-
Notifications
You must be signed in to change notification settings - Fork 0
Architecture
This will be a native Android application written in Javascript through the React Native framework. The base React libraries, React-Native libraries, Expo libraries, and navigation library will be used to build the application with native widgets (FlatList, Pressable, ScrollView, etc.). The React Native Vision Camera library will help provide barcode scanning functionality. User accounts and their application information will be handled using a Firebase real-time database, and Firebase Authentication will handle password authentication. Kroger's public API will be used to retrieve detailed information on products and store locations.
Additional Libraries used:
- react-native-base64
- expo-constants
- react-native-dotenv
The package manager used will be npm (Node Package Manager).
-
Jackson: API work; setting up the API connection, initially retrieving product information from the API.
- Implementing data refresh functionality to keep product info up-to-date, especially for products currently in cart.
-
Mark: General Product Screen navigation bars and functionality; taking API info and displaying it as scrollable products with pertinent info displayed.
- Setting up camera/barcode scanning.
- Creating a tutorial to be displayed on app start up.
-
Danny: Creating the shopping cart; totaling prices with delivery fees; simulating checkout process; storing cart data locally on device.
- Setting up warnings on out-of-stock products before ordering.
- Setting up order history.
-
Zack: Implementing product search bar, with filters and sorts.
- Setting up individual product info screens, including 'adding to favorites' functionality.
- Adding a favorite products list per account.
-
Claude: Establishing login in/account creation functionality and screens; database info retrieval/pushing.
- Creating store selection pages/functionality.
- Setting up settings functionality.
An .apk will be made available on the GitHub release.
For improved clarity, pop out the image in another tab.
State will be not be maintained for this particular database. Product information will be loaded from the database on app start up. See the structure below for details.
- Information on specific products can be retrieved:
- Fetch API GET
- Price GET
- Item ID GET
- Stock Level GET
- Size GET
- Location GET
- Description GET
- etc.
- Batches of products can also be searched for through the API. These can be narrowed down using query parameters, including:
- filter.term (a search term to filter product results, e.g. milk)
- filter.locationID (the locationID of the store, e.g. 01400943; only display products from that location)
- filter.productID (the ID of the product, e.g. 0001111060903)
- filter.limit (the number of products to return)
- etc.
State will be maintained both locally (in memory) and through a database. Cart information, user location/home address, a user's preferred store, and user setting states will be kept locally, while most other user information will be stored via the Firebase database. See the structure below for details. Passwords will be handled by the authentication console.
-
users
- userID (String)
- firstName (String)
- lastName (String)
- email (String)
- billingAddress
- address1 (String)
- address2 (String)
- city (String)
- state (String)
- ...
- deliveryAddress
- ...
-
orders
- userID (String)
- priorOrders
- priorOrder1
- checkoutDate (Date)
- productIDs (String)
- totalPrice (double)
- ...
- priorOrder1
-
carts
- userID (String)
- cartContents
- productIDs (String)
- totalPrice (double)
-
favorites
- userID (String)
- productIDs (String)
Here are a few common queries that will be ran on this database:
- Retrieving all relevant user info (users, orders, favorites, settings, etc.) upon log-in via userID
- Checking if an email is already being used in the database while creating account
- Verifying local user information such as cart contents matches the database (and rectifying it if unsychronized)
Joins will be necessary given that this database hierarchy avoids nesting with the JSON. userID will serve as the key to join the subsets of data together.
