j?j:g+f));return 1===d?(b=a[c-1],e.push(k[b>>2]+k[63&b<<4]+"==")):2===d&&(b=(a[c-2]<<8)+a[c-1],e.push(k[b>>10]+k[63&b>>4]+k[63&b<<2]+"=")),e.join("")}c.byteLength=function(a){var b=d(a),c=b[0],e=b[1];return 3*(c+e)/4-e},c.toByteArray=f,c.fromByteArray=j;for(var k=[],l=[],m="undefined"==typeof Uint8Array?Array:Uint8Array,n="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/",o=0,p=n.length;o 0) {
+ throw new Error('Invalid string. Length must be a multiple of 4')
+ }
+
+ // Trim off extra bytes after placeholder bytes are found
+ // See: https://github.com/beatgammit/base64-js/issues/42
+ var validLen = b64.indexOf('=')
+ if (validLen === -1) validLen = len
+
+ var placeHoldersLen = validLen === len
+ ? 0
+ : 4 - (validLen % 4)
+
+ return [validLen, placeHoldersLen]
+}
+
+// base64 is 4/3 + up to two characters of the original data
+function byteLength (b64) {
+ var lens = getLens(b64)
+ var validLen = lens[0]
+ var placeHoldersLen = lens[1]
+ return ((validLen + placeHoldersLen) * 3 / 4) - placeHoldersLen
+}
+
+function _byteLength (b64, validLen, placeHoldersLen) {
+ return ((validLen + placeHoldersLen) * 3 / 4) - placeHoldersLen
+}
+
+function toByteArray (b64) {
+ var tmp
+ var lens = getLens(b64)
+ var validLen = lens[0]
+ var placeHoldersLen = lens[1]
+
+ var arr = new Arr(_byteLength(b64, validLen, placeHoldersLen))
+
+ var curByte = 0
+
+ // if there are placeholders, only get up to the last complete 4 chars
+ var len = placeHoldersLen > 0
+ ? validLen - 4
+ : validLen
+
+ var i
+ for (i = 0; i < len; i += 4) {
+ tmp =
+ (revLookup[b64.charCodeAt(i)] << 18) |
+ (revLookup[b64.charCodeAt(i + 1)] << 12) |
+ (revLookup[b64.charCodeAt(i + 2)] << 6) |
+ revLookup[b64.charCodeAt(i + 3)]
+ arr[curByte++] = (tmp >> 16) & 0xFF
+ arr[curByte++] = (tmp >> 8) & 0xFF
+ arr[curByte++] = tmp & 0xFF
+ }
+
+ if (placeHoldersLen === 2) {
+ tmp =
+ (revLookup[b64.charCodeAt(i)] << 2) |
+ (revLookup[b64.charCodeAt(i + 1)] >> 4)
+ arr[curByte++] = tmp & 0xFF
+ }
+
+ if (placeHoldersLen === 1) {
+ tmp =
+ (revLookup[b64.charCodeAt(i)] << 10) |
+ (revLookup[b64.charCodeAt(i + 1)] << 4) |
+ (revLookup[b64.charCodeAt(i + 2)] >> 2)
+ arr[curByte++] = (tmp >> 8) & 0xFF
+ arr[curByte++] = tmp & 0xFF
+ }
+
+ return arr
+}
+
+function tripletToBase64 (num) {
+ return lookup[num >> 18 & 0x3F] +
+ lookup[num >> 12 & 0x3F] +
+ lookup[num >> 6 & 0x3F] +
+ lookup[num & 0x3F]
+}
+
+function encodeChunk (uint8, start, end) {
+ var tmp
+ var output = []
+ for (var i = start; i < end; i += 3) {
+ tmp =
+ ((uint8[i] << 16) & 0xFF0000) +
+ ((uint8[i + 1] << 8) & 0xFF00) +
+ (uint8[i + 2] & 0xFF)
+ output.push(tripletToBase64(tmp))
+ }
+ return output.join('')
+}
+
+function fromByteArray (uint8) {
+ var tmp
+ var len = uint8.length
+ var extraBytes = len % 3 // if we have 1 byte left, pad 2 bytes
+ var parts = []
+ var maxChunkLength = 16383 // must be multiple of 3
+
+ // go through the array every three bytes, we'll deal with trailing stuff later
+ for (var i = 0, len2 = len - extraBytes; i < len2; i += maxChunkLength) {
+ parts.push(encodeChunk(uint8, i, (i + maxChunkLength) > len2 ? len2 : (i + maxChunkLength)))
+ }
+
+ // pad the end with zeros, but make sure to not forget the extra bytes
+ if (extraBytes === 1) {
+ tmp = uint8[len - 1]
+ parts.push(
+ lookup[tmp >> 2] +
+ lookup[(tmp << 4) & 0x3F] +
+ '=='
+ )
+ } else if (extraBytes === 2) {
+ tmp = (uint8[len - 2] << 8) + uint8[len - 1]
+ parts.push(
+ lookup[tmp >> 10] +
+ lookup[(tmp >> 4) & 0x3F] +
+ lookup[(tmp << 2) & 0x3F] +
+ '='
+ )
+ }
+
+ return parts.join('')
+}
diff --git a/node_modules/base64-js/package.json b/node_modules/base64-js/package.json
new file mode 100644
index 0000000..4cbe2aa
--- /dev/null
+++ b/node_modules/base64-js/package.json
@@ -0,0 +1,75 @@
+{
+ "_from": "base64-js@^1.3.1",
+ "_id": "base64-js@1.5.1",
+ "_inBundle": false,
+ "_integrity": "sha512-AKpaYlHn8t4SVbOHCy+b5+KKgvR4vrsD8vbvrbiQJps7fKDTkjkDry6ji0rUJjC0kzbNePLwzxq8iypo41qeWA==",
+ "_location": "/base64-js",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "base64-js@^1.3.1",
+ "name": "base64-js",
+ "escapedName": "base64-js",
+ "rawSpec": "^1.3.1",
+ "saveSpec": null,
+ "fetchSpec": "^1.3.1"
+ },
+ "_requiredBy": [
+ "/buffer"
+ ],
+ "_resolved": "https://registry.npmjs.org/base64-js/-/base64-js-1.5.1.tgz",
+ "_shasum": "1b1b440160a5bf7ad40b650f095963481903930a",
+ "_spec": "base64-js@^1.3.1",
+ "_where": "/Users/jasonagus/Desktop/Guide Project/node_modules/buffer",
+ "author": {
+ "name": "T. Jameson Little",
+ "email": "t.jameson.little@gmail.com"
+ },
+ "bugs": {
+ "url": "https://github.com/beatgammit/base64-js/issues"
+ },
+ "bundleDependencies": false,
+ "deprecated": false,
+ "description": "Base64 encoding/decoding in pure JS",
+ "devDependencies": {
+ "babel-minify": "^0.5.1",
+ "benchmark": "^2.1.4",
+ "browserify": "^16.3.0",
+ "standard": "*",
+ "tape": "4.x"
+ },
+ "funding": [
+ {
+ "type": "github",
+ "url": "https://github.com/sponsors/feross"
+ },
+ {
+ "type": "patreon",
+ "url": "https://www.patreon.com/feross"
+ },
+ {
+ "type": "consulting",
+ "url": "https://feross.org/support"
+ }
+ ],
+ "homepage": "https://github.com/beatgammit/base64-js",
+ "keywords": [
+ "base64"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "base64-js",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/beatgammit/base64-js.git"
+ },
+ "scripts": {
+ "build": "browserify -s base64js -r ./ | minify > base64js.min.js",
+ "lint": "standard",
+ "test": "npm run lint && npm run unit",
+ "unit": "tape test/*.js"
+ },
+ "typings": "index.d.ts",
+ "version": "1.5.1"
+}
diff --git a/node_modules/bl/.jshintrc b/node_modules/bl/.jshintrc
deleted file mode 100644
index be119b2..0000000
--- a/node_modules/bl/.jshintrc
+++ /dev/null
@@ -1,60 +0,0 @@
-{
- "predef": [ ]
- , "bitwise": false
- , "camelcase": false
- , "curly": false
- , "eqeqeq": false
- , "forin": false
- , "immed": false
- , "latedef": false
- , "noarg": true
- , "noempty": true
- , "nonew": true
- , "plusplus": false
- , "quotmark": true
- , "regexp": false
- , "undef": true
- , "unused": true
- , "strict": false
- , "trailing": true
- , "maxlen": 120
- , "asi": true
- , "boss": true
- , "debug": true
- , "eqnull": true
- , "esnext": false
- , "evil": true
- , "expr": true
- , "funcscope": false
- , "globalstrict": false
- , "iterator": false
- , "lastsemic": true
- , "laxbreak": true
- , "laxcomma": true
- , "loopfunc": true
- , "multistr": false
- , "onecase": false
- , "proto": false
- , "regexdash": false
- , "scripturl": true
- , "smarttabs": false
- , "shadow": false
- , "sub": true
- , "supernew": false
- , "validthis": true
- , "browser": true
- , "couch": false
- , "devel": false
- , "dojo": false
- , "mootools": false
- , "node": true
- , "nonstandard": true
- , "prototypejs": false
- , "rhino": false
- , "worker": true
- , "wsh": false
- , "nomen": false
- , "onevar": false
- , "passfail": false
- , "esversion": 3
-}
\ No newline at end of file
diff --git a/node_modules/bl/.travis.yml b/node_modules/bl/.travis.yml
deleted file mode 100644
index 1044a09..0000000
--- a/node_modules/bl/.travis.yml
+++ /dev/null
@@ -1,15 +0,0 @@
-sudo: false
-language: node_js
-node_js:
- - '4'
- - '6'
- - '8'
- - '9'
- - '10'
-branches:
- only:
- - master
-notifications:
- email:
- - rod@vagg.org
- - matteo.collina@gmail.com
diff --git a/node_modules/bl/LICENSE.md b/node_modules/bl/LICENSE.md
deleted file mode 100644
index dea757d..0000000
--- a/node_modules/bl/LICENSE.md
+++ /dev/null
@@ -1,13 +0,0 @@
-The MIT License (MIT)
-=====================
-
-Copyright (c) 2013-2018 bl contributors
-----------------------------------
-
-*bl contributors listed at *
-
-Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/bl/README.md b/node_modules/bl/README.md
deleted file mode 100644
index 79dca35..0000000
--- a/node_modules/bl/README.md
+++ /dev/null
@@ -1,218 +0,0 @@
-# bl *(BufferList)*
-
-[](https://travis-ci.org/rvagg/bl)
-
-**A Node.js Buffer list collector, reader and streamer thingy.**
-
-[](https://nodei.co/npm/bl/)
-[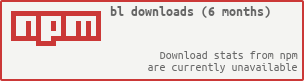](https://nodei.co/npm/bl/)
-
-**bl** is a storage object for collections of Node Buffers, exposing them with the main Buffer readable API. Also works as a duplex stream so you can collect buffers from a stream that emits them and emit buffers to a stream that consumes them!
-
-The original buffers are kept intact and copies are only done as necessary. Any reads that require the use of a single original buffer will return a slice of that buffer only (which references the same memory as the original buffer). Reads that span buffers perform concatenation as required and return the results transparently.
-
-```js
-const BufferList = require('bl')
-
-var bl = new BufferList()
-bl.append(Buffer.from('abcd'))
-bl.append(Buffer.from('efg'))
-bl.append('hi') // bl will also accept & convert Strings
-bl.append(Buffer.from('j'))
-bl.append(Buffer.from([ 0x3, 0x4 ]))
-
-console.log(bl.length) // 12
-
-console.log(bl.slice(0, 10).toString('ascii')) // 'abcdefghij'
-console.log(bl.slice(3, 10).toString('ascii')) // 'defghij'
-console.log(bl.slice(3, 6).toString('ascii')) // 'def'
-console.log(bl.slice(3, 8).toString('ascii')) // 'defgh'
-console.log(bl.slice(5, 10).toString('ascii')) // 'fghij'
-
-console.log(bl.indexOf('def')) // 3
-console.log(bl.indexOf('asdf')) // -1
-
-// or just use toString!
-console.log(bl.toString()) // 'abcdefghij\u0003\u0004'
-console.log(bl.toString('ascii', 3, 8)) // 'defgh'
-console.log(bl.toString('ascii', 5, 10)) // 'fghij'
-
-// other standard Buffer readables
-console.log(bl.readUInt16BE(10)) // 0x0304
-console.log(bl.readUInt16LE(10)) // 0x0403
-```
-
-Give it a callback in the constructor and use it just like **[concat-stream](https://github.com/maxogden/node-concat-stream)**:
-
-```js
-const bl = require('bl')
- , fs = require('fs')
-
-fs.createReadStream('README.md')
- .pipe(bl(function (err, data) { // note 'new' isn't strictly required
- // `data` is a complete Buffer object containing the full data
- console.log(data.toString())
- }))
-```
-
-Note that when you use the *callback* method like this, the resulting `data` parameter is a concatenation of all `Buffer` objects in the list. If you want to avoid the overhead of this concatenation (in cases of extreme performance consciousness), then avoid the *callback* method and just listen to `'end'` instead, like a standard Stream.
-
-Or to fetch a URL using [hyperquest](https://github.com/substack/hyperquest) (should work with [request](http://github.com/mikeal/request) and even plain Node http too!):
-```js
-const hyperquest = require('hyperquest')
- , bl = require('bl')
- , url = 'https://raw.github.com/rvagg/bl/master/README.md'
-
-hyperquest(url).pipe(bl(function (err, data) {
- console.log(data.toString())
-}))
-```
-
-Or, use it as a readable stream to recompose a list of Buffers to an output source:
-
-```js
-const BufferList = require('bl')
- , fs = require('fs')
-
-var bl = new BufferList()
-bl.append(Buffer.from('abcd'))
-bl.append(Buffer.from('efg'))
-bl.append(Buffer.from('hi'))
-bl.append(Buffer.from('j'))
-
-bl.pipe(fs.createWriteStream('gibberish.txt'))
-```
-
-## API
-
- * new BufferList([ callback ])
- * bl.length
- * bl.append(buffer)
- * bl.get(index)
- * bl.indexOf(value[, byteOffset][, encoding])
- * bl.slice([ start[, end ] ])
- * bl.shallowSlice([ start[, end ] ])
- * bl.copy(dest, [ destStart, [ srcStart [, srcEnd ] ] ])
- * bl.duplicate()
- * bl.consume(bytes)
- * bl.toString([encoding, [ start, [ end ]]])
- * bl.readDoubleBE()
, bl.readDoubleLE()
, bl.readFloatBE()
, bl.readFloatLE()
, bl.readInt32BE()
, bl.readInt32LE()
, bl.readUInt32BE()
, bl.readUInt32LE()
, bl.readInt16BE()
, bl.readInt16LE()
, bl.readUInt16BE()
, bl.readUInt16LE()
, bl.readInt8()
, bl.readUInt8()
- * Streams
-
---------------------------------------------------------
-
-### new BufferList([ callback | Buffer | Buffer array | BufferList | BufferList array | String ])
-The constructor takes an optional callback, if supplied, the callback will be called with an error argument followed by a reference to the **bl** instance, when `bl.end()` is called (i.e. from a piped stream). This is a convenient method of collecting the entire contents of a stream, particularly when the stream is *chunky*, such as a network stream.
-
-Normally, no arguments are required for the constructor, but you can initialise the list by passing in a single `Buffer` object or an array of `Buffer` object.
-
-`new` is not strictly required, if you don't instantiate a new object, it will be done automatically for you so you can create a new instance simply with:
-
-```js
-var bl = require('bl')
-var myinstance = bl()
-
-// equivalent to:
-
-var BufferList = require('bl')
-var myinstance = new BufferList()
-```
-
---------------------------------------------------------
-
-### bl.length
-Get the length of the list in bytes. This is the sum of the lengths of all of the buffers contained in the list, minus any initial offset for a semi-consumed buffer at the beginning. Should accurately represent the total number of bytes that can be read from the list.
-
---------------------------------------------------------
-
-### bl.append(Buffer | Buffer array | BufferList | BufferList array | String)
-`append(buffer)` adds an additional buffer or BufferList to the internal list. `this` is returned so it can be chained.
-
---------------------------------------------------------
-
-### bl.get(index)
-`get()` will return the byte at the specified index.
-
---------------------------------------------------------
-
-### bl.indexOf(value[, byteOffset][, encoding])
-`get()` will return the byte at the specified index.
-`indexOf()` method returns the first index at which a given element can be found in the BufferList, or -1 if it is not present.
-
---------------------------------------------------------
-
-### bl.slice([ start, [ end ] ])
-`slice()` returns a new `Buffer` object containing the bytes within the range specified. Both `start` and `end` are optional and will default to the beginning and end of the list respectively.
-
-If the requested range spans a single internal buffer then a slice of that buffer will be returned which shares the original memory range of that Buffer. If the range spans multiple buffers then copy operations will likely occur to give you a uniform Buffer.
-
---------------------------------------------------------
-
-### bl.shallowSlice([ start, [ end ] ])
-`shallowSlice()` returns a new `BufferList` object containing the bytes within the range specified. Both `start` and `end` are optional and will default to the beginning and end of the list respectively.
-
-No copies will be performed. All buffers in the result share memory with the original list.
-
---------------------------------------------------------
-
-### bl.copy(dest, [ destStart, [ srcStart [, srcEnd ] ] ])
-`copy()` copies the content of the list in the `dest` buffer, starting from `destStart` and containing the bytes within the range specified with `srcStart` to `srcEnd`. `destStart`, `start` and `end` are optional and will default to the beginning of the `dest` buffer, and the beginning and end of the list respectively.
-
---------------------------------------------------------
-
-### bl.duplicate()
-`duplicate()` performs a **shallow-copy** of the list. The internal Buffers remains the same, so if you change the underlying Buffers, the change will be reflected in both the original and the duplicate. This method is needed if you want to call `consume()` or `pipe()` and still keep the original list.Example:
-
-```js
-var bl = new BufferList()
-
-bl.append('hello')
-bl.append(' world')
-bl.append('\n')
-
-bl.duplicate().pipe(process.stdout, { end: false })
-
-console.log(bl.toString())
-```
-
---------------------------------------------------------
-
-### bl.consume(bytes)
-`consume()` will shift bytes *off the start of the list*. The number of bytes consumed don't need to line up with the sizes of the internal Buffers—initial offsets will be calculated accordingly in order to give you a consistent view of the data.
-
---------------------------------------------------------
-
-### bl.toString([encoding, [ start, [ end ]]])
-`toString()` will return a string representation of the buffer. The optional `start` and `end` arguments are passed on to `slice()`, while the `encoding` is passed on to `toString()` of the resulting Buffer. See the [Buffer#toString()](http://nodejs.org/docs/latest/api/buffer.html#buffer_buf_tostring_encoding_start_end) documentation for more information.
-
---------------------------------------------------------
-
-### bl.readDoubleBE(), bl.readDoubleLE(), bl.readFloatBE(), bl.readFloatLE(), bl.readInt32BE(), bl.readInt32LE(), bl.readUInt32BE(), bl.readUInt32LE(), bl.readInt16BE(), bl.readInt16LE(), bl.readUInt16BE(), bl.readUInt16LE(), bl.readInt8(), bl.readUInt8()
-
-All of the standard byte-reading methods of the `Buffer` interface are implemented and will operate across internal Buffer boundaries transparently.
-
-See the [Buffer](http://nodejs.org/docs/latest/api/buffer.html)
documentation for how these work.
-
---------------------------------------------------------
-
-### Streams
-**bl** is a Node **[Duplex Stream](http://nodejs.org/docs/latest/api/stream.html#stream_class_stream_duplex)**, so it can be read from and written to like a standard Node stream. You can also `pipe()` to and from a **bl** instance.
-
---------------------------------------------------------
-
-## Contributors
-
-**bl** is brought to you by the following hackers:
-
- * [Rod Vagg](https://github.com/rvagg)
- * [Matteo Collina](https://github.com/mcollina)
- * [Jarett Cruger](https://github.com/jcrugzz)
-
-=======
-
-
-## License & copyright
-
-Copyright (c) 2013-2018 bl contributors (listed above).
-
-bl is licensed under the MIT license. All rights not explicitly granted in the MIT license are reserved. See the included LICENSE.md file for more details.
diff --git a/node_modules/bl/bl.js b/node_modules/bl/bl.js
deleted file mode 100644
index 52c3740..0000000
--- a/node_modules/bl/bl.js
+++ /dev/null
@@ -1,392 +0,0 @@
-'use strict'
-var DuplexStream = require('readable-stream').Duplex
- , util = require('util')
- , Buffer = require('safe-buffer').Buffer
-
-function BufferList (callback) {
- if (!(this instanceof BufferList))
- return new BufferList(callback)
-
- this._bufs = []
- this.length = 0
-
- if (typeof callback == 'function') {
- this._callback = callback
-
- var piper = function piper (err) {
- if (this._callback) {
- this._callback(err)
- this._callback = null
- }
- }.bind(this)
-
- this.on('pipe', function onPipe (src) {
- src.on('error', piper)
- })
- this.on('unpipe', function onUnpipe (src) {
- src.removeListener('error', piper)
- })
- } else {
- this.append(callback)
- }
-
- DuplexStream.call(this)
-}
-
-
-util.inherits(BufferList, DuplexStream)
-
-
-BufferList.prototype._offset = function _offset (offset) {
- var tot = 0, i = 0, _t
- if (offset === 0) return [ 0, 0 ]
- for (; i < this._bufs.length; i++) {
- _t = tot + this._bufs[i].length
- if (offset < _t || i == this._bufs.length - 1) {
- return [ i, offset - tot ]
- }
- tot = _t
- }
-}
-
-BufferList.prototype._reverseOffset = function (blOffset) {
- var bufferId = blOffset[0]
- var offset = blOffset[1]
- for (var i = 0; i < bufferId; i++) {
- offset += this._bufs[i].length
- }
- return offset
-}
-
-BufferList.prototype.append = function append (buf) {
- var i = 0
-
- if (Buffer.isBuffer(buf)) {
- this._appendBuffer(buf)
- } else if (Array.isArray(buf)) {
- for (; i < buf.length; i++)
- this.append(buf[i])
- } else if (buf instanceof BufferList) {
- // unwrap argument into individual BufferLists
- for (; i < buf._bufs.length; i++)
- this.append(buf._bufs[i])
- } else if (buf != null) {
- // coerce number arguments to strings, since Buffer(number) does
- // uninitialized memory allocation
- if (typeof buf == 'number')
- buf = buf.toString()
-
- this._appendBuffer(Buffer.from(buf))
- }
-
- return this
-}
-
-
-BufferList.prototype._appendBuffer = function appendBuffer (buf) {
- this._bufs.push(buf)
- this.length += buf.length
-}
-
-
-BufferList.prototype._write = function _write (buf, encoding, callback) {
- this._appendBuffer(buf)
-
- if (typeof callback == 'function')
- callback()
-}
-
-
-BufferList.prototype._read = function _read (size) {
- if (!this.length)
- return this.push(null)
-
- size = Math.min(size, this.length)
- this.push(this.slice(0, size))
- this.consume(size)
-}
-
-
-BufferList.prototype.end = function end (chunk) {
- DuplexStream.prototype.end.call(this, chunk)
-
- if (this._callback) {
- this._callback(null, this.slice())
- this._callback = null
- }
-}
-
-
-BufferList.prototype.get = function get (index) {
- if (index > this.length || index < 0) {
- return undefined
- }
- var offset = this._offset(index)
- return this._bufs[offset[0]][offset[1]]
-}
-
-
-BufferList.prototype.slice = function slice (start, end) {
- if (typeof start == 'number' && start < 0)
- start += this.length
- if (typeof end == 'number' && end < 0)
- end += this.length
- return this.copy(null, 0, start, end)
-}
-
-
-BufferList.prototype.copy = function copy (dst, dstStart, srcStart, srcEnd) {
- if (typeof srcStart != 'number' || srcStart < 0)
- srcStart = 0
- if (typeof srcEnd != 'number' || srcEnd > this.length)
- srcEnd = this.length
- if (srcStart >= this.length)
- return dst || Buffer.alloc(0)
- if (srcEnd <= 0)
- return dst || Buffer.alloc(0)
-
- var copy = !!dst
- , off = this._offset(srcStart)
- , len = srcEnd - srcStart
- , bytes = len
- , bufoff = (copy && dstStart) || 0
- , start = off[1]
- , l
- , i
-
- // copy/slice everything
- if (srcStart === 0 && srcEnd == this.length) {
- if (!copy) { // slice, but full concat if multiple buffers
- return this._bufs.length === 1
- ? this._bufs[0]
- : Buffer.concat(this._bufs, this.length)
- }
-
- // copy, need to copy individual buffers
- for (i = 0; i < this._bufs.length; i++) {
- this._bufs[i].copy(dst, bufoff)
- bufoff += this._bufs[i].length
- }
-
- return dst
- }
-
- // easy, cheap case where it's a subset of one of the buffers
- if (bytes <= this._bufs[off[0]].length - start) {
- return copy
- ? this._bufs[off[0]].copy(dst, dstStart, start, start + bytes)
- : this._bufs[off[0]].slice(start, start + bytes)
- }
-
- if (!copy) // a slice, we need something to copy in to
- dst = Buffer.allocUnsafe(len)
-
- for (i = off[0]; i < this._bufs.length; i++) {
- l = this._bufs[i].length - start
-
- if (bytes > l) {
- this._bufs[i].copy(dst, bufoff, start)
- bufoff += l
- } else {
- this._bufs[i].copy(dst, bufoff, start, start + bytes)
- bufoff += l
- break
- }
-
- bytes -= l
-
- if (start)
- start = 0
- }
-
- // safeguard so that we don't return uninitialized memory
- if (dst.length > bufoff) return dst.slice(0, bufoff)
-
- return dst
-}
-
-BufferList.prototype.shallowSlice = function shallowSlice (start, end) {
- start = start || 0
- end = typeof end !== 'number' ? this.length : end
-
- if (start < 0)
- start += this.length
- if (end < 0)
- end += this.length
-
- if (start === end) {
- return new BufferList()
- }
- var startOffset = this._offset(start)
- , endOffset = this._offset(end)
- , buffers = this._bufs.slice(startOffset[0], endOffset[0] + 1)
-
- if (endOffset[1] == 0)
- buffers.pop()
- else
- buffers[buffers.length-1] = buffers[buffers.length-1].slice(0, endOffset[1])
-
- if (startOffset[1] != 0)
- buffers[0] = buffers[0].slice(startOffset[1])
-
- return new BufferList(buffers)
-}
-
-BufferList.prototype.toString = function toString (encoding, start, end) {
- return this.slice(start, end).toString(encoding)
-}
-
-BufferList.prototype.consume = function consume (bytes) {
- // first, normalize the argument, in accordance with how Buffer does it
- bytes = Math.trunc(bytes)
- // do nothing if not a positive number
- if (Number.isNaN(bytes) || bytes <= 0) return this
-
- while (this._bufs.length) {
- if (bytes >= this._bufs[0].length) {
- bytes -= this._bufs[0].length
- this.length -= this._bufs[0].length
- this._bufs.shift()
- } else {
- this._bufs[0] = this._bufs[0].slice(bytes)
- this.length -= bytes
- break
- }
- }
- return this
-}
-
-
-BufferList.prototype.duplicate = function duplicate () {
- var i = 0
- , copy = new BufferList()
-
- for (; i < this._bufs.length; i++)
- copy.append(this._bufs[i])
-
- return copy
-}
-
-
-BufferList.prototype.destroy = function destroy () {
- this._bufs.length = 0
- this.length = 0
- this.push(null)
-}
-
-
-BufferList.prototype.indexOf = function (search, offset, encoding) {
- if (encoding === undefined && typeof offset === 'string') {
- encoding = offset
- offset = undefined
- }
- if (typeof search === 'function' || Array.isArray(search)) {
- throw new TypeError('The "value" argument must be one of type string, Buffer, BufferList, or Uint8Array.')
- } else if (typeof search === 'number') {
- search = Buffer.from([search])
- } else if (typeof search === 'string') {
- search = Buffer.from(search, encoding)
- } else if (search instanceof BufferList) {
- search = search.slice()
- } else if (!Buffer.isBuffer(search)) {
- search = Buffer.from(search)
- }
-
- offset = Number(offset || 0)
- if (isNaN(offset)) {
- offset = 0
- }
-
- if (offset < 0) {
- offset = this.length + offset
- }
-
- if (offset < 0) {
- offset = 0
- }
-
- if (search.length === 0) {
- return offset > this.length ? this.length : offset
- }
-
- var blOffset = this._offset(offset)
- var blIndex = blOffset[0] // index of which internal buffer we're working on
- var buffOffset = blOffset[1] // offset of the internal buffer we're working on
-
- // scan over each buffer
- for (blIndex; blIndex < this._bufs.length; blIndex++) {
- var buff = this._bufs[blIndex]
- while(buffOffset < buff.length) {
- var availableWindow = buff.length - buffOffset
- if (availableWindow >= search.length) {
- var nativeSearchResult = buff.indexOf(search, buffOffset)
- if (nativeSearchResult !== -1) {
- return this._reverseOffset([blIndex, nativeSearchResult])
- }
- buffOffset = buff.length - search.length + 1 // end of native search window
- } else {
- var revOffset = this._reverseOffset([blIndex, buffOffset])
- if (this._match(revOffset, search)) {
- return revOffset
- }
- buffOffset++
- }
- }
- buffOffset = 0
- }
- return -1
-}
-
-BufferList.prototype._match = function(offset, search) {
- if (this.length - offset < search.length) {
- return false
- }
- for (var searchOffset = 0; searchOffset < search.length ; searchOffset++) {
- if(this.get(offset + searchOffset) !== search[searchOffset]){
- return false
- }
- }
- return true
-}
-
-
-;(function () {
- var methods = {
- 'readDoubleBE' : 8
- , 'readDoubleLE' : 8
- , 'readFloatBE' : 4
- , 'readFloatLE' : 4
- , 'readInt32BE' : 4
- , 'readInt32LE' : 4
- , 'readUInt32BE' : 4
- , 'readUInt32LE' : 4
- , 'readInt16BE' : 2
- , 'readInt16LE' : 2
- , 'readUInt16BE' : 2
- , 'readUInt16LE' : 2
- , 'readInt8' : 1
- , 'readUInt8' : 1
- , 'readIntBE' : null
- , 'readIntLE' : null
- , 'readUIntBE' : null
- , 'readUIntLE' : null
- }
-
- for (var m in methods) {
- (function (m) {
- if (methods[m] === null) {
- BufferList.prototype[m] = function (offset, byteLength) {
- return this.slice(offset, offset + byteLength)[m](0, byteLength)
- }
- }
- else {
- BufferList.prototype[m] = function (offset) {
- return this.slice(offset, offset + methods[m])[m](0)
- }
- }
- }(m))
- }
-}())
-
-
-module.exports = BufferList
diff --git a/node_modules/bl/package.json b/node_modules/bl/package.json
deleted file mode 100644
index 6c0ddb8..0000000
--- a/node_modules/bl/package.json
+++ /dev/null
@@ -1,63 +0,0 @@
-{
- "_from": "bl@^2.2.1",
- "_id": "bl@2.2.1",
- "_inBundle": false,
- "_integrity": "sha512-6Pesp1w0DEX1N550i/uGV/TqucVL4AM/pgThFSN/Qq9si1/DF9aIHs1BxD8V/QU0HoeHO6cQRTAuYnLPKq1e4g==",
- "_location": "/bl",
- "_phantomChildren": {},
- "_requested": {
- "type": "range",
- "registry": true,
- "raw": "bl@^2.2.1",
- "name": "bl",
- "escapedName": "bl",
- "rawSpec": "^2.2.1",
- "saveSpec": null,
- "fetchSpec": "^2.2.1"
- },
- "_requiredBy": [
- "/mongodb"
- ],
- "_resolved": "https://registry.npmjs.org/bl/-/bl-2.2.1.tgz",
- "_shasum": "8c11a7b730655c5d56898cdc871224f40fd901d5",
- "_spec": "bl@^2.2.1",
- "_where": "/Users/jasonagus/Desktop/Skyblock Guide/node_modules/mongodb",
- "authors": [
- "Rod Vagg (https://github.com/rvagg)",
- "Matteo Collina (https://github.com/mcollina)",
- "Jarett Cruger (https://github.com/jcrugzz)"
- ],
- "bugs": {
- "url": "https://github.com/rvagg/bl/issues"
- },
- "bundleDependencies": false,
- "dependencies": {
- "readable-stream": "^2.3.5",
- "safe-buffer": "^5.1.1"
- },
- "deprecated": false,
- "description": "Buffer List: collect buffers and access with a standard readable Buffer interface, streamable too!",
- "devDependencies": {
- "faucet": "0.0.1",
- "hash_file": "~0.1.1",
- "tape": "~4.9.0"
- },
- "homepage": "https://github.com/rvagg/bl",
- "keywords": [
- "buffer",
- "buffers",
- "stream",
- "awesomesauce"
- ],
- "license": "MIT",
- "main": "bl.js",
- "name": "bl",
- "repository": {
- "type": "git",
- "url": "git+https://github.com/rvagg/bl.git"
- },
- "scripts": {
- "test": "node test/test.js | faucet"
- },
- "version": "2.2.1"
-}
diff --git a/node_modules/bl/test/indexOf.js b/node_modules/bl/test/indexOf.js
deleted file mode 100644
index 68435e1..0000000
--- a/node_modules/bl/test/indexOf.js
+++ /dev/null
@@ -1,463 +0,0 @@
-'use strict'
-
-var tape = require('tape')
- , BufferList = require('../')
- , Buffer = require('safe-buffer').Buffer
-
-tape('indexOf single byte needle', t => {
- const bl = new BufferList(['abcdefg', 'abcdefg', '12345'])
- t.equal(bl.indexOf('e'), 4)
- t.equal(bl.indexOf('e', 5), 11)
- t.equal(bl.indexOf('e', 12), -1)
- t.equal(bl.indexOf('5'), 18)
- t.end()
-})
-
-tape('indexOf multiple byte needle', t => {
- const bl = new BufferList(['abcdefg', 'abcdefg'])
- t.equal(bl.indexOf('ef'), 4)
- t.equal(bl.indexOf('ef', 5), 11)
- t.end()
-})
-
-tape('indexOf multiple byte needles across buffer boundaries', t => {
- const bl = new BufferList(['abcdefg', 'abcdefg'])
- t.equal(bl.indexOf('fgabc'), 5)
- t.end()
-})
-
-tape('indexOf takes a buffer list search', t => {
- const bl = new BufferList(['abcdefg', 'abcdefg'])
- const search = new BufferList('fgabc')
- t.equal(bl.indexOf(search), 5)
- t.end()
-})
-
-tape('indexOf a zero byte needle', t => {
- const b = new BufferList('abcdef')
- const buf_empty = Buffer.from('')
- t.equal(b.indexOf(''), 0)
- t.equal(b.indexOf('', 1), 1)
- t.equal(b.indexOf('', b.length + 1), b.length)
- t.equal(b.indexOf('', Infinity), b.length)
- t.equal(b.indexOf(buf_empty), 0)
- t.equal(b.indexOf(buf_empty, 1), 1)
- t.equal(b.indexOf(buf_empty, b.length + 1), b.length)
- t.equal(b.indexOf(buf_empty, Infinity), b.length)
- t.end()
-})
-
-tape('indexOf buffers smaller and larger than the needle', t => {
- const bl = new BufferList(['abcdefg', 'a', 'bcdefg', 'a', 'bcfgab'])
- t.equal(bl.indexOf('fgabc'), 5)
- t.equal(bl.indexOf('fgabc', 6), 12)
- t.equal(bl.indexOf('fgabc', 13), -1)
- t.end()
-})
-
-// only present in node 6+
-;(process.version.substr(1).split('.')[0] >= 6) && tape('indexOf latin1 and binary encoding', t => {
- const b = new BufferList('abcdef')
-
- // test latin1 encoding
- t.equal(
- new BufferList(Buffer.from(b.toString('latin1'), 'latin1'))
- .indexOf('d', 0, 'latin1'),
- 3
- )
- t.equal(
- new BufferList(Buffer.from(b.toString('latin1'), 'latin1'))
- .indexOf(Buffer.from('d', 'latin1'), 0, 'latin1'),
- 3
- )
- t.equal(
- new BufferList(Buffer.from('aa\u00e8aa', 'latin1'))
- .indexOf('\u00e8', 'latin1'),
- 2
- )
- t.equal(
- new BufferList(Buffer.from('\u00e8', 'latin1'))
- .indexOf('\u00e8', 'latin1'),
- 0
- )
- t.equal(
- new BufferList(Buffer.from('\u00e8', 'latin1'))
- .indexOf(Buffer.from('\u00e8', 'latin1'), 'latin1'),
- 0
- )
-
- // test binary encoding
- t.equal(
- new BufferList(Buffer.from(b.toString('binary'), 'binary'))
- .indexOf('d', 0, 'binary'),
- 3
- )
- t.equal(
- new BufferList(Buffer.from(b.toString('binary'), 'binary'))
- .indexOf(Buffer.from('d', 'binary'), 0, 'binary'),
- 3
- )
- t.equal(
- new BufferList(Buffer.from('aa\u00e8aa', 'binary'))
- .indexOf('\u00e8', 'binary'),
- 2
- )
- t.equal(
- new BufferList(Buffer.from('\u00e8', 'binary'))
- .indexOf('\u00e8', 'binary'),
- 0
- )
- t.equal(
- new BufferList(Buffer.from('\u00e8', 'binary'))
- .indexOf(Buffer.from('\u00e8', 'binary'), 'binary'),
- 0
- )
- t.end()
-})
-
-tape('indexOf the entire nodejs10 buffer test suite', t => {
- const b = new BufferList('abcdef')
- const buf_a = Buffer.from('a')
- const buf_bc = Buffer.from('bc')
- const buf_f = Buffer.from('f')
- const buf_z = Buffer.from('z')
-
- const stringComparison = 'abcdef'
-
- t.equal(b.indexOf('a'), 0)
- t.equal(b.indexOf('a', 1), -1)
- t.equal(b.indexOf('a', -1), -1)
- t.equal(b.indexOf('a', -4), -1)
- t.equal(b.indexOf('a', -b.length), 0)
- t.equal(b.indexOf('a', NaN), 0)
- t.equal(b.indexOf('a', -Infinity), 0)
- t.equal(b.indexOf('a', Infinity), -1)
- t.equal(b.indexOf('bc'), 1)
- t.equal(b.indexOf('bc', 2), -1)
- t.equal(b.indexOf('bc', -1), -1)
- t.equal(b.indexOf('bc', -3), -1)
- t.equal(b.indexOf('bc', -5), 1)
- t.equal(b.indexOf('bc', NaN), 1)
- t.equal(b.indexOf('bc', -Infinity), 1)
- t.equal(b.indexOf('bc', Infinity), -1)
- t.equal(b.indexOf('f'), b.length - 1)
- t.equal(b.indexOf('z'), -1)
- // empty search tests
- t.equal(b.indexOf(buf_a), 0)
- t.equal(b.indexOf(buf_a, 1), -1)
- t.equal(b.indexOf(buf_a, -1), -1)
- t.equal(b.indexOf(buf_a, -4), -1)
- t.equal(b.indexOf(buf_a, -b.length), 0)
- t.equal(b.indexOf(buf_a, NaN), 0)
- t.equal(b.indexOf(buf_a, -Infinity), 0)
- t.equal(b.indexOf(buf_a, Infinity), -1)
- t.equal(b.indexOf(buf_bc), 1)
- t.equal(b.indexOf(buf_bc, 2), -1)
- t.equal(b.indexOf(buf_bc, -1), -1)
- t.equal(b.indexOf(buf_bc, -3), -1)
- t.equal(b.indexOf(buf_bc, -5), 1)
- t.equal(b.indexOf(buf_bc, NaN), 1)
- t.equal(b.indexOf(buf_bc, -Infinity), 1)
- t.equal(b.indexOf(buf_bc, Infinity), -1)
- t.equal(b.indexOf(buf_f), b.length - 1)
- t.equal(b.indexOf(buf_z), -1)
- t.equal(b.indexOf(0x61), 0)
- t.equal(b.indexOf(0x61, 1), -1)
- t.equal(b.indexOf(0x61, -1), -1)
- t.equal(b.indexOf(0x61, -4), -1)
- t.equal(b.indexOf(0x61, -b.length), 0)
- t.equal(b.indexOf(0x61, NaN), 0)
- t.equal(b.indexOf(0x61, -Infinity), 0)
- t.equal(b.indexOf(0x61, Infinity), -1)
- t.equal(b.indexOf(0x0), -1)
-
- // test offsets
- t.equal(b.indexOf('d', 2), 3)
- t.equal(b.indexOf('f', 5), 5)
- t.equal(b.indexOf('f', -1), 5)
- t.equal(b.indexOf('f', 6), -1)
-
- t.equal(b.indexOf(Buffer.from('d'), 2), 3)
- t.equal(b.indexOf(Buffer.from('f'), 5), 5)
- t.equal(b.indexOf(Buffer.from('f'), -1), 5)
- t.equal(b.indexOf(Buffer.from('f'), 6), -1)
-
- t.equal(Buffer.from('ff').indexOf(Buffer.from('f'), 1, 'ucs2'), -1)
-
- // test invalid and uppercase encoding
- t.equal(b.indexOf('b', 'utf8'), 1)
- t.equal(b.indexOf('b', 'UTF8'), 1)
- t.equal(b.indexOf('62', 'HEX'), 1)
- t.throws(() => b.indexOf('bad', 'enc'), TypeError)
-
- // test hex encoding
- t.equal(
- Buffer.from(b.toString('hex'), 'hex')
- .indexOf('64', 0, 'hex'),
- 3
- )
- t.equal(
- Buffer.from(b.toString('hex'), 'hex')
- .indexOf(Buffer.from('64', 'hex'), 0, 'hex'),
- 3
- )
-
- // test base64 encoding
- t.equal(
- Buffer.from(b.toString('base64'), 'base64')
- .indexOf('ZA==', 0, 'base64'),
- 3
- )
- t.equal(
- Buffer.from(b.toString('base64'), 'base64')
- .indexOf(Buffer.from('ZA==', 'base64'), 0, 'base64'),
- 3
- )
-
- // test ascii encoding
- t.equal(
- Buffer.from(b.toString('ascii'), 'ascii')
- .indexOf('d', 0, 'ascii'),
- 3
- )
- t.equal(
- Buffer.from(b.toString('ascii'), 'ascii')
- .indexOf(Buffer.from('d', 'ascii'), 0, 'ascii'),
- 3
- )
-
- // test optional offset with passed encoding
- t.equal(Buffer.from('aaaa0').indexOf('30', 'hex'), 4)
- t.equal(Buffer.from('aaaa00a').indexOf('3030', 'hex'), 4)
-
- {
- // test usc2 encoding
- const twoByteString = Buffer.from('\u039a\u0391\u03a3\u03a3\u0395', 'ucs2')
-
- t.equal(8, twoByteString.indexOf('\u0395', 4, 'ucs2'))
- t.equal(6, twoByteString.indexOf('\u03a3', -4, 'ucs2'))
- t.equal(4, twoByteString.indexOf('\u03a3', -6, 'ucs2'))
- t.equal(4, twoByteString.indexOf(
- Buffer.from('\u03a3', 'ucs2'), -6, 'ucs2'))
- t.equal(-1, twoByteString.indexOf('\u03a3', -2, 'ucs2'))
- }
-
- const mixedByteStringUcs2 =
- Buffer.from('\u039a\u0391abc\u03a3\u03a3\u0395', 'ucs2')
- t.equal(6, mixedByteStringUcs2.indexOf('bc', 0, 'ucs2'))
- t.equal(10, mixedByteStringUcs2.indexOf('\u03a3', 0, 'ucs2'))
- t.equal(-1, mixedByteStringUcs2.indexOf('\u0396', 0, 'ucs2'))
-
- t.equal(
- 6, mixedByteStringUcs2.indexOf(Buffer.from('bc', 'ucs2'), 0, 'ucs2'))
- t.equal(
- 10, mixedByteStringUcs2.indexOf(Buffer.from('\u03a3', 'ucs2'), 0, 'ucs2'))
- t.equal(
- -1, mixedByteStringUcs2.indexOf(Buffer.from('\u0396', 'ucs2'), 0, 'ucs2'))
-
- {
- const twoByteString = Buffer.from('\u039a\u0391\u03a3\u03a3\u0395', 'ucs2')
-
- // Test single char pattern
- t.equal(0, twoByteString.indexOf('\u039a', 0, 'ucs2'))
- let index = twoByteString.indexOf('\u0391', 0, 'ucs2')
- t.equal(2, index, `Alpha - at index ${index}`)
- index = twoByteString.indexOf('\u03a3', 0, 'ucs2')
- t.equal(4, index, `First Sigma - at index ${index}`)
- index = twoByteString.indexOf('\u03a3', 6, 'ucs2')
- t.equal(6, index, `Second Sigma - at index ${index}`)
- index = twoByteString.indexOf('\u0395', 0, 'ucs2')
- t.equal(8, index, `Epsilon - at index ${index}`)
- index = twoByteString.indexOf('\u0392', 0, 'ucs2')
- t.equal(-1, index, `Not beta - at index ${index}`)
-
- // Test multi-char pattern
- index = twoByteString.indexOf('\u039a\u0391', 0, 'ucs2')
- t.equal(0, index, `Lambda Alpha - at index ${index}`)
- index = twoByteString.indexOf('\u0391\u03a3', 0, 'ucs2')
- t.equal(2, index, `Alpha Sigma - at index ${index}`)
- index = twoByteString.indexOf('\u03a3\u03a3', 0, 'ucs2')
- t.equal(4, index, `Sigma Sigma - at index ${index}`)
- index = twoByteString.indexOf('\u03a3\u0395', 0, 'ucs2')
- t.equal(6, index, `Sigma Epsilon - at index ${index}`)
- }
-
- const mixedByteStringUtf8 = Buffer.from('\u039a\u0391abc\u03a3\u03a3\u0395')
- t.equal(5, mixedByteStringUtf8.indexOf('bc'))
- t.equal(5, mixedByteStringUtf8.indexOf('bc', 5))
- t.equal(5, mixedByteStringUtf8.indexOf('bc', -8))
- t.equal(7, mixedByteStringUtf8.indexOf('\u03a3'))
- t.equal(-1, mixedByteStringUtf8.indexOf('\u0396'))
-
-
- // Test complex string indexOf algorithms. Only trigger for long strings.
- // Long string that isn't a simple repeat of a shorter string.
- let longString = 'A'
- for (let i = 66; i < 76; i++) { // from 'B' to 'K'
- longString = longString + String.fromCharCode(i) + longString
- }
-
- const longBufferString = Buffer.from(longString)
-
- // pattern of 15 chars, repeated every 16 chars in long
- let pattern = 'ABACABADABACABA'
- for (let i = 0; i < longBufferString.length - pattern.length; i += 7) {
- const index = longBufferString.indexOf(pattern, i)
- t.equal((i + 15) & ~0xf, index,
- `Long ABACABA...-string at index ${i}`)
- }
-
- let index = longBufferString.indexOf('AJABACA')
- t.equal(510, index, `Long AJABACA, First J - at index ${index}`)
- index = longBufferString.indexOf('AJABACA', 511)
- t.equal(1534, index, `Long AJABACA, Second J - at index ${index}`)
-
- pattern = 'JABACABADABACABA'
- index = longBufferString.indexOf(pattern)
- t.equal(511, index, `Long JABACABA..., First J - at index ${index}`)
- index = longBufferString.indexOf(pattern, 512)
- t.equal(
- 1535, index, `Long JABACABA..., Second J - at index ${index}`)
-
- // Search for a non-ASCII string in a pure ASCII string.
- const asciiString = Buffer.from(
- 'arglebargleglopglyfarglebargleglopglyfarglebargleglopglyf')
- t.equal(-1, asciiString.indexOf('\x2061'))
- t.equal(3, asciiString.indexOf('leb', 0))
-
- // Search in string containing many non-ASCII chars.
- const allCodePoints = []
- for (let i = 0; i < 65536; i++) allCodePoints[i] = i
- const allCharsString = String.fromCharCode.apply(String, allCodePoints)
- const allCharsBufferUtf8 = Buffer.from(allCharsString)
- const allCharsBufferUcs2 = Buffer.from(allCharsString, 'ucs2')
-
- // Search for string long enough to trigger complex search with ASCII pattern
- // and UC16 subject.
- t.equal(-1, allCharsBufferUtf8.indexOf('notfound'))
- t.equal(-1, allCharsBufferUcs2.indexOf('notfound'))
-
- // Needle is longer than haystack, but only because it's encoded as UTF-16
- t.equal(Buffer.from('aaaa').indexOf('a'.repeat(4), 'ucs2'), -1)
-
- t.equal(Buffer.from('aaaa').indexOf('a'.repeat(4), 'utf8'), 0)
- t.equal(Buffer.from('aaaa').indexOf('你好', 'ucs2'), -1)
-
- // Haystack has odd length, but the needle is UCS2.
- t.equal(Buffer.from('aaaaa').indexOf('b', 'ucs2'), -1)
-
- {
- // Find substrings in Utf8.
- const lengths = [1, 3, 15]; // Single char, simple and complex.
- const indices = [0x5, 0x60, 0x400, 0x680, 0x7ee, 0xFF02, 0x16610, 0x2f77b]
- for (let lengthIndex = 0; lengthIndex < lengths.length; lengthIndex++) {
- for (let i = 0; i < indices.length; i++) {
- const index = indices[i]
- let length = lengths[lengthIndex]
-
- if (index + length > 0x7F) {
- length = 2 * length
- }
-
- if (index + length > 0x7FF) {
- length = 3 * length
- }
-
- if (index + length > 0xFFFF) {
- length = 4 * length
- }
-
- const patternBufferUtf8 = allCharsBufferUtf8.slice(index, index + length)
- t.equal(index, allCharsBufferUtf8.indexOf(patternBufferUtf8))
-
- const patternStringUtf8 = patternBufferUtf8.toString()
- t.equal(index, allCharsBufferUtf8.indexOf(patternStringUtf8))
- }
- }
- }
-
- {
- // Find substrings in Usc2.
- const lengths = [2, 4, 16]; // Single char, simple and complex.
- const indices = [0x5, 0x65, 0x105, 0x205, 0x285, 0x2005, 0x2085, 0xfff0]
- for (let lengthIndex = 0; lengthIndex < lengths.length; lengthIndex++) {
- for (let i = 0; i < indices.length; i++) {
- const index = indices[i] * 2
- const length = lengths[lengthIndex]
-
- const patternBufferUcs2 =
- allCharsBufferUcs2.slice(index, index + length)
- t.equal(
- index, allCharsBufferUcs2.indexOf(patternBufferUcs2, 0, 'ucs2'))
-
- const patternStringUcs2 = patternBufferUcs2.toString('ucs2')
- t.equal(
- index, allCharsBufferUcs2.indexOf(patternStringUcs2, 0, 'ucs2'))
- }
- }
- }
-
- [
- () => {},
- {},
- []
- ].forEach(val => {
- debugger
- t.throws(() => b.indexOf(val), TypeError, `"${JSON.stringify(val)}" should throw`)
- })
-
- // Test weird offset arguments.
- // The following offsets coerce to NaN or 0, searching the whole Buffer
- t.equal(b.indexOf('b', undefined), 1)
- t.equal(b.indexOf('b', {}), 1)
- t.equal(b.indexOf('b', 0), 1)
- t.equal(b.indexOf('b', null), 1)
- t.equal(b.indexOf('b', []), 1)
-
- // The following offset coerces to 2, in other words +[2] === 2
- t.equal(b.indexOf('b', [2]), -1)
-
- // Behavior should match String.indexOf()
- t.equal(
- b.indexOf('b', undefined),
- stringComparison.indexOf('b', undefined))
- t.equal(
- b.indexOf('b', {}),
- stringComparison.indexOf('b', {}))
- t.equal(
- b.indexOf('b', 0),
- stringComparison.indexOf('b', 0))
- t.equal(
- b.indexOf('b', null),
- stringComparison.indexOf('b', null))
- t.equal(
- b.indexOf('b', []),
- stringComparison.indexOf('b', []))
- t.equal(
- b.indexOf('b', [2]),
- stringComparison.indexOf('b', [2]))
-
- // test truncation of Number arguments to uint8
- {
- const buf = Buffer.from('this is a test')
- t.equal(buf.indexOf(0x6973), 3)
- t.equal(buf.indexOf(0x697320), 4)
- t.equal(buf.indexOf(0x69732069), 2)
- t.equal(buf.indexOf(0x697374657374), 0)
- t.equal(buf.indexOf(0x69737374), 0)
- t.equal(buf.indexOf(0x69737465), 11)
- t.equal(buf.indexOf(0x69737465), 11)
- t.equal(buf.indexOf(-140), 0)
- t.equal(buf.indexOf(-152), 1)
- t.equal(buf.indexOf(0xff), -1)
- t.equal(buf.indexOf(0xffff), -1)
- }
-
- // Test that Uint8Array arguments are okay.
- {
- const needle = new Uint8Array([ 0x66, 0x6f, 0x6f ])
- const haystack = new BufferList(Buffer.from('a foo b foo'))
- t.equal(haystack.indexOf(needle), 2)
- }
- t.end()
-})
diff --git a/node_modules/bl/test/test.js b/node_modules/bl/test/test.js
deleted file mode 100644
index 42fcad4..0000000
--- a/node_modules/bl/test/test.js
+++ /dev/null
@@ -1,782 +0,0 @@
-'use strict'
-
-var tape = require('tape')
- , crypto = require('crypto')
- , fs = require('fs')
- , hash = require('hash_file')
- , BufferList = require('../')
- , Buffer = require('safe-buffer').Buffer
-
- , encodings =
- ('hex utf8 utf-8 ascii binary base64'
- + (process.browser ? '' : ' ucs2 ucs-2 utf16le utf-16le')).split(' ')
-
-// run the indexOf tests
-require('./indexOf')
-
-tape('single bytes from single buffer', function (t) {
- var bl = new BufferList()
- bl.append(Buffer.from('abcd'))
-
- t.equal(bl.length, 4)
- t.equal(bl.get(-1), undefined)
- t.equal(bl.get(0), 97)
- t.equal(bl.get(1), 98)
- t.equal(bl.get(2), 99)
- t.equal(bl.get(3), 100)
- t.equal(bl.get(4), undefined)
-
- t.end()
-})
-
-tape('single bytes from multiple buffers', function (t) {
- var bl = new BufferList()
- bl.append(Buffer.from('abcd'))
- bl.append(Buffer.from('efg'))
- bl.append(Buffer.from('hi'))
- bl.append(Buffer.from('j'))
-
- t.equal(bl.length, 10)
-
- t.equal(bl.get(0), 97)
- t.equal(bl.get(1), 98)
- t.equal(bl.get(2), 99)
- t.equal(bl.get(3), 100)
- t.equal(bl.get(4), 101)
- t.equal(bl.get(5), 102)
- t.equal(bl.get(6), 103)
- t.equal(bl.get(7), 104)
- t.equal(bl.get(8), 105)
- t.equal(bl.get(9), 106)
- t.end()
-})
-
-tape('multi bytes from single buffer', function (t) {
- var bl = new BufferList()
- bl.append(Buffer.from('abcd'))
-
- t.equal(bl.length, 4)
-
- t.equal(bl.slice(0, 4).toString('ascii'), 'abcd')
- t.equal(bl.slice(0, 3).toString('ascii'), 'abc')
- t.equal(bl.slice(1, 4).toString('ascii'), 'bcd')
- t.equal(bl.slice(-4, -1).toString('ascii'), 'abc')
-
- t.end()
-})
-
-tape('multi bytes from single buffer (negative indexes)', function (t) {
- var bl = new BufferList()
- bl.append(Buffer.from('buffer'))
-
- t.equal(bl.length, 6)
-
- t.equal(bl.slice(-6, -1).toString('ascii'), 'buffe')
- t.equal(bl.slice(-6, -2).toString('ascii'), 'buff')
- t.equal(bl.slice(-5, -2).toString('ascii'), 'uff')
-
- t.end()
-})
-
-tape('multiple bytes from multiple buffers', function (t) {
- var bl = new BufferList()
-
- bl.append(Buffer.from('abcd'))
- bl.append(Buffer.from('efg'))
- bl.append(Buffer.from('hi'))
- bl.append(Buffer.from('j'))
-
- t.equal(bl.length, 10)
-
- t.equal(bl.slice(0, 10).toString('ascii'), 'abcdefghij')
- t.equal(bl.slice(3, 10).toString('ascii'), 'defghij')
- t.equal(bl.slice(3, 6).toString('ascii'), 'def')
- t.equal(bl.slice(3, 8).toString('ascii'), 'defgh')
- t.equal(bl.slice(5, 10).toString('ascii'), 'fghij')
- t.equal(bl.slice(-7, -4).toString('ascii'), 'def')
-
- t.end()
-})
-
-tape('multiple bytes from multiple buffer lists', function (t) {
- var bl = new BufferList()
-
- bl.append(new BufferList([ Buffer.from('abcd'), Buffer.from('efg') ]))
- bl.append(new BufferList([ Buffer.from('hi'), Buffer.from('j') ]))
-
- t.equal(bl.length, 10)
-
- t.equal(bl.slice(0, 10).toString('ascii'), 'abcdefghij')
-
- t.equal(bl.slice(3, 10).toString('ascii'), 'defghij')
- t.equal(bl.slice(3, 6).toString('ascii'), 'def')
- t.equal(bl.slice(3, 8).toString('ascii'), 'defgh')
- t.equal(bl.slice(5, 10).toString('ascii'), 'fghij')
-
- t.end()
-})
-
-// same data as previous test, just using nested constructors
-tape('multiple bytes from crazy nested buffer lists', function (t) {
- var bl = new BufferList()
-
- bl.append(new BufferList([
- new BufferList([
- new BufferList(Buffer.from('abc'))
- , Buffer.from('d')
- , new BufferList(Buffer.from('efg'))
- ])
- , new BufferList([ Buffer.from('hi') ])
- , new BufferList(Buffer.from('j'))
- ]))
-
- t.equal(bl.length, 10)
-
- t.equal(bl.slice(0, 10).toString('ascii'), 'abcdefghij')
-
- t.equal(bl.slice(3, 10).toString('ascii'), 'defghij')
- t.equal(bl.slice(3, 6).toString('ascii'), 'def')
- t.equal(bl.slice(3, 8).toString('ascii'), 'defgh')
- t.equal(bl.slice(5, 10).toString('ascii'), 'fghij')
-
- t.end()
-})
-
-tape('append accepts arrays of Buffers', function (t) {
- var bl = new BufferList()
- bl.append(Buffer.from('abc'))
- bl.append([ Buffer.from('def') ])
- bl.append([ Buffer.from('ghi'), Buffer.from('jkl') ])
- bl.append([ Buffer.from('mnop'), Buffer.from('qrstu'), Buffer.from('vwxyz') ])
- t.equal(bl.length, 26)
- t.equal(bl.slice().toString('ascii'), 'abcdefghijklmnopqrstuvwxyz')
- t.end()
-})
-
-tape('append accepts arrays of BufferLists', function (t) {
- var bl = new BufferList()
- bl.append(Buffer.from('abc'))
- bl.append([ new BufferList('def') ])
- bl.append(new BufferList([ Buffer.from('ghi'), new BufferList('jkl') ]))
- bl.append([ Buffer.from('mnop'), new BufferList([ Buffer.from('qrstu'), Buffer.from('vwxyz') ]) ])
- t.equal(bl.length, 26)
- t.equal(bl.slice().toString('ascii'), 'abcdefghijklmnopqrstuvwxyz')
- t.end()
-})
-
-tape('append chainable', function (t) {
- var bl = new BufferList()
- t.ok(bl.append(Buffer.from('abcd')) === bl)
- t.ok(bl.append([ Buffer.from('abcd') ]) === bl)
- t.ok(bl.append(new BufferList(Buffer.from('abcd'))) === bl)
- t.ok(bl.append([ new BufferList(Buffer.from('abcd')) ]) === bl)
- t.end()
-})
-
-tape('append chainable (test results)', function (t) {
- var bl = new BufferList('abc')
- .append([ new BufferList('def') ])
- .append(new BufferList([ Buffer.from('ghi'), new BufferList('jkl') ]))
- .append([ Buffer.from('mnop'), new BufferList([ Buffer.from('qrstu'), Buffer.from('vwxyz') ]) ])
-
- t.equal(bl.length, 26)
- t.equal(bl.slice().toString('ascii'), 'abcdefghijklmnopqrstuvwxyz')
- t.end()
-})
-
-tape('consuming from multiple buffers', function (t) {
- var bl = new BufferList()
-
- bl.append(Buffer.from('abcd'))
- bl.append(Buffer.from('efg'))
- bl.append(Buffer.from('hi'))
- bl.append(Buffer.from('j'))
-
- t.equal(bl.length, 10)
-
- t.equal(bl.slice(0, 10).toString('ascii'), 'abcdefghij')
-
- bl.consume(3)
- t.equal(bl.length, 7)
- t.equal(bl.slice(0, 7).toString('ascii'), 'defghij')
-
- bl.consume(2)
- t.equal(bl.length, 5)
- t.equal(bl.slice(0, 5).toString('ascii'), 'fghij')
-
- bl.consume(1)
- t.equal(bl.length, 4)
- t.equal(bl.slice(0, 4).toString('ascii'), 'ghij')
-
- bl.consume(1)
- t.equal(bl.length, 3)
- t.equal(bl.slice(0, 3).toString('ascii'), 'hij')
-
- bl.consume(2)
- t.equal(bl.length, 1)
- t.equal(bl.slice(0, 1).toString('ascii'), 'j')
-
- t.end()
-})
-
-tape('complete consumption', function (t) {
- var bl = new BufferList()
-
- bl.append(Buffer.from('a'))
- bl.append(Buffer.from('b'))
-
- bl.consume(2)
-
- t.equal(bl.length, 0)
- t.equal(bl._bufs.length, 0)
-
- t.end()
-})
-
-tape('test readUInt8 / readInt8', function (t) {
- var buf1 = Buffer.alloc(1)
- , buf2 = Buffer.alloc(3)
- , buf3 = Buffer.alloc(3)
- , bl = new BufferList()
-
- buf2[1] = 0x3
- buf2[2] = 0x4
- buf3[0] = 0x23
- buf3[1] = 0x42
-
- bl.append(buf1)
- bl.append(buf2)
- bl.append(buf3)
-
- t.equal(bl.readUInt8(2), 0x3)
- t.equal(bl.readInt8(2), 0x3)
- t.equal(bl.readUInt8(3), 0x4)
- t.equal(bl.readInt8(3), 0x4)
- t.equal(bl.readUInt8(4), 0x23)
- t.equal(bl.readInt8(4), 0x23)
- t.equal(bl.readUInt8(5), 0x42)
- t.equal(bl.readInt8(5), 0x42)
- t.end()
-})
-
-tape('test readUInt16LE / readUInt16BE / readInt16LE / readInt16BE', function (t) {
- var buf1 = Buffer.alloc(1)
- , buf2 = Buffer.alloc(3)
- , buf3 = Buffer.alloc(3)
- , bl = new BufferList()
-
- buf2[1] = 0x3
- buf2[2] = 0x4
- buf3[0] = 0x23
- buf3[1] = 0x42
-
- bl.append(buf1)
- bl.append(buf2)
- bl.append(buf3)
-
- t.equal(bl.readUInt16BE(2), 0x0304)
- t.equal(bl.readUInt16LE(2), 0x0403)
- t.equal(bl.readInt16BE(2), 0x0304)
- t.equal(bl.readInt16LE(2), 0x0403)
- t.equal(bl.readUInt16BE(3), 0x0423)
- t.equal(bl.readUInt16LE(3), 0x2304)
- t.equal(bl.readInt16BE(3), 0x0423)
- t.equal(bl.readInt16LE(3), 0x2304)
- t.equal(bl.readUInt16BE(4), 0x2342)
- t.equal(bl.readUInt16LE(4), 0x4223)
- t.equal(bl.readInt16BE(4), 0x2342)
- t.equal(bl.readInt16LE(4), 0x4223)
- t.end()
-})
-
-tape('test readUInt32LE / readUInt32BE / readInt32LE / readInt32BE', function (t) {
- var buf1 = Buffer.alloc(1)
- , buf2 = Buffer.alloc(3)
- , buf3 = Buffer.alloc(3)
- , bl = new BufferList()
-
- buf2[1] = 0x3
- buf2[2] = 0x4
- buf3[0] = 0x23
- buf3[1] = 0x42
-
- bl.append(buf1)
- bl.append(buf2)
- bl.append(buf3)
-
- t.equal(bl.readUInt32BE(2), 0x03042342)
- t.equal(bl.readUInt32LE(2), 0x42230403)
- t.equal(bl.readInt32BE(2), 0x03042342)
- t.equal(bl.readInt32LE(2), 0x42230403)
- t.end()
-})
-
-tape('test readUIntLE / readUIntBE / readIntLE / readIntBE', function (t) {
- var buf1 = Buffer.alloc(1)
- , buf2 = Buffer.alloc(3)
- , buf3 = Buffer.alloc(3)
- , bl = new BufferList()
-
- buf2[0] = 0x2
- buf2[1] = 0x3
- buf2[2] = 0x4
- buf3[0] = 0x23
- buf3[1] = 0x42
- buf3[2] = 0x61
-
- bl.append(buf1)
- bl.append(buf2)
- bl.append(buf3)
-
- t.equal(bl.readUIntBE(1, 1), 0x02)
- t.equal(bl.readUIntBE(1, 2), 0x0203)
- t.equal(bl.readUIntBE(1, 3), 0x020304)
- t.equal(bl.readUIntBE(1, 4), 0x02030423)
- t.equal(bl.readUIntBE(1, 5), 0x0203042342)
- t.equal(bl.readUIntBE(1, 6), 0x020304234261)
- t.equal(bl.readUIntLE(1, 1), 0x02)
- t.equal(bl.readUIntLE(1, 2), 0x0302)
- t.equal(bl.readUIntLE(1, 3), 0x040302)
- t.equal(bl.readUIntLE(1, 4), 0x23040302)
- t.equal(bl.readUIntLE(1, 5), 0x4223040302)
- t.equal(bl.readUIntLE(1, 6), 0x614223040302)
- t.equal(bl.readIntBE(1, 1), 0x02)
- t.equal(bl.readIntBE(1, 2), 0x0203)
- t.equal(bl.readIntBE(1, 3), 0x020304)
- t.equal(bl.readIntBE(1, 4), 0x02030423)
- t.equal(bl.readIntBE(1, 5), 0x0203042342)
- t.equal(bl.readIntBE(1, 6), 0x020304234261)
- t.equal(bl.readIntLE(1, 1), 0x02)
- t.equal(bl.readIntLE(1, 2), 0x0302)
- t.equal(bl.readIntLE(1, 3), 0x040302)
- t.equal(bl.readIntLE(1, 4), 0x23040302)
- t.equal(bl.readIntLE(1, 5), 0x4223040302)
- t.equal(bl.readIntLE(1, 6), 0x614223040302)
- t.end()
-})
-
-tape('test readFloatLE / readFloatBE', function (t) {
- var buf1 = Buffer.alloc(1)
- , buf2 = Buffer.alloc(3)
- , buf3 = Buffer.alloc(3)
- , bl = new BufferList()
-
- buf2[1] = 0x00
- buf2[2] = 0x00
- buf3[0] = 0x80
- buf3[1] = 0x3f
-
- bl.append(buf1)
- bl.append(buf2)
- bl.append(buf3)
-
- t.equal(bl.readFloatLE(2), 0x01)
- t.end()
-})
-
-tape('test readDoubleLE / readDoubleBE', function (t) {
- var buf1 = Buffer.alloc(1)
- , buf2 = Buffer.alloc(3)
- , buf3 = Buffer.alloc(10)
- , bl = new BufferList()
-
- buf2[1] = 0x55
- buf2[2] = 0x55
- buf3[0] = 0x55
- buf3[1] = 0x55
- buf3[2] = 0x55
- buf3[3] = 0x55
- buf3[4] = 0xd5
- buf3[5] = 0x3f
-
- bl.append(buf1)
- bl.append(buf2)
- bl.append(buf3)
-
- t.equal(bl.readDoubleLE(2), 0.3333333333333333)
- t.end()
-})
-
-tape('test toString', function (t) {
- var bl = new BufferList()
-
- bl.append(Buffer.from('abcd'))
- bl.append(Buffer.from('efg'))
- bl.append(Buffer.from('hi'))
- bl.append(Buffer.from('j'))
-
- t.equal(bl.toString('ascii', 0, 10), 'abcdefghij')
- t.equal(bl.toString('ascii', 3, 10), 'defghij')
- t.equal(bl.toString('ascii', 3, 6), 'def')
- t.equal(bl.toString('ascii', 3, 8), 'defgh')
- t.equal(bl.toString('ascii', 5, 10), 'fghij')
-
- t.end()
-})
-
-tape('test toString encoding', function (t) {
- var bl = new BufferList()
- , b = Buffer.from('abcdefghij\xff\x00')
-
- bl.append(Buffer.from('abcd'))
- bl.append(Buffer.from('efg'))
- bl.append(Buffer.from('hi'))
- bl.append(Buffer.from('j'))
- bl.append(Buffer.from('\xff\x00'))
-
- encodings.forEach(function (enc) {
- t.equal(bl.toString(enc), b.toString(enc), enc)
- })
-
- t.end()
-})
-
-tape('uninitialized memory', function (t) {
- const secret = crypto.randomBytes(256)
- for (let i = 0; i < 1e6; i++) {
- const clone = Buffer.from(secret)
- const bl = new BufferList()
- bl.append(Buffer.from('a'))
- bl.consume(-1024)
- const buf = bl.slice(1)
- if (buf.indexOf(clone) !== -1) {
- t.fail(`Match (at ${i})`)
- break
- }
- }
- t.end()
-})
-
-!process.browser && tape('test stream', function (t) {
- var random = crypto.randomBytes(65534)
- , rndhash = hash(random, 'md5')
- , md5sum = crypto.createHash('md5')
- , bl = new BufferList(function (err, buf) {
- t.ok(Buffer.isBuffer(buf))
- t.ok(err === null)
- t.equal(rndhash, hash(bl.slice(), 'md5'))
- t.equal(rndhash, hash(buf, 'md5'))
-
- bl.pipe(fs.createWriteStream('/tmp/bl_test_rnd_out.dat'))
- .on('close', function () {
- var s = fs.createReadStream('/tmp/bl_test_rnd_out.dat')
- s.on('data', md5sum.update.bind(md5sum))
- s.on('end', function() {
- t.equal(rndhash, md5sum.digest('hex'), 'woohoo! correct hash!')
- t.end()
- })
- })
-
- })
-
- fs.writeFileSync('/tmp/bl_test_rnd.dat', random)
- fs.createReadStream('/tmp/bl_test_rnd.dat').pipe(bl)
-})
-
-tape('instantiation with Buffer', function (t) {
- var buf = crypto.randomBytes(1024)
- , buf2 = crypto.randomBytes(1024)
- , b = BufferList(buf)
-
- t.equal(buf.toString('hex'), b.slice().toString('hex'), 'same buffer')
- b = BufferList([ buf, buf2 ])
- t.equal(b.slice().toString('hex'), Buffer.concat([ buf, buf2 ]).toString('hex'), 'same buffer')
- t.end()
-})
-
-tape('test String appendage', function (t) {
- var bl = new BufferList()
- , b = Buffer.from('abcdefghij\xff\x00')
-
- bl.append('abcd')
- bl.append('efg')
- bl.append('hi')
- bl.append('j')
- bl.append('\xff\x00')
-
- encodings.forEach(function (enc) {
- t.equal(bl.toString(enc), b.toString(enc))
- })
-
- t.end()
-})
-
-tape('test Number appendage', function (t) {
- var bl = new BufferList()
- , b = Buffer.from('1234567890')
-
- bl.append(1234)
- bl.append(567)
- bl.append(89)
- bl.append(0)
-
- encodings.forEach(function (enc) {
- t.equal(bl.toString(enc), b.toString(enc))
- })
-
- t.end()
-})
-
-tape('write nothing, should get empty buffer', function (t) {
- t.plan(3)
- BufferList(function (err, data) {
- t.notOk(err, 'no error')
- t.ok(Buffer.isBuffer(data), 'got a buffer')
- t.equal(0, data.length, 'got a zero-length buffer')
- t.end()
- }).end()
-})
-
-tape('unicode string', function (t) {
- t.plan(2)
- var inp1 = '\u2600'
- , inp2 = '\u2603'
- , exp = inp1 + ' and ' + inp2
- , bl = BufferList()
- bl.write(inp1)
- bl.write(' and ')
- bl.write(inp2)
- t.equal(exp, bl.toString())
- t.equal(Buffer.from(exp).toString('hex'), bl.toString('hex'))
-})
-
-tape('should emit finish', function (t) {
- var source = BufferList()
- , dest = BufferList()
-
- source.write('hello')
- source.pipe(dest)
-
- dest.on('finish', function () {
- t.equal(dest.toString('utf8'), 'hello')
- t.end()
- })
-})
-
-tape('basic copy', function (t) {
- var buf = crypto.randomBytes(1024)
- , buf2 = Buffer.alloc(1024)
- , b = BufferList(buf)
-
- b.copy(buf2)
- t.equal(b.slice().toString('hex'), buf2.toString('hex'), 'same buffer')
- t.end()
-})
-
-tape('copy after many appends', function (t) {
- var buf = crypto.randomBytes(512)
- , buf2 = Buffer.alloc(1024)
- , b = BufferList(buf)
-
- b.append(buf)
- b.copy(buf2)
- t.equal(b.slice().toString('hex'), buf2.toString('hex'), 'same buffer')
- t.end()
-})
-
-tape('copy at a precise position', function (t) {
- var buf = crypto.randomBytes(1004)
- , buf2 = Buffer.alloc(1024)
- , b = BufferList(buf)
-
- b.copy(buf2, 20)
- t.equal(b.slice().toString('hex'), buf2.slice(20).toString('hex'), 'same buffer')
- t.end()
-})
-
-tape('copy starting from a precise location', function (t) {
- var buf = crypto.randomBytes(10)
- , buf2 = Buffer.alloc(5)
- , b = BufferList(buf)
-
- b.copy(buf2, 0, 5)
- t.equal(b.slice(5).toString('hex'), buf2.toString('hex'), 'same buffer')
- t.end()
-})
-
-tape('copy in an interval', function (t) {
- var rnd = crypto.randomBytes(10)
- , b = BufferList(rnd) // put the random bytes there
- , actual = Buffer.alloc(3)
- , expected = Buffer.alloc(3)
-
- rnd.copy(expected, 0, 5, 8)
- b.copy(actual, 0, 5, 8)
-
- t.equal(actual.toString('hex'), expected.toString('hex'), 'same buffer')
- t.end()
-})
-
-tape('copy an interval between two buffers', function (t) {
- var buf = crypto.randomBytes(10)
- , buf2 = Buffer.alloc(10)
- , b = BufferList(buf)
-
- b.append(buf)
- b.copy(buf2, 0, 5, 15)
-
- t.equal(b.slice(5, 15).toString('hex'), buf2.toString('hex'), 'same buffer')
- t.end()
-})
-
-tape('shallow slice across buffer boundaries', function (t) {
- var bl = new BufferList(['First', 'Second', 'Third'])
-
- t.equal(bl.shallowSlice(3, 13).toString(), 'stSecondTh')
- t.end()
-})
-
-tape('shallow slice within single buffer', function (t) {
- t.plan(2)
- var bl = new BufferList(['First', 'Second', 'Third'])
-
- t.equal(bl.shallowSlice(5, 10).toString(), 'Secon')
- t.equal(bl.shallowSlice(7, 10).toString(), 'con')
- t.end()
-})
-
-tape('shallow slice single buffer', function (t) {
- t.plan(3)
- var bl = new BufferList(['First', 'Second', 'Third'])
-
- t.equal(bl.shallowSlice(0, 5).toString(), 'First')
- t.equal(bl.shallowSlice(5, 11).toString(), 'Second')
- t.equal(bl.shallowSlice(11, 16).toString(), 'Third')
-})
-
-tape('shallow slice with negative or omitted indices', function (t) {
- t.plan(4)
- var bl = new BufferList(['First', 'Second', 'Third'])
-
- t.equal(bl.shallowSlice().toString(), 'FirstSecondThird')
- t.equal(bl.shallowSlice(5).toString(), 'SecondThird')
- t.equal(bl.shallowSlice(5, -3).toString(), 'SecondTh')
- t.equal(bl.shallowSlice(-8).toString(), 'ondThird')
-})
-
-tape('shallow slice does not make a copy', function (t) {
- t.plan(1)
- var buffers = [Buffer.from('First'), Buffer.from('Second'), Buffer.from('Third')]
- var bl = (new BufferList(buffers)).shallowSlice(5, -3)
-
- buffers[1].fill('h')
- buffers[2].fill('h')
-
- t.equal(bl.toString(), 'hhhhhhhh')
-})
-
-tape('shallow slice with 0 length', function (t) {
- t.plan(1)
- var buffers = [Buffer.from('First'), Buffer.from('Second'), Buffer.from('Third')]
- var bl = (new BufferList(buffers)).shallowSlice(0, 0)
- t.equal(bl.length, 0)
-})
-
-tape('shallow slice with 0 length from middle', function (t) {
- t.plan(1)
- var buffers = [Buffer.from('First'), Buffer.from('Second'), Buffer.from('Third')]
- var bl = (new BufferList(buffers)).shallowSlice(10, 10)
- t.equal(bl.length, 0)
-})
-
-tape('duplicate', function (t) {
- t.plan(2)
-
- var bl = new BufferList('abcdefghij\xff\x00')
- , dup = bl.duplicate()
-
- t.equal(bl.prototype, dup.prototype)
- t.equal(bl.toString('hex'), dup.toString('hex'))
-})
-
-tape('destroy no pipe', function (t) {
- t.plan(2)
-
- var bl = new BufferList('alsdkfja;lsdkfja;lsdk')
- bl.destroy()
-
- t.equal(bl._bufs.length, 0)
- t.equal(bl.length, 0)
-})
-
-!process.browser && tape('destroy with pipe before read end', function (t) {
- t.plan(2)
-
- var bl = new BufferList()
- fs.createReadStream(__dirname + '/test.js')
- .pipe(bl)
-
- bl.destroy()
-
- t.equal(bl._bufs.length, 0)
- t.equal(bl.length, 0)
-
-})
-
-!process.browser && tape('destroy with pipe before read end with race', function (t) {
- t.plan(2)
-
- var bl = new BufferList()
- fs.createReadStream(__dirname + '/test.js')
- .pipe(bl)
-
- setTimeout(function () {
- bl.destroy()
- setTimeout(function () {
- t.equal(bl._bufs.length, 0)
- t.equal(bl.length, 0)
- }, 500)
- }, 500)
-})
-
-!process.browser && tape('destroy with pipe after read end', function (t) {
- t.plan(2)
-
- var bl = new BufferList()
- fs.createReadStream(__dirname + '/test.js')
- .on('end', onEnd)
- .pipe(bl)
-
- function onEnd () {
- bl.destroy()
-
- t.equal(bl._bufs.length, 0)
- t.equal(bl.length, 0)
- }
-})
-
-!process.browser && tape('destroy with pipe while writing to a destination', function (t) {
- t.plan(4)
-
- var bl = new BufferList()
- , ds = new BufferList()
-
- fs.createReadStream(__dirname + '/test.js')
- .on('end', onEnd)
- .pipe(bl)
-
- function onEnd () {
- bl.pipe(ds)
-
- setTimeout(function () {
- bl.destroy()
-
- t.equals(bl._bufs.length, 0)
- t.equals(bl.length, 0)
-
- ds.destroy()
-
- t.equals(bl._bufs.length, 0)
- t.equals(bl.length, 0)
-
- }, 100)
- }
-})
-
-!process.browser && tape('handle error', function (t) {
- t.plan(2)
- fs.createReadStream('/does/not/exist').pipe(BufferList(function (err, data) {
- t.ok(err instanceof Error, 'has error')
- t.notOk(data, 'no data')
- }))
-})
diff --git a/node_modules/bson/HISTORY.md b/node_modules/bson/HISTORY.md
index 815edf7..1ab0477 100644
--- a/node_modules/bson/HISTORY.md
+++ b/node_modules/bson/HISTORY.md
@@ -2,94 +2,315 @@
All notable changes to this project will be documented in this file. See [standard-version](https://github.com/conventional-changelog/standard-version) for commit guidelines.
-### [1.1.5](https://github.com/mongodb/js-bson/compare/v1.1.4...v1.1.5) (2020-08-10)
+### [4.4.1](https://github.com/mongodb/js-bson/compare/v4.4.0...v4.4.1) (2021-07-06)
### Bug Fixes
-* **object-id:** harden the duck-typing ([b526145](https://github.com/mongodb/js-bson/commit/b5261450c3bc4abb2e2fb19b5b1a7aba27982d44))
+* **NODE-3247:** DBRef special handling ([#443](https://github.com/mongodb/js-bson/issues/443)) ([f5d984d](https://github.com/mongodb/js-bson/commit/f5d984d88b2e20310ec5cc3a39b91b0fd1e0b3c9))
+* **NODE-3282:** BSONRegExp options not alphabetized ([#441](https://github.com/mongodb/js-bson/issues/441)) ([18c3512](https://github.com/mongodb/js-bson/commit/18c3512befe54908e4b816056dbde0d1b998d81b))
+* **NODE-3376:** use standard JS methods for copying Buffers ([#444](https://github.com/mongodb/js-bson/issues/444)) ([804050d](https://github.com/mongodb/js-bson/commit/804050d40b03a02116995e63671e05ffa033dc45))
+* **NODE-3390:** serialize non-finite doubles correctly in EJSON ([#445](https://github.com/mongodb/js-bson/issues/445)) ([7eb7998](https://github.com/mongodb/js-bson/commit/7eb79981e16d73a391c567b7f9748943997a424d))
-
-## [1.1.3](https://github.com/mongodb/js-bson/compare/v1.1.2...v1.1.3) (2019-11-09)
+## [4.4.0](https://github.com/mongodb/js-bson/compare/v4.3.0...v4.4.0) (2021-05-18)
-Reverts 1.1.2
-
-## [1.1.2](https://github.com/mongodb/js-bson/compare/v1.1.1...v1.1.2) (2019-11-08)
+### Features
+
+* **NODE-3264:** allow Decimal128(string), Long(string), Long(bigint) ([#437](https://github.com/mongodb/js-bson/issues/437)) ([392c1bc](https://github.com/mongodb/js-bson/commit/392c1bcbe003b185f38d64a8a24bc21a6661cb26))
+* make circular input errors for EJSON expressive ([#433](https://github.com/mongodb/js-bson/issues/433)) ([7b351cc](https://github.com/mongodb/js-bson/commit/7b351cc217786e5ee992f0fb64588f9c3fddd828))
+
+
+### Bug Fixes
+
+* make Long inspect result evaluable ([3a2eff1](https://github.com/mongodb/js-bson/commit/3a2eff127175c7f94c9ccc940074537b7ad972f1))
+* **NODE-3153:** correctly deserialize `__proto__` properties ([#431](https://github.com/mongodb/js-bson/issues/431)) ([f34cabc](https://github.com/mongodb/js-bson/commit/f34cabc31e66bc809d8e3cc6b0d203739f40aa41))
+* accept Uint8Array where Buffer is accepted ([#432](https://github.com/mongodb/js-bson/issues/432)) ([4613763](https://github.com/mongodb/js-bson/commit/46137636ac8e59010ba3bfdd317d5d13d9d3066d))
+* clean up instanceof usage ([9b6d52a](https://github.com/mongodb/js-bson/commit/9b6d52a84a20641b22732355e56c3bae3fe857f1))
+* improve ArrayBuffer brand check in ensureBuffer ([#429](https://github.com/mongodb/js-bson/issues/429)) ([99722f6](https://github.com/mongodb/js-bson/commit/99722f66d9f5eeb0ab57e74bab26049a425fa3e8))
+
+## [4.3.0](https://github.com/mongodb/js-bson/compare/v4.2.3...v4.3.0) (2021-04-06)
+
+
+### Features
+
+* UUID convenience class ([#425](https://github.com/mongodb/js-bson/issues/425)) ([76e1826](https://github.com/mongodb/js-bson/commit/76e1826eed852d4cca9fafabbcf826af1367c9af))
+
+### [4.2.3](https://github.com/mongodb/js-bson/compare/v4.2.2...v4.2.3) (2021-03-02)
+
+
+### Bug Fixes
+
+* allow library to be loaded in web workeds ([#423](https://github.com/mongodb/js-bson/issues/423)) ([023f57e](https://github.com/mongodb/js-bson/commit/5ae057d3c6dd87e1407dcdc7b8d9da668023f57e))
+
+* make inspection result of BSON types evaluable ([#416](https://github.com/mongodb/js-bson/issues/416)) ([616665f](https://github.com/mongodb/js-bson/commit/616665f5e6f7dd06a88de450aaccaa203fa6c652))
+* permit BSON types to be created without new ([#424](https://github.com/mongodb/js-bson/issues/424)) ([d2bc284](https://github.com/mongodb/js-bson/commit/d2bc284943649ac27116701a4ed91ff731a4bdf7))
+
+### [4.2.2](https://github.com/mongodb/js-bson/compare/v4.2.1...v4.2.2) (2020-12-01)
+
+
+### Bug Fixes
+
+* remove tslib usage and fix Long method alias ([#415](https://github.com/mongodb/js-bson/issues/415)) ([2d9a8e6](https://github.com/mongodb/js-bson/commit/2d9a8e678417ec43a0b82377743ab9c30a3c3b6b))
+
+### [4.2.1](https://github.com/mongodb/js-bson/compare/v4.2.0...v4.2.1) (2020-12-01)
+
+
+### Bug Fixes
+
+* backwards compatibility with older BSON package versions ([#411](https://github.com/mongodb/js-bson/issues/411)) ([5167be2](https://github.com/mongodb/js-bson/commit/5167be2752369d5832057f7b69da0e240ed4c204))
+* Downlevel type definitions ([#410](https://github.com/mongodb/js-bson/issues/410)) ([203402f](https://github.com/mongodb/js-bson/commit/203402f5cd9dd496a4103dc1008bad382d3c69c4))
+* make inspect method for ObjectId work ([#412](https://github.com/mongodb/js-bson/issues/412)) ([a585a0c](https://github.com/mongodb/js-bson/commit/a585a0cf8cd1212617fb8f37581194e6c31e33fa))
+* remove stringify overloads ([2df6b42](https://github.com/mongodb/js-bson/commit/2df6b42de4cbf81a307d2db144d15470d543976e))
+
+## [4.2.0](https://github.com/mongodb/js-bson/compare/v4.1.0...v4.2.0) (2020-10-13)
+
+
+### Features
+
+* add extended json parsing for $uuid ([b1b2a0e](https://github.com/mongodb/js-bson/commit/b1b2a0ee5f497c971aa28961cf80bde522fc1779))
+* convert to TypeScript ([#393](https://github.com/mongodb/js-bson/issues/393)) ([9aad874](https://github.com/mongodb/js-bson/commit/9aad8746bbb2159012193a53a206509130a95fb0))
+* Improve TS Typings ([#389](https://github.com/mongodb/js-bson/issues/389)) ([ae9ae2d](https://github.com/mongodb/js-bson/commit/ae9ae2df0d5d0a88adf27523d7fca7f3ad59a57a))
+
+
+### Bug Fixes
+
+* adds interfaces for EJSON objects ([7f5f1a3](https://github.com/mongodb/js-bson/commit/7f5f1a38d99d1d50b8bf261cc72916f5bce506ae))
+* Correct API Extractor config to omit definition file from dist ([#407](https://github.com/mongodb/js-bson/issues/407)) ([ace8647](https://github.com/mongodb/js-bson/commit/ace8647646e20df61e77d0ce8ed7ea84a3ff7738))
+* coverage ([992e2e0](https://github.com/mongodb/js-bson/commit/992e2e040806701d1c69e09d07186a6e1deacc0e))
+* deprecate cacheFunctionsCrc32 ([ea83bf5](https://github.com/mongodb/js-bson/commit/ea83bf5200f4a936692f710063941ba802386da4))
+* Rework rollup config to output named and default exports ([#404](https://github.com/mongodb/js-bson/issues/404)) ([a48676b](https://github.com/mongodb/js-bson/commit/a48676b0d442e06a71a413500194d35a7bea7587))
+* Throw on BigInt type values ([#397](https://github.com/mongodb/js-bson/issues/397)) ([2dd54e5](https://github.com/mongodb/js-bson/commit/2dd54e5275fc72dd8cd579a1636d2a73b7b0e790))
+* type issues with SerializeOptions and Long methods accepting Timestamp ([c18ba71](https://github.com/mongodb/js-bson/commit/c18ba71229129c8ea34e40265a9503c10e29a9e0))
+
+
+# [4.1.0](https://github.com/mongodb/js-bson/compare/v4.0.4...v4.1.0) (2020-08-10)
+
+
+### Bug Fixes
+
+* spelling in deserializer errors ([4c6f2e4](https://github.com/mongodb/js-bson/commit/4c6f2e4))
+* **object-id:** harden the duck-typing ([4b800ae](https://github.com/mongodb/js-bson/commit/4b800ae))
+* parse value of Int32 in constructor ([5cda40f](https://github.com/mongodb/js-bson/commit/5cda40f))
+* Reduce floating point precision required of extended json implementations ([#369](https://github.com/mongodb/js-bson/issues/369)) ([5e35d1a](https://github.com/mongodb/js-bson/commit/5e35d1a))
+
+
+### Features
+
+* add support for primitives to EJSON.stringify ([329857d](https://github.com/mongodb/js-bson/commit/329857d))
+
+
+
+
+## [4.0.4](https://github.com/mongodb/js-bson/compare/v4.0.3...v4.0.4) (2020-03-26)
+
+
+### Bug Fixes
+
+* improve EJSON generation for previously skipped edge cases ([30f5a8f](https://github.com/mongodb/js-bson/commit/30f5a8f))
+* only upgrade `symbol` to `string` if `promoteValues` is true ([067a7ba](https://github.com/mongodb/js-bson/commit/067a7ba))
+
+
+
+
+## [4.0.3](https://github.com/mongodb/js-bson/compare/v4.0.2...v4.0.3) (2020-01-09)
+
+
+### Bug Fixes
+
+* support Number object in Int32 and Double constructors ([fe3f0dc](https://github.com/mongodb/js-bson/commit/fe3f0dc))
+* **Timestamp:** make sure timestamp is always unsigned ([36b2d43](https://github.com/mongodb/js-bson/commit/36b2d43))
+
+
+
+
+## [4.0.2](https://github.com/mongodb/js-bson/compare/v4.0.0...v4.0.2) (2019-03-08)
### Bug Fixes
-* **_bsontype:** only check bsontype if it is a prototype member. ([dd8a349](https://github.com/mongodb/js-bson/commit/dd8a349))
+* **buffer:** don't use deprecated Buffer constructors ([7bb9c57](https://github.com/mongodb/js-bson/commit/7bb9c57))
+* **Buffer:** import buffer for binary, decimal128, and fnv1a ([6be7b8d](https://github.com/mongodb/js-bson/commit/6be7b8d))
+* **ejson:** enable serialization of legacy `ObjectID` ([ba98ccb](https://github.com/mongodb/js-bson/commit/ba98ccb)), closes [#303](https://github.com/mongodb/js-bson/issues/303)
+* **ejson:** support array for replacer parameter in `EJSON.stringify` ([9f43809](https://github.com/mongodb/js-bson/commit/9f43809)), closes [#303](https://github.com/mongodb/js-bson/issues/303) [#302](https://github.com/mongodb/js-bson/issues/302) [#303](https://github.com/mongodb/js-bson/issues/303)
+* **ejson-serialize:** prevent double serialization for nested documents ([ab790c9](https://github.com/mongodb/js-bson/commit/ab790c9)), closes [#303](https://github.com/mongodb/js-bson/issues/303)
+* **object-id:** correct serialization of old ObjectID types ([8d57a8c](https://github.com/mongodb/js-bson/commit/8d57a8c))
+* **timestamp:** getTimestamp support times beyond 2038 ([a0820d5](https://github.com/mongodb/js-bson/commit/a0820d5))
+* 4.x-1.x interop (incl. ObjectID _bsontype) ([f4b16d9](https://github.com/mongodb/js-bson/commit/f4b16d9))
-
-## [1.1.1](https://github.com/mongodb/js-bson/compare/v1.1.0...v1.1.1) (2019-03-08)
+
+## [4.0.1](https://github.com/mongodb/js-bson/compare/v4.0.0...v4.0.1) (2018-12-06)
### Bug Fixes
-* **object-id:** support 4.x->1.x interop for MinKey and ObjectId ([53419a5](https://github.com/mongodb/js-bson/commit/53419a5))
+* **object-id:** correct serialization of old ObjectID types ([8d57a8c](https://github.com/mongodb/js-bson/commit/8d57a8c))
+
+
+
+
+# [4.0.0](https://github.com/mongodb/js-bson/compare/v3.0.2...v4.0.0) (2018-11-13)
+
+### Migration Guide
+
+Please see the [migration guide](https://github.com/mongodb/js-bson/blob/master/docs/upgrade-to-v4.md) for detailed discussion of breaking changes in this release.
+
+### Bug Fixes
+
+* **buffer:** replace deprecated Buffer constructor ([5acdebf](https://github.com/mongodb/js-bson/commit/5acdebf))
+* **dbPointer:** fix utf8 bug for dbPointer ([018c769](https://github.com/mongodb/js-bson/commit/018c769))
+* **deserialize:** fix deserialization of 0xFFFD ([c682ae3](https://github.com/mongodb/js-bson/commit/c682ae3)), closes [#277](https://github.com/mongodb/js-bson/issues/277)
+* **ext-json:** deserialize doubles as `Number` in relaxed mode ([a767fa1](https://github.com/mongodb/js-bson/commit/a767fa1))
+* **ObjectId:** will now throw if an invalid character is passed ([6f30b4e](https://github.com/mongodb/js-bson/commit/6f30b4e))
+* **ObjectID:** ObjectId.isValid should check buffer length ([06af813](https://github.com/mongodb/js-bson/commit/06af813))
+* **package:** `browser` section needs to point to correct index ([08337e3](https://github.com/mongodb/js-bson/commit/08337e3))
+* **random-bytes:** fallback to insecure path if require is null ([963b12b](https://github.com/mongodb/js-bson/commit/963b12b))
+* **random-bytes:** wrap crypto require in try/catch for fallback ([47fd5f7](https://github.com/mongodb/js-bson/commit/47fd5f7))
+* **serializer:** do not use checkKeys for $clusterTime ([cbb4724](https://github.com/mongodb/js-bson/commit/cbb4724))
+* **serializer:** map insert expects only string keys ([aba3a18](https://github.com/mongodb/js-bson/commit/aba3a18))
+
+
+### Code Refactoring
+
+* **symbol:** rename Symbol to BSONSymbol ([5d5b3d2](https://github.com/mongodb/js-bson/commit/5d5b3d2))
### Features
-* replace new Buffer with modern versions ([24aefba](https://github.com/mongodb/js-bson/commit/24aefba))
+* **BSON:** simplify and flatten module exports ([f8920c6](https://github.com/mongodb/js-bson/commit/f8920c6))
+* **bsontype:** move all `_bsontypes` to non-enumerable properties ([16f5bf6](https://github.com/mongodb/js-bson/commit/16f5bf6))
+* **ext-json:** add extended JSON codecs directly to BSON types ([10e5f00](https://github.com/mongodb/js-bson/commit/10e5f00))
+* **ext-json:** add extended JSON support to the bson library ([d6b71ab](https://github.com/mongodb/js-bson/commit/d6b71ab))
+* **ext-json:** export EJSON at top level of module ([c356a5a](https://github.com/mongodb/js-bson/commit/c356a5a))
+* **karma:** test bson in the browser ([cd593ca](https://github.com/mongodb/js-bson/commit/cd593ca))
+* **long:** replace long implementatin with long.js ([545900d](https://github.com/mongodb/js-bson/commit/545900d))
+
+### BREAKING CHANGES
+* **ObjectId:** Where code was previously silently erroring, users may now
+experience TypeErrors
+* **symbol:** This was conflicting with the ES6 Symbol type
-
-# [1.1.0](https://github.com/mongodb/js-bson/compare/v1.0.9...v1.1.0) (2018-08-13)
+
+
+
+## [3.0.2](https://github.com/mongodb/js-bson/compare/v3.0.1...v3.0.2) (2018-07-13)
### Bug Fixes
-* **serializer:** do not use checkKeys for $clusterTime ([573e141](https://github.com/mongodb/js-bson/commit/573e141))
+* **revert:** Reverting v3.0.1 ([efb0720](https://github.com/mongodb/js-bson/commit/efb0720))
+
+
+
+
+# [3.0.0](https://github.com/mongodb/js-bson/compare/v2.0.8...v3.0.0) (2018-06-13)
+
+
+### Features
+* **ObjectID:** use FNV-1a hash for objectId ([4f545b1](https://github.com/mongodb/js-bson/commit/4f545b1))
+* **rollup:** initial commit of rollup-generated bundle ([474b8f7](https://github.com/mongodb/js-bson/commit/474b8f7))
+* **rollup:** switch from webpack to rollup for bundling ([98068fa](https://github.com/mongodb/js-bson/commit/98068fa))
-
-## [1.0.9](https://github.com/mongodb/js-bson/compare/v1.0.8...v1.0.9) (2018-06-07)
+
+
+## [2.0.8](https://github.com/mongodb/js-bson/compare/v2.0.7...v2.0.8) (2018-06-06)
### Bug Fixes
-* **serializer:** remove use of `const` ([5feb12f](https://github.com/mongodb/js-bson/commit/5feb12f))
+* **readme:** clarify documentation about deserialize methods ([e311056](https://github.com/mongodb/js-bson/commit/e311056))
+* **serialization:** normalize function stringification ([21eb0b0](https://github.com/mongodb/js-bson/commit/21eb0b0))
-
-## [1.0.7](https://github.com/mongodb/js-bson/compare/v1.0.6...v1.0.7) (2018-06-06)
+
+## [2.0.7](https://github.com/mongodb/js-bson/compare/v2.0.6...v2.0.7) (2018-05-31)
### Bug Fixes
-* **binary:** add type checking for buffer ([26b05b5](https://github.com/mongodb/js-bson/commit/26b05b5))
-* **bson:** fix custom inspect property ([080323b](https://github.com/mongodb/js-bson/commit/080323b))
-* **readme:** clarify documentation about deserialize methods ([20f764c](https://github.com/mongodb/js-bson/commit/20f764c))
-* **serialization:** normalize function stringification ([1320c10](https://github.com/mongodb/js-bson/commit/1320c10))
+* **binary:** add type checking for buffer ([cbfb25d](https://github.com/mongodb/js-bson/commit/cbfb25d))
+
+
+
+
+## [2.0.6](https://github.com/mongodb/js-bson/compare/v2.0.5...v2.0.6) (2018-04-27)
+
+
+### Bug Fixes
+* **deserializeStream:** allow multiple documents to be deserialized ([6fc5984](https://github.com/mongodb/js-bson/commit/6fc5984)), closes [#244](https://github.com/mongodb/js-bson/issues/244)
-
-## [1.0.6](https://github.com/mongodb/js-bson/compare/v1.0.5...v1.0.6) (2018-03-12)
+
+
+## [2.0.5](https://github.com/mongodb/js-bson/compare/v2.0.4...v2.0.5) (2018-04-06)
+
+
+### Bug Fixes
+
+* **regexp:** properly construct new BSONRegExp when constructor called without new ([#242](https://github.com/mongodb/js-bson/issues/242)) ([93ae799](https://github.com/mongodb/js-bson/commit/93ae799))
+
+
+
+
+## [2.0.4](https://github.com/mongodb/js-bson/compare/v2.0.3...v2.0.4) (2018-03-12)
+
+
+
+
+## [2.0.3](https://github.com/mongodb/js-bson/compare/v2.0.2...v2.0.3) (2018-03-12)
+
+
+### Features
+
+* **serialization:** support arbitrary sizes for the internal serialization buffer ([a6bd45c](https://github.com/mongodb/js-bson/commit/a6bd45c))
+
+
+
+
+## [2.0.2](https://github.com/mongodb/js-bson/compare/v2.0.1...v2.0.2) (2018-03-02)
+
+
+### Bug Fixes
+
+* make sure all functions are named consistently ([6df9022](https://github.com/mongodb/js-bson/commit/6df9022))
+
+
+
+
+## [2.0.1](https://github.com/mongodb/js-bson/compare/v2.0.0...v2.0.1) (2018-02-28)
+
+
+### Bug Fixes
+
+* **serializer:** ensure RegExp options are alphabetically sorted ([d60659d](https://github.com/mongodb/js-bson/commit/d60659d))
### Features
-* **serialization:** support arbitrary sizes for the internal serialization buffer ([abe97bc](https://github.com/mongodb/js-bson/commit/abe97bc))
+* **db-ref:** support passing a namespace into a DBRef ctor ([604831b](https://github.com/mongodb/js-bson/commit/604831b))
-
-## 1.0.5 (2018-02-26)
+
+# 2.0.0 (2018-02-26)
### Bug Fixes
-* **decimal128:** add basic guard against REDOS attacks ([bd61c45](https://github.com/mongodb/js-bson/commit/bd61c45))
+* **browser:** fixing browser property in package.json ([095fba9](https://github.com/mongodb/js-bson/commit/095fba9))
+* **dbref:** only upgrade objects with allowed $keys to DBRefs ([98eb9e2](https://github.com/mongodb/js-bson/commit/98eb9e2))
+* **decimal128:** add basic guard against REDOS attacks ([511ecc4](https://github.com/mongodb/js-bson/commit/511ecc4))
+* **Decimal128:** update toString and fromString methods to correctly handle the case of too many significant digits ([25ed43e](https://github.com/mongodb/js-bson/commit/25ed43e))
* **objectid:** if pid is 1, use random value ([e188ae6](https://github.com/mongodb/js-bson/commit/e188ae6))
+* **serializeWithBufferAndIndex:** write documents to start of intermediate buffer ([b4e4ac5](https://github.com/mongodb/js-bson/commit/b4e4ac5))
diff --git a/node_modules/bson/README.md b/node_modules/bson/README.md
index 0688341..cd7242f 100644
--- a/node_modules/bson/README.md
+++ b/node_modules/bson/README.md
@@ -1,11 +1,28 @@
# BSON parser
-BSON is short for Binary JSON and is the binary-encoded serialization of JSON-like documents. You can learn more about it in [the specification](http://bsonspec.org).
+BSON is short for "Binary JSON," and is the binary-encoded serialization of JSON-like documents. You can learn more about it in [the specification](http://bsonspec.org).
-This browser version of the BSON parser is compiled using [webpack](https://webpack.js.org/) and the current version is pre-compiled in the `browser_build` directory.
+This browser version of the BSON parser is compiled using [rollup](https://rollupjs.org/) and the current version is pre-compiled in the `dist` directory.
This is the default BSON parser, however, there is a C++ Node.js addon version as well that does not support the browser. It can be found at [mongod-js/bson-ext](https://github.com/mongodb-js/bson-ext).
+### Table of Contents
+- [Usage](#usage)
+- [Bugs/Feature Requests](#bugs--feature-requests)
+- [Installation](#installation)
+- [Documentation](#documentation)
+- [FAQ](#faq)
+
+## Bugs / Feature Requests
+
+Think you've found a bug? Want to see a new feature in `bson`? Please open a case in our issue management tool, JIRA:
+
+1. Create an account and login: [jira.mongodb.org](https://jira.mongodb.org)
+2. Navigate to the NODE project: [jira.mongodb.org/browse/NODE](https://jira.mongodb.org/browse/NODE)
+3. Click **Create Issue** - Please provide as much information as possible about the issue and how to reproduce it.
+
+Bug reports in JIRA for all driver projects (i.e. NODE, PYTHON, CSHARP, JAVA) and the Core Server (i.e. SERVER) project are **public**.
+
## Usage
To build a new version perform the following operations:
@@ -15,135 +32,324 @@ npm install
npm run build
```
-A simple example of how to use BSON in the browser:
+### Node (no bundling)
+A simple example of how to use BSON in `Node.js`:
+
+```js
+const BSON = require('bson');
+const Long = BSON.Long;
+
+// Serialize a document
+const doc = { long: Long.fromNumber(100) };
+const data = BSON.serialize(doc);
+console.log('data:', data);
+
+// Deserialize the resulting Buffer
+const doc_2 = BSON.deserialize(data);
+console.log('doc_2:', doc_2);
+```
+
+### Browser (no bundling)
+
+If you are not using a bundler like webpack, you can include `dist/bson.bundle.js` using a script tag. It includes polyfills for built-in node types like `Buffer`.
```html
-
+
```
-A simple example of how to use BSON in `Node.js`:
+### Using webpack
+
+If using webpack, you can use your normal import/require syntax of your project to pull in the `bson` library.
+
+ES6 Example:
```js
-// Get BSON parser class
-var BSON = require('bson')
-// Get the Long type
-var Long = BSON.Long;
-// Create a bson parser instance
-var bson = new BSON();
+import { Long, serialize, deserialize } from 'bson';
-// Serialize document
-var doc = { long: Long.fromNumber(100) }
+// Serialize a document
+const doc = { long: Long.fromNumber(100) };
+const data = serialize(doc);
+console.log('data:', data);
+
+// De serialize it again
+const doc_2 = deserialize(data);
+console.log('doc_2:', doc_2);
+```
+
+ES5 Example:
+
+```js
+const BSON = require('bson');
+const Long = BSON.Long;
// Serialize a document
-var data = bson.serialize(doc)
-console.log('data:', data)
+const doc = { long: Long.fromNumber(100) };
+const data = BSON.serialize(doc);
+console.log('data:', data);
// Deserialize the resulting Buffer
-var doc_2 = bson.deserialize(data)
-console.log('doc_2:', doc_2)
+const doc_2 = BSON.deserialize(data);
+console.log('doc_2:', doc_2);
```
+Depending on your settings, webpack will under the hood resolve to one of the following:
+
+- `dist/bson.browser.esm.js` If your project is in the browser and using ES6 modules (Default for `webworker` and `web` targets)
+- `dist/bson.browser.umd.js` If your project is in the browser and not using ES6 modules
+- `dist/bson.esm.js` If your project is in Node.js and using ES6 modules (Default for `node` targets)
+- `lib/bson.js` (the normal include path) If your project is in Node.js and not using ES6 modules
+
+For more information, see [this page on webpack's `resolve.mainFields`](https://webpack.js.org/configuration/resolve/#resolvemainfields) and [the `package.json` for this project](./package.json#L52)
+
+### Usage with Angular
+
+Starting with Angular 6, Angular CLI removed the shim for `global` and other node built-in variables (original comment [here](https://github.com/angular/angular-cli/issues/9827#issuecomment-386154063)). If you are using BSON with Angular, you may need to add the following shim to your `polyfills.ts` file:
+
+```js
+// In polyfills.ts
+(window as any).global = window;
+```
+
+- [Original Comment by Angular CLI](https://github.com/angular/angular-cli/issues/9827#issuecomment-386154063)
+- [Original Source for Solution](https://stackoverflow.com/a/50488337/4930088)
+
## Installation
`npm install bson`
-## API
+## Documentation
+
+### Objects
+
+
+- EJSON :
object
+
+
+
+### Functions
+
+
+- setInternalBufferSize(size)
+Sets the size of the internal serialization buffer.
+
+- serialize(object) ⇒
Buffer
+Serialize a Javascript object.
+
+- serializeWithBufferAndIndex(object, buffer) ⇒
Number
+Serialize a Javascript object using a predefined Buffer and index into the buffer, useful when pre-allocating the space for serialization.
+
+- deserialize(buffer) ⇒
Object
+Deserialize data as BSON.
+
+- calculateObjectSize(object) ⇒
Number
+Calculate the bson size for a passed in Javascript object.
+
+- deserializeStream(data, startIndex, numberOfDocuments, documents, docStartIndex, [options]) ⇒
Number
+Deserialize stream data as BSON documents.
+
+
+
+
+
+### EJSON
+
+* [EJSON](#EJSON)
+
+ * [.parse(text, [options])](#EJSON.parse)
+
+ * [.stringify(value, [replacer], [space], [options])](#EJSON.stringify)
+
+ * [.serialize(bson, [options])](#EJSON.serialize)
+
+ * [.deserialize(ejson, [options])](#EJSON.deserialize)
+
+
+
+
+#### *EJSON*.parse(text, [options])
+
+| Param | Type | Default | Description |
+| --- | --- | --- | --- |
+| text | string
| | |
+| [options] | object
| | Optional settings |
+| [options.relaxed] | boolean
| true
| Attempt to return native JS types where possible, rather than BSON types (if true) |
+
+Parse an Extended JSON string, constructing the JavaScript value or object described by that
+string.
+
+**Example**
+```js
+const { EJSON } = require('bson');
+const text = '{ "int32": { "$numberInt": "10" } }';
+
+// prints { int32: { [String: '10'] _bsontype: 'Int32', value: '10' } }
+console.log(EJSON.parse(text, { relaxed: false }));
+
+// prints { int32: 10 }
+console.log(EJSON.parse(text));
+```
+
+
+#### *EJSON*.stringify(value, [replacer], [space], [options])
+
+| Param | Type | Default | Description |
+| --- | --- | --- | --- |
+| value | object
| | The value to convert to extended JSON |
+| [replacer] | function
\| array
| | A function that alters the behavior of the stringification process, or an array of String and Number objects that serve as a whitelist for selecting/filtering the properties of the value object to be included in the JSON string. If this value is null or not provided, all properties of the object are included in the resulting JSON string |
+| [space] | string
\| number
| | A String or Number object that's used to insert white space into the output JSON string for readability purposes. |
+| [options] | object
| | Optional settings |
+| [options.relaxed] | boolean
| true
| Enabled Extended JSON's `relaxed` mode |
+| [options.legacy] | boolean
| true
| Output in Extended JSON v1 |
+
+Converts a BSON document to an Extended JSON string, optionally replacing values if a replacer
+function is specified or optionally including only the specified properties if a replacer array
+is specified.
+
+**Example**
+```js
+const { EJSON } = require('bson');
+const Int32 = require('mongodb').Int32;
+const doc = { int32: new Int32(10) };
+
+// prints '{"int32":{"$numberInt":"10"}}'
+console.log(EJSON.stringify(doc, { relaxed: false }));
+
+// prints '{"int32":10}'
+console.log(EJSON.stringify(doc));
+```
+
+
+#### *EJSON*.serialize(bson, [options])
+
+| Param | Type | Description |
+| --- | --- | --- |
+| bson | object
| The object to serialize |
+| [options] | object
| Optional settings passed to the `stringify` function |
+
+Serializes an object to an Extended JSON string, and reparse it as a JavaScript object.
+
+
+
+#### *EJSON*.deserialize(ejson, [options])
+
+| Param | Type | Description |
+| --- | --- | --- |
+| ejson | object
| The Extended JSON object to deserialize |
+| [options] | object
| Optional settings passed to the parse method |
+
+Deserializes an Extended JSON object into a plain JavaScript object with native/BSON types
+
+
+
+### setInternalBufferSize(size)
+
+| Param | Type | Description |
+| --- | --- | --- |
+| size | number
| The desired size for the internal serialization buffer |
+
+Sets the size of the internal serialization buffer.
+
+
+
+### serialize(object)
-### BSON types
+| Param | Type | Default | Description |
+| --- | --- | --- | --- |
+| object | Object
| | the Javascript object to serialize. |
+| [options.checkKeys] | Boolean
| | the serializer will check if keys are valid. |
+| [options.serializeFunctions] | Boolean
| false
| serialize the javascript functions **(default:false)**. |
+| [options.ignoreUndefined] | Boolean
| true
| ignore undefined fields **(default:true)**. |
-For all BSON types documentation, please refer to the following sources:
- * [MongoDB BSON Type Reference](https://docs.mongodb.com/manual/reference/bson-types/)
- * [BSON Spec](https://bsonspec.org/)
+Serialize a Javascript object.
-### BSON serialization and deserialiation
+**Returns**: Buffer
- returns the Buffer object containing the serialized object.
+
-**`new BSON()`** - Creates a new BSON serializer/deserializer you can use to serialize and deserialize BSON.
+### serializeWithBufferAndIndex(object, buffer)
-#### BSON.serialize
+| Param | Type | Default | Description |
+| --- | --- | --- | --- |
+| object | Object
| | the Javascript object to serialize. |
+| buffer | Buffer
| | the Buffer you pre-allocated to store the serialized BSON object. |
+| [options.checkKeys] | Boolean
| | the serializer will check if keys are valid. |
+| [options.serializeFunctions] | Boolean
| false
| serialize the javascript functions **(default:false)**. |
+| [options.ignoreUndefined] | Boolean
| true
| ignore undefined fields **(default:true)**. |
+| [options.index] | Number
| | the index in the buffer where we wish to start serializing into. |
-The BSON `serialize` method takes a JavaScript object and an optional options object and returns a Node.js Buffer.
+Serialize a Javascript object using a predefined Buffer and index into the buffer, useful when pre-allocating the space for serialization.
- * `BSON.serialize(object, options)`
- * @param {Object} object the JavaScript object to serialize.
- * @param {Boolean} [options.checkKeys=false] the serializer will check if keys are valid.
- * @param {Boolean} [options.serializeFunctions=false] serialize the JavaScript functions.
- * @param {Boolean} [options.ignoreUndefined=true]
- * @return {Buffer} returns a Buffer instance.
+**Returns**: Number
- returns the index pointing to the last written byte in the buffer.
+
-#### BSON.serializeWithBufferAndIndex
+### deserialize(buffer)
-The BSON `serializeWithBufferAndIndex` method takes an object, a target buffer instance and an optional options object and returns the end serialization index in the final buffer.
+| Param | Type | Default | Description |
+| --- | --- | --- | --- |
+| buffer | Buffer
| | the buffer containing the serialized set of BSON documents. |
+| [options.evalFunctions] | Object
| false
| evaluate functions in the BSON document scoped to the object deserialized. |
+| [options.cacheFunctions] | Object
| false
| cache evaluated functions for reuse. |
+| [options.promoteLongs] | Object
| true
| when deserializing a Long will fit it into a Number if it's smaller than 53 bits |
+| [options.promoteBuffers] | Object
| false
| when deserializing a Binary will return it as a node.js Buffer instance. |
+| [options.promoteValues] | Object
| false
| when deserializing will promote BSON values to their Node.js closest equivalent types. |
+| [options.fieldsAsRaw] | Object
|
| allow to specify if there what fields we wish to return as unserialized raw buffer. |
+| [options.bsonRegExp] | Object
| false
| return BSON regular expressions as BSONRegExp instances. |
+| [options.allowObjectSmallerThanBufferSize] | boolean
| false
| allows the buffer to be larger than the parsed BSON object |
- * `BSON.serializeWithBufferAndIndex(object, buffer, options)`
- * @param {Object} object the JavaScript object to serialize.
- * @param {Buffer} buffer the Buffer you pre-allocated to store the serialized BSON object.
- * @param {Boolean} [options.checkKeys=false] the serializer will check if keys are valid.
- * @param {Boolean} [options.serializeFunctions=false] serialize the JavaScript functions.
- * @param {Boolean} [options.ignoreUndefined=true] ignore undefined fields.
- * @param {Number} [options.index=0] the index in the buffer where we wish to start serializing into.
- * @return {Number} returns the index pointing to the last written byte in the buffer.
+Deserialize data as BSON.
-#### BSON.calculateObjectSize
+**Returns**: Object
- returns the deserialized Javascript Object.
+
-The BSON `calculateObjectSize` method takes a JavaScript object and an optional options object and returns the size of the BSON object.
+### calculateObjectSize(object)
- * `BSON.calculateObjectSize(object, options)`
- * @param {Object} object the JavaScript object to serialize.
- * @param {Boolean} [options.serializeFunctions=false] serialize the JavaScript functions.
- * @param {Boolean} [options.ignoreUndefined=true]
- * @return {Buffer} returns a Buffer instance.
+| Param | Type | Default | Description |
+| --- | --- | --- | --- |
+| object | Object
| | the Javascript object to calculate the BSON byte size for. |
+| [options.serializeFunctions] | Boolean
| false
| serialize the javascript functions **(default:false)**. |
+| [options.ignoreUndefined] | Boolean
| true
| ignore undefined fields **(default:true)**. |
-#### BSON.deserialize
+Calculate the bson size for a passed in Javascript object.
-The BSON `deserialize` method takes a Node.js Buffer and an optional options object and returns a deserialized JavaScript object.
+**Returns**: Number
- returns the number of bytes the BSON object will take up.
+
- * `BSON.deserialize(buffer, options)`
- * @param {Object} [options.evalFunctions=false] evaluate functions in the BSON document scoped to the object deserialized.
- * @param {Object} [options.cacheFunctions=false] cache evaluated functions for reuse.
- * @param {Object} [options.cacheFunctionsCrc32=false] use a crc32 code for caching, otherwise use the string of the function.
- * @param {Object} [options.promoteLongs=true] when deserializing a Long will fit it into a Number if it's smaller than 53 bits
- * @param {Object} [options.promoteBuffers=false] when deserializing a Binary will return it as a Node.js Buffer instance.
- * @param {Object} [options.promoteValues=false] when deserializing will promote BSON values to their Node.js closest equivalent types.
- * @param {Object} [options.fieldsAsRaw=null] allow to specify if there what fields we wish to return as unserialized raw buffer.
- * @param {Object} [options.bsonRegExp=false] return BSON regular expressions as BSONRegExp instances.
- * @return {Object} returns the deserialized Javascript Object.
+### deserializeStream(data, startIndex, numberOfDocuments, documents, docStartIndex, [options])
-#### BSON.deserializeStream
+| Param | Type | Default | Description |
+| --- | --- | --- | --- |
+| data | Buffer
| | the buffer containing the serialized set of BSON documents. |
+| startIndex | Number
| | the start index in the data Buffer where the deserialization is to start. |
+| numberOfDocuments | Number
| | number of documents to deserialize. |
+| documents | Array
| | an array where to store the deserialized documents. |
+| docStartIndex | Number
| | the index in the documents array from where to start inserting documents. |
+| [options] | Object
| | additional options used for the deserialization. |
+| [options.evalFunctions] | Object
| false
| evaluate functions in the BSON document scoped to the object deserialized. |
+| [options.cacheFunctions] | Object
| false
| cache evaluated functions for reuse. |
+| [options.promoteLongs] | Object
| true
| when deserializing a Long will fit it into a Number if it's smaller than 53 bits |
+| [options.promoteBuffers] | Object
| false
| when deserializing a Binary will return it as a node.js Buffer instance. |
+| [options.promoteValues] | Object
| false
| when deserializing will promote BSON values to their Node.js closest equivalent types. |
+| [options.fieldsAsRaw] | Object
|
| allow to specify if there what fields we wish to return as unserialized raw buffer. |
+| [options.bsonRegExp] | Object
| false
| return BSON regular expressions as BSONRegExp instances. |
-The BSON `deserializeStream` method takes a Node.js Buffer, `startIndex` and allow more control over deserialization of a Buffer containing concatenated BSON documents.
+Deserialize stream data as BSON documents.
- * `BSON.deserializeStream(buffer, startIndex, numberOfDocuments, documents, docStartIndex, options)`
- * @param {Buffer} buffer the buffer containing the serialized set of BSON documents.
- * @param {Number} startIndex the start index in the data Buffer where the deserialization is to start.
- * @param {Number} numberOfDocuments number of documents to deserialize.
- * @param {Array} documents an array where to store the deserialized documents.
- * @param {Number} docStartIndex the index in the documents array from where to start inserting documents.
- * @param {Object} [options.evalFunctions=false] evaluate functions in the BSON document scoped to the object deserialized.
- * @param {Object} [options.cacheFunctions=false] cache evaluated functions for reuse.
- * @param {Object} [options.cacheFunctionsCrc32=false] use a crc32 code for caching, otherwise use the string of the function.
- * @param {Object} [options.promoteLongs=true] when deserializing a Long will fit it into a Number if it's smaller than 53 bits
- * @param {Object} [options.promoteBuffers=false] when deserializing a Binary will return it as a Node.js Buffer instance.
- * @param {Object} [options.promoteValues=false] when deserializing will promote BSON values to their Node.js closest equivalent types.
- * @param {Object} [options.fieldsAsRaw=null] allow to specify if there what fields we wish to return as unserialized raw buffer.
- * @param {Object} [options.bsonRegExp=false] return BSON regular expressions as BSONRegExp instances.
- * @return {Number} returns the next index in the buffer after deserialization **x** numbers of documents.
+**Returns**: Number
- returns the next index in the buffer after deserialization **x** numbers of documents.
## FAQ
@@ -156,7 +362,7 @@ The `undefined` BSON type has been [deprecated for many years](http://bsonspec.o
This library looks for `toBSON()` functions on every path, and calls the `toBSON()` function to get the value to serialize.
```javascript
-var bson = new BSON();
+const BSON = require('bson');
class CustomSerialize {
toBSON() {
@@ -166,5 +372,5 @@ class CustomSerialize {
const obj = { answer: new CustomSerialize() };
// "{ answer: 42 }"
-console.log(bson.deserialize(bson.serialize(obj)));
+console.log(BSON.deserialize(BSON.serialize(obj)));
```
diff --git a/node_modules/bson/bower.json b/node_modules/bson/bower.json
index be4510b..5f3f3a1 100644
--- a/node_modules/bson/bower.json
+++ b/node_modules/bson/bower.json
@@ -7,7 +7,7 @@
"parser"
],
"author": "Christian Amor Kvalheim ",
- "main": "./browser_build/bson.js",
+ "main": "./dist/bson.js",
"license": "Apache-2.0",
"moduleType": [
"globals",
@@ -22,5 +22,5 @@
"test",
"tools"
],
- "version": "1.1.5"
+ "version": "4.4.1"
}
diff --git a/node_modules/bson/browser_build/bson.js b/node_modules/bson/browser_build/bson.js
deleted file mode 100644
index 9540a86..0000000
--- a/node_modules/bson/browser_build/bson.js
+++ /dev/null
@@ -1,18218 +0,0 @@
-(function webpackUniversalModuleDefinition(root, factory) {
- if(typeof exports === 'object' && typeof module === 'object')
- module.exports = factory();
- else if(typeof define === 'function' && define.amd)
- define([], factory);
- else {
- var a = factory();
- for(var i in a) (typeof exports === 'object' ? exports : root)[i] = a[i];
- }
-})(this, function() {
-return /******/ (function(modules) { // webpackBootstrap
-/******/ // The module cache
-/******/ var installedModules = {};
-
-/******/ // The require function
-/******/ function __webpack_require__(moduleId) {
-
-/******/ // Check if module is in cache
-/******/ if(installedModules[moduleId])
-/******/ return installedModules[moduleId].exports;
-
-/******/ // Create a new module (and put it into the cache)
-/******/ var module = installedModules[moduleId] = {
-/******/ exports: {},
-/******/ id: moduleId,
-/******/ loaded: false
-/******/ };
-
-/******/ // Execute the module function
-/******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
-
-/******/ // Flag the module as loaded
-/******/ module.loaded = true;
-
-/******/ // Return the exports of the module
-/******/ return module.exports;
-/******/ }
-
-
-/******/ // expose the modules object (__webpack_modules__)
-/******/ __webpack_require__.m = modules;
-
-/******/ // expose the module cache
-/******/ __webpack_require__.c = installedModules;
-
-/******/ // __webpack_public_path__
-/******/ __webpack_require__.p = "/";
-
-/******/ // Load entry module and return exports
-/******/ return __webpack_require__(0);
-/******/ })
-/************************************************************************/
-/******/ ([
-/* 0 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(1);
- module.exports = __webpack_require__(332);
-
-
-/***/ }),
-/* 1 */
-/***/ (function(module, exports, __webpack_require__) {
-
- /* WEBPACK VAR INJECTION */(function(global) {"use strict";
-
- __webpack_require__(2);
-
- __webpack_require__(328);
-
- __webpack_require__(329);
-
- if (global._babelPolyfill) {
- throw new Error("only one instance of babel-polyfill is allowed");
- }
- global._babelPolyfill = true;
-
- var DEFINE_PROPERTY = "defineProperty";
- function define(O, key, value) {
- O[key] || Object[DEFINE_PROPERTY](O, key, {
- writable: true,
- configurable: true,
- value: value
- });
- }
-
- define(String.prototype, "padLeft", "".padStart);
- define(String.prototype, "padRight", "".padEnd);
-
- "pop,reverse,shift,keys,values,entries,indexOf,every,some,forEach,map,filter,find,findIndex,includes,join,slice,concat,push,splice,unshift,sort,lastIndexOf,reduce,reduceRight,copyWithin,fill".split(",").forEach(function (key) {
- [][key] && define(Array, key, Function.call.bind([][key]));
- });
- /* WEBPACK VAR INJECTION */}.call(exports, (function() { return this; }())))
-
-/***/ }),
-/* 2 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(3);
- __webpack_require__(53);
- __webpack_require__(54);
- __webpack_require__(55);
- __webpack_require__(56);
- __webpack_require__(58);
- __webpack_require__(60);
- __webpack_require__(61);
- __webpack_require__(62);
- __webpack_require__(63);
- __webpack_require__(64);
- __webpack_require__(65);
- __webpack_require__(66);
- __webpack_require__(67);
- __webpack_require__(68);
- __webpack_require__(70);
- __webpack_require__(72);
- __webpack_require__(74);
- __webpack_require__(76);
- __webpack_require__(79);
- __webpack_require__(80);
- __webpack_require__(81);
- __webpack_require__(85);
- __webpack_require__(87);
- __webpack_require__(89);
- __webpack_require__(92);
- __webpack_require__(93);
- __webpack_require__(94);
- __webpack_require__(95);
- __webpack_require__(97);
- __webpack_require__(98);
- __webpack_require__(99);
- __webpack_require__(100);
- __webpack_require__(101);
- __webpack_require__(102);
- __webpack_require__(103);
- __webpack_require__(105);
- __webpack_require__(106);
- __webpack_require__(107);
- __webpack_require__(109);
- __webpack_require__(110);
- __webpack_require__(111);
- __webpack_require__(113);
- __webpack_require__(115);
- __webpack_require__(116);
- __webpack_require__(117);
- __webpack_require__(118);
- __webpack_require__(119);
- __webpack_require__(120);
- __webpack_require__(121);
- __webpack_require__(122);
- __webpack_require__(123);
- __webpack_require__(124);
- __webpack_require__(125);
- __webpack_require__(126);
- __webpack_require__(127);
- __webpack_require__(132);
- __webpack_require__(133);
- __webpack_require__(137);
- __webpack_require__(138);
- __webpack_require__(139);
- __webpack_require__(140);
- __webpack_require__(142);
- __webpack_require__(143);
- __webpack_require__(144);
- __webpack_require__(145);
- __webpack_require__(146);
- __webpack_require__(147);
- __webpack_require__(148);
- __webpack_require__(149);
- __webpack_require__(150);
- __webpack_require__(151);
- __webpack_require__(152);
- __webpack_require__(153);
- __webpack_require__(154);
- __webpack_require__(155);
- __webpack_require__(156);
- __webpack_require__(158);
- __webpack_require__(159);
- __webpack_require__(161);
- __webpack_require__(162);
- __webpack_require__(168);
- __webpack_require__(169);
- __webpack_require__(171);
- __webpack_require__(172);
- __webpack_require__(173);
- __webpack_require__(177);
- __webpack_require__(178);
- __webpack_require__(179);
- __webpack_require__(180);
- __webpack_require__(181);
- __webpack_require__(183);
- __webpack_require__(184);
- __webpack_require__(185);
- __webpack_require__(186);
- __webpack_require__(189);
- __webpack_require__(191);
- __webpack_require__(192);
- __webpack_require__(193);
- __webpack_require__(195);
- __webpack_require__(197);
- __webpack_require__(199);
- __webpack_require__(201);
- __webpack_require__(202);
- __webpack_require__(203);
- __webpack_require__(207);
- __webpack_require__(208);
- __webpack_require__(209);
- __webpack_require__(211);
- __webpack_require__(221);
- __webpack_require__(225);
- __webpack_require__(226);
- __webpack_require__(228);
- __webpack_require__(229);
- __webpack_require__(233);
- __webpack_require__(234);
- __webpack_require__(236);
- __webpack_require__(237);
- __webpack_require__(238);
- __webpack_require__(239);
- __webpack_require__(240);
- __webpack_require__(241);
- __webpack_require__(242);
- __webpack_require__(243);
- __webpack_require__(244);
- __webpack_require__(245);
- __webpack_require__(246);
- __webpack_require__(247);
- __webpack_require__(248);
- __webpack_require__(249);
- __webpack_require__(250);
- __webpack_require__(251);
- __webpack_require__(252);
- __webpack_require__(253);
- __webpack_require__(254);
- __webpack_require__(256);
- __webpack_require__(257);
- __webpack_require__(258);
- __webpack_require__(259);
- __webpack_require__(260);
- __webpack_require__(262);
- __webpack_require__(263);
- __webpack_require__(264);
- __webpack_require__(266);
- __webpack_require__(267);
- __webpack_require__(268);
- __webpack_require__(269);
- __webpack_require__(270);
- __webpack_require__(271);
- __webpack_require__(272);
- __webpack_require__(273);
- __webpack_require__(275);
- __webpack_require__(276);
- __webpack_require__(278);
- __webpack_require__(279);
- __webpack_require__(280);
- __webpack_require__(281);
- __webpack_require__(284);
- __webpack_require__(285);
- __webpack_require__(287);
- __webpack_require__(288);
- __webpack_require__(289);
- __webpack_require__(290);
- __webpack_require__(292);
- __webpack_require__(293);
- __webpack_require__(294);
- __webpack_require__(295);
- __webpack_require__(296);
- __webpack_require__(297);
- __webpack_require__(298);
- __webpack_require__(299);
- __webpack_require__(300);
- __webpack_require__(301);
- __webpack_require__(303);
- __webpack_require__(304);
- __webpack_require__(305);
- __webpack_require__(306);
- __webpack_require__(307);
- __webpack_require__(308);
- __webpack_require__(309);
- __webpack_require__(310);
- __webpack_require__(311);
- __webpack_require__(312);
- __webpack_require__(313);
- __webpack_require__(315);
- __webpack_require__(316);
- __webpack_require__(317);
- __webpack_require__(318);
- __webpack_require__(319);
- __webpack_require__(320);
- __webpack_require__(321);
- __webpack_require__(322);
- __webpack_require__(323);
- __webpack_require__(324);
- __webpack_require__(325);
- __webpack_require__(326);
- __webpack_require__(327);
- module.exports = __webpack_require__(9);
-
-
-/***/ }),
-/* 3 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // ECMAScript 6 symbols shim
- var global = __webpack_require__(4);
- var has = __webpack_require__(5);
- var DESCRIPTORS = __webpack_require__(6);
- var $export = __webpack_require__(8);
- var redefine = __webpack_require__(18);
- var META = __webpack_require__(25).KEY;
- var $fails = __webpack_require__(7);
- var shared = __webpack_require__(21);
- var setToStringTag = __webpack_require__(26);
- var uid = __webpack_require__(19);
- var wks = __webpack_require__(27);
- var wksExt = __webpack_require__(28);
- var wksDefine = __webpack_require__(29);
- var enumKeys = __webpack_require__(30);
- var isArray = __webpack_require__(45);
- var anObject = __webpack_require__(12);
- var isObject = __webpack_require__(13);
- var toObject = __webpack_require__(46);
- var toIObject = __webpack_require__(33);
- var toPrimitive = __webpack_require__(16);
- var createDesc = __webpack_require__(17);
- var _create = __webpack_require__(47);
- var gOPNExt = __webpack_require__(50);
- var $GOPD = __webpack_require__(52);
- var $GOPS = __webpack_require__(43);
- var $DP = __webpack_require__(11);
- var $keys = __webpack_require__(31);
- var gOPD = $GOPD.f;
- var dP = $DP.f;
- var gOPN = gOPNExt.f;
- var $Symbol = global.Symbol;
- var $JSON = global.JSON;
- var _stringify = $JSON && $JSON.stringify;
- var PROTOTYPE = 'prototype';
- var HIDDEN = wks('_hidden');
- var TO_PRIMITIVE = wks('toPrimitive');
- var isEnum = {}.propertyIsEnumerable;
- var SymbolRegistry = shared('symbol-registry');
- var AllSymbols = shared('symbols');
- var OPSymbols = shared('op-symbols');
- var ObjectProto = Object[PROTOTYPE];
- var USE_NATIVE = typeof $Symbol == 'function' && !!$GOPS.f;
- var QObject = global.QObject;
- // Don't use setters in Qt Script, https://github.com/zloirock/core-js/issues/173
- var setter = !QObject || !QObject[PROTOTYPE] || !QObject[PROTOTYPE].findChild;
-
- // fallback for old Android, https://code.google.com/p/v8/issues/detail?id=687
- var setSymbolDesc = DESCRIPTORS && $fails(function () {
- return _create(dP({}, 'a', {
- get: function () { return dP(this, 'a', { value: 7 }).a; }
- })).a != 7;
- }) ? function (it, key, D) {
- var protoDesc = gOPD(ObjectProto, key);
- if (protoDesc) delete ObjectProto[key];
- dP(it, key, D);
- if (protoDesc && it !== ObjectProto) dP(ObjectProto, key, protoDesc);
- } : dP;
-
- var wrap = function (tag) {
- var sym = AllSymbols[tag] = _create($Symbol[PROTOTYPE]);
- sym._k = tag;
- return sym;
- };
-
- var isSymbol = USE_NATIVE && typeof $Symbol.iterator == 'symbol' ? function (it) {
- return typeof it == 'symbol';
- } : function (it) {
- return it instanceof $Symbol;
- };
-
- var $defineProperty = function defineProperty(it, key, D) {
- if (it === ObjectProto) $defineProperty(OPSymbols, key, D);
- anObject(it);
- key = toPrimitive(key, true);
- anObject(D);
- if (has(AllSymbols, key)) {
- if (!D.enumerable) {
- if (!has(it, HIDDEN)) dP(it, HIDDEN, createDesc(1, {}));
- it[HIDDEN][key] = true;
- } else {
- if (has(it, HIDDEN) && it[HIDDEN][key]) it[HIDDEN][key] = false;
- D = _create(D, { enumerable: createDesc(0, false) });
- } return setSymbolDesc(it, key, D);
- } return dP(it, key, D);
- };
- var $defineProperties = function defineProperties(it, P) {
- anObject(it);
- var keys = enumKeys(P = toIObject(P));
- var i = 0;
- var l = keys.length;
- var key;
- while (l > i) $defineProperty(it, key = keys[i++], P[key]);
- return it;
- };
- var $create = function create(it, P) {
- return P === undefined ? _create(it) : $defineProperties(_create(it), P);
- };
- var $propertyIsEnumerable = function propertyIsEnumerable(key) {
- var E = isEnum.call(this, key = toPrimitive(key, true));
- if (this === ObjectProto && has(AllSymbols, key) && !has(OPSymbols, key)) return false;
- return E || !has(this, key) || !has(AllSymbols, key) || has(this, HIDDEN) && this[HIDDEN][key] ? E : true;
- };
- var $getOwnPropertyDescriptor = function getOwnPropertyDescriptor(it, key) {
- it = toIObject(it);
- key = toPrimitive(key, true);
- if (it === ObjectProto && has(AllSymbols, key) && !has(OPSymbols, key)) return;
- var D = gOPD(it, key);
- if (D && has(AllSymbols, key) && !(has(it, HIDDEN) && it[HIDDEN][key])) D.enumerable = true;
- return D;
- };
- var $getOwnPropertyNames = function getOwnPropertyNames(it) {
- var names = gOPN(toIObject(it));
- var result = [];
- var i = 0;
- var key;
- while (names.length > i) {
- if (!has(AllSymbols, key = names[i++]) && key != HIDDEN && key != META) result.push(key);
- } return result;
- };
- var $getOwnPropertySymbols = function getOwnPropertySymbols(it) {
- var IS_OP = it === ObjectProto;
- var names = gOPN(IS_OP ? OPSymbols : toIObject(it));
- var result = [];
- var i = 0;
- var key;
- while (names.length > i) {
- if (has(AllSymbols, key = names[i++]) && (IS_OP ? has(ObjectProto, key) : true)) result.push(AllSymbols[key]);
- } return result;
- };
-
- // 19.4.1.1 Symbol([description])
- if (!USE_NATIVE) {
- $Symbol = function Symbol() {
- if (this instanceof $Symbol) throw TypeError('Symbol is not a constructor!');
- var tag = uid(arguments.length > 0 ? arguments[0] : undefined);
- var $set = function (value) {
- if (this === ObjectProto) $set.call(OPSymbols, value);
- if (has(this, HIDDEN) && has(this[HIDDEN], tag)) this[HIDDEN][tag] = false;
- setSymbolDesc(this, tag, createDesc(1, value));
- };
- if (DESCRIPTORS && setter) setSymbolDesc(ObjectProto, tag, { configurable: true, set: $set });
- return wrap(tag);
- };
- redefine($Symbol[PROTOTYPE], 'toString', function toString() {
- return this._k;
- });
-
- $GOPD.f = $getOwnPropertyDescriptor;
- $DP.f = $defineProperty;
- __webpack_require__(51).f = gOPNExt.f = $getOwnPropertyNames;
- __webpack_require__(44).f = $propertyIsEnumerable;
- $GOPS.f = $getOwnPropertySymbols;
-
- if (DESCRIPTORS && !__webpack_require__(22)) {
- redefine(ObjectProto, 'propertyIsEnumerable', $propertyIsEnumerable, true);
- }
-
- wksExt.f = function (name) {
- return wrap(wks(name));
- };
- }
-
- $export($export.G + $export.W + $export.F * !USE_NATIVE, { Symbol: $Symbol });
-
- for (var es6Symbols = (
- // 19.4.2.2, 19.4.2.3, 19.4.2.4, 19.4.2.6, 19.4.2.8, 19.4.2.9, 19.4.2.10, 19.4.2.11, 19.4.2.12, 19.4.2.13, 19.4.2.14
- 'hasInstance,isConcatSpreadable,iterator,match,replace,search,species,split,toPrimitive,toStringTag,unscopables'
- ).split(','), j = 0; es6Symbols.length > j;)wks(es6Symbols[j++]);
-
- for (var wellKnownSymbols = $keys(wks.store), k = 0; wellKnownSymbols.length > k;) wksDefine(wellKnownSymbols[k++]);
-
- $export($export.S + $export.F * !USE_NATIVE, 'Symbol', {
- // 19.4.2.1 Symbol.for(key)
- 'for': function (key) {
- return has(SymbolRegistry, key += '')
- ? SymbolRegistry[key]
- : SymbolRegistry[key] = $Symbol(key);
- },
- // 19.4.2.5 Symbol.keyFor(sym)
- keyFor: function keyFor(sym) {
- if (!isSymbol(sym)) throw TypeError(sym + ' is not a symbol!');
- for (var key in SymbolRegistry) if (SymbolRegistry[key] === sym) return key;
- },
- useSetter: function () { setter = true; },
- useSimple: function () { setter = false; }
- });
-
- $export($export.S + $export.F * !USE_NATIVE, 'Object', {
- // 19.1.2.2 Object.create(O [, Properties])
- create: $create,
- // 19.1.2.4 Object.defineProperty(O, P, Attributes)
- defineProperty: $defineProperty,
- // 19.1.2.3 Object.defineProperties(O, Properties)
- defineProperties: $defineProperties,
- // 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
- getOwnPropertyDescriptor: $getOwnPropertyDescriptor,
- // 19.1.2.7 Object.getOwnPropertyNames(O)
- getOwnPropertyNames: $getOwnPropertyNames,
- // 19.1.2.8 Object.getOwnPropertySymbols(O)
- getOwnPropertySymbols: $getOwnPropertySymbols
- });
-
- // Chrome 38 and 39 `Object.getOwnPropertySymbols` fails on primitives
- // https://bugs.chromium.org/p/v8/issues/detail?id=3443
- var FAILS_ON_PRIMITIVES = $fails(function () { $GOPS.f(1); });
-
- $export($export.S + $export.F * FAILS_ON_PRIMITIVES, 'Object', {
- getOwnPropertySymbols: function getOwnPropertySymbols(it) {
- return $GOPS.f(toObject(it));
- }
- });
-
- // 24.3.2 JSON.stringify(value [, replacer [, space]])
- $JSON && $export($export.S + $export.F * (!USE_NATIVE || $fails(function () {
- var S = $Symbol();
- // MS Edge converts symbol values to JSON as {}
- // WebKit converts symbol values to JSON as null
- // V8 throws on boxed symbols
- return _stringify([S]) != '[null]' || _stringify({ a: S }) != '{}' || _stringify(Object(S)) != '{}';
- })), 'JSON', {
- stringify: function stringify(it) {
- var args = [it];
- var i = 1;
- var replacer, $replacer;
- while (arguments.length > i) args.push(arguments[i++]);
- $replacer = replacer = args[1];
- if (!isObject(replacer) && it === undefined || isSymbol(it)) return; // IE8 returns string on undefined
- if (!isArray(replacer)) replacer = function (key, value) {
- if (typeof $replacer == 'function') value = $replacer.call(this, key, value);
- if (!isSymbol(value)) return value;
- };
- args[1] = replacer;
- return _stringify.apply($JSON, args);
- }
- });
-
- // 19.4.3.4 Symbol.prototype[@@toPrimitive](hint)
- $Symbol[PROTOTYPE][TO_PRIMITIVE] || __webpack_require__(10)($Symbol[PROTOTYPE], TO_PRIMITIVE, $Symbol[PROTOTYPE].valueOf);
- // 19.4.3.5 Symbol.prototype[@@toStringTag]
- setToStringTag($Symbol, 'Symbol');
- // 20.2.1.9 Math[@@toStringTag]
- setToStringTag(Math, 'Math', true);
- // 24.3.3 JSON[@@toStringTag]
- setToStringTag(global.JSON, 'JSON', true);
-
-
-/***/ }),
-/* 4 */
-/***/ (function(module, exports) {
-
- // https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
- var global = module.exports = typeof window != 'undefined' && window.Math == Math
- ? window : typeof self != 'undefined' && self.Math == Math ? self
- // eslint-disable-next-line no-new-func
- : Function('return this')();
- if (typeof __g == 'number') __g = global; // eslint-disable-line no-undef
-
-
-/***/ }),
-/* 5 */
-/***/ (function(module, exports) {
-
- var hasOwnProperty = {}.hasOwnProperty;
- module.exports = function (it, key) {
- return hasOwnProperty.call(it, key);
- };
-
-
-/***/ }),
-/* 6 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // Thank's IE8 for his funny defineProperty
- module.exports = !__webpack_require__(7)(function () {
- return Object.defineProperty({}, 'a', { get: function () { return 7; } }).a != 7;
- });
-
-
-/***/ }),
-/* 7 */
-/***/ (function(module, exports) {
-
- module.exports = function (exec) {
- try {
- return !!exec();
- } catch (e) {
- return true;
- }
- };
-
-
-/***/ }),
-/* 8 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var global = __webpack_require__(4);
- var core = __webpack_require__(9);
- var hide = __webpack_require__(10);
- var redefine = __webpack_require__(18);
- var ctx = __webpack_require__(23);
- var PROTOTYPE = 'prototype';
-
- var $export = function (type, name, source) {
- var IS_FORCED = type & $export.F;
- var IS_GLOBAL = type & $export.G;
- var IS_STATIC = type & $export.S;
- var IS_PROTO = type & $export.P;
- var IS_BIND = type & $export.B;
- var target = IS_GLOBAL ? global : IS_STATIC ? global[name] || (global[name] = {}) : (global[name] || {})[PROTOTYPE];
- var exports = IS_GLOBAL ? core : core[name] || (core[name] = {});
- var expProto = exports[PROTOTYPE] || (exports[PROTOTYPE] = {});
- var key, own, out, exp;
- if (IS_GLOBAL) source = name;
- for (key in source) {
- // contains in native
- own = !IS_FORCED && target && target[key] !== undefined;
- // export native or passed
- out = (own ? target : source)[key];
- // bind timers to global for call from export context
- exp = IS_BIND && own ? ctx(out, global) : IS_PROTO && typeof out == 'function' ? ctx(Function.call, out) : out;
- // extend global
- if (target) redefine(target, key, out, type & $export.U);
- // export
- if (exports[key] != out) hide(exports, key, exp);
- if (IS_PROTO && expProto[key] != out) expProto[key] = out;
- }
- };
- global.core = core;
- // type bitmap
- $export.F = 1; // forced
- $export.G = 2; // global
- $export.S = 4; // static
- $export.P = 8; // proto
- $export.B = 16; // bind
- $export.W = 32; // wrap
- $export.U = 64; // safe
- $export.R = 128; // real proto method for `library`
- module.exports = $export;
-
-
-/***/ }),
-/* 9 */
-/***/ (function(module, exports) {
-
- var core = module.exports = { version: '2.6.10' };
- if (typeof __e == 'number') __e = core; // eslint-disable-line no-undef
-
-
-/***/ }),
-/* 10 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var dP = __webpack_require__(11);
- var createDesc = __webpack_require__(17);
- module.exports = __webpack_require__(6) ? function (object, key, value) {
- return dP.f(object, key, createDesc(1, value));
- } : function (object, key, value) {
- object[key] = value;
- return object;
- };
-
-
-/***/ }),
-/* 11 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var anObject = __webpack_require__(12);
- var IE8_DOM_DEFINE = __webpack_require__(14);
- var toPrimitive = __webpack_require__(16);
- var dP = Object.defineProperty;
-
- exports.f = __webpack_require__(6) ? Object.defineProperty : function defineProperty(O, P, Attributes) {
- anObject(O);
- P = toPrimitive(P, true);
- anObject(Attributes);
- if (IE8_DOM_DEFINE) try {
- return dP(O, P, Attributes);
- } catch (e) { /* empty */ }
- if ('get' in Attributes || 'set' in Attributes) throw TypeError('Accessors not supported!');
- if ('value' in Attributes) O[P] = Attributes.value;
- return O;
- };
-
-
-/***/ }),
-/* 12 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var isObject = __webpack_require__(13);
- module.exports = function (it) {
- if (!isObject(it)) throw TypeError(it + ' is not an object!');
- return it;
- };
-
-
-/***/ }),
-/* 13 */
-/***/ (function(module, exports) {
-
- module.exports = function (it) {
- return typeof it === 'object' ? it !== null : typeof it === 'function';
- };
-
-
-/***/ }),
-/* 14 */
-/***/ (function(module, exports, __webpack_require__) {
-
- module.exports = !__webpack_require__(6) && !__webpack_require__(7)(function () {
- return Object.defineProperty(__webpack_require__(15)('div'), 'a', { get: function () { return 7; } }).a != 7;
- });
-
-
-/***/ }),
-/* 15 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var isObject = __webpack_require__(13);
- var document = __webpack_require__(4).document;
- // typeof document.createElement is 'object' in old IE
- var is = isObject(document) && isObject(document.createElement);
- module.exports = function (it) {
- return is ? document.createElement(it) : {};
- };
-
-
-/***/ }),
-/* 16 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 7.1.1 ToPrimitive(input [, PreferredType])
- var isObject = __webpack_require__(13);
- // instead of the ES6 spec version, we didn't implement @@toPrimitive case
- // and the second argument - flag - preferred type is a string
- module.exports = function (it, S) {
- if (!isObject(it)) return it;
- var fn, val;
- if (S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it))) return val;
- if (typeof (fn = it.valueOf) == 'function' && !isObject(val = fn.call(it))) return val;
- if (!S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it))) return val;
- throw TypeError("Can't convert object to primitive value");
- };
-
-
-/***/ }),
-/* 17 */
-/***/ (function(module, exports) {
-
- module.exports = function (bitmap, value) {
- return {
- enumerable: !(bitmap & 1),
- configurable: !(bitmap & 2),
- writable: !(bitmap & 4),
- value: value
- };
- };
-
-
-/***/ }),
-/* 18 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var global = __webpack_require__(4);
- var hide = __webpack_require__(10);
- var has = __webpack_require__(5);
- var SRC = __webpack_require__(19)('src');
- var $toString = __webpack_require__(20);
- var TO_STRING = 'toString';
- var TPL = ('' + $toString).split(TO_STRING);
-
- __webpack_require__(9).inspectSource = function (it) {
- return $toString.call(it);
- };
-
- (module.exports = function (O, key, val, safe) {
- var isFunction = typeof val == 'function';
- if (isFunction) has(val, 'name') || hide(val, 'name', key);
- if (O[key] === val) return;
- if (isFunction) has(val, SRC) || hide(val, SRC, O[key] ? '' + O[key] : TPL.join(String(key)));
- if (O === global) {
- O[key] = val;
- } else if (!safe) {
- delete O[key];
- hide(O, key, val);
- } else if (O[key]) {
- O[key] = val;
- } else {
- hide(O, key, val);
- }
- // add fake Function#toString for correct work wrapped methods / constructors with methods like LoDash isNative
- })(Function.prototype, TO_STRING, function toString() {
- return typeof this == 'function' && this[SRC] || $toString.call(this);
- });
-
-
-/***/ }),
-/* 19 */
-/***/ (function(module, exports) {
-
- var id = 0;
- var px = Math.random();
- module.exports = function (key) {
- return 'Symbol('.concat(key === undefined ? '' : key, ')_', (++id + px).toString(36));
- };
-
-
-/***/ }),
-/* 20 */
-/***/ (function(module, exports, __webpack_require__) {
-
- module.exports = __webpack_require__(21)('native-function-to-string', Function.toString);
-
-
-/***/ }),
-/* 21 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var core = __webpack_require__(9);
- var global = __webpack_require__(4);
- var SHARED = '__core-js_shared__';
- var store = global[SHARED] || (global[SHARED] = {});
-
- (module.exports = function (key, value) {
- return store[key] || (store[key] = value !== undefined ? value : {});
- })('versions', []).push({
- version: core.version,
- mode: __webpack_require__(22) ? 'pure' : 'global',
- copyright: '© 2019 Denis Pushkarev (zloirock.ru)'
- });
-
-
-/***/ }),
-/* 22 */
-/***/ (function(module, exports) {
-
- module.exports = false;
-
-
-/***/ }),
-/* 23 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // optional / simple context binding
- var aFunction = __webpack_require__(24);
- module.exports = function (fn, that, length) {
- aFunction(fn);
- if (that === undefined) return fn;
- switch (length) {
- case 1: return function (a) {
- return fn.call(that, a);
- };
- case 2: return function (a, b) {
- return fn.call(that, a, b);
- };
- case 3: return function (a, b, c) {
- return fn.call(that, a, b, c);
- };
- }
- return function (/* ...args */) {
- return fn.apply(that, arguments);
- };
- };
-
-
-/***/ }),
-/* 24 */
-/***/ (function(module, exports) {
-
- module.exports = function (it) {
- if (typeof it != 'function') throw TypeError(it + ' is not a function!');
- return it;
- };
-
-
-/***/ }),
-/* 25 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var META = __webpack_require__(19)('meta');
- var isObject = __webpack_require__(13);
- var has = __webpack_require__(5);
- var setDesc = __webpack_require__(11).f;
- var id = 0;
- var isExtensible = Object.isExtensible || function () {
- return true;
- };
- var FREEZE = !__webpack_require__(7)(function () {
- return isExtensible(Object.preventExtensions({}));
- });
- var setMeta = function (it) {
- setDesc(it, META, { value: {
- i: 'O' + ++id, // object ID
- w: {} // weak collections IDs
- } });
- };
- var fastKey = function (it, create) {
- // return primitive with prefix
- if (!isObject(it)) return typeof it == 'symbol' ? it : (typeof it == 'string' ? 'S' : 'P') + it;
- if (!has(it, META)) {
- // can't set metadata to uncaught frozen object
- if (!isExtensible(it)) return 'F';
- // not necessary to add metadata
- if (!create) return 'E';
- // add missing metadata
- setMeta(it);
- // return object ID
- } return it[META].i;
- };
- var getWeak = function (it, create) {
- if (!has(it, META)) {
- // can't set metadata to uncaught frozen object
- if (!isExtensible(it)) return true;
- // not necessary to add metadata
- if (!create) return false;
- // add missing metadata
- setMeta(it);
- // return hash weak collections IDs
- } return it[META].w;
- };
- // add metadata on freeze-family methods calling
- var onFreeze = function (it) {
- if (FREEZE && meta.NEED && isExtensible(it) && !has(it, META)) setMeta(it);
- return it;
- };
- var meta = module.exports = {
- KEY: META,
- NEED: false,
- fastKey: fastKey,
- getWeak: getWeak,
- onFreeze: onFreeze
- };
-
-
-/***/ }),
-/* 26 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var def = __webpack_require__(11).f;
- var has = __webpack_require__(5);
- var TAG = __webpack_require__(27)('toStringTag');
-
- module.exports = function (it, tag, stat) {
- if (it && !has(it = stat ? it : it.prototype, TAG)) def(it, TAG, { configurable: true, value: tag });
- };
-
-
-/***/ }),
-/* 27 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var store = __webpack_require__(21)('wks');
- var uid = __webpack_require__(19);
- var Symbol = __webpack_require__(4).Symbol;
- var USE_SYMBOL = typeof Symbol == 'function';
-
- var $exports = module.exports = function (name) {
- return store[name] || (store[name] =
- USE_SYMBOL && Symbol[name] || (USE_SYMBOL ? Symbol : uid)('Symbol.' + name));
- };
-
- $exports.store = store;
-
-
-/***/ }),
-/* 28 */
-/***/ (function(module, exports, __webpack_require__) {
-
- exports.f = __webpack_require__(27);
-
-
-/***/ }),
-/* 29 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var global = __webpack_require__(4);
- var core = __webpack_require__(9);
- var LIBRARY = __webpack_require__(22);
- var wksExt = __webpack_require__(28);
- var defineProperty = __webpack_require__(11).f;
- module.exports = function (name) {
- var $Symbol = core.Symbol || (core.Symbol = LIBRARY ? {} : global.Symbol || {});
- if (name.charAt(0) != '_' && !(name in $Symbol)) defineProperty($Symbol, name, { value: wksExt.f(name) });
- };
-
-
-/***/ }),
-/* 30 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // all enumerable object keys, includes symbols
- var getKeys = __webpack_require__(31);
- var gOPS = __webpack_require__(43);
- var pIE = __webpack_require__(44);
- module.exports = function (it) {
- var result = getKeys(it);
- var getSymbols = gOPS.f;
- if (getSymbols) {
- var symbols = getSymbols(it);
- var isEnum = pIE.f;
- var i = 0;
- var key;
- while (symbols.length > i) if (isEnum.call(it, key = symbols[i++])) result.push(key);
- } return result;
- };
-
-
-/***/ }),
-/* 31 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.14 / 15.2.3.14 Object.keys(O)
- var $keys = __webpack_require__(32);
- var enumBugKeys = __webpack_require__(42);
-
- module.exports = Object.keys || function keys(O) {
- return $keys(O, enumBugKeys);
- };
-
-
-/***/ }),
-/* 32 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var has = __webpack_require__(5);
- var toIObject = __webpack_require__(33);
- var arrayIndexOf = __webpack_require__(37)(false);
- var IE_PROTO = __webpack_require__(41)('IE_PROTO');
-
- module.exports = function (object, names) {
- var O = toIObject(object);
- var i = 0;
- var result = [];
- var key;
- for (key in O) if (key != IE_PROTO) has(O, key) && result.push(key);
- // Don't enum bug & hidden keys
- while (names.length > i) if (has(O, key = names[i++])) {
- ~arrayIndexOf(result, key) || result.push(key);
- }
- return result;
- };
-
-
-/***/ }),
-/* 33 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // to indexed object, toObject with fallback for non-array-like ES3 strings
- var IObject = __webpack_require__(34);
- var defined = __webpack_require__(36);
- module.exports = function (it) {
- return IObject(defined(it));
- };
-
-
-/***/ }),
-/* 34 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // fallback for non-array-like ES3 and non-enumerable old V8 strings
- var cof = __webpack_require__(35);
- // eslint-disable-next-line no-prototype-builtins
- module.exports = Object('z').propertyIsEnumerable(0) ? Object : function (it) {
- return cof(it) == 'String' ? it.split('') : Object(it);
- };
-
-
-/***/ }),
-/* 35 */
-/***/ (function(module, exports) {
-
- var toString = {}.toString;
-
- module.exports = function (it) {
- return toString.call(it).slice(8, -1);
- };
-
-
-/***/ }),
-/* 36 */
-/***/ (function(module, exports) {
-
- // 7.2.1 RequireObjectCoercible(argument)
- module.exports = function (it) {
- if (it == undefined) throw TypeError("Can't call method on " + it);
- return it;
- };
-
-
-/***/ }),
-/* 37 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // false -> Array#indexOf
- // true -> Array#includes
- var toIObject = __webpack_require__(33);
- var toLength = __webpack_require__(38);
- var toAbsoluteIndex = __webpack_require__(40);
- module.exports = function (IS_INCLUDES) {
- return function ($this, el, fromIndex) {
- var O = toIObject($this);
- var length = toLength(O.length);
- var index = toAbsoluteIndex(fromIndex, length);
- var value;
- // Array#includes uses SameValueZero equality algorithm
- // eslint-disable-next-line no-self-compare
- if (IS_INCLUDES && el != el) while (length > index) {
- value = O[index++];
- // eslint-disable-next-line no-self-compare
- if (value != value) return true;
- // Array#indexOf ignores holes, Array#includes - not
- } else for (;length > index; index++) if (IS_INCLUDES || index in O) {
- if (O[index] === el) return IS_INCLUDES || index || 0;
- } return !IS_INCLUDES && -1;
- };
- };
-
-
-/***/ }),
-/* 38 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 7.1.15 ToLength
- var toInteger = __webpack_require__(39);
- var min = Math.min;
- module.exports = function (it) {
- return it > 0 ? min(toInteger(it), 0x1fffffffffffff) : 0; // pow(2, 53) - 1 == 9007199254740991
- };
-
-
-/***/ }),
-/* 39 */
-/***/ (function(module, exports) {
-
- // 7.1.4 ToInteger
- var ceil = Math.ceil;
- var floor = Math.floor;
- module.exports = function (it) {
- return isNaN(it = +it) ? 0 : (it > 0 ? floor : ceil)(it);
- };
-
-
-/***/ }),
-/* 40 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var toInteger = __webpack_require__(39);
- var max = Math.max;
- var min = Math.min;
- module.exports = function (index, length) {
- index = toInteger(index);
- return index < 0 ? max(index + length, 0) : min(index, length);
- };
-
-
-/***/ }),
-/* 41 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var shared = __webpack_require__(21)('keys');
- var uid = __webpack_require__(19);
- module.exports = function (key) {
- return shared[key] || (shared[key] = uid(key));
- };
-
-
-/***/ }),
-/* 42 */
-/***/ (function(module, exports) {
-
- // IE 8- don't enum bug keys
- module.exports = (
- 'constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,toLocaleString,toString,valueOf'
- ).split(',');
-
-
-/***/ }),
-/* 43 */
-/***/ (function(module, exports) {
-
- exports.f = Object.getOwnPropertySymbols;
-
-
-/***/ }),
-/* 44 */
-/***/ (function(module, exports) {
-
- exports.f = {}.propertyIsEnumerable;
-
-
-/***/ }),
-/* 45 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 7.2.2 IsArray(argument)
- var cof = __webpack_require__(35);
- module.exports = Array.isArray || function isArray(arg) {
- return cof(arg) == 'Array';
- };
-
-
-/***/ }),
-/* 46 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 7.1.13 ToObject(argument)
- var defined = __webpack_require__(36);
- module.exports = function (it) {
- return Object(defined(it));
- };
-
-
-/***/ }),
-/* 47 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.2 / 15.2.3.5 Object.create(O [, Properties])
- var anObject = __webpack_require__(12);
- var dPs = __webpack_require__(48);
- var enumBugKeys = __webpack_require__(42);
- var IE_PROTO = __webpack_require__(41)('IE_PROTO');
- var Empty = function () { /* empty */ };
- var PROTOTYPE = 'prototype';
-
- // Create object with fake `null` prototype: use iframe Object with cleared prototype
- var createDict = function () {
- // Thrash, waste and sodomy: IE GC bug
- var iframe = __webpack_require__(15)('iframe');
- var i = enumBugKeys.length;
- var lt = '<';
- var gt = '>';
- var iframeDocument;
- iframe.style.display = 'none';
- __webpack_require__(49).appendChild(iframe);
- iframe.src = 'javascript:'; // eslint-disable-line no-script-url
- // createDict = iframe.contentWindow.Object;
- // html.removeChild(iframe);
- iframeDocument = iframe.contentWindow.document;
- iframeDocument.open();
- iframeDocument.write(lt + 'script' + gt + 'document.F=Object' + lt + '/script' + gt);
- iframeDocument.close();
- createDict = iframeDocument.F;
- while (i--) delete createDict[PROTOTYPE][enumBugKeys[i]];
- return createDict();
- };
-
- module.exports = Object.create || function create(O, Properties) {
- var result;
- if (O !== null) {
- Empty[PROTOTYPE] = anObject(O);
- result = new Empty();
- Empty[PROTOTYPE] = null;
- // add "__proto__" for Object.getPrototypeOf polyfill
- result[IE_PROTO] = O;
- } else result = createDict();
- return Properties === undefined ? result : dPs(result, Properties);
- };
-
-
-/***/ }),
-/* 48 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var dP = __webpack_require__(11);
- var anObject = __webpack_require__(12);
- var getKeys = __webpack_require__(31);
-
- module.exports = __webpack_require__(6) ? Object.defineProperties : function defineProperties(O, Properties) {
- anObject(O);
- var keys = getKeys(Properties);
- var length = keys.length;
- var i = 0;
- var P;
- while (length > i) dP.f(O, P = keys[i++], Properties[P]);
- return O;
- };
-
-
-/***/ }),
-/* 49 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var document = __webpack_require__(4).document;
- module.exports = document && document.documentElement;
-
-
-/***/ }),
-/* 50 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // fallback for IE11 buggy Object.getOwnPropertyNames with iframe and window
- var toIObject = __webpack_require__(33);
- var gOPN = __webpack_require__(51).f;
- var toString = {}.toString;
-
- var windowNames = typeof window == 'object' && window && Object.getOwnPropertyNames
- ? Object.getOwnPropertyNames(window) : [];
-
- var getWindowNames = function (it) {
- try {
- return gOPN(it);
- } catch (e) {
- return windowNames.slice();
- }
- };
-
- module.exports.f = function getOwnPropertyNames(it) {
- return windowNames && toString.call(it) == '[object Window]' ? getWindowNames(it) : gOPN(toIObject(it));
- };
-
-
-/***/ }),
-/* 51 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.7 / 15.2.3.4 Object.getOwnPropertyNames(O)
- var $keys = __webpack_require__(32);
- var hiddenKeys = __webpack_require__(42).concat('length', 'prototype');
-
- exports.f = Object.getOwnPropertyNames || function getOwnPropertyNames(O) {
- return $keys(O, hiddenKeys);
- };
-
-
-/***/ }),
-/* 52 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var pIE = __webpack_require__(44);
- var createDesc = __webpack_require__(17);
- var toIObject = __webpack_require__(33);
- var toPrimitive = __webpack_require__(16);
- var has = __webpack_require__(5);
- var IE8_DOM_DEFINE = __webpack_require__(14);
- var gOPD = Object.getOwnPropertyDescriptor;
-
- exports.f = __webpack_require__(6) ? gOPD : function getOwnPropertyDescriptor(O, P) {
- O = toIObject(O);
- P = toPrimitive(P, true);
- if (IE8_DOM_DEFINE) try {
- return gOPD(O, P);
- } catch (e) { /* empty */ }
- if (has(O, P)) return createDesc(!pIE.f.call(O, P), O[P]);
- };
-
-
-/***/ }),
-/* 53 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- // 19.1.2.2 / 15.2.3.5 Object.create(O [, Properties])
- $export($export.S, 'Object', { create: __webpack_require__(47) });
-
-
-/***/ }),
-/* 54 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- // 19.1.2.4 / 15.2.3.6 Object.defineProperty(O, P, Attributes)
- $export($export.S + $export.F * !__webpack_require__(6), 'Object', { defineProperty: __webpack_require__(11).f });
-
-
-/***/ }),
-/* 55 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- // 19.1.2.3 / 15.2.3.7 Object.defineProperties(O, Properties)
- $export($export.S + $export.F * !__webpack_require__(6), 'Object', { defineProperties: __webpack_require__(48) });
-
-
-/***/ }),
-/* 56 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
- var toIObject = __webpack_require__(33);
- var $getOwnPropertyDescriptor = __webpack_require__(52).f;
-
- __webpack_require__(57)('getOwnPropertyDescriptor', function () {
- return function getOwnPropertyDescriptor(it, key) {
- return $getOwnPropertyDescriptor(toIObject(it), key);
- };
- });
-
-
-/***/ }),
-/* 57 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // most Object methods by ES6 should accept primitives
- var $export = __webpack_require__(8);
- var core = __webpack_require__(9);
- var fails = __webpack_require__(7);
- module.exports = function (KEY, exec) {
- var fn = (core.Object || {})[KEY] || Object[KEY];
- var exp = {};
- exp[KEY] = exec(fn);
- $export($export.S + $export.F * fails(function () { fn(1); }), 'Object', exp);
- };
-
-
-/***/ }),
-/* 58 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.9 Object.getPrototypeOf(O)
- var toObject = __webpack_require__(46);
- var $getPrototypeOf = __webpack_require__(59);
-
- __webpack_require__(57)('getPrototypeOf', function () {
- return function getPrototypeOf(it) {
- return $getPrototypeOf(toObject(it));
- };
- });
-
-
-/***/ }),
-/* 59 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.9 / 15.2.3.2 Object.getPrototypeOf(O)
- var has = __webpack_require__(5);
- var toObject = __webpack_require__(46);
- var IE_PROTO = __webpack_require__(41)('IE_PROTO');
- var ObjectProto = Object.prototype;
-
- module.exports = Object.getPrototypeOf || function (O) {
- O = toObject(O);
- if (has(O, IE_PROTO)) return O[IE_PROTO];
- if (typeof O.constructor == 'function' && O instanceof O.constructor) {
- return O.constructor.prototype;
- } return O instanceof Object ? ObjectProto : null;
- };
-
-
-/***/ }),
-/* 60 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.14 Object.keys(O)
- var toObject = __webpack_require__(46);
- var $keys = __webpack_require__(31);
-
- __webpack_require__(57)('keys', function () {
- return function keys(it) {
- return $keys(toObject(it));
- };
- });
-
-
-/***/ }),
-/* 61 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.7 Object.getOwnPropertyNames(O)
- __webpack_require__(57)('getOwnPropertyNames', function () {
- return __webpack_require__(50).f;
- });
-
-
-/***/ }),
-/* 62 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.5 Object.freeze(O)
- var isObject = __webpack_require__(13);
- var meta = __webpack_require__(25).onFreeze;
-
- __webpack_require__(57)('freeze', function ($freeze) {
- return function freeze(it) {
- return $freeze && isObject(it) ? $freeze(meta(it)) : it;
- };
- });
-
-
-/***/ }),
-/* 63 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.17 Object.seal(O)
- var isObject = __webpack_require__(13);
- var meta = __webpack_require__(25).onFreeze;
-
- __webpack_require__(57)('seal', function ($seal) {
- return function seal(it) {
- return $seal && isObject(it) ? $seal(meta(it)) : it;
- };
- });
-
-
-/***/ }),
-/* 64 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.15 Object.preventExtensions(O)
- var isObject = __webpack_require__(13);
- var meta = __webpack_require__(25).onFreeze;
-
- __webpack_require__(57)('preventExtensions', function ($preventExtensions) {
- return function preventExtensions(it) {
- return $preventExtensions && isObject(it) ? $preventExtensions(meta(it)) : it;
- };
- });
-
-
-/***/ }),
-/* 65 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.12 Object.isFrozen(O)
- var isObject = __webpack_require__(13);
-
- __webpack_require__(57)('isFrozen', function ($isFrozen) {
- return function isFrozen(it) {
- return isObject(it) ? $isFrozen ? $isFrozen(it) : false : true;
- };
- });
-
-
-/***/ }),
-/* 66 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.13 Object.isSealed(O)
- var isObject = __webpack_require__(13);
-
- __webpack_require__(57)('isSealed', function ($isSealed) {
- return function isSealed(it) {
- return isObject(it) ? $isSealed ? $isSealed(it) : false : true;
- };
- });
-
-
-/***/ }),
-/* 67 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.2.11 Object.isExtensible(O)
- var isObject = __webpack_require__(13);
-
- __webpack_require__(57)('isExtensible', function ($isExtensible) {
- return function isExtensible(it) {
- return isObject(it) ? $isExtensible ? $isExtensible(it) : true : false;
- };
- });
-
-
-/***/ }),
-/* 68 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.3.1 Object.assign(target, source)
- var $export = __webpack_require__(8);
-
- $export($export.S + $export.F, 'Object', { assign: __webpack_require__(69) });
-
-
-/***/ }),
-/* 69 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // 19.1.2.1 Object.assign(target, source, ...)
- var DESCRIPTORS = __webpack_require__(6);
- var getKeys = __webpack_require__(31);
- var gOPS = __webpack_require__(43);
- var pIE = __webpack_require__(44);
- var toObject = __webpack_require__(46);
- var IObject = __webpack_require__(34);
- var $assign = Object.assign;
-
- // should work with symbols and should have deterministic property order (V8 bug)
- module.exports = !$assign || __webpack_require__(7)(function () {
- var A = {};
- var B = {};
- // eslint-disable-next-line no-undef
- var S = Symbol();
- var K = 'abcdefghijklmnopqrst';
- A[S] = 7;
- K.split('').forEach(function (k) { B[k] = k; });
- return $assign({}, A)[S] != 7 || Object.keys($assign({}, B)).join('') != K;
- }) ? function assign(target, source) { // eslint-disable-line no-unused-vars
- var T = toObject(target);
- var aLen = arguments.length;
- var index = 1;
- var getSymbols = gOPS.f;
- var isEnum = pIE.f;
- while (aLen > index) {
- var S = IObject(arguments[index++]);
- var keys = getSymbols ? getKeys(S).concat(getSymbols(S)) : getKeys(S);
- var length = keys.length;
- var j = 0;
- var key;
- while (length > j) {
- key = keys[j++];
- if (!DESCRIPTORS || isEnum.call(S, key)) T[key] = S[key];
- }
- } return T;
- } : $assign;
-
-
-/***/ }),
-/* 70 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.3.10 Object.is(value1, value2)
- var $export = __webpack_require__(8);
- $export($export.S, 'Object', { is: __webpack_require__(71) });
-
-
-/***/ }),
-/* 71 */
-/***/ (function(module, exports) {
-
- // 7.2.9 SameValue(x, y)
- module.exports = Object.is || function is(x, y) {
- // eslint-disable-next-line no-self-compare
- return x === y ? x !== 0 || 1 / x === 1 / y : x != x && y != y;
- };
-
-
-/***/ }),
-/* 72 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.1.3.19 Object.setPrototypeOf(O, proto)
- var $export = __webpack_require__(8);
- $export($export.S, 'Object', { setPrototypeOf: __webpack_require__(73).set });
-
-
-/***/ }),
-/* 73 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // Works with __proto__ only. Old v8 can't work with null proto objects.
- /* eslint-disable no-proto */
- var isObject = __webpack_require__(13);
- var anObject = __webpack_require__(12);
- var check = function (O, proto) {
- anObject(O);
- if (!isObject(proto) && proto !== null) throw TypeError(proto + ": can't set as prototype!");
- };
- module.exports = {
- set: Object.setPrototypeOf || ('__proto__' in {} ? // eslint-disable-line
- function (test, buggy, set) {
- try {
- set = __webpack_require__(23)(Function.call, __webpack_require__(52).f(Object.prototype, '__proto__').set, 2);
- set(test, []);
- buggy = !(test instanceof Array);
- } catch (e) { buggy = true; }
- return function setPrototypeOf(O, proto) {
- check(O, proto);
- if (buggy) O.__proto__ = proto;
- else set(O, proto);
- return O;
- };
- }({}, false) : undefined),
- check: check
- };
-
-
-/***/ }),
-/* 74 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // 19.1.3.6 Object.prototype.toString()
- var classof = __webpack_require__(75);
- var test = {};
- test[__webpack_require__(27)('toStringTag')] = 'z';
- if (test + '' != '[object z]') {
- __webpack_require__(18)(Object.prototype, 'toString', function toString() {
- return '[object ' + classof(this) + ']';
- }, true);
- }
-
-
-/***/ }),
-/* 75 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // getting tag from 19.1.3.6 Object.prototype.toString()
- var cof = __webpack_require__(35);
- var TAG = __webpack_require__(27)('toStringTag');
- // ES3 wrong here
- var ARG = cof(function () { return arguments; }()) == 'Arguments';
-
- // fallback for IE11 Script Access Denied error
- var tryGet = function (it, key) {
- try {
- return it[key];
- } catch (e) { /* empty */ }
- };
-
- module.exports = function (it) {
- var O, T, B;
- return it === undefined ? 'Undefined' : it === null ? 'Null'
- // @@toStringTag case
- : typeof (T = tryGet(O = Object(it), TAG)) == 'string' ? T
- // builtinTag case
- : ARG ? cof(O)
- // ES3 arguments fallback
- : (B = cof(O)) == 'Object' && typeof O.callee == 'function' ? 'Arguments' : B;
- };
-
-
-/***/ }),
-/* 76 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 19.2.3.2 / 15.3.4.5 Function.prototype.bind(thisArg, args...)
- var $export = __webpack_require__(8);
-
- $export($export.P, 'Function', { bind: __webpack_require__(77) });
-
-
-/***/ }),
-/* 77 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var aFunction = __webpack_require__(24);
- var isObject = __webpack_require__(13);
- var invoke = __webpack_require__(78);
- var arraySlice = [].slice;
- var factories = {};
-
- var construct = function (F, len, args) {
- if (!(len in factories)) {
- for (var n = [], i = 0; i < len; i++) n[i] = 'a[' + i + ']';
- // eslint-disable-next-line no-new-func
- factories[len] = Function('F,a', 'return new F(' + n.join(',') + ')');
- } return factories[len](F, args);
- };
-
- module.exports = Function.bind || function bind(that /* , ...args */) {
- var fn = aFunction(this);
- var partArgs = arraySlice.call(arguments, 1);
- var bound = function (/* args... */) {
- var args = partArgs.concat(arraySlice.call(arguments));
- return this instanceof bound ? construct(fn, args.length, args) : invoke(fn, args, that);
- };
- if (isObject(fn.prototype)) bound.prototype = fn.prototype;
- return bound;
- };
-
-
-/***/ }),
-/* 78 */
-/***/ (function(module, exports) {
-
- // fast apply, http://jsperf.lnkit.com/fast-apply/5
- module.exports = function (fn, args, that) {
- var un = that === undefined;
- switch (args.length) {
- case 0: return un ? fn()
- : fn.call(that);
- case 1: return un ? fn(args[0])
- : fn.call(that, args[0]);
- case 2: return un ? fn(args[0], args[1])
- : fn.call(that, args[0], args[1]);
- case 3: return un ? fn(args[0], args[1], args[2])
- : fn.call(that, args[0], args[1], args[2]);
- case 4: return un ? fn(args[0], args[1], args[2], args[3])
- : fn.call(that, args[0], args[1], args[2], args[3]);
- } return fn.apply(that, args);
- };
-
-
-/***/ }),
-/* 79 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var dP = __webpack_require__(11).f;
- var FProto = Function.prototype;
- var nameRE = /^\s*function ([^ (]*)/;
- var NAME = 'name';
-
- // 19.2.4.2 name
- NAME in FProto || __webpack_require__(6) && dP(FProto, NAME, {
- configurable: true,
- get: function () {
- try {
- return ('' + this).match(nameRE)[1];
- } catch (e) {
- return '';
- }
- }
- });
-
-
-/***/ }),
-/* 80 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var isObject = __webpack_require__(13);
- var getPrototypeOf = __webpack_require__(59);
- var HAS_INSTANCE = __webpack_require__(27)('hasInstance');
- var FunctionProto = Function.prototype;
- // 19.2.3.6 Function.prototype[@@hasInstance](V)
- if (!(HAS_INSTANCE in FunctionProto)) __webpack_require__(11).f(FunctionProto, HAS_INSTANCE, { value: function (O) {
- if (typeof this != 'function' || !isObject(O)) return false;
- if (!isObject(this.prototype)) return O instanceof this;
- // for environment w/o native `@@hasInstance` logic enough `instanceof`, but add this:
- while (O = getPrototypeOf(O)) if (this.prototype === O) return true;
- return false;
- } });
-
-
-/***/ }),
-/* 81 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- var $parseInt = __webpack_require__(82);
- // 18.2.5 parseInt(string, radix)
- $export($export.G + $export.F * (parseInt != $parseInt), { parseInt: $parseInt });
-
-
-/***/ }),
-/* 82 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $parseInt = __webpack_require__(4).parseInt;
- var $trim = __webpack_require__(83).trim;
- var ws = __webpack_require__(84);
- var hex = /^[-+]?0[xX]/;
-
- module.exports = $parseInt(ws + '08') !== 8 || $parseInt(ws + '0x16') !== 22 ? function parseInt(str, radix) {
- var string = $trim(String(str), 3);
- return $parseInt(string, (radix >>> 0) || (hex.test(string) ? 16 : 10));
- } : $parseInt;
-
-
-/***/ }),
-/* 83 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- var defined = __webpack_require__(36);
- var fails = __webpack_require__(7);
- var spaces = __webpack_require__(84);
- var space = '[' + spaces + ']';
- var non = '\u200b\u0085';
- var ltrim = RegExp('^' + space + space + '*');
- var rtrim = RegExp(space + space + '*$');
-
- var exporter = function (KEY, exec, ALIAS) {
- var exp = {};
- var FORCE = fails(function () {
- return !!spaces[KEY]() || non[KEY]() != non;
- });
- var fn = exp[KEY] = FORCE ? exec(trim) : spaces[KEY];
- if (ALIAS) exp[ALIAS] = fn;
- $export($export.P + $export.F * FORCE, 'String', exp);
- };
-
- // 1 -> String#trimLeft
- // 2 -> String#trimRight
- // 3 -> String#trim
- var trim = exporter.trim = function (string, TYPE) {
- string = String(defined(string));
- if (TYPE & 1) string = string.replace(ltrim, '');
- if (TYPE & 2) string = string.replace(rtrim, '');
- return string;
- };
-
- module.exports = exporter;
-
-
-/***/ }),
-/* 84 */
-/***/ (function(module, exports) {
-
- module.exports = '\x09\x0A\x0B\x0C\x0D\x20\xA0\u1680\u180E\u2000\u2001\u2002\u2003' +
- '\u2004\u2005\u2006\u2007\u2008\u2009\u200A\u202F\u205F\u3000\u2028\u2029\uFEFF';
-
-
-/***/ }),
-/* 85 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- var $parseFloat = __webpack_require__(86);
- // 18.2.4 parseFloat(string)
- $export($export.G + $export.F * (parseFloat != $parseFloat), { parseFloat: $parseFloat });
-
-
-/***/ }),
-/* 86 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $parseFloat = __webpack_require__(4).parseFloat;
- var $trim = __webpack_require__(83).trim;
-
- module.exports = 1 / $parseFloat(__webpack_require__(84) + '-0') !== -Infinity ? function parseFloat(str) {
- var string = $trim(String(str), 3);
- var result = $parseFloat(string);
- return result === 0 && string.charAt(0) == '-' ? -0 : result;
- } : $parseFloat;
-
-
-/***/ }),
-/* 87 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var global = __webpack_require__(4);
- var has = __webpack_require__(5);
- var cof = __webpack_require__(35);
- var inheritIfRequired = __webpack_require__(88);
- var toPrimitive = __webpack_require__(16);
- var fails = __webpack_require__(7);
- var gOPN = __webpack_require__(51).f;
- var gOPD = __webpack_require__(52).f;
- var dP = __webpack_require__(11).f;
- var $trim = __webpack_require__(83).trim;
- var NUMBER = 'Number';
- var $Number = global[NUMBER];
- var Base = $Number;
- var proto = $Number.prototype;
- // Opera ~12 has broken Object#toString
- var BROKEN_COF = cof(__webpack_require__(47)(proto)) == NUMBER;
- var TRIM = 'trim' in String.prototype;
-
- // 7.1.3 ToNumber(argument)
- var toNumber = function (argument) {
- var it = toPrimitive(argument, false);
- if (typeof it == 'string' && it.length > 2) {
- it = TRIM ? it.trim() : $trim(it, 3);
- var first = it.charCodeAt(0);
- var third, radix, maxCode;
- if (first === 43 || first === 45) {
- third = it.charCodeAt(2);
- if (third === 88 || third === 120) return NaN; // Number('+0x1') should be NaN, old V8 fix
- } else if (first === 48) {
- switch (it.charCodeAt(1)) {
- case 66: case 98: radix = 2; maxCode = 49; break; // fast equal /^0b[01]+$/i
- case 79: case 111: radix = 8; maxCode = 55; break; // fast equal /^0o[0-7]+$/i
- default: return +it;
- }
- for (var digits = it.slice(2), i = 0, l = digits.length, code; i < l; i++) {
- code = digits.charCodeAt(i);
- // parseInt parses a string to a first unavailable symbol
- // but ToNumber should return NaN if a string contains unavailable symbols
- if (code < 48 || code > maxCode) return NaN;
- } return parseInt(digits, radix);
- }
- } return +it;
- };
-
- if (!$Number(' 0o1') || !$Number('0b1') || $Number('+0x1')) {
- $Number = function Number(value) {
- var it = arguments.length < 1 ? 0 : value;
- var that = this;
- return that instanceof $Number
- // check on 1..constructor(foo) case
- && (BROKEN_COF ? fails(function () { proto.valueOf.call(that); }) : cof(that) != NUMBER)
- ? inheritIfRequired(new Base(toNumber(it)), that, $Number) : toNumber(it);
- };
- for (var keys = __webpack_require__(6) ? gOPN(Base) : (
- // ES3:
- 'MAX_VALUE,MIN_VALUE,NaN,NEGATIVE_INFINITY,POSITIVE_INFINITY,' +
- // ES6 (in case, if modules with ES6 Number statics required before):
- 'EPSILON,isFinite,isInteger,isNaN,isSafeInteger,MAX_SAFE_INTEGER,' +
- 'MIN_SAFE_INTEGER,parseFloat,parseInt,isInteger'
- ).split(','), j = 0, key; keys.length > j; j++) {
- if (has(Base, key = keys[j]) && !has($Number, key)) {
- dP($Number, key, gOPD(Base, key));
- }
- }
- $Number.prototype = proto;
- proto.constructor = $Number;
- __webpack_require__(18)(global, NUMBER, $Number);
- }
-
-
-/***/ }),
-/* 88 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var isObject = __webpack_require__(13);
- var setPrototypeOf = __webpack_require__(73).set;
- module.exports = function (that, target, C) {
- var S = target.constructor;
- var P;
- if (S !== C && typeof S == 'function' && (P = S.prototype) !== C.prototype && isObject(P) && setPrototypeOf) {
- setPrototypeOf(that, P);
- } return that;
- };
-
-
-/***/ }),
-/* 89 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var toInteger = __webpack_require__(39);
- var aNumberValue = __webpack_require__(90);
- var repeat = __webpack_require__(91);
- var $toFixed = 1.0.toFixed;
- var floor = Math.floor;
- var data = [0, 0, 0, 0, 0, 0];
- var ERROR = 'Number.toFixed: incorrect invocation!';
- var ZERO = '0';
-
- var multiply = function (n, c) {
- var i = -1;
- var c2 = c;
- while (++i < 6) {
- c2 += n * data[i];
- data[i] = c2 % 1e7;
- c2 = floor(c2 / 1e7);
- }
- };
- var divide = function (n) {
- var i = 6;
- var c = 0;
- while (--i >= 0) {
- c += data[i];
- data[i] = floor(c / n);
- c = (c % n) * 1e7;
- }
- };
- var numToString = function () {
- var i = 6;
- var s = '';
- while (--i >= 0) {
- if (s !== '' || i === 0 || data[i] !== 0) {
- var t = String(data[i]);
- s = s === '' ? t : s + repeat.call(ZERO, 7 - t.length) + t;
- }
- } return s;
- };
- var pow = function (x, n, acc) {
- return n === 0 ? acc : n % 2 === 1 ? pow(x, n - 1, acc * x) : pow(x * x, n / 2, acc);
- };
- var log = function (x) {
- var n = 0;
- var x2 = x;
- while (x2 >= 4096) {
- n += 12;
- x2 /= 4096;
- }
- while (x2 >= 2) {
- n += 1;
- x2 /= 2;
- } return n;
- };
-
- $export($export.P + $export.F * (!!$toFixed && (
- 0.00008.toFixed(3) !== '0.000' ||
- 0.9.toFixed(0) !== '1' ||
- 1.255.toFixed(2) !== '1.25' ||
- 1000000000000000128.0.toFixed(0) !== '1000000000000000128'
- ) || !__webpack_require__(7)(function () {
- // V8 ~ Android 4.3-
- $toFixed.call({});
- })), 'Number', {
- toFixed: function toFixed(fractionDigits) {
- var x = aNumberValue(this, ERROR);
- var f = toInteger(fractionDigits);
- var s = '';
- var m = ZERO;
- var e, z, j, k;
- if (f < 0 || f > 20) throw RangeError(ERROR);
- // eslint-disable-next-line no-self-compare
- if (x != x) return 'NaN';
- if (x <= -1e21 || x >= 1e21) return String(x);
- if (x < 0) {
- s = '-';
- x = -x;
- }
- if (x > 1e-21) {
- e = log(x * pow(2, 69, 1)) - 69;
- z = e < 0 ? x * pow(2, -e, 1) : x / pow(2, e, 1);
- z *= 0x10000000000000;
- e = 52 - e;
- if (e > 0) {
- multiply(0, z);
- j = f;
- while (j >= 7) {
- multiply(1e7, 0);
- j -= 7;
- }
- multiply(pow(10, j, 1), 0);
- j = e - 1;
- while (j >= 23) {
- divide(1 << 23);
- j -= 23;
- }
- divide(1 << j);
- multiply(1, 1);
- divide(2);
- m = numToString();
- } else {
- multiply(0, z);
- multiply(1 << -e, 0);
- m = numToString() + repeat.call(ZERO, f);
- }
- }
- if (f > 0) {
- k = m.length;
- m = s + (k <= f ? '0.' + repeat.call(ZERO, f - k) + m : m.slice(0, k - f) + '.' + m.slice(k - f));
- } else {
- m = s + m;
- } return m;
- }
- });
-
-
-/***/ }),
-/* 90 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var cof = __webpack_require__(35);
- module.exports = function (it, msg) {
- if (typeof it != 'number' && cof(it) != 'Number') throw TypeError(msg);
- return +it;
- };
-
-
-/***/ }),
-/* 91 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var toInteger = __webpack_require__(39);
- var defined = __webpack_require__(36);
-
- module.exports = function repeat(count) {
- var str = String(defined(this));
- var res = '';
- var n = toInteger(count);
- if (n < 0 || n == Infinity) throw RangeError("Count can't be negative");
- for (;n > 0; (n >>>= 1) && (str += str)) if (n & 1) res += str;
- return res;
- };
-
-
-/***/ }),
-/* 92 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $fails = __webpack_require__(7);
- var aNumberValue = __webpack_require__(90);
- var $toPrecision = 1.0.toPrecision;
-
- $export($export.P + $export.F * ($fails(function () {
- // IE7-
- return $toPrecision.call(1, undefined) !== '1';
- }) || !$fails(function () {
- // V8 ~ Android 4.3-
- $toPrecision.call({});
- })), 'Number', {
- toPrecision: function toPrecision(precision) {
- var that = aNumberValue(this, 'Number#toPrecision: incorrect invocation!');
- return precision === undefined ? $toPrecision.call(that) : $toPrecision.call(that, precision);
- }
- });
-
-
-/***/ }),
-/* 93 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.1.2.1 Number.EPSILON
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Number', { EPSILON: Math.pow(2, -52) });
-
-
-/***/ }),
-/* 94 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.1.2.2 Number.isFinite(number)
- var $export = __webpack_require__(8);
- var _isFinite = __webpack_require__(4).isFinite;
-
- $export($export.S, 'Number', {
- isFinite: function isFinite(it) {
- return typeof it == 'number' && _isFinite(it);
- }
- });
-
-
-/***/ }),
-/* 95 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.1.2.3 Number.isInteger(number)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Number', { isInteger: __webpack_require__(96) });
-
-
-/***/ }),
-/* 96 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.1.2.3 Number.isInteger(number)
- var isObject = __webpack_require__(13);
- var floor = Math.floor;
- module.exports = function isInteger(it) {
- return !isObject(it) && isFinite(it) && floor(it) === it;
- };
-
-
-/***/ }),
-/* 97 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.1.2.4 Number.isNaN(number)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Number', {
- isNaN: function isNaN(number) {
- // eslint-disable-next-line no-self-compare
- return number != number;
- }
- });
-
-
-/***/ }),
-/* 98 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.1.2.5 Number.isSafeInteger(number)
- var $export = __webpack_require__(8);
- var isInteger = __webpack_require__(96);
- var abs = Math.abs;
-
- $export($export.S, 'Number', {
- isSafeInteger: function isSafeInteger(number) {
- return isInteger(number) && abs(number) <= 0x1fffffffffffff;
- }
- });
-
-
-/***/ }),
-/* 99 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.1.2.6 Number.MAX_SAFE_INTEGER
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Number', { MAX_SAFE_INTEGER: 0x1fffffffffffff });
-
-
-/***/ }),
-/* 100 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.1.2.10 Number.MIN_SAFE_INTEGER
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Number', { MIN_SAFE_INTEGER: -0x1fffffffffffff });
-
-
-/***/ }),
-/* 101 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- var $parseFloat = __webpack_require__(86);
- // 20.1.2.12 Number.parseFloat(string)
- $export($export.S + $export.F * (Number.parseFloat != $parseFloat), 'Number', { parseFloat: $parseFloat });
-
-
-/***/ }),
-/* 102 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- var $parseInt = __webpack_require__(82);
- // 20.1.2.13 Number.parseInt(string, radix)
- $export($export.S + $export.F * (Number.parseInt != $parseInt), 'Number', { parseInt: $parseInt });
-
-
-/***/ }),
-/* 103 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.3 Math.acosh(x)
- var $export = __webpack_require__(8);
- var log1p = __webpack_require__(104);
- var sqrt = Math.sqrt;
- var $acosh = Math.acosh;
-
- $export($export.S + $export.F * !($acosh
- // V8 bug: https://code.google.com/p/v8/issues/detail?id=3509
- && Math.floor($acosh(Number.MAX_VALUE)) == 710
- // Tor Browser bug: Math.acosh(Infinity) -> NaN
- && $acosh(Infinity) == Infinity
- ), 'Math', {
- acosh: function acosh(x) {
- return (x = +x) < 1 ? NaN : x > 94906265.62425156
- ? Math.log(x) + Math.LN2
- : log1p(x - 1 + sqrt(x - 1) * sqrt(x + 1));
- }
- });
-
-
-/***/ }),
-/* 104 */
-/***/ (function(module, exports) {
-
- // 20.2.2.20 Math.log1p(x)
- module.exports = Math.log1p || function log1p(x) {
- return (x = +x) > -1e-8 && x < 1e-8 ? x - x * x / 2 : Math.log(1 + x);
- };
-
-
-/***/ }),
-/* 105 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.5 Math.asinh(x)
- var $export = __webpack_require__(8);
- var $asinh = Math.asinh;
-
- function asinh(x) {
- return !isFinite(x = +x) || x == 0 ? x : x < 0 ? -asinh(-x) : Math.log(x + Math.sqrt(x * x + 1));
- }
-
- // Tor Browser bug: Math.asinh(0) -> -0
- $export($export.S + $export.F * !($asinh && 1 / $asinh(0) > 0), 'Math', { asinh: asinh });
-
-
-/***/ }),
-/* 106 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.7 Math.atanh(x)
- var $export = __webpack_require__(8);
- var $atanh = Math.atanh;
-
- // Tor Browser bug: Math.atanh(-0) -> 0
- $export($export.S + $export.F * !($atanh && 1 / $atanh(-0) < 0), 'Math', {
- atanh: function atanh(x) {
- return (x = +x) == 0 ? x : Math.log((1 + x) / (1 - x)) / 2;
- }
- });
-
-
-/***/ }),
-/* 107 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.9 Math.cbrt(x)
- var $export = __webpack_require__(8);
- var sign = __webpack_require__(108);
-
- $export($export.S, 'Math', {
- cbrt: function cbrt(x) {
- return sign(x = +x) * Math.pow(Math.abs(x), 1 / 3);
- }
- });
-
-
-/***/ }),
-/* 108 */
-/***/ (function(module, exports) {
-
- // 20.2.2.28 Math.sign(x)
- module.exports = Math.sign || function sign(x) {
- // eslint-disable-next-line no-self-compare
- return (x = +x) == 0 || x != x ? x : x < 0 ? -1 : 1;
- };
-
-
-/***/ }),
-/* 109 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.11 Math.clz32(x)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', {
- clz32: function clz32(x) {
- return (x >>>= 0) ? 31 - Math.floor(Math.log(x + 0.5) * Math.LOG2E) : 32;
- }
- });
-
-
-/***/ }),
-/* 110 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.12 Math.cosh(x)
- var $export = __webpack_require__(8);
- var exp = Math.exp;
-
- $export($export.S, 'Math', {
- cosh: function cosh(x) {
- return (exp(x = +x) + exp(-x)) / 2;
- }
- });
-
-
-/***/ }),
-/* 111 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.14 Math.expm1(x)
- var $export = __webpack_require__(8);
- var $expm1 = __webpack_require__(112);
-
- $export($export.S + $export.F * ($expm1 != Math.expm1), 'Math', { expm1: $expm1 });
-
-
-/***/ }),
-/* 112 */
-/***/ (function(module, exports) {
-
- // 20.2.2.14 Math.expm1(x)
- var $expm1 = Math.expm1;
- module.exports = (!$expm1
- // Old FF bug
- || $expm1(10) > 22025.465794806719 || $expm1(10) < 22025.4657948067165168
- // Tor Browser bug
- || $expm1(-2e-17) != -2e-17
- ) ? function expm1(x) {
- return (x = +x) == 0 ? x : x > -1e-6 && x < 1e-6 ? x + x * x / 2 : Math.exp(x) - 1;
- } : $expm1;
-
-
-/***/ }),
-/* 113 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.16 Math.fround(x)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', { fround: __webpack_require__(114) });
-
-
-/***/ }),
-/* 114 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.16 Math.fround(x)
- var sign = __webpack_require__(108);
- var pow = Math.pow;
- var EPSILON = pow(2, -52);
- var EPSILON32 = pow(2, -23);
- var MAX32 = pow(2, 127) * (2 - EPSILON32);
- var MIN32 = pow(2, -126);
-
- var roundTiesToEven = function (n) {
- return n + 1 / EPSILON - 1 / EPSILON;
- };
-
- module.exports = Math.fround || function fround(x) {
- var $abs = Math.abs(x);
- var $sign = sign(x);
- var a, result;
- if ($abs < MIN32) return $sign * roundTiesToEven($abs / MIN32 / EPSILON32) * MIN32 * EPSILON32;
- a = (1 + EPSILON32 / EPSILON) * $abs;
- result = a - (a - $abs);
- // eslint-disable-next-line no-self-compare
- if (result > MAX32 || result != result) return $sign * Infinity;
- return $sign * result;
- };
-
-
-/***/ }),
-/* 115 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.17 Math.hypot([value1[, value2[, … ]]])
- var $export = __webpack_require__(8);
- var abs = Math.abs;
-
- $export($export.S, 'Math', {
- hypot: function hypot(value1, value2) { // eslint-disable-line no-unused-vars
- var sum = 0;
- var i = 0;
- var aLen = arguments.length;
- var larg = 0;
- var arg, div;
- while (i < aLen) {
- arg = abs(arguments[i++]);
- if (larg < arg) {
- div = larg / arg;
- sum = sum * div * div + 1;
- larg = arg;
- } else if (arg > 0) {
- div = arg / larg;
- sum += div * div;
- } else sum += arg;
- }
- return larg === Infinity ? Infinity : larg * Math.sqrt(sum);
- }
- });
-
-
-/***/ }),
-/* 116 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.18 Math.imul(x, y)
- var $export = __webpack_require__(8);
- var $imul = Math.imul;
-
- // some WebKit versions fails with big numbers, some has wrong arity
- $export($export.S + $export.F * __webpack_require__(7)(function () {
- return $imul(0xffffffff, 5) != -5 || $imul.length != 2;
- }), 'Math', {
- imul: function imul(x, y) {
- var UINT16 = 0xffff;
- var xn = +x;
- var yn = +y;
- var xl = UINT16 & xn;
- var yl = UINT16 & yn;
- return 0 | xl * yl + ((UINT16 & xn >>> 16) * yl + xl * (UINT16 & yn >>> 16) << 16 >>> 0);
- }
- });
-
-
-/***/ }),
-/* 117 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.21 Math.log10(x)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', {
- log10: function log10(x) {
- return Math.log(x) * Math.LOG10E;
- }
- });
-
-
-/***/ }),
-/* 118 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.20 Math.log1p(x)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', { log1p: __webpack_require__(104) });
-
-
-/***/ }),
-/* 119 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.22 Math.log2(x)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', {
- log2: function log2(x) {
- return Math.log(x) / Math.LN2;
- }
- });
-
-
-/***/ }),
-/* 120 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.28 Math.sign(x)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', { sign: __webpack_require__(108) });
-
-
-/***/ }),
-/* 121 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.30 Math.sinh(x)
- var $export = __webpack_require__(8);
- var expm1 = __webpack_require__(112);
- var exp = Math.exp;
-
- // V8 near Chromium 38 has a problem with very small numbers
- $export($export.S + $export.F * __webpack_require__(7)(function () {
- return !Math.sinh(-2e-17) != -2e-17;
- }), 'Math', {
- sinh: function sinh(x) {
- return Math.abs(x = +x) < 1
- ? (expm1(x) - expm1(-x)) / 2
- : (exp(x - 1) - exp(-x - 1)) * (Math.E / 2);
- }
- });
-
-
-/***/ }),
-/* 122 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.33 Math.tanh(x)
- var $export = __webpack_require__(8);
- var expm1 = __webpack_require__(112);
- var exp = Math.exp;
-
- $export($export.S, 'Math', {
- tanh: function tanh(x) {
- var a = expm1(x = +x);
- var b = expm1(-x);
- return a == Infinity ? 1 : b == Infinity ? -1 : (a - b) / (exp(x) + exp(-x));
- }
- });
-
-
-/***/ }),
-/* 123 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.2.2.34 Math.trunc(x)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', {
- trunc: function trunc(it) {
- return (it > 0 ? Math.floor : Math.ceil)(it);
- }
- });
-
-
-/***/ }),
-/* 124 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- var toAbsoluteIndex = __webpack_require__(40);
- var fromCharCode = String.fromCharCode;
- var $fromCodePoint = String.fromCodePoint;
-
- // length should be 1, old FF problem
- $export($export.S + $export.F * (!!$fromCodePoint && $fromCodePoint.length != 1), 'String', {
- // 21.1.2.2 String.fromCodePoint(...codePoints)
- fromCodePoint: function fromCodePoint(x) { // eslint-disable-line no-unused-vars
- var res = [];
- var aLen = arguments.length;
- var i = 0;
- var code;
- while (aLen > i) {
- code = +arguments[i++];
- if (toAbsoluteIndex(code, 0x10ffff) !== code) throw RangeError(code + ' is not a valid code point');
- res.push(code < 0x10000
- ? fromCharCode(code)
- : fromCharCode(((code -= 0x10000) >> 10) + 0xd800, code % 0x400 + 0xdc00)
- );
- } return res.join('');
- }
- });
-
-
-/***/ }),
-/* 125 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- var toIObject = __webpack_require__(33);
- var toLength = __webpack_require__(38);
-
- $export($export.S, 'String', {
- // 21.1.2.4 String.raw(callSite, ...substitutions)
- raw: function raw(callSite) {
- var tpl = toIObject(callSite.raw);
- var len = toLength(tpl.length);
- var aLen = arguments.length;
- var res = [];
- var i = 0;
- while (len > i) {
- res.push(String(tpl[i++]));
- if (i < aLen) res.push(String(arguments[i]));
- } return res.join('');
- }
- });
-
-
-/***/ }),
-/* 126 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // 21.1.3.25 String.prototype.trim()
- __webpack_require__(83)('trim', function ($trim) {
- return function trim() {
- return $trim(this, 3);
- };
- });
-
-
-/***/ }),
-/* 127 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $at = __webpack_require__(128)(true);
-
- // 21.1.3.27 String.prototype[@@iterator]()
- __webpack_require__(129)(String, 'String', function (iterated) {
- this._t = String(iterated); // target
- this._i = 0; // next index
- // 21.1.5.2.1 %StringIteratorPrototype%.next()
- }, function () {
- var O = this._t;
- var index = this._i;
- var point;
- if (index >= O.length) return { value: undefined, done: true };
- point = $at(O, index);
- this._i += point.length;
- return { value: point, done: false };
- });
-
-
-/***/ }),
-/* 128 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var toInteger = __webpack_require__(39);
- var defined = __webpack_require__(36);
- // true -> String#at
- // false -> String#codePointAt
- module.exports = function (TO_STRING) {
- return function (that, pos) {
- var s = String(defined(that));
- var i = toInteger(pos);
- var l = s.length;
- var a, b;
- if (i < 0 || i >= l) return TO_STRING ? '' : undefined;
- a = s.charCodeAt(i);
- return a < 0xd800 || a > 0xdbff || i + 1 === l || (b = s.charCodeAt(i + 1)) < 0xdc00 || b > 0xdfff
- ? TO_STRING ? s.charAt(i) : a
- : TO_STRING ? s.slice(i, i + 2) : (a - 0xd800 << 10) + (b - 0xdc00) + 0x10000;
- };
- };
-
-
-/***/ }),
-/* 129 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var LIBRARY = __webpack_require__(22);
- var $export = __webpack_require__(8);
- var redefine = __webpack_require__(18);
- var hide = __webpack_require__(10);
- var Iterators = __webpack_require__(130);
- var $iterCreate = __webpack_require__(131);
- var setToStringTag = __webpack_require__(26);
- var getPrototypeOf = __webpack_require__(59);
- var ITERATOR = __webpack_require__(27)('iterator');
- var BUGGY = !([].keys && 'next' in [].keys()); // Safari has buggy iterators w/o `next`
- var FF_ITERATOR = '@@iterator';
- var KEYS = 'keys';
- var VALUES = 'values';
-
- var returnThis = function () { return this; };
-
- module.exports = function (Base, NAME, Constructor, next, DEFAULT, IS_SET, FORCED) {
- $iterCreate(Constructor, NAME, next);
- var getMethod = function (kind) {
- if (!BUGGY && kind in proto) return proto[kind];
- switch (kind) {
- case KEYS: return function keys() { return new Constructor(this, kind); };
- case VALUES: return function values() { return new Constructor(this, kind); };
- } return function entries() { return new Constructor(this, kind); };
- };
- var TAG = NAME + ' Iterator';
- var DEF_VALUES = DEFAULT == VALUES;
- var VALUES_BUG = false;
- var proto = Base.prototype;
- var $native = proto[ITERATOR] || proto[FF_ITERATOR] || DEFAULT && proto[DEFAULT];
- var $default = $native || getMethod(DEFAULT);
- var $entries = DEFAULT ? !DEF_VALUES ? $default : getMethod('entries') : undefined;
- var $anyNative = NAME == 'Array' ? proto.entries || $native : $native;
- var methods, key, IteratorPrototype;
- // Fix native
- if ($anyNative) {
- IteratorPrototype = getPrototypeOf($anyNative.call(new Base()));
- if (IteratorPrototype !== Object.prototype && IteratorPrototype.next) {
- // Set @@toStringTag to native iterators
- setToStringTag(IteratorPrototype, TAG, true);
- // fix for some old engines
- if (!LIBRARY && typeof IteratorPrototype[ITERATOR] != 'function') hide(IteratorPrototype, ITERATOR, returnThis);
- }
- }
- // fix Array#{values, @@iterator}.name in V8 / FF
- if (DEF_VALUES && $native && $native.name !== VALUES) {
- VALUES_BUG = true;
- $default = function values() { return $native.call(this); };
- }
- // Define iterator
- if ((!LIBRARY || FORCED) && (BUGGY || VALUES_BUG || !proto[ITERATOR])) {
- hide(proto, ITERATOR, $default);
- }
- // Plug for library
- Iterators[NAME] = $default;
- Iterators[TAG] = returnThis;
- if (DEFAULT) {
- methods = {
- values: DEF_VALUES ? $default : getMethod(VALUES),
- keys: IS_SET ? $default : getMethod(KEYS),
- entries: $entries
- };
- if (FORCED) for (key in methods) {
- if (!(key in proto)) redefine(proto, key, methods[key]);
- } else $export($export.P + $export.F * (BUGGY || VALUES_BUG), NAME, methods);
- }
- return methods;
- };
-
-
-/***/ }),
-/* 130 */
-/***/ (function(module, exports) {
-
- module.exports = {};
-
-
-/***/ }),
-/* 131 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var create = __webpack_require__(47);
- var descriptor = __webpack_require__(17);
- var setToStringTag = __webpack_require__(26);
- var IteratorPrototype = {};
-
- // 25.1.2.1.1 %IteratorPrototype%[@@iterator]()
- __webpack_require__(10)(IteratorPrototype, __webpack_require__(27)('iterator'), function () { return this; });
-
- module.exports = function (Constructor, NAME, next) {
- Constructor.prototype = create(IteratorPrototype, { next: descriptor(1, next) });
- setToStringTag(Constructor, NAME + ' Iterator');
- };
-
-
-/***/ }),
-/* 132 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $at = __webpack_require__(128)(false);
- $export($export.P, 'String', {
- // 21.1.3.3 String.prototype.codePointAt(pos)
- codePointAt: function codePointAt(pos) {
- return $at(this, pos);
- }
- });
-
-
-/***/ }),
-/* 133 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 21.1.3.6 String.prototype.endsWith(searchString [, endPosition])
- 'use strict';
- var $export = __webpack_require__(8);
- var toLength = __webpack_require__(38);
- var context = __webpack_require__(134);
- var ENDS_WITH = 'endsWith';
- var $endsWith = ''[ENDS_WITH];
-
- $export($export.P + $export.F * __webpack_require__(136)(ENDS_WITH), 'String', {
- endsWith: function endsWith(searchString /* , endPosition = @length */) {
- var that = context(this, searchString, ENDS_WITH);
- var endPosition = arguments.length > 1 ? arguments[1] : undefined;
- var len = toLength(that.length);
- var end = endPosition === undefined ? len : Math.min(toLength(endPosition), len);
- var search = String(searchString);
- return $endsWith
- ? $endsWith.call(that, search, end)
- : that.slice(end - search.length, end) === search;
- }
- });
-
-
-/***/ }),
-/* 134 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // helper for String#{startsWith, endsWith, includes}
- var isRegExp = __webpack_require__(135);
- var defined = __webpack_require__(36);
-
- module.exports = function (that, searchString, NAME) {
- if (isRegExp(searchString)) throw TypeError('String#' + NAME + " doesn't accept regex!");
- return String(defined(that));
- };
-
-
-/***/ }),
-/* 135 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 7.2.8 IsRegExp(argument)
- var isObject = __webpack_require__(13);
- var cof = __webpack_require__(35);
- var MATCH = __webpack_require__(27)('match');
- module.exports = function (it) {
- var isRegExp;
- return isObject(it) && ((isRegExp = it[MATCH]) !== undefined ? !!isRegExp : cof(it) == 'RegExp');
- };
-
-
-/***/ }),
-/* 136 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var MATCH = __webpack_require__(27)('match');
- module.exports = function (KEY) {
- var re = /./;
- try {
- '/./'[KEY](re);
- } catch (e) {
- try {
- re[MATCH] = false;
- return !'/./'[KEY](re);
- } catch (f) { /* empty */ }
- } return true;
- };
-
-
-/***/ }),
-/* 137 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 21.1.3.7 String.prototype.includes(searchString, position = 0)
- 'use strict';
- var $export = __webpack_require__(8);
- var context = __webpack_require__(134);
- var INCLUDES = 'includes';
-
- $export($export.P + $export.F * __webpack_require__(136)(INCLUDES), 'String', {
- includes: function includes(searchString /* , position = 0 */) {
- return !!~context(this, searchString, INCLUDES)
- .indexOf(searchString, arguments.length > 1 ? arguments[1] : undefined);
- }
- });
-
-
-/***/ }),
-/* 138 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
-
- $export($export.P, 'String', {
- // 21.1.3.13 String.prototype.repeat(count)
- repeat: __webpack_require__(91)
- });
-
-
-/***/ }),
-/* 139 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 21.1.3.18 String.prototype.startsWith(searchString [, position ])
- 'use strict';
- var $export = __webpack_require__(8);
- var toLength = __webpack_require__(38);
- var context = __webpack_require__(134);
- var STARTS_WITH = 'startsWith';
- var $startsWith = ''[STARTS_WITH];
-
- $export($export.P + $export.F * __webpack_require__(136)(STARTS_WITH), 'String', {
- startsWith: function startsWith(searchString /* , position = 0 */) {
- var that = context(this, searchString, STARTS_WITH);
- var index = toLength(Math.min(arguments.length > 1 ? arguments[1] : undefined, that.length));
- var search = String(searchString);
- return $startsWith
- ? $startsWith.call(that, search, index)
- : that.slice(index, index + search.length) === search;
- }
- });
-
-
-/***/ }),
-/* 140 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.2 String.prototype.anchor(name)
- __webpack_require__(141)('anchor', function (createHTML) {
- return function anchor(name) {
- return createHTML(this, 'a', 'name', name);
- };
- });
-
-
-/***/ }),
-/* 141 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- var fails = __webpack_require__(7);
- var defined = __webpack_require__(36);
- var quot = /"/g;
- // B.2.3.2.1 CreateHTML(string, tag, attribute, value)
- var createHTML = function (string, tag, attribute, value) {
- var S = String(defined(string));
- var p1 = '<' + tag;
- if (attribute !== '') p1 += ' ' + attribute + '="' + String(value).replace(quot, '"') + '"';
- return p1 + '>' + S + '' + tag + '>';
- };
- module.exports = function (NAME, exec) {
- var O = {};
- O[NAME] = exec(createHTML);
- $export($export.P + $export.F * fails(function () {
- var test = ''[NAME]('"');
- return test !== test.toLowerCase() || test.split('"').length > 3;
- }), 'String', O);
- };
-
-
-/***/ }),
-/* 142 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.3 String.prototype.big()
- __webpack_require__(141)('big', function (createHTML) {
- return function big() {
- return createHTML(this, 'big', '', '');
- };
- });
-
-
-/***/ }),
-/* 143 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.4 String.prototype.blink()
- __webpack_require__(141)('blink', function (createHTML) {
- return function blink() {
- return createHTML(this, 'blink', '', '');
- };
- });
-
-
-/***/ }),
-/* 144 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.5 String.prototype.bold()
- __webpack_require__(141)('bold', function (createHTML) {
- return function bold() {
- return createHTML(this, 'b', '', '');
- };
- });
-
-
-/***/ }),
-/* 145 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.6 String.prototype.fixed()
- __webpack_require__(141)('fixed', function (createHTML) {
- return function fixed() {
- return createHTML(this, 'tt', '', '');
- };
- });
-
-
-/***/ }),
-/* 146 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.7 String.prototype.fontcolor(color)
- __webpack_require__(141)('fontcolor', function (createHTML) {
- return function fontcolor(color) {
- return createHTML(this, 'font', 'color', color);
- };
- });
-
-
-/***/ }),
-/* 147 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.8 String.prototype.fontsize(size)
- __webpack_require__(141)('fontsize', function (createHTML) {
- return function fontsize(size) {
- return createHTML(this, 'font', 'size', size);
- };
- });
-
-
-/***/ }),
-/* 148 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.9 String.prototype.italics()
- __webpack_require__(141)('italics', function (createHTML) {
- return function italics() {
- return createHTML(this, 'i', '', '');
- };
- });
-
-
-/***/ }),
-/* 149 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.10 String.prototype.link(url)
- __webpack_require__(141)('link', function (createHTML) {
- return function link(url) {
- return createHTML(this, 'a', 'href', url);
- };
- });
-
-
-/***/ }),
-/* 150 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.11 String.prototype.small()
- __webpack_require__(141)('small', function (createHTML) {
- return function small() {
- return createHTML(this, 'small', '', '');
- };
- });
-
-
-/***/ }),
-/* 151 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.12 String.prototype.strike()
- __webpack_require__(141)('strike', function (createHTML) {
- return function strike() {
- return createHTML(this, 'strike', '', '');
- };
- });
-
-
-/***/ }),
-/* 152 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.13 String.prototype.sub()
- __webpack_require__(141)('sub', function (createHTML) {
- return function sub() {
- return createHTML(this, 'sub', '', '');
- };
- });
-
-
-/***/ }),
-/* 153 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // B.2.3.14 String.prototype.sup()
- __webpack_require__(141)('sup', function (createHTML) {
- return function sup() {
- return createHTML(this, 'sup', '', '');
- };
- });
-
-
-/***/ }),
-/* 154 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.3.3.1 / 15.9.4.4 Date.now()
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Date', { now: function () { return new Date().getTime(); } });
-
-
-/***/ }),
-/* 155 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var toObject = __webpack_require__(46);
- var toPrimitive = __webpack_require__(16);
-
- $export($export.P + $export.F * __webpack_require__(7)(function () {
- return new Date(NaN).toJSON() !== null
- || Date.prototype.toJSON.call({ toISOString: function () { return 1; } }) !== 1;
- }), 'Date', {
- // eslint-disable-next-line no-unused-vars
- toJSON: function toJSON(key) {
- var O = toObject(this);
- var pv = toPrimitive(O);
- return typeof pv == 'number' && !isFinite(pv) ? null : O.toISOString();
- }
- });
-
-
-/***/ }),
-/* 156 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 20.3.4.36 / 15.9.5.43 Date.prototype.toISOString()
- var $export = __webpack_require__(8);
- var toISOString = __webpack_require__(157);
-
- // PhantomJS / old WebKit has a broken implementations
- $export($export.P + $export.F * (Date.prototype.toISOString !== toISOString), 'Date', {
- toISOString: toISOString
- });
-
-
-/***/ }),
-/* 157 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // 20.3.4.36 / 15.9.5.43 Date.prototype.toISOString()
- var fails = __webpack_require__(7);
- var getTime = Date.prototype.getTime;
- var $toISOString = Date.prototype.toISOString;
-
- var lz = function (num) {
- return num > 9 ? num : '0' + num;
- };
-
- // PhantomJS / old WebKit has a broken implementations
- module.exports = (fails(function () {
- return $toISOString.call(new Date(-5e13 - 1)) != '0385-07-25T07:06:39.999Z';
- }) || !fails(function () {
- $toISOString.call(new Date(NaN));
- })) ? function toISOString() {
- if (!isFinite(getTime.call(this))) throw RangeError('Invalid time value');
- var d = this;
- var y = d.getUTCFullYear();
- var m = d.getUTCMilliseconds();
- var s = y < 0 ? '-' : y > 9999 ? '+' : '';
- return s + ('00000' + Math.abs(y)).slice(s ? -6 : -4) +
- '-' + lz(d.getUTCMonth() + 1) + '-' + lz(d.getUTCDate()) +
- 'T' + lz(d.getUTCHours()) + ':' + lz(d.getUTCMinutes()) +
- ':' + lz(d.getUTCSeconds()) + '.' + (m > 99 ? m : '0' + lz(m)) + 'Z';
- } : $toISOString;
-
-
-/***/ }),
-/* 158 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var DateProto = Date.prototype;
- var INVALID_DATE = 'Invalid Date';
- var TO_STRING = 'toString';
- var $toString = DateProto[TO_STRING];
- var getTime = DateProto.getTime;
- if (new Date(NaN) + '' != INVALID_DATE) {
- __webpack_require__(18)(DateProto, TO_STRING, function toString() {
- var value = getTime.call(this);
- // eslint-disable-next-line no-self-compare
- return value === value ? $toString.call(this) : INVALID_DATE;
- });
- }
-
-
-/***/ }),
-/* 159 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var TO_PRIMITIVE = __webpack_require__(27)('toPrimitive');
- var proto = Date.prototype;
-
- if (!(TO_PRIMITIVE in proto)) __webpack_require__(10)(proto, TO_PRIMITIVE, __webpack_require__(160));
-
-
-/***/ }),
-/* 160 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var anObject = __webpack_require__(12);
- var toPrimitive = __webpack_require__(16);
- var NUMBER = 'number';
-
- module.exports = function (hint) {
- if (hint !== 'string' && hint !== NUMBER && hint !== 'default') throw TypeError('Incorrect hint');
- return toPrimitive(anObject(this), hint != NUMBER);
- };
-
-
-/***/ }),
-/* 161 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 22.1.2.2 / 15.4.3.2 Array.isArray(arg)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Array', { isArray: __webpack_require__(45) });
-
-
-/***/ }),
-/* 162 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var ctx = __webpack_require__(23);
- var $export = __webpack_require__(8);
- var toObject = __webpack_require__(46);
- var call = __webpack_require__(163);
- var isArrayIter = __webpack_require__(164);
- var toLength = __webpack_require__(38);
- var createProperty = __webpack_require__(165);
- var getIterFn = __webpack_require__(166);
-
- $export($export.S + $export.F * !__webpack_require__(167)(function (iter) { Array.from(iter); }), 'Array', {
- // 22.1.2.1 Array.from(arrayLike, mapfn = undefined, thisArg = undefined)
- from: function from(arrayLike /* , mapfn = undefined, thisArg = undefined */) {
- var O = toObject(arrayLike);
- var C = typeof this == 'function' ? this : Array;
- var aLen = arguments.length;
- var mapfn = aLen > 1 ? arguments[1] : undefined;
- var mapping = mapfn !== undefined;
- var index = 0;
- var iterFn = getIterFn(O);
- var length, result, step, iterator;
- if (mapping) mapfn = ctx(mapfn, aLen > 2 ? arguments[2] : undefined, 2);
- // if object isn't iterable or it's array with default iterator - use simple case
- if (iterFn != undefined && !(C == Array && isArrayIter(iterFn))) {
- for (iterator = iterFn.call(O), result = new C(); !(step = iterator.next()).done; index++) {
- createProperty(result, index, mapping ? call(iterator, mapfn, [step.value, index], true) : step.value);
- }
- } else {
- length = toLength(O.length);
- for (result = new C(length); length > index; index++) {
- createProperty(result, index, mapping ? mapfn(O[index], index) : O[index]);
- }
- }
- result.length = index;
- return result;
- }
- });
-
-
-/***/ }),
-/* 163 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // call something on iterator step with safe closing on error
- var anObject = __webpack_require__(12);
- module.exports = function (iterator, fn, value, entries) {
- try {
- return entries ? fn(anObject(value)[0], value[1]) : fn(value);
- // 7.4.6 IteratorClose(iterator, completion)
- } catch (e) {
- var ret = iterator['return'];
- if (ret !== undefined) anObject(ret.call(iterator));
- throw e;
- }
- };
-
-
-/***/ }),
-/* 164 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // check on default Array iterator
- var Iterators = __webpack_require__(130);
- var ITERATOR = __webpack_require__(27)('iterator');
- var ArrayProto = Array.prototype;
-
- module.exports = function (it) {
- return it !== undefined && (Iterators.Array === it || ArrayProto[ITERATOR] === it);
- };
-
-
-/***/ }),
-/* 165 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $defineProperty = __webpack_require__(11);
- var createDesc = __webpack_require__(17);
-
- module.exports = function (object, index, value) {
- if (index in object) $defineProperty.f(object, index, createDesc(0, value));
- else object[index] = value;
- };
-
-
-/***/ }),
-/* 166 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var classof = __webpack_require__(75);
- var ITERATOR = __webpack_require__(27)('iterator');
- var Iterators = __webpack_require__(130);
- module.exports = __webpack_require__(9).getIteratorMethod = function (it) {
- if (it != undefined) return it[ITERATOR]
- || it['@@iterator']
- || Iterators[classof(it)];
- };
-
-
-/***/ }),
-/* 167 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var ITERATOR = __webpack_require__(27)('iterator');
- var SAFE_CLOSING = false;
-
- try {
- var riter = [7][ITERATOR]();
- riter['return'] = function () { SAFE_CLOSING = true; };
- // eslint-disable-next-line no-throw-literal
- Array.from(riter, function () { throw 2; });
- } catch (e) { /* empty */ }
-
- module.exports = function (exec, skipClosing) {
- if (!skipClosing && !SAFE_CLOSING) return false;
- var safe = false;
- try {
- var arr = [7];
- var iter = arr[ITERATOR]();
- iter.next = function () { return { done: safe = true }; };
- arr[ITERATOR] = function () { return iter; };
- exec(arr);
- } catch (e) { /* empty */ }
- return safe;
- };
-
-
-/***/ }),
-/* 168 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var createProperty = __webpack_require__(165);
-
- // WebKit Array.of isn't generic
- $export($export.S + $export.F * __webpack_require__(7)(function () {
- function F() { /* empty */ }
- return !(Array.of.call(F) instanceof F);
- }), 'Array', {
- // 22.1.2.3 Array.of( ...items)
- of: function of(/* ...args */) {
- var index = 0;
- var aLen = arguments.length;
- var result = new (typeof this == 'function' ? this : Array)(aLen);
- while (aLen > index) createProperty(result, index, arguments[index++]);
- result.length = aLen;
- return result;
- }
- });
-
-
-/***/ }),
-/* 169 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // 22.1.3.13 Array.prototype.join(separator)
- var $export = __webpack_require__(8);
- var toIObject = __webpack_require__(33);
- var arrayJoin = [].join;
-
- // fallback for not array-like strings
- $export($export.P + $export.F * (__webpack_require__(34) != Object || !__webpack_require__(170)(arrayJoin)), 'Array', {
- join: function join(separator) {
- return arrayJoin.call(toIObject(this), separator === undefined ? ',' : separator);
- }
- });
-
-
-/***/ }),
-/* 170 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var fails = __webpack_require__(7);
-
- module.exports = function (method, arg) {
- return !!method && fails(function () {
- // eslint-disable-next-line no-useless-call
- arg ? method.call(null, function () { /* empty */ }, 1) : method.call(null);
- });
- };
-
-
-/***/ }),
-/* 171 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var html = __webpack_require__(49);
- var cof = __webpack_require__(35);
- var toAbsoluteIndex = __webpack_require__(40);
- var toLength = __webpack_require__(38);
- var arraySlice = [].slice;
-
- // fallback for not array-like ES3 strings and DOM objects
- $export($export.P + $export.F * __webpack_require__(7)(function () {
- if (html) arraySlice.call(html);
- }), 'Array', {
- slice: function slice(begin, end) {
- var len = toLength(this.length);
- var klass = cof(this);
- end = end === undefined ? len : end;
- if (klass == 'Array') return arraySlice.call(this, begin, end);
- var start = toAbsoluteIndex(begin, len);
- var upTo = toAbsoluteIndex(end, len);
- var size = toLength(upTo - start);
- var cloned = new Array(size);
- var i = 0;
- for (; i < size; i++) cloned[i] = klass == 'String'
- ? this.charAt(start + i)
- : this[start + i];
- return cloned;
- }
- });
-
-
-/***/ }),
-/* 172 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var aFunction = __webpack_require__(24);
- var toObject = __webpack_require__(46);
- var fails = __webpack_require__(7);
- var $sort = [].sort;
- var test = [1, 2, 3];
-
- $export($export.P + $export.F * (fails(function () {
- // IE8-
- test.sort(undefined);
- }) || !fails(function () {
- // V8 bug
- test.sort(null);
- // Old WebKit
- }) || !__webpack_require__(170)($sort)), 'Array', {
- // 22.1.3.25 Array.prototype.sort(comparefn)
- sort: function sort(comparefn) {
- return comparefn === undefined
- ? $sort.call(toObject(this))
- : $sort.call(toObject(this), aFunction(comparefn));
- }
- });
-
-
-/***/ }),
-/* 173 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $forEach = __webpack_require__(174)(0);
- var STRICT = __webpack_require__(170)([].forEach, true);
-
- $export($export.P + $export.F * !STRICT, 'Array', {
- // 22.1.3.10 / 15.4.4.18 Array.prototype.forEach(callbackfn [, thisArg])
- forEach: function forEach(callbackfn /* , thisArg */) {
- return $forEach(this, callbackfn, arguments[1]);
- }
- });
-
-
-/***/ }),
-/* 174 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 0 -> Array#forEach
- // 1 -> Array#map
- // 2 -> Array#filter
- // 3 -> Array#some
- // 4 -> Array#every
- // 5 -> Array#find
- // 6 -> Array#findIndex
- var ctx = __webpack_require__(23);
- var IObject = __webpack_require__(34);
- var toObject = __webpack_require__(46);
- var toLength = __webpack_require__(38);
- var asc = __webpack_require__(175);
- module.exports = function (TYPE, $create) {
- var IS_MAP = TYPE == 1;
- var IS_FILTER = TYPE == 2;
- var IS_SOME = TYPE == 3;
- var IS_EVERY = TYPE == 4;
- var IS_FIND_INDEX = TYPE == 6;
- var NO_HOLES = TYPE == 5 || IS_FIND_INDEX;
- var create = $create || asc;
- return function ($this, callbackfn, that) {
- var O = toObject($this);
- var self = IObject(O);
- var f = ctx(callbackfn, that, 3);
- var length = toLength(self.length);
- var index = 0;
- var result = IS_MAP ? create($this, length) : IS_FILTER ? create($this, 0) : undefined;
- var val, res;
- for (;length > index; index++) if (NO_HOLES || index in self) {
- val = self[index];
- res = f(val, index, O);
- if (TYPE) {
- if (IS_MAP) result[index] = res; // map
- else if (res) switch (TYPE) {
- case 3: return true; // some
- case 5: return val; // find
- case 6: return index; // findIndex
- case 2: result.push(val); // filter
- } else if (IS_EVERY) return false; // every
- }
- }
- return IS_FIND_INDEX ? -1 : IS_SOME || IS_EVERY ? IS_EVERY : result;
- };
- };
-
-
-/***/ }),
-/* 175 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 9.4.2.3 ArraySpeciesCreate(originalArray, length)
- var speciesConstructor = __webpack_require__(176);
-
- module.exports = function (original, length) {
- return new (speciesConstructor(original))(length);
- };
-
-
-/***/ }),
-/* 176 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var isObject = __webpack_require__(13);
- var isArray = __webpack_require__(45);
- var SPECIES = __webpack_require__(27)('species');
-
- module.exports = function (original) {
- var C;
- if (isArray(original)) {
- C = original.constructor;
- // cross-realm fallback
- if (typeof C == 'function' && (C === Array || isArray(C.prototype))) C = undefined;
- if (isObject(C)) {
- C = C[SPECIES];
- if (C === null) C = undefined;
- }
- } return C === undefined ? Array : C;
- };
-
-
-/***/ }),
-/* 177 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $map = __webpack_require__(174)(1);
-
- $export($export.P + $export.F * !__webpack_require__(170)([].map, true), 'Array', {
- // 22.1.3.15 / 15.4.4.19 Array.prototype.map(callbackfn [, thisArg])
- map: function map(callbackfn /* , thisArg */) {
- return $map(this, callbackfn, arguments[1]);
- }
- });
-
-
-/***/ }),
-/* 178 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $filter = __webpack_require__(174)(2);
-
- $export($export.P + $export.F * !__webpack_require__(170)([].filter, true), 'Array', {
- // 22.1.3.7 / 15.4.4.20 Array.prototype.filter(callbackfn [, thisArg])
- filter: function filter(callbackfn /* , thisArg */) {
- return $filter(this, callbackfn, arguments[1]);
- }
- });
-
-
-/***/ }),
-/* 179 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $some = __webpack_require__(174)(3);
-
- $export($export.P + $export.F * !__webpack_require__(170)([].some, true), 'Array', {
- // 22.1.3.23 / 15.4.4.17 Array.prototype.some(callbackfn [, thisArg])
- some: function some(callbackfn /* , thisArg */) {
- return $some(this, callbackfn, arguments[1]);
- }
- });
-
-
-/***/ }),
-/* 180 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $every = __webpack_require__(174)(4);
-
- $export($export.P + $export.F * !__webpack_require__(170)([].every, true), 'Array', {
- // 22.1.3.5 / 15.4.4.16 Array.prototype.every(callbackfn [, thisArg])
- every: function every(callbackfn /* , thisArg */) {
- return $every(this, callbackfn, arguments[1]);
- }
- });
-
-
-/***/ }),
-/* 181 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $reduce = __webpack_require__(182);
-
- $export($export.P + $export.F * !__webpack_require__(170)([].reduce, true), 'Array', {
- // 22.1.3.18 / 15.4.4.21 Array.prototype.reduce(callbackfn [, initialValue])
- reduce: function reduce(callbackfn /* , initialValue */) {
- return $reduce(this, callbackfn, arguments.length, arguments[1], false);
- }
- });
-
-
-/***/ }),
-/* 182 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var aFunction = __webpack_require__(24);
- var toObject = __webpack_require__(46);
- var IObject = __webpack_require__(34);
- var toLength = __webpack_require__(38);
-
- module.exports = function (that, callbackfn, aLen, memo, isRight) {
- aFunction(callbackfn);
- var O = toObject(that);
- var self = IObject(O);
- var length = toLength(O.length);
- var index = isRight ? length - 1 : 0;
- var i = isRight ? -1 : 1;
- if (aLen < 2) for (;;) {
- if (index in self) {
- memo = self[index];
- index += i;
- break;
- }
- index += i;
- if (isRight ? index < 0 : length <= index) {
- throw TypeError('Reduce of empty array with no initial value');
- }
- }
- for (;isRight ? index >= 0 : length > index; index += i) if (index in self) {
- memo = callbackfn(memo, self[index], index, O);
- }
- return memo;
- };
-
-
-/***/ }),
-/* 183 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $reduce = __webpack_require__(182);
-
- $export($export.P + $export.F * !__webpack_require__(170)([].reduceRight, true), 'Array', {
- // 22.1.3.19 / 15.4.4.22 Array.prototype.reduceRight(callbackfn [, initialValue])
- reduceRight: function reduceRight(callbackfn /* , initialValue */) {
- return $reduce(this, callbackfn, arguments.length, arguments[1], true);
- }
- });
-
-
-/***/ }),
-/* 184 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $indexOf = __webpack_require__(37)(false);
- var $native = [].indexOf;
- var NEGATIVE_ZERO = !!$native && 1 / [1].indexOf(1, -0) < 0;
-
- $export($export.P + $export.F * (NEGATIVE_ZERO || !__webpack_require__(170)($native)), 'Array', {
- // 22.1.3.11 / 15.4.4.14 Array.prototype.indexOf(searchElement [, fromIndex])
- indexOf: function indexOf(searchElement /* , fromIndex = 0 */) {
- return NEGATIVE_ZERO
- // convert -0 to +0
- ? $native.apply(this, arguments) || 0
- : $indexOf(this, searchElement, arguments[1]);
- }
- });
-
-
-/***/ }),
-/* 185 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var toIObject = __webpack_require__(33);
- var toInteger = __webpack_require__(39);
- var toLength = __webpack_require__(38);
- var $native = [].lastIndexOf;
- var NEGATIVE_ZERO = !!$native && 1 / [1].lastIndexOf(1, -0) < 0;
-
- $export($export.P + $export.F * (NEGATIVE_ZERO || !__webpack_require__(170)($native)), 'Array', {
- // 22.1.3.14 / 15.4.4.15 Array.prototype.lastIndexOf(searchElement [, fromIndex])
- lastIndexOf: function lastIndexOf(searchElement /* , fromIndex = @[*-1] */) {
- // convert -0 to +0
- if (NEGATIVE_ZERO) return $native.apply(this, arguments) || 0;
- var O = toIObject(this);
- var length = toLength(O.length);
- var index = length - 1;
- if (arguments.length > 1) index = Math.min(index, toInteger(arguments[1]));
- if (index < 0) index = length + index;
- for (;index >= 0; index--) if (index in O) if (O[index] === searchElement) return index || 0;
- return -1;
- }
- });
-
-
-/***/ }),
-/* 186 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
- var $export = __webpack_require__(8);
-
- $export($export.P, 'Array', { copyWithin: __webpack_require__(187) });
-
- __webpack_require__(188)('copyWithin');
-
-
-/***/ }),
-/* 187 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
- 'use strict';
- var toObject = __webpack_require__(46);
- var toAbsoluteIndex = __webpack_require__(40);
- var toLength = __webpack_require__(38);
-
- module.exports = [].copyWithin || function copyWithin(target /* = 0 */, start /* = 0, end = @length */) {
- var O = toObject(this);
- var len = toLength(O.length);
- var to = toAbsoluteIndex(target, len);
- var from = toAbsoluteIndex(start, len);
- var end = arguments.length > 2 ? arguments[2] : undefined;
- var count = Math.min((end === undefined ? len : toAbsoluteIndex(end, len)) - from, len - to);
- var inc = 1;
- if (from < to && to < from + count) {
- inc = -1;
- from += count - 1;
- to += count - 1;
- }
- while (count-- > 0) {
- if (from in O) O[to] = O[from];
- else delete O[to];
- to += inc;
- from += inc;
- } return O;
- };
-
-
-/***/ }),
-/* 188 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 22.1.3.31 Array.prototype[@@unscopables]
- var UNSCOPABLES = __webpack_require__(27)('unscopables');
- var ArrayProto = Array.prototype;
- if (ArrayProto[UNSCOPABLES] == undefined) __webpack_require__(10)(ArrayProto, UNSCOPABLES, {});
- module.exports = function (key) {
- ArrayProto[UNSCOPABLES][key] = true;
- };
-
-
-/***/ }),
-/* 189 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
- var $export = __webpack_require__(8);
-
- $export($export.P, 'Array', { fill: __webpack_require__(190) });
-
- __webpack_require__(188)('fill');
-
-
-/***/ }),
-/* 190 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
- 'use strict';
- var toObject = __webpack_require__(46);
- var toAbsoluteIndex = __webpack_require__(40);
- var toLength = __webpack_require__(38);
- module.exports = function fill(value /* , start = 0, end = @length */) {
- var O = toObject(this);
- var length = toLength(O.length);
- var aLen = arguments.length;
- var index = toAbsoluteIndex(aLen > 1 ? arguments[1] : undefined, length);
- var end = aLen > 2 ? arguments[2] : undefined;
- var endPos = end === undefined ? length : toAbsoluteIndex(end, length);
- while (endPos > index) O[index++] = value;
- return O;
- };
-
-
-/***/ }),
-/* 191 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // 22.1.3.8 Array.prototype.find(predicate, thisArg = undefined)
- var $export = __webpack_require__(8);
- var $find = __webpack_require__(174)(5);
- var KEY = 'find';
- var forced = true;
- // Shouldn't skip holes
- if (KEY in []) Array(1)[KEY](function () { forced = false; });
- $export($export.P + $export.F * forced, 'Array', {
- find: function find(callbackfn /* , that = undefined */) {
- return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
- }
- });
- __webpack_require__(188)(KEY);
-
-
-/***/ }),
-/* 192 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // 22.1.3.9 Array.prototype.findIndex(predicate, thisArg = undefined)
- var $export = __webpack_require__(8);
- var $find = __webpack_require__(174)(6);
- var KEY = 'findIndex';
- var forced = true;
- // Shouldn't skip holes
- if (KEY in []) Array(1)[KEY](function () { forced = false; });
- $export($export.P + $export.F * forced, 'Array', {
- findIndex: function findIndex(callbackfn /* , that = undefined */) {
- return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
- }
- });
- __webpack_require__(188)(KEY);
-
-
-/***/ }),
-/* 193 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(194)('Array');
-
-
-/***/ }),
-/* 194 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var global = __webpack_require__(4);
- var dP = __webpack_require__(11);
- var DESCRIPTORS = __webpack_require__(6);
- var SPECIES = __webpack_require__(27)('species');
-
- module.exports = function (KEY) {
- var C = global[KEY];
- if (DESCRIPTORS && C && !C[SPECIES]) dP.f(C, SPECIES, {
- configurable: true,
- get: function () { return this; }
- });
- };
-
-
-/***/ }),
-/* 195 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var addToUnscopables = __webpack_require__(188);
- var step = __webpack_require__(196);
- var Iterators = __webpack_require__(130);
- var toIObject = __webpack_require__(33);
-
- // 22.1.3.4 Array.prototype.entries()
- // 22.1.3.13 Array.prototype.keys()
- // 22.1.3.29 Array.prototype.values()
- // 22.1.3.30 Array.prototype[@@iterator]()
- module.exports = __webpack_require__(129)(Array, 'Array', function (iterated, kind) {
- this._t = toIObject(iterated); // target
- this._i = 0; // next index
- this._k = kind; // kind
- // 22.1.5.2.1 %ArrayIteratorPrototype%.next()
- }, function () {
- var O = this._t;
- var kind = this._k;
- var index = this._i++;
- if (!O || index >= O.length) {
- this._t = undefined;
- return step(1);
- }
- if (kind == 'keys') return step(0, index);
- if (kind == 'values') return step(0, O[index]);
- return step(0, [index, O[index]]);
- }, 'values');
-
- // argumentsList[@@iterator] is %ArrayProto_values% (9.4.4.6, 9.4.4.7)
- Iterators.Arguments = Iterators.Array;
-
- addToUnscopables('keys');
- addToUnscopables('values');
- addToUnscopables('entries');
-
-
-/***/ }),
-/* 196 */
-/***/ (function(module, exports) {
-
- module.exports = function (done, value) {
- return { value: value, done: !!done };
- };
-
-
-/***/ }),
-/* 197 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var global = __webpack_require__(4);
- var inheritIfRequired = __webpack_require__(88);
- var dP = __webpack_require__(11).f;
- var gOPN = __webpack_require__(51).f;
- var isRegExp = __webpack_require__(135);
- var $flags = __webpack_require__(198);
- var $RegExp = global.RegExp;
- var Base = $RegExp;
- var proto = $RegExp.prototype;
- var re1 = /a/g;
- var re2 = /a/g;
- // "new" creates a new object, old webkit buggy here
- var CORRECT_NEW = new $RegExp(re1) !== re1;
-
- if (__webpack_require__(6) && (!CORRECT_NEW || __webpack_require__(7)(function () {
- re2[__webpack_require__(27)('match')] = false;
- // RegExp constructor can alter flags and IsRegExp works correct with @@match
- return $RegExp(re1) != re1 || $RegExp(re2) == re2 || $RegExp(re1, 'i') != '/a/i';
- }))) {
- $RegExp = function RegExp(p, f) {
- var tiRE = this instanceof $RegExp;
- var piRE = isRegExp(p);
- var fiU = f === undefined;
- return !tiRE && piRE && p.constructor === $RegExp && fiU ? p
- : inheritIfRequired(CORRECT_NEW
- ? new Base(piRE && !fiU ? p.source : p, f)
- : Base((piRE = p instanceof $RegExp) ? p.source : p, piRE && fiU ? $flags.call(p) : f)
- , tiRE ? this : proto, $RegExp);
- };
- var proxy = function (key) {
- key in $RegExp || dP($RegExp, key, {
- configurable: true,
- get: function () { return Base[key]; },
- set: function (it) { Base[key] = it; }
- });
- };
- for (var keys = gOPN(Base), i = 0; keys.length > i;) proxy(keys[i++]);
- proto.constructor = $RegExp;
- $RegExp.prototype = proto;
- __webpack_require__(18)(global, 'RegExp', $RegExp);
- }
-
- __webpack_require__(194)('RegExp');
-
-
-/***/ }),
-/* 198 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // 21.2.5.3 get RegExp.prototype.flags
- var anObject = __webpack_require__(12);
- module.exports = function () {
- var that = anObject(this);
- var result = '';
- if (that.global) result += 'g';
- if (that.ignoreCase) result += 'i';
- if (that.multiline) result += 'm';
- if (that.unicode) result += 'u';
- if (that.sticky) result += 'y';
- return result;
- };
-
-
-/***/ }),
-/* 199 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var regexpExec = __webpack_require__(200);
- __webpack_require__(8)({
- target: 'RegExp',
- proto: true,
- forced: regexpExec !== /./.exec
- }, {
- exec: regexpExec
- });
-
-
-/***/ }),
-/* 200 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
-
- var regexpFlags = __webpack_require__(198);
-
- var nativeExec = RegExp.prototype.exec;
- // This always refers to the native implementation, because the
- // String#replace polyfill uses ./fix-regexp-well-known-symbol-logic.js,
- // which loads this file before patching the method.
- var nativeReplace = String.prototype.replace;
-
- var patchedExec = nativeExec;
-
- var LAST_INDEX = 'lastIndex';
-
- var UPDATES_LAST_INDEX_WRONG = (function () {
- var re1 = /a/,
- re2 = /b*/g;
- nativeExec.call(re1, 'a');
- nativeExec.call(re2, 'a');
- return re1[LAST_INDEX] !== 0 || re2[LAST_INDEX] !== 0;
- })();
-
- // nonparticipating capturing group, copied from es5-shim's String#split patch.
- var NPCG_INCLUDED = /()??/.exec('')[1] !== undefined;
-
- var PATCH = UPDATES_LAST_INDEX_WRONG || NPCG_INCLUDED;
-
- if (PATCH) {
- patchedExec = function exec(str) {
- var re = this;
- var lastIndex, reCopy, match, i;
-
- if (NPCG_INCLUDED) {
- reCopy = new RegExp('^' + re.source + '$(?!\\s)', regexpFlags.call(re));
- }
- if (UPDATES_LAST_INDEX_WRONG) lastIndex = re[LAST_INDEX];
-
- match = nativeExec.call(re, str);
-
- if (UPDATES_LAST_INDEX_WRONG && match) {
- re[LAST_INDEX] = re.global ? match.index + match[0].length : lastIndex;
- }
- if (NPCG_INCLUDED && match && match.length > 1) {
- // Fix browsers whose `exec` methods don't consistently return `undefined`
- // for NPCG, like IE8. NOTE: This doesn' work for /(.?)?/
- // eslint-disable-next-line no-loop-func
- nativeReplace.call(match[0], reCopy, function () {
- for (i = 1; i < arguments.length - 2; i++) {
- if (arguments[i] === undefined) match[i] = undefined;
- }
- });
- }
-
- return match;
- };
- }
-
- module.exports = patchedExec;
-
-
-/***/ }),
-/* 201 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- __webpack_require__(202);
- var anObject = __webpack_require__(12);
- var $flags = __webpack_require__(198);
- var DESCRIPTORS = __webpack_require__(6);
- var TO_STRING = 'toString';
- var $toString = /./[TO_STRING];
-
- var define = function (fn) {
- __webpack_require__(18)(RegExp.prototype, TO_STRING, fn, true);
- };
-
- // 21.2.5.14 RegExp.prototype.toString()
- if (__webpack_require__(7)(function () { return $toString.call({ source: 'a', flags: 'b' }) != '/a/b'; })) {
- define(function toString() {
- var R = anObject(this);
- return '/'.concat(R.source, '/',
- 'flags' in R ? R.flags : !DESCRIPTORS && R instanceof RegExp ? $flags.call(R) : undefined);
- });
- // FF44- RegExp#toString has a wrong name
- } else if ($toString.name != TO_STRING) {
- define(function toString() {
- return $toString.call(this);
- });
- }
-
-
-/***/ }),
-/* 202 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 21.2.5.3 get RegExp.prototype.flags()
- if (__webpack_require__(6) && /./g.flags != 'g') __webpack_require__(11).f(RegExp.prototype, 'flags', {
- configurable: true,
- get: __webpack_require__(198)
- });
-
-
-/***/ }),
-/* 203 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
-
- var anObject = __webpack_require__(12);
- var toLength = __webpack_require__(38);
- var advanceStringIndex = __webpack_require__(204);
- var regExpExec = __webpack_require__(205);
-
- // @@match logic
- __webpack_require__(206)('match', 1, function (defined, MATCH, $match, maybeCallNative) {
- return [
- // `String.prototype.match` method
- // https://tc39.github.io/ecma262/#sec-string.prototype.match
- function match(regexp) {
- var O = defined(this);
- var fn = regexp == undefined ? undefined : regexp[MATCH];
- return fn !== undefined ? fn.call(regexp, O) : new RegExp(regexp)[MATCH](String(O));
- },
- // `RegExp.prototype[@@match]` method
- // https://tc39.github.io/ecma262/#sec-regexp.prototype-@@match
- function (regexp) {
- var res = maybeCallNative($match, regexp, this);
- if (res.done) return res.value;
- var rx = anObject(regexp);
- var S = String(this);
- if (!rx.global) return regExpExec(rx, S);
- var fullUnicode = rx.unicode;
- rx.lastIndex = 0;
- var A = [];
- var n = 0;
- var result;
- while ((result = regExpExec(rx, S)) !== null) {
- var matchStr = String(result[0]);
- A[n] = matchStr;
- if (matchStr === '') rx.lastIndex = advanceStringIndex(S, toLength(rx.lastIndex), fullUnicode);
- n++;
- }
- return n === 0 ? null : A;
- }
- ];
- });
-
-
-/***/ }),
-/* 204 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var at = __webpack_require__(128)(true);
-
- // `AdvanceStringIndex` abstract operation
- // https://tc39.github.io/ecma262/#sec-advancestringindex
- module.exports = function (S, index, unicode) {
- return index + (unicode ? at(S, index).length : 1);
- };
-
-
-/***/ }),
-/* 205 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
-
- var classof = __webpack_require__(75);
- var builtinExec = RegExp.prototype.exec;
-
- // `RegExpExec` abstract operation
- // https://tc39.github.io/ecma262/#sec-regexpexec
- module.exports = function (R, S) {
- var exec = R.exec;
- if (typeof exec === 'function') {
- var result = exec.call(R, S);
- if (typeof result !== 'object') {
- throw new TypeError('RegExp exec method returned something other than an Object or null');
- }
- return result;
- }
- if (classof(R) !== 'RegExp') {
- throw new TypeError('RegExp#exec called on incompatible receiver');
- }
- return builtinExec.call(R, S);
- };
-
-
-/***/ }),
-/* 206 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- __webpack_require__(199);
- var redefine = __webpack_require__(18);
- var hide = __webpack_require__(10);
- var fails = __webpack_require__(7);
- var defined = __webpack_require__(36);
- var wks = __webpack_require__(27);
- var regexpExec = __webpack_require__(200);
-
- var SPECIES = wks('species');
-
- var REPLACE_SUPPORTS_NAMED_GROUPS = !fails(function () {
- // #replace needs built-in support for named groups.
- // #match works fine because it just return the exec results, even if it has
- // a "grops" property.
- var re = /./;
- re.exec = function () {
- var result = [];
- result.groups = { a: '7' };
- return result;
- };
- return ''.replace(re, '$') !== '7';
- });
-
- var SPLIT_WORKS_WITH_OVERWRITTEN_EXEC = (function () {
- // Chrome 51 has a buggy "split" implementation when RegExp#exec !== nativeExec
- var re = /(?:)/;
- var originalExec = re.exec;
- re.exec = function () { return originalExec.apply(this, arguments); };
- var result = 'ab'.split(re);
- return result.length === 2 && result[0] === 'a' && result[1] === 'b';
- })();
-
- module.exports = function (KEY, length, exec) {
- var SYMBOL = wks(KEY);
-
- var DELEGATES_TO_SYMBOL = !fails(function () {
- // String methods call symbol-named RegEp methods
- var O = {};
- O[SYMBOL] = function () { return 7; };
- return ''[KEY](O) != 7;
- });
-
- var DELEGATES_TO_EXEC = DELEGATES_TO_SYMBOL ? !fails(function () {
- // Symbol-named RegExp methods call .exec
- var execCalled = false;
- var re = /a/;
- re.exec = function () { execCalled = true; return null; };
- if (KEY === 'split') {
- // RegExp[@@split] doesn't call the regex's exec method, but first creates
- // a new one. We need to return the patched regex when creating the new one.
- re.constructor = {};
- re.constructor[SPECIES] = function () { return re; };
- }
- re[SYMBOL]('');
- return !execCalled;
- }) : undefined;
-
- if (
- !DELEGATES_TO_SYMBOL ||
- !DELEGATES_TO_EXEC ||
- (KEY === 'replace' && !REPLACE_SUPPORTS_NAMED_GROUPS) ||
- (KEY === 'split' && !SPLIT_WORKS_WITH_OVERWRITTEN_EXEC)
- ) {
- var nativeRegExpMethod = /./[SYMBOL];
- var fns = exec(
- defined,
- SYMBOL,
- ''[KEY],
- function maybeCallNative(nativeMethod, regexp, str, arg2, forceStringMethod) {
- if (regexp.exec === regexpExec) {
- if (DELEGATES_TO_SYMBOL && !forceStringMethod) {
- // The native String method already delegates to @@method (this
- // polyfilled function), leasing to infinite recursion.
- // We avoid it by directly calling the native @@method method.
- return { done: true, value: nativeRegExpMethod.call(regexp, str, arg2) };
- }
- return { done: true, value: nativeMethod.call(str, regexp, arg2) };
- }
- return { done: false };
- }
- );
- var strfn = fns[0];
- var rxfn = fns[1];
-
- redefine(String.prototype, KEY, strfn);
- hide(RegExp.prototype, SYMBOL, length == 2
- // 21.2.5.8 RegExp.prototype[@@replace](string, replaceValue)
- // 21.2.5.11 RegExp.prototype[@@split](string, limit)
- ? function (string, arg) { return rxfn.call(string, this, arg); }
- // 21.2.5.6 RegExp.prototype[@@match](string)
- // 21.2.5.9 RegExp.prototype[@@search](string)
- : function (string) { return rxfn.call(string, this); }
- );
- }
- };
-
-
-/***/ }),
-/* 207 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
-
- var anObject = __webpack_require__(12);
- var toObject = __webpack_require__(46);
- var toLength = __webpack_require__(38);
- var toInteger = __webpack_require__(39);
- var advanceStringIndex = __webpack_require__(204);
- var regExpExec = __webpack_require__(205);
- var max = Math.max;
- var min = Math.min;
- var floor = Math.floor;
- var SUBSTITUTION_SYMBOLS = /\$([$&`']|\d\d?|<[^>]*>)/g;
- var SUBSTITUTION_SYMBOLS_NO_NAMED = /\$([$&`']|\d\d?)/g;
-
- var maybeToString = function (it) {
- return it === undefined ? it : String(it);
- };
-
- // @@replace logic
- __webpack_require__(206)('replace', 2, function (defined, REPLACE, $replace, maybeCallNative) {
- return [
- // `String.prototype.replace` method
- // https://tc39.github.io/ecma262/#sec-string.prototype.replace
- function replace(searchValue, replaceValue) {
- var O = defined(this);
- var fn = searchValue == undefined ? undefined : searchValue[REPLACE];
- return fn !== undefined
- ? fn.call(searchValue, O, replaceValue)
- : $replace.call(String(O), searchValue, replaceValue);
- },
- // `RegExp.prototype[@@replace]` method
- // https://tc39.github.io/ecma262/#sec-regexp.prototype-@@replace
- function (regexp, replaceValue) {
- var res = maybeCallNative($replace, regexp, this, replaceValue);
- if (res.done) return res.value;
-
- var rx = anObject(regexp);
- var S = String(this);
- var functionalReplace = typeof replaceValue === 'function';
- if (!functionalReplace) replaceValue = String(replaceValue);
- var global = rx.global;
- if (global) {
- var fullUnicode = rx.unicode;
- rx.lastIndex = 0;
- }
- var results = [];
- while (true) {
- var result = regExpExec(rx, S);
- if (result === null) break;
- results.push(result);
- if (!global) break;
- var matchStr = String(result[0]);
- if (matchStr === '') rx.lastIndex = advanceStringIndex(S, toLength(rx.lastIndex), fullUnicode);
- }
- var accumulatedResult = '';
- var nextSourcePosition = 0;
- for (var i = 0; i < results.length; i++) {
- result = results[i];
- var matched = String(result[0]);
- var position = max(min(toInteger(result.index), S.length), 0);
- var captures = [];
- // NOTE: This is equivalent to
- // captures = result.slice(1).map(maybeToString)
- // but for some reason `nativeSlice.call(result, 1, result.length)` (called in
- // the slice polyfill when slicing native arrays) "doesn't work" in safari 9 and
- // causes a crash (https://pastebin.com/N21QzeQA) when trying to debug it.
- for (var j = 1; j < result.length; j++) captures.push(maybeToString(result[j]));
- var namedCaptures = result.groups;
- if (functionalReplace) {
- var replacerArgs = [matched].concat(captures, position, S);
- if (namedCaptures !== undefined) replacerArgs.push(namedCaptures);
- var replacement = String(replaceValue.apply(undefined, replacerArgs));
- } else {
- replacement = getSubstitution(matched, S, position, captures, namedCaptures, replaceValue);
- }
- if (position >= nextSourcePosition) {
- accumulatedResult += S.slice(nextSourcePosition, position) + replacement;
- nextSourcePosition = position + matched.length;
- }
- }
- return accumulatedResult + S.slice(nextSourcePosition);
- }
- ];
-
- // https://tc39.github.io/ecma262/#sec-getsubstitution
- function getSubstitution(matched, str, position, captures, namedCaptures, replacement) {
- var tailPos = position + matched.length;
- var m = captures.length;
- var symbols = SUBSTITUTION_SYMBOLS_NO_NAMED;
- if (namedCaptures !== undefined) {
- namedCaptures = toObject(namedCaptures);
- symbols = SUBSTITUTION_SYMBOLS;
- }
- return $replace.call(replacement, symbols, function (match, ch) {
- var capture;
- switch (ch.charAt(0)) {
- case '$': return '$';
- case '&': return matched;
- case '`': return str.slice(0, position);
- case "'": return str.slice(tailPos);
- case '<':
- capture = namedCaptures[ch.slice(1, -1)];
- break;
- default: // \d\d?
- var n = +ch;
- if (n === 0) return match;
- if (n > m) {
- var f = floor(n / 10);
- if (f === 0) return match;
- if (f <= m) return captures[f - 1] === undefined ? ch.charAt(1) : captures[f - 1] + ch.charAt(1);
- return match;
- }
- capture = captures[n - 1];
- }
- return capture === undefined ? '' : capture;
- });
- }
- });
-
-
-/***/ }),
-/* 208 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
-
- var anObject = __webpack_require__(12);
- var sameValue = __webpack_require__(71);
- var regExpExec = __webpack_require__(205);
-
- // @@search logic
- __webpack_require__(206)('search', 1, function (defined, SEARCH, $search, maybeCallNative) {
- return [
- // `String.prototype.search` method
- // https://tc39.github.io/ecma262/#sec-string.prototype.search
- function search(regexp) {
- var O = defined(this);
- var fn = regexp == undefined ? undefined : regexp[SEARCH];
- return fn !== undefined ? fn.call(regexp, O) : new RegExp(regexp)[SEARCH](String(O));
- },
- // `RegExp.prototype[@@search]` method
- // https://tc39.github.io/ecma262/#sec-regexp.prototype-@@search
- function (regexp) {
- var res = maybeCallNative($search, regexp, this);
- if (res.done) return res.value;
- var rx = anObject(regexp);
- var S = String(this);
- var previousLastIndex = rx.lastIndex;
- if (!sameValue(previousLastIndex, 0)) rx.lastIndex = 0;
- var result = regExpExec(rx, S);
- if (!sameValue(rx.lastIndex, previousLastIndex)) rx.lastIndex = previousLastIndex;
- return result === null ? -1 : result.index;
- }
- ];
- });
-
-
-/***/ }),
-/* 209 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
-
- var isRegExp = __webpack_require__(135);
- var anObject = __webpack_require__(12);
- var speciesConstructor = __webpack_require__(210);
- var advanceStringIndex = __webpack_require__(204);
- var toLength = __webpack_require__(38);
- var callRegExpExec = __webpack_require__(205);
- var regexpExec = __webpack_require__(200);
- var fails = __webpack_require__(7);
- var $min = Math.min;
- var $push = [].push;
- var $SPLIT = 'split';
- var LENGTH = 'length';
- var LAST_INDEX = 'lastIndex';
- var MAX_UINT32 = 0xffffffff;
-
- // babel-minify transpiles RegExp('x', 'y') -> /x/y and it causes SyntaxError
- var SUPPORTS_Y = !fails(function () { RegExp(MAX_UINT32, 'y'); });
-
- // @@split logic
- __webpack_require__(206)('split', 2, function (defined, SPLIT, $split, maybeCallNative) {
- var internalSplit;
- if (
- 'abbc'[$SPLIT](/(b)*/)[1] == 'c' ||
- 'test'[$SPLIT](/(?:)/, -1)[LENGTH] != 4 ||
- 'ab'[$SPLIT](/(?:ab)*/)[LENGTH] != 2 ||
- '.'[$SPLIT](/(.?)(.?)/)[LENGTH] != 4 ||
- '.'[$SPLIT](/()()/)[LENGTH] > 1 ||
- ''[$SPLIT](/.?/)[LENGTH]
- ) {
- // based on es5-shim implementation, need to rework it
- internalSplit = function (separator, limit) {
- var string = String(this);
- if (separator === undefined && limit === 0) return [];
- // If `separator` is not a regex, use native split
- if (!isRegExp(separator)) return $split.call(string, separator, limit);
- var output = [];
- var flags = (separator.ignoreCase ? 'i' : '') +
- (separator.multiline ? 'm' : '') +
- (separator.unicode ? 'u' : '') +
- (separator.sticky ? 'y' : '');
- var lastLastIndex = 0;
- var splitLimit = limit === undefined ? MAX_UINT32 : limit >>> 0;
- // Make `global` and avoid `lastIndex` issues by working with a copy
- var separatorCopy = new RegExp(separator.source, flags + 'g');
- var match, lastIndex, lastLength;
- while (match = regexpExec.call(separatorCopy, string)) {
- lastIndex = separatorCopy[LAST_INDEX];
- if (lastIndex > lastLastIndex) {
- output.push(string.slice(lastLastIndex, match.index));
- if (match[LENGTH] > 1 && match.index < string[LENGTH]) $push.apply(output, match.slice(1));
- lastLength = match[0][LENGTH];
- lastLastIndex = lastIndex;
- if (output[LENGTH] >= splitLimit) break;
- }
- if (separatorCopy[LAST_INDEX] === match.index) separatorCopy[LAST_INDEX]++; // Avoid an infinite loop
- }
- if (lastLastIndex === string[LENGTH]) {
- if (lastLength || !separatorCopy.test('')) output.push('');
- } else output.push(string.slice(lastLastIndex));
- return output[LENGTH] > splitLimit ? output.slice(0, splitLimit) : output;
- };
- // Chakra, V8
- } else if ('0'[$SPLIT](undefined, 0)[LENGTH]) {
- internalSplit = function (separator, limit) {
- return separator === undefined && limit === 0 ? [] : $split.call(this, separator, limit);
- };
- } else {
- internalSplit = $split;
- }
-
- return [
- // `String.prototype.split` method
- // https://tc39.github.io/ecma262/#sec-string.prototype.split
- function split(separator, limit) {
- var O = defined(this);
- var splitter = separator == undefined ? undefined : separator[SPLIT];
- return splitter !== undefined
- ? splitter.call(separator, O, limit)
- : internalSplit.call(String(O), separator, limit);
- },
- // `RegExp.prototype[@@split]` method
- // https://tc39.github.io/ecma262/#sec-regexp.prototype-@@split
- //
- // NOTE: This cannot be properly polyfilled in engines that don't support
- // the 'y' flag.
- function (regexp, limit) {
- var res = maybeCallNative(internalSplit, regexp, this, limit, internalSplit !== $split);
- if (res.done) return res.value;
-
- var rx = anObject(regexp);
- var S = String(this);
- var C = speciesConstructor(rx, RegExp);
-
- var unicodeMatching = rx.unicode;
- var flags = (rx.ignoreCase ? 'i' : '') +
- (rx.multiline ? 'm' : '') +
- (rx.unicode ? 'u' : '') +
- (SUPPORTS_Y ? 'y' : 'g');
-
- // ^(? + rx + ) is needed, in combination with some S slicing, to
- // simulate the 'y' flag.
- var splitter = new C(SUPPORTS_Y ? rx : '^(?:' + rx.source + ')', flags);
- var lim = limit === undefined ? MAX_UINT32 : limit >>> 0;
- if (lim === 0) return [];
- if (S.length === 0) return callRegExpExec(splitter, S) === null ? [S] : [];
- var p = 0;
- var q = 0;
- var A = [];
- while (q < S.length) {
- splitter.lastIndex = SUPPORTS_Y ? q : 0;
- var z = callRegExpExec(splitter, SUPPORTS_Y ? S : S.slice(q));
- var e;
- if (
- z === null ||
- (e = $min(toLength(splitter.lastIndex + (SUPPORTS_Y ? 0 : q)), S.length)) === p
- ) {
- q = advanceStringIndex(S, q, unicodeMatching);
- } else {
- A.push(S.slice(p, q));
- if (A.length === lim) return A;
- for (var i = 1; i <= z.length - 1; i++) {
- A.push(z[i]);
- if (A.length === lim) return A;
- }
- q = p = e;
- }
- }
- A.push(S.slice(p));
- return A;
- }
- ];
- });
-
-
-/***/ }),
-/* 210 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 7.3.20 SpeciesConstructor(O, defaultConstructor)
- var anObject = __webpack_require__(12);
- var aFunction = __webpack_require__(24);
- var SPECIES = __webpack_require__(27)('species');
- module.exports = function (O, D) {
- var C = anObject(O).constructor;
- var S;
- return C === undefined || (S = anObject(C)[SPECIES]) == undefined ? D : aFunction(S);
- };
-
-
-/***/ }),
-/* 211 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var LIBRARY = __webpack_require__(22);
- var global = __webpack_require__(4);
- var ctx = __webpack_require__(23);
- var classof = __webpack_require__(75);
- var $export = __webpack_require__(8);
- var isObject = __webpack_require__(13);
- var aFunction = __webpack_require__(24);
- var anInstance = __webpack_require__(212);
- var forOf = __webpack_require__(213);
- var speciesConstructor = __webpack_require__(210);
- var task = __webpack_require__(214).set;
- var microtask = __webpack_require__(215)();
- var newPromiseCapabilityModule = __webpack_require__(216);
- var perform = __webpack_require__(217);
- var userAgent = __webpack_require__(218);
- var promiseResolve = __webpack_require__(219);
- var PROMISE = 'Promise';
- var TypeError = global.TypeError;
- var process = global.process;
- var versions = process && process.versions;
- var v8 = versions && versions.v8 || '';
- var $Promise = global[PROMISE];
- var isNode = classof(process) == 'process';
- var empty = function () { /* empty */ };
- var Internal, newGenericPromiseCapability, OwnPromiseCapability, Wrapper;
- var newPromiseCapability = newGenericPromiseCapability = newPromiseCapabilityModule.f;
-
- var USE_NATIVE = !!function () {
- try {
- // correct subclassing with @@species support
- var promise = $Promise.resolve(1);
- var FakePromise = (promise.constructor = {})[__webpack_require__(27)('species')] = function (exec) {
- exec(empty, empty);
- };
- // unhandled rejections tracking support, NodeJS Promise without it fails @@species test
- return (isNode || typeof PromiseRejectionEvent == 'function')
- && promise.then(empty) instanceof FakePromise
- // v8 6.6 (Node 10 and Chrome 66) have a bug with resolving custom thenables
- // https://bugs.chromium.org/p/chromium/issues/detail?id=830565
- // we can't detect it synchronously, so just check versions
- && v8.indexOf('6.6') !== 0
- && userAgent.indexOf('Chrome/66') === -1;
- } catch (e) { /* empty */ }
- }();
-
- // helpers
- var isThenable = function (it) {
- var then;
- return isObject(it) && typeof (then = it.then) == 'function' ? then : false;
- };
- var notify = function (promise, isReject) {
- if (promise._n) return;
- promise._n = true;
- var chain = promise._c;
- microtask(function () {
- var value = promise._v;
- var ok = promise._s == 1;
- var i = 0;
- var run = function (reaction) {
- var handler = ok ? reaction.ok : reaction.fail;
- var resolve = reaction.resolve;
- var reject = reaction.reject;
- var domain = reaction.domain;
- var result, then, exited;
- try {
- if (handler) {
- if (!ok) {
- if (promise._h == 2) onHandleUnhandled(promise);
- promise._h = 1;
- }
- if (handler === true) result = value;
- else {
- if (domain) domain.enter();
- result = handler(value); // may throw
- if (domain) {
- domain.exit();
- exited = true;
- }
- }
- if (result === reaction.promise) {
- reject(TypeError('Promise-chain cycle'));
- } else if (then = isThenable(result)) {
- then.call(result, resolve, reject);
- } else resolve(result);
- } else reject(value);
- } catch (e) {
- if (domain && !exited) domain.exit();
- reject(e);
- }
- };
- while (chain.length > i) run(chain[i++]); // variable length - can't use forEach
- promise._c = [];
- promise._n = false;
- if (isReject && !promise._h) onUnhandled(promise);
- });
- };
- var onUnhandled = function (promise) {
- task.call(global, function () {
- var value = promise._v;
- var unhandled = isUnhandled(promise);
- var result, handler, console;
- if (unhandled) {
- result = perform(function () {
- if (isNode) {
- process.emit('unhandledRejection', value, promise);
- } else if (handler = global.onunhandledrejection) {
- handler({ promise: promise, reason: value });
- } else if ((console = global.console) && console.error) {
- console.error('Unhandled promise rejection', value);
- }
- });
- // Browsers should not trigger `rejectionHandled` event if it was handled here, NodeJS - should
- promise._h = isNode || isUnhandled(promise) ? 2 : 1;
- } promise._a = undefined;
- if (unhandled && result.e) throw result.v;
- });
- };
- var isUnhandled = function (promise) {
- return promise._h !== 1 && (promise._a || promise._c).length === 0;
- };
- var onHandleUnhandled = function (promise) {
- task.call(global, function () {
- var handler;
- if (isNode) {
- process.emit('rejectionHandled', promise);
- } else if (handler = global.onrejectionhandled) {
- handler({ promise: promise, reason: promise._v });
- }
- });
- };
- var $reject = function (value) {
- var promise = this;
- if (promise._d) return;
- promise._d = true;
- promise = promise._w || promise; // unwrap
- promise._v = value;
- promise._s = 2;
- if (!promise._a) promise._a = promise._c.slice();
- notify(promise, true);
- };
- var $resolve = function (value) {
- var promise = this;
- var then;
- if (promise._d) return;
- promise._d = true;
- promise = promise._w || promise; // unwrap
- try {
- if (promise === value) throw TypeError("Promise can't be resolved itself");
- if (then = isThenable(value)) {
- microtask(function () {
- var wrapper = { _w: promise, _d: false }; // wrap
- try {
- then.call(value, ctx($resolve, wrapper, 1), ctx($reject, wrapper, 1));
- } catch (e) {
- $reject.call(wrapper, e);
- }
- });
- } else {
- promise._v = value;
- promise._s = 1;
- notify(promise, false);
- }
- } catch (e) {
- $reject.call({ _w: promise, _d: false }, e); // wrap
- }
- };
-
- // constructor polyfill
- if (!USE_NATIVE) {
- // 25.4.3.1 Promise(executor)
- $Promise = function Promise(executor) {
- anInstance(this, $Promise, PROMISE, '_h');
- aFunction(executor);
- Internal.call(this);
- try {
- executor(ctx($resolve, this, 1), ctx($reject, this, 1));
- } catch (err) {
- $reject.call(this, err);
- }
- };
- // eslint-disable-next-line no-unused-vars
- Internal = function Promise(executor) {
- this._c = []; // <- awaiting reactions
- this._a = undefined; // <- checked in isUnhandled reactions
- this._s = 0; // <- state
- this._d = false; // <- done
- this._v = undefined; // <- value
- this._h = 0; // <- rejection state, 0 - default, 1 - handled, 2 - unhandled
- this._n = false; // <- notify
- };
- Internal.prototype = __webpack_require__(220)($Promise.prototype, {
- // 25.4.5.3 Promise.prototype.then(onFulfilled, onRejected)
- then: function then(onFulfilled, onRejected) {
- var reaction = newPromiseCapability(speciesConstructor(this, $Promise));
- reaction.ok = typeof onFulfilled == 'function' ? onFulfilled : true;
- reaction.fail = typeof onRejected == 'function' && onRejected;
- reaction.domain = isNode ? process.domain : undefined;
- this._c.push(reaction);
- if (this._a) this._a.push(reaction);
- if (this._s) notify(this, false);
- return reaction.promise;
- },
- // 25.4.5.1 Promise.prototype.catch(onRejected)
- 'catch': function (onRejected) {
- return this.then(undefined, onRejected);
- }
- });
- OwnPromiseCapability = function () {
- var promise = new Internal();
- this.promise = promise;
- this.resolve = ctx($resolve, promise, 1);
- this.reject = ctx($reject, promise, 1);
- };
- newPromiseCapabilityModule.f = newPromiseCapability = function (C) {
- return C === $Promise || C === Wrapper
- ? new OwnPromiseCapability(C)
- : newGenericPromiseCapability(C);
- };
- }
-
- $export($export.G + $export.W + $export.F * !USE_NATIVE, { Promise: $Promise });
- __webpack_require__(26)($Promise, PROMISE);
- __webpack_require__(194)(PROMISE);
- Wrapper = __webpack_require__(9)[PROMISE];
-
- // statics
- $export($export.S + $export.F * !USE_NATIVE, PROMISE, {
- // 25.4.4.5 Promise.reject(r)
- reject: function reject(r) {
- var capability = newPromiseCapability(this);
- var $$reject = capability.reject;
- $$reject(r);
- return capability.promise;
- }
- });
- $export($export.S + $export.F * (LIBRARY || !USE_NATIVE), PROMISE, {
- // 25.4.4.6 Promise.resolve(x)
- resolve: function resolve(x) {
- return promiseResolve(LIBRARY && this === Wrapper ? $Promise : this, x);
- }
- });
- $export($export.S + $export.F * !(USE_NATIVE && __webpack_require__(167)(function (iter) {
- $Promise.all(iter)['catch'](empty);
- })), PROMISE, {
- // 25.4.4.1 Promise.all(iterable)
- all: function all(iterable) {
- var C = this;
- var capability = newPromiseCapability(C);
- var resolve = capability.resolve;
- var reject = capability.reject;
- var result = perform(function () {
- var values = [];
- var index = 0;
- var remaining = 1;
- forOf(iterable, false, function (promise) {
- var $index = index++;
- var alreadyCalled = false;
- values.push(undefined);
- remaining++;
- C.resolve(promise).then(function (value) {
- if (alreadyCalled) return;
- alreadyCalled = true;
- values[$index] = value;
- --remaining || resolve(values);
- }, reject);
- });
- --remaining || resolve(values);
- });
- if (result.e) reject(result.v);
- return capability.promise;
- },
- // 25.4.4.4 Promise.race(iterable)
- race: function race(iterable) {
- var C = this;
- var capability = newPromiseCapability(C);
- var reject = capability.reject;
- var result = perform(function () {
- forOf(iterable, false, function (promise) {
- C.resolve(promise).then(capability.resolve, reject);
- });
- });
- if (result.e) reject(result.v);
- return capability.promise;
- }
- });
-
-
-/***/ }),
-/* 212 */
-/***/ (function(module, exports) {
-
- module.exports = function (it, Constructor, name, forbiddenField) {
- if (!(it instanceof Constructor) || (forbiddenField !== undefined && forbiddenField in it)) {
- throw TypeError(name + ': incorrect invocation!');
- } return it;
- };
-
-
-/***/ }),
-/* 213 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var ctx = __webpack_require__(23);
- var call = __webpack_require__(163);
- var isArrayIter = __webpack_require__(164);
- var anObject = __webpack_require__(12);
- var toLength = __webpack_require__(38);
- var getIterFn = __webpack_require__(166);
- var BREAK = {};
- var RETURN = {};
- var exports = module.exports = function (iterable, entries, fn, that, ITERATOR) {
- var iterFn = ITERATOR ? function () { return iterable; } : getIterFn(iterable);
- var f = ctx(fn, that, entries ? 2 : 1);
- var index = 0;
- var length, step, iterator, result;
- if (typeof iterFn != 'function') throw TypeError(iterable + ' is not iterable!');
- // fast case for arrays with default iterator
- if (isArrayIter(iterFn)) for (length = toLength(iterable.length); length > index; index++) {
- result = entries ? f(anObject(step = iterable[index])[0], step[1]) : f(iterable[index]);
- if (result === BREAK || result === RETURN) return result;
- } else for (iterator = iterFn.call(iterable); !(step = iterator.next()).done;) {
- result = call(iterator, f, step.value, entries);
- if (result === BREAK || result === RETURN) return result;
- }
- };
- exports.BREAK = BREAK;
- exports.RETURN = RETURN;
-
-
-/***/ }),
-/* 214 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var ctx = __webpack_require__(23);
- var invoke = __webpack_require__(78);
- var html = __webpack_require__(49);
- var cel = __webpack_require__(15);
- var global = __webpack_require__(4);
- var process = global.process;
- var setTask = global.setImmediate;
- var clearTask = global.clearImmediate;
- var MessageChannel = global.MessageChannel;
- var Dispatch = global.Dispatch;
- var counter = 0;
- var queue = {};
- var ONREADYSTATECHANGE = 'onreadystatechange';
- var defer, channel, port;
- var run = function () {
- var id = +this;
- // eslint-disable-next-line no-prototype-builtins
- if (queue.hasOwnProperty(id)) {
- var fn = queue[id];
- delete queue[id];
- fn();
- }
- };
- var listener = function (event) {
- run.call(event.data);
- };
- // Node.js 0.9+ & IE10+ has setImmediate, otherwise:
- if (!setTask || !clearTask) {
- setTask = function setImmediate(fn) {
- var args = [];
- var i = 1;
- while (arguments.length > i) args.push(arguments[i++]);
- queue[++counter] = function () {
- // eslint-disable-next-line no-new-func
- invoke(typeof fn == 'function' ? fn : Function(fn), args);
- };
- defer(counter);
- return counter;
- };
- clearTask = function clearImmediate(id) {
- delete queue[id];
- };
- // Node.js 0.8-
- if (__webpack_require__(35)(process) == 'process') {
- defer = function (id) {
- process.nextTick(ctx(run, id, 1));
- };
- // Sphere (JS game engine) Dispatch API
- } else if (Dispatch && Dispatch.now) {
- defer = function (id) {
- Dispatch.now(ctx(run, id, 1));
- };
- // Browsers with MessageChannel, includes WebWorkers
- } else if (MessageChannel) {
- channel = new MessageChannel();
- port = channel.port2;
- channel.port1.onmessage = listener;
- defer = ctx(port.postMessage, port, 1);
- // Browsers with postMessage, skip WebWorkers
- // IE8 has postMessage, but it's sync & typeof its postMessage is 'object'
- } else if (global.addEventListener && typeof postMessage == 'function' && !global.importScripts) {
- defer = function (id) {
- global.postMessage(id + '', '*');
- };
- global.addEventListener('message', listener, false);
- // IE8-
- } else if (ONREADYSTATECHANGE in cel('script')) {
- defer = function (id) {
- html.appendChild(cel('script'))[ONREADYSTATECHANGE] = function () {
- html.removeChild(this);
- run.call(id);
- };
- };
- // Rest old browsers
- } else {
- defer = function (id) {
- setTimeout(ctx(run, id, 1), 0);
- };
- }
- }
- module.exports = {
- set: setTask,
- clear: clearTask
- };
-
-
-/***/ }),
-/* 215 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var global = __webpack_require__(4);
- var macrotask = __webpack_require__(214).set;
- var Observer = global.MutationObserver || global.WebKitMutationObserver;
- var process = global.process;
- var Promise = global.Promise;
- var isNode = __webpack_require__(35)(process) == 'process';
-
- module.exports = function () {
- var head, last, notify;
-
- var flush = function () {
- var parent, fn;
- if (isNode && (parent = process.domain)) parent.exit();
- while (head) {
- fn = head.fn;
- head = head.next;
- try {
- fn();
- } catch (e) {
- if (head) notify();
- else last = undefined;
- throw e;
- }
- } last = undefined;
- if (parent) parent.enter();
- };
-
- // Node.js
- if (isNode) {
- notify = function () {
- process.nextTick(flush);
- };
- // browsers with MutationObserver, except iOS Safari - https://github.com/zloirock/core-js/issues/339
- } else if (Observer && !(global.navigator && global.navigator.standalone)) {
- var toggle = true;
- var node = document.createTextNode('');
- new Observer(flush).observe(node, { characterData: true }); // eslint-disable-line no-new
- notify = function () {
- node.data = toggle = !toggle;
- };
- // environments with maybe non-completely correct, but existent Promise
- } else if (Promise && Promise.resolve) {
- // Promise.resolve without an argument throws an error in LG WebOS 2
- var promise = Promise.resolve(undefined);
- notify = function () {
- promise.then(flush);
- };
- // for other environments - macrotask based on:
- // - setImmediate
- // - MessageChannel
- // - window.postMessag
- // - onreadystatechange
- // - setTimeout
- } else {
- notify = function () {
- // strange IE + webpack dev server bug - use .call(global)
- macrotask.call(global, flush);
- };
- }
-
- return function (fn) {
- var task = { fn: fn, next: undefined };
- if (last) last.next = task;
- if (!head) {
- head = task;
- notify();
- } last = task;
- };
- };
-
-
-/***/ }),
-/* 216 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // 25.4.1.5 NewPromiseCapability(C)
- var aFunction = __webpack_require__(24);
-
- function PromiseCapability(C) {
- var resolve, reject;
- this.promise = new C(function ($$resolve, $$reject) {
- if (resolve !== undefined || reject !== undefined) throw TypeError('Bad Promise constructor');
- resolve = $$resolve;
- reject = $$reject;
- });
- this.resolve = aFunction(resolve);
- this.reject = aFunction(reject);
- }
-
- module.exports.f = function (C) {
- return new PromiseCapability(C);
- };
-
-
-/***/ }),
-/* 217 */
-/***/ (function(module, exports) {
-
- module.exports = function (exec) {
- try {
- return { e: false, v: exec() };
- } catch (e) {
- return { e: true, v: e };
- }
- };
-
-
-/***/ }),
-/* 218 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var global = __webpack_require__(4);
- var navigator = global.navigator;
-
- module.exports = navigator && navigator.userAgent || '';
-
-
-/***/ }),
-/* 219 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var anObject = __webpack_require__(12);
- var isObject = __webpack_require__(13);
- var newPromiseCapability = __webpack_require__(216);
-
- module.exports = function (C, x) {
- anObject(C);
- if (isObject(x) && x.constructor === C) return x;
- var promiseCapability = newPromiseCapability.f(C);
- var resolve = promiseCapability.resolve;
- resolve(x);
- return promiseCapability.promise;
- };
-
-
-/***/ }),
-/* 220 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var redefine = __webpack_require__(18);
- module.exports = function (target, src, safe) {
- for (var key in src) redefine(target, key, src[key], safe);
- return target;
- };
-
-
-/***/ }),
-/* 221 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var strong = __webpack_require__(222);
- var validate = __webpack_require__(223);
- var MAP = 'Map';
-
- // 23.1 Map Objects
- module.exports = __webpack_require__(224)(MAP, function (get) {
- return function Map() { return get(this, arguments.length > 0 ? arguments[0] : undefined); };
- }, {
- // 23.1.3.6 Map.prototype.get(key)
- get: function get(key) {
- var entry = strong.getEntry(validate(this, MAP), key);
- return entry && entry.v;
- },
- // 23.1.3.9 Map.prototype.set(key, value)
- set: function set(key, value) {
- return strong.def(validate(this, MAP), key === 0 ? 0 : key, value);
- }
- }, strong, true);
-
-
-/***/ }),
-/* 222 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var dP = __webpack_require__(11).f;
- var create = __webpack_require__(47);
- var redefineAll = __webpack_require__(220);
- var ctx = __webpack_require__(23);
- var anInstance = __webpack_require__(212);
- var forOf = __webpack_require__(213);
- var $iterDefine = __webpack_require__(129);
- var step = __webpack_require__(196);
- var setSpecies = __webpack_require__(194);
- var DESCRIPTORS = __webpack_require__(6);
- var fastKey = __webpack_require__(25).fastKey;
- var validate = __webpack_require__(223);
- var SIZE = DESCRIPTORS ? '_s' : 'size';
-
- var getEntry = function (that, key) {
- // fast case
- var index = fastKey(key);
- var entry;
- if (index !== 'F') return that._i[index];
- // frozen object case
- for (entry = that._f; entry; entry = entry.n) {
- if (entry.k == key) return entry;
- }
- };
-
- module.exports = {
- getConstructor: function (wrapper, NAME, IS_MAP, ADDER) {
- var C = wrapper(function (that, iterable) {
- anInstance(that, C, NAME, '_i');
- that._t = NAME; // collection type
- that._i = create(null); // index
- that._f = undefined; // first entry
- that._l = undefined; // last entry
- that[SIZE] = 0; // size
- if (iterable != undefined) forOf(iterable, IS_MAP, that[ADDER], that);
- });
- redefineAll(C.prototype, {
- // 23.1.3.1 Map.prototype.clear()
- // 23.2.3.2 Set.prototype.clear()
- clear: function clear() {
- for (var that = validate(this, NAME), data = that._i, entry = that._f; entry; entry = entry.n) {
- entry.r = true;
- if (entry.p) entry.p = entry.p.n = undefined;
- delete data[entry.i];
- }
- that._f = that._l = undefined;
- that[SIZE] = 0;
- },
- // 23.1.3.3 Map.prototype.delete(key)
- // 23.2.3.4 Set.prototype.delete(value)
- 'delete': function (key) {
- var that = validate(this, NAME);
- var entry = getEntry(that, key);
- if (entry) {
- var next = entry.n;
- var prev = entry.p;
- delete that._i[entry.i];
- entry.r = true;
- if (prev) prev.n = next;
- if (next) next.p = prev;
- if (that._f == entry) that._f = next;
- if (that._l == entry) that._l = prev;
- that[SIZE]--;
- } return !!entry;
- },
- // 23.2.3.6 Set.prototype.forEach(callbackfn, thisArg = undefined)
- // 23.1.3.5 Map.prototype.forEach(callbackfn, thisArg = undefined)
- forEach: function forEach(callbackfn /* , that = undefined */) {
- validate(this, NAME);
- var f = ctx(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3);
- var entry;
- while (entry = entry ? entry.n : this._f) {
- f(entry.v, entry.k, this);
- // revert to the last existing entry
- while (entry && entry.r) entry = entry.p;
- }
- },
- // 23.1.3.7 Map.prototype.has(key)
- // 23.2.3.7 Set.prototype.has(value)
- has: function has(key) {
- return !!getEntry(validate(this, NAME), key);
- }
- });
- if (DESCRIPTORS) dP(C.prototype, 'size', {
- get: function () {
- return validate(this, NAME)[SIZE];
- }
- });
- return C;
- },
- def: function (that, key, value) {
- var entry = getEntry(that, key);
- var prev, index;
- // change existing entry
- if (entry) {
- entry.v = value;
- // create new entry
- } else {
- that._l = entry = {
- i: index = fastKey(key, true), // <- index
- k: key, // <- key
- v: value, // <- value
- p: prev = that._l, // <- previous entry
- n: undefined, // <- next entry
- r: false // <- removed
- };
- if (!that._f) that._f = entry;
- if (prev) prev.n = entry;
- that[SIZE]++;
- // add to index
- if (index !== 'F') that._i[index] = entry;
- } return that;
- },
- getEntry: getEntry,
- setStrong: function (C, NAME, IS_MAP) {
- // add .keys, .values, .entries, [@@iterator]
- // 23.1.3.4, 23.1.3.8, 23.1.3.11, 23.1.3.12, 23.2.3.5, 23.2.3.8, 23.2.3.10, 23.2.3.11
- $iterDefine(C, NAME, function (iterated, kind) {
- this._t = validate(iterated, NAME); // target
- this._k = kind; // kind
- this._l = undefined; // previous
- }, function () {
- var that = this;
- var kind = that._k;
- var entry = that._l;
- // revert to the last existing entry
- while (entry && entry.r) entry = entry.p;
- // get next entry
- if (!that._t || !(that._l = entry = entry ? entry.n : that._t._f)) {
- // or finish the iteration
- that._t = undefined;
- return step(1);
- }
- // return step by kind
- if (kind == 'keys') return step(0, entry.k);
- if (kind == 'values') return step(0, entry.v);
- return step(0, [entry.k, entry.v]);
- }, IS_MAP ? 'entries' : 'values', !IS_MAP, true);
-
- // add [@@species], 23.1.2.2, 23.2.2.2
- setSpecies(NAME);
- }
- };
-
-
-/***/ }),
-/* 223 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var isObject = __webpack_require__(13);
- module.exports = function (it, TYPE) {
- if (!isObject(it) || it._t !== TYPE) throw TypeError('Incompatible receiver, ' + TYPE + ' required!');
- return it;
- };
-
-
-/***/ }),
-/* 224 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var global = __webpack_require__(4);
- var $export = __webpack_require__(8);
- var redefine = __webpack_require__(18);
- var redefineAll = __webpack_require__(220);
- var meta = __webpack_require__(25);
- var forOf = __webpack_require__(213);
- var anInstance = __webpack_require__(212);
- var isObject = __webpack_require__(13);
- var fails = __webpack_require__(7);
- var $iterDetect = __webpack_require__(167);
- var setToStringTag = __webpack_require__(26);
- var inheritIfRequired = __webpack_require__(88);
-
- module.exports = function (NAME, wrapper, methods, common, IS_MAP, IS_WEAK) {
- var Base = global[NAME];
- var C = Base;
- var ADDER = IS_MAP ? 'set' : 'add';
- var proto = C && C.prototype;
- var O = {};
- var fixMethod = function (KEY) {
- var fn = proto[KEY];
- redefine(proto, KEY,
- KEY == 'delete' ? function (a) {
- return IS_WEAK && !isObject(a) ? false : fn.call(this, a === 0 ? 0 : a);
- } : KEY == 'has' ? function has(a) {
- return IS_WEAK && !isObject(a) ? false : fn.call(this, a === 0 ? 0 : a);
- } : KEY == 'get' ? function get(a) {
- return IS_WEAK && !isObject(a) ? undefined : fn.call(this, a === 0 ? 0 : a);
- } : KEY == 'add' ? function add(a) { fn.call(this, a === 0 ? 0 : a); return this; }
- : function set(a, b) { fn.call(this, a === 0 ? 0 : a, b); return this; }
- );
- };
- if (typeof C != 'function' || !(IS_WEAK || proto.forEach && !fails(function () {
- new C().entries().next();
- }))) {
- // create collection constructor
- C = common.getConstructor(wrapper, NAME, IS_MAP, ADDER);
- redefineAll(C.prototype, methods);
- meta.NEED = true;
- } else {
- var instance = new C();
- // early implementations not supports chaining
- var HASNT_CHAINING = instance[ADDER](IS_WEAK ? {} : -0, 1) != instance;
- // V8 ~ Chromium 40- weak-collections throws on primitives, but should return false
- var THROWS_ON_PRIMITIVES = fails(function () { instance.has(1); });
- // most early implementations doesn't supports iterables, most modern - not close it correctly
- var ACCEPT_ITERABLES = $iterDetect(function (iter) { new C(iter); }); // eslint-disable-line no-new
- // for early implementations -0 and +0 not the same
- var BUGGY_ZERO = !IS_WEAK && fails(function () {
- // V8 ~ Chromium 42- fails only with 5+ elements
- var $instance = new C();
- var index = 5;
- while (index--) $instance[ADDER](index, index);
- return !$instance.has(-0);
- });
- if (!ACCEPT_ITERABLES) {
- C = wrapper(function (target, iterable) {
- anInstance(target, C, NAME);
- var that = inheritIfRequired(new Base(), target, C);
- if (iterable != undefined) forOf(iterable, IS_MAP, that[ADDER], that);
- return that;
- });
- C.prototype = proto;
- proto.constructor = C;
- }
- if (THROWS_ON_PRIMITIVES || BUGGY_ZERO) {
- fixMethod('delete');
- fixMethod('has');
- IS_MAP && fixMethod('get');
- }
- if (BUGGY_ZERO || HASNT_CHAINING) fixMethod(ADDER);
- // weak collections should not contains .clear method
- if (IS_WEAK && proto.clear) delete proto.clear;
- }
-
- setToStringTag(C, NAME);
-
- O[NAME] = C;
- $export($export.G + $export.W + $export.F * (C != Base), O);
-
- if (!IS_WEAK) common.setStrong(C, NAME, IS_MAP);
-
- return C;
- };
-
-
-/***/ }),
-/* 225 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var strong = __webpack_require__(222);
- var validate = __webpack_require__(223);
- var SET = 'Set';
-
- // 23.2 Set Objects
- module.exports = __webpack_require__(224)(SET, function (get) {
- return function Set() { return get(this, arguments.length > 0 ? arguments[0] : undefined); };
- }, {
- // 23.2.3.1 Set.prototype.add(value)
- add: function add(value) {
- return strong.def(validate(this, SET), value = value === 0 ? 0 : value, value);
- }
- }, strong);
-
-
-/***/ }),
-/* 226 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var global = __webpack_require__(4);
- var each = __webpack_require__(174)(0);
- var redefine = __webpack_require__(18);
- var meta = __webpack_require__(25);
- var assign = __webpack_require__(69);
- var weak = __webpack_require__(227);
- var isObject = __webpack_require__(13);
- var validate = __webpack_require__(223);
- var NATIVE_WEAK_MAP = __webpack_require__(223);
- var IS_IE11 = !global.ActiveXObject && 'ActiveXObject' in global;
- var WEAK_MAP = 'WeakMap';
- var getWeak = meta.getWeak;
- var isExtensible = Object.isExtensible;
- var uncaughtFrozenStore = weak.ufstore;
- var InternalMap;
-
- var wrapper = function (get) {
- return function WeakMap() {
- return get(this, arguments.length > 0 ? arguments[0] : undefined);
- };
- };
-
- var methods = {
- // 23.3.3.3 WeakMap.prototype.get(key)
- get: function get(key) {
- if (isObject(key)) {
- var data = getWeak(key);
- if (data === true) return uncaughtFrozenStore(validate(this, WEAK_MAP)).get(key);
- return data ? data[this._i] : undefined;
- }
- },
- // 23.3.3.5 WeakMap.prototype.set(key, value)
- set: function set(key, value) {
- return weak.def(validate(this, WEAK_MAP), key, value);
- }
- };
-
- // 23.3 WeakMap Objects
- var $WeakMap = module.exports = __webpack_require__(224)(WEAK_MAP, wrapper, methods, weak, true, true);
-
- // IE11 WeakMap frozen keys fix
- if (NATIVE_WEAK_MAP && IS_IE11) {
- InternalMap = weak.getConstructor(wrapper, WEAK_MAP);
- assign(InternalMap.prototype, methods);
- meta.NEED = true;
- each(['delete', 'has', 'get', 'set'], function (key) {
- var proto = $WeakMap.prototype;
- var method = proto[key];
- redefine(proto, key, function (a, b) {
- // store frozen objects on internal weakmap shim
- if (isObject(a) && !isExtensible(a)) {
- if (!this._f) this._f = new InternalMap();
- var result = this._f[key](a, b);
- return key == 'set' ? this : result;
- // store all the rest on native weakmap
- } return method.call(this, a, b);
- });
- });
- }
-
-
-/***/ }),
-/* 227 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var redefineAll = __webpack_require__(220);
- var getWeak = __webpack_require__(25).getWeak;
- var anObject = __webpack_require__(12);
- var isObject = __webpack_require__(13);
- var anInstance = __webpack_require__(212);
- var forOf = __webpack_require__(213);
- var createArrayMethod = __webpack_require__(174);
- var $has = __webpack_require__(5);
- var validate = __webpack_require__(223);
- var arrayFind = createArrayMethod(5);
- var arrayFindIndex = createArrayMethod(6);
- var id = 0;
-
- // fallback for uncaught frozen keys
- var uncaughtFrozenStore = function (that) {
- return that._l || (that._l = new UncaughtFrozenStore());
- };
- var UncaughtFrozenStore = function () {
- this.a = [];
- };
- var findUncaughtFrozen = function (store, key) {
- return arrayFind(store.a, function (it) {
- return it[0] === key;
- });
- };
- UncaughtFrozenStore.prototype = {
- get: function (key) {
- var entry = findUncaughtFrozen(this, key);
- if (entry) return entry[1];
- },
- has: function (key) {
- return !!findUncaughtFrozen(this, key);
- },
- set: function (key, value) {
- var entry = findUncaughtFrozen(this, key);
- if (entry) entry[1] = value;
- else this.a.push([key, value]);
- },
- 'delete': function (key) {
- var index = arrayFindIndex(this.a, function (it) {
- return it[0] === key;
- });
- if (~index) this.a.splice(index, 1);
- return !!~index;
- }
- };
-
- module.exports = {
- getConstructor: function (wrapper, NAME, IS_MAP, ADDER) {
- var C = wrapper(function (that, iterable) {
- anInstance(that, C, NAME, '_i');
- that._t = NAME; // collection type
- that._i = id++; // collection id
- that._l = undefined; // leak store for uncaught frozen objects
- if (iterable != undefined) forOf(iterable, IS_MAP, that[ADDER], that);
- });
- redefineAll(C.prototype, {
- // 23.3.3.2 WeakMap.prototype.delete(key)
- // 23.4.3.3 WeakSet.prototype.delete(value)
- 'delete': function (key) {
- if (!isObject(key)) return false;
- var data = getWeak(key);
- if (data === true) return uncaughtFrozenStore(validate(this, NAME))['delete'](key);
- return data && $has(data, this._i) && delete data[this._i];
- },
- // 23.3.3.4 WeakMap.prototype.has(key)
- // 23.4.3.4 WeakSet.prototype.has(value)
- has: function has(key) {
- if (!isObject(key)) return false;
- var data = getWeak(key);
- if (data === true) return uncaughtFrozenStore(validate(this, NAME)).has(key);
- return data && $has(data, this._i);
- }
- });
- return C;
- },
- def: function (that, key, value) {
- var data = getWeak(anObject(key), true);
- if (data === true) uncaughtFrozenStore(that).set(key, value);
- else data[that._i] = value;
- return that;
- },
- ufstore: uncaughtFrozenStore
- };
-
-
-/***/ }),
-/* 228 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var weak = __webpack_require__(227);
- var validate = __webpack_require__(223);
- var WEAK_SET = 'WeakSet';
-
- // 23.4 WeakSet Objects
- __webpack_require__(224)(WEAK_SET, function (get) {
- return function WeakSet() { return get(this, arguments.length > 0 ? arguments[0] : undefined); };
- }, {
- // 23.4.3.1 WeakSet.prototype.add(value)
- add: function add(value) {
- return weak.def(validate(this, WEAK_SET), value, true);
- }
- }, weak, false, true);
-
-
-/***/ }),
-/* 229 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var $typed = __webpack_require__(230);
- var buffer = __webpack_require__(231);
- var anObject = __webpack_require__(12);
- var toAbsoluteIndex = __webpack_require__(40);
- var toLength = __webpack_require__(38);
- var isObject = __webpack_require__(13);
- var ArrayBuffer = __webpack_require__(4).ArrayBuffer;
- var speciesConstructor = __webpack_require__(210);
- var $ArrayBuffer = buffer.ArrayBuffer;
- var $DataView = buffer.DataView;
- var $isView = $typed.ABV && ArrayBuffer.isView;
- var $slice = $ArrayBuffer.prototype.slice;
- var VIEW = $typed.VIEW;
- var ARRAY_BUFFER = 'ArrayBuffer';
-
- $export($export.G + $export.W + $export.F * (ArrayBuffer !== $ArrayBuffer), { ArrayBuffer: $ArrayBuffer });
-
- $export($export.S + $export.F * !$typed.CONSTR, ARRAY_BUFFER, {
- // 24.1.3.1 ArrayBuffer.isView(arg)
- isView: function isView(it) {
- return $isView && $isView(it) || isObject(it) && VIEW in it;
- }
- });
-
- $export($export.P + $export.U + $export.F * __webpack_require__(7)(function () {
- return !new $ArrayBuffer(2).slice(1, undefined).byteLength;
- }), ARRAY_BUFFER, {
- // 24.1.4.3 ArrayBuffer.prototype.slice(start, end)
- slice: function slice(start, end) {
- if ($slice !== undefined && end === undefined) return $slice.call(anObject(this), start); // FF fix
- var len = anObject(this).byteLength;
- var first = toAbsoluteIndex(start, len);
- var fin = toAbsoluteIndex(end === undefined ? len : end, len);
- var result = new (speciesConstructor(this, $ArrayBuffer))(toLength(fin - first));
- var viewS = new $DataView(this);
- var viewT = new $DataView(result);
- var index = 0;
- while (first < fin) {
- viewT.setUint8(index++, viewS.getUint8(first++));
- } return result;
- }
- });
-
- __webpack_require__(194)(ARRAY_BUFFER);
-
-
-/***/ }),
-/* 230 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var global = __webpack_require__(4);
- var hide = __webpack_require__(10);
- var uid = __webpack_require__(19);
- var TYPED = uid('typed_array');
- var VIEW = uid('view');
- var ABV = !!(global.ArrayBuffer && global.DataView);
- var CONSTR = ABV;
- var i = 0;
- var l = 9;
- var Typed;
-
- var TypedArrayConstructors = (
- 'Int8Array,Uint8Array,Uint8ClampedArray,Int16Array,Uint16Array,Int32Array,Uint32Array,Float32Array,Float64Array'
- ).split(',');
-
- while (i < l) {
- if (Typed = global[TypedArrayConstructors[i++]]) {
- hide(Typed.prototype, TYPED, true);
- hide(Typed.prototype, VIEW, true);
- } else CONSTR = false;
- }
-
- module.exports = {
- ABV: ABV,
- CONSTR: CONSTR,
- TYPED: TYPED,
- VIEW: VIEW
- };
-
-
-/***/ }),
-/* 231 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var global = __webpack_require__(4);
- var DESCRIPTORS = __webpack_require__(6);
- var LIBRARY = __webpack_require__(22);
- var $typed = __webpack_require__(230);
- var hide = __webpack_require__(10);
- var redefineAll = __webpack_require__(220);
- var fails = __webpack_require__(7);
- var anInstance = __webpack_require__(212);
- var toInteger = __webpack_require__(39);
- var toLength = __webpack_require__(38);
- var toIndex = __webpack_require__(232);
- var gOPN = __webpack_require__(51).f;
- var dP = __webpack_require__(11).f;
- var arrayFill = __webpack_require__(190);
- var setToStringTag = __webpack_require__(26);
- var ARRAY_BUFFER = 'ArrayBuffer';
- var DATA_VIEW = 'DataView';
- var PROTOTYPE = 'prototype';
- var WRONG_LENGTH = 'Wrong length!';
- var WRONG_INDEX = 'Wrong index!';
- var $ArrayBuffer = global[ARRAY_BUFFER];
- var $DataView = global[DATA_VIEW];
- var Math = global.Math;
- var RangeError = global.RangeError;
- // eslint-disable-next-line no-shadow-restricted-names
- var Infinity = global.Infinity;
- var BaseBuffer = $ArrayBuffer;
- var abs = Math.abs;
- var pow = Math.pow;
- var floor = Math.floor;
- var log = Math.log;
- var LN2 = Math.LN2;
- var BUFFER = 'buffer';
- var BYTE_LENGTH = 'byteLength';
- var BYTE_OFFSET = 'byteOffset';
- var $BUFFER = DESCRIPTORS ? '_b' : BUFFER;
- var $LENGTH = DESCRIPTORS ? '_l' : BYTE_LENGTH;
- var $OFFSET = DESCRIPTORS ? '_o' : BYTE_OFFSET;
-
- // IEEE754 conversions based on https://github.com/feross/ieee754
- function packIEEE754(value, mLen, nBytes) {
- var buffer = new Array(nBytes);
- var eLen = nBytes * 8 - mLen - 1;
- var eMax = (1 << eLen) - 1;
- var eBias = eMax >> 1;
- var rt = mLen === 23 ? pow(2, -24) - pow(2, -77) : 0;
- var i = 0;
- var s = value < 0 || value === 0 && 1 / value < 0 ? 1 : 0;
- var e, m, c;
- value = abs(value);
- // eslint-disable-next-line no-self-compare
- if (value != value || value === Infinity) {
- // eslint-disable-next-line no-self-compare
- m = value != value ? 1 : 0;
- e = eMax;
- } else {
- e = floor(log(value) / LN2);
- if (value * (c = pow(2, -e)) < 1) {
- e--;
- c *= 2;
- }
- if (e + eBias >= 1) {
- value += rt / c;
- } else {
- value += rt * pow(2, 1 - eBias);
- }
- if (value * c >= 2) {
- e++;
- c /= 2;
- }
- if (e + eBias >= eMax) {
- m = 0;
- e = eMax;
- } else if (e + eBias >= 1) {
- m = (value * c - 1) * pow(2, mLen);
- e = e + eBias;
- } else {
- m = value * pow(2, eBias - 1) * pow(2, mLen);
- e = 0;
- }
- }
- for (; mLen >= 8; buffer[i++] = m & 255, m /= 256, mLen -= 8);
- e = e << mLen | m;
- eLen += mLen;
- for (; eLen > 0; buffer[i++] = e & 255, e /= 256, eLen -= 8);
- buffer[--i] |= s * 128;
- return buffer;
- }
- function unpackIEEE754(buffer, mLen, nBytes) {
- var eLen = nBytes * 8 - mLen - 1;
- var eMax = (1 << eLen) - 1;
- var eBias = eMax >> 1;
- var nBits = eLen - 7;
- var i = nBytes - 1;
- var s = buffer[i--];
- var e = s & 127;
- var m;
- s >>= 7;
- for (; nBits > 0; e = e * 256 + buffer[i], i--, nBits -= 8);
- m = e & (1 << -nBits) - 1;
- e >>= -nBits;
- nBits += mLen;
- for (; nBits > 0; m = m * 256 + buffer[i], i--, nBits -= 8);
- if (e === 0) {
- e = 1 - eBias;
- } else if (e === eMax) {
- return m ? NaN : s ? -Infinity : Infinity;
- } else {
- m = m + pow(2, mLen);
- e = e - eBias;
- } return (s ? -1 : 1) * m * pow(2, e - mLen);
- }
-
- function unpackI32(bytes) {
- return bytes[3] << 24 | bytes[2] << 16 | bytes[1] << 8 | bytes[0];
- }
- function packI8(it) {
- return [it & 0xff];
- }
- function packI16(it) {
- return [it & 0xff, it >> 8 & 0xff];
- }
- function packI32(it) {
- return [it & 0xff, it >> 8 & 0xff, it >> 16 & 0xff, it >> 24 & 0xff];
- }
- function packF64(it) {
- return packIEEE754(it, 52, 8);
- }
- function packF32(it) {
- return packIEEE754(it, 23, 4);
- }
-
- function addGetter(C, key, internal) {
- dP(C[PROTOTYPE], key, { get: function () { return this[internal]; } });
- }
-
- function get(view, bytes, index, isLittleEndian) {
- var numIndex = +index;
- var intIndex = toIndex(numIndex);
- if (intIndex + bytes > view[$LENGTH]) throw RangeError(WRONG_INDEX);
- var store = view[$BUFFER]._b;
- var start = intIndex + view[$OFFSET];
- var pack = store.slice(start, start + bytes);
- return isLittleEndian ? pack : pack.reverse();
- }
- function set(view, bytes, index, conversion, value, isLittleEndian) {
- var numIndex = +index;
- var intIndex = toIndex(numIndex);
- if (intIndex + bytes > view[$LENGTH]) throw RangeError(WRONG_INDEX);
- var store = view[$BUFFER]._b;
- var start = intIndex + view[$OFFSET];
- var pack = conversion(+value);
- for (var i = 0; i < bytes; i++) store[start + i] = pack[isLittleEndian ? i : bytes - i - 1];
- }
-
- if (!$typed.ABV) {
- $ArrayBuffer = function ArrayBuffer(length) {
- anInstance(this, $ArrayBuffer, ARRAY_BUFFER);
- var byteLength = toIndex(length);
- this._b = arrayFill.call(new Array(byteLength), 0);
- this[$LENGTH] = byteLength;
- };
-
- $DataView = function DataView(buffer, byteOffset, byteLength) {
- anInstance(this, $DataView, DATA_VIEW);
- anInstance(buffer, $ArrayBuffer, DATA_VIEW);
- var bufferLength = buffer[$LENGTH];
- var offset = toInteger(byteOffset);
- if (offset < 0 || offset > bufferLength) throw RangeError('Wrong offset!');
- byteLength = byteLength === undefined ? bufferLength - offset : toLength(byteLength);
- if (offset + byteLength > bufferLength) throw RangeError(WRONG_LENGTH);
- this[$BUFFER] = buffer;
- this[$OFFSET] = offset;
- this[$LENGTH] = byteLength;
- };
-
- if (DESCRIPTORS) {
- addGetter($ArrayBuffer, BYTE_LENGTH, '_l');
- addGetter($DataView, BUFFER, '_b');
- addGetter($DataView, BYTE_LENGTH, '_l');
- addGetter($DataView, BYTE_OFFSET, '_o');
- }
-
- redefineAll($DataView[PROTOTYPE], {
- getInt8: function getInt8(byteOffset) {
- return get(this, 1, byteOffset)[0] << 24 >> 24;
- },
- getUint8: function getUint8(byteOffset) {
- return get(this, 1, byteOffset)[0];
- },
- getInt16: function getInt16(byteOffset /* , littleEndian */) {
- var bytes = get(this, 2, byteOffset, arguments[1]);
- return (bytes[1] << 8 | bytes[0]) << 16 >> 16;
- },
- getUint16: function getUint16(byteOffset /* , littleEndian */) {
- var bytes = get(this, 2, byteOffset, arguments[1]);
- return bytes[1] << 8 | bytes[0];
- },
- getInt32: function getInt32(byteOffset /* , littleEndian */) {
- return unpackI32(get(this, 4, byteOffset, arguments[1]));
- },
- getUint32: function getUint32(byteOffset /* , littleEndian */) {
- return unpackI32(get(this, 4, byteOffset, arguments[1])) >>> 0;
- },
- getFloat32: function getFloat32(byteOffset /* , littleEndian */) {
- return unpackIEEE754(get(this, 4, byteOffset, arguments[1]), 23, 4);
- },
- getFloat64: function getFloat64(byteOffset /* , littleEndian */) {
- return unpackIEEE754(get(this, 8, byteOffset, arguments[1]), 52, 8);
- },
- setInt8: function setInt8(byteOffset, value) {
- set(this, 1, byteOffset, packI8, value);
- },
- setUint8: function setUint8(byteOffset, value) {
- set(this, 1, byteOffset, packI8, value);
- },
- setInt16: function setInt16(byteOffset, value /* , littleEndian */) {
- set(this, 2, byteOffset, packI16, value, arguments[2]);
- },
- setUint16: function setUint16(byteOffset, value /* , littleEndian */) {
- set(this, 2, byteOffset, packI16, value, arguments[2]);
- },
- setInt32: function setInt32(byteOffset, value /* , littleEndian */) {
- set(this, 4, byteOffset, packI32, value, arguments[2]);
- },
- setUint32: function setUint32(byteOffset, value /* , littleEndian */) {
- set(this, 4, byteOffset, packI32, value, arguments[2]);
- },
- setFloat32: function setFloat32(byteOffset, value /* , littleEndian */) {
- set(this, 4, byteOffset, packF32, value, arguments[2]);
- },
- setFloat64: function setFloat64(byteOffset, value /* , littleEndian */) {
- set(this, 8, byteOffset, packF64, value, arguments[2]);
- }
- });
- } else {
- if (!fails(function () {
- $ArrayBuffer(1);
- }) || !fails(function () {
- new $ArrayBuffer(-1); // eslint-disable-line no-new
- }) || fails(function () {
- new $ArrayBuffer(); // eslint-disable-line no-new
- new $ArrayBuffer(1.5); // eslint-disable-line no-new
- new $ArrayBuffer(NaN); // eslint-disable-line no-new
- return $ArrayBuffer.name != ARRAY_BUFFER;
- })) {
- $ArrayBuffer = function ArrayBuffer(length) {
- anInstance(this, $ArrayBuffer);
- return new BaseBuffer(toIndex(length));
- };
- var ArrayBufferProto = $ArrayBuffer[PROTOTYPE] = BaseBuffer[PROTOTYPE];
- for (var keys = gOPN(BaseBuffer), j = 0, key; keys.length > j;) {
- if (!((key = keys[j++]) in $ArrayBuffer)) hide($ArrayBuffer, key, BaseBuffer[key]);
- }
- if (!LIBRARY) ArrayBufferProto.constructor = $ArrayBuffer;
- }
- // iOS Safari 7.x bug
- var view = new $DataView(new $ArrayBuffer(2));
- var $setInt8 = $DataView[PROTOTYPE].setInt8;
- view.setInt8(0, 2147483648);
- view.setInt8(1, 2147483649);
- if (view.getInt8(0) || !view.getInt8(1)) redefineAll($DataView[PROTOTYPE], {
- setInt8: function setInt8(byteOffset, value) {
- $setInt8.call(this, byteOffset, value << 24 >> 24);
- },
- setUint8: function setUint8(byteOffset, value) {
- $setInt8.call(this, byteOffset, value << 24 >> 24);
- }
- }, true);
- }
- setToStringTag($ArrayBuffer, ARRAY_BUFFER);
- setToStringTag($DataView, DATA_VIEW);
- hide($DataView[PROTOTYPE], $typed.VIEW, true);
- exports[ARRAY_BUFFER] = $ArrayBuffer;
- exports[DATA_VIEW] = $DataView;
-
-
-/***/ }),
-/* 232 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://tc39.github.io/ecma262/#sec-toindex
- var toInteger = __webpack_require__(39);
- var toLength = __webpack_require__(38);
- module.exports = function (it) {
- if (it === undefined) return 0;
- var number = toInteger(it);
- var length = toLength(number);
- if (number !== length) throw RangeError('Wrong length!');
- return length;
- };
-
-
-/***/ }),
-/* 233 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- $export($export.G + $export.W + $export.F * !__webpack_require__(230).ABV, {
- DataView: __webpack_require__(231).DataView
- });
-
-
-/***/ }),
-/* 234 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(235)('Int8', 1, function (init) {
- return function Int8Array(data, byteOffset, length) {
- return init(this, data, byteOffset, length);
- };
- });
-
-
-/***/ }),
-/* 235 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- if (__webpack_require__(6)) {
- var LIBRARY = __webpack_require__(22);
- var global = __webpack_require__(4);
- var fails = __webpack_require__(7);
- var $export = __webpack_require__(8);
- var $typed = __webpack_require__(230);
- var $buffer = __webpack_require__(231);
- var ctx = __webpack_require__(23);
- var anInstance = __webpack_require__(212);
- var propertyDesc = __webpack_require__(17);
- var hide = __webpack_require__(10);
- var redefineAll = __webpack_require__(220);
- var toInteger = __webpack_require__(39);
- var toLength = __webpack_require__(38);
- var toIndex = __webpack_require__(232);
- var toAbsoluteIndex = __webpack_require__(40);
- var toPrimitive = __webpack_require__(16);
- var has = __webpack_require__(5);
- var classof = __webpack_require__(75);
- var isObject = __webpack_require__(13);
- var toObject = __webpack_require__(46);
- var isArrayIter = __webpack_require__(164);
- var create = __webpack_require__(47);
- var getPrototypeOf = __webpack_require__(59);
- var gOPN = __webpack_require__(51).f;
- var getIterFn = __webpack_require__(166);
- var uid = __webpack_require__(19);
- var wks = __webpack_require__(27);
- var createArrayMethod = __webpack_require__(174);
- var createArrayIncludes = __webpack_require__(37);
- var speciesConstructor = __webpack_require__(210);
- var ArrayIterators = __webpack_require__(195);
- var Iterators = __webpack_require__(130);
- var $iterDetect = __webpack_require__(167);
- var setSpecies = __webpack_require__(194);
- var arrayFill = __webpack_require__(190);
- var arrayCopyWithin = __webpack_require__(187);
- var $DP = __webpack_require__(11);
- var $GOPD = __webpack_require__(52);
- var dP = $DP.f;
- var gOPD = $GOPD.f;
- var RangeError = global.RangeError;
- var TypeError = global.TypeError;
- var Uint8Array = global.Uint8Array;
- var ARRAY_BUFFER = 'ArrayBuffer';
- var SHARED_BUFFER = 'Shared' + ARRAY_BUFFER;
- var BYTES_PER_ELEMENT = 'BYTES_PER_ELEMENT';
- var PROTOTYPE = 'prototype';
- var ArrayProto = Array[PROTOTYPE];
- var $ArrayBuffer = $buffer.ArrayBuffer;
- var $DataView = $buffer.DataView;
- var arrayForEach = createArrayMethod(0);
- var arrayFilter = createArrayMethod(2);
- var arraySome = createArrayMethod(3);
- var arrayEvery = createArrayMethod(4);
- var arrayFind = createArrayMethod(5);
- var arrayFindIndex = createArrayMethod(6);
- var arrayIncludes = createArrayIncludes(true);
- var arrayIndexOf = createArrayIncludes(false);
- var arrayValues = ArrayIterators.values;
- var arrayKeys = ArrayIterators.keys;
- var arrayEntries = ArrayIterators.entries;
- var arrayLastIndexOf = ArrayProto.lastIndexOf;
- var arrayReduce = ArrayProto.reduce;
- var arrayReduceRight = ArrayProto.reduceRight;
- var arrayJoin = ArrayProto.join;
- var arraySort = ArrayProto.sort;
- var arraySlice = ArrayProto.slice;
- var arrayToString = ArrayProto.toString;
- var arrayToLocaleString = ArrayProto.toLocaleString;
- var ITERATOR = wks('iterator');
- var TAG = wks('toStringTag');
- var TYPED_CONSTRUCTOR = uid('typed_constructor');
- var DEF_CONSTRUCTOR = uid('def_constructor');
- var ALL_CONSTRUCTORS = $typed.CONSTR;
- var TYPED_ARRAY = $typed.TYPED;
- var VIEW = $typed.VIEW;
- var WRONG_LENGTH = 'Wrong length!';
-
- var $map = createArrayMethod(1, function (O, length) {
- return allocate(speciesConstructor(O, O[DEF_CONSTRUCTOR]), length);
- });
-
- var LITTLE_ENDIAN = fails(function () {
- // eslint-disable-next-line no-undef
- return new Uint8Array(new Uint16Array([1]).buffer)[0] === 1;
- });
-
- var FORCED_SET = !!Uint8Array && !!Uint8Array[PROTOTYPE].set && fails(function () {
- new Uint8Array(1).set({});
- });
-
- var toOffset = function (it, BYTES) {
- var offset = toInteger(it);
- if (offset < 0 || offset % BYTES) throw RangeError('Wrong offset!');
- return offset;
- };
-
- var validate = function (it) {
- if (isObject(it) && TYPED_ARRAY in it) return it;
- throw TypeError(it + ' is not a typed array!');
- };
-
- var allocate = function (C, length) {
- if (!(isObject(C) && TYPED_CONSTRUCTOR in C)) {
- throw TypeError('It is not a typed array constructor!');
- } return new C(length);
- };
-
- var speciesFromList = function (O, list) {
- return fromList(speciesConstructor(O, O[DEF_CONSTRUCTOR]), list);
- };
-
- var fromList = function (C, list) {
- var index = 0;
- var length = list.length;
- var result = allocate(C, length);
- while (length > index) result[index] = list[index++];
- return result;
- };
-
- var addGetter = function (it, key, internal) {
- dP(it, key, { get: function () { return this._d[internal]; } });
- };
-
- var $from = function from(source /* , mapfn, thisArg */) {
- var O = toObject(source);
- var aLen = arguments.length;
- var mapfn = aLen > 1 ? arguments[1] : undefined;
- var mapping = mapfn !== undefined;
- var iterFn = getIterFn(O);
- var i, length, values, result, step, iterator;
- if (iterFn != undefined && !isArrayIter(iterFn)) {
- for (iterator = iterFn.call(O), values = [], i = 0; !(step = iterator.next()).done; i++) {
- values.push(step.value);
- } O = values;
- }
- if (mapping && aLen > 2) mapfn = ctx(mapfn, arguments[2], 2);
- for (i = 0, length = toLength(O.length), result = allocate(this, length); length > i; i++) {
- result[i] = mapping ? mapfn(O[i], i) : O[i];
- }
- return result;
- };
-
- var $of = function of(/* ...items */) {
- var index = 0;
- var length = arguments.length;
- var result = allocate(this, length);
- while (length > index) result[index] = arguments[index++];
- return result;
- };
-
- // iOS Safari 6.x fails here
- var TO_LOCALE_BUG = !!Uint8Array && fails(function () { arrayToLocaleString.call(new Uint8Array(1)); });
-
- var $toLocaleString = function toLocaleString() {
- return arrayToLocaleString.apply(TO_LOCALE_BUG ? arraySlice.call(validate(this)) : validate(this), arguments);
- };
-
- var proto = {
- copyWithin: function copyWithin(target, start /* , end */) {
- return arrayCopyWithin.call(validate(this), target, start, arguments.length > 2 ? arguments[2] : undefined);
- },
- every: function every(callbackfn /* , thisArg */) {
- return arrayEvery(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined);
- },
- fill: function fill(value /* , start, end */) { // eslint-disable-line no-unused-vars
- return arrayFill.apply(validate(this), arguments);
- },
- filter: function filter(callbackfn /* , thisArg */) {
- return speciesFromList(this, arrayFilter(validate(this), callbackfn,
- arguments.length > 1 ? arguments[1] : undefined));
- },
- find: function find(predicate /* , thisArg */) {
- return arrayFind(validate(this), predicate, arguments.length > 1 ? arguments[1] : undefined);
- },
- findIndex: function findIndex(predicate /* , thisArg */) {
- return arrayFindIndex(validate(this), predicate, arguments.length > 1 ? arguments[1] : undefined);
- },
- forEach: function forEach(callbackfn /* , thisArg */) {
- arrayForEach(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined);
- },
- indexOf: function indexOf(searchElement /* , fromIndex */) {
- return arrayIndexOf(validate(this), searchElement, arguments.length > 1 ? arguments[1] : undefined);
- },
- includes: function includes(searchElement /* , fromIndex */) {
- return arrayIncludes(validate(this), searchElement, arguments.length > 1 ? arguments[1] : undefined);
- },
- join: function join(separator) { // eslint-disable-line no-unused-vars
- return arrayJoin.apply(validate(this), arguments);
- },
- lastIndexOf: function lastIndexOf(searchElement /* , fromIndex */) { // eslint-disable-line no-unused-vars
- return arrayLastIndexOf.apply(validate(this), arguments);
- },
- map: function map(mapfn /* , thisArg */) {
- return $map(validate(this), mapfn, arguments.length > 1 ? arguments[1] : undefined);
- },
- reduce: function reduce(callbackfn /* , initialValue */) { // eslint-disable-line no-unused-vars
- return arrayReduce.apply(validate(this), arguments);
- },
- reduceRight: function reduceRight(callbackfn /* , initialValue */) { // eslint-disable-line no-unused-vars
- return arrayReduceRight.apply(validate(this), arguments);
- },
- reverse: function reverse() {
- var that = this;
- var length = validate(that).length;
- var middle = Math.floor(length / 2);
- var index = 0;
- var value;
- while (index < middle) {
- value = that[index];
- that[index++] = that[--length];
- that[length] = value;
- } return that;
- },
- some: function some(callbackfn /* , thisArg */) {
- return arraySome(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined);
- },
- sort: function sort(comparefn) {
- return arraySort.call(validate(this), comparefn);
- },
- subarray: function subarray(begin, end) {
- var O = validate(this);
- var length = O.length;
- var $begin = toAbsoluteIndex(begin, length);
- return new (speciesConstructor(O, O[DEF_CONSTRUCTOR]))(
- O.buffer,
- O.byteOffset + $begin * O.BYTES_PER_ELEMENT,
- toLength((end === undefined ? length : toAbsoluteIndex(end, length)) - $begin)
- );
- }
- };
-
- var $slice = function slice(start, end) {
- return speciesFromList(this, arraySlice.call(validate(this), start, end));
- };
-
- var $set = function set(arrayLike /* , offset */) {
- validate(this);
- var offset = toOffset(arguments[1], 1);
- var length = this.length;
- var src = toObject(arrayLike);
- var len = toLength(src.length);
- var index = 0;
- if (len + offset > length) throw RangeError(WRONG_LENGTH);
- while (index < len) this[offset + index] = src[index++];
- };
-
- var $iterators = {
- entries: function entries() {
- return arrayEntries.call(validate(this));
- },
- keys: function keys() {
- return arrayKeys.call(validate(this));
- },
- values: function values() {
- return arrayValues.call(validate(this));
- }
- };
-
- var isTAIndex = function (target, key) {
- return isObject(target)
- && target[TYPED_ARRAY]
- && typeof key != 'symbol'
- && key in target
- && String(+key) == String(key);
- };
- var $getDesc = function getOwnPropertyDescriptor(target, key) {
- return isTAIndex(target, key = toPrimitive(key, true))
- ? propertyDesc(2, target[key])
- : gOPD(target, key);
- };
- var $setDesc = function defineProperty(target, key, desc) {
- if (isTAIndex(target, key = toPrimitive(key, true))
- && isObject(desc)
- && has(desc, 'value')
- && !has(desc, 'get')
- && !has(desc, 'set')
- // TODO: add validation descriptor w/o calling accessors
- && !desc.configurable
- && (!has(desc, 'writable') || desc.writable)
- && (!has(desc, 'enumerable') || desc.enumerable)
- ) {
- target[key] = desc.value;
- return target;
- } return dP(target, key, desc);
- };
-
- if (!ALL_CONSTRUCTORS) {
- $GOPD.f = $getDesc;
- $DP.f = $setDesc;
- }
-
- $export($export.S + $export.F * !ALL_CONSTRUCTORS, 'Object', {
- getOwnPropertyDescriptor: $getDesc,
- defineProperty: $setDesc
- });
-
- if (fails(function () { arrayToString.call({}); })) {
- arrayToString = arrayToLocaleString = function toString() {
- return arrayJoin.call(this);
- };
- }
-
- var $TypedArrayPrototype$ = redefineAll({}, proto);
- redefineAll($TypedArrayPrototype$, $iterators);
- hide($TypedArrayPrototype$, ITERATOR, $iterators.values);
- redefineAll($TypedArrayPrototype$, {
- slice: $slice,
- set: $set,
- constructor: function () { /* noop */ },
- toString: arrayToString,
- toLocaleString: $toLocaleString
- });
- addGetter($TypedArrayPrototype$, 'buffer', 'b');
- addGetter($TypedArrayPrototype$, 'byteOffset', 'o');
- addGetter($TypedArrayPrototype$, 'byteLength', 'l');
- addGetter($TypedArrayPrototype$, 'length', 'e');
- dP($TypedArrayPrototype$, TAG, {
- get: function () { return this[TYPED_ARRAY]; }
- });
-
- // eslint-disable-next-line max-statements
- module.exports = function (KEY, BYTES, wrapper, CLAMPED) {
- CLAMPED = !!CLAMPED;
- var NAME = KEY + (CLAMPED ? 'Clamped' : '') + 'Array';
- var GETTER = 'get' + KEY;
- var SETTER = 'set' + KEY;
- var TypedArray = global[NAME];
- var Base = TypedArray || {};
- var TAC = TypedArray && getPrototypeOf(TypedArray);
- var FORCED = !TypedArray || !$typed.ABV;
- var O = {};
- var TypedArrayPrototype = TypedArray && TypedArray[PROTOTYPE];
- var getter = function (that, index) {
- var data = that._d;
- return data.v[GETTER](index * BYTES + data.o, LITTLE_ENDIAN);
- };
- var setter = function (that, index, value) {
- var data = that._d;
- if (CLAMPED) value = (value = Math.round(value)) < 0 ? 0 : value > 0xff ? 0xff : value & 0xff;
- data.v[SETTER](index * BYTES + data.o, value, LITTLE_ENDIAN);
- };
- var addElement = function (that, index) {
- dP(that, index, {
- get: function () {
- return getter(this, index);
- },
- set: function (value) {
- return setter(this, index, value);
- },
- enumerable: true
- });
- };
- if (FORCED) {
- TypedArray = wrapper(function (that, data, $offset, $length) {
- anInstance(that, TypedArray, NAME, '_d');
- var index = 0;
- var offset = 0;
- var buffer, byteLength, length, klass;
- if (!isObject(data)) {
- length = toIndex(data);
- byteLength = length * BYTES;
- buffer = new $ArrayBuffer(byteLength);
- } else if (data instanceof $ArrayBuffer || (klass = classof(data)) == ARRAY_BUFFER || klass == SHARED_BUFFER) {
- buffer = data;
- offset = toOffset($offset, BYTES);
- var $len = data.byteLength;
- if ($length === undefined) {
- if ($len % BYTES) throw RangeError(WRONG_LENGTH);
- byteLength = $len - offset;
- if (byteLength < 0) throw RangeError(WRONG_LENGTH);
- } else {
- byteLength = toLength($length) * BYTES;
- if (byteLength + offset > $len) throw RangeError(WRONG_LENGTH);
- }
- length = byteLength / BYTES;
- } else if (TYPED_ARRAY in data) {
- return fromList(TypedArray, data);
- } else {
- return $from.call(TypedArray, data);
- }
- hide(that, '_d', {
- b: buffer,
- o: offset,
- l: byteLength,
- e: length,
- v: new $DataView(buffer)
- });
- while (index < length) addElement(that, index++);
- });
- TypedArrayPrototype = TypedArray[PROTOTYPE] = create($TypedArrayPrototype$);
- hide(TypedArrayPrototype, 'constructor', TypedArray);
- } else if (!fails(function () {
- TypedArray(1);
- }) || !fails(function () {
- new TypedArray(-1); // eslint-disable-line no-new
- }) || !$iterDetect(function (iter) {
- new TypedArray(); // eslint-disable-line no-new
- new TypedArray(null); // eslint-disable-line no-new
- new TypedArray(1.5); // eslint-disable-line no-new
- new TypedArray(iter); // eslint-disable-line no-new
- }, true)) {
- TypedArray = wrapper(function (that, data, $offset, $length) {
- anInstance(that, TypedArray, NAME);
- var klass;
- // `ws` module bug, temporarily remove validation length for Uint8Array
- // https://github.com/websockets/ws/pull/645
- if (!isObject(data)) return new Base(toIndex(data));
- if (data instanceof $ArrayBuffer || (klass = classof(data)) == ARRAY_BUFFER || klass == SHARED_BUFFER) {
- return $length !== undefined
- ? new Base(data, toOffset($offset, BYTES), $length)
- : $offset !== undefined
- ? new Base(data, toOffset($offset, BYTES))
- : new Base(data);
- }
- if (TYPED_ARRAY in data) return fromList(TypedArray, data);
- return $from.call(TypedArray, data);
- });
- arrayForEach(TAC !== Function.prototype ? gOPN(Base).concat(gOPN(TAC)) : gOPN(Base), function (key) {
- if (!(key in TypedArray)) hide(TypedArray, key, Base[key]);
- });
- TypedArray[PROTOTYPE] = TypedArrayPrototype;
- if (!LIBRARY) TypedArrayPrototype.constructor = TypedArray;
- }
- var $nativeIterator = TypedArrayPrototype[ITERATOR];
- var CORRECT_ITER_NAME = !!$nativeIterator
- && ($nativeIterator.name == 'values' || $nativeIterator.name == undefined);
- var $iterator = $iterators.values;
- hide(TypedArray, TYPED_CONSTRUCTOR, true);
- hide(TypedArrayPrototype, TYPED_ARRAY, NAME);
- hide(TypedArrayPrototype, VIEW, true);
- hide(TypedArrayPrototype, DEF_CONSTRUCTOR, TypedArray);
-
- if (CLAMPED ? new TypedArray(1)[TAG] != NAME : !(TAG in TypedArrayPrototype)) {
- dP(TypedArrayPrototype, TAG, {
- get: function () { return NAME; }
- });
- }
-
- O[NAME] = TypedArray;
-
- $export($export.G + $export.W + $export.F * (TypedArray != Base), O);
-
- $export($export.S, NAME, {
- BYTES_PER_ELEMENT: BYTES
- });
-
- $export($export.S + $export.F * fails(function () { Base.of.call(TypedArray, 1); }), NAME, {
- from: $from,
- of: $of
- });
-
- if (!(BYTES_PER_ELEMENT in TypedArrayPrototype)) hide(TypedArrayPrototype, BYTES_PER_ELEMENT, BYTES);
-
- $export($export.P, NAME, proto);
-
- setSpecies(NAME);
-
- $export($export.P + $export.F * FORCED_SET, NAME, { set: $set });
-
- $export($export.P + $export.F * !CORRECT_ITER_NAME, NAME, $iterators);
-
- if (!LIBRARY && TypedArrayPrototype.toString != arrayToString) TypedArrayPrototype.toString = arrayToString;
-
- $export($export.P + $export.F * fails(function () {
- new TypedArray(1).slice();
- }), NAME, { slice: $slice });
-
- $export($export.P + $export.F * (fails(function () {
- return [1, 2].toLocaleString() != new TypedArray([1, 2]).toLocaleString();
- }) || !fails(function () {
- TypedArrayPrototype.toLocaleString.call([1, 2]);
- })), NAME, { toLocaleString: $toLocaleString });
-
- Iterators[NAME] = CORRECT_ITER_NAME ? $nativeIterator : $iterator;
- if (!LIBRARY && !CORRECT_ITER_NAME) hide(TypedArrayPrototype, ITERATOR, $iterator);
- };
- } else module.exports = function () { /* empty */ };
-
-
-/***/ }),
-/* 236 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(235)('Uint8', 1, function (init) {
- return function Uint8Array(data, byteOffset, length) {
- return init(this, data, byteOffset, length);
- };
- });
-
-
-/***/ }),
-/* 237 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(235)('Uint8', 1, function (init) {
- return function Uint8ClampedArray(data, byteOffset, length) {
- return init(this, data, byteOffset, length);
- };
- }, true);
-
-
-/***/ }),
-/* 238 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(235)('Int16', 2, function (init) {
- return function Int16Array(data, byteOffset, length) {
- return init(this, data, byteOffset, length);
- };
- });
-
-
-/***/ }),
-/* 239 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(235)('Uint16', 2, function (init) {
- return function Uint16Array(data, byteOffset, length) {
- return init(this, data, byteOffset, length);
- };
- });
-
-
-/***/ }),
-/* 240 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(235)('Int32', 4, function (init) {
- return function Int32Array(data, byteOffset, length) {
- return init(this, data, byteOffset, length);
- };
- });
-
-
-/***/ }),
-/* 241 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(235)('Uint32', 4, function (init) {
- return function Uint32Array(data, byteOffset, length) {
- return init(this, data, byteOffset, length);
- };
- });
-
-
-/***/ }),
-/* 242 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(235)('Float32', 4, function (init) {
- return function Float32Array(data, byteOffset, length) {
- return init(this, data, byteOffset, length);
- };
- });
-
-
-/***/ }),
-/* 243 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(235)('Float64', 8, function (init) {
- return function Float64Array(data, byteOffset, length) {
- return init(this, data, byteOffset, length);
- };
- });
-
-
-/***/ }),
-/* 244 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.1 Reflect.apply(target, thisArgument, argumentsList)
- var $export = __webpack_require__(8);
- var aFunction = __webpack_require__(24);
- var anObject = __webpack_require__(12);
- var rApply = (__webpack_require__(4).Reflect || {}).apply;
- var fApply = Function.apply;
- // MS Edge argumentsList argument is optional
- $export($export.S + $export.F * !__webpack_require__(7)(function () {
- rApply(function () { /* empty */ });
- }), 'Reflect', {
- apply: function apply(target, thisArgument, argumentsList) {
- var T = aFunction(target);
- var L = anObject(argumentsList);
- return rApply ? rApply(T, thisArgument, L) : fApply.call(T, thisArgument, L);
- }
- });
-
-
-/***/ }),
-/* 245 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.2 Reflect.construct(target, argumentsList [, newTarget])
- var $export = __webpack_require__(8);
- var create = __webpack_require__(47);
- var aFunction = __webpack_require__(24);
- var anObject = __webpack_require__(12);
- var isObject = __webpack_require__(13);
- var fails = __webpack_require__(7);
- var bind = __webpack_require__(77);
- var rConstruct = (__webpack_require__(4).Reflect || {}).construct;
-
- // MS Edge supports only 2 arguments and argumentsList argument is optional
- // FF Nightly sets third argument as `new.target`, but does not create `this` from it
- var NEW_TARGET_BUG = fails(function () {
- function F() { /* empty */ }
- return !(rConstruct(function () { /* empty */ }, [], F) instanceof F);
- });
- var ARGS_BUG = !fails(function () {
- rConstruct(function () { /* empty */ });
- });
-
- $export($export.S + $export.F * (NEW_TARGET_BUG || ARGS_BUG), 'Reflect', {
- construct: function construct(Target, args /* , newTarget */) {
- aFunction(Target);
- anObject(args);
- var newTarget = arguments.length < 3 ? Target : aFunction(arguments[2]);
- if (ARGS_BUG && !NEW_TARGET_BUG) return rConstruct(Target, args, newTarget);
- if (Target == newTarget) {
- // w/o altered newTarget, optimization for 0-4 arguments
- switch (args.length) {
- case 0: return new Target();
- case 1: return new Target(args[0]);
- case 2: return new Target(args[0], args[1]);
- case 3: return new Target(args[0], args[1], args[2]);
- case 4: return new Target(args[0], args[1], args[2], args[3]);
- }
- // w/o altered newTarget, lot of arguments case
- var $args = [null];
- $args.push.apply($args, args);
- return new (bind.apply(Target, $args))();
- }
- // with altered newTarget, not support built-in constructors
- var proto = newTarget.prototype;
- var instance = create(isObject(proto) ? proto : Object.prototype);
- var result = Function.apply.call(Target, instance, args);
- return isObject(result) ? result : instance;
- }
- });
-
-
-/***/ }),
-/* 246 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.3 Reflect.defineProperty(target, propertyKey, attributes)
- var dP = __webpack_require__(11);
- var $export = __webpack_require__(8);
- var anObject = __webpack_require__(12);
- var toPrimitive = __webpack_require__(16);
-
- // MS Edge has broken Reflect.defineProperty - throwing instead of returning false
- $export($export.S + $export.F * __webpack_require__(7)(function () {
- // eslint-disable-next-line no-undef
- Reflect.defineProperty(dP.f({}, 1, { value: 1 }), 1, { value: 2 });
- }), 'Reflect', {
- defineProperty: function defineProperty(target, propertyKey, attributes) {
- anObject(target);
- propertyKey = toPrimitive(propertyKey, true);
- anObject(attributes);
- try {
- dP.f(target, propertyKey, attributes);
- return true;
- } catch (e) {
- return false;
- }
- }
- });
-
-
-/***/ }),
-/* 247 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.4 Reflect.deleteProperty(target, propertyKey)
- var $export = __webpack_require__(8);
- var gOPD = __webpack_require__(52).f;
- var anObject = __webpack_require__(12);
-
- $export($export.S, 'Reflect', {
- deleteProperty: function deleteProperty(target, propertyKey) {
- var desc = gOPD(anObject(target), propertyKey);
- return desc && !desc.configurable ? false : delete target[propertyKey];
- }
- });
-
-
-/***/ }),
-/* 248 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // 26.1.5 Reflect.enumerate(target)
- var $export = __webpack_require__(8);
- var anObject = __webpack_require__(12);
- var Enumerate = function (iterated) {
- this._t = anObject(iterated); // target
- this._i = 0; // next index
- var keys = this._k = []; // keys
- var key;
- for (key in iterated) keys.push(key);
- };
- __webpack_require__(131)(Enumerate, 'Object', function () {
- var that = this;
- var keys = that._k;
- var key;
- do {
- if (that._i >= keys.length) return { value: undefined, done: true };
- } while (!((key = keys[that._i++]) in that._t));
- return { value: key, done: false };
- });
-
- $export($export.S, 'Reflect', {
- enumerate: function enumerate(target) {
- return new Enumerate(target);
- }
- });
-
-
-/***/ }),
-/* 249 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.6 Reflect.get(target, propertyKey [, receiver])
- var gOPD = __webpack_require__(52);
- var getPrototypeOf = __webpack_require__(59);
- var has = __webpack_require__(5);
- var $export = __webpack_require__(8);
- var isObject = __webpack_require__(13);
- var anObject = __webpack_require__(12);
-
- function get(target, propertyKey /* , receiver */) {
- var receiver = arguments.length < 3 ? target : arguments[2];
- var desc, proto;
- if (anObject(target) === receiver) return target[propertyKey];
- if (desc = gOPD.f(target, propertyKey)) return has(desc, 'value')
- ? desc.value
- : desc.get !== undefined
- ? desc.get.call(receiver)
- : undefined;
- if (isObject(proto = getPrototypeOf(target))) return get(proto, propertyKey, receiver);
- }
-
- $export($export.S, 'Reflect', { get: get });
-
-
-/***/ }),
-/* 250 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.7 Reflect.getOwnPropertyDescriptor(target, propertyKey)
- var gOPD = __webpack_require__(52);
- var $export = __webpack_require__(8);
- var anObject = __webpack_require__(12);
-
- $export($export.S, 'Reflect', {
- getOwnPropertyDescriptor: function getOwnPropertyDescriptor(target, propertyKey) {
- return gOPD.f(anObject(target), propertyKey);
- }
- });
-
-
-/***/ }),
-/* 251 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.8 Reflect.getPrototypeOf(target)
- var $export = __webpack_require__(8);
- var getProto = __webpack_require__(59);
- var anObject = __webpack_require__(12);
-
- $export($export.S, 'Reflect', {
- getPrototypeOf: function getPrototypeOf(target) {
- return getProto(anObject(target));
- }
- });
-
-
-/***/ }),
-/* 252 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.9 Reflect.has(target, propertyKey)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Reflect', {
- has: function has(target, propertyKey) {
- return propertyKey in target;
- }
- });
-
-
-/***/ }),
-/* 253 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.10 Reflect.isExtensible(target)
- var $export = __webpack_require__(8);
- var anObject = __webpack_require__(12);
- var $isExtensible = Object.isExtensible;
-
- $export($export.S, 'Reflect', {
- isExtensible: function isExtensible(target) {
- anObject(target);
- return $isExtensible ? $isExtensible(target) : true;
- }
- });
-
-
-/***/ }),
-/* 254 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.11 Reflect.ownKeys(target)
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Reflect', { ownKeys: __webpack_require__(255) });
-
-
-/***/ }),
-/* 255 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // all object keys, includes non-enumerable and symbols
- var gOPN = __webpack_require__(51);
- var gOPS = __webpack_require__(43);
- var anObject = __webpack_require__(12);
- var Reflect = __webpack_require__(4).Reflect;
- module.exports = Reflect && Reflect.ownKeys || function ownKeys(it) {
- var keys = gOPN.f(anObject(it));
- var getSymbols = gOPS.f;
- return getSymbols ? keys.concat(getSymbols(it)) : keys;
- };
-
-
-/***/ }),
-/* 256 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.12 Reflect.preventExtensions(target)
- var $export = __webpack_require__(8);
- var anObject = __webpack_require__(12);
- var $preventExtensions = Object.preventExtensions;
-
- $export($export.S, 'Reflect', {
- preventExtensions: function preventExtensions(target) {
- anObject(target);
- try {
- if ($preventExtensions) $preventExtensions(target);
- return true;
- } catch (e) {
- return false;
- }
- }
- });
-
-
-/***/ }),
-/* 257 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.13 Reflect.set(target, propertyKey, V [, receiver])
- var dP = __webpack_require__(11);
- var gOPD = __webpack_require__(52);
- var getPrototypeOf = __webpack_require__(59);
- var has = __webpack_require__(5);
- var $export = __webpack_require__(8);
- var createDesc = __webpack_require__(17);
- var anObject = __webpack_require__(12);
- var isObject = __webpack_require__(13);
-
- function set(target, propertyKey, V /* , receiver */) {
- var receiver = arguments.length < 4 ? target : arguments[3];
- var ownDesc = gOPD.f(anObject(target), propertyKey);
- var existingDescriptor, proto;
- if (!ownDesc) {
- if (isObject(proto = getPrototypeOf(target))) {
- return set(proto, propertyKey, V, receiver);
- }
- ownDesc = createDesc(0);
- }
- if (has(ownDesc, 'value')) {
- if (ownDesc.writable === false || !isObject(receiver)) return false;
- if (existingDescriptor = gOPD.f(receiver, propertyKey)) {
- if (existingDescriptor.get || existingDescriptor.set || existingDescriptor.writable === false) return false;
- existingDescriptor.value = V;
- dP.f(receiver, propertyKey, existingDescriptor);
- } else dP.f(receiver, propertyKey, createDesc(0, V));
- return true;
- }
- return ownDesc.set === undefined ? false : (ownDesc.set.call(receiver, V), true);
- }
-
- $export($export.S, 'Reflect', { set: set });
-
-
-/***/ }),
-/* 258 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // 26.1.14 Reflect.setPrototypeOf(target, proto)
- var $export = __webpack_require__(8);
- var setProto = __webpack_require__(73);
-
- if (setProto) $export($export.S, 'Reflect', {
- setPrototypeOf: function setPrototypeOf(target, proto) {
- setProto.check(target, proto);
- try {
- setProto.set(target, proto);
- return true;
- } catch (e) {
- return false;
- }
- }
- });
-
-
-/***/ }),
-/* 259 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://github.com/tc39/Array.prototype.includes
- var $export = __webpack_require__(8);
- var $includes = __webpack_require__(37)(true);
-
- $export($export.P, 'Array', {
- includes: function includes(el /* , fromIndex = 0 */) {
- return $includes(this, el, arguments.length > 1 ? arguments[1] : undefined);
- }
- });
-
- __webpack_require__(188)('includes');
-
-
-/***/ }),
-/* 260 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://tc39.github.io/proposal-flatMap/#sec-Array.prototype.flatMap
- var $export = __webpack_require__(8);
- var flattenIntoArray = __webpack_require__(261);
- var toObject = __webpack_require__(46);
- var toLength = __webpack_require__(38);
- var aFunction = __webpack_require__(24);
- var arraySpeciesCreate = __webpack_require__(175);
-
- $export($export.P, 'Array', {
- flatMap: function flatMap(callbackfn /* , thisArg */) {
- var O = toObject(this);
- var sourceLen, A;
- aFunction(callbackfn);
- sourceLen = toLength(O.length);
- A = arraySpeciesCreate(O, 0);
- flattenIntoArray(A, O, O, sourceLen, 0, 1, callbackfn, arguments[1]);
- return A;
- }
- });
-
- __webpack_require__(188)('flatMap');
-
-
-/***/ }),
-/* 261 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://tc39.github.io/proposal-flatMap/#sec-FlattenIntoArray
- var isArray = __webpack_require__(45);
- var isObject = __webpack_require__(13);
- var toLength = __webpack_require__(38);
- var ctx = __webpack_require__(23);
- var IS_CONCAT_SPREADABLE = __webpack_require__(27)('isConcatSpreadable');
-
- function flattenIntoArray(target, original, source, sourceLen, start, depth, mapper, thisArg) {
- var targetIndex = start;
- var sourceIndex = 0;
- var mapFn = mapper ? ctx(mapper, thisArg, 3) : false;
- var element, spreadable;
-
- while (sourceIndex < sourceLen) {
- if (sourceIndex in source) {
- element = mapFn ? mapFn(source[sourceIndex], sourceIndex, original) : source[sourceIndex];
-
- spreadable = false;
- if (isObject(element)) {
- spreadable = element[IS_CONCAT_SPREADABLE];
- spreadable = spreadable !== undefined ? !!spreadable : isArray(element);
- }
-
- if (spreadable && depth > 0) {
- targetIndex = flattenIntoArray(target, original, element, toLength(element.length), targetIndex, depth - 1) - 1;
- } else {
- if (targetIndex >= 0x1fffffffffffff) throw TypeError();
- target[targetIndex] = element;
- }
-
- targetIndex++;
- }
- sourceIndex++;
- }
- return targetIndex;
- }
-
- module.exports = flattenIntoArray;
-
-
-/***/ }),
-/* 262 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://tc39.github.io/proposal-flatMap/#sec-Array.prototype.flatten
- var $export = __webpack_require__(8);
- var flattenIntoArray = __webpack_require__(261);
- var toObject = __webpack_require__(46);
- var toLength = __webpack_require__(38);
- var toInteger = __webpack_require__(39);
- var arraySpeciesCreate = __webpack_require__(175);
-
- $export($export.P, 'Array', {
- flatten: function flatten(/* depthArg = 1 */) {
- var depthArg = arguments[0];
- var O = toObject(this);
- var sourceLen = toLength(O.length);
- var A = arraySpeciesCreate(O, 0);
- flattenIntoArray(A, O, O, sourceLen, 0, depthArg === undefined ? 1 : toInteger(depthArg));
- return A;
- }
- });
-
- __webpack_require__(188)('flatten');
-
-
-/***/ }),
-/* 263 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://github.com/mathiasbynens/String.prototype.at
- var $export = __webpack_require__(8);
- var $at = __webpack_require__(128)(true);
-
- $export($export.P, 'String', {
- at: function at(pos) {
- return $at(this, pos);
- }
- });
-
-
-/***/ }),
-/* 264 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://github.com/tc39/proposal-string-pad-start-end
- var $export = __webpack_require__(8);
- var $pad = __webpack_require__(265);
- var userAgent = __webpack_require__(218);
-
- // https://github.com/zloirock/core-js/issues/280
- var WEBKIT_BUG = /Version\/10\.\d+(\.\d+)?( Mobile\/\w+)? Safari\//.test(userAgent);
-
- $export($export.P + $export.F * WEBKIT_BUG, 'String', {
- padStart: function padStart(maxLength /* , fillString = ' ' */) {
- return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, true);
- }
- });
-
-
-/***/ }),
-/* 265 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/tc39/proposal-string-pad-start-end
- var toLength = __webpack_require__(38);
- var repeat = __webpack_require__(91);
- var defined = __webpack_require__(36);
-
- module.exports = function (that, maxLength, fillString, left) {
- var S = String(defined(that));
- var stringLength = S.length;
- var fillStr = fillString === undefined ? ' ' : String(fillString);
- var intMaxLength = toLength(maxLength);
- if (intMaxLength <= stringLength || fillStr == '') return S;
- var fillLen = intMaxLength - stringLength;
- var stringFiller = repeat.call(fillStr, Math.ceil(fillLen / fillStr.length));
- if (stringFiller.length > fillLen) stringFiller = stringFiller.slice(0, fillLen);
- return left ? stringFiller + S : S + stringFiller;
- };
-
-
-/***/ }),
-/* 266 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://github.com/tc39/proposal-string-pad-start-end
- var $export = __webpack_require__(8);
- var $pad = __webpack_require__(265);
- var userAgent = __webpack_require__(218);
-
- // https://github.com/zloirock/core-js/issues/280
- var WEBKIT_BUG = /Version\/10\.\d+(\.\d+)?( Mobile\/\w+)? Safari\//.test(userAgent);
-
- $export($export.P + $export.F * WEBKIT_BUG, 'String', {
- padEnd: function padEnd(maxLength /* , fillString = ' ' */) {
- return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, false);
- }
- });
-
-
-/***/ }),
-/* 267 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://github.com/sebmarkbage/ecmascript-string-left-right-trim
- __webpack_require__(83)('trimLeft', function ($trim) {
- return function trimLeft() {
- return $trim(this, 1);
- };
- }, 'trimStart');
-
-
-/***/ }),
-/* 268 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://github.com/sebmarkbage/ecmascript-string-left-right-trim
- __webpack_require__(83)('trimRight', function ($trim) {
- return function trimRight() {
- return $trim(this, 2);
- };
- }, 'trimEnd');
-
-
-/***/ }),
-/* 269 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://tc39.github.io/String.prototype.matchAll/
- var $export = __webpack_require__(8);
- var defined = __webpack_require__(36);
- var toLength = __webpack_require__(38);
- var isRegExp = __webpack_require__(135);
- var getFlags = __webpack_require__(198);
- var RegExpProto = RegExp.prototype;
-
- var $RegExpStringIterator = function (regexp, string) {
- this._r = regexp;
- this._s = string;
- };
-
- __webpack_require__(131)($RegExpStringIterator, 'RegExp String', function next() {
- var match = this._r.exec(this._s);
- return { value: match, done: match === null };
- });
-
- $export($export.P, 'String', {
- matchAll: function matchAll(regexp) {
- defined(this);
- if (!isRegExp(regexp)) throw TypeError(regexp + ' is not a regexp!');
- var S = String(this);
- var flags = 'flags' in RegExpProto ? String(regexp.flags) : getFlags.call(regexp);
- var rx = new RegExp(regexp.source, ~flags.indexOf('g') ? flags : 'g' + flags);
- rx.lastIndex = toLength(regexp.lastIndex);
- return new $RegExpStringIterator(rx, S);
- }
- });
-
-
-/***/ }),
-/* 270 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(29)('asyncIterator');
-
-
-/***/ }),
-/* 271 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(29)('observable');
-
-
-/***/ }),
-/* 272 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/tc39/proposal-object-getownpropertydescriptors
- var $export = __webpack_require__(8);
- var ownKeys = __webpack_require__(255);
- var toIObject = __webpack_require__(33);
- var gOPD = __webpack_require__(52);
- var createProperty = __webpack_require__(165);
-
- $export($export.S, 'Object', {
- getOwnPropertyDescriptors: function getOwnPropertyDescriptors(object) {
- var O = toIObject(object);
- var getDesc = gOPD.f;
- var keys = ownKeys(O);
- var result = {};
- var i = 0;
- var key, desc;
- while (keys.length > i) {
- desc = getDesc(O, key = keys[i++]);
- if (desc !== undefined) createProperty(result, key, desc);
- }
- return result;
- }
- });
-
-
-/***/ }),
-/* 273 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/tc39/proposal-object-values-entries
- var $export = __webpack_require__(8);
- var $values = __webpack_require__(274)(false);
-
- $export($export.S, 'Object', {
- values: function values(it) {
- return $values(it);
- }
- });
-
-
-/***/ }),
-/* 274 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var DESCRIPTORS = __webpack_require__(6);
- var getKeys = __webpack_require__(31);
- var toIObject = __webpack_require__(33);
- var isEnum = __webpack_require__(44).f;
- module.exports = function (isEntries) {
- return function (it) {
- var O = toIObject(it);
- var keys = getKeys(O);
- var length = keys.length;
- var i = 0;
- var result = [];
- var key;
- while (length > i) {
- key = keys[i++];
- if (!DESCRIPTORS || isEnum.call(O, key)) {
- result.push(isEntries ? [key, O[key]] : O[key]);
- }
- }
- return result;
- };
- };
-
-
-/***/ }),
-/* 275 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/tc39/proposal-object-values-entries
- var $export = __webpack_require__(8);
- var $entries = __webpack_require__(274)(true);
-
- $export($export.S, 'Object', {
- entries: function entries(it) {
- return $entries(it);
- }
- });
-
-
-/***/ }),
-/* 276 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var toObject = __webpack_require__(46);
- var aFunction = __webpack_require__(24);
- var $defineProperty = __webpack_require__(11);
-
- // B.2.2.2 Object.prototype.__defineGetter__(P, getter)
- __webpack_require__(6) && $export($export.P + __webpack_require__(277), 'Object', {
- __defineGetter__: function __defineGetter__(P, getter) {
- $defineProperty.f(toObject(this), P, { get: aFunction(getter), enumerable: true, configurable: true });
- }
- });
-
-
-/***/ }),
-/* 277 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // Forced replacement prototype accessors methods
- module.exports = __webpack_require__(22) || !__webpack_require__(7)(function () {
- var K = Math.random();
- // In FF throws only define methods
- // eslint-disable-next-line no-undef, no-useless-call
- __defineSetter__.call(null, K, function () { /* empty */ });
- delete __webpack_require__(4)[K];
- });
-
-
-/***/ }),
-/* 278 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var toObject = __webpack_require__(46);
- var aFunction = __webpack_require__(24);
- var $defineProperty = __webpack_require__(11);
-
- // B.2.2.3 Object.prototype.__defineSetter__(P, setter)
- __webpack_require__(6) && $export($export.P + __webpack_require__(277), 'Object', {
- __defineSetter__: function __defineSetter__(P, setter) {
- $defineProperty.f(toObject(this), P, { set: aFunction(setter), enumerable: true, configurable: true });
- }
- });
-
-
-/***/ }),
-/* 279 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var toObject = __webpack_require__(46);
- var toPrimitive = __webpack_require__(16);
- var getPrototypeOf = __webpack_require__(59);
- var getOwnPropertyDescriptor = __webpack_require__(52).f;
-
- // B.2.2.4 Object.prototype.__lookupGetter__(P)
- __webpack_require__(6) && $export($export.P + __webpack_require__(277), 'Object', {
- __lookupGetter__: function __lookupGetter__(P) {
- var O = toObject(this);
- var K = toPrimitive(P, true);
- var D;
- do {
- if (D = getOwnPropertyDescriptor(O, K)) return D.get;
- } while (O = getPrototypeOf(O));
- }
- });
-
-
-/***/ }),
-/* 280 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- var $export = __webpack_require__(8);
- var toObject = __webpack_require__(46);
- var toPrimitive = __webpack_require__(16);
- var getPrototypeOf = __webpack_require__(59);
- var getOwnPropertyDescriptor = __webpack_require__(52).f;
-
- // B.2.2.5 Object.prototype.__lookupSetter__(P)
- __webpack_require__(6) && $export($export.P + __webpack_require__(277), 'Object', {
- __lookupSetter__: function __lookupSetter__(P) {
- var O = toObject(this);
- var K = toPrimitive(P, true);
- var D;
- do {
- if (D = getOwnPropertyDescriptor(O, K)) return D.set;
- } while (O = getPrototypeOf(O));
- }
- });
-
-
-/***/ }),
-/* 281 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/DavidBruant/Map-Set.prototype.toJSON
- var $export = __webpack_require__(8);
-
- $export($export.P + $export.R, 'Map', { toJSON: __webpack_require__(282)('Map') });
-
-
-/***/ }),
-/* 282 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/DavidBruant/Map-Set.prototype.toJSON
- var classof = __webpack_require__(75);
- var from = __webpack_require__(283);
- module.exports = function (NAME) {
- return function toJSON() {
- if (classof(this) != NAME) throw TypeError(NAME + "#toJSON isn't generic");
- return from(this);
- };
- };
-
-
-/***/ }),
-/* 283 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var forOf = __webpack_require__(213);
-
- module.exports = function (iter, ITERATOR) {
- var result = [];
- forOf(iter, false, result.push, result, ITERATOR);
- return result;
- };
-
-
-/***/ }),
-/* 284 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/DavidBruant/Map-Set.prototype.toJSON
- var $export = __webpack_require__(8);
-
- $export($export.P + $export.R, 'Set', { toJSON: __webpack_require__(282)('Set') });
-
-
-/***/ }),
-/* 285 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://tc39.github.io/proposal-setmap-offrom/#sec-map.of
- __webpack_require__(286)('Map');
-
-
-/***/ }),
-/* 286 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://tc39.github.io/proposal-setmap-offrom/
- var $export = __webpack_require__(8);
-
- module.exports = function (COLLECTION) {
- $export($export.S, COLLECTION, { of: function of() {
- var length = arguments.length;
- var A = new Array(length);
- while (length--) A[length] = arguments[length];
- return new this(A);
- } });
- };
-
-
-/***/ }),
-/* 287 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://tc39.github.io/proposal-setmap-offrom/#sec-set.of
- __webpack_require__(286)('Set');
-
-
-/***/ }),
-/* 288 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://tc39.github.io/proposal-setmap-offrom/#sec-weakmap.of
- __webpack_require__(286)('WeakMap');
-
-
-/***/ }),
-/* 289 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://tc39.github.io/proposal-setmap-offrom/#sec-weakset.of
- __webpack_require__(286)('WeakSet');
-
-
-/***/ }),
-/* 290 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://tc39.github.io/proposal-setmap-offrom/#sec-map.from
- __webpack_require__(291)('Map');
-
-
-/***/ }),
-/* 291 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://tc39.github.io/proposal-setmap-offrom/
- var $export = __webpack_require__(8);
- var aFunction = __webpack_require__(24);
- var ctx = __webpack_require__(23);
- var forOf = __webpack_require__(213);
-
- module.exports = function (COLLECTION) {
- $export($export.S, COLLECTION, { from: function from(source /* , mapFn, thisArg */) {
- var mapFn = arguments[1];
- var mapping, A, n, cb;
- aFunction(this);
- mapping = mapFn !== undefined;
- if (mapping) aFunction(mapFn);
- if (source == undefined) return new this();
- A = [];
- if (mapping) {
- n = 0;
- cb = ctx(mapFn, arguments[2], 2);
- forOf(source, false, function (nextItem) {
- A.push(cb(nextItem, n++));
- });
- } else {
- forOf(source, false, A.push, A);
- }
- return new this(A);
- } });
- };
-
-
-/***/ }),
-/* 292 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://tc39.github.io/proposal-setmap-offrom/#sec-set.from
- __webpack_require__(291)('Set');
-
-
-/***/ }),
-/* 293 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://tc39.github.io/proposal-setmap-offrom/#sec-weakmap.from
- __webpack_require__(291)('WeakMap');
-
-
-/***/ }),
-/* 294 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://tc39.github.io/proposal-setmap-offrom/#sec-weakset.from
- __webpack_require__(291)('WeakSet');
-
-
-/***/ }),
-/* 295 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/tc39/proposal-global
- var $export = __webpack_require__(8);
-
- $export($export.G, { global: __webpack_require__(4) });
-
-
-/***/ }),
-/* 296 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/tc39/proposal-global
- var $export = __webpack_require__(8);
-
- $export($export.S, 'System', { global: __webpack_require__(4) });
-
-
-/***/ }),
-/* 297 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/ljharb/proposal-is-error
- var $export = __webpack_require__(8);
- var cof = __webpack_require__(35);
-
- $export($export.S, 'Error', {
- isError: function isError(it) {
- return cof(it) === 'Error';
- }
- });
-
-
-/***/ }),
-/* 298 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://rwaldron.github.io/proposal-math-extensions/
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', {
- clamp: function clamp(x, lower, upper) {
- return Math.min(upper, Math.max(lower, x));
- }
- });
-
-
-/***/ }),
-/* 299 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://rwaldron.github.io/proposal-math-extensions/
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', { DEG_PER_RAD: Math.PI / 180 });
-
-
-/***/ }),
-/* 300 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://rwaldron.github.io/proposal-math-extensions/
- var $export = __webpack_require__(8);
- var RAD_PER_DEG = 180 / Math.PI;
-
- $export($export.S, 'Math', {
- degrees: function degrees(radians) {
- return radians * RAD_PER_DEG;
- }
- });
-
-
-/***/ }),
-/* 301 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://rwaldron.github.io/proposal-math-extensions/
- var $export = __webpack_require__(8);
- var scale = __webpack_require__(302);
- var fround = __webpack_require__(114);
-
- $export($export.S, 'Math', {
- fscale: function fscale(x, inLow, inHigh, outLow, outHigh) {
- return fround(scale(x, inLow, inHigh, outLow, outHigh));
- }
- });
-
-
-/***/ }),
-/* 302 */
-/***/ (function(module, exports) {
-
- // https://rwaldron.github.io/proposal-math-extensions/
- module.exports = Math.scale || function scale(x, inLow, inHigh, outLow, outHigh) {
- if (
- arguments.length === 0
- // eslint-disable-next-line no-self-compare
- || x != x
- // eslint-disable-next-line no-self-compare
- || inLow != inLow
- // eslint-disable-next-line no-self-compare
- || inHigh != inHigh
- // eslint-disable-next-line no-self-compare
- || outLow != outLow
- // eslint-disable-next-line no-self-compare
- || outHigh != outHigh
- ) return NaN;
- if (x === Infinity || x === -Infinity) return x;
- return (x - inLow) * (outHigh - outLow) / (inHigh - inLow) + outLow;
- };
-
-
-/***/ }),
-/* 303 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://gist.github.com/BrendanEich/4294d5c212a6d2254703
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', {
- iaddh: function iaddh(x0, x1, y0, y1) {
- var $x0 = x0 >>> 0;
- var $x1 = x1 >>> 0;
- var $y0 = y0 >>> 0;
- return $x1 + (y1 >>> 0) + (($x0 & $y0 | ($x0 | $y0) & ~($x0 + $y0 >>> 0)) >>> 31) | 0;
- }
- });
-
-
-/***/ }),
-/* 304 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://gist.github.com/BrendanEich/4294d5c212a6d2254703
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', {
- isubh: function isubh(x0, x1, y0, y1) {
- var $x0 = x0 >>> 0;
- var $x1 = x1 >>> 0;
- var $y0 = y0 >>> 0;
- return $x1 - (y1 >>> 0) - ((~$x0 & $y0 | ~($x0 ^ $y0) & $x0 - $y0 >>> 0) >>> 31) | 0;
- }
- });
-
-
-/***/ }),
-/* 305 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://gist.github.com/BrendanEich/4294d5c212a6d2254703
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', {
- imulh: function imulh(u, v) {
- var UINT16 = 0xffff;
- var $u = +u;
- var $v = +v;
- var u0 = $u & UINT16;
- var v0 = $v & UINT16;
- var u1 = $u >> 16;
- var v1 = $v >> 16;
- var t = (u1 * v0 >>> 0) + (u0 * v0 >>> 16);
- return u1 * v1 + (t >> 16) + ((u0 * v1 >>> 0) + (t & UINT16) >> 16);
- }
- });
-
-
-/***/ }),
-/* 306 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://rwaldron.github.io/proposal-math-extensions/
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', { RAD_PER_DEG: 180 / Math.PI });
-
-
-/***/ }),
-/* 307 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://rwaldron.github.io/proposal-math-extensions/
- var $export = __webpack_require__(8);
- var DEG_PER_RAD = Math.PI / 180;
-
- $export($export.S, 'Math', {
- radians: function radians(degrees) {
- return degrees * DEG_PER_RAD;
- }
- });
-
-
-/***/ }),
-/* 308 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://rwaldron.github.io/proposal-math-extensions/
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', { scale: __webpack_require__(302) });
-
-
-/***/ }),
-/* 309 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://gist.github.com/BrendanEich/4294d5c212a6d2254703
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', {
- umulh: function umulh(u, v) {
- var UINT16 = 0xffff;
- var $u = +u;
- var $v = +v;
- var u0 = $u & UINT16;
- var v0 = $v & UINT16;
- var u1 = $u >>> 16;
- var v1 = $v >>> 16;
- var t = (u1 * v0 >>> 0) + (u0 * v0 >>> 16);
- return u1 * v1 + (t >>> 16) + ((u0 * v1 >>> 0) + (t & UINT16) >>> 16);
- }
- });
-
-
-/***/ }),
-/* 310 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // http://jfbastien.github.io/papers/Math.signbit.html
- var $export = __webpack_require__(8);
-
- $export($export.S, 'Math', { signbit: function signbit(x) {
- // eslint-disable-next-line no-self-compare
- return (x = +x) != x ? x : x == 0 ? 1 / x == Infinity : x > 0;
- } });
-
-
-/***/ }),
-/* 311 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/tc39/proposal-promise-finally
- 'use strict';
- var $export = __webpack_require__(8);
- var core = __webpack_require__(9);
- var global = __webpack_require__(4);
- var speciesConstructor = __webpack_require__(210);
- var promiseResolve = __webpack_require__(219);
-
- $export($export.P + $export.R, 'Promise', { 'finally': function (onFinally) {
- var C = speciesConstructor(this, core.Promise || global.Promise);
- var isFunction = typeof onFinally == 'function';
- return this.then(
- isFunction ? function (x) {
- return promiseResolve(C, onFinally()).then(function () { return x; });
- } : onFinally,
- isFunction ? function (e) {
- return promiseResolve(C, onFinally()).then(function () { throw e; });
- } : onFinally
- );
- } });
-
-
-/***/ }),
-/* 312 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://github.com/tc39/proposal-promise-try
- var $export = __webpack_require__(8);
- var newPromiseCapability = __webpack_require__(216);
- var perform = __webpack_require__(217);
-
- $export($export.S, 'Promise', { 'try': function (callbackfn) {
- var promiseCapability = newPromiseCapability.f(this);
- var result = perform(callbackfn);
- (result.e ? promiseCapability.reject : promiseCapability.resolve)(result.v);
- return promiseCapability.promise;
- } });
-
-
-/***/ }),
-/* 313 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var metadata = __webpack_require__(314);
- var anObject = __webpack_require__(12);
- var toMetaKey = metadata.key;
- var ordinaryDefineOwnMetadata = metadata.set;
-
- metadata.exp({ defineMetadata: function defineMetadata(metadataKey, metadataValue, target, targetKey) {
- ordinaryDefineOwnMetadata(metadataKey, metadataValue, anObject(target), toMetaKey(targetKey));
- } });
-
-
-/***/ }),
-/* 314 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var Map = __webpack_require__(221);
- var $export = __webpack_require__(8);
- var shared = __webpack_require__(21)('metadata');
- var store = shared.store || (shared.store = new (__webpack_require__(226))());
-
- var getOrCreateMetadataMap = function (target, targetKey, create) {
- var targetMetadata = store.get(target);
- if (!targetMetadata) {
- if (!create) return undefined;
- store.set(target, targetMetadata = new Map());
- }
- var keyMetadata = targetMetadata.get(targetKey);
- if (!keyMetadata) {
- if (!create) return undefined;
- targetMetadata.set(targetKey, keyMetadata = new Map());
- } return keyMetadata;
- };
- var ordinaryHasOwnMetadata = function (MetadataKey, O, P) {
- var metadataMap = getOrCreateMetadataMap(O, P, false);
- return metadataMap === undefined ? false : metadataMap.has(MetadataKey);
- };
- var ordinaryGetOwnMetadata = function (MetadataKey, O, P) {
- var metadataMap = getOrCreateMetadataMap(O, P, false);
- return metadataMap === undefined ? undefined : metadataMap.get(MetadataKey);
- };
- var ordinaryDefineOwnMetadata = function (MetadataKey, MetadataValue, O, P) {
- getOrCreateMetadataMap(O, P, true).set(MetadataKey, MetadataValue);
- };
- var ordinaryOwnMetadataKeys = function (target, targetKey) {
- var metadataMap = getOrCreateMetadataMap(target, targetKey, false);
- var keys = [];
- if (metadataMap) metadataMap.forEach(function (_, key) { keys.push(key); });
- return keys;
- };
- var toMetaKey = function (it) {
- return it === undefined || typeof it == 'symbol' ? it : String(it);
- };
- var exp = function (O) {
- $export($export.S, 'Reflect', O);
- };
-
- module.exports = {
- store: store,
- map: getOrCreateMetadataMap,
- has: ordinaryHasOwnMetadata,
- get: ordinaryGetOwnMetadata,
- set: ordinaryDefineOwnMetadata,
- keys: ordinaryOwnMetadataKeys,
- key: toMetaKey,
- exp: exp
- };
-
-
-/***/ }),
-/* 315 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var metadata = __webpack_require__(314);
- var anObject = __webpack_require__(12);
- var toMetaKey = metadata.key;
- var getOrCreateMetadataMap = metadata.map;
- var store = metadata.store;
-
- metadata.exp({ deleteMetadata: function deleteMetadata(metadataKey, target /* , targetKey */) {
- var targetKey = arguments.length < 3 ? undefined : toMetaKey(arguments[2]);
- var metadataMap = getOrCreateMetadataMap(anObject(target), targetKey, false);
- if (metadataMap === undefined || !metadataMap['delete'](metadataKey)) return false;
- if (metadataMap.size) return true;
- var targetMetadata = store.get(target);
- targetMetadata['delete'](targetKey);
- return !!targetMetadata.size || store['delete'](target);
- } });
-
-
-/***/ }),
-/* 316 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var metadata = __webpack_require__(314);
- var anObject = __webpack_require__(12);
- var getPrototypeOf = __webpack_require__(59);
- var ordinaryHasOwnMetadata = metadata.has;
- var ordinaryGetOwnMetadata = metadata.get;
- var toMetaKey = metadata.key;
-
- var ordinaryGetMetadata = function (MetadataKey, O, P) {
- var hasOwn = ordinaryHasOwnMetadata(MetadataKey, O, P);
- if (hasOwn) return ordinaryGetOwnMetadata(MetadataKey, O, P);
- var parent = getPrototypeOf(O);
- return parent !== null ? ordinaryGetMetadata(MetadataKey, parent, P) : undefined;
- };
-
- metadata.exp({ getMetadata: function getMetadata(metadataKey, target /* , targetKey */) {
- return ordinaryGetMetadata(metadataKey, anObject(target), arguments.length < 3 ? undefined : toMetaKey(arguments[2]));
- } });
-
-
-/***/ }),
-/* 317 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var Set = __webpack_require__(225);
- var from = __webpack_require__(283);
- var metadata = __webpack_require__(314);
- var anObject = __webpack_require__(12);
- var getPrototypeOf = __webpack_require__(59);
- var ordinaryOwnMetadataKeys = metadata.keys;
- var toMetaKey = metadata.key;
-
- var ordinaryMetadataKeys = function (O, P) {
- var oKeys = ordinaryOwnMetadataKeys(O, P);
- var parent = getPrototypeOf(O);
- if (parent === null) return oKeys;
- var pKeys = ordinaryMetadataKeys(parent, P);
- return pKeys.length ? oKeys.length ? from(new Set(oKeys.concat(pKeys))) : pKeys : oKeys;
- };
-
- metadata.exp({ getMetadataKeys: function getMetadataKeys(target /* , targetKey */) {
- return ordinaryMetadataKeys(anObject(target), arguments.length < 2 ? undefined : toMetaKey(arguments[1]));
- } });
-
-
-/***/ }),
-/* 318 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var metadata = __webpack_require__(314);
- var anObject = __webpack_require__(12);
- var ordinaryGetOwnMetadata = metadata.get;
- var toMetaKey = metadata.key;
-
- metadata.exp({ getOwnMetadata: function getOwnMetadata(metadataKey, target /* , targetKey */) {
- return ordinaryGetOwnMetadata(metadataKey, anObject(target)
- , arguments.length < 3 ? undefined : toMetaKey(arguments[2]));
- } });
-
-
-/***/ }),
-/* 319 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var metadata = __webpack_require__(314);
- var anObject = __webpack_require__(12);
- var ordinaryOwnMetadataKeys = metadata.keys;
- var toMetaKey = metadata.key;
-
- metadata.exp({ getOwnMetadataKeys: function getOwnMetadataKeys(target /* , targetKey */) {
- return ordinaryOwnMetadataKeys(anObject(target), arguments.length < 2 ? undefined : toMetaKey(arguments[1]));
- } });
-
-
-/***/ }),
-/* 320 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var metadata = __webpack_require__(314);
- var anObject = __webpack_require__(12);
- var getPrototypeOf = __webpack_require__(59);
- var ordinaryHasOwnMetadata = metadata.has;
- var toMetaKey = metadata.key;
-
- var ordinaryHasMetadata = function (MetadataKey, O, P) {
- var hasOwn = ordinaryHasOwnMetadata(MetadataKey, O, P);
- if (hasOwn) return true;
- var parent = getPrototypeOf(O);
- return parent !== null ? ordinaryHasMetadata(MetadataKey, parent, P) : false;
- };
-
- metadata.exp({ hasMetadata: function hasMetadata(metadataKey, target /* , targetKey */) {
- return ordinaryHasMetadata(metadataKey, anObject(target), arguments.length < 3 ? undefined : toMetaKey(arguments[2]));
- } });
-
-
-/***/ }),
-/* 321 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var metadata = __webpack_require__(314);
- var anObject = __webpack_require__(12);
- var ordinaryHasOwnMetadata = metadata.has;
- var toMetaKey = metadata.key;
-
- metadata.exp({ hasOwnMetadata: function hasOwnMetadata(metadataKey, target /* , targetKey */) {
- return ordinaryHasOwnMetadata(metadataKey, anObject(target)
- , arguments.length < 3 ? undefined : toMetaKey(arguments[2]));
- } });
-
-
-/***/ }),
-/* 322 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $metadata = __webpack_require__(314);
- var anObject = __webpack_require__(12);
- var aFunction = __webpack_require__(24);
- var toMetaKey = $metadata.key;
- var ordinaryDefineOwnMetadata = $metadata.set;
-
- $metadata.exp({ metadata: function metadata(metadataKey, metadataValue) {
- return function decorator(target, targetKey) {
- ordinaryDefineOwnMetadata(
- metadataKey, metadataValue,
- (targetKey !== undefined ? anObject : aFunction)(target),
- toMetaKey(targetKey)
- );
- };
- } });
-
-
-/***/ }),
-/* 323 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/rwaldron/tc39-notes/blob/master/es6/2014-09/sept-25.md#510-globalasap-for-enqueuing-a-microtask
- var $export = __webpack_require__(8);
- var microtask = __webpack_require__(215)();
- var process = __webpack_require__(4).process;
- var isNode = __webpack_require__(35)(process) == 'process';
-
- $export($export.G, {
- asap: function asap(fn) {
- var domain = isNode && process.domain;
- microtask(domain ? domain.bind(fn) : fn);
- }
- });
-
-
-/***/ }),
-/* 324 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
- // https://github.com/zenparsing/es-observable
- var $export = __webpack_require__(8);
- var global = __webpack_require__(4);
- var core = __webpack_require__(9);
- var microtask = __webpack_require__(215)();
- var OBSERVABLE = __webpack_require__(27)('observable');
- var aFunction = __webpack_require__(24);
- var anObject = __webpack_require__(12);
- var anInstance = __webpack_require__(212);
- var redefineAll = __webpack_require__(220);
- var hide = __webpack_require__(10);
- var forOf = __webpack_require__(213);
- var RETURN = forOf.RETURN;
-
- var getMethod = function (fn) {
- return fn == null ? undefined : aFunction(fn);
- };
-
- var cleanupSubscription = function (subscription) {
- var cleanup = subscription._c;
- if (cleanup) {
- subscription._c = undefined;
- cleanup();
- }
- };
-
- var subscriptionClosed = function (subscription) {
- return subscription._o === undefined;
- };
-
- var closeSubscription = function (subscription) {
- if (!subscriptionClosed(subscription)) {
- subscription._o = undefined;
- cleanupSubscription(subscription);
- }
- };
-
- var Subscription = function (observer, subscriber) {
- anObject(observer);
- this._c = undefined;
- this._o = observer;
- observer = new SubscriptionObserver(this);
- try {
- var cleanup = subscriber(observer);
- var subscription = cleanup;
- if (cleanup != null) {
- if (typeof cleanup.unsubscribe === 'function') cleanup = function () { subscription.unsubscribe(); };
- else aFunction(cleanup);
- this._c = cleanup;
- }
- } catch (e) {
- observer.error(e);
- return;
- } if (subscriptionClosed(this)) cleanupSubscription(this);
- };
-
- Subscription.prototype = redefineAll({}, {
- unsubscribe: function unsubscribe() { closeSubscription(this); }
- });
-
- var SubscriptionObserver = function (subscription) {
- this._s = subscription;
- };
-
- SubscriptionObserver.prototype = redefineAll({}, {
- next: function next(value) {
- var subscription = this._s;
- if (!subscriptionClosed(subscription)) {
- var observer = subscription._o;
- try {
- var m = getMethod(observer.next);
- if (m) return m.call(observer, value);
- } catch (e) {
- try {
- closeSubscription(subscription);
- } finally {
- throw e;
- }
- }
- }
- },
- error: function error(value) {
- var subscription = this._s;
- if (subscriptionClosed(subscription)) throw value;
- var observer = subscription._o;
- subscription._o = undefined;
- try {
- var m = getMethod(observer.error);
- if (!m) throw value;
- value = m.call(observer, value);
- } catch (e) {
- try {
- cleanupSubscription(subscription);
- } finally {
- throw e;
- }
- } cleanupSubscription(subscription);
- return value;
- },
- complete: function complete(value) {
- var subscription = this._s;
- if (!subscriptionClosed(subscription)) {
- var observer = subscription._o;
- subscription._o = undefined;
- try {
- var m = getMethod(observer.complete);
- value = m ? m.call(observer, value) : undefined;
- } catch (e) {
- try {
- cleanupSubscription(subscription);
- } finally {
- throw e;
- }
- } cleanupSubscription(subscription);
- return value;
- }
- }
- });
-
- var $Observable = function Observable(subscriber) {
- anInstance(this, $Observable, 'Observable', '_f')._f = aFunction(subscriber);
- };
-
- redefineAll($Observable.prototype, {
- subscribe: function subscribe(observer) {
- return new Subscription(observer, this._f);
- },
- forEach: function forEach(fn) {
- var that = this;
- return new (core.Promise || global.Promise)(function (resolve, reject) {
- aFunction(fn);
- var subscription = that.subscribe({
- next: function (value) {
- try {
- return fn(value);
- } catch (e) {
- reject(e);
- subscription.unsubscribe();
- }
- },
- error: reject,
- complete: resolve
- });
- });
- }
- });
-
- redefineAll($Observable, {
- from: function from(x) {
- var C = typeof this === 'function' ? this : $Observable;
- var method = getMethod(anObject(x)[OBSERVABLE]);
- if (method) {
- var observable = anObject(method.call(x));
- return observable.constructor === C ? observable : new C(function (observer) {
- return observable.subscribe(observer);
- });
- }
- return new C(function (observer) {
- var done = false;
- microtask(function () {
- if (!done) {
- try {
- if (forOf(x, false, function (it) {
- observer.next(it);
- if (done) return RETURN;
- }) === RETURN) return;
- } catch (e) {
- if (done) throw e;
- observer.error(e);
- return;
- } observer.complete();
- }
- });
- return function () { done = true; };
- });
- },
- of: function of() {
- for (var i = 0, l = arguments.length, items = new Array(l); i < l;) items[i] = arguments[i++];
- return new (typeof this === 'function' ? this : $Observable)(function (observer) {
- var done = false;
- microtask(function () {
- if (!done) {
- for (var j = 0; j < items.length; ++j) {
- observer.next(items[j]);
- if (done) return;
- } observer.complete();
- }
- });
- return function () { done = true; };
- });
- }
- });
-
- hide($Observable.prototype, OBSERVABLE, function () { return this; });
-
- $export($export.G, { Observable: $Observable });
-
- __webpack_require__(194)('Observable');
-
-
-/***/ }),
-/* 325 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // ie9- setTimeout & setInterval additional parameters fix
- var global = __webpack_require__(4);
- var $export = __webpack_require__(8);
- var userAgent = __webpack_require__(218);
- var slice = [].slice;
- var MSIE = /MSIE .\./.test(userAgent); // <- dirty ie9- check
- var wrap = function (set) {
- return function (fn, time /* , ...args */) {
- var boundArgs = arguments.length > 2;
- var args = boundArgs ? slice.call(arguments, 2) : false;
- return set(boundArgs ? function () {
- // eslint-disable-next-line no-new-func
- (typeof fn == 'function' ? fn : Function(fn)).apply(this, args);
- } : fn, time);
- };
- };
- $export($export.G + $export.B + $export.F * MSIE, {
- setTimeout: wrap(global.setTimeout),
- setInterval: wrap(global.setInterval)
- });
-
-
-/***/ }),
-/* 326 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $export = __webpack_require__(8);
- var $task = __webpack_require__(214);
- $export($export.G + $export.B, {
- setImmediate: $task.set,
- clearImmediate: $task.clear
- });
-
-
-/***/ }),
-/* 327 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var $iterators = __webpack_require__(195);
- var getKeys = __webpack_require__(31);
- var redefine = __webpack_require__(18);
- var global = __webpack_require__(4);
- var hide = __webpack_require__(10);
- var Iterators = __webpack_require__(130);
- var wks = __webpack_require__(27);
- var ITERATOR = wks('iterator');
- var TO_STRING_TAG = wks('toStringTag');
- var ArrayValues = Iterators.Array;
-
- var DOMIterables = {
- CSSRuleList: true, // TODO: Not spec compliant, should be false.
- CSSStyleDeclaration: false,
- CSSValueList: false,
- ClientRectList: false,
- DOMRectList: false,
- DOMStringList: false,
- DOMTokenList: true,
- DataTransferItemList: false,
- FileList: false,
- HTMLAllCollection: false,
- HTMLCollection: false,
- HTMLFormElement: false,
- HTMLSelectElement: false,
- MediaList: true, // TODO: Not spec compliant, should be false.
- MimeTypeArray: false,
- NamedNodeMap: false,
- NodeList: true,
- PaintRequestList: false,
- Plugin: false,
- PluginArray: false,
- SVGLengthList: false,
- SVGNumberList: false,
- SVGPathSegList: false,
- SVGPointList: false,
- SVGStringList: false,
- SVGTransformList: false,
- SourceBufferList: false,
- StyleSheetList: true, // TODO: Not spec compliant, should be false.
- TextTrackCueList: false,
- TextTrackList: false,
- TouchList: false
- };
-
- for (var collections = getKeys(DOMIterables), i = 0; i < collections.length; i++) {
- var NAME = collections[i];
- var explicit = DOMIterables[NAME];
- var Collection = global[NAME];
- var proto = Collection && Collection.prototype;
- var key;
- if (proto) {
- if (!proto[ITERATOR]) hide(proto, ITERATOR, ArrayValues);
- if (!proto[TO_STRING_TAG]) hide(proto, TO_STRING_TAG, NAME);
- Iterators[NAME] = ArrayValues;
- if (explicit) for (key in $iterators) if (!proto[key]) redefine(proto, key, $iterators[key], true);
- }
- }
-
-
-/***/ }),
-/* 328 */
-/***/ (function(module, exports) {
-
- /* WEBPACK VAR INJECTION */(function(global) {/**
- * Copyright (c) 2014, Facebook, Inc.
- * All rights reserved.
- *
- * This source code is licensed under the BSD-style license found in the
- * https://raw.github.com/facebook/regenerator/master/LICENSE file. An
- * additional grant of patent rights can be found in the PATENTS file in
- * the same directory.
- */
-
- !(function(global) {
- "use strict";
-
- var Op = Object.prototype;
- var hasOwn = Op.hasOwnProperty;
- var undefined; // More compressible than void 0.
- var $Symbol = typeof Symbol === "function" ? Symbol : {};
- var iteratorSymbol = $Symbol.iterator || "@@iterator";
- var asyncIteratorSymbol = $Symbol.asyncIterator || "@@asyncIterator";
- var toStringTagSymbol = $Symbol.toStringTag || "@@toStringTag";
-
- var inModule = typeof module === "object";
- var runtime = global.regeneratorRuntime;
- if (runtime) {
- if (inModule) {
- // If regeneratorRuntime is defined globally and we're in a module,
- // make the exports object identical to regeneratorRuntime.
- module.exports = runtime;
- }
- // Don't bother evaluating the rest of this file if the runtime was
- // already defined globally.
- return;
- }
-
- // Define the runtime globally (as expected by generated code) as either
- // module.exports (if we're in a module) or a new, empty object.
- runtime = global.regeneratorRuntime = inModule ? module.exports : {};
-
- function wrap(innerFn, outerFn, self, tryLocsList) {
- // If outerFn provided and outerFn.prototype is a Generator, then outerFn.prototype instanceof Generator.
- var protoGenerator = outerFn && outerFn.prototype instanceof Generator ? outerFn : Generator;
- var generator = Object.create(protoGenerator.prototype);
- var context = new Context(tryLocsList || []);
-
- // The ._invoke method unifies the implementations of the .next,
- // .throw, and .return methods.
- generator._invoke = makeInvokeMethod(innerFn, self, context);
-
- return generator;
- }
- runtime.wrap = wrap;
-
- // Try/catch helper to minimize deoptimizations. Returns a completion
- // record like context.tryEntries[i].completion. This interface could
- // have been (and was previously) designed to take a closure to be
- // invoked without arguments, but in all the cases we care about we
- // already have an existing method we want to call, so there's no need
- // to create a new function object. We can even get away with assuming
- // the method takes exactly one argument, since that happens to be true
- // in every case, so we don't have to touch the arguments object. The
- // only additional allocation required is the completion record, which
- // has a stable shape and so hopefully should be cheap to allocate.
- function tryCatch(fn, obj, arg) {
- try {
- return { type: "normal", arg: fn.call(obj, arg) };
- } catch (err) {
- return { type: "throw", arg: err };
- }
- }
-
- var GenStateSuspendedStart = "suspendedStart";
- var GenStateSuspendedYield = "suspendedYield";
- var GenStateExecuting = "executing";
- var GenStateCompleted = "completed";
-
- // Returning this object from the innerFn has the same effect as
- // breaking out of the dispatch switch statement.
- var ContinueSentinel = {};
-
- // Dummy constructor functions that we use as the .constructor and
- // .constructor.prototype properties for functions that return Generator
- // objects. For full spec compliance, you may wish to configure your
- // minifier not to mangle the names of these two functions.
- function Generator() {}
- function GeneratorFunction() {}
- function GeneratorFunctionPrototype() {}
-
- // This is a polyfill for %IteratorPrototype% for environments that
- // don't natively support it.
- var IteratorPrototype = {};
- IteratorPrototype[iteratorSymbol] = function () {
- return this;
- };
-
- var getProto = Object.getPrototypeOf;
- var NativeIteratorPrototype = getProto && getProto(getProto(values([])));
- if (NativeIteratorPrototype &&
- NativeIteratorPrototype !== Op &&
- hasOwn.call(NativeIteratorPrototype, iteratorSymbol)) {
- // This environment has a native %IteratorPrototype%; use it instead
- // of the polyfill.
- IteratorPrototype = NativeIteratorPrototype;
- }
-
- var Gp = GeneratorFunctionPrototype.prototype =
- Generator.prototype = Object.create(IteratorPrototype);
- GeneratorFunction.prototype = Gp.constructor = GeneratorFunctionPrototype;
- GeneratorFunctionPrototype.constructor = GeneratorFunction;
- GeneratorFunctionPrototype[toStringTagSymbol] =
- GeneratorFunction.displayName = "GeneratorFunction";
-
- // Helper for defining the .next, .throw, and .return methods of the
- // Iterator interface in terms of a single ._invoke method.
- function defineIteratorMethods(prototype) {
- ["next", "throw", "return"].forEach(function(method) {
- prototype[method] = function(arg) {
- return this._invoke(method, arg);
- };
- });
- }
-
- runtime.isGeneratorFunction = function(genFun) {
- var ctor = typeof genFun === "function" && genFun.constructor;
- return ctor
- ? ctor === GeneratorFunction ||
- // For the native GeneratorFunction constructor, the best we can
- // do is to check its .name property.
- (ctor.displayName || ctor.name) === "GeneratorFunction"
- : false;
- };
-
- runtime.mark = function(genFun) {
- if (Object.setPrototypeOf) {
- Object.setPrototypeOf(genFun, GeneratorFunctionPrototype);
- } else {
- genFun.__proto__ = GeneratorFunctionPrototype;
- if (!(toStringTagSymbol in genFun)) {
- genFun[toStringTagSymbol] = "GeneratorFunction";
- }
- }
- genFun.prototype = Object.create(Gp);
- return genFun;
- };
-
- // Within the body of any async function, `await x` is transformed to
- // `yield regeneratorRuntime.awrap(x)`, so that the runtime can test
- // `hasOwn.call(value, "__await")` to determine if the yielded value is
- // meant to be awaited.
- runtime.awrap = function(arg) {
- return { __await: arg };
- };
-
- function AsyncIterator(generator) {
- function invoke(method, arg, resolve, reject) {
- var record = tryCatch(generator[method], generator, arg);
- if (record.type === "throw") {
- reject(record.arg);
- } else {
- var result = record.arg;
- var value = result.value;
- if (value &&
- typeof value === "object" &&
- hasOwn.call(value, "__await")) {
- return Promise.resolve(value.__await).then(function(value) {
- invoke("next", value, resolve, reject);
- }, function(err) {
- invoke("throw", err, resolve, reject);
- });
- }
-
- return Promise.resolve(value).then(function(unwrapped) {
- // When a yielded Promise is resolved, its final value becomes
- // the .value of the Promise<{value,done}> result for the
- // current iteration. If the Promise is rejected, however, the
- // result for this iteration will be rejected with the same
- // reason. Note that rejections of yielded Promises are not
- // thrown back into the generator function, as is the case
- // when an awaited Promise is rejected. This difference in
- // behavior between yield and await is important, because it
- // allows the consumer to decide what to do with the yielded
- // rejection (swallow it and continue, manually .throw it back
- // into the generator, abandon iteration, whatever). With
- // await, by contrast, there is no opportunity to examine the
- // rejection reason outside the generator function, so the
- // only option is to throw it from the await expression, and
- // let the generator function handle the exception.
- result.value = unwrapped;
- resolve(result);
- }, reject);
- }
- }
-
- if (typeof global.process === "object" && global.process.domain) {
- invoke = global.process.domain.bind(invoke);
- }
-
- var previousPromise;
-
- function enqueue(method, arg) {
- function callInvokeWithMethodAndArg() {
- return new Promise(function(resolve, reject) {
- invoke(method, arg, resolve, reject);
- });
- }
-
- return previousPromise =
- // If enqueue has been called before, then we want to wait until
- // all previous Promises have been resolved before calling invoke,
- // so that results are always delivered in the correct order. If
- // enqueue has not been called before, then it is important to
- // call invoke immediately, without waiting on a callback to fire,
- // so that the async generator function has the opportunity to do
- // any necessary setup in a predictable way. This predictability
- // is why the Promise constructor synchronously invokes its
- // executor callback, and why async functions synchronously
- // execute code before the first await. Since we implement simple
- // async functions in terms of async generators, it is especially
- // important to get this right, even though it requires care.
- previousPromise ? previousPromise.then(
- callInvokeWithMethodAndArg,
- // Avoid propagating failures to Promises returned by later
- // invocations of the iterator.
- callInvokeWithMethodAndArg
- ) : callInvokeWithMethodAndArg();
- }
-
- // Define the unified helper method that is used to implement .next,
- // .throw, and .return (see defineIteratorMethods).
- this._invoke = enqueue;
- }
-
- defineIteratorMethods(AsyncIterator.prototype);
- AsyncIterator.prototype[asyncIteratorSymbol] = function () {
- return this;
- };
- runtime.AsyncIterator = AsyncIterator;
-
- // Note that simple async functions are implemented on top of
- // AsyncIterator objects; they just return a Promise for the value of
- // the final result produced by the iterator.
- runtime.async = function(innerFn, outerFn, self, tryLocsList) {
- var iter = new AsyncIterator(
- wrap(innerFn, outerFn, self, tryLocsList)
- );
-
- return runtime.isGeneratorFunction(outerFn)
- ? iter // If outerFn is a generator, return the full iterator.
- : iter.next().then(function(result) {
- return result.done ? result.value : iter.next();
- });
- };
-
- function makeInvokeMethod(innerFn, self, context) {
- var state = GenStateSuspendedStart;
-
- return function invoke(method, arg) {
- if (state === GenStateExecuting) {
- throw new Error("Generator is already running");
- }
-
- if (state === GenStateCompleted) {
- if (method === "throw") {
- throw arg;
- }
-
- // Be forgiving, per 25.3.3.3.3 of the spec:
- // https://people.mozilla.org/~jorendorff/es6-draft.html#sec-generatorresume
- return doneResult();
- }
-
- context.method = method;
- context.arg = arg;
-
- while (true) {
- var delegate = context.delegate;
- if (delegate) {
- var delegateResult = maybeInvokeDelegate(delegate, context);
- if (delegateResult) {
- if (delegateResult === ContinueSentinel) continue;
- return delegateResult;
- }
- }
-
- if (context.method === "next") {
- // Setting context._sent for legacy support of Babel's
- // function.sent implementation.
- context.sent = context._sent = context.arg;
-
- } else if (context.method === "throw") {
- if (state === GenStateSuspendedStart) {
- state = GenStateCompleted;
- throw context.arg;
- }
-
- context.dispatchException(context.arg);
-
- } else if (context.method === "return") {
- context.abrupt("return", context.arg);
- }
-
- state = GenStateExecuting;
-
- var record = tryCatch(innerFn, self, context);
- if (record.type === "normal") {
- // If an exception is thrown from innerFn, we leave state ===
- // GenStateExecuting and loop back for another invocation.
- state = context.done
- ? GenStateCompleted
- : GenStateSuspendedYield;
-
- if (record.arg === ContinueSentinel) {
- continue;
- }
-
- return {
- value: record.arg,
- done: context.done
- };
-
- } else if (record.type === "throw") {
- state = GenStateCompleted;
- // Dispatch the exception by looping back around to the
- // context.dispatchException(context.arg) call above.
- context.method = "throw";
- context.arg = record.arg;
- }
- }
- };
- }
-
- // Call delegate.iterator[context.method](context.arg) and handle the
- // result, either by returning a { value, done } result from the
- // delegate iterator, or by modifying context.method and context.arg,
- // setting context.delegate to null, and returning the ContinueSentinel.
- function maybeInvokeDelegate(delegate, context) {
- var method = delegate.iterator[context.method];
- if (method === undefined) {
- // A .throw or .return when the delegate iterator has no .throw
- // method always terminates the yield* loop.
- context.delegate = null;
-
- if (context.method === "throw") {
- if (delegate.iterator.return) {
- // If the delegate iterator has a return method, give it a
- // chance to clean up.
- context.method = "return";
- context.arg = undefined;
- maybeInvokeDelegate(delegate, context);
-
- if (context.method === "throw") {
- // If maybeInvokeDelegate(context) changed context.method from
- // "return" to "throw", let that override the TypeError below.
- return ContinueSentinel;
- }
- }
-
- context.method = "throw";
- context.arg = new TypeError(
- "The iterator does not provide a 'throw' method");
- }
-
- return ContinueSentinel;
- }
-
- var record = tryCatch(method, delegate.iterator, context.arg);
-
- if (record.type === "throw") {
- context.method = "throw";
- context.arg = record.arg;
- context.delegate = null;
- return ContinueSentinel;
- }
-
- var info = record.arg;
-
- if (! info) {
- context.method = "throw";
- context.arg = new TypeError("iterator result is not an object");
- context.delegate = null;
- return ContinueSentinel;
- }
-
- if (info.done) {
- // Assign the result of the finished delegate to the temporary
- // variable specified by delegate.resultName (see delegateYield).
- context[delegate.resultName] = info.value;
-
- // Resume execution at the desired location (see delegateYield).
- context.next = delegate.nextLoc;
-
- // If context.method was "throw" but the delegate handled the
- // exception, let the outer generator proceed normally. If
- // context.method was "next", forget context.arg since it has been
- // "consumed" by the delegate iterator. If context.method was
- // "return", allow the original .return call to continue in the
- // outer generator.
- if (context.method !== "return") {
- context.method = "next";
- context.arg = undefined;
- }
-
- } else {
- // Re-yield the result returned by the delegate method.
- return info;
- }
-
- // The delegate iterator is finished, so forget it and continue with
- // the outer generator.
- context.delegate = null;
- return ContinueSentinel;
- }
-
- // Define Generator.prototype.{next,throw,return} in terms of the
- // unified ._invoke helper method.
- defineIteratorMethods(Gp);
-
- Gp[toStringTagSymbol] = "Generator";
-
- // A Generator should always return itself as the iterator object when the
- // @@iterator function is called on it. Some browsers' implementations of the
- // iterator prototype chain incorrectly implement this, causing the Generator
- // object to not be returned from this call. This ensures that doesn't happen.
- // See https://github.com/facebook/regenerator/issues/274 for more details.
- Gp[iteratorSymbol] = function() {
- return this;
- };
-
- Gp.toString = function() {
- return "[object Generator]";
- };
-
- function pushTryEntry(locs) {
- var entry = { tryLoc: locs[0] };
-
- if (1 in locs) {
- entry.catchLoc = locs[1];
- }
-
- if (2 in locs) {
- entry.finallyLoc = locs[2];
- entry.afterLoc = locs[3];
- }
-
- this.tryEntries.push(entry);
- }
-
- function resetTryEntry(entry) {
- var record = entry.completion || {};
- record.type = "normal";
- delete record.arg;
- entry.completion = record;
- }
-
- function Context(tryLocsList) {
- // The root entry object (effectively a try statement without a catch
- // or a finally block) gives us a place to store values thrown from
- // locations where there is no enclosing try statement.
- this.tryEntries = [{ tryLoc: "root" }];
- tryLocsList.forEach(pushTryEntry, this);
- this.reset(true);
- }
-
- runtime.keys = function(object) {
- var keys = [];
- for (var key in object) {
- keys.push(key);
- }
- keys.reverse();
-
- // Rather than returning an object with a next method, we keep
- // things simple and return the next function itself.
- return function next() {
- while (keys.length) {
- var key = keys.pop();
- if (key in object) {
- next.value = key;
- next.done = false;
- return next;
- }
- }
-
- // To avoid creating an additional object, we just hang the .value
- // and .done properties off the next function object itself. This
- // also ensures that the minifier will not anonymize the function.
- next.done = true;
- return next;
- };
- };
-
- function values(iterable) {
- if (iterable) {
- var iteratorMethod = iterable[iteratorSymbol];
- if (iteratorMethod) {
- return iteratorMethod.call(iterable);
- }
-
- if (typeof iterable.next === "function") {
- return iterable;
- }
-
- if (!isNaN(iterable.length)) {
- var i = -1, next = function next() {
- while (++i < iterable.length) {
- if (hasOwn.call(iterable, i)) {
- next.value = iterable[i];
- next.done = false;
- return next;
- }
- }
-
- next.value = undefined;
- next.done = true;
-
- return next;
- };
-
- return next.next = next;
- }
- }
-
- // Return an iterator with no values.
- return { next: doneResult };
- }
- runtime.values = values;
-
- function doneResult() {
- return { value: undefined, done: true };
- }
-
- Context.prototype = {
- constructor: Context,
-
- reset: function(skipTempReset) {
- this.prev = 0;
- this.next = 0;
- // Resetting context._sent for legacy support of Babel's
- // function.sent implementation.
- this.sent = this._sent = undefined;
- this.done = false;
- this.delegate = null;
-
- this.method = "next";
- this.arg = undefined;
-
- this.tryEntries.forEach(resetTryEntry);
-
- if (!skipTempReset) {
- for (var name in this) {
- // Not sure about the optimal order of these conditions:
- if (name.charAt(0) === "t" &&
- hasOwn.call(this, name) &&
- !isNaN(+name.slice(1))) {
- this[name] = undefined;
- }
- }
- }
- },
-
- stop: function() {
- this.done = true;
-
- var rootEntry = this.tryEntries[0];
- var rootRecord = rootEntry.completion;
- if (rootRecord.type === "throw") {
- throw rootRecord.arg;
- }
-
- return this.rval;
- },
-
- dispatchException: function(exception) {
- if (this.done) {
- throw exception;
- }
-
- var context = this;
- function handle(loc, caught) {
- record.type = "throw";
- record.arg = exception;
- context.next = loc;
-
- if (caught) {
- // If the dispatched exception was caught by a catch block,
- // then let that catch block handle the exception normally.
- context.method = "next";
- context.arg = undefined;
- }
-
- return !! caught;
- }
-
- for (var i = this.tryEntries.length - 1; i >= 0; --i) {
- var entry = this.tryEntries[i];
- var record = entry.completion;
-
- if (entry.tryLoc === "root") {
- // Exception thrown outside of any try block that could handle
- // it, so set the completion value of the entire function to
- // throw the exception.
- return handle("end");
- }
-
- if (entry.tryLoc <= this.prev) {
- var hasCatch = hasOwn.call(entry, "catchLoc");
- var hasFinally = hasOwn.call(entry, "finallyLoc");
-
- if (hasCatch && hasFinally) {
- if (this.prev < entry.catchLoc) {
- return handle(entry.catchLoc, true);
- } else if (this.prev < entry.finallyLoc) {
- return handle(entry.finallyLoc);
- }
-
- } else if (hasCatch) {
- if (this.prev < entry.catchLoc) {
- return handle(entry.catchLoc, true);
- }
-
- } else if (hasFinally) {
- if (this.prev < entry.finallyLoc) {
- return handle(entry.finallyLoc);
- }
-
- } else {
- throw new Error("try statement without catch or finally");
- }
- }
- }
- },
-
- abrupt: function(type, arg) {
- for (var i = this.tryEntries.length - 1; i >= 0; --i) {
- var entry = this.tryEntries[i];
- if (entry.tryLoc <= this.prev &&
- hasOwn.call(entry, "finallyLoc") &&
- this.prev < entry.finallyLoc) {
- var finallyEntry = entry;
- break;
- }
- }
-
- if (finallyEntry &&
- (type === "break" ||
- type === "continue") &&
- finallyEntry.tryLoc <= arg &&
- arg <= finallyEntry.finallyLoc) {
- // Ignore the finally entry if control is not jumping to a
- // location outside the try/catch block.
- finallyEntry = null;
- }
-
- var record = finallyEntry ? finallyEntry.completion : {};
- record.type = type;
- record.arg = arg;
-
- if (finallyEntry) {
- this.method = "next";
- this.next = finallyEntry.finallyLoc;
- return ContinueSentinel;
- }
-
- return this.complete(record);
- },
-
- complete: function(record, afterLoc) {
- if (record.type === "throw") {
- throw record.arg;
- }
-
- if (record.type === "break" ||
- record.type === "continue") {
- this.next = record.arg;
- } else if (record.type === "return") {
- this.rval = this.arg = record.arg;
- this.method = "return";
- this.next = "end";
- } else if (record.type === "normal" && afterLoc) {
- this.next = afterLoc;
- }
-
- return ContinueSentinel;
- },
-
- finish: function(finallyLoc) {
- for (var i = this.tryEntries.length - 1; i >= 0; --i) {
- var entry = this.tryEntries[i];
- if (entry.finallyLoc === finallyLoc) {
- this.complete(entry.completion, entry.afterLoc);
- resetTryEntry(entry);
- return ContinueSentinel;
- }
- }
- },
-
- "catch": function(tryLoc) {
- for (var i = this.tryEntries.length - 1; i >= 0; --i) {
- var entry = this.tryEntries[i];
- if (entry.tryLoc === tryLoc) {
- var record = entry.completion;
- if (record.type === "throw") {
- var thrown = record.arg;
- resetTryEntry(entry);
- }
- return thrown;
- }
- }
-
- // The context.catch method must only be called with a location
- // argument that corresponds to a known catch block.
- throw new Error("illegal catch attempt");
- },
-
- delegateYield: function(iterable, resultName, nextLoc) {
- this.delegate = {
- iterator: values(iterable),
- resultName: resultName,
- nextLoc: nextLoc
- };
-
- if (this.method === "next") {
- // Deliberately forget the last sent value so that we don't
- // accidentally pass it on to the delegate.
- this.arg = undefined;
- }
-
- return ContinueSentinel;
- }
- };
- })(
- // Among the various tricks for obtaining a reference to the global
- // object, this seems to be the most reliable technique that does not
- // use indirect eval (which violates Content Security Policy).
- typeof global === "object" ? global :
- typeof window === "object" ? window :
- typeof self === "object" ? self : this
- );
-
- /* WEBPACK VAR INJECTION */}.call(exports, (function() { return this; }())))
-
-/***/ }),
-/* 329 */
-/***/ (function(module, exports, __webpack_require__) {
-
- __webpack_require__(330);
- module.exports = __webpack_require__(9).RegExp.escape;
-
-
-/***/ }),
-/* 330 */
-/***/ (function(module, exports, __webpack_require__) {
-
- // https://github.com/benjamingr/RexExp.escape
- var $export = __webpack_require__(8);
- var $re = __webpack_require__(331)(/[\\^$*+?.()|[\]{}]/g, '\\$&');
-
- $export($export.S, 'RegExp', { escape: function escape(it) { return $re(it); } });
-
-
-/***/ }),
-/* 331 */
-/***/ (function(module, exports) {
-
- module.exports = function (regExp, replace) {
- var replacer = replace === Object(replace) ? function (part) {
- return replace[part];
- } : replace;
- return function (it) {
- return String(it).replace(regExp, replacer);
- };
- };
-
-
-/***/ }),
-/* 332 */
-/***/ (function(module, exports, __webpack_require__) {
-
- var BSON = __webpack_require__(333),
- Binary = __webpack_require__(356),
- Code = __webpack_require__(351),
- DBRef = __webpack_require__(355),
- Decimal128 = __webpack_require__(352),
- Double = __webpack_require__(336),
- Int32 = __webpack_require__(350),
- Long = __webpack_require__(335),
- Map = __webpack_require__(334),
- MaxKey = __webpack_require__(354),
- MinKey = __webpack_require__(353),
- ObjectId = __webpack_require__(338),
- BSONRegExp = __webpack_require__(348),
- Symbol = __webpack_require__(349),
- Timestamp = __webpack_require__(337);
-
- // BSON MAX VALUES
- BSON.BSON_INT32_MAX = 0x7fffffff;
- BSON.BSON_INT32_MIN = -0x80000000;
-
- BSON.BSON_INT64_MAX = Math.pow(2, 63) - 1;
- BSON.BSON_INT64_MIN = -Math.pow(2, 63);
-
- // JS MAX PRECISE VALUES
- BSON.JS_INT_MAX = 0x20000000000000; // Any integer up to 2^53 can be precisely represented by a double.
- BSON.JS_INT_MIN = -0x20000000000000; // Any integer down to -2^53 can be precisely represented by a double.
-
- // Add BSON types to function creation
- BSON.Binary = Binary;
- BSON.Code = Code;
- BSON.DBRef = DBRef;
- BSON.Decimal128 = Decimal128;
- BSON.Double = Double;
- BSON.Int32 = Int32;
- BSON.Long = Long;
- BSON.Map = Map;
- BSON.MaxKey = MaxKey;
- BSON.MinKey = MinKey;
- BSON.ObjectId = ObjectId;
- BSON.ObjectID = ObjectId;
- BSON.BSONRegExp = BSONRegExp;
- BSON.Symbol = Symbol;
- BSON.Timestamp = Timestamp;
-
- // Return the BSON
- module.exports = BSON;
-
-/***/ }),
-/* 333 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
-
- var Map = __webpack_require__(334),
- Long = __webpack_require__(335),
- Double = __webpack_require__(336),
- Timestamp = __webpack_require__(337),
- ObjectID = __webpack_require__(338),
- BSONRegExp = __webpack_require__(348),
- Symbol = __webpack_require__(349),
- Int32 = __webpack_require__(350),
- Code = __webpack_require__(351),
- Decimal128 = __webpack_require__(352),
- MinKey = __webpack_require__(353),
- MaxKey = __webpack_require__(354),
- DBRef = __webpack_require__(355),
- Binary = __webpack_require__(356);
-
- // Parts of the parser
- var deserialize = __webpack_require__(357),
- serializer = __webpack_require__(358),
- calculateObjectSize = __webpack_require__(360),
- utils = __webpack_require__(344);
-
- /**
- * @ignore
- * @api private
- */
- // Default Max Size
- var MAXSIZE = 1024 * 1024 * 17;
-
- // Current Internal Temporary Serialization Buffer
- var buffer = utils.allocBuffer(MAXSIZE);
-
- var BSON = function () {};
-
- /**
- * Serialize a Javascript object.
- *
- * @param {Object} object the Javascript object to serialize.
- * @param {Boolean} [options.checkKeys] the serializer will check if keys are valid.
- * @param {Boolean} [options.serializeFunctions=false] serialize the javascript functions **(default:false)**.
- * @param {Boolean} [options.ignoreUndefined=true] ignore undefined fields **(default:true)**.
- * @param {Number} [options.minInternalBufferSize=1024*1024*17] minimum size of the internal temporary serialization buffer **(default:1024*1024*17)**.
- * @return {Buffer} returns the Buffer object containing the serialized object.
- * @api public
- */
- BSON.prototype.serialize = function serialize(object, options) {
- options = options || {};
- // Unpack the options
- var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
- var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
- var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
- var minInternalBufferSize = typeof options.minInternalBufferSize === 'number' ? options.minInternalBufferSize : MAXSIZE;
-
- // Resize the internal serialization buffer if needed
- if (buffer.length < minInternalBufferSize) {
- buffer = utils.allocBuffer(minInternalBufferSize);
- }
-
- // Attempt to serialize
- var serializationIndex = serializer(buffer, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined, []);
- // Create the final buffer
- var finishedBuffer = utils.allocBuffer(serializationIndex);
- // Copy into the finished buffer
- buffer.copy(finishedBuffer, 0, 0, finishedBuffer.length);
- // Return the buffer
- return finishedBuffer;
- };
-
- /**
- * Serialize a Javascript object using a predefined Buffer and index into the buffer, useful when pre-allocating the space for serialization.
- *
- * @param {Object} object the Javascript object to serialize.
- * @param {Buffer} buffer the Buffer you pre-allocated to store the serialized BSON object.
- * @param {Boolean} [options.checkKeys] the serializer will check if keys are valid.
- * @param {Boolean} [options.serializeFunctions=false] serialize the javascript functions **(default:false)**.
- * @param {Boolean} [options.ignoreUndefined=true] ignore undefined fields **(default:true)**.
- * @param {Number} [options.index] the index in the buffer where we wish to start serializing into.
- * @return {Number} returns the index pointing to the last written byte in the buffer.
- * @api public
- */
- BSON.prototype.serializeWithBufferAndIndex = function (object, finalBuffer, options) {
- options = options || {};
- // Unpack the options
- var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
- var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
- var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
- var startIndex = typeof options.index === 'number' ? options.index : 0;
-
- // Attempt to serialize
- var serializationIndex = serializer(finalBuffer, object, checkKeys, startIndex || 0, 0, serializeFunctions, ignoreUndefined);
-
- // Return the index
- return serializationIndex - 1;
- };
-
- /**
- * Deserialize data as BSON.
- *
- * @param {Buffer} buffer the buffer containing the serialized set of BSON documents.
- * @param {Object} [options.evalFunctions=false] evaluate functions in the BSON document scoped to the object deserialized.
- * @param {Object} [options.cacheFunctions=false] cache evaluated functions for reuse.
- * @param {Object} [options.cacheFunctionsCrc32=false] use a crc32 code for caching, otherwise use the string of the function.
- * @param {Object} [options.promoteLongs=true] when deserializing a Long will fit it into a Number if it's smaller than 53 bits
- * @param {Object} [options.promoteBuffers=false] when deserializing a Binary will return it as a node.js Buffer instance.
- * @param {Object} [options.promoteValues=false] when deserializing will promote BSON values to their Node.js closest equivalent types.
- * @param {Object} [options.fieldsAsRaw=null] allow to specify if there what fields we wish to return as unserialized raw buffer.
- * @param {Object} [options.bsonRegExp=false] return BSON regular expressions as BSONRegExp instances.
- * @return {Object} returns the deserialized Javascript Object.
- * @api public
- */
- BSON.prototype.deserialize = function (buffer, options) {
- return deserialize(buffer, options);
- };
-
- /**
- * Calculate the bson size for a passed in Javascript object.
- *
- * @param {Object} object the Javascript object to calculate the BSON byte size for.
- * @param {Boolean} [options.serializeFunctions=false] serialize the javascript functions **(default:false)**.
- * @param {Boolean} [options.ignoreUndefined=true] ignore undefined fields **(default:true)**.
- * @return {Number} returns the number of bytes the BSON object will take up.
- * @api public
- */
- BSON.prototype.calculateObjectSize = function (object, options) {
- options = options || {};
-
- var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
- var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
-
- return calculateObjectSize(object, serializeFunctions, ignoreUndefined);
- };
-
- /**
- * Deserialize stream data as BSON documents.
- *
- * @param {Buffer} data the buffer containing the serialized set of BSON documents.
- * @param {Number} startIndex the start index in the data Buffer where the deserialization is to start.
- * @param {Number} numberOfDocuments number of documents to deserialize.
- * @param {Array} documents an array where to store the deserialized documents.
- * @param {Number} docStartIndex the index in the documents array from where to start inserting documents.
- * @param {Object} [options] additional options used for the deserialization.
- * @param {Object} [options.evalFunctions=false] evaluate functions in the BSON document scoped to the object deserialized.
- * @param {Object} [options.cacheFunctions=false] cache evaluated functions for reuse.
- * @param {Object} [options.cacheFunctionsCrc32=false] use a crc32 code for caching, otherwise use the string of the function.
- * @param {Object} [options.promoteLongs=true] when deserializing a Long will fit it into a Number if it's smaller than 53 bits
- * @param {Object} [options.promoteBuffers=false] when deserializing a Binary will return it as a node.js Buffer instance.
- * @param {Object} [options.promoteValues=false] when deserializing will promote BSON values to their Node.js closest equivalent types.
- * @param {Object} [options.fieldsAsRaw=null] allow to specify if there what fields we wish to return as unserialized raw buffer.
- * @param {Object} [options.bsonRegExp=false] return BSON regular expressions as BSONRegExp instances.
- * @return {Number} returns the next index in the buffer after deserialization **x** numbers of documents.
- * @api public
- */
- BSON.prototype.deserializeStream = function (data, startIndex, numberOfDocuments, documents, docStartIndex, options) {
- options = options != null ? options : {};
- var index = startIndex;
- // Loop over all documents
- for (var i = 0; i < numberOfDocuments; i++) {
- // Find size of the document
- var size = data[index] | data[index + 1] << 8 | data[index + 2] << 16 | data[index + 3] << 24;
- // Update options with index
- options['index'] = index;
- // Parse the document at this point
- documents[docStartIndex + i] = this.deserialize(data, options);
- // Adjust index by the document size
- index = index + size;
- }
-
- // Return object containing end index of parsing and list of documents
- return index;
- };
-
- /**
- * @ignore
- * @api private
- */
- // BSON MAX VALUES
- BSON.BSON_INT32_MAX = 0x7fffffff;
- BSON.BSON_INT32_MIN = -0x80000000;
-
- BSON.BSON_INT64_MAX = Math.pow(2, 63) - 1;
- BSON.BSON_INT64_MIN = -Math.pow(2, 63);
-
- // JS MAX PRECISE VALUES
- BSON.JS_INT_MAX = 0x20000000000000; // Any integer up to 2^53 can be precisely represented by a double.
- BSON.JS_INT_MIN = -0x20000000000000; // Any integer down to -2^53 can be precisely represented by a double.
-
- // Internal long versions
- // var JS_INT_MAX_LONG = Long.fromNumber(0x20000000000000); // Any integer up to 2^53 can be precisely represented by a double.
- // var JS_INT_MIN_LONG = Long.fromNumber(-0x20000000000000); // Any integer down to -2^53 can be precisely represented by a double.
-
- /**
- * Number BSON Type
- *
- * @classconstant BSON_DATA_NUMBER
- **/
- BSON.BSON_DATA_NUMBER = 1;
- /**
- * String BSON Type
- *
- * @classconstant BSON_DATA_STRING
- **/
- BSON.BSON_DATA_STRING = 2;
- /**
- * Object BSON Type
- *
- * @classconstant BSON_DATA_OBJECT
- **/
- BSON.BSON_DATA_OBJECT = 3;
- /**
- * Array BSON Type
- *
- * @classconstant BSON_DATA_ARRAY
- **/
- BSON.BSON_DATA_ARRAY = 4;
- /**
- * Binary BSON Type
- *
- * @classconstant BSON_DATA_BINARY
- **/
- BSON.BSON_DATA_BINARY = 5;
- /**
- * ObjectID BSON Type
- *
- * @classconstant BSON_DATA_OID
- **/
- BSON.BSON_DATA_OID = 7;
- /**
- * Boolean BSON Type
- *
- * @classconstant BSON_DATA_BOOLEAN
- **/
- BSON.BSON_DATA_BOOLEAN = 8;
- /**
- * Date BSON Type
- *
- * @classconstant BSON_DATA_DATE
- **/
- BSON.BSON_DATA_DATE = 9;
- /**
- * null BSON Type
- *
- * @classconstant BSON_DATA_NULL
- **/
- BSON.BSON_DATA_NULL = 10;
- /**
- * RegExp BSON Type
- *
- * @classconstant BSON_DATA_REGEXP
- **/
- BSON.BSON_DATA_REGEXP = 11;
- /**
- * Code BSON Type
- *
- * @classconstant BSON_DATA_CODE
- **/
- BSON.BSON_DATA_CODE = 13;
- /**
- * Symbol BSON Type
- *
- * @classconstant BSON_DATA_SYMBOL
- **/
- BSON.BSON_DATA_SYMBOL = 14;
- /**
- * Code with Scope BSON Type
- *
- * @classconstant BSON_DATA_CODE_W_SCOPE
- **/
- BSON.BSON_DATA_CODE_W_SCOPE = 15;
- /**
- * 32 bit Integer BSON Type
- *
- * @classconstant BSON_DATA_INT
- **/
- BSON.BSON_DATA_INT = 16;
- /**
- * Timestamp BSON Type
- *
- * @classconstant BSON_DATA_TIMESTAMP
- **/
- BSON.BSON_DATA_TIMESTAMP = 17;
- /**
- * Long BSON Type
- *
- * @classconstant BSON_DATA_LONG
- **/
- BSON.BSON_DATA_LONG = 18;
- /**
- * MinKey BSON Type
- *
- * @classconstant BSON_DATA_MIN_KEY
- **/
- BSON.BSON_DATA_MIN_KEY = 0xff;
- /**
- * MaxKey BSON Type
- *
- * @classconstant BSON_DATA_MAX_KEY
- **/
- BSON.BSON_DATA_MAX_KEY = 0x7f;
-
- /**
- * Binary Default Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_DEFAULT
- **/
- BSON.BSON_BINARY_SUBTYPE_DEFAULT = 0;
- /**
- * Binary Function Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_FUNCTION
- **/
- BSON.BSON_BINARY_SUBTYPE_FUNCTION = 1;
- /**
- * Binary Byte Array Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_BYTE_ARRAY
- **/
- BSON.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
- /**
- * Binary UUID Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_UUID
- **/
- BSON.BSON_BINARY_SUBTYPE_UUID = 3;
- /**
- * Binary MD5 Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_MD5
- **/
- BSON.BSON_BINARY_SUBTYPE_MD5 = 4;
- /**
- * Binary User Defined Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_USER_DEFINED
- **/
- BSON.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
-
- // Return BSON
- module.exports = BSON;
- module.exports.Code = Code;
- module.exports.Map = Map;
- module.exports.Symbol = Symbol;
- module.exports.BSON = BSON;
- module.exports.DBRef = DBRef;
- module.exports.Binary = Binary;
- module.exports.ObjectID = ObjectID;
- module.exports.Long = Long;
- module.exports.Timestamp = Timestamp;
- module.exports.Double = Double;
- module.exports.Int32 = Int32;
- module.exports.MinKey = MinKey;
- module.exports.MaxKey = MaxKey;
- module.exports.BSONRegExp = BSONRegExp;
- module.exports.Decimal128 = Decimal128;
-
-/***/ }),
-/* 334 */
-/***/ (function(module, exports) {
-
- /* WEBPACK VAR INJECTION */(function(global) {'use strict';
-
- // We have an ES6 Map available, return the native instance
-
- if (typeof global.Map !== 'undefined') {
- module.exports = global.Map;
- module.exports.Map = global.Map;
- } else {
- // We will return a polyfill
- var Map = function (array) {
- this._keys = [];
- this._values = {};
-
- for (var i = 0; i < array.length; i++) {
- if (array[i] == null) continue; // skip null and undefined
- var entry = array[i];
- var key = entry[0];
- var value = entry[1];
- // Add the key to the list of keys in order
- this._keys.push(key);
- // Add the key and value to the values dictionary with a point
- // to the location in the ordered keys list
- this._values[key] = { v: value, i: this._keys.length - 1 };
- }
- };
-
- Map.prototype.clear = function () {
- this._keys = [];
- this._values = {};
- };
-
- Map.prototype.delete = function (key) {
- var value = this._values[key];
- if (value == null) return false;
- // Delete entry
- delete this._values[key];
- // Remove the key from the ordered keys list
- this._keys.splice(value.i, 1);
- return true;
- };
-
- Map.prototype.entries = function () {
- var self = this;
- var index = 0;
-
- return {
- next: function () {
- var key = self._keys[index++];
- return {
- value: key !== undefined ? [key, self._values[key].v] : undefined,
- done: key !== undefined ? false : true
- };
- }
- };
- };
-
- Map.prototype.forEach = function (callback, self) {
- self = self || this;
-
- for (var i = 0; i < this._keys.length; i++) {
- var key = this._keys[i];
- // Call the forEach callback
- callback.call(self, this._values[key].v, key, self);
- }
- };
-
- Map.prototype.get = function (key) {
- return this._values[key] ? this._values[key].v : undefined;
- };
-
- Map.prototype.has = function (key) {
- return this._values[key] != null;
- };
-
- Map.prototype.keys = function () {
- var self = this;
- var index = 0;
-
- return {
- next: function () {
- var key = self._keys[index++];
- return {
- value: key !== undefined ? key : undefined,
- done: key !== undefined ? false : true
- };
- }
- };
- };
-
- Map.prototype.set = function (key, value) {
- if (this._values[key]) {
- this._values[key].v = value;
- return this;
- }
-
- // Add the key to the list of keys in order
- this._keys.push(key);
- // Add the key and value to the values dictionary with a point
- // to the location in the ordered keys list
- this._values[key] = { v: value, i: this._keys.length - 1 };
- return this;
- };
-
- Map.prototype.values = function () {
- var self = this;
- var index = 0;
-
- return {
- next: function () {
- var key = self._keys[index++];
- return {
- value: key !== undefined ? self._values[key].v : undefined,
- done: key !== undefined ? false : true
- };
- }
- };
- };
-
- // Last ismaster
- Object.defineProperty(Map.prototype, 'size', {
- enumerable: true,
- get: function () {
- return this._keys.length;
- }
- });
-
- module.exports = Map;
- module.exports.Map = Map;
- }
- /* WEBPACK VAR INJECTION */}.call(exports, (function() { return this; }())))
-
-/***/ }),
-/* 335 */
-/***/ (function(module, exports) {
-
- // Licensed under the Apache License, Version 2.0 (the "License");
- // you may not use this file except in compliance with the License.
- // You may obtain a copy of the License at
- //
- // http://www.apache.org/licenses/LICENSE-2.0
- //
- // Unless required by applicable law or agreed to in writing, software
- // distributed under the License is distributed on an "AS IS" BASIS,
- // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
- // See the License for the specific language governing permissions and
- // limitations under the License.
- //
- // Copyright 2009 Google Inc. All Rights Reserved
-
- /**
- * Defines a Long class for representing a 64-bit two's-complement
- * integer value, which faithfully simulates the behavior of a Java "Long". This
- * implementation is derived from LongLib in GWT.
- *
- * Constructs a 64-bit two's-complement integer, given its low and high 32-bit
- * values as *signed* integers. See the from* functions below for more
- * convenient ways of constructing Longs.
- *
- * The internal representation of a Long is the two given signed, 32-bit values.
- * We use 32-bit pieces because these are the size of integers on which
- * Javascript performs bit-operations. For operations like addition and
- * multiplication, we split each number into 16-bit pieces, which can easily be
- * multiplied within Javascript's floating-point representation without overflow
- * or change in sign.
- *
- * In the algorithms below, we frequently reduce the negative case to the
- * positive case by negating the input(s) and then post-processing the result.
- * Note that we must ALWAYS check specially whether those values are MIN_VALUE
- * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
- * a positive number, it overflows back into a negative). Not handling this
- * case would often result in infinite recursion.
- *
- * @class
- * @param {number} low the low (signed) 32 bits of the Long.
- * @param {number} high the high (signed) 32 bits of the Long.
- * @return {Long}
- */
- function Long(low, high) {
- if (!(this instanceof Long)) return new Long(low, high);
-
- this._bsontype = 'Long';
- /**
- * @type {number}
- * @ignore
- */
- this.low_ = low | 0; // force into 32 signed bits.
-
- /**
- * @type {number}
- * @ignore
- */
- this.high_ = high | 0; // force into 32 signed bits.
- }
-
- /**
- * Return the int value.
- *
- * @method
- * @return {number} the value, assuming it is a 32-bit integer.
- */
- Long.prototype.toInt = function () {
- return this.low_;
- };
-
- /**
- * Return the Number value.
- *
- * @method
- * @return {number} the closest floating-point representation to this value.
- */
- Long.prototype.toNumber = function () {
- return this.high_ * Long.TWO_PWR_32_DBL_ + this.getLowBitsUnsigned();
- };
-
- /**
- * Return the JSON value.
- *
- * @method
- * @return {string} the JSON representation.
- */
- Long.prototype.toJSON = function () {
- return this.toString();
- };
-
- /**
- * Return the String value.
- *
- * @method
- * @param {number} [opt_radix] the radix in which the text should be written.
- * @return {string} the textual representation of this value.
- */
- Long.prototype.toString = function (opt_radix) {
- var radix = opt_radix || 10;
- if (radix < 2 || 36 < radix) {
- throw Error('radix out of range: ' + radix);
- }
-
- if (this.isZero()) {
- return '0';
- }
-
- if (this.isNegative()) {
- if (this.equals(Long.MIN_VALUE)) {
- // We need to change the Long value before it can be negated, so we remove
- // the bottom-most digit in this base and then recurse to do the rest.
- var radixLong = Long.fromNumber(radix);
- var div = this.div(radixLong);
- var rem = div.multiply(radixLong).subtract(this);
- return div.toString(radix) + rem.toInt().toString(radix);
- } else {
- return '-' + this.negate().toString(radix);
- }
- }
-
- // Do several (6) digits each time through the loop, so as to
- // minimize the calls to the very expensive emulated div.
- var radixToPower = Long.fromNumber(Math.pow(radix, 6));
-
- rem = this;
- var result = '';
-
- while (!rem.isZero()) {
- var remDiv = rem.div(radixToPower);
- var intval = rem.subtract(remDiv.multiply(radixToPower)).toInt();
- var digits = intval.toString(radix);
-
- rem = remDiv;
- if (rem.isZero()) {
- return digits + result;
- } else {
- while (digits.length < 6) {
- digits = '0' + digits;
- }
- result = '' + digits + result;
- }
- }
- };
-
- /**
- * Return the high 32-bits value.
- *
- * @method
- * @return {number} the high 32-bits as a signed value.
- */
- Long.prototype.getHighBits = function () {
- return this.high_;
- };
-
- /**
- * Return the low 32-bits value.
- *
- * @method
- * @return {number} the low 32-bits as a signed value.
- */
- Long.prototype.getLowBits = function () {
- return this.low_;
- };
-
- /**
- * Return the low unsigned 32-bits value.
- *
- * @method
- * @return {number} the low 32-bits as an unsigned value.
- */
- Long.prototype.getLowBitsUnsigned = function () {
- return this.low_ >= 0 ? this.low_ : Long.TWO_PWR_32_DBL_ + this.low_;
- };
-
- /**
- * Returns the number of bits needed to represent the absolute value of this Long.
- *
- * @method
- * @return {number} Returns the number of bits needed to represent the absolute value of this Long.
- */
- Long.prototype.getNumBitsAbs = function () {
- if (this.isNegative()) {
- if (this.equals(Long.MIN_VALUE)) {
- return 64;
- } else {
- return this.negate().getNumBitsAbs();
- }
- } else {
- var val = this.high_ !== 0 ? this.high_ : this.low_;
- for (var bit = 31; bit > 0; bit--) {
- if ((val & 1 << bit) !== 0) {
- break;
- }
- }
- return this.high_ !== 0 ? bit + 33 : bit + 1;
- }
- };
-
- /**
- * Return whether this value is zero.
- *
- * @method
- * @return {boolean} whether this value is zero.
- */
- Long.prototype.isZero = function () {
- return this.high_ === 0 && this.low_ === 0;
- };
-
- /**
- * Return whether this value is negative.
- *
- * @method
- * @return {boolean} whether this value is negative.
- */
- Long.prototype.isNegative = function () {
- return this.high_ < 0;
- };
-
- /**
- * Return whether this value is odd.
- *
- * @method
- * @return {boolean} whether this value is odd.
- */
- Long.prototype.isOdd = function () {
- return (this.low_ & 1) === 1;
- };
-
- /**
- * Return whether this Long equals the other
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long equals the other
- */
- Long.prototype.equals = function (other) {
- return this.high_ === other.high_ && this.low_ === other.low_;
- };
-
- /**
- * Return whether this Long does not equal the other.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long does not equal the other.
- */
- Long.prototype.notEquals = function (other) {
- return this.high_ !== other.high_ || this.low_ !== other.low_;
- };
-
- /**
- * Return whether this Long is less than the other.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long is less than the other.
- */
- Long.prototype.lessThan = function (other) {
- return this.compare(other) < 0;
- };
-
- /**
- * Return whether this Long is less than or equal to the other.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long is less than or equal to the other.
- */
- Long.prototype.lessThanOrEqual = function (other) {
- return this.compare(other) <= 0;
- };
-
- /**
- * Return whether this Long is greater than the other.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long is greater than the other.
- */
- Long.prototype.greaterThan = function (other) {
- return this.compare(other) > 0;
- };
-
- /**
- * Return whether this Long is greater than or equal to the other.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long is greater than or equal to the other.
- */
- Long.prototype.greaterThanOrEqual = function (other) {
- return this.compare(other) >= 0;
- };
-
- /**
- * Compares this Long with the given one.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} 0 if they are the same, 1 if the this is greater, and -1 if the given one is greater.
- */
- Long.prototype.compare = function (other) {
- if (this.equals(other)) {
- return 0;
- }
-
- var thisNeg = this.isNegative();
- var otherNeg = other.isNegative();
- if (thisNeg && !otherNeg) {
- return -1;
- }
- if (!thisNeg && otherNeg) {
- return 1;
- }
-
- // at this point, the signs are the same, so subtraction will not overflow
- if (this.subtract(other).isNegative()) {
- return -1;
- } else {
- return 1;
- }
- };
-
- /**
- * The negation of this value.
- *
- * @method
- * @return {Long} the negation of this value.
- */
- Long.prototype.negate = function () {
- if (this.equals(Long.MIN_VALUE)) {
- return Long.MIN_VALUE;
- } else {
- return this.not().add(Long.ONE);
- }
- };
-
- /**
- * Returns the sum of this and the given Long.
- *
- * @method
- * @param {Long} other Long to add to this one.
- * @return {Long} the sum of this and the given Long.
- */
- Long.prototype.add = function (other) {
- // Divide each number into 4 chunks of 16 bits, and then sum the chunks.
-
- var a48 = this.high_ >>> 16;
- var a32 = this.high_ & 0xffff;
- var a16 = this.low_ >>> 16;
- var a00 = this.low_ & 0xffff;
-
- var b48 = other.high_ >>> 16;
- var b32 = other.high_ & 0xffff;
- var b16 = other.low_ >>> 16;
- var b00 = other.low_ & 0xffff;
-
- var c48 = 0,
- c32 = 0,
- c16 = 0,
- c00 = 0;
- c00 += a00 + b00;
- c16 += c00 >>> 16;
- c00 &= 0xffff;
- c16 += a16 + b16;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c32 += a32 + b32;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c48 += a48 + b48;
- c48 &= 0xffff;
- return Long.fromBits(c16 << 16 | c00, c48 << 16 | c32);
- };
-
- /**
- * Returns the difference of this and the given Long.
- *
- * @method
- * @param {Long} other Long to subtract from this.
- * @return {Long} the difference of this and the given Long.
- */
- Long.prototype.subtract = function (other) {
- return this.add(other.negate());
- };
-
- /**
- * Returns the product of this and the given Long.
- *
- * @method
- * @param {Long} other Long to multiply with this.
- * @return {Long} the product of this and the other.
- */
- Long.prototype.multiply = function (other) {
- if (this.isZero()) {
- return Long.ZERO;
- } else if (other.isZero()) {
- return Long.ZERO;
- }
-
- if (this.equals(Long.MIN_VALUE)) {
- return other.isOdd() ? Long.MIN_VALUE : Long.ZERO;
- } else if (other.equals(Long.MIN_VALUE)) {
- return this.isOdd() ? Long.MIN_VALUE : Long.ZERO;
- }
-
- if (this.isNegative()) {
- if (other.isNegative()) {
- return this.negate().multiply(other.negate());
- } else {
- return this.negate().multiply(other).negate();
- }
- } else if (other.isNegative()) {
- return this.multiply(other.negate()).negate();
- }
-
- // If both Longs are small, use float multiplication
- if (this.lessThan(Long.TWO_PWR_24_) && other.lessThan(Long.TWO_PWR_24_)) {
- return Long.fromNumber(this.toNumber() * other.toNumber());
- }
-
- // Divide each Long into 4 chunks of 16 bits, and then add up 4x4 products.
- // We can skip products that would overflow.
-
- var a48 = this.high_ >>> 16;
- var a32 = this.high_ & 0xffff;
- var a16 = this.low_ >>> 16;
- var a00 = this.low_ & 0xffff;
-
- var b48 = other.high_ >>> 16;
- var b32 = other.high_ & 0xffff;
- var b16 = other.low_ >>> 16;
- var b00 = other.low_ & 0xffff;
-
- var c48 = 0,
- c32 = 0,
- c16 = 0,
- c00 = 0;
- c00 += a00 * b00;
- c16 += c00 >>> 16;
- c00 &= 0xffff;
- c16 += a16 * b00;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c16 += a00 * b16;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c32 += a32 * b00;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c32 += a16 * b16;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c32 += a00 * b32;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;
- c48 &= 0xffff;
- return Long.fromBits(c16 << 16 | c00, c48 << 16 | c32);
- };
-
- /**
- * Returns this Long divided by the given one.
- *
- * @method
- * @param {Long} other Long by which to divide.
- * @return {Long} this Long divided by the given one.
- */
- Long.prototype.div = function (other) {
- if (other.isZero()) {
- throw Error('division by zero');
- } else if (this.isZero()) {
- return Long.ZERO;
- }
-
- if (this.equals(Long.MIN_VALUE)) {
- if (other.equals(Long.ONE) || other.equals(Long.NEG_ONE)) {
- return Long.MIN_VALUE; // recall that -MIN_VALUE == MIN_VALUE
- } else if (other.equals(Long.MIN_VALUE)) {
- return Long.ONE;
- } else {
- // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.
- var halfThis = this.shiftRight(1);
- var approx = halfThis.div(other).shiftLeft(1);
- if (approx.equals(Long.ZERO)) {
- return other.isNegative() ? Long.ONE : Long.NEG_ONE;
- } else {
- var rem = this.subtract(other.multiply(approx));
- var result = approx.add(rem.div(other));
- return result;
- }
- }
- } else if (other.equals(Long.MIN_VALUE)) {
- return Long.ZERO;
- }
-
- if (this.isNegative()) {
- if (other.isNegative()) {
- return this.negate().div(other.negate());
- } else {
- return this.negate().div(other).negate();
- }
- } else if (other.isNegative()) {
- return this.div(other.negate()).negate();
- }
-
- // Repeat the following until the remainder is less than other: find a
- // floating-point that approximates remainder / other *from below*, add this
- // into the result, and subtract it from the remainder. It is critical that
- // the approximate value is less than or equal to the real value so that the
- // remainder never becomes negative.
- var res = Long.ZERO;
- rem = this;
- while (rem.greaterThanOrEqual(other)) {
- // Approximate the result of division. This may be a little greater or
- // smaller than the actual value.
- approx = Math.max(1, Math.floor(rem.toNumber() / other.toNumber()));
-
- // We will tweak the approximate result by changing it in the 48-th digit or
- // the smallest non-fractional digit, whichever is larger.
- var log2 = Math.ceil(Math.log(approx) / Math.LN2);
- var delta = log2 <= 48 ? 1 : Math.pow(2, log2 - 48);
-
- // Decrease the approximation until it is smaller than the remainder. Note
- // that if it is too large, the product overflows and is negative.
- var approxRes = Long.fromNumber(approx);
- var approxRem = approxRes.multiply(other);
- while (approxRem.isNegative() || approxRem.greaterThan(rem)) {
- approx -= delta;
- approxRes = Long.fromNumber(approx);
- approxRem = approxRes.multiply(other);
- }
-
- // We know the answer can't be zero... and actually, zero would cause
- // infinite recursion since we would make no progress.
- if (approxRes.isZero()) {
- approxRes = Long.ONE;
- }
-
- res = res.add(approxRes);
- rem = rem.subtract(approxRem);
- }
- return res;
- };
-
- /**
- * Returns this Long modulo the given one.
- *
- * @method
- * @param {Long} other Long by which to mod.
- * @return {Long} this Long modulo the given one.
- */
- Long.prototype.modulo = function (other) {
- return this.subtract(this.div(other).multiply(other));
- };
-
- /**
- * The bitwise-NOT of this value.
- *
- * @method
- * @return {Long} the bitwise-NOT of this value.
- */
- Long.prototype.not = function () {
- return Long.fromBits(~this.low_, ~this.high_);
- };
-
- /**
- * Returns the bitwise-AND of this Long and the given one.
- *
- * @method
- * @param {Long} other the Long with which to AND.
- * @return {Long} the bitwise-AND of this and the other.
- */
- Long.prototype.and = function (other) {
- return Long.fromBits(this.low_ & other.low_, this.high_ & other.high_);
- };
-
- /**
- * Returns the bitwise-OR of this Long and the given one.
- *
- * @method
- * @param {Long} other the Long with which to OR.
- * @return {Long} the bitwise-OR of this and the other.
- */
- Long.prototype.or = function (other) {
- return Long.fromBits(this.low_ | other.low_, this.high_ | other.high_);
- };
-
- /**
- * Returns the bitwise-XOR of this Long and the given one.
- *
- * @method
- * @param {Long} other the Long with which to XOR.
- * @return {Long} the bitwise-XOR of this and the other.
- */
- Long.prototype.xor = function (other) {
- return Long.fromBits(this.low_ ^ other.low_, this.high_ ^ other.high_);
- };
-
- /**
- * Returns this Long with bits shifted to the left by the given amount.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Long} this shifted to the left by the given amount.
- */
- Long.prototype.shiftLeft = function (numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var low = this.low_;
- if (numBits < 32) {
- var high = this.high_;
- return Long.fromBits(low << numBits, high << numBits | low >>> 32 - numBits);
- } else {
- return Long.fromBits(0, low << numBits - 32);
- }
- }
- };
-
- /**
- * Returns this Long with bits shifted to the right by the given amount.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Long} this shifted to the right by the given amount.
- */
- Long.prototype.shiftRight = function (numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var high = this.high_;
- if (numBits < 32) {
- var low = this.low_;
- return Long.fromBits(low >>> numBits | high << 32 - numBits, high >> numBits);
- } else {
- return Long.fromBits(high >> numBits - 32, high >= 0 ? 0 : -1);
- }
- }
- };
-
- /**
- * Returns this Long with bits shifted to the right by the given amount, with the new top bits matching the current sign bit.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Long} this shifted to the right by the given amount, with zeros placed into the new leading bits.
- */
- Long.prototype.shiftRightUnsigned = function (numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var high = this.high_;
- if (numBits < 32) {
- var low = this.low_;
- return Long.fromBits(low >>> numBits | high << 32 - numBits, high >>> numBits);
- } else if (numBits === 32) {
- return Long.fromBits(high, 0);
- } else {
- return Long.fromBits(high >>> numBits - 32, 0);
- }
- }
- };
-
- /**
- * Returns a Long representing the given (32-bit) integer value.
- *
- * @method
- * @param {number} value the 32-bit integer in question.
- * @return {Long} the corresponding Long value.
- */
- Long.fromInt = function (value) {
- if (-128 <= value && value < 128) {
- var cachedObj = Long.INT_CACHE_[value];
- if (cachedObj) {
- return cachedObj;
- }
- }
-
- var obj = new Long(value | 0, value < 0 ? -1 : 0);
- if (-128 <= value && value < 128) {
- Long.INT_CACHE_[value] = obj;
- }
- return obj;
- };
-
- /**
- * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
- *
- * @method
- * @param {number} value the number in question.
- * @return {Long} the corresponding Long value.
- */
- Long.fromNumber = function (value) {
- if (isNaN(value) || !isFinite(value)) {
- return Long.ZERO;
- } else if (value <= -Long.TWO_PWR_63_DBL_) {
- return Long.MIN_VALUE;
- } else if (value + 1 >= Long.TWO_PWR_63_DBL_) {
- return Long.MAX_VALUE;
- } else if (value < 0) {
- return Long.fromNumber(-value).negate();
- } else {
- return new Long(value % Long.TWO_PWR_32_DBL_ | 0, value / Long.TWO_PWR_32_DBL_ | 0);
- }
- };
-
- /**
- * Returns a Long representing the 64-bit integer that comes by concatenating the given high and low bits. Each is assumed to use 32 bits.
- *
- * @method
- * @param {number} lowBits the low 32-bits.
- * @param {number} highBits the high 32-bits.
- * @return {Long} the corresponding Long value.
- */
- Long.fromBits = function (lowBits, highBits) {
- return new Long(lowBits, highBits);
- };
-
- /**
- * Returns a Long representation of the given string, written using the given radix.
- *
- * @method
- * @param {string} str the textual representation of the Long.
- * @param {number} opt_radix the radix in which the text is written.
- * @return {Long} the corresponding Long value.
- */
- Long.fromString = function (str, opt_radix) {
- if (str.length === 0) {
- throw Error('number format error: empty string');
- }
-
- var radix = opt_radix || 10;
- if (radix < 2 || 36 < radix) {
- throw Error('radix out of range: ' + radix);
- }
-
- if (str.charAt(0) === '-') {
- return Long.fromString(str.substring(1), radix).negate();
- } else if (str.indexOf('-') >= 0) {
- throw Error('number format error: interior "-" character: ' + str);
- }
-
- // Do several (8) digits each time through the loop, so as to
- // minimize the calls to the very expensive emulated div.
- var radixToPower = Long.fromNumber(Math.pow(radix, 8));
-
- var result = Long.ZERO;
- for (var i = 0; i < str.length; i += 8) {
- var size = Math.min(8, str.length - i);
- var value = parseInt(str.substring(i, i + size), radix);
- if (size < 8) {
- var power = Long.fromNumber(Math.pow(radix, size));
- result = result.multiply(power).add(Long.fromNumber(value));
- } else {
- result = result.multiply(radixToPower);
- result = result.add(Long.fromNumber(value));
- }
- }
- return result;
- };
-
- // NOTE: Common constant values ZERO, ONE, NEG_ONE, etc. are defined below the
- // from* methods on which they depend.
-
- /**
- * A cache of the Long representations of small integer values.
- * @type {Object}
- * @ignore
- */
- Long.INT_CACHE_ = {};
-
- // NOTE: the compiler should inline these constant values below and then remove
- // these variables, so there should be no runtime penalty for these.
-
- /**
- * Number used repeated below in calculations. This must appear before the
- * first call to any from* function below.
- * @type {number}
- * @ignore
- */
- Long.TWO_PWR_16_DBL_ = 1 << 16;
-
- /**
- * @type {number}
- * @ignore
- */
- Long.TWO_PWR_24_DBL_ = 1 << 24;
-
- /**
- * @type {number}
- * @ignore
- */
- Long.TWO_PWR_32_DBL_ = Long.TWO_PWR_16_DBL_ * Long.TWO_PWR_16_DBL_;
-
- /**
- * @type {number}
- * @ignore
- */
- Long.TWO_PWR_31_DBL_ = Long.TWO_PWR_32_DBL_ / 2;
-
- /**
- * @type {number}
- * @ignore
- */
- Long.TWO_PWR_48_DBL_ = Long.TWO_PWR_32_DBL_ * Long.TWO_PWR_16_DBL_;
-
- /**
- * @type {number}
- * @ignore
- */
- Long.TWO_PWR_64_DBL_ = Long.TWO_PWR_32_DBL_ * Long.TWO_PWR_32_DBL_;
-
- /**
- * @type {number}
- * @ignore
- */
- Long.TWO_PWR_63_DBL_ = Long.TWO_PWR_64_DBL_ / 2;
-
- /** @type {Long} */
- Long.ZERO = Long.fromInt(0);
-
- /** @type {Long} */
- Long.ONE = Long.fromInt(1);
-
- /** @type {Long} */
- Long.NEG_ONE = Long.fromInt(-1);
-
- /** @type {Long} */
- Long.MAX_VALUE = Long.fromBits(0xffffffff | 0, 0x7fffffff | 0);
-
- /** @type {Long} */
- Long.MIN_VALUE = Long.fromBits(0, 0x80000000 | 0);
-
- /**
- * @type {Long}
- * @ignore
- */
- Long.TWO_PWR_24_ = Long.fromInt(1 << 24);
-
- /**
- * Expose.
- */
- module.exports = Long;
- module.exports.Long = Long;
-
-/***/ }),
-/* 336 */
-/***/ (function(module, exports) {
-
- /**
- * A class representation of the BSON Double type.
- *
- * @class
- * @param {number} value the number we want to represent as a double.
- * @return {Double}
- */
- function Double(value) {
- if (!(this instanceof Double)) return new Double(value);
-
- this._bsontype = 'Double';
- this.value = value;
- }
-
- /**
- * Access the number value.
- *
- * @method
- * @return {number} returns the wrapped double number.
- */
- Double.prototype.valueOf = function () {
- return this.value;
- };
-
- /**
- * @ignore
- */
- Double.prototype.toJSON = function () {
- return this.value;
- };
-
- module.exports = Double;
- module.exports.Double = Double;
-
-/***/ }),
-/* 337 */
-/***/ (function(module, exports) {
-
- // Licensed under the Apache License, Version 2.0 (the "License");
- // you may not use this file except in compliance with the License.
- // You may obtain a copy of the License at
- //
- // http://www.apache.org/licenses/LICENSE-2.0
- //
- // Unless required by applicable law or agreed to in writing, software
- // distributed under the License is distributed on an "AS IS" BASIS,
- // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
- // See the License for the specific language governing permissions and
- // limitations under the License.
- //
- // Copyright 2009 Google Inc. All Rights Reserved
-
- /**
- * This type is for INTERNAL use in MongoDB only and should not be used in applications.
- * The appropriate corresponding type is the JavaScript Date type.
- *
- * Defines a Timestamp class for representing a 64-bit two's-complement
- * integer value, which faithfully simulates the behavior of a Java "Timestamp". This
- * implementation is derived from TimestampLib in GWT.
- *
- * Constructs a 64-bit two's-complement integer, given its low and high 32-bit
- * values as *signed* integers. See the from* functions below for more
- * convenient ways of constructing Timestamps.
- *
- * The internal representation of a Timestamp is the two given signed, 32-bit values.
- * We use 32-bit pieces because these are the size of integers on which
- * Javascript performs bit-operations. For operations like addition and
- * multiplication, we split each number into 16-bit pieces, which can easily be
- * multiplied within Javascript's floating-point representation without overflow
- * or change in sign.
- *
- * In the algorithms below, we frequently reduce the negative case to the
- * positive case by negating the input(s) and then post-processing the result.
- * Note that we must ALWAYS check specially whether those values are MIN_VALUE
- * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
- * a positive number, it overflows back into a negative). Not handling this
- * case would often result in infinite recursion.
- *
- * @class
- * @param {number} low the low (signed) 32 bits of the Timestamp.
- * @param {number} high the high (signed) 32 bits of the Timestamp.
- */
- function Timestamp(low, high) {
- if (!(this instanceof Timestamp)) return new Timestamp(low, high);
- this._bsontype = 'Timestamp';
- /**
- * @type {number}
- * @ignore
- */
- this.low_ = low | 0; // force into 32 signed bits.
-
- /**
- * @type {number}
- * @ignore
- */
- this.high_ = high | 0; // force into 32 signed bits.
- }
-
- /**
- * Return the int value.
- *
- * @return {number} the value, assuming it is a 32-bit integer.
- */
- Timestamp.prototype.toInt = function () {
- return this.low_;
- };
-
- /**
- * Return the Number value.
- *
- * @method
- * @return {number} the closest floating-point representation to this value.
- */
- Timestamp.prototype.toNumber = function () {
- return this.high_ * Timestamp.TWO_PWR_32_DBL_ + this.getLowBitsUnsigned();
- };
-
- /**
- * Return the JSON value.
- *
- * @method
- * @return {string} the JSON representation.
- */
- Timestamp.prototype.toJSON = function () {
- return this.toString();
- };
-
- /**
- * Return the String value.
- *
- * @method
- * @param {number} [opt_radix] the radix in which the text should be written.
- * @return {string} the textual representation of this value.
- */
- Timestamp.prototype.toString = function (opt_radix) {
- var radix = opt_radix || 10;
- if (radix < 2 || 36 < radix) {
- throw Error('radix out of range: ' + radix);
- }
-
- if (this.isZero()) {
- return '0';
- }
-
- if (this.isNegative()) {
- if (this.equals(Timestamp.MIN_VALUE)) {
- // We need to change the Timestamp value before it can be negated, so we remove
- // the bottom-most digit in this base and then recurse to do the rest.
- var radixTimestamp = Timestamp.fromNumber(radix);
- var div = this.div(radixTimestamp);
- var rem = div.multiply(radixTimestamp).subtract(this);
- return div.toString(radix) + rem.toInt().toString(radix);
- } else {
- return '-' + this.negate().toString(radix);
- }
- }
-
- // Do several (6) digits each time through the loop, so as to
- // minimize the calls to the very expensive emulated div.
- var radixToPower = Timestamp.fromNumber(Math.pow(radix, 6));
-
- rem = this;
- var result = '';
-
- while (!rem.isZero()) {
- var remDiv = rem.div(radixToPower);
- var intval = rem.subtract(remDiv.multiply(radixToPower)).toInt();
- var digits = intval.toString(radix);
-
- rem = remDiv;
- if (rem.isZero()) {
- return digits + result;
- } else {
- while (digits.length < 6) {
- digits = '0' + digits;
- }
- result = '' + digits + result;
- }
- }
- };
-
- /**
- * Return the high 32-bits value.
- *
- * @method
- * @return {number} the high 32-bits as a signed value.
- */
- Timestamp.prototype.getHighBits = function () {
- return this.high_;
- };
-
- /**
- * Return the low 32-bits value.
- *
- * @method
- * @return {number} the low 32-bits as a signed value.
- */
- Timestamp.prototype.getLowBits = function () {
- return this.low_;
- };
-
- /**
- * Return the low unsigned 32-bits value.
- *
- * @method
- * @return {number} the low 32-bits as an unsigned value.
- */
- Timestamp.prototype.getLowBitsUnsigned = function () {
- return this.low_ >= 0 ? this.low_ : Timestamp.TWO_PWR_32_DBL_ + this.low_;
- };
-
- /**
- * Returns the number of bits needed to represent the absolute value of this Timestamp.
- *
- * @method
- * @return {number} Returns the number of bits needed to represent the absolute value of this Timestamp.
- */
- Timestamp.prototype.getNumBitsAbs = function () {
- if (this.isNegative()) {
- if (this.equals(Timestamp.MIN_VALUE)) {
- return 64;
- } else {
- return this.negate().getNumBitsAbs();
- }
- } else {
- var val = this.high_ !== 0 ? this.high_ : this.low_;
- for (var bit = 31; bit > 0; bit--) {
- if ((val & 1 << bit) !== 0) {
- break;
- }
- }
- return this.high_ !== 0 ? bit + 33 : bit + 1;
- }
- };
-
- /**
- * Return whether this value is zero.
- *
- * @method
- * @return {boolean} whether this value is zero.
- */
- Timestamp.prototype.isZero = function () {
- return this.high_ === 0 && this.low_ === 0;
- };
-
- /**
- * Return whether this value is negative.
- *
- * @method
- * @return {boolean} whether this value is negative.
- */
- Timestamp.prototype.isNegative = function () {
- return this.high_ < 0;
- };
-
- /**
- * Return whether this value is odd.
- *
- * @method
- * @return {boolean} whether this value is odd.
- */
- Timestamp.prototype.isOdd = function () {
- return (this.low_ & 1) === 1;
- };
-
- /**
- * Return whether this Timestamp equals the other
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp equals the other
- */
- Timestamp.prototype.equals = function (other) {
- return this.high_ === other.high_ && this.low_ === other.low_;
- };
-
- /**
- * Return whether this Timestamp does not equal the other.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp does not equal the other.
- */
- Timestamp.prototype.notEquals = function (other) {
- return this.high_ !== other.high_ || this.low_ !== other.low_;
- };
-
- /**
- * Return whether this Timestamp is less than the other.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp is less than the other.
- */
- Timestamp.prototype.lessThan = function (other) {
- return this.compare(other) < 0;
- };
-
- /**
- * Return whether this Timestamp is less than or equal to the other.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp is less than or equal to the other.
- */
- Timestamp.prototype.lessThanOrEqual = function (other) {
- return this.compare(other) <= 0;
- };
-
- /**
- * Return whether this Timestamp is greater than the other.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp is greater than the other.
- */
- Timestamp.prototype.greaterThan = function (other) {
- return this.compare(other) > 0;
- };
-
- /**
- * Return whether this Timestamp is greater than or equal to the other.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp is greater than or equal to the other.
- */
- Timestamp.prototype.greaterThanOrEqual = function (other) {
- return this.compare(other) >= 0;
- };
-
- /**
- * Compares this Timestamp with the given one.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} 0 if they are the same, 1 if the this is greater, and -1 if the given one is greater.
- */
- Timestamp.prototype.compare = function (other) {
- if (this.equals(other)) {
- return 0;
- }
-
- var thisNeg = this.isNegative();
- var otherNeg = other.isNegative();
- if (thisNeg && !otherNeg) {
- return -1;
- }
- if (!thisNeg && otherNeg) {
- return 1;
- }
-
- // at this point, the signs are the same, so subtraction will not overflow
- if (this.subtract(other).isNegative()) {
- return -1;
- } else {
- return 1;
- }
- };
-
- /**
- * The negation of this value.
- *
- * @method
- * @return {Timestamp} the negation of this value.
- */
- Timestamp.prototype.negate = function () {
- if (this.equals(Timestamp.MIN_VALUE)) {
- return Timestamp.MIN_VALUE;
- } else {
- return this.not().add(Timestamp.ONE);
- }
- };
-
- /**
- * Returns the sum of this and the given Timestamp.
- *
- * @method
- * @param {Timestamp} other Timestamp to add to this one.
- * @return {Timestamp} the sum of this and the given Timestamp.
- */
- Timestamp.prototype.add = function (other) {
- // Divide each number into 4 chunks of 16 bits, and then sum the chunks.
-
- var a48 = this.high_ >>> 16;
- var a32 = this.high_ & 0xffff;
- var a16 = this.low_ >>> 16;
- var a00 = this.low_ & 0xffff;
-
- var b48 = other.high_ >>> 16;
- var b32 = other.high_ & 0xffff;
- var b16 = other.low_ >>> 16;
- var b00 = other.low_ & 0xffff;
-
- var c48 = 0,
- c32 = 0,
- c16 = 0,
- c00 = 0;
- c00 += a00 + b00;
- c16 += c00 >>> 16;
- c00 &= 0xffff;
- c16 += a16 + b16;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c32 += a32 + b32;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c48 += a48 + b48;
- c48 &= 0xffff;
- return Timestamp.fromBits(c16 << 16 | c00, c48 << 16 | c32);
- };
-
- /**
- * Returns the difference of this and the given Timestamp.
- *
- * @method
- * @param {Timestamp} other Timestamp to subtract from this.
- * @return {Timestamp} the difference of this and the given Timestamp.
- */
- Timestamp.prototype.subtract = function (other) {
- return this.add(other.negate());
- };
-
- /**
- * Returns the product of this and the given Timestamp.
- *
- * @method
- * @param {Timestamp} other Timestamp to multiply with this.
- * @return {Timestamp} the product of this and the other.
- */
- Timestamp.prototype.multiply = function (other) {
- if (this.isZero()) {
- return Timestamp.ZERO;
- } else if (other.isZero()) {
- return Timestamp.ZERO;
- }
-
- if (this.equals(Timestamp.MIN_VALUE)) {
- return other.isOdd() ? Timestamp.MIN_VALUE : Timestamp.ZERO;
- } else if (other.equals(Timestamp.MIN_VALUE)) {
- return this.isOdd() ? Timestamp.MIN_VALUE : Timestamp.ZERO;
- }
-
- if (this.isNegative()) {
- if (other.isNegative()) {
- return this.negate().multiply(other.negate());
- } else {
- return this.negate().multiply(other).negate();
- }
- } else if (other.isNegative()) {
- return this.multiply(other.negate()).negate();
- }
-
- // If both Timestamps are small, use float multiplication
- if (this.lessThan(Timestamp.TWO_PWR_24_) && other.lessThan(Timestamp.TWO_PWR_24_)) {
- return Timestamp.fromNumber(this.toNumber() * other.toNumber());
- }
-
- // Divide each Timestamp into 4 chunks of 16 bits, and then add up 4x4 products.
- // We can skip products that would overflow.
-
- var a48 = this.high_ >>> 16;
- var a32 = this.high_ & 0xffff;
- var a16 = this.low_ >>> 16;
- var a00 = this.low_ & 0xffff;
-
- var b48 = other.high_ >>> 16;
- var b32 = other.high_ & 0xffff;
- var b16 = other.low_ >>> 16;
- var b00 = other.low_ & 0xffff;
-
- var c48 = 0,
- c32 = 0,
- c16 = 0,
- c00 = 0;
- c00 += a00 * b00;
- c16 += c00 >>> 16;
- c00 &= 0xffff;
- c16 += a16 * b00;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c16 += a00 * b16;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c32 += a32 * b00;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c32 += a16 * b16;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c32 += a00 * b32;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;
- c48 &= 0xffff;
- return Timestamp.fromBits(c16 << 16 | c00, c48 << 16 | c32);
- };
-
- /**
- * Returns this Timestamp divided by the given one.
- *
- * @method
- * @param {Timestamp} other Timestamp by which to divide.
- * @return {Timestamp} this Timestamp divided by the given one.
- */
- Timestamp.prototype.div = function (other) {
- if (other.isZero()) {
- throw Error('division by zero');
- } else if (this.isZero()) {
- return Timestamp.ZERO;
- }
-
- if (this.equals(Timestamp.MIN_VALUE)) {
- if (other.equals(Timestamp.ONE) || other.equals(Timestamp.NEG_ONE)) {
- return Timestamp.MIN_VALUE; // recall that -MIN_VALUE == MIN_VALUE
- } else if (other.equals(Timestamp.MIN_VALUE)) {
- return Timestamp.ONE;
- } else {
- // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.
- var halfThis = this.shiftRight(1);
- var approx = halfThis.div(other).shiftLeft(1);
- if (approx.equals(Timestamp.ZERO)) {
- return other.isNegative() ? Timestamp.ONE : Timestamp.NEG_ONE;
- } else {
- var rem = this.subtract(other.multiply(approx));
- var result = approx.add(rem.div(other));
- return result;
- }
- }
- } else if (other.equals(Timestamp.MIN_VALUE)) {
- return Timestamp.ZERO;
- }
-
- if (this.isNegative()) {
- if (other.isNegative()) {
- return this.negate().div(other.negate());
- } else {
- return this.negate().div(other).negate();
- }
- } else if (other.isNegative()) {
- return this.div(other.negate()).negate();
- }
-
- // Repeat the following until the remainder is less than other: find a
- // floating-point that approximates remainder / other *from below*, add this
- // into the result, and subtract it from the remainder. It is critical that
- // the approximate value is less than or equal to the real value so that the
- // remainder never becomes negative.
- var res = Timestamp.ZERO;
- rem = this;
- while (rem.greaterThanOrEqual(other)) {
- // Approximate the result of division. This may be a little greater or
- // smaller than the actual value.
- approx = Math.max(1, Math.floor(rem.toNumber() / other.toNumber()));
-
- // We will tweak the approximate result by changing it in the 48-th digit or
- // the smallest non-fractional digit, whichever is larger.
- var log2 = Math.ceil(Math.log(approx) / Math.LN2);
- var delta = log2 <= 48 ? 1 : Math.pow(2, log2 - 48);
-
- // Decrease the approximation until it is smaller than the remainder. Note
- // that if it is too large, the product overflows and is negative.
- var approxRes = Timestamp.fromNumber(approx);
- var approxRem = approxRes.multiply(other);
- while (approxRem.isNegative() || approxRem.greaterThan(rem)) {
- approx -= delta;
- approxRes = Timestamp.fromNumber(approx);
- approxRem = approxRes.multiply(other);
- }
-
- // We know the answer can't be zero... and actually, zero would cause
- // infinite recursion since we would make no progress.
- if (approxRes.isZero()) {
- approxRes = Timestamp.ONE;
- }
-
- res = res.add(approxRes);
- rem = rem.subtract(approxRem);
- }
- return res;
- };
-
- /**
- * Returns this Timestamp modulo the given one.
- *
- * @method
- * @param {Timestamp} other Timestamp by which to mod.
- * @return {Timestamp} this Timestamp modulo the given one.
- */
- Timestamp.prototype.modulo = function (other) {
- return this.subtract(this.div(other).multiply(other));
- };
-
- /**
- * The bitwise-NOT of this value.
- *
- * @method
- * @return {Timestamp} the bitwise-NOT of this value.
- */
- Timestamp.prototype.not = function () {
- return Timestamp.fromBits(~this.low_, ~this.high_);
- };
-
- /**
- * Returns the bitwise-AND of this Timestamp and the given one.
- *
- * @method
- * @param {Timestamp} other the Timestamp with which to AND.
- * @return {Timestamp} the bitwise-AND of this and the other.
- */
- Timestamp.prototype.and = function (other) {
- return Timestamp.fromBits(this.low_ & other.low_, this.high_ & other.high_);
- };
-
- /**
- * Returns the bitwise-OR of this Timestamp and the given one.
- *
- * @method
- * @param {Timestamp} other the Timestamp with which to OR.
- * @return {Timestamp} the bitwise-OR of this and the other.
- */
- Timestamp.prototype.or = function (other) {
- return Timestamp.fromBits(this.low_ | other.low_, this.high_ | other.high_);
- };
-
- /**
- * Returns the bitwise-XOR of this Timestamp and the given one.
- *
- * @method
- * @param {Timestamp} other the Timestamp with which to XOR.
- * @return {Timestamp} the bitwise-XOR of this and the other.
- */
- Timestamp.prototype.xor = function (other) {
- return Timestamp.fromBits(this.low_ ^ other.low_, this.high_ ^ other.high_);
- };
-
- /**
- * Returns this Timestamp with bits shifted to the left by the given amount.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Timestamp} this shifted to the left by the given amount.
- */
- Timestamp.prototype.shiftLeft = function (numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var low = this.low_;
- if (numBits < 32) {
- var high = this.high_;
- return Timestamp.fromBits(low << numBits, high << numBits | low >>> 32 - numBits);
- } else {
- return Timestamp.fromBits(0, low << numBits - 32);
- }
- }
- };
-
- /**
- * Returns this Timestamp with bits shifted to the right by the given amount.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Timestamp} this shifted to the right by the given amount.
- */
- Timestamp.prototype.shiftRight = function (numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var high = this.high_;
- if (numBits < 32) {
- var low = this.low_;
- return Timestamp.fromBits(low >>> numBits | high << 32 - numBits, high >> numBits);
- } else {
- return Timestamp.fromBits(high >> numBits - 32, high >= 0 ? 0 : -1);
- }
- }
- };
-
- /**
- * Returns this Timestamp with bits shifted to the right by the given amount, with the new top bits matching the current sign bit.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Timestamp} this shifted to the right by the given amount, with zeros placed into the new leading bits.
- */
- Timestamp.prototype.shiftRightUnsigned = function (numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var high = this.high_;
- if (numBits < 32) {
- var low = this.low_;
- return Timestamp.fromBits(low >>> numBits | high << 32 - numBits, high >>> numBits);
- } else if (numBits === 32) {
- return Timestamp.fromBits(high, 0);
- } else {
- return Timestamp.fromBits(high >>> numBits - 32, 0);
- }
- }
- };
-
- /**
- * Returns a Timestamp representing the given (32-bit) integer value.
- *
- * @method
- * @param {number} value the 32-bit integer in question.
- * @return {Timestamp} the corresponding Timestamp value.
- */
- Timestamp.fromInt = function (value) {
- if (-128 <= value && value < 128) {
- var cachedObj = Timestamp.INT_CACHE_[value];
- if (cachedObj) {
- return cachedObj;
- }
- }
-
- var obj = new Timestamp(value | 0, value < 0 ? -1 : 0);
- if (-128 <= value && value < 128) {
- Timestamp.INT_CACHE_[value] = obj;
- }
- return obj;
- };
-
- /**
- * Returns a Timestamp representing the given value, provided that it is a finite number. Otherwise, zero is returned.
- *
- * @method
- * @param {number} value the number in question.
- * @return {Timestamp} the corresponding Timestamp value.
- */
- Timestamp.fromNumber = function (value) {
- if (isNaN(value) || !isFinite(value)) {
- return Timestamp.ZERO;
- } else if (value <= -Timestamp.TWO_PWR_63_DBL_) {
- return Timestamp.MIN_VALUE;
- } else if (value + 1 >= Timestamp.TWO_PWR_63_DBL_) {
- return Timestamp.MAX_VALUE;
- } else if (value < 0) {
- return Timestamp.fromNumber(-value).negate();
- } else {
- return new Timestamp(value % Timestamp.TWO_PWR_32_DBL_ | 0, value / Timestamp.TWO_PWR_32_DBL_ | 0);
- }
- };
-
- /**
- * Returns a Timestamp representing the 64-bit integer that comes by concatenating the given high and low bits. Each is assumed to use 32 bits.
- *
- * @method
- * @param {number} lowBits the low 32-bits.
- * @param {number} highBits the high 32-bits.
- * @return {Timestamp} the corresponding Timestamp value.
- */
- Timestamp.fromBits = function (lowBits, highBits) {
- return new Timestamp(lowBits, highBits);
- };
-
- /**
- * Returns a Timestamp representation of the given string, written using the given radix.
- *
- * @method
- * @param {string} str the textual representation of the Timestamp.
- * @param {number} opt_radix the radix in which the text is written.
- * @return {Timestamp} the corresponding Timestamp value.
- */
- Timestamp.fromString = function (str, opt_radix) {
- if (str.length === 0) {
- throw Error('number format error: empty string');
- }
-
- var radix = opt_radix || 10;
- if (radix < 2 || 36 < radix) {
- throw Error('radix out of range: ' + radix);
- }
-
- if (str.charAt(0) === '-') {
- return Timestamp.fromString(str.substring(1), radix).negate();
- } else if (str.indexOf('-') >= 0) {
- throw Error('number format error: interior "-" character: ' + str);
- }
-
- // Do several (8) digits each time through the loop, so as to
- // minimize the calls to the very expensive emulated div.
- var radixToPower = Timestamp.fromNumber(Math.pow(radix, 8));
-
- var result = Timestamp.ZERO;
- for (var i = 0; i < str.length; i += 8) {
- var size = Math.min(8, str.length - i);
- var value = parseInt(str.substring(i, i + size), radix);
- if (size < 8) {
- var power = Timestamp.fromNumber(Math.pow(radix, size));
- result = result.multiply(power).add(Timestamp.fromNumber(value));
- } else {
- result = result.multiply(radixToPower);
- result = result.add(Timestamp.fromNumber(value));
- }
- }
- return result;
- };
-
- // NOTE: Common constant values ZERO, ONE, NEG_ONE, etc. are defined below the
- // from* methods on which they depend.
-
- /**
- * A cache of the Timestamp representations of small integer values.
- * @type {Object}
- * @ignore
- */
- Timestamp.INT_CACHE_ = {};
-
- // NOTE: the compiler should inline these constant values below and then remove
- // these variables, so there should be no runtime penalty for these.
-
- /**
- * Number used repeated below in calculations. This must appear before the
- * first call to any from* function below.
- * @type {number}
- * @ignore
- */
- Timestamp.TWO_PWR_16_DBL_ = 1 << 16;
-
- /**
- * @type {number}
- * @ignore
- */
- Timestamp.TWO_PWR_24_DBL_ = 1 << 24;
-
- /**
- * @type {number}
- * @ignore
- */
- Timestamp.TWO_PWR_32_DBL_ = Timestamp.TWO_PWR_16_DBL_ * Timestamp.TWO_PWR_16_DBL_;
-
- /**
- * @type {number}
- * @ignore
- */
- Timestamp.TWO_PWR_31_DBL_ = Timestamp.TWO_PWR_32_DBL_ / 2;
-
- /**
- * @type {number}
- * @ignore
- */
- Timestamp.TWO_PWR_48_DBL_ = Timestamp.TWO_PWR_32_DBL_ * Timestamp.TWO_PWR_16_DBL_;
-
- /**
- * @type {number}
- * @ignore
- */
- Timestamp.TWO_PWR_64_DBL_ = Timestamp.TWO_PWR_32_DBL_ * Timestamp.TWO_PWR_32_DBL_;
-
- /**
- * @type {number}
- * @ignore
- */
- Timestamp.TWO_PWR_63_DBL_ = Timestamp.TWO_PWR_64_DBL_ / 2;
-
- /** @type {Timestamp} */
- Timestamp.ZERO = Timestamp.fromInt(0);
-
- /** @type {Timestamp} */
- Timestamp.ONE = Timestamp.fromInt(1);
-
- /** @type {Timestamp} */
- Timestamp.NEG_ONE = Timestamp.fromInt(-1);
-
- /** @type {Timestamp} */
- Timestamp.MAX_VALUE = Timestamp.fromBits(0xffffffff | 0, 0x7fffffff | 0);
-
- /** @type {Timestamp} */
- Timestamp.MIN_VALUE = Timestamp.fromBits(0, 0x80000000 | 0);
-
- /**
- * @type {Timestamp}
- * @ignore
- */
- Timestamp.TWO_PWR_24_ = Timestamp.fromInt(1 << 24);
-
- /**
- * Expose.
- */
- module.exports = Timestamp;
- module.exports.Timestamp = Timestamp;
-
-/***/ }),
-/* 338 */
-/***/ (function(module, exports, __webpack_require__) {
-
- /* WEBPACK VAR INJECTION */(function(Buffer, process) {// Custom inspect property name / symbol.
- var inspect = 'inspect';
-
- var utils = __webpack_require__(344);
-
- /**
- * Machine id.
- *
- * Create a random 3-byte value (i.e. unique for this
- * process). Other drivers use a md5 of the machine id here, but
- * that would mean an asyc call to gethostname, so we don't bother.
- * @ignore
- */
- var MACHINE_ID = parseInt(Math.random() * 0xffffff, 10);
-
- // Regular expression that checks for hex value
- var checkForHexRegExp = new RegExp('^[0-9a-fA-F]{24}$');
-
- // Check if buffer exists
- try {
- if (Buffer && Buffer.from) {
- var hasBufferType = true;
- inspect = __webpack_require__(345).inspect.custom || 'inspect';
- }
- } catch (err) {
- hasBufferType = false;
- }
-
- /**
- * Create a new ObjectID instance
- *
- * @class
- * @param {(string|number)} id Can be a 24 byte hex string, 12 byte binary string or a Number.
- * @property {number} generationTime The generation time of this ObjectId instance
- * @return {ObjectID} instance of ObjectID.
- */
- var ObjectID = function ObjectID(id) {
- // Duck-typing to support ObjectId from different npm packages
- if (id instanceof ObjectID) return id;
- if (!(this instanceof ObjectID)) return new ObjectID(id);
-
- this._bsontype = 'ObjectID';
-
- // The most common usecase (blank id, new objectId instance)
- if (id == null || typeof id === 'number') {
- // Generate a new id
- this.id = this.generate(id);
- // If we are caching the hex string
- if (ObjectID.cacheHexString) this.__id = this.toString('hex');
- // Return the object
- return;
- }
-
- // Check if the passed in id is valid
- var valid = ObjectID.isValid(id);
-
- // Throw an error if it's not a valid setup
- if (!valid && id != null) {
- throw new Error('Argument passed in must be a single String of 12 bytes or a string of 24 hex characters');
- } else if (valid && typeof id === 'string' && id.length === 24 && hasBufferType) {
- return new ObjectID(utils.toBuffer(id, 'hex'));
- } else if (valid && typeof id === 'string' && id.length === 24) {
- return ObjectID.createFromHexString(id);
- } else if (id != null && id.length === 12) {
- // assume 12 byte string
- this.id = id;
- } else if (id != null && id.toHexString) {
- // Duck-typing to support ObjectId from different npm packages
- return id;
- } else {
- throw new Error('Argument passed in must be a single String of 12 bytes or a string of 24 hex characters');
- }
-
- if (ObjectID.cacheHexString) this.__id = this.toString('hex');
- };
-
- // Allow usage of ObjectId as well as ObjectID
- // var ObjectId = ObjectID;
-
- // Precomputed hex table enables speedy hex string conversion
- var hexTable = [];
- for (var i = 0; i < 256; i++) {
- hexTable[i] = (i <= 15 ? '0' : '') + i.toString(16);
- }
-
- /**
- * Return the ObjectID id as a 24 byte hex string representation
- *
- * @method
- * @return {string} return the 24 byte hex string representation.
- */
- ObjectID.prototype.toHexString = function () {
- if (ObjectID.cacheHexString && this.__id) return this.__id;
-
- var hexString = '';
- if (!this.id || !this.id.length) {
- throw new Error('invalid ObjectId, ObjectId.id must be either a string or a Buffer, but is [' + JSON.stringify(this.id) + ']');
- }
-
- if (this.id instanceof _Buffer) {
- hexString = convertToHex(this.id);
- if (ObjectID.cacheHexString) this.__id = hexString;
- return hexString;
- }
-
- for (var i = 0; i < this.id.length; i++) {
- hexString += hexTable[this.id.charCodeAt(i)];
- }
-
- if (ObjectID.cacheHexString) this.__id = hexString;
- return hexString;
- };
-
- /**
- * Update the ObjectID index used in generating new ObjectID's on the driver
- *
- * @method
- * @return {number} returns next index value.
- * @ignore
- */
- ObjectID.prototype.get_inc = function () {
- return ObjectID.index = (ObjectID.index + 1) % 0xffffff;
- };
-
- /**
- * Update the ObjectID index used in generating new ObjectID's on the driver
- *
- * @method
- * @return {number} returns next index value.
- * @ignore
- */
- ObjectID.prototype.getInc = function () {
- return this.get_inc();
- };
-
- /**
- * Generate a 12 byte id buffer used in ObjectID's
- *
- * @method
- * @param {number} [time] optional parameter allowing to pass in a second based timestamp.
- * @return {Buffer} return the 12 byte id buffer string.
- */
- ObjectID.prototype.generate = function (time) {
- if ('number' !== typeof time) {
- time = ~~(Date.now() / 1000);
- }
-
- // Use pid
- var pid = (typeof process === 'undefined' || process.pid === 1 ? Math.floor(Math.random() * 100000) : process.pid) % 0xffff;
- var inc = this.get_inc();
- // Buffer used
- var buffer = utils.allocBuffer(12);
- // Encode time
- buffer[3] = time & 0xff;
- buffer[2] = time >> 8 & 0xff;
- buffer[1] = time >> 16 & 0xff;
- buffer[0] = time >> 24 & 0xff;
- // Encode machine
- buffer[6] = MACHINE_ID & 0xff;
- buffer[5] = MACHINE_ID >> 8 & 0xff;
- buffer[4] = MACHINE_ID >> 16 & 0xff;
- // Encode pid
- buffer[8] = pid & 0xff;
- buffer[7] = pid >> 8 & 0xff;
- // Encode index
- buffer[11] = inc & 0xff;
- buffer[10] = inc >> 8 & 0xff;
- buffer[9] = inc >> 16 & 0xff;
- // Return the buffer
- return buffer;
- };
-
- /**
- * Converts the id into a 24 byte hex string for printing
- *
- * @param {String} format The Buffer toString format parameter.
- * @return {String} return the 24 byte hex string representation.
- * @ignore
- */
- ObjectID.prototype.toString = function (format) {
- // Is the id a buffer then use the buffer toString method to return the format
- if (this.id && this.id.copy) {
- return this.id.toString(typeof format === 'string' ? format : 'hex');
- }
-
- // if(this.buffer )
- return this.toHexString();
- };
-
- /**
- * Converts to a string representation of this Id.
- *
- * @return {String} return the 24 byte hex string representation.
- * @ignore
- */
- ObjectID.prototype[inspect] = ObjectID.prototype.toString;
-
- /**
- * Converts to its JSON representation.
- *
- * @return {String} return the 24 byte hex string representation.
- * @ignore
- */
- ObjectID.prototype.toJSON = function () {
- return this.toHexString();
- };
-
- /**
- * Compares the equality of this ObjectID with `otherID`.
- *
- * @method
- * @param {object} otherID ObjectID instance to compare against.
- * @return {boolean} the result of comparing two ObjectID's
- */
- ObjectID.prototype.equals = function equals(otherId) {
- // var id;
-
- if (otherId instanceof ObjectID) {
- return this.toString() === otherId.toString();
- } else if (typeof otherId === 'string' && ObjectID.isValid(otherId) && otherId.length === 12 && this.id instanceof _Buffer) {
- return otherId === this.id.toString('binary');
- } else if (typeof otherId === 'string' && ObjectID.isValid(otherId) && otherId.length === 24) {
- return otherId.toLowerCase() === this.toHexString();
- } else if (typeof otherId === 'string' && ObjectID.isValid(otherId) && otherId.length === 12) {
- return otherId === this.id;
- } else if (otherId != null && (otherId instanceof ObjectID || otherId.toHexString)) {
- return otherId.toHexString() === this.toHexString();
- } else {
- return false;
- }
- };
-
- /**
- * Returns the generation date (accurate up to the second) that this ID was generated.
- *
- * @method
- * @return {date} the generation date
- */
- ObjectID.prototype.getTimestamp = function () {
- var timestamp = new Date();
- var time = this.id[3] | this.id[2] << 8 | this.id[1] << 16 | this.id[0] << 24;
- timestamp.setTime(Math.floor(time) * 1000);
- return timestamp;
- };
-
- /**
- * @ignore
- */
- ObjectID.index = ~~(Math.random() * 0xffffff);
-
- /**
- * @ignore
- */
- ObjectID.createPk = function createPk() {
- return new ObjectID();
- };
-
- /**
- * Creates an ObjectID from a second based number, with the rest of the ObjectID zeroed out. Used for comparisons or sorting the ObjectID.
- *
- * @method
- * @param {number} time an integer number representing a number of seconds.
- * @return {ObjectID} return the created ObjectID
- */
- ObjectID.createFromTime = function createFromTime(time) {
- var buffer = utils.toBuffer([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]);
- // Encode time into first 4 bytes
- buffer[3] = time & 0xff;
- buffer[2] = time >> 8 & 0xff;
- buffer[1] = time >> 16 & 0xff;
- buffer[0] = time >> 24 & 0xff;
- // Return the new objectId
- return new ObjectID(buffer);
- };
-
- // Lookup tables
- //var encodeLookup = '0123456789abcdef'.split('');
- var decodeLookup = [];
- i = 0;
- while (i < 10) decodeLookup[0x30 + i] = i++;
- while (i < 16) decodeLookup[0x41 - 10 + i] = decodeLookup[0x61 - 10 + i] = i++;
-
- var _Buffer = Buffer;
- var convertToHex = function (bytes) {
- return bytes.toString('hex');
- };
-
- /**
- * Creates an ObjectID from a hex string representation of an ObjectID.
- *
- * @method
- * @param {string} hexString create a ObjectID from a passed in 24 byte hexstring.
- * @return {ObjectID} return the created ObjectID
- */
- ObjectID.createFromHexString = function createFromHexString(string) {
- // Throw an error if it's not a valid setup
- if (typeof string === 'undefined' || string != null && string.length !== 24) {
- throw new Error('Argument passed in must be a single String of 12 bytes or a string of 24 hex characters');
- }
-
- // Use Buffer.from method if available
- if (hasBufferType) return new ObjectID(utils.toBuffer(string, 'hex'));
-
- // Calculate lengths
- var array = new _Buffer(12);
- var n = 0;
- var i = 0;
-
- while (i < 24) {
- array[n++] = decodeLookup[string.charCodeAt(i++)] << 4 | decodeLookup[string.charCodeAt(i++)];
- }
-
- return new ObjectID(array);
- };
-
- /**
- * Checks if a value is a valid bson ObjectId
- *
- * @method
- * @return {boolean} return true if the value is a valid bson ObjectId, return false otherwise.
- */
- ObjectID.isValid = function isValid(id) {
- if (id == null) return false;
-
- if (typeof id === 'number') {
- return true;
- }
-
- if (typeof id === 'string') {
- return id.length === 12 || id.length === 24 && checkForHexRegExp.test(id);
- }
-
- if (id instanceof ObjectID) {
- return true;
- }
-
- if (id instanceof _Buffer) {
- return true;
- }
-
- // Duck-Typing detection of ObjectId like objects
- if (id.toHexString) {
- return id.id.length === 12 || id.id.length === 24 && checkForHexRegExp.test(id.id);
- }
-
- return false;
- };
-
- /**
- * @ignore
- */
- Object.defineProperty(ObjectID.prototype, 'generationTime', {
- enumerable: true,
- get: function () {
- return this.id[3] | this.id[2] << 8 | this.id[1] << 16 | this.id[0] << 24;
- },
- set: function (value) {
- // Encode time into first 4 bytes
- this.id[3] = value & 0xff;
- this.id[2] = value >> 8 & 0xff;
- this.id[1] = value >> 16 & 0xff;
- this.id[0] = value >> 24 & 0xff;
- }
- });
-
- /**
- * Expose.
- */
- module.exports = ObjectID;
- module.exports.ObjectID = ObjectID;
- module.exports.ObjectId = ObjectID;
- /* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(339).Buffer, __webpack_require__(343)))
-
-/***/ }),
-/* 339 */
-/***/ (function(module, exports, __webpack_require__) {
-
- /* WEBPACK VAR INJECTION */(function(global) {/*!
- * The buffer module from node.js, for the browser.
- *
- * @author Feross Aboukhadijeh
- * @license MIT
- */
- /* eslint-disable no-proto */
-
- 'use strict'
-
- var base64 = __webpack_require__(340)
- var ieee754 = __webpack_require__(341)
- var isArray = __webpack_require__(342)
-
- exports.Buffer = Buffer
- exports.SlowBuffer = SlowBuffer
- exports.INSPECT_MAX_BYTES = 50
-
- /**
- * If `Buffer.TYPED_ARRAY_SUPPORT`:
- * === true Use Uint8Array implementation (fastest)
- * === false Use Object implementation (most compatible, even IE6)
- *
- * Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,
- * Opera 11.6+, iOS 4.2+.
- *
- * Due to various browser bugs, sometimes the Object implementation will be used even
- * when the browser supports typed arrays.
- *
- * Note:
- *
- * - Firefox 4-29 lacks support for adding new properties to `Uint8Array` instances,
- * See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438.
- *
- * - Chrome 9-10 is missing the `TypedArray.prototype.subarray` function.
- *
- * - IE10 has a broken `TypedArray.prototype.subarray` function which returns arrays of
- * incorrect length in some situations.
-
- * We detect these buggy browsers and set `Buffer.TYPED_ARRAY_SUPPORT` to `false` so they
- * get the Object implementation, which is slower but behaves correctly.
- */
- Buffer.TYPED_ARRAY_SUPPORT = global.TYPED_ARRAY_SUPPORT !== undefined
- ? global.TYPED_ARRAY_SUPPORT
- : typedArraySupport()
-
- /*
- * Export kMaxLength after typed array support is determined.
- */
- exports.kMaxLength = kMaxLength()
-
- function typedArraySupport () {
- try {
- var arr = new Uint8Array(1)
- arr.__proto__ = {__proto__: Uint8Array.prototype, foo: function () { return 42 }}
- return arr.foo() === 42 && // typed array instances can be augmented
- typeof arr.subarray === 'function' && // chrome 9-10 lack `subarray`
- arr.subarray(1, 1).byteLength === 0 // ie10 has broken `subarray`
- } catch (e) {
- return false
- }
- }
-
- function kMaxLength () {
- return Buffer.TYPED_ARRAY_SUPPORT
- ? 0x7fffffff
- : 0x3fffffff
- }
-
- function createBuffer (that, length) {
- if (kMaxLength() < length) {
- throw new RangeError('Invalid typed array length')
- }
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- // Return an augmented `Uint8Array` instance, for best performance
- that = new Uint8Array(length)
- that.__proto__ = Buffer.prototype
- } else {
- // Fallback: Return an object instance of the Buffer class
- if (that === null) {
- that = new Buffer(length)
- }
- that.length = length
- }
-
- return that
- }
-
- /**
- * The Buffer constructor returns instances of `Uint8Array` that have their
- * prototype changed to `Buffer.prototype`. Furthermore, `Buffer` is a subclass of
- * `Uint8Array`, so the returned instances will have all the node `Buffer` methods
- * and the `Uint8Array` methods. Square bracket notation works as expected -- it
- * returns a single octet.
- *
- * The `Uint8Array` prototype remains unmodified.
- */
-
- function Buffer (arg, encodingOrOffset, length) {
- if (!Buffer.TYPED_ARRAY_SUPPORT && !(this instanceof Buffer)) {
- return new Buffer(arg, encodingOrOffset, length)
- }
-
- // Common case.
- if (typeof arg === 'number') {
- if (typeof encodingOrOffset === 'string') {
- throw new Error(
- 'If encoding is specified then the first argument must be a string'
- )
- }
- return allocUnsafe(this, arg)
- }
- return from(this, arg, encodingOrOffset, length)
- }
-
- Buffer.poolSize = 8192 // not used by this implementation
-
- // TODO: Legacy, not needed anymore. Remove in next major version.
- Buffer._augment = function (arr) {
- arr.__proto__ = Buffer.prototype
- return arr
- }
-
- function from (that, value, encodingOrOffset, length) {
- if (typeof value === 'number') {
- throw new TypeError('"value" argument must not be a number')
- }
-
- if (typeof ArrayBuffer !== 'undefined' && value instanceof ArrayBuffer) {
- return fromArrayBuffer(that, value, encodingOrOffset, length)
- }
-
- if (typeof value === 'string') {
- return fromString(that, value, encodingOrOffset)
- }
-
- return fromObject(that, value)
- }
-
- /**
- * Functionally equivalent to Buffer(arg, encoding) but throws a TypeError
- * if value is a number.
- * Buffer.from(str[, encoding])
- * Buffer.from(array)
- * Buffer.from(buffer)
- * Buffer.from(arrayBuffer[, byteOffset[, length]])
- **/
- Buffer.from = function (value, encodingOrOffset, length) {
- return from(null, value, encodingOrOffset, length)
- }
-
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- Buffer.prototype.__proto__ = Uint8Array.prototype
- Buffer.__proto__ = Uint8Array
- if (typeof Symbol !== 'undefined' && Symbol.species &&
- Buffer[Symbol.species] === Buffer) {
- // Fix subarray() in ES2016. See: https://github.com/feross/buffer/pull/97
- Object.defineProperty(Buffer, Symbol.species, {
- value: null,
- configurable: true
- })
- }
- }
-
- function assertSize (size) {
- if (typeof size !== 'number') {
- throw new TypeError('"size" argument must be a number')
- } else if (size < 0) {
- throw new RangeError('"size" argument must not be negative')
- }
- }
-
- function alloc (that, size, fill, encoding) {
- assertSize(size)
- if (size <= 0) {
- return createBuffer(that, size)
- }
- if (fill !== undefined) {
- // Only pay attention to encoding if it's a string. This
- // prevents accidentally sending in a number that would
- // be interpretted as a start offset.
- return typeof encoding === 'string'
- ? createBuffer(that, size).fill(fill, encoding)
- : createBuffer(that, size).fill(fill)
- }
- return createBuffer(that, size)
- }
-
- /**
- * Creates a new filled Buffer instance.
- * alloc(size[, fill[, encoding]])
- **/
- Buffer.alloc = function (size, fill, encoding) {
- return alloc(null, size, fill, encoding)
- }
-
- function allocUnsafe (that, size) {
- assertSize(size)
- that = createBuffer(that, size < 0 ? 0 : checked(size) | 0)
- if (!Buffer.TYPED_ARRAY_SUPPORT) {
- for (var i = 0; i < size; ++i) {
- that[i] = 0
- }
- }
- return that
- }
-
- /**
- * Equivalent to Buffer(num), by default creates a non-zero-filled Buffer instance.
- * */
- Buffer.allocUnsafe = function (size) {
- return allocUnsafe(null, size)
- }
- /**
- * Equivalent to SlowBuffer(num), by default creates a non-zero-filled Buffer instance.
- */
- Buffer.allocUnsafeSlow = function (size) {
- return allocUnsafe(null, size)
- }
-
- function fromString (that, string, encoding) {
- if (typeof encoding !== 'string' || encoding === '') {
- encoding = 'utf8'
- }
-
- if (!Buffer.isEncoding(encoding)) {
- throw new TypeError('"encoding" must be a valid string encoding')
- }
-
- var length = byteLength(string, encoding) | 0
- that = createBuffer(that, length)
-
- var actual = that.write(string, encoding)
-
- if (actual !== length) {
- // Writing a hex string, for example, that contains invalid characters will
- // cause everything after the first invalid character to be ignored. (e.g.
- // 'abxxcd' will be treated as 'ab')
- that = that.slice(0, actual)
- }
-
- return that
- }
-
- function fromArrayLike (that, array) {
- var length = array.length < 0 ? 0 : checked(array.length) | 0
- that = createBuffer(that, length)
- for (var i = 0; i < length; i += 1) {
- that[i] = array[i] & 255
- }
- return that
- }
-
- function fromArrayBuffer (that, array, byteOffset, length) {
- array.byteLength // this throws if `array` is not a valid ArrayBuffer
-
- if (byteOffset < 0 || array.byteLength < byteOffset) {
- throw new RangeError('\'offset\' is out of bounds')
- }
-
- if (array.byteLength < byteOffset + (length || 0)) {
- throw new RangeError('\'length\' is out of bounds')
- }
-
- if (byteOffset === undefined && length === undefined) {
- array = new Uint8Array(array)
- } else if (length === undefined) {
- array = new Uint8Array(array, byteOffset)
- } else {
- array = new Uint8Array(array, byteOffset, length)
- }
-
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- // Return an augmented `Uint8Array` instance, for best performance
- that = array
- that.__proto__ = Buffer.prototype
- } else {
- // Fallback: Return an object instance of the Buffer class
- that = fromArrayLike(that, array)
- }
- return that
- }
-
- function fromObject (that, obj) {
- if (Buffer.isBuffer(obj)) {
- var len = checked(obj.length) | 0
- that = createBuffer(that, len)
-
- if (that.length === 0) {
- return that
- }
-
- obj.copy(that, 0, 0, len)
- return that
- }
-
- if (obj) {
- if ((typeof ArrayBuffer !== 'undefined' &&
- obj.buffer instanceof ArrayBuffer) || 'length' in obj) {
- if (typeof obj.length !== 'number' || isnan(obj.length)) {
- return createBuffer(that, 0)
- }
- return fromArrayLike(that, obj)
- }
-
- if (obj.type === 'Buffer' && isArray(obj.data)) {
- return fromArrayLike(that, obj.data)
- }
- }
-
- throw new TypeError('First argument must be a string, Buffer, ArrayBuffer, Array, or array-like object.')
- }
-
- function checked (length) {
- // Note: cannot use `length < kMaxLength()` here because that fails when
- // length is NaN (which is otherwise coerced to zero.)
- if (length >= kMaxLength()) {
- throw new RangeError('Attempt to allocate Buffer larger than maximum ' +
- 'size: 0x' + kMaxLength().toString(16) + ' bytes')
- }
- return length | 0
- }
-
- function SlowBuffer (length) {
- if (+length != length) { // eslint-disable-line eqeqeq
- length = 0
- }
- return Buffer.alloc(+length)
- }
-
- Buffer.isBuffer = function isBuffer (b) {
- return !!(b != null && b._isBuffer)
- }
-
- Buffer.compare = function compare (a, b) {
- if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {
- throw new TypeError('Arguments must be Buffers')
- }
-
- if (a === b) return 0
-
- var x = a.length
- var y = b.length
-
- for (var i = 0, len = Math.min(x, y); i < len; ++i) {
- if (a[i] !== b[i]) {
- x = a[i]
- y = b[i]
- break
- }
- }
-
- if (x < y) return -1
- if (y < x) return 1
- return 0
- }
-
- Buffer.isEncoding = function isEncoding (encoding) {
- switch (String(encoding).toLowerCase()) {
- case 'hex':
- case 'utf8':
- case 'utf-8':
- case 'ascii':
- case 'latin1':
- case 'binary':
- case 'base64':
- case 'ucs2':
- case 'ucs-2':
- case 'utf16le':
- case 'utf-16le':
- return true
- default:
- return false
- }
- }
-
- Buffer.concat = function concat (list, length) {
- if (!isArray(list)) {
- throw new TypeError('"list" argument must be an Array of Buffers')
- }
-
- if (list.length === 0) {
- return Buffer.alloc(0)
- }
-
- var i
- if (length === undefined) {
- length = 0
- for (i = 0; i < list.length; ++i) {
- length += list[i].length
- }
- }
-
- var buffer = Buffer.allocUnsafe(length)
- var pos = 0
- for (i = 0; i < list.length; ++i) {
- var buf = list[i]
- if (!Buffer.isBuffer(buf)) {
- throw new TypeError('"list" argument must be an Array of Buffers')
- }
- buf.copy(buffer, pos)
- pos += buf.length
- }
- return buffer
- }
-
- function byteLength (string, encoding) {
- if (Buffer.isBuffer(string)) {
- return string.length
- }
- if (typeof ArrayBuffer !== 'undefined' && typeof ArrayBuffer.isView === 'function' &&
- (ArrayBuffer.isView(string) || string instanceof ArrayBuffer)) {
- return string.byteLength
- }
- if (typeof string !== 'string') {
- string = '' + string
- }
-
- var len = string.length
- if (len === 0) return 0
-
- // Use a for loop to avoid recursion
- var loweredCase = false
- for (;;) {
- switch (encoding) {
- case 'ascii':
- case 'latin1':
- case 'binary':
- return len
- case 'utf8':
- case 'utf-8':
- case undefined:
- return utf8ToBytes(string).length
- case 'ucs2':
- case 'ucs-2':
- case 'utf16le':
- case 'utf-16le':
- return len * 2
- case 'hex':
- return len >>> 1
- case 'base64':
- return base64ToBytes(string).length
- default:
- if (loweredCase) return utf8ToBytes(string).length // assume utf8
- encoding = ('' + encoding).toLowerCase()
- loweredCase = true
- }
- }
- }
- Buffer.byteLength = byteLength
-
- function slowToString (encoding, start, end) {
- var loweredCase = false
-
- // No need to verify that "this.length <= MAX_UINT32" since it's a read-only
- // property of a typed array.
-
- // This behaves neither like String nor Uint8Array in that we set start/end
- // to their upper/lower bounds if the value passed is out of range.
- // undefined is handled specially as per ECMA-262 6th Edition,
- // Section 13.3.3.7 Runtime Semantics: KeyedBindingInitialization.
- if (start === undefined || start < 0) {
- start = 0
- }
- // Return early if start > this.length. Done here to prevent potential uint32
- // coercion fail below.
- if (start > this.length) {
- return ''
- }
-
- if (end === undefined || end > this.length) {
- end = this.length
- }
-
- if (end <= 0) {
- return ''
- }
-
- // Force coersion to uint32. This will also coerce falsey/NaN values to 0.
- end >>>= 0
- start >>>= 0
-
- if (end <= start) {
- return ''
- }
-
- if (!encoding) encoding = 'utf8'
-
- while (true) {
- switch (encoding) {
- case 'hex':
- return hexSlice(this, start, end)
-
- case 'utf8':
- case 'utf-8':
- return utf8Slice(this, start, end)
-
- case 'ascii':
- return asciiSlice(this, start, end)
-
- case 'latin1':
- case 'binary':
- return latin1Slice(this, start, end)
-
- case 'base64':
- return base64Slice(this, start, end)
-
- case 'ucs2':
- case 'ucs-2':
- case 'utf16le':
- case 'utf-16le':
- return utf16leSlice(this, start, end)
-
- default:
- if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)
- encoding = (encoding + '').toLowerCase()
- loweredCase = true
- }
- }
- }
-
- // The property is used by `Buffer.isBuffer` and `is-buffer` (in Safari 5-7) to detect
- // Buffer instances.
- Buffer.prototype._isBuffer = true
-
- function swap (b, n, m) {
- var i = b[n]
- b[n] = b[m]
- b[m] = i
- }
-
- Buffer.prototype.swap16 = function swap16 () {
- var len = this.length
- if (len % 2 !== 0) {
- throw new RangeError('Buffer size must be a multiple of 16-bits')
- }
- for (var i = 0; i < len; i += 2) {
- swap(this, i, i + 1)
- }
- return this
- }
-
- Buffer.prototype.swap32 = function swap32 () {
- var len = this.length
- if (len % 4 !== 0) {
- throw new RangeError('Buffer size must be a multiple of 32-bits')
- }
- for (var i = 0; i < len; i += 4) {
- swap(this, i, i + 3)
- swap(this, i + 1, i + 2)
- }
- return this
- }
-
- Buffer.prototype.swap64 = function swap64 () {
- var len = this.length
- if (len % 8 !== 0) {
- throw new RangeError('Buffer size must be a multiple of 64-bits')
- }
- for (var i = 0; i < len; i += 8) {
- swap(this, i, i + 7)
- swap(this, i + 1, i + 6)
- swap(this, i + 2, i + 5)
- swap(this, i + 3, i + 4)
- }
- return this
- }
-
- Buffer.prototype.toString = function toString () {
- var length = this.length | 0
- if (length === 0) return ''
- if (arguments.length === 0) return utf8Slice(this, 0, length)
- return slowToString.apply(this, arguments)
- }
-
- Buffer.prototype.equals = function equals (b) {
- if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer')
- if (this === b) return true
- return Buffer.compare(this, b) === 0
- }
-
- Buffer.prototype.inspect = function inspect () {
- var str = ''
- var max = exports.INSPECT_MAX_BYTES
- if (this.length > 0) {
- str = this.toString('hex', 0, max).match(/.{2}/g).join(' ')
- if (this.length > max) str += ' ... '
- }
- return ''
- }
-
- Buffer.prototype.compare = function compare (target, start, end, thisStart, thisEnd) {
- if (!Buffer.isBuffer(target)) {
- throw new TypeError('Argument must be a Buffer')
- }
-
- if (start === undefined) {
- start = 0
- }
- if (end === undefined) {
- end = target ? target.length : 0
- }
- if (thisStart === undefined) {
- thisStart = 0
- }
- if (thisEnd === undefined) {
- thisEnd = this.length
- }
-
- if (start < 0 || end > target.length || thisStart < 0 || thisEnd > this.length) {
- throw new RangeError('out of range index')
- }
-
- if (thisStart >= thisEnd && start >= end) {
- return 0
- }
- if (thisStart >= thisEnd) {
- return -1
- }
- if (start >= end) {
- return 1
- }
-
- start >>>= 0
- end >>>= 0
- thisStart >>>= 0
- thisEnd >>>= 0
-
- if (this === target) return 0
-
- var x = thisEnd - thisStart
- var y = end - start
- var len = Math.min(x, y)
-
- var thisCopy = this.slice(thisStart, thisEnd)
- var targetCopy = target.slice(start, end)
-
- for (var i = 0; i < len; ++i) {
- if (thisCopy[i] !== targetCopy[i]) {
- x = thisCopy[i]
- y = targetCopy[i]
- break
- }
- }
-
- if (x < y) return -1
- if (y < x) return 1
- return 0
- }
-
- // Finds either the first index of `val` in `buffer` at offset >= `byteOffset`,
- // OR the last index of `val` in `buffer` at offset <= `byteOffset`.
- //
- // Arguments:
- // - buffer - a Buffer to search
- // - val - a string, Buffer, or number
- // - byteOffset - an index into `buffer`; will be clamped to an int32
- // - encoding - an optional encoding, relevant is val is a string
- // - dir - true for indexOf, false for lastIndexOf
- function bidirectionalIndexOf (buffer, val, byteOffset, encoding, dir) {
- // Empty buffer means no match
- if (buffer.length === 0) return -1
-
- // Normalize byteOffset
- if (typeof byteOffset === 'string') {
- encoding = byteOffset
- byteOffset = 0
- } else if (byteOffset > 0x7fffffff) {
- byteOffset = 0x7fffffff
- } else if (byteOffset < -0x80000000) {
- byteOffset = -0x80000000
- }
- byteOffset = +byteOffset // Coerce to Number.
- if (isNaN(byteOffset)) {
- // byteOffset: it it's undefined, null, NaN, "foo", etc, search whole buffer
- byteOffset = dir ? 0 : (buffer.length - 1)
- }
-
- // Normalize byteOffset: negative offsets start from the end of the buffer
- if (byteOffset < 0) byteOffset = buffer.length + byteOffset
- if (byteOffset >= buffer.length) {
- if (dir) return -1
- else byteOffset = buffer.length - 1
- } else if (byteOffset < 0) {
- if (dir) byteOffset = 0
- else return -1
- }
-
- // Normalize val
- if (typeof val === 'string') {
- val = Buffer.from(val, encoding)
- }
-
- // Finally, search either indexOf (if dir is true) or lastIndexOf
- if (Buffer.isBuffer(val)) {
- // Special case: looking for empty string/buffer always fails
- if (val.length === 0) {
- return -1
- }
- return arrayIndexOf(buffer, val, byteOffset, encoding, dir)
- } else if (typeof val === 'number') {
- val = val & 0xFF // Search for a byte value [0-255]
- if (Buffer.TYPED_ARRAY_SUPPORT &&
- typeof Uint8Array.prototype.indexOf === 'function') {
- if (dir) {
- return Uint8Array.prototype.indexOf.call(buffer, val, byteOffset)
- } else {
- return Uint8Array.prototype.lastIndexOf.call(buffer, val, byteOffset)
- }
- }
- return arrayIndexOf(buffer, [ val ], byteOffset, encoding, dir)
- }
-
- throw new TypeError('val must be string, number or Buffer')
- }
-
- function arrayIndexOf (arr, val, byteOffset, encoding, dir) {
- var indexSize = 1
- var arrLength = arr.length
- var valLength = val.length
-
- if (encoding !== undefined) {
- encoding = String(encoding).toLowerCase()
- if (encoding === 'ucs2' || encoding === 'ucs-2' ||
- encoding === 'utf16le' || encoding === 'utf-16le') {
- if (arr.length < 2 || val.length < 2) {
- return -1
- }
- indexSize = 2
- arrLength /= 2
- valLength /= 2
- byteOffset /= 2
- }
- }
-
- function read (buf, i) {
- if (indexSize === 1) {
- return buf[i]
- } else {
- return buf.readUInt16BE(i * indexSize)
- }
- }
-
- var i
- if (dir) {
- var foundIndex = -1
- for (i = byteOffset; i < arrLength; i++) {
- if (read(arr, i) === read(val, foundIndex === -1 ? 0 : i - foundIndex)) {
- if (foundIndex === -1) foundIndex = i
- if (i - foundIndex + 1 === valLength) return foundIndex * indexSize
- } else {
- if (foundIndex !== -1) i -= i - foundIndex
- foundIndex = -1
- }
- }
- } else {
- if (byteOffset + valLength > arrLength) byteOffset = arrLength - valLength
- for (i = byteOffset; i >= 0; i--) {
- var found = true
- for (var j = 0; j < valLength; j++) {
- if (read(arr, i + j) !== read(val, j)) {
- found = false
- break
- }
- }
- if (found) return i
- }
- }
-
- return -1
- }
-
- Buffer.prototype.includes = function includes (val, byteOffset, encoding) {
- return this.indexOf(val, byteOffset, encoding) !== -1
- }
-
- Buffer.prototype.indexOf = function indexOf (val, byteOffset, encoding) {
- return bidirectionalIndexOf(this, val, byteOffset, encoding, true)
- }
-
- Buffer.prototype.lastIndexOf = function lastIndexOf (val, byteOffset, encoding) {
- return bidirectionalIndexOf(this, val, byteOffset, encoding, false)
- }
-
- function hexWrite (buf, string, offset, length) {
- offset = Number(offset) || 0
- var remaining = buf.length - offset
- if (!length) {
- length = remaining
- } else {
- length = Number(length)
- if (length > remaining) {
- length = remaining
- }
- }
-
- // must be an even number of digits
- var strLen = string.length
- if (strLen % 2 !== 0) throw new TypeError('Invalid hex string')
-
- if (length > strLen / 2) {
- length = strLen / 2
- }
- for (var i = 0; i < length; ++i) {
- var parsed = parseInt(string.substr(i * 2, 2), 16)
- if (isNaN(parsed)) return i
- buf[offset + i] = parsed
- }
- return i
- }
-
- function utf8Write (buf, string, offset, length) {
- return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length)
- }
-
- function asciiWrite (buf, string, offset, length) {
- return blitBuffer(asciiToBytes(string), buf, offset, length)
- }
-
- function latin1Write (buf, string, offset, length) {
- return asciiWrite(buf, string, offset, length)
- }
-
- function base64Write (buf, string, offset, length) {
- return blitBuffer(base64ToBytes(string), buf, offset, length)
- }
-
- function ucs2Write (buf, string, offset, length) {
- return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length)
- }
-
- Buffer.prototype.write = function write (string, offset, length, encoding) {
- // Buffer#write(string)
- if (offset === undefined) {
- encoding = 'utf8'
- length = this.length
- offset = 0
- // Buffer#write(string, encoding)
- } else if (length === undefined && typeof offset === 'string') {
- encoding = offset
- length = this.length
- offset = 0
- // Buffer#write(string, offset[, length][, encoding])
- } else if (isFinite(offset)) {
- offset = offset | 0
- if (isFinite(length)) {
- length = length | 0
- if (encoding === undefined) encoding = 'utf8'
- } else {
- encoding = length
- length = undefined
- }
- // legacy write(string, encoding, offset, length) - remove in v0.13
- } else {
- throw new Error(
- 'Buffer.write(string, encoding, offset[, length]) is no longer supported'
- )
- }
-
- var remaining = this.length - offset
- if (length === undefined || length > remaining) length = remaining
-
- if ((string.length > 0 && (length < 0 || offset < 0)) || offset > this.length) {
- throw new RangeError('Attempt to write outside buffer bounds')
- }
-
- if (!encoding) encoding = 'utf8'
-
- var loweredCase = false
- for (;;) {
- switch (encoding) {
- case 'hex':
- return hexWrite(this, string, offset, length)
-
- case 'utf8':
- case 'utf-8':
- return utf8Write(this, string, offset, length)
-
- case 'ascii':
- return asciiWrite(this, string, offset, length)
-
- case 'latin1':
- case 'binary':
- return latin1Write(this, string, offset, length)
-
- case 'base64':
- // Warning: maxLength not taken into account in base64Write
- return base64Write(this, string, offset, length)
-
- case 'ucs2':
- case 'ucs-2':
- case 'utf16le':
- case 'utf-16le':
- return ucs2Write(this, string, offset, length)
-
- default:
- if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)
- encoding = ('' + encoding).toLowerCase()
- loweredCase = true
- }
- }
- }
-
- Buffer.prototype.toJSON = function toJSON () {
- return {
- type: 'Buffer',
- data: Array.prototype.slice.call(this._arr || this, 0)
- }
- }
-
- function base64Slice (buf, start, end) {
- if (start === 0 && end === buf.length) {
- return base64.fromByteArray(buf)
- } else {
- return base64.fromByteArray(buf.slice(start, end))
- }
- }
-
- function utf8Slice (buf, start, end) {
- end = Math.min(buf.length, end)
- var res = []
-
- var i = start
- while (i < end) {
- var firstByte = buf[i]
- var codePoint = null
- var bytesPerSequence = (firstByte > 0xEF) ? 4
- : (firstByte > 0xDF) ? 3
- : (firstByte > 0xBF) ? 2
- : 1
-
- if (i + bytesPerSequence <= end) {
- var secondByte, thirdByte, fourthByte, tempCodePoint
-
- switch (bytesPerSequence) {
- case 1:
- if (firstByte < 0x80) {
- codePoint = firstByte
- }
- break
- case 2:
- secondByte = buf[i + 1]
- if ((secondByte & 0xC0) === 0x80) {
- tempCodePoint = (firstByte & 0x1F) << 0x6 | (secondByte & 0x3F)
- if (tempCodePoint > 0x7F) {
- codePoint = tempCodePoint
- }
- }
- break
- case 3:
- secondByte = buf[i + 1]
- thirdByte = buf[i + 2]
- if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {
- tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | (thirdByte & 0x3F)
- if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {
- codePoint = tempCodePoint
- }
- }
- break
- case 4:
- secondByte = buf[i + 1]
- thirdByte = buf[i + 2]
- fourthByte = buf[i + 3]
- if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {
- tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | (fourthByte & 0x3F)
- if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {
- codePoint = tempCodePoint
- }
- }
- }
- }
-
- if (codePoint === null) {
- // we did not generate a valid codePoint so insert a
- // replacement char (U+FFFD) and advance only 1 byte
- codePoint = 0xFFFD
- bytesPerSequence = 1
- } else if (codePoint > 0xFFFF) {
- // encode to utf16 (surrogate pair dance)
- codePoint -= 0x10000
- res.push(codePoint >>> 10 & 0x3FF | 0xD800)
- codePoint = 0xDC00 | codePoint & 0x3FF
- }
-
- res.push(codePoint)
- i += bytesPerSequence
- }
-
- return decodeCodePointsArray(res)
- }
-
- // Based on http://stackoverflow.com/a/22747272/680742, the browser with
- // the lowest limit is Chrome, with 0x10000 args.
- // We go 1 magnitude less, for safety
- var MAX_ARGUMENTS_LENGTH = 0x1000
-
- function decodeCodePointsArray (codePoints) {
- var len = codePoints.length
- if (len <= MAX_ARGUMENTS_LENGTH) {
- return String.fromCharCode.apply(String, codePoints) // avoid extra slice()
- }
-
- // Decode in chunks to avoid "call stack size exceeded".
- var res = ''
- var i = 0
- while (i < len) {
- res += String.fromCharCode.apply(
- String,
- codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH)
- )
- }
- return res
- }
-
- function asciiSlice (buf, start, end) {
- var ret = ''
- end = Math.min(buf.length, end)
-
- for (var i = start; i < end; ++i) {
- ret += String.fromCharCode(buf[i] & 0x7F)
- }
- return ret
- }
-
- function latin1Slice (buf, start, end) {
- var ret = ''
- end = Math.min(buf.length, end)
-
- for (var i = start; i < end; ++i) {
- ret += String.fromCharCode(buf[i])
- }
- return ret
- }
-
- function hexSlice (buf, start, end) {
- var len = buf.length
-
- if (!start || start < 0) start = 0
- if (!end || end < 0 || end > len) end = len
-
- var out = ''
- for (var i = start; i < end; ++i) {
- out += toHex(buf[i])
- }
- return out
- }
-
- function utf16leSlice (buf, start, end) {
- var bytes = buf.slice(start, end)
- var res = ''
- for (var i = 0; i < bytes.length; i += 2) {
- res += String.fromCharCode(bytes[i] + bytes[i + 1] * 256)
- }
- return res
- }
-
- Buffer.prototype.slice = function slice (start, end) {
- var len = this.length
- start = ~~start
- end = end === undefined ? len : ~~end
-
- if (start < 0) {
- start += len
- if (start < 0) start = 0
- } else if (start > len) {
- start = len
- }
-
- if (end < 0) {
- end += len
- if (end < 0) end = 0
- } else if (end > len) {
- end = len
- }
-
- if (end < start) end = start
-
- var newBuf
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- newBuf = this.subarray(start, end)
- newBuf.__proto__ = Buffer.prototype
- } else {
- var sliceLen = end - start
- newBuf = new Buffer(sliceLen, undefined)
- for (var i = 0; i < sliceLen; ++i) {
- newBuf[i] = this[i + start]
- }
- }
-
- return newBuf
- }
-
- /*
- * Need to make sure that buffer isn't trying to write out of bounds.
- */
- function checkOffset (offset, ext, length) {
- if ((offset % 1) !== 0 || offset < 0) throw new RangeError('offset is not uint')
- if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length')
- }
-
- Buffer.prototype.readUIntLE = function readUIntLE (offset, byteLength, noAssert) {
- offset = offset | 0
- byteLength = byteLength | 0
- if (!noAssert) checkOffset(offset, byteLength, this.length)
-
- var val = this[offset]
- var mul = 1
- var i = 0
- while (++i < byteLength && (mul *= 0x100)) {
- val += this[offset + i] * mul
- }
-
- return val
- }
-
- Buffer.prototype.readUIntBE = function readUIntBE (offset, byteLength, noAssert) {
- offset = offset | 0
- byteLength = byteLength | 0
- if (!noAssert) {
- checkOffset(offset, byteLength, this.length)
- }
-
- var val = this[offset + --byteLength]
- var mul = 1
- while (byteLength > 0 && (mul *= 0x100)) {
- val += this[offset + --byteLength] * mul
- }
-
- return val
- }
-
- Buffer.prototype.readUInt8 = function readUInt8 (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 1, this.length)
- return this[offset]
- }
-
- Buffer.prototype.readUInt16LE = function readUInt16LE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 2, this.length)
- return this[offset] | (this[offset + 1] << 8)
- }
-
- Buffer.prototype.readUInt16BE = function readUInt16BE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 2, this.length)
- return (this[offset] << 8) | this[offset + 1]
- }
-
- Buffer.prototype.readUInt32LE = function readUInt32LE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 4, this.length)
-
- return ((this[offset]) |
- (this[offset + 1] << 8) |
- (this[offset + 2] << 16)) +
- (this[offset + 3] * 0x1000000)
- }
-
- Buffer.prototype.readUInt32BE = function readUInt32BE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 4, this.length)
-
- return (this[offset] * 0x1000000) +
- ((this[offset + 1] << 16) |
- (this[offset + 2] << 8) |
- this[offset + 3])
- }
-
- Buffer.prototype.readIntLE = function readIntLE (offset, byteLength, noAssert) {
- offset = offset | 0
- byteLength = byteLength | 0
- if (!noAssert) checkOffset(offset, byteLength, this.length)
-
- var val = this[offset]
- var mul = 1
- var i = 0
- while (++i < byteLength && (mul *= 0x100)) {
- val += this[offset + i] * mul
- }
- mul *= 0x80
-
- if (val >= mul) val -= Math.pow(2, 8 * byteLength)
-
- return val
- }
-
- Buffer.prototype.readIntBE = function readIntBE (offset, byteLength, noAssert) {
- offset = offset | 0
- byteLength = byteLength | 0
- if (!noAssert) checkOffset(offset, byteLength, this.length)
-
- var i = byteLength
- var mul = 1
- var val = this[offset + --i]
- while (i > 0 && (mul *= 0x100)) {
- val += this[offset + --i] * mul
- }
- mul *= 0x80
-
- if (val >= mul) val -= Math.pow(2, 8 * byteLength)
-
- return val
- }
-
- Buffer.prototype.readInt8 = function readInt8 (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 1, this.length)
- if (!(this[offset] & 0x80)) return (this[offset])
- return ((0xff - this[offset] + 1) * -1)
- }
-
- Buffer.prototype.readInt16LE = function readInt16LE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 2, this.length)
- var val = this[offset] | (this[offset + 1] << 8)
- return (val & 0x8000) ? val | 0xFFFF0000 : val
- }
-
- Buffer.prototype.readInt16BE = function readInt16BE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 2, this.length)
- var val = this[offset + 1] | (this[offset] << 8)
- return (val & 0x8000) ? val | 0xFFFF0000 : val
- }
-
- Buffer.prototype.readInt32LE = function readInt32LE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 4, this.length)
-
- return (this[offset]) |
- (this[offset + 1] << 8) |
- (this[offset + 2] << 16) |
- (this[offset + 3] << 24)
- }
-
- Buffer.prototype.readInt32BE = function readInt32BE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 4, this.length)
-
- return (this[offset] << 24) |
- (this[offset + 1] << 16) |
- (this[offset + 2] << 8) |
- (this[offset + 3])
- }
-
- Buffer.prototype.readFloatLE = function readFloatLE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 4, this.length)
- return ieee754.read(this, offset, true, 23, 4)
- }
-
- Buffer.prototype.readFloatBE = function readFloatBE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 4, this.length)
- return ieee754.read(this, offset, false, 23, 4)
- }
-
- Buffer.prototype.readDoubleLE = function readDoubleLE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 8, this.length)
- return ieee754.read(this, offset, true, 52, 8)
- }
-
- Buffer.prototype.readDoubleBE = function readDoubleBE (offset, noAssert) {
- if (!noAssert) checkOffset(offset, 8, this.length)
- return ieee754.read(this, offset, false, 52, 8)
- }
-
- function checkInt (buf, value, offset, ext, max, min) {
- if (!Buffer.isBuffer(buf)) throw new TypeError('"buffer" argument must be a Buffer instance')
- if (value > max || value < min) throw new RangeError('"value" argument is out of bounds')
- if (offset + ext > buf.length) throw new RangeError('Index out of range')
- }
-
- Buffer.prototype.writeUIntLE = function writeUIntLE (value, offset, byteLength, noAssert) {
- value = +value
- offset = offset | 0
- byteLength = byteLength | 0
- if (!noAssert) {
- var maxBytes = Math.pow(2, 8 * byteLength) - 1
- checkInt(this, value, offset, byteLength, maxBytes, 0)
- }
-
- var mul = 1
- var i = 0
- this[offset] = value & 0xFF
- while (++i < byteLength && (mul *= 0x100)) {
- this[offset + i] = (value / mul) & 0xFF
- }
-
- return offset + byteLength
- }
-
- Buffer.prototype.writeUIntBE = function writeUIntBE (value, offset, byteLength, noAssert) {
- value = +value
- offset = offset | 0
- byteLength = byteLength | 0
- if (!noAssert) {
- var maxBytes = Math.pow(2, 8 * byteLength) - 1
- checkInt(this, value, offset, byteLength, maxBytes, 0)
- }
-
- var i = byteLength - 1
- var mul = 1
- this[offset + i] = value & 0xFF
- while (--i >= 0 && (mul *= 0x100)) {
- this[offset + i] = (value / mul) & 0xFF
- }
-
- return offset + byteLength
- }
-
- Buffer.prototype.writeUInt8 = function writeUInt8 (value, offset, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0)
- if (!Buffer.TYPED_ARRAY_SUPPORT) value = Math.floor(value)
- this[offset] = (value & 0xff)
- return offset + 1
- }
-
- function objectWriteUInt16 (buf, value, offset, littleEndian) {
- if (value < 0) value = 0xffff + value + 1
- for (var i = 0, j = Math.min(buf.length - offset, 2); i < j; ++i) {
- buf[offset + i] = (value & (0xff << (8 * (littleEndian ? i : 1 - i)))) >>>
- (littleEndian ? i : 1 - i) * 8
- }
- }
-
- Buffer.prototype.writeUInt16LE = function writeUInt16LE (value, offset, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- this[offset] = (value & 0xff)
- this[offset + 1] = (value >>> 8)
- } else {
- objectWriteUInt16(this, value, offset, true)
- }
- return offset + 2
- }
-
- Buffer.prototype.writeUInt16BE = function writeUInt16BE (value, offset, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- this[offset] = (value >>> 8)
- this[offset + 1] = (value & 0xff)
- } else {
- objectWriteUInt16(this, value, offset, false)
- }
- return offset + 2
- }
-
- function objectWriteUInt32 (buf, value, offset, littleEndian) {
- if (value < 0) value = 0xffffffff + value + 1
- for (var i = 0, j = Math.min(buf.length - offset, 4); i < j; ++i) {
- buf[offset + i] = (value >>> (littleEndian ? i : 3 - i) * 8) & 0xff
- }
- }
-
- Buffer.prototype.writeUInt32LE = function writeUInt32LE (value, offset, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- this[offset + 3] = (value >>> 24)
- this[offset + 2] = (value >>> 16)
- this[offset + 1] = (value >>> 8)
- this[offset] = (value & 0xff)
- } else {
- objectWriteUInt32(this, value, offset, true)
- }
- return offset + 4
- }
-
- Buffer.prototype.writeUInt32BE = function writeUInt32BE (value, offset, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- this[offset] = (value >>> 24)
- this[offset + 1] = (value >>> 16)
- this[offset + 2] = (value >>> 8)
- this[offset + 3] = (value & 0xff)
- } else {
- objectWriteUInt32(this, value, offset, false)
- }
- return offset + 4
- }
-
- Buffer.prototype.writeIntLE = function writeIntLE (value, offset, byteLength, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) {
- var limit = Math.pow(2, 8 * byteLength - 1)
-
- checkInt(this, value, offset, byteLength, limit - 1, -limit)
- }
-
- var i = 0
- var mul = 1
- var sub = 0
- this[offset] = value & 0xFF
- while (++i < byteLength && (mul *= 0x100)) {
- if (value < 0 && sub === 0 && this[offset + i - 1] !== 0) {
- sub = 1
- }
- this[offset + i] = ((value / mul) >> 0) - sub & 0xFF
- }
-
- return offset + byteLength
- }
-
- Buffer.prototype.writeIntBE = function writeIntBE (value, offset, byteLength, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) {
- var limit = Math.pow(2, 8 * byteLength - 1)
-
- checkInt(this, value, offset, byteLength, limit - 1, -limit)
- }
-
- var i = byteLength - 1
- var mul = 1
- var sub = 0
- this[offset + i] = value & 0xFF
- while (--i >= 0 && (mul *= 0x100)) {
- if (value < 0 && sub === 0 && this[offset + i + 1] !== 0) {
- sub = 1
- }
- this[offset + i] = ((value / mul) >> 0) - sub & 0xFF
- }
-
- return offset + byteLength
- }
-
- Buffer.prototype.writeInt8 = function writeInt8 (value, offset, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80)
- if (!Buffer.TYPED_ARRAY_SUPPORT) value = Math.floor(value)
- if (value < 0) value = 0xff + value + 1
- this[offset] = (value & 0xff)
- return offset + 1
- }
-
- Buffer.prototype.writeInt16LE = function writeInt16LE (value, offset, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- this[offset] = (value & 0xff)
- this[offset + 1] = (value >>> 8)
- } else {
- objectWriteUInt16(this, value, offset, true)
- }
- return offset + 2
- }
-
- Buffer.prototype.writeInt16BE = function writeInt16BE (value, offset, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- this[offset] = (value >>> 8)
- this[offset + 1] = (value & 0xff)
- } else {
- objectWriteUInt16(this, value, offset, false)
- }
- return offset + 2
- }
-
- Buffer.prototype.writeInt32LE = function writeInt32LE (value, offset, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- this[offset] = (value & 0xff)
- this[offset + 1] = (value >>> 8)
- this[offset + 2] = (value >>> 16)
- this[offset + 3] = (value >>> 24)
- } else {
- objectWriteUInt32(this, value, offset, true)
- }
- return offset + 4
- }
-
- Buffer.prototype.writeInt32BE = function writeInt32BE (value, offset, noAssert) {
- value = +value
- offset = offset | 0
- if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)
- if (value < 0) value = 0xffffffff + value + 1
- if (Buffer.TYPED_ARRAY_SUPPORT) {
- this[offset] = (value >>> 24)
- this[offset + 1] = (value >>> 16)
- this[offset + 2] = (value >>> 8)
- this[offset + 3] = (value & 0xff)
- } else {
- objectWriteUInt32(this, value, offset, false)
- }
- return offset + 4
- }
-
- function checkIEEE754 (buf, value, offset, ext, max, min) {
- if (offset + ext > buf.length) throw new RangeError('Index out of range')
- if (offset < 0) throw new RangeError('Index out of range')
- }
-
- function writeFloat (buf, value, offset, littleEndian, noAssert) {
- if (!noAssert) {
- checkIEEE754(buf, value, offset, 4, 3.4028234663852886e+38, -3.4028234663852886e+38)
- }
- ieee754.write(buf, value, offset, littleEndian, 23, 4)
- return offset + 4
- }
-
- Buffer.prototype.writeFloatLE = function writeFloatLE (value, offset, noAssert) {
- return writeFloat(this, value, offset, true, noAssert)
- }
-
- Buffer.prototype.writeFloatBE = function writeFloatBE (value, offset, noAssert) {
- return writeFloat(this, value, offset, false, noAssert)
- }
-
- function writeDouble (buf, value, offset, littleEndian, noAssert) {
- if (!noAssert) {
- checkIEEE754(buf, value, offset, 8, 1.7976931348623157E+308, -1.7976931348623157E+308)
- }
- ieee754.write(buf, value, offset, littleEndian, 52, 8)
- return offset + 8
- }
-
- Buffer.prototype.writeDoubleLE = function writeDoubleLE (value, offset, noAssert) {
- return writeDouble(this, value, offset, true, noAssert)
- }
-
- Buffer.prototype.writeDoubleBE = function writeDoubleBE (value, offset, noAssert) {
- return writeDouble(this, value, offset, false, noAssert)
- }
-
- // copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)
- Buffer.prototype.copy = function copy (target, targetStart, start, end) {
- if (!start) start = 0
- if (!end && end !== 0) end = this.length
- if (targetStart >= target.length) targetStart = target.length
- if (!targetStart) targetStart = 0
- if (end > 0 && end < start) end = start
-
- // Copy 0 bytes; we're done
- if (end === start) return 0
- if (target.length === 0 || this.length === 0) return 0
-
- // Fatal error conditions
- if (targetStart < 0) {
- throw new RangeError('targetStart out of bounds')
- }
- if (start < 0 || start >= this.length) throw new RangeError('sourceStart out of bounds')
- if (end < 0) throw new RangeError('sourceEnd out of bounds')
-
- // Are we oob?
- if (end > this.length) end = this.length
- if (target.length - targetStart < end - start) {
- end = target.length - targetStart + start
- }
-
- var len = end - start
- var i
-
- if (this === target && start < targetStart && targetStart < end) {
- // descending copy from end
- for (i = len - 1; i >= 0; --i) {
- target[i + targetStart] = this[i + start]
- }
- } else if (len < 1000 || !Buffer.TYPED_ARRAY_SUPPORT) {
- // ascending copy from start
- for (i = 0; i < len; ++i) {
- target[i + targetStart] = this[i + start]
- }
- } else {
- Uint8Array.prototype.set.call(
- target,
- this.subarray(start, start + len),
- targetStart
- )
- }
-
- return len
- }
-
- // Usage:
- // buffer.fill(number[, offset[, end]])
- // buffer.fill(buffer[, offset[, end]])
- // buffer.fill(string[, offset[, end]][, encoding])
- Buffer.prototype.fill = function fill (val, start, end, encoding) {
- // Handle string cases:
- if (typeof val === 'string') {
- if (typeof start === 'string') {
- encoding = start
- start = 0
- end = this.length
- } else if (typeof end === 'string') {
- encoding = end
- end = this.length
- }
- if (val.length === 1) {
- var code = val.charCodeAt(0)
- if (code < 256) {
- val = code
- }
- }
- if (encoding !== undefined && typeof encoding !== 'string') {
- throw new TypeError('encoding must be a string')
- }
- if (typeof encoding === 'string' && !Buffer.isEncoding(encoding)) {
- throw new TypeError('Unknown encoding: ' + encoding)
- }
- } else if (typeof val === 'number') {
- val = val & 255
- }
-
- // Invalid ranges are not set to a default, so can range check early.
- if (start < 0 || this.length < start || this.length < end) {
- throw new RangeError('Out of range index')
- }
-
- if (end <= start) {
- return this
- }
-
- start = start >>> 0
- end = end === undefined ? this.length : end >>> 0
-
- if (!val) val = 0
-
- var i
- if (typeof val === 'number') {
- for (i = start; i < end; ++i) {
- this[i] = val
- }
- } else {
- var bytes = Buffer.isBuffer(val)
- ? val
- : utf8ToBytes(new Buffer(val, encoding).toString())
- var len = bytes.length
- for (i = 0; i < end - start; ++i) {
- this[i + start] = bytes[i % len]
- }
- }
-
- return this
- }
-
- // HELPER FUNCTIONS
- // ================
-
- var INVALID_BASE64_RE = /[^+\/0-9A-Za-z-_]/g
-
- function base64clean (str) {
- // Node strips out invalid characters like \n and \t from the string, base64-js does not
- str = stringtrim(str).replace(INVALID_BASE64_RE, '')
- // Node converts strings with length < 2 to ''
- if (str.length < 2) return ''
- // Node allows for non-padded base64 strings (missing trailing ===), base64-js does not
- while (str.length % 4 !== 0) {
- str = str + '='
- }
- return str
- }
-
- function stringtrim (str) {
- if (str.trim) return str.trim()
- return str.replace(/^\s+|\s+$/g, '')
- }
-
- function toHex (n) {
- if (n < 16) return '0' + n.toString(16)
- return n.toString(16)
- }
-
- function utf8ToBytes (string, units) {
- units = units || Infinity
- var codePoint
- var length = string.length
- var leadSurrogate = null
- var bytes = []
-
- for (var i = 0; i < length; ++i) {
- codePoint = string.charCodeAt(i)
-
- // is surrogate component
- if (codePoint > 0xD7FF && codePoint < 0xE000) {
- // last char was a lead
- if (!leadSurrogate) {
- // no lead yet
- if (codePoint > 0xDBFF) {
- // unexpected trail
- if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
- continue
- } else if (i + 1 === length) {
- // unpaired lead
- if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
- continue
- }
-
- // valid lead
- leadSurrogate = codePoint
-
- continue
- }
-
- // 2 leads in a row
- if (codePoint < 0xDC00) {
- if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
- leadSurrogate = codePoint
- continue
- }
-
- // valid surrogate pair
- codePoint = (leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00) + 0x10000
- } else if (leadSurrogate) {
- // valid bmp char, but last char was a lead
- if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
- }
-
- leadSurrogate = null
-
- // encode utf8
- if (codePoint < 0x80) {
- if ((units -= 1) < 0) break
- bytes.push(codePoint)
- } else if (codePoint < 0x800) {
- if ((units -= 2) < 0) break
- bytes.push(
- codePoint >> 0x6 | 0xC0,
- codePoint & 0x3F | 0x80
- )
- } else if (codePoint < 0x10000) {
- if ((units -= 3) < 0) break
- bytes.push(
- codePoint >> 0xC | 0xE0,
- codePoint >> 0x6 & 0x3F | 0x80,
- codePoint & 0x3F | 0x80
- )
- } else if (codePoint < 0x110000) {
- if ((units -= 4) < 0) break
- bytes.push(
- codePoint >> 0x12 | 0xF0,
- codePoint >> 0xC & 0x3F | 0x80,
- codePoint >> 0x6 & 0x3F | 0x80,
- codePoint & 0x3F | 0x80
- )
- } else {
- throw new Error('Invalid code point')
- }
- }
-
- return bytes
- }
-
- function asciiToBytes (str) {
- var byteArray = []
- for (var i = 0; i < str.length; ++i) {
- // Node's code seems to be doing this and not & 0x7F..
- byteArray.push(str.charCodeAt(i) & 0xFF)
- }
- return byteArray
- }
-
- function utf16leToBytes (str, units) {
- var c, hi, lo
- var byteArray = []
- for (var i = 0; i < str.length; ++i) {
- if ((units -= 2) < 0) break
-
- c = str.charCodeAt(i)
- hi = c >> 8
- lo = c % 256
- byteArray.push(lo)
- byteArray.push(hi)
- }
-
- return byteArray
- }
-
- function base64ToBytes (str) {
- return base64.toByteArray(base64clean(str))
- }
-
- function blitBuffer (src, dst, offset, length) {
- for (var i = 0; i < length; ++i) {
- if ((i + offset >= dst.length) || (i >= src.length)) break
- dst[i + offset] = src[i]
- }
- return i
- }
-
- function isnan (val) {
- return val !== val // eslint-disable-line no-self-compare
- }
-
- /* WEBPACK VAR INJECTION */}.call(exports, (function() { return this; }())))
-
-/***/ }),
-/* 340 */
-/***/ (function(module, exports) {
-
- 'use strict'
-
- exports.byteLength = byteLength
- exports.toByteArray = toByteArray
- exports.fromByteArray = fromByteArray
-
- var lookup = []
- var revLookup = []
- var Arr = typeof Uint8Array !== 'undefined' ? Uint8Array : Array
-
- var code = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/'
- for (var i = 0, len = code.length; i < len; ++i) {
- lookup[i] = code[i]
- revLookup[code.charCodeAt(i)] = i
- }
-
- // Support decoding URL-safe base64 strings, as Node.js does.
- // See: https://en.wikipedia.org/wiki/Base64#URL_applications
- revLookup['-'.charCodeAt(0)] = 62
- revLookup['_'.charCodeAt(0)] = 63
-
- function getLens (b64) {
- var len = b64.length
-
- if (len % 4 > 0) {
- throw new Error('Invalid string. Length must be a multiple of 4')
- }
-
- // Trim off extra bytes after placeholder bytes are found
- // See: https://github.com/beatgammit/base64-js/issues/42
- var validLen = b64.indexOf('=')
- if (validLen === -1) validLen = len
-
- var placeHoldersLen = validLen === len
- ? 0
- : 4 - (validLen % 4)
-
- return [validLen, placeHoldersLen]
- }
-
- // base64 is 4/3 + up to two characters of the original data
- function byteLength (b64) {
- var lens = getLens(b64)
- var validLen = lens[0]
- var placeHoldersLen = lens[1]
- return ((validLen + placeHoldersLen) * 3 / 4) - placeHoldersLen
- }
-
- function _byteLength (b64, validLen, placeHoldersLen) {
- return ((validLen + placeHoldersLen) * 3 / 4) - placeHoldersLen
- }
-
- function toByteArray (b64) {
- var tmp
- var lens = getLens(b64)
- var validLen = lens[0]
- var placeHoldersLen = lens[1]
-
- var arr = new Arr(_byteLength(b64, validLen, placeHoldersLen))
-
- var curByte = 0
-
- // if there are placeholders, only get up to the last complete 4 chars
- var len = placeHoldersLen > 0
- ? validLen - 4
- : validLen
-
- var i
- for (i = 0; i < len; i += 4) {
- tmp =
- (revLookup[b64.charCodeAt(i)] << 18) |
- (revLookup[b64.charCodeAt(i + 1)] << 12) |
- (revLookup[b64.charCodeAt(i + 2)] << 6) |
- revLookup[b64.charCodeAt(i + 3)]
- arr[curByte++] = (tmp >> 16) & 0xFF
- arr[curByte++] = (tmp >> 8) & 0xFF
- arr[curByte++] = tmp & 0xFF
- }
-
- if (placeHoldersLen === 2) {
- tmp =
- (revLookup[b64.charCodeAt(i)] << 2) |
- (revLookup[b64.charCodeAt(i + 1)] >> 4)
- arr[curByte++] = tmp & 0xFF
- }
-
- if (placeHoldersLen === 1) {
- tmp =
- (revLookup[b64.charCodeAt(i)] << 10) |
- (revLookup[b64.charCodeAt(i + 1)] << 4) |
- (revLookup[b64.charCodeAt(i + 2)] >> 2)
- arr[curByte++] = (tmp >> 8) & 0xFF
- arr[curByte++] = tmp & 0xFF
- }
-
- return arr
- }
-
- function tripletToBase64 (num) {
- return lookup[num >> 18 & 0x3F] +
- lookup[num >> 12 & 0x3F] +
- lookup[num >> 6 & 0x3F] +
- lookup[num & 0x3F]
- }
-
- function encodeChunk (uint8, start, end) {
- var tmp
- var output = []
- for (var i = start; i < end; i += 3) {
- tmp =
- ((uint8[i] << 16) & 0xFF0000) +
- ((uint8[i + 1] << 8) & 0xFF00) +
- (uint8[i + 2] & 0xFF)
- output.push(tripletToBase64(tmp))
- }
- return output.join('')
- }
-
- function fromByteArray (uint8) {
- var tmp
- var len = uint8.length
- var extraBytes = len % 3 // if we have 1 byte left, pad 2 bytes
- var parts = []
- var maxChunkLength = 16383 // must be multiple of 3
-
- // go through the array every three bytes, we'll deal with trailing stuff later
- for (var i = 0, len2 = len - extraBytes; i < len2; i += maxChunkLength) {
- parts.push(encodeChunk(
- uint8, i, (i + maxChunkLength) > len2 ? len2 : (i + maxChunkLength)
- ))
- }
-
- // pad the end with zeros, but make sure to not forget the extra bytes
- if (extraBytes === 1) {
- tmp = uint8[len - 1]
- parts.push(
- lookup[tmp >> 2] +
- lookup[(tmp << 4) & 0x3F] +
- '=='
- )
- } else if (extraBytes === 2) {
- tmp = (uint8[len - 2] << 8) + uint8[len - 1]
- parts.push(
- lookup[tmp >> 10] +
- lookup[(tmp >> 4) & 0x3F] +
- lookup[(tmp << 2) & 0x3F] +
- '='
- )
- }
-
- return parts.join('')
- }
-
-
-/***/ }),
-/* 341 */
-/***/ (function(module, exports) {
-
- exports.read = function (buffer, offset, isLE, mLen, nBytes) {
- var e, m
- var eLen = (nBytes * 8) - mLen - 1
- var eMax = (1 << eLen) - 1
- var eBias = eMax >> 1
- var nBits = -7
- var i = isLE ? (nBytes - 1) : 0
- var d = isLE ? -1 : 1
- var s = buffer[offset + i]
-
- i += d
-
- e = s & ((1 << (-nBits)) - 1)
- s >>= (-nBits)
- nBits += eLen
- for (; nBits > 0; e = (e * 256) + buffer[offset + i], i += d, nBits -= 8) {}
-
- m = e & ((1 << (-nBits)) - 1)
- e >>= (-nBits)
- nBits += mLen
- for (; nBits > 0; m = (m * 256) + buffer[offset + i], i += d, nBits -= 8) {}
-
- if (e === 0) {
- e = 1 - eBias
- } else if (e === eMax) {
- return m ? NaN : ((s ? -1 : 1) * Infinity)
- } else {
- m = m + Math.pow(2, mLen)
- e = e - eBias
- }
- return (s ? -1 : 1) * m * Math.pow(2, e - mLen)
- }
-
- exports.write = function (buffer, value, offset, isLE, mLen, nBytes) {
- var e, m, c
- var eLen = (nBytes * 8) - mLen - 1
- var eMax = (1 << eLen) - 1
- var eBias = eMax >> 1
- var rt = (mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0)
- var i = isLE ? 0 : (nBytes - 1)
- var d = isLE ? 1 : -1
- var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0
-
- value = Math.abs(value)
-
- if (isNaN(value) || value === Infinity) {
- m = isNaN(value) ? 1 : 0
- e = eMax
- } else {
- e = Math.floor(Math.log(value) / Math.LN2)
- if (value * (c = Math.pow(2, -e)) < 1) {
- e--
- c *= 2
- }
- if (e + eBias >= 1) {
- value += rt / c
- } else {
- value += rt * Math.pow(2, 1 - eBias)
- }
- if (value * c >= 2) {
- e++
- c /= 2
- }
-
- if (e + eBias >= eMax) {
- m = 0
- e = eMax
- } else if (e + eBias >= 1) {
- m = ((value * c) - 1) * Math.pow(2, mLen)
- e = e + eBias
- } else {
- m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen)
- e = 0
- }
- }
-
- for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}
-
- e = (e << mLen) | m
- eLen += mLen
- for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}
-
- buffer[offset + i - d] |= s * 128
- }
-
-
-/***/ }),
-/* 342 */
-/***/ (function(module, exports) {
-
- var toString = {}.toString;
-
- module.exports = Array.isArray || function (arr) {
- return toString.call(arr) == '[object Array]';
- };
-
-
-/***/ }),
-/* 343 */
-/***/ (function(module, exports) {
-
- // shim for using process in browser
- var process = module.exports = {};
-
- // cached from whatever global is present so that test runners that stub it
- // don't break things. But we need to wrap it in a try catch in case it is
- // wrapped in strict mode code which doesn't define any globals. It's inside a
- // function because try/catches deoptimize in certain engines.
-
- var cachedSetTimeout;
- var cachedClearTimeout;
-
- function defaultSetTimout() {
- throw new Error('setTimeout has not been defined');
- }
- function defaultClearTimeout () {
- throw new Error('clearTimeout has not been defined');
- }
- (function () {
- try {
- if (typeof setTimeout === 'function') {
- cachedSetTimeout = setTimeout;
- } else {
- cachedSetTimeout = defaultSetTimout;
- }
- } catch (e) {
- cachedSetTimeout = defaultSetTimout;
- }
- try {
- if (typeof clearTimeout === 'function') {
- cachedClearTimeout = clearTimeout;
- } else {
- cachedClearTimeout = defaultClearTimeout;
- }
- } catch (e) {
- cachedClearTimeout = defaultClearTimeout;
- }
- } ())
- function runTimeout(fun) {
- if (cachedSetTimeout === setTimeout) {
- //normal enviroments in sane situations
- return setTimeout(fun, 0);
- }
- // if setTimeout wasn't available but was latter defined
- if ((cachedSetTimeout === defaultSetTimout || !cachedSetTimeout) && setTimeout) {
- cachedSetTimeout = setTimeout;
- return setTimeout(fun, 0);
- }
- try {
- // when when somebody has screwed with setTimeout but no I.E. maddness
- return cachedSetTimeout(fun, 0);
- } catch(e){
- try {
- // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally
- return cachedSetTimeout.call(null, fun, 0);
- } catch(e){
- // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error
- return cachedSetTimeout.call(this, fun, 0);
- }
- }
-
-
- }
- function runClearTimeout(marker) {
- if (cachedClearTimeout === clearTimeout) {
- //normal enviroments in sane situations
- return clearTimeout(marker);
- }
- // if clearTimeout wasn't available but was latter defined
- if ((cachedClearTimeout === defaultClearTimeout || !cachedClearTimeout) && clearTimeout) {
- cachedClearTimeout = clearTimeout;
- return clearTimeout(marker);
- }
- try {
- // when when somebody has screwed with setTimeout but no I.E. maddness
- return cachedClearTimeout(marker);
- } catch (e){
- try {
- // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally
- return cachedClearTimeout.call(null, marker);
- } catch (e){
- // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error.
- // Some versions of I.E. have different rules for clearTimeout vs setTimeout
- return cachedClearTimeout.call(this, marker);
- }
- }
-
-
-
- }
- var queue = [];
- var draining = false;
- var currentQueue;
- var queueIndex = -1;
-
- function cleanUpNextTick() {
- if (!draining || !currentQueue) {
- return;
- }
- draining = false;
- if (currentQueue.length) {
- queue = currentQueue.concat(queue);
- } else {
- queueIndex = -1;
- }
- if (queue.length) {
- drainQueue();
- }
- }
-
- function drainQueue() {
- if (draining) {
- return;
- }
- var timeout = runTimeout(cleanUpNextTick);
- draining = true;
-
- var len = queue.length;
- while(len) {
- currentQueue = queue;
- queue = [];
- while (++queueIndex < len) {
- if (currentQueue) {
- currentQueue[queueIndex].run();
- }
- }
- queueIndex = -1;
- len = queue.length;
- }
- currentQueue = null;
- draining = false;
- runClearTimeout(timeout);
- }
-
- process.nextTick = function (fun) {
- var args = new Array(arguments.length - 1);
- if (arguments.length > 1) {
- for (var i = 1; i < arguments.length; i++) {
- args[i - 1] = arguments[i];
- }
- }
- queue.push(new Item(fun, args));
- if (queue.length === 1 && !draining) {
- runTimeout(drainQueue);
- }
- };
-
- // v8 likes predictible objects
- function Item(fun, array) {
- this.fun = fun;
- this.array = array;
- }
- Item.prototype.run = function () {
- this.fun.apply(null, this.array);
- };
- process.title = 'browser';
- process.browser = true;
- process.env = {};
- process.argv = [];
- process.version = ''; // empty string to avoid regexp issues
- process.versions = {};
-
- function noop() {}
-
- process.on = noop;
- process.addListener = noop;
- process.once = noop;
- process.off = noop;
- process.removeListener = noop;
- process.removeAllListeners = noop;
- process.emit = noop;
- process.prependListener = noop;
- process.prependOnceListener = noop;
-
- process.listeners = function (name) { return [] }
-
- process.binding = function (name) {
- throw new Error('process.binding is not supported');
- };
-
- process.cwd = function () { return '/' };
- process.chdir = function (dir) {
- throw new Error('process.chdir is not supported');
- };
- process.umask = function() { return 0; };
-
-
-/***/ }),
-/* 344 */
-/***/ (function(module, exports, __webpack_require__) {
-
- /* WEBPACK VAR INJECTION */(function(Buffer) {'use strict';
-
- /**
- * Normalizes our expected stringified form of a function across versions of node
- * @param {Function} fn The function to stringify
- */
-
- function normalizedFunctionString(fn) {
- return fn.toString().replace(/function *\(/, 'function (');
- }
-
- function newBuffer(item, encoding) {
- return new Buffer(item, encoding);
- }
-
- function allocBuffer() {
- return Buffer.alloc.apply(Buffer, arguments);
- }
-
- function toBuffer() {
- return Buffer.from.apply(Buffer, arguments);
- }
-
- module.exports = {
- normalizedFunctionString: normalizedFunctionString,
- allocBuffer: typeof Buffer.alloc === 'function' ? allocBuffer : newBuffer,
- toBuffer: typeof Buffer.from === 'function' ? toBuffer : newBuffer
- };
- /* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(339).Buffer))
-
-/***/ }),
-/* 345 */
-/***/ (function(module, exports, __webpack_require__) {
-
- /* WEBPACK VAR INJECTION */(function(global, process) {// Copyright Joyent, Inc. and other Node contributors.
- //
- // Permission is hereby granted, free of charge, to any person obtaining a
- // copy of this software and associated documentation files (the
- // "Software"), to deal in the Software without restriction, including
- // without limitation the rights to use, copy, modify, merge, publish,
- // distribute, sublicense, and/or sell copies of the Software, and to permit
- // persons to whom the Software is furnished to do so, subject to the
- // following conditions:
- //
- // The above copyright notice and this permission notice shall be included
- // in all copies or substantial portions of the Software.
- //
- // THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
- // OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
- // MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN
- // NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
- // DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
- // OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
- // USE OR OTHER DEALINGS IN THE SOFTWARE.
-
- var formatRegExp = /%[sdj%]/g;
- exports.format = function(f) {
- if (!isString(f)) {
- var objects = [];
- for (var i = 0; i < arguments.length; i++) {
- objects.push(inspect(arguments[i]));
- }
- return objects.join(' ');
- }
-
- var i = 1;
- var args = arguments;
- var len = args.length;
- var str = String(f).replace(formatRegExp, function(x) {
- if (x === '%%') return '%';
- if (i >= len) return x;
- switch (x) {
- case '%s': return String(args[i++]);
- case '%d': return Number(args[i++]);
- case '%j':
- try {
- return JSON.stringify(args[i++]);
- } catch (_) {
- return '[Circular]';
- }
- default:
- return x;
- }
- });
- for (var x = args[i]; i < len; x = args[++i]) {
- if (isNull(x) || !isObject(x)) {
- str += ' ' + x;
- } else {
- str += ' ' + inspect(x);
- }
- }
- return str;
- };
-
-
- // Mark that a method should not be used.
- // Returns a modified function which warns once by default.
- // If --no-deprecation is set, then it is a no-op.
- exports.deprecate = function(fn, msg) {
- // Allow for deprecating things in the process of starting up.
- if (isUndefined(global.process)) {
- return function() {
- return exports.deprecate(fn, msg).apply(this, arguments);
- };
- }
-
- if (process.noDeprecation === true) {
- return fn;
- }
-
- var warned = false;
- function deprecated() {
- if (!warned) {
- if (process.throwDeprecation) {
- throw new Error(msg);
- } else if (process.traceDeprecation) {
- console.trace(msg);
- } else {
- console.error(msg);
- }
- warned = true;
- }
- return fn.apply(this, arguments);
- }
-
- return deprecated;
- };
-
-
- var debugs = {};
- var debugEnviron;
- exports.debuglog = function(set) {
- if (isUndefined(debugEnviron))
- debugEnviron = process.env.NODE_DEBUG || '';
- set = set.toUpperCase();
- if (!debugs[set]) {
- if (new RegExp('\\b' + set + '\\b', 'i').test(debugEnviron)) {
- var pid = process.pid;
- debugs[set] = function() {
- var msg = exports.format.apply(exports, arguments);
- console.error('%s %d: %s', set, pid, msg);
- };
- } else {
- debugs[set] = function() {};
- }
- }
- return debugs[set];
- };
-
-
- /**
- * Echos the value of a value. Trys to print the value out
- * in the best way possible given the different types.
- *
- * @param {Object} obj The object to print out.
- * @param {Object} opts Optional options object that alters the output.
- */
- /* legacy: obj, showHidden, depth, colors*/
- function inspect(obj, opts) {
- // default options
- var ctx = {
- seen: [],
- stylize: stylizeNoColor
- };
- // legacy...
- if (arguments.length >= 3) ctx.depth = arguments[2];
- if (arguments.length >= 4) ctx.colors = arguments[3];
- if (isBoolean(opts)) {
- // legacy...
- ctx.showHidden = opts;
- } else if (opts) {
- // got an "options" object
- exports._extend(ctx, opts);
- }
- // set default options
- if (isUndefined(ctx.showHidden)) ctx.showHidden = false;
- if (isUndefined(ctx.depth)) ctx.depth = 2;
- if (isUndefined(ctx.colors)) ctx.colors = false;
- if (isUndefined(ctx.customInspect)) ctx.customInspect = true;
- if (ctx.colors) ctx.stylize = stylizeWithColor;
- return formatValue(ctx, obj, ctx.depth);
- }
- exports.inspect = inspect;
-
-
- // http://en.wikipedia.org/wiki/ANSI_escape_code#graphics
- inspect.colors = {
- 'bold' : [1, 22],
- 'italic' : [3, 23],
- 'underline' : [4, 24],
- 'inverse' : [7, 27],
- 'white' : [37, 39],
- 'grey' : [90, 39],
- 'black' : [30, 39],
- 'blue' : [34, 39],
- 'cyan' : [36, 39],
- 'green' : [32, 39],
- 'magenta' : [35, 39],
- 'red' : [31, 39],
- 'yellow' : [33, 39]
- };
-
- // Don't use 'blue' not visible on cmd.exe
- inspect.styles = {
- 'special': 'cyan',
- 'number': 'yellow',
- 'boolean': 'yellow',
- 'undefined': 'grey',
- 'null': 'bold',
- 'string': 'green',
- 'date': 'magenta',
- // "name": intentionally not styling
- 'regexp': 'red'
- };
-
-
- function stylizeWithColor(str, styleType) {
- var style = inspect.styles[styleType];
-
- if (style) {
- return '\u001b[' + inspect.colors[style][0] + 'm' + str +
- '\u001b[' + inspect.colors[style][1] + 'm';
- } else {
- return str;
- }
- }
-
-
- function stylizeNoColor(str, styleType) {
- return str;
- }
-
-
- function arrayToHash(array) {
- var hash = {};
-
- array.forEach(function(val, idx) {
- hash[val] = true;
- });
-
- return hash;
- }
-
-
- function formatValue(ctx, value, recurseTimes) {
- // Provide a hook for user-specified inspect functions.
- // Check that value is an object with an inspect function on it
- if (ctx.customInspect &&
- value &&
- isFunction(value.inspect) &&
- // Filter out the util module, it's inspect function is special
- value.inspect !== exports.inspect &&
- // Also filter out any prototype objects using the circular check.
- !(value.constructor && value.constructor.prototype === value)) {
- var ret = value.inspect(recurseTimes, ctx);
- if (!isString(ret)) {
- ret = formatValue(ctx, ret, recurseTimes);
- }
- return ret;
- }
-
- // Primitive types cannot have properties
- var primitive = formatPrimitive(ctx, value);
- if (primitive) {
- return primitive;
- }
-
- // Look up the keys of the object.
- var keys = Object.keys(value);
- var visibleKeys = arrayToHash(keys);
-
- if (ctx.showHidden) {
- keys = Object.getOwnPropertyNames(value);
- }
-
- // IE doesn't make error fields non-enumerable
- // http://msdn.microsoft.com/en-us/library/ie/dww52sbt(v=vs.94).aspx
- if (isError(value)
- && (keys.indexOf('message') >= 0 || keys.indexOf('description') >= 0)) {
- return formatError(value);
- }
-
- // Some type of object without properties can be shortcutted.
- if (keys.length === 0) {
- if (isFunction(value)) {
- var name = value.name ? ': ' + value.name : '';
- return ctx.stylize('[Function' + name + ']', 'special');
- }
- if (isRegExp(value)) {
- return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
- }
- if (isDate(value)) {
- return ctx.stylize(Date.prototype.toString.call(value), 'date');
- }
- if (isError(value)) {
- return formatError(value);
- }
- }
-
- var base = '', array = false, braces = ['{', '}'];
-
- // Make Array say that they are Array
- if (isArray(value)) {
- array = true;
- braces = ['[', ']'];
- }
-
- // Make functions say that they are functions
- if (isFunction(value)) {
- var n = value.name ? ': ' + value.name : '';
- base = ' [Function' + n + ']';
- }
-
- // Make RegExps say that they are RegExps
- if (isRegExp(value)) {
- base = ' ' + RegExp.prototype.toString.call(value);
- }
-
- // Make dates with properties first say the date
- if (isDate(value)) {
- base = ' ' + Date.prototype.toUTCString.call(value);
- }
-
- // Make error with message first say the error
- if (isError(value)) {
- base = ' ' + formatError(value);
- }
-
- if (keys.length === 0 && (!array || value.length == 0)) {
- return braces[0] + base + braces[1];
- }
-
- if (recurseTimes < 0) {
- if (isRegExp(value)) {
- return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
- } else {
- return ctx.stylize('[Object]', 'special');
- }
- }
-
- ctx.seen.push(value);
-
- var output;
- if (array) {
- output = formatArray(ctx, value, recurseTimes, visibleKeys, keys);
- } else {
- output = keys.map(function(key) {
- return formatProperty(ctx, value, recurseTimes, visibleKeys, key, array);
- });
- }
-
- ctx.seen.pop();
-
- return reduceToSingleString(output, base, braces);
- }
-
-
- function formatPrimitive(ctx, value) {
- if (isUndefined(value))
- return ctx.stylize('undefined', 'undefined');
- if (isString(value)) {
- var simple = '\'' + JSON.stringify(value).replace(/^"|"$/g, '')
- .replace(/'/g, "\\'")
- .replace(/\\"/g, '"') + '\'';
- return ctx.stylize(simple, 'string');
- }
- if (isNumber(value))
- return ctx.stylize('' + value, 'number');
- if (isBoolean(value))
- return ctx.stylize('' + value, 'boolean');
- // For some reason typeof null is "object", so special case here.
- if (isNull(value))
- return ctx.stylize('null', 'null');
- }
-
-
- function formatError(value) {
- return '[' + Error.prototype.toString.call(value) + ']';
- }
-
-
- function formatArray(ctx, value, recurseTimes, visibleKeys, keys) {
- var output = [];
- for (var i = 0, l = value.length; i < l; ++i) {
- if (hasOwnProperty(value, String(i))) {
- output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,
- String(i), true));
- } else {
- output.push('');
- }
- }
- keys.forEach(function(key) {
- if (!key.match(/^\d+$/)) {
- output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,
- key, true));
- }
- });
- return output;
- }
-
-
- function formatProperty(ctx, value, recurseTimes, visibleKeys, key, array) {
- var name, str, desc;
- desc = Object.getOwnPropertyDescriptor(value, key) || { value: value[key] };
- if (desc.get) {
- if (desc.set) {
- str = ctx.stylize('[Getter/Setter]', 'special');
- } else {
- str = ctx.stylize('[Getter]', 'special');
- }
- } else {
- if (desc.set) {
- str = ctx.stylize('[Setter]', 'special');
- }
- }
- if (!hasOwnProperty(visibleKeys, key)) {
- name = '[' + key + ']';
- }
- if (!str) {
- if (ctx.seen.indexOf(desc.value) < 0) {
- if (isNull(recurseTimes)) {
- str = formatValue(ctx, desc.value, null);
- } else {
- str = formatValue(ctx, desc.value, recurseTimes - 1);
- }
- if (str.indexOf('\n') > -1) {
- if (array) {
- str = str.split('\n').map(function(line) {
- return ' ' + line;
- }).join('\n').substr(2);
- } else {
- str = '\n' + str.split('\n').map(function(line) {
- return ' ' + line;
- }).join('\n');
- }
- }
- } else {
- str = ctx.stylize('[Circular]', 'special');
- }
- }
- if (isUndefined(name)) {
- if (array && key.match(/^\d+$/)) {
- return str;
- }
- name = JSON.stringify('' + key);
- if (name.match(/^"([a-zA-Z_][a-zA-Z_0-9]*)"$/)) {
- name = name.substr(1, name.length - 2);
- name = ctx.stylize(name, 'name');
- } else {
- name = name.replace(/'/g, "\\'")
- .replace(/\\"/g, '"')
- .replace(/(^"|"$)/g, "'");
- name = ctx.stylize(name, 'string');
- }
- }
-
- return name + ': ' + str;
- }
-
-
- function reduceToSingleString(output, base, braces) {
- var numLinesEst = 0;
- var length = output.reduce(function(prev, cur) {
- numLinesEst++;
- if (cur.indexOf('\n') >= 0) numLinesEst++;
- return prev + cur.replace(/\u001b\[\d\d?m/g, '').length + 1;
- }, 0);
-
- if (length > 60) {
- return braces[0] +
- (base === '' ? '' : base + '\n ') +
- ' ' +
- output.join(',\n ') +
- ' ' +
- braces[1];
- }
-
- return braces[0] + base + ' ' + output.join(', ') + ' ' + braces[1];
- }
-
-
- // NOTE: These type checking functions intentionally don't use `instanceof`
- // because it is fragile and can be easily faked with `Object.create()`.
- function isArray(ar) {
- return Array.isArray(ar);
- }
- exports.isArray = isArray;
-
- function isBoolean(arg) {
- return typeof arg === 'boolean';
- }
- exports.isBoolean = isBoolean;
-
- function isNull(arg) {
- return arg === null;
- }
- exports.isNull = isNull;
-
- function isNullOrUndefined(arg) {
- return arg == null;
- }
- exports.isNullOrUndefined = isNullOrUndefined;
-
- function isNumber(arg) {
- return typeof arg === 'number';
- }
- exports.isNumber = isNumber;
-
- function isString(arg) {
- return typeof arg === 'string';
- }
- exports.isString = isString;
-
- function isSymbol(arg) {
- return typeof arg === 'symbol';
- }
- exports.isSymbol = isSymbol;
-
- function isUndefined(arg) {
- return arg === void 0;
- }
- exports.isUndefined = isUndefined;
-
- function isRegExp(re) {
- return isObject(re) && objectToString(re) === '[object RegExp]';
- }
- exports.isRegExp = isRegExp;
-
- function isObject(arg) {
- return typeof arg === 'object' && arg !== null;
- }
- exports.isObject = isObject;
-
- function isDate(d) {
- return isObject(d) && objectToString(d) === '[object Date]';
- }
- exports.isDate = isDate;
-
- function isError(e) {
- return isObject(e) &&
- (objectToString(e) === '[object Error]' || e instanceof Error);
- }
- exports.isError = isError;
-
- function isFunction(arg) {
- return typeof arg === 'function';
- }
- exports.isFunction = isFunction;
-
- function isPrimitive(arg) {
- return arg === null ||
- typeof arg === 'boolean' ||
- typeof arg === 'number' ||
- typeof arg === 'string' ||
- typeof arg === 'symbol' || // ES6 symbol
- typeof arg === 'undefined';
- }
- exports.isPrimitive = isPrimitive;
-
- exports.isBuffer = __webpack_require__(346);
-
- function objectToString(o) {
- return Object.prototype.toString.call(o);
- }
-
-
- function pad(n) {
- return n < 10 ? '0' + n.toString(10) : n.toString(10);
- }
-
-
- var months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep',
- 'Oct', 'Nov', 'Dec'];
-
- // 26 Feb 16:19:34
- function timestamp() {
- var d = new Date();
- var time = [pad(d.getHours()),
- pad(d.getMinutes()),
- pad(d.getSeconds())].join(':');
- return [d.getDate(), months[d.getMonth()], time].join(' ');
- }
-
-
- // log is just a thin wrapper to console.log that prepends a timestamp
- exports.log = function() {
- console.log('%s - %s', timestamp(), exports.format.apply(exports, arguments));
- };
-
-
- /**
- * Inherit the prototype methods from one constructor into another.
- *
- * The Function.prototype.inherits from lang.js rewritten as a standalone
- * function (not on Function.prototype). NOTE: If this file is to be loaded
- * during bootstrapping this function needs to be rewritten using some native
- * functions as prototype setup using normal JavaScript does not work as
- * expected during bootstrapping (see mirror.js in r114903).
- *
- * @param {function} ctor Constructor function which needs to inherit the
- * prototype.
- * @param {function} superCtor Constructor function to inherit prototype from.
- */
- exports.inherits = __webpack_require__(347);
-
- exports._extend = function(origin, add) {
- // Don't do anything if add isn't an object
- if (!add || !isObject(add)) return origin;
-
- var keys = Object.keys(add);
- var i = keys.length;
- while (i--) {
- origin[keys[i]] = add[keys[i]];
- }
- return origin;
- };
-
- function hasOwnProperty(obj, prop) {
- return Object.prototype.hasOwnProperty.call(obj, prop);
- }
-
- /* WEBPACK VAR INJECTION */}.call(exports, (function() { return this; }()), __webpack_require__(343)))
-
-/***/ }),
-/* 346 */
-/***/ (function(module, exports) {
-
- module.exports = function isBuffer(arg) {
- return arg && typeof arg === 'object'
- && typeof arg.copy === 'function'
- && typeof arg.fill === 'function'
- && typeof arg.readUInt8 === 'function';
- }
-
-/***/ }),
-/* 347 */
-/***/ (function(module, exports) {
-
- if (typeof Object.create === 'function') {
- // implementation from standard node.js 'util' module
- module.exports = function inherits(ctor, superCtor) {
- ctor.super_ = superCtor
- ctor.prototype = Object.create(superCtor.prototype, {
- constructor: {
- value: ctor,
- enumerable: false,
- writable: true,
- configurable: true
- }
- });
- };
- } else {
- // old school shim for old browsers
- module.exports = function inherits(ctor, superCtor) {
- ctor.super_ = superCtor
- var TempCtor = function () {}
- TempCtor.prototype = superCtor.prototype
- ctor.prototype = new TempCtor()
- ctor.prototype.constructor = ctor
- }
- }
-
-
-/***/ }),
-/* 348 */
-/***/ (function(module, exports) {
-
- /**
- * A class representation of the BSON RegExp type.
- *
- * @class
- * @return {BSONRegExp} A MinKey instance
- */
- function BSONRegExp(pattern, options) {
- if (!(this instanceof BSONRegExp)) return new BSONRegExp();
-
- // Execute
- this._bsontype = 'BSONRegExp';
- this.pattern = pattern || '';
- this.options = options || '';
-
- // Validate options
- for (var i = 0; i < this.options.length; i++) {
- if (!(this.options[i] === 'i' || this.options[i] === 'm' || this.options[i] === 'x' || this.options[i] === 'l' || this.options[i] === 's' || this.options[i] === 'u')) {
- throw new Error('the regular expression options [' + this.options[i] + '] is not supported');
- }
- }
- }
-
- module.exports = BSONRegExp;
- module.exports.BSONRegExp = BSONRegExp;
-
-/***/ }),
-/* 349 */
-/***/ (function(module, exports, __webpack_require__) {
-
- /* WEBPACK VAR INJECTION */(function(Buffer) {// Custom inspect property name / symbol.
- var inspect = Buffer ? __webpack_require__(345).inspect.custom || 'inspect' : 'inspect';
-
- /**
- * A class representation of the BSON Symbol type.
- *
- * @class
- * @deprecated
- * @param {string} value the string representing the symbol.
- * @return {Symbol}
- */
- function Symbol(value) {
- if (!(this instanceof Symbol)) return new Symbol(value);
- this._bsontype = 'Symbol';
- this.value = value;
- }
-
- /**
- * Access the wrapped string value.
- *
- * @method
- * @return {String} returns the wrapped string.
- */
- Symbol.prototype.valueOf = function () {
- return this.value;
- };
-
- /**
- * @ignore
- */
- Symbol.prototype.toString = function () {
- return this.value;
- };
-
- /**
- * @ignore
- */
- Symbol.prototype[inspect] = function () {
- return this.value;
- };
-
- /**
- * @ignore
- */
- Symbol.prototype.toJSON = function () {
- return this.value;
- };
-
- module.exports = Symbol;
- module.exports.Symbol = Symbol;
- /* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(339).Buffer))
-
-/***/ }),
-/* 350 */
-/***/ (function(module, exports) {
-
- /**
- * A class representation of a BSON Int32 type.
- *
- * @class
- * @param {number} value the number we want to represent as an int32.
- * @return {Int32}
- */
- var Int32 = function (value) {
- if (!(this instanceof Int32)) return new Int32(value);
-
- this._bsontype = 'Int32';
- this.value = value;
- };
-
- /**
- * Access the number value.
- *
- * @method
- * @return {number} returns the wrapped int32 number.
- */
- Int32.prototype.valueOf = function () {
- return this.value;
- };
-
- /**
- * @ignore
- */
- Int32.prototype.toJSON = function () {
- return this.value;
- };
-
- module.exports = Int32;
- module.exports.Int32 = Int32;
-
-/***/ }),
-/* 351 */
-/***/ (function(module, exports) {
-
- /**
- * A class representation of the BSON Code type.
- *
- * @class
- * @param {(string|function)} code a string or function.
- * @param {Object} [scope] an optional scope for the function.
- * @return {Code}
- */
- var Code = function Code(code, scope) {
- if (!(this instanceof Code)) return new Code(code, scope);
- this._bsontype = 'Code';
- this.code = code;
- this.scope = scope;
- };
-
- /**
- * @ignore
- */
- Code.prototype.toJSON = function () {
- return { scope: this.scope, code: this.code };
- };
-
- module.exports = Code;
- module.exports.Code = Code;
-
-/***/ }),
-/* 352 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
-
- var Long = __webpack_require__(335);
-
- var PARSE_STRING_REGEXP = /^(\+|-)?(\d+|(\d*\.\d*))?(E|e)?([-+])?(\d+)?$/;
- var PARSE_INF_REGEXP = /^(\+|-)?(Infinity|inf)$/i;
- var PARSE_NAN_REGEXP = /^(\+|-)?NaN$/i;
-
- var EXPONENT_MAX = 6111;
- var EXPONENT_MIN = -6176;
- var EXPONENT_BIAS = 6176;
- var MAX_DIGITS = 34;
-
- // Nan value bits as 32 bit values (due to lack of longs)
- var NAN_BUFFER = [0x7c, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00].reverse();
- // Infinity value bits 32 bit values (due to lack of longs)
- var INF_NEGATIVE_BUFFER = [0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00].reverse();
- var INF_POSITIVE_BUFFER = [0x78, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00].reverse();
-
- var EXPONENT_REGEX = /^([-+])?(\d+)?$/;
-
- var utils = __webpack_require__(344);
-
- // Detect if the value is a digit
- var isDigit = function (value) {
- return !isNaN(parseInt(value, 10));
- };
-
- // Divide two uint128 values
- var divideu128 = function (value) {
- var DIVISOR = Long.fromNumber(1000 * 1000 * 1000);
- var _rem = Long.fromNumber(0);
- var i = 0;
-
- if (!value.parts[0] && !value.parts[1] && !value.parts[2] && !value.parts[3]) {
- return { quotient: value, rem: _rem };
- }
-
- for (i = 0; i <= 3; i++) {
- // Adjust remainder to match value of next dividend
- _rem = _rem.shiftLeft(32);
- // Add the divided to _rem
- _rem = _rem.add(new Long(value.parts[i], 0));
- value.parts[i] = _rem.div(DIVISOR).low_;
- _rem = _rem.modulo(DIVISOR);
- }
-
- return { quotient: value, rem: _rem };
- };
-
- // Multiply two Long values and return the 128 bit value
- var multiply64x2 = function (left, right) {
- if (!left && !right) {
- return { high: Long.fromNumber(0), low: Long.fromNumber(0) };
- }
-
- var leftHigh = left.shiftRightUnsigned(32);
- var leftLow = new Long(left.getLowBits(), 0);
- var rightHigh = right.shiftRightUnsigned(32);
- var rightLow = new Long(right.getLowBits(), 0);
-
- var productHigh = leftHigh.multiply(rightHigh);
- var productMid = leftHigh.multiply(rightLow);
- var productMid2 = leftLow.multiply(rightHigh);
- var productLow = leftLow.multiply(rightLow);
-
- productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
- productMid = new Long(productMid.getLowBits(), 0).add(productMid2).add(productLow.shiftRightUnsigned(32));
-
- productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
- productLow = productMid.shiftLeft(32).add(new Long(productLow.getLowBits(), 0));
-
- // Return the 128 bit result
- return { high: productHigh, low: productLow };
- };
-
- var lessThan = function (left, right) {
- // Make values unsigned
- var uhleft = left.high_ >>> 0;
- var uhright = right.high_ >>> 0;
-
- // Compare high bits first
- if (uhleft < uhright) {
- return true;
- } else if (uhleft === uhright) {
- var ulleft = left.low_ >>> 0;
- var ulright = right.low_ >>> 0;
- if (ulleft < ulright) return true;
- }
-
- return false;
- };
-
- // var longtoHex = function(value) {
- // var buffer = utils.allocBuffer(8);
- // var index = 0;
- // // Encode the low 64 bits of the decimal
- // // Encode low bits
- // buffer[index++] = value.low_ & 0xff;
- // buffer[index++] = (value.low_ >> 8) & 0xff;
- // buffer[index++] = (value.low_ >> 16) & 0xff;
- // buffer[index++] = (value.low_ >> 24) & 0xff;
- // // Encode high bits
- // buffer[index++] = value.high_ & 0xff;
- // buffer[index++] = (value.high_ >> 8) & 0xff;
- // buffer[index++] = (value.high_ >> 16) & 0xff;
- // buffer[index++] = (value.high_ >> 24) & 0xff;
- // return buffer.reverse().toString('hex');
- // };
-
- // var int32toHex = function(value) {
- // var buffer = utils.allocBuffer(4);
- // var index = 0;
- // // Encode the low 64 bits of the decimal
- // // Encode low bits
- // buffer[index++] = value & 0xff;
- // buffer[index++] = (value >> 8) & 0xff;
- // buffer[index++] = (value >> 16) & 0xff;
- // buffer[index++] = (value >> 24) & 0xff;
- // return buffer.reverse().toString('hex');
- // };
-
- /**
- * A class representation of the BSON Decimal128 type.
- *
- * @class
- * @param {Buffer} bytes a buffer containing the raw Decimal128 bytes.
- * @return {Double}
- */
- var Decimal128 = function (bytes) {
- this._bsontype = 'Decimal128';
- this.bytes = bytes;
- };
-
- /**
- * Create a Decimal128 instance from a string representation
- *
- * @method
- * @param {string} string a numeric string representation.
- * @return {Decimal128} returns a Decimal128 instance.
- */
- Decimal128.fromString = function (string) {
- // Parse state tracking
- var isNegative = false;
- var sawRadix = false;
- var foundNonZero = false;
-
- // Total number of significant digits (no leading or trailing zero)
- var significantDigits = 0;
- // Total number of significand digits read
- var nDigitsRead = 0;
- // Total number of digits (no leading zeros)
- var nDigits = 0;
- // The number of the digits after radix
- var radixPosition = 0;
- // The index of the first non-zero in *str*
- var firstNonZero = 0;
-
- // Digits Array
- var digits = [0];
- // The number of digits in digits
- var nDigitsStored = 0;
- // Insertion pointer for digits
- var digitsInsert = 0;
- // The index of the first non-zero digit
- var firstDigit = 0;
- // The index of the last digit
- var lastDigit = 0;
-
- // Exponent
- var exponent = 0;
- // loop index over array
- var i = 0;
- // The high 17 digits of the significand
- var significandHigh = [0, 0];
- // The low 17 digits of the significand
- var significandLow = [0, 0];
- // The biased exponent
- var biasedExponent = 0;
-
- // Read index
- var index = 0;
-
- // Trim the string
- string = string.trim();
-
- // Naively prevent against REDOS attacks.
- // TODO: implementing a custom parsing for this, or refactoring the regex would yield
- // further gains.
- if (string.length >= 7000) {
- throw new Error('' + string + ' not a valid Decimal128 string');
- }
-
- // Results
- var stringMatch = string.match(PARSE_STRING_REGEXP);
- var infMatch = string.match(PARSE_INF_REGEXP);
- var nanMatch = string.match(PARSE_NAN_REGEXP);
-
- // Validate the string
- if (!stringMatch && !infMatch && !nanMatch || string.length === 0) {
- throw new Error('' + string + ' not a valid Decimal128 string');
- }
-
- // Check if we have an illegal exponent format
- if (stringMatch && stringMatch[4] && stringMatch[2] === undefined) {
- throw new Error('' + string + ' not a valid Decimal128 string');
- }
-
- // Get the negative or positive sign
- if (string[index] === '+' || string[index] === '-') {
- isNegative = string[index++] === '-';
- }
-
- // Check if user passed Infinity or NaN
- if (!isDigit(string[index]) && string[index] !== '.') {
- if (string[index] === 'i' || string[index] === 'I') {
- return new Decimal128(utils.toBuffer(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
- } else if (string[index] === 'N') {
- return new Decimal128(utils.toBuffer(NAN_BUFFER));
- }
- }
-
- // Read all the digits
- while (isDigit(string[index]) || string[index] === '.') {
- if (string[index] === '.') {
- if (sawRadix) {
- return new Decimal128(utils.toBuffer(NAN_BUFFER));
- }
-
- sawRadix = true;
- index = index + 1;
- continue;
- }
-
- if (nDigitsStored < 34) {
- if (string[index] !== '0' || foundNonZero) {
- if (!foundNonZero) {
- firstNonZero = nDigitsRead;
- }
-
- foundNonZero = true;
-
- // Only store 34 digits
- digits[digitsInsert++] = parseInt(string[index], 10);
- nDigitsStored = nDigitsStored + 1;
- }
- }
-
- if (foundNonZero) {
- nDigits = nDigits + 1;
- }
-
- if (sawRadix) {
- radixPosition = radixPosition + 1;
- }
-
- nDigitsRead = nDigitsRead + 1;
- index = index + 1;
- }
-
- if (sawRadix && !nDigitsRead) {
- throw new Error('' + string + ' not a valid Decimal128 string');
- }
-
- // Read exponent if exists
- if (string[index] === 'e' || string[index] === 'E') {
- // Read exponent digits
- var match = string.substr(++index).match(EXPONENT_REGEX);
-
- // No digits read
- if (!match || !match[2]) {
- return new Decimal128(utils.toBuffer(NAN_BUFFER));
- }
-
- // Get exponent
- exponent = parseInt(match[0], 10);
-
- // Adjust the index
- index = index + match[0].length;
- }
-
- // Return not a number
- if (string[index]) {
- return new Decimal128(utils.toBuffer(NAN_BUFFER));
- }
-
- // Done reading input
- // Find first non-zero digit in digits
- firstDigit = 0;
-
- if (!nDigitsStored) {
- firstDigit = 0;
- lastDigit = 0;
- digits[0] = 0;
- nDigits = 1;
- nDigitsStored = 1;
- significantDigits = 0;
- } else {
- lastDigit = nDigitsStored - 1;
- significantDigits = nDigits;
-
- if (exponent !== 0 && significantDigits !== 1) {
- while (string[firstNonZero + significantDigits - 1] === '0') {
- significantDigits = significantDigits - 1;
- }
- }
- }
-
- // Normalization of exponent
- // Correct exponent based on radix position, and shift significand as needed
- // to represent user input
-
- // Overflow prevention
- if (exponent <= radixPosition && radixPosition - exponent > 1 << 14) {
- exponent = EXPONENT_MIN;
- } else {
- exponent = exponent - radixPosition;
- }
-
- // Attempt to normalize the exponent
- while (exponent > EXPONENT_MAX) {
- // Shift exponent to significand and decrease
- lastDigit = lastDigit + 1;
-
- if (lastDigit - firstDigit > MAX_DIGITS) {
- // Check if we have a zero then just hard clamp, otherwise fail
- var digitsString = digits.join('');
- if (digitsString.match(/^0+$/)) {
- exponent = EXPONENT_MAX;
- break;
- } else {
- return new Decimal128(utils.toBuffer(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
- }
- }
-
- exponent = exponent - 1;
- }
-
- while (exponent < EXPONENT_MIN || nDigitsStored < nDigits) {
- // Shift last digit
- if (lastDigit === 0) {
- exponent = EXPONENT_MIN;
- significantDigits = 0;
- break;
- }
-
- if (nDigitsStored < nDigits) {
- // adjust to match digits not stored
- nDigits = nDigits - 1;
- } else {
- // adjust to round
- lastDigit = lastDigit - 1;
- }
-
- if (exponent < EXPONENT_MAX) {
- exponent = exponent + 1;
- } else {
- // Check if we have a zero then just hard clamp, otherwise fail
- digitsString = digits.join('');
- if (digitsString.match(/^0+$/)) {
- exponent = EXPONENT_MAX;
- break;
- } else {
- return new Decimal128(utils.toBuffer(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
- }
- }
- }
-
- // Round
- // We've normalized the exponent, but might still need to round.
- if (lastDigit - firstDigit + 1 < significantDigits && string[significantDigits] !== '0') {
- var endOfString = nDigitsRead;
-
- // If we have seen a radix point, 'string' is 1 longer than we have
- // documented with ndigits_read, so inc the position of the first nonzero
- // digit and the position that digits are read to.
- if (sawRadix && exponent === EXPONENT_MIN) {
- firstNonZero = firstNonZero + 1;
- endOfString = endOfString + 1;
- }
-
- var roundDigit = parseInt(string[firstNonZero + lastDigit + 1], 10);
- var roundBit = 0;
-
- if (roundDigit >= 5) {
- roundBit = 1;
-
- if (roundDigit === 5) {
- roundBit = digits[lastDigit] % 2 === 1;
-
- for (i = firstNonZero + lastDigit + 2; i < endOfString; i++) {
- if (parseInt(string[i], 10)) {
- roundBit = 1;
- break;
- }
- }
- }
- }
-
- if (roundBit) {
- var dIdx = lastDigit;
-
- for (; dIdx >= 0; dIdx--) {
- if (++digits[dIdx] > 9) {
- digits[dIdx] = 0;
-
- // overflowed most significant digit
- if (dIdx === 0) {
- if (exponent < EXPONENT_MAX) {
- exponent = exponent + 1;
- digits[dIdx] = 1;
- } else {
- return new Decimal128(utils.toBuffer(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
- }
- }
- } else {
- break;
- }
- }
- }
- }
-
- // Encode significand
- // The high 17 digits of the significand
- significandHigh = Long.fromNumber(0);
- // The low 17 digits of the significand
- significandLow = Long.fromNumber(0);
-
- // read a zero
- if (significantDigits === 0) {
- significandHigh = Long.fromNumber(0);
- significandLow = Long.fromNumber(0);
- } else if (lastDigit - firstDigit < 17) {
- dIdx = firstDigit;
- significandLow = Long.fromNumber(digits[dIdx++]);
- significandHigh = new Long(0, 0);
-
- for (; dIdx <= lastDigit; dIdx++) {
- significandLow = significandLow.multiply(Long.fromNumber(10));
- significandLow = significandLow.add(Long.fromNumber(digits[dIdx]));
- }
- } else {
- dIdx = firstDigit;
- significandHigh = Long.fromNumber(digits[dIdx++]);
-
- for (; dIdx <= lastDigit - 17; dIdx++) {
- significandHigh = significandHigh.multiply(Long.fromNumber(10));
- significandHigh = significandHigh.add(Long.fromNumber(digits[dIdx]));
- }
-
- significandLow = Long.fromNumber(digits[dIdx++]);
-
- for (; dIdx <= lastDigit; dIdx++) {
- significandLow = significandLow.multiply(Long.fromNumber(10));
- significandLow = significandLow.add(Long.fromNumber(digits[dIdx]));
- }
- }
-
- var significand = multiply64x2(significandHigh, Long.fromString('100000000000000000'));
-
- significand.low = significand.low.add(significandLow);
-
- if (lessThan(significand.low, significandLow)) {
- significand.high = significand.high.add(Long.fromNumber(1));
- }
-
- // Biased exponent
- biasedExponent = exponent + EXPONENT_BIAS;
- var dec = { low: Long.fromNumber(0), high: Long.fromNumber(0) };
-
- // Encode combination, exponent, and significand.
- if (significand.high.shiftRightUnsigned(49).and(Long.fromNumber(1)).equals(Long.fromNumber)) {
- // Encode '11' into bits 1 to 3
- dec.high = dec.high.or(Long.fromNumber(0x3).shiftLeft(61));
- dec.high = dec.high.or(Long.fromNumber(biasedExponent).and(Long.fromNumber(0x3fff).shiftLeft(47)));
- dec.high = dec.high.or(significand.high.and(Long.fromNumber(0x7fffffffffff)));
- } else {
- dec.high = dec.high.or(Long.fromNumber(biasedExponent & 0x3fff).shiftLeft(49));
- dec.high = dec.high.or(significand.high.and(Long.fromNumber(0x1ffffffffffff)));
- }
-
- dec.low = significand.low;
-
- // Encode sign
- if (isNegative) {
- dec.high = dec.high.or(Long.fromString('9223372036854775808'));
- }
-
- // Encode into a buffer
- var buffer = utils.allocBuffer(16);
- index = 0;
-
- // Encode the low 64 bits of the decimal
- // Encode low bits
- buffer[index++] = dec.low.low_ & 0xff;
- buffer[index++] = dec.low.low_ >> 8 & 0xff;
- buffer[index++] = dec.low.low_ >> 16 & 0xff;
- buffer[index++] = dec.low.low_ >> 24 & 0xff;
- // Encode high bits
- buffer[index++] = dec.low.high_ & 0xff;
- buffer[index++] = dec.low.high_ >> 8 & 0xff;
- buffer[index++] = dec.low.high_ >> 16 & 0xff;
- buffer[index++] = dec.low.high_ >> 24 & 0xff;
-
- // Encode the high 64 bits of the decimal
- // Encode low bits
- buffer[index++] = dec.high.low_ & 0xff;
- buffer[index++] = dec.high.low_ >> 8 & 0xff;
- buffer[index++] = dec.high.low_ >> 16 & 0xff;
- buffer[index++] = dec.high.low_ >> 24 & 0xff;
- // Encode high bits
- buffer[index++] = dec.high.high_ & 0xff;
- buffer[index++] = dec.high.high_ >> 8 & 0xff;
- buffer[index++] = dec.high.high_ >> 16 & 0xff;
- buffer[index++] = dec.high.high_ >> 24 & 0xff;
-
- // Return the new Decimal128
- return new Decimal128(buffer);
- };
-
- // Extract least significant 5 bits
- var COMBINATION_MASK = 0x1f;
- // Extract least significant 14 bits
- var EXPONENT_MASK = 0x3fff;
- // Value of combination field for Inf
- var COMBINATION_INFINITY = 30;
- // Value of combination field for NaN
- var COMBINATION_NAN = 31;
- // Value of combination field for NaN
- // var COMBINATION_SNAN = 32;
- // decimal128 exponent bias
- EXPONENT_BIAS = 6176;
-
- /**
- * Create a string representation of the raw Decimal128 value
- *
- * @method
- * @return {string} returns a Decimal128 string representation.
- */
- Decimal128.prototype.toString = function () {
- // Note: bits in this routine are referred to starting at 0,
- // from the sign bit, towards the coefficient.
-
- // bits 0 - 31
- var high;
- // bits 32 - 63
- var midh;
- // bits 64 - 95
- var midl;
- // bits 96 - 127
- var low;
- // bits 1 - 5
- var combination;
- // decoded biased exponent (14 bits)
- var biased_exponent;
- // the number of significand digits
- var significand_digits = 0;
- // the base-10 digits in the significand
- var significand = new Array(36);
- for (var i = 0; i < significand.length; i++) significand[i] = 0;
- // read pointer into significand
- var index = 0;
-
- // unbiased exponent
- var exponent;
- // the exponent if scientific notation is used
- var scientific_exponent;
-
- // true if the number is zero
- var is_zero = false;
-
- // the most signifcant significand bits (50-46)
- var significand_msb;
- // temporary storage for significand decoding
- var significand128 = { parts: new Array(4) };
- // indexing variables
- i;
- var j, k;
-
- // Output string
- var string = [];
-
- // Unpack index
- index = 0;
-
- // Buffer reference
- var buffer = this.bytes;
-
- // Unpack the low 64bits into a long
- low = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- midl = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
-
- // Unpack the high 64bits into a long
- midh = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- high = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
-
- // Unpack index
- index = 0;
-
- // Create the state of the decimal
- var dec = {
- low: new Long(low, midl),
- high: new Long(midh, high)
- };
-
- if (dec.high.lessThan(Long.ZERO)) {
- string.push('-');
- }
-
- // Decode combination field and exponent
- combination = high >> 26 & COMBINATION_MASK;
-
- if (combination >> 3 === 3) {
- // Check for 'special' values
- if (combination === COMBINATION_INFINITY) {
- return string.join('') + 'Infinity';
- } else if (combination === COMBINATION_NAN) {
- return 'NaN';
- } else {
- biased_exponent = high >> 15 & EXPONENT_MASK;
- significand_msb = 0x08 + (high >> 14 & 0x01);
- }
- } else {
- significand_msb = high >> 14 & 0x07;
- biased_exponent = high >> 17 & EXPONENT_MASK;
- }
-
- exponent = biased_exponent - EXPONENT_BIAS;
-
- // Create string of significand digits
-
- // Convert the 114-bit binary number represented by
- // (significand_high, significand_low) to at most 34 decimal
- // digits through modulo and division.
- significand128.parts[0] = (high & 0x3fff) + ((significand_msb & 0xf) << 14);
- significand128.parts[1] = midh;
- significand128.parts[2] = midl;
- significand128.parts[3] = low;
-
- if (significand128.parts[0] === 0 && significand128.parts[1] === 0 && significand128.parts[2] === 0 && significand128.parts[3] === 0) {
- is_zero = true;
- } else {
- for (k = 3; k >= 0; k--) {
- var least_digits = 0;
- // Peform the divide
- var result = divideu128(significand128);
- significand128 = result.quotient;
- least_digits = result.rem.low_;
-
- // We now have the 9 least significant digits (in base 2).
- // Convert and output to string.
- if (!least_digits) continue;
-
- for (j = 8; j >= 0; j--) {
- // significand[k * 9 + j] = Math.round(least_digits % 10);
- significand[k * 9 + j] = least_digits % 10;
- // least_digits = Math.round(least_digits / 10);
- least_digits = Math.floor(least_digits / 10);
- }
- }
- }
-
- // Output format options:
- // Scientific - [-]d.dddE(+/-)dd or [-]dE(+/-)dd
- // Regular - ddd.ddd
-
- if (is_zero) {
- significand_digits = 1;
- significand[index] = 0;
- } else {
- significand_digits = 36;
- i = 0;
-
- while (!significand[index]) {
- i++;
- significand_digits = significand_digits - 1;
- index = index + 1;
- }
- }
-
- scientific_exponent = significand_digits - 1 + exponent;
-
- // The scientific exponent checks are dictated by the string conversion
- // specification and are somewhat arbitrary cutoffs.
- //
- // We must check exponent > 0, because if this is the case, the number
- // has trailing zeros. However, we *cannot* output these trailing zeros,
- // because doing so would change the precision of the value, and would
- // change stored data if the string converted number is round tripped.
-
- if (scientific_exponent >= 34 || scientific_exponent <= -7 || exponent > 0) {
- // Scientific format
- string.push(significand[index++]);
- significand_digits = significand_digits - 1;
-
- if (significand_digits) {
- string.push('.');
- }
-
- for (i = 0; i < significand_digits; i++) {
- string.push(significand[index++]);
- }
-
- // Exponent
- string.push('E');
- if (scientific_exponent > 0) {
- string.push('+' + scientific_exponent);
- } else {
- string.push(scientific_exponent);
- }
- } else {
- // Regular format with no decimal place
- if (exponent >= 0) {
- for (i = 0; i < significand_digits; i++) {
- string.push(significand[index++]);
- }
- } else {
- var radix_position = significand_digits + exponent;
-
- // non-zero digits before radix
- if (radix_position > 0) {
- for (i = 0; i < radix_position; i++) {
- string.push(significand[index++]);
- }
- } else {
- string.push('0');
- }
-
- string.push('.');
- // add leading zeros after radix
- while (radix_position++ < 0) {
- string.push('0');
- }
-
- for (i = 0; i < significand_digits - Math.max(radix_position - 1, 0); i++) {
- string.push(significand[index++]);
- }
- }
- }
-
- return string.join('');
- };
-
- Decimal128.prototype.toJSON = function () {
- return { $numberDecimal: this.toString() };
- };
-
- module.exports = Decimal128;
- module.exports.Decimal128 = Decimal128;
-
-/***/ }),
-/* 353 */
-/***/ (function(module, exports) {
-
- /**
- * A class representation of the BSON MinKey type.
- *
- * @class
- * @return {MinKey} A MinKey instance
- */
- function MinKey() {
- if (!(this instanceof MinKey)) return new MinKey();
-
- this._bsontype = 'MinKey';
- }
-
- module.exports = MinKey;
- module.exports.MinKey = MinKey;
-
-/***/ }),
-/* 354 */
-/***/ (function(module, exports) {
-
- /**
- * A class representation of the BSON MaxKey type.
- *
- * @class
- * @return {MaxKey} A MaxKey instance
- */
- function MaxKey() {
- if (!(this instanceof MaxKey)) return new MaxKey();
-
- this._bsontype = 'MaxKey';
- }
-
- module.exports = MaxKey;
- module.exports.MaxKey = MaxKey;
-
-/***/ }),
-/* 355 */
-/***/ (function(module, exports) {
-
- /**
- * A class representation of the BSON DBRef type.
- *
- * @class
- * @param {string} namespace the collection name.
- * @param {ObjectID} oid the reference ObjectID.
- * @param {string} [db] optional db name, if omitted the reference is local to the current db.
- * @return {DBRef}
- */
- function DBRef(namespace, oid, db) {
- if (!(this instanceof DBRef)) return new DBRef(namespace, oid, db);
-
- this._bsontype = 'DBRef';
- this.namespace = namespace;
- this.oid = oid;
- this.db = db;
- }
-
- /**
- * @ignore
- * @api private
- */
- DBRef.prototype.toJSON = function () {
- return {
- $ref: this.namespace,
- $id: this.oid,
- $db: this.db == null ? '' : this.db
- };
- };
-
- module.exports = DBRef;
- module.exports.DBRef = DBRef;
-
-/***/ }),
-/* 356 */
-/***/ (function(module, exports, __webpack_require__) {
-
- /* WEBPACK VAR INJECTION */(function(global) {/**
- * Module dependencies.
- * @ignore
- */
-
- // Test if we're in Node via presence of "global" not absence of "window"
- // to support hybrid environments like Electron
- if (typeof global !== 'undefined') {
- var Buffer = __webpack_require__(339).Buffer; // TODO just use global Buffer
- }
-
- var utils = __webpack_require__(344);
-
- /**
- * A class representation of the BSON Binary type.
- *
- * Sub types
- * - **BSON.BSON_BINARY_SUBTYPE_DEFAULT**, default BSON type.
- * - **BSON.BSON_BINARY_SUBTYPE_FUNCTION**, BSON function type.
- * - **BSON.BSON_BINARY_SUBTYPE_BYTE_ARRAY**, BSON byte array type.
- * - **BSON.BSON_BINARY_SUBTYPE_UUID**, BSON uuid type.
- * - **BSON.BSON_BINARY_SUBTYPE_MD5**, BSON md5 type.
- * - **BSON.BSON_BINARY_SUBTYPE_USER_DEFINED**, BSON user defined type.
- *
- * @class
- * @param {Buffer} buffer a buffer object containing the binary data.
- * @param {Number} [subType] the option binary type.
- * @return {Binary}
- */
- function Binary(buffer, subType) {
- if (!(this instanceof Binary)) return new Binary(buffer, subType);
-
- if (buffer != null && !(typeof buffer === 'string') && !Buffer.isBuffer(buffer) && !(buffer instanceof Uint8Array) && !Array.isArray(buffer)) {
- throw new Error('only String, Buffer, Uint8Array or Array accepted');
- }
-
- this._bsontype = 'Binary';
-
- if (buffer instanceof Number) {
- this.sub_type = buffer;
- this.position = 0;
- } else {
- this.sub_type = subType == null ? BSON_BINARY_SUBTYPE_DEFAULT : subType;
- this.position = 0;
- }
-
- if (buffer != null && !(buffer instanceof Number)) {
- // Only accept Buffer, Uint8Array or Arrays
- if (typeof buffer === 'string') {
- // Different ways of writing the length of the string for the different types
- if (typeof Buffer !== 'undefined') {
- this.buffer = utils.toBuffer(buffer);
- } else if (typeof Uint8Array !== 'undefined' || Object.prototype.toString.call(buffer) === '[object Array]') {
- this.buffer = writeStringToArray(buffer);
- } else {
- throw new Error('only String, Buffer, Uint8Array or Array accepted');
- }
- } else {
- this.buffer = buffer;
- }
- this.position = buffer.length;
- } else {
- if (typeof Buffer !== 'undefined') {
- this.buffer = utils.allocBuffer(Binary.BUFFER_SIZE);
- } else if (typeof Uint8Array !== 'undefined') {
- this.buffer = new Uint8Array(new ArrayBuffer(Binary.BUFFER_SIZE));
- } else {
- this.buffer = new Array(Binary.BUFFER_SIZE);
- }
- // Set position to start of buffer
- this.position = 0;
- }
- }
-
- /**
- * Updates this binary with byte_value.
- *
- * @method
- * @param {string} byte_value a single byte we wish to write.
- */
- Binary.prototype.put = function put(byte_value) {
- // If it's a string and a has more than one character throw an error
- if (byte_value['length'] != null && typeof byte_value !== 'number' && byte_value.length !== 1) throw new Error('only accepts single character String, Uint8Array or Array');
- if (typeof byte_value !== 'number' && byte_value < 0 || byte_value > 255) throw new Error('only accepts number in a valid unsigned byte range 0-255');
-
- // Decode the byte value once
- var decoded_byte = null;
- if (typeof byte_value === 'string') {
- decoded_byte = byte_value.charCodeAt(0);
- } else if (byte_value['length'] != null) {
- decoded_byte = byte_value[0];
- } else {
- decoded_byte = byte_value;
- }
-
- if (this.buffer.length > this.position) {
- this.buffer[this.position++] = decoded_byte;
- } else {
- if (typeof Buffer !== 'undefined' && Buffer.isBuffer(this.buffer)) {
- // Create additional overflow buffer
- var buffer = utils.allocBuffer(Binary.BUFFER_SIZE + this.buffer.length);
- // Combine the two buffers together
- this.buffer.copy(buffer, 0, 0, this.buffer.length);
- this.buffer = buffer;
- this.buffer[this.position++] = decoded_byte;
- } else {
- buffer = null;
- // Create a new buffer (typed or normal array)
- if (Object.prototype.toString.call(this.buffer) === '[object Uint8Array]') {
- buffer = new Uint8Array(new ArrayBuffer(Binary.BUFFER_SIZE + this.buffer.length));
- } else {
- buffer = new Array(Binary.BUFFER_SIZE + this.buffer.length);
- }
-
- // We need to copy all the content to the new array
- for (var i = 0; i < this.buffer.length; i++) {
- buffer[i] = this.buffer[i];
- }
-
- // Reassign the buffer
- this.buffer = buffer;
- // Write the byte
- this.buffer[this.position++] = decoded_byte;
- }
- }
- };
-
- /**
- * Writes a buffer or string to the binary.
- *
- * @method
- * @param {(Buffer|string)} string a string or buffer to be written to the Binary BSON object.
- * @param {number} offset specify the binary of where to write the content.
- * @return {null}
- */
- Binary.prototype.write = function write(string, offset) {
- offset = typeof offset === 'number' ? offset : this.position;
-
- // If the buffer is to small let's extend the buffer
- if (this.buffer.length < offset + string.length) {
- var buffer = null;
- // If we are in node.js
- if (typeof Buffer !== 'undefined' && Buffer.isBuffer(this.buffer)) {
- buffer = utils.allocBuffer(this.buffer.length + string.length);
- this.buffer.copy(buffer, 0, 0, this.buffer.length);
- } else if (Object.prototype.toString.call(this.buffer) === '[object Uint8Array]') {
- // Create a new buffer
- buffer = new Uint8Array(new ArrayBuffer(this.buffer.length + string.length));
- // Copy the content
- for (var i = 0; i < this.position; i++) {
- buffer[i] = this.buffer[i];
- }
- }
-
- // Assign the new buffer
- this.buffer = buffer;
- }
-
- if (typeof Buffer !== 'undefined' && Buffer.isBuffer(string) && Buffer.isBuffer(this.buffer)) {
- string.copy(this.buffer, offset, 0, string.length);
- this.position = offset + string.length > this.position ? offset + string.length : this.position;
- // offset = string.length
- } else if (typeof Buffer !== 'undefined' && typeof string === 'string' && Buffer.isBuffer(this.buffer)) {
- this.buffer.write(string, offset, 'binary');
- this.position = offset + string.length > this.position ? offset + string.length : this.position;
- // offset = string.length;
- } else if (Object.prototype.toString.call(string) === '[object Uint8Array]' || Object.prototype.toString.call(string) === '[object Array]' && typeof string !== 'string') {
- for (i = 0; i < string.length; i++) {
- this.buffer[offset++] = string[i];
- }
-
- this.position = offset > this.position ? offset : this.position;
- } else if (typeof string === 'string') {
- for (i = 0; i < string.length; i++) {
- this.buffer[offset++] = string.charCodeAt(i);
- }
-
- this.position = offset > this.position ? offset : this.position;
- }
- };
-
- /**
- * Reads **length** bytes starting at **position**.
- *
- * @method
- * @param {number} position read from the given position in the Binary.
- * @param {number} length the number of bytes to read.
- * @return {Buffer}
- */
- Binary.prototype.read = function read(position, length) {
- length = length && length > 0 ? length : this.position;
-
- // Let's return the data based on the type we have
- if (this.buffer['slice']) {
- return this.buffer.slice(position, position + length);
- } else {
- // Create a buffer to keep the result
- var buffer = typeof Uint8Array !== 'undefined' ? new Uint8Array(new ArrayBuffer(length)) : new Array(length);
- for (var i = 0; i < length; i++) {
- buffer[i] = this.buffer[position++];
- }
- }
- // Return the buffer
- return buffer;
- };
-
- /**
- * Returns the value of this binary as a string.
- *
- * @method
- * @return {string}
- */
- Binary.prototype.value = function value(asRaw) {
- asRaw = asRaw == null ? false : asRaw;
-
- // Optimize to serialize for the situation where the data == size of buffer
- if (asRaw && typeof Buffer !== 'undefined' && Buffer.isBuffer(this.buffer) && this.buffer.length === this.position) return this.buffer;
-
- // If it's a node.js buffer object
- if (typeof Buffer !== 'undefined' && Buffer.isBuffer(this.buffer)) {
- return asRaw ? this.buffer.slice(0, this.position) : this.buffer.toString('binary', 0, this.position);
- } else {
- if (asRaw) {
- // we support the slice command use it
- if (this.buffer['slice'] != null) {
- return this.buffer.slice(0, this.position);
- } else {
- // Create a new buffer to copy content to
- var newBuffer = Object.prototype.toString.call(this.buffer) === '[object Uint8Array]' ? new Uint8Array(new ArrayBuffer(this.position)) : new Array(this.position);
- // Copy content
- for (var i = 0; i < this.position; i++) {
- newBuffer[i] = this.buffer[i];
- }
- // Return the buffer
- return newBuffer;
- }
- } else {
- return convertArraytoUtf8BinaryString(this.buffer, 0, this.position);
- }
- }
- };
-
- /**
- * Length.
- *
- * @method
- * @return {number} the length of the binary.
- */
- Binary.prototype.length = function length() {
- return this.position;
- };
-
- /**
- * @ignore
- */
- Binary.prototype.toJSON = function () {
- return this.buffer != null ? this.buffer.toString('base64') : '';
- };
-
- /**
- * @ignore
- */
- Binary.prototype.toString = function (format) {
- return this.buffer != null ? this.buffer.slice(0, this.position).toString(format) : '';
- };
-
- /**
- * Binary default subtype
- * @ignore
- */
- var BSON_BINARY_SUBTYPE_DEFAULT = 0;
-
- /**
- * @ignore
- */
- var writeStringToArray = function (data) {
- // Create a buffer
- var buffer = typeof Uint8Array !== 'undefined' ? new Uint8Array(new ArrayBuffer(data.length)) : new Array(data.length);
- // Write the content to the buffer
- for (var i = 0; i < data.length; i++) {
- buffer[i] = data.charCodeAt(i);
- }
- // Write the string to the buffer
- return buffer;
- };
-
- /**
- * Convert Array ot Uint8Array to Binary String
- *
- * @ignore
- */
- var convertArraytoUtf8BinaryString = function (byteArray, startIndex, endIndex) {
- var result = '';
- for (var i = startIndex; i < endIndex; i++) {
- result = result + String.fromCharCode(byteArray[i]);
- }
- return result;
- };
-
- Binary.BUFFER_SIZE = 256;
-
- /**
- * Default BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
- Binary.SUBTYPE_DEFAULT = 0;
- /**
- * Function BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
- Binary.SUBTYPE_FUNCTION = 1;
- /**
- * Byte Array BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
- Binary.SUBTYPE_BYTE_ARRAY = 2;
- /**
- * OLD UUID BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
- Binary.SUBTYPE_UUID_OLD = 3;
- /**
- * UUID BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
- Binary.SUBTYPE_UUID = 4;
- /**
- * MD5 BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
- Binary.SUBTYPE_MD5 = 5;
- /**
- * User BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
- Binary.SUBTYPE_USER_DEFINED = 128;
-
- /**
- * Expose.
- */
- module.exports = Binary;
- module.exports.Binary = Binary;
- /* WEBPACK VAR INJECTION */}.call(exports, (function() { return this; }())))
-
-/***/ }),
-/* 357 */
-/***/ (function(module, exports, __webpack_require__) {
-
- 'use strict';
-
- var Long = __webpack_require__(335).Long,
- Double = __webpack_require__(336).Double,
- Timestamp = __webpack_require__(337).Timestamp,
- ObjectID = __webpack_require__(338).ObjectID,
- Symbol = __webpack_require__(349).Symbol,
- Code = __webpack_require__(351).Code,
- MinKey = __webpack_require__(353).MinKey,
- MaxKey = __webpack_require__(354).MaxKey,
- Decimal128 = __webpack_require__(352),
- Int32 = __webpack_require__(350),
- DBRef = __webpack_require__(355).DBRef,
- BSONRegExp = __webpack_require__(348).BSONRegExp,
- Binary = __webpack_require__(356).Binary;
-
- var utils = __webpack_require__(344);
-
- var deserialize = function (buffer, options, isArray) {
- options = options == null ? {} : options;
- var index = options && options.index ? options.index : 0;
- // Read the document size
- var size = buffer[index] | buffer[index + 1] << 8 | buffer[index + 2] << 16 | buffer[index + 3] << 24;
-
- // Ensure buffer is valid size
- if (size < 5 || buffer.length < size || size + index > buffer.length) {
- throw new Error('corrupt bson message');
- }
-
- // Illegal end value
- if (buffer[index + size - 1] !== 0) {
- throw new Error("One object, sized correctly, with a spot for an EOO, but the EOO isn't 0x00");
- }
-
- // Start deserializtion
- return deserializeObject(buffer, index, options, isArray);
- };
-
- var deserializeObject = function (buffer, index, options, isArray) {
- var evalFunctions = options['evalFunctions'] == null ? false : options['evalFunctions'];
- var cacheFunctions = options['cacheFunctions'] == null ? false : options['cacheFunctions'];
- var cacheFunctionsCrc32 = options['cacheFunctionsCrc32'] == null ? false : options['cacheFunctionsCrc32'];
-
- if (!cacheFunctionsCrc32) var crc32 = null;
-
- var fieldsAsRaw = options['fieldsAsRaw'] == null ? null : options['fieldsAsRaw'];
-
- // Return raw bson buffer instead of parsing it
- var raw = options['raw'] == null ? false : options['raw'];
-
- // Return BSONRegExp objects instead of native regular expressions
- var bsonRegExp = typeof options['bsonRegExp'] === 'boolean' ? options['bsonRegExp'] : false;
-
- // Controls the promotion of values vs wrapper classes
- var promoteBuffers = options['promoteBuffers'] == null ? false : options['promoteBuffers'];
- var promoteLongs = options['promoteLongs'] == null ? true : options['promoteLongs'];
- var promoteValues = options['promoteValues'] == null ? true : options['promoteValues'];
-
- // Set the start index
- var startIndex = index;
-
- // Validate that we have at least 4 bytes of buffer
- if (buffer.length < 5) throw new Error('corrupt bson message < 5 bytes long');
-
- // Read the document size
- var size = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
-
- // Ensure buffer is valid size
- if (size < 5 || size > buffer.length) throw new Error('corrupt bson message');
-
- // Create holding object
- var object = isArray ? [] : {};
- // Used for arrays to skip having to perform utf8 decoding
- var arrayIndex = 0;
-
- var done = false;
-
- // While we have more left data left keep parsing
- // while (buffer[index + 1] !== 0) {
- while (!done) {
- // Read the type
- var elementType = buffer[index++];
- // If we get a zero it's the last byte, exit
- if (elementType === 0) break;
-
- // Get the start search index
- var i = index;
- // Locate the end of the c string
- while (buffer[i] !== 0x00 && i < buffer.length) {
- i++;
- }
-
- // If are at the end of the buffer there is a problem with the document
- if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
- var name = isArray ? arrayIndex++ : buffer.toString('utf8', index, i);
-
- index = i + 1;
-
- if (elementType === BSON.BSON_DATA_STRING) {
- var stringSize = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- if (stringSize <= 0 || stringSize > buffer.length - index || buffer[index + stringSize - 1] !== 0) throw new Error('bad string length in bson');
- object[name] = buffer.toString('utf8', index, index + stringSize - 1);
- index = index + stringSize;
- } else if (elementType === BSON.BSON_DATA_OID) {
- var oid = utils.allocBuffer(12);
- buffer.copy(oid, 0, index, index + 12);
- object[name] = new ObjectID(oid);
- index = index + 12;
- } else if (elementType === BSON.BSON_DATA_INT && promoteValues === false) {
- object[name] = new Int32(buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24);
- } else if (elementType === BSON.BSON_DATA_INT) {
- object[name] = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- } else if (elementType === BSON.BSON_DATA_NUMBER && promoteValues === false) {
- object[name] = new Double(buffer.readDoubleLE(index));
- index = index + 8;
- } else if (elementType === BSON.BSON_DATA_NUMBER) {
- object[name] = buffer.readDoubleLE(index);
- index = index + 8;
- } else if (elementType === BSON.BSON_DATA_DATE) {
- var lowBits = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- var highBits = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- object[name] = new Date(new Long(lowBits, highBits).toNumber());
- } else if (elementType === BSON.BSON_DATA_BOOLEAN) {
- if (buffer[index] !== 0 && buffer[index] !== 1) throw new Error('illegal boolean type value');
- object[name] = buffer[index++] === 1;
- } else if (elementType === BSON.BSON_DATA_OBJECT) {
- var _index = index;
- var objectSize = buffer[index] | buffer[index + 1] << 8 | buffer[index + 2] << 16 | buffer[index + 3] << 24;
- if (objectSize <= 0 || objectSize > buffer.length - index) throw new Error('bad embedded document length in bson');
-
- // We have a raw value
- if (raw) {
- object[name] = buffer.slice(index, index + objectSize);
- } else {
- object[name] = deserializeObject(buffer, _index, options, false);
- }
-
- index = index + objectSize;
- } else if (elementType === BSON.BSON_DATA_ARRAY) {
- _index = index;
- objectSize = buffer[index] | buffer[index + 1] << 8 | buffer[index + 2] << 16 | buffer[index + 3] << 24;
- var arrayOptions = options;
-
- // Stop index
- var stopIndex = index + objectSize;
-
- // All elements of array to be returned as raw bson
- if (fieldsAsRaw && fieldsAsRaw[name]) {
- arrayOptions = {};
- for (var n in options) arrayOptions[n] = options[n];
- arrayOptions['raw'] = true;
- }
-
- object[name] = deserializeObject(buffer, _index, arrayOptions, true);
- index = index + objectSize;
-
- if (buffer[index - 1] !== 0) throw new Error('invalid array terminator byte');
- if (index !== stopIndex) throw new Error('corrupted array bson');
- } else if (elementType === BSON.BSON_DATA_UNDEFINED) {
- object[name] = undefined;
- } else if (elementType === BSON.BSON_DATA_NULL) {
- object[name] = null;
- } else if (elementType === BSON.BSON_DATA_LONG) {
- // Unpack the low and high bits
- lowBits = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- highBits = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- var long = new Long(lowBits, highBits);
- // Promote the long if possible
- if (promoteLongs && promoteValues === true) {
- object[name] = long.lessThanOrEqual(JS_INT_MAX_LONG) && long.greaterThanOrEqual(JS_INT_MIN_LONG) ? long.toNumber() : long;
- } else {
- object[name] = long;
- }
- } else if (elementType === BSON.BSON_DATA_DECIMAL128) {
- // Buffer to contain the decimal bytes
- var bytes = utils.allocBuffer(16);
- // Copy the next 16 bytes into the bytes buffer
- buffer.copy(bytes, 0, index, index + 16);
- // Update index
- index = index + 16;
- // Assign the new Decimal128 value
- var decimal128 = new Decimal128(bytes);
- // If we have an alternative mapper use that
- object[name] = decimal128.toObject ? decimal128.toObject() : decimal128;
- } else if (elementType === BSON.BSON_DATA_BINARY) {
- var binarySize = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- var totalBinarySize = binarySize;
- var subType = buffer[index++];
-
- // Did we have a negative binary size, throw
- if (binarySize < 0) throw new Error('Negative binary type element size found');
-
- // Is the length longer than the document
- if (binarySize > buffer.length) throw new Error('Binary type size larger than document size');
-
- // Decode as raw Buffer object if options specifies it
- if (buffer['slice'] != null) {
- // If we have subtype 2 skip the 4 bytes for the size
- if (subType === Binary.SUBTYPE_BYTE_ARRAY) {
- binarySize = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- if (binarySize < 0) throw new Error('Negative binary type element size found for subtype 0x02');
- if (binarySize > totalBinarySize - 4) throw new Error('Binary type with subtype 0x02 contains to long binary size');
- if (binarySize < totalBinarySize - 4) throw new Error('Binary type with subtype 0x02 contains to short binary size');
- }
-
- if (promoteBuffers && promoteValues) {
- object[name] = buffer.slice(index, index + binarySize);
- } else {
- object[name] = new Binary(buffer.slice(index, index + binarySize), subType);
- }
- } else {
- var _buffer = typeof Uint8Array !== 'undefined' ? new Uint8Array(new ArrayBuffer(binarySize)) : new Array(binarySize);
- // If we have subtype 2 skip the 4 bytes for the size
- if (subType === Binary.SUBTYPE_BYTE_ARRAY) {
- binarySize = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- if (binarySize < 0) throw new Error('Negative binary type element size found for subtype 0x02');
- if (binarySize > totalBinarySize - 4) throw new Error('Binary type with subtype 0x02 contains to long binary size');
- if (binarySize < totalBinarySize - 4) throw new Error('Binary type with subtype 0x02 contains to short binary size');
- }
-
- // Copy the data
- for (i = 0; i < binarySize; i++) {
- _buffer[i] = buffer[index + i];
- }
-
- if (promoteBuffers && promoteValues) {
- object[name] = _buffer;
- } else {
- object[name] = new Binary(_buffer, subType);
- }
- }
-
- // Update the index
- index = index + binarySize;
- } else if (elementType === BSON.BSON_DATA_REGEXP && bsonRegExp === false) {
- // Get the start search index
- i = index;
- // Locate the end of the c string
- while (buffer[i] !== 0x00 && i < buffer.length) {
- i++;
- }
- // If are at the end of the buffer there is a problem with the document
- if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
- // Return the C string
- var source = buffer.toString('utf8', index, i);
- // Create the regexp
- index = i + 1;
-
- // Get the start search index
- i = index;
- // Locate the end of the c string
- while (buffer[i] !== 0x00 && i < buffer.length) {
- i++;
- }
- // If are at the end of the buffer there is a problem with the document
- if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
- // Return the C string
- var regExpOptions = buffer.toString('utf8', index, i);
- index = i + 1;
-
- // For each option add the corresponding one for javascript
- var optionsArray = new Array(regExpOptions.length);
-
- // Parse options
- for (i = 0; i < regExpOptions.length; i++) {
- switch (regExpOptions[i]) {
- case 'm':
- optionsArray[i] = 'm';
- break;
- case 's':
- optionsArray[i] = 'g';
- break;
- case 'i':
- optionsArray[i] = 'i';
- break;
- }
- }
-
- object[name] = new RegExp(source, optionsArray.join(''));
- } else if (elementType === BSON.BSON_DATA_REGEXP && bsonRegExp === true) {
- // Get the start search index
- i = index;
- // Locate the end of the c string
- while (buffer[i] !== 0x00 && i < buffer.length) {
- i++;
- }
- // If are at the end of the buffer there is a problem with the document
- if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
- // Return the C string
- source = buffer.toString('utf8', index, i);
- index = i + 1;
-
- // Get the start search index
- i = index;
- // Locate the end of the c string
- while (buffer[i] !== 0x00 && i < buffer.length) {
- i++;
- }
- // If are at the end of the buffer there is a problem with the document
- if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
- // Return the C string
- regExpOptions = buffer.toString('utf8', index, i);
- index = i + 1;
-
- // Set the object
- object[name] = new BSONRegExp(source, regExpOptions);
- } else if (elementType === BSON.BSON_DATA_SYMBOL) {
- stringSize = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- if (stringSize <= 0 || stringSize > buffer.length - index || buffer[index + stringSize - 1] !== 0) throw new Error('bad string length in bson');
- object[name] = new Symbol(buffer.toString('utf8', index, index + stringSize - 1));
- index = index + stringSize;
- } else if (elementType === BSON.BSON_DATA_TIMESTAMP) {
- lowBits = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- highBits = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- object[name] = new Timestamp(lowBits, highBits);
- } else if (elementType === BSON.BSON_DATA_MIN_KEY) {
- object[name] = new MinKey();
- } else if (elementType === BSON.BSON_DATA_MAX_KEY) {
- object[name] = new MaxKey();
- } else if (elementType === BSON.BSON_DATA_CODE) {
- stringSize = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- if (stringSize <= 0 || stringSize > buffer.length - index || buffer[index + stringSize - 1] !== 0) throw new Error('bad string length in bson');
- var functionString = buffer.toString('utf8', index, index + stringSize - 1);
-
- // If we are evaluating the functions
- if (evalFunctions) {
- // If we have cache enabled let's look for the md5 of the function in the cache
- if (cacheFunctions) {
- var hash = cacheFunctionsCrc32 ? crc32(functionString) : functionString;
- // Got to do this to avoid V8 deoptimizing the call due to finding eval
- object[name] = isolateEvalWithHash(functionCache, hash, functionString, object);
- } else {
- object[name] = isolateEval(functionString);
- }
- } else {
- object[name] = new Code(functionString);
- }
-
- // Update parse index position
- index = index + stringSize;
- } else if (elementType === BSON.BSON_DATA_CODE_W_SCOPE) {
- var totalSize = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
-
- // Element cannot be shorter than totalSize + stringSize + documentSize + terminator
- if (totalSize < 4 + 4 + 4 + 1) {
- throw new Error('code_w_scope total size shorter minimum expected length');
- }
-
- // Get the code string size
- stringSize = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- // Check if we have a valid string
- if (stringSize <= 0 || stringSize > buffer.length - index || buffer[index + stringSize - 1] !== 0) throw new Error('bad string length in bson');
-
- // Javascript function
- functionString = buffer.toString('utf8', index, index + stringSize - 1);
- // Update parse index position
- index = index + stringSize;
- // Parse the element
- _index = index;
- // Decode the size of the object document
- objectSize = buffer[index] | buffer[index + 1] << 8 | buffer[index + 2] << 16 | buffer[index + 3] << 24;
- // Decode the scope object
- var scopeObject = deserializeObject(buffer, _index, options, false);
- // Adjust the index
- index = index + objectSize;
-
- // Check if field length is to short
- if (totalSize < 4 + 4 + objectSize + stringSize) {
- throw new Error('code_w_scope total size is to short, truncating scope');
- }
-
- // Check if totalSize field is to long
- if (totalSize > 4 + 4 + objectSize + stringSize) {
- throw new Error('code_w_scope total size is to long, clips outer document');
- }
-
- // If we are evaluating the functions
- if (evalFunctions) {
- // If we have cache enabled let's look for the md5 of the function in the cache
- if (cacheFunctions) {
- hash = cacheFunctionsCrc32 ? crc32(functionString) : functionString;
- // Got to do this to avoid V8 deoptimizing the call due to finding eval
- object[name] = isolateEvalWithHash(functionCache, hash, functionString, object);
- } else {
- object[name] = isolateEval(functionString);
- }
-
- object[name].scope = scopeObject;
- } else {
- object[name] = new Code(functionString, scopeObject);
- }
- } else if (elementType === BSON.BSON_DATA_DBPOINTER) {
- // Get the code string size
- stringSize = buffer[index++] | buffer[index++] << 8 | buffer[index++] << 16 | buffer[index++] << 24;
- // Check if we have a valid string
- if (stringSize <= 0 || stringSize > buffer.length - index || buffer[index + stringSize - 1] !== 0) throw new Error('bad string length in bson');
- // Namespace
- var namespace = buffer.toString('utf8', index, index + stringSize - 1);
- // Update parse index position
- index = index + stringSize;
-
- // Read the oid
- var oidBuffer = utils.allocBuffer(12);
- buffer.copy(oidBuffer, 0, index, index + 12);
- oid = new ObjectID(oidBuffer);
-
- // Update the index
- index = index + 12;
-
- // Split the namespace
- var parts = namespace.split('.');
- var db = parts.shift();
- var collection = parts.join('.');
- // Upgrade to DBRef type
- object[name] = new DBRef(collection, oid, db);
- } else {
- throw new Error('Detected unknown BSON type ' + elementType.toString(16) + ' for fieldname "' + name + '", are you using the latest BSON parser');
- }
- }
-
- // Check if the deserialization was against a valid array/object
- if (size !== index - startIndex) {
- if (isArray) throw new Error('corrupt array bson');
- throw new Error('corrupt object bson');
- }
-
- // Check if we have a db ref object
- if (object['$id'] != null) object = new DBRef(object['$ref'], object['$id'], object['$db']);
- return object;
- };
-
- /**
- * Ensure eval is isolated.
- *
- * @ignore
- * @api private
- */
- var isolateEvalWithHash = function (functionCache, hash, functionString, object) {
- // Contains the value we are going to set
- var value = null;
-
- // Check for cache hit, eval if missing and return cached function
- if (functionCache[hash] == null) {
- eval('value = ' + functionString);
- functionCache[hash] = value;
- }
- // Set the object
- return functionCache[hash].bind(object);
- };
-
- /**
- * Ensure eval is isolated.
- *
- * @ignore
- * @api private
- */
- var isolateEval = function (functionString) {
- // Contains the value we are going to set
- var value = null;
- // Eval the function
- eval('value = ' + functionString);
- return value;
- };
-
- var BSON = {};
-
- /**
- * Contains the function cache if we have that enable to allow for avoiding the eval step on each deserialization, comparison is by md5
- *
- * @ignore
- * @api private
- */
- var functionCache = BSON.functionCache = {};
-
- /**
- * Number BSON Type
- *
- * @classconstant BSON_DATA_NUMBER
- **/
- BSON.BSON_DATA_NUMBER = 1;
- /**
- * String BSON Type
- *
- * @classconstant BSON_DATA_STRING
- **/
- BSON.BSON_DATA_STRING = 2;
- /**
- * Object BSON Type
- *
- * @classconstant BSON_DATA_OBJECT
- **/
- BSON.BSON_DATA_OBJECT = 3;
- /**
- * Array BSON Type
- *
- * @classconstant BSON_DATA_ARRAY
- **/
- BSON.BSON_DATA_ARRAY = 4;
- /**
- * Binary BSON Type
- *
- * @classconstant BSON_DATA_BINARY
- **/
- BSON.BSON_DATA_BINARY = 5;
- /**
- * Binary BSON Type
- *
- * @classconstant BSON_DATA_UNDEFINED
- **/
- BSON.BSON_DATA_UNDEFINED = 6;
- /**
- * ObjectID BSON Type
- *
- * @classconstant BSON_DATA_OID
- **/
- BSON.BSON_DATA_OID = 7;
- /**
- * Boolean BSON Type
- *
- * @classconstant BSON_DATA_BOOLEAN
- **/
- BSON.BSON_DATA_BOOLEAN = 8;
- /**
- * Date BSON Type
- *
- * @classconstant BSON_DATA_DATE
- **/
- BSON.BSON_DATA_DATE = 9;
- /**
- * null BSON Type
- *
- * @classconstant BSON_DATA_NULL
- **/
- BSON.BSON_DATA_NULL = 10;
- /**
- * RegExp BSON Type
- *
- * @classconstant BSON_DATA_REGEXP
- **/
- BSON.BSON_DATA_REGEXP = 11;
- /**
- * Code BSON Type
- *
- * @classconstant BSON_DATA_DBPOINTER
- **/
- BSON.BSON_DATA_DBPOINTER = 12;
- /**
- * Code BSON Type
- *
- * @classconstant BSON_DATA_CODE
- **/
- BSON.BSON_DATA_CODE = 13;
- /**
- * Symbol BSON Type
- *
- * @classconstant BSON_DATA_SYMBOL
- **/
- BSON.BSON_DATA_SYMBOL = 14;
- /**
- * Code with Scope BSON Type
- *
- * @classconstant BSON_DATA_CODE_W_SCOPE
- **/
- BSON.BSON_DATA_CODE_W_SCOPE = 15;
- /**
- * 32 bit Integer BSON Type
- *
- * @classconstant BSON_DATA_INT
- **/
- BSON.BSON_DATA_INT = 16;
- /**
- * Timestamp BSON Type
- *
- * @classconstant BSON_DATA_TIMESTAMP
- **/
- BSON.BSON_DATA_TIMESTAMP = 17;
- /**
- * Long BSON Type
- *
- * @classconstant BSON_DATA_LONG
- **/
- BSON.BSON_DATA_LONG = 18;
- /**
- * Long BSON Type
- *
- * @classconstant BSON_DATA_DECIMAL128
- **/
- BSON.BSON_DATA_DECIMAL128 = 19;
- /**
- * MinKey BSON Type
- *
- * @classconstant BSON_DATA_MIN_KEY
- **/
- BSON.BSON_DATA_MIN_KEY = 0xff;
- /**
- * MaxKey BSON Type
- *
- * @classconstant BSON_DATA_MAX_KEY
- **/
- BSON.BSON_DATA_MAX_KEY = 0x7f;
-
- /**
- * Binary Default Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_DEFAULT
- **/
- BSON.BSON_BINARY_SUBTYPE_DEFAULT = 0;
- /**
- * Binary Function Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_FUNCTION
- **/
- BSON.BSON_BINARY_SUBTYPE_FUNCTION = 1;
- /**
- * Binary Byte Array Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_BYTE_ARRAY
- **/
- BSON.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
- /**
- * Binary UUID Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_UUID
- **/
- BSON.BSON_BINARY_SUBTYPE_UUID = 3;
- /**
- * Binary MD5 Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_MD5
- **/
- BSON.BSON_BINARY_SUBTYPE_MD5 = 4;
- /**
- * Binary User Defined Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_USER_DEFINED
- **/
- BSON.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
-
- // BSON MAX VALUES
- BSON.BSON_INT32_MAX = 0x7fffffff;
- BSON.BSON_INT32_MIN = -0x80000000;
-
- BSON.BSON_INT64_MAX = Math.pow(2, 63) - 1;
- BSON.BSON_INT64_MIN = -Math.pow(2, 63);
-
- // JS MAX PRECISE VALUES
- BSON.JS_INT_MAX = 0x20000000000000; // Any integer up to 2^53 can be precisely represented by a double.
- BSON.JS_INT_MIN = -0x20000000000000; // Any integer down to -2^53 can be precisely represented by a double.
-
- // Internal long versions
- var JS_INT_MAX_LONG = Long.fromNumber(0x20000000000000); // Any integer up to 2^53 can be precisely represented by a double.
- var JS_INT_MIN_LONG = Long.fromNumber(-0x20000000000000); // Any integer down to -2^53 can be precisely represented by a double.
-
- module.exports = deserialize;
-
-/***/ }),
-/* 358 */
-/***/ (function(module, exports, __webpack_require__) {
-
- /* WEBPACK VAR INJECTION */(function(Buffer) {'use strict';
-
- var writeIEEE754 = __webpack_require__(359).writeIEEE754,
- Long = __webpack_require__(335).Long,
- Map = __webpack_require__(334),
- Binary = __webpack_require__(356).Binary;
-
- var normalizedFunctionString = __webpack_require__(344).normalizedFunctionString;
-
- // try {
- // var _Buffer = Uint8Array;
- // } catch (e) {
- // _Buffer = Buffer;
- // }
-
- var regexp = /\x00/; // eslint-disable-line no-control-regex
- var ignoreKeys = ['$db', '$ref', '$id', '$clusterTime'];
-
- // To ensure that 0.4 of node works correctly
- var isDate = function isDate(d) {
- return typeof d === 'object' && Object.prototype.toString.call(d) === '[object Date]';
- };
-
- var isRegExp = function isRegExp(d) {
- return Object.prototype.toString.call(d) === '[object RegExp]';
- };
-
- var serializeString = function (buffer, key, value, index, isArray) {
- // Encode String type
- buffer[index++] = BSON.BSON_DATA_STRING;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes + 1;
- buffer[index - 1] = 0;
- // Write the string
- var size = buffer.write(value, index + 4, 'utf8');
- // Write the size of the string to buffer
- buffer[index + 3] = size + 1 >> 24 & 0xff;
- buffer[index + 2] = size + 1 >> 16 & 0xff;
- buffer[index + 1] = size + 1 >> 8 & 0xff;
- buffer[index] = size + 1 & 0xff;
- // Update index
- index = index + 4 + size;
- // Write zero
- buffer[index++] = 0;
- return index;
- };
-
- var serializeNumber = function (buffer, key, value, index, isArray) {
- // We have an integer value
- if (Math.floor(value) === value && value >= BSON.JS_INT_MIN && value <= BSON.JS_INT_MAX) {
- // If the value fits in 32 bits encode as int, if it fits in a double
- // encode it as a double, otherwise long
- if (value >= BSON.BSON_INT32_MIN && value <= BSON.BSON_INT32_MAX) {
- // Set int type 32 bits or less
- buffer[index++] = BSON.BSON_DATA_INT;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write the int value
- buffer[index++] = value & 0xff;
- buffer[index++] = value >> 8 & 0xff;
- buffer[index++] = value >> 16 & 0xff;
- buffer[index++] = value >> 24 & 0xff;
- } else if (value >= BSON.JS_INT_MIN && value <= BSON.JS_INT_MAX) {
- // Encode as double
- buffer[index++] = BSON.BSON_DATA_NUMBER;
- // Number of written bytes
- numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write float
- writeIEEE754(buffer, value, index, 'little', 52, 8);
- // Ajust index
- index = index + 8;
- } else {
- // Set long type
- buffer[index++] = BSON.BSON_DATA_LONG;
- // Number of written bytes
- numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- var longVal = Long.fromNumber(value);
- var lowBits = longVal.getLowBits();
- var highBits = longVal.getHighBits();
- // Encode low bits
- buffer[index++] = lowBits & 0xff;
- buffer[index++] = lowBits >> 8 & 0xff;
- buffer[index++] = lowBits >> 16 & 0xff;
- buffer[index++] = lowBits >> 24 & 0xff;
- // Encode high bits
- buffer[index++] = highBits & 0xff;
- buffer[index++] = highBits >> 8 & 0xff;
- buffer[index++] = highBits >> 16 & 0xff;
- buffer[index++] = highBits >> 24 & 0xff;
- }
- } else {
- // Encode as double
- buffer[index++] = BSON.BSON_DATA_NUMBER;
- // Number of written bytes
- numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write float
- writeIEEE754(buffer, value, index, 'little', 52, 8);
- // Ajust index
- index = index + 8;
- }
-
- return index;
- };
-
- var serializeNull = function (buffer, key, value, index, isArray) {
- // Set long type
- buffer[index++] = BSON.BSON_DATA_NULL;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- return index;
- };
-
- var serializeBoolean = function (buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_BOOLEAN;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Encode the boolean value
- buffer[index++] = value ? 1 : 0;
- return index;
- };
-
- var serializeDate = function (buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_DATE;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
-
- // Write the date
- var dateInMilis = Long.fromNumber(value.getTime());
- var lowBits = dateInMilis.getLowBits();
- var highBits = dateInMilis.getHighBits();
- // Encode low bits
- buffer[index++] = lowBits & 0xff;
- buffer[index++] = lowBits >> 8 & 0xff;
- buffer[index++] = lowBits >> 16 & 0xff;
- buffer[index++] = lowBits >> 24 & 0xff;
- // Encode high bits
- buffer[index++] = highBits & 0xff;
- buffer[index++] = highBits >> 8 & 0xff;
- buffer[index++] = highBits >> 16 & 0xff;
- buffer[index++] = highBits >> 24 & 0xff;
- return index;
- };
-
- var serializeRegExp = function (buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_REGEXP;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- if (value.source && value.source.match(regexp) != null) {
- throw Error('value ' + value.source + ' must not contain null bytes');
- }
- // Adjust the index
- index = index + buffer.write(value.source, index, 'utf8');
- // Write zero
- buffer[index++] = 0x00;
- // Write the parameters
- if (value.global) buffer[index++] = 0x73; // s
- if (value.ignoreCase) buffer[index++] = 0x69; // i
- if (value.multiline) buffer[index++] = 0x6d; // m
- // Add ending zero
- buffer[index++] = 0x00;
- return index;
- };
-
- var serializeBSONRegExp = function (buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_REGEXP;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
-
- // Check the pattern for 0 bytes
- if (value.pattern.match(regexp) != null) {
- // The BSON spec doesn't allow keys with null bytes because keys are
- // null-terminated.
- throw Error('pattern ' + value.pattern + ' must not contain null bytes');
- }
-
- // Adjust the index
- index = index + buffer.write(value.pattern, index, 'utf8');
- // Write zero
- buffer[index++] = 0x00;
- // Write the options
- index = index + buffer.write(value.options.split('').sort().join(''), index, 'utf8');
- // Add ending zero
- buffer[index++] = 0x00;
- return index;
- };
-
- var serializeMinMax = function (buffer, key, value, index, isArray) {
- // Write the type of either min or max key
- if (value === null) {
- buffer[index++] = BSON.BSON_DATA_NULL;
- } else if (value._bsontype === 'MinKey') {
- buffer[index++] = BSON.BSON_DATA_MIN_KEY;
- } else {
- buffer[index++] = BSON.BSON_DATA_MAX_KEY;
- }
-
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- return index;
- };
-
- var serializeObjectId = function (buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_OID;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
-
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
-
- // Write the objectId into the shared buffer
- if (typeof value.id === 'string') {
- buffer.write(value.id, index, 'binary');
- } else if (value.id && value.id.copy) {
- value.id.copy(buffer, index, 0, 12);
- } else {
- throw new Error('object [' + JSON.stringify(value) + '] is not a valid ObjectId');
- }
-
- // Ajust index
- return index + 12;
- };
-
- var serializeBuffer = function (buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_BINARY;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Get size of the buffer (current write point)
- var size = value.length;
- // Write the size of the string to buffer
- buffer[index++] = size & 0xff;
- buffer[index++] = size >> 8 & 0xff;
- buffer[index++] = size >> 16 & 0xff;
- buffer[index++] = size >> 24 & 0xff;
- // Write the default subtype
- buffer[index++] = BSON.BSON_BINARY_SUBTYPE_DEFAULT;
- // Copy the content form the binary field to the buffer
- value.copy(buffer, index, 0, size);
- // Adjust the index
- index = index + size;
- return index;
- };
-
- var serializeObject = function (buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray, path) {
- for (var i = 0; i < path.length; i++) {
- if (path[i] === value) throw new Error('cyclic dependency detected');
- }
-
- // Push value to stack
- path.push(value);
- // Write the type
- buffer[index++] = Array.isArray(value) ? BSON.BSON_DATA_ARRAY : BSON.BSON_DATA_OBJECT;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- var endIndex = serializeInto(buffer, value, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined, path);
- // Pop stack
- path.pop();
- // Write size
- return endIndex;
- };
-
- var serializeDecimal128 = function (buffer, key, value, index, isArray) {
- buffer[index++] = BSON.BSON_DATA_DECIMAL128;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write the data from the value
- value.bytes.copy(buffer, index, 0, 16);
- return index + 16;
- };
-
- var serializeLong = function (buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = value._bsontype === 'Long' ? BSON.BSON_DATA_LONG : BSON.BSON_DATA_TIMESTAMP;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write the date
- var lowBits = value.getLowBits();
- var highBits = value.getHighBits();
- // Encode low bits
- buffer[index++] = lowBits & 0xff;
- buffer[index++] = lowBits >> 8 & 0xff;
- buffer[index++] = lowBits >> 16 & 0xff;
- buffer[index++] = lowBits >> 24 & 0xff;
- // Encode high bits
- buffer[index++] = highBits & 0xff;
- buffer[index++] = highBits >> 8 & 0xff;
- buffer[index++] = highBits >> 16 & 0xff;
- buffer[index++] = highBits >> 24 & 0xff;
- return index;
- };
-
- var serializeInt32 = function (buffer, key, value, index, isArray) {
- // Set int type 32 bits or less
- buffer[index++] = BSON.BSON_DATA_INT;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write the int value
- buffer[index++] = value & 0xff;
- buffer[index++] = value >> 8 & 0xff;
- buffer[index++] = value >> 16 & 0xff;
- buffer[index++] = value >> 24 & 0xff;
- return index;
- };
-
- var serializeDouble = function (buffer, key, value, index, isArray) {
- // Encode as double
- buffer[index++] = BSON.BSON_DATA_NUMBER;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write float
- writeIEEE754(buffer, value, index, 'little', 52, 8);
- // Ajust index
- index = index + 8;
- return index;
- };
-
- var serializeFunction = function (buffer, key, value, index, checkKeys, depth, isArray) {
- buffer[index++] = BSON.BSON_DATA_CODE;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Function string
- var functionString = normalizedFunctionString(value);
-
- // Write the string
- var size = buffer.write(functionString, index + 4, 'utf8') + 1;
- // Write the size of the string to buffer
- buffer[index] = size & 0xff;
- buffer[index + 1] = size >> 8 & 0xff;
- buffer[index + 2] = size >> 16 & 0xff;
- buffer[index + 3] = size >> 24 & 0xff;
- // Update index
- index = index + 4 + size - 1;
- // Write zero
- buffer[index++] = 0;
- return index;
- };
-
- var serializeCode = function (buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray) {
- if (value.scope && typeof value.scope === 'object') {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_CODE_W_SCOPE;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
-
- // Starting index
- var startIndex = index;
-
- // Serialize the function
- // Get the function string
- var functionString = typeof value.code === 'string' ? value.code : value.code.toString();
- // Index adjustment
- index = index + 4;
- // Write string into buffer
- var codeSize = buffer.write(functionString, index + 4, 'utf8') + 1;
- // Write the size of the string to buffer
- buffer[index] = codeSize & 0xff;
- buffer[index + 1] = codeSize >> 8 & 0xff;
- buffer[index + 2] = codeSize >> 16 & 0xff;
- buffer[index + 3] = codeSize >> 24 & 0xff;
- // Write end 0
- buffer[index + 4 + codeSize - 1] = 0;
- // Write the
- index = index + codeSize + 4;
-
- //
- // Serialize the scope value
- var endIndex = serializeInto(buffer, value.scope, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined);
- index = endIndex - 1;
-
- // Writ the total
- var totalSize = endIndex - startIndex;
-
- // Write the total size of the object
- buffer[startIndex++] = totalSize & 0xff;
- buffer[startIndex++] = totalSize >> 8 & 0xff;
- buffer[startIndex++] = totalSize >> 16 & 0xff;
- buffer[startIndex++] = totalSize >> 24 & 0xff;
- // Write trailing zero
- buffer[index++] = 0;
- } else {
- buffer[index++] = BSON.BSON_DATA_CODE;
- // Number of written bytes
- numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Function string
- functionString = value.code.toString();
- // Write the string
- var size = buffer.write(functionString, index + 4, 'utf8') + 1;
- // Write the size of the string to buffer
- buffer[index] = size & 0xff;
- buffer[index + 1] = size >> 8 & 0xff;
- buffer[index + 2] = size >> 16 & 0xff;
- buffer[index + 3] = size >> 24 & 0xff;
- // Update index
- index = index + 4 + size - 1;
- // Write zero
- buffer[index++] = 0;
- }
-
- return index;
- };
-
- var serializeBinary = function (buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_BINARY;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Extract the buffer
- var data = value.value(true);
- // Calculate size
- var size = value.position;
- // Add the deprecated 02 type 4 bytes of size to total
- if (value.sub_type === Binary.SUBTYPE_BYTE_ARRAY) size = size + 4;
- // Write the size of the string to buffer
- buffer[index++] = size & 0xff;
- buffer[index++] = size >> 8 & 0xff;
- buffer[index++] = size >> 16 & 0xff;
- buffer[index++] = size >> 24 & 0xff;
- // Write the subtype to the buffer
- buffer[index++] = value.sub_type;
-
- // If we have binary type 2 the 4 first bytes are the size
- if (value.sub_type === Binary.SUBTYPE_BYTE_ARRAY) {
- size = size - 4;
- buffer[index++] = size & 0xff;
- buffer[index++] = size >> 8 & 0xff;
- buffer[index++] = size >> 16 & 0xff;
- buffer[index++] = size >> 24 & 0xff;
- }
-
- // Write the data to the object
- data.copy(buffer, index, 0, value.position);
- // Adjust the index
- index = index + value.position;
- return index;
- };
-
- var serializeSymbol = function (buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_SYMBOL;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write the string
- var size = buffer.write(value.value, index + 4, 'utf8') + 1;
- // Write the size of the string to buffer
- buffer[index] = size & 0xff;
- buffer[index + 1] = size >> 8 & 0xff;
- buffer[index + 2] = size >> 16 & 0xff;
- buffer[index + 3] = size >> 24 & 0xff;
- // Update index
- index = index + 4 + size - 1;
- // Write zero
- buffer[index++] = 0x00;
- return index;
- };
-
- var serializeDBRef = function (buffer, key, value, index, depth, serializeFunctions, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_OBJECT;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray ? buffer.write(key, index, 'utf8') : buffer.write(key, index, 'ascii');
-
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
-
- var startIndex = index;
- var endIndex;
-
- // Serialize object
- if (null != value.db) {
- endIndex = serializeInto(buffer, {
- $ref: value.namespace,
- $id: value.oid,
- $db: value.db
- }, false, index, depth + 1, serializeFunctions);
- } else {
- endIndex = serializeInto(buffer, {
- $ref: value.namespace,
- $id: value.oid
- }, false, index, depth + 1, serializeFunctions);
- }
-
- // Calculate object size
- var size = endIndex - startIndex;
- // Write the size
- buffer[startIndex++] = size & 0xff;
- buffer[startIndex++] = size >> 8 & 0xff;
- buffer[startIndex++] = size >> 16 & 0xff;
- buffer[startIndex++] = size >> 24 & 0xff;
- // Set index
- return endIndex;
- };
-
- var serializeInto = function serializeInto(buffer, object, checkKeys, startingIndex, depth, serializeFunctions, ignoreUndefined, path) {
- startingIndex = startingIndex || 0;
- path = path || [];
-
- // Push the object to the path
- path.push(object);
-
- // Start place to serialize into
- var index = startingIndex + 4;
- // var self = this;
-
- // Special case isArray
- if (Array.isArray(object)) {
- // Get object keys
- for (var i = 0; i < object.length; i++) {
- var key = '' + i;
- var value = object[i];
-
- // Is there an override value
- if (value && value.toBSON) {
- if (typeof value.toBSON !== 'function') throw new Error('toBSON is not a function');
- value = value.toBSON();
- }
-
- var type = typeof value;
- if (type === 'string') {
- index = serializeString(buffer, key, value, index, true);
- } else if (type === 'number') {
- index = serializeNumber(buffer, key, value, index, true);
- } else if (type === 'boolean') {
- index = serializeBoolean(buffer, key, value, index, true);
- } else if (value instanceof Date || isDate(value)) {
- index = serializeDate(buffer, key, value, index, true);
- } else if (value === undefined) {
- index = serializeNull(buffer, key, value, index, true);
- } else if (value === null) {
- index = serializeNull(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'ObjectID' || value['_bsontype'] === 'ObjectId') {
- index = serializeObjectId(buffer, key, value, index, true);
- } else if (Buffer.isBuffer(value)) {
- index = serializeBuffer(buffer, key, value, index, true);
- } else if (value instanceof RegExp || isRegExp(value)) {
- index = serializeRegExp(buffer, key, value, index, true);
- } else if (type === 'object' && value['_bsontype'] == null) {
- index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true, path);
- } else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
- index = serializeDecimal128(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
- index = serializeLong(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'Double') {
- index = serializeDouble(buffer, key, value, index, true);
- } else if (typeof value === 'function' && serializeFunctions) {
- index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions, true);
- } else if (value['_bsontype'] === 'Code') {
- index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true);
- } else if (value['_bsontype'] === 'Binary') {
- index = serializeBinary(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'Symbol') {
- index = serializeSymbol(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'DBRef') {
- index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions, true);
- } else if (value['_bsontype'] === 'BSONRegExp') {
- index = serializeBSONRegExp(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'Int32') {
- index = serializeInt32(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
- index = serializeMinMax(buffer, key, value, index, true);
- } else if (typeof value['_bsontype'] !== 'undefined') {
- throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
- }
- }
- } else if (object instanceof Map) {
- var iterator = object.entries();
- var done = false;
-
- while (!done) {
- // Unpack the next entry
- var entry = iterator.next();
- done = entry.done;
- // Are we done, then skip and terminate
- if (done) continue;
-
- // Get the entry values
- key = entry.value[0];
- value = entry.value[1];
-
- // Check the type of the value
- type = typeof value;
-
- // Check the key and throw error if it's illegal
- if (typeof key === 'string' && ignoreKeys.indexOf(key) === -1) {
- if (key.match(regexp) != null) {
- // The BSON spec doesn't allow keys with null bytes because keys are
- // null-terminated.
- throw Error('key ' + key + ' must not contain null bytes');
- }
-
- if (checkKeys) {
- if ('$' === key[0]) {
- throw Error('key ' + key + " must not start with '$'");
- } else if (~key.indexOf('.')) {
- throw Error('key ' + key + " must not contain '.'");
- }
- }
- }
-
- if (type === 'string') {
- index = serializeString(buffer, key, value, index);
- } else if (type === 'number') {
- index = serializeNumber(buffer, key, value, index);
- } else if (type === 'boolean') {
- index = serializeBoolean(buffer, key, value, index);
- } else if (value instanceof Date || isDate(value)) {
- index = serializeDate(buffer, key, value, index);
- // } else if (value === undefined && ignoreUndefined === true) {
- } else if (value === null || value === undefined && ignoreUndefined === false) {
- index = serializeNull(buffer, key, value, index);
- } else if (value['_bsontype'] === 'ObjectID' || value['_bsontype'] === 'ObjectId') {
- index = serializeObjectId(buffer, key, value, index);
- } else if (Buffer.isBuffer(value)) {
- index = serializeBuffer(buffer, key, value, index);
- } else if (value instanceof RegExp || isRegExp(value)) {
- index = serializeRegExp(buffer, key, value, index);
- } else if (type === 'object' && value['_bsontype'] == null) {
- index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
- } else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
- index = serializeDecimal128(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
- index = serializeLong(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Double') {
- index = serializeDouble(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Code') {
- index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
- } else if (typeof value === 'function' && serializeFunctions) {
- index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
- } else if (value['_bsontype'] === 'Binary') {
- index = serializeBinary(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Symbol') {
- index = serializeSymbol(buffer, key, value, index);
- } else if (value['_bsontype'] === 'DBRef') {
- index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
- } else if (value['_bsontype'] === 'BSONRegExp') {
- index = serializeBSONRegExp(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Int32') {
- index = serializeInt32(buffer, key, value, index);
- } else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
- index = serializeMinMax(buffer, key, value, index);
- } else if (typeof value['_bsontype'] !== 'undefined') {
- throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
- }
- }
- } else {
- // Did we provide a custom serialization method
- if (object.toBSON) {
- if (typeof object.toBSON !== 'function') throw new Error('toBSON is not a function');
- object = object.toBSON();
- if (object != null && typeof object !== 'object') throw new Error('toBSON function did not return an object');
- }
-
- // Iterate over all the keys
- for (key in object) {
- value = object[key];
- // Is there an override value
- if (value && value.toBSON) {
- if (typeof value.toBSON !== 'function') throw new Error('toBSON is not a function');
- value = value.toBSON();
- }
-
- // Check the type of the value
- type = typeof value;
-
- // Check the key and throw error if it's illegal
- if (typeof key === 'string' && ignoreKeys.indexOf(key) === -1) {
- if (key.match(regexp) != null) {
- // The BSON spec doesn't allow keys with null bytes because keys are
- // null-terminated.
- throw Error('key ' + key + ' must not contain null bytes');
- }
-
- if (checkKeys) {
- if ('$' === key[0]) {
- throw Error('key ' + key + " must not start with '$'");
- } else if (~key.indexOf('.')) {
- throw Error('key ' + key + " must not contain '.'");
- }
- }
- }
-
- if (type === 'string') {
- index = serializeString(buffer, key, value, index);
- } else if (type === 'number') {
- index = serializeNumber(buffer, key, value, index);
- } else if (type === 'boolean') {
- index = serializeBoolean(buffer, key, value, index);
- } else if (value instanceof Date || isDate(value)) {
- index = serializeDate(buffer, key, value, index);
- } else if (value === undefined) {
- if (ignoreUndefined === false) index = serializeNull(buffer, key, value, index);
- } else if (value === null) {
- index = serializeNull(buffer, key, value, index);
- } else if (value['_bsontype'] === 'ObjectID' || value['_bsontype'] === 'ObjectId') {
- index = serializeObjectId(buffer, key, value, index);
- } else if (Buffer.isBuffer(value)) {
- index = serializeBuffer(buffer, key, value, index);
- } else if (value instanceof RegExp || isRegExp(value)) {
- index = serializeRegExp(buffer, key, value, index);
- } else if (type === 'object' && value['_bsontype'] == null) {
- index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
- } else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
- index = serializeDecimal128(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
- index = serializeLong(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Double') {
- index = serializeDouble(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Code') {
- index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
- } else if (typeof value === 'function' && serializeFunctions) {
- index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
- } else if (value['_bsontype'] === 'Binary') {
- index = serializeBinary(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Symbol') {
- index = serializeSymbol(buffer, key, value, index);
- } else if (value['_bsontype'] === 'DBRef') {
- index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
- } else if (value['_bsontype'] === 'BSONRegExp') {
- index = serializeBSONRegExp(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Int32') {
- index = serializeInt32(buffer, key, value, index);
- } else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
- index = serializeMinMax(buffer, key, value, index);
- } else if (typeof value['_bsontype'] !== 'undefined') {
- throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
- }
- }
- }
-
- // Remove the path
- path.pop();
-
- // Final padding byte for object
- buffer[index++] = 0x00;
-
- // Final size
- var size = index - startingIndex;
- // Write the size of the object
- buffer[startingIndex++] = size & 0xff;
- buffer[startingIndex++] = size >> 8 & 0xff;
- buffer[startingIndex++] = size >> 16 & 0xff;
- buffer[startingIndex++] = size >> 24 & 0xff;
- return index;
- };
-
- var BSON = {};
-
- /**
- * Contains the function cache if we have that enable to allow for avoiding the eval step on each deserialization, comparison is by md5
- *
- * @ignore
- * @api private
- */
- // var functionCache = (BSON.functionCache = {});
-
- /**
- * Number BSON Type
- *
- * @classconstant BSON_DATA_NUMBER
- **/
- BSON.BSON_DATA_NUMBER = 1;
- /**
- * String BSON Type
- *
- * @classconstant BSON_DATA_STRING
- **/
- BSON.BSON_DATA_STRING = 2;
- /**
- * Object BSON Type
- *
- * @classconstant BSON_DATA_OBJECT
- **/
- BSON.BSON_DATA_OBJECT = 3;
- /**
- * Array BSON Type
- *
- * @classconstant BSON_DATA_ARRAY
- **/
- BSON.BSON_DATA_ARRAY = 4;
- /**
- * Binary BSON Type
- *
- * @classconstant BSON_DATA_BINARY
- **/
- BSON.BSON_DATA_BINARY = 5;
- /**
- * ObjectID BSON Type, deprecated
- *
- * @classconstant BSON_DATA_UNDEFINED
- **/
- BSON.BSON_DATA_UNDEFINED = 6;
- /**
- * ObjectID BSON Type
- *
- * @classconstant BSON_DATA_OID
- **/
- BSON.BSON_DATA_OID = 7;
- /**
- * Boolean BSON Type
- *
- * @classconstant BSON_DATA_BOOLEAN
- **/
- BSON.BSON_DATA_BOOLEAN = 8;
- /**
- * Date BSON Type
- *
- * @classconstant BSON_DATA_DATE
- **/
- BSON.BSON_DATA_DATE = 9;
- /**
- * null BSON Type
- *
- * @classconstant BSON_DATA_NULL
- **/
- BSON.BSON_DATA_NULL = 10;
- /**
- * RegExp BSON Type
- *
- * @classconstant BSON_DATA_REGEXP
- **/
- BSON.BSON_DATA_REGEXP = 11;
- /**
- * Code BSON Type
- *
- * @classconstant BSON_DATA_CODE
- **/
- BSON.BSON_DATA_CODE = 13;
- /**
- * Symbol BSON Type
- *
- * @classconstant BSON_DATA_SYMBOL
- **/
- BSON.BSON_DATA_SYMBOL = 14;
- /**
- * Code with Scope BSON Type
- *
- * @classconstant BSON_DATA_CODE_W_SCOPE
- **/
- BSON.BSON_DATA_CODE_W_SCOPE = 15;
- /**
- * 32 bit Integer BSON Type
- *
- * @classconstant BSON_DATA_INT
- **/
- BSON.BSON_DATA_INT = 16;
- /**
- * Timestamp BSON Type
- *
- * @classconstant BSON_DATA_TIMESTAMP
- **/
- BSON.BSON_DATA_TIMESTAMP = 17;
- /**
- * Long BSON Type
- *
- * @classconstant BSON_DATA_LONG
- **/
- BSON.BSON_DATA_LONG = 18;
- /**
- * Long BSON Type
- *
- * @classconstant BSON_DATA_DECIMAL128
- **/
- BSON.BSON_DATA_DECIMAL128 = 19;
- /**
- * MinKey BSON Type
- *
- * @classconstant BSON_DATA_MIN_KEY
- **/
- BSON.BSON_DATA_MIN_KEY = 0xff;
- /**
- * MaxKey BSON Type
- *
- * @classconstant BSON_DATA_MAX_KEY
- **/
- BSON.BSON_DATA_MAX_KEY = 0x7f;
- /**
- * Binary Default Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_DEFAULT
- **/
- BSON.BSON_BINARY_SUBTYPE_DEFAULT = 0;
- /**
- * Binary Function Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_FUNCTION
- **/
- BSON.BSON_BINARY_SUBTYPE_FUNCTION = 1;
- /**
- * Binary Byte Array Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_BYTE_ARRAY
- **/
- BSON.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
- /**
- * Binary UUID Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_UUID
- **/
- BSON.BSON_BINARY_SUBTYPE_UUID = 3;
- /**
- * Binary MD5 Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_MD5
- **/
- BSON.BSON_BINARY_SUBTYPE_MD5 = 4;
- /**
- * Binary User Defined Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_USER_DEFINED
- **/
- BSON.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
-
- // BSON MAX VALUES
- BSON.BSON_INT32_MAX = 0x7fffffff;
- BSON.BSON_INT32_MIN = -0x80000000;
-
- BSON.BSON_INT64_MAX = Math.pow(2, 63) - 1;
- BSON.BSON_INT64_MIN = -Math.pow(2, 63);
-
- // JS MAX PRECISE VALUES
- BSON.JS_INT_MAX = 0x20000000000000; // Any integer up to 2^53 can be precisely represented by a double.
- BSON.JS_INT_MIN = -0x20000000000000; // Any integer down to -2^53 can be precisely represented by a double.
-
- // Internal long versions
- // var JS_INT_MAX_LONG = Long.fromNumber(0x20000000000000); // Any integer up to 2^53 can be precisely represented by a double.
- // var JS_INT_MIN_LONG = Long.fromNumber(-0x20000000000000); // Any integer down to -2^53 can be precisely represented by a double.
-
- module.exports = serializeInto;
- /* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(339).Buffer))
-
-/***/ }),
-/* 359 */
-/***/ (function(module, exports) {
-
- // Copyright (c) 2008, Fair Oaks Labs, Inc.
- // All rights reserved.
- //
- // Redistribution and use in source and binary forms, with or without
- // modification, are permitted provided that the following conditions are met:
- //
- // * Redistributions of source code must retain the above copyright notice,
- // this list of conditions and the following disclaimer.
- //
- // * Redistributions in binary form must reproduce the above copyright notice,
- // this list of conditions and the following disclaimer in the documentation
- // and/or other materials provided with the distribution.
- //
- // * Neither the name of Fair Oaks Labs, Inc. nor the names of its contributors
- // may be used to endorse or promote products derived from this software
- // without specific prior written permission.
- //
- // THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
- // AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
- // IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
- // ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
- // LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
- // CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
- // SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
- // INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
- // CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
- // ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
- // POSSIBILITY OF SUCH DAMAGE.
- //
- //
- // Modifications to writeIEEE754 to support negative zeroes made by Brian White
-
- var readIEEE754 = function (buffer, offset, endian, mLen, nBytes) {
- var e,
- m,
- bBE = endian === 'big',
- eLen = nBytes * 8 - mLen - 1,
- eMax = (1 << eLen) - 1,
- eBias = eMax >> 1,
- nBits = -7,
- i = bBE ? 0 : nBytes - 1,
- d = bBE ? 1 : -1,
- s = buffer[offset + i];
-
- i += d;
-
- e = s & (1 << -nBits) - 1;
- s >>= -nBits;
- nBits += eLen;
- for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8);
-
- m = e & (1 << -nBits) - 1;
- e >>= -nBits;
- nBits += mLen;
- for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8);
-
- if (e === 0) {
- e = 1 - eBias;
- } else if (e === eMax) {
- return m ? NaN : (s ? -1 : 1) * Infinity;
- } else {
- m = m + Math.pow(2, mLen);
- e = e - eBias;
- }
- return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
- };
-
- var writeIEEE754 = function (buffer, value, offset, endian, mLen, nBytes) {
- var e,
- m,
- c,
- bBE = endian === 'big',
- eLen = nBytes * 8 - mLen - 1,
- eMax = (1 << eLen) - 1,
- eBias = eMax >> 1,
- rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0,
- i = bBE ? nBytes - 1 : 0,
- d = bBE ? -1 : 1,
- s = value < 0 || value === 0 && 1 / value < 0 ? 1 : 0;
-
- value = Math.abs(value);
-
- if (isNaN(value) || value === Infinity) {
- m = isNaN(value) ? 1 : 0;
- e = eMax;
- } else {
- e = Math.floor(Math.log(value) / Math.LN2);
- if (value * (c = Math.pow(2, -e)) < 1) {
- e--;
- c *= 2;
- }
- if (e + eBias >= 1) {
- value += rt / c;
- } else {
- value += rt * Math.pow(2, 1 - eBias);
- }
- if (value * c >= 2) {
- e++;
- c /= 2;
- }
-
- if (e + eBias >= eMax) {
- m = 0;
- e = eMax;
- } else if (e + eBias >= 1) {
- m = (value * c - 1) * Math.pow(2, mLen);
- e = e + eBias;
- } else {
- m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
- e = 0;
- }
- }
-
- for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8);
-
- e = e << mLen | m;
- eLen += mLen;
- for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8);
-
- buffer[offset + i - d] |= s * 128;
- };
-
- exports.readIEEE754 = readIEEE754;
- exports.writeIEEE754 = writeIEEE754;
-
-/***/ }),
-/* 360 */
-/***/ (function(module, exports, __webpack_require__) {
-
- /* WEBPACK VAR INJECTION */(function(Buffer) {'use strict';
-
- var Long = __webpack_require__(335).Long,
- Double = __webpack_require__(336).Double,
- Timestamp = __webpack_require__(337).Timestamp,
- ObjectID = __webpack_require__(338).ObjectID,
- Symbol = __webpack_require__(349).Symbol,
- BSONRegExp = __webpack_require__(348).BSONRegExp,
- Code = __webpack_require__(351).Code,
- Decimal128 = __webpack_require__(352),
- MinKey = __webpack_require__(353).MinKey,
- MaxKey = __webpack_require__(354).MaxKey,
- DBRef = __webpack_require__(355).DBRef,
- Binary = __webpack_require__(356).Binary;
-
- var normalizedFunctionString = __webpack_require__(344).normalizedFunctionString;
-
- // To ensure that 0.4 of node works correctly
- var isDate = function isDate(d) {
- return typeof d === 'object' && Object.prototype.toString.call(d) === '[object Date]';
- };
-
- var calculateObjectSize = function calculateObjectSize(object, serializeFunctions, ignoreUndefined) {
- var totalLength = 4 + 1;
-
- if (Array.isArray(object)) {
- for (var i = 0; i < object.length; i++) {
- totalLength += calculateElement(i.toString(), object[i], serializeFunctions, true, ignoreUndefined);
- }
- } else {
- // If we have toBSON defined, override the current object
- if (object.toBSON) {
- object = object.toBSON();
- }
-
- // Calculate size
- for (var key in object) {
- totalLength += calculateElement(key, object[key], serializeFunctions, false, ignoreUndefined);
- }
- }
-
- return totalLength;
- };
-
- /**
- * @ignore
- * @api private
- */
- function calculateElement(name, value, serializeFunctions, isArray, ignoreUndefined) {
- // If we have toBSON defined, override the current object
- if (value && value.toBSON) {
- value = value.toBSON();
- }
-
- switch (typeof value) {
- case 'string':
- return 1 + Buffer.byteLength(name, 'utf8') + 1 + 4 + Buffer.byteLength(value, 'utf8') + 1;
- case 'number':
- if (Math.floor(value) === value && value >= BSON.JS_INT_MIN && value <= BSON.JS_INT_MAX) {
- if (value >= BSON.BSON_INT32_MIN && value <= BSON.BSON_INT32_MAX) {
- // 32 bit
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (4 + 1);
- } else {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
- }
- } else {
- // 64 bit
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
- }
- case 'undefined':
- if (isArray || !ignoreUndefined) return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
- return 0;
- case 'boolean':
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 1);
- case 'object':
- if (value == null || value instanceof MinKey || value instanceof MaxKey || value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
- } else if (value instanceof ObjectID || value['_bsontype'] === 'ObjectID' || value['_bsontype'] === 'ObjectId') {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (12 + 1);
- } else if (value instanceof Date || isDate(value)) {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
- } else if (typeof Buffer !== 'undefined' && Buffer.isBuffer(value)) {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 4 + 1) + value.length;
- } else if (value instanceof Long || value instanceof Double || value instanceof Timestamp || value['_bsontype'] === 'Long' || value['_bsontype'] === 'Double' || value['_bsontype'] === 'Timestamp') {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
- } else if (value instanceof Decimal128 || value['_bsontype'] === 'Decimal128') {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (16 + 1);
- } else if (value instanceof Code || value['_bsontype'] === 'Code') {
- // Calculate size depending on the availability of a scope
- if (value.scope != null && Object.keys(value.scope).length > 0) {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1 + 4 + 4 + Buffer.byteLength(value.code.toString(), 'utf8') + 1 + calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined);
- } else {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1 + 4 + Buffer.byteLength(value.code.toString(), 'utf8') + 1;
- }
- } else if (value instanceof Binary || value['_bsontype'] === 'Binary') {
- // Check what kind of subtype we have
- if (value.sub_type === Binary.SUBTYPE_BYTE_ARRAY) {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (value.position + 1 + 4 + 1 + 4);
- } else {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (value.position + 1 + 4 + 1);
- }
- } else if (value instanceof Symbol || value['_bsontype'] === 'Symbol') {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + Buffer.byteLength(value.value, 'utf8') + 4 + 1 + 1;
- } else if (value instanceof DBRef || value['_bsontype'] === 'DBRef') {
- // Set up correct object for serialization
- var ordered_values = {
- $ref: value.namespace,
- $id: value.oid
- };
-
- // Add db reference if it exists
- if (null != value.db) {
- ordered_values['$db'] = value.db;
- }
-
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1 + calculateObjectSize(ordered_values, serializeFunctions, ignoreUndefined);
- } else if (value instanceof RegExp || Object.prototype.toString.call(value) === '[object RegExp]') {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1 + Buffer.byteLength(value.source, 'utf8') + 1 + (value.global ? 1 : 0) + (value.ignoreCase ? 1 : 0) + (value.multiline ? 1 : 0) + 1;
- } else if (value instanceof BSONRegExp || value['_bsontype'] === 'BSONRegExp') {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1 + Buffer.byteLength(value.pattern, 'utf8') + 1 + Buffer.byteLength(value.options, 'utf8') + 1;
- } else {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + calculateObjectSize(value, serializeFunctions, ignoreUndefined) + 1;
- }
- case 'function':
- // WTF for 0.4.X where typeof /someregexp/ === 'function'
- if (value instanceof RegExp || Object.prototype.toString.call(value) === '[object RegExp]' || String.call(value) === '[object RegExp]') {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1 + Buffer.byteLength(value.source, 'utf8') + 1 + (value.global ? 1 : 0) + (value.ignoreCase ? 1 : 0) + (value.multiline ? 1 : 0) + 1;
- } else {
- if (serializeFunctions && value.scope != null && Object.keys(value.scope).length > 0) {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1 + 4 + 4 + Buffer.byteLength(normalizedFunctionString(value), 'utf8') + 1 + calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined);
- } else if (serializeFunctions) {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1 + 4 + Buffer.byteLength(normalizedFunctionString(value), 'utf8') + 1;
- }
- }
- }
-
- return 0;
- }
-
- var BSON = {};
-
- // BSON MAX VALUES
- BSON.BSON_INT32_MAX = 0x7fffffff;
- BSON.BSON_INT32_MIN = -0x80000000;
-
- // JS MAX PRECISE VALUES
- BSON.JS_INT_MAX = 0x20000000000000; // Any integer up to 2^53 can be precisely represented by a double.
- BSON.JS_INT_MIN = -0x20000000000000; // Any integer down to -2^53 can be precisely represented by a double.
-
- module.exports = calculateObjectSize;
- /* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(339).Buffer))
-
-/***/ })
-/******/ ])
-});
-;
\ No newline at end of file
diff --git a/node_modules/bson/browser_build/package.json b/node_modules/bson/browser_build/package.json
deleted file mode 100644
index 980db7f..0000000
--- a/node_modules/bson/browser_build/package.json
+++ /dev/null
@@ -1,8 +0,0 @@
-{ "name" : "bson"
-, "description" : "A bson parser for node.js and the browser"
-, "main": "../"
-, "directories" : { "lib" : "../lib/bson" }
-, "engines" : { "node" : ">=0.6.0" }
-, "licenses" : [ { "type" : "Apache License, Version 2.0"
- , "url" : "http://www.apache.org/licenses/LICENSE-2.0" } ]
-}
diff --git a/node_modules/bson/bson.d.ts b/node_modules/bson/bson.d.ts
new file mode 100644
index 0000000..b1402f8
--- /dev/null
+++ b/node_modules/bson/bson.d.ts
@@ -0,0 +1,1054 @@
+import { Buffer } from 'buffer';
+/**
+ * A class representation of the BSON Binary type.
+ * @public
+ */
+export declare class Binary {
+ _bsontype: 'Binary';
+ /* Excluded from this release type: BSON_BINARY_SUBTYPE_DEFAULT */
+ /** Initial buffer default size */
+ static readonly BUFFER_SIZE = 256;
+ /** Default BSON type */
+ static readonly SUBTYPE_DEFAULT = 0;
+ /** Function BSON type */
+ static readonly SUBTYPE_FUNCTION = 1;
+ /** Byte Array BSON type */
+ static readonly SUBTYPE_BYTE_ARRAY = 2;
+ /** Deprecated UUID BSON type @deprecated Please use SUBTYPE_UUID */
+ static readonly SUBTYPE_UUID_OLD = 3;
+ /** UUID BSON type */
+ static readonly SUBTYPE_UUID = 4;
+ /** MD5 BSON type */
+ static readonly SUBTYPE_MD5 = 5;
+ /** User BSON type */
+ static readonly SUBTYPE_USER_DEFINED = 128;
+ buffer: Buffer;
+ sub_type: number;
+ position: number;
+ /**
+ * @param buffer - a buffer object containing the binary data.
+ * @param subType - the option binary type.
+ */
+ constructor(buffer?: string | BinarySequence, subType?: number);
+ /**
+ * Updates this binary with byte_value.
+ *
+ * @param byteValue - a single byte we wish to write.
+ */
+ put(byteValue: string | number | Uint8Array | Buffer | number[]): void;
+ /**
+ * Writes a buffer or string to the binary.
+ *
+ * @param sequence - a string or buffer to be written to the Binary BSON object.
+ * @param offset - specify the binary of where to write the content.
+ */
+ write(sequence: string | BinarySequence, offset: number): void;
+ /**
+ * Reads **length** bytes starting at **position**.
+ *
+ * @param position - read from the given position in the Binary.
+ * @param length - the number of bytes to read.
+ */
+ read(position: number, length: number): BinarySequence;
+ /**
+ * Returns the value of this binary as a string.
+ * @param asRaw - Will skip converting to a string
+ * @remarks
+ * This is handy when calling this function conditionally for some key value pairs and not others
+ */
+ value(asRaw?: boolean): string | BinarySequence;
+ /** the length of the binary sequence */
+ length(): number;
+ /* Excluded from this release type: toJSON */
+ /* Excluded from this release type: toString */
+ /* Excluded from this release type: toExtendedJSON */
+ /* Excluded from this release type: toUUID */
+ /* Excluded from this release type: fromExtendedJSON */
+ inspect(): string;
+}
+/** @public */
+export declare interface BinaryExtended {
+ $binary: {
+ subType: string;
+ base64: string;
+ };
+}
+/** @public */
+export declare interface BinaryExtendedLegacy {
+ $type: string;
+ $binary: string;
+}
+/** @public */
+export declare type BinarySequence = Uint8Array | Buffer | number[];
+/**
+ * BSON default export
+ * @deprecated Please use named exports
+ * @privateRemarks
+ * We want to someday deprecate the default export,
+ * so none of the new TS types are being exported on the default
+ * @public
+ */
+declare const BSON: {
+ Binary: typeof Binary;
+ Code: typeof Code;
+ DBRef: typeof DBRef;
+ Decimal128: typeof Decimal128;
+ Double: typeof Double;
+ Int32: typeof Int32;
+ Long: typeof Long;
+ UUID: typeof UUID;
+ Map: MapConstructor;
+ MaxKey: typeof MaxKey;
+ MinKey: typeof MinKey;
+ ObjectId: typeof ObjectId;
+ ObjectID: typeof ObjectId;
+ BSONRegExp: typeof BSONRegExp;
+ BSONSymbol: typeof BSONSymbol;
+ Timestamp: typeof Timestamp;
+ EJSON: typeof EJSON;
+ setInternalBufferSize: typeof setInternalBufferSize;
+ serialize: typeof serialize;
+ serializeWithBufferAndIndex: typeof serializeWithBufferAndIndex;
+ deserialize: typeof deserialize;
+ calculateObjectSize: typeof calculateObjectSize;
+ deserializeStream: typeof deserializeStream;
+};
+export default BSON;
+/* Excluded from this release type: BSON_BINARY_SUBTYPE_BYTE_ARRAY */
+/* Excluded from this release type: BSON_BINARY_SUBTYPE_DEFAULT */
+/* Excluded from this release type: BSON_BINARY_SUBTYPE_FUNCTION */
+/* Excluded from this release type: BSON_BINARY_SUBTYPE_MD5 */
+/* Excluded from this release type: BSON_BINARY_SUBTYPE_USER_DEFINED */
+/* Excluded from this release type: BSON_BINARY_SUBTYPE_UUID */
+/* Excluded from this release type: BSON_BINARY_SUBTYPE_UUID_NEW */
+/* Excluded from this release type: BSON_DATA_ARRAY */
+/* Excluded from this release type: BSON_DATA_BINARY */
+/* Excluded from this release type: BSON_DATA_BOOLEAN */
+/* Excluded from this release type: BSON_DATA_CODE */
+/* Excluded from this release type: BSON_DATA_CODE_W_SCOPE */
+/* Excluded from this release type: BSON_DATA_DATE */
+/* Excluded from this release type: BSON_DATA_DBPOINTER */
+/* Excluded from this release type: BSON_DATA_DECIMAL128 */
+/* Excluded from this release type: BSON_DATA_INT */
+/* Excluded from this release type: BSON_DATA_LONG */
+/* Excluded from this release type: BSON_DATA_MAX_KEY */
+/* Excluded from this release type: BSON_DATA_MIN_KEY */
+/* Excluded from this release type: BSON_DATA_NULL */
+/* Excluded from this release type: BSON_DATA_NUMBER */
+/* Excluded from this release type: BSON_DATA_OBJECT */
+/* Excluded from this release type: BSON_DATA_OID */
+/* Excluded from this release type: BSON_DATA_REGEXP */
+/* Excluded from this release type: BSON_DATA_STRING */
+/* Excluded from this release type: BSON_DATA_SYMBOL */
+/* Excluded from this release type: BSON_DATA_TIMESTAMP */
+/* Excluded from this release type: BSON_DATA_UNDEFINED */
+/* Excluded from this release type: BSON_INT32_MAX */
+/* Excluded from this release type: BSON_INT32_MIN */
+/* Excluded from this release type: BSON_INT64_MAX */
+/* Excluded from this release type: BSON_INT64_MIN */
+/**
+ * A class representation of the BSON RegExp type.
+ * @public
+ */
+export declare class BSONRegExp {
+ _bsontype: 'BSONRegExp';
+ pattern: string;
+ options: string;
+ /**
+ * @param pattern - The regular expression pattern to match
+ * @param options - The regular expression options
+ */
+ constructor(pattern: string, options?: string);
+ static parseOptions(options?: string): string;
+}
+/** @public */
+export declare interface BSONRegExpExtended {
+ $regularExpression: {
+ pattern: string;
+ options: string;
+ };
+}
+/** @public */
+export declare interface BSONRegExpExtendedLegacy {
+ $regex: string | BSONRegExp;
+ $options: string;
+}
+/**
+ * A class representation of the BSON Symbol type.
+ * @public
+ */
+export declare class BSONSymbol {
+ _bsontype: 'Symbol';
+ value: string;
+ /**
+ * @param value - the string representing the symbol.
+ */
+ constructor(value: string);
+ /** Access the wrapped string value. */
+ valueOf(): string;
+}
+/** @public */
+export declare interface BSONSymbolExtended {
+ $symbol: string;
+}
+/**
+ * Calculate the bson size for a passed in Javascript object.
+ *
+ * @param object - the Javascript object to calculate the BSON byte size for
+ * @returns size of BSON object in bytes
+ * @public
+ */
+export declare function calculateObjectSize(object: Document, options?: CalculateObjectSizeOptions): number;
+/** @public */
+export declare type CalculateObjectSizeOptions = Pick;
+/**
+ * A class representation of the BSON Code type.
+ * @public
+ */
+export declare class Code {
+ _bsontype: 'Code';
+ code: string | Function;
+ scope?: Document;
+ /**
+ * @param code - a string or function.
+ * @param scope - an optional scope for the function.
+ */
+ constructor(code: string | Function, scope?: Document);
+ /* Excluded from this release type: toJSON */
+ /* Excluded from this release type: toExtendedJSON */
+ /* Excluded from this release type: fromExtendedJSON */
+ inspect(): string;
+}
+/** @public */
+export declare interface CodeExtended {
+ $code: string | Function;
+ $scope?: Document;
+}
+/**
+ * A class representation of the BSON DBRef type.
+ * @public
+ */
+export declare class DBRef {
+ _bsontype: 'DBRef';
+ collection: string;
+ oid: ObjectId;
+ db?: string;
+ fields: Document;
+ /**
+ * @param collection - the collection name.
+ * @param oid - the reference ObjectId.
+ * @param db - optional db name, if omitted the reference is local to the current db.
+ */
+ constructor(collection: string, oid: ObjectId, db?: string, fields?: Document);
+ /* Excluded from this release type: namespace */
+ /* Excluded from this release type: namespace */
+ /* Excluded from this release type: toJSON */
+ /* Excluded from this release type: toExtendedJSON */
+ /* Excluded from this release type: fromExtendedJSON */
+ inspect(): string;
+}
+/** @public */
+export declare interface DBRefLike {
+ $ref: string;
+ $id: ObjectId;
+ $db?: string;
+}
+/**
+ * A class representation of the BSON Decimal128 type.
+ * @public
+ */
+export declare class Decimal128 {
+ _bsontype: 'Decimal128';
+ readonly bytes: Buffer;
+ /**
+ * @param bytes - a buffer containing the raw Decimal128 bytes in little endian order,
+ * or a string representation as returned by .toString()
+ */
+ constructor(bytes: Buffer | string);
+ /**
+ * Create a Decimal128 instance from a string representation
+ *
+ * @param representation - a numeric string representation.
+ */
+ static fromString(representation: string): Decimal128;
+ /** Create a string representation of the raw Decimal128 value */
+ toString(): string;
+ toJSON(): Decimal128Extended;
+ /* Excluded from this release type: toExtendedJSON */
+ /* Excluded from this release type: fromExtendedJSON */
+ inspect(): string;
+}
+/** @public */
+export declare interface Decimal128Extended {
+ $numberDecimal: string;
+}
+/**
+ * Deserialize data as BSON.
+ *
+ * @param buffer - the buffer containing the serialized set of BSON documents.
+ * @returns returns the deserialized Javascript Object.
+ * @public
+ */
+export declare function deserialize(buffer: Buffer | ArrayBufferView | ArrayBuffer, options?: DeserializeOptions): Document;
+/** @public */
+export declare interface DeserializeOptions {
+ /** evaluate functions in the BSON document scoped to the object deserialized. */
+ evalFunctions?: boolean;
+ /** cache evaluated functions for reuse. */
+ cacheFunctions?: boolean;
+ /**
+ * use a crc32 code for caching, otherwise use the string of the function.
+ * @deprecated this option to use the crc32 function never worked as intended
+ * due to the fact that the crc32 function itself was never implemented.
+ * */
+ cacheFunctionsCrc32?: boolean;
+ /** when deserializing a Long will fit it into a Number if it's smaller than 53 bits */
+ promoteLongs?: boolean;
+ /** when deserializing a Binary will return it as a node.js Buffer instance. */
+ promoteBuffers?: boolean;
+ /** when deserializing will promote BSON values to their Node.js closest equivalent types. */
+ promoteValues?: boolean;
+ /** allow to specify if there what fields we wish to return as unserialized raw buffer. */
+ fieldsAsRaw?: Document;
+ /** return BSON regular expressions as BSONRegExp instances. */
+ bsonRegExp?: boolean;
+ /** allows the buffer to be larger than the parsed BSON object */
+ allowObjectSmallerThanBufferSize?: boolean;
+ /** Offset into buffer to begin reading document from */
+ index?: number;
+ raw?: boolean;
+}
+/**
+ * Deserialize stream data as BSON documents.
+ *
+ * @param data - the buffer containing the serialized set of BSON documents.
+ * @param startIndex - the start index in the data Buffer where the deserialization is to start.
+ * @param numberOfDocuments - number of documents to deserialize.
+ * @param documents - an array where to store the deserialized documents.
+ * @param docStartIndex - the index in the documents array from where to start inserting documents.
+ * @param options - additional options used for the deserialization.
+ * @returns next index in the buffer after deserialization **x** numbers of documents.
+ * @public
+ */
+export declare function deserializeStream(data: Buffer | ArrayBufferView | ArrayBuffer, startIndex: number, numberOfDocuments: number, documents: Document[], docStartIndex: number, options: DeserializeOptions): number;
+/** @public */
+export declare interface Document {
+ [key: string]: any;
+}
+/**
+ * A class representation of the BSON Double type.
+ * @public
+ */
+export declare class Double {
+ _bsontype: 'Double';
+ value: number;
+ /**
+ * Create a Double type
+ *
+ * @param value - the number we want to represent as a double.
+ */
+ constructor(value: number);
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped double number.
+ */
+ valueOf(): number;
+ /* Excluded from this release type: toJSON */
+ /* Excluded from this release type: toExtendedJSON */
+ /* Excluded from this release type: fromExtendedJSON */
+ inspect(): string;
+}
+/** @public */
+export declare interface DoubleExtended {
+ $numberDouble: string;
+}
+/**
+ * EJSON parse / stringify API
+ * @public
+ */
+export declare namespace EJSON {
+ export interface Options {
+ /** Output using the Extended JSON v1 spec */
+ legacy?: boolean;
+ /** Enable Extended JSON's `relaxed` mode, which attempts to return native JS types where possible, rather than BSON types */
+ relaxed?: boolean;
+ /**
+ * Disable Extended JSON's `relaxed` mode, which attempts to return BSON types where possible, rather than native JS types
+ * @deprecated Please use the relaxed property instead
+ */
+ strict?: boolean;
+ }
+ /**
+ * Parse an Extended JSON string, constructing the JavaScript value or object described by that
+ * string.
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const text = '{ "int32": { "$numberInt": "10" } }';
+ *
+ * // prints { int32: { [String: '10'] _bsontype: 'Int32', value: '10' } }
+ * console.log(EJSON.parse(text, { relaxed: false }));
+ *
+ * // prints { int32: 10 }
+ * console.log(EJSON.parse(text));
+ * ```
+ */
+ export function parse(text: string, options?: EJSON.Options): SerializableTypes;
+ export type JSONPrimitive = string | number | boolean | null;
+ export type SerializableTypes = Document | Array | JSONPrimitive;
+ /**
+ * Converts a BSON document to an Extended JSON string, optionally replacing values if a replacer
+ * function is specified or optionally including only the specified properties if a replacer array
+ * is specified.
+ *
+ * @param value - The value to convert to extended JSON
+ * @param replacer - A function that alters the behavior of the stringification process, or an array of String and Number objects that serve as a whitelist for selecting/filtering the properties of the value object to be included in the JSON string. If this value is null or not provided, all properties of the object are included in the resulting JSON string
+ * @param space - A String or Number object that's used to insert white space into the output JSON string for readability purposes.
+ * @param options - Optional settings
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const Int32 = require('mongodb').Int32;
+ * const doc = { int32: new Int32(10) };
+ *
+ * // prints '{"int32":{"$numberInt":"10"}}'
+ * console.log(EJSON.stringify(doc, { relaxed: false }));
+ *
+ * // prints '{"int32":10}'
+ * console.log(EJSON.stringify(doc));
+ * ```
+ */
+ export function stringify(value: SerializableTypes, replacer?: (number | string)[] | ((this: any, key: string, value: any) => any) | EJSON.Options, space?: string | number, options?: EJSON.Options): string;
+ /**
+ * Serializes an object to an Extended JSON string, and reparse it as a JavaScript object.
+ *
+ * @param value - The object to serialize
+ * @param options - Optional settings passed to the `stringify` function
+ */
+ export function serialize(value: SerializableTypes, options?: EJSON.Options): Document;
+ /**
+ * Deserializes an Extended JSON object into a plain JavaScript object with native/BSON types
+ *
+ * @param ejson - The Extended JSON object to deserialize
+ * @param options - Optional settings passed to the parse method
+ */
+ export function deserialize(ejson: Document, options?: EJSON.Options): SerializableTypes;
+}
+/** @public */
+export declare type EJSONOptions = EJSON.Options;
+/**
+ * A class representation of a BSON Int32 type.
+ * @public
+ */
+export declare class Int32 {
+ _bsontype: 'Int32';
+ value: number;
+ /**
+ * Create an Int32 type
+ *
+ * @param value - the number we want to represent as an int32.
+ */
+ constructor(value: number | string);
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped int32 number.
+ */
+ valueOf(): number;
+ /* Excluded from this release type: toJSON */
+ /* Excluded from this release type: toExtendedJSON */
+ /* Excluded from this release type: fromExtendedJSON */
+ inspect(): string;
+}
+/** @public */
+export declare interface Int32Extended {
+ $numberInt: string;
+}
+declare const kId: unique symbol;
+declare const kId_2: unique symbol;
+/**
+ * A class representing a 64-bit integer
+ * @public
+ * @remarks
+ * The internal representation of a long is the two given signed, 32-bit values.
+ * We use 32-bit pieces because these are the size of integers on which
+ * Javascript performs bit-operations. For operations like addition and
+ * multiplication, we split each number into 16 bit pieces, which can easily be
+ * multiplied within Javascript's floating-point representation without overflow
+ * or change in sign.
+ * In the algorithms below, we frequently reduce the negative case to the
+ * positive case by negating the input(s) and then post-processing the result.
+ * Note that we must ALWAYS check specially whether those values are MIN_VALUE
+ * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
+ * a positive number, it overflows back into a negative). Not handling this
+ * case would often result in infinite recursion.
+ * Common constant values ZERO, ONE, NEG_ONE, etc. are found as static properties on this class.
+ */
+export declare class Long {
+ _bsontype: 'Long';
+ /** An indicator used to reliably determine if an object is a Long or not. */
+ __isLong__: true;
+ /**
+ * The high 32 bits as a signed value.
+ */
+ high: number;
+ /**
+ * The low 32 bits as a signed value.
+ */
+ low: number;
+ /**
+ * Whether unsigned or not.
+ */
+ unsigned: boolean;
+ /**
+ * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers.
+ * See the from* functions below for more convenient ways of constructing Longs.
+ *
+ * Acceptable signatures are:
+ * - Long(low, high, unsigned?)
+ * - Long(bigint, unsigned?)
+ * - Long(string, unsigned?)
+ *
+ * @param low - The low (signed) 32 bits of the long
+ * @param high - The high (signed) 32 bits of the long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ constructor(low?: number | bigint | string, high?: number | boolean, unsigned?: boolean);
+ static TWO_PWR_24: Long;
+ /** Maximum unsigned value. */
+ static MAX_UNSIGNED_VALUE: Long;
+ /** Signed zero */
+ static ZERO: Long;
+ /** Unsigned zero. */
+ static UZERO: Long;
+ /** Signed one. */
+ static ONE: Long;
+ /** Unsigned one. */
+ static UONE: Long;
+ /** Signed negative one. */
+ static NEG_ONE: Long;
+ /** Maximum signed value. */
+ static MAX_VALUE: Long;
+ /** Minimum signed value. */
+ static MIN_VALUE: Long;
+ /**
+ * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits.
+ * Each is assumed to use 32 bits.
+ * @param lowBits - The low 32 bits
+ * @param highBits - The high 32 bits
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromBits(lowBits: number, highBits: number, unsigned?: boolean): Long;
+ /**
+ * Returns a Long representing the given 32 bit integer value.
+ * @param value - The 32 bit integer in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromInt(value: number, unsigned?: boolean): Long;
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromNumber(value: number, unsigned?: boolean): Long;
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromBigInt(value: bigint, unsigned?: boolean): Long;
+ /**
+ * Returns a Long representation of the given string, written using the specified radix.
+ * @param str - The textual representation of the Long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param radix - The radix in which the text is written (2-36), defaults to 10
+ * @returns The corresponding Long value
+ */
+ static fromString(str: string, unsigned?: boolean, radix?: number): Long;
+ /**
+ * Creates a Long from its byte representation.
+ * @param bytes - Byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns The corresponding Long value
+ */
+ static fromBytes(bytes: number[], unsigned?: boolean, le?: boolean): Long;
+ /**
+ * Creates a Long from its little endian byte representation.
+ * @param bytes - Little endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromBytesLE(bytes: number[], unsigned?: boolean): Long;
+ /**
+ * Creates a Long from its big endian byte representation.
+ * @param bytes - Big endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromBytesBE(bytes: number[], unsigned?: boolean): Long;
+ /**
+ * Tests if the specified object is a Long.
+ */
+ static isLong(value: any): value is Long;
+ /**
+ * Converts the specified value to a Long.
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ static fromValue(val: number | string | {
+ low: number;
+ high: number;
+ unsigned?: boolean;
+ }, unsigned?: boolean): Long;
+ /** Returns the sum of this and the specified Long. */
+ add(addend: string | number | Long | Timestamp): Long;
+ /**
+ * Returns the sum of this and the specified Long.
+ * @returns Sum
+ */
+ and(other: string | number | Long | Timestamp): Long;
+ /**
+ * Compares this Long's value with the specified's.
+ * @returns 0 if they are the same, 1 if the this is greater and -1 if the given one is greater
+ */
+ compare(other: string | number | Long | Timestamp): 0 | 1 | -1;
+ /** This is an alias of {@link Long.compare} */
+ comp(other: string | number | Long | Timestamp): 0 | 1 | -1;
+ /**
+ * Returns this Long divided by the specified. The result is signed if this Long is signed or unsigned if this Long is unsigned.
+ * @returns Quotient
+ */
+ divide(divisor: string | number | Long | Timestamp): Long;
+ /**This is an alias of {@link Long.divide} */
+ div(divisor: string | number | Long | Timestamp): Long;
+ /**
+ * Tests if this Long's value equals the specified's.
+ * @param other - Other value
+ */
+ equals(other: string | number | Long | Timestamp): boolean;
+ /** This is an alias of {@link Long.equals} */
+ eq(other: string | number | Long | Timestamp): boolean;
+ /** Gets the high 32 bits as a signed integer. */
+ getHighBits(): number;
+ /** Gets the high 32 bits as an unsigned integer. */
+ getHighBitsUnsigned(): number;
+ /** Gets the low 32 bits as a signed integer. */
+ getLowBits(): number;
+ /** Gets the low 32 bits as an unsigned integer. */
+ getLowBitsUnsigned(): number;
+ /** Gets the number of bits needed to represent the absolute value of this Long. */
+ getNumBitsAbs(): number;
+ /** Tests if this Long's value is greater than the specified's. */
+ greaterThan(other: string | number | Long | Timestamp): boolean;
+ /** This is an alias of {@link Long.greaterThan} */
+ gt(other: string | number | Long | Timestamp): boolean;
+ /** Tests if this Long's value is greater than or equal the specified's. */
+ greaterThanOrEqual(other: string | number | Long | Timestamp): boolean;
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ gte(other: string | number | Long | Timestamp): boolean;
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ ge(other: string | number | Long | Timestamp): boolean;
+ /** Tests if this Long's value is even. */
+ isEven(): boolean;
+ /** Tests if this Long's value is negative. */
+ isNegative(): boolean;
+ /** Tests if this Long's value is odd. */
+ isOdd(): boolean;
+ /** Tests if this Long's value is positive. */
+ isPositive(): boolean;
+ /** Tests if this Long's value equals zero. */
+ isZero(): boolean;
+ /** Tests if this Long's value is less than the specified's. */
+ lessThan(other: string | number | Long | Timestamp): boolean;
+ /** This is an alias of {@link Long#lessThan}. */
+ lt(other: string | number | Long | Timestamp): boolean;
+ /** Tests if this Long's value is less than or equal the specified's. */
+ lessThanOrEqual(other: string | number | Long | Timestamp): boolean;
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ lte(other: string | number | Long | Timestamp): boolean;
+ /** Returns this Long modulo the specified. */
+ modulo(divisor: string | number | Long | Timestamp): Long;
+ /** This is an alias of {@link Long.modulo} */
+ mod(divisor: string | number | Long | Timestamp): Long;
+ /** This is an alias of {@link Long.modulo} */
+ rem(divisor: string | number | Long | Timestamp): Long;
+ /**
+ * Returns the product of this and the specified Long.
+ * @param multiplier - Multiplier
+ * @returns Product
+ */
+ multiply(multiplier: string | number | Long | Timestamp): Long;
+ /** This is an alias of {@link Long.multiply} */
+ mul(multiplier: string | number | Long | Timestamp): Long;
+ /** Returns the Negation of this Long's value. */
+ negate(): Long;
+ /** This is an alias of {@link Long.negate} */
+ neg(): Long;
+ /** Returns the bitwise NOT of this Long. */
+ not(): Long;
+ /** Tests if this Long's value differs from the specified's. */
+ notEquals(other: string | number | Long | Timestamp): boolean;
+ /** This is an alias of {@link Long.notEquals} */
+ neq(other: string | number | Long | Timestamp): boolean;
+ /** This is an alias of {@link Long.notEquals} */
+ ne(other: string | number | Long | Timestamp): boolean;
+ /**
+ * Returns the bitwise OR of this Long and the specified.
+ */
+ or(other: number | string | Long): Long;
+ /**
+ * Returns this Long with bits shifted to the left by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ shiftLeft(numBits: number | Long): Long;
+ /** This is an alias of {@link Long.shiftLeft} */
+ shl(numBits: number | Long): Long;
+ /**
+ * Returns this Long with bits arithmetically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ shiftRight(numBits: number | Long): Long;
+ /** This is an alias of {@link Long.shiftRight} */
+ shr(numBits: number | Long): Long;
+ /**
+ * Returns this Long with bits logically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ shiftRightUnsigned(numBits: Long | number): Long;
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ shr_u(numBits: number | Long): Long;
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ shru(numBits: number | Long): Long;
+ /**
+ * Returns the difference of this and the specified Long.
+ * @param subtrahend - Subtrahend
+ * @returns Difference
+ */
+ subtract(subtrahend: string | number | Long | Timestamp): Long;
+ /** This is an alias of {@link Long.subtract} */
+ sub(subtrahend: string | number | Long | Timestamp): Long;
+ /** Converts the Long to a 32 bit integer, assuming it is a 32 bit integer. */
+ toInt(): number;
+ /** Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa). */
+ toNumber(): number;
+ /** Converts the Long to a BigInt (arbitrary precision). */
+ toBigInt(): bigint;
+ /**
+ * Converts this Long to its byte representation.
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns Byte representation
+ */
+ toBytes(le?: boolean): number[];
+ /**
+ * Converts this Long to its little endian byte representation.
+ * @returns Little endian byte representation
+ */
+ toBytesLE(): number[];
+ /**
+ * Converts this Long to its big endian byte representation.
+ * @returns Big endian byte representation
+ */
+ toBytesBE(): number[];
+ /**
+ * Converts this Long to signed.
+ */
+ toSigned(): Long;
+ /**
+ * Converts the Long to a string written in the specified radix.
+ * @param radix - Radix (2-36), defaults to 10
+ * @throws RangeError If `radix` is out of range
+ */
+ toString(radix?: number): string;
+ /** Converts this Long to unsigned. */
+ toUnsigned(): Long;
+ /** Returns the bitwise XOR of this Long and the given one. */
+ xor(other: Long | number | string): Long;
+ /** This is an alias of {@link Long.isZero} */
+ eqz(): boolean;
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ le(other: string | number | Long | Timestamp): boolean;
+ toExtendedJSON(options?: EJSONOptions): number | LongExtended;
+ static fromExtendedJSON(doc: {
+ $numberLong: string;
+ }, options?: EJSONOptions): number | Long;
+ inspect(): string;
+}
+/** @public */
+export declare interface LongExtended {
+ $numberLong: string;
+}
+/** @public */
+export declare type LongWithoutOverrides = new (low: number | Long, high?: number, unsigned?: boolean) => {
+ [P in Exclude]: Long[P];
+};
+/** @public */
+export declare const LongWithoutOverridesClass: LongWithoutOverrides;
+/** @public */
+declare let Map_2: MapConstructor;
+export { Map_2 as Map };
+/**
+ * A class representation of the BSON MaxKey type.
+ * @public
+ */
+export declare class MaxKey {
+ _bsontype: 'MaxKey';
+ constructor();
+ /* Excluded from this release type: toExtendedJSON */
+ /* Excluded from this release type: fromExtendedJSON */
+ inspect(): string;
+}
+/** @public */
+export declare interface MaxKeyExtended {
+ $maxKey: 1;
+}
+/**
+ * A class representation of the BSON MinKey type.
+ * @public
+ */
+export declare class MinKey {
+ _bsontype: 'MinKey';
+ constructor();
+ /* Excluded from this release type: toExtendedJSON */
+ /* Excluded from this release type: fromExtendedJSON */
+ inspect(): string;
+}
+/** @public */
+export declare interface MinKeyExtended {
+ $minKey: 1;
+}
+/**
+ * A class representation of the BSON ObjectId type.
+ * @public
+ */
+declare class ObjectId {
+ _bsontype: 'ObjectId';
+ /* Excluded from this release type: index */
+ static cacheHexString: boolean;
+ /* Excluded from this release type: [kId] */
+ /* Excluded from this release type: __id */
+ /**
+ * Create an ObjectId type
+ *
+ * @param id - Can be a 24 character hex string, 12 byte binary Buffer, or a number.
+ */
+ constructor(id?: string | Buffer | number | ObjectIdLike | ObjectId);
+ /*
+ * The ObjectId bytes
+ * @readonly
+ */
+ id: Buffer;
+ /*
+ * The generation time of this ObjectId instance
+ * @deprecated Please use getTimestamp / createFromTime which returns an int32 epoch
+ */
+ generationTime: number;
+ /** Returns the ObjectId id as a 24 character hex string representation */
+ toHexString(): string;
+ /* Excluded from this release type: getInc */
+ /**
+ * Generate a 12 byte id buffer used in ObjectId's
+ *
+ * @param time - pass in a second based timestamp.
+ */
+ static generate(time?: number): Buffer;
+ /* Excluded from this release type: toString */
+ /* Excluded from this release type: toJSON */
+ /**
+ * Compares the equality of this ObjectId with `otherID`.
+ *
+ * @param otherId - ObjectId instance to compare against.
+ */
+ equals(otherId: string | ObjectId | ObjectIdLike): boolean;
+ /** Returns the generation date (accurate up to the second) that this ID was generated. */
+ getTimestamp(): Date;
+ /* Excluded from this release type: createPk */
+ /**
+ * Creates an ObjectId from a second based number, with the rest of the ObjectId zeroed out. Used for comparisons or sorting the ObjectId.
+ *
+ * @param time - an integer number representing a number of seconds.
+ */
+ static createFromTime(time: number): ObjectId;
+ /**
+ * Creates an ObjectId from a hex string representation of an ObjectId.
+ *
+ * @param hexString - create a ObjectId from a passed in 24 character hexstring.
+ */
+ static createFromHexString(hexString: string): ObjectId;
+ /**
+ * Checks if a value is a valid bson ObjectId
+ *
+ * @param id - ObjectId instance to validate.
+ */
+ static isValid(id: number | string | ObjectId | Uint8Array | ObjectIdLike): boolean;
+ /* Excluded from this release type: toExtendedJSON */
+ /* Excluded from this release type: fromExtendedJSON */
+ inspect(): string;
+}
+export { ObjectId as ObjectID };
+export { ObjectId };
+/** @public */
+export declare interface ObjectIdExtended {
+ $oid: string;
+}
+/** @public */
+export declare interface ObjectIdLike {
+ id: string | Buffer;
+ __id?: string;
+ toHexString(): string;
+}
+/**
+ * Serialize a Javascript object.
+ *
+ * @param object - the Javascript object to serialize.
+ * @returns Buffer object containing the serialized object.
+ * @public
+ */
+export declare function serialize(object: Document, options?: SerializeOptions): Buffer;
+/** @public */
+export declare interface SerializeOptions {
+ /** the serializer will check if keys are valid. */
+ checkKeys?: boolean;
+ /** serialize the javascript functions **(default:false)**. */
+ serializeFunctions?: boolean;
+ /** serialize will not emit undefined fields **(default:true)** */
+ ignoreUndefined?: boolean;
+ /* Excluded from this release type: minInternalBufferSize */
+ /** the index in the buffer where we wish to start serializing into */
+ index?: number;
+}
+/**
+ * Serialize a Javascript object using a predefined Buffer and index into the buffer,
+ * useful when pre-allocating the space for serialization.
+ *
+ * @param object - the Javascript object to serialize.
+ * @param finalBuffer - the Buffer you pre-allocated to store the serialized BSON object.
+ * @returns the index pointing to the last written byte in the buffer.
+ * @public
+ */
+export declare function serializeWithBufferAndIndex(object: Document, finalBuffer: Buffer, options?: SerializeOptions): number;
+/**
+ * Sets the size of the internal serialization buffer.
+ *
+ * @param size - The desired size for the internal serialization buffer
+ * @public
+ */
+export declare function setInternalBufferSize(size: number): void;
+/** @public */
+export declare class Timestamp extends LongWithoutOverridesClass {
+ _bsontype: 'Timestamp';
+ static readonly MAX_VALUE: Long;
+ /**
+ * @param low - A 64-bit Long representing the Timestamp.
+ */
+ constructor(low: Long);
+ /**
+ * @param low - the low (signed) 32 bits of the Timestamp.
+ * @param high - the high (signed) 32 bits of the Timestamp.
+ */
+ constructor(low: Long);
+ constructor(low: number, high: number);
+ toJSON(): {
+ $timestamp: string;
+ };
+ /** Returns a Timestamp represented by the given (32-bit) integer value. */
+ static fromInt(value: number): Timestamp;
+ /** Returns a Timestamp representing the given number value, provided that it is a finite number. Otherwise, zero is returned. */
+ static fromNumber(value: number): Timestamp;
+ /**
+ * Returns a Timestamp for the given high and low bits. Each is assumed to use 32 bits.
+ *
+ * @param lowBits - the low 32-bits.
+ * @param highBits - the high 32-bits.
+ */
+ static fromBits(lowBits: number, highBits: number): Timestamp;
+ /**
+ * Returns a Timestamp from the given string, optionally using the given radix.
+ *
+ * @param str - the textual representation of the Timestamp.
+ * @param optRadix - the radix in which the text is written.
+ */
+ static fromString(str: string, optRadix: number): Timestamp;
+ /* Excluded from this release type: toExtendedJSON */
+ /* Excluded from this release type: fromExtendedJSON */
+ inspect(): string;
+}
+/** @public */
+export declare interface TimestampExtended {
+ $timestamp: {
+ t: number;
+ i: number;
+ };
+}
+/** @public */
+export declare type TimestampOverrides = '_bsontype' | 'toExtendedJSON' | 'fromExtendedJSON' | 'inspect';
+/**
+ * A class representation of the BSON UUID type.
+ * @public
+ */
+export declare class UUID {
+ _bsontype: 'UUID';
+ static cacheHexString: boolean;
+ /* Excluded from this release type: [kId] */
+ /* Excluded from this release type: __id */
+ /**
+ * Create an UUID type
+ *
+ * @param input - Can be a 32 or 36 character hex string (dashes excluded/included) or a 16 byte binary Buffer.
+ */
+ constructor(input?: string | Buffer | UUID);
+ /*
+ * The UUID bytes
+ * @readonly
+ */
+ id: Buffer;
+ /**
+ * Generate a 16 byte uuid v4 buffer used in UUIDs
+ */
+ /**
+ * Returns the UUID id as a 32 or 36 character hex string representation, excluding/including dashes (defaults to 36 character dash separated)
+ * @param includeDashes - should the string exclude dash-separators.
+ * */
+ toHexString(includeDashes?: boolean): string;
+ /* Excluded from this release type: toString */
+ /* Excluded from this release type: toJSON */
+ /**
+ * Compares the equality of this UUID with `otherID`.
+ *
+ * @param otherId - UUID instance to compare against.
+ */
+ equals(otherId: string | Buffer | UUID): boolean;
+ /**
+ * Creates a Binary instance from the current UUID.
+ */
+ toBinary(): Binary;
+ /**
+ * Generates a populated buffer containing a v4 uuid
+ */
+ static generate(): Buffer;
+ /**
+ * Checks if a value is a valid bson UUID
+ * @param input - UUID, string or Buffer to validate.
+ */
+ static isValid(input: string | Buffer | UUID): boolean;
+ /**
+ * Creates an UUID from a hex string representation of an UUID.
+ * @param hexString - 32 or 36 character hex string (dashes excluded/included).
+ */
+ static createFromHexString(hexString: string): UUID;
+ inspect(): string;
+}
+/** @public */
+export declare type UUIDExtended = {
+ $uuid: string;
+};
+export {};
diff --git a/node_modules/bson/dist/bson.browser.esm.js b/node_modules/bson/dist/bson.browser.esm.js
new file mode 100644
index 0000000..bb088fb
--- /dev/null
+++ b/node_modules/bson/dist/bson.browser.esm.js
@@ -0,0 +1,8952 @@
+var commonjsGlobal = typeof globalThis !== 'undefined' ? globalThis : typeof window !== 'undefined' ? window : typeof global !== 'undefined' ? global : typeof self !== 'undefined' ? self : {};
+
+function unwrapExports (x) {
+ return x && x.__esModule && Object.prototype.hasOwnProperty.call(x, 'default') ? x['default'] : x;
+}
+
+function createCommonjsModule(fn, module) {
+ return module = { exports: {} }, fn(module, module.exports), module.exports;
+}
+
+var byteLength_1 = byteLength;
+var toByteArray_1 = toByteArray;
+var fromByteArray_1 = fromByteArray;
+var lookup = [];
+var revLookup = [];
+var Arr = typeof Uint8Array !== 'undefined' ? Uint8Array : Array;
+var code$1 = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/';
+
+for (var i = 0, len = code$1.length; i < len; ++i) {
+ lookup[i] = code$1[i];
+ revLookup[code$1.charCodeAt(i)] = i;
+} // Support decoding URL-safe base64 strings, as Node.js does.
+// See: https://en.wikipedia.org/wiki/Base64#URL_applications
+
+
+revLookup['-'.charCodeAt(0)] = 62;
+revLookup['_'.charCodeAt(0)] = 63;
+
+function getLens(b64) {
+ var len = b64.length;
+
+ if (len % 4 > 0) {
+ throw new Error('Invalid string. Length must be a multiple of 4');
+ } // Trim off extra bytes after placeholder bytes are found
+ // See: https://github.com/beatgammit/base64-js/issues/42
+
+
+ var validLen = b64.indexOf('=');
+ if (validLen === -1) validLen = len;
+ var placeHoldersLen = validLen === len ? 0 : 4 - validLen % 4;
+ return [validLen, placeHoldersLen];
+} // base64 is 4/3 + up to two characters of the original data
+
+
+function byteLength(b64) {
+ var lens = getLens(b64);
+ var validLen = lens[0];
+ var placeHoldersLen = lens[1];
+ return (validLen + placeHoldersLen) * 3 / 4 - placeHoldersLen;
+}
+
+function _byteLength(b64, validLen, placeHoldersLen) {
+ return (validLen + placeHoldersLen) * 3 / 4 - placeHoldersLen;
+}
+
+function toByteArray(b64) {
+ var tmp;
+ var lens = getLens(b64);
+ var validLen = lens[0];
+ var placeHoldersLen = lens[1];
+ var arr = new Arr(_byteLength(b64, validLen, placeHoldersLen));
+ var curByte = 0; // if there are placeholders, only get up to the last complete 4 chars
+
+ var len = placeHoldersLen > 0 ? validLen - 4 : validLen;
+ var i;
+
+ for (i = 0; i < len; i += 4) {
+ tmp = revLookup[b64.charCodeAt(i)] << 18 | revLookup[b64.charCodeAt(i + 1)] << 12 | revLookup[b64.charCodeAt(i + 2)] << 6 | revLookup[b64.charCodeAt(i + 3)];
+ arr[curByte++] = tmp >> 16 & 0xFF;
+ arr[curByte++] = tmp >> 8 & 0xFF;
+ arr[curByte++] = tmp & 0xFF;
+ }
+
+ if (placeHoldersLen === 2) {
+ tmp = revLookup[b64.charCodeAt(i)] << 2 | revLookup[b64.charCodeAt(i + 1)] >> 4;
+ arr[curByte++] = tmp & 0xFF;
+ }
+
+ if (placeHoldersLen === 1) {
+ tmp = revLookup[b64.charCodeAt(i)] << 10 | revLookup[b64.charCodeAt(i + 1)] << 4 | revLookup[b64.charCodeAt(i + 2)] >> 2;
+ arr[curByte++] = tmp >> 8 & 0xFF;
+ arr[curByte++] = tmp & 0xFF;
+ }
+
+ return arr;
+}
+
+function tripletToBase64(num) {
+ return lookup[num >> 18 & 0x3F] + lookup[num >> 12 & 0x3F] + lookup[num >> 6 & 0x3F] + lookup[num & 0x3F];
+}
+
+function encodeChunk(uint8, start, end) {
+ var tmp;
+ var output = [];
+
+ for (var i = start; i < end; i += 3) {
+ tmp = (uint8[i] << 16 & 0xFF0000) + (uint8[i + 1] << 8 & 0xFF00) + (uint8[i + 2] & 0xFF);
+ output.push(tripletToBase64(tmp));
+ }
+
+ return output.join('');
+}
+
+function fromByteArray(uint8) {
+ var tmp;
+ var len = uint8.length;
+ var extraBytes = len % 3; // if we have 1 byte left, pad 2 bytes
+
+ var parts = [];
+ var maxChunkLength = 16383; // must be multiple of 3
+ // go through the array every three bytes, we'll deal with trailing stuff later
+
+ for (var i = 0, len2 = len - extraBytes; i < len2; i += maxChunkLength) {
+ parts.push(encodeChunk(uint8, i, i + maxChunkLength > len2 ? len2 : i + maxChunkLength));
+ } // pad the end with zeros, but make sure to not forget the extra bytes
+
+
+ if (extraBytes === 1) {
+ tmp = uint8[len - 1];
+ parts.push(lookup[tmp >> 2] + lookup[tmp << 4 & 0x3F] + '==');
+ } else if (extraBytes === 2) {
+ tmp = (uint8[len - 2] << 8) + uint8[len - 1];
+ parts.push(lookup[tmp >> 10] + lookup[tmp >> 4 & 0x3F] + lookup[tmp << 2 & 0x3F] + '=');
+ }
+
+ return parts.join('');
+}
+
+var base64Js = {
+ byteLength: byteLength_1,
+ toByteArray: toByteArray_1,
+ fromByteArray: fromByteArray_1
+};
+
+/*! ieee754. BSD-3-Clause License. Feross Aboukhadijeh */
+var read = function read(buffer, offset, isLE, mLen, nBytes) {
+ var e, m;
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var nBits = -7;
+ var i = isLE ? nBytes - 1 : 0;
+ var d = isLE ? -1 : 1;
+ var s = buffer[offset + i];
+ i += d;
+ e = s & (1 << -nBits) - 1;
+ s >>= -nBits;
+ nBits += eLen;
+
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8) {}
+
+ m = e & (1 << -nBits) - 1;
+ e >>= -nBits;
+ nBits += mLen;
+
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8) {}
+
+ if (e === 0) {
+ e = 1 - eBias;
+ } else if (e === eMax) {
+ return m ? NaN : (s ? -1 : 1) * Infinity;
+ } else {
+ m = m + Math.pow(2, mLen);
+ e = e - eBias;
+ }
+
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
+};
+
+var write = function write(buffer, value, offset, isLE, mLen, nBytes) {
+ var e, m, c;
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0;
+ var i = isLE ? 0 : nBytes - 1;
+ var d = isLE ? 1 : -1;
+ var s = value < 0 || value === 0 && 1 / value < 0 ? 1 : 0;
+ value = Math.abs(value);
+
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0;
+ e = eMax;
+ } else {
+ e = Math.floor(Math.log(value) / Math.LN2);
+
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--;
+ c *= 2;
+ }
+
+ if (e + eBias >= 1) {
+ value += rt / c;
+ } else {
+ value += rt * Math.pow(2, 1 - eBias);
+ }
+
+ if (value * c >= 2) {
+ e++;
+ c /= 2;
+ }
+
+ if (e + eBias >= eMax) {
+ m = 0;
+ e = eMax;
+ } else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen);
+ e = e + eBias;
+ } else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
+ e = 0;
+ }
+ }
+
+ for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}
+
+ e = e << mLen | m;
+ eLen += mLen;
+
+ for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}
+
+ buffer[offset + i - d] |= s * 128;
+};
+
+var ieee754 = {
+ read: read,
+ write: write
+};
+
+var buffer = createCommonjsModule(function (module, exports) {
+
+ var customInspectSymbol = typeof Symbol === 'function' && typeof Symbol['for'] === 'function' ? // eslint-disable-line dot-notation
+ Symbol['for']('nodejs.util.inspect.custom') // eslint-disable-line dot-notation
+ : null;
+ exports.Buffer = Buffer;
+ exports.SlowBuffer = SlowBuffer;
+ exports.INSPECT_MAX_BYTES = 50;
+ var K_MAX_LENGTH = 0x7fffffff;
+ exports.kMaxLength = K_MAX_LENGTH;
+ /**
+ * If `Buffer.TYPED_ARRAY_SUPPORT`:
+ * === true Use Uint8Array implementation (fastest)
+ * === false Print warning and recommend using `buffer` v4.x which has an Object
+ * implementation (most compatible, even IE6)
+ *
+ * Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,
+ * Opera 11.6+, iOS 4.2+.
+ *
+ * We report that the browser does not support typed arrays if the are not subclassable
+ * using __proto__. Firefox 4-29 lacks support for adding new properties to `Uint8Array`
+ * (See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438). IE 10 lacks support
+ * for __proto__ and has a buggy typed array implementation.
+ */
+
+ Buffer.TYPED_ARRAY_SUPPORT = typedArraySupport();
+
+ if (!Buffer.TYPED_ARRAY_SUPPORT && typeof console !== 'undefined' && typeof console.error === 'function') {
+ console.error('This browser lacks typed array (Uint8Array) support which is required by ' + '`buffer` v5.x. Use `buffer` v4.x if you require old browser support.');
+ }
+
+ function typedArraySupport() {
+ // Can typed array instances can be augmented?
+ try {
+ var arr = new Uint8Array(1);
+ var proto = {
+ foo: function foo() {
+ return 42;
+ }
+ };
+ Object.setPrototypeOf(proto, Uint8Array.prototype);
+ Object.setPrototypeOf(arr, proto);
+ return arr.foo() === 42;
+ } catch (e) {
+ return false;
+ }
+ }
+
+ Object.defineProperty(Buffer.prototype, 'parent', {
+ enumerable: true,
+ get: function get() {
+ if (!Buffer.isBuffer(this)) return undefined;
+ return this.buffer;
+ }
+ });
+ Object.defineProperty(Buffer.prototype, 'offset', {
+ enumerable: true,
+ get: function get() {
+ if (!Buffer.isBuffer(this)) return undefined;
+ return this.byteOffset;
+ }
+ });
+
+ function createBuffer(length) {
+ if (length > K_MAX_LENGTH) {
+ throw new RangeError('The value "' + length + '" is invalid for option "size"');
+ } // Return an augmented `Uint8Array` instance
+
+
+ var buf = new Uint8Array(length);
+ Object.setPrototypeOf(buf, Buffer.prototype);
+ return buf;
+ }
+ /**
+ * The Buffer constructor returns instances of `Uint8Array` that have their
+ * prototype changed to `Buffer.prototype`. Furthermore, `Buffer` is a subclass of
+ * `Uint8Array`, so the returned instances will have all the node `Buffer` methods
+ * and the `Uint8Array` methods. Square bracket notation works as expected -- it
+ * returns a single octet.
+ *
+ * The `Uint8Array` prototype remains unmodified.
+ */
+
+
+ function Buffer(arg, encodingOrOffset, length) {
+ // Common case.
+ if (typeof arg === 'number') {
+ if (typeof encodingOrOffset === 'string') {
+ throw new TypeError('The "string" argument must be of type string. Received type number');
+ }
+
+ return allocUnsafe(arg);
+ }
+
+ return from(arg, encodingOrOffset, length);
+ }
+
+ Buffer.poolSize = 8192; // not used by this implementation
+
+ function from(value, encodingOrOffset, length) {
+ if (typeof value === 'string') {
+ return fromString(value, encodingOrOffset);
+ }
+
+ if (ArrayBuffer.isView(value)) {
+ return fromArrayView(value);
+ }
+
+ if (value == null) {
+ throw new TypeError('The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' + 'or Array-like Object. Received type ' + babelHelpers["typeof"](value));
+ }
+
+ if (isInstance(value, ArrayBuffer) || value && isInstance(value.buffer, ArrayBuffer)) {
+ return fromArrayBuffer(value, encodingOrOffset, length);
+ }
+
+ if (typeof SharedArrayBuffer !== 'undefined' && (isInstance(value, SharedArrayBuffer) || value && isInstance(value.buffer, SharedArrayBuffer))) {
+ return fromArrayBuffer(value, encodingOrOffset, length);
+ }
+
+ if (typeof value === 'number') {
+ throw new TypeError('The "value" argument must not be of type number. Received type number');
+ }
+
+ var valueOf = value.valueOf && value.valueOf();
+
+ if (valueOf != null && valueOf !== value) {
+ return Buffer.from(valueOf, encodingOrOffset, length);
+ }
+
+ var b = fromObject(value);
+ if (b) return b;
+
+ if (typeof Symbol !== 'undefined' && Symbol.toPrimitive != null && typeof value[Symbol.toPrimitive] === 'function') {
+ return Buffer.from(value[Symbol.toPrimitive]('string'), encodingOrOffset, length);
+ }
+
+ throw new TypeError('The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' + 'or Array-like Object. Received type ' + babelHelpers["typeof"](value));
+ }
+ /**
+ * Functionally equivalent to Buffer(arg, encoding) but throws a TypeError
+ * if value is a number.
+ * Buffer.from(str[, encoding])
+ * Buffer.from(array)
+ * Buffer.from(buffer)
+ * Buffer.from(arrayBuffer[, byteOffset[, length]])
+ **/
+
+
+ Buffer.from = function (value, encodingOrOffset, length) {
+ return from(value, encodingOrOffset, length);
+ }; // Note: Change prototype *after* Buffer.from is defined to workaround Chrome bug:
+ // https://github.com/feross/buffer/pull/148
+
+
+ Object.setPrototypeOf(Buffer.prototype, Uint8Array.prototype);
+ Object.setPrototypeOf(Buffer, Uint8Array);
+
+ function assertSize(size) {
+ if (typeof size !== 'number') {
+ throw new TypeError('"size" argument must be of type number');
+ } else if (size < 0) {
+ throw new RangeError('The value "' + size + '" is invalid for option "size"');
+ }
+ }
+
+ function alloc(size, fill, encoding) {
+ assertSize(size);
+
+ if (size <= 0) {
+ return createBuffer(size);
+ }
+
+ if (fill !== undefined) {
+ // Only pay attention to encoding if it's a string. This
+ // prevents accidentally sending in a number that would
+ // be interpreted as a start offset.
+ return typeof encoding === 'string' ? createBuffer(size).fill(fill, encoding) : createBuffer(size).fill(fill);
+ }
+
+ return createBuffer(size);
+ }
+ /**
+ * Creates a new filled Buffer instance.
+ * alloc(size[, fill[, encoding]])
+ **/
+
+
+ Buffer.alloc = function (size, fill, encoding) {
+ return alloc(size, fill, encoding);
+ };
+
+ function allocUnsafe(size) {
+ assertSize(size);
+ return createBuffer(size < 0 ? 0 : checked(size) | 0);
+ }
+ /**
+ * Equivalent to Buffer(num), by default creates a non-zero-filled Buffer instance.
+ * */
+
+
+ Buffer.allocUnsafe = function (size) {
+ return allocUnsafe(size);
+ };
+ /**
+ * Equivalent to SlowBuffer(num), by default creates a non-zero-filled Buffer instance.
+ */
+
+
+ Buffer.allocUnsafeSlow = function (size) {
+ return allocUnsafe(size);
+ };
+
+ function fromString(string, encoding) {
+ if (typeof encoding !== 'string' || encoding === '') {
+ encoding = 'utf8';
+ }
+
+ if (!Buffer.isEncoding(encoding)) {
+ throw new TypeError('Unknown encoding: ' + encoding);
+ }
+
+ var length = byteLength(string, encoding) | 0;
+ var buf = createBuffer(length);
+ var actual = buf.write(string, encoding);
+
+ if (actual !== length) {
+ // Writing a hex string, for example, that contains invalid characters will
+ // cause everything after the first invalid character to be ignored. (e.g.
+ // 'abxxcd' will be treated as 'ab')
+ buf = buf.slice(0, actual);
+ }
+
+ return buf;
+ }
+
+ function fromArrayLike(array) {
+ var length = array.length < 0 ? 0 : checked(array.length) | 0;
+ var buf = createBuffer(length);
+
+ for (var i = 0; i < length; i += 1) {
+ buf[i] = array[i] & 255;
+ }
+
+ return buf;
+ }
+
+ function fromArrayView(arrayView) {
+ if (isInstance(arrayView, Uint8Array)) {
+ var copy = new Uint8Array(arrayView);
+ return fromArrayBuffer(copy.buffer, copy.byteOffset, copy.byteLength);
+ }
+
+ return fromArrayLike(arrayView);
+ }
+
+ function fromArrayBuffer(array, byteOffset, length) {
+ if (byteOffset < 0 || array.byteLength < byteOffset) {
+ throw new RangeError('"offset" is outside of buffer bounds');
+ }
+
+ if (array.byteLength < byteOffset + (length || 0)) {
+ throw new RangeError('"length" is outside of buffer bounds');
+ }
+
+ var buf;
+
+ if (byteOffset === undefined && length === undefined) {
+ buf = new Uint8Array(array);
+ } else if (length === undefined) {
+ buf = new Uint8Array(array, byteOffset);
+ } else {
+ buf = new Uint8Array(array, byteOffset, length);
+ } // Return an augmented `Uint8Array` instance
+
+
+ Object.setPrototypeOf(buf, Buffer.prototype);
+ return buf;
+ }
+
+ function fromObject(obj) {
+ if (Buffer.isBuffer(obj)) {
+ var len = checked(obj.length) | 0;
+ var buf = createBuffer(len);
+
+ if (buf.length === 0) {
+ return buf;
+ }
+
+ obj.copy(buf, 0, 0, len);
+ return buf;
+ }
+
+ if (obj.length !== undefined) {
+ if (typeof obj.length !== 'number' || numberIsNaN(obj.length)) {
+ return createBuffer(0);
+ }
+
+ return fromArrayLike(obj);
+ }
+
+ if (obj.type === 'Buffer' && Array.isArray(obj.data)) {
+ return fromArrayLike(obj.data);
+ }
+ }
+
+ function checked(length) {
+ // Note: cannot use `length < K_MAX_LENGTH` here because that fails when
+ // length is NaN (which is otherwise coerced to zero.)
+ if (length >= K_MAX_LENGTH) {
+ throw new RangeError('Attempt to allocate Buffer larger than maximum ' + 'size: 0x' + K_MAX_LENGTH.toString(16) + ' bytes');
+ }
+
+ return length | 0;
+ }
+
+ function SlowBuffer(length) {
+ if (+length != length) {
+ // eslint-disable-line eqeqeq
+ length = 0;
+ }
+
+ return Buffer.alloc(+length);
+ }
+
+ Buffer.isBuffer = function isBuffer(b) {
+ return b != null && b._isBuffer === true && b !== Buffer.prototype; // so Buffer.isBuffer(Buffer.prototype) will be false
+ };
+
+ Buffer.compare = function compare(a, b) {
+ if (isInstance(a, Uint8Array)) a = Buffer.from(a, a.offset, a.byteLength);
+ if (isInstance(b, Uint8Array)) b = Buffer.from(b, b.offset, b.byteLength);
+
+ if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {
+ throw new TypeError('The "buf1", "buf2" arguments must be one of type Buffer or Uint8Array');
+ }
+
+ if (a === b) return 0;
+ var x = a.length;
+ var y = b.length;
+
+ for (var i = 0, len = Math.min(x, y); i < len; ++i) {
+ if (a[i] !== b[i]) {
+ x = a[i];
+ y = b[i];
+ break;
+ }
+ }
+
+ if (x < y) return -1;
+ if (y < x) return 1;
+ return 0;
+ };
+
+ Buffer.isEncoding = function isEncoding(encoding) {
+ switch (String(encoding).toLowerCase()) {
+ case 'hex':
+ case 'utf8':
+ case 'utf-8':
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ case 'base64':
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return true;
+
+ default:
+ return false;
+ }
+ };
+
+ Buffer.concat = function concat(list, length) {
+ if (!Array.isArray(list)) {
+ throw new TypeError('"list" argument must be an Array of Buffers');
+ }
+
+ if (list.length === 0) {
+ return Buffer.alloc(0);
+ }
+
+ var i;
+
+ if (length === undefined) {
+ length = 0;
+
+ for (i = 0; i < list.length; ++i) {
+ length += list[i].length;
+ }
+ }
+
+ var buffer = Buffer.allocUnsafe(length);
+ var pos = 0;
+
+ for (i = 0; i < list.length; ++i) {
+ var buf = list[i];
+
+ if (isInstance(buf, Uint8Array)) {
+ if (pos + buf.length > buffer.length) {
+ Buffer.from(buf).copy(buffer, pos);
+ } else {
+ Uint8Array.prototype.set.call(buffer, buf, pos);
+ }
+ } else if (!Buffer.isBuffer(buf)) {
+ throw new TypeError('"list" argument must be an Array of Buffers');
+ } else {
+ buf.copy(buffer, pos);
+ }
+
+ pos += buf.length;
+ }
+
+ return buffer;
+ };
+
+ function byteLength(string, encoding) {
+ if (Buffer.isBuffer(string)) {
+ return string.length;
+ }
+
+ if (ArrayBuffer.isView(string) || isInstance(string, ArrayBuffer)) {
+ return string.byteLength;
+ }
+
+ if (typeof string !== 'string') {
+ throw new TypeError('The "string" argument must be one of type string, Buffer, or ArrayBuffer. ' + 'Received type ' + babelHelpers["typeof"](string));
+ }
+
+ var len = string.length;
+ var mustMatch = arguments.length > 2 && arguments[2] === true;
+ if (!mustMatch && len === 0) return 0; // Use a for loop to avoid recursion
+
+ var loweredCase = false;
+
+ for (;;) {
+ switch (encoding) {
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ return len;
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8ToBytes(string).length;
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return len * 2;
+
+ case 'hex':
+ return len >>> 1;
+
+ case 'base64':
+ return base64ToBytes(string).length;
+
+ default:
+ if (loweredCase) {
+ return mustMatch ? -1 : utf8ToBytes(string).length; // assume utf8
+ }
+
+ encoding = ('' + encoding).toLowerCase();
+ loweredCase = true;
+ }
+ }
+ }
+
+ Buffer.byteLength = byteLength;
+
+ function slowToString(encoding, start, end) {
+ var loweredCase = false; // No need to verify that "this.length <= MAX_UINT32" since it's a read-only
+ // property of a typed array.
+ // This behaves neither like String nor Uint8Array in that we set start/end
+ // to their upper/lower bounds if the value passed is out of range.
+ // undefined is handled specially as per ECMA-262 6th Edition,
+ // Section 13.3.3.7 Runtime Semantics: KeyedBindingInitialization.
+
+ if (start === undefined || start < 0) {
+ start = 0;
+ } // Return early if start > this.length. Done here to prevent potential uint32
+ // coercion fail below.
+
+
+ if (start > this.length) {
+ return '';
+ }
+
+ if (end === undefined || end > this.length) {
+ end = this.length;
+ }
+
+ if (end <= 0) {
+ return '';
+ } // Force coercion to uint32. This will also coerce falsey/NaN values to 0.
+
+
+ end >>>= 0;
+ start >>>= 0;
+
+ if (end <= start) {
+ return '';
+ }
+
+ if (!encoding) encoding = 'utf8';
+
+ while (true) {
+ switch (encoding) {
+ case 'hex':
+ return hexSlice(this, start, end);
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Slice(this, start, end);
+
+ case 'ascii':
+ return asciiSlice(this, start, end);
+
+ case 'latin1':
+ case 'binary':
+ return latin1Slice(this, start, end);
+
+ case 'base64':
+ return base64Slice(this, start, end);
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return utf16leSlice(this, start, end);
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding);
+ encoding = (encoding + '').toLowerCase();
+ loweredCase = true;
+ }
+ }
+ } // This property is used by `Buffer.isBuffer` (and the `is-buffer` npm package)
+ // to detect a Buffer instance. It's not possible to use `instanceof Buffer`
+ // reliably in a browserify context because there could be multiple different
+ // copies of the 'buffer' package in use. This method works even for Buffer
+ // instances that were created from another copy of the `buffer` package.
+ // See: https://github.com/feross/buffer/issues/154
+
+
+ Buffer.prototype._isBuffer = true;
+
+ function swap(b, n, m) {
+ var i = b[n];
+ b[n] = b[m];
+ b[m] = i;
+ }
+
+ Buffer.prototype.swap16 = function swap16() {
+ var len = this.length;
+
+ if (len % 2 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 16-bits');
+ }
+
+ for (var i = 0; i < len; i += 2) {
+ swap(this, i, i + 1);
+ }
+
+ return this;
+ };
+
+ Buffer.prototype.swap32 = function swap32() {
+ var len = this.length;
+
+ if (len % 4 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 32-bits');
+ }
+
+ for (var i = 0; i < len; i += 4) {
+ swap(this, i, i + 3);
+ swap(this, i + 1, i + 2);
+ }
+
+ return this;
+ };
+
+ Buffer.prototype.swap64 = function swap64() {
+ var len = this.length;
+
+ if (len % 8 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 64-bits');
+ }
+
+ for (var i = 0; i < len; i += 8) {
+ swap(this, i, i + 7);
+ swap(this, i + 1, i + 6);
+ swap(this, i + 2, i + 5);
+ swap(this, i + 3, i + 4);
+ }
+
+ return this;
+ };
+
+ Buffer.prototype.toString = function toString() {
+ var length = this.length;
+ if (length === 0) return '';
+ if (arguments.length === 0) return utf8Slice(this, 0, length);
+ return slowToString.apply(this, arguments);
+ };
+
+ Buffer.prototype.toLocaleString = Buffer.prototype.toString;
+
+ Buffer.prototype.equals = function equals(b) {
+ if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer');
+ if (this === b) return true;
+ return Buffer.compare(this, b) === 0;
+ };
+
+ Buffer.prototype.inspect = function inspect() {
+ var str = '';
+ var max = exports.INSPECT_MAX_BYTES;
+ str = this.toString('hex', 0, max).replace(/(.{2})/g, '$1 ').trim();
+ if (this.length > max) str += ' ... ';
+ return '';
+ };
+
+ if (customInspectSymbol) {
+ Buffer.prototype[customInspectSymbol] = Buffer.prototype.inspect;
+ }
+
+ Buffer.prototype.compare = function compare(target, start, end, thisStart, thisEnd) {
+ if (isInstance(target, Uint8Array)) {
+ target = Buffer.from(target, target.offset, target.byteLength);
+ }
+
+ if (!Buffer.isBuffer(target)) {
+ throw new TypeError('The "target" argument must be one of type Buffer or Uint8Array. ' + 'Received type ' + babelHelpers["typeof"](target));
+ }
+
+ if (start === undefined) {
+ start = 0;
+ }
+
+ if (end === undefined) {
+ end = target ? target.length : 0;
+ }
+
+ if (thisStart === undefined) {
+ thisStart = 0;
+ }
+
+ if (thisEnd === undefined) {
+ thisEnd = this.length;
+ }
+
+ if (start < 0 || end > target.length || thisStart < 0 || thisEnd > this.length) {
+ throw new RangeError('out of range index');
+ }
+
+ if (thisStart >= thisEnd && start >= end) {
+ return 0;
+ }
+
+ if (thisStart >= thisEnd) {
+ return -1;
+ }
+
+ if (start >= end) {
+ return 1;
+ }
+
+ start >>>= 0;
+ end >>>= 0;
+ thisStart >>>= 0;
+ thisEnd >>>= 0;
+ if (this === target) return 0;
+ var x = thisEnd - thisStart;
+ var y = end - start;
+ var len = Math.min(x, y);
+ var thisCopy = this.slice(thisStart, thisEnd);
+ var targetCopy = target.slice(start, end);
+
+ for (var i = 0; i < len; ++i) {
+ if (thisCopy[i] !== targetCopy[i]) {
+ x = thisCopy[i];
+ y = targetCopy[i];
+ break;
+ }
+ }
+
+ if (x < y) return -1;
+ if (y < x) return 1;
+ return 0;
+ }; // Finds either the first index of `val` in `buffer` at offset >= `byteOffset`,
+ // OR the last index of `val` in `buffer` at offset <= `byteOffset`.
+ //
+ // Arguments:
+ // - buffer - a Buffer to search
+ // - val - a string, Buffer, or number
+ // - byteOffset - an index into `buffer`; will be clamped to an int32
+ // - encoding - an optional encoding, relevant is val is a string
+ // - dir - true for indexOf, false for lastIndexOf
+
+
+ function bidirectionalIndexOf(buffer, val, byteOffset, encoding, dir) {
+ // Empty buffer means no match
+ if (buffer.length === 0) return -1; // Normalize byteOffset
+
+ if (typeof byteOffset === 'string') {
+ encoding = byteOffset;
+ byteOffset = 0;
+ } else if (byteOffset > 0x7fffffff) {
+ byteOffset = 0x7fffffff;
+ } else if (byteOffset < -0x80000000) {
+ byteOffset = -0x80000000;
+ }
+
+ byteOffset = +byteOffset; // Coerce to Number.
+
+ if (numberIsNaN(byteOffset)) {
+ // byteOffset: it it's undefined, null, NaN, "foo", etc, search whole buffer
+ byteOffset = dir ? 0 : buffer.length - 1;
+ } // Normalize byteOffset: negative offsets start from the end of the buffer
+
+
+ if (byteOffset < 0) byteOffset = buffer.length + byteOffset;
+
+ if (byteOffset >= buffer.length) {
+ if (dir) return -1;else byteOffset = buffer.length - 1;
+ } else if (byteOffset < 0) {
+ if (dir) byteOffset = 0;else return -1;
+ } // Normalize val
+
+
+ if (typeof val === 'string') {
+ val = Buffer.from(val, encoding);
+ } // Finally, search either indexOf (if dir is true) or lastIndexOf
+
+
+ if (Buffer.isBuffer(val)) {
+ // Special case: looking for empty string/buffer always fails
+ if (val.length === 0) {
+ return -1;
+ }
+
+ return arrayIndexOf(buffer, val, byteOffset, encoding, dir);
+ } else if (typeof val === 'number') {
+ val = val & 0xFF; // Search for a byte value [0-255]
+
+ if (typeof Uint8Array.prototype.indexOf === 'function') {
+ if (dir) {
+ return Uint8Array.prototype.indexOf.call(buffer, val, byteOffset);
+ } else {
+ return Uint8Array.prototype.lastIndexOf.call(buffer, val, byteOffset);
+ }
+ }
+
+ return arrayIndexOf(buffer, [val], byteOffset, encoding, dir);
+ }
+
+ throw new TypeError('val must be string, number or Buffer');
+ }
+
+ function arrayIndexOf(arr, val, byteOffset, encoding, dir) {
+ var indexSize = 1;
+ var arrLength = arr.length;
+ var valLength = val.length;
+
+ if (encoding !== undefined) {
+ encoding = String(encoding).toLowerCase();
+
+ if (encoding === 'ucs2' || encoding === 'ucs-2' || encoding === 'utf16le' || encoding === 'utf-16le') {
+ if (arr.length < 2 || val.length < 2) {
+ return -1;
+ }
+
+ indexSize = 2;
+ arrLength /= 2;
+ valLength /= 2;
+ byteOffset /= 2;
+ }
+ }
+
+ function read(buf, i) {
+ if (indexSize === 1) {
+ return buf[i];
+ } else {
+ return buf.readUInt16BE(i * indexSize);
+ }
+ }
+
+ var i;
+
+ if (dir) {
+ var foundIndex = -1;
+
+ for (i = byteOffset; i < arrLength; i++) {
+ if (read(arr, i) === read(val, foundIndex === -1 ? 0 : i - foundIndex)) {
+ if (foundIndex === -1) foundIndex = i;
+ if (i - foundIndex + 1 === valLength) return foundIndex * indexSize;
+ } else {
+ if (foundIndex !== -1) i -= i - foundIndex;
+ foundIndex = -1;
+ }
+ }
+ } else {
+ if (byteOffset + valLength > arrLength) byteOffset = arrLength - valLength;
+
+ for (i = byteOffset; i >= 0; i--) {
+ var found = true;
+
+ for (var j = 0; j < valLength; j++) {
+ if (read(arr, i + j) !== read(val, j)) {
+ found = false;
+ break;
+ }
+ }
+
+ if (found) return i;
+ }
+ }
+
+ return -1;
+ }
+
+ Buffer.prototype.includes = function includes(val, byteOffset, encoding) {
+ return this.indexOf(val, byteOffset, encoding) !== -1;
+ };
+
+ Buffer.prototype.indexOf = function indexOf(val, byteOffset, encoding) {
+ return bidirectionalIndexOf(this, val, byteOffset, encoding, true);
+ };
+
+ Buffer.prototype.lastIndexOf = function lastIndexOf(val, byteOffset, encoding) {
+ return bidirectionalIndexOf(this, val, byteOffset, encoding, false);
+ };
+
+ function hexWrite(buf, string, offset, length) {
+ offset = Number(offset) || 0;
+ var remaining = buf.length - offset;
+
+ if (!length) {
+ length = remaining;
+ } else {
+ length = Number(length);
+
+ if (length > remaining) {
+ length = remaining;
+ }
+ }
+
+ var strLen = string.length;
+
+ if (length > strLen / 2) {
+ length = strLen / 2;
+ }
+
+ for (var i = 0; i < length; ++i) {
+ var parsed = parseInt(string.substr(i * 2, 2), 16);
+ if (numberIsNaN(parsed)) return i;
+ buf[offset + i] = parsed;
+ }
+
+ return i;
+ }
+
+ function utf8Write(buf, string, offset, length) {
+ return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length);
+ }
+
+ function asciiWrite(buf, string, offset, length) {
+ return blitBuffer(asciiToBytes(string), buf, offset, length);
+ }
+
+ function base64Write(buf, string, offset, length) {
+ return blitBuffer(base64ToBytes(string), buf, offset, length);
+ }
+
+ function ucs2Write(buf, string, offset, length) {
+ return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length);
+ }
+
+ Buffer.prototype.write = function write(string, offset, length, encoding) {
+ // Buffer#write(string)
+ if (offset === undefined) {
+ encoding = 'utf8';
+ length = this.length;
+ offset = 0; // Buffer#write(string, encoding)
+ } else if (length === undefined && typeof offset === 'string') {
+ encoding = offset;
+ length = this.length;
+ offset = 0; // Buffer#write(string, offset[, length][, encoding])
+ } else if (isFinite(offset)) {
+ offset = offset >>> 0;
+
+ if (isFinite(length)) {
+ length = length >>> 0;
+ if (encoding === undefined) encoding = 'utf8';
+ } else {
+ encoding = length;
+ length = undefined;
+ }
+ } else {
+ throw new Error('Buffer.write(string, encoding, offset[, length]) is no longer supported');
+ }
+
+ var remaining = this.length - offset;
+ if (length === undefined || length > remaining) length = remaining;
+
+ if (string.length > 0 && (length < 0 || offset < 0) || offset > this.length) {
+ throw new RangeError('Attempt to write outside buffer bounds');
+ }
+
+ if (!encoding) encoding = 'utf8';
+ var loweredCase = false;
+
+ for (;;) {
+ switch (encoding) {
+ case 'hex':
+ return hexWrite(this, string, offset, length);
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Write(this, string, offset, length);
+
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ return asciiWrite(this, string, offset, length);
+
+ case 'base64':
+ // Warning: maxLength not taken into account in base64Write
+ return base64Write(this, string, offset, length);
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return ucs2Write(this, string, offset, length);
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding);
+ encoding = ('' + encoding).toLowerCase();
+ loweredCase = true;
+ }
+ }
+ };
+
+ Buffer.prototype.toJSON = function toJSON() {
+ return {
+ type: 'Buffer',
+ data: Array.prototype.slice.call(this._arr || this, 0)
+ };
+ };
+
+ function base64Slice(buf, start, end) {
+ if (start === 0 && end === buf.length) {
+ return base64Js.fromByteArray(buf);
+ } else {
+ return base64Js.fromByteArray(buf.slice(start, end));
+ }
+ }
+
+ function utf8Slice(buf, start, end) {
+ end = Math.min(buf.length, end);
+ var res = [];
+ var i = start;
+
+ while (i < end) {
+ var firstByte = buf[i];
+ var codePoint = null;
+ var bytesPerSequence = firstByte > 0xEF ? 4 : firstByte > 0xDF ? 3 : firstByte > 0xBF ? 2 : 1;
+
+ if (i + bytesPerSequence <= end) {
+ var secondByte, thirdByte, fourthByte, tempCodePoint;
+
+ switch (bytesPerSequence) {
+ case 1:
+ if (firstByte < 0x80) {
+ codePoint = firstByte;
+ }
+
+ break;
+
+ case 2:
+ secondByte = buf[i + 1];
+
+ if ((secondByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0x1F) << 0x6 | secondByte & 0x3F;
+
+ if (tempCodePoint > 0x7F) {
+ codePoint = tempCodePoint;
+ }
+ }
+
+ break;
+
+ case 3:
+ secondByte = buf[i + 1];
+ thirdByte = buf[i + 2];
+
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | thirdByte & 0x3F;
+
+ if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {
+ codePoint = tempCodePoint;
+ }
+ }
+
+ break;
+
+ case 4:
+ secondByte = buf[i + 1];
+ thirdByte = buf[i + 2];
+ fourthByte = buf[i + 3];
+
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | fourthByte & 0x3F;
+
+ if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {
+ codePoint = tempCodePoint;
+ }
+ }
+
+ }
+ }
+
+ if (codePoint === null) {
+ // we did not generate a valid codePoint so insert a
+ // replacement char (U+FFFD) and advance only 1 byte
+ codePoint = 0xFFFD;
+ bytesPerSequence = 1;
+ } else if (codePoint > 0xFFFF) {
+ // encode to utf16 (surrogate pair dance)
+ codePoint -= 0x10000;
+ res.push(codePoint >>> 10 & 0x3FF | 0xD800);
+ codePoint = 0xDC00 | codePoint & 0x3FF;
+ }
+
+ res.push(codePoint);
+ i += bytesPerSequence;
+ }
+
+ return decodeCodePointsArray(res);
+ } // Based on http://stackoverflow.com/a/22747272/680742, the browser with
+ // the lowest limit is Chrome, with 0x10000 args.
+ // We go 1 magnitude less, for safety
+
+
+ var MAX_ARGUMENTS_LENGTH = 0x1000;
+
+ function decodeCodePointsArray(codePoints) {
+ var len = codePoints.length;
+
+ if (len <= MAX_ARGUMENTS_LENGTH) {
+ return String.fromCharCode.apply(String, codePoints); // avoid extra slice()
+ } // Decode in chunks to avoid "call stack size exceeded".
+
+
+ var res = '';
+ var i = 0;
+
+ while (i < len) {
+ res += String.fromCharCode.apply(String, codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH));
+ }
+
+ return res;
+ }
+
+ function asciiSlice(buf, start, end) {
+ var ret = '';
+ end = Math.min(buf.length, end);
+
+ for (var i = start; i < end; ++i) {
+ ret += String.fromCharCode(buf[i] & 0x7F);
+ }
+
+ return ret;
+ }
+
+ function latin1Slice(buf, start, end) {
+ var ret = '';
+ end = Math.min(buf.length, end);
+
+ for (var i = start; i < end; ++i) {
+ ret += String.fromCharCode(buf[i]);
+ }
+
+ return ret;
+ }
+
+ function hexSlice(buf, start, end) {
+ var len = buf.length;
+ if (!start || start < 0) start = 0;
+ if (!end || end < 0 || end > len) end = len;
+ var out = '';
+
+ for (var i = start; i < end; ++i) {
+ out += hexSliceLookupTable[buf[i]];
+ }
+
+ return out;
+ }
+
+ function utf16leSlice(buf, start, end) {
+ var bytes = buf.slice(start, end);
+ var res = ''; // If bytes.length is odd, the last 8 bits must be ignored (same as node.js)
+
+ for (var i = 0; i < bytes.length - 1; i += 2) {
+ res += String.fromCharCode(bytes[i] + bytes[i + 1] * 256);
+ }
+
+ return res;
+ }
+
+ Buffer.prototype.slice = function slice(start, end) {
+ var len = this.length;
+ start = ~~start;
+ end = end === undefined ? len : ~~end;
+
+ if (start < 0) {
+ start += len;
+ if (start < 0) start = 0;
+ } else if (start > len) {
+ start = len;
+ }
+
+ if (end < 0) {
+ end += len;
+ if (end < 0) end = 0;
+ } else if (end > len) {
+ end = len;
+ }
+
+ if (end < start) end = start;
+ var newBuf = this.subarray(start, end); // Return an augmented `Uint8Array` instance
+
+ Object.setPrototypeOf(newBuf, Buffer.prototype);
+ return newBuf;
+ };
+ /*
+ * Need to make sure that buffer isn't trying to write out of bounds.
+ */
+
+
+ function checkOffset(offset, ext, length) {
+ if (offset % 1 !== 0 || offset < 0) throw new RangeError('offset is not uint');
+ if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length');
+ }
+
+ Buffer.prototype.readUintLE = Buffer.prototype.readUIntLE = function readUIntLE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+ if (!noAssert) checkOffset(offset, byteLength, this.length);
+ var val = this[offset];
+ var mul = 1;
+ var i = 0;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul;
+ }
+
+ return val;
+ };
+
+ Buffer.prototype.readUintBE = Buffer.prototype.readUIntBE = function readUIntBE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+
+ if (!noAssert) {
+ checkOffset(offset, byteLength, this.length);
+ }
+
+ var val = this[offset + --byteLength];
+ var mul = 1;
+
+ while (byteLength > 0 && (mul *= 0x100)) {
+ val += this[offset + --byteLength] * mul;
+ }
+
+ return val;
+ };
+
+ Buffer.prototype.readUint8 = Buffer.prototype.readUInt8 = function readUInt8(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 1, this.length);
+ return this[offset];
+ };
+
+ Buffer.prototype.readUint16LE = Buffer.prototype.readUInt16LE = function readUInt16LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ return this[offset] | this[offset + 1] << 8;
+ };
+
+ Buffer.prototype.readUint16BE = Buffer.prototype.readUInt16BE = function readUInt16BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ return this[offset] << 8 | this[offset + 1];
+ };
+
+ Buffer.prototype.readUint32LE = Buffer.prototype.readUInt32LE = function readUInt32LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return (this[offset] | this[offset + 1] << 8 | this[offset + 2] << 16) + this[offset + 3] * 0x1000000;
+ };
+
+ Buffer.prototype.readUint32BE = Buffer.prototype.readUInt32BE = function readUInt32BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return this[offset] * 0x1000000 + (this[offset + 1] << 16 | this[offset + 2] << 8 | this[offset + 3]);
+ };
+
+ Buffer.prototype.readIntLE = function readIntLE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+ if (!noAssert) checkOffset(offset, byteLength, this.length);
+ var val = this[offset];
+ var mul = 1;
+ var i = 0;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul;
+ }
+
+ mul *= 0x80;
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength);
+ return val;
+ };
+
+ Buffer.prototype.readIntBE = function readIntBE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+ if (!noAssert) checkOffset(offset, byteLength, this.length);
+ var i = byteLength;
+ var mul = 1;
+ var val = this[offset + --i];
+
+ while (i > 0 && (mul *= 0x100)) {
+ val += this[offset + --i] * mul;
+ }
+
+ mul *= 0x80;
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength);
+ return val;
+ };
+
+ Buffer.prototype.readInt8 = function readInt8(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 1, this.length);
+ if (!(this[offset] & 0x80)) return this[offset];
+ return (0xff - this[offset] + 1) * -1;
+ };
+
+ Buffer.prototype.readInt16LE = function readInt16LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ var val = this[offset] | this[offset + 1] << 8;
+ return val & 0x8000 ? val | 0xFFFF0000 : val;
+ };
+
+ Buffer.prototype.readInt16BE = function readInt16BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ var val = this[offset + 1] | this[offset] << 8;
+ return val & 0x8000 ? val | 0xFFFF0000 : val;
+ };
+
+ Buffer.prototype.readInt32LE = function readInt32LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return this[offset] | this[offset + 1] << 8 | this[offset + 2] << 16 | this[offset + 3] << 24;
+ };
+
+ Buffer.prototype.readInt32BE = function readInt32BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return this[offset] << 24 | this[offset + 1] << 16 | this[offset + 2] << 8 | this[offset + 3];
+ };
+
+ Buffer.prototype.readFloatLE = function readFloatLE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return ieee754.read(this, offset, true, 23, 4);
+ };
+
+ Buffer.prototype.readFloatBE = function readFloatBE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return ieee754.read(this, offset, false, 23, 4);
+ };
+
+ Buffer.prototype.readDoubleLE = function readDoubleLE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 8, this.length);
+ return ieee754.read(this, offset, true, 52, 8);
+ };
+
+ Buffer.prototype.readDoubleBE = function readDoubleBE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 8, this.length);
+ return ieee754.read(this, offset, false, 52, 8);
+ };
+
+ function checkInt(buf, value, offset, ext, max, min) {
+ if (!Buffer.isBuffer(buf)) throw new TypeError('"buffer" argument must be a Buffer instance');
+ if (value > max || value < min) throw new RangeError('"value" argument is out of bounds');
+ if (offset + ext > buf.length) throw new RangeError('Index out of range');
+ }
+
+ Buffer.prototype.writeUintLE = Buffer.prototype.writeUIntLE = function writeUIntLE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+
+ if (!noAssert) {
+ var maxBytes = Math.pow(2, 8 * byteLength) - 1;
+ checkInt(this, value, offset, byteLength, maxBytes, 0);
+ }
+
+ var mul = 1;
+ var i = 0;
+ this[offset] = value & 0xFF;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ this[offset + i] = value / mul & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeUintBE = Buffer.prototype.writeUIntBE = function writeUIntBE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+
+ if (!noAssert) {
+ var maxBytes = Math.pow(2, 8 * byteLength) - 1;
+ checkInt(this, value, offset, byteLength, maxBytes, 0);
+ }
+
+ var i = byteLength - 1;
+ var mul = 1;
+ this[offset + i] = value & 0xFF;
+
+ while (--i >= 0 && (mul *= 0x100)) {
+ this[offset + i] = value / mul & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeUint8 = Buffer.prototype.writeUInt8 = function writeUInt8(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0);
+ this[offset] = value & 0xff;
+ return offset + 1;
+ };
+
+ Buffer.prototype.writeUint16LE = Buffer.prototype.writeUInt16LE = function writeUInt16LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0);
+ this[offset] = value & 0xff;
+ this[offset + 1] = value >>> 8;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeUint16BE = Buffer.prototype.writeUInt16BE = function writeUInt16BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0);
+ this[offset] = value >>> 8;
+ this[offset + 1] = value & 0xff;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeUint32LE = Buffer.prototype.writeUInt32LE = function writeUInt32LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0);
+ this[offset + 3] = value >>> 24;
+ this[offset + 2] = value >>> 16;
+ this[offset + 1] = value >>> 8;
+ this[offset] = value & 0xff;
+ return offset + 4;
+ };
+
+ Buffer.prototype.writeUint32BE = Buffer.prototype.writeUInt32BE = function writeUInt32BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0);
+ this[offset] = value >>> 24;
+ this[offset + 1] = value >>> 16;
+ this[offset + 2] = value >>> 8;
+ this[offset + 3] = value & 0xff;
+ return offset + 4;
+ };
+
+ Buffer.prototype.writeIntLE = function writeIntLE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ var limit = Math.pow(2, 8 * byteLength - 1);
+ checkInt(this, value, offset, byteLength, limit - 1, -limit);
+ }
+
+ var i = 0;
+ var mul = 1;
+ var sub = 0;
+ this[offset] = value & 0xFF;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ if (value < 0 && sub === 0 && this[offset + i - 1] !== 0) {
+ sub = 1;
+ }
+
+ this[offset + i] = (value / mul >> 0) - sub & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeIntBE = function writeIntBE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ var limit = Math.pow(2, 8 * byteLength - 1);
+ checkInt(this, value, offset, byteLength, limit - 1, -limit);
+ }
+
+ var i = byteLength - 1;
+ var mul = 1;
+ var sub = 0;
+ this[offset + i] = value & 0xFF;
+
+ while (--i >= 0 && (mul *= 0x100)) {
+ if (value < 0 && sub === 0 && this[offset + i + 1] !== 0) {
+ sub = 1;
+ }
+
+ this[offset + i] = (value / mul >> 0) - sub & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeInt8 = function writeInt8(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80);
+ if (value < 0) value = 0xff + value + 1;
+ this[offset] = value & 0xff;
+ return offset + 1;
+ };
+
+ Buffer.prototype.writeInt16LE = function writeInt16LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000);
+ this[offset] = value & 0xff;
+ this[offset + 1] = value >>> 8;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeInt16BE = function writeInt16BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000);
+ this[offset] = value >>> 8;
+ this[offset + 1] = value & 0xff;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeInt32LE = function writeInt32LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000);
+ this[offset] = value & 0xff;
+ this[offset + 1] = value >>> 8;
+ this[offset + 2] = value >>> 16;
+ this[offset + 3] = value >>> 24;
+ return offset + 4;
+ };
+
+ Buffer.prototype.writeInt32BE = function writeInt32BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000);
+ if (value < 0) value = 0xffffffff + value + 1;
+ this[offset] = value >>> 24;
+ this[offset + 1] = value >>> 16;
+ this[offset + 2] = value >>> 8;
+ this[offset + 3] = value & 0xff;
+ return offset + 4;
+ };
+
+ function checkIEEE754(buf, value, offset, ext, max, min) {
+ if (offset + ext > buf.length) throw new RangeError('Index out of range');
+ if (offset < 0) throw new RangeError('Index out of range');
+ }
+
+ function writeFloat(buf, value, offset, littleEndian, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 4);
+ }
+
+ ieee754.write(buf, value, offset, littleEndian, 23, 4);
+ return offset + 4;
+ }
+
+ Buffer.prototype.writeFloatLE = function writeFloatLE(value, offset, noAssert) {
+ return writeFloat(this, value, offset, true, noAssert);
+ };
+
+ Buffer.prototype.writeFloatBE = function writeFloatBE(value, offset, noAssert) {
+ return writeFloat(this, value, offset, false, noAssert);
+ };
+
+ function writeDouble(buf, value, offset, littleEndian, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 8);
+ }
+
+ ieee754.write(buf, value, offset, littleEndian, 52, 8);
+ return offset + 8;
+ }
+
+ Buffer.prototype.writeDoubleLE = function writeDoubleLE(value, offset, noAssert) {
+ return writeDouble(this, value, offset, true, noAssert);
+ };
+
+ Buffer.prototype.writeDoubleBE = function writeDoubleBE(value, offset, noAssert) {
+ return writeDouble(this, value, offset, false, noAssert);
+ }; // copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)
+
+
+ Buffer.prototype.copy = function copy(target, targetStart, start, end) {
+ if (!Buffer.isBuffer(target)) throw new TypeError('argument should be a Buffer');
+ if (!start) start = 0;
+ if (!end && end !== 0) end = this.length;
+ if (targetStart >= target.length) targetStart = target.length;
+ if (!targetStart) targetStart = 0;
+ if (end > 0 && end < start) end = start; // Copy 0 bytes; we're done
+
+ if (end === start) return 0;
+ if (target.length === 0 || this.length === 0) return 0; // Fatal error conditions
+
+ if (targetStart < 0) {
+ throw new RangeError('targetStart out of bounds');
+ }
+
+ if (start < 0 || start >= this.length) throw new RangeError('Index out of range');
+ if (end < 0) throw new RangeError('sourceEnd out of bounds'); // Are we oob?
+
+ if (end > this.length) end = this.length;
+
+ if (target.length - targetStart < end - start) {
+ end = target.length - targetStart + start;
+ }
+
+ var len = end - start;
+
+ if (this === target && typeof Uint8Array.prototype.copyWithin === 'function') {
+ // Use built-in when available, missing from IE11
+ this.copyWithin(targetStart, start, end);
+ } else {
+ Uint8Array.prototype.set.call(target, this.subarray(start, end), targetStart);
+ }
+
+ return len;
+ }; // Usage:
+ // buffer.fill(number[, offset[, end]])
+ // buffer.fill(buffer[, offset[, end]])
+ // buffer.fill(string[, offset[, end]][, encoding])
+
+
+ Buffer.prototype.fill = function fill(val, start, end, encoding) {
+ // Handle string cases:
+ if (typeof val === 'string') {
+ if (typeof start === 'string') {
+ encoding = start;
+ start = 0;
+ end = this.length;
+ } else if (typeof end === 'string') {
+ encoding = end;
+ end = this.length;
+ }
+
+ if (encoding !== undefined && typeof encoding !== 'string') {
+ throw new TypeError('encoding must be a string');
+ }
+
+ if (typeof encoding === 'string' && !Buffer.isEncoding(encoding)) {
+ throw new TypeError('Unknown encoding: ' + encoding);
+ }
+
+ if (val.length === 1) {
+ var code = val.charCodeAt(0);
+
+ if (encoding === 'utf8' && code < 128 || encoding === 'latin1') {
+ // Fast path: If `val` fits into a single byte, use that numeric value.
+ val = code;
+ }
+ }
+ } else if (typeof val === 'number') {
+ val = val & 255;
+ } else if (typeof val === 'boolean') {
+ val = Number(val);
+ } // Invalid ranges are not set to a default, so can range check early.
+
+
+ if (start < 0 || this.length < start || this.length < end) {
+ throw new RangeError('Out of range index');
+ }
+
+ if (end <= start) {
+ return this;
+ }
+
+ start = start >>> 0;
+ end = end === undefined ? this.length : end >>> 0;
+ if (!val) val = 0;
+ var i;
+
+ if (typeof val === 'number') {
+ for (i = start; i < end; ++i) {
+ this[i] = val;
+ }
+ } else {
+ var bytes = Buffer.isBuffer(val) ? val : Buffer.from(val, encoding);
+ var len = bytes.length;
+
+ if (len === 0) {
+ throw new TypeError('The value "' + val + '" is invalid for argument "value"');
+ }
+
+ for (i = 0; i < end - start; ++i) {
+ this[i + start] = bytes[i % len];
+ }
+ }
+
+ return this;
+ }; // HELPER FUNCTIONS
+ // ================
+
+
+ var INVALID_BASE64_RE = /[^+/0-9A-Za-z-_]/g;
+
+ function base64clean(str) {
+ // Node takes equal signs as end of the Base64 encoding
+ str = str.split('=')[0]; // Node strips out invalid characters like \n and \t from the string, base64-js does not
+
+ str = str.trim().replace(INVALID_BASE64_RE, ''); // Node converts strings with length < 2 to ''
+
+ if (str.length < 2) return ''; // Node allows for non-padded base64 strings (missing trailing ===), base64-js does not
+
+ while (str.length % 4 !== 0) {
+ str = str + '=';
+ }
+
+ return str;
+ }
+
+ function utf8ToBytes(string, units) {
+ units = units || Infinity;
+ var codePoint;
+ var length = string.length;
+ var leadSurrogate = null;
+ var bytes = [];
+
+ for (var i = 0; i < length; ++i) {
+ codePoint = string.charCodeAt(i); // is surrogate component
+
+ if (codePoint > 0xD7FF && codePoint < 0xE000) {
+ // last char was a lead
+ if (!leadSurrogate) {
+ // no lead yet
+ if (codePoint > 0xDBFF) {
+ // unexpected trail
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ continue;
+ } else if (i + 1 === length) {
+ // unpaired lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ continue;
+ } // valid lead
+
+
+ leadSurrogate = codePoint;
+ continue;
+ } // 2 leads in a row
+
+
+ if (codePoint < 0xDC00) {
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ leadSurrogate = codePoint;
+ continue;
+ } // valid surrogate pair
+
+
+ codePoint = (leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00) + 0x10000;
+ } else if (leadSurrogate) {
+ // valid bmp char, but last char was a lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ }
+
+ leadSurrogate = null; // encode utf8
+
+ if (codePoint < 0x80) {
+ if ((units -= 1) < 0) break;
+ bytes.push(codePoint);
+ } else if (codePoint < 0x800) {
+ if ((units -= 2) < 0) break;
+ bytes.push(codePoint >> 0x6 | 0xC0, codePoint & 0x3F | 0x80);
+ } else if (codePoint < 0x10000) {
+ if ((units -= 3) < 0) break;
+ bytes.push(codePoint >> 0xC | 0xE0, codePoint >> 0x6 & 0x3F | 0x80, codePoint & 0x3F | 0x80);
+ } else if (codePoint < 0x110000) {
+ if ((units -= 4) < 0) break;
+ bytes.push(codePoint >> 0x12 | 0xF0, codePoint >> 0xC & 0x3F | 0x80, codePoint >> 0x6 & 0x3F | 0x80, codePoint & 0x3F | 0x80);
+ } else {
+ throw new Error('Invalid code point');
+ }
+ }
+
+ return bytes;
+ }
+
+ function asciiToBytes(str) {
+ var byteArray = [];
+
+ for (var i = 0; i < str.length; ++i) {
+ // Node's code seems to be doing this and not & 0x7F..
+ byteArray.push(str.charCodeAt(i) & 0xFF);
+ }
+
+ return byteArray;
+ }
+
+ function utf16leToBytes(str, units) {
+ var c, hi, lo;
+ var byteArray = [];
+
+ for (var i = 0; i < str.length; ++i) {
+ if ((units -= 2) < 0) break;
+ c = str.charCodeAt(i);
+ hi = c >> 8;
+ lo = c % 256;
+ byteArray.push(lo);
+ byteArray.push(hi);
+ }
+
+ return byteArray;
+ }
+
+ function base64ToBytes(str) {
+ return base64Js.toByteArray(base64clean(str));
+ }
+
+ function blitBuffer(src, dst, offset, length) {
+ for (var i = 0; i < length; ++i) {
+ if (i + offset >= dst.length || i >= src.length) break;
+ dst[i + offset] = src[i];
+ }
+
+ return i;
+ } // ArrayBuffer or Uint8Array objects from other contexts (i.e. iframes) do not pass
+ // the `instanceof` check but they should be treated as of that type.
+ // See: https://github.com/feross/buffer/issues/166
+
+
+ function isInstance(obj, type) {
+ return obj instanceof type || obj != null && obj.constructor != null && obj.constructor.name != null && obj.constructor.name === type.name;
+ }
+
+ function numberIsNaN(obj) {
+ // For IE11 support
+ return obj !== obj; // eslint-disable-line no-self-compare
+ } // Create lookup table for `toString('hex')`
+ // See: https://github.com/feross/buffer/issues/219
+
+
+ var hexSliceLookupTable = function () {
+ var alphabet = '0123456789abcdef';
+ var table = new Array(256);
+
+ for (var i = 0; i < 16; ++i) {
+ var i16 = i * 16;
+
+ for (var j = 0; j < 16; ++j) {
+ table[i16 + j] = alphabet[i] + alphabet[j];
+ }
+ }
+
+ return table;
+ }();
+});
+buffer.Buffer;
+buffer.SlowBuffer;
+buffer.INSPECT_MAX_BYTES;
+buffer.kMaxLength;
+
+var require$$0 = {};
+
+// shim for using process in browser
+
+var performance = global.performance || {};
+
+performance.now || performance.mozNow || performance.msNow || performance.oNow || performance.webkitNow || function () {
+ return new Date().getTime();
+}; // generate timestamp or delta
+
+var inherits;
+
+if (typeof Object.create === 'function') {
+ inherits = function inherits(ctor, superCtor) {
+ // implementation from standard node.js 'util' module
+ ctor.super_ = superCtor;
+ ctor.prototype = Object.create(superCtor.prototype, {
+ constructor: {
+ value: ctor,
+ enumerable: false,
+ writable: true,
+ configurable: true
+ }
+ });
+ };
+} else {
+ inherits = function inherits(ctor, superCtor) {
+ ctor.super_ = superCtor;
+
+ var TempCtor = function TempCtor() {};
+
+ TempCtor.prototype = superCtor.prototype;
+ ctor.prototype = new TempCtor();
+ ctor.prototype.constructor = ctor;
+ };
+}
+
+var inherits$1 = inherits;
+
+// Copyright Joyent, Inc. and other Node contributors.
+var formatRegExp = /%[sdj%]/g;
+function format(f) {
+ if (!isString(f)) {
+ var objects = [];
+
+ for (var i = 0; i < arguments.length; i++) {
+ objects.push(inspect(arguments[i]));
+ }
+
+ return objects.join(' ');
+ }
+
+ var i = 1;
+ var args = arguments;
+ var len = args.length;
+ var str = String(f).replace(formatRegExp, function (x) {
+ if (x === '%%') return '%';
+ if (i >= len) return x;
+
+ switch (x) {
+ case '%s':
+ return String(args[i++]);
+
+ case '%d':
+ return Number(args[i++]);
+
+ case '%j':
+ try {
+ return JSON.stringify(args[i++]);
+ } catch (_) {
+ return '[Circular]';
+ }
+
+ default:
+ return x;
+ }
+ });
+
+ for (var x = args[i]; i < len; x = args[++i]) {
+ if (isNull(x) || !isObject(x)) {
+ str += ' ' + x;
+ } else {
+ str += ' ' + inspect(x);
+ }
+ }
+
+ return str;
+}
+// Returns a modified function which warns once by default.
+// If --no-deprecation is set, then it is a no-op.
+
+function deprecate(fn, msg) {
+ // Allow for deprecating things in the process of starting up.
+ if (isUndefined(global.process)) {
+ return function () {
+ return deprecate(fn, msg).apply(this, arguments);
+ };
+ }
+
+ var warned = false;
+
+ function deprecated() {
+ if (!warned) {
+ {
+ console.error(msg);
+ }
+
+ warned = true;
+ }
+
+ return fn.apply(this, arguments);
+ }
+
+ return deprecated;
+}
+var debugs = {};
+var debugEnviron;
+function debuglog(set) {
+ if (isUndefined(debugEnviron)) debugEnviron = '';
+ set = set.toUpperCase();
+
+ if (!debugs[set]) {
+ if (new RegExp('\\b' + set + '\\b', 'i').test(debugEnviron)) {
+ var pid = 0;
+
+ debugs[set] = function () {
+ var msg = format.apply(null, arguments);
+ console.error('%s %d: %s', set, pid, msg);
+ };
+ } else {
+ debugs[set] = function () {};
+ }
+ }
+
+ return debugs[set];
+}
+/**
+ * Echos the value of a value. Trys to print the value out
+ * in the best way possible given the different types.
+ *
+ * @param {Object} obj The object to print out.
+ * @param {Object} opts Optional options object that alters the output.
+ */
+
+/* legacy: obj, showHidden, depth, colors*/
+
+function inspect(obj, opts) {
+ // default options
+ var ctx = {
+ seen: [],
+ stylize: stylizeNoColor
+ }; // legacy...
+
+ if (arguments.length >= 3) ctx.depth = arguments[2];
+ if (arguments.length >= 4) ctx.colors = arguments[3];
+
+ if (isBoolean(opts)) {
+ // legacy...
