diff --git a/.github/workflows/coverage.yml b/.github/workflows/coverage.yml
index d5de0bdb..09b136c2 100644
--- a/.github/workflows/coverage.yml
+++ b/.github/workflows/coverage.yml
@@ -24,5 +24,6 @@ jobs:
working-directory: hive
- uses: codecov/codecov-action@v4
with:
+ fail_ci_if_error: true
token: ${{ secrets.CODECOV_TOKEN }}
file: hive/coverage/lcov.info
diff --git a/hive/CHANGELOG.md b/hive/CHANGELOG.md
index 624f123b..b395f0ed 100644
--- a/hive/CHANGELOG.md
+++ b/hive/CHANGELOG.md
@@ -1,3 +1,8 @@
+## 2.5.0+2
+
+- Adds documentation for adding new fields (@vizakenjack)
+- Updates README badges
+
## 2.5.0+1
- Documentation updates for `HiveField` in support of `hive_ce_generator` changes
diff --git a/hive/README.md b/hive/README.md
index 4c26cc14..78b36ea9 100644
--- a/hive/README.md
+++ b/hive/README.md
@@ -1,9 +1,9 @@
-
+
Fast, Enjoyable & Secure NoSQL Database
-[](https://github.com/hivedb/hive/actions) [](https://codecov.io/gh/hivedb/hive) [](https://pub.dev/packages/hive) [](https://github.com/hivedb/hive/blob/master/LICENSE)
+[](https://github.com/IO-Design-Team/hive_ce/actions/workflows/test.yml) [](https://codecov.io/gh/IO-Design-Team/hive_ce) [](https://pub.dev/packages/hive_ce) [](https://github.com/IO-Design-Team/hive_ce/blob/master/LICENSE)
Hive is a lightweight and blazing fast key-value database written in pure Dart. Inspired by [Bitcask](https://en.wikipedia.org/wiki/Bitcask).
@@ -16,6 +16,7 @@ If you need queries, multi-isolate support or links between objects check out [I
The `hive_ce` package is a drop in replacement for Hive v2. Make the following replacements in your project:
pubspec.yaml
+
```yaml
# old
dependencies:
@@ -35,6 +36,7 @@ dev_dependencies:
```
Dart files
+
```dart
// old
import 'package:hive/hive.dart';
@@ -152,6 +154,7 @@ class Person extends HiveObject {
```
Add the following to your pubspec.yaml
+
```yaml
dev_dependencies:
build_runner: latest
@@ -159,11 +162,13 @@ dev_dependencies:
```
And run the following command to generate the type adapter
+
```bash
flutter pub run build_runner build --delete-conflicting-outputs
```
This will generate all of your `TypeAdapter`s as well as a Hive extension to register them all in one go
+
```dart
import 'package:your_package/hive_registrar.g.dart';
@@ -193,6 +198,80 @@ person.save();
print(box.getAt(0)) // Dave - 30
```
+## Add fields to objects
+
+When adding a new non-nullable field to an existing object, you need to specify a default value to ensure compatibility with existing data.
+
+For example, consider an existing database with a `Person` object:
+
+```dart
+@HiveType(typeId: 0)
+class Person extends HiveObject {
+ Person({required this.name, required this.age});
+
+ @HiveField(0)
+ String name;
+
+ @HiveField(1)
+ int age;
+}
+```
+
+If you want to add a `balance` field:
+
+```dart
+@HiveType(typeId: 0)
+class Person extends HiveObject {
+ Person({required this.name, required this.age, required this.balance});
+
+ @HiveField(0)
+ String name;
+
+ @HiveField(1)
+ int age;
+
+ @HiveField(2)
+ int balance;
+}
+```
+
+Without proper handling, this change will cause null errors in the existing application when accessing the new field.
+
+To resolve this issue, you can set a default value in the constructor (this requires hive_ce_generator 1.5.0+)
+
+```dart
+@HiveType(typeId: 0)
+class Person extends HiveObject {
+ Person({required this.name, required this.age, this.balance = 0});
+
+ @HiveField(0)
+ String name;
+
+ @HiveField(1)
+ int age;
+
+ @HiveField(2)
+ int balance;
+}
+```
+
+Or specify it in the `HiveField` annotation:
+
+```dart
+@HiveField(2, defaultValue: 0)
+int balance;
+```
+
+Alternatively, you can write custom migration code to handle the transition.
+
+After modifying the schema, remember to run the build runner to generate the necessary code:
+
+```console
+flutter pub run build_runner build --delete-conflicting-outputs
+```
+
+This will update your Hive adapters to reflect the changes in your object structure.
+
## Hive ❤️ Flutter
Hive was written with Flutter in mind. It is a perfect fit if you need a lightweight datastore for your app. After adding the required dependencies and initializing Hive, you can use Hive in your project:
@@ -223,10 +302,10 @@ Boxes are cached and therefore fast enough to be used directly in the `build()`
## Benchmark
-| 1000 read iterations | 1000 write iterations |
-| :--------------------------------------------------------------------------------------------------: | :-----------------------------------------------------------------------------------------: |
-|  |  |
-| SharedPreferences is on par with Hive when it comes to read performance. SQLite performs much worse. | Hive greatly outperforms SQLite and SharedPreferences when it comes to writing or deleting. |
+| 1000 read iterations | 1000 write iterations |
+| :--------------------------------------------------------------------------------------------------: | :----------------------------------------------------------------------------------------------: |
+| 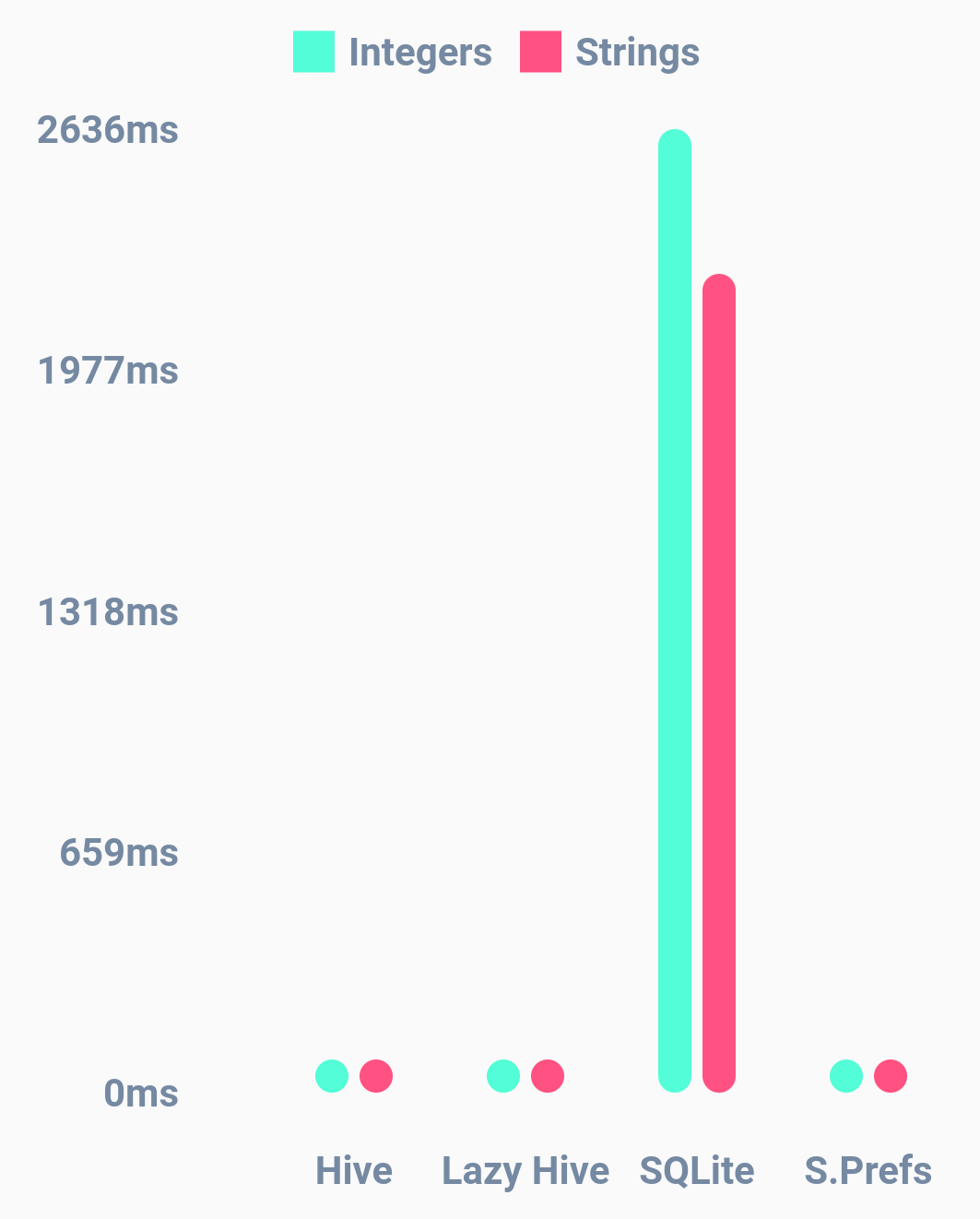 | 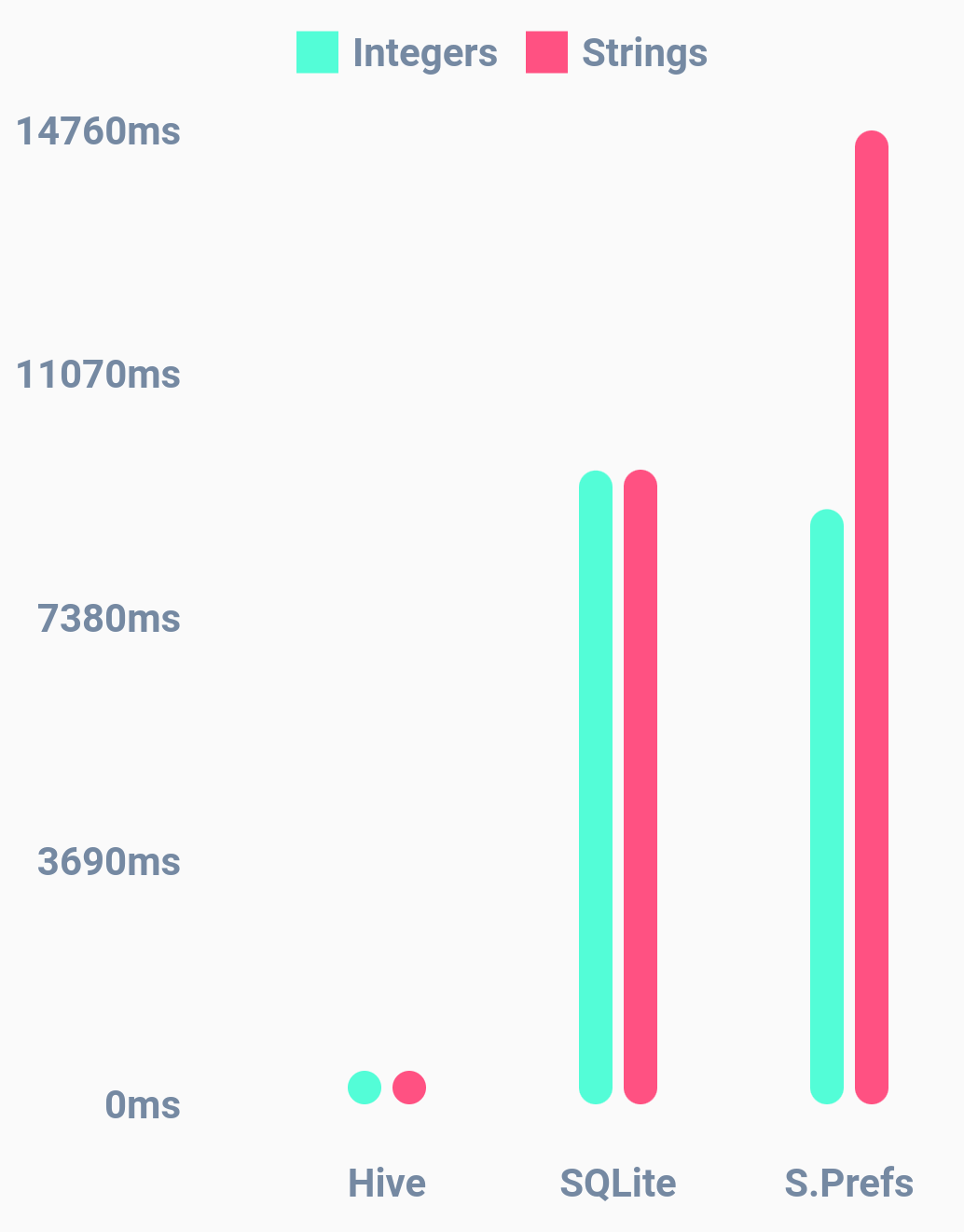 |
+| SharedPreferences is on par with Hive when it comes to read performance. SQLite performs much worse. | Hive greatly outperforms SQLite and SharedPreferences when it comes to writing or deleting. |
The benchmark was performed on a Oneplus 6T with Android Q. You can [run the benchmark yourself](https://github.com/hivedb/hive_benchmark).
diff --git a/hive/pubspec.yaml b/hive/pubspec.yaml
index 7ce5311a..ca923669 100644
--- a/hive/pubspec.yaml
+++ b/hive/pubspec.yaml
@@ -1,6 +1,6 @@
name: hive_ce
description: Hive Community Edition - A spiritual continuation of Hive v2
-version: 2.5.0+1
+version: 2.5.0+2
homepage: https://github.com/IO-Design-Team/hive_ce/tree/main/hive
documentation: https://docs.hivedb.dev/