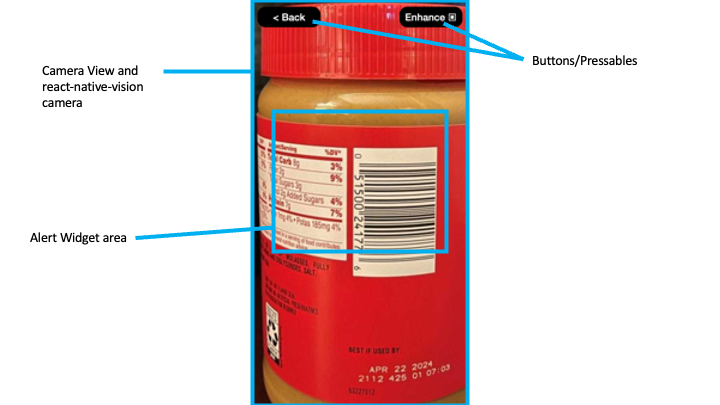
React-Native-Vision-Camera is a popular library for photo, video, and snapshot capture through React Native. When paired with ML Kit's Vision APIs or a JavaScript worklet, this library will allow for product barcode scanning. The productID obtained from the barcode will be used to look up the product, assuming it exists in the database. The Enhance Pressable applies a filter to the camera feed to help those with low vision find the barcode. If a barcode cannot be scanned or there isn't a corresponding product in the database, an Alert will pop up and notify the user.
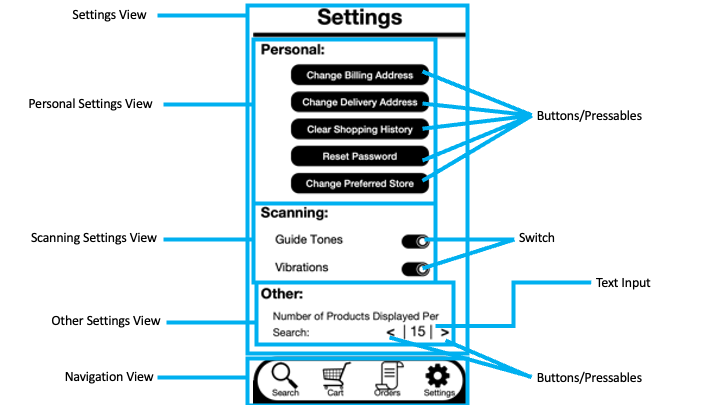
Switches are boolean. They can either be switched left or right. Being switched to the right means that the associated setting is set to 'on'. The 'Number of Products Displayer Per Search' quantity can be incremented/decremented using the arrow buttons, or by tapping the quantity itself and directly inputting a new valid value (nonnegative, nonzero integer, etc.).
Splitting the settings into multiple views allows for easier formatting within sections and distinction between sections. If future settings or sections are added, the Settings View itself will use a ScrollView for scrolling capability.
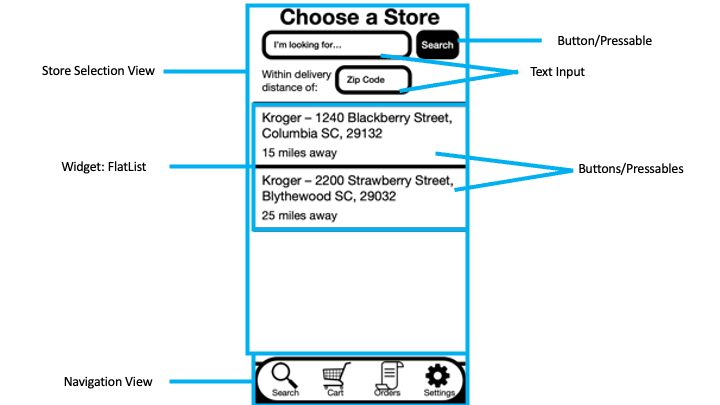
The FlatList widget allows the app to display more than four stores, which is necessary in highly urbanized areas; FlatLists are scrollable, so every store and its details can be seen. Stores will be sorted by closest to zip code first. Each displayed store is a Pressable, which sets the user's preferred store on press. The Store Selection screen allows the navigation bar to display.
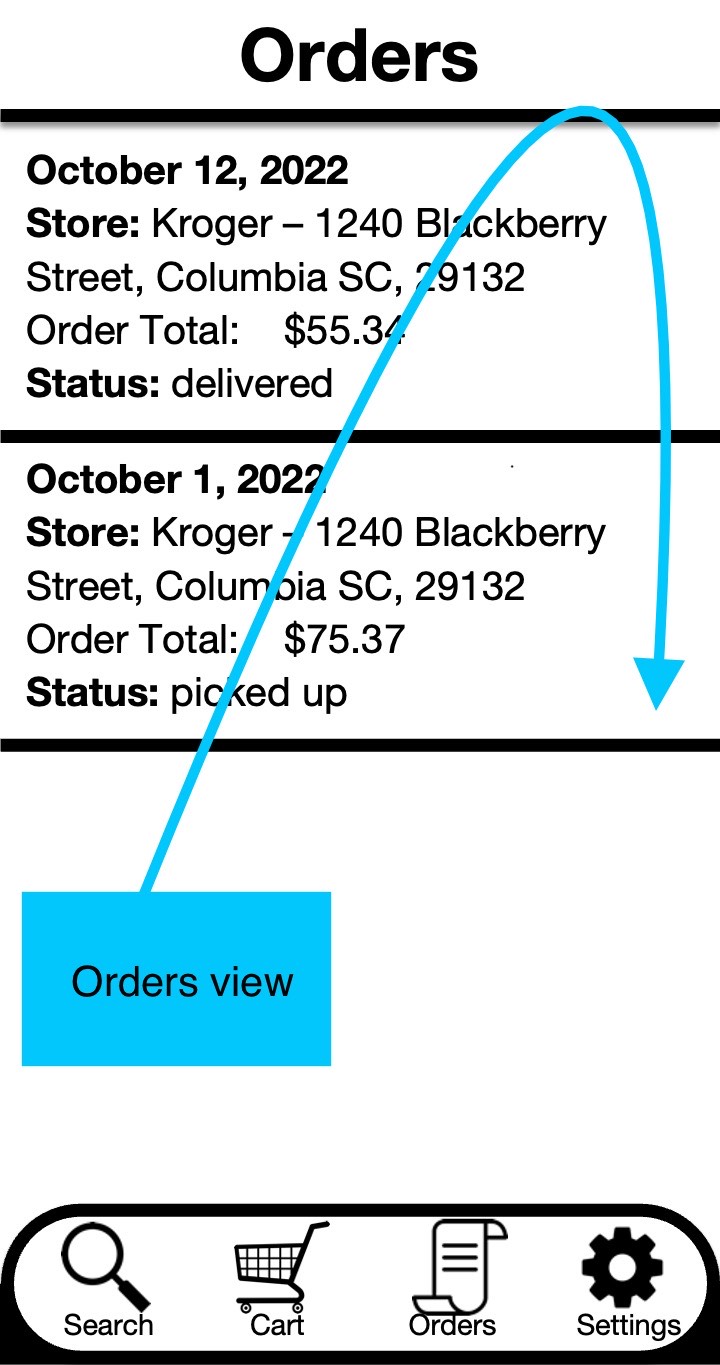
The Orders View utilizes a FlatList to display a scrollable feed of past orders. Orders are stored in most recent order. The Orders Screen allows the navigation bar to display.
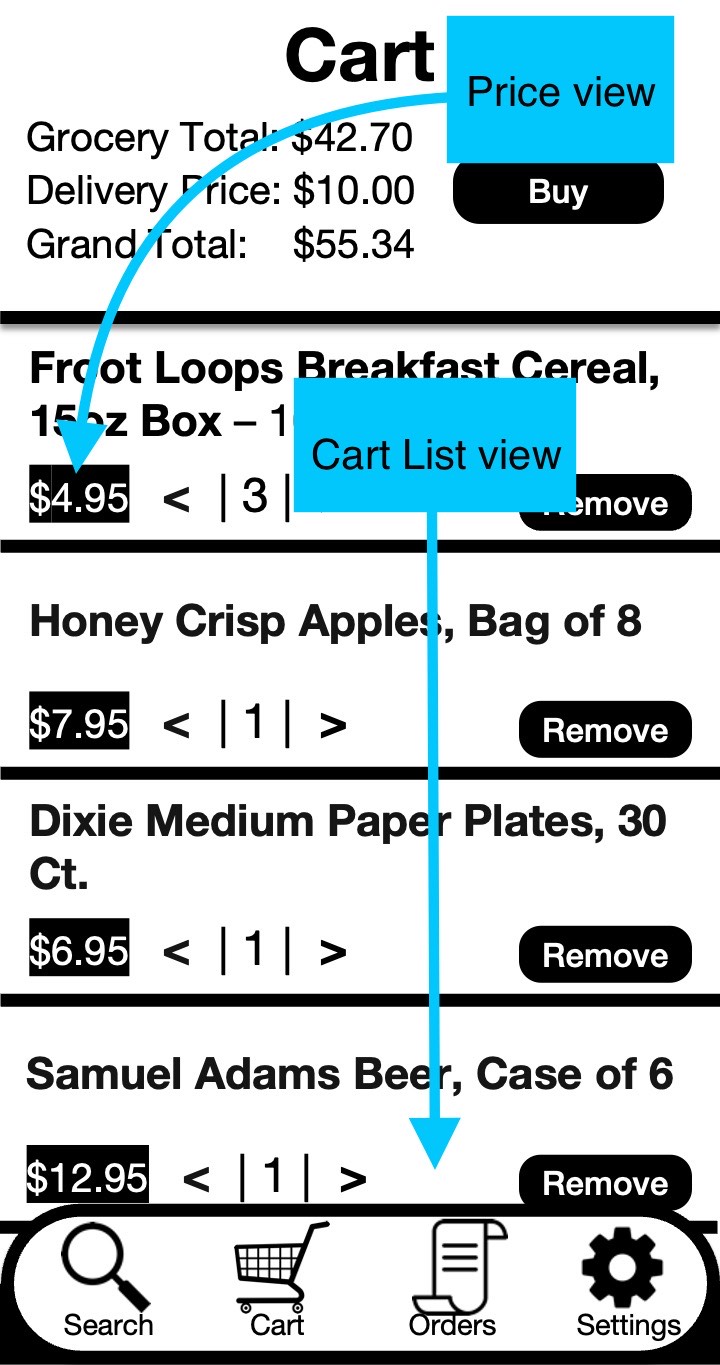
The Price View consists of the 'Buy' Pressable as well as the grocery total, delivery price, and grand total values. The Cart List utilizes a FlatList to display a scrollable feed of products in the cart. Each product itself is a Pressable, allowing for the user to go to that product's information page on press. Additionally, each product's quantity can be directly edited/incremented/decremented from the Cart List view or removed from the cart using the Remove Pressable. Products in the cart are stored in first-in order. The Cart Screen allows the navigation bar to display.
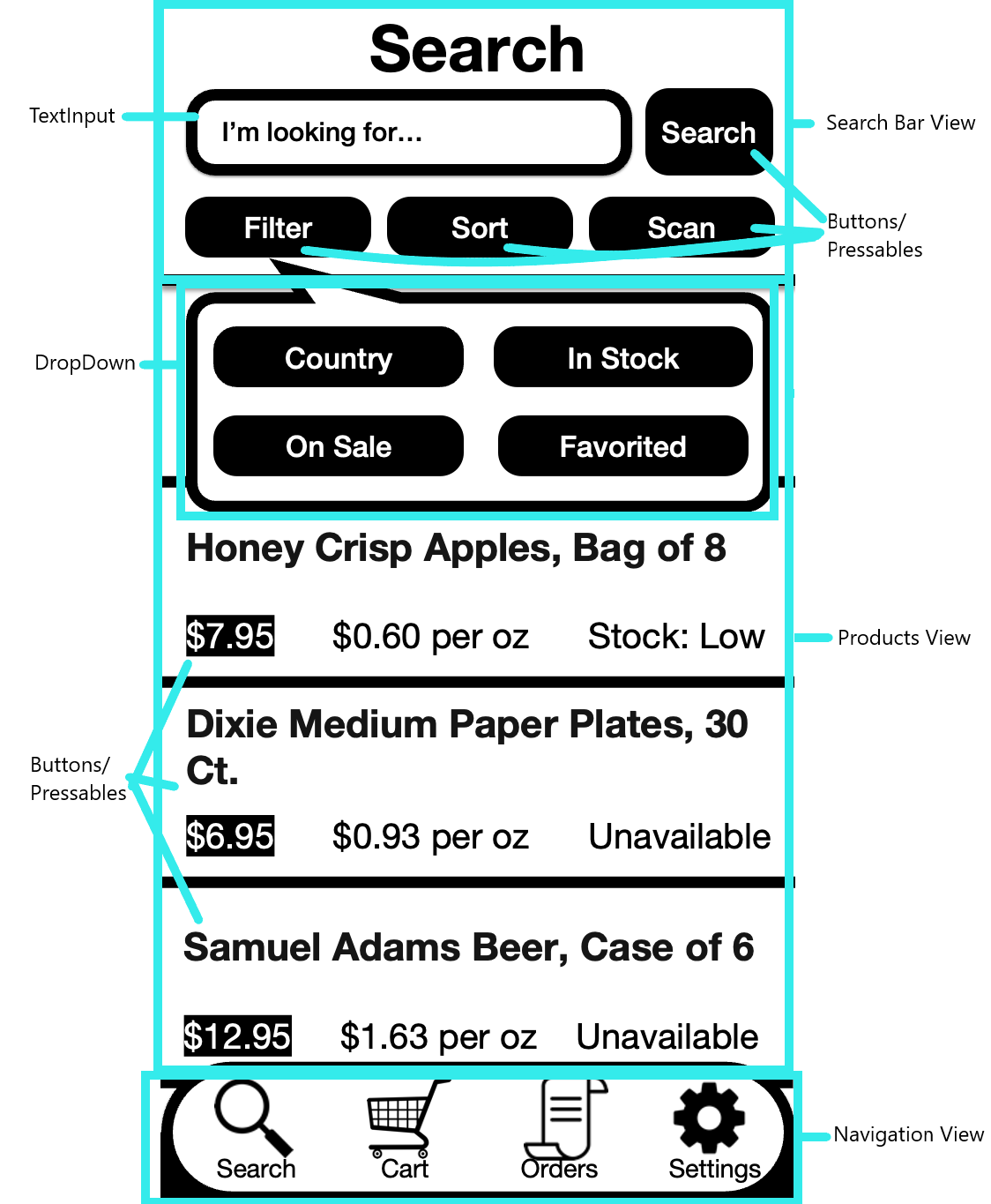
The DropDown views contains buttons. The DropDown views are hidden until the Filter or Sort buttons are pressed in the Search Bar view. Only the DropDown view for the Filter button is shown here. The DropDown view for the Sort button follows the same view format. The Products View utilizes a FlatList to display a scrollable feed of products in the database. Each product itself is a Pressable, allows for the user to go to that product's information page on press. The Search Screen allows the navigation bar to display.
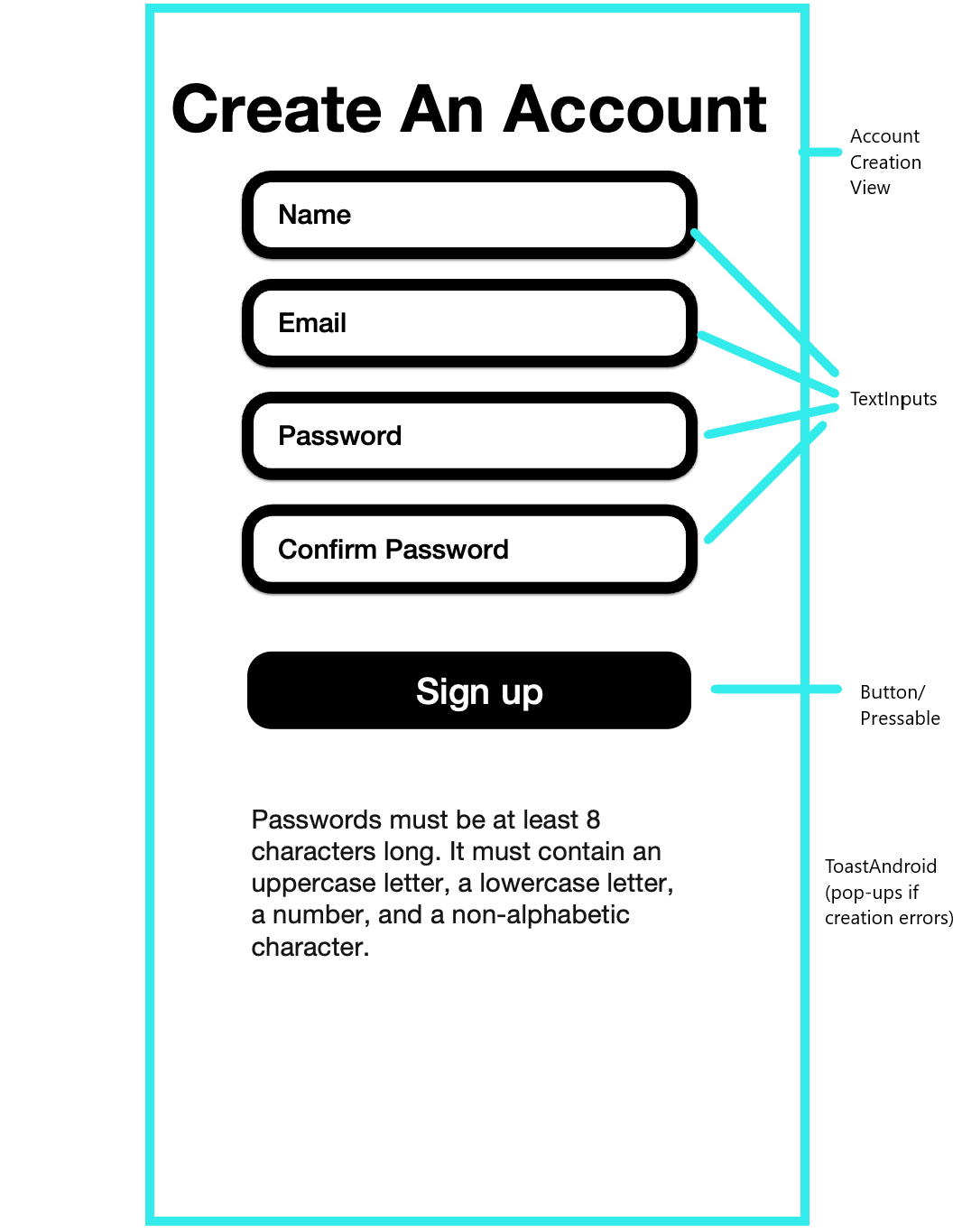
ToastAndroid is a widget that displays a small popup window with text that is unobtrusive. If a password doesn't meet requirements or an email is already taken, a pop-up will appear.
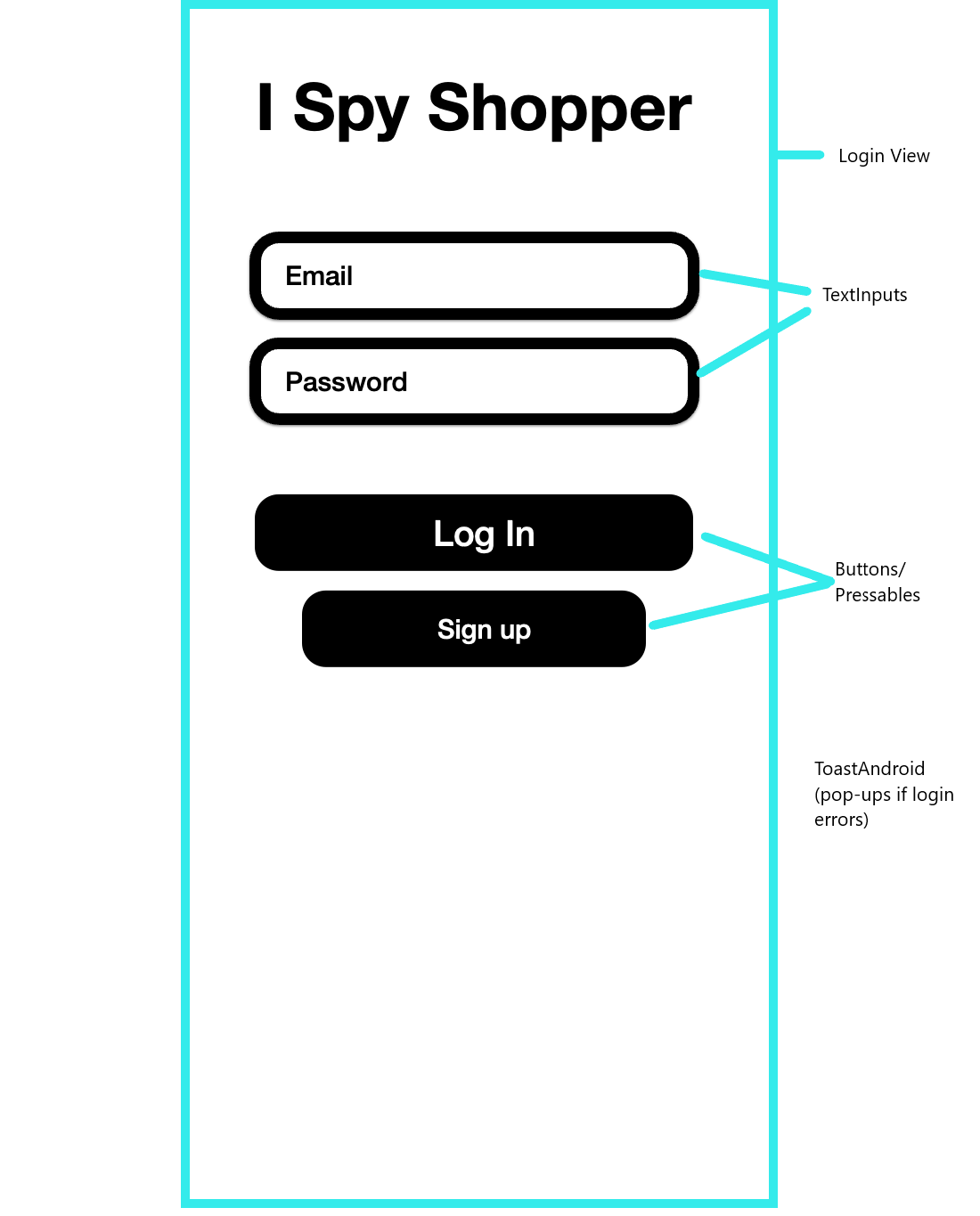
ToastAndroid is a widget that displays a small popup window with text that is unobtrusive. If logging in fails due to an incorrect password or failed authentication, a pop-up will appear.
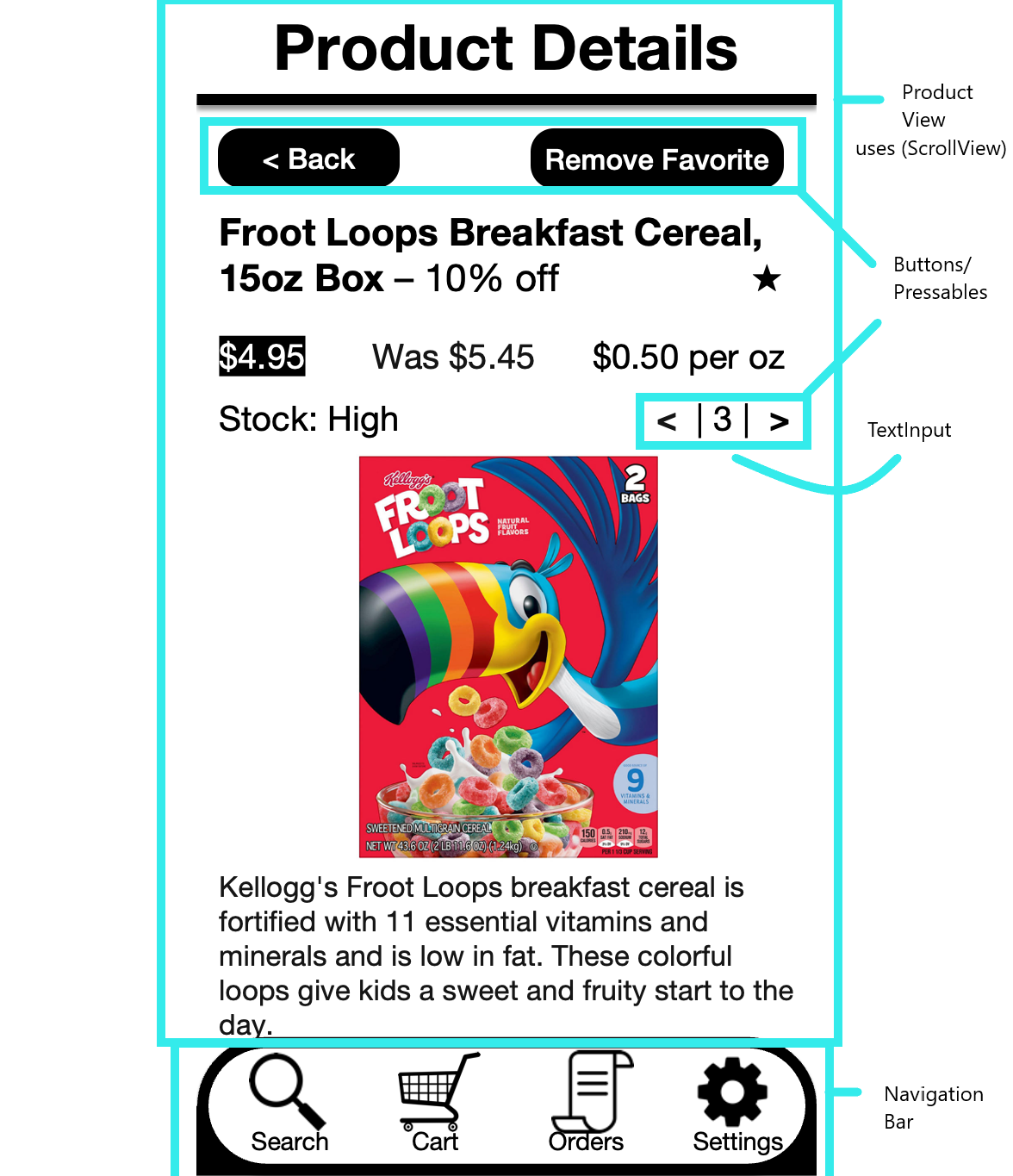
The Product Details screen displays all available information about the product. It utilizes a ScrollView to allow for scrolling to see the rest of the information. A 'favorites' Pressable allows for a user to add a product to their favorites list or remove it from their favorites if already favorited. Like the previous quantity set-ups, the quantity can be incremented/decremented using the arrow buttons, or by tapping the quantity itself and directly inputting a new valid value (nonnegative, nonzero integer, etc.). The Product Details Screen allows the navigation bar to display.