+ ctx.showHidden = opts;
+ } else if (opts) {
+ // got an "options" object
+ _extend(ctx, opts);
+ } // set default options
+
+
+ if (isUndefined(ctx.showHidden)) ctx.showHidden = false;
+ if (isUndefined(ctx.depth)) ctx.depth = 2;
+ if (isUndefined(ctx.colors)) ctx.colors = false;
+ if (isUndefined(ctx.customInspect)) ctx.customInspect = true;
+ if (ctx.colors) ctx.stylize = stylizeWithColor;
+ return formatValue(ctx, obj, ctx.depth);
+} // http://en.wikipedia.org/wiki/ANSI_escape_code#graphics
+
+inspect.colors = {
+ 'bold': [1, 22],
+ 'italic': [3, 23],
+ 'underline': [4, 24],
+ 'inverse': [7, 27],
+ 'white': [37, 39],
+ 'grey': [90, 39],
+ 'black': [30, 39],
+ 'blue': [34, 39],
+ 'cyan': [36, 39],
+ 'green': [32, 39],
+ 'magenta': [35, 39],
+ 'red': [31, 39],
+ 'yellow': [33, 39]
+}; // Don't use 'blue' not visible on cmd.exe
+
+inspect.styles = {
+ 'special': 'cyan',
+ 'number': 'yellow',
+ 'boolean': 'yellow',
+ 'undefined': 'grey',
+ 'null': 'bold',
+ 'string': 'green',
+ 'date': 'magenta',
+ // "name": intentionally not styling
+ 'regexp': 'red'
+};
+
+function stylizeWithColor(str, styleType) {
+ var style = inspect.styles[styleType];
+
+ if (style) {
+ return "\x1B[" + inspect.colors[style][0] + 'm' + str + "\x1B[" + inspect.colors[style][1] + 'm';
+ } else {
+ return str;
+ }
+}
+
+function stylizeNoColor(str, styleType) {
+ return str;
+}
+
+function arrayToHash(array) {
+ var hash = {};
+ array.forEach(function (val, idx) {
+ hash[val] = true;
+ });
+ return hash;
+}
+
+function formatValue(ctx, value, recurseTimes) {
+ // Provide a hook for user-specified inspect functions.
+ // Check that value is an object with an inspect function on it
+ if (ctx.customInspect && value && isFunction(value.inspect) && // Filter out the util module, it's inspect function is special
+ value.inspect !== inspect && // Also filter out any prototype objects using the circular check.
+ !(value.constructor && value.constructor.prototype === value)) {
+ var ret = value.inspect(recurseTimes, ctx);
+
+ if (!isString(ret)) {
+ ret = formatValue(ctx, ret, recurseTimes);
+ }
+
+ return ret;
+ } // Primitive types cannot have properties
+
+
+ var primitive = formatPrimitive(ctx, value);
+
+ if (primitive) {
+ return primitive;
+ } // Look up the keys of the object.
+
+
+ var keys = Object.keys(value);
+ var visibleKeys = arrayToHash(keys);
+
+ if (ctx.showHidden) {
+ keys = Object.getOwnPropertyNames(value);
+ } // IE doesn't make error fields non-enumerable
+ // http://msdn.microsoft.com/en-us/library/ie/dww52sbt(v=vs.94).aspx
+
+
+ if (isError(value) && (keys.indexOf('message') >= 0 || keys.indexOf('description') >= 0)) {
+ return formatError(value);
+ } // Some type of object without properties can be shortcutted.
+
+
+ if (keys.length === 0) {
+ if (isFunction(value)) {
+ var name = value.name ? ': ' + value.name : '';
+ return ctx.stylize('[Function' + name + ']', 'special');
+ }
+
+ if (isRegExp(value)) {
+ return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
+ }
+
+ if (isDate(value)) {
+ return ctx.stylize(Date.prototype.toString.call(value), 'date');
+ }
+
+ if (isError(value)) {
+ return formatError(value);
+ }
+ }
+
+ var base = '',
+ array = false,
+ braces = ['{', '}']; // Make Array say that they are Array
+
+ if (isArray(value)) {
+ array = true;
+ braces = ['[', ']'];
+ } // Make functions say that they are functions
+
+
+ if (isFunction(value)) {
+ var n = value.name ? ': ' + value.name : '';
+ base = ' [Function' + n + ']';
+ } // Make RegExps say that they are RegExps
+
+
+ if (isRegExp(value)) {
+ base = ' ' + RegExp.prototype.toString.call(value);
+ } // Make dates with properties first say the date
+
+
+ if (isDate(value)) {
+ base = ' ' + Date.prototype.toUTCString.call(value);
+ } // Make error with message first say the error
+
+
+ if (isError(value)) {
+ base = ' ' + formatError(value);
+ }
+
+ if (keys.length === 0 && (!array || value.length == 0)) {
+ return braces[0] + base + braces[1];
+ }
+
+ if (recurseTimes < 0) {
+ if (isRegExp(value)) {
+ return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
+ } else {
+ return ctx.stylize('[Object]', 'special');
+ }
+ }
+
+ ctx.seen.push(value);
+ var output;
+
+ if (array) {
+ output = formatArray(ctx, value, recurseTimes, visibleKeys, keys);
+ } else {
+ output = keys.map(function (key) {
+ return formatProperty(ctx, value, recurseTimes, visibleKeys, key, array);
+ });
+ }
+
+ ctx.seen.pop();
+ return reduceToSingleString(output, base, braces);
+}
+
+function formatPrimitive(ctx, value) {
+ if (isUndefined(value)) return ctx.stylize('undefined', 'undefined');
+
+ if (isString(value)) {
+ var simple = '\'' + JSON.stringify(value).replace(/^"|"$/g, '').replace(/'/g, "\\'").replace(/\\"/g, '"') + '\'';
+ return ctx.stylize(simple, 'string');
+ }
+
+ if (isNumber(value)) return ctx.stylize('' + value, 'number');
+ if (isBoolean(value)) return ctx.stylize('' + value, 'boolean'); // For some reason typeof null is "object", so special case here.
+
+ if (isNull(value)) return ctx.stylize('null', 'null');
+}
+
+function formatError(value) {
+ return '[' + Error.prototype.toString.call(value) + ']';
+}
+
+function formatArray(ctx, value, recurseTimes, visibleKeys, keys) {
+ var output = [];
+
+ for (var i = 0, l = value.length; i < l; ++i) {
+ if (hasOwnProperty(value, String(i))) {
+ output.push(formatProperty(ctx, value, recurseTimes, visibleKeys, String(i), true));
+ } else {
+ output.push('');
+ }
+ }
+
+ keys.forEach(function (key) {
+ if (!key.match(/^\d+$/)) {
+ output.push(formatProperty(ctx, value, recurseTimes, visibleKeys, key, true));
+ }
+ });
+ return output;
+}
+
+function formatProperty(ctx, value, recurseTimes, visibleKeys, key, array) {
+ var name, str, desc;
+ desc = Object.getOwnPropertyDescriptor(value, key) || {
+ value: value[key]
+ };
+
+ if (desc.get) {
+ if (desc.set) {
+ str = ctx.stylize('[Getter/Setter]', 'special');
+ } else {
+ str = ctx.stylize('[Getter]', 'special');
+ }
+ } else {
+ if (desc.set) {
+ str = ctx.stylize('[Setter]', 'special');
+ }
+ }
+
+ if (!hasOwnProperty(visibleKeys, key)) {
+ name = '[' + key + ']';
+ }
+
+ if (!str) {
+ if (ctx.seen.indexOf(desc.value) < 0) {
+ if (isNull(recurseTimes)) {
+ str = formatValue(ctx, desc.value, null);
+ } else {
+ str = formatValue(ctx, desc.value, recurseTimes - 1);
+ }
+
+ if (str.indexOf('\n') > -1) {
+ if (array) {
+ str = str.split('\n').map(function (line) {
+ return ' ' + line;
+ }).join('\n').substr(2);
+ } else {
+ str = '\n' + str.split('\n').map(function (line) {
+ return ' ' + line;
+ }).join('\n');
+ }
+ }
+ } else {
+ str = ctx.stylize('[Circular]', 'special');
+ }
+ }
+
+ if (isUndefined(name)) {
+ if (array && key.match(/^\d+$/)) {
+ return str;
+ }
+
+ name = JSON.stringify('' + key);
+
+ if (name.match(/^"([a-zA-Z_][a-zA-Z_0-9]*)"$/)) {
+ name = name.substr(1, name.length - 2);
+ name = ctx.stylize(name, 'name');
+ } else {
+ name = name.replace(/'/g, "\\'").replace(/\\"/g, '"').replace(/(^"|"$)/g, "'");
+ name = ctx.stylize(name, 'string');
+ }
+ }
+
+ return name + ': ' + str;
+}
+
+function reduceToSingleString(output, base, braces) {
+ var length = output.reduce(function (prev, cur) {
+ if (cur.indexOf('\n') >= 0) ;
+ return prev + cur.replace(/\u001b\[\d\d?m/g, '').length + 1;
+ }, 0);
+
+ if (length > 60) {
+ return braces[0] + (base === '' ? '' : base + '\n ') + ' ' + output.join(',\n ') + ' ' + braces[1];
+ }
+
+ return braces[0] + base + ' ' + output.join(', ') + ' ' + braces[1];
+} // NOTE: These type checking functions intentionally don't use `instanceof`
+// because it is fragile and can be easily faked with `Object.create()`.
+
+
+function isArray(ar) {
+ return Array.isArray(ar);
+}
+function isBoolean(arg) {
+ return typeof arg === 'boolean';
+}
+function isNull(arg) {
+ return arg === null;
+}
+function isNullOrUndefined(arg) {
+ return arg == null;
+}
+function isNumber(arg) {
+ return typeof arg === 'number';
+}
+function isString(arg) {
+ return typeof arg === 'string';
+}
+function isSymbol(arg) {
+ return babelHelpers["typeof"](arg) === 'symbol';
+}
+function isUndefined(arg) {
+ return arg === void 0;
+}
+function isRegExp(re) {
+ return isObject(re) && objectToString(re) === '[object RegExp]';
+}
+function isObject(arg) {
+ return babelHelpers["typeof"](arg) === 'object' && arg !== null;
+}
+function isDate(d) {
+ return isObject(d) && objectToString(d) === '[object Date]';
+}
+function isError(e) {
+ return isObject(e) && (objectToString(e) === '[object Error]' || e instanceof Error);
+}
+function isFunction(arg) {
+ return typeof arg === 'function';
+}
+function isPrimitive(arg) {
+ return arg === null || typeof arg === 'boolean' || typeof arg === 'number' || typeof arg === 'string' || babelHelpers["typeof"](arg) === 'symbol' || // ES6 symbol
+ typeof arg === 'undefined';
+}
+function isBuffer(maybeBuf) {
+ return Buffer.isBuffer(maybeBuf);
+}
+
+function objectToString(o) {
+ return Object.prototype.toString.call(o);
+}
+
+function pad(n) {
+ return n < 10 ? '0' + n.toString(10) : n.toString(10);
+}
+
+var months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']; // 26 Feb 16:19:34
+
+function timestamp$1() {
+ var d = new Date();
+ var time = [pad(d.getHours()), pad(d.getMinutes()), pad(d.getSeconds())].join(':');
+ return [d.getDate(), months[d.getMonth()], time].join(' ');
+} // log is just a thin wrapper to console.log that prepends a timestamp
+
+
+function log() {
+ console.log('%s - %s', timestamp$1(), format.apply(null, arguments));
+}
+function _extend(origin, add) {
+ // Don't do anything if add isn't an object
+ if (!add || !isObject(add)) return origin;
+ var keys = Object.keys(add);
+ var i = keys.length;
+
+ while (i--) {
+ origin[keys[i]] = add[keys[i]];
+ }
+
+ return origin;
+}
+
+function hasOwnProperty(obj, prop) {
+ return Object.prototype.hasOwnProperty.call(obj, prop);
+}
+
+var require$$1 = {
+ inherits: inherits$1,
+ _extend: _extend,
+ log: log,
+ isBuffer: isBuffer,
+ isPrimitive: isPrimitive,
+ isFunction: isFunction,
+ isError: isError,
+ isDate: isDate,
+ isObject: isObject,
+ isRegExp: isRegExp,
+ isUndefined: isUndefined,
+ isSymbol: isSymbol,
+ isString: isString,
+ isNumber: isNumber,
+ isNullOrUndefined: isNullOrUndefined,
+ isNull: isNull,
+ isBoolean: isBoolean,
+ isArray: isArray,
+ inspect: inspect,
+ deprecate: deprecate,
+ format: format,
+ debuglog: debuglog
+};
+
+var utils = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.deprecate = exports.isObjectLike = exports.isDate = exports.haveBuffer = exports.isMap = exports.isRegExp = exports.isBigUInt64Array = exports.isBigInt64Array = exports.isUint8Array = exports.isAnyArrayBuffer = exports.randomBytes = exports.normalizedFunctionString = void 0;
+
+/**
+ * Normalizes our expected stringified form of a function across versions of node
+ * @param fn - The function to stringify
+ */
+function normalizedFunctionString(fn) {
+ return fn.toString().replace('function(', 'function (');
+}
+exports.normalizedFunctionString = normalizedFunctionString;
+var isReactNative = typeof commonjsGlobal.navigator === 'object' && commonjsGlobal.navigator.product === 'ReactNative';
+var insecureWarning = isReactNative
+ ? 'BSON: For React Native please polyfill crypto.getRandomValues, e.g. using: https://www.npmjs.com/package/react-native-get-random-values.'
+ : 'BSON: No cryptographic implementation for random bytes present, falling back to a less secure implementation.';
+var insecureRandomBytes = function insecureRandomBytes(size) {
+ console.warn(insecureWarning);
+ var result = buffer.Buffer.alloc(size);
+ for (var i = 0; i < size; ++i)
+ result[i] = Math.floor(Math.random() * 256);
+ return result;
+};
+var detectRandomBytes = function () {
+ if (typeof window !== 'undefined') {
+ // browser crypto implementation(s)
+ var target_1 = window.crypto || window.msCrypto; // allow for IE11
+ if (target_1 && target_1.getRandomValues) {
+ return function (size) { return target_1.getRandomValues(buffer.Buffer.alloc(size)); };
+ }
+ }
+ if (typeof commonjsGlobal !== 'undefined' && commonjsGlobal.crypto && commonjsGlobal.crypto.getRandomValues) {
+ // allow for RN packages such as https://www.npmjs.com/package/react-native-get-random-values to populate global
+ return function (size) { return commonjsGlobal.crypto.getRandomValues(buffer.Buffer.alloc(size)); };
+ }
+ var requiredRandomBytes;
+ try {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ requiredRandomBytes = require$$0.randomBytes;
+ }
+ catch (e) {
+ // keep the fallback
+ }
+ // NOTE: in transpiled cases the above require might return null/undefined
+ return requiredRandomBytes || insecureRandomBytes;
+};
+exports.randomBytes = detectRandomBytes();
+function isAnyArrayBuffer(value) {
+ return ['[object ArrayBuffer]', '[object SharedArrayBuffer]'].includes(Object.prototype.toString.call(value));
+}
+exports.isAnyArrayBuffer = isAnyArrayBuffer;
+function isUint8Array(value) {
+ return Object.prototype.toString.call(value) === '[object Uint8Array]';
+}
+exports.isUint8Array = isUint8Array;
+function isBigInt64Array(value) {
+ return Object.prototype.toString.call(value) === '[object BigInt64Array]';
+}
+exports.isBigInt64Array = isBigInt64Array;
+function isBigUInt64Array(value) {
+ return Object.prototype.toString.call(value) === '[object BigUint64Array]';
+}
+exports.isBigUInt64Array = isBigUInt64Array;
+function isRegExp(d) {
+ return Object.prototype.toString.call(d) === '[object RegExp]';
+}
+exports.isRegExp = isRegExp;
+function isMap(d) {
+ return Object.prototype.toString.call(d) === '[object Map]';
+}
+exports.isMap = isMap;
+/** Call to check if your environment has `Buffer` */
+function haveBuffer() {
+ return typeof commonjsGlobal !== 'undefined' && typeof commonjsGlobal.Buffer !== 'undefined';
+}
+exports.haveBuffer = haveBuffer;
+// To ensure that 0.4 of node works correctly
+function isDate(d) {
+ return isObjectLike(d) && Object.prototype.toString.call(d) === '[object Date]';
+}
+exports.isDate = isDate;
+/**
+ * @internal
+ * this is to solve the `'someKey' in x` problem where x is unknown.
+ * https://github.com/typescript-eslint/typescript-eslint/issues/1071#issuecomment-541955753
+ */
+function isObjectLike(candidate) {
+ return typeof candidate === 'object' && candidate !== null;
+}
+exports.isObjectLike = isObjectLike;
+function deprecate(fn, message) {
+ if (typeof window === 'undefined' && typeof self === 'undefined') {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ return require$$1.deprecate(fn, message);
+ }
+ var warned = false;
+ function deprecated() {
+ var args = [];
+ for (var _i = 0; _i < arguments.length; _i++) {
+ args[_i] = arguments[_i];
+ }
+ if (!warned) {
+ console.warn(message);
+ warned = true;
+ }
+ return fn.apply(this, args);
+ }
+ return deprecated;
+}
+exports.deprecate = deprecate;
+
+});
+
+unwrapExports(utils);
+utils.deprecate;
+utils.isObjectLike;
+utils.isDate;
+utils.haveBuffer;
+utils.isMap;
+utils.isRegExp;
+utils.isBigUInt64Array;
+utils.isBigInt64Array;
+utils.isUint8Array;
+utils.isAnyArrayBuffer;
+utils.randomBytes;
+utils.normalizedFunctionString;
+
+var ensure_buffer = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.ensureBuffer = void 0;
+
+
+/**
+ * Makes sure that, if a Uint8Array is passed in, it is wrapped in a Buffer.
+ *
+ * @param potentialBuffer - The potential buffer
+ * @returns Buffer the input if potentialBuffer is a buffer, or a buffer that
+ * wraps a passed in Uint8Array
+ * @throws TypeError If anything other than a Buffer or Uint8Array is passed in
+ */
+function ensureBuffer(potentialBuffer) {
+ if (ArrayBuffer.isView(potentialBuffer)) {
+ return buffer.Buffer.from(potentialBuffer.buffer, potentialBuffer.byteOffset, potentialBuffer.byteLength);
+ }
+ if (utils.isAnyArrayBuffer(potentialBuffer)) {
+ return buffer.Buffer.from(potentialBuffer);
+ }
+ throw new TypeError('Must use either Buffer or TypedArray');
+}
+exports.ensureBuffer = ensureBuffer;
+
+});
+
+unwrapExports(ensure_buffer);
+ensure_buffer.ensureBuffer;
+
+var uuid_utils = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.bufferToUuidHexString = exports.uuidHexStringToBuffer = exports.uuidValidateString = void 0;
+
+// Validation regex for v4 uuid (validates with or without dashes)
+var VALIDATION_REGEX = /^(?:[0-9a-f]{8}-[0-9a-f]{4}-4[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}|[0-9a-f]{12}4[0-9a-f]{3}[89ab][0-9a-f]{15})$/i;
+var uuidValidateString = function (str) {
+ return typeof str === 'string' && VALIDATION_REGEX.test(str);
+};
+exports.uuidValidateString = uuidValidateString;
+var uuidHexStringToBuffer = function (hexString) {
+ if (!exports.uuidValidateString(hexString)) {
+ throw new TypeError('UUID string representations must be a 32 or 36 character hex string (dashes excluded/included). Format: "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx" or "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx".');
+ }
+ var sanitizedHexString = hexString.replace(/-/g, '');
+ return buffer.Buffer.from(sanitizedHexString, 'hex');
+};
+exports.uuidHexStringToBuffer = uuidHexStringToBuffer;
+var bufferToUuidHexString = function (buffer, includeDashes) {
+ if (includeDashes === void 0) { includeDashes = true; }
+ return includeDashes
+ ? buffer.toString('hex', 0, 4) +
+ '-' +
+ buffer.toString('hex', 4, 6) +
+ '-' +
+ buffer.toString('hex', 6, 8) +
+ '-' +
+ buffer.toString('hex', 8, 10) +
+ '-' +
+ buffer.toString('hex', 10, 16)
+ : buffer.toString('hex');
+};
+exports.bufferToUuidHexString = bufferToUuidHexString;
+
+});
+
+unwrapExports(uuid_utils);
+uuid_utils.bufferToUuidHexString;
+uuid_utils.uuidHexStringToBuffer;
+uuid_utils.uuidValidateString;
+
+var uuid = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.UUID = void 0;
+
+
+
+
+
+var BYTE_LENGTH = 16;
+var kId = Symbol('id');
+/**
+ * A class representation of the BSON UUID type.
+ * @public
+ */
+var UUID = /** @class */ (function () {
+ /**
+ * Create an UUID type
+ *
+ * @param input - Can be a 32 or 36 character hex string (dashes excluded/included) or a 16 byte binary Buffer.
+ */
+ function UUID(input) {
+ if (typeof input === 'undefined') {
+ // The most common use case (blank id, new UUID() instance)
+ this.id = UUID.generate();
+ }
+ else if (input instanceof UUID) {
+ this[kId] = buffer.Buffer.from(input.id);
+ this.__id = input.__id;
+ }
+ else if (ArrayBuffer.isView(input) && input.byteLength === BYTE_LENGTH) {
+ this.id = ensure_buffer.ensureBuffer(input);
+ }
+ else if (typeof input === 'string') {
+ this.id = uuid_utils.uuidHexStringToBuffer(input);
+ }
+ else {
+ throw new TypeError('Argument passed in UUID constructor must be a UUID, a 16 byte Buffer or a 32/36 character hex string (dashes excluded/included, format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx).');
+ }
+ }
+ Object.defineProperty(UUID.prototype, "id", {
+ /**
+ * The UUID bytes
+ * @readonly
+ */
+ get: function () {
+ return this[kId];
+ },
+ set: function (value) {
+ this[kId] = value;
+ if (UUID.cacheHexString) {
+ this.__id = uuid_utils.bufferToUuidHexString(value);
+ }
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /**
+ * Generate a 16 byte uuid v4 buffer used in UUIDs
+ */
+ /**
+ * Returns the UUID id as a 32 or 36 character hex string representation, excluding/including dashes (defaults to 36 character dash separated)
+ * @param includeDashes - should the string exclude dash-separators.
+ * */
+ UUID.prototype.toHexString = function (includeDashes) {
+ if (includeDashes === void 0) { includeDashes = true; }
+ if (UUID.cacheHexString && this.__id) {
+ return this.__id;
+ }
+ var uuidHexString = uuid_utils.bufferToUuidHexString(this.id, includeDashes);
+ if (UUID.cacheHexString) {
+ this.__id = uuidHexString;
+ }
+ return uuidHexString;
+ };
+ /**
+ * Converts the id into a 36 character (dashes included) hex string, unless a encoding is specified.
+ * @internal
+ */
+ UUID.prototype.toString = function (encoding) {
+ return encoding ? this.id.toString(encoding) : this.toHexString();
+ };
+ /**
+ * Converts the id into its JSON string representation. A 36 character (dashes included) hex string in the format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx
+ * @internal
+ */
+ UUID.prototype.toJSON = function () {
+ return this.toHexString();
+ };
+ /**
+ * Compares the equality of this UUID with `otherID`.
+ *
+ * @param otherId - UUID instance to compare against.
+ */
+ UUID.prototype.equals = function (otherId) {
+ if (!otherId) {
+ return false;
+ }
+ if (otherId instanceof UUID) {
+ return otherId.id.equals(this.id);
+ }
+ try {
+ return new UUID(otherId).id.equals(this.id);
+ }
+ catch (_a) {
+ return false;
+ }
+ };
+ /**
+ * Creates a Binary instance from the current UUID.
+ */
+ UUID.prototype.toBinary = function () {
+ return new binary.Binary(this.id, binary.Binary.SUBTYPE_UUID);
+ };
+ /**
+ * Generates a populated buffer containing a v4 uuid
+ */
+ UUID.generate = function () {
+ var bytes = utils.randomBytes(BYTE_LENGTH);
+ // Per 4.4, set bits for version and `clock_seq_hi_and_reserved`
+ // Kindly borrowed from https://github.com/uuidjs/uuid/blob/master/src/v4.js
+ bytes[6] = (bytes[6] & 0x0f) | 0x40;
+ bytes[8] = (bytes[8] & 0x3f) | 0x80;
+ return buffer.Buffer.from(bytes);
+ };
+ /**
+ * Checks if a value is a valid bson UUID
+ * @param input - UUID, string or Buffer to validate.
+ */
+ UUID.isValid = function (input) {
+ if (!input) {
+ return false;
+ }
+ if (input instanceof UUID) {
+ return true;
+ }
+ if (typeof input === 'string') {
+ return uuid_utils.uuidValidateString(input);
+ }
+ if (utils.isUint8Array(input)) {
+ // check for length & uuid version (https://tools.ietf.org/html/rfc4122#section-4.1.3)
+ if (input.length !== BYTE_LENGTH) {
+ return false;
+ }
+ try {
+ // get this byte as hex: xxxxxxxx-xxxx-XXxx-xxxx-xxxxxxxxxxxx
+ // check first part as uuid version: xxxxxxxx-xxxx-Xxxx-xxxx-xxxxxxxxxxxx
+ return parseInt(input[6].toString(16)[0], 10) === binary.Binary.SUBTYPE_UUID;
+ }
+ catch (_a) {
+ return false;
+ }
+ }
+ return false;
+ };
+ /**
+ * Creates an UUID from a hex string representation of an UUID.
+ * @param hexString - 32 or 36 character hex string (dashes excluded/included).
+ */
+ UUID.createFromHexString = function (hexString) {
+ var buffer = uuid_utils.uuidHexStringToBuffer(hexString);
+ return new UUID(buffer);
+ };
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 36 character hex string representation.
+ * @internal
+ */
+ UUID.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ UUID.prototype.inspect = function () {
+ return "new UUID(\"" + this.toHexString() + "\")";
+ };
+ return UUID;
+}());
+exports.UUID = UUID;
+Object.defineProperty(UUID.prototype, '_bsontype', { value: 'UUID' });
+
+});
+
+unwrapExports(uuid);
+uuid.UUID;
+
+var binary = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Binary = void 0;
+
+
+
+
+/**
+ * A class representation of the BSON Binary type.
+ * @public
+ */
+var Binary = /** @class */ (function () {
+ /**
+ * @param buffer - a buffer object containing the binary data.
+ * @param subType - the option binary type.
+ */
+ function Binary(buffer$1, subType) {
+ if (!(this instanceof Binary))
+ return new Binary(buffer$1, subType);
+ if (!(buffer$1 == null) &&
+ !(typeof buffer$1 === 'string') &&
+ !ArrayBuffer.isView(buffer$1) &&
+ !(buffer$1 instanceof ArrayBuffer) &&
+ !Array.isArray(buffer$1)) {
+ throw new TypeError('Binary can only be constructed from string, Buffer, TypedArray, or Array');
+ }
+ this.sub_type = subType !== null && subType !== void 0 ? subType : Binary.BSON_BINARY_SUBTYPE_DEFAULT;
+ if (buffer$1 == null) {
+ // create an empty binary buffer
+ this.buffer = buffer.Buffer.alloc(Binary.BUFFER_SIZE);
+ this.position = 0;
+ }
+ else {
+ if (typeof buffer$1 === 'string') {
+ // string
+ this.buffer = buffer.Buffer.from(buffer$1, 'binary');
+ }
+ else if (Array.isArray(buffer$1)) {
+ // number[]
+ this.buffer = buffer.Buffer.from(buffer$1);
+ }
+ else {
+ // Buffer | TypedArray | ArrayBuffer
+ this.buffer = ensure_buffer.ensureBuffer(buffer$1);
+ }
+ this.position = this.buffer.byteLength;
+ }
+ }
+ /**
+ * Updates this binary with byte_value.
+ *
+ * @param byteValue - a single byte we wish to write.
+ */
+ Binary.prototype.put = function (byteValue) {
+ // If it's a string and a has more than one character throw an error
+ if (typeof byteValue === 'string' && byteValue.length !== 1) {
+ throw new TypeError('only accepts single character String');
+ }
+ else if (typeof byteValue !== 'number' && byteValue.length !== 1)
+ throw new TypeError('only accepts single character Uint8Array or Array');
+ // Decode the byte value once
+ var decodedByte;
+ if (typeof byteValue === 'string') {
+ decodedByte = byteValue.charCodeAt(0);
+ }
+ else if (typeof byteValue === 'number') {
+ decodedByte = byteValue;
+ }
+ else {
+ decodedByte = byteValue[0];
+ }
+ if (decodedByte < 0 || decodedByte > 255) {
+ throw new TypeError('only accepts number in a valid unsigned byte range 0-255');
+ }
+ if (this.buffer.length > this.position) {
+ this.buffer[this.position++] = decodedByte;
+ }
+ else {
+ var buffer$1 = buffer.Buffer.alloc(Binary.BUFFER_SIZE + this.buffer.length);
+ // Combine the two buffers together
+ this.buffer.copy(buffer$1, 0, 0, this.buffer.length);
+ this.buffer = buffer$1;
+ this.buffer[this.position++] = decodedByte;
+ }
+ };
+ /**
+ * Writes a buffer or string to the binary.
+ *
+ * @param sequence - a string or buffer to be written to the Binary BSON object.
+ * @param offset - specify the binary of where to write the content.
+ */
+ Binary.prototype.write = function (sequence, offset) {
+ offset = typeof offset === 'number' ? offset : this.position;
+ // If the buffer is to small let's extend the buffer
+ if (this.buffer.length < offset + sequence.length) {
+ var buffer$1 = buffer.Buffer.alloc(this.buffer.length + sequence.length);
+ this.buffer.copy(buffer$1, 0, 0, this.buffer.length);
+ // Assign the new buffer
+ this.buffer = buffer$1;
+ }
+ if (ArrayBuffer.isView(sequence)) {
+ this.buffer.set(ensure_buffer.ensureBuffer(sequence), offset);
+ this.position =
+ offset + sequence.byteLength > this.position ? offset + sequence.length : this.position;
+ }
+ else if (typeof sequence === 'string') {
+ this.buffer.write(sequence, offset, sequence.length, 'binary');
+ this.position =
+ offset + sequence.length > this.position ? offset + sequence.length : this.position;
+ }
+ };
+ /**
+ * Reads **length** bytes starting at **position**.
+ *
+ * @param position - read from the given position in the Binary.
+ * @param length - the number of bytes to read.
+ */
+ Binary.prototype.read = function (position, length) {
+ length = length && length > 0 ? length : this.position;
+ // Let's return the data based on the type we have
+ return this.buffer.slice(position, position + length);
+ };
+ /**
+ * Returns the value of this binary as a string.
+ * @param asRaw - Will skip converting to a string
+ * @remarks
+ * This is handy when calling this function conditionally for some key value pairs and not others
+ */
+ Binary.prototype.value = function (asRaw) {
+ asRaw = !!asRaw;
+ // Optimize to serialize for the situation where the data == size of buffer
+ if (asRaw && this.buffer.length === this.position) {
+ return this.buffer;
+ }
+ // If it's a node.js buffer object
+ if (asRaw) {
+ return this.buffer.slice(0, this.position);
+ }
+ return this.buffer.toString('binary', 0, this.position);
+ };
+ /** the length of the binary sequence */
+ Binary.prototype.length = function () {
+ return this.position;
+ };
+ /** @internal */
+ Binary.prototype.toJSON = function () {
+ return this.buffer.toString('base64');
+ };
+ /** @internal */
+ Binary.prototype.toString = function (format) {
+ return this.buffer.toString(format);
+ };
+ /** @internal */
+ Binary.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ var base64String = this.buffer.toString('base64');
+ var subType = Number(this.sub_type).toString(16);
+ if (options.legacy) {
+ return {
+ $binary: base64String,
+ $type: subType.length === 1 ? '0' + subType : subType
+ };
+ }
+ return {
+ $binary: {
+ base64: base64String,
+ subType: subType.length === 1 ? '0' + subType : subType
+ }
+ };
+ };
+ /** @internal */
+ Binary.prototype.toUUID = function () {
+ if (this.sub_type === Binary.SUBTYPE_UUID) {
+ return new uuid.UUID(this.buffer.slice(0, this.position));
+ }
+ throw new Error("Binary sub_type \"" + this.sub_type + "\" is not supported for converting to UUID. Only \"" + Binary.SUBTYPE_UUID + "\" is currently supported.");
+ };
+ /** @internal */
+ Binary.fromExtendedJSON = function (doc, options) {
+ options = options || {};
+ var data;
+ var type;
+ if ('$binary' in doc) {
+ if (options.legacy && typeof doc.$binary === 'string' && '$type' in doc) {
+ type = doc.$type ? parseInt(doc.$type, 16) : 0;
+ data = buffer.Buffer.from(doc.$binary, 'base64');
+ }
+ else {
+ if (typeof doc.$binary !== 'string') {
+ type = doc.$binary.subType ? parseInt(doc.$binary.subType, 16) : 0;
+ data = buffer.Buffer.from(doc.$binary.base64, 'base64');
+ }
+ }
+ }
+ else if ('$uuid' in doc) {
+ type = 4;
+ data = uuid_utils.uuidHexStringToBuffer(doc.$uuid);
+ }
+ if (!data) {
+ throw new TypeError("Unexpected Binary Extended JSON format " + JSON.stringify(doc));
+ }
+ return new Binary(data, type);
+ };
+ /** @internal */
+ Binary.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Binary.prototype.inspect = function () {
+ var asBuffer = this.value(true);
+ return "new Binary(Buffer.from(\"" + asBuffer.toString('hex') + "\", \"hex\"), " + this.sub_type + ")";
+ };
+ /**
+ * Binary default subtype
+ * @internal
+ */
+ Binary.BSON_BINARY_SUBTYPE_DEFAULT = 0;
+ /** Initial buffer default size */
+ Binary.BUFFER_SIZE = 256;
+ /** Default BSON type */
+ Binary.SUBTYPE_DEFAULT = 0;
+ /** Function BSON type */
+ Binary.SUBTYPE_FUNCTION = 1;
+ /** Byte Array BSON type */
+ Binary.SUBTYPE_BYTE_ARRAY = 2;
+ /** Deprecated UUID BSON type @deprecated Please use SUBTYPE_UUID */
+ Binary.SUBTYPE_UUID_OLD = 3;
+ /** UUID BSON type */
+ Binary.SUBTYPE_UUID = 4;
+ /** MD5 BSON type */
+ Binary.SUBTYPE_MD5 = 5;
+ /** User BSON type */
+ Binary.SUBTYPE_USER_DEFINED = 128;
+ return Binary;
+}());
+exports.Binary = Binary;
+Object.defineProperty(Binary.prototype, '_bsontype', { value: 'Binary' });
+
+});
+
+unwrapExports(binary);
+binary.Binary;
+
+var code = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Code = void 0;
+/**
+ * A class representation of the BSON Code type.
+ * @public
+ */
+var Code = /** @class */ (function () {
+ /**
+ * @param code - a string or function.
+ * @param scope - an optional scope for the function.
+ */
+ function Code(code, scope) {
+ if (!(this instanceof Code))
+ return new Code(code, scope);
+ this.code = code;
+ this.scope = scope;
+ }
+ /** @internal */
+ Code.prototype.toJSON = function () {
+ return { code: this.code, scope: this.scope };
+ };
+ /** @internal */
+ Code.prototype.toExtendedJSON = function () {
+ if (this.scope) {
+ return { $code: this.code, $scope: this.scope };
+ }
+ return { $code: this.code };
+ };
+ /** @internal */
+ Code.fromExtendedJSON = function (doc) {
+ return new Code(doc.$code, doc.$scope);
+ };
+ /** @internal */
+ Code.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Code.prototype.inspect = function () {
+ var codeJson = this.toJSON();
+ return "new Code(\"" + codeJson.code + "\"" + (codeJson.scope ? ", " + JSON.stringify(codeJson.scope) : '') + ")";
+ };
+ return Code;
+}());
+exports.Code = Code;
+Object.defineProperty(Code.prototype, '_bsontype', { value: 'Code' });
+
+});
+
+unwrapExports(code);
+code.Code;
+
+var db_ref = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.DBRef = exports.isDBRefLike = void 0;
+
+/** @internal */
+function isDBRefLike(value) {
+ return (utils.isObjectLike(value) &&
+ value.$id != null &&
+ typeof value.$ref === 'string' &&
+ (value.$db == null || typeof value.$db === 'string'));
+}
+exports.isDBRefLike = isDBRefLike;
+/**
+ * A class representation of the BSON DBRef type.
+ * @public
+ */
+var DBRef = /** @class */ (function () {
+ /**
+ * @param collection - the collection name.
+ * @param oid - the reference ObjectId.
+ * @param db - optional db name, if omitted the reference is local to the current db.
+ */
+ function DBRef(collection, oid, db, fields) {
+ if (!(this instanceof DBRef))
+ return new DBRef(collection, oid, db, fields);
+ // check if namespace has been provided
+ var parts = collection.split('.');
+ if (parts.length === 2) {
+ db = parts.shift();
+ // eslint-disable-next-line @typescript-eslint/no-non-null-assertion
+ collection = parts.shift();
+ }
+ this.collection = collection;
+ this.oid = oid;
+ this.db = db;
+ this.fields = fields || {};
+ }
+ Object.defineProperty(DBRef.prototype, "namespace", {
+ // Property provided for compatibility with the 1.x parser
+ // the 1.x parser used a "namespace" property, while 4.x uses "collection"
+ /** @internal */
+ get: function () {
+ return this.collection;
+ },
+ set: function (value) {
+ this.collection = value;
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /** @internal */
+ DBRef.prototype.toJSON = function () {
+ var o = Object.assign({
+ $ref: this.collection,
+ $id: this.oid
+ }, this.fields);
+ if (this.db != null)
+ o.$db = this.db;
+ return o;
+ };
+ /** @internal */
+ DBRef.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ var o = {
+ $ref: this.collection,
+ $id: this.oid
+ };
+ if (options.legacy) {
+ return o;
+ }
+ if (this.db)
+ o.$db = this.db;
+ o = Object.assign(o, this.fields);
+ return o;
+ };
+ /** @internal */
+ DBRef.fromExtendedJSON = function (doc) {
+ var copy = Object.assign({}, doc);
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new DBRef(doc.$ref, doc.$id, doc.$db, copy);
+ };
+ /** @internal */
+ DBRef.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ DBRef.prototype.inspect = function () {
+ // NOTE: if OID is an ObjectId class it will just print the oid string.
+ var oid = this.oid === undefined || this.oid.toString === undefined ? this.oid : this.oid.toString();
+ return "new DBRef(\"" + this.namespace + "\", new ObjectId(\"" + oid + "\")" + (this.db ? ", \"" + this.db + "\"" : '') + ")";
+ };
+ return DBRef;
+}());
+exports.DBRef = DBRef;
+Object.defineProperty(DBRef.prototype, '_bsontype', { value: 'DBRef' });
+
+});
+
+unwrapExports(db_ref);
+db_ref.DBRef;
+db_ref.isDBRefLike;
+
+var long_1 = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Long = void 0;
+
+/**
+ * wasm optimizations, to do native i64 multiplication and divide
+ */
+var wasm = undefined;
+try {
+ wasm = new WebAssembly.Instance(new WebAssembly.Module(
+ // prettier-ignore
+ new Uint8Array([0, 97, 115, 109, 1, 0, 0, 0, 1, 13, 2, 96, 0, 1, 127, 96, 4, 127, 127, 127, 127, 1, 127, 3, 7, 6, 0, 1, 1, 1, 1, 1, 6, 6, 1, 127, 1, 65, 0, 11, 7, 50, 6, 3, 109, 117, 108, 0, 1, 5, 100, 105, 118, 95, 115, 0, 2, 5, 100, 105, 118, 95, 117, 0, 3, 5, 114, 101, 109, 95, 115, 0, 4, 5, 114, 101, 109, 95, 117, 0, 5, 8, 103, 101, 116, 95, 104, 105, 103, 104, 0, 0, 10, 191, 1, 6, 4, 0, 35, 0, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 126, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 127, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 128, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 129, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 130, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11])), {}).exports;
+}
+catch (_a) {
+ // no wasm support
+}
+var TWO_PWR_16_DBL = 1 << 16;
+var TWO_PWR_24_DBL = 1 << 24;
+var TWO_PWR_32_DBL = TWO_PWR_16_DBL * TWO_PWR_16_DBL;
+var TWO_PWR_64_DBL = TWO_PWR_32_DBL * TWO_PWR_32_DBL;
+var TWO_PWR_63_DBL = TWO_PWR_64_DBL / 2;
+/** A cache of the Long representations of small integer values. */
+var INT_CACHE = {};
+/** A cache of the Long representations of small unsigned integer values. */
+var UINT_CACHE = {};
+/**
+ * A class representing a 64-bit integer
+ * @public
+ * @remarks
+ * The internal representation of a long is the two given signed, 32-bit values.
+ * We use 32-bit pieces because these are the size of integers on which
+ * Javascript performs bit-operations. For operations like addition and
+ * multiplication, we split each number into 16 bit pieces, which can easily be
+ * multiplied within Javascript's floating-point representation without overflow
+ * or change in sign.
+ * In the algorithms below, we frequently reduce the negative case to the
+ * positive case by negating the input(s) and then post-processing the result.
+ * Note that we must ALWAYS check specially whether those values are MIN_VALUE
+ * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
+ * a positive number, it overflows back into a negative). Not handling this
+ * case would often result in infinite recursion.
+ * Common constant values ZERO, ONE, NEG_ONE, etc. are found as static properties on this class.
+ */
+var Long = /** @class */ (function () {
+ /**
+ * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers.
+ * See the from* functions below for more convenient ways of constructing Longs.
+ *
+ * Acceptable signatures are:
+ * - Long(low, high, unsigned?)
+ * - Long(bigint, unsigned?)
+ * - Long(string, unsigned?)
+ *
+ * @param low - The low (signed) 32 bits of the long
+ * @param high - The high (signed) 32 bits of the long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ function Long(low, high, unsigned) {
+ if (low === void 0) { low = 0; }
+ if (!(this instanceof Long))
+ return new Long(low, high, unsigned);
+ if (typeof low === 'bigint') {
+ Object.assign(this, Long.fromBigInt(low, !!high));
+ }
+ else if (typeof low === 'string') {
+ Object.assign(this, Long.fromString(low, !!high));
+ }
+ else {
+ this.low = low | 0;
+ this.high = high | 0;
+ this.unsigned = !!unsigned;
+ }
+ Object.defineProperty(this, '__isLong__', {
+ value: true,
+ configurable: false,
+ writable: false,
+ enumerable: false
+ });
+ }
+ /**
+ * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits.
+ * Each is assumed to use 32 bits.
+ * @param lowBits - The low 32 bits
+ * @param highBits - The high 32 bits
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBits = function (lowBits, highBits, unsigned) {
+ return new Long(lowBits, highBits, unsigned);
+ };
+ /**
+ * Returns a Long representing the given 32 bit integer value.
+ * @param value - The 32 bit integer in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromInt = function (value, unsigned) {
+ var obj, cachedObj, cache;
+ if (unsigned) {
+ value >>>= 0;
+ if ((cache = 0 <= value && value < 256)) {
+ cachedObj = UINT_CACHE[value];
+ if (cachedObj)
+ return cachedObj;
+ }
+ obj = Long.fromBits(value, (value | 0) < 0 ? -1 : 0, true);
+ if (cache)
+ UINT_CACHE[value] = obj;
+ return obj;
+ }
+ else {
+ value |= 0;
+ if ((cache = -128 <= value && value < 128)) {
+ cachedObj = INT_CACHE[value];
+ if (cachedObj)
+ return cachedObj;
+ }
+ obj = Long.fromBits(value, value < 0 ? -1 : 0, false);
+ if (cache)
+ INT_CACHE[value] = obj;
+ return obj;
+ }
+ };
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromNumber = function (value, unsigned) {
+ if (isNaN(value))
+ return unsigned ? Long.UZERO : Long.ZERO;
+ if (unsigned) {
+ if (value < 0)
+ return Long.UZERO;
+ if (value >= TWO_PWR_64_DBL)
+ return Long.MAX_UNSIGNED_VALUE;
+ }
+ else {
+ if (value <= -TWO_PWR_63_DBL)
+ return Long.MIN_VALUE;
+ if (value + 1 >= TWO_PWR_63_DBL)
+ return Long.MAX_VALUE;
+ }
+ if (value < 0)
+ return Long.fromNumber(-value, unsigned).neg();
+ return Long.fromBits(value % TWO_PWR_32_DBL | 0, (value / TWO_PWR_32_DBL) | 0, unsigned);
+ };
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBigInt = function (value, unsigned) {
+ return Long.fromString(value.toString(), unsigned);
+ };
+ /**
+ * Returns a Long representation of the given string, written using the specified radix.
+ * @param str - The textual representation of the Long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param radix - The radix in which the text is written (2-36), defaults to 10
+ * @returns The corresponding Long value
+ */
+ Long.fromString = function (str, unsigned, radix) {
+ if (str.length === 0)
+ throw Error('empty string');
+ if (str === 'NaN' || str === 'Infinity' || str === '+Infinity' || str === '-Infinity')
+ return Long.ZERO;
+ if (typeof unsigned === 'number') {
+ // For goog.math.long compatibility
+ (radix = unsigned), (unsigned = false);
+ }
+ else {
+ unsigned = !!unsigned;
+ }
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix)
+ throw RangeError('radix');
+ var p;
+ if ((p = str.indexOf('-')) > 0)
+ throw Error('interior hyphen');
+ else if (p === 0) {
+ return Long.fromString(str.substring(1), unsigned, radix).neg();
+ }
+ // Do several (8) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ var radixToPower = Long.fromNumber(Math.pow(radix, 8));
+ var result = Long.ZERO;
+ for (var i = 0; i < str.length; i += 8) {
+ var size = Math.min(8, str.length - i), value = parseInt(str.substring(i, i + size), radix);
+ if (size < 8) {
+ var power = Long.fromNumber(Math.pow(radix, size));
+ result = result.mul(power).add(Long.fromNumber(value));
+ }
+ else {
+ result = result.mul(radixToPower);
+ result = result.add(Long.fromNumber(value));
+ }
+ }
+ result.unsigned = unsigned;
+ return result;
+ };
+ /**
+ * Creates a Long from its byte representation.
+ * @param bytes - Byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns The corresponding Long value
+ */
+ Long.fromBytes = function (bytes, unsigned, le) {
+ return le ? Long.fromBytesLE(bytes, unsigned) : Long.fromBytesBE(bytes, unsigned);
+ };
+ /**
+ * Creates a Long from its little endian byte representation.
+ * @param bytes - Little endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBytesLE = function (bytes, unsigned) {
+ return new Long(bytes[0] | (bytes[1] << 8) | (bytes[2] << 16) | (bytes[3] << 24), bytes[4] | (bytes[5] << 8) | (bytes[6] << 16) | (bytes[7] << 24), unsigned);
+ };
+ /**
+ * Creates a Long from its big endian byte representation.
+ * @param bytes - Big endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBytesBE = function (bytes, unsigned) {
+ return new Long((bytes[4] << 24) | (bytes[5] << 16) | (bytes[6] << 8) | bytes[7], (bytes[0] << 24) | (bytes[1] << 16) | (bytes[2] << 8) | bytes[3], unsigned);
+ };
+ /**
+ * Tests if the specified object is a Long.
+ */
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any, @typescript-eslint/explicit-module-boundary-types
+ Long.isLong = function (value) {
+ return utils.isObjectLike(value) && value['__isLong__'] === true;
+ };
+ /**
+ * Converts the specified value to a Long.
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ Long.fromValue = function (val, unsigned) {
+ if (typeof val === 'number')
+ return Long.fromNumber(val, unsigned);
+ if (typeof val === 'string')
+ return Long.fromString(val, unsigned);
+ // Throws for non-objects, converts non-instanceof Long:
+ return Long.fromBits(val.low, val.high, typeof unsigned === 'boolean' ? unsigned : val.unsigned);
+ };
+ /** Returns the sum of this and the specified Long. */
+ Long.prototype.add = function (addend) {
+ if (!Long.isLong(addend))
+ addend = Long.fromValue(addend);
+ // Divide each number into 4 chunks of 16 bits, and then sum the chunks.
+ var a48 = this.high >>> 16;
+ var a32 = this.high & 0xffff;
+ var a16 = this.low >>> 16;
+ var a00 = this.low & 0xffff;
+ var b48 = addend.high >>> 16;
+ var b32 = addend.high & 0xffff;
+ var b16 = addend.low >>> 16;
+ var b00 = addend.low & 0xffff;
+ var c48 = 0, c32 = 0, c16 = 0, c00 = 0;
+ c00 += a00 + b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 + b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 + b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 + b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ };
+ /**
+ * Returns the sum of this and the specified Long.
+ * @returns Sum
+ */
+ Long.prototype.and = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low & other.low, this.high & other.high, this.unsigned);
+ };
+ /**
+ * Compares this Long's value with the specified's.
+ * @returns 0 if they are the same, 1 if the this is greater and -1 if the given one is greater
+ */
+ Long.prototype.compare = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ if (this.eq(other))
+ return 0;
+ var thisNeg = this.isNegative(), otherNeg = other.isNegative();
+ if (thisNeg && !otherNeg)
+ return -1;
+ if (!thisNeg && otherNeg)
+ return 1;
+ // At this point the sign bits are the same
+ if (!this.unsigned)
+ return this.sub(other).isNegative() ? -1 : 1;
+ // Both are positive if at least one is unsigned
+ return other.high >>> 0 > this.high >>> 0 ||
+ (other.high === this.high && other.low >>> 0 > this.low >>> 0)
+ ? -1
+ : 1;
+ };
+ /** This is an alias of {@link Long.compare} */
+ Long.prototype.comp = function (other) {
+ return this.compare(other);
+ };
+ /**
+ * Returns this Long divided by the specified. The result is signed if this Long is signed or unsigned if this Long is unsigned.
+ * @returns Quotient
+ */
+ Long.prototype.divide = function (divisor) {
+ if (!Long.isLong(divisor))
+ divisor = Long.fromValue(divisor);
+ if (divisor.isZero())
+ throw Error('division by zero');
+ // use wasm support if present
+ if (wasm) {
+ // guard against signed division overflow: the largest
+ // negative number / -1 would be 1 larger than the largest
+ // positive number, due to two's complement.
+ if (!this.unsigned &&
+ this.high === -0x80000000 &&
+ divisor.low === -1 &&
+ divisor.high === -1) {
+ // be consistent with non-wasm code path
+ return this;
+ }
+ var low = (this.unsigned ? wasm.div_u : wasm.div_s)(this.low, this.high, divisor.low, divisor.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ if (this.isZero())
+ return this.unsigned ? Long.UZERO : Long.ZERO;
+ var approx, rem, res;
+ if (!this.unsigned) {
+ // This section is only relevant for signed longs and is derived from the
+ // closure library as a whole.
+ if (this.eq(Long.MIN_VALUE)) {
+ if (divisor.eq(Long.ONE) || divisor.eq(Long.NEG_ONE))
+ return Long.MIN_VALUE;
+ // recall that -MIN_VALUE == MIN_VALUE
+ else if (divisor.eq(Long.MIN_VALUE))
+ return Long.ONE;
+ else {
+ // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.
+ var halfThis = this.shr(1);
+ approx = halfThis.div(divisor).shl(1);
+ if (approx.eq(Long.ZERO)) {
+ return divisor.isNegative() ? Long.ONE : Long.NEG_ONE;
+ }
+ else {
+ rem = this.sub(divisor.mul(approx));
+ res = approx.add(rem.div(divisor));
+ return res;
+ }
+ }
+ }
+ else if (divisor.eq(Long.MIN_VALUE))
+ return this.unsigned ? Long.UZERO : Long.ZERO;
+ if (this.isNegative()) {
+ if (divisor.isNegative())
+ return this.neg().div(divisor.neg());
+ return this.neg().div(divisor).neg();
+ }
+ else if (divisor.isNegative())
+ return this.div(divisor.neg()).neg();
+ res = Long.ZERO;
+ }
+ else {
+ // The algorithm below has not been made for unsigned longs. It's therefore
+ // required to take special care of the MSB prior to running it.
+ if (!divisor.unsigned)
+ divisor = divisor.toUnsigned();
+ if (divisor.gt(this))
+ return Long.UZERO;
+ if (divisor.gt(this.shru(1)))
+ // 15 >>> 1 = 7 ; with divisor = 8 ; true
+ return Long.UONE;
+ res = Long.UZERO;
+ }
+ // Repeat the following until the remainder is less than other: find a
+ // floating-point that approximates remainder / other *from below*, add this
+ // into the result, and subtract it from the remainder. It is critical that
+ // the approximate value is less than or equal to the real value so that the
+ // remainder never becomes negative.
+ rem = this;
+ while (rem.gte(divisor)) {
+ // Approximate the result of division. This may be a little greater or
+ // smaller than the actual value.
+ approx = Math.max(1, Math.floor(rem.toNumber() / divisor.toNumber()));
+ // We will tweak the approximate result by changing it in the 48-th digit or
+ // the smallest non-fractional digit, whichever is larger.
+ var log2 = Math.ceil(Math.log(approx) / Math.LN2);
+ var delta = log2 <= 48 ? 1 : Math.pow(2, log2 - 48);
+ // Decrease the approximation until it is smaller than the remainder. Note
+ // that if it is too large, the product overflows and is negative.
+ var approxRes = Long.fromNumber(approx);
+ var approxRem = approxRes.mul(divisor);
+ while (approxRem.isNegative() || approxRem.gt(rem)) {
+ approx -= delta;
+ approxRes = Long.fromNumber(approx, this.unsigned);
+ approxRem = approxRes.mul(divisor);
+ }
+ // We know the answer can't be zero... and actually, zero would cause
+ // infinite recursion since we would make no progress.
+ if (approxRes.isZero())
+ approxRes = Long.ONE;
+ res = res.add(approxRes);
+ rem = rem.sub(approxRem);
+ }
+ return res;
+ };
+ /**This is an alias of {@link Long.divide} */
+ Long.prototype.div = function (divisor) {
+ return this.divide(divisor);
+ };
+ /**
+ * Tests if this Long's value equals the specified's.
+ * @param other - Other value
+ */
+ Long.prototype.equals = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ if (this.unsigned !== other.unsigned && this.high >>> 31 === 1 && other.high >>> 31 === 1)
+ return false;
+ return this.high === other.high && this.low === other.low;
+ };
+ /** This is an alias of {@link Long.equals} */
+ Long.prototype.eq = function (other) {
+ return this.equals(other);
+ };
+ /** Gets the high 32 bits as a signed integer. */
+ Long.prototype.getHighBits = function () {
+ return this.high;
+ };
+ /** Gets the high 32 bits as an unsigned integer. */
+ Long.prototype.getHighBitsUnsigned = function () {
+ return this.high >>> 0;
+ };
+ /** Gets the low 32 bits as a signed integer. */
+ Long.prototype.getLowBits = function () {
+ return this.low;
+ };
+ /** Gets the low 32 bits as an unsigned integer. */
+ Long.prototype.getLowBitsUnsigned = function () {
+ return this.low >>> 0;
+ };
+ /** Gets the number of bits needed to represent the absolute value of this Long. */
+ Long.prototype.getNumBitsAbs = function () {
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ return this.eq(Long.MIN_VALUE) ? 64 : this.neg().getNumBitsAbs();
+ }
+ var val = this.high !== 0 ? this.high : this.low;
+ var bit;
+ for (bit = 31; bit > 0; bit--)
+ if ((val & (1 << bit)) !== 0)
+ break;
+ return this.high !== 0 ? bit + 33 : bit + 1;
+ };
+ /** Tests if this Long's value is greater than the specified's. */
+ Long.prototype.greaterThan = function (other) {
+ return this.comp(other) > 0;
+ };
+ /** This is an alias of {@link Long.greaterThan} */
+ Long.prototype.gt = function (other) {
+ return this.greaterThan(other);
+ };
+ /** Tests if this Long's value is greater than or equal the specified's. */
+ Long.prototype.greaterThanOrEqual = function (other) {
+ return this.comp(other) >= 0;
+ };
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ Long.prototype.gte = function (other) {
+ return this.greaterThanOrEqual(other);
+ };
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ Long.prototype.ge = function (other) {
+ return this.greaterThanOrEqual(other);
+ };
+ /** Tests if this Long's value is even. */
+ Long.prototype.isEven = function () {
+ return (this.low & 1) === 0;
+ };
+ /** Tests if this Long's value is negative. */
+ Long.prototype.isNegative = function () {
+ return !this.unsigned && this.high < 0;
+ };
+ /** Tests if this Long's value is odd. */
+ Long.prototype.isOdd = function () {
+ return (this.low & 1) === 1;
+ };
+ /** Tests if this Long's value is positive. */
+ Long.prototype.isPositive = function () {
+ return this.unsigned || this.high >= 0;
+ };
+ /** Tests if this Long's value equals zero. */
+ Long.prototype.isZero = function () {
+ return this.high === 0 && this.low === 0;
+ };
+ /** Tests if this Long's value is less than the specified's. */
+ Long.prototype.lessThan = function (other) {
+ return this.comp(other) < 0;
+ };
+ /** This is an alias of {@link Long#lessThan}. */
+ Long.prototype.lt = function (other) {
+ return this.lessThan(other);
+ };
+ /** Tests if this Long's value is less than or equal the specified's. */
+ Long.prototype.lessThanOrEqual = function (other) {
+ return this.comp(other) <= 0;
+ };
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ Long.prototype.lte = function (other) {
+ return this.lessThanOrEqual(other);
+ };
+ /** Returns this Long modulo the specified. */
+ Long.prototype.modulo = function (divisor) {
+ if (!Long.isLong(divisor))
+ divisor = Long.fromValue(divisor);
+ // use wasm support if present
+ if (wasm) {
+ var low = (this.unsigned ? wasm.rem_u : wasm.rem_s)(this.low, this.high, divisor.low, divisor.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ return this.sub(this.div(divisor).mul(divisor));
+ };
+ /** This is an alias of {@link Long.modulo} */
+ Long.prototype.mod = function (divisor) {
+ return this.modulo(divisor);
+ };
+ /** This is an alias of {@link Long.modulo} */
+ Long.prototype.rem = function (divisor) {
+ return this.modulo(divisor);
+ };
+ /**
+ * Returns the product of this and the specified Long.
+ * @param multiplier - Multiplier
+ * @returns Product
+ */
+ Long.prototype.multiply = function (multiplier) {
+ if (this.isZero())
+ return Long.ZERO;
+ if (!Long.isLong(multiplier))
+ multiplier = Long.fromValue(multiplier);
+ // use wasm support if present
+ if (wasm) {
+ var low = wasm.mul(this.low, this.high, multiplier.low, multiplier.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ if (multiplier.isZero())
+ return Long.ZERO;
+ if (this.eq(Long.MIN_VALUE))
+ return multiplier.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (multiplier.eq(Long.MIN_VALUE))
+ return this.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (this.isNegative()) {
+ if (multiplier.isNegative())
+ return this.neg().mul(multiplier.neg());
+ else
+ return this.neg().mul(multiplier).neg();
+ }
+ else if (multiplier.isNegative())
+ return this.mul(multiplier.neg()).neg();
+ // If both longs are small, use float multiplication
+ if (this.lt(Long.TWO_PWR_24) && multiplier.lt(Long.TWO_PWR_24))
+ return Long.fromNumber(this.toNumber() * multiplier.toNumber(), this.unsigned);
+ // Divide each long into 4 chunks of 16 bits, and then add up 4x4 products.
+ // We can skip products that would overflow.
+ var a48 = this.high >>> 16;
+ var a32 = this.high & 0xffff;
+ var a16 = this.low >>> 16;
+ var a00 = this.low & 0xffff;
+ var b48 = multiplier.high >>> 16;
+ var b32 = multiplier.high & 0xffff;
+ var b16 = multiplier.low >>> 16;
+ var b00 = multiplier.low & 0xffff;
+ var c48 = 0, c32 = 0, c16 = 0, c00 = 0;
+ c00 += a00 * b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 * b00;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c16 += a00 * b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 * b00;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a16 * b16;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a00 * b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ };
+ /** This is an alias of {@link Long.multiply} */
+ Long.prototype.mul = function (multiplier) {
+ return this.multiply(multiplier);
+ };
+ /** Returns the Negation of this Long's value. */
+ Long.prototype.negate = function () {
+ if (!this.unsigned && this.eq(Long.MIN_VALUE))
+ return Long.MIN_VALUE;
+ return this.not().add(Long.ONE);
+ };
+ /** This is an alias of {@link Long.negate} */
+ Long.prototype.neg = function () {
+ return this.negate();
+ };
+ /** Returns the bitwise NOT of this Long. */
+ Long.prototype.not = function () {
+ return Long.fromBits(~this.low, ~this.high, this.unsigned);
+ };
+ /** Tests if this Long's value differs from the specified's. */
+ Long.prototype.notEquals = function (other) {
+ return !this.equals(other);
+ };
+ /** This is an alias of {@link Long.notEquals} */
+ Long.prototype.neq = function (other) {
+ return this.notEquals(other);
+ };
+ /** This is an alias of {@link Long.notEquals} */
+ Long.prototype.ne = function (other) {
+ return this.notEquals(other);
+ };
+ /**
+ * Returns the bitwise OR of this Long and the specified.
+ */
+ Long.prototype.or = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low | other.low, this.high | other.high, this.unsigned);
+ };
+ /**
+ * Returns this Long with bits shifted to the left by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftLeft = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ if ((numBits &= 63) === 0)
+ return this;
+ else if (numBits < 32)
+ return Long.fromBits(this.low << numBits, (this.high << numBits) | (this.low >>> (32 - numBits)), this.unsigned);
+ else
+ return Long.fromBits(0, this.low << (numBits - 32), this.unsigned);
+ };
+ /** This is an alias of {@link Long.shiftLeft} */
+ Long.prototype.shl = function (numBits) {
+ return this.shiftLeft(numBits);
+ };
+ /**
+ * Returns this Long with bits arithmetically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftRight = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ if ((numBits &= 63) === 0)
+ return this;
+ else if (numBits < 32)
+ return Long.fromBits((this.low >>> numBits) | (this.high << (32 - numBits)), this.high >> numBits, this.unsigned);
+ else
+ return Long.fromBits(this.high >> (numBits - 32), this.high >= 0 ? 0 : -1, this.unsigned);
+ };
+ /** This is an alias of {@link Long.shiftRight} */
+ Long.prototype.shr = function (numBits) {
+ return this.shiftRight(numBits);
+ };
+ /**
+ * Returns this Long with bits logically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftRightUnsigned = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ numBits &= 63;
+ if (numBits === 0)
+ return this;
+ else {
+ var high = this.high;
+ if (numBits < 32) {
+ var low = this.low;
+ return Long.fromBits((low >>> numBits) | (high << (32 - numBits)), high >>> numBits, this.unsigned);
+ }
+ else if (numBits === 32)
+ return Long.fromBits(high, 0, this.unsigned);
+ else
+ return Long.fromBits(high >>> (numBits - 32), 0, this.unsigned);
+ }
+ };
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ Long.prototype.shr_u = function (numBits) {
+ return this.shiftRightUnsigned(numBits);
+ };
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ Long.prototype.shru = function (numBits) {
+ return this.shiftRightUnsigned(numBits);
+ };
+ /**
+ * Returns the difference of this and the specified Long.
+ * @param subtrahend - Subtrahend
+ * @returns Difference
+ */
+ Long.prototype.subtract = function (subtrahend) {
+ if (!Long.isLong(subtrahend))
+ subtrahend = Long.fromValue(subtrahend);
+ return this.add(subtrahend.neg());
+ };
+ /** This is an alias of {@link Long.subtract} */
+ Long.prototype.sub = function (subtrahend) {
+ return this.subtract(subtrahend);
+ };
+ /** Converts the Long to a 32 bit integer, assuming it is a 32 bit integer. */
+ Long.prototype.toInt = function () {
+ return this.unsigned ? this.low >>> 0 : this.low;
+ };
+ /** Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa). */
+ Long.prototype.toNumber = function () {
+ if (this.unsigned)
+ return (this.high >>> 0) * TWO_PWR_32_DBL + (this.low >>> 0);
+ return this.high * TWO_PWR_32_DBL + (this.low >>> 0);
+ };
+ /** Converts the Long to a BigInt (arbitrary precision). */
+ Long.prototype.toBigInt = function () {
+ return BigInt(this.toString());
+ };
+ /**
+ * Converts this Long to its byte representation.
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns Byte representation
+ */
+ Long.prototype.toBytes = function (le) {
+ return le ? this.toBytesLE() : this.toBytesBE();
+ };
+ /**
+ * Converts this Long to its little endian byte representation.
+ * @returns Little endian byte representation
+ */
+ Long.prototype.toBytesLE = function () {
+ var hi = this.high, lo = this.low;
+ return [
+ lo & 0xff,
+ (lo >>> 8) & 0xff,
+ (lo >>> 16) & 0xff,
+ lo >>> 24,
+ hi & 0xff,
+ (hi >>> 8) & 0xff,
+ (hi >>> 16) & 0xff,
+ hi >>> 24
+ ];
+ };
+ /**
+ * Converts this Long to its big endian byte representation.
+ * @returns Big endian byte representation
+ */
+ Long.prototype.toBytesBE = function () {
+ var hi = this.high, lo = this.low;
+ return [
+ hi >>> 24,
+ (hi >>> 16) & 0xff,
+ (hi >>> 8) & 0xff,
+ hi & 0xff,
+ lo >>> 24,
+ (lo >>> 16) & 0xff,
+ (lo >>> 8) & 0xff,
+ lo & 0xff
+ ];
+ };
+ /**
+ * Converts this Long to signed.
+ */
+ Long.prototype.toSigned = function () {
+ if (!this.unsigned)
+ return this;
+ return Long.fromBits(this.low, this.high, false);
+ };
+ /**
+ * Converts the Long to a string written in the specified radix.
+ * @param radix - Radix (2-36), defaults to 10
+ * @throws RangeError If `radix` is out of range
+ */
+ Long.prototype.toString = function (radix) {
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix)
+ throw RangeError('radix');
+ if (this.isZero())
+ return '0';
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ if (this.eq(Long.MIN_VALUE)) {
+ // We need to change the Long value before it can be negated, so we remove
+ // the bottom-most digit in this base and then recurse to do the rest.
+ var radixLong = Long.fromNumber(radix), div = this.div(radixLong), rem1 = div.mul(radixLong).sub(this);
+ return div.toString(radix) + rem1.toInt().toString(radix);
+ }
+ else
+ return '-' + this.neg().toString(radix);
+ }
+ // Do several (6) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ var radixToPower = Long.fromNumber(Math.pow(radix, 6), this.unsigned);
+ // eslint-disable-next-line @typescript-eslint/no-this-alias
+ var rem = this;
+ var result = '';
+ // eslint-disable-next-line no-constant-condition
+ while (true) {
+ var remDiv = rem.div(radixToPower);
+ var intval = rem.sub(remDiv.mul(radixToPower)).toInt() >>> 0;
+ var digits = intval.toString(radix);
+ rem = remDiv;
+ if (rem.isZero()) {
+ return digits + result;
+ }
+ else {
+ while (digits.length < 6)
+ digits = '0' + digits;
+ result = '' + digits + result;
+ }
+ }
+ };
+ /** Converts this Long to unsigned. */
+ Long.prototype.toUnsigned = function () {
+ if (this.unsigned)
+ return this;
+ return Long.fromBits(this.low, this.high, true);
+ };
+ /** Returns the bitwise XOR of this Long and the given one. */
+ Long.prototype.xor = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low ^ other.low, this.high ^ other.high, this.unsigned);
+ };
+ /** This is an alias of {@link Long.isZero} */
+ Long.prototype.eqz = function () {
+ return this.isZero();
+ };
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ Long.prototype.le = function (other) {
+ return this.lessThanOrEqual(other);
+ };
+ /*
+ ****************************************************************
+ * BSON SPECIFIC ADDITIONS *
+ ****************************************************************
+ */
+ Long.prototype.toExtendedJSON = function (options) {
+ if (options && options.relaxed)
+ return this.toNumber();
+ return { $numberLong: this.toString() };
+ };
+ Long.fromExtendedJSON = function (doc, options) {
+ var result = Long.fromString(doc.$numberLong);
+ return options && options.relaxed ? result.toNumber() : result;
+ };
+ /** @internal */
+ Long.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Long.prototype.inspect = function () {
+ return "new Long(\"" + this.toString() + "\"" + (this.unsigned ? ', true' : '') + ")";
+ };
+ Long.TWO_PWR_24 = Long.fromInt(TWO_PWR_24_DBL);
+ /** Maximum unsigned value. */
+ Long.MAX_UNSIGNED_VALUE = Long.fromBits(0xffffffff | 0, 0xffffffff | 0, true);
+ /** Signed zero */
+ Long.ZERO = Long.fromInt(0);
+ /** Unsigned zero. */
+ Long.UZERO = Long.fromInt(0, true);
+ /** Signed one. */
+ Long.ONE = Long.fromInt(1);
+ /** Unsigned one. */
+ Long.UONE = Long.fromInt(1, true);
+ /** Signed negative one. */
+ Long.NEG_ONE = Long.fromInt(-1);
+ /** Maximum signed value. */
+ Long.MAX_VALUE = Long.fromBits(0xffffffff | 0, 0x7fffffff | 0, false);
+ /** Minimum signed value. */
+ Long.MIN_VALUE = Long.fromBits(0, 0x80000000 | 0, false);
+ return Long;
+}());
+exports.Long = Long;
+Object.defineProperty(Long.prototype, '__isLong__', { value: true });
+Object.defineProperty(Long.prototype, '_bsontype', { value: 'Long' });
+
+});
+
+unwrapExports(long_1);
+long_1.Long;
+
+var decimal128 = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Decimal128 = void 0;
+
+
+var PARSE_STRING_REGEXP = /^(\+|-)?(\d+|(\d*\.\d*))?(E|e)?([-+])?(\d+)?$/;
+var PARSE_INF_REGEXP = /^(\+|-)?(Infinity|inf)$/i;
+var PARSE_NAN_REGEXP = /^(\+|-)?NaN$/i;
+var EXPONENT_MAX = 6111;
+var EXPONENT_MIN = -6176;
+var EXPONENT_BIAS = 6176;
+var MAX_DIGITS = 34;
+// Nan value bits as 32 bit values (due to lack of longs)
+var NAN_BUFFER = [
+ 0x7c,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+// Infinity value bits 32 bit values (due to lack of longs)
+var INF_NEGATIVE_BUFFER = [
+ 0xf8,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+var INF_POSITIVE_BUFFER = [
+ 0x78,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+var EXPONENT_REGEX = /^([-+])?(\d+)?$/;
+// Extract least significant 5 bits
+var COMBINATION_MASK = 0x1f;
+// Extract least significant 14 bits
+var EXPONENT_MASK = 0x3fff;
+// Value of combination field for Inf
+var COMBINATION_INFINITY = 30;
+// Value of combination field for NaN
+var COMBINATION_NAN = 31;
+// Detect if the value is a digit
+function isDigit(value) {
+ return !isNaN(parseInt(value, 10));
+}
+// Divide two uint128 values
+function divideu128(value) {
+ var DIVISOR = long_1.Long.fromNumber(1000 * 1000 * 1000);
+ var _rem = long_1.Long.fromNumber(0);
+ if (!value.parts[0] && !value.parts[1] && !value.parts[2] && !value.parts[3]) {
+ return { quotient: value, rem: _rem };
+ }
+ for (var i = 0; i <= 3; i++) {
+ // Adjust remainder to match value of next dividend
+ _rem = _rem.shiftLeft(32);
+ // Add the divided to _rem
+ _rem = _rem.add(new long_1.Long(value.parts[i], 0));
+ value.parts[i] = _rem.div(DIVISOR).low;
+ _rem = _rem.modulo(DIVISOR);
+ }
+ return { quotient: value, rem: _rem };
+}
+// Multiply two Long values and return the 128 bit value
+function multiply64x2(left, right) {
+ if (!left && !right) {
+ return { high: long_1.Long.fromNumber(0), low: long_1.Long.fromNumber(0) };
+ }
+ var leftHigh = left.shiftRightUnsigned(32);
+ var leftLow = new long_1.Long(left.getLowBits(), 0);
+ var rightHigh = right.shiftRightUnsigned(32);
+ var rightLow = new long_1.Long(right.getLowBits(), 0);
+ var productHigh = leftHigh.multiply(rightHigh);
+ var productMid = leftHigh.multiply(rightLow);
+ var productMid2 = leftLow.multiply(rightHigh);
+ var productLow = leftLow.multiply(rightLow);
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productMid = new long_1.Long(productMid.getLowBits(), 0)
+ .add(productMid2)
+ .add(productLow.shiftRightUnsigned(32));
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productLow = productMid.shiftLeft(32).add(new long_1.Long(productLow.getLowBits(), 0));
+ // Return the 128 bit result
+ return { high: productHigh, low: productLow };
+}
+function lessThan(left, right) {
+ // Make values unsigned
+ var uhleft = left.high >>> 0;
+ var uhright = right.high >>> 0;
+ // Compare high bits first
+ if (uhleft < uhright) {
+ return true;
+ }
+ else if (uhleft === uhright) {
+ var ulleft = left.low >>> 0;
+ var ulright = right.low >>> 0;
+ if (ulleft < ulright)
+ return true;
+ }
+ return false;
+}
+function invalidErr(string, message) {
+ throw new TypeError("\"" + string + "\" is not a valid Decimal128 string - " + message);
+}
+/**
+ * A class representation of the BSON Decimal128 type.
+ * @public
+ */
+var Decimal128 = /** @class */ (function () {
+ /**
+ * @param bytes - a buffer containing the raw Decimal128 bytes in little endian order,
+ * or a string representation as returned by .toString()
+ */
+ function Decimal128(bytes) {
+ if (!(this instanceof Decimal128))
+ return new Decimal128(bytes);
+ if (typeof bytes === 'string') {
+ this.bytes = Decimal128.fromString(bytes).bytes;
+ }
+ else {
+ this.bytes = bytes;
+ }
+ }
+ /**
+ * Create a Decimal128 instance from a string representation
+ *
+ * @param representation - a numeric string representation.
+ */
+ Decimal128.fromString = function (representation) {
+ // Parse state tracking
+ var isNegative = false;
+ var sawRadix = false;
+ var foundNonZero = false;
+ // Total number of significant digits (no leading or trailing zero)
+ var significantDigits = 0;
+ // Total number of significand digits read
+ var nDigitsRead = 0;
+ // Total number of digits (no leading zeros)
+ var nDigits = 0;
+ // The number of the digits after radix
+ var radixPosition = 0;
+ // The index of the first non-zero in *str*
+ var firstNonZero = 0;
+ // Digits Array
+ var digits = [0];
+ // The number of digits in digits
+ var nDigitsStored = 0;
+ // Insertion pointer for digits
+ var digitsInsert = 0;
+ // The index of the first non-zero digit
+ var firstDigit = 0;
+ // The index of the last digit
+ var lastDigit = 0;
+ // Exponent
+ var exponent = 0;
+ // loop index over array
+ var i = 0;
+ // The high 17 digits of the significand
+ var significandHigh = new long_1.Long(0, 0);
+ // The low 17 digits of the significand
+ var significandLow = new long_1.Long(0, 0);
+ // The biased exponent
+ var biasedExponent = 0;
+ // Read index
+ var index = 0;
+ // Naively prevent against REDOS attacks.
+ // TODO: implementing a custom parsing for this, or refactoring the regex would yield
+ // further gains.
+ if (representation.length >= 7000) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+ // Results
+ var stringMatch = representation.match(PARSE_STRING_REGEXP);
+ var infMatch = representation.match(PARSE_INF_REGEXP);
+ var nanMatch = representation.match(PARSE_NAN_REGEXP);
+ // Validate the string
+ if ((!stringMatch && !infMatch && !nanMatch) || representation.length === 0) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+ if (stringMatch) {
+ // full_match = stringMatch[0]
+ // sign = stringMatch[1]
+ var unsignedNumber = stringMatch[2];
+ // stringMatch[3] is undefined if a whole number (ex "1", 12")
+ // but defined if a number w/ decimal in it (ex "1.0, 12.2")
+ var e = stringMatch[4];
+ var expSign = stringMatch[5];
+ var expNumber = stringMatch[6];
+ // they provided e, but didn't give an exponent number. for ex "1e"
+ if (e && expNumber === undefined)
+ invalidErr(representation, 'missing exponent power');
+ // they provided e, but didn't give a number before it. for ex "e1"
+ if (e && unsignedNumber === undefined)
+ invalidErr(representation, 'missing exponent base');
+ if (e === undefined && (expSign || expNumber)) {
+ invalidErr(representation, 'missing e before exponent');
+ }
+ }
+ // Get the negative or positive sign
+ if (representation[index] === '+' || representation[index] === '-') {
+ isNegative = representation[index++] === '-';
+ }
+ // Check if user passed Infinity or NaN
+ if (!isDigit(representation[index]) && representation[index] !== '.') {
+ if (representation[index] === 'i' || representation[index] === 'I') {
+ return new Decimal128(buffer.Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ }
+ else if (representation[index] === 'N') {
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ }
+ }
+ // Read all the digits
+ while (isDigit(representation[index]) || representation[index] === '.') {
+ if (representation[index] === '.') {
+ if (sawRadix)
+ invalidErr(representation, 'contains multiple periods');
+ sawRadix = true;
+ index = index + 1;
+ continue;
+ }
+ if (nDigitsStored < 34) {
+ if (representation[index] !== '0' || foundNonZero) {
+ if (!foundNonZero) {
+ firstNonZero = nDigitsRead;
+ }
+ foundNonZero = true;
+ // Only store 34 digits
+ digits[digitsInsert++] = parseInt(representation[index], 10);
+ nDigitsStored = nDigitsStored + 1;
+ }
+ }
+ if (foundNonZero)
+ nDigits = nDigits + 1;
+ if (sawRadix)
+ radixPosition = radixPosition + 1;
+ nDigitsRead = nDigitsRead + 1;
+ index = index + 1;
+ }
+ if (sawRadix && !nDigitsRead)
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ // Read exponent if exists
+ if (representation[index] === 'e' || representation[index] === 'E') {
+ // Read exponent digits
+ var match = representation.substr(++index).match(EXPONENT_REGEX);
+ // No digits read
+ if (!match || !match[2])
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ // Get exponent
+ exponent = parseInt(match[0], 10);
+ // Adjust the index
+ index = index + match[0].length;
+ }
+ // Return not a number
+ if (representation[index])
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ // Done reading input
+ // Find first non-zero digit in digits
+ firstDigit = 0;
+ if (!nDigitsStored) {
+ firstDigit = 0;
+ lastDigit = 0;
+ digits[0] = 0;
+ nDigits = 1;
+ nDigitsStored = 1;
+ significantDigits = 0;
+ }
+ else {
+ lastDigit = nDigitsStored - 1;
+ significantDigits = nDigits;
+ if (significantDigits !== 1) {
+ while (representation[firstNonZero + significantDigits - 1] === '0') {
+ significantDigits = significantDigits - 1;
+ }
+ }
+ }
+ // Normalization of exponent
+ // Correct exponent based on radix position, and shift significand as needed
+ // to represent user input
+ // Overflow prevention
+ if (exponent <= radixPosition && radixPosition - exponent > 1 << 14) {
+ exponent = EXPONENT_MIN;
+ }
+ else {
+ exponent = exponent - radixPosition;
+ }
+ // Attempt to normalize the exponent
+ while (exponent > EXPONENT_MAX) {
+ // Shift exponent to significand and decrease
+ lastDigit = lastDigit + 1;
+ if (lastDigit - firstDigit > MAX_DIGITS) {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ var digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ exponent = exponent - 1;
+ }
+ while (exponent < EXPONENT_MIN || nDigitsStored < nDigits) {
+ // Shift last digit. can only do this if < significant digits than # stored.
+ if (lastDigit === 0 && significantDigits < nDigitsStored) {
+ exponent = EXPONENT_MIN;
+ significantDigits = 0;
+ break;
+ }
+ if (nDigitsStored < nDigits) {
+ // adjust to match digits not stored
+ nDigits = nDigits - 1;
+ }
+ else {
+ // adjust to round
+ lastDigit = lastDigit - 1;
+ }
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ }
+ else {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ var digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ }
+ // Round
+ // We've normalized the exponent, but might still need to round.
+ if (lastDigit - firstDigit + 1 < significantDigits) {
+ var endOfString = nDigitsRead;
+ // If we have seen a radix point, 'string' is 1 longer than we have
+ // documented with ndigits_read, so inc the position of the first nonzero
+ // digit and the position that digits are read to.
+ if (sawRadix) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ // if negative, we need to increment again to account for - sign at start.
+ if (isNegative) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ var roundDigit = parseInt(representation[firstNonZero + lastDigit + 1], 10);
+ var roundBit = 0;
+ if (roundDigit >= 5) {
+ roundBit = 1;
+ if (roundDigit === 5) {
+ roundBit = digits[lastDigit] % 2 === 1 ? 1 : 0;
+ for (i = firstNonZero + lastDigit + 2; i < endOfString; i++) {
+ if (parseInt(representation[i], 10)) {
+ roundBit = 1;
+ break;
+ }
+ }
+ }
+ }
+ if (roundBit) {
+ var dIdx = lastDigit;
+ for (; dIdx >= 0; dIdx--) {
+ if (++digits[dIdx] > 9) {
+ digits[dIdx] = 0;
+ // overflowed most significant digit
+ if (dIdx === 0) {
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ digits[dIdx] = 1;
+ }
+ else {
+ return new Decimal128(buffer.Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ }
+ }
+ }
+ }
+ }
+ }
+ // Encode significand
+ // The high 17 digits of the significand
+ significandHigh = long_1.Long.fromNumber(0);
+ // The low 17 digits of the significand
+ significandLow = long_1.Long.fromNumber(0);
+ // read a zero
+ if (significantDigits === 0) {
+ significandHigh = long_1.Long.fromNumber(0);
+ significandLow = long_1.Long.fromNumber(0);
+ }
+ else if (lastDigit - firstDigit < 17) {
+ var dIdx = firstDigit;
+ significandLow = long_1.Long.fromNumber(digits[dIdx++]);
+ significandHigh = new long_1.Long(0, 0);
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(long_1.Long.fromNumber(10));
+ significandLow = significandLow.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ }
+ else {
+ var dIdx = firstDigit;
+ significandHigh = long_1.Long.fromNumber(digits[dIdx++]);
+ for (; dIdx <= lastDigit - 17; dIdx++) {
+ significandHigh = significandHigh.multiply(long_1.Long.fromNumber(10));
+ significandHigh = significandHigh.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ significandLow = long_1.Long.fromNumber(digits[dIdx++]);
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(long_1.Long.fromNumber(10));
+ significandLow = significandLow.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ }
+ var significand = multiply64x2(significandHigh, long_1.Long.fromString('100000000000000000'));
+ significand.low = significand.low.add(significandLow);
+ if (lessThan(significand.low, significandLow)) {
+ significand.high = significand.high.add(long_1.Long.fromNumber(1));
+ }
+ // Biased exponent
+ biasedExponent = exponent + EXPONENT_BIAS;
+ var dec = { low: long_1.Long.fromNumber(0), high: long_1.Long.fromNumber(0) };
+ // Encode combination, exponent, and significand.
+ if (significand.high.shiftRightUnsigned(49).and(long_1.Long.fromNumber(1)).equals(long_1.Long.fromNumber(1))) {
+ // Encode '11' into bits 1 to 3
+ dec.high = dec.high.or(long_1.Long.fromNumber(0x3).shiftLeft(61));
+ dec.high = dec.high.or(long_1.Long.fromNumber(biasedExponent).and(long_1.Long.fromNumber(0x3fff).shiftLeft(47)));
+ dec.high = dec.high.or(significand.high.and(long_1.Long.fromNumber(0x7fffffffffff)));
+ }
+ else {
+ dec.high = dec.high.or(long_1.Long.fromNumber(biasedExponent & 0x3fff).shiftLeft(49));
+ dec.high = dec.high.or(significand.high.and(long_1.Long.fromNumber(0x1ffffffffffff)));
+ }
+ dec.low = significand.low;
+ // Encode sign
+ if (isNegative) {
+ dec.high = dec.high.or(long_1.Long.fromString('9223372036854775808'));
+ }
+ // Encode into a buffer
+ var buffer$1 = buffer.Buffer.alloc(16);
+ index = 0;
+ // Encode the low 64 bits of the decimal
+ // Encode low bits
+ buffer$1[index++] = dec.low.low & 0xff;
+ buffer$1[index++] = (dec.low.low >> 8) & 0xff;
+ buffer$1[index++] = (dec.low.low >> 16) & 0xff;
+ buffer$1[index++] = (dec.low.low >> 24) & 0xff;
+ // Encode high bits
+ buffer$1[index++] = dec.low.high & 0xff;
+ buffer$1[index++] = (dec.low.high >> 8) & 0xff;
+ buffer$1[index++] = (dec.low.high >> 16) & 0xff;
+ buffer$1[index++] = (dec.low.high >> 24) & 0xff;
+ // Encode the high 64 bits of the decimal
+ // Encode low bits
+ buffer$1[index++] = dec.high.low & 0xff;
+ buffer$1[index++] = (dec.high.low >> 8) & 0xff;
+ buffer$1[index++] = (dec.high.low >> 16) & 0xff;
+ buffer$1[index++] = (dec.high.low >> 24) & 0xff;
+ // Encode high bits
+ buffer$1[index++] = dec.high.high & 0xff;
+ buffer$1[index++] = (dec.high.high >> 8) & 0xff;
+ buffer$1[index++] = (dec.high.high >> 16) & 0xff;
+ buffer$1[index++] = (dec.high.high >> 24) & 0xff;
+ // Return the new Decimal128
+ return new Decimal128(buffer$1);
+ };
+ /** Create a string representation of the raw Decimal128 value */
+ Decimal128.prototype.toString = function () {
+ // Note: bits in this routine are referred to starting at 0,
+ // from the sign bit, towards the coefficient.
+ // decoded biased exponent (14 bits)
+ var biased_exponent;
+ // the number of significand digits
+ var significand_digits = 0;
+ // the base-10 digits in the significand
+ var significand = new Array(36);
+ for (var i = 0; i < significand.length; i++)
+ significand[i] = 0;
+ // read pointer into significand
+ var index = 0;
+ // true if the number is zero
+ var is_zero = false;
+ // the most significant significand bits (50-46)
+ var significand_msb;
+ // temporary storage for significand decoding
+ var significand128 = { parts: [0, 0, 0, 0] };
+ // indexing variables
+ var j, k;
+ // Output string
+ var string = [];
+ // Unpack index
+ index = 0;
+ // Buffer reference
+ var buffer = this.bytes;
+ // Unpack the low 64bits into a long
+ // bits 96 - 127
+ var low = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 64 - 95
+ var midl = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Unpack the high 64bits into a long
+ // bits 32 - 63
+ var midh = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 0 - 31
+ var high = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Unpack index
+ index = 0;
+ // Create the state of the decimal
+ var dec = {
+ low: new long_1.Long(low, midl),
+ high: new long_1.Long(midh, high)
+ };
+ if (dec.high.lessThan(long_1.Long.ZERO)) {
+ string.push('-');
+ }
+ // Decode combination field and exponent
+ // bits 1 - 5
+ var combination = (high >> 26) & COMBINATION_MASK;
+ if (combination >> 3 === 3) {
+ // Check for 'special' values
+ if (combination === COMBINATION_INFINITY) {
+ return string.join('') + 'Infinity';
+ }
+ else if (combination === COMBINATION_NAN) {
+ return 'NaN';
+ }
+ else {
+ biased_exponent = (high >> 15) & EXPONENT_MASK;
+ significand_msb = 0x08 + ((high >> 14) & 0x01);
+ }
+ }
+ else {
+ significand_msb = (high >> 14) & 0x07;
+ biased_exponent = (high >> 17) & EXPONENT_MASK;
+ }
+ // unbiased exponent
+ var exponent = biased_exponent - EXPONENT_BIAS;
+ // Create string of significand digits
+ // Convert the 114-bit binary number represented by
+ // (significand_high, significand_low) to at most 34 decimal
+ // digits through modulo and division.
+ significand128.parts[0] = (high & 0x3fff) + ((significand_msb & 0xf) << 14);
+ significand128.parts[1] = midh;
+ significand128.parts[2] = midl;
+ significand128.parts[3] = low;
+ if (significand128.parts[0] === 0 &&
+ significand128.parts[1] === 0 &&
+ significand128.parts[2] === 0 &&
+ significand128.parts[3] === 0) {
+ is_zero = true;
+ }
+ else {
+ for (k = 3; k >= 0; k--) {
+ var least_digits = 0;
+ // Perform the divide
+ var result = divideu128(significand128);
+ significand128 = result.quotient;
+ least_digits = result.rem.low;
+ // We now have the 9 least significant digits (in base 2).
+ // Convert and output to string.
+ if (!least_digits)
+ continue;
+ for (j = 8; j >= 0; j--) {
+ // significand[k * 9 + j] = Math.round(least_digits % 10);
+ significand[k * 9 + j] = least_digits % 10;
+ // least_digits = Math.round(least_digits / 10);
+ least_digits = Math.floor(least_digits / 10);
+ }
+ }
+ }
+ // Output format options:
+ // Scientific - [-]d.dddE(+/-)dd or [-]dE(+/-)dd
+ // Regular - ddd.ddd
+ if (is_zero) {
+ significand_digits = 1;
+ significand[index] = 0;
+ }
+ else {
+ significand_digits = 36;
+ while (!significand[index]) {
+ significand_digits = significand_digits - 1;
+ index = index + 1;
+ }
+ }
+ // the exponent if scientific notation is used
+ var scientific_exponent = significand_digits - 1 + exponent;
+ // The scientific exponent checks are dictated by the string conversion
+ // specification and are somewhat arbitrary cutoffs.
+ //
+ // We must check exponent > 0, because if this is the case, the number
+ // has trailing zeros. However, we *cannot* output these trailing zeros,
+ // because doing so would change the precision of the value, and would
+ // change stored data if the string converted number is round tripped.
+ if (scientific_exponent >= 34 || scientific_exponent <= -7 || exponent > 0) {
+ // Scientific format
+ // if there are too many significant digits, we should just be treating numbers
+ // as + or - 0 and using the non-scientific exponent (this is for the "invalid
+ // representation should be treated as 0/-0" spec cases in decimal128-1.json)
+ if (significand_digits > 34) {
+ string.push("" + 0);
+ if (exponent > 0)
+ string.push('E+' + exponent);
+ else if (exponent < 0)
+ string.push('E' + exponent);
+ return string.join('');
+ }
+ string.push("" + significand[index++]);
+ significand_digits = significand_digits - 1;
+ if (significand_digits) {
+ string.push('.');
+ }
+ for (var i = 0; i < significand_digits; i++) {
+ string.push("" + significand[index++]);
+ }
+ // Exponent
+ string.push('E');
+ if (scientific_exponent > 0) {
+ string.push('+' + scientific_exponent);
+ }
+ else {
+ string.push("" + scientific_exponent);
+ }
+ }
+ else {
+ // Regular format with no decimal place
+ if (exponent >= 0) {
+ for (var i = 0; i < significand_digits; i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ else {
+ var radix_position = significand_digits + exponent;
+ // non-zero digits before radix
+ if (radix_position > 0) {
+ for (var i = 0; i < radix_position; i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ else {
+ string.push('0');
+ }
+ string.push('.');
+ // add leading zeros after radix
+ while (radix_position++ < 0) {
+ string.push('0');
+ }
+ for (var i = 0; i < significand_digits - Math.max(radix_position - 1, 0); i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ }
+ return string.join('');
+ };
+ Decimal128.prototype.toJSON = function () {
+ return { $numberDecimal: this.toString() };
+ };
+ /** @internal */
+ Decimal128.prototype.toExtendedJSON = function () {
+ return { $numberDecimal: this.toString() };
+ };
+ /** @internal */
+ Decimal128.fromExtendedJSON = function (doc) {
+ return Decimal128.fromString(doc.$numberDecimal);
+ };
+ /** @internal */
+ Decimal128.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Decimal128.prototype.inspect = function () {
+ return "new Decimal128(\"" + this.toString() + "\")";
+ };
+ return Decimal128;
+}());
+exports.Decimal128 = Decimal128;
+Object.defineProperty(Decimal128.prototype, '_bsontype', { value: 'Decimal128' });
+
+});
+
+unwrapExports(decimal128);
+decimal128.Decimal128;
+
+var double_1 = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Double = void 0;
+/**
+ * A class representation of the BSON Double type.
+ * @public
+ */
+var Double = /** @class */ (function () {
+ /**
+ * Create a Double type
+ *
+ * @param value - the number we want to represent as a double.
+ */
+ function Double(value) {
+ if (!(this instanceof Double))
+ return new Double(value);
+ if (value instanceof Number) {
+ value = value.valueOf();
+ }
+ this.value = +value;
+ }
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped double number.
+ */
+ Double.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ Double.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ Double.prototype.toExtendedJSON = function (options) {
+ if (options && (options.legacy || (options.relaxed && isFinite(this.value)))) {
+ return this.value;
+ }
+ // NOTE: JavaScript has +0 and -0, apparently to model limit calculations. If a user
+ // explicitly provided `-0` then we need to ensure the sign makes it into the output
+ if (Object.is(Math.sign(this.value), -0)) {
+ return { $numberDouble: "-" + this.value.toFixed(1) };
+ }
+ var $numberDouble;
+ if (Number.isInteger(this.value)) {
+ $numberDouble = this.value.toFixed(1);
+ if ($numberDouble.length >= 13) {
+ $numberDouble = this.value.toExponential(13).toUpperCase();
+ }
+ }
+ else {
+ $numberDouble = this.value.toString();
+ }
+ return { $numberDouble: $numberDouble };
+ };
+ /** @internal */
+ Double.fromExtendedJSON = function (doc, options) {
+ var doubleValue = parseFloat(doc.$numberDouble);
+ return options && options.relaxed ? doubleValue : new Double(doubleValue);
+ };
+ /** @internal */
+ Double.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Double.prototype.inspect = function () {
+ var eJSON = this.toExtendedJSON();
+ return "new Double(" + eJSON.$numberDouble + ")";
+ };
+ return Double;
+}());
+exports.Double = Double;
+Object.defineProperty(Double.prototype, '_bsontype', { value: 'Double' });
+
+});
+
+unwrapExports(double_1);
+double_1.Double;
+
+var int_32 = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Int32 = void 0;
+/**
+ * A class representation of a BSON Int32 type.
+ * @public
+ */
+var Int32 = /** @class */ (function () {
+ /**
+ * Create an Int32 type
+ *
+ * @param value - the number we want to represent as an int32.
+ */
+ function Int32(value) {
+ if (!(this instanceof Int32))
+ return new Int32(value);
+ if (value instanceof Number) {
+ value = value.valueOf();
+ }
+ this.value = +value;
+ }
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped int32 number.
+ */
+ Int32.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ Int32.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ Int32.prototype.toExtendedJSON = function (options) {
+ if (options && (options.relaxed || options.legacy))
+ return this.value;
+ return { $numberInt: this.value.toString() };
+ };
+ /** @internal */
+ Int32.fromExtendedJSON = function (doc, options) {
+ return options && options.relaxed ? parseInt(doc.$numberInt, 10) : new Int32(doc.$numberInt);
+ };
+ /** @internal */
+ Int32.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Int32.prototype.inspect = function () {
+ return "new Int32(" + this.valueOf() + ")";
+ };
+ return Int32;
+}());
+exports.Int32 = Int32;
+Object.defineProperty(Int32.prototype, '_bsontype', { value: 'Int32' });
+
+});
+
+unwrapExports(int_32);
+int_32.Int32;
+
+var max_key = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.MaxKey = void 0;
+/**
+ * A class representation of the BSON MaxKey type.
+ * @public
+ */
+var MaxKey = /** @class */ (function () {
+ function MaxKey() {
+ if (!(this instanceof MaxKey))
+ return new MaxKey();
+ }
+ /** @internal */
+ MaxKey.prototype.toExtendedJSON = function () {
+ return { $maxKey: 1 };
+ };
+ /** @internal */
+ MaxKey.fromExtendedJSON = function () {
+ return new MaxKey();
+ };
+ /** @internal */
+ MaxKey.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ MaxKey.prototype.inspect = function () {
+ return 'new MaxKey()';
+ };
+ return MaxKey;
+}());
+exports.MaxKey = MaxKey;
+Object.defineProperty(MaxKey.prototype, '_bsontype', { value: 'MaxKey' });
+
+});
+
+unwrapExports(max_key);
+max_key.MaxKey;
+
+var min_key = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.MinKey = void 0;
+/**
+ * A class representation of the BSON MinKey type.
+ * @public
+ */
+var MinKey = /** @class */ (function () {
+ function MinKey() {
+ if (!(this instanceof MinKey))
+ return new MinKey();
+ }
+ /** @internal */
+ MinKey.prototype.toExtendedJSON = function () {
+ return { $minKey: 1 };
+ };
+ /** @internal */
+ MinKey.fromExtendedJSON = function () {
+ return new MinKey();
+ };
+ /** @internal */
+ MinKey.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ MinKey.prototype.inspect = function () {
+ return 'new MinKey()';
+ };
+ return MinKey;
+}());
+exports.MinKey = MinKey;
+Object.defineProperty(MinKey.prototype, '_bsontype', { value: 'MinKey' });
+
+});
+
+unwrapExports(min_key);
+min_key.MinKey;
+
+var objectid = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.ObjectId = void 0;
+
+
+
+// Regular expression that checks for hex value
+var checkForHexRegExp = new RegExp('^[0-9a-fA-F]{24}$');
+// Unique sequence for the current process (initialized on first use)
+var PROCESS_UNIQUE = null;
+var kId = Symbol('id');
+/**
+ * A class representation of the BSON ObjectId type.
+ * @public
+ */
+var ObjectId = /** @class */ (function () {
+ /**
+ * Create an ObjectId type
+ *
+ * @param id - Can be a 24 character hex string, 12 byte binary Buffer, or a number.
+ */
+ function ObjectId(id) {
+ if (!(this instanceof ObjectId))
+ return new ObjectId(id);
+ // Duck-typing to support ObjectId from different npm packages
+ if (id instanceof ObjectId) {
+ this[kId] = id.id;
+ this.__id = id.__id;
+ }
+ if (typeof id === 'object' && id && 'id' in id) {
+ if ('toHexString' in id && typeof id.toHexString === 'function') {
+ this[kId] = buffer.Buffer.from(id.toHexString(), 'hex');
+ }
+ else {
+ this[kId] = typeof id.id === 'string' ? buffer.Buffer.from(id.id) : id.id;
+ }
+ }
+ // The most common use case (blank id, new objectId instance)
+ if (id == null || typeof id === 'number') {
+ // Generate a new id
+ this[kId] = ObjectId.generate(typeof id === 'number' ? id : undefined);
+ // If we are caching the hex string
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+ if (ArrayBuffer.isView(id) && id.byteLength === 12) {
+ this[kId] = ensure_buffer.ensureBuffer(id);
+ }
+ if (typeof id === 'string') {
+ if (id.length === 12) {
+ var bytes = buffer.Buffer.from(id);
+ if (bytes.byteLength === 12) {
+ this[kId] = bytes;
+ }
+ }
+ else if (id.length === 24 && checkForHexRegExp.test(id)) {
+ this[kId] = buffer.Buffer.from(id, 'hex');
+ }
+ else {
+ throw new TypeError('Argument passed in must be a Buffer or string of 12 bytes or a string of 24 hex characters');
+ }
+ }
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+ Object.defineProperty(ObjectId.prototype, "id", {
+ /**
+ * The ObjectId bytes
+ * @readonly
+ */
+ get: function () {
+ return this[kId];
+ },
+ set: function (value) {
+ this[kId] = value;
+ if (ObjectId.cacheHexString) {
+ this.__id = value.toString('hex');
+ }
+ },
+ enumerable: false,
+ configurable: true
+ });
+ Object.defineProperty(ObjectId.prototype, "generationTime", {
+ /**
+ * The generation time of this ObjectId instance
+ * @deprecated Please use getTimestamp / createFromTime which returns an int32 epoch
+ */
+ get: function () {
+ return this.id.readInt32BE(0);
+ },
+ set: function (value) {
+ // Encode time into first 4 bytes
+ this.id.writeUInt32BE(value, 0);
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /** Returns the ObjectId id as a 24 character hex string representation */
+ ObjectId.prototype.toHexString = function () {
+ if (ObjectId.cacheHexString && this.__id) {
+ return this.__id;
+ }
+ var hexString = this.id.toString('hex');
+ if (ObjectId.cacheHexString && !this.__id) {
+ this.__id = hexString;
+ }
+ return hexString;
+ };
+ /**
+ * Update the ObjectId index
+ * @privateRemarks
+ * Used in generating new ObjectId's on the driver
+ * @internal
+ */
+ ObjectId.getInc = function () {
+ return (ObjectId.index = (ObjectId.index + 1) % 0xffffff);
+ };
+ /**
+ * Generate a 12 byte id buffer used in ObjectId's
+ *
+ * @param time - pass in a second based timestamp.
+ */
+ ObjectId.generate = function (time) {
+ if ('number' !== typeof time) {
+ time = ~~(Date.now() / 1000);
+ }
+ var inc = ObjectId.getInc();
+ var buffer$1 = buffer.Buffer.alloc(12);
+ // 4-byte timestamp
+ buffer$1.writeUInt32BE(time, 0);
+ // set PROCESS_UNIQUE if yet not initialized
+ if (PROCESS_UNIQUE === null) {
+ PROCESS_UNIQUE = utils.randomBytes(5);
+ }
+ // 5-byte process unique
+ buffer$1[4] = PROCESS_UNIQUE[0];
+ buffer$1[5] = PROCESS_UNIQUE[1];
+ buffer$1[6] = PROCESS_UNIQUE[2];
+ buffer$1[7] = PROCESS_UNIQUE[3];
+ buffer$1[8] = PROCESS_UNIQUE[4];
+ // 3-byte counter
+ buffer$1[11] = inc & 0xff;
+ buffer$1[10] = (inc >> 8) & 0xff;
+ buffer$1[9] = (inc >> 16) & 0xff;
+ return buffer$1;
+ };
+ /**
+ * Converts the id into a 24 character hex string for printing
+ *
+ * @param format - The Buffer toString format parameter.
+ * @internal
+ */
+ ObjectId.prototype.toString = function (format) {
+ // Is the id a buffer then use the buffer toString method to return the format
+ if (format)
+ return this.id.toString(format);
+ return this.toHexString();
+ };
+ /**
+ * Converts to its JSON the 24 character hex string representation.
+ * @internal
+ */
+ ObjectId.prototype.toJSON = function () {
+ return this.toHexString();
+ };
+ /**
+ * Compares the equality of this ObjectId with `otherID`.
+ *
+ * @param otherId - ObjectId instance to compare against.
+ */
+ ObjectId.prototype.equals = function (otherId) {
+ if (otherId === undefined || otherId === null) {
+ return false;
+ }
+ if (otherId instanceof ObjectId) {
+ return this.toString() === otherId.toString();
+ }
+ if (typeof otherId === 'string' &&
+ ObjectId.isValid(otherId) &&
+ otherId.length === 12 &&
+ utils.isUint8Array(this.id)) {
+ return otherId === buffer.Buffer.prototype.toString.call(this.id, 'latin1');
+ }
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 24) {
+ return otherId.toLowerCase() === this.toHexString();
+ }
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 12) {
+ return buffer.Buffer.from(otherId).equals(this.id);
+ }
+ if (typeof otherId === 'object' &&
+ 'toHexString' in otherId &&
+ typeof otherId.toHexString === 'function') {
+ return otherId.toHexString() === this.toHexString();
+ }
+ return false;
+ };
+ /** Returns the generation date (accurate up to the second) that this ID was generated. */
+ ObjectId.prototype.getTimestamp = function () {
+ var timestamp = new Date();
+ var time = this.id.readUInt32BE(0);
+ timestamp.setTime(Math.floor(time) * 1000);
+ return timestamp;
+ };
+ /** @internal */
+ ObjectId.createPk = function () {
+ return new ObjectId();
+ };
+ /**
+ * Creates an ObjectId from a second based number, with the rest of the ObjectId zeroed out. Used for comparisons or sorting the ObjectId.
+ *
+ * @param time - an integer number representing a number of seconds.
+ */
+ ObjectId.createFromTime = function (time) {
+ var buffer$1 = buffer.Buffer.from([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]);
+ // Encode time into first 4 bytes
+ buffer$1.writeUInt32BE(time, 0);
+ // Return the new objectId
+ return new ObjectId(buffer$1);
+ };
+ /**
+ * Creates an ObjectId from a hex string representation of an ObjectId.
+ *
+ * @param hexString - create a ObjectId from a passed in 24 character hexstring.
+ */
+ ObjectId.createFromHexString = function (hexString) {
+ // Throw an error if it's not a valid setup
+ if (typeof hexString === 'undefined' || (hexString != null && hexString.length !== 24)) {
+ throw new TypeError('Argument passed in must be a single String of 12 bytes or a string of 24 hex characters');
+ }
+ return new ObjectId(buffer.Buffer.from(hexString, 'hex'));
+ };
+ /**
+ * Checks if a value is a valid bson ObjectId
+ *
+ * @param id - ObjectId instance to validate.
+ */
+ ObjectId.isValid = function (id) {
+ if (id == null)
+ return false;
+ if (typeof id === 'number') {
+ return true;
+ }
+ if (typeof id === 'string') {
+ return id.length === 12 || (id.length === 24 && checkForHexRegExp.test(id));
+ }
+ if (id instanceof ObjectId) {
+ return true;
+ }
+ if (utils.isUint8Array(id) && id.length === 12) {
+ return true;
+ }
+ // Duck-Typing detection of ObjectId like objects
+ if (typeof id === 'object' && 'toHexString' in id && typeof id.toHexString === 'function') {
+ if (typeof id.id === 'string') {
+ return id.id.length === 12;
+ }
+ return id.toHexString().length === 24 && checkForHexRegExp.test(id.id.toString('hex'));
+ }
+ return false;
+ };
+ /** @internal */
+ ObjectId.prototype.toExtendedJSON = function () {
+ if (this.toHexString)
+ return { $oid: this.toHexString() };
+ return { $oid: this.toString('hex') };
+ };
+ /** @internal */
+ ObjectId.fromExtendedJSON = function (doc) {
+ return new ObjectId(doc.$oid);
+ };
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 24 character hex string representation.
+ * @internal
+ */
+ ObjectId.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ ObjectId.prototype.inspect = function () {
+ return "new ObjectId(\"" + this.toHexString() + "\")";
+ };
+ /** @internal */
+ ObjectId.index = ~~(Math.random() * 0xffffff);
+ return ObjectId;
+}());
+exports.ObjectId = ObjectId;
+// Deprecated methods
+Object.defineProperty(ObjectId.prototype, 'generate', {
+ value: utils.deprecate(function (time) { return ObjectId.generate(time); }, 'Please use the static `ObjectId.generate(time)` instead')
+});
+Object.defineProperty(ObjectId.prototype, 'getInc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+});
+Object.defineProperty(ObjectId.prototype, 'get_inc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+});
+Object.defineProperty(ObjectId, 'get_inc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+});
+Object.defineProperty(ObjectId.prototype, '_bsontype', { value: 'ObjectID' });
+
+});
+
+unwrapExports(objectid);
+objectid.ObjectId;
+
+var regexp = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.BSONRegExp = void 0;
+function alphabetize(str) {
+ return str.split('').sort().join('');
+}
+/**
+ * A class representation of the BSON RegExp type.
+ * @public
+ */
+var BSONRegExp = /** @class */ (function () {
+ /**
+ * @param pattern - The regular expression pattern to match
+ * @param options - The regular expression options
+ */
+ function BSONRegExp(pattern, options) {
+ if (!(this instanceof BSONRegExp))
+ return new BSONRegExp(pattern, options);
+ this.pattern = pattern;
+ this.options = alphabetize(options !== null && options !== void 0 ? options : '');
+ // Validate options
+ for (var i = 0; i < this.options.length; i++) {
+ if (!(this.options[i] === 'i' ||
+ this.options[i] === 'm' ||
+ this.options[i] === 'x' ||
+ this.options[i] === 'l' ||
+ this.options[i] === 's' ||
+ this.options[i] === 'u')) {
+ throw new Error("The regular expression option [" + this.options[i] + "] is not supported");
+ }
+ }
+ }
+ BSONRegExp.parseOptions = function (options) {
+ return options ? options.split('').sort().join('') : '';
+ };
+ /** @internal */
+ BSONRegExp.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ if (options.legacy) {
+ return { $regex: this.pattern, $options: this.options };
+ }
+ return { $regularExpression: { pattern: this.pattern, options: this.options } };
+ };
+ /** @internal */
+ BSONRegExp.fromExtendedJSON = function (doc) {
+ if ('$regex' in doc) {
+ if (typeof doc.$regex !== 'string') {
+ // This is for $regex query operators that have extended json values.
+ if (doc.$regex._bsontype === 'BSONRegExp') {
+ return doc;
+ }
+ }
+ else {
+ return new BSONRegExp(doc.$regex, BSONRegExp.parseOptions(doc.$options));
+ }
+ }
+ if ('$regularExpression' in doc) {
+ return new BSONRegExp(doc.$regularExpression.pattern, BSONRegExp.parseOptions(doc.$regularExpression.options));
+ }
+ throw new TypeError("Unexpected BSONRegExp EJSON object form: " + JSON.stringify(doc));
+ };
+ return BSONRegExp;
+}());
+exports.BSONRegExp = BSONRegExp;
+Object.defineProperty(BSONRegExp.prototype, '_bsontype', { value: 'BSONRegExp' });
+
+});
+
+unwrapExports(regexp);
+regexp.BSONRegExp;
+
+var symbol = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.BSONSymbol = void 0;
+/**
+ * A class representation of the BSON Symbol type.
+ * @public
+ */
+var BSONSymbol = /** @class */ (function () {
+ /**
+ * @param value - the string representing the symbol.
+ */
+ function BSONSymbol(value) {
+ if (!(this instanceof BSONSymbol))
+ return new BSONSymbol(value);
+ this.value = value;
+ }
+ /** Access the wrapped string value. */
+ BSONSymbol.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.toString = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.inspect = function () {
+ return "new BSONSymbol(\"" + this.value + "\")";
+ };
+ /** @internal */
+ BSONSymbol.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.toExtendedJSON = function () {
+ return { $symbol: this.value };
+ };
+ /** @internal */
+ BSONSymbol.fromExtendedJSON = function (doc) {
+ return new BSONSymbol(doc.$symbol);
+ };
+ /** @internal */
+ BSONSymbol.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ return BSONSymbol;
+}());
+exports.BSONSymbol = BSONSymbol;
+Object.defineProperty(BSONSymbol.prototype, '_bsontype', { value: 'Symbol' });
+
+});
+
+unwrapExports(symbol);
+symbol.BSONSymbol;
+
+/*! *****************************************************************************
+Copyright (c) Microsoft Corporation.
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH
+REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY
+AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,
+INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM
+LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR
+OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR
+PERFORMANCE OF THIS SOFTWARE.
+***************************************************************************** */
+
+/* global Reflect, Promise */
+var _extendStatics = function extendStatics(d, b) {
+ _extendStatics = Object.setPrototypeOf || {
+ __proto__: []
+ } instanceof Array && function (d, b) {
+ d.__proto__ = b;
+ } || function (d, b) {
+ for (var p in b) {
+ if (b.hasOwnProperty(p)) d[p] = b[p];
+ }
+ };
+
+ return _extendStatics(d, b);
+};
+
+function __extends(d, b) {
+ _extendStatics(d, b);
+
+ function __() {
+ this.constructor = d;
+ }
+
+ d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
+}
+
+var _assign = function __assign() {
+ _assign = Object.assign || function __assign(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+
+ for (var p in s) {
+ if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p];
+ }
+ }
+
+ return t;
+ };
+
+ return _assign.apply(this, arguments);
+};
+function __rest(s, e) {
+ var t = {};
+
+ for (var p in s) {
+ if (Object.prototype.hasOwnProperty.call(s, p) && e.indexOf(p) < 0) t[p] = s[p];
+ }
+
+ if (s != null && typeof Object.getOwnPropertySymbols === "function") for (var i = 0, p = Object.getOwnPropertySymbols(s); i < p.length; i++) {
+ if (e.indexOf(p[i]) < 0 && Object.prototype.propertyIsEnumerable.call(s, p[i])) t[p[i]] = s[p[i]];
+ }
+ return t;
+}
+function __decorate(decorators, target, key, desc) {
+ var c = arguments.length,
+ r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc,
+ d;
+ if ((typeof Reflect === "undefined" ? "undefined" : babelHelpers["typeof"](Reflect)) === "object" && typeof Reflect.decorate === "function") r = Reflect.decorate(decorators, target, key, desc);else for (var i = decorators.length - 1; i >= 0; i--) {
+ if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;
+ }
+ return c > 3 && r && Object.defineProperty(target, key, r), r;
+}
+function __param(paramIndex, decorator) {
+ return function (target, key) {
+ decorator(target, key, paramIndex);
+ };
+}
+function __metadata(metadataKey, metadataValue) {
+ if ((typeof Reflect === "undefined" ? "undefined" : babelHelpers["typeof"](Reflect)) === "object" && typeof Reflect.metadata === "function") return Reflect.metadata(metadataKey, metadataValue);
+}
+function __awaiter(thisArg, _arguments, P, generator) {
+ function adopt(value) {
+ return value instanceof P ? value : new P(function (resolve) {
+ resolve(value);
+ });
+ }
+
+ return new (P || (P = Promise))(function (resolve, reject) {
+ function fulfilled(value) {
+ try {
+ step(generator.next(value));
+ } catch (e) {
+ reject(e);
+ }
+ }
+
+ function rejected(value) {
+ try {
+ step(generator["throw"](value));
+ } catch (e) {
+ reject(e);
+ }
+ }
+
+ function step(result) {
+ result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected);
+ }
+
+ step((generator = generator.apply(thisArg, _arguments || [])).next());
+ });
+}
+function __generator(thisArg, body) {
+ var _ = {
+ label: 0,
+ sent: function sent() {
+ if (t[0] & 1) throw t[1];
+ return t[1];
+ },
+ trys: [],
+ ops: []
+ },
+ f,
+ y,
+ t,
+ g;
+ return g = {
+ next: verb(0),
+ "throw": verb(1),
+ "return": verb(2)
+ }, typeof Symbol === "function" && (g[Symbol.iterator] = function () {
+ return this;
+ }), g;
+
+ function verb(n) {
+ return function (v) {
+ return step([n, v]);
+ };
+ }
+
+ function step(op) {
+ if (f) throw new TypeError("Generator is already executing.");
+
+ while (_) {
+ try {
+ if (f = 1, y && (t = op[0] & 2 ? y["return"] : op[0] ? y["throw"] || ((t = y["return"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done) return t;
+ if (y = 0, t) op = [op[0] & 2, t.value];
+
+ switch (op[0]) {
+ case 0:
+ case 1:
+ t = op;
+ break;
+
+ case 4:
+ _.label++;
+ return {
+ value: op[1],
+ done: false
+ };
+
+ case 5:
+ _.label++;
+ y = op[1];
+ op = [0];
+ continue;
+
+ case 7:
+ op = _.ops.pop();
+
+ _.trys.pop();
+
+ continue;
+
+ default:
+ if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) {
+ _ = 0;
+ continue;
+ }
+
+ if (op[0] === 3 && (!t || op[1] > t[0] && op[1] < t[3])) {
+ _.label = op[1];
+ break;
+ }
+
+ if (op[0] === 6 && _.label < t[1]) {
+ _.label = t[1];
+ t = op;
+ break;
+ }
+
+ if (t && _.label < t[2]) {
+ _.label = t[2];
+
+ _.ops.push(op);
+
+ break;
+ }
+
+ if (t[2]) _.ops.pop();
+
+ _.trys.pop();
+
+ continue;
+ }
+
+ op = body.call(thisArg, _);
+ } catch (e) {
+ op = [6, e];
+ y = 0;
+ } finally {
+ f = t = 0;
+ }
+ }
+
+ if (op[0] & 5) throw op[1];
+ return {
+ value: op[0] ? op[1] : void 0,
+ done: true
+ };
+ }
+}
+function __createBinding(o, m, k, k2) {
+ if (k2 === undefined) k2 = k;
+ o[k2] = m[k];
+}
+function __exportStar(m, exports) {
+ for (var p in m) {
+ if (p !== "default" && !exports.hasOwnProperty(p)) exports[p] = m[p];
+ }
+}
+function __values(o) {
+ var s = typeof Symbol === "function" && Symbol.iterator,
+ m = s && o[s],
+ i = 0;
+ if (m) return m.call(o);
+ if (o && typeof o.length === "number") return {
+ next: function next() {
+ if (o && i >= o.length) o = void 0;
+ return {
+ value: o && o[i++],
+ done: !o
+ };
+ }
+ };
+ throw new TypeError(s ? "Object is not iterable." : "Symbol.iterator is not defined.");
+}
+function __read(o, n) {
+ var m = typeof Symbol === "function" && o[Symbol.iterator];
+ if (!m) return o;
+ var i = m.call(o),
+ r,
+ ar = [],
+ e;
+
+ try {
+ while ((n === void 0 || n-- > 0) && !(r = i.next()).done) {
+ ar.push(r.value);
+ }
+ } catch (error) {
+ e = {
+ error: error
+ };
+ } finally {
+ try {
+ if (r && !r.done && (m = i["return"])) m.call(i);
+ } finally {
+ if (e) throw e.error;
+ }
+ }
+
+ return ar;
+}
+function __spread() {
+ for (var ar = [], i = 0; i < arguments.length; i++) {
+ ar = ar.concat(__read(arguments[i]));
+ }
+
+ return ar;
+}
+function __spreadArrays() {
+ for (var s = 0, i = 0, il = arguments.length; i < il; i++) {
+ s += arguments[i].length;
+ }
+
+ for (var r = Array(s), k = 0, i = 0; i < il; i++) {
+ for (var a = arguments[i], j = 0, jl = a.length; j < jl; j++, k++) {
+ r[k] = a[j];
+ }
+ }
+
+ return r;
+}
+function __await(v) {
+ return this instanceof __await ? (this.v = v, this) : new __await(v);
+}
+function __asyncGenerator(thisArg, _arguments, generator) {
+ if (!Symbol.asyncIterator) throw new TypeError("Symbol.asyncIterator is not defined.");
+ var g = generator.apply(thisArg, _arguments || []),
+ i,
+ q = [];
+ return i = {}, verb("next"), verb("throw"), verb("return"), i[Symbol.asyncIterator] = function () {
+ return this;
+ }, i;
+
+ function verb(n) {
+ if (g[n]) i[n] = function (v) {
+ return new Promise(function (a, b) {
+ q.push([n, v, a, b]) > 1 || resume(n, v);
+ });
+ };
+ }
+
+ function resume(n, v) {
+ try {
+ step(g[n](v));
+ } catch (e) {
+ settle(q[0][3], e);
+ }
+ }
+
+ function step(r) {
+ r.value instanceof __await ? Promise.resolve(r.value.v).then(fulfill, reject) : settle(q[0][2], r);
+ }
+
+ function fulfill(value) {
+ resume("next", value);
+ }
+
+ function reject(value) {
+ resume("throw", value);
+ }
+
+ function settle(f, v) {
+ if (f(v), q.shift(), q.length) resume(q[0][0], q[0][1]);
+ }
+}
+function __asyncDelegator(o) {
+ var i, p;
+ return i = {}, verb("next"), verb("throw", function (e) {
+ throw e;
+ }), verb("return"), i[Symbol.iterator] = function () {
+ return this;
+ }, i;
+
+ function verb(n, f) {
+ i[n] = o[n] ? function (v) {
+ return (p = !p) ? {
+ value: __await(o[n](v)),
+ done: n === "return"
+ } : f ? f(v) : v;
+ } : f;
+ }
+}
+function __asyncValues(o) {
+ if (!Symbol.asyncIterator) throw new TypeError("Symbol.asyncIterator is not defined.");
+ var m = o[Symbol.asyncIterator],
+ i;
+ return m ? m.call(o) : (o = typeof __values === "function" ? __values(o) : o[Symbol.iterator](), i = {}, verb("next"), verb("throw"), verb("return"), i[Symbol.asyncIterator] = function () {
+ return this;
+ }, i);
+
+ function verb(n) {
+ i[n] = o[n] && function (v) {
+ return new Promise(function (resolve, reject) {
+ v = o[n](v), settle(resolve, reject, v.done, v.value);
+ });
+ };
+ }
+
+ function settle(resolve, reject, d, v) {
+ Promise.resolve(v).then(function (v) {
+ resolve({
+ value: v,
+ done: d
+ });
+ }, reject);
+ }
+}
+function __makeTemplateObject(cooked, raw) {
+ if (Object.defineProperty) {
+ Object.defineProperty(cooked, "raw", {
+ value: raw
+ });
+ } else {
+ cooked.raw = raw;
+ }
+
+ return cooked;
+}
+function __importStar(mod) {
+ if (mod && mod.__esModule) return mod;
+ var result = {};
+ if (mod != null) for (var k in mod) {
+ if (Object.hasOwnProperty.call(mod, k)) result[k] = mod[k];
+ }
+ result["default"] = mod;
+ return result;
+}
+function __importDefault(mod) {
+ return mod && mod.__esModule ? mod : {
+ "default": mod
+ };
+}
+function __classPrivateFieldGet(receiver, privateMap) {
+ if (!privateMap.has(receiver)) {
+ throw new TypeError("attempted to get private field on non-instance");
+ }
+
+ return privateMap.get(receiver);
+}
+function __classPrivateFieldSet(receiver, privateMap, value) {
+ if (!privateMap.has(receiver)) {
+ throw new TypeError("attempted to set private field on non-instance");
+ }
+
+ privateMap.set(receiver, value);
+ return value;
+}
+
+var tslib_es6 = /*#__PURE__*/Object.freeze({
+ __proto__: null,
+ __extends: __extends,
+ get __assign () { return _assign; },
+ __rest: __rest,
+ __decorate: __decorate,
+ __param: __param,
+ __metadata: __metadata,
+ __awaiter: __awaiter,
+ __generator: __generator,
+ __createBinding: __createBinding,
+ __exportStar: __exportStar,
+ __values: __values,
+ __read: __read,
+ __spread: __spread,
+ __spreadArrays: __spreadArrays,
+ __await: __await,
+ __asyncGenerator: __asyncGenerator,
+ __asyncDelegator: __asyncDelegator,
+ __asyncValues: __asyncValues,
+ __makeTemplateObject: __makeTemplateObject,
+ __importStar: __importStar,
+ __importDefault: __importDefault,
+ __classPrivateFieldGet: __classPrivateFieldGet,
+ __classPrivateFieldSet: __classPrivateFieldSet
+});
+
+var timestamp = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Timestamp = exports.LongWithoutOverridesClass = void 0;
+
+
+/** @public */
+exports.LongWithoutOverridesClass = long_1.Long;
+/** @public */
+var Timestamp = /** @class */ (function (_super) {
+ tslib_es6.__extends(Timestamp, _super);
+ function Timestamp(low, high) {
+ var _this = this;
+ // eslint-disable-next-line @typescript-eslint/ban-ts-comment
+ ///@ts-expect-error
+ if (!(_this instanceof Timestamp))
+ return new Timestamp(low, high);
+ if (long_1.Long.isLong(low)) {
+ _this = _super.call(this, low.low, low.high, true) || this;
+ }
+ else {
+ _this = _super.call(this, low, high, true) || this;
+ }
+ Object.defineProperty(_this, '_bsontype', {
+ value: 'Timestamp',
+ writable: false,
+ configurable: false,
+ enumerable: false
+ });
+ return _this;
+ }
+ Timestamp.prototype.toJSON = function () {
+ return {
+ $timestamp: this.toString()
+ };
+ };
+ /** Returns a Timestamp represented by the given (32-bit) integer value. */
+ Timestamp.fromInt = function (value) {
+ return new Timestamp(long_1.Long.fromInt(value, true));
+ };
+ /** Returns a Timestamp representing the given number value, provided that it is a finite number. Otherwise, zero is returned. */
+ Timestamp.fromNumber = function (value) {
+ return new Timestamp(long_1.Long.fromNumber(value, true));
+ };
+ /**
+ * Returns a Timestamp for the given high and low bits. Each is assumed to use 32 bits.
+ *
+ * @param lowBits - the low 32-bits.
+ * @param highBits - the high 32-bits.
+ */
+ Timestamp.fromBits = function (lowBits, highBits) {
+ return new Timestamp(lowBits, highBits);
+ };
+ /**
+ * Returns a Timestamp from the given string, optionally using the given radix.
+ *
+ * @param str - the textual representation of the Timestamp.
+ * @param optRadix - the radix in which the text is written.
+ */
+ Timestamp.fromString = function (str, optRadix) {
+ return new Timestamp(long_1.Long.fromString(str, true, optRadix));
+ };
+ /** @internal */
+ Timestamp.prototype.toExtendedJSON = function () {
+ return { $timestamp: { t: this.high >>> 0, i: this.low >>> 0 } };
+ };
+ /** @internal */
+ Timestamp.fromExtendedJSON = function (doc) {
+ return new Timestamp(doc.$timestamp.i, doc.$timestamp.t);
+ };
+ /** @internal */
+ Timestamp.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Timestamp.prototype.inspect = function () {
+ return "new Timestamp(" + this.getLowBits().toString() + ", " + this.getHighBits().toString() + ")";
+ };
+ Timestamp.MAX_VALUE = long_1.Long.MAX_UNSIGNED_VALUE;
+ return Timestamp;
+}(exports.LongWithoutOverridesClass));
+exports.Timestamp = Timestamp;
+
+});
+
+unwrapExports(timestamp);
+timestamp.Timestamp;
+timestamp.LongWithoutOverridesClass;
+
+var extended_json = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.EJSON = exports.isBSONType = void 0;
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+function isBSONType(value) {
+ return (utils.isObjectLike(value) && Reflect.has(value, '_bsontype') && typeof value._bsontype === 'string');
+}
+exports.isBSONType = isBSONType;
+// INT32 boundaries
+var BSON_INT32_MAX = 0x7fffffff;
+var BSON_INT32_MIN = -0x80000000;
+// INT64 boundaries
+var BSON_INT64_MAX = 0x7fffffffffffffff;
+var BSON_INT64_MIN = -0x8000000000000000;
+// all the types where we don't need to do any special processing and can just pass the EJSON
+//straight to type.fromExtendedJSON
+var keysToCodecs = {
+ $oid: objectid.ObjectId,
+ $binary: binary.Binary,
+ $uuid: binary.Binary,
+ $symbol: symbol.BSONSymbol,
+ $numberInt: int_32.Int32,
+ $numberDecimal: decimal128.Decimal128,
+ $numberDouble: double_1.Double,
+ $numberLong: long_1.Long,
+ $minKey: min_key.MinKey,
+ $maxKey: max_key.MaxKey,
+ $regex: regexp.BSONRegExp,
+ $regularExpression: regexp.BSONRegExp,
+ $timestamp: timestamp.Timestamp
+};
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function deserializeValue(value, options) {
+ if (options === void 0) { options = {}; }
+ if (typeof value === 'number') {
+ if (options.relaxed || options.legacy) {
+ return value;
+ }
+ // if it's an integer, should interpret as smallest BSON integer
+ // that can represent it exactly. (if out of range, interpret as double.)
+ if (Math.floor(value) === value) {
+ if (value >= BSON_INT32_MIN && value <= BSON_INT32_MAX)
+ return new int_32.Int32(value);
+ if (value >= BSON_INT64_MIN && value <= BSON_INT64_MAX)
+ return long_1.Long.fromNumber(value);
+ }
+ // If the number is a non-integer or out of integer range, should interpret as BSON Double.
+ return new double_1.Double(value);
+ }
+ // from here on out we're looking for bson types, so bail if its not an object
+ if (value == null || typeof value !== 'object')
+ return value;
+ // upgrade deprecated undefined to null
+ if (value.$undefined)
+ return null;
+ var keys = Object.keys(value).filter(function (k) { return k.startsWith('$') && value[k] != null; });
+ for (var i = 0; i < keys.length; i++) {
+ var c = keysToCodecs[keys[i]];
+ if (c)
+ return c.fromExtendedJSON(value, options);
+ }
+ if (value.$date != null) {
+ var d = value.$date;
+ var date = new Date();
+ if (options.legacy) {
+ if (typeof d === 'number')
+ date.setTime(d);
+ else if (typeof d === 'string')
+ date.setTime(Date.parse(d));
+ }
+ else {
+ if (typeof d === 'string')
+ date.setTime(Date.parse(d));
+ else if (long_1.Long.isLong(d))
+ date.setTime(d.toNumber());
+ else if (typeof d === 'number' && options.relaxed)
+ date.setTime(d);
+ }
+ return date;
+ }
+ if (value.$code != null) {
+ var copy = Object.assign({}, value);
+ if (value.$scope) {
+ copy.$scope = deserializeValue(value.$scope);
+ }
+ return code.Code.fromExtendedJSON(value);
+ }
+ if (db_ref.isDBRefLike(value) || value.$dbPointer) {
+ var v = value.$ref ? value : value.$dbPointer;
+ // we run into this in a "degenerate EJSON" case (with $id and $ref order flipped)
+ // because of the order JSON.parse goes through the document
+ if (v instanceof db_ref.DBRef)
+ return v;
+ var dollarKeys = Object.keys(v).filter(function (k) { return k.startsWith('$'); });
+ var valid_1 = true;
+ dollarKeys.forEach(function (k) {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1)
+ valid_1 = false;
+ });
+ // only make DBRef if $ keys are all valid
+ if (valid_1)
+ return db_ref.DBRef.fromExtendedJSON(v);
+ }
+ return value;
+}
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeArray(array, options) {
+ return array.map(function (v, index) {
+ options.seenObjects.push({ propertyName: "index " + index, obj: null });
+ try {
+ return serializeValue(v, options);
+ }
+ finally {
+ options.seenObjects.pop();
+ }
+ });
+}
+function getISOString(date) {
+ var isoStr = date.toISOString();
+ // we should only show milliseconds in timestamp if they're non-zero
+ return date.getUTCMilliseconds() !== 0 ? isoStr : isoStr.slice(0, -5) + 'Z';
+}
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeValue(value, options) {
+ if ((typeof value === 'object' || typeof value === 'function') && value !== null) {
+ var index = options.seenObjects.findIndex(function (entry) { return entry.obj === value; });
+ if (index !== -1) {
+ var props = options.seenObjects.map(function (entry) { return entry.propertyName; });
+ var leadingPart = props
+ .slice(0, index)
+ .map(function (prop) { return prop + " -> "; })
+ .join('');
+ var alreadySeen = props[index];
+ var circularPart = ' -> ' +
+ props
+ .slice(index + 1, props.length - 1)
+ .map(function (prop) { return prop + " -> "; })
+ .join('');
+ var current = props[props.length - 1];
+ var leadingSpace = ' '.repeat(leadingPart.length + alreadySeen.length / 2);
+ var dashes = '-'.repeat(circularPart.length + (alreadySeen.length + current.length) / 2 - 1);
+ throw new TypeError('Converting circular structure to EJSON:\n' +
+ (" " + leadingPart + alreadySeen + circularPart + current + "\n") +
+ (" " + leadingSpace + "\\" + dashes + "/"));
+ }
+ options.seenObjects[options.seenObjects.length - 1].obj = value;
+ }
+ if (Array.isArray(value))
+ return serializeArray(value, options);
+ if (value === undefined)
+ return null;
+ if (value instanceof Date || utils.isDate(value)) {
+ var dateNum = value.getTime(),
+ // is it in year range 1970-9999?
+ inRange = dateNum > -1 && dateNum < 253402318800000;
+ if (options.legacy) {
+ return options.relaxed && inRange
+ ? { $date: value.getTime() }
+ : { $date: getISOString(value) };
+ }
+ return options.relaxed && inRange
+ ? { $date: getISOString(value) }
+ : { $date: { $numberLong: value.getTime().toString() } };
+ }
+ if (typeof value === 'number' && (!options.relaxed || !isFinite(value))) {
+ // it's an integer
+ if (Math.floor(value) === value) {
+ var int32Range = value >= BSON_INT32_MIN && value <= BSON_INT32_MAX, int64Range = value >= BSON_INT64_MIN && value <= BSON_INT64_MAX;
+ // interpret as being of the smallest BSON integer type that can represent the number exactly
+ if (int32Range)
+ return { $numberInt: value.toString() };
+ if (int64Range)
+ return { $numberLong: value.toString() };
+ }
+ return { $numberDouble: value.toString() };
+ }
+ if (value instanceof RegExp || utils.isRegExp(value)) {
+ var flags = value.flags;
+ if (flags === undefined) {
+ var match = value.toString().match(/[gimuy]*$/);
+ if (match) {
+ flags = match[0];
+ }
+ }
+ var rx = new regexp.BSONRegExp(value.source, flags);
+ return rx.toExtendedJSON(options);
+ }
+ if (value != null && typeof value === 'object')
+ return serializeDocument(value, options);
+ return value;
+}
+var BSON_TYPE_MAPPINGS = {
+ Binary: function (o) { return new binary.Binary(o.value(), o.sub_type); },
+ Code: function (o) { return new code.Code(o.code, o.scope); },
+ DBRef: function (o) { return new db_ref.DBRef(o.collection || o.namespace, o.oid, o.db, o.fields); },
+ Decimal128: function (o) { return new decimal128.Decimal128(o.bytes); },
+ Double: function (o) { return new double_1.Double(o.value); },
+ Int32: function (o) { return new int_32.Int32(o.value); },
+ Long: function (o) {
+ return long_1.Long.fromBits(
+ // underscore variants for 1.x backwards compatibility
+ o.low != null ? o.low : o.low_, o.low != null ? o.high : o.high_, o.low != null ? o.unsigned : o.unsigned_);
+ },
+ MaxKey: function () { return new max_key.MaxKey(); },
+ MinKey: function () { return new min_key.MinKey(); },
+ ObjectID: function (o) { return new objectid.ObjectId(o); },
+ ObjectId: function (o) { return new objectid.ObjectId(o); },
+ BSONRegExp: function (o) { return new regexp.BSONRegExp(o.pattern, o.options); },
+ Symbol: function (o) { return new symbol.BSONSymbol(o.value); },
+ Timestamp: function (o) { return timestamp.Timestamp.fromBits(o.low, o.high); }
+};
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeDocument(doc, options) {
+ if (doc == null || typeof doc !== 'object')
+ throw new Error('not an object instance');
+ var bsontype = doc._bsontype;
+ if (typeof bsontype === 'undefined') {
+ // It's a regular object. Recursively serialize its property values.
+ var _doc = {};
+ for (var name in doc) {
+ options.seenObjects.push({ propertyName: name, obj: null });
+ try {
+ _doc[name] = serializeValue(doc[name], options);
+ }
+ finally {
+ options.seenObjects.pop();
+ }
+ }
+ return _doc;
+ }
+ else if (isBSONType(doc)) {
+ // the "document" is really just a BSON type object
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ var outDoc = doc;
+ if (typeof outDoc.toExtendedJSON !== 'function') {
+ // There's no EJSON serialization function on the object. It's probably an
+ // object created by a previous version of this library (or another library)
+ // that's duck-typing objects to look like they were generated by this library).
+ // Copy the object into this library's version of that type.
+ var mapper = BSON_TYPE_MAPPINGS[doc._bsontype];
+ if (!mapper) {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + doc._bsontype);
+ }
+ outDoc = mapper(outDoc);
+ }
+ // Two BSON types may have nested objects that may need to be serialized too
+ if (bsontype === 'Code' && outDoc.scope) {
+ outDoc = new code.Code(outDoc.code, serializeValue(outDoc.scope, options));
+ }
+ else if (bsontype === 'DBRef' && outDoc.oid) {
+ outDoc = new db_ref.DBRef(serializeValue(outDoc.collection, options), serializeValue(outDoc.oid, options), serializeValue(outDoc.db, options), serializeValue(outDoc.fields, options));
+ }
+ return outDoc.toExtendedJSON(options);
+ }
+ else {
+ throw new Error('_bsontype must be a string, but was: ' + typeof bsontype);
+ }
+}
+(function (EJSON) {
+ /**
+ * Parse an Extended JSON string, constructing the JavaScript value or object described by that
+ * string.
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const text = '{ "int32": { "$numberInt": "10" } }';
+ *
+ * // prints { int32: { [String: '10'] _bsontype: 'Int32', value: '10' } }
+ * console.log(EJSON.parse(text, { relaxed: false }));
+ *
+ * // prints { int32: 10 }
+ * console.log(EJSON.parse(text));
+ * ```
+ */
+ function parse(text, options) {
+ var finalOptions = Object.assign({}, { relaxed: true, legacy: false }, options);
+ // relaxed implies not strict
+ if (typeof finalOptions.relaxed === 'boolean')
+ finalOptions.strict = !finalOptions.relaxed;
+ if (typeof finalOptions.strict === 'boolean')
+ finalOptions.relaxed = !finalOptions.strict;
+ return JSON.parse(text, function (_key, value) { return deserializeValue(value, finalOptions); });
+ }
+ EJSON.parse = parse;
+ /**
+ * Converts a BSON document to an Extended JSON string, optionally replacing values if a replacer
+ * function is specified or optionally including only the specified properties if a replacer array
+ * is specified.
+ *
+ * @param value - The value to convert to extended JSON
+ * @param replacer - A function that alters the behavior of the stringification process, or an array of String and Number objects that serve as a whitelist for selecting/filtering the properties of the value object to be included in the JSON string. If this value is null or not provided, all properties of the object are included in the resulting JSON string
+ * @param space - A String or Number object that's used to insert white space into the output JSON string for readability purposes.
+ * @param options - Optional settings
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const Int32 = require('mongodb').Int32;
+ * const doc = { int32: new Int32(10) };
+ *
+ * // prints '{"int32":{"$numberInt":"10"}}'
+ * console.log(EJSON.stringify(doc, { relaxed: false }));
+ *
+ * // prints '{"int32":10}'
+ * console.log(EJSON.stringify(doc));
+ * ```
+ */
+ function stringify(value,
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ replacer, space, options) {
+ if (space != null && typeof space === 'object') {
+ options = space;
+ space = 0;
+ }
+ if (replacer != null && typeof replacer === 'object' && !Array.isArray(replacer)) {
+ options = replacer;
+ replacer = undefined;
+ space = 0;
+ }
+ var serializeOptions = Object.assign({ relaxed: true, legacy: false }, options, {
+ seenObjects: [{ propertyName: '(root)', obj: null }]
+ });
+ var doc = serializeValue(value, serializeOptions);
+ return JSON.stringify(doc, replacer, space);
+ }
+ EJSON.stringify = stringify;
+ /**
+ * Serializes an object to an Extended JSON string, and reparse it as a JavaScript object.
+ *
+ * @param value - The object to serialize
+ * @param options - Optional settings passed to the `stringify` function
+ */
+ function serialize(value, options) {
+ options = options || {};
+ return JSON.parse(stringify(value, options));
+ }
+ EJSON.serialize = serialize;
+ /**
+ * Deserializes an Extended JSON object into a plain JavaScript object with native/BSON types
+ *
+ * @param ejson - The Extended JSON object to deserialize
+ * @param options - Optional settings passed to the parse method
+ */
+ function deserialize(ejson, options) {
+ options = options || {};
+ return parse(JSON.stringify(ejson), options);
+ }
+ EJSON.deserialize = deserialize;
+})(exports.EJSON || (exports.EJSON = {}));
+
+});
+
+unwrapExports(extended_json);
+extended_json.EJSON;
+extended_json.isBSONType;
+
+var map = createCommonjsModule(function (module, exports) {
+/* eslint-disable @typescript-eslint/no-explicit-any */
+// We have an ES6 Map available, return the native instance
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Map = void 0;
+/** @public */
+var bsonMap;
+exports.Map = bsonMap;
+var check = function (potentialGlobal) {
+ // eslint-disable-next-line eqeqeq
+ return potentialGlobal && potentialGlobal.Math == Math && potentialGlobal;
+};
+// https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+function getGlobal() {
+ // eslint-disable-next-line no-undef
+ return (check(typeof globalThis === 'object' && globalThis) ||
+ check(typeof window === 'object' && window) ||
+ check(typeof self === 'object' && self) ||
+ check(typeof commonjsGlobal === 'object' && commonjsGlobal) ||
+ Function('return this')());
+}
+var bsonGlobal = getGlobal();
+if (Object.prototype.hasOwnProperty.call(bsonGlobal, 'Map')) {
+ exports.Map = bsonMap = bsonGlobal.Map;
+}
+else {
+ // We will return a polyfill
+ exports.Map = bsonMap = /** @class */ (function () {
+ function Map(array) {
+ if (array === void 0) { array = []; }
+ this._keys = [];
+ this._values = {};
+ for (var i = 0; i < array.length; i++) {
+ if (array[i] == null)
+ continue; // skip null and undefined
+ var entry = array[i];
+ var key = entry[0];
+ var value = entry[1];
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ }
+ }
+ Map.prototype.clear = function () {
+ this._keys = [];
+ this._values = {};
+ };
+ Map.prototype.delete = function (key) {
+ var value = this._values[key];
+ if (value == null)
+ return false;
+ // Delete entry
+ delete this._values[key];
+ // Remove the key from the ordered keys list
+ this._keys.splice(value.i, 1);
+ return true;
+ };
+ Map.prototype.entries = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? [key, _this._values[key].v] : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Map.prototype.forEach = function (callback, self) {
+ self = self || this;
+ for (var i = 0; i < this._keys.length; i++) {
+ var key = this._keys[i];
+ // Call the forEach callback
+ callback.call(self, this._values[key].v, key, self);
+ }
+ };
+ Map.prototype.get = function (key) {
+ return this._values[key] ? this._values[key].v : undefined;
+ };
+ Map.prototype.has = function (key) {
+ return this._values[key] != null;
+ };
+ Map.prototype.keys = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? key : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Map.prototype.set = function (key, value) {
+ if (this._values[key]) {
+ this._values[key].v = value;
+ return this;
+ }
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ return this;
+ };
+ Map.prototype.values = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? _this._values[key].v : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Object.defineProperty(Map.prototype, "size", {
+ get: function () {
+ return this._keys.length;
+ },
+ enumerable: false,
+ configurable: true
+ });
+ return Map;
+ }());
+}
+
+});
+
+unwrapExports(map);
+map.Map;
+
+var constants = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.BSON_BINARY_SUBTYPE_USER_DEFINED = exports.BSON_BINARY_SUBTYPE_MD5 = exports.BSON_BINARY_SUBTYPE_UUID_NEW = exports.BSON_BINARY_SUBTYPE_UUID = exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = exports.BSON_BINARY_SUBTYPE_FUNCTION = exports.BSON_BINARY_SUBTYPE_DEFAULT = exports.BSON_DATA_MAX_KEY = exports.BSON_DATA_MIN_KEY = exports.BSON_DATA_DECIMAL128 = exports.BSON_DATA_LONG = exports.BSON_DATA_TIMESTAMP = exports.BSON_DATA_INT = exports.BSON_DATA_CODE_W_SCOPE = exports.BSON_DATA_SYMBOL = exports.BSON_DATA_CODE = exports.BSON_DATA_DBPOINTER = exports.BSON_DATA_REGEXP = exports.BSON_DATA_NULL = exports.BSON_DATA_DATE = exports.BSON_DATA_BOOLEAN = exports.BSON_DATA_OID = exports.BSON_DATA_UNDEFINED = exports.BSON_DATA_BINARY = exports.BSON_DATA_ARRAY = exports.BSON_DATA_OBJECT = exports.BSON_DATA_STRING = exports.BSON_DATA_NUMBER = exports.JS_INT_MIN = exports.JS_INT_MAX = exports.BSON_INT64_MIN = exports.BSON_INT64_MAX = exports.BSON_INT32_MIN = exports.BSON_INT32_MAX = void 0;
+/** @internal */
+exports.BSON_INT32_MAX = 0x7fffffff;
+/** @internal */
+exports.BSON_INT32_MIN = -0x80000000;
+/** @internal */
+exports.BSON_INT64_MAX = Math.pow(2, 63) - 1;
+/** @internal */
+exports.BSON_INT64_MIN = -Math.pow(2, 63);
+/**
+ * Any integer up to 2^53 can be precisely represented by a double.
+ * @internal
+ */
+exports.JS_INT_MAX = Math.pow(2, 53);
+/**
+ * Any integer down to -2^53 can be precisely represented by a double.
+ * @internal
+ */
+exports.JS_INT_MIN = -Math.pow(2, 53);
+/** Number BSON Type @internal */
+exports.BSON_DATA_NUMBER = 1;
+/** String BSON Type @internal */
+exports.BSON_DATA_STRING = 2;
+/** Object BSON Type @internal */
+exports.BSON_DATA_OBJECT = 3;
+/** Array BSON Type @internal */
+exports.BSON_DATA_ARRAY = 4;
+/** Binary BSON Type @internal */
+exports.BSON_DATA_BINARY = 5;
+/** Binary BSON Type @internal */
+exports.BSON_DATA_UNDEFINED = 6;
+/** ObjectId BSON Type @internal */
+exports.BSON_DATA_OID = 7;
+/** Boolean BSON Type @internal */
+exports.BSON_DATA_BOOLEAN = 8;
+/** Date BSON Type @internal */
+exports.BSON_DATA_DATE = 9;
+/** null BSON Type @internal */
+exports.BSON_DATA_NULL = 10;
+/** RegExp BSON Type @internal */
+exports.BSON_DATA_REGEXP = 11;
+/** Code BSON Type @internal */
+exports.BSON_DATA_DBPOINTER = 12;
+/** Code BSON Type @internal */
+exports.BSON_DATA_CODE = 13;
+/** Symbol BSON Type @internal */
+exports.BSON_DATA_SYMBOL = 14;
+/** Code with Scope BSON Type @internal */
+exports.BSON_DATA_CODE_W_SCOPE = 15;
+/** 32 bit Integer BSON Type @internal */
+exports.BSON_DATA_INT = 16;
+/** Timestamp BSON Type @internal */
+exports.BSON_DATA_TIMESTAMP = 17;
+/** Long BSON Type @internal */
+exports.BSON_DATA_LONG = 18;
+/** Decimal128 BSON Type @internal */
+exports.BSON_DATA_DECIMAL128 = 19;
+/** MinKey BSON Type @internal */
+exports.BSON_DATA_MIN_KEY = 0xff;
+/** MaxKey BSON Type @internal */
+exports.BSON_DATA_MAX_KEY = 0x7f;
+/** Binary Default Type @internal */
+exports.BSON_BINARY_SUBTYPE_DEFAULT = 0;
+/** Binary Function Type @internal */
+exports.BSON_BINARY_SUBTYPE_FUNCTION = 1;
+/** Binary Byte Array Type @internal */
+exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
+/** Binary Deprecated UUID Type @deprecated Please use BSON_BINARY_SUBTYPE_UUID_NEW @internal */
+exports.BSON_BINARY_SUBTYPE_UUID = 3;
+/** Binary UUID Type @internal */
+exports.BSON_BINARY_SUBTYPE_UUID_NEW = 4;
+/** Binary MD5 Type @internal */
+exports.BSON_BINARY_SUBTYPE_MD5 = 5;
+/** Binary User Defined Type @internal */
+exports.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
+
+});
+
+unwrapExports(constants);
+constants.BSON_BINARY_SUBTYPE_USER_DEFINED;
+constants.BSON_BINARY_SUBTYPE_MD5;
+constants.BSON_BINARY_SUBTYPE_UUID_NEW;
+constants.BSON_BINARY_SUBTYPE_UUID;
+constants.BSON_BINARY_SUBTYPE_BYTE_ARRAY;
+constants.BSON_BINARY_SUBTYPE_FUNCTION;
+constants.BSON_BINARY_SUBTYPE_DEFAULT;
+constants.BSON_DATA_MAX_KEY;
+constants.BSON_DATA_MIN_KEY;
+constants.BSON_DATA_DECIMAL128;
+constants.BSON_DATA_LONG;
+constants.BSON_DATA_TIMESTAMP;
+constants.BSON_DATA_INT;
+constants.BSON_DATA_CODE_W_SCOPE;
+constants.BSON_DATA_SYMBOL;
+constants.BSON_DATA_CODE;
+constants.BSON_DATA_DBPOINTER;
+constants.BSON_DATA_REGEXP;
+constants.BSON_DATA_NULL;
+constants.BSON_DATA_DATE;
+constants.BSON_DATA_BOOLEAN;
+constants.BSON_DATA_OID;
+constants.BSON_DATA_UNDEFINED;
+constants.BSON_DATA_BINARY;
+constants.BSON_DATA_ARRAY;
+constants.BSON_DATA_OBJECT;
+constants.BSON_DATA_STRING;
+constants.BSON_DATA_NUMBER;
+constants.JS_INT_MIN;
+constants.JS_INT_MAX;
+constants.BSON_INT64_MIN;
+constants.BSON_INT64_MAX;
+constants.BSON_INT32_MIN;
+constants.BSON_INT32_MAX;
+
+var calculate_size = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.calculateObjectSize = void 0;
+
+
+
+
+function calculateObjectSize(object, serializeFunctions, ignoreUndefined) {
+ var totalLength = 4 + 1;
+ if (Array.isArray(object)) {
+ for (var i = 0; i < object.length; i++) {
+ totalLength += calculateElement(i.toString(), object[i], serializeFunctions, true, ignoreUndefined);
+ }
+ }
+ else {
+ // If we have toBSON defined, override the current object
+ if (object.toBSON) {
+ object = object.toBSON();
+ }
+ // Calculate size
+ for (var key in object) {
+ totalLength += calculateElement(key, object[key], serializeFunctions, false, ignoreUndefined);
+ }
+ }
+ return totalLength;
+}
+exports.calculateObjectSize = calculateObjectSize;
+/** @internal */
+function calculateElement(name,
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+value, serializeFunctions, isArray, ignoreUndefined) {
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (isArray === void 0) { isArray = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = false; }
+ // If we have toBSON defined, override the current object
+ if (value && value.toBSON) {
+ value = value.toBSON();
+ }
+ switch (typeof value) {
+ case 'string':
+ return 1 + buffer.Buffer.byteLength(name, 'utf8') + 1 + 4 + buffer.Buffer.byteLength(value, 'utf8') + 1;
+ case 'number':
+ if (Math.floor(value) === value &&
+ value >= constants.JS_INT_MIN &&
+ value <= constants.JS_INT_MAX) {
+ if (value >= constants.BSON_INT32_MIN && value <= constants.BSON_INT32_MAX) {
+ // 32 bit
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (4 + 1);
+ }
+ else {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ }
+ else {
+ // 64 bit
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ case 'undefined':
+ if (isArray || !ignoreUndefined)
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ return 0;
+ case 'boolean':
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 1);
+ case 'object':
+ if (value == null || value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (12 + 1);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ else if (ArrayBuffer.isView(value) ||
+ value instanceof ArrayBuffer ||
+ utils.isAnyArrayBuffer(value)) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 4 + 1) + value.byteLength);
+ }
+ else if (value['_bsontype'] === 'Long' ||
+ value['_bsontype'] === 'Double' ||
+ value['_bsontype'] === 'Timestamp') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ else if (value['_bsontype'] === 'Decimal128') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (16 + 1);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ // Calculate size depending on the availability of a scope
+ if (value.scope != null && Object.keys(value.scope).length > 0) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ buffer.Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined));
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ buffer.Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1);
+ }
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ // Check what kind of subtype we have
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ (value.position + 1 + 4 + 1 + 4));
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (value.position + 1 + 4 + 1));
+ }
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ buffer.Buffer.byteLength(value.value, 'utf8') +
+ 4 +
+ 1 +
+ 1);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ // Set up correct object for serialization
+ var ordered_values = Object.assign({
+ $ref: value.collection,
+ $id: value.oid
+ }, value.fields);
+ // Add db reference if it exists
+ if (value.db != null) {
+ ordered_values['$db'] = value.db;
+ }
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ calculateObjectSize(ordered_values, serializeFunctions, ignoreUndefined));
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.pattern, 'utf8') +
+ 1 +
+ buffer.Buffer.byteLength(value.options, 'utf8') +
+ 1);
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ calculateObjectSize(value, serializeFunctions, ignoreUndefined) +
+ 1);
+ }
+ case 'function':
+ // WTF for 0.4.X where typeof /someregexp/ === 'function'
+ if (value instanceof RegExp || utils.isRegExp(value) || String.call(value) === '[object RegExp]') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1);
+ }
+ else {
+ if (serializeFunctions && value.scope != null && Object.keys(value.scope).length > 0) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ buffer.Buffer.byteLength(utils.normalizedFunctionString(value), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined));
+ }
+ else if (serializeFunctions) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ buffer.Buffer.byteLength(utils.normalizedFunctionString(value), 'utf8') +
+ 1);
+ }
+ }
+ }
+ return 0;
+}
+
+});
+
+unwrapExports(calculate_size);
+calculate_size.calculateObjectSize;
+
+var validate_utf8 = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.validateUtf8 = void 0;
+var FIRST_BIT = 0x80;
+var FIRST_TWO_BITS = 0xc0;
+var FIRST_THREE_BITS = 0xe0;
+var FIRST_FOUR_BITS = 0xf0;
+var FIRST_FIVE_BITS = 0xf8;
+var TWO_BIT_CHAR = 0xc0;
+var THREE_BIT_CHAR = 0xe0;
+var FOUR_BIT_CHAR = 0xf0;
+var CONTINUING_CHAR = 0x80;
+/**
+ * Determines if the passed in bytes are valid utf8
+ * @param bytes - An array of 8-bit bytes. Must be indexable and have length property
+ * @param start - The index to start validating
+ * @param end - The index to end validating
+ */
+function validateUtf8(bytes, start, end) {
+ var continuation = 0;
+ for (var i = start; i < end; i += 1) {
+ var byte = bytes[i];
+ if (continuation) {
+ if ((byte & FIRST_TWO_BITS) !== CONTINUING_CHAR) {
+ return false;
+ }
+ continuation -= 1;
+ }
+ else if (byte & FIRST_BIT) {
+ if ((byte & FIRST_THREE_BITS) === TWO_BIT_CHAR) {
+ continuation = 1;
+ }
+ else if ((byte & FIRST_FOUR_BITS) === THREE_BIT_CHAR) {
+ continuation = 2;
+ }
+ else if ((byte & FIRST_FIVE_BITS) === FOUR_BIT_CHAR) {
+ continuation = 3;
+ }
+ else {
+ return false;
+ }
+ }
+ }
+ return !continuation;
+}
+exports.validateUtf8 = validateUtf8;
+
+});
+
+unwrapExports(validate_utf8);
+validate_utf8.validateUtf8;
+
+var deserializer = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.deserialize = void 0;
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+// Internal long versions
+var JS_INT_MAX_LONG = long_1.Long.fromNumber(constants.JS_INT_MAX);
+var JS_INT_MIN_LONG = long_1.Long.fromNumber(constants.JS_INT_MIN);
+var functionCache = {};
+function deserialize(buffer, options, isArray) {
+ options = options == null ? {} : options;
+ var index = options && options.index ? options.index : 0;
+ // Read the document size
+ var size = buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ if (size < 5) {
+ throw new Error("bson size must be >= 5, is " + size);
+ }
+ if (options.allowObjectSmallerThanBufferSize && buffer.length < size) {
+ throw new Error("buffer length " + buffer.length + " must be >= bson size " + size);
+ }
+ if (!options.allowObjectSmallerThanBufferSize && buffer.length !== size) {
+ throw new Error("buffer length " + buffer.length + " must === bson size " + size);
+ }
+ if (size + index > buffer.byteLength) {
+ throw new Error("(bson size " + size + " + options.index " + index + " must be <= buffer length " + buffer.byteLength + ")");
+ }
+ // Illegal end value
+ if (buffer[index + size - 1] !== 0) {
+ throw new Error("One object, sized correctly, with a spot for an EOO, but the EOO isn't 0x00");
+ }
+ // Start deserializtion
+ return deserializeObject(buffer, index, options, isArray);
+}
+exports.deserialize = deserialize;
+function deserializeObject(buffer$1, index, options, isArray) {
+ if (isArray === void 0) { isArray = false; }
+ var evalFunctions = options['evalFunctions'] == null ? false : options['evalFunctions'];
+ var cacheFunctions = options['cacheFunctions'] == null ? false : options['cacheFunctions'];
+ var fieldsAsRaw = options['fieldsAsRaw'] == null ? null : options['fieldsAsRaw'];
+ // Return raw bson buffer instead of parsing it
+ var raw = options['raw'] == null ? false : options['raw'];
+ // Return BSONRegExp objects instead of native regular expressions
+ var bsonRegExp = typeof options['bsonRegExp'] === 'boolean' ? options['bsonRegExp'] : false;
+ // Controls the promotion of values vs wrapper classes
+ var promoteBuffers = options['promoteBuffers'] == null ? false : options['promoteBuffers'];
+ var promoteLongs = options['promoteLongs'] == null ? true : options['promoteLongs'];
+ var promoteValues = options['promoteValues'] == null ? true : options['promoteValues'];
+ // Set the start index
+ var startIndex = index;
+ // Validate that we have at least 4 bytes of buffer
+ if (buffer$1.length < 5)
+ throw new Error('corrupt bson message < 5 bytes long');
+ // Read the document size
+ var size = buffer$1[index++] | (buffer$1[index++] << 8) | (buffer$1[index++] << 16) | (buffer$1[index++] << 24);
+ // Ensure buffer is valid size
+ if (size < 5 || size > buffer$1.length)
+ throw new Error('corrupt bson message');
+ // Create holding object
+ var object = isArray ? [] : {};
+ // Used for arrays to skip having to perform utf8 decoding
+ var arrayIndex = 0;
+ var done = false;
+ // While we have more left data left keep parsing
+ while (!done) {
+ // Read the type
+ var elementType = buffer$1[index++];
+ // If we get a zero it's the last byte, exit
+ if (elementType === 0)
+ break;
+ // Get the start search index
+ var i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.byteLength)
+ throw new Error('Bad BSON Document: illegal CString');
+ var name = isArray ? arrayIndex++ : buffer$1.toString('utf8', index, i);
+ var value = void 0;
+ index = i + 1;
+ if (elementType === constants.BSON_DATA_STRING) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ if (!validate_utf8.validateUtf8(buffer$1, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ value = buffer$1.toString('utf8', index, index + stringSize - 1);
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_OID) {
+ var oid = buffer.Buffer.alloc(12);
+ buffer$1.copy(oid, 0, index, index + 12);
+ value = new objectid.ObjectId(oid);
+ index = index + 12;
+ }
+ else if (elementType === constants.BSON_DATA_INT && promoteValues === false) {
+ value = new int_32.Int32(buffer$1[index++] | (buffer$1[index++] << 8) | (buffer$1[index++] << 16) | (buffer$1[index++] << 24));
+ }
+ else if (elementType === constants.BSON_DATA_INT) {
+ value =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ }
+ else if (elementType === constants.BSON_DATA_NUMBER && promoteValues === false) {
+ value = new double_1.Double(buffer$1.readDoubleLE(index));
+ index = index + 8;
+ }
+ else if (elementType === constants.BSON_DATA_NUMBER) {
+ value = buffer$1.readDoubleLE(index);
+ index = index + 8;
+ }
+ else if (elementType === constants.BSON_DATA_DATE) {
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ value = new Date(new long_1.Long(lowBits, highBits).toNumber());
+ }
+ else if (elementType === constants.BSON_DATA_BOOLEAN) {
+ if (buffer$1[index] !== 0 && buffer$1[index] !== 1)
+ throw new Error('illegal boolean type value');
+ value = buffer$1[index++] === 1;
+ }
+ else if (elementType === constants.BSON_DATA_OBJECT) {
+ var _index = index;
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ if (objectSize <= 0 || objectSize > buffer$1.length - index)
+ throw new Error('bad embedded document length in bson');
+ // We have a raw value
+ if (raw) {
+ value = buffer$1.slice(index, index + objectSize);
+ }
+ else {
+ value = deserializeObject(buffer$1, _index, options, false);
+ }
+ index = index + objectSize;
+ }
+ else if (elementType === constants.BSON_DATA_ARRAY) {
+ var _index = index;
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ var arrayOptions = options;
+ // Stop index
+ var stopIndex = index + objectSize;
+ // All elements of array to be returned as raw bson
+ if (fieldsAsRaw && fieldsAsRaw[name]) {
+ arrayOptions = {};
+ for (var n in options) {
+ arrayOptions[n] = options[n];
+ }
+ arrayOptions['raw'] = true;
+ }
+ value = deserializeObject(buffer$1, _index, arrayOptions, true);
+ index = index + objectSize;
+ if (buffer$1[index - 1] !== 0)
+ throw new Error('invalid array terminator byte');
+ if (index !== stopIndex)
+ throw new Error('corrupted array bson');
+ }
+ else if (elementType === constants.BSON_DATA_UNDEFINED) {
+ value = undefined;
+ }
+ else if (elementType === constants.BSON_DATA_NULL) {
+ value = null;
+ }
+ else if (elementType === constants.BSON_DATA_LONG) {
+ // Unpack the low and high bits
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var long = new long_1.Long(lowBits, highBits);
+ // Promote the long if possible
+ if (promoteLongs && promoteValues === true) {
+ value =
+ long.lessThanOrEqual(JS_INT_MAX_LONG) && long.greaterThanOrEqual(JS_INT_MIN_LONG)
+ ? long.toNumber()
+ : long;
+ }
+ else {
+ value = long;
+ }
+ }
+ else if (elementType === constants.BSON_DATA_DECIMAL128) {
+ // Buffer to contain the decimal bytes
+ var bytes = buffer.Buffer.alloc(16);
+ // Copy the next 16 bytes into the bytes buffer
+ buffer$1.copy(bytes, 0, index, index + 16);
+ // Update index
+ index = index + 16;
+ // Assign the new Decimal128 value
+ var decimal128$1 = new decimal128.Decimal128(bytes);
+ // If we have an alternative mapper use that
+ if ('toObject' in decimal128$1 && typeof decimal128$1.toObject === 'function') {
+ value = decimal128$1.toObject();
+ }
+ else {
+ value = decimal128$1;
+ }
+ }
+ else if (elementType === constants.BSON_DATA_BINARY) {
+ var binarySize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var totalBinarySize = binarySize;
+ var subType = buffer$1[index++];
+ // Did we have a negative binary size, throw
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found');
+ // Is the length longer than the document
+ if (binarySize > buffer$1.byteLength)
+ throw new Error('Binary type size larger than document size');
+ // Decode as raw Buffer object if options specifies it
+ if (buffer$1['slice'] != null) {
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+ if (promoteBuffers && promoteValues) {
+ value = buffer$1.slice(index, index + binarySize);
+ }
+ else {
+ value = new binary.Binary(buffer$1.slice(index, index + binarySize), subType);
+ }
+ }
+ else {
+ var _buffer = buffer.Buffer.alloc(binarySize);
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+ // Copy the data
+ for (i = 0; i < binarySize; i++) {
+ _buffer[i] = buffer$1[index + i];
+ }
+ if (promoteBuffers && promoteValues) {
+ value = _buffer;
+ }
+ else {
+ value = new binary.Binary(_buffer, subType);
+ }
+ }
+ // Update the index
+ index = index + binarySize;
+ }
+ else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === false) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var source = buffer$1.toString('utf8', index, i);
+ // Create the regexp
+ index = i + 1;
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var regExpOptions = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // For each option add the corresponding one for javascript
+ var optionsArray = new Array(regExpOptions.length);
+ // Parse options
+ for (i = 0; i < regExpOptions.length; i++) {
+ switch (regExpOptions[i]) {
+ case 'm':
+ optionsArray[i] = 'm';
+ break;
+ case 's':
+ optionsArray[i] = 'g';
+ break;
+ case 'i':
+ optionsArray[i] = 'i';
+ break;
+ }
+ }
+ value = new RegExp(source, optionsArray.join(''));
+ }
+ else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === true) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var source = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var regExpOptions = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // Set the object
+ value = new regexp.BSONRegExp(source, regExpOptions);
+ }
+ else if (elementType === constants.BSON_DATA_SYMBOL) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ var symbol$1 = buffer$1.toString('utf8', index, index + stringSize - 1);
+ value = promoteValues ? symbol$1 : new symbol.BSONSymbol(symbol$1);
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_TIMESTAMP) {
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ value = new timestamp.Timestamp(lowBits, highBits);
+ }
+ else if (elementType === constants.BSON_DATA_MIN_KEY) {
+ value = new min_key.MinKey();
+ }
+ else if (elementType === constants.BSON_DATA_MAX_KEY) {
+ value = new max_key.MaxKey();
+ }
+ else if (elementType === constants.BSON_DATA_CODE) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ var functionString = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ }
+ else {
+ value = isolateEval(functionString);
+ }
+ }
+ else {
+ value = new code.Code(functionString);
+ }
+ // Update parse index position
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_CODE_W_SCOPE) {
+ var totalSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Element cannot be shorter than totalSize + stringSize + documentSize + terminator
+ if (totalSize < 4 + 4 + 4 + 1) {
+ throw new Error('code_w_scope total size shorter minimum expected length');
+ }
+ // Get the code string size
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Check if we have a valid string
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ // Javascript function
+ var functionString = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Parse the element
+ var _index = index;
+ // Decode the size of the object document
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ // Decode the scope object
+ var scopeObject = deserializeObject(buffer$1, _index, options, false);
+ // Adjust the index
+ index = index + objectSize;
+ // Check if field length is too short
+ if (totalSize < 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too short, truncating scope');
+ }
+ // Check if totalSize field is too long
+ if (totalSize > 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too long, clips outer document');
+ }
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ }
+ else {
+ value = isolateEval(functionString);
+ }
+ value.scope = scopeObject;
+ }
+ else {
+ value = new code.Code(functionString, scopeObject);
+ }
+ }
+ else if (elementType === constants.BSON_DATA_DBPOINTER) {
+ // Get the code string size
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Check if we have a valid string
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ // Namespace
+ if (!validate_utf8.validateUtf8(buffer$1, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ var namespace = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Read the oid
+ var oidBuffer = buffer.Buffer.alloc(12);
+ buffer$1.copy(oidBuffer, 0, index, index + 12);
+ var oid = new objectid.ObjectId(oidBuffer);
+ // Update the index
+ index = index + 12;
+ // Upgrade to DBRef type
+ value = new db_ref.DBRef(namespace, oid);
+ }
+ else {
+ throw new Error('Detected unknown BSON type ' + elementType.toString(16) + ' for fieldname "' + name + '"');
+ }
+ if (name === '__proto__') {
+ Object.defineProperty(object, name, {
+ value: value,
+ writable: true,
+ enumerable: true,
+ configurable: true
+ });
+ }
+ else {
+ object[name] = value;
+ }
+ }
+ // Check if the deserialization was against a valid array/object
+ if (size !== index - startIndex) {
+ if (isArray)
+ throw new Error('corrupt array bson');
+ throw new Error('corrupt object bson');
+ }
+ // check if object's $ keys are those of a DBRef
+ var dollarKeys = Object.keys(object).filter(function (k) { return k.startsWith('$'); });
+ var valid = true;
+ dollarKeys.forEach(function (k) {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1)
+ valid = false;
+ });
+ // if a $key not in "$ref", "$id", "$db", don't make a DBRef
+ if (!valid)
+ return object;
+ if (db_ref.isDBRefLike(object)) {
+ var copy = Object.assign({}, object);
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new db_ref.DBRef(object.$ref, object.$id, object.$db, copy);
+ }
+ return object;
+}
+/**
+ * Ensure eval is isolated, store the result in functionCache.
+ *
+ * @internal
+ */
+function isolateEval(functionString, functionCache, object) {
+ if (!functionCache)
+ return new Function(functionString);
+ // Check for cache hit, eval if missing and return cached function
+ if (functionCache[functionString] == null) {
+ functionCache[functionString] = new Function(functionString);
+ }
+ // Set the object
+ return functionCache[functionString].bind(object);
+}
+
+});
+
+unwrapExports(deserializer);
+deserializer.deserialize;
+
+var float_parser = createCommonjsModule(function (module, exports) {
+// Copyright (c) 2008, Fair Oaks Labs, Inc.
+// All rights reserved.
+//
+// Redistribution and use in source and binary forms, with or without
+// modification, are permitted provided that the following conditions are met:
+//
+// * Redistributions of source code must retain the above copyright notice,
+// this list of conditions and the following disclaimer.
+//
+// * Redistributions in binary form must reproduce the above copyright notice,
+// this list of conditions and the following disclaimer in the documentation
+// and/or other materials provided with the distribution.
+//
+// * Neither the name of Fair Oaks Labs, Inc. nor the names of its contributors
+// may be used to endorse or promote products derived from this software
+// without specific prior written permission.
+//
+// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+// AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
+// ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
+// LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
+// CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
+// SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
+// INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
+// CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
+// ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
+// POSSIBILITY OF SUCH DAMAGE.
+//
+//
+// Modifications to writeIEEE754 to support negative zeroes made by Brian White
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.writeIEEE754 = exports.readIEEE754 = void 0;
+function readIEEE754(buffer, offset, endian, mLen, nBytes) {
+ var e;
+ var m;
+ var bBE = endian === 'big';
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var nBits = -7;
+ var i = bBE ? 0 : nBytes - 1;
+ var d = bBE ? 1 : -1;
+ var s = buffer[offset + i];
+ i += d;
+ e = s & ((1 << -nBits) - 1);
+ s >>= -nBits;
+ nBits += eLen;
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8)
+ ;
+ m = e & ((1 << -nBits) - 1);
+ e >>= -nBits;
+ nBits += mLen;
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8)
+ ;
+ if (e === 0) {
+ e = 1 - eBias;
+ }
+ else if (e === eMax) {
+ return m ? NaN : (s ? -1 : 1) * Infinity;
+ }
+ else {
+ m = m + Math.pow(2, mLen);
+ e = e - eBias;
+ }
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
+}
+exports.readIEEE754 = readIEEE754;
+function writeIEEE754(buffer, value, offset, endian, mLen, nBytes) {
+ var e;
+ var m;
+ var c;
+ var bBE = endian === 'big';
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0;
+ var i = bBE ? nBytes - 1 : 0;
+ var d = bBE ? -1 : 1;
+ var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0;
+ value = Math.abs(value);
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0;
+ e = eMax;
+ }
+ else {
+ e = Math.floor(Math.log(value) / Math.LN2);
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--;
+ c *= 2;
+ }
+ if (e + eBias >= 1) {
+ value += rt / c;
+ }
+ else {
+ value += rt * Math.pow(2, 1 - eBias);
+ }
+ if (value * c >= 2) {
+ e++;
+ c /= 2;
+ }
+ if (e + eBias >= eMax) {
+ m = 0;
+ e = eMax;
+ }
+ else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen);
+ e = e + eBias;
+ }
+ else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
+ e = 0;
+ }
+ }
+ if (isNaN(value))
+ m = 0;
+ while (mLen >= 8) {
+ buffer[offset + i] = m & 0xff;
+ i += d;
+ m /= 256;
+ mLen -= 8;
+ }
+ e = (e << mLen) | m;
+ if (isNaN(value))
+ e += 8;
+ eLen += mLen;
+ while (eLen > 0) {
+ buffer[offset + i] = e & 0xff;
+ i += d;
+ e /= 256;
+ eLen -= 8;
+ }
+ buffer[offset + i - d] |= s * 128;
+}
+exports.writeIEEE754 = writeIEEE754;
+
+});
+
+unwrapExports(float_parser);
+float_parser.writeIEEE754;
+float_parser.readIEEE754;
+
+var serializer = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.serializeInto = void 0;
+
+
+
+
+
+
+
+
+var regexp = /\x00/; // eslint-disable-line no-control-regex
+var ignoreKeys = new Set(['$db', '$ref', '$id', '$clusterTime']);
+/*
+ * isArray indicates if we are writing to a BSON array (type 0x04)
+ * which forces the "key" which really an array index as a string to be written as ascii
+ * This will catch any errors in index as a string generation
+ */
+function serializeString(buffer, key, value, index, isArray) {
+ // Encode String type
+ buffer[index++] = constants.BSON_DATA_STRING;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes + 1;
+ buffer[index - 1] = 0;
+ // Write the string
+ var size = buffer.write(value, index + 4, undefined, 'utf8');
+ // Write the size of the string to buffer
+ buffer[index + 3] = ((size + 1) >> 24) & 0xff;
+ buffer[index + 2] = ((size + 1) >> 16) & 0xff;
+ buffer[index + 1] = ((size + 1) >> 8) & 0xff;
+ buffer[index] = (size + 1) & 0xff;
+ // Update index
+ index = index + 4 + size;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+}
+function serializeNumber(buffer, key, value, index, isArray) {
+ // We have an integer value
+ // TODO(NODE-2529): Add support for big int
+ if (Number.isInteger(value) &&
+ value >= constants.BSON_INT32_MIN &&
+ value <= constants.BSON_INT32_MAX) {
+ // If the value fits in 32 bits encode as int32
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ }
+ else {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ float_parser.writeIEEE754(buffer, value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ }
+ return index;
+}
+function serializeNull(buffer, key, _, index, isArray) {
+ // Set long type
+ buffer[index++] = constants.BSON_DATA_NULL;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+}
+function serializeBoolean(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BOOLEAN;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Encode the boolean value
+ buffer[index++] = value ? 1 : 0;
+ return index;
+}
+function serializeDate(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_DATE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ var dateInMilis = long_1.Long.fromNumber(value.getTime());
+ var lowBits = dateInMilis.getLowBits();
+ var highBits = dateInMilis.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+}
+function serializeRegExp(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ if (value.source && value.source.match(regexp) != null) {
+ throw Error('value ' + value.source + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.source, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the parameters
+ if (value.ignoreCase)
+ buffer[index++] = 0x69; // i
+ if (value.global)
+ buffer[index++] = 0x73; // s
+ if (value.multiline)
+ buffer[index++] = 0x6d; // m
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+}
+function serializeBSONRegExp(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Check the pattern for 0 bytes
+ if (value.pattern.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('pattern ' + value.pattern + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.pattern, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the options
+ index = index + buffer.write(value.options.split('').sort().join(''), index, undefined, 'utf8');
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+}
+function serializeMinMax(buffer, key, value, index, isArray) {
+ // Write the type of either min or max key
+ if (value === null) {
+ buffer[index++] = constants.BSON_DATA_NULL;
+ }
+ else if (value._bsontype === 'MinKey') {
+ buffer[index++] = constants.BSON_DATA_MIN_KEY;
+ }
+ else {
+ buffer[index++] = constants.BSON_DATA_MAX_KEY;
+ }
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+}
+function serializeObjectId(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OID;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the objectId into the shared buffer
+ if (typeof value.id === 'string') {
+ buffer.write(value.id, index, undefined, 'binary');
+ }
+ else if (utils.isUint8Array(value.id)) {
+ // Use the standard JS methods here because buffer.copy() is buggy with the
+ // browser polyfill
+ buffer.set(value.id.subarray(0, 12), index);
+ }
+ else {
+ throw new TypeError('object [' + JSON.stringify(value) + '] is not a valid ObjectId');
+ }
+ // Adjust index
+ return index + 12;
+}
+function serializeBuffer(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Get size of the buffer (current write point)
+ var size = value.length;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the default subtype
+ buffer[index++] = constants.BSON_BINARY_SUBTYPE_DEFAULT;
+ // Copy the content form the binary field to the buffer
+ buffer.set(ensure_buffer.ensureBuffer(value), index);
+ // Adjust the index
+ index = index + size;
+ return index;
+}
+function serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray, path) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (isArray === void 0) { isArray = false; }
+ if (path === void 0) { path = []; }
+ for (var i = 0; i < path.length; i++) {
+ if (path[i] === value)
+ throw new Error('cyclic dependency detected');
+ }
+ // Push value to stack
+ path.push(value);
+ // Write the type
+ buffer[index++] = Array.isArray(value) ? constants.BSON_DATA_ARRAY : constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ var endIndex = serializeInto(buffer, value, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined, path);
+ // Pop stack
+ path.pop();
+ return endIndex;
+}
+function serializeDecimal128(buffer, key, value, index, isArray) {
+ buffer[index++] = constants.BSON_DATA_DECIMAL128;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the data from the value
+ // Prefer the standard JS methods because their typechecking is not buggy,
+ // unlike the `buffer` polyfill's.
+ buffer.set(value.bytes.subarray(0, 16), index);
+ return index + 16;
+}
+function serializeLong(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] =
+ value._bsontype === 'Long' ? constants.BSON_DATA_LONG : constants.BSON_DATA_TIMESTAMP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ var lowBits = value.getLowBits();
+ var highBits = value.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+}
+function serializeInt32(buffer, key, value, index, isArray) {
+ value = value.valueOf();
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ return index;
+}
+function serializeDouble(buffer, key, value, index, isArray) {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ float_parser.writeIEEE754(buffer, value.value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ return index;
+}
+function serializeFunction(buffer, key, value, index, _checkKeys, _depth, isArray) {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ var functionString = utils.normalizedFunctionString(value);
+ // Write the string
+ var size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+}
+function serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (isArray === void 0) { isArray = false; }
+ if (value.scope && typeof value.scope === 'object') {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_CODE_W_SCOPE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Starting index
+ var startIndex = index;
+ // Serialize the function
+ // Get the function string
+ var functionString = typeof value.code === 'string' ? value.code : value.code.toString();
+ // Index adjustment
+ index = index + 4;
+ // Write string into buffer
+ var codeSize = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = codeSize & 0xff;
+ buffer[index + 1] = (codeSize >> 8) & 0xff;
+ buffer[index + 2] = (codeSize >> 16) & 0xff;
+ buffer[index + 3] = (codeSize >> 24) & 0xff;
+ // Write end 0
+ buffer[index + 4 + codeSize - 1] = 0;
+ // Write the
+ index = index + codeSize + 4;
+ //
+ // Serialize the scope value
+ var endIndex = serializeInto(buffer, value.scope, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined);
+ index = endIndex - 1;
+ // Writ the total
+ var totalSize = endIndex - startIndex;
+ // Write the total size of the object
+ buffer[startIndex++] = totalSize & 0xff;
+ buffer[startIndex++] = (totalSize >> 8) & 0xff;
+ buffer[startIndex++] = (totalSize >> 16) & 0xff;
+ buffer[startIndex++] = (totalSize >> 24) & 0xff;
+ // Write trailing zero
+ buffer[index++] = 0;
+ }
+ else {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ var functionString = value.code.toString();
+ // Write the string
+ var size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ }
+ return index;
+}
+function serializeBinary(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Extract the buffer
+ var data = value.value(true);
+ // Calculate size
+ var size = value.position;
+ // Add the deprecated 02 type 4 bytes of size to total
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY)
+ size = size + 4;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the subtype to the buffer
+ buffer[index++] = value.sub_type;
+ // If we have binary type 2 the 4 first bytes are the size
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ size = size - 4;
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ }
+ // Write the data to the object
+ buffer.set(data, index);
+ // Adjust the index
+ index = index + value.position;
+ return index;
+}
+function serializeSymbol(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_SYMBOL;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the string
+ var size = buffer.write(value.value, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0x00;
+ return index;
+}
+function serializeDBRef(buffer, key, value, index, depth, serializeFunctions, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ var startIndex = index;
+ var output = {
+ $ref: value.collection || value.namespace,
+ $id: value.oid
+ };
+ if (value.db != null) {
+ output.$db = value.db;
+ }
+ output = Object.assign(output, value.fields);
+ var endIndex = serializeInto(buffer, output, false, index, depth + 1, serializeFunctions);
+ // Calculate object size
+ var size = endIndex - startIndex;
+ // Write the size
+ buffer[startIndex++] = size & 0xff;
+ buffer[startIndex++] = (size >> 8) & 0xff;
+ buffer[startIndex++] = (size >> 16) & 0xff;
+ buffer[startIndex++] = (size >> 24) & 0xff;
+ // Set index
+ return endIndex;
+}
+function serializeInto(buffer, object, checkKeys, startingIndex, depth, serializeFunctions, ignoreUndefined, path) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (startingIndex === void 0) { startingIndex = 0; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (path === void 0) { path = []; }
+ startingIndex = startingIndex || 0;
+ path = path || [];
+ // Push the object to the path
+ path.push(object);
+ // Start place to serialize into
+ var index = startingIndex + 4;
+ // Special case isArray
+ if (Array.isArray(object)) {
+ // Get object keys
+ for (var i = 0; i < object.length; i++) {
+ var key = '' + i;
+ var value = object[i];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+ if (typeof value === 'string') {
+ index = serializeString(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'number') {
+ index = serializeNumber(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (typeof value === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index, true);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index, true);
+ }
+ else if (value === undefined) {
+ index = serializeNull(buffer, key, value, index, true);
+ }
+ else if (value === null) {
+ index = serializeNull(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index, true);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index, true);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true, path);
+ }
+ else if (typeof value === 'object' &&
+ extended_json.isBSONType(value) &&
+ value._bsontype === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, true);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions, true);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index, true);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ else if (object instanceof map.Map || utils.isMap(object)) {
+ var iterator = object.entries();
+ var done = false;
+ while (!done) {
+ // Unpack the next entry
+ var entry = iterator.next();
+ done = !!entry.done;
+ // Are we done, then skip and terminate
+ if (done)
+ continue;
+ // Get the entry values
+ var key = entry.value[0];
+ var value = entry.value[1];
+ // Check the type of the value
+ var type = typeof value;
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ }
+ else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ }
+ else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ }
+ else if (type === 'bigint' || utils.isBigInt64Array(value) || utils.isBigUInt64Array(value)) {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ }
+ else if (value === null || (value === undefined && ignoreUndefined === false)) {
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ }
+ else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
+ }
+ else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ else {
+ // Did we provide a custom serialization method
+ if (object.toBSON) {
+ if (typeof object.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ object = object.toBSON();
+ if (object != null && typeof object !== 'object')
+ throw new TypeError('toBSON function did not return an object');
+ }
+ // Iterate over all the keys
+ for (var key in object) {
+ var value = object[key];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+ // Check the type of the value
+ var type = typeof value;
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ }
+ else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ }
+ else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ }
+ else if (type === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ }
+ else if (value === undefined) {
+ if (ignoreUndefined === false)
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value === null) {
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ }
+ else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
+ }
+ else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ // Remove the path
+ path.pop();
+ // Final padding byte for object
+ buffer[index++] = 0x00;
+ // Final size
+ var size = index - startingIndex;
+ // Write the size of the object
+ buffer[startingIndex++] = size & 0xff;
+ buffer[startingIndex++] = (size >> 8) & 0xff;
+ buffer[startingIndex++] = (size >> 16) & 0xff;
+ buffer[startingIndex++] = (size >> 24) & 0xff;
+ return index;
+}
+exports.serializeInto = serializeInto;
+
+});
+
+unwrapExports(serializer);
+serializer.serializeInto;
+
+var bson = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.ObjectID = exports.Decimal128 = exports.BSONRegExp = exports.MaxKey = exports.MinKey = exports.Int32 = exports.Double = exports.Timestamp = exports.Long = exports.UUID = exports.ObjectId = exports.Binary = exports.DBRef = exports.BSONSymbol = exports.Map = exports.Code = exports.LongWithoutOverridesClass = exports.EJSON = exports.BSON_INT64_MIN = exports.BSON_INT64_MAX = exports.BSON_INT32_MIN = exports.BSON_INT32_MAX = exports.BSON_DATA_UNDEFINED = exports.BSON_DATA_TIMESTAMP = exports.BSON_DATA_SYMBOL = exports.BSON_DATA_STRING = exports.BSON_DATA_REGEXP = exports.BSON_DATA_OID = exports.BSON_DATA_OBJECT = exports.BSON_DATA_NUMBER = exports.BSON_DATA_NULL = exports.BSON_DATA_MIN_KEY = exports.BSON_DATA_MAX_KEY = exports.BSON_DATA_LONG = exports.BSON_DATA_INT = exports.BSON_DATA_DECIMAL128 = exports.BSON_DATA_DBPOINTER = exports.BSON_DATA_DATE = exports.BSON_DATA_CODE_W_SCOPE = exports.BSON_DATA_CODE = exports.BSON_DATA_BOOLEAN = exports.BSON_DATA_BINARY = exports.BSON_DATA_ARRAY = exports.BSON_BINARY_SUBTYPE_UUID_NEW = exports.BSON_BINARY_SUBTYPE_UUID = exports.BSON_BINARY_SUBTYPE_USER_DEFINED = exports.BSON_BINARY_SUBTYPE_MD5 = exports.BSON_BINARY_SUBTYPE_FUNCTION = exports.BSON_BINARY_SUBTYPE_DEFAULT = exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = void 0;
+exports.deserializeStream = exports.calculateObjectSize = exports.deserialize = exports.serializeWithBufferAndIndex = exports.serialize = exports.setInternalBufferSize = void 0;
+
+
+Object.defineProperty(exports, "Binary", { enumerable: true, get: function () { return binary.Binary; } });
+
+Object.defineProperty(exports, "Code", { enumerable: true, get: function () { return code.Code; } });
+
+Object.defineProperty(exports, "DBRef", { enumerable: true, get: function () { return db_ref.DBRef; } });
+
+Object.defineProperty(exports, "Decimal128", { enumerable: true, get: function () { return decimal128.Decimal128; } });
+
+Object.defineProperty(exports, "Double", { enumerable: true, get: function () { return double_1.Double; } });
+
+
+
+Object.defineProperty(exports, "Int32", { enumerable: true, get: function () { return int_32.Int32; } });
+
+Object.defineProperty(exports, "Long", { enumerable: true, get: function () { return long_1.Long; } });
+
+Object.defineProperty(exports, "Map", { enumerable: true, get: function () { return map.Map; } });
+
+Object.defineProperty(exports, "MaxKey", { enumerable: true, get: function () { return max_key.MaxKey; } });
+
+Object.defineProperty(exports, "MinKey", { enumerable: true, get: function () { return min_key.MinKey; } });
+
+Object.defineProperty(exports, "ObjectId", { enumerable: true, get: function () { return objectid.ObjectId; } });
+Object.defineProperty(exports, "ObjectID", { enumerable: true, get: function () { return objectid.ObjectId; } });
+
+// Parts of the parser
+
+
+
+Object.defineProperty(exports, "BSONRegExp", { enumerable: true, get: function () { return regexp.BSONRegExp; } });
+
+Object.defineProperty(exports, "BSONSymbol", { enumerable: true, get: function () { return symbol.BSONSymbol; } });
+
+Object.defineProperty(exports, "Timestamp", { enumerable: true, get: function () { return timestamp.Timestamp; } });
+
+Object.defineProperty(exports, "UUID", { enumerable: true, get: function () { return uuid.UUID; } });
+
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_BYTE_ARRAY", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_BYTE_ARRAY; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_DEFAULT", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_DEFAULT; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_FUNCTION", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_FUNCTION; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_MD5", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_MD5; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_USER_DEFINED", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_USER_DEFINED; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_UUID", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_UUID; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_UUID_NEW", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_UUID_NEW; } });
+Object.defineProperty(exports, "BSON_DATA_ARRAY", { enumerable: true, get: function () { return constants.BSON_DATA_ARRAY; } });
+Object.defineProperty(exports, "BSON_DATA_BINARY", { enumerable: true, get: function () { return constants.BSON_DATA_BINARY; } });
+Object.defineProperty(exports, "BSON_DATA_BOOLEAN", { enumerable: true, get: function () { return constants.BSON_DATA_BOOLEAN; } });
+Object.defineProperty(exports, "BSON_DATA_CODE", { enumerable: true, get: function () { return constants.BSON_DATA_CODE; } });
+Object.defineProperty(exports, "BSON_DATA_CODE_W_SCOPE", { enumerable: true, get: function () { return constants.BSON_DATA_CODE_W_SCOPE; } });
+Object.defineProperty(exports, "BSON_DATA_DATE", { enumerable: true, get: function () { return constants.BSON_DATA_DATE; } });
+Object.defineProperty(exports, "BSON_DATA_DBPOINTER", { enumerable: true, get: function () { return constants.BSON_DATA_DBPOINTER; } });
+Object.defineProperty(exports, "BSON_DATA_DECIMAL128", { enumerable: true, get: function () { return constants.BSON_DATA_DECIMAL128; } });
+Object.defineProperty(exports, "BSON_DATA_INT", { enumerable: true, get: function () { return constants.BSON_DATA_INT; } });
+Object.defineProperty(exports, "BSON_DATA_LONG", { enumerable: true, get: function () { return constants.BSON_DATA_LONG; } });
+Object.defineProperty(exports, "BSON_DATA_MAX_KEY", { enumerable: true, get: function () { return constants.BSON_DATA_MAX_KEY; } });
+Object.defineProperty(exports, "BSON_DATA_MIN_KEY", { enumerable: true, get: function () { return constants.BSON_DATA_MIN_KEY; } });
+Object.defineProperty(exports, "BSON_DATA_NULL", { enumerable: true, get: function () { return constants.BSON_DATA_NULL; } });
+Object.defineProperty(exports, "BSON_DATA_NUMBER", { enumerable: true, get: function () { return constants.BSON_DATA_NUMBER; } });
+Object.defineProperty(exports, "BSON_DATA_OBJECT", { enumerable: true, get: function () { return constants.BSON_DATA_OBJECT; } });
+Object.defineProperty(exports, "BSON_DATA_OID", { enumerable: true, get: function () { return constants.BSON_DATA_OID; } });
+Object.defineProperty(exports, "BSON_DATA_REGEXP", { enumerable: true, get: function () { return constants.BSON_DATA_REGEXP; } });
+Object.defineProperty(exports, "BSON_DATA_STRING", { enumerable: true, get: function () { return constants.BSON_DATA_STRING; } });
+Object.defineProperty(exports, "BSON_DATA_SYMBOL", { enumerable: true, get: function () { return constants.BSON_DATA_SYMBOL; } });
+Object.defineProperty(exports, "BSON_DATA_TIMESTAMP", { enumerable: true, get: function () { return constants.BSON_DATA_TIMESTAMP; } });
+Object.defineProperty(exports, "BSON_DATA_UNDEFINED", { enumerable: true, get: function () { return constants.BSON_DATA_UNDEFINED; } });
+Object.defineProperty(exports, "BSON_INT32_MAX", { enumerable: true, get: function () { return constants.BSON_INT32_MAX; } });
+Object.defineProperty(exports, "BSON_INT32_MIN", { enumerable: true, get: function () { return constants.BSON_INT32_MIN; } });
+Object.defineProperty(exports, "BSON_INT64_MAX", { enumerable: true, get: function () { return constants.BSON_INT64_MAX; } });
+Object.defineProperty(exports, "BSON_INT64_MIN", { enumerable: true, get: function () { return constants.BSON_INT64_MIN; } });
+var extended_json_2 = extended_json;
+Object.defineProperty(exports, "EJSON", { enumerable: true, get: function () { return extended_json_2.EJSON; } });
+var timestamp_2 = timestamp;
+Object.defineProperty(exports, "LongWithoutOverridesClass", { enumerable: true, get: function () { return timestamp_2.LongWithoutOverridesClass; } });
+/** @internal */
+// Default Max Size
+var MAXSIZE = 1024 * 1024 * 17;
+// Current Internal Temporary Serialization Buffer
+var buffer$1 = buffer.Buffer.alloc(MAXSIZE);
+/**
+ * Sets the size of the internal serialization buffer.
+ *
+ * @param size - The desired size for the internal serialization buffer
+ * @public
+ */
+function setInternalBufferSize(size) {
+ // Resize the internal serialization buffer if needed
+ if (buffer$1.length < size) {
+ buffer$1 = buffer.Buffer.alloc(size);
+ }
+}
+exports.setInternalBufferSize = setInternalBufferSize;
+/**
+ * Serialize a Javascript object.
+ *
+ * @param object - the Javascript object to serialize.
+ * @returns Buffer object containing the serialized object.
+ * @public
+ */
+function serialize(object, options) {
+ if (options === void 0) { options = {}; }
+ // Unpack the options
+ var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ var minInternalBufferSize = typeof options.minInternalBufferSize === 'number' ? options.minInternalBufferSize : MAXSIZE;
+ // Resize the internal serialization buffer if needed
+ if (buffer$1.length < minInternalBufferSize) {
+ buffer$1 = buffer.Buffer.alloc(minInternalBufferSize);
+ }
+ // Attempt to serialize
+ var serializationIndex = serializer.serializeInto(buffer$1, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined, []);
+ // Create the final buffer
+ var finishedBuffer = buffer.Buffer.alloc(serializationIndex);
+ // Copy into the finished buffer
+ buffer$1.copy(finishedBuffer, 0, 0, finishedBuffer.length);
+ // Return the buffer
+ return finishedBuffer;
+}
+exports.serialize = serialize;
+/**
+ * Serialize a Javascript object using a predefined Buffer and index into the buffer,
+ * useful when pre-allocating the space for serialization.
+ *
+ * @param object - the Javascript object to serialize.
+ * @param finalBuffer - the Buffer you pre-allocated to store the serialized BSON object.
+ * @returns the index pointing to the last written byte in the buffer.
+ * @public
+ */
+function serializeWithBufferAndIndex(object, finalBuffer, options) {
+ if (options === void 0) { options = {}; }
+ // Unpack the options
+ var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ var startIndex = typeof options.index === 'number' ? options.index : 0;
+ // Attempt to serialize
+ var serializationIndex = serializer.serializeInto(buffer$1, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined);
+ buffer$1.copy(finalBuffer, startIndex, 0, serializationIndex);
+ // Return the index
+ return startIndex + serializationIndex - 1;
+}
+exports.serializeWithBufferAndIndex = serializeWithBufferAndIndex;
+/**
+ * Deserialize data as BSON.
+ *
+ * @param buffer - the buffer containing the serialized set of BSON documents.
+ * @returns returns the deserialized Javascript Object.
+ * @public
+ */
+function deserialize(buffer, options) {
+ if (options === void 0) { options = {}; }
+ return deserializer.deserialize(ensure_buffer.ensureBuffer(buffer), options);
+}
+exports.deserialize = deserialize;
+/**
+ * Calculate the bson size for a passed in Javascript object.
+ *
+ * @param object - the Javascript object to calculate the BSON byte size for
+ * @returns size of BSON object in bytes
+ * @public
+ */
+function calculateObjectSize(object, options) {
+ if (options === void 0) { options = {}; }
+ options = options || {};
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ return calculate_size.calculateObjectSize(object, serializeFunctions, ignoreUndefined);
+}
+exports.calculateObjectSize = calculateObjectSize;
+/**
+ * Deserialize stream data as BSON documents.
+ *
+ * @param data - the buffer containing the serialized set of BSON documents.
+ * @param startIndex - the start index in the data Buffer where the deserialization is to start.
+ * @param numberOfDocuments - number of documents to deserialize.
+ * @param documents - an array where to store the deserialized documents.
+ * @param docStartIndex - the index in the documents array from where to start inserting documents.
+ * @param options - additional options used for the deserialization.
+ * @returns next index in the buffer after deserialization **x** numbers of documents.
+ * @public
+ */
+function deserializeStream(data, startIndex, numberOfDocuments, documents, docStartIndex, options) {
+ var internalOptions = Object.assign({ allowObjectSmallerThanBufferSize: true, index: 0 }, options);
+ var bufferData = ensure_buffer.ensureBuffer(data);
+ var index = startIndex;
+ // Loop over all documents
+ for (var i = 0; i < numberOfDocuments; i++) {
+ // Find size of the document
+ var size = bufferData[index] |
+ (bufferData[index + 1] << 8) |
+ (bufferData[index + 2] << 16) |
+ (bufferData[index + 3] << 24);
+ // Update options with index
+ internalOptions.index = index;
+ // Parse the document at this point
+ documents[docStartIndex + i] = deserializer.deserialize(bufferData, internalOptions);
+ // Adjust index by the document size
+ index = index + size;
+ }
+ // Return object containing end index of parsing and list of documents
+ return index;
+}
+exports.deserializeStream = deserializeStream;
+/**
+ * BSON default export
+ * @deprecated Please use named exports
+ * @privateRemarks
+ * We want to someday deprecate the default export,
+ * so none of the new TS types are being exported on the default
+ * @public
+ */
+var BSON = {
+ Binary: binary.Binary,
+ Code: code.Code,
+ DBRef: db_ref.DBRef,
+ Decimal128: decimal128.Decimal128,
+ Double: double_1.Double,
+ Int32: int_32.Int32,
+ Long: long_1.Long,
+ UUID: uuid.UUID,
+ Map: map.Map,
+ MaxKey: max_key.MaxKey,
+ MinKey: min_key.MinKey,
+ ObjectId: objectid.ObjectId,
+ ObjectID: objectid.ObjectId,
+ BSONRegExp: regexp.BSONRegExp,
+ BSONSymbol: symbol.BSONSymbol,
+ Timestamp: timestamp.Timestamp,
+ EJSON: extended_json.EJSON,
+ setInternalBufferSize: setInternalBufferSize,
+ serialize: serialize,
+ serializeWithBufferAndIndex: serializeWithBufferAndIndex,
+ deserialize: deserialize,
+ calculateObjectSize: calculateObjectSize,
+ deserializeStream: deserializeStream
+};
+exports.default = BSON;
+
+});
+
+var bson$1 = unwrapExports(bson);
+var bson_1 = bson.ObjectID;
+var bson_2 = bson.Decimal128;
+var bson_3 = bson.BSONRegExp;
+var bson_4 = bson.MaxKey;
+var bson_5 = bson.MinKey;
+var bson_6 = bson.Int32;
+var bson_7 = bson.Double;
+var bson_8 = bson.Timestamp;
+var bson_9 = bson.Long;
+var bson_10 = bson.UUID;
+var bson_11 = bson.ObjectId;
+var bson_12 = bson.Binary;
+var bson_13 = bson.DBRef;
+var bson_14 = bson.BSONSymbol;
+var bson_15 = bson.Map;
+var bson_16 = bson.Code;
+var bson_17 = bson.LongWithoutOverridesClass;
+var bson_18 = bson.EJSON;
+var bson_19 = bson.BSON_INT64_MIN;
+var bson_20 = bson.BSON_INT64_MAX;
+var bson_21 = bson.BSON_INT32_MIN;
+var bson_22 = bson.BSON_INT32_MAX;
+var bson_23 = bson.BSON_DATA_UNDEFINED;
+var bson_24 = bson.BSON_DATA_TIMESTAMP;
+var bson_25 = bson.BSON_DATA_SYMBOL;
+var bson_26 = bson.BSON_DATA_STRING;
+var bson_27 = bson.BSON_DATA_REGEXP;
+var bson_28 = bson.BSON_DATA_OID;
+var bson_29 = bson.BSON_DATA_OBJECT;
+var bson_30 = bson.BSON_DATA_NUMBER;
+var bson_31 = bson.BSON_DATA_NULL;
+var bson_32 = bson.BSON_DATA_MIN_KEY;
+var bson_33 = bson.BSON_DATA_MAX_KEY;
+var bson_34 = bson.BSON_DATA_LONG;
+var bson_35 = bson.BSON_DATA_INT;
+var bson_36 = bson.BSON_DATA_DECIMAL128;
+var bson_37 = bson.BSON_DATA_DBPOINTER;
+var bson_38 = bson.BSON_DATA_DATE;
+var bson_39 = bson.BSON_DATA_CODE_W_SCOPE;
+var bson_40 = bson.BSON_DATA_CODE;
+var bson_41 = bson.BSON_DATA_BOOLEAN;
+var bson_42 = bson.BSON_DATA_BINARY;
+var bson_43 = bson.BSON_DATA_ARRAY;
+var bson_44 = bson.BSON_BINARY_SUBTYPE_UUID_NEW;
+var bson_45 = bson.BSON_BINARY_SUBTYPE_UUID;
+var bson_46 = bson.BSON_BINARY_SUBTYPE_USER_DEFINED;
+var bson_47 = bson.BSON_BINARY_SUBTYPE_MD5;
+var bson_48 = bson.BSON_BINARY_SUBTYPE_FUNCTION;
+var bson_49 = bson.BSON_BINARY_SUBTYPE_DEFAULT;
+var bson_50 = bson.BSON_BINARY_SUBTYPE_BYTE_ARRAY;
+var bson_51 = bson.deserializeStream;
+var bson_52 = bson.calculateObjectSize;
+var bson_53 = bson.deserialize;
+var bson_54 = bson.serializeWithBufferAndIndex;
+var bson_55 = bson.serialize;
+var bson_56 = bson.setInternalBufferSize;
+
+export default bson$1;
+export { bson_3 as BSONRegExp, bson_14 as BSONSymbol, bson_50 as BSON_BINARY_SUBTYPE_BYTE_ARRAY, bson_49 as BSON_BINARY_SUBTYPE_DEFAULT, bson_48 as BSON_BINARY_SUBTYPE_FUNCTION, bson_47 as BSON_BINARY_SUBTYPE_MD5, bson_46 as BSON_BINARY_SUBTYPE_USER_DEFINED, bson_45 as BSON_BINARY_SUBTYPE_UUID, bson_44 as BSON_BINARY_SUBTYPE_UUID_NEW, bson_43 as BSON_DATA_ARRAY, bson_42 as BSON_DATA_BINARY, bson_41 as BSON_DATA_BOOLEAN, bson_40 as BSON_DATA_CODE, bson_39 as BSON_DATA_CODE_W_SCOPE, bson_38 as BSON_DATA_DATE, bson_37 as BSON_DATA_DBPOINTER, bson_36 as BSON_DATA_DECIMAL128, bson_35 as BSON_DATA_INT, bson_34 as BSON_DATA_LONG, bson_33 as BSON_DATA_MAX_KEY, bson_32 as BSON_DATA_MIN_KEY, bson_31 as BSON_DATA_NULL, bson_30 as BSON_DATA_NUMBER, bson_29 as BSON_DATA_OBJECT, bson_28 as BSON_DATA_OID, bson_27 as BSON_DATA_REGEXP, bson_26 as BSON_DATA_STRING, bson_25 as BSON_DATA_SYMBOL, bson_24 as BSON_DATA_TIMESTAMP, bson_23 as BSON_DATA_UNDEFINED, bson_22 as BSON_INT32_MAX, bson_21 as BSON_INT32_MIN, bson_20 as BSON_INT64_MAX, bson_19 as BSON_INT64_MIN, bson_12 as Binary, bson_16 as Code, bson_13 as DBRef, bson_2 as Decimal128, bson_7 as Double, bson_18 as EJSON, bson_6 as Int32, bson_9 as Long, bson_17 as LongWithoutOverridesClass, bson_15 as Map, bson_4 as MaxKey, bson_5 as MinKey, bson_1 as ObjectID, bson_11 as ObjectId, bson_8 as Timestamp, bson_10 as UUID, bson_52 as calculateObjectSize, bson_53 as deserialize, bson_51 as deserializeStream, bson_55 as serialize, bson_54 as serializeWithBufferAndIndex, bson_56 as setInternalBufferSize };
+//# sourceMappingURL=bson.browser.esm.js.map
diff --git a/node_modules/bson/dist/bson.browser.esm.js.map b/node_modules/bson/dist/bson.browser.esm.js.map
new file mode 100644
index 0000000..83843ff
--- /dev/null
+++ b/node_modules/bson/dist/bson.browser.esm.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"bson.browser.esm.js","sources":["../node_modules/base64-js/index.js","../node_modules/ieee754/index.js","../node_modules/buffer/index.js","../node_modules/rollup-plugin-node-builtins/src/es6/empty.js","../node_modules/process-es6/browser.js","../node_modules/rollup-plugin-node-builtins/src/es6/inherits.js","../node_modules/rollup-plugin-node-builtins/src/es6/util.js","../src/parser/utils.ts","../src/ensure_buffer.ts","../src/uuid_utils.ts","../src/uuid.ts","../src/binary.ts","../src/code.ts","../src/db_ref.ts","../src/long.ts","../src/decimal128.ts","../src/double.ts","../src/int_32.ts","../src/max_key.ts","../src/min_key.ts","../src/objectid.ts","../src/regexp.ts","../src/symbol.ts","../node_modules/tslib/tslib.es6.js","../src/timestamp.ts","../src/extended_json.ts","../src/map.ts","../src/constants.ts","../src/parser/calculate_size.ts","../src/validate_utf8.ts","../src/parser/deserializer.ts","../src/float_parser.ts","../src/parser/serializer.ts","../src/bson.ts"],"sourcesContent":["'use strict'\n\nexports.byteLength = byteLength\nexports.toByteArray = toByteArray\nexports.fromByteArray = fromByteArray\n\nvar lookup = []\nvar revLookup = []\nvar Arr = typeof Uint8Array !== 'undefined' ? Uint8Array : Array\n\nvar code = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/'\nfor (var i = 0, len = code.length; i < len; ++i) {\n lookup[i] = code[i]\n revLookup[code.charCodeAt(i)] = i\n}\n\n// Support decoding URL-safe base64 strings, as Node.js does.\n// See: https://en.wikipedia.org/wiki/Base64#URL_applications\nrevLookup['-'.charCodeAt(0)] = 62\nrevLookup['_'.charCodeAt(0)] = 63\n\nfunction getLens (b64) {\n var len = b64.length\n\n if (len % 4 > 0) {\n throw new Error('Invalid string. Length must be a multiple of 4')\n }\n\n // Trim off extra bytes after placeholder bytes are found\n // See: https://github.com/beatgammit/base64-js/issues/42\n var validLen = b64.indexOf('=')\n if (validLen === -1) validLen = len\n\n var placeHoldersLen = validLen === len\n ? 0\n : 4 - (validLen % 4)\n\n return [validLen, placeHoldersLen]\n}\n\n// base64 is 4/3 + up to two characters of the original data\nfunction byteLength (b64) {\n var lens = getLens(b64)\n var validLen = lens[0]\n var placeHoldersLen = lens[1]\n return ((validLen + placeHoldersLen) * 3 / 4) - placeHoldersLen\n}\n\nfunction _byteLength (b64, validLen, placeHoldersLen) {\n return ((validLen + placeHoldersLen) * 3 / 4) - placeHoldersLen\n}\n\nfunction toByteArray (b64) {\n var tmp\n var lens = getLens(b64)\n var validLen = lens[0]\n var placeHoldersLen = lens[1]\n\n var arr = new Arr(_byteLength(b64, validLen, placeHoldersLen))\n\n var curByte = 0\n\n // if there are placeholders, only get up to the last complete 4 chars\n var len = placeHoldersLen > 0\n ? validLen - 4\n : validLen\n\n var i\n for (i = 0; i < len; i += 4) {\n tmp =\n (revLookup[b64.charCodeAt(i)] << 18) |\n (revLookup[b64.charCodeAt(i + 1)] << 12) |\n (revLookup[b64.charCodeAt(i + 2)] << 6) |\n revLookup[b64.charCodeAt(i + 3)]\n arr[curByte++] = (tmp >> 16) & 0xFF\n arr[curByte++] = (tmp >> 8) & 0xFF\n arr[curByte++] = tmp & 0xFF\n }\n\n if (placeHoldersLen === 2) {\n tmp =\n (revLookup[b64.charCodeAt(i)] << 2) |\n (revLookup[b64.charCodeAt(i + 1)] >> 4)\n arr[curByte++] = tmp & 0xFF\n }\n\n if (placeHoldersLen === 1) {\n tmp =\n (revLookup[b64.charCodeAt(i)] << 10) |\n (revLookup[b64.charCodeAt(i + 1)] << 4) |\n (revLookup[b64.charCodeAt(i + 2)] >> 2)\n arr[curByte++] = (tmp >> 8) & 0xFF\n arr[curByte++] = tmp & 0xFF\n }\n\n return arr\n}\n\nfunction tripletToBase64 (num) {\n return lookup[num >> 18 & 0x3F] +\n lookup[num >> 12 & 0x3F] +\n lookup[num >> 6 & 0x3F] +\n lookup[num & 0x3F]\n}\n\nfunction encodeChunk (uint8, start, end) {\n var tmp\n var output = []\n for (var i = start; i < end; i += 3) {\n tmp =\n ((uint8[i] << 16) & 0xFF0000) +\n ((uint8[i + 1] << 8) & 0xFF00) +\n (uint8[i + 2] & 0xFF)\n output.push(tripletToBase64(tmp))\n }\n return output.join('')\n}\n\nfunction fromByteArray (uint8) {\n var tmp\n var len = uint8.length\n var extraBytes = len % 3 // if we have 1 byte left, pad 2 bytes\n var parts = []\n var maxChunkLength = 16383 // must be multiple of 3\n\n // go through the array every three bytes, we'll deal with trailing stuff later\n for (var i = 0, len2 = len - extraBytes; i < len2; i += maxChunkLength) {\n parts.push(encodeChunk(uint8, i, (i + maxChunkLength) > len2 ? len2 : (i + maxChunkLength)))\n }\n\n // pad the end with zeros, but make sure to not forget the extra bytes\n if (extraBytes === 1) {\n tmp = uint8[len - 1]\n parts.push(\n lookup[tmp >> 2] +\n lookup[(tmp << 4) & 0x3F] +\n '=='\n )\n } else if (extraBytes === 2) {\n tmp = (uint8[len - 2] << 8) + uint8[len - 1]\n parts.push(\n lookup[tmp >> 10] +\n lookup[(tmp >> 4) & 0x3F] +\n lookup[(tmp << 2) & 0x3F] +\n '='\n )\n }\n\n return parts.join('')\n}\n","/*! ieee754. BSD-3-Clause License. Feross Aboukhadijeh */\nexports.read = function (buffer, offset, isLE, mLen, nBytes) {\n var e, m\n var eLen = (nBytes * 8) - mLen - 1\n var eMax = (1 << eLen) - 1\n var eBias = eMax >> 1\n var nBits = -7\n var i = isLE ? (nBytes - 1) : 0\n var d = isLE ? -1 : 1\n var s = buffer[offset + i]\n\n i += d\n\n e = s & ((1 << (-nBits)) - 1)\n s >>= (-nBits)\n nBits += eLen\n for (; nBits > 0; e = (e * 256) + buffer[offset + i], i += d, nBits -= 8) {}\n\n m = e & ((1 << (-nBits)) - 1)\n e >>= (-nBits)\n nBits += mLen\n for (; nBits > 0; m = (m * 256) + buffer[offset + i], i += d, nBits -= 8) {}\n\n if (e === 0) {\n e = 1 - eBias\n } else if (e === eMax) {\n return m ? NaN : ((s ? -1 : 1) * Infinity)\n } else {\n m = m + Math.pow(2, mLen)\n e = e - eBias\n }\n return (s ? -1 : 1) * m * Math.pow(2, e - mLen)\n}\n\nexports.write = function (buffer, value, offset, isLE, mLen, nBytes) {\n var e, m, c\n var eLen = (nBytes * 8) - mLen - 1\n var eMax = (1 << eLen) - 1\n var eBias = eMax >> 1\n var rt = (mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0)\n var i = isLE ? 0 : (nBytes - 1)\n var d = isLE ? 1 : -1\n var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0\n\n value = Math.abs(value)\n\n if (isNaN(value) || value === Infinity) {\n m = isNaN(value) ? 1 : 0\n e = eMax\n } else {\n e = Math.floor(Math.log(value) / Math.LN2)\n if (value * (c = Math.pow(2, -e)) < 1) {\n e--\n c *= 2\n }\n if (e + eBias >= 1) {\n value += rt / c\n } else {\n value += rt * Math.pow(2, 1 - eBias)\n }\n if (value * c >= 2) {\n e++\n c /= 2\n }\n\n if (e + eBias >= eMax) {\n m = 0\n e = eMax\n } else if (e + eBias >= 1) {\n m = ((value * c) - 1) * Math.pow(2, mLen)\n e = e + eBias\n } else {\n m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen)\n e = 0\n }\n }\n\n for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}\n\n e = (e << mLen) | m\n eLen += mLen\n for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}\n\n buffer[offset + i - d] |= s * 128\n}\n","/*!\n * The buffer module from node.js, for the browser.\n *\n * @author Feross Aboukhadijeh \n * @license MIT\n */\n/* eslint-disable no-proto */\n\n'use strict'\n\nvar base64 = require('base64-js')\nvar ieee754 = require('ieee754')\nvar customInspectSymbol =\n (typeof Symbol === 'function' && typeof Symbol['for'] === 'function') // eslint-disable-line dot-notation\n ? Symbol['for']('nodejs.util.inspect.custom') // eslint-disable-line dot-notation\n : null\n\nexports.Buffer = Buffer\nexports.SlowBuffer = SlowBuffer\nexports.INSPECT_MAX_BYTES = 50\n\nvar K_MAX_LENGTH = 0x7fffffff\nexports.kMaxLength = K_MAX_LENGTH\n\n/**\n * If `Buffer.TYPED_ARRAY_SUPPORT`:\n * === true Use Uint8Array implementation (fastest)\n * === false Print warning and recommend using `buffer` v4.x which has an Object\n * implementation (most compatible, even IE6)\n *\n * Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,\n * Opera 11.6+, iOS 4.2+.\n *\n * We report that the browser does not support typed arrays if the are not subclassable\n * using __proto__. Firefox 4-29 lacks support for adding new properties to `Uint8Array`\n * (See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438). IE 10 lacks support\n * for __proto__ and has a buggy typed array implementation.\n */\nBuffer.TYPED_ARRAY_SUPPORT = typedArraySupport()\n\nif (!Buffer.TYPED_ARRAY_SUPPORT && typeof console !== 'undefined' &&\n typeof console.error === 'function') {\n console.error(\n 'This browser lacks typed array (Uint8Array) support which is required by ' +\n '`buffer` v5.x. Use `buffer` v4.x if you require old browser support.'\n )\n}\n\nfunction typedArraySupport () {\n // Can typed array instances can be augmented?\n try {\n var arr = new Uint8Array(1)\n var proto = { foo: function () { return 42 } }\n Object.setPrototypeOf(proto, Uint8Array.prototype)\n Object.setPrototypeOf(arr, proto)\n return arr.foo() === 42\n } catch (e) {\n return false\n }\n}\n\nObject.defineProperty(Buffer.prototype, 'parent', {\n enumerable: true,\n get: function () {\n if (!Buffer.isBuffer(this)) return undefined\n return this.buffer\n }\n})\n\nObject.defineProperty(Buffer.prototype, 'offset', {\n enumerable: true,\n get: function () {\n if (!Buffer.isBuffer(this)) return undefined\n return this.byteOffset\n }\n})\n\nfunction createBuffer (length) {\n if (length > K_MAX_LENGTH) {\n throw new RangeError('The value \"' + length + '\" is invalid for option \"size\"')\n }\n // Return an augmented `Uint8Array` instance\n var buf = new Uint8Array(length)\n Object.setPrototypeOf(buf, Buffer.prototype)\n return buf\n}\n\n/**\n * The Buffer constructor returns instances of `Uint8Array` that have their\n * prototype changed to `Buffer.prototype`. Furthermore, `Buffer` is a subclass of\n * `Uint8Array`, so the returned instances will have all the node `Buffer` methods\n * and the `Uint8Array` methods. Square bracket notation works as expected -- it\n * returns a single octet.\n *\n * The `Uint8Array` prototype remains unmodified.\n */\n\nfunction Buffer (arg, encodingOrOffset, length) {\n // Common case.\n if (typeof arg === 'number') {\n if (typeof encodingOrOffset === 'string') {\n throw new TypeError(\n 'The \"string\" argument must be of type string. Received type number'\n )\n }\n return allocUnsafe(arg)\n }\n return from(arg, encodingOrOffset, length)\n}\n\nBuffer.poolSize = 8192 // not used by this implementation\n\nfunction from (value, encodingOrOffset, length) {\n if (typeof value === 'string') {\n return fromString(value, encodingOrOffset)\n }\n\n if (ArrayBuffer.isView(value)) {\n return fromArrayView(value)\n }\n\n if (value == null) {\n throw new TypeError(\n 'The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' +\n 'or Array-like Object. Received type ' + (typeof value)\n )\n }\n\n if (isInstance(value, ArrayBuffer) ||\n (value && isInstance(value.buffer, ArrayBuffer))) {\n return fromArrayBuffer(value, encodingOrOffset, length)\n }\n\n if (typeof SharedArrayBuffer !== 'undefined' &&\n (isInstance(value, SharedArrayBuffer) ||\n (value && isInstance(value.buffer, SharedArrayBuffer)))) {\n return fromArrayBuffer(value, encodingOrOffset, length)\n }\n\n if (typeof value === 'number') {\n throw new TypeError(\n 'The \"value\" argument must not be of type number. Received type number'\n )\n }\n\n var valueOf = value.valueOf && value.valueOf()\n if (valueOf != null && valueOf !== value) {\n return Buffer.from(valueOf, encodingOrOffset, length)\n }\n\n var b = fromObject(value)\n if (b) return b\n\n if (typeof Symbol !== 'undefined' && Symbol.toPrimitive != null &&\n typeof value[Symbol.toPrimitive] === 'function') {\n return Buffer.from(\n value[Symbol.toPrimitive]('string'), encodingOrOffset, length\n )\n }\n\n throw new TypeError(\n 'The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' +\n 'or Array-like Object. Received type ' + (typeof value)\n )\n}\n\n/**\n * Functionally equivalent to Buffer(arg, encoding) but throws a TypeError\n * if value is a number.\n * Buffer.from(str[, encoding])\n * Buffer.from(array)\n * Buffer.from(buffer)\n * Buffer.from(arrayBuffer[, byteOffset[, length]])\n **/\nBuffer.from = function (value, encodingOrOffset, length) {\n return from(value, encodingOrOffset, length)\n}\n\n// Note: Change prototype *after* Buffer.from is defined to workaround Chrome bug:\n// https://github.com/feross/buffer/pull/148\nObject.setPrototypeOf(Buffer.prototype, Uint8Array.prototype)\nObject.setPrototypeOf(Buffer, Uint8Array)\n\nfunction assertSize (size) {\n if (typeof size !== 'number') {\n throw new TypeError('\"size\" argument must be of type number')\n } else if (size < 0) {\n throw new RangeError('The value \"' + size + '\" is invalid for option \"size\"')\n }\n}\n\nfunction alloc (size, fill, encoding) {\n assertSize(size)\n if (size <= 0) {\n return createBuffer(size)\n }\n if (fill !== undefined) {\n // Only pay attention to encoding if it's a string. This\n // prevents accidentally sending in a number that would\n // be interpreted as a start offset.\n return typeof encoding === 'string'\n ? createBuffer(size).fill(fill, encoding)\n : createBuffer(size).fill(fill)\n }\n return createBuffer(size)\n}\n\n/**\n * Creates a new filled Buffer instance.\n * alloc(size[, fill[, encoding]])\n **/\nBuffer.alloc = function (size, fill, encoding) {\n return alloc(size, fill, encoding)\n}\n\nfunction allocUnsafe (size) {\n assertSize(size)\n return createBuffer(size < 0 ? 0 : checked(size) | 0)\n}\n\n/**\n * Equivalent to Buffer(num), by default creates a non-zero-filled Buffer instance.\n * */\nBuffer.allocUnsafe = function (size) {\n return allocUnsafe(size)\n}\n/**\n * Equivalent to SlowBuffer(num), by default creates a non-zero-filled Buffer instance.\n */\nBuffer.allocUnsafeSlow = function (size) {\n return allocUnsafe(size)\n}\n\nfunction fromString (string, encoding) {\n if (typeof encoding !== 'string' || encoding === '') {\n encoding = 'utf8'\n }\n\n if (!Buffer.isEncoding(encoding)) {\n throw new TypeError('Unknown encoding: ' + encoding)\n }\n\n var length = byteLength(string, encoding) | 0\n var buf = createBuffer(length)\n\n var actual = buf.write(string, encoding)\n\n if (actual !== length) {\n // Writing a hex string, for example, that contains invalid characters will\n // cause everything after the first invalid character to be ignored. (e.g.\n // 'abxxcd' will be treated as 'ab')\n buf = buf.slice(0, actual)\n }\n\n return buf\n}\n\nfunction fromArrayLike (array) {\n var length = array.length < 0 ? 0 : checked(array.length) | 0\n var buf = createBuffer(length)\n for (var i = 0; i < length; i += 1) {\n buf[i] = array[i] & 255\n }\n return buf\n}\n\nfunction fromArrayView (arrayView) {\n if (isInstance(arrayView, Uint8Array)) {\n var copy = new Uint8Array(arrayView)\n return fromArrayBuffer(copy.buffer, copy.byteOffset, copy.byteLength)\n }\n return fromArrayLike(arrayView)\n}\n\nfunction fromArrayBuffer (array, byteOffset, length) {\n if (byteOffset < 0 || array.byteLength < byteOffset) {\n throw new RangeError('\"offset\" is outside of buffer bounds')\n }\n\n if (array.byteLength < byteOffset + (length || 0)) {\n throw new RangeError('\"length\" is outside of buffer bounds')\n }\n\n var buf\n if (byteOffset === undefined && length === undefined) {\n buf = new Uint8Array(array)\n } else if (length === undefined) {\n buf = new Uint8Array(array, byteOffset)\n } else {\n buf = new Uint8Array(array, byteOffset, length)\n }\n\n // Return an augmented `Uint8Array` instance\n Object.setPrototypeOf(buf, Buffer.prototype)\n\n return buf\n}\n\nfunction fromObject (obj) {\n if (Buffer.isBuffer(obj)) {\n var len = checked(obj.length) | 0\n var buf = createBuffer(len)\n\n if (buf.length === 0) {\n return buf\n }\n\n obj.copy(buf, 0, 0, len)\n return buf\n }\n\n if (obj.length !== undefined) {\n if (typeof obj.length !== 'number' || numberIsNaN(obj.length)) {\n return createBuffer(0)\n }\n return fromArrayLike(obj)\n }\n\n if (obj.type === 'Buffer' && Array.isArray(obj.data)) {\n return fromArrayLike(obj.data)\n }\n}\n\nfunction checked (length) {\n // Note: cannot use `length < K_MAX_LENGTH` here because that fails when\n // length is NaN (which is otherwise coerced to zero.)\n if (length >= K_MAX_LENGTH) {\n throw new RangeError('Attempt to allocate Buffer larger than maximum ' +\n 'size: 0x' + K_MAX_LENGTH.toString(16) + ' bytes')\n }\n return length | 0\n}\n\nfunction SlowBuffer (length) {\n if (+length != length) { // eslint-disable-line eqeqeq\n length = 0\n }\n return Buffer.alloc(+length)\n}\n\nBuffer.isBuffer = function isBuffer (b) {\n return b != null && b._isBuffer === true &&\n b !== Buffer.prototype // so Buffer.isBuffer(Buffer.prototype) will be false\n}\n\nBuffer.compare = function compare (a, b) {\n if (isInstance(a, Uint8Array)) a = Buffer.from(a, a.offset, a.byteLength)\n if (isInstance(b, Uint8Array)) b = Buffer.from(b, b.offset, b.byteLength)\n if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {\n throw new TypeError(\n 'The \"buf1\", \"buf2\" arguments must be one of type Buffer or Uint8Array'\n )\n }\n\n if (a === b) return 0\n\n var x = a.length\n var y = b.length\n\n for (var i = 0, len = Math.min(x, y); i < len; ++i) {\n if (a[i] !== b[i]) {\n x = a[i]\n y = b[i]\n break\n }\n }\n\n if (x < y) return -1\n if (y < x) return 1\n return 0\n}\n\nBuffer.isEncoding = function isEncoding (encoding) {\n switch (String(encoding).toLowerCase()) {\n case 'hex':\n case 'utf8':\n case 'utf-8':\n case 'ascii':\n case 'latin1':\n case 'binary':\n case 'base64':\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return true\n default:\n return false\n }\n}\n\nBuffer.concat = function concat (list, length) {\n if (!Array.isArray(list)) {\n throw new TypeError('\"list\" argument must be an Array of Buffers')\n }\n\n if (list.length === 0) {\n return Buffer.alloc(0)\n }\n\n var i\n if (length === undefined) {\n length = 0\n for (i = 0; i < list.length; ++i) {\n length += list[i].length\n }\n }\n\n var buffer = Buffer.allocUnsafe(length)\n var pos = 0\n for (i = 0; i < list.length; ++i) {\n var buf = list[i]\n if (isInstance(buf, Uint8Array)) {\n if (pos + buf.length > buffer.length) {\n Buffer.from(buf).copy(buffer, pos)\n } else {\n Uint8Array.prototype.set.call(\n buffer,\n buf,\n pos\n )\n }\n } else if (!Buffer.isBuffer(buf)) {\n throw new TypeError('\"list\" argument must be an Array of Buffers')\n } else {\n buf.copy(buffer, pos)\n }\n pos += buf.length\n }\n return buffer\n}\n\nfunction byteLength (string, encoding) {\n if (Buffer.isBuffer(string)) {\n return string.length\n }\n if (ArrayBuffer.isView(string) || isInstance(string, ArrayBuffer)) {\n return string.byteLength\n }\n if (typeof string !== 'string') {\n throw new TypeError(\n 'The \"string\" argument must be one of type string, Buffer, or ArrayBuffer. ' +\n 'Received type ' + typeof string\n )\n }\n\n var len = string.length\n var mustMatch = (arguments.length > 2 && arguments[2] === true)\n if (!mustMatch && len === 0) return 0\n\n // Use a for loop to avoid recursion\n var loweredCase = false\n for (;;) {\n switch (encoding) {\n case 'ascii':\n case 'latin1':\n case 'binary':\n return len\n case 'utf8':\n case 'utf-8':\n return utf8ToBytes(string).length\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return len * 2\n case 'hex':\n return len >>> 1\n case 'base64':\n return base64ToBytes(string).length\n default:\n if (loweredCase) {\n return mustMatch ? -1 : utf8ToBytes(string).length // assume utf8\n }\n encoding = ('' + encoding).toLowerCase()\n loweredCase = true\n }\n }\n}\nBuffer.byteLength = byteLength\n\nfunction slowToString (encoding, start, end) {\n var loweredCase = false\n\n // No need to verify that \"this.length <= MAX_UINT32\" since it's a read-only\n // property of a typed array.\n\n // This behaves neither like String nor Uint8Array in that we set start/end\n // to their upper/lower bounds if the value passed is out of range.\n // undefined is handled specially as per ECMA-262 6th Edition,\n // Section 13.3.3.7 Runtime Semantics: KeyedBindingInitialization.\n if (start === undefined || start < 0) {\n start = 0\n }\n // Return early if start > this.length. Done here to prevent potential uint32\n // coercion fail below.\n if (start > this.length) {\n return ''\n }\n\n if (end === undefined || end > this.length) {\n end = this.length\n }\n\n if (end <= 0) {\n return ''\n }\n\n // Force coercion to uint32. This will also coerce falsey/NaN values to 0.\n end >>>= 0\n start >>>= 0\n\n if (end <= start) {\n return ''\n }\n\n if (!encoding) encoding = 'utf8'\n\n while (true) {\n switch (encoding) {\n case 'hex':\n return hexSlice(this, start, end)\n\n case 'utf8':\n case 'utf-8':\n return utf8Slice(this, start, end)\n\n case 'ascii':\n return asciiSlice(this, start, end)\n\n case 'latin1':\n case 'binary':\n return latin1Slice(this, start, end)\n\n case 'base64':\n return base64Slice(this, start, end)\n\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return utf16leSlice(this, start, end)\n\n default:\n if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)\n encoding = (encoding + '').toLowerCase()\n loweredCase = true\n }\n }\n}\n\n// This property is used by `Buffer.isBuffer` (and the `is-buffer` npm package)\n// to detect a Buffer instance. It's not possible to use `instanceof Buffer`\n// reliably in a browserify context because there could be multiple different\n// copies of the 'buffer' package in use. This method works even for Buffer\n// instances that were created from another copy of the `buffer` package.\n// See: https://github.com/feross/buffer/issues/154\nBuffer.prototype._isBuffer = true\n\nfunction swap (b, n, m) {\n var i = b[n]\n b[n] = b[m]\n b[m] = i\n}\n\nBuffer.prototype.swap16 = function swap16 () {\n var len = this.length\n if (len % 2 !== 0) {\n throw new RangeError('Buffer size must be a multiple of 16-bits')\n }\n for (var i = 0; i < len; i += 2) {\n swap(this, i, i + 1)\n }\n return this\n}\n\nBuffer.prototype.swap32 = function swap32 () {\n var len = this.length\n if (len % 4 !== 0) {\n throw new RangeError('Buffer size must be a multiple of 32-bits')\n }\n for (var i = 0; i < len; i += 4) {\n swap(this, i, i + 3)\n swap(this, i + 1, i + 2)\n }\n return this\n}\n\nBuffer.prototype.swap64 = function swap64 () {\n var len = this.length\n if (len % 8 !== 0) {\n throw new RangeError('Buffer size must be a multiple of 64-bits')\n }\n for (var i = 0; i < len; i += 8) {\n swap(this, i, i + 7)\n swap(this, i + 1, i + 6)\n swap(this, i + 2, i + 5)\n swap(this, i + 3, i + 4)\n }\n return this\n}\n\nBuffer.prototype.toString = function toString () {\n var length = this.length\n if (length === 0) return ''\n if (arguments.length === 0) return utf8Slice(this, 0, length)\n return slowToString.apply(this, arguments)\n}\n\nBuffer.prototype.toLocaleString = Buffer.prototype.toString\n\nBuffer.prototype.equals = function equals (b) {\n if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer')\n if (this === b) return true\n return Buffer.compare(this, b) === 0\n}\n\nBuffer.prototype.inspect = function inspect () {\n var str = ''\n var max = exports.INSPECT_MAX_BYTES\n str = this.toString('hex', 0, max).replace(/(.{2})/g, '$1 ').trim()\n if (this.length > max) str += ' ... '\n return ''\n}\nif (customInspectSymbol) {\n Buffer.prototype[customInspectSymbol] = Buffer.prototype.inspect\n}\n\nBuffer.prototype.compare = function compare (target, start, end, thisStart, thisEnd) {\n if (isInstance(target, Uint8Array)) {\n target = Buffer.from(target, target.offset, target.byteLength)\n }\n if (!Buffer.isBuffer(target)) {\n throw new TypeError(\n 'The \"target\" argument must be one of type Buffer or Uint8Array. ' +\n 'Received type ' + (typeof target)\n )\n }\n\n if (start === undefined) {\n start = 0\n }\n if (end === undefined) {\n end = target ? target.length : 0\n }\n if (thisStart === undefined) {\n thisStart = 0\n }\n if (thisEnd === undefined) {\n thisEnd = this.length\n }\n\n if (start < 0 || end > target.length || thisStart < 0 || thisEnd > this.length) {\n throw new RangeError('out of range index')\n }\n\n if (thisStart >= thisEnd && start >= end) {\n return 0\n }\n if (thisStart >= thisEnd) {\n return -1\n }\n if (start >= end) {\n return 1\n }\n\n start >>>= 0\n end >>>= 0\n thisStart >>>= 0\n thisEnd >>>= 0\n\n if (this === target) return 0\n\n var x = thisEnd - thisStart\n var y = end - start\n var len = Math.min(x, y)\n\n var thisCopy = this.slice(thisStart, thisEnd)\n var targetCopy = target.slice(start, end)\n\n for (var i = 0; i < len; ++i) {\n if (thisCopy[i] !== targetCopy[i]) {\n x = thisCopy[i]\n y = targetCopy[i]\n break\n }\n }\n\n if (x < y) return -1\n if (y < x) return 1\n return 0\n}\n\n// Finds either the first index of `val` in `buffer` at offset >= `byteOffset`,\n// OR the last index of `val` in `buffer` at offset <= `byteOffset`.\n//\n// Arguments:\n// - buffer - a Buffer to search\n// - val - a string, Buffer, or number\n// - byteOffset - an index into `buffer`; will be clamped to an int32\n// - encoding - an optional encoding, relevant is val is a string\n// - dir - true for indexOf, false for lastIndexOf\nfunction bidirectionalIndexOf (buffer, val, byteOffset, encoding, dir) {\n // Empty buffer means no match\n if (buffer.length === 0) return -1\n\n // Normalize byteOffset\n if (typeof byteOffset === 'string') {\n encoding = byteOffset\n byteOffset = 0\n } else if (byteOffset > 0x7fffffff) {\n byteOffset = 0x7fffffff\n } else if (byteOffset < -0x80000000) {\n byteOffset = -0x80000000\n }\n byteOffset = +byteOffset // Coerce to Number.\n if (numberIsNaN(byteOffset)) {\n // byteOffset: it it's undefined, null, NaN, \"foo\", etc, search whole buffer\n byteOffset = dir ? 0 : (buffer.length - 1)\n }\n\n // Normalize byteOffset: negative offsets start from the end of the buffer\n if (byteOffset < 0) byteOffset = buffer.length + byteOffset\n if (byteOffset >= buffer.length) {\n if (dir) return -1\n else byteOffset = buffer.length - 1\n } else if (byteOffset < 0) {\n if (dir) byteOffset = 0\n else return -1\n }\n\n // Normalize val\n if (typeof val === 'string') {\n val = Buffer.from(val, encoding)\n }\n\n // Finally, search either indexOf (if dir is true) or lastIndexOf\n if (Buffer.isBuffer(val)) {\n // Special case: looking for empty string/buffer always fails\n if (val.length === 0) {\n return -1\n }\n return arrayIndexOf(buffer, val, byteOffset, encoding, dir)\n } else if (typeof val === 'number') {\n val = val & 0xFF // Search for a byte value [0-255]\n if (typeof Uint8Array.prototype.indexOf === 'function') {\n if (dir) {\n return Uint8Array.prototype.indexOf.call(buffer, val, byteOffset)\n } else {\n return Uint8Array.prototype.lastIndexOf.call(buffer, val, byteOffset)\n }\n }\n return arrayIndexOf(buffer, [val], byteOffset, encoding, dir)\n }\n\n throw new TypeError('val must be string, number or Buffer')\n}\n\nfunction arrayIndexOf (arr, val, byteOffset, encoding, dir) {\n var indexSize = 1\n var arrLength = arr.length\n var valLength = val.length\n\n if (encoding !== undefined) {\n encoding = String(encoding).toLowerCase()\n if (encoding === 'ucs2' || encoding === 'ucs-2' ||\n encoding === 'utf16le' || encoding === 'utf-16le') {\n if (arr.length < 2 || val.length < 2) {\n return -1\n }\n indexSize = 2\n arrLength /= 2\n valLength /= 2\n byteOffset /= 2\n }\n }\n\n function read (buf, i) {\n if (indexSize === 1) {\n return buf[i]\n } else {\n return buf.readUInt16BE(i * indexSize)\n }\n }\n\n var i\n if (dir) {\n var foundIndex = -1\n for (i = byteOffset; i < arrLength; i++) {\n if (read(arr, i) === read(val, foundIndex === -1 ? 0 : i - foundIndex)) {\n if (foundIndex === -1) foundIndex = i\n if (i - foundIndex + 1 === valLength) return foundIndex * indexSize\n } else {\n if (foundIndex !== -1) i -= i - foundIndex\n foundIndex = -1\n }\n }\n } else {\n if (byteOffset + valLength > arrLength) byteOffset = arrLength - valLength\n for (i = byteOffset; i >= 0; i--) {\n var found = true\n for (var j = 0; j < valLength; j++) {\n if (read(arr, i + j) !== read(val, j)) {\n found = false\n break\n }\n }\n if (found) return i\n }\n }\n\n return -1\n}\n\nBuffer.prototype.includes = function includes (val, byteOffset, encoding) {\n return this.indexOf(val, byteOffset, encoding) !== -1\n}\n\nBuffer.prototype.indexOf = function indexOf (val, byteOffset, encoding) {\n return bidirectionalIndexOf(this, val, byteOffset, encoding, true)\n}\n\nBuffer.prototype.lastIndexOf = function lastIndexOf (val, byteOffset, encoding) {\n return bidirectionalIndexOf(this, val, byteOffset, encoding, false)\n}\n\nfunction hexWrite (buf, string, offset, length) {\n offset = Number(offset) || 0\n var remaining = buf.length - offset\n if (!length) {\n length = remaining\n } else {\n length = Number(length)\n if (length > remaining) {\n length = remaining\n }\n }\n\n var strLen = string.length\n\n if (length > strLen / 2) {\n length = strLen / 2\n }\n for (var i = 0; i < length; ++i) {\n var parsed = parseInt(string.substr(i * 2, 2), 16)\n if (numberIsNaN(parsed)) return i\n buf[offset + i] = parsed\n }\n return i\n}\n\nfunction utf8Write (buf, string, offset, length) {\n return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length)\n}\n\nfunction asciiWrite (buf, string, offset, length) {\n return blitBuffer(asciiToBytes(string), buf, offset, length)\n}\n\nfunction base64Write (buf, string, offset, length) {\n return blitBuffer(base64ToBytes(string), buf, offset, length)\n}\n\nfunction ucs2Write (buf, string, offset, length) {\n return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length)\n}\n\nBuffer.prototype.write = function write (string, offset, length, encoding) {\n // Buffer#write(string)\n if (offset === undefined) {\n encoding = 'utf8'\n length = this.length\n offset = 0\n // Buffer#write(string, encoding)\n } else if (length === undefined && typeof offset === 'string') {\n encoding = offset\n length = this.length\n offset = 0\n // Buffer#write(string, offset[, length][, encoding])\n } else if (isFinite(offset)) {\n offset = offset >>> 0\n if (isFinite(length)) {\n length = length >>> 0\n if (encoding === undefined) encoding = 'utf8'\n } else {\n encoding = length\n length = undefined\n }\n } else {\n throw new Error(\n 'Buffer.write(string, encoding, offset[, length]) is no longer supported'\n )\n }\n\n var remaining = this.length - offset\n if (length === undefined || length > remaining) length = remaining\n\n if ((string.length > 0 && (length < 0 || offset < 0)) || offset > this.length) {\n throw new RangeError('Attempt to write outside buffer bounds')\n }\n\n if (!encoding) encoding = 'utf8'\n\n var loweredCase = false\n for (;;) {\n switch (encoding) {\n case 'hex':\n return hexWrite(this, string, offset, length)\n\n case 'utf8':\n case 'utf-8':\n return utf8Write(this, string, offset, length)\n\n case 'ascii':\n case 'latin1':\n case 'binary':\n return asciiWrite(this, string, offset, length)\n\n case 'base64':\n // Warning: maxLength not taken into account in base64Write\n return base64Write(this, string, offset, length)\n\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return ucs2Write(this, string, offset, length)\n\n default:\n if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)\n encoding = ('' + encoding).toLowerCase()\n loweredCase = true\n }\n }\n}\n\nBuffer.prototype.toJSON = function toJSON () {\n return {\n type: 'Buffer',\n data: Array.prototype.slice.call(this._arr || this, 0)\n }\n}\n\nfunction base64Slice (buf, start, end) {\n if (start === 0 && end === buf.length) {\n return base64.fromByteArray(buf)\n } else {\n return base64.fromByteArray(buf.slice(start, end))\n }\n}\n\nfunction utf8Slice (buf, start, end) {\n end = Math.min(buf.length, end)\n var res = []\n\n var i = start\n while (i < end) {\n var firstByte = buf[i]\n var codePoint = null\n var bytesPerSequence = (firstByte > 0xEF)\n ? 4\n : (firstByte > 0xDF)\n ? 3\n : (firstByte > 0xBF)\n ? 2\n : 1\n\n if (i + bytesPerSequence <= end) {\n var secondByte, thirdByte, fourthByte, tempCodePoint\n\n switch (bytesPerSequence) {\n case 1:\n if (firstByte < 0x80) {\n codePoint = firstByte\n }\n break\n case 2:\n secondByte = buf[i + 1]\n if ((secondByte & 0xC0) === 0x80) {\n tempCodePoint = (firstByte & 0x1F) << 0x6 | (secondByte & 0x3F)\n if (tempCodePoint > 0x7F) {\n codePoint = tempCodePoint\n }\n }\n break\n case 3:\n secondByte = buf[i + 1]\n thirdByte = buf[i + 2]\n if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {\n tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | (thirdByte & 0x3F)\n if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {\n codePoint = tempCodePoint\n }\n }\n break\n case 4:\n secondByte = buf[i + 1]\n thirdByte = buf[i + 2]\n fourthByte = buf[i + 3]\n if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {\n tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | (fourthByte & 0x3F)\n if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {\n codePoint = tempCodePoint\n }\n }\n }\n }\n\n if (codePoint === null) {\n // we did not generate a valid codePoint so insert a\n // replacement char (U+FFFD) and advance only 1 byte\n codePoint = 0xFFFD\n bytesPerSequence = 1\n } else if (codePoint > 0xFFFF) {\n // encode to utf16 (surrogate pair dance)\n codePoint -= 0x10000\n res.push(codePoint >>> 10 & 0x3FF | 0xD800)\n codePoint = 0xDC00 | codePoint & 0x3FF\n }\n\n res.push(codePoint)\n i += bytesPerSequence\n }\n\n return decodeCodePointsArray(res)\n}\n\n// Based on http://stackoverflow.com/a/22747272/680742, the browser with\n// the lowest limit is Chrome, with 0x10000 args.\n// We go 1 magnitude less, for safety\nvar MAX_ARGUMENTS_LENGTH = 0x1000\n\nfunction decodeCodePointsArray (codePoints) {\n var len = codePoints.length\n if (len <= MAX_ARGUMENTS_LENGTH) {\n return String.fromCharCode.apply(String, codePoints) // avoid extra slice()\n }\n\n // Decode in chunks to avoid \"call stack size exceeded\".\n var res = ''\n var i = 0\n while (i < len) {\n res += String.fromCharCode.apply(\n String,\n codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH)\n )\n }\n return res\n}\n\nfunction asciiSlice (buf, start, end) {\n var ret = ''\n end = Math.min(buf.length, end)\n\n for (var i = start; i < end; ++i) {\n ret += String.fromCharCode(buf[i] & 0x7F)\n }\n return ret\n}\n\nfunction latin1Slice (buf, start, end) {\n var ret = ''\n end = Math.min(buf.length, end)\n\n for (var i = start; i < end; ++i) {\n ret += String.fromCharCode(buf[i])\n }\n return ret\n}\n\nfunction hexSlice (buf, start, end) {\n var len = buf.length\n\n if (!start || start < 0) start = 0\n if (!end || end < 0 || end > len) end = len\n\n var out = ''\n for (var i = start; i < end; ++i) {\n out += hexSliceLookupTable[buf[i]]\n }\n return out\n}\n\nfunction utf16leSlice (buf, start, end) {\n var bytes = buf.slice(start, end)\n var res = ''\n // If bytes.length is odd, the last 8 bits must be ignored (same as node.js)\n for (var i = 0; i < bytes.length - 1; i += 2) {\n res += String.fromCharCode(bytes[i] + (bytes[i + 1] * 256))\n }\n return res\n}\n\nBuffer.prototype.slice = function slice (start, end) {\n var len = this.length\n start = ~~start\n end = end === undefined ? len : ~~end\n\n if (start < 0) {\n start += len\n if (start < 0) start = 0\n } else if (start > len) {\n start = len\n }\n\n if (end < 0) {\n end += len\n if (end < 0) end = 0\n } else if (end > len) {\n end = len\n }\n\n if (end < start) end = start\n\n var newBuf = this.subarray(start, end)\n // Return an augmented `Uint8Array` instance\n Object.setPrototypeOf(newBuf, Buffer.prototype)\n\n return newBuf\n}\n\n/*\n * Need to make sure that buffer isn't trying to write out of bounds.\n */\nfunction checkOffset (offset, ext, length) {\n if ((offset % 1) !== 0 || offset < 0) throw new RangeError('offset is not uint')\n if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length')\n}\n\nBuffer.prototype.readUintLE =\nBuffer.prototype.readUIntLE = function readUIntLE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) checkOffset(offset, byteLength, this.length)\n\n var val = this[offset]\n var mul = 1\n var i = 0\n while (++i < byteLength && (mul *= 0x100)) {\n val += this[offset + i] * mul\n }\n\n return val\n}\n\nBuffer.prototype.readUintBE =\nBuffer.prototype.readUIntBE = function readUIntBE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) {\n checkOffset(offset, byteLength, this.length)\n }\n\n var val = this[offset + --byteLength]\n var mul = 1\n while (byteLength > 0 && (mul *= 0x100)) {\n val += this[offset + --byteLength] * mul\n }\n\n return val\n}\n\nBuffer.prototype.readUint8 =\nBuffer.prototype.readUInt8 = function readUInt8 (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 1, this.length)\n return this[offset]\n}\n\nBuffer.prototype.readUint16LE =\nBuffer.prototype.readUInt16LE = function readUInt16LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n return this[offset] | (this[offset + 1] << 8)\n}\n\nBuffer.prototype.readUint16BE =\nBuffer.prototype.readUInt16BE = function readUInt16BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n return (this[offset] << 8) | this[offset + 1]\n}\n\nBuffer.prototype.readUint32LE =\nBuffer.prototype.readUInt32LE = function readUInt32LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return ((this[offset]) |\n (this[offset + 1] << 8) |\n (this[offset + 2] << 16)) +\n (this[offset + 3] * 0x1000000)\n}\n\nBuffer.prototype.readUint32BE =\nBuffer.prototype.readUInt32BE = function readUInt32BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return (this[offset] * 0x1000000) +\n ((this[offset + 1] << 16) |\n (this[offset + 2] << 8) |\n this[offset + 3])\n}\n\nBuffer.prototype.readIntLE = function readIntLE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) checkOffset(offset, byteLength, this.length)\n\n var val = this[offset]\n var mul = 1\n var i = 0\n while (++i < byteLength && (mul *= 0x100)) {\n val += this[offset + i] * mul\n }\n mul *= 0x80\n\n if (val >= mul) val -= Math.pow(2, 8 * byteLength)\n\n return val\n}\n\nBuffer.prototype.readIntBE = function readIntBE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) checkOffset(offset, byteLength, this.length)\n\n var i = byteLength\n var mul = 1\n var val = this[offset + --i]\n while (i > 0 && (mul *= 0x100)) {\n val += this[offset + --i] * mul\n }\n mul *= 0x80\n\n if (val >= mul) val -= Math.pow(2, 8 * byteLength)\n\n return val\n}\n\nBuffer.prototype.readInt8 = function readInt8 (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 1, this.length)\n if (!(this[offset] & 0x80)) return (this[offset])\n return ((0xff - this[offset] + 1) * -1)\n}\n\nBuffer.prototype.readInt16LE = function readInt16LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n var val = this[offset] | (this[offset + 1] << 8)\n return (val & 0x8000) ? val | 0xFFFF0000 : val\n}\n\nBuffer.prototype.readInt16BE = function readInt16BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n var val = this[offset + 1] | (this[offset] << 8)\n return (val & 0x8000) ? val | 0xFFFF0000 : val\n}\n\nBuffer.prototype.readInt32LE = function readInt32LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return (this[offset]) |\n (this[offset + 1] << 8) |\n (this[offset + 2] << 16) |\n (this[offset + 3] << 24)\n}\n\nBuffer.prototype.readInt32BE = function readInt32BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return (this[offset] << 24) |\n (this[offset + 1] << 16) |\n (this[offset + 2] << 8) |\n (this[offset + 3])\n}\n\nBuffer.prototype.readFloatLE = function readFloatLE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n return ieee754.read(this, offset, true, 23, 4)\n}\n\nBuffer.prototype.readFloatBE = function readFloatBE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n return ieee754.read(this, offset, false, 23, 4)\n}\n\nBuffer.prototype.readDoubleLE = function readDoubleLE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 8, this.length)\n return ieee754.read(this, offset, true, 52, 8)\n}\n\nBuffer.prototype.readDoubleBE = function readDoubleBE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 8, this.length)\n return ieee754.read(this, offset, false, 52, 8)\n}\n\nfunction checkInt (buf, value, offset, ext, max, min) {\n if (!Buffer.isBuffer(buf)) throw new TypeError('\"buffer\" argument must be a Buffer instance')\n if (value > max || value < min) throw new RangeError('\"value\" argument is out of bounds')\n if (offset + ext > buf.length) throw new RangeError('Index out of range')\n}\n\nBuffer.prototype.writeUintLE =\nBuffer.prototype.writeUIntLE = function writeUIntLE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) {\n var maxBytes = Math.pow(2, 8 * byteLength) - 1\n checkInt(this, value, offset, byteLength, maxBytes, 0)\n }\n\n var mul = 1\n var i = 0\n this[offset] = value & 0xFF\n while (++i < byteLength && (mul *= 0x100)) {\n this[offset + i] = (value / mul) & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeUintBE =\nBuffer.prototype.writeUIntBE = function writeUIntBE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) {\n var maxBytes = Math.pow(2, 8 * byteLength) - 1\n checkInt(this, value, offset, byteLength, maxBytes, 0)\n }\n\n var i = byteLength - 1\n var mul = 1\n this[offset + i] = value & 0xFF\n while (--i >= 0 && (mul *= 0x100)) {\n this[offset + i] = (value / mul) & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeUint8 =\nBuffer.prototype.writeUInt8 = function writeUInt8 (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0)\n this[offset] = (value & 0xff)\n return offset + 1\n}\n\nBuffer.prototype.writeUint16LE =\nBuffer.prototype.writeUInt16LE = function writeUInt16LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)\n this[offset] = (value & 0xff)\n this[offset + 1] = (value >>> 8)\n return offset + 2\n}\n\nBuffer.prototype.writeUint16BE =\nBuffer.prototype.writeUInt16BE = function writeUInt16BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)\n this[offset] = (value >>> 8)\n this[offset + 1] = (value & 0xff)\n return offset + 2\n}\n\nBuffer.prototype.writeUint32LE =\nBuffer.prototype.writeUInt32LE = function writeUInt32LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)\n this[offset + 3] = (value >>> 24)\n this[offset + 2] = (value >>> 16)\n this[offset + 1] = (value >>> 8)\n this[offset] = (value & 0xff)\n return offset + 4\n}\n\nBuffer.prototype.writeUint32BE =\nBuffer.prototype.writeUInt32BE = function writeUInt32BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)\n this[offset] = (value >>> 24)\n this[offset + 1] = (value >>> 16)\n this[offset + 2] = (value >>> 8)\n this[offset + 3] = (value & 0xff)\n return offset + 4\n}\n\nBuffer.prototype.writeIntLE = function writeIntLE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n var limit = Math.pow(2, (8 * byteLength) - 1)\n\n checkInt(this, value, offset, byteLength, limit - 1, -limit)\n }\n\n var i = 0\n var mul = 1\n var sub = 0\n this[offset] = value & 0xFF\n while (++i < byteLength && (mul *= 0x100)) {\n if (value < 0 && sub === 0 && this[offset + i - 1] !== 0) {\n sub = 1\n }\n this[offset + i] = ((value / mul) >> 0) - sub & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeIntBE = function writeIntBE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n var limit = Math.pow(2, (8 * byteLength) - 1)\n\n checkInt(this, value, offset, byteLength, limit - 1, -limit)\n }\n\n var i = byteLength - 1\n var mul = 1\n var sub = 0\n this[offset + i] = value & 0xFF\n while (--i >= 0 && (mul *= 0x100)) {\n if (value < 0 && sub === 0 && this[offset + i + 1] !== 0) {\n sub = 1\n }\n this[offset + i] = ((value / mul) >> 0) - sub & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeInt8 = function writeInt8 (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80)\n if (value < 0) value = 0xff + value + 1\n this[offset] = (value & 0xff)\n return offset + 1\n}\n\nBuffer.prototype.writeInt16LE = function writeInt16LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)\n this[offset] = (value & 0xff)\n this[offset + 1] = (value >>> 8)\n return offset + 2\n}\n\nBuffer.prototype.writeInt16BE = function writeInt16BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)\n this[offset] = (value >>> 8)\n this[offset + 1] = (value & 0xff)\n return offset + 2\n}\n\nBuffer.prototype.writeInt32LE = function writeInt32LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)\n this[offset] = (value & 0xff)\n this[offset + 1] = (value >>> 8)\n this[offset + 2] = (value >>> 16)\n this[offset + 3] = (value >>> 24)\n return offset + 4\n}\n\nBuffer.prototype.writeInt32BE = function writeInt32BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)\n if (value < 0) value = 0xffffffff + value + 1\n this[offset] = (value >>> 24)\n this[offset + 1] = (value >>> 16)\n this[offset + 2] = (value >>> 8)\n this[offset + 3] = (value & 0xff)\n return offset + 4\n}\n\nfunction checkIEEE754 (buf, value, offset, ext, max, min) {\n if (offset + ext > buf.length) throw new RangeError('Index out of range')\n if (offset < 0) throw new RangeError('Index out of range')\n}\n\nfunction writeFloat (buf, value, offset, littleEndian, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n checkIEEE754(buf, value, offset, 4, 3.4028234663852886e+38, -3.4028234663852886e+38)\n }\n ieee754.write(buf, value, offset, littleEndian, 23, 4)\n return offset + 4\n}\n\nBuffer.prototype.writeFloatLE = function writeFloatLE (value, offset, noAssert) {\n return writeFloat(this, value, offset, true, noAssert)\n}\n\nBuffer.prototype.writeFloatBE = function writeFloatBE (value, offset, noAssert) {\n return writeFloat(this, value, offset, false, noAssert)\n}\n\nfunction writeDouble (buf, value, offset, littleEndian, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n checkIEEE754(buf, value, offset, 8, 1.7976931348623157E+308, -1.7976931348623157E+308)\n }\n ieee754.write(buf, value, offset, littleEndian, 52, 8)\n return offset + 8\n}\n\nBuffer.prototype.writeDoubleLE = function writeDoubleLE (value, offset, noAssert) {\n return writeDouble(this, value, offset, true, noAssert)\n}\n\nBuffer.prototype.writeDoubleBE = function writeDoubleBE (value, offset, noAssert) {\n return writeDouble(this, value, offset, false, noAssert)\n}\n\n// copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)\nBuffer.prototype.copy = function copy (target, targetStart, start, end) {\n if (!Buffer.isBuffer(target)) throw new TypeError('argument should be a Buffer')\n if (!start) start = 0\n if (!end && end !== 0) end = this.length\n if (targetStart >= target.length) targetStart = target.length\n if (!targetStart) targetStart = 0\n if (end > 0 && end < start) end = start\n\n // Copy 0 bytes; we're done\n if (end === start) return 0\n if (target.length === 0 || this.length === 0) return 0\n\n // Fatal error conditions\n if (targetStart < 0) {\n throw new RangeError('targetStart out of bounds')\n }\n if (start < 0 || start >= this.length) throw new RangeError('Index out of range')\n if (end < 0) throw new RangeError('sourceEnd out of bounds')\n\n // Are we oob?\n if (end > this.length) end = this.length\n if (target.length - targetStart < end - start) {\n end = target.length - targetStart + start\n }\n\n var len = end - start\n\n if (this === target && typeof Uint8Array.prototype.copyWithin === 'function') {\n // Use built-in when available, missing from IE11\n this.copyWithin(targetStart, start, end)\n } else {\n Uint8Array.prototype.set.call(\n target,\n this.subarray(start, end),\n targetStart\n )\n }\n\n return len\n}\n\n// Usage:\n// buffer.fill(number[, offset[, end]])\n// buffer.fill(buffer[, offset[, end]])\n// buffer.fill(string[, offset[, end]][, encoding])\nBuffer.prototype.fill = function fill (val, start, end, encoding) {\n // Handle string cases:\n if (typeof val === 'string') {\n if (typeof start === 'string') {\n encoding = start\n start = 0\n end = this.length\n } else if (typeof end === 'string') {\n encoding = end\n end = this.length\n }\n if (encoding !== undefined && typeof encoding !== 'string') {\n throw new TypeError('encoding must be a string')\n }\n if (typeof encoding === 'string' && !Buffer.isEncoding(encoding)) {\n throw new TypeError('Unknown encoding: ' + encoding)\n }\n if (val.length === 1) {\n var code = val.charCodeAt(0)\n if ((encoding === 'utf8' && code < 128) ||\n encoding === 'latin1') {\n // Fast path: If `val` fits into a single byte, use that numeric value.\n val = code\n }\n }\n } else if (typeof val === 'number') {\n val = val & 255\n } else if (typeof val === 'boolean') {\n val = Number(val)\n }\n\n // Invalid ranges are not set to a default, so can range check early.\n if (start < 0 || this.length < start || this.length < end) {\n throw new RangeError('Out of range index')\n }\n\n if (end <= start) {\n return this\n }\n\n start = start >>> 0\n end = end === undefined ? this.length : end >>> 0\n\n if (!val) val = 0\n\n var i\n if (typeof val === 'number') {\n for (i = start; i < end; ++i) {\n this[i] = val\n }\n } else {\n var bytes = Buffer.isBuffer(val)\n ? val\n : Buffer.from(val, encoding)\n var len = bytes.length\n if (len === 0) {\n throw new TypeError('The value \"' + val +\n '\" is invalid for argument \"value\"')\n }\n for (i = 0; i < end - start; ++i) {\n this[i + start] = bytes[i % len]\n }\n }\n\n return this\n}\n\n// HELPER FUNCTIONS\n// ================\n\nvar INVALID_BASE64_RE = /[^+/0-9A-Za-z-_]/g\n\nfunction base64clean (str) {\n // Node takes equal signs as end of the Base64 encoding\n str = str.split('=')[0]\n // Node strips out invalid characters like \\n and \\t from the string, base64-js does not\n str = str.trim().replace(INVALID_BASE64_RE, '')\n // Node converts strings with length < 2 to ''\n if (str.length < 2) return ''\n // Node allows for non-padded base64 strings (missing trailing ===), base64-js does not\n while (str.length % 4 !== 0) {\n str = str + '='\n }\n return str\n}\n\nfunction utf8ToBytes (string, units) {\n units = units || Infinity\n var codePoint\n var length = string.length\n var leadSurrogate = null\n var bytes = []\n\n for (var i = 0; i < length; ++i) {\n codePoint = string.charCodeAt(i)\n\n // is surrogate component\n if (codePoint > 0xD7FF && codePoint < 0xE000) {\n // last char was a lead\n if (!leadSurrogate) {\n // no lead yet\n if (codePoint > 0xDBFF) {\n // unexpected trail\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n continue\n } else if (i + 1 === length) {\n // unpaired lead\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n continue\n }\n\n // valid lead\n leadSurrogate = codePoint\n\n continue\n }\n\n // 2 leads in a row\n if (codePoint < 0xDC00) {\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n leadSurrogate = codePoint\n continue\n }\n\n // valid surrogate pair\n codePoint = (leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00) + 0x10000\n } else if (leadSurrogate) {\n // valid bmp char, but last char was a lead\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n }\n\n leadSurrogate = null\n\n // encode utf8\n if (codePoint < 0x80) {\n if ((units -= 1) < 0) break\n bytes.push(codePoint)\n } else if (codePoint < 0x800) {\n if ((units -= 2) < 0) break\n bytes.push(\n codePoint >> 0x6 | 0xC0,\n codePoint & 0x3F | 0x80\n )\n } else if (codePoint < 0x10000) {\n if ((units -= 3) < 0) break\n bytes.push(\n codePoint >> 0xC | 0xE0,\n codePoint >> 0x6 & 0x3F | 0x80,\n codePoint & 0x3F | 0x80\n )\n } else if (codePoint < 0x110000) {\n if ((units -= 4) < 0) break\n bytes.push(\n codePoint >> 0x12 | 0xF0,\n codePoint >> 0xC & 0x3F | 0x80,\n codePoint >> 0x6 & 0x3F | 0x80,\n codePoint & 0x3F | 0x80\n )\n } else {\n throw new Error('Invalid code point')\n }\n }\n\n return bytes\n}\n\nfunction asciiToBytes (str) {\n var byteArray = []\n for (var i = 0; i < str.length; ++i) {\n // Node's code seems to be doing this and not & 0x7F..\n byteArray.push(str.charCodeAt(i) & 0xFF)\n }\n return byteArray\n}\n\nfunction utf16leToBytes (str, units) {\n var c, hi, lo\n var byteArray = []\n for (var i = 0; i < str.length; ++i) {\n if ((units -= 2) < 0) break\n\n c = str.charCodeAt(i)\n hi = c >> 8\n lo = c % 256\n byteArray.push(lo)\n byteArray.push(hi)\n }\n\n return byteArray\n}\n\nfunction base64ToBytes (str) {\n return base64.toByteArray(base64clean(str))\n}\n\nfunction blitBuffer (src, dst, offset, length) {\n for (var i = 0; i < length; ++i) {\n if ((i + offset >= dst.length) || (i >= src.length)) break\n dst[i + offset] = src[i]\n }\n return i\n}\n\n// ArrayBuffer or Uint8Array objects from other contexts (i.e. iframes) do not pass\n// the `instanceof` check but they should be treated as of that type.\n// See: https://github.com/feross/buffer/issues/166\nfunction isInstance (obj, type) {\n return obj instanceof type ||\n (obj != null && obj.constructor != null && obj.constructor.name != null &&\n obj.constructor.name === type.name)\n}\nfunction numberIsNaN (obj) {\n // For IE11 support\n return obj !== obj // eslint-disable-line no-self-compare\n}\n\n// Create lookup table for `toString('hex')`\n// See: https://github.com/feross/buffer/issues/219\nvar hexSliceLookupTable = (function () {\n var alphabet = '0123456789abcdef'\n var table = new Array(256)\n for (var i = 0; i < 16; ++i) {\n var i16 = i * 16\n for (var j = 0; j < 16; ++j) {\n table[i16 + j] = alphabet[i] + alphabet[j]\n }\n }\n return table\n})()\n","export default {};\n","// shim for using process in browser\n// based off https://github.com/defunctzombie/node-process/blob/master/browser.js\n\nfunction defaultSetTimout() {\n throw new Error('setTimeout has not been defined');\n}\nfunction defaultClearTimeout () {\n throw new Error('clearTimeout has not been defined');\n}\nvar cachedSetTimeout = defaultSetTimout;\nvar cachedClearTimeout = defaultClearTimeout;\nif (typeof global.setTimeout === 'function') {\n cachedSetTimeout = setTimeout;\n}\nif (typeof global.clearTimeout === 'function') {\n cachedClearTimeout = clearTimeout;\n}\n\nfunction runTimeout(fun) {\n if (cachedSetTimeout === setTimeout) {\n //normal enviroments in sane situations\n return setTimeout(fun, 0);\n }\n // if setTimeout wasn't available but was latter defined\n if ((cachedSetTimeout === defaultSetTimout || !cachedSetTimeout) && setTimeout) {\n cachedSetTimeout = setTimeout;\n return setTimeout(fun, 0);\n }\n try {\n // when when somebody has screwed with setTimeout but no I.E. maddness\n return cachedSetTimeout(fun, 0);\n } catch(e){\n try {\n // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally\n return cachedSetTimeout.call(null, fun, 0);\n } catch(e){\n // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error\n return cachedSetTimeout.call(this, fun, 0);\n }\n }\n\n\n}\nfunction runClearTimeout(marker) {\n if (cachedClearTimeout === clearTimeout) {\n //normal enviroments in sane situations\n return clearTimeout(marker);\n }\n // if clearTimeout wasn't available but was latter defined\n if ((cachedClearTimeout === defaultClearTimeout || !cachedClearTimeout) && clearTimeout) {\n cachedClearTimeout = clearTimeout;\n return clearTimeout(marker);\n }\n try {\n // when when somebody has screwed with setTimeout but no I.E. maddness\n return cachedClearTimeout(marker);\n } catch (e){\n try {\n // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally\n return cachedClearTimeout.call(null, marker);\n } catch (e){\n // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error.\n // Some versions of I.E. have different rules for clearTimeout vs setTimeout\n return cachedClearTimeout.call(this, marker);\n }\n }\n\n\n\n}\nvar queue = [];\nvar draining = false;\nvar currentQueue;\nvar queueIndex = -1;\n\nfunction cleanUpNextTick() {\n if (!draining || !currentQueue) {\n return;\n }\n draining = false;\n if (currentQueue.length) {\n queue = currentQueue.concat(queue);\n } else {\n queueIndex = -1;\n }\n if (queue.length) {\n drainQueue();\n }\n}\n\nfunction drainQueue() {\n if (draining) {\n return;\n }\n var timeout = runTimeout(cleanUpNextTick);\n draining = true;\n\n var len = queue.length;\n while(len) {\n currentQueue = queue;\n queue = [];\n while (++queueIndex < len) {\n if (currentQueue) {\n currentQueue[queueIndex].run();\n }\n }\n queueIndex = -1;\n len = queue.length;\n }\n currentQueue = null;\n draining = false;\n runClearTimeout(timeout);\n}\nexport function nextTick(fun) {\n var args = new Array(arguments.length - 1);\n if (arguments.length > 1) {\n for (var i = 1; i < arguments.length; i++) {\n args[i - 1] = arguments[i];\n }\n }\n queue.push(new Item(fun, args));\n if (queue.length === 1 && !draining) {\n runTimeout(drainQueue);\n }\n}\n// v8 likes predictible objects\nfunction Item(fun, array) {\n this.fun = fun;\n this.array = array;\n}\nItem.prototype.run = function () {\n this.fun.apply(null, this.array);\n};\nexport var title = 'browser';\nexport var platform = 'browser';\nexport var browser = true;\nexport var env = {};\nexport var argv = [];\nexport var version = ''; // empty string to avoid regexp issues\nexport var versions = {};\nexport var release = {};\nexport var config = {};\n\nfunction noop() {}\n\nexport var on = noop;\nexport var addListener = noop;\nexport var once = noop;\nexport var off = noop;\nexport var removeListener = noop;\nexport var removeAllListeners = noop;\nexport var emit = noop;\n\nexport function binding(name) {\n throw new Error('process.binding is not supported');\n}\n\nexport function cwd () { return '/' }\nexport function chdir (dir) {\n throw new Error('process.chdir is not supported');\n};\nexport function umask() { return 0; }\n\n// from https://github.com/kumavis/browser-process-hrtime/blob/master/index.js\nvar performance = global.performance || {}\nvar performanceNow =\n performance.now ||\n performance.mozNow ||\n performance.msNow ||\n performance.oNow ||\n performance.webkitNow ||\n function(){ return (new Date()).getTime() }\n\n// generate timestamp or delta\n// see http://nodejs.org/api/process.html#process_process_hrtime\nexport function hrtime(previousTimestamp){\n var clocktime = performanceNow.call(performance)*1e-3\n var seconds = Math.floor(clocktime)\n var nanoseconds = Math.floor((clocktime%1)*1e9)\n if (previousTimestamp) {\n seconds = seconds - previousTimestamp[0]\n nanoseconds = nanoseconds - previousTimestamp[1]\n if (nanoseconds<0) {\n seconds--\n nanoseconds += 1e9\n }\n }\n return [seconds,nanoseconds]\n}\n\nvar startTime = new Date();\nexport function uptime() {\n var currentTime = new Date();\n var dif = currentTime - startTime;\n return dif / 1000;\n}\n\nexport default {\n nextTick: nextTick,\n title: title,\n browser: browser,\n env: env,\n argv: argv,\n version: version,\n versions: versions,\n on: on,\n addListener: addListener,\n once: once,\n off: off,\n removeListener: removeListener,\n removeAllListeners: removeAllListeners,\n emit: emit,\n binding: binding,\n cwd: cwd,\n chdir: chdir,\n umask: umask,\n hrtime: hrtime,\n platform: platform,\n release: release,\n config: config,\n uptime: uptime\n};\n","\nvar inherits;\nif (typeof Object.create === 'function'){\n inherits = function inherits(ctor, superCtor) {\n // implementation from standard node.js 'util' module\n ctor.super_ = superCtor\n ctor.prototype = Object.create(superCtor.prototype, {\n constructor: {\n value: ctor,\n enumerable: false,\n writable: true,\n configurable: true\n }\n });\n };\n} else {\n inherits = function inherits(ctor, superCtor) {\n ctor.super_ = superCtor\n var TempCtor = function () {}\n TempCtor.prototype = superCtor.prototype\n ctor.prototype = new TempCtor()\n ctor.prototype.constructor = ctor\n }\n}\nexport default inherits;\n","// Copyright Joyent, Inc. and other Node contributors.\n//\n// Permission is hereby granted, free of charge, to any person obtaining a\n// copy of this software and associated documentation files (the\n// \"Software\"), to deal in the Software without restriction, including\n// without limitation the rights to use, copy, modify, merge, publish,\n// distribute, sublicense, and/or sell copies of the Software, and to permit\n// persons to whom the Software is furnished to do so, subject to the\n// following conditions:\n//\n// The above copyright notice and this permission notice shall be included\n// in all copies or substantial portions of the Software.\n//\n// THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS\n// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF\n// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN\n// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,\n// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR\n// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE\n// USE OR OTHER DEALINGS IN THE SOFTWARE.\nimport process from 'process';\nvar formatRegExp = /%[sdj%]/g;\nexport function format(f) {\n if (!isString(f)) {\n var objects = [];\n for (var i = 0; i < arguments.length; i++) {\n objects.push(inspect(arguments[i]));\n }\n return objects.join(' ');\n }\n\n var i = 1;\n var args = arguments;\n var len = args.length;\n var str = String(f).replace(formatRegExp, function(x) {\n if (x === '%%') return '%';\n if (i >= len) return x;\n switch (x) {\n case '%s': return String(args[i++]);\n case '%d': return Number(args[i++]);\n case '%j':\n try {\n return JSON.stringify(args[i++]);\n } catch (_) {\n return '[Circular]';\n }\n default:\n return x;\n }\n });\n for (var x = args[i]; i < len; x = args[++i]) {\n if (isNull(x) || !isObject(x)) {\n str += ' ' + x;\n } else {\n str += ' ' + inspect(x);\n }\n }\n return str;\n};\n\n\n// Mark that a method should not be used.\n// Returns a modified function which warns once by default.\n// If --no-deprecation is set, then it is a no-op.\nexport function deprecate(fn, msg) {\n // Allow for deprecating things in the process of starting up.\n if (isUndefined(global.process)) {\n return function() {\n return deprecate(fn, msg).apply(this, arguments);\n };\n }\n\n if (process.noDeprecation === true) {\n return fn;\n }\n\n var warned = false;\n function deprecated() {\n if (!warned) {\n if (process.throwDeprecation) {\n throw new Error(msg);\n } else if (process.traceDeprecation) {\n console.trace(msg);\n } else {\n console.error(msg);\n }\n warned = true;\n }\n return fn.apply(this, arguments);\n }\n\n return deprecated;\n};\n\n\nvar debugs = {};\nvar debugEnviron;\nexport function debuglog(set) {\n if (isUndefined(debugEnviron))\n debugEnviron = process.env.NODE_DEBUG || '';\n set = set.toUpperCase();\n if (!debugs[set]) {\n if (new RegExp('\\\\b' + set + '\\\\b', 'i').test(debugEnviron)) {\n var pid = 0;\n debugs[set] = function() {\n var msg = format.apply(null, arguments);\n console.error('%s %d: %s', set, pid, msg);\n };\n } else {\n debugs[set] = function() {};\n }\n }\n return debugs[set];\n};\n\n\n/**\n * Echos the value of a value. Trys to print the value out\n * in the best way possible given the different types.\n *\n * @param {Object} obj The object to print out.\n * @param {Object} opts Optional options object that alters the output.\n */\n/* legacy: obj, showHidden, depth, colors*/\nexport function inspect(obj, opts) {\n // default options\n var ctx = {\n seen: [],\n stylize: stylizeNoColor\n };\n // legacy...\n if (arguments.length >= 3) ctx.depth = arguments[2];\n if (arguments.length >= 4) ctx.colors = arguments[3];\n if (isBoolean(opts)) {\n // legacy...\n ctx.showHidden = opts;\n } else if (opts) {\n // got an \"options\" object\n _extend(ctx, opts);\n }\n // set default options\n if (isUndefined(ctx.showHidden)) ctx.showHidden = false;\n if (isUndefined(ctx.depth)) ctx.depth = 2;\n if (isUndefined(ctx.colors)) ctx.colors = false;\n if (isUndefined(ctx.customInspect)) ctx.customInspect = true;\n if (ctx.colors) ctx.stylize = stylizeWithColor;\n return formatValue(ctx, obj, ctx.depth);\n}\n\n// http://en.wikipedia.org/wiki/ANSI_escape_code#graphics\ninspect.colors = {\n 'bold' : [1, 22],\n 'italic' : [3, 23],\n 'underline' : [4, 24],\n 'inverse' : [7, 27],\n 'white' : [37, 39],\n 'grey' : [90, 39],\n 'black' : [30, 39],\n 'blue' : [34, 39],\n 'cyan' : [36, 39],\n 'green' : [32, 39],\n 'magenta' : [35, 39],\n 'red' : [31, 39],\n 'yellow' : [33, 39]\n};\n\n// Don't use 'blue' not visible on cmd.exe\ninspect.styles = {\n 'special': 'cyan',\n 'number': 'yellow',\n 'boolean': 'yellow',\n 'undefined': 'grey',\n 'null': 'bold',\n 'string': 'green',\n 'date': 'magenta',\n // \"name\": intentionally not styling\n 'regexp': 'red'\n};\n\n\nfunction stylizeWithColor(str, styleType) {\n var style = inspect.styles[styleType];\n\n if (style) {\n return '\\u001b[' + inspect.colors[style][0] + 'm' + str +\n '\\u001b[' + inspect.colors[style][1] + 'm';\n } else {\n return str;\n }\n}\n\n\nfunction stylizeNoColor(str, styleType) {\n return str;\n}\n\n\nfunction arrayToHash(array) {\n var hash = {};\n\n array.forEach(function(val, idx) {\n hash[val] = true;\n });\n\n return hash;\n}\n\n\nfunction formatValue(ctx, value, recurseTimes) {\n // Provide a hook for user-specified inspect functions.\n // Check that value is an object with an inspect function on it\n if (ctx.customInspect &&\n value &&\n isFunction(value.inspect) &&\n // Filter out the util module, it's inspect function is special\n value.inspect !== inspect &&\n // Also filter out any prototype objects using the circular check.\n !(value.constructor && value.constructor.prototype === value)) {\n var ret = value.inspect(recurseTimes, ctx);\n if (!isString(ret)) {\n ret = formatValue(ctx, ret, recurseTimes);\n }\n return ret;\n }\n\n // Primitive types cannot have properties\n var primitive = formatPrimitive(ctx, value);\n if (primitive) {\n return primitive;\n }\n\n // Look up the keys of the object.\n var keys = Object.keys(value);\n var visibleKeys = arrayToHash(keys);\n\n if (ctx.showHidden) {\n keys = Object.getOwnPropertyNames(value);\n }\n\n // IE doesn't make error fields non-enumerable\n // http://msdn.microsoft.com/en-us/library/ie/dww52sbt(v=vs.94).aspx\n if (isError(value)\n && (keys.indexOf('message') >= 0 || keys.indexOf('description') >= 0)) {\n return formatError(value);\n }\n\n // Some type of object without properties can be shortcutted.\n if (keys.length === 0) {\n if (isFunction(value)) {\n var name = value.name ? ': ' + value.name : '';\n return ctx.stylize('[Function' + name + ']', 'special');\n }\n if (isRegExp(value)) {\n return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');\n }\n if (isDate(value)) {\n return ctx.stylize(Date.prototype.toString.call(value), 'date');\n }\n if (isError(value)) {\n return formatError(value);\n }\n }\n\n var base = '', array = false, braces = ['{', '}'];\n\n // Make Array say that they are Array\n if (isArray(value)) {\n array = true;\n braces = ['[', ']'];\n }\n\n // Make functions say that they are functions\n if (isFunction(value)) {\n var n = value.name ? ': ' + value.name : '';\n base = ' [Function' + n + ']';\n }\n\n // Make RegExps say that they are RegExps\n if (isRegExp(value)) {\n base = ' ' + RegExp.prototype.toString.call(value);\n }\n\n // Make dates with properties first say the date\n if (isDate(value)) {\n base = ' ' + Date.prototype.toUTCString.call(value);\n }\n\n // Make error with message first say the error\n if (isError(value)) {\n base = ' ' + formatError(value);\n }\n\n if (keys.length === 0 && (!array || value.length == 0)) {\n return braces[0] + base + braces[1];\n }\n\n if (recurseTimes < 0) {\n if (isRegExp(value)) {\n return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');\n } else {\n return ctx.stylize('[Object]', 'special');\n }\n }\n\n ctx.seen.push(value);\n\n var output;\n if (array) {\n output = formatArray(ctx, value, recurseTimes, visibleKeys, keys);\n } else {\n output = keys.map(function(key) {\n return formatProperty(ctx, value, recurseTimes, visibleKeys, key, array);\n });\n }\n\n ctx.seen.pop();\n\n return reduceToSingleString(output, base, braces);\n}\n\n\nfunction formatPrimitive(ctx, value) {\n if (isUndefined(value))\n return ctx.stylize('undefined', 'undefined');\n if (isString(value)) {\n var simple = '\\'' + JSON.stringify(value).replace(/^\"|\"$/g, '')\n .replace(/'/g, \"\\\\'\")\n .replace(/\\\\\"/g, '\"') + '\\'';\n return ctx.stylize(simple, 'string');\n }\n if (isNumber(value))\n return ctx.stylize('' + value, 'number');\n if (isBoolean(value))\n return ctx.stylize('' + value, 'boolean');\n // For some reason typeof null is \"object\", so special case here.\n if (isNull(value))\n return ctx.stylize('null', 'null');\n}\n\n\nfunction formatError(value) {\n return '[' + Error.prototype.toString.call(value) + ']';\n}\n\n\nfunction formatArray(ctx, value, recurseTimes, visibleKeys, keys) {\n var output = [];\n for (var i = 0, l = value.length; i < l; ++i) {\n if (hasOwnProperty(value, String(i))) {\n output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,\n String(i), true));\n } else {\n output.push('');\n }\n }\n keys.forEach(function(key) {\n if (!key.match(/^\\d+$/)) {\n output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,\n key, true));\n }\n });\n return output;\n}\n\n\nfunction formatProperty(ctx, value, recurseTimes, visibleKeys, key, array) {\n var name, str, desc;\n desc = Object.getOwnPropertyDescriptor(value, key) || { value: value[key] };\n if (desc.get) {\n if (desc.set) {\n str = ctx.stylize('[Getter/Setter]', 'special');\n } else {\n str = ctx.stylize('[Getter]', 'special');\n }\n } else {\n if (desc.set) {\n str = ctx.stylize('[Setter]', 'special');\n }\n }\n if (!hasOwnProperty(visibleKeys, key)) {\n name = '[' + key + ']';\n }\n if (!str) {\n if (ctx.seen.indexOf(desc.value) < 0) {\n if (isNull(recurseTimes)) {\n str = formatValue(ctx, desc.value, null);\n } else {\n str = formatValue(ctx, desc.value, recurseTimes - 1);\n }\n if (str.indexOf('\\n') > -1) {\n if (array) {\n str = str.split('\\n').map(function(line) {\n return ' ' + line;\n }).join('\\n').substr(2);\n } else {\n str = '\\n' + str.split('\\n').map(function(line) {\n return ' ' + line;\n }).join('\\n');\n }\n }\n } else {\n str = ctx.stylize('[Circular]', 'special');\n }\n }\n if (isUndefined(name)) {\n if (array && key.match(/^\\d+$/)) {\n return str;\n }\n name = JSON.stringify('' + key);\n if (name.match(/^\"([a-zA-Z_][a-zA-Z_0-9]*)\"$/)) {\n name = name.substr(1, name.length - 2);\n name = ctx.stylize(name, 'name');\n } else {\n name = name.replace(/'/g, \"\\\\'\")\n .replace(/\\\\\"/g, '\"')\n .replace(/(^\"|\"$)/g, \"'\");\n name = ctx.stylize(name, 'string');\n }\n }\n\n return name + ': ' + str;\n}\n\n\nfunction reduceToSingleString(output, base, braces) {\n var numLinesEst = 0;\n var length = output.reduce(function(prev, cur) {\n numLinesEst++;\n if (cur.indexOf('\\n') >= 0) numLinesEst++;\n return prev + cur.replace(/\\u001b\\[\\d\\d?m/g, '').length + 1;\n }, 0);\n\n if (length > 60) {\n return braces[0] +\n (base === '' ? '' : base + '\\n ') +\n ' ' +\n output.join(',\\n ') +\n ' ' +\n braces[1];\n }\n\n return braces[0] + base + ' ' + output.join(', ') + ' ' + braces[1];\n}\n\n\n// NOTE: These type checking functions intentionally don't use `instanceof`\n// because it is fragile and can be easily faked with `Object.create()`.\nexport function isArray(ar) {\n return Array.isArray(ar);\n}\n\nexport function isBoolean(arg) {\n return typeof arg === 'boolean';\n}\n\nexport function isNull(arg) {\n return arg === null;\n}\n\nexport function isNullOrUndefined(arg) {\n return arg == null;\n}\n\nexport function isNumber(arg) {\n return typeof arg === 'number';\n}\n\nexport function isString(arg) {\n return typeof arg === 'string';\n}\n\nexport function isSymbol(arg) {\n return typeof arg === 'symbol';\n}\n\nexport function isUndefined(arg) {\n return arg === void 0;\n}\n\nexport function isRegExp(re) {\n return isObject(re) && objectToString(re) === '[object RegExp]';\n}\n\nexport function isObject(arg) {\n return typeof arg === 'object' && arg !== null;\n}\n\nexport function isDate(d) {\n return isObject(d) && objectToString(d) === '[object Date]';\n}\n\nexport function isError(e) {\n return isObject(e) &&\n (objectToString(e) === '[object Error]' || e instanceof Error);\n}\n\nexport function isFunction(arg) {\n return typeof arg === 'function';\n}\n\nexport function isPrimitive(arg) {\n return arg === null ||\n typeof arg === 'boolean' ||\n typeof arg === 'number' ||\n typeof arg === 'string' ||\n typeof arg === 'symbol' || // ES6 symbol\n typeof arg === 'undefined';\n}\n\nexport function isBuffer(maybeBuf) {\n return Buffer.isBuffer(maybeBuf);\n}\n\nfunction objectToString(o) {\n return Object.prototype.toString.call(o);\n}\n\n\nfunction pad(n) {\n return n < 10 ? '0' + n.toString(10) : n.toString(10);\n}\n\n\nvar months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep',\n 'Oct', 'Nov', 'Dec'];\n\n// 26 Feb 16:19:34\nfunction timestamp() {\n var d = new Date();\n var time = [pad(d.getHours()),\n pad(d.getMinutes()),\n pad(d.getSeconds())].join(':');\n return [d.getDate(), months[d.getMonth()], time].join(' ');\n}\n\n\n// log is just a thin wrapper to console.log that prepends a timestamp\nexport function log() {\n console.log('%s - %s', timestamp(), format.apply(null, arguments));\n}\n\n\n/**\n * Inherit the prototype methods from one constructor into another.\n *\n * The Function.prototype.inherits from lang.js rewritten as a standalone\n * function (not on Function.prototype). NOTE: If this file is to be loaded\n * during bootstrapping this function needs to be rewritten using some native\n * functions as prototype setup using normal JavaScript does not work as\n * expected during bootstrapping (see mirror.js in r114903).\n *\n * @param {function} ctor Constructor function which needs to inherit the\n * prototype.\n * @param {function} superCtor Constructor function to inherit prototype from.\n */\nimport inherits from './inherits';\nexport {inherits}\n\nexport function _extend(origin, add) {\n // Don't do anything if add isn't an object\n if (!add || !isObject(add)) return origin;\n\n var keys = Object.keys(add);\n var i = keys.length;\n while (i--) {\n origin[keys[i]] = add[keys[i]];\n }\n return origin;\n};\n\nfunction hasOwnProperty(obj, prop) {\n return Object.prototype.hasOwnProperty.call(obj, prop);\n}\n\nexport default {\n inherits: inherits,\n _extend: _extend,\n log: log,\n isBuffer: isBuffer,\n isPrimitive: isPrimitive,\n isFunction: isFunction,\n isError: isError,\n isDate: isDate,\n isObject: isObject,\n isRegExp: isRegExp,\n isUndefined: isUndefined,\n isSymbol: isSymbol,\n isString: isString,\n isNumber: isNumber,\n isNullOrUndefined: isNullOrUndefined,\n isNull: isNull,\n isBoolean: isBoolean,\n isArray: isArray,\n inspect: inspect,\n deprecate: deprecate,\n format: format,\n debuglog: debuglog\n}\n",null,null,null,null,null,null,null,null,null,null,null,null,null,null,null,null,"/*! *****************************************************************************\r\nCopyright (c) Microsoft Corporation.\r\n\r\nPermission to use, copy, modify, and/or distribute this software for any\r\npurpose with or without fee is hereby granted.\r\n\r\nTHE SOFTWARE IS PROVIDED \"AS IS\" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH\r\nREGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY\r\nAND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,\r\nINDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM\r\nLOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR\r\nOTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR\r\nPERFORMANCE OF THIS SOFTWARE.\r\n***************************************************************************** */\r\n/* global Reflect, Promise */\r\n\r\nvar extendStatics = function(d, b) {\r\n extendStatics = Object.setPrototypeOf ||\r\n ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||\r\n function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; };\r\n return extendStatics(d, b);\r\n};\r\n\r\nexport function __extends(d, b) {\r\n extendStatics(d, b);\r\n function __() { this.constructor = d; }\r\n d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());\r\n}\r\n\r\nexport var __assign = function() {\r\n __assign = Object.assign || function __assign(t) {\r\n for (var s, i = 1, n = arguments.length; i < n; i++) {\r\n s = arguments[i];\r\n for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p];\r\n }\r\n return t;\r\n }\r\n return __assign.apply(this, arguments);\r\n}\r\n\r\nexport function __rest(s, e) {\r\n var t = {};\r\n for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p) && e.indexOf(p) < 0)\r\n t[p] = s[p];\r\n if (s != null && typeof Object.getOwnPropertySymbols === \"function\")\r\n for (var i = 0, p = Object.getOwnPropertySymbols(s); i < p.length; i++) {\r\n if (e.indexOf(p[i]) < 0 && Object.prototype.propertyIsEnumerable.call(s, p[i]))\r\n t[p[i]] = s[p[i]];\r\n }\r\n return t;\r\n}\r\n\r\nexport function __decorate(decorators, target, key, desc) {\r\n var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;\r\n if (typeof Reflect === \"object\" && typeof Reflect.decorate === \"function\") r = Reflect.decorate(decorators, target, key, desc);\r\n else for (var i = decorators.length - 1; i >= 0; i--) if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;\r\n return c > 3 && r && Object.defineProperty(target, key, r), r;\r\n}\r\n\r\nexport function __param(paramIndex, decorator) {\r\n return function (target, key) { decorator(target, key, paramIndex); }\r\n}\r\n\r\nexport function __metadata(metadataKey, metadataValue) {\r\n if (typeof Reflect === \"object\" && typeof Reflect.metadata === \"function\") return Reflect.metadata(metadataKey, metadataValue);\r\n}\r\n\r\nexport function __awaiter(thisArg, _arguments, P, generator) {\r\n function adopt(value) { return value instanceof P ? value : new P(function (resolve) { resolve(value); }); }\r\n return new (P || (P = Promise))(function (resolve, reject) {\r\n function fulfilled(value) { try { step(generator.next(value)); } catch (e) { reject(e); } }\r\n function rejected(value) { try { step(generator[\"throw\"](value)); } catch (e) { reject(e); } }\r\n function step(result) { result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected); }\r\n step((generator = generator.apply(thisArg, _arguments || [])).next());\r\n });\r\n}\r\n\r\nexport function __generator(thisArg, body) {\r\n var _ = { label: 0, sent: function() { if (t[0] & 1) throw t[1]; return t[1]; }, trys: [], ops: [] }, f, y, t, g;\r\n return g = { next: verb(0), \"throw\": verb(1), \"return\": verb(2) }, typeof Symbol === \"function\" && (g[Symbol.iterator] = function() { return this; }), g;\r\n function verb(n) { return function (v) { return step([n, v]); }; }\r\n function step(op) {\r\n if (f) throw new TypeError(\"Generator is already executing.\");\r\n while (_) try {\r\n if (f = 1, y && (t = op[0] & 2 ? y[\"return\"] : op[0] ? y[\"throw\"] || ((t = y[\"return\"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done) return t;\r\n if (y = 0, t) op = [op[0] & 2, t.value];\r\n switch (op[0]) {\r\n case 0: case 1: t = op; break;\r\n case 4: _.label++; return { value: op[1], done: false };\r\n case 5: _.label++; y = op[1]; op = [0]; continue;\r\n case 7: op = _.ops.pop(); _.trys.pop(); continue;\r\n default:\r\n if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) { _ = 0; continue; }\r\n if (op[0] === 3 && (!t || (op[1] > t[0] && op[1] < t[3]))) { _.label = op[1]; break; }\r\n if (op[0] === 6 && _.label < t[1]) { _.label = t[1]; t = op; break; }\r\n if (t && _.label < t[2]) { _.label = t[2]; _.ops.push(op); break; }\r\n if (t[2]) _.ops.pop();\r\n _.trys.pop(); continue;\r\n }\r\n op = body.call(thisArg, _);\r\n } catch (e) { op = [6, e]; y = 0; } finally { f = t = 0; }\r\n if (op[0] & 5) throw op[1]; return { value: op[0] ? op[1] : void 0, done: true };\r\n }\r\n}\r\n\r\nexport function __createBinding(o, m, k, k2) {\r\n if (k2 === undefined) k2 = k;\r\n o[k2] = m[k];\r\n}\r\n\r\nexport function __exportStar(m, exports) {\r\n for (var p in m) if (p !== \"default\" && !exports.hasOwnProperty(p)) exports[p] = m[p];\r\n}\r\n\r\nexport function __values(o) {\r\n var s = typeof Symbol === \"function\" && Symbol.iterator, m = s && o[s], i = 0;\r\n if (m) return m.call(o);\r\n if (o && typeof o.length === \"number\") return {\r\n next: function () {\r\n if (o && i >= o.length) o = void 0;\r\n return { value: o && o[i++], done: !o };\r\n }\r\n };\r\n throw new TypeError(s ? \"Object is not iterable.\" : \"Symbol.iterator is not defined.\");\r\n}\r\n\r\nexport function __read(o, n) {\r\n var m = typeof Symbol === \"function\" && o[Symbol.iterator];\r\n if (!m) return o;\r\n var i = m.call(o), r, ar = [], e;\r\n try {\r\n while ((n === void 0 || n-- > 0) && !(r = i.next()).done) ar.push(r.value);\r\n }\r\n catch (error) { e = { error: error }; }\r\n finally {\r\n try {\r\n if (r && !r.done && (m = i[\"return\"])) m.call(i);\r\n }\r\n finally { if (e) throw e.error; }\r\n }\r\n return ar;\r\n}\r\n\r\nexport function __spread() {\r\n for (var ar = [], i = 0; i < arguments.length; i++)\r\n ar = ar.concat(__read(arguments[i]));\r\n return ar;\r\n}\r\n\r\nexport function __spreadArrays() {\r\n for (var s = 0, i = 0, il = arguments.length; i < il; i++) s += arguments[i].length;\r\n for (var r = Array(s), k = 0, i = 0; i < il; i++)\r\n for (var a = arguments[i], j = 0, jl = a.length; j < jl; j++, k++)\r\n r[k] = a[j];\r\n return r;\r\n};\r\n\r\nexport function __await(v) {\r\n return this instanceof __await ? (this.v = v, this) : new __await(v);\r\n}\r\n\r\nexport function __asyncGenerator(thisArg, _arguments, generator) {\r\n if (!Symbol.asyncIterator) throw new TypeError(\"Symbol.asyncIterator is not defined.\");\r\n var g = generator.apply(thisArg, _arguments || []), i, q = [];\r\n return i = {}, verb(\"next\"), verb(\"throw\"), verb(\"return\"), i[Symbol.asyncIterator] = function () { return this; }, i;\r\n function verb(n) { if (g[n]) i[n] = function (v) { return new Promise(function (a, b) { q.push([n, v, a, b]) > 1 || resume(n, v); }); }; }\r\n function resume(n, v) { try { step(g[n](v)); } catch (e) { settle(q[0][3], e); } }\r\n function step(r) { r.value instanceof __await ? Promise.resolve(r.value.v).then(fulfill, reject) : settle(q[0][2], r); }\r\n function fulfill(value) { resume(\"next\", value); }\r\n function reject(value) { resume(\"throw\", value); }\r\n function settle(f, v) { if (f(v), q.shift(), q.length) resume(q[0][0], q[0][1]); }\r\n}\r\n\r\nexport function __asyncDelegator(o) {\r\n var i, p;\r\n return i = {}, verb(\"next\"), verb(\"throw\", function (e) { throw e; }), verb(\"return\"), i[Symbol.iterator] = function () { return this; }, i;\r\n function verb(n, f) { i[n] = o[n] ? function (v) { return (p = !p) ? { value: __await(o[n](v)), done: n === \"return\" } : f ? f(v) : v; } : f; }\r\n}\r\n\r\nexport function __asyncValues(o) {\r\n if (!Symbol.asyncIterator) throw new TypeError(\"Symbol.asyncIterator is not defined.\");\r\n var m = o[Symbol.asyncIterator], i;\r\n return m ? m.call(o) : (o = typeof __values === \"function\" ? __values(o) : o[Symbol.iterator](), i = {}, verb(\"next\"), verb(\"throw\"), verb(\"return\"), i[Symbol.asyncIterator] = function () { return this; }, i);\r\n function verb(n) { i[n] = o[n] && function (v) { return new Promise(function (resolve, reject) { v = o[n](v), settle(resolve, reject, v.done, v.value); }); }; }\r\n function settle(resolve, reject, d, v) { Promise.resolve(v).then(function(v) { resolve({ value: v, done: d }); }, reject); }\r\n}\r\n\r\nexport function __makeTemplateObject(cooked, raw) {\r\n if (Object.defineProperty) { Object.defineProperty(cooked, \"raw\", { value: raw }); } else { cooked.raw = raw; }\r\n return cooked;\r\n};\r\n\r\nexport function __importStar(mod) {\r\n if (mod && mod.__esModule) return mod;\r\n var result = {};\r\n if (mod != null) for (var k in mod) if (Object.hasOwnProperty.call(mod, k)) result[k] = mod[k];\r\n result.default = mod;\r\n return result;\r\n}\r\n\r\nexport function __importDefault(mod) {\r\n return (mod && mod.__esModule) ? mod : { default: mod };\r\n}\r\n\r\nexport function __classPrivateFieldGet(receiver, privateMap) {\r\n if (!privateMap.has(receiver)) {\r\n throw new TypeError(\"attempted to get private field on non-instance\");\r\n }\r\n return privateMap.get(receiver);\r\n}\r\n\r\nexport function __classPrivateFieldSet(receiver, privateMap, value) {\r\n if (!privateMap.has(receiver)) {\r\n throw new TypeError(\"attempted to set private field on non-instance\");\r\n }\r\n privateMap.set(receiver, value);\r\n return value;\r\n}\r\n",null,null,null,null,null,null,null,null,null,null],"names":["byteLength","toByteArray","fromByteArray","lookup","revLookup","Arr","Uint8Array","Array","code","i","len","length","charCodeAt","getLens","b64","Error","validLen","indexOf","placeHoldersLen","lens","_byteLength","tmp","arr","curByte","tripletToBase64","num","encodeChunk","uint8","start","end","output","push","join","extraBytes","parts","maxChunkLength","len2","buffer","offset","isLE","mLen","nBytes","e","m","eLen","eMax","eBias","nBits","d","s","NaN","Infinity","Math","pow","value","c","rt","abs","isNaN","floor","log","LN2","customInspectSymbol","Symbol","exports","Buffer","SlowBuffer","K_MAX_LENGTH","TYPED_ARRAY_SUPPORT","typedArraySupport","console","error","proto","foo","Object","setPrototypeOf","prototype","defineProperty","enumerable","get","isBuffer","undefined","byteOffset","createBuffer","RangeError","buf","arg","encodingOrOffset","TypeError","allocUnsafe","from","poolSize","fromString","ArrayBuffer","isView","fromArrayView","isInstance","fromArrayBuffer","SharedArrayBuffer","valueOf","b","fromObject","toPrimitive","assertSize","size","alloc","fill","encoding","checked","allocUnsafeSlow","string","isEncoding","actual","write","slice","fromArrayLike","array","arrayView","copy","obj","numberIsNaN","type","isArray","data","toString","_isBuffer","compare","a","x","y","min","String","toLowerCase","concat","list","pos","set","call","mustMatch","arguments","loweredCase","utf8ToBytes","base64ToBytes","slowToString","hexSlice","utf8Slice","asciiSlice","latin1Slice","base64Slice","utf16leSlice","swap","n","swap16","swap32","swap64","apply","toLocaleString","equals","inspect","str","max","INSPECT_MAX_BYTES","replace","trim","target","thisStart","thisEnd","thisCopy","targetCopy","bidirectionalIndexOf","val","dir","arrayIndexOf","lastIndexOf","indexSize","arrLength","valLength","read","readUInt16BE","foundIndex","found","j","includes","hexWrite","Number","remaining","strLen","parsed","parseInt","substr","utf8Write","blitBuffer","asciiWrite","asciiToBytes","base64Write","ucs2Write","utf16leToBytes","isFinite","toJSON","_arr","base64","res","firstByte","codePoint","bytesPerSequence","secondByte","thirdByte","fourthByte","tempCodePoint","decodeCodePointsArray","MAX_ARGUMENTS_LENGTH","codePoints","fromCharCode","ret","out","hexSliceLookupTable","bytes","newBuf","subarray","checkOffset","ext","readUintLE","readUIntLE","noAssert","mul","readUintBE","readUIntBE","readUint8","readUInt8","readUint16LE","readUInt16LE","readUint16BE","readUint32LE","readUInt32LE","readUint32BE","readUInt32BE","readIntLE","readIntBE","readInt8","readInt16LE","readInt16BE","readInt32LE","readInt32BE","readFloatLE","ieee754","readFloatBE","readDoubleLE","readDoubleBE","checkInt","writeUintLE","writeUIntLE","maxBytes","writeUintBE","writeUIntBE","writeUint8","writeUInt8","writeUint16LE","writeUInt16LE","writeUint16BE","writeUInt16BE","writeUint32LE","writeUInt32LE","writeUint32BE","writeUInt32BE","writeIntLE","limit","sub","writeIntBE","writeInt8","writeInt16LE","writeInt16BE","writeInt32LE","writeInt32BE","checkIEEE754","writeFloat","littleEndian","writeFloatLE","writeFloatBE","writeDouble","writeDoubleLE","writeDoubleBE","targetStart","copyWithin","INVALID_BASE64_RE","base64clean","split","units","leadSurrogate","byteArray","hi","lo","src","dst","constructor","name","alphabet","table","i16","performance","global","now","mozNow","msNow","oNow","webkitNow","Date","getTime","inherits","create","ctor","superCtor","super_","writable","configurable","TempCtor","formatRegExp","format","f","isString","objects","args","JSON","stringify","_","isNull","isObject","deprecate","fn","msg","isUndefined","process","warned","deprecated","debugs","debugEnviron","debuglog","toUpperCase","RegExp","test","pid","opts","ctx","seen","stylize","stylizeNoColor","depth","colors","isBoolean","showHidden","_extend","customInspect","stylizeWithColor","formatValue","styles","styleType","style","arrayToHash","hash","forEach","idx","recurseTimes","isFunction","primitive","formatPrimitive","keys","visibleKeys","getOwnPropertyNames","isError","formatError","isRegExp","isDate","base","braces","toUTCString","formatArray","map","key","formatProperty","pop","reduceToSingleString","simple","isNumber","l","hasOwnProperty","match","desc","getOwnPropertyDescriptor","line","reduce","prev","cur","numLinesEst","ar","isNullOrUndefined","isSymbol","re","objectToString","isPrimitive","maybeBuf","o","pad","months","timestamp","time","getHours","getMinutes","getSeconds","getDate","getMonth","origin","add","prop","buffer_1","utils_1","ensure_buffer_1","uuid_utils_1","binary_1","uuid_1","extendStatics","__proto__","p","__extends","__","__assign","assign","t","__rest","getOwnPropertySymbols","propertyIsEnumerable","__decorate","decorators","r","Reflect","decorate","__param","paramIndex","decorator","__metadata","metadataKey","metadataValue","metadata","__awaiter","thisArg","_arguments","P","generator","adopt","resolve","Promise","reject","fulfilled","step","next","rejected","result","done","then","__generator","body","label","sent","trys","ops","g","verb","iterator","v","op","__createBinding","k","k2","__exportStar","__values","__read","__spread","__spreadArrays","il","jl","__await","__asyncGenerator","asyncIterator","q","resume","settle","fulfill","shift","__asyncDelegator","__asyncValues","__makeTemplateObject","cooked","raw","__importStar","mod","__esModule","__importDefault","__classPrivateFieldGet","receiver","privateMap","has","__classPrivateFieldSet","tslib_1","objectid_1","symbol_1","int_32_1","decimal128_1","min_key_1","max_key_1","regexp_1","timestamp_1","code_1","db_ref_1","validate_utf8_1","decimal128","symbol","float_parser_1","extended_json_1","map_1","serializer_1","deserializer_1","calculate_size_1"],"mappings":";;;;;;;;;;AAEA,gBAAkB,GAAGA,UAArB;AACA,iBAAmB,GAAGC,WAAtB;AACA,mBAAqB,GAAGC,aAAxB;AAEA,IAAIC,MAAM,GAAG,EAAb;AACA,IAAIC,SAAS,GAAG,EAAhB;AACA,IAAIC,GAAG,GAAG,OAAOC,UAAP,KAAsB,WAAtB,GAAoCA,UAApC,GAAiDC,KAA3D;AAEA,IAAIC,MAAI,GAAG,kEAAX;;AACA,KAAK,IAAIC,CAAC,GAAG,CAAR,EAAWC,GAAG,GAAGF,MAAI,CAACG,MAA3B,EAAmCF,CAAC,GAAGC,GAAvC,EAA4C,EAAED,CAA9C,EAAiD;AAC/CN,EAAAA,MAAM,CAACM,CAAD,CAAN,GAAYD,MAAI,CAACC,CAAD,CAAhB;AACAL,EAAAA,SAAS,CAACI,MAAI,CAACI,UAAL,CAAgBH,CAAhB,CAAD,CAAT,GAAgCA,CAAhC;AACD;AAGD;;;AACAL,SAAS,CAAC,IAAIQ,UAAJ,CAAe,CAAf,CAAD,CAAT,GAA+B,EAA/B;AACAR,SAAS,CAAC,IAAIQ,UAAJ,CAAe,CAAf,CAAD,CAAT,GAA+B,EAA/B;;AAEA,SAASC,OAAT,CAAkBC,GAAlB,EAAuB;AACrB,MAAIJ,GAAG,GAAGI,GAAG,CAACH,MAAd;;AAEA,MAAID,GAAG,GAAG,CAAN,GAAU,CAAd,EAAiB;AACf,UAAM,IAAIK,KAAJ,CAAU,gDAAV,CAAN;AACD,GALoB;;;;AASrB,MAAIC,QAAQ,GAAGF,GAAG,CAACG,OAAJ,CAAY,GAAZ,CAAf;AACA,MAAID,QAAQ,KAAK,CAAC,CAAlB,EAAqBA,QAAQ,GAAGN,GAAX;AAErB,MAAIQ,eAAe,GAAGF,QAAQ,KAAKN,GAAb,GAClB,CADkB,GAElB,IAAKM,QAAQ,GAAG,CAFpB;AAIA,SAAO,CAACA,QAAD,EAAWE,eAAX,CAAP;AACD;;;AAGD,SAASlB,UAAT,CAAqBc,GAArB,EAA0B;AACxB,MAAIK,IAAI,GAAGN,OAAO,CAACC,GAAD,CAAlB;AACA,MAAIE,QAAQ,GAAGG,IAAI,CAAC,CAAD,CAAnB;AACA,MAAID,eAAe,GAAGC,IAAI,CAAC,CAAD,CAA1B;AACA,SAAQ,CAACH,QAAQ,GAAGE,eAAZ,IAA+B,CAA/B,GAAmC,CAApC,GAAyCA,eAAhD;AACD;;AAED,SAASE,WAAT,CAAsBN,GAAtB,EAA2BE,QAA3B,EAAqCE,eAArC,EAAsD;AACpD,SAAQ,CAACF,QAAQ,GAAGE,eAAZ,IAA+B,CAA/B,GAAmC,CAApC,GAAyCA,eAAhD;AACD;;AAED,SAASjB,WAAT,CAAsBa,GAAtB,EAA2B;AACzB,MAAIO,GAAJ;AACA,MAAIF,IAAI,GAAGN,OAAO,CAACC,GAAD,CAAlB;AACA,MAAIE,QAAQ,GAAGG,IAAI,CAAC,CAAD,CAAnB;AACA,MAAID,eAAe,GAAGC,IAAI,CAAC,CAAD,CAA1B;AAEA,MAAIG,GAAG,GAAG,IAAIjB,GAAJ,CAAQe,WAAW,CAACN,GAAD,EAAME,QAAN,EAAgBE,eAAhB,CAAnB,CAAV;AAEA,MAAIK,OAAO,GAAG,CAAd,CARyB;;AAWzB,MAAIb,GAAG,GAAGQ,eAAe,GAAG,CAAlB,GACNF,QAAQ,GAAG,CADL,GAENA,QAFJ;AAIA,MAAIP,CAAJ;;AACA,OAAKA,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAGC,GAAhB,EAAqBD,CAAC,IAAI,CAA1B,EAA6B;AAC3BY,IAAAA,GAAG,GACAjB,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAf,CAAD,CAAT,IAAgC,EAAjC,GACCL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,EADrC,GAECL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CAFrC,GAGAL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAJX;AAKAa,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAkBF,GAAG,IAAI,EAAR,GAAc,IAA/B;AACAC,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAkBF,GAAG,IAAI,CAAR,GAAa,IAA9B;AACAC,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAiBF,GAAG,GAAG,IAAvB;AACD;;AAED,MAAIH,eAAe,KAAK,CAAxB,EAA2B;AACzBG,IAAAA,GAAG,GACAjB,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAf,CAAD,CAAT,IAAgC,CAAjC,GACCL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CAFvC;AAGAa,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAiBF,GAAG,GAAG,IAAvB;AACD;;AAED,MAAIH,eAAe,KAAK,CAAxB,EAA2B;AACzBG,IAAAA,GAAG,GACAjB,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAf,CAAD,CAAT,IAAgC,EAAjC,GACCL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CADrC,GAECL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CAHvC;AAIAa,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAkBF,GAAG,IAAI,CAAR,GAAa,IAA9B;AACAC,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAiBF,GAAG,GAAG,IAAvB;AACD;;AAED,SAAOC,GAAP;AACD;;AAED,SAASE,eAAT,CAA0BC,GAA1B,EAA+B;AAC7B,SAAOtB,MAAM,CAACsB,GAAG,IAAI,EAAP,GAAY,IAAb,CAAN,GACLtB,MAAM,CAACsB,GAAG,IAAI,EAAP,GAAY,IAAb,CADD,GAELtB,MAAM,CAACsB,GAAG,IAAI,CAAP,GAAW,IAAZ,CAFD,GAGLtB,MAAM,CAACsB,GAAG,GAAG,IAAP,CAHR;AAID;;AAED,SAASC,WAAT,CAAsBC,KAAtB,EAA6BC,KAA7B,EAAoCC,GAApC,EAAyC;AACvC,MAAIR,GAAJ;AACA,MAAIS,MAAM,GAAG,EAAb;;AACA,OAAK,IAAIrB,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6BpB,CAAC,IAAI,CAAlC,EAAqC;AACnCY,IAAAA,GAAG,GACD,CAAEM,KAAK,CAAClB,CAAD,CAAL,IAAY,EAAb,GAAmB,QAApB,KACEkB,KAAK,CAAClB,CAAC,GAAG,CAAL,CAAL,IAAgB,CAAjB,GAAsB,MADvB,KAECkB,KAAK,CAAClB,CAAC,GAAG,CAAL,CAAL,GAAe,IAFhB,CADF;AAIAqB,IAAAA,MAAM,CAACC,IAAP,CAAYP,eAAe,CAACH,GAAD,CAA3B;AACD;;AACD,SAAOS,MAAM,CAACE,IAAP,CAAY,EAAZ,CAAP;AACD;;AAED,SAAS9B,aAAT,CAAwByB,KAAxB,EAA+B;AAC7B,MAAIN,GAAJ;AACA,MAAIX,GAAG,GAAGiB,KAAK,CAAChB,MAAhB;AACA,MAAIsB,UAAU,GAAGvB,GAAG,GAAG,CAAvB,CAH6B;;AAI7B,MAAIwB,KAAK,GAAG,EAAZ;AACA,MAAIC,cAAc,GAAG,KAArB,CAL6B;;;AAQ7B,OAAK,IAAI1B,CAAC,GAAG,CAAR,EAAW2B,IAAI,GAAG1B,GAAG,GAAGuB,UAA7B,EAAyCxB,CAAC,GAAG2B,IAA7C,EAAmD3B,CAAC,IAAI0B,cAAxD,EAAwE;AACtED,IAAAA,KAAK,CAACH,IAAN,CAAWL,WAAW,CAACC,KAAD,EAAQlB,CAAR,EAAYA,CAAC,GAAG0B,cAAL,GAAuBC,IAAvB,GAA8BA,IAA9B,GAAsC3B,CAAC,GAAG0B,cAArD,CAAtB;AACD,GAV4B;;;AAa7B,MAAIF,UAAU,KAAK,CAAnB,EAAsB;AACpBZ,IAAAA,GAAG,GAAGM,KAAK,CAACjB,GAAG,GAAG,CAAP,CAAX;AACAwB,IAAAA,KAAK,CAACH,IAAN,CACE5B,MAAM,CAACkB,GAAG,IAAI,CAAR,CAAN,GACAlB,MAAM,CAAEkB,GAAG,IAAI,CAAR,GAAa,IAAd,CADN,GAEA,IAHF;AAKD,GAPD,MAOO,IAAIY,UAAU,KAAK,CAAnB,EAAsB;AAC3BZ,IAAAA,GAAG,GAAG,CAACM,KAAK,CAACjB,GAAG,GAAG,CAAP,CAAL,IAAkB,CAAnB,IAAwBiB,KAAK,CAACjB,GAAG,GAAG,CAAP,CAAnC;AACAwB,IAAAA,KAAK,CAACH,IAAN,CACE5B,MAAM,CAACkB,GAAG,IAAI,EAAR,CAAN,GACAlB,MAAM,CAAEkB,GAAG,IAAI,CAAR,GAAa,IAAd,CADN,GAEAlB,MAAM,CAAEkB,GAAG,IAAI,CAAR,GAAa,IAAd,CAFN,GAGA,GAJF;AAMD;;AAED,SAAOa,KAAK,CAACF,IAAN,CAAW,EAAX,CAAP;;;;;;;;;ACpJF;AACA,QAAY,GAAG,aAAA,CAAUK,MAAV,EAAkBC,MAAlB,EAA0BC,IAA1B,EAAgCC,IAAhC,EAAsCC,MAAtC,EAA8C;AAC3D,MAAIC,CAAJ,EAAOC,CAAP;AACA,MAAIC,IAAI,GAAIH,MAAM,GAAG,CAAV,GAAeD,IAAf,GAAsB,CAAjC;AACA,MAAIK,IAAI,GAAG,CAAC,KAAKD,IAAN,IAAc,CAAzB;AACA,MAAIE,KAAK,GAAGD,IAAI,IAAI,CAApB;AACA,MAAIE,KAAK,GAAG,CAAC,CAAb;AACA,MAAItC,CAAC,GAAG8B,IAAI,GAAIE,MAAM,GAAG,CAAb,GAAkB,CAA9B;AACA,MAAIO,CAAC,GAAGT,IAAI,GAAG,CAAC,CAAJ,GAAQ,CAApB;AACA,MAAIU,CAAC,GAAGZ,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAd;AAEAA,EAAAA,CAAC,IAAIuC,CAAL;AAEAN,EAAAA,CAAC,GAAGO,CAAC,GAAI,CAAC,KAAM,CAACF,KAAR,IAAkB,CAA3B;AACAE,EAAAA,CAAC,KAAM,CAACF,KAAR;AACAA,EAAAA,KAAK,IAAIH,IAAT;;AACA,SAAOG,KAAK,GAAG,CAAf,EAAkBL,CAAC,GAAIA,CAAC,GAAG,GAAL,GAAYL,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAtB,EAAoCA,CAAC,IAAIuC,CAAzC,EAA4CD,KAAK,IAAI,CAAvE,EAA0E;;AAE1EJ,EAAAA,CAAC,GAAGD,CAAC,GAAI,CAAC,KAAM,CAACK,KAAR,IAAkB,CAA3B;AACAL,EAAAA,CAAC,KAAM,CAACK,KAAR;AACAA,EAAAA,KAAK,IAAIP,IAAT;;AACA,SAAOO,KAAK,GAAG,CAAf,EAAkBJ,CAAC,GAAIA,CAAC,GAAG,GAAL,GAAYN,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAtB,EAAoCA,CAAC,IAAIuC,CAAzC,EAA4CD,KAAK,IAAI,CAAvE,EAA0E;;AAE1E,MAAIL,CAAC,KAAK,CAAV,EAAa;AACXA,IAAAA,CAAC,GAAG,IAAII,KAAR;AACD,GAFD,MAEO,IAAIJ,CAAC,KAAKG,IAAV,EAAgB;AACrB,WAAOF,CAAC,GAAGO,GAAH,GAAU,CAACD,CAAC,GAAG,CAAC,CAAJ,GAAQ,CAAV,IAAeE,QAAjC;AACD,GAFM,MAEA;AACLR,IAAAA,CAAC,GAAGA,CAAC,GAAGS,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYb,IAAZ,CAAR;AACAE,IAAAA,CAAC,GAAGA,CAAC,GAAGI,KAAR;AACD;;AACD,SAAO,CAACG,CAAC,GAAG,CAAC,CAAJ,GAAQ,CAAV,IAAeN,CAAf,GAAmBS,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYX,CAAC,GAAGF,IAAhB,CAA1B;AACD,CA/BD;;AAiCA,SAAa,GAAG,cAAA,CAAUH,MAAV,EAAkBiB,KAAlB,EAAyBhB,MAAzB,EAAiCC,IAAjC,EAAuCC,IAAvC,EAA6CC,MAA7C,EAAqD;AACnE,MAAIC,CAAJ,EAAOC,CAAP,EAAUY,CAAV;AACA,MAAIX,IAAI,GAAIH,MAAM,GAAG,CAAV,GAAeD,IAAf,GAAsB,CAAjC;AACA,MAAIK,IAAI,GAAG,CAAC,KAAKD,IAAN,IAAc,CAAzB;AACA,MAAIE,KAAK,GAAGD,IAAI,IAAI,CAApB;AACA,MAAIW,EAAE,GAAIhB,IAAI,KAAK,EAAT,GAAcY,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,CAAC,EAAb,IAAmBD,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,CAAC,EAAb,CAAjC,GAAoD,CAA9D;AACA,MAAI5C,CAAC,GAAG8B,IAAI,GAAG,CAAH,GAAQE,MAAM,GAAG,CAA7B;AACA,MAAIO,CAAC,GAAGT,IAAI,GAAG,CAAH,GAAO,CAAC,CAApB;AACA,MAAIU,CAAC,GAAGK,KAAK,GAAG,CAAR,IAAcA,KAAK,KAAK,CAAV,IAAe,IAAIA,KAAJ,GAAY,CAAzC,GAA8C,CAA9C,GAAkD,CAA1D;AAEAA,EAAAA,KAAK,GAAGF,IAAI,CAACK,GAAL,CAASH,KAAT,CAAR;;AAEA,MAAII,KAAK,CAACJ,KAAD,CAAL,IAAgBA,KAAK,KAAKH,QAA9B,EAAwC;AACtCR,IAAAA,CAAC,GAAGe,KAAK,CAACJ,KAAD,CAAL,GAAe,CAAf,GAAmB,CAAvB;AACAZ,IAAAA,CAAC,GAAGG,IAAJ;AACD,GAHD,MAGO;AACLH,IAAAA,CAAC,GAAGU,IAAI,CAACO,KAAL,CAAWP,IAAI,CAACQ,GAAL,CAASN,KAAT,IAAkBF,IAAI,CAACS,GAAlC,CAAJ;;AACA,QAAIP,KAAK,IAAIC,CAAC,GAAGH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,CAACX,CAAb,CAAR,CAAL,GAAgC,CAApC,EAAuC;AACrCA,MAAAA,CAAC;AACDa,MAAAA,CAAC,IAAI,CAAL;AACD;;AACD,QAAIb,CAAC,GAAGI,KAAJ,IAAa,CAAjB,EAAoB;AAClBQ,MAAAA,KAAK,IAAIE,EAAE,GAAGD,CAAd;AACD,KAFD,MAEO;AACLD,MAAAA,KAAK,IAAIE,EAAE,GAAGJ,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIP,KAAhB,CAAd;AACD;;AACD,QAAIQ,KAAK,GAAGC,CAAR,IAAa,CAAjB,EAAoB;AAClBb,MAAAA,CAAC;AACDa,MAAAA,CAAC,IAAI,CAAL;AACD;;AAED,QAAIb,CAAC,GAAGI,KAAJ,IAAaD,IAAjB,EAAuB;AACrBF,MAAAA,CAAC,GAAG,CAAJ;AACAD,MAAAA,CAAC,GAAGG,IAAJ;AACD,KAHD,MAGO,IAAIH,CAAC,GAAGI,KAAJ,IAAa,CAAjB,EAAoB;AACzBH,MAAAA,CAAC,GAAG,CAAEW,KAAK,GAAGC,CAAT,GAAc,CAAf,IAAoBH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYb,IAAZ,CAAxB;AACAE,MAAAA,CAAC,GAAGA,CAAC,GAAGI,KAAR;AACD,KAHM,MAGA;AACLH,MAAAA,CAAC,GAAGW,KAAK,GAAGF,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYP,KAAK,GAAG,CAApB,CAAR,GAAiCM,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYb,IAAZ,CAArC;AACAE,MAAAA,CAAC,GAAG,CAAJ;AACD;AACF;;AAED,SAAOF,IAAI,IAAI,CAAf,EAAkBH,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAN,GAAqBkC,CAAC,GAAG,IAAzB,EAA+BlC,CAAC,IAAIuC,CAApC,EAAuCL,CAAC,IAAI,GAA5C,EAAiDH,IAAI,IAAI,CAA3E,EAA8E;;AAE9EE,EAAAA,CAAC,GAAIA,CAAC,IAAIF,IAAN,GAAcG,CAAlB;AACAC,EAAAA,IAAI,IAAIJ,IAAR;;AACA,SAAOI,IAAI,GAAG,CAAd,EAAiBP,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAN,GAAqBiC,CAAC,GAAG,IAAzB,EAA+BjC,CAAC,IAAIuC,CAApC,EAAuCN,CAAC,IAAI,GAA5C,EAAiDE,IAAI,IAAI,CAA1E,EAA6E;;AAE7EP,EAAAA,MAAM,CAACC,MAAM,GAAG7B,CAAT,GAAauC,CAAd,CAAN,IAA0BC,CAAC,GAAG,GAA9B;CAjDF;;;;;;;;;ACtBA,MAAIa,mBAAmB,GACpB,OAAOC,MAAP,KAAkB,UAAlB,IAAgC,OAAOA,MAAM,CAAC,KAAD,CAAb,KAAyB,UAA1D;AACIA,EAAAA,MAAM,CAAC,KAAD,CAAN,CAAc,4BAAd,CADJ;AAAA,IAEI,IAHN;AAKAC,EAAAA,cAAA,GAAiBC,MAAjB;AACAD,EAAAA,kBAAA,GAAqBE,UAArB;AACAF,EAAAA,yBAAA,GAA4B,EAA5B;AAEA,MAAIG,YAAY,GAAG,UAAnB;AACAH,EAAAA,kBAAA,GAAqBG,YAArB;AAEA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;AACAF,EAAAA,MAAM,CAACG,mBAAP,GAA6BC,iBAAiB,EAA9C;;AAEA,MAAI,CAACJ,MAAM,CAACG,mBAAR,IAA+B,OAAOE,OAAP,KAAmB,WAAlD,IACA,OAAOA,OAAO,CAACC,KAAf,KAAyB,UAD7B,EACyC;AACvCD,IAAAA,OAAO,CAACC,KAAR,CACE,8EACA,sEAFF;AAID;;AAED,WAASF,iBAAT,GAA8B;;AAE5B,QAAI;AACF,UAAI/C,GAAG,GAAG,IAAIhB,UAAJ,CAAe,CAAf,CAAV;AACA,UAAIkE,KAAK,GAAG;AAAEC,QAAAA,GAAG,EAAE,eAAY;AAAE,iBAAO,EAAP;AAAW;AAAhC,OAAZ;AACAC,MAAAA,MAAM,CAACC,cAAP,CAAsBH,KAAtB,EAA6BlE,UAAU,CAACsE,SAAxC;AACAF,MAAAA,MAAM,CAACC,cAAP,CAAsBrD,GAAtB,EAA2BkD,KAA3B;AACA,aAAOlD,GAAG,CAACmD,GAAJ,OAAc,EAArB;AACD,KAND,CAME,OAAO/B,CAAP,EAAU;AACV,aAAO,KAAP;AACD;AACF;;AAEDgC,EAAAA,MAAM,CAACG,cAAP,CAAsBZ,MAAM,CAACW,SAA7B,EAAwC,QAAxC,EAAkD;AAChDE,IAAAA,UAAU,EAAE,IADoC;AAEhDC,IAAAA,GAAG,EAAE,eAAY;AACf,UAAI,CAACd,MAAM,CAACe,QAAP,CAAgB,IAAhB,CAAL,EAA4B,OAAOC,SAAP;AAC5B,aAAO,KAAK5C,MAAZ;AACD;AAL+C,GAAlD;AAQAqC,EAAAA,MAAM,CAACG,cAAP,CAAsBZ,MAAM,CAACW,SAA7B,EAAwC,QAAxC,EAAkD;AAChDE,IAAAA,UAAU,EAAE,IADoC;AAEhDC,IAAAA,GAAG,EAAE,eAAY;AACf,UAAI,CAACd,MAAM,CAACe,QAAP,CAAgB,IAAhB,CAAL,EAA4B,OAAOC,SAAP;AAC5B,aAAO,KAAKC,UAAZ;AACD;AAL+C,GAAlD;;AAQA,WAASC,YAAT,CAAuBxE,MAAvB,EAA+B;AAC7B,QAAIA,MAAM,GAAGwD,YAAb,EAA2B;AACzB,YAAM,IAAIiB,UAAJ,CAAe,gBAAgBzE,MAAhB,GAAyB,gCAAxC,CAAN;AACD,KAH4B;;;AAK7B,QAAI0E,GAAG,GAAG,IAAI/E,UAAJ,CAAeK,MAAf,CAAV;AACA+D,IAAAA,MAAM,CAACC,cAAP,CAAsBU,GAAtB,EAA2BpB,MAAM,CAACW,SAAlC;AACA,WAAOS,GAAP;AACD;AAED;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;;AAEA,WAASpB,MAAT,CAAiBqB,GAAjB,EAAsBC,gBAAtB,EAAwC5E,MAAxC,EAAgD;;AAE9C,QAAI,OAAO2E,GAAP,KAAe,QAAnB,EAA6B;AAC3B,UAAI,OAAOC,gBAAP,KAA4B,QAAhC,EAA0C;AACxC,cAAM,IAAIC,SAAJ,CACJ,oEADI,CAAN;AAGD;;AACD,aAAOC,WAAW,CAACH,GAAD,CAAlB;AACD;;AACD,WAAOI,IAAI,CAACJ,GAAD,EAAMC,gBAAN,EAAwB5E,MAAxB,CAAX;AACD;;AAEDsD,EAAAA,MAAM,CAAC0B,QAAP,GAAkB,IAAlB;;AAEA,WAASD,IAAT,CAAepC,KAAf,EAAsBiC,gBAAtB,EAAwC5E,MAAxC,EAAgD;AAC9C,QAAI,OAAO2C,KAAP,KAAiB,QAArB,EAA+B;AAC7B,aAAOsC,UAAU,CAACtC,KAAD,EAAQiC,gBAAR,CAAjB;AACD;;AAED,QAAIM,WAAW,CAACC,MAAZ,CAAmBxC,KAAnB,CAAJ,EAA+B;AAC7B,aAAOyC,aAAa,CAACzC,KAAD,CAApB;AACD;;AAED,QAAIA,KAAK,IAAI,IAAb,EAAmB;AACjB,YAAM,IAAIkC,SAAJ,CACJ,gFACA,sCADA,0BACiDlC,KADjD,CADI,CAAN;AAID;;AAED,QAAI0C,UAAU,CAAC1C,KAAD,EAAQuC,WAAR,CAAV,IACCvC,KAAK,IAAI0C,UAAU,CAAC1C,KAAK,CAACjB,MAAP,EAAewD,WAAf,CADxB,EACsD;AACpD,aAAOI,eAAe,CAAC3C,KAAD,EAAQiC,gBAAR,EAA0B5E,MAA1B,CAAtB;AACD;;AAED,QAAI,OAAOuF,iBAAP,KAA6B,WAA7B,KACCF,UAAU,CAAC1C,KAAD,EAAQ4C,iBAAR,CAAV,IACA5C,KAAK,IAAI0C,UAAU,CAAC1C,KAAK,CAACjB,MAAP,EAAe6D,iBAAf,CAFpB,CAAJ,EAE6D;AAC3D,aAAOD,eAAe,CAAC3C,KAAD,EAAQiC,gBAAR,EAA0B5E,MAA1B,CAAtB;AACD;;AAED,QAAI,OAAO2C,KAAP,KAAiB,QAArB,EAA+B;AAC7B,YAAM,IAAIkC,SAAJ,CACJ,uEADI,CAAN;AAGD;;AAED,QAAIW,OAAO,GAAG7C,KAAK,CAAC6C,OAAN,IAAiB7C,KAAK,CAAC6C,OAAN,EAA/B;;AACA,QAAIA,OAAO,IAAI,IAAX,IAAmBA,OAAO,KAAK7C,KAAnC,EAA0C;AACxC,aAAOW,MAAM,CAACyB,IAAP,CAAYS,OAAZ,EAAqBZ,gBAArB,EAAuC5E,MAAvC,CAAP;AACD;;AAED,QAAIyF,CAAC,GAAGC,UAAU,CAAC/C,KAAD,CAAlB;AACA,QAAI8C,CAAJ,EAAO,OAAOA,CAAP;;AAEP,QAAI,OAAOrC,MAAP,KAAkB,WAAlB,IAAiCA,MAAM,CAACuC,WAAP,IAAsB,IAAvD,IACA,OAAOhD,KAAK,CAACS,MAAM,CAACuC,WAAR,CAAZ,KAAqC,UADzC,EACqD;AACnD,aAAOrC,MAAM,CAACyB,IAAP,CACLpC,KAAK,CAACS,MAAM,CAACuC,WAAR,CAAL,CAA0B,QAA1B,CADK,EACgCf,gBADhC,EACkD5E,MADlD,CAAP;AAGD;;AAED,UAAM,IAAI6E,SAAJ,CACJ,gFACA,sCADA,0BACiDlC,KADjD,CADI,CAAN;AAID;AAED;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;;AACAW,EAAAA,MAAM,CAACyB,IAAP,GAAc,UAAUpC,KAAV,EAAiBiC,gBAAjB,EAAmC5E,MAAnC,EAA2C;AACvD,WAAO+E,IAAI,CAACpC,KAAD,EAAQiC,gBAAR,EAA0B5E,MAA1B,CAAX;AACD,GAFD;AAKA;;;AACA+D,EAAAA,MAAM,CAACC,cAAP,CAAsBV,MAAM,CAACW,SAA7B,EAAwCtE,UAAU,CAACsE,SAAnD;AACAF,EAAAA,MAAM,CAACC,cAAP,CAAsBV,MAAtB,EAA8B3D,UAA9B;;AAEA,WAASiG,UAAT,CAAqBC,IAArB,EAA2B;AACzB,QAAI,OAAOA,IAAP,KAAgB,QAApB,EAA8B;AAC5B,YAAM,IAAIhB,SAAJ,CAAc,wCAAd,CAAN;AACD,KAFD,MAEO,IAAIgB,IAAI,GAAG,CAAX,EAAc;AACnB,YAAM,IAAIpB,UAAJ,CAAe,gBAAgBoB,IAAhB,GAAuB,gCAAtC,CAAN;AACD;AACF;;AAED,WAASC,KAAT,CAAgBD,IAAhB,EAAsBE,IAAtB,EAA4BC,QAA5B,EAAsC;AACpCJ,IAAAA,UAAU,CAACC,IAAD,CAAV;;AACA,QAAIA,IAAI,IAAI,CAAZ,EAAe;AACb,aAAOrB,YAAY,CAACqB,IAAD,CAAnB;AACD;;AACD,QAAIE,IAAI,KAAKzB,SAAb,EAAwB;;;;AAItB,aAAO,OAAO0B,QAAP,KAAoB,QAApB,GACHxB,YAAY,CAACqB,IAAD,CAAZ,CAAmBE,IAAnB,CAAwBA,IAAxB,EAA8BC,QAA9B,CADG,GAEHxB,YAAY,CAACqB,IAAD,CAAZ,CAAmBE,IAAnB,CAAwBA,IAAxB,CAFJ;AAGD;;AACD,WAAOvB,YAAY,CAACqB,IAAD,CAAnB;AACD;AAED;AACA;AACA;AACA;;;AACAvC,EAAAA,MAAM,CAACwC,KAAP,GAAe,UAAUD,IAAV,EAAgBE,IAAhB,EAAsBC,QAAtB,EAAgC;AAC7C,WAAOF,KAAK,CAACD,IAAD,EAAOE,IAAP,EAAaC,QAAb,CAAZ;AACD,GAFD;;AAIA,WAASlB,WAAT,CAAsBe,IAAtB,EAA4B;AAC1BD,IAAAA,UAAU,CAACC,IAAD,CAAV;AACA,WAAOrB,YAAY,CAACqB,IAAI,GAAG,CAAP,GAAW,CAAX,GAAeI,OAAO,CAACJ,IAAD,CAAP,GAAgB,CAAhC,CAAnB;AACD;AAED;AACA;AACA;;;AACAvC,EAAAA,MAAM,CAACwB,WAAP,GAAqB,UAAUe,IAAV,EAAgB;AACnC,WAAOf,WAAW,CAACe,IAAD,CAAlB;AACD,GAFD;AAGA;AACA;AACA;;;AACAvC,EAAAA,MAAM,CAAC4C,eAAP,GAAyB,UAAUL,IAAV,EAAgB;AACvC,WAAOf,WAAW,CAACe,IAAD,CAAlB;AACD,GAFD;;AAIA,WAASZ,UAAT,CAAqBkB,MAArB,EAA6BH,QAA7B,EAAuC;AACrC,QAAI,OAAOA,QAAP,KAAoB,QAApB,IAAgCA,QAAQ,KAAK,EAAjD,EAAqD;AACnDA,MAAAA,QAAQ,GAAG,MAAX;AACD;;AAED,QAAI,CAAC1C,MAAM,CAAC8C,UAAP,CAAkBJ,QAAlB,CAAL,EAAkC;AAChC,YAAM,IAAInB,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;AACD;;AAED,QAAIhG,MAAM,GAAGX,UAAU,CAAC8G,MAAD,EAASH,QAAT,CAAV,GAA+B,CAA5C;AACA,QAAItB,GAAG,GAAGF,YAAY,CAACxE,MAAD,CAAtB;AAEA,QAAIqG,MAAM,GAAG3B,GAAG,CAAC4B,KAAJ,CAAUH,MAAV,EAAkBH,QAAlB,CAAb;;AAEA,QAAIK,MAAM,KAAKrG,MAAf,EAAuB;;;;AAIrB0E,MAAAA,GAAG,GAAGA,GAAG,CAAC6B,KAAJ,CAAU,CAAV,EAAaF,MAAb,CAAN;AACD;;AAED,WAAO3B,GAAP;AACD;;AAED,WAAS8B,aAAT,CAAwBC,KAAxB,EAA+B;AAC7B,QAAIzG,MAAM,GAAGyG,KAAK,CAACzG,MAAN,GAAe,CAAf,GAAmB,CAAnB,GAAuBiG,OAAO,CAACQ,KAAK,CAACzG,MAAP,CAAP,GAAwB,CAA5D;AACA,QAAI0E,GAAG,GAAGF,YAAY,CAACxE,MAAD,CAAtB;;AACA,SAAK,IAAIF,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4BF,CAAC,IAAI,CAAjC,EAAoC;AAClC4E,MAAAA,GAAG,CAAC5E,CAAD,CAAH,GAAS2G,KAAK,CAAC3G,CAAD,CAAL,GAAW,GAApB;AACD;;AACD,WAAO4E,GAAP;AACD;;AAED,WAASU,aAAT,CAAwBsB,SAAxB,EAAmC;AACjC,QAAIrB,UAAU,CAACqB,SAAD,EAAY/G,UAAZ,CAAd,EAAuC;AACrC,UAAIgH,IAAI,GAAG,IAAIhH,UAAJ,CAAe+G,SAAf,CAAX;AACA,aAAOpB,eAAe,CAACqB,IAAI,CAACjF,MAAN,EAAciF,IAAI,CAACpC,UAAnB,EAA+BoC,IAAI,CAACtH,UAApC,CAAtB;AACD;;AACD,WAAOmH,aAAa,CAACE,SAAD,CAApB;AACD;;AAED,WAASpB,eAAT,CAA0BmB,KAA1B,EAAiClC,UAAjC,EAA6CvE,MAA7C,EAAqD;AACnD,QAAIuE,UAAU,GAAG,CAAb,IAAkBkC,KAAK,CAACpH,UAAN,GAAmBkF,UAAzC,EAAqD;AACnD,YAAM,IAAIE,UAAJ,CAAe,sCAAf,CAAN;AACD;;AAED,QAAIgC,KAAK,CAACpH,UAAN,GAAmBkF,UAAU,IAAIvE,MAAM,IAAI,CAAd,CAAjC,EAAmD;AACjD,YAAM,IAAIyE,UAAJ,CAAe,sCAAf,CAAN;AACD;;AAED,QAAIC,GAAJ;;AACA,QAAIH,UAAU,KAAKD,SAAf,IAA4BtE,MAAM,KAAKsE,SAA3C,EAAsD;AACpDI,MAAAA,GAAG,GAAG,IAAI/E,UAAJ,CAAe8G,KAAf,CAAN;AACD,KAFD,MAEO,IAAIzG,MAAM,KAAKsE,SAAf,EAA0B;AAC/BI,MAAAA,GAAG,GAAG,IAAI/E,UAAJ,CAAe8G,KAAf,EAAsBlC,UAAtB,CAAN;AACD,KAFM,MAEA;AACLG,MAAAA,GAAG,GAAG,IAAI/E,UAAJ,CAAe8G,KAAf,EAAsBlC,UAAtB,EAAkCvE,MAAlC,CAAN;AACD,KAhBkD;;;AAmBnD+D,IAAAA,MAAM,CAACC,cAAP,CAAsBU,GAAtB,EAA2BpB,MAAM,CAACW,SAAlC;AAEA,WAAOS,GAAP;AACD;;AAED,WAASgB,UAAT,CAAqBkB,GAArB,EAA0B;AACxB,QAAItD,MAAM,CAACe,QAAP,CAAgBuC,GAAhB,CAAJ,EAA0B;AACxB,UAAI7G,GAAG,GAAGkG,OAAO,CAACW,GAAG,CAAC5G,MAAL,CAAP,GAAsB,CAAhC;AACA,UAAI0E,GAAG,GAAGF,YAAY,CAACzE,GAAD,CAAtB;;AAEA,UAAI2E,GAAG,CAAC1E,MAAJ,KAAe,CAAnB,EAAsB;AACpB,eAAO0E,GAAP;AACD;;AAEDkC,MAAAA,GAAG,CAACD,IAAJ,CAASjC,GAAT,EAAc,CAAd,EAAiB,CAAjB,EAAoB3E,GAApB;AACA,aAAO2E,GAAP;AACD;;AAED,QAAIkC,GAAG,CAAC5G,MAAJ,KAAesE,SAAnB,EAA8B;AAC5B,UAAI,OAAOsC,GAAG,CAAC5G,MAAX,KAAsB,QAAtB,IAAkC6G,WAAW,CAACD,GAAG,CAAC5G,MAAL,CAAjD,EAA+D;AAC7D,eAAOwE,YAAY,CAAC,CAAD,CAAnB;AACD;;AACD,aAAOgC,aAAa,CAACI,GAAD,CAApB;AACD;;AAED,QAAIA,GAAG,CAACE,IAAJ,KAAa,QAAb,IAAyBlH,KAAK,CAACmH,OAAN,CAAcH,GAAG,CAACI,IAAlB,CAA7B,EAAsD;AACpD,aAAOR,aAAa,CAACI,GAAG,CAACI,IAAL,CAApB;AACD;AACF;;AAED,WAASf,OAAT,CAAkBjG,MAAlB,EAA0B;;;AAGxB,QAAIA,MAAM,IAAIwD,YAAd,EAA4B;AAC1B,YAAM,IAAIiB,UAAJ,CAAe,oDACA,UADA,GACajB,YAAY,CAACyD,QAAb,CAAsB,EAAtB,CADb,GACyC,QADxD,CAAN;AAED;;AACD,WAAOjH,MAAM,GAAG,CAAhB;AACD;;AAED,WAASuD,UAAT,CAAqBvD,MAArB,EAA6B;AAC3B,QAAI,CAACA,MAAD,IAAWA,MAAf,EAAuB;;AACrBA,MAAAA,MAAM,GAAG,CAAT;AACD;;AACD,WAAOsD,MAAM,CAACwC,KAAP,CAAa,CAAC9F,MAAd,CAAP;AACD;;AAEDsD,EAAAA,MAAM,CAACe,QAAP,GAAkB,SAASA,QAAT,CAAmBoB,CAAnB,EAAsB;AACtC,WAAOA,CAAC,IAAI,IAAL,IAAaA,CAAC,CAACyB,SAAF,KAAgB,IAA7B,IACLzB,CAAC,KAAKnC,MAAM,CAACW,SADf,CADsC;AAGvC,GAHD;;AAKAX,EAAAA,MAAM,CAAC6D,OAAP,GAAiB,SAASA,OAAT,CAAkBC,CAAlB,EAAqB3B,CAArB,EAAwB;AACvC,QAAIJ,UAAU,CAAC+B,CAAD,EAAIzH,UAAJ,CAAd,EAA+ByH,CAAC,GAAG9D,MAAM,CAACyB,IAAP,CAAYqC,CAAZ,EAAeA,CAAC,CAACzF,MAAjB,EAAyByF,CAAC,CAAC/H,UAA3B,CAAJ;AAC/B,QAAIgG,UAAU,CAACI,CAAD,EAAI9F,UAAJ,CAAd,EAA+B8F,CAAC,GAAGnC,MAAM,CAACyB,IAAP,CAAYU,CAAZ,EAAeA,CAAC,CAAC9D,MAAjB,EAAyB8D,CAAC,CAACpG,UAA3B,CAAJ;;AAC/B,QAAI,CAACiE,MAAM,CAACe,QAAP,CAAgB+C,CAAhB,CAAD,IAAuB,CAAC9D,MAAM,CAACe,QAAP,CAAgBoB,CAAhB,CAA5B,EAAgD;AAC9C,YAAM,IAAIZ,SAAJ,CACJ,uEADI,CAAN;AAGD;;AAED,QAAIuC,CAAC,KAAK3B,CAAV,EAAa,OAAO,CAAP;AAEb,QAAI4B,CAAC,GAAGD,CAAC,CAACpH,MAAV;AACA,QAAIsH,CAAC,GAAG7B,CAAC,CAACzF,MAAV;;AAEA,SAAK,IAAIF,CAAC,GAAG,CAAR,EAAWC,GAAG,GAAG0C,IAAI,CAAC8E,GAAL,CAASF,CAAT,EAAYC,CAAZ,CAAtB,EAAsCxH,CAAC,GAAGC,GAA1C,EAA+C,EAAED,CAAjD,EAAoD;AAClD,UAAIsH,CAAC,CAACtH,CAAD,CAAD,KAAS2F,CAAC,CAAC3F,CAAD,CAAd,EAAmB;AACjBuH,QAAAA,CAAC,GAAGD,CAAC,CAACtH,CAAD,CAAL;AACAwH,QAAAA,CAAC,GAAG7B,CAAC,CAAC3F,CAAD,CAAL;AACA;AACD;AACF;;AAED,QAAIuH,CAAC,GAAGC,CAAR,EAAW,OAAO,CAAC,CAAR;AACX,QAAIA,CAAC,GAAGD,CAAR,EAAW,OAAO,CAAP;AACX,WAAO,CAAP;AACD,GAzBD;;AA2BA/D,EAAAA,MAAM,CAAC8C,UAAP,GAAoB,SAASA,UAAT,CAAqBJ,QAArB,EAA+B;AACjD,YAAQwB,MAAM,CAACxB,QAAD,CAAN,CAAiByB,WAAjB,EAAR;AACE,WAAK,KAAL;AACA,WAAK,MAAL;AACA,WAAK,OAAL;AACA,WAAK,OAAL;AACA,WAAK,QAAL;AACA,WAAK,QAAL;AACA,WAAK,QAAL;AACA,WAAK,MAAL;AACA,WAAK,OAAL;AACA,WAAK,SAAL;AACA,WAAK,UAAL;AACE,eAAO,IAAP;;AACF;AACE,eAAO,KAAP;AAdJ;AAgBD,GAjBD;;AAmBAnE,EAAAA,MAAM,CAACoE,MAAP,GAAgB,SAASA,MAAT,CAAiBC,IAAjB,EAAuB3H,MAAvB,EAA+B;AAC7C,QAAI,CAACJ,KAAK,CAACmH,OAAN,CAAcY,IAAd,CAAL,EAA0B;AACxB,YAAM,IAAI9C,SAAJ,CAAc,6CAAd,CAAN;AACD;;AAED,QAAI8C,IAAI,CAAC3H,MAAL,KAAgB,CAApB,EAAuB;AACrB,aAAOsD,MAAM,CAACwC,KAAP,CAAa,CAAb,CAAP;AACD;;AAED,QAAIhG,CAAJ;;AACA,QAAIE,MAAM,KAAKsE,SAAf,EAA0B;AACxBtE,MAAAA,MAAM,GAAG,CAAT;;AACA,WAAKF,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAG6H,IAAI,CAAC3H,MAArB,EAA6B,EAAEF,CAA/B,EAAkC;AAChCE,QAAAA,MAAM,IAAI2H,IAAI,CAAC7H,CAAD,CAAJ,CAAQE,MAAlB;AACD;AACF;;AAED,QAAI0B,MAAM,GAAG4B,MAAM,CAACwB,WAAP,CAAmB9E,MAAnB,CAAb;AACA,QAAI4H,GAAG,GAAG,CAAV;;AACA,SAAK9H,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAG6H,IAAI,CAAC3H,MAArB,EAA6B,EAAEF,CAA/B,EAAkC;AAChC,UAAI4E,GAAG,GAAGiD,IAAI,CAAC7H,CAAD,CAAd;;AACA,UAAIuF,UAAU,CAACX,GAAD,EAAM/E,UAAN,CAAd,EAAiC;AAC/B,YAAIiI,GAAG,GAAGlD,GAAG,CAAC1E,MAAV,GAAmB0B,MAAM,CAAC1B,MAA9B,EAAsC;AACpCsD,UAAAA,MAAM,CAACyB,IAAP,CAAYL,GAAZ,EAAiBiC,IAAjB,CAAsBjF,MAAtB,EAA8BkG,GAA9B;AACD,SAFD,MAEO;AACLjI,UAAAA,UAAU,CAACsE,SAAX,CAAqB4D,GAArB,CAAyBC,IAAzB,CACEpG,MADF,EAEEgD,GAFF,EAGEkD,GAHF;AAKD;AACF,OAVD,MAUO,IAAI,CAACtE,MAAM,CAACe,QAAP,CAAgBK,GAAhB,CAAL,EAA2B;AAChC,cAAM,IAAIG,SAAJ,CAAc,6CAAd,CAAN;AACD,OAFM,MAEA;AACLH,QAAAA,GAAG,CAACiC,IAAJ,CAASjF,MAAT,EAAiBkG,GAAjB;AACD;;AACDA,MAAAA,GAAG,IAAIlD,GAAG,CAAC1E,MAAX;AACD;;AACD,WAAO0B,MAAP;AACD,GAvCD;;AAyCA,WAASrC,UAAT,CAAqB8G,MAArB,EAA6BH,QAA7B,EAAuC;AACrC,QAAI1C,MAAM,CAACe,QAAP,CAAgB8B,MAAhB,CAAJ,EAA6B;AAC3B,aAAOA,MAAM,CAACnG,MAAd;AACD;;AACD,QAAIkF,WAAW,CAACC,MAAZ,CAAmBgB,MAAnB,KAA8Bd,UAAU,CAACc,MAAD,EAASjB,WAAT,CAA5C,EAAmE;AACjE,aAAOiB,MAAM,CAAC9G,UAAd;AACD;;AACD,QAAI,OAAO8G,MAAP,KAAkB,QAAtB,EAAgC;AAC9B,YAAM,IAAItB,SAAJ,CACJ,+EACA,gBADA,0BAC0BsB,MAD1B,CADI,CAAN;AAID;;AAED,QAAIpG,GAAG,GAAGoG,MAAM,CAACnG,MAAjB;AACA,QAAI+H,SAAS,GAAIC,SAAS,CAAChI,MAAV,GAAmB,CAAnB,IAAwBgI,SAAS,CAAC,CAAD,CAAT,KAAiB,IAA1D;AACA,QAAI,CAACD,SAAD,IAAchI,GAAG,KAAK,CAA1B,EAA6B,OAAO,CAAP,CAhBQ;;AAmBrC,QAAIkI,WAAW,GAAG,KAAlB;;AACA,aAAS;AACP,cAAQjC,QAAR;AACE,aAAK,OAAL;AACA,aAAK,QAAL;AACA,aAAK,QAAL;AACE,iBAAOjG,GAAP;;AACF,aAAK,MAAL;AACA,aAAK,OAAL;AACE,iBAAOmI,WAAW,CAAC/B,MAAD,CAAX,CAAoBnG,MAA3B;;AACF,aAAK,MAAL;AACA,aAAK,OAAL;AACA,aAAK,SAAL;AACA,aAAK,UAAL;AACE,iBAAOD,GAAG,GAAG,CAAb;;AACF,aAAK,KAAL;AACE,iBAAOA,GAAG,KAAK,CAAf;;AACF,aAAK,QAAL;AACE,iBAAOoI,aAAa,CAAChC,MAAD,CAAb,CAAsBnG,MAA7B;;AACF;AACE,cAAIiI,WAAJ,EAAiB;AACf,mBAAOF,SAAS,GAAG,CAAC,CAAJ,GAAQG,WAAW,CAAC/B,MAAD,CAAX,CAAoBnG,MAA5C,CADe;AAEhB;;AACDgG,UAAAA,QAAQ,GAAG,CAAC,KAAKA,QAAN,EAAgByB,WAAhB,EAAX;AACAQ,UAAAA,WAAW,GAAG,IAAd;AAtBJ;AAwBD;AACF;;AACD3E,EAAAA,MAAM,CAACjE,UAAP,GAAoBA,UAApB;;AAEA,WAAS+I,YAAT,CAAuBpC,QAAvB,EAAiC/E,KAAjC,EAAwCC,GAAxC,EAA6C;AAC3C,QAAI+G,WAAW,GAAG,KAAlB,CAD2C;;;;;;;AAU3C,QAAIhH,KAAK,KAAKqD,SAAV,IAAuBrD,KAAK,GAAG,CAAnC,EAAsC;AACpCA,MAAAA,KAAK,GAAG,CAAR;AACD,KAZ0C;;;;AAe3C,QAAIA,KAAK,GAAG,KAAKjB,MAAjB,EAAyB;AACvB,aAAO,EAAP;AACD;;AAED,QAAIkB,GAAG,KAAKoD,SAAR,IAAqBpD,GAAG,GAAG,KAAKlB,MAApC,EAA4C;AAC1CkB,MAAAA,GAAG,GAAG,KAAKlB,MAAX;AACD;;AAED,QAAIkB,GAAG,IAAI,CAAX,EAAc;AACZ,aAAO,EAAP;AACD,KAzB0C;;;AA4B3CA,IAAAA,GAAG,MAAM,CAAT;AACAD,IAAAA,KAAK,MAAM,CAAX;;AAEA,QAAIC,GAAG,IAAID,KAAX,EAAkB;AAChB,aAAO,EAAP;AACD;;AAED,QAAI,CAAC+E,QAAL,EAAeA,QAAQ,GAAG,MAAX;;AAEf,WAAO,IAAP,EAAa;AACX,cAAQA,QAAR;AACE,aAAK,KAAL;AACE,iBAAOqC,QAAQ,CAAC,IAAD,EAAOpH,KAAP,EAAcC,GAAd,CAAf;;AAEF,aAAK,MAAL;AACA,aAAK,OAAL;AACE,iBAAOoH,SAAS,CAAC,IAAD,EAAOrH,KAAP,EAAcC,GAAd,CAAhB;;AAEF,aAAK,OAAL;AACE,iBAAOqH,UAAU,CAAC,IAAD,EAAOtH,KAAP,EAAcC,GAAd,CAAjB;;AAEF,aAAK,QAAL;AACA,aAAK,QAAL;AACE,iBAAOsH,WAAW,CAAC,IAAD,EAAOvH,KAAP,EAAcC,GAAd,CAAlB;;AAEF,aAAK,QAAL;AACE,iBAAOuH,WAAW,CAAC,IAAD,EAAOxH,KAAP,EAAcC,GAAd,CAAlB;;AAEF,aAAK,MAAL;AACA,aAAK,OAAL;AACA,aAAK,SAAL;AACA,aAAK,UAAL;AACE,iBAAOwH,YAAY,CAAC,IAAD,EAAOzH,KAAP,EAAcC,GAAd,CAAnB;;AAEF;AACE,cAAI+G,WAAJ,EAAiB,MAAM,IAAIpD,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;AACjBA,UAAAA,QAAQ,GAAG,CAACA,QAAQ,GAAG,EAAZ,EAAgByB,WAAhB,EAAX;AACAQ,UAAAA,WAAW,GAAG,IAAd;AA3BJ;AA6BD;AACF;AAGD;AACA;AACA;AACA;AACA;;;AACA3E,EAAAA,MAAM,CAACW,SAAP,CAAiBiD,SAAjB,GAA6B,IAA7B;;AAEA,WAASyB,IAAT,CAAelD,CAAf,EAAkBmD,CAAlB,EAAqB5G,CAArB,EAAwB;AACtB,QAAIlC,CAAC,GAAG2F,CAAC,CAACmD,CAAD,CAAT;AACAnD,IAAAA,CAAC,CAACmD,CAAD,CAAD,GAAOnD,CAAC,CAACzD,CAAD,CAAR;AACAyD,IAAAA,CAAC,CAACzD,CAAD,CAAD,GAAOlC,CAAP;AACD;;AAEDwD,EAAAA,MAAM,CAACW,SAAP,CAAiB4E,MAAjB,GAA0B,SAASA,MAAT,GAAmB;AAC3C,QAAI9I,GAAG,GAAG,KAAKC,MAAf;;AACA,QAAID,GAAG,GAAG,CAAN,KAAY,CAAhB,EAAmB;AACjB,YAAM,IAAI0E,UAAJ,CAAe,2CAAf,CAAN;AACD;;AACD,SAAK,IAAI3E,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyBD,CAAC,IAAI,CAA9B,EAAiC;AAC/B6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAP,EAAUA,CAAC,GAAG,CAAd,CAAJ;AACD;;AACD,WAAO,IAAP;AACD,GATD;;AAWAwD,EAAAA,MAAM,CAACW,SAAP,CAAiB6E,MAAjB,GAA0B,SAASA,MAAT,GAAmB;AAC3C,QAAI/I,GAAG,GAAG,KAAKC,MAAf;;AACA,QAAID,GAAG,GAAG,CAAN,KAAY,CAAhB,EAAmB;AACjB,YAAM,IAAI0E,UAAJ,CAAe,2CAAf,CAAN;AACD;;AACD,SAAK,IAAI3E,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyBD,CAAC,IAAI,CAA9B,EAAiC;AAC/B6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAP,EAAUA,CAAC,GAAG,CAAd,CAAJ;AACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;AACD;;AACD,WAAO,IAAP;AACD,GAVD;;AAYAwD,EAAAA,MAAM,CAACW,SAAP,CAAiB8E,MAAjB,GAA0B,SAASA,MAAT,GAAmB;AAC3C,QAAIhJ,GAAG,GAAG,KAAKC,MAAf;;AACA,QAAID,GAAG,GAAG,CAAN,KAAY,CAAhB,EAAmB;AACjB,YAAM,IAAI0E,UAAJ,CAAe,2CAAf,CAAN;AACD;;AACD,SAAK,IAAI3E,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyBD,CAAC,IAAI,CAA9B,EAAiC;AAC/B6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAP,EAAUA,CAAC,GAAG,CAAd,CAAJ;AACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;AACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;AACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;AACD;;AACD,WAAO,IAAP;AACD,GAZD;;AAcAwD,EAAAA,MAAM,CAACW,SAAP,CAAiBgD,QAAjB,GAA4B,SAASA,QAAT,GAAqB;AAC/C,QAAIjH,MAAM,GAAG,KAAKA,MAAlB;AACA,QAAIA,MAAM,KAAK,CAAf,EAAkB,OAAO,EAAP;AAClB,QAAIgI,SAAS,CAAChI,MAAV,KAAqB,CAAzB,EAA4B,OAAOsI,SAAS,CAAC,IAAD,EAAO,CAAP,EAAUtI,MAAV,CAAhB;AAC5B,WAAOoI,YAAY,CAACY,KAAb,CAAmB,IAAnB,EAAyBhB,SAAzB,CAAP;AACD,GALD;;AAOA1E,EAAAA,MAAM,CAACW,SAAP,CAAiBgF,cAAjB,GAAkC3F,MAAM,CAACW,SAAP,CAAiBgD,QAAnD;;AAEA3D,EAAAA,MAAM,CAACW,SAAP,CAAiBiF,MAAjB,GAA0B,SAASA,MAAT,CAAiBzD,CAAjB,EAAoB;AAC5C,QAAI,CAACnC,MAAM,CAACe,QAAP,CAAgBoB,CAAhB,CAAL,EAAyB,MAAM,IAAIZ,SAAJ,CAAc,2BAAd,CAAN;AACzB,QAAI,SAASY,CAAb,EAAgB,OAAO,IAAP;AAChB,WAAOnC,MAAM,CAAC6D,OAAP,CAAe,IAAf,EAAqB1B,CAArB,MAA4B,CAAnC;AACD,GAJD;;AAMAnC,EAAAA,MAAM,CAACW,SAAP,CAAiBkF,OAAjB,GAA2B,SAASA,OAAT,GAAoB;AAC7C,QAAIC,GAAG,GAAG,EAAV;AACA,QAAIC,GAAG,GAAGhG,OAAO,CAACiG,iBAAlB;AACAF,IAAAA,GAAG,GAAG,KAAKnC,QAAL,CAAc,KAAd,EAAqB,CAArB,EAAwBoC,GAAxB,EAA6BE,OAA7B,CAAqC,SAArC,EAAgD,KAAhD,EAAuDC,IAAvD,EAAN;AACA,QAAI,KAAKxJ,MAAL,GAAcqJ,GAAlB,EAAuBD,GAAG,IAAI,OAAP;AACvB,WAAO,aAAaA,GAAb,GAAmB,GAA1B;AACD,GAND;;AAOA,MAAIjG,mBAAJ,EAAyB;AACvBG,IAAAA,MAAM,CAACW,SAAP,CAAiBd,mBAAjB,IAAwCG,MAAM,CAACW,SAAP,CAAiBkF,OAAzD;AACD;;AAED7F,EAAAA,MAAM,CAACW,SAAP,CAAiBkD,OAAjB,GAA2B,SAASA,OAAT,CAAkBsC,MAAlB,EAA0BxI,KAA1B,EAAiCC,GAAjC,EAAsCwI,SAAtC,EAAiDC,OAAjD,EAA0D;AACnF,QAAItE,UAAU,CAACoE,MAAD,EAAS9J,UAAT,CAAd,EAAoC;AAClC8J,MAAAA,MAAM,GAAGnG,MAAM,CAACyB,IAAP,CAAY0E,MAAZ,EAAoBA,MAAM,CAAC9H,MAA3B,EAAmC8H,MAAM,CAACpK,UAA1C,CAAT;AACD;;AACD,QAAI,CAACiE,MAAM,CAACe,QAAP,CAAgBoF,MAAhB,CAAL,EAA8B;AAC5B,YAAM,IAAI5E,SAAJ,CACJ,qEACA,gBADA,0BAC2B4E,MAD3B,CADI,CAAN;AAID;;AAED,QAAIxI,KAAK,KAAKqD,SAAd,EAAyB;AACvBrD,MAAAA,KAAK,GAAG,CAAR;AACD;;AACD,QAAIC,GAAG,KAAKoD,SAAZ,EAAuB;AACrBpD,MAAAA,GAAG,GAAGuI,MAAM,GAAGA,MAAM,CAACzJ,MAAV,GAAmB,CAA/B;AACD;;AACD,QAAI0J,SAAS,KAAKpF,SAAlB,EAA6B;AAC3BoF,MAAAA,SAAS,GAAG,CAAZ;AACD;;AACD,QAAIC,OAAO,KAAKrF,SAAhB,EAA2B;AACzBqF,MAAAA,OAAO,GAAG,KAAK3J,MAAf;AACD;;AAED,QAAIiB,KAAK,GAAG,CAAR,IAAaC,GAAG,GAAGuI,MAAM,CAACzJ,MAA1B,IAAoC0J,SAAS,GAAG,CAAhD,IAAqDC,OAAO,GAAG,KAAK3J,MAAxE,EAAgF;AAC9E,YAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;AACD;;AAED,QAAIiF,SAAS,IAAIC,OAAb,IAAwB1I,KAAK,IAAIC,GAArC,EAA0C;AACxC,aAAO,CAAP;AACD;;AACD,QAAIwI,SAAS,IAAIC,OAAjB,EAA0B;AACxB,aAAO,CAAC,CAAR;AACD;;AACD,QAAI1I,KAAK,IAAIC,GAAb,EAAkB;AAChB,aAAO,CAAP;AACD;;AAEDD,IAAAA,KAAK,MAAM,CAAX;AACAC,IAAAA,GAAG,MAAM,CAAT;AACAwI,IAAAA,SAAS,MAAM,CAAf;AACAC,IAAAA,OAAO,MAAM,CAAb;AAEA,QAAI,SAASF,MAAb,EAAqB,OAAO,CAAP;AAErB,QAAIpC,CAAC,GAAGsC,OAAO,GAAGD,SAAlB;AACA,QAAIpC,CAAC,GAAGpG,GAAG,GAAGD,KAAd;AACA,QAAIlB,GAAG,GAAG0C,IAAI,CAAC8E,GAAL,CAASF,CAAT,EAAYC,CAAZ,CAAV;AAEA,QAAIsC,QAAQ,GAAG,KAAKrD,KAAL,CAAWmD,SAAX,EAAsBC,OAAtB,CAAf;AACA,QAAIE,UAAU,GAAGJ,MAAM,CAAClD,KAAP,CAAatF,KAAb,EAAoBC,GAApB,CAAjB;;AAEA,SAAK,IAAIpB,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyB,EAAED,CAA3B,EAA8B;AAC5B,UAAI8J,QAAQ,CAAC9J,CAAD,CAAR,KAAgB+J,UAAU,CAAC/J,CAAD,CAA9B,EAAmC;AACjCuH,QAAAA,CAAC,GAAGuC,QAAQ,CAAC9J,CAAD,CAAZ;AACAwH,QAAAA,CAAC,GAAGuC,UAAU,CAAC/J,CAAD,CAAd;AACA;AACD;AACF;;AAED,QAAIuH,CAAC,GAAGC,CAAR,EAAW,OAAO,CAAC,CAAR;AACX,QAAIA,CAAC,GAAGD,CAAR,EAAW,OAAO,CAAP;AACX,WAAO,CAAP;AACD,GA/DD;AAkEA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;;AACA,WAASyC,oBAAT,CAA+BpI,MAA/B,EAAuCqI,GAAvC,EAA4CxF,UAA5C,EAAwDyB,QAAxD,EAAkEgE,GAAlE,EAAuE;;AAErE,QAAItI,MAAM,CAAC1B,MAAP,KAAkB,CAAtB,EAAyB,OAAO,CAAC,CAAR,CAF4C;;AAKrE,QAAI,OAAOuE,UAAP,KAAsB,QAA1B,EAAoC;AAClCyB,MAAAA,QAAQ,GAAGzB,UAAX;AACAA,MAAAA,UAAU,GAAG,CAAb;AACD,KAHD,MAGO,IAAIA,UAAU,GAAG,UAAjB,EAA6B;AAClCA,MAAAA,UAAU,GAAG,UAAb;AACD,KAFM,MAEA,IAAIA,UAAU,GAAG,CAAC,UAAlB,EAA8B;AACnCA,MAAAA,UAAU,GAAG,CAAC,UAAd;AACD;;AACDA,IAAAA,UAAU,GAAG,CAACA,UAAd,CAbqE;;AAcrE,QAAIsC,WAAW,CAACtC,UAAD,CAAf,EAA6B;;AAE3BA,MAAAA,UAAU,GAAGyF,GAAG,GAAG,CAAH,GAAQtI,MAAM,CAAC1B,MAAP,GAAgB,CAAxC;AACD,KAjBoE;;;AAoBrE,QAAIuE,UAAU,GAAG,CAAjB,EAAoBA,UAAU,GAAG7C,MAAM,CAAC1B,MAAP,GAAgBuE,UAA7B;;AACpB,QAAIA,UAAU,IAAI7C,MAAM,CAAC1B,MAAzB,EAAiC;AAC/B,UAAIgK,GAAJ,EAAS,OAAO,CAAC,CAAR,CAAT,KACKzF,UAAU,GAAG7C,MAAM,CAAC1B,MAAP,GAAgB,CAA7B;AACN,KAHD,MAGO,IAAIuE,UAAU,GAAG,CAAjB,EAAoB;AACzB,UAAIyF,GAAJ,EAASzF,UAAU,GAAG,CAAb,CAAT,KACK,OAAO,CAAC,CAAR;AACN,KA3BoE;;;AA8BrE,QAAI,OAAOwF,GAAP,KAAe,QAAnB,EAA6B;AAC3BA,MAAAA,GAAG,GAAGzG,MAAM,CAACyB,IAAP,CAAYgF,GAAZ,EAAiB/D,QAAjB,CAAN;AACD,KAhCoE;;;AAmCrE,QAAI1C,MAAM,CAACe,QAAP,CAAgB0F,GAAhB,CAAJ,EAA0B;;AAExB,UAAIA,GAAG,CAAC/J,MAAJ,KAAe,CAAnB,EAAsB;AACpB,eAAO,CAAC,CAAR;AACD;;AACD,aAAOiK,YAAY,CAACvI,MAAD,EAASqI,GAAT,EAAcxF,UAAd,EAA0ByB,QAA1B,EAAoCgE,GAApC,CAAnB;AACD,KAND,MAMO,IAAI,OAAOD,GAAP,KAAe,QAAnB,EAA6B;AAClCA,MAAAA,GAAG,GAAGA,GAAG,GAAG,IAAZ,CADkC;;AAElC,UAAI,OAAOpK,UAAU,CAACsE,SAAX,CAAqB3D,OAA5B,KAAwC,UAA5C,EAAwD;AACtD,YAAI0J,GAAJ,EAAS;AACP,iBAAOrK,UAAU,CAACsE,SAAX,CAAqB3D,OAArB,CAA6BwH,IAA7B,CAAkCpG,MAAlC,EAA0CqI,GAA1C,EAA+CxF,UAA/C,CAAP;AACD,SAFD,MAEO;AACL,iBAAO5E,UAAU,CAACsE,SAAX,CAAqBiG,WAArB,CAAiCpC,IAAjC,CAAsCpG,MAAtC,EAA8CqI,GAA9C,EAAmDxF,UAAnD,CAAP;AACD;AACF;;AACD,aAAO0F,YAAY,CAACvI,MAAD,EAAS,CAACqI,GAAD,CAAT,EAAgBxF,UAAhB,EAA4ByB,QAA5B,EAAsCgE,GAAtC,CAAnB;AACD;;AAED,UAAM,IAAInF,SAAJ,CAAc,sCAAd,CAAN;AACD;;AAED,WAASoF,YAAT,CAAuBtJ,GAAvB,EAA4BoJ,GAA5B,EAAiCxF,UAAjC,EAA6CyB,QAA7C,EAAuDgE,GAAvD,EAA4D;AAC1D,QAAIG,SAAS,GAAG,CAAhB;AACA,QAAIC,SAAS,GAAGzJ,GAAG,CAACX,MAApB;AACA,QAAIqK,SAAS,GAAGN,GAAG,CAAC/J,MAApB;;AAEA,QAAIgG,QAAQ,KAAK1B,SAAjB,EAA4B;AAC1B0B,MAAAA,QAAQ,GAAGwB,MAAM,CAACxB,QAAD,CAAN,CAAiByB,WAAjB,EAAX;;AACA,UAAIzB,QAAQ,KAAK,MAAb,IAAuBA,QAAQ,KAAK,OAApC,IACAA,QAAQ,KAAK,SADb,IAC0BA,QAAQ,KAAK,UAD3C,EACuD;AACrD,YAAIrF,GAAG,CAACX,MAAJ,GAAa,CAAb,IAAkB+J,GAAG,CAAC/J,MAAJ,GAAa,CAAnC,EAAsC;AACpC,iBAAO,CAAC,CAAR;AACD;;AACDmK,QAAAA,SAAS,GAAG,CAAZ;AACAC,QAAAA,SAAS,IAAI,CAAb;AACAC,QAAAA,SAAS,IAAI,CAAb;AACA9F,QAAAA,UAAU,IAAI,CAAd;AACD;AACF;;AAED,aAAS+F,IAAT,CAAe5F,GAAf,EAAoB5E,CAApB,EAAuB;AACrB,UAAIqK,SAAS,KAAK,CAAlB,EAAqB;AACnB,eAAOzF,GAAG,CAAC5E,CAAD,CAAV;AACD,OAFD,MAEO;AACL,eAAO4E,GAAG,CAAC6F,YAAJ,CAAiBzK,CAAC,GAAGqK,SAArB,CAAP;AACD;AACF;;AAED,QAAIrK,CAAJ;;AACA,QAAIkK,GAAJ,EAAS;AACP,UAAIQ,UAAU,GAAG,CAAC,CAAlB;;AACA,WAAK1K,CAAC,GAAGyE,UAAT,EAAqBzE,CAAC,GAAGsK,SAAzB,EAAoCtK,CAAC,EAArC,EAAyC;AACvC,YAAIwK,IAAI,CAAC3J,GAAD,EAAMb,CAAN,CAAJ,KAAiBwK,IAAI,CAACP,GAAD,EAAMS,UAAU,KAAK,CAAC,CAAhB,GAAoB,CAApB,GAAwB1K,CAAC,GAAG0K,UAAlC,CAAzB,EAAwE;AACtE,cAAIA,UAAU,KAAK,CAAC,CAApB,EAAuBA,UAAU,GAAG1K,CAAb;AACvB,cAAIA,CAAC,GAAG0K,UAAJ,GAAiB,CAAjB,KAAuBH,SAA3B,EAAsC,OAAOG,UAAU,GAAGL,SAApB;AACvC,SAHD,MAGO;AACL,cAAIK,UAAU,KAAK,CAAC,CAApB,EAAuB1K,CAAC,IAAIA,CAAC,GAAG0K,UAAT;AACvBA,UAAAA,UAAU,GAAG,CAAC,CAAd;AACD;AACF;AACF,KAXD,MAWO;AACL,UAAIjG,UAAU,GAAG8F,SAAb,GAAyBD,SAA7B,EAAwC7F,UAAU,GAAG6F,SAAS,GAAGC,SAAzB;;AACxC,WAAKvK,CAAC,GAAGyE,UAAT,EAAqBzE,CAAC,IAAI,CAA1B,EAA6BA,CAAC,EAA9B,EAAkC;AAChC,YAAI2K,KAAK,GAAG,IAAZ;;AACA,aAAK,IAAIC,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGL,SAApB,EAA+BK,CAAC,EAAhC,EAAoC;AAClC,cAAIJ,IAAI,CAAC3J,GAAD,EAAMb,CAAC,GAAG4K,CAAV,CAAJ,KAAqBJ,IAAI,CAACP,GAAD,EAAMW,CAAN,CAA7B,EAAuC;AACrCD,YAAAA,KAAK,GAAG,KAAR;AACA;AACD;AACF;;AACD,YAAIA,KAAJ,EAAW,OAAO3K,CAAP;AACZ;AACF;;AAED,WAAO,CAAC,CAAR;AACD;;AAEDwD,EAAAA,MAAM,CAACW,SAAP,CAAiB0G,QAAjB,GAA4B,SAASA,QAAT,CAAmBZ,GAAnB,EAAwBxF,UAAxB,EAAoCyB,QAApC,EAA8C;AACxE,WAAO,KAAK1F,OAAL,CAAayJ,GAAb,EAAkBxF,UAAlB,EAA8ByB,QAA9B,MAA4C,CAAC,CAApD;AACD,GAFD;;AAIA1C,EAAAA,MAAM,CAACW,SAAP,CAAiB3D,OAAjB,GAA2B,SAASA,OAAT,CAAkByJ,GAAlB,EAAuBxF,UAAvB,EAAmCyB,QAAnC,EAA6C;AACtE,WAAO8D,oBAAoB,CAAC,IAAD,EAAOC,GAAP,EAAYxF,UAAZ,EAAwByB,QAAxB,EAAkC,IAAlC,CAA3B;AACD,GAFD;;AAIA1C,EAAAA,MAAM,CAACW,SAAP,CAAiBiG,WAAjB,GAA+B,SAASA,WAAT,CAAsBH,GAAtB,EAA2BxF,UAA3B,EAAuCyB,QAAvC,EAAiD;AAC9E,WAAO8D,oBAAoB,CAAC,IAAD,EAAOC,GAAP,EAAYxF,UAAZ,EAAwByB,QAAxB,EAAkC,KAAlC,CAA3B;AACD,GAFD;;AAIA,WAAS4E,QAAT,CAAmBlG,GAAnB,EAAwByB,MAAxB,EAAgCxE,MAAhC,EAAwC3B,MAAxC,EAAgD;AAC9C2B,IAAAA,MAAM,GAAGkJ,MAAM,CAAClJ,MAAD,CAAN,IAAkB,CAA3B;AACA,QAAImJ,SAAS,GAAGpG,GAAG,CAAC1E,MAAJ,GAAa2B,MAA7B;;AACA,QAAI,CAAC3B,MAAL,EAAa;AACXA,MAAAA,MAAM,GAAG8K,SAAT;AACD,KAFD,MAEO;AACL9K,MAAAA,MAAM,GAAG6K,MAAM,CAAC7K,MAAD,CAAf;;AACA,UAAIA,MAAM,GAAG8K,SAAb,EAAwB;AACtB9K,QAAAA,MAAM,GAAG8K,SAAT;AACD;AACF;;AAED,QAAIC,MAAM,GAAG5E,MAAM,CAACnG,MAApB;;AAEA,QAAIA,MAAM,GAAG+K,MAAM,GAAG,CAAtB,EAAyB;AACvB/K,MAAAA,MAAM,GAAG+K,MAAM,GAAG,CAAlB;AACD;;AACD,SAAK,IAAIjL,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4B,EAAEF,CAA9B,EAAiC;AAC/B,UAAIkL,MAAM,GAAGC,QAAQ,CAAC9E,MAAM,CAAC+E,MAAP,CAAcpL,CAAC,GAAG,CAAlB,EAAqB,CAArB,CAAD,EAA0B,EAA1B,CAArB;AACA,UAAI+G,WAAW,CAACmE,MAAD,CAAf,EAAyB,OAAOlL,CAAP;AACzB4E,MAAAA,GAAG,CAAC/C,MAAM,GAAG7B,CAAV,CAAH,GAAkBkL,MAAlB;AACD;;AACD,WAAOlL,CAAP;AACD;;AAED,WAASqL,SAAT,CAAoBzG,GAApB,EAAyByB,MAAzB,EAAiCxE,MAAjC,EAAyC3B,MAAzC,EAAiD;AAC/C,WAAOoL,UAAU,CAAClD,WAAW,CAAC/B,MAAD,EAASzB,GAAG,CAAC1E,MAAJ,GAAa2B,MAAtB,CAAZ,EAA2C+C,GAA3C,EAAgD/C,MAAhD,EAAwD3B,MAAxD,CAAjB;AACD;;AAED,WAASqL,UAAT,CAAqB3G,GAArB,EAA0ByB,MAA1B,EAAkCxE,MAAlC,EAA0C3B,MAA1C,EAAkD;AAChD,WAAOoL,UAAU,CAACE,YAAY,CAACnF,MAAD,CAAb,EAAuBzB,GAAvB,EAA4B/C,MAA5B,EAAoC3B,MAApC,CAAjB;AACD;;AAED,WAASuL,WAAT,CAAsB7G,GAAtB,EAA2ByB,MAA3B,EAAmCxE,MAAnC,EAA2C3B,MAA3C,EAAmD;AACjD,WAAOoL,UAAU,CAACjD,aAAa,CAAChC,MAAD,CAAd,EAAwBzB,GAAxB,EAA6B/C,MAA7B,EAAqC3B,MAArC,CAAjB;AACD;;AAED,WAASwL,SAAT,CAAoB9G,GAApB,EAAyByB,MAAzB,EAAiCxE,MAAjC,EAAyC3B,MAAzC,EAAiD;AAC/C,WAAOoL,UAAU,CAACK,cAAc,CAACtF,MAAD,EAASzB,GAAG,CAAC1E,MAAJ,GAAa2B,MAAtB,CAAf,EAA8C+C,GAA9C,EAAmD/C,MAAnD,EAA2D3B,MAA3D,CAAjB;AACD;;AAEDsD,EAAAA,MAAM,CAACW,SAAP,CAAiBqC,KAAjB,GAAyB,SAASA,KAAT,CAAgBH,MAAhB,EAAwBxE,MAAxB,EAAgC3B,MAAhC,EAAwCgG,QAAxC,EAAkD;;AAEzE,QAAIrE,MAAM,KAAK2C,SAAf,EAA0B;AACxB0B,MAAAA,QAAQ,GAAG,MAAX;AACAhG,MAAAA,MAAM,GAAG,KAAKA,MAAd;AACA2B,MAAAA,MAAM,GAAG,CAAT,CAHwB;AAKzB,KALD,MAKO,IAAI3B,MAAM,KAAKsE,SAAX,IAAwB,OAAO3C,MAAP,KAAkB,QAA9C,EAAwD;AAC7DqE,MAAAA,QAAQ,GAAGrE,MAAX;AACA3B,MAAAA,MAAM,GAAG,KAAKA,MAAd;AACA2B,MAAAA,MAAM,GAAG,CAAT,CAH6D;AAK9D,KALM,MAKA,IAAI+J,QAAQ,CAAC/J,MAAD,CAAZ,EAAsB;AAC3BA,MAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;AACA,UAAI+J,QAAQ,CAAC1L,MAAD,CAAZ,EAAsB;AACpBA,QAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,YAAIgG,QAAQ,KAAK1B,SAAjB,EAA4B0B,QAAQ,GAAG,MAAX;AAC7B,OAHD,MAGO;AACLA,QAAAA,QAAQ,GAAGhG,MAAX;AACAA,QAAAA,MAAM,GAAGsE,SAAT;AACD;AACF,KATM,MASA;AACL,YAAM,IAAIlE,KAAJ,CACJ,yEADI,CAAN;AAGD;;AAED,QAAI0K,SAAS,GAAG,KAAK9K,MAAL,GAAc2B,MAA9B;AACA,QAAI3B,MAAM,KAAKsE,SAAX,IAAwBtE,MAAM,GAAG8K,SAArC,EAAgD9K,MAAM,GAAG8K,SAAT;;AAEhD,QAAK3E,MAAM,CAACnG,MAAP,GAAgB,CAAhB,KAAsBA,MAAM,GAAG,CAAT,IAAc2B,MAAM,GAAG,CAA7C,CAAD,IAAqDA,MAAM,GAAG,KAAK3B,MAAvE,EAA+E;AAC7E,YAAM,IAAIyE,UAAJ,CAAe,wCAAf,CAAN;AACD;;AAED,QAAI,CAACuB,QAAL,EAAeA,QAAQ,GAAG,MAAX;AAEf,QAAIiC,WAAW,GAAG,KAAlB;;AACA,aAAS;AACP,cAAQjC,QAAR;AACE,aAAK,KAAL;AACE,iBAAO4E,QAAQ,CAAC,IAAD,EAAOzE,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAf;;AAEF,aAAK,MAAL;AACA,aAAK,OAAL;AACE,iBAAOmL,SAAS,CAAC,IAAD,EAAOhF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAhB;;AAEF,aAAK,OAAL;AACA,aAAK,QAAL;AACA,aAAK,QAAL;AACE,iBAAOqL,UAAU,CAAC,IAAD,EAAOlF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAjB;;AAEF,aAAK,QAAL;;AAEE,iBAAOuL,WAAW,CAAC,IAAD,EAAOpF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAlB;;AAEF,aAAK,MAAL;AACA,aAAK,OAAL;AACA,aAAK,SAAL;AACA,aAAK,UAAL;AACE,iBAAOwL,SAAS,CAAC,IAAD,EAAOrF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAhB;;AAEF;AACE,cAAIiI,WAAJ,EAAiB,MAAM,IAAIpD,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;AACjBA,UAAAA,QAAQ,GAAG,CAAC,KAAKA,QAAN,EAAgByB,WAAhB,EAAX;AACAQ,UAAAA,WAAW,GAAG,IAAd;AA1BJ;AA4BD;AACF,GAnED;;AAqEA3E,EAAAA,MAAM,CAACW,SAAP,CAAiB0H,MAAjB,GAA0B,SAASA,MAAT,GAAmB;AAC3C,WAAO;AACL7E,MAAAA,IAAI,EAAE,QADD;AAELE,MAAAA,IAAI,EAAEpH,KAAK,CAACqE,SAAN,CAAgBsC,KAAhB,CAAsBuB,IAAtB,CAA2B,KAAK8D,IAAL,IAAa,IAAxC,EAA8C,CAA9C;AAFD,KAAP;AAID,GALD;;AAOA,WAASnD,WAAT,CAAsB/D,GAAtB,EAA2BzD,KAA3B,EAAkCC,GAAlC,EAAuC;AACrC,QAAID,KAAK,KAAK,CAAV,IAAeC,GAAG,KAAKwD,GAAG,CAAC1E,MAA/B,EAAuC;AACrC,aAAO6L,QAAM,CAACtM,aAAP,CAAqBmF,GAArB,CAAP;AACD,KAFD,MAEO;AACL,aAAOmH,QAAM,CAACtM,aAAP,CAAqBmF,GAAG,CAAC6B,KAAJ,CAAUtF,KAAV,EAAiBC,GAAjB,CAArB,CAAP;AACD;AACF;;AAED,WAASoH,SAAT,CAAoB5D,GAApB,EAAyBzD,KAAzB,EAAgCC,GAAhC,EAAqC;AACnCA,IAAAA,GAAG,GAAGuB,IAAI,CAAC8E,GAAL,CAAS7C,GAAG,CAAC1E,MAAb,EAAqBkB,GAArB,CAAN;AACA,QAAI4K,GAAG,GAAG,EAAV;AAEA,QAAIhM,CAAC,GAAGmB,KAAR;;AACA,WAAOnB,CAAC,GAAGoB,GAAX,EAAgB;AACd,UAAI6K,SAAS,GAAGrH,GAAG,CAAC5E,CAAD,CAAnB;AACA,UAAIkM,SAAS,GAAG,IAAhB;AACA,UAAIC,gBAAgB,GAAIF,SAAS,GAAG,IAAb,GACnB,CADmB,GAElBA,SAAS,GAAG,IAAb,GACI,CADJ,GAEKA,SAAS,GAAG,IAAb,GACI,CADJ,GAEI,CANZ;;AAQA,UAAIjM,CAAC,GAAGmM,gBAAJ,IAAwB/K,GAA5B,EAAiC;AAC/B,YAAIgL,UAAJ,EAAgBC,SAAhB,EAA2BC,UAA3B,EAAuCC,aAAvC;;AAEA,gBAAQJ,gBAAR;AACE,eAAK,CAAL;AACE,gBAAIF,SAAS,GAAG,IAAhB,EAAsB;AACpBC,cAAAA,SAAS,GAAGD,SAAZ;AACD;;AACD;;AACF,eAAK,CAAL;AACEG,YAAAA,UAAU,GAAGxH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;;AACA,gBAAI,CAACoM,UAAU,GAAG,IAAd,MAAwB,IAA5B,EAAkC;AAChCG,cAAAA,aAAa,GAAG,CAACN,SAAS,GAAG,IAAb,KAAsB,GAAtB,GAA6BG,UAAU,GAAG,IAA1D;;AACA,kBAAIG,aAAa,GAAG,IAApB,EAA0B;AACxBL,gBAAAA,SAAS,GAAGK,aAAZ;AACD;AACF;;AACD;;AACF,eAAK,CAAL;AACEH,YAAAA,UAAU,GAAGxH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;AACAqM,YAAAA,SAAS,GAAGzH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAf;;AACA,gBAAI,CAACoM,UAAU,GAAG,IAAd,MAAwB,IAAxB,IAAgC,CAACC,SAAS,GAAG,IAAb,MAAuB,IAA3D,EAAiE;AAC/DE,cAAAA,aAAa,GAAG,CAACN,SAAS,GAAG,GAAb,KAAqB,GAArB,GAA2B,CAACG,UAAU,GAAG,IAAd,KAAuB,GAAlD,GAAyDC,SAAS,GAAG,IAArF;;AACA,kBAAIE,aAAa,GAAG,KAAhB,KAA0BA,aAAa,GAAG,MAAhB,IAA0BA,aAAa,GAAG,MAApE,CAAJ,EAAiF;AAC/EL,gBAAAA,SAAS,GAAGK,aAAZ;AACD;AACF;;AACD;;AACF,eAAK,CAAL;AACEH,YAAAA,UAAU,GAAGxH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;AACAqM,YAAAA,SAAS,GAAGzH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAf;AACAsM,YAAAA,UAAU,GAAG1H,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;;AACA,gBAAI,CAACoM,UAAU,GAAG,IAAd,MAAwB,IAAxB,IAAgC,CAACC,SAAS,GAAG,IAAb,MAAuB,IAAvD,IAA+D,CAACC,UAAU,GAAG,IAAd,MAAwB,IAA3F,EAAiG;AAC/FC,cAAAA,aAAa,GAAG,CAACN,SAAS,GAAG,GAAb,KAAqB,IAArB,GAA4B,CAACG,UAAU,GAAG,IAAd,KAAuB,GAAnD,GAAyD,CAACC,SAAS,GAAG,IAAb,KAAsB,GAA/E,GAAsFC,UAAU,GAAG,IAAnH;;AACA,kBAAIC,aAAa,GAAG,MAAhB,IAA0BA,aAAa,GAAG,QAA9C,EAAwD;AACtDL,gBAAAA,SAAS,GAAGK,aAAZ;AACD;AACF;;AAlCL;AAoCD;;AAED,UAAIL,SAAS,KAAK,IAAlB,EAAwB;;;AAGtBA,QAAAA,SAAS,GAAG,MAAZ;AACAC,QAAAA,gBAAgB,GAAG,CAAnB;AACD,OALD,MAKO,IAAID,SAAS,GAAG,MAAhB,EAAwB;;AAE7BA,QAAAA,SAAS,IAAI,OAAb;AACAF,QAAAA,GAAG,CAAC1K,IAAJ,CAAS4K,SAAS,KAAK,EAAd,GAAmB,KAAnB,GAA2B,MAApC;AACAA,QAAAA,SAAS,GAAG,SAASA,SAAS,GAAG,KAAjC;AACD;;AAEDF,MAAAA,GAAG,CAAC1K,IAAJ,CAAS4K,SAAT;AACAlM,MAAAA,CAAC,IAAImM,gBAAL;AACD;;AAED,WAAOK,qBAAqB,CAACR,GAAD,CAA5B;AACD;AAGD;AACA;;;AACA,MAAIS,oBAAoB,GAAG,MAA3B;;AAEA,WAASD,qBAAT,CAAgCE,UAAhC,EAA4C;AAC1C,QAAIzM,GAAG,GAAGyM,UAAU,CAACxM,MAArB;;AACA,QAAID,GAAG,IAAIwM,oBAAX,EAAiC;AAC/B,aAAO/E,MAAM,CAACiF,YAAP,CAAoBzD,KAApB,CAA0BxB,MAA1B,EAAkCgF,UAAlC,CAAP,CAD+B;AAEhC,KAJyC;;;AAO1C,QAAIV,GAAG,GAAG,EAAV;AACA,QAAIhM,CAAC,GAAG,CAAR;;AACA,WAAOA,CAAC,GAAGC,GAAX,EAAgB;AACd+L,MAAAA,GAAG,IAAItE,MAAM,CAACiF,YAAP,CAAoBzD,KAApB,CACLxB,MADK,EAELgF,UAAU,CAACjG,KAAX,CAAiBzG,CAAjB,EAAoBA,CAAC,IAAIyM,oBAAzB,CAFK,CAAP;AAID;;AACD,WAAOT,GAAP;AACD;;AAED,WAASvD,UAAT,CAAqB7D,GAArB,EAA0BzD,KAA1B,EAAiCC,GAAjC,EAAsC;AACpC,QAAIwL,GAAG,GAAG,EAAV;AACAxL,IAAAA,GAAG,GAAGuB,IAAI,CAAC8E,GAAL,CAAS7C,GAAG,CAAC1E,MAAb,EAAqBkB,GAArB,CAAN;;AAEA,SAAK,IAAIpB,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6B,EAAEpB,CAA/B,EAAkC;AAChC4M,MAAAA,GAAG,IAAIlF,MAAM,CAACiF,YAAP,CAAoB/H,GAAG,CAAC5E,CAAD,CAAH,GAAS,IAA7B,CAAP;AACD;;AACD,WAAO4M,GAAP;AACD;;AAED,WAASlE,WAAT,CAAsB9D,GAAtB,EAA2BzD,KAA3B,EAAkCC,GAAlC,EAAuC;AACrC,QAAIwL,GAAG,GAAG,EAAV;AACAxL,IAAAA,GAAG,GAAGuB,IAAI,CAAC8E,GAAL,CAAS7C,GAAG,CAAC1E,MAAb,EAAqBkB,GAArB,CAAN;;AAEA,SAAK,IAAIpB,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6B,EAAEpB,CAA/B,EAAkC;AAChC4M,MAAAA,GAAG,IAAIlF,MAAM,CAACiF,YAAP,CAAoB/H,GAAG,CAAC5E,CAAD,CAAvB,CAAP;AACD;;AACD,WAAO4M,GAAP;AACD;;AAED,WAASrE,QAAT,CAAmB3D,GAAnB,EAAwBzD,KAAxB,EAA+BC,GAA/B,EAAoC;AAClC,QAAInB,GAAG,GAAG2E,GAAG,CAAC1E,MAAd;AAEA,QAAI,CAACiB,KAAD,IAAUA,KAAK,GAAG,CAAtB,EAAyBA,KAAK,GAAG,CAAR;AACzB,QAAI,CAACC,GAAD,IAAQA,GAAG,GAAG,CAAd,IAAmBA,GAAG,GAAGnB,GAA7B,EAAkCmB,GAAG,GAAGnB,GAAN;AAElC,QAAI4M,GAAG,GAAG,EAAV;;AACA,SAAK,IAAI7M,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6B,EAAEpB,CAA/B,EAAkC;AAChC6M,MAAAA,GAAG,IAAIC,mBAAmB,CAAClI,GAAG,CAAC5E,CAAD,CAAJ,CAA1B;AACD;;AACD,WAAO6M,GAAP;AACD;;AAED,WAASjE,YAAT,CAAuBhE,GAAvB,EAA4BzD,KAA5B,EAAmCC,GAAnC,EAAwC;AACtC,QAAI2L,KAAK,GAAGnI,GAAG,CAAC6B,KAAJ,CAAUtF,KAAV,EAAiBC,GAAjB,CAAZ;AACA,QAAI4K,GAAG,GAAG,EAAV,CAFsC;;AAItC,SAAK,IAAIhM,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAG+M,KAAK,CAAC7M,MAAN,GAAe,CAAnC,EAAsCF,CAAC,IAAI,CAA3C,EAA8C;AAC5CgM,MAAAA,GAAG,IAAItE,MAAM,CAACiF,YAAP,CAAoBI,KAAK,CAAC/M,CAAD,CAAL,GAAY+M,KAAK,CAAC/M,CAAC,GAAG,CAAL,CAAL,GAAe,GAA/C,CAAP;AACD;;AACD,WAAOgM,GAAP;AACD;;AAEDxI,EAAAA,MAAM,CAACW,SAAP,CAAiBsC,KAAjB,GAAyB,SAASA,KAAT,CAAgBtF,KAAhB,EAAuBC,GAAvB,EAA4B;AACnD,QAAInB,GAAG,GAAG,KAAKC,MAAf;AACAiB,IAAAA,KAAK,GAAG,CAAC,CAACA,KAAV;AACAC,IAAAA,GAAG,GAAGA,GAAG,KAAKoD,SAAR,GAAoBvE,GAApB,GAA0B,CAAC,CAACmB,GAAlC;;AAEA,QAAID,KAAK,GAAG,CAAZ,EAAe;AACbA,MAAAA,KAAK,IAAIlB,GAAT;AACA,UAAIkB,KAAK,GAAG,CAAZ,EAAeA,KAAK,GAAG,CAAR;AAChB,KAHD,MAGO,IAAIA,KAAK,GAAGlB,GAAZ,EAAiB;AACtBkB,MAAAA,KAAK,GAAGlB,GAAR;AACD;;AAED,QAAImB,GAAG,GAAG,CAAV,EAAa;AACXA,MAAAA,GAAG,IAAInB,GAAP;AACA,UAAImB,GAAG,GAAG,CAAV,EAAaA,GAAG,GAAG,CAAN;AACd,KAHD,MAGO,IAAIA,GAAG,GAAGnB,GAAV,EAAe;AACpBmB,MAAAA,GAAG,GAAGnB,GAAN;AACD;;AAED,QAAImB,GAAG,GAAGD,KAAV,EAAiBC,GAAG,GAAGD,KAAN;AAEjB,QAAI6L,MAAM,GAAG,KAAKC,QAAL,CAAc9L,KAAd,EAAqBC,GAArB,CAAb,CArBmD;;AAuBnD6C,IAAAA,MAAM,CAACC,cAAP,CAAsB8I,MAAtB,EAA8BxJ,MAAM,CAACW,SAArC;AAEA,WAAO6I,MAAP;AACD,GA1BD;AA4BA;AACA;AACA;;;AACA,WAASE,WAAT,CAAsBrL,MAAtB,EAA8BsL,GAA9B,EAAmCjN,MAAnC,EAA2C;AACzC,QAAK2B,MAAM,GAAG,CAAV,KAAiB,CAAjB,IAAsBA,MAAM,GAAG,CAAnC,EAAsC,MAAM,IAAI8C,UAAJ,CAAe,oBAAf,CAAN;AACtC,QAAI9C,MAAM,GAAGsL,GAAT,GAAejN,MAAnB,EAA2B,MAAM,IAAIyE,UAAJ,CAAe,uCAAf,CAAN;AAC5B;;AAEDnB,EAAAA,MAAM,CAACW,SAAP,CAAiBiJ,UAAjB,GACA5J,MAAM,CAACW,SAAP,CAAiBkJ,UAAjB,GAA8B,SAASA,UAAT,CAAqBxL,MAArB,EAA6BtC,UAA7B,EAAyC+N,QAAzC,EAAmD;AAC/EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;AACA,QAAI,CAAC+N,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;AAEf,QAAI+J,GAAG,GAAG,KAAKpI,MAAL,CAAV;AACA,QAAI0L,GAAG,GAAG,CAAV;AACA,QAAIvN,CAAC,GAAG,CAAR;;AACA,WAAO,EAAEA,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;AACzCtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG7B,CAAd,IAAmBuN,GAA1B;AACD;;AAED,WAAOtD,GAAP;AACD,GAdD;;AAgBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBqJ,UAAjB,GACAhK,MAAM,CAACW,SAAP,CAAiBsJ,UAAjB,GAA8B,SAASA,UAAT,CAAqB5L,MAArB,EAA6BtC,UAA7B,EAAyC+N,QAAzC,EAAmD;AAC/EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;;AACA,QAAI,CAAC+N,QAAL,EAAe;AACbJ,MAAAA,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;AACD;;AAED,QAAI+J,GAAG,GAAG,KAAKpI,MAAM,GAAG,EAAEtC,UAAhB,CAAV;AACA,QAAIgO,GAAG,GAAG,CAAV;;AACA,WAAOhO,UAAU,GAAG,CAAb,KAAmBgO,GAAG,IAAI,KAA1B,CAAP,EAAyC;AACvCtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG,EAAEtC,UAAhB,IAA8BgO,GAArC;AACD;;AAED,WAAOtD,GAAP;AACD,GAfD;;AAiBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBuJ,SAAjB,GACAlK,MAAM,CAACW,SAAP,CAAiBwJ,SAAjB,GAA6B,SAASA,SAAT,CAAoB9L,MAApB,EAA4ByL,QAA5B,EAAsC;AACjEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AACf,WAAO,KAAK2B,MAAL,CAAP;AACD,GALD;;AAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiByJ,YAAjB,GACApK,MAAM,CAACW,SAAP,CAAiB0J,YAAjB,GAAgC,SAASA,YAAT,CAAuBhM,MAAvB,EAA+ByL,QAA/B,EAAyC;AACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AACf,WAAO,KAAK2B,MAAL,IAAgB,KAAKA,MAAM,GAAG,CAAd,KAAoB,CAA3C;AACD,GALD;;AAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB2J,YAAjB,GACAtK,MAAM,CAACW,SAAP,CAAiBsG,YAAjB,GAAgC,SAASA,YAAT,CAAuB5I,MAAvB,EAA+ByL,QAA/B,EAAyC;AACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AACf,WAAQ,KAAK2B,MAAL,KAAgB,CAAjB,GAAsB,KAAKA,MAAM,GAAG,CAAd,CAA7B;AACD,GALD;;AAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB4J,YAAjB,GACAvK,MAAM,CAACW,SAAP,CAAiB6J,YAAjB,GAAgC,SAASA,YAAT,CAAuBnM,MAAvB,EAA+ByL,QAA/B,EAAyC;AACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AAEf,WAAO,CAAE,KAAK2B,MAAL,CAAD,GACH,KAAKA,MAAM,GAAG,CAAd,KAAoB,CADjB,GAEH,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAFlB,IAGF,KAAKA,MAAM,GAAG,CAAd,IAAmB,SAHxB;AAID,GATD;;AAWA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB8J,YAAjB,GACAzK,MAAM,CAACW,SAAP,CAAiB+J,YAAjB,GAAgC,SAASA,YAAT,CAAuBrM,MAAvB,EAA+ByL,QAA/B,EAAyC;AACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AAEf,WAAQ,KAAK2B,MAAL,IAAe,SAAhB,IACH,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAArB,GACA,KAAKA,MAAM,GAAG,CAAd,KAAoB,CADpB,GAED,KAAKA,MAAM,GAAG,CAAd,CAHK,CAAP;AAID,GATD;;AAWA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBgK,SAAjB,GAA6B,SAASA,SAAT,CAAoBtM,MAApB,EAA4BtC,UAA5B,EAAwC+N,QAAxC,EAAkD;AAC7EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;AACA,QAAI,CAAC+N,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;AAEf,QAAI+J,GAAG,GAAG,KAAKpI,MAAL,CAAV;AACA,QAAI0L,GAAG,GAAG,CAAV;AACA,QAAIvN,CAAC,GAAG,CAAR;;AACA,WAAO,EAAEA,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;AACzCtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG7B,CAAd,IAAmBuN,GAA1B;AACD;;AACDA,IAAAA,GAAG,IAAI,IAAP;AAEA,QAAItD,GAAG,IAAIsD,GAAX,EAAgBtD,GAAG,IAAItH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,CAAP;AAEhB,WAAO0K,GAAP;AACD,GAhBD;;AAkBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBiK,SAAjB,GAA6B,SAASA,SAAT,CAAoBvM,MAApB,EAA4BtC,UAA5B,EAAwC+N,QAAxC,EAAkD;AAC7EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;AACA,QAAI,CAAC+N,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;AAEf,QAAIF,CAAC,GAAGT,UAAR;AACA,QAAIgO,GAAG,GAAG,CAAV;AACA,QAAItD,GAAG,GAAG,KAAKpI,MAAM,GAAG,EAAE7B,CAAhB,CAAV;;AACA,WAAOA,CAAC,GAAG,CAAJ,KAAUuN,GAAG,IAAI,KAAjB,CAAP,EAAgC;AAC9BtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG,EAAE7B,CAAhB,IAAqBuN,GAA5B;AACD;;AACDA,IAAAA,GAAG,IAAI,IAAP;AAEA,QAAItD,GAAG,IAAIsD,GAAX,EAAgBtD,GAAG,IAAItH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,CAAP;AAEhB,WAAO0K,GAAP;AACD,GAhBD;;AAkBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBkK,QAAjB,GAA4B,SAASA,QAAT,CAAmBxM,MAAnB,EAA2ByL,QAA3B,EAAqC;AAC/DzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AACf,QAAI,EAAE,KAAK2B,MAAL,IAAe,IAAjB,CAAJ,EAA4B,OAAQ,KAAKA,MAAL,CAAR;AAC5B,WAAQ,CAAC,OAAO,KAAKA,MAAL,CAAP,GAAsB,CAAvB,IAA4B,CAAC,CAArC;AACD,GALD;;AAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBmK,WAAjB,GAA+B,SAASA,WAAT,CAAsBzM,MAAtB,EAA8ByL,QAA9B,EAAwC;AACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AACf,QAAI+J,GAAG,GAAG,KAAKpI,MAAL,IAAgB,KAAKA,MAAM,GAAG,CAAd,KAAoB,CAA9C;AACA,WAAQoI,GAAG,GAAG,MAAP,GAAiBA,GAAG,GAAG,UAAvB,GAAoCA,GAA3C;AACD,GALD;;AAOAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBoK,WAAjB,GAA+B,SAASA,WAAT,CAAsB1M,MAAtB,EAA8ByL,QAA9B,EAAwC;AACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AACf,QAAI+J,GAAG,GAAG,KAAKpI,MAAM,GAAG,CAAd,IAAoB,KAAKA,MAAL,KAAgB,CAA9C;AACA,WAAQoI,GAAG,GAAG,MAAP,GAAiBA,GAAG,GAAG,UAAvB,GAAoCA,GAA3C;AACD,GALD;;AAOAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBqK,WAAjB,GAA+B,SAASA,WAAT,CAAsB3M,MAAtB,EAA8ByL,QAA9B,EAAwC;AACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AAEf,WAAQ,KAAK2B,MAAL,CAAD,GACJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,CADhB,GAEJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAFhB,GAGJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAHvB;AAID,GARD;;AAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBsK,WAAjB,GAA+B,SAASA,WAAT,CAAsB5M,MAAtB,EAA8ByL,QAA9B,EAAwC;AACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AAEf,WAAQ,KAAK2B,MAAL,KAAgB,EAAjB,GACJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,EADhB,GAEJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,CAFhB,GAGJ,KAAKA,MAAM,GAAG,CAAd,CAHH;AAID,GARD;;AAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBuK,WAAjB,GAA+B,SAASA,WAAT,CAAsB7M,MAAtB,EAA8ByL,QAA9B,EAAwC;AACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,IAA3B,EAAiC,EAAjC,EAAqC,CAArC,CAAP;AACD,GAJD;;AAMA2B,EAAAA,MAAM,CAACW,SAAP,CAAiByK,WAAjB,GAA+B,SAASA,WAAT,CAAsB/M,MAAtB,EAA8ByL,QAA9B,EAAwC;AACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,KAA3B,EAAkC,EAAlC,EAAsC,CAAtC,CAAP;AACD,GAJD;;AAMA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB0K,YAAjB,GAAgC,SAASA,YAAT,CAAuBhN,MAAvB,EAA+ByL,QAA/B,EAAyC;AACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,IAA3B,EAAiC,EAAjC,EAAqC,CAArC,CAAP;AACD,GAJD;;AAMA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB2K,YAAjB,GAAgC,SAASA,YAAT,CAAuBjN,MAAvB,EAA+ByL,QAA/B,EAAyC;AACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;AACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,KAA3B,EAAkC,EAAlC,EAAsC,CAAtC,CAAP;AACD,GAJD;;AAMA,WAASkN,QAAT,CAAmBnK,GAAnB,EAAwB/B,KAAxB,EAA+BhB,MAA/B,EAAuCsL,GAAvC,EAA4C5D,GAA5C,EAAiD9B,GAAjD,EAAsD;AACpD,QAAI,CAACjE,MAAM,CAACe,QAAP,CAAgBK,GAAhB,CAAL,EAA2B,MAAM,IAAIG,SAAJ,CAAc,6CAAd,CAAN;AAC3B,QAAIlC,KAAK,GAAG0G,GAAR,IAAe1G,KAAK,GAAG4E,GAA3B,EAAgC,MAAM,IAAI9C,UAAJ,CAAe,mCAAf,CAAN;AAChC,QAAI9C,MAAM,GAAGsL,GAAT,GAAevI,GAAG,CAAC1E,MAAvB,EAA+B,MAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;AAChC;;AAEDnB,EAAAA,MAAM,CAACW,SAAP,CAAiB6K,WAAjB,GACAxL,MAAM,CAACW,SAAP,CAAiB8K,WAAjB,GAA+B,SAASA,WAAT,CAAsBpM,KAAtB,EAA6BhB,MAA7B,EAAqCtC,UAArC,EAAiD+N,QAAjD,EAA2D;AACxFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;;AACA,QAAI,CAAC+N,QAAL,EAAe;AACb,UAAI4B,QAAQ,GAAGvM,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,IAA8B,CAA7C;AACAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkC2P,QAAlC,EAA4C,CAA5C,CAAR;AACD;;AAED,QAAI3B,GAAG,GAAG,CAAV;AACA,QAAIvN,CAAC,GAAG,CAAR;AACA,SAAK6B,MAAL,IAAegB,KAAK,GAAG,IAAvB;;AACA,WAAO,EAAE7C,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;AACzC,WAAK1L,MAAM,GAAG7B,CAAd,IAAoB6C,KAAK,GAAG0K,GAAT,GAAgB,IAAnC;AACD;;AAED,WAAO1L,MAAM,GAAGtC,UAAhB;AACD,GAlBD;;AAoBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiBgL,WAAjB,GACA3L,MAAM,CAACW,SAAP,CAAiBiL,WAAjB,GAA+B,SAASA,WAAT,CAAsBvM,KAAtB,EAA6BhB,MAA7B,EAAqCtC,UAArC,EAAiD+N,QAAjD,EAA2D;AACxFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;;AACA,QAAI,CAAC+N,QAAL,EAAe;AACb,UAAI4B,QAAQ,GAAGvM,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,IAA8B,CAA7C;AACAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkC2P,QAAlC,EAA4C,CAA5C,CAAR;AACD;;AAED,QAAIlP,CAAC,GAAGT,UAAU,GAAG,CAArB;AACA,QAAIgO,GAAG,GAAG,CAAV;AACA,SAAK1L,MAAM,GAAG7B,CAAd,IAAmB6C,KAAK,GAAG,IAA3B;;AACA,WAAO,EAAE7C,CAAF,IAAO,CAAP,KAAauN,GAAG,IAAI,KAApB,CAAP,EAAmC;AACjC,WAAK1L,MAAM,GAAG7B,CAAd,IAAoB6C,KAAK,GAAG0K,GAAT,GAAgB,IAAnC;AACD;;AAED,WAAO1L,MAAM,GAAGtC,UAAhB;AACD,GAlBD;;AAoBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiBkL,UAAjB,GACA7L,MAAM,CAACW,SAAP,CAAiBmL,UAAjB,GAA8B,SAASA,UAAT,CAAqBzM,KAArB,EAA4BhB,MAA5B,EAAoCyL,QAApC,EAA8C;AAC1EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,IAAzB,EAA+B,CAA/B,CAAR;AACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;AACA,WAAOhB,MAAM,GAAG,CAAhB;AACD,GAPD;;AASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBoL,aAAjB,GACA/L,MAAM,CAACW,SAAP,CAAiBqL,aAAjB,GAAiC,SAASA,aAAT,CAAwB3M,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;AAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAjC,CAAR;AACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;AACA,WAAOhB,MAAM,GAAG,CAAhB;AACD,GARD;;AAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBsL,aAAjB,GACAjM,MAAM,CAACW,SAAP,CAAiBuL,aAAjB,GAAiC,SAASA,aAAT,CAAwB7M,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;AAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAjC,CAAR;AACf,SAAKA,MAAL,IAAgBgB,KAAK,KAAK,CAA1B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;AACA,WAAOhB,MAAM,GAAG,CAAhB;AACD,GARD;;AAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBwL,aAAjB,GACAnM,MAAM,CAACW,SAAP,CAAiByL,aAAjB,GAAiC,SAASA,aAAT,CAAwB/M,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;AAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAArC,CAAR;AACf,SAAKA,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;AACA,SAAKhB,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;AACA,WAAOhB,MAAM,GAAG,CAAhB;AACD,GAVD;;AAYA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB0L,aAAjB,GACArM,MAAM,CAACW,SAAP,CAAiB2L,aAAjB,GAAiC,SAASA,aAAT,CAAwBjN,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;AAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAArC,CAAR;AACf,SAAKA,MAAL,IAAgBgB,KAAK,KAAK,EAA1B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;AACA,WAAOhB,MAAM,GAAG,CAAhB;AACD,GAVD;;AAYA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB4L,UAAjB,GAA8B,SAASA,UAAT,CAAqBlN,KAArB,EAA4BhB,MAA5B,EAAoCtC,UAApC,EAAgD+N,QAAhD,EAA0D;AACtFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;AACA,QAAI,CAACyL,QAAL,EAAe;AACb,UAAI0C,KAAK,GAAGrN,IAAI,CAACC,GAAL,CAAS,CAAT,EAAa,IAAIrD,UAAL,GAAmB,CAA/B,CAAZ;AAEAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkCyQ,KAAK,GAAG,CAA1C,EAA6C,CAACA,KAA9C,CAAR;AACD;;AAED,QAAIhQ,CAAC,GAAG,CAAR;AACA,QAAIuN,GAAG,GAAG,CAAV;AACA,QAAI0C,GAAG,GAAG,CAAV;AACA,SAAKpO,MAAL,IAAegB,KAAK,GAAG,IAAvB;;AACA,WAAO,EAAE7C,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;AACzC,UAAI1K,KAAK,GAAG,CAAR,IAAaoN,GAAG,KAAK,CAArB,IAA0B,KAAKpO,MAAM,GAAG7B,CAAT,GAAa,CAAlB,MAAyB,CAAvD,EAA0D;AACxDiQ,QAAAA,GAAG,GAAG,CAAN;AACD;;AACD,WAAKpO,MAAM,GAAG7B,CAAd,IAAmB,CAAE6C,KAAK,GAAG0K,GAAT,IAAiB,CAAlB,IAAuB0C,GAAvB,GAA6B,IAAhD;AACD;;AAED,WAAOpO,MAAM,GAAGtC,UAAhB;AACD,GArBD;;AAuBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiB+L,UAAjB,GAA8B,SAASA,UAAT,CAAqBrN,KAArB,EAA4BhB,MAA5B,EAAoCtC,UAApC,EAAgD+N,QAAhD,EAA0D;AACtFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;AACA,QAAI,CAACyL,QAAL,EAAe;AACb,UAAI0C,KAAK,GAAGrN,IAAI,CAACC,GAAL,CAAS,CAAT,EAAa,IAAIrD,UAAL,GAAmB,CAA/B,CAAZ;AAEAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkCyQ,KAAK,GAAG,CAA1C,EAA6C,CAACA,KAA9C,CAAR;AACD;;AAED,QAAIhQ,CAAC,GAAGT,UAAU,GAAG,CAArB;AACA,QAAIgO,GAAG,GAAG,CAAV;AACA,QAAI0C,GAAG,GAAG,CAAV;AACA,SAAKpO,MAAM,GAAG7B,CAAd,IAAmB6C,KAAK,GAAG,IAA3B;;AACA,WAAO,EAAE7C,CAAF,IAAO,CAAP,KAAauN,GAAG,IAAI,KAApB,CAAP,EAAmC;AACjC,UAAI1K,KAAK,GAAG,CAAR,IAAaoN,GAAG,KAAK,CAArB,IAA0B,KAAKpO,MAAM,GAAG7B,CAAT,GAAa,CAAlB,MAAyB,CAAvD,EAA0D;AACxDiQ,QAAAA,GAAG,GAAG,CAAN;AACD;;AACD,WAAKpO,MAAM,GAAG7B,CAAd,IAAmB,CAAE6C,KAAK,GAAG0K,GAAT,IAAiB,CAAlB,IAAuB0C,GAAvB,GAA6B,IAAhD;AACD;;AAED,WAAOpO,MAAM,GAAGtC,UAAhB;AACD,GArBD;;AAuBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiBgM,SAAjB,GAA6B,SAASA,SAAT,CAAoBtN,KAApB,EAA2BhB,MAA3B,EAAmCyL,QAAnC,EAA6C;AACxEzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,IAAzB,EAA+B,CAAC,IAAhC,CAAR;AACf,QAAIgB,KAAK,GAAG,CAAZ,EAAeA,KAAK,GAAG,OAAOA,KAAP,GAAe,CAAvB;AACf,SAAKhB,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;AACA,WAAOhB,MAAM,GAAG,CAAhB;AACD,GAPD;;AASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBiM,YAAjB,GAAgC,SAASA,YAAT,CAAuBvN,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;AAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAC,MAAlC,CAAR;AACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;AACA,WAAOhB,MAAM,GAAG,CAAhB;AACD,GAPD;;AASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBkM,YAAjB,GAAgC,SAASA,YAAT,CAAuBxN,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;AAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAC,MAAlC,CAAR;AACf,SAAKA,MAAL,IAAgBgB,KAAK,KAAK,CAA1B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;AACA,WAAOhB,MAAM,GAAG,CAAhB;AACD,GAPD;;AASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBmM,YAAjB,GAAgC,SAASA,YAAT,CAAuBzN,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;AAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAAC,UAAtC,CAAR;AACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;AACA,WAAOhB,MAAM,GAAG,CAAhB;AACD,GATD;;AAWA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBoM,YAAjB,GAAgC,SAASA,YAAT,CAAuB1N,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;AAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;AACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAAC,UAAtC,CAAR;AACf,QAAIgB,KAAK,GAAG,CAAZ,EAAeA,KAAK,GAAG,aAAaA,KAAb,GAAqB,CAA7B;AACf,SAAKhB,MAAL,IAAgBgB,KAAK,KAAK,EAA1B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;AACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;AACA,WAAOhB,MAAM,GAAG,CAAhB;AACD,GAVD;;AAYA,WAAS2O,YAAT,CAAuB5L,GAAvB,EAA4B/B,KAA5B,EAAmChB,MAAnC,EAA2CsL,GAA3C,EAAgD5D,GAAhD,EAAqD9B,GAArD,EAA0D;AACxD,QAAI5F,MAAM,GAAGsL,GAAT,GAAevI,GAAG,CAAC1E,MAAvB,EAA+B,MAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;AAC/B,QAAI9C,MAAM,GAAG,CAAb,EAAgB,MAAM,IAAI8C,UAAJ,CAAe,oBAAf,CAAN;AACjB;;AAED,WAAS8L,UAAT,CAAqB7L,GAArB,EAA0B/B,KAA1B,EAAiChB,MAAjC,EAAyC6O,YAAzC,EAAuDpD,QAAvD,EAAiE;AAC/DzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;AACA,QAAI,CAACyL,QAAL,EAAe;AACbkD,MAAAA,YAAY,CAAC5L,GAAD,EAAM/B,KAAN,EAAahB,MAAb,EAAqB,CAArB,CAAZ;AACD;;AACD8M,IAAAA,OAAO,CAACnI,KAAR,CAAc5B,GAAd,EAAmB/B,KAAnB,EAA0BhB,MAA1B,EAAkC6O,YAAlC,EAAgD,EAAhD,EAAoD,CAApD;AACA,WAAO7O,MAAM,GAAG,CAAhB;AACD;;AAED2B,EAAAA,MAAM,CAACW,SAAP,CAAiBwM,YAAjB,GAAgC,SAASA,YAAT,CAAuB9N,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;AAC9E,WAAOmD,UAAU,CAAC,IAAD,EAAO5N,KAAP,EAAchB,MAAd,EAAsB,IAAtB,EAA4ByL,QAA5B,CAAjB;AACD,GAFD;;AAIA9J,EAAAA,MAAM,CAACW,SAAP,CAAiByM,YAAjB,GAAgC,SAASA,YAAT,CAAuB/N,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;AAC9E,WAAOmD,UAAU,CAAC,IAAD,EAAO5N,KAAP,EAAchB,MAAd,EAAsB,KAAtB,EAA6ByL,QAA7B,CAAjB;AACD,GAFD;;AAIA,WAASuD,WAAT,CAAsBjM,GAAtB,EAA2B/B,KAA3B,EAAkChB,MAAlC,EAA0C6O,YAA1C,EAAwDpD,QAAxD,EAAkE;AAChEzK,IAAAA,KAAK,GAAG,CAACA,KAAT;AACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;AACA,QAAI,CAACyL,QAAL,EAAe;AACbkD,MAAAA,YAAY,CAAC5L,GAAD,EAAM/B,KAAN,EAAahB,MAAb,EAAqB,CAArB,CAAZ;AACD;;AACD8M,IAAAA,OAAO,CAACnI,KAAR,CAAc5B,GAAd,EAAmB/B,KAAnB,EAA0BhB,MAA1B,EAAkC6O,YAAlC,EAAgD,EAAhD,EAAoD,CAApD;AACA,WAAO7O,MAAM,GAAG,CAAhB;AACD;;AAED2B,EAAAA,MAAM,CAACW,SAAP,CAAiB2M,aAAjB,GAAiC,SAASA,aAAT,CAAwBjO,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;AAChF,WAAOuD,WAAW,CAAC,IAAD,EAAOhO,KAAP,EAAchB,MAAd,EAAsB,IAAtB,EAA4ByL,QAA5B,CAAlB;AACD,GAFD;;AAIA9J,EAAAA,MAAM,CAACW,SAAP,CAAiB4M,aAAjB,GAAiC,SAASA,aAAT,CAAwBlO,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;AAChF,WAAOuD,WAAW,CAAC,IAAD,EAAOhO,KAAP,EAAchB,MAAd,EAAsB,KAAtB,EAA6ByL,QAA7B,CAAlB;AACD,GAFD;;;AAKA9J,EAAAA,MAAM,CAACW,SAAP,CAAiB0C,IAAjB,GAAwB,SAASA,IAAT,CAAe8C,MAAf,EAAuBqH,WAAvB,EAAoC7P,KAApC,EAA2CC,GAA3C,EAAgD;AACtE,QAAI,CAACoC,MAAM,CAACe,QAAP,CAAgBoF,MAAhB,CAAL,EAA8B,MAAM,IAAI5E,SAAJ,CAAc,6BAAd,CAAN;AAC9B,QAAI,CAAC5D,KAAL,EAAYA,KAAK,GAAG,CAAR;AACZ,QAAI,CAACC,GAAD,IAAQA,GAAG,KAAK,CAApB,EAAuBA,GAAG,GAAG,KAAKlB,MAAX;AACvB,QAAI8Q,WAAW,IAAIrH,MAAM,CAACzJ,MAA1B,EAAkC8Q,WAAW,GAAGrH,MAAM,CAACzJ,MAArB;AAClC,QAAI,CAAC8Q,WAAL,EAAkBA,WAAW,GAAG,CAAd;AAClB,QAAI5P,GAAG,GAAG,CAAN,IAAWA,GAAG,GAAGD,KAArB,EAA4BC,GAAG,GAAGD,KAAN,CAN0C;;AAStE,QAAIC,GAAG,KAAKD,KAAZ,EAAmB,OAAO,CAAP;AACnB,QAAIwI,MAAM,CAACzJ,MAAP,KAAkB,CAAlB,IAAuB,KAAKA,MAAL,KAAgB,CAA3C,EAA8C,OAAO,CAAP,CAVwB;;AAatE,QAAI8Q,WAAW,GAAG,CAAlB,EAAqB;AACnB,YAAM,IAAIrM,UAAJ,CAAe,2BAAf,CAAN;AACD;;AACD,QAAIxD,KAAK,GAAG,CAAR,IAAaA,KAAK,IAAI,KAAKjB,MAA/B,EAAuC,MAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;AACvC,QAAIvD,GAAG,GAAG,CAAV,EAAa,MAAM,IAAIuD,UAAJ,CAAe,yBAAf,CAAN,CAjByD;;AAoBtE,QAAIvD,GAAG,GAAG,KAAKlB,MAAf,EAAuBkB,GAAG,GAAG,KAAKlB,MAAX;;AACvB,QAAIyJ,MAAM,CAACzJ,MAAP,GAAgB8Q,WAAhB,GAA8B5P,GAAG,GAAGD,KAAxC,EAA+C;AAC7CC,MAAAA,GAAG,GAAGuI,MAAM,CAACzJ,MAAP,GAAgB8Q,WAAhB,GAA8B7P,KAApC;AACD;;AAED,QAAIlB,GAAG,GAAGmB,GAAG,GAAGD,KAAhB;;AAEA,QAAI,SAASwI,MAAT,IAAmB,OAAO9J,UAAU,CAACsE,SAAX,CAAqB8M,UAA5B,KAA2C,UAAlE,EAA8E;;AAE5E,WAAKA,UAAL,CAAgBD,WAAhB,EAA6B7P,KAA7B,EAAoCC,GAApC;AACD,KAHD,MAGO;AACLvB,MAAAA,UAAU,CAACsE,SAAX,CAAqB4D,GAArB,CAAyBC,IAAzB,CACE2B,MADF,EAEE,KAAKsD,QAAL,CAAc9L,KAAd,EAAqBC,GAArB,CAFF,EAGE4P,WAHF;AAKD;;AAED,WAAO/Q,GAAP;AACD,GAvCD;AA0CA;AACA;AACA;;;AACAuD,EAAAA,MAAM,CAACW,SAAP,CAAiB8B,IAAjB,GAAwB,SAASA,IAAT,CAAegE,GAAf,EAAoB9I,KAApB,EAA2BC,GAA3B,EAAgC8E,QAAhC,EAA0C;;AAEhE,QAAI,OAAO+D,GAAP,KAAe,QAAnB,EAA6B;AAC3B,UAAI,OAAO9I,KAAP,KAAiB,QAArB,EAA+B;AAC7B+E,QAAAA,QAAQ,GAAG/E,KAAX;AACAA,QAAAA,KAAK,GAAG,CAAR;AACAC,QAAAA,GAAG,GAAG,KAAKlB,MAAX;AACD,OAJD,MAIO,IAAI,OAAOkB,GAAP,KAAe,QAAnB,EAA6B;AAClC8E,QAAAA,QAAQ,GAAG9E,GAAX;AACAA,QAAAA,GAAG,GAAG,KAAKlB,MAAX;AACD;;AACD,UAAIgG,QAAQ,KAAK1B,SAAb,IAA0B,OAAO0B,QAAP,KAAoB,QAAlD,EAA4D;AAC1D,cAAM,IAAInB,SAAJ,CAAc,2BAAd,CAAN;AACD;;AACD,UAAI,OAAOmB,QAAP,KAAoB,QAApB,IAAgC,CAAC1C,MAAM,CAAC8C,UAAP,CAAkBJ,QAAlB,CAArC,EAAkE;AAChE,cAAM,IAAInB,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;AACD;;AACD,UAAI+D,GAAG,CAAC/J,MAAJ,KAAe,CAAnB,EAAsB;AACpB,YAAIH,IAAI,GAAGkK,GAAG,CAAC9J,UAAJ,CAAe,CAAf,CAAX;;AACA,YAAK+F,QAAQ,KAAK,MAAb,IAAuBnG,IAAI,GAAG,GAA/B,IACAmG,QAAQ,KAAK,QADjB,EAC2B;;AAEzB+D,UAAAA,GAAG,GAAGlK,IAAN;AACD;AACF;AACF,KAvBD,MAuBO,IAAI,OAAOkK,GAAP,KAAe,QAAnB,EAA6B;AAClCA,MAAAA,GAAG,GAAGA,GAAG,GAAG,GAAZ;AACD,KAFM,MAEA,IAAI,OAAOA,GAAP,KAAe,SAAnB,EAA8B;AACnCA,MAAAA,GAAG,GAAGc,MAAM,CAACd,GAAD,CAAZ;AACD,KA7B+D;;;AAgChE,QAAI9I,KAAK,GAAG,CAAR,IAAa,KAAKjB,MAAL,GAAciB,KAA3B,IAAoC,KAAKjB,MAAL,GAAckB,GAAtD,EAA2D;AACzD,YAAM,IAAIuD,UAAJ,CAAe,oBAAf,CAAN;AACD;;AAED,QAAIvD,GAAG,IAAID,KAAX,EAAkB;AAChB,aAAO,IAAP;AACD;;AAEDA,IAAAA,KAAK,GAAGA,KAAK,KAAK,CAAlB;AACAC,IAAAA,GAAG,GAAGA,GAAG,KAAKoD,SAAR,GAAoB,KAAKtE,MAAzB,GAAkCkB,GAAG,KAAK,CAAhD;AAEA,QAAI,CAAC6I,GAAL,EAAUA,GAAG,GAAG,CAAN;AAEV,QAAIjK,CAAJ;;AACA,QAAI,OAAOiK,GAAP,KAAe,QAAnB,EAA6B;AAC3B,WAAKjK,CAAC,GAAGmB,KAAT,EAAgBnB,CAAC,GAAGoB,GAApB,EAAyB,EAAEpB,CAA3B,EAA8B;AAC5B,aAAKA,CAAL,IAAUiK,GAAV;AACD;AACF,KAJD,MAIO;AACL,UAAI8C,KAAK,GAAGvJ,MAAM,CAACe,QAAP,CAAgB0F,GAAhB,IACRA,GADQ,GAERzG,MAAM,CAACyB,IAAP,CAAYgF,GAAZ,EAAiB/D,QAAjB,CAFJ;AAGA,UAAIjG,GAAG,GAAG8M,KAAK,CAAC7M,MAAhB;;AACA,UAAID,GAAG,KAAK,CAAZ,EAAe;AACb,cAAM,IAAI8E,SAAJ,CAAc,gBAAgBkF,GAAhB,GAClB,mCADI,CAAN;AAED;;AACD,WAAKjK,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAGoB,GAAG,GAAGD,KAAtB,EAA6B,EAAEnB,CAA/B,EAAkC;AAChC,aAAKA,CAAC,GAAGmB,KAAT,IAAkB4L,KAAK,CAAC/M,CAAC,GAAGC,GAAL,CAAvB;AACD;AACF;;AAED,WAAO,IAAP;AACD,GAjED;AAoEA;;;AAEA,MAAIiR,iBAAiB,GAAG,mBAAxB;;AAEA,WAASC,WAAT,CAAsB7H,GAAtB,EAA2B;;AAEzBA,IAAAA,GAAG,GAAGA,GAAG,CAAC8H,KAAJ,CAAU,GAAV,EAAe,CAAf,CAAN,CAFyB;;AAIzB9H,IAAAA,GAAG,GAAGA,GAAG,CAACI,IAAJ,GAAWD,OAAX,CAAmByH,iBAAnB,EAAsC,EAAtC,CAAN,CAJyB;;AAMzB,QAAI5H,GAAG,CAACpJ,MAAJ,GAAa,CAAjB,EAAoB,OAAO,EAAP,CANK;;AAQzB,WAAOoJ,GAAG,CAACpJ,MAAJ,GAAa,CAAb,KAAmB,CAA1B,EAA6B;AAC3BoJ,MAAAA,GAAG,GAAGA,GAAG,GAAG,GAAZ;AACD;;AACD,WAAOA,GAAP;AACD;;AAED,WAASlB,WAAT,CAAsB/B,MAAtB,EAA8BgL,KAA9B,EAAqC;AACnCA,IAAAA,KAAK,GAAGA,KAAK,IAAI3O,QAAjB;AACA,QAAIwJ,SAAJ;AACA,QAAIhM,MAAM,GAAGmG,MAAM,CAACnG,MAApB;AACA,QAAIoR,aAAa,GAAG,IAApB;AACA,QAAIvE,KAAK,GAAG,EAAZ;;AAEA,SAAK,IAAI/M,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4B,EAAEF,CAA9B,EAAiC;AAC/BkM,MAAAA,SAAS,GAAG7F,MAAM,CAAClG,UAAP,CAAkBH,CAAlB,CAAZ,CAD+B;;AAI/B,UAAIkM,SAAS,GAAG,MAAZ,IAAsBA,SAAS,GAAG,MAAtC,EAA8C;;AAE5C,YAAI,CAACoF,aAAL,EAAoB;;AAElB,cAAIpF,SAAS,GAAG,MAAhB,EAAwB;;AAEtB,gBAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;AACvB;AACD,WAJD,MAIO,IAAItB,CAAC,GAAG,CAAJ,KAAUE,MAAd,EAAsB;;AAE3B,gBAAI,CAACmR,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;AACvB;AACD,WAViB;;;AAalBgQ,UAAAA,aAAa,GAAGpF,SAAhB;AAEA;AACD,SAlB2C;;;AAqB5C,YAAIA,SAAS,GAAG,MAAhB,EAAwB;AACtB,cAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;AACvBgQ,UAAAA,aAAa,GAAGpF,SAAhB;AACA;AACD,SAzB2C;;;AA4B5CA,QAAAA,SAAS,GAAG,CAACoF,aAAa,GAAG,MAAhB,IAA0B,EAA1B,GAA+BpF,SAAS,GAAG,MAA5C,IAAsD,OAAlE;AACD,OA7BD,MA6BO,IAAIoF,aAAJ,EAAmB;;AAExB,YAAI,CAACD,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;AACxB;;AAEDgQ,MAAAA,aAAa,GAAG,IAAhB,CAtC+B;;AAyC/B,UAAIpF,SAAS,GAAG,IAAhB,EAAsB;AACpB,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;AACtBtE,QAAAA,KAAK,CAACzL,IAAN,CAAW4K,SAAX;AACD,OAHD,MAGO,IAAIA,SAAS,GAAG,KAAhB,EAAuB;AAC5B,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;AACtBtE,QAAAA,KAAK,CAACzL,IAAN,CACE4K,SAAS,IAAI,GAAb,GAAmB,IADrB,EAEEA,SAAS,GAAG,IAAZ,GAAmB,IAFrB;AAID,OANM,MAMA,IAAIA,SAAS,GAAG,OAAhB,EAAyB;AAC9B,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;AACtBtE,QAAAA,KAAK,CAACzL,IAAN,CACE4K,SAAS,IAAI,GAAb,GAAmB,IADrB,EAEEA,SAAS,IAAI,GAAb,GAAmB,IAAnB,GAA0B,IAF5B,EAGEA,SAAS,GAAG,IAAZ,GAAmB,IAHrB;AAKD,OAPM,MAOA,IAAIA,SAAS,GAAG,QAAhB,EAA0B;AAC/B,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;AACtBtE,QAAAA,KAAK,CAACzL,IAAN,CACE4K,SAAS,IAAI,IAAb,GAAoB,IADtB,EAEEA,SAAS,IAAI,GAAb,GAAmB,IAAnB,GAA0B,IAF5B,EAGEA,SAAS,IAAI,GAAb,GAAmB,IAAnB,GAA0B,IAH5B,EAIEA,SAAS,GAAG,IAAZ,GAAmB,IAJrB;AAMD,OARM,MAQA;AACL,cAAM,IAAI5L,KAAJ,CAAU,oBAAV,CAAN;AACD;AACF;;AAED,WAAOyM,KAAP;AACD;;AAED,WAASvB,YAAT,CAAuBlC,GAAvB,EAA4B;AAC1B,QAAIiI,SAAS,GAAG,EAAhB;;AACA,SAAK,IAAIvR,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGsJ,GAAG,CAACpJ,MAAxB,EAAgC,EAAEF,CAAlC,EAAqC;;AAEnCuR,MAAAA,SAAS,CAACjQ,IAAV,CAAegI,GAAG,CAACnJ,UAAJ,CAAeH,CAAf,IAAoB,IAAnC;AACD;;AACD,WAAOuR,SAAP;AACD;;AAED,WAAS5F,cAAT,CAAyBrC,GAAzB,EAA8B+H,KAA9B,EAAqC;AACnC,QAAIvO,CAAJ,EAAO0O,EAAP,EAAWC,EAAX;AACA,QAAIF,SAAS,GAAG,EAAhB;;AACA,SAAK,IAAIvR,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGsJ,GAAG,CAACpJ,MAAxB,EAAgC,EAAEF,CAAlC,EAAqC;AACnC,UAAI,CAACqR,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;AAEtBvO,MAAAA,CAAC,GAAGwG,GAAG,CAACnJ,UAAJ,CAAeH,CAAf,CAAJ;AACAwR,MAAAA,EAAE,GAAG1O,CAAC,IAAI,CAAV;AACA2O,MAAAA,EAAE,GAAG3O,CAAC,GAAG,GAAT;AACAyO,MAAAA,SAAS,CAACjQ,IAAV,CAAemQ,EAAf;AACAF,MAAAA,SAAS,CAACjQ,IAAV,CAAekQ,EAAf;AACD;;AAED,WAAOD,SAAP;AACD;;AAED,WAASlJ,aAAT,CAAwBiB,GAAxB,EAA6B;AAC3B,WAAOyC,QAAM,CAACvM,WAAP,CAAmB2R,WAAW,CAAC7H,GAAD,CAA9B,CAAP;AACD;;AAED,WAASgC,UAAT,CAAqBoG,GAArB,EAA0BC,GAA1B,EAA+B9P,MAA/B,EAAuC3B,MAAvC,EAA+C;AAC7C,SAAK,IAAIF,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4B,EAAEF,CAA9B,EAAiC;AAC/B,UAAKA,CAAC,GAAG6B,MAAJ,IAAc8P,GAAG,CAACzR,MAAnB,IAA+BF,CAAC,IAAI0R,GAAG,CAACxR,MAA5C,EAAqD;AACrDyR,MAAAA,GAAG,CAAC3R,CAAC,GAAG6B,MAAL,CAAH,GAAkB6P,GAAG,CAAC1R,CAAD,CAArB;AACD;;AACD,WAAOA,CAAP;AACD;AAGD;AACA;;;AACA,WAASuF,UAAT,CAAqBuB,GAArB,EAA0BE,IAA1B,EAAgC;AAC9B,WAAOF,GAAG,YAAYE,IAAf,IACJF,GAAG,IAAI,IAAP,IAAeA,GAAG,CAAC8K,WAAJ,IAAmB,IAAlC,IAA0C9K,GAAG,CAAC8K,WAAJ,CAAgBC,IAAhB,IAAwB,IAAlE,IACC/K,GAAG,CAAC8K,WAAJ,CAAgBC,IAAhB,KAAyB7K,IAAI,CAAC6K,IAFlC;AAGD;;AACD,WAAS9K,WAAT,CAAsBD,GAAtB,EAA2B;;AAEzB,WAAOA,GAAG,KAAKA,GAAf,CAFyB;AAG1B;AAGD;;;AACA,MAAIgG,mBAAmB,GAAI,YAAY;AACrC,QAAIgF,QAAQ,GAAG,kBAAf;AACA,QAAIC,KAAK,GAAG,IAAIjS,KAAJ,CAAU,GAAV,CAAZ;;AACA,SAAK,IAAIE,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAG,EAApB,EAAwB,EAAEA,CAA1B,EAA6B;AAC3B,UAAIgS,GAAG,GAAGhS,CAAC,GAAG,EAAd;;AACA,WAAK,IAAI4K,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAG,EAApB,EAAwB,EAAEA,CAA1B,EAA6B;AAC3BmH,QAAAA,KAAK,CAACC,GAAG,GAAGpH,CAAP,CAAL,GAAiBkH,QAAQ,CAAC9R,CAAD,CAAR,GAAc8R,QAAQ,CAAClH,CAAD,CAAvC;AACD;AACF;;AACD,WAAOmH,KAAP;AACD,GAVyB,EAA1B;;;;;;;AC9wDA,iBAAe,EAAf;;ACAA;;AAoKA,IAAIE,WAAW,GAAGC,MAAM,CAACD,WAAP,IAAsB,EAAxC;;AAEEA,WAAW,CAACE,GAAZ,IACAF,WAAW,CAACG,MADZ,IAEAH,WAAW,CAACI,KAFZ,IAGAJ,WAAW,CAACK,IAHZ,IAIAL,WAAW,CAACM,SAJZ,IAKA,YAAU;AAAE,SAAQ,IAAIC,IAAJ,EAAD,CAAaC,OAAb,EAAP;AAA+B;;AC1K7C,IAAIC,QAAJ;;AACA,IAAI,OAAOzO,MAAM,CAAC0O,MAAd,KAAyB,UAA7B,EAAwC;AACtCD,EAAAA,QAAQ,GAAG,SAASA,QAAT,CAAkBE,IAAlB,EAAwBC,SAAxB,EAAmC;AAC5C;AACAD,IAAAA,IAAI,CAACE,MAAL,GAAcD,SAAd;AACAD,IAAAA,IAAI,CAACzO,SAAL,GAAiBF,MAAM,CAAC0O,MAAP,CAAcE,SAAS,CAAC1O,SAAxB,EAAmC;AAClDyN,MAAAA,WAAW,EAAE;AACX/O,QAAAA,KAAK,EAAE+P,IADI;AAEXvO,QAAAA,UAAU,EAAE,KAFD;AAGX0O,QAAAA,QAAQ,EAAE,IAHC;AAIXC,QAAAA,YAAY,EAAE;AAJH;AADqC,KAAnC,CAAjB;AAQD,GAXD;AAYD,CAbD,MAaO;AACLN,EAAAA,QAAQ,GAAG,SAASA,QAAT,CAAkBE,IAAlB,EAAwBC,SAAxB,EAAmC;AAC5CD,IAAAA,IAAI,CAACE,MAAL,GAAcD,SAAd;;AACA,QAAII,QAAQ,GAAG,SAAXA,QAAW,GAAY,EAA3B;;AACAA,IAAAA,QAAQ,CAAC9O,SAAT,GAAqB0O,SAAS,CAAC1O,SAA/B;AACAyO,IAAAA,IAAI,CAACzO,SAAL,GAAiB,IAAI8O,QAAJ,EAAjB;AACAL,IAAAA,IAAI,CAACzO,SAAL,CAAeyN,WAAf,GAA6BgB,IAA7B;AACD,GAND;AAOD;;AACD,iBAAeF,QAAf;;ACxBA;AAqBA,IAAIQ,YAAY,GAAG,UAAnB;AACO,SAASC,MAAT,CAAgBC,CAAhB,EAAmB;AACxB,MAAI,CAACC,QAAQ,CAACD,CAAD,CAAb,EAAkB;AAChB,QAAIE,OAAO,GAAG,EAAd;;AACA,SAAK,IAAItT,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGkI,SAAS,CAAChI,MAA9B,EAAsCF,CAAC,EAAvC,EAA2C;AACzCsT,MAAAA,OAAO,CAAChS,IAAR,CAAa+H,OAAO,CAACnB,SAAS,CAAClI,CAAD,CAAV,CAApB;AACD;;AACD,WAAOsT,OAAO,CAAC/R,IAAR,CAAa,GAAb,CAAP;AACD;;AAED,MAAIvB,CAAC,GAAG,CAAR;AACA,MAAIuT,IAAI,GAAGrL,SAAX;AACA,MAAIjI,GAAG,GAAGsT,IAAI,CAACrT,MAAf;AACA,MAAIoJ,GAAG,GAAG5B,MAAM,CAAC0L,CAAD,CAAN,CAAU3J,OAAV,CAAkByJ,YAAlB,EAAgC,UAAS3L,CAAT,EAAY;AACpD,QAAIA,CAAC,KAAK,IAAV,EAAgB,OAAO,GAAP;AAChB,QAAIvH,CAAC,IAAIC,GAAT,EAAc,OAAOsH,CAAP;;AACd,YAAQA,CAAR;AACE,WAAK,IAAL;AAAW,eAAOG,MAAM,CAAC6L,IAAI,CAACvT,CAAC,EAAF,CAAL,CAAb;;AACX,WAAK,IAAL;AAAW,eAAO+K,MAAM,CAACwI,IAAI,CAACvT,CAAC,EAAF,CAAL,CAAb;;AACX,WAAK,IAAL;AACE,YAAI;AACF,iBAAOwT,IAAI,CAACC,SAAL,CAAeF,IAAI,CAACvT,CAAC,EAAF,CAAnB,CAAP;AACD,SAFD,CAEE,OAAO0T,CAAP,EAAU;AACV,iBAAO,YAAP;AACD;;AACH;AACE,eAAOnM,CAAP;AAVJ;AAYD,GAfS,CAAV;;AAgBA,OAAK,IAAIA,CAAC,GAAGgM,IAAI,CAACvT,CAAD,CAAjB,EAAsBA,CAAC,GAAGC,GAA1B,EAA+BsH,CAAC,GAAGgM,IAAI,CAAC,EAAEvT,CAAH,CAAvC,EAA8C;AAC5C,QAAI2T,MAAM,CAACpM,CAAD,CAAN,IAAa,CAACqM,QAAQ,CAACrM,CAAD,CAA1B,EAA+B;AAC7B+B,MAAAA,GAAG,IAAI,MAAM/B,CAAb;AACD,KAFD,MAEO;AACL+B,MAAAA,GAAG,IAAI,MAAMD,OAAO,CAAC9B,CAAD,CAApB;AACD;AACF;;AACD,SAAO+B,GAAP;AACD;AAID;AACA;;AACO,SAASuK,SAAT,CAAmBC,EAAnB,EAAuBC,GAAvB,EAA4B;AACjC;AACA,MAAIC,WAAW,CAAC9B,MAAM,CAAC+B,OAAR,CAAf,EAAiC;AAC/B,WAAO,YAAW;AAChB,aAAOJ,SAAS,CAACC,EAAD,EAAKC,GAAL,CAAT,CAAmB7K,KAAnB,CAAyB,IAAzB,EAA+BhB,SAA/B,CAAP;AACD,KAFD;AAGD;;AAMD,MAAIgM,MAAM,GAAG,KAAb;;AACA,WAASC,UAAT,GAAsB;AACpB,QAAI,CAACD,MAAL,EAAa;AACX,MAIO;AACLrQ,QAAAA,OAAO,CAACC,KAAR,CAAciQ,GAAd;AACD;;AACDG,MAAAA,MAAM,GAAG,IAAT;AACD;;AACD,WAAOJ,EAAE,CAAC5K,KAAH,CAAS,IAAT,EAAehB,SAAf,CAAP;AACD;;AAED,SAAOiM,UAAP;AACD;AAGD,IAAIC,MAAM,GAAG,EAAb;AACA,IAAIC,YAAJ;AACO,SAASC,QAAT,CAAkBvM,GAAlB,EAAuB;AAC5B,MAAIiM,WAAW,CAACK,YAAD,CAAf,EACEA,YAAY,GAA6B,EAAzC;AACFtM,EAAAA,GAAG,GAAGA,GAAG,CAACwM,WAAJ,EAAN;;AACA,MAAI,CAACH,MAAM,CAACrM,GAAD,CAAX,EAAkB;AAChB,QAAI,IAAIyM,MAAJ,CAAW,QAAQzM,GAAR,GAAc,KAAzB,EAAgC,GAAhC,EAAqC0M,IAArC,CAA0CJ,YAA1C,CAAJ,EAA6D;AAC3D,UAAIK,GAAG,GAAG,CAAV;;AACAN,MAAAA,MAAM,CAACrM,GAAD,CAAN,GAAc,YAAW;AACvB,YAAIgM,GAAG,GAAGZ,MAAM,CAACjK,KAAP,CAAa,IAAb,EAAmBhB,SAAnB,CAAV;AACArE,QAAAA,OAAO,CAACC,KAAR,CAAc,WAAd,EAA2BiE,GAA3B,EAAgC2M,GAAhC,EAAqCX,GAArC;AACD,OAHD;AAID,KAND,MAMO;AACLK,MAAAA,MAAM,CAACrM,GAAD,CAAN,GAAc,YAAW,EAAzB;AACD;AACF;;AACD,SAAOqM,MAAM,CAACrM,GAAD,CAAb;AACD;AAGD;AACA;AACA;AACA;AACA;AACA;AACA;;AACA;;AACO,SAASsB,OAAT,CAAiBvC,GAAjB,EAAsB6N,IAAtB,EAA4B;AACjC;AACA,MAAIC,GAAG,GAAG;AACRC,IAAAA,IAAI,EAAE,EADE;AAERC,IAAAA,OAAO,EAAEC;AAFD,GAAV,CAFiC;;AAOjC,MAAI7M,SAAS,CAAChI,MAAV,IAAoB,CAAxB,EAA2B0U,GAAG,CAACI,KAAJ,GAAY9M,SAAS,CAAC,CAAD,CAArB;AAC3B,MAAIA,SAAS,CAAChI,MAAV,IAAoB,CAAxB,EAA2B0U,GAAG,CAACK,MAAJ,GAAa/M,SAAS,CAAC,CAAD,CAAtB;;AAC3B,MAAIgN,SAAS,CAACP,IAAD,CAAb,EAAqB;AACnB;AACAC,IAAAA,GAAG,CAACO,UAAJ,GAAiBR,IAAjB;AACD,GAHD,MAGO,IAAIA,IAAJ,EAAU;AACf;AACAS,IAAAA,OAAO,CAACR,GAAD,EAAMD,IAAN,CAAP;AACD,GAfgC;;;AAiBjC,MAAIX,WAAW,CAACY,GAAG,CAACO,UAAL,CAAf,EAAiCP,GAAG,CAACO,UAAJ,GAAiB,KAAjB;AACjC,MAAInB,WAAW,CAACY,GAAG,CAACI,KAAL,CAAf,EAA4BJ,GAAG,CAACI,KAAJ,GAAY,CAAZ;AAC5B,MAAIhB,WAAW,CAACY,GAAG,CAACK,MAAL,CAAf,EAA6BL,GAAG,CAACK,MAAJ,GAAa,KAAb;AAC7B,MAAIjB,WAAW,CAACY,GAAG,CAACS,aAAL,CAAf,EAAoCT,GAAG,CAACS,aAAJ,GAAoB,IAApB;AACpC,MAAIT,GAAG,CAACK,MAAR,EAAgBL,GAAG,CAACE,OAAJ,GAAcQ,gBAAd;AAChB,SAAOC,WAAW,CAACX,GAAD,EAAM9N,GAAN,EAAW8N,GAAG,CAACI,KAAf,CAAlB;AACD;;AAGD3L,OAAO,CAAC4L,MAAR,GAAiB;AACf,UAAS,CAAC,CAAD,EAAI,EAAJ,CADM;AAEf,YAAW,CAAC,CAAD,EAAI,EAAJ,CAFI;AAGf,eAAc,CAAC,CAAD,EAAI,EAAJ,CAHC;AAIf,aAAY,CAAC,CAAD,EAAI,EAAJ,CAJG;AAKf,WAAU,CAAC,EAAD,EAAK,EAAL,CALK;AAMf,UAAS,CAAC,EAAD,EAAK,EAAL,CANM;AAOf,WAAU,CAAC,EAAD,EAAK,EAAL,CAPK;AAQf,UAAS,CAAC,EAAD,EAAK,EAAL,CARM;AASf,UAAS,CAAC,EAAD,EAAK,EAAL,CATM;AAUf,WAAU,CAAC,EAAD,EAAK,EAAL,CAVK;AAWf,aAAY,CAAC,EAAD,EAAK,EAAL,CAXG;AAYf,SAAQ,CAAC,EAAD,EAAK,EAAL,CAZO;AAaf,YAAW,CAAC,EAAD,EAAK,EAAL;AAbI,CAAjB;;AAiBA5L,OAAO,CAACmM,MAAR,GAAiB;AACf,aAAW,MADI;AAEf,YAAU,QAFK;AAGf,aAAW,QAHI;AAIf,eAAa,MAJE;AAKf,UAAQ,MALO;AAMf,YAAU,OANK;AAOf,UAAQ,SAPO;AAQf;AACA,YAAU;AATK,CAAjB;;AAaA,SAASF,gBAAT,CAA0BhM,GAA1B,EAA+BmM,SAA/B,EAA0C;AACxC,MAAIC,KAAK,GAAGrM,OAAO,CAACmM,MAAR,CAAeC,SAAf,CAAZ;;AAEA,MAAIC,KAAJ,EAAW;AACT,WAAO,UAAYrM,OAAO,CAAC4L,MAAR,CAAeS,KAAf,EAAsB,CAAtB,CAAZ,GAAuC,GAAvC,GAA6CpM,GAA7C,GACA,OADA,GACYD,OAAO,CAAC4L,MAAR,CAAeS,KAAf,EAAsB,CAAtB,CADZ,GACuC,GAD9C;AAED,GAHD,MAGO;AACL,WAAOpM,GAAP;AACD;AACF;;AAGD,SAASyL,cAAT,CAAwBzL,GAAxB,EAA6BmM,SAA7B,EAAwC;AACtC,SAAOnM,GAAP;AACD;;AAGD,SAASqM,WAAT,CAAqBhP,KAArB,EAA4B;AAC1B,MAAIiP,IAAI,GAAG,EAAX;AAEAjP,EAAAA,KAAK,CAACkP,OAAN,CAAc,UAAS5L,GAAT,EAAc6L,GAAd,EAAmB;AAC/BF,IAAAA,IAAI,CAAC3L,GAAD,CAAJ,GAAY,IAAZ;AACD,GAFD;AAIA,SAAO2L,IAAP;AACD;;AAGD,SAASL,WAAT,CAAqBX,GAArB,EAA0B/R,KAA1B,EAAiCkT,YAAjC,EAA+C;AAC7C;AACA;AACA,MAAInB,GAAG,CAACS,aAAJ,IACAxS,KADA,IAEAmT,UAAU,CAACnT,KAAK,CAACwG,OAAP,CAFV;AAIAxG,EAAAA,KAAK,CAACwG,OAAN,KAAkBA,OAJlB;AAMA,IAAExG,KAAK,CAAC+O,WAAN,IAAqB/O,KAAK,CAAC+O,WAAN,CAAkBzN,SAAlB,KAAgCtB,KAAvD,CANJ,EAMmE;AACjE,QAAI+J,GAAG,GAAG/J,KAAK,CAACwG,OAAN,CAAc0M,YAAd,EAA4BnB,GAA5B,CAAV;;AACA,QAAI,CAACvB,QAAQ,CAACzG,GAAD,CAAb,EAAoB;AAClBA,MAAAA,GAAG,GAAG2I,WAAW,CAACX,GAAD,EAAMhI,GAAN,EAAWmJ,YAAX,CAAjB;AACD;;AACD,WAAOnJ,GAAP;AACD,GAf4C;;;AAkB7C,MAAIqJ,SAAS,GAAGC,eAAe,CAACtB,GAAD,EAAM/R,KAAN,CAA/B;;AACA,MAAIoT,SAAJ,EAAe;AACb,WAAOA,SAAP;AACD,GArB4C;;;AAwB7C,MAAIE,IAAI,GAAGlS,MAAM,CAACkS,IAAP,CAAYtT,KAAZ,CAAX;AACA,MAAIuT,WAAW,GAAGT,WAAW,CAACQ,IAAD,CAA7B;;AAEA,MAAIvB,GAAG,CAACO,UAAR,EAAoB;AAClBgB,IAAAA,IAAI,GAAGlS,MAAM,CAACoS,mBAAP,CAA2BxT,KAA3B,CAAP;AACD,GA7B4C;AAgC7C;;;AACA,MAAIyT,OAAO,CAACzT,KAAD,CAAP,KACIsT,IAAI,CAAC3V,OAAL,CAAa,SAAb,KAA2B,CAA3B,IAAgC2V,IAAI,CAAC3V,OAAL,CAAa,aAAb,KAA+B,CADnE,CAAJ,EAC2E;AACzE,WAAO+V,WAAW,CAAC1T,KAAD,CAAlB;AACD,GApC4C;;;AAuC7C,MAAIsT,IAAI,CAACjW,MAAL,KAAgB,CAApB,EAAuB;AACrB,QAAI8V,UAAU,CAACnT,KAAD,CAAd,EAAuB;AACrB,UAAIgP,IAAI,GAAGhP,KAAK,CAACgP,IAAN,GAAa,OAAOhP,KAAK,CAACgP,IAA1B,GAAiC,EAA5C;AACA,aAAO+C,GAAG,CAACE,OAAJ,CAAY,cAAcjD,IAAd,GAAqB,GAAjC,EAAsC,SAAtC,CAAP;AACD;;AACD,QAAI2E,QAAQ,CAAC3T,KAAD,CAAZ,EAAqB;AACnB,aAAO+R,GAAG,CAACE,OAAJ,CAAYN,MAAM,CAACrQ,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BnF,KAA/B,CAAZ,EAAmD,QAAnD,CAAP;AACD;;AACD,QAAI4T,MAAM,CAAC5T,KAAD,CAAV,EAAmB;AACjB,aAAO+R,GAAG,CAACE,OAAJ,CAAYtC,IAAI,CAACrO,SAAL,CAAegD,QAAf,CAAwBa,IAAxB,CAA6BnF,KAA7B,CAAZ,EAAiD,MAAjD,CAAP;AACD;;AACD,QAAIyT,OAAO,CAACzT,KAAD,CAAX,EAAoB;AAClB,aAAO0T,WAAW,CAAC1T,KAAD,CAAlB;AACD;AACF;;AAED,MAAI6T,IAAI,GAAG,EAAX;AAAA,MAAe/P,KAAK,GAAG,KAAvB;AAAA,MAA8BgQ,MAAM,GAAG,CAAC,GAAD,EAAM,GAAN,CAAvC,CAvD6C;;AA0D7C,MAAI1P,OAAO,CAACpE,KAAD,CAAX,EAAoB;AAClB8D,IAAAA,KAAK,GAAG,IAAR;AACAgQ,IAAAA,MAAM,GAAG,CAAC,GAAD,EAAM,GAAN,CAAT;AACD,GA7D4C;;;AAgE7C,MAAIX,UAAU,CAACnT,KAAD,CAAd,EAAuB;AACrB,QAAIiG,CAAC,GAAGjG,KAAK,CAACgP,IAAN,GAAa,OAAOhP,KAAK,CAACgP,IAA1B,GAAiC,EAAzC;AACA6E,IAAAA,IAAI,GAAG,eAAe5N,CAAf,GAAmB,GAA1B;AACD,GAnE4C;;;AAsE7C,MAAI0N,QAAQ,CAAC3T,KAAD,CAAZ,EAAqB;AACnB6T,IAAAA,IAAI,GAAG,MAAMlC,MAAM,CAACrQ,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BnF,KAA/B,CAAb;AACD,GAxE4C;;;AA2E7C,MAAI4T,MAAM,CAAC5T,KAAD,CAAV,EAAmB;AACjB6T,IAAAA,IAAI,GAAG,MAAMlE,IAAI,CAACrO,SAAL,CAAeyS,WAAf,CAA2B5O,IAA3B,CAAgCnF,KAAhC,CAAb;AACD,GA7E4C;;;AAgF7C,MAAIyT,OAAO,CAACzT,KAAD,CAAX,EAAoB;AAClB6T,IAAAA,IAAI,GAAG,MAAMH,WAAW,CAAC1T,KAAD,CAAxB;AACD;;AAED,MAAIsT,IAAI,CAACjW,MAAL,KAAgB,CAAhB,KAAsB,CAACyG,KAAD,IAAU9D,KAAK,CAAC3C,MAAN,IAAgB,CAAhD,CAAJ,EAAwD;AACtD,WAAOyW,MAAM,CAAC,CAAD,CAAN,GAAYD,IAAZ,GAAmBC,MAAM,CAAC,CAAD,CAAhC;AACD;;AAED,MAAIZ,YAAY,GAAG,CAAnB,EAAsB;AACpB,QAAIS,QAAQ,CAAC3T,KAAD,CAAZ,EAAqB;AACnB,aAAO+R,GAAG,CAACE,OAAJ,CAAYN,MAAM,CAACrQ,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BnF,KAA/B,CAAZ,EAAmD,QAAnD,CAAP;AACD,KAFD,MAEO;AACL,aAAO+R,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAP;AACD;AACF;;AAEDF,EAAAA,GAAG,CAACC,IAAJ,CAASvT,IAAT,CAAcuB,KAAd;AAEA,MAAIxB,MAAJ;;AACA,MAAIsF,KAAJ,EAAW;AACTtF,IAAAA,MAAM,GAAGwV,WAAW,CAACjC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EAAwCD,IAAxC,CAApB;AACD,GAFD,MAEO;AACL9U,IAAAA,MAAM,GAAG8U,IAAI,CAACW,GAAL,CAAS,UAASC,GAAT,EAAc;AAC9B,aAAOC,cAAc,CAACpC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EAAwCW,GAAxC,EAA6CpQ,KAA7C,CAArB;AACD,KAFQ,CAAT;AAGD;;AAEDiO,EAAAA,GAAG,CAACC,IAAJ,CAASoC,GAAT;AAEA,SAAOC,oBAAoB,CAAC7V,MAAD,EAASqV,IAAT,EAAeC,MAAf,CAA3B;AACD;;AAGD,SAAST,eAAT,CAAyBtB,GAAzB,EAA8B/R,KAA9B,EAAqC;AACnC,MAAImR,WAAW,CAACnR,KAAD,CAAf,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,WAAZ,EAAyB,WAAzB,CAAP;;AACF,MAAIzB,QAAQ,CAACxQ,KAAD,CAAZ,EAAqB;AACnB,QAAIsU,MAAM,GAAG,OAAO3D,IAAI,CAACC,SAAL,CAAe5Q,KAAf,EAAsB4G,OAAtB,CAA8B,QAA9B,EAAwC,EAAxC,EACsBA,OADtB,CAC8B,IAD9B,EACoC,KADpC,EAEsBA,OAFtB,CAE8B,MAF9B,EAEsC,GAFtC,CAAP,GAEoD,IAFjE;AAGA,WAAOmL,GAAG,CAACE,OAAJ,CAAYqC,MAAZ,EAAoB,QAApB,CAAP;AACD;;AACD,MAAIC,QAAQ,CAACvU,KAAD,CAAZ,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,KAAKjS,KAAjB,EAAwB,QAAxB,CAAP;AACF,MAAIqS,SAAS,CAACrS,KAAD,CAAb,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,KAAKjS,KAAjB,EAAwB,SAAxB,CAAP,CAZiC;;AAcnC,MAAI8Q,MAAM,CAAC9Q,KAAD,CAAV,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,MAAZ,EAAoB,MAApB,CAAP;AACH;;AAGD,SAASyB,WAAT,CAAqB1T,KAArB,EAA4B;AAC1B,SAAO,MAAMvC,KAAK,CAAC6D,SAAN,CAAgBgD,QAAhB,CAAyBa,IAAzB,CAA8BnF,KAA9B,CAAN,GAA6C,GAApD;AACD;;AAGD,SAASgU,WAAT,CAAqBjC,GAArB,EAA0B/R,KAA1B,EAAiCkT,YAAjC,EAA+CK,WAA/C,EAA4DD,IAA5D,EAAkE;AAChE,MAAI9U,MAAM,GAAG,EAAb;;AACA,OAAK,IAAIrB,CAAC,GAAG,CAAR,EAAWqX,CAAC,GAAGxU,KAAK,CAAC3C,MAA1B,EAAkCF,CAAC,GAAGqX,CAAtC,EAAyC,EAAErX,CAA3C,EAA8C;AAC5C,QAAIsX,cAAc,CAACzU,KAAD,EAAQ6E,MAAM,CAAC1H,CAAD,CAAd,CAAlB,EAAsC;AACpCqB,MAAAA,MAAM,CAACC,IAAP,CAAY0V,cAAc,CAACpC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EACtB1O,MAAM,CAAC1H,CAAD,CADgB,EACX,IADW,CAA1B;AAED,KAHD,MAGO;AACLqB,MAAAA,MAAM,CAACC,IAAP,CAAY,EAAZ;AACD;AACF;;AACD6U,EAAAA,IAAI,CAACN,OAAL,CAAa,UAASkB,GAAT,EAAc;AACzB,QAAI,CAACA,GAAG,CAACQ,KAAJ,CAAU,OAAV,CAAL,EAAyB;AACvBlW,MAAAA,MAAM,CAACC,IAAP,CAAY0V,cAAc,CAACpC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EACtBW,GADsB,EACjB,IADiB,CAA1B;AAED;AACF,GALD;AAMA,SAAO1V,MAAP;AACD;;AAGD,SAAS2V,cAAT,CAAwBpC,GAAxB,EAA6B/R,KAA7B,EAAoCkT,YAApC,EAAkDK,WAAlD,EAA+DW,GAA/D,EAAoEpQ,KAApE,EAA2E;AACzE,MAAIkL,IAAJ,EAAUvI,GAAV,EAAekO,IAAf;AACAA,EAAAA,IAAI,GAAGvT,MAAM,CAACwT,wBAAP,CAAgC5U,KAAhC,EAAuCkU,GAAvC,KAA+C;AAAElU,IAAAA,KAAK,EAAEA,KAAK,CAACkU,GAAD;AAAd,GAAtD;;AACA,MAAIS,IAAI,CAAClT,GAAT,EAAc;AACZ,QAAIkT,IAAI,CAACzP,GAAT,EAAc;AACZuB,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,iBAAZ,EAA+B,SAA/B,CAAN;AACD,KAFD,MAEO;AACLxL,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAN;AACD;AACF,GAND,MAMO;AACL,QAAI0C,IAAI,CAACzP,GAAT,EAAc;AACZuB,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAN;AACD;AACF;;AACD,MAAI,CAACwC,cAAc,CAAClB,WAAD,EAAcW,GAAd,CAAnB,EAAuC;AACrClF,IAAAA,IAAI,GAAG,MAAMkF,GAAN,GAAY,GAAnB;AACD;;AACD,MAAI,CAACzN,GAAL,EAAU;AACR,QAAIsL,GAAG,CAACC,IAAJ,CAASrU,OAAT,CAAiBgX,IAAI,CAAC3U,KAAtB,IAA+B,CAAnC,EAAsC;AACpC,UAAI8Q,MAAM,CAACoC,YAAD,CAAV,EAA0B;AACxBzM,QAAAA,GAAG,GAAGiM,WAAW,CAACX,GAAD,EAAM4C,IAAI,CAAC3U,KAAX,EAAkB,IAAlB,CAAjB;AACD,OAFD,MAEO;AACLyG,QAAAA,GAAG,GAAGiM,WAAW,CAACX,GAAD,EAAM4C,IAAI,CAAC3U,KAAX,EAAkBkT,YAAY,GAAG,CAAjC,CAAjB;AACD;;AACD,UAAIzM,GAAG,CAAC9I,OAAJ,CAAY,IAAZ,IAAoB,CAAC,CAAzB,EAA4B;AAC1B,YAAImