-
-
Notifications
You must be signed in to change notification settings - Fork 220
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Prims Algorithm Tutorial #711
base: main
Are you sure you want to change the base?
Conversation
- Included an overview, the complexities of the algorithm - Explained one leetcode problem in depth (I couldn't find too many and I wanted to leave some for the suggested problems that were simple and similar) - Included two suggested problems. - Highlighted any references used.
Incorporated the requested changes.
Incorporated the requested changes. Do let me know if they're okay. |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
the structure is fine. please add one more example with the same structure.
- Added a second example problem for the same.
Added a second example problem, also highlighted the solutions for the problems that had one. |
Made a few modifications to the code, indentation to make it copy-pastable in leetcode
I made a second commit changing a little bit of the indentation and layout of the code to make it directly copy-pastable in leetcode. Do let me know if it's okay! |
Hello! I just wanted to follow up on the PR. |
Ah, got it. Will make sure to do that next time! |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Made necessary changes to run locally
Apologies for the delay, was a bit caught up with work. Made the changes and tested it locally. Seems to be working fine now. |
Hi there! Just wanted to follow up on the PR! |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
- please apply LaTex instead of using backticks.
- for the content, i will review in depth in weekend.
- Changed all backtick highlighting to LaTeX - Modified the introduction to the problems (made it an explanation instead of just listing input and output) - Changed value for TabItems to 'py'
Made the requested changes. My apologies for not checking with the format of things. I think it should be compliant now. |
Hi, just wanted to follow up on the pull request. |
Hi! Just wanted to know if I needed to make any other changes in this. |
@Infonioknight hey sorry for the late update. been a bit busy these months. will try to review on weekend. thanks. |
@wingkwong no worries at all! Do take care. |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
## Suggested Pre-requisites | ||
Before moving on to learn the Prims algorithm, it is suggested that you know what a [Minimum Spanning Tree (MST)](../graph-theory/minimum-spanning-tree.md) is. |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
can you also work on MST page?
|
||
### Complexity and Use-Cases | ||
When implemented using a priority queue (usually a binary heap), the time complexity of Prim’s algorithm is $O(E + V log V)$, where $E$ is the number of edges and $V$ is the number of vertices. This makes Prim's algorithm highly efficient for **dense** graphs, where the number of edges is close to the maximum possible. For sparse graphs (a graph with a relatively small number of edges compared to the maximum number of possible edges), other algorithms like [Kruskal’s](kruskals-algorithm.md) may perform better. |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
may elaborate how to get
|
||
### Complexity and Use-Cases | ||
When implemented using a priority queue (usually a binary heap), the time complexity of Prim’s algorithm is $O(E + V log V)$, where $E$ is the number of edges and $V$ is the number of vertices. This makes Prim's algorithm highly efficient for **dense** graphs, where the number of edges is close to the maximum possible. For sparse graphs (a graph with a relatively small number of edges compared to the maximum number of possible edges), other algorithms like [Kruskal’s](kruskals-algorithm.md) may perform better. |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
This makes Prim's algorithm highly efficient for dense graphs,
may explain why it is efficient for dense graphs but not sparse ones.
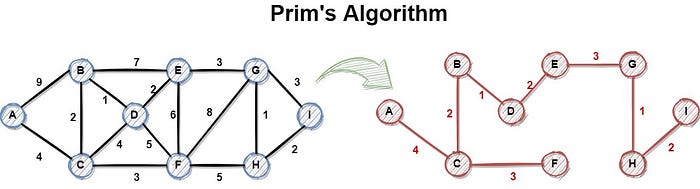 | ||
|
||
_Source: https://miro.medium.com/max/700/1*7kpPIPcmXr38Juh0umM6fA.jpeg_ |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
refer to the post title instead.
3: [(6, 1), (1, 2)] | ||
``` | ||
Where each tuple consists of '(cost, destination)'. |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
'(cost, destination)'
-> $(cost, destination)$
## Example 2: [1584 - Min Cost to Connect All Points](https://leetcode.com/problems/min-cost-to-connect-all-points) | ||
### Instructions: | ||
You are given an array $points$ representing integer coordinates of some points on a 2D-plane, where $points[i]$ = $[xi, yi]$. |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
xi
-> x_i
yi
-> y_i
apply to j
as well.
for i in range(len(points)): | ||
current = tuple(points[i]) | ||
for j in range(i+1, len(points)): |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
i+1
-> i + 1
|
||
adjacency_list = {} | ||
for i in range(len(points)): |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
len(points)
-> n
since it will be used more than one time
heapq.heappush(connection_queue, connection) | ||
|
||
while len(seen) < n and connection_queue: | ||
cost, current = heapq.heappop(connection_queue) | ||
if current in seen: | ||
continue | ||
|
||
res += cost | ||
seen.add(current) | ||
|
||
for connection in adjacency_list[current]: | ||
if connection[1] not in seen: | ||
heapq.heappush(connection_queue, connection) | ||
|
||
return res |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
fix indentation
seen = set([start]) | ||
|
||
for connection in adjacency_list[start]: |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
include comments for the below code like L314 - L323. (apply to other code block)
Change Summary
Checklist
If you haven't fulfilled the below requirements or even delete the entire checklist, your PR won't be reviewed and will be closed without notice. Regular contributors (with 10+ PRs) can skip this part.
General
Tutorial
solutionLink
blank.Solutions
## Approach 1: Two Pointers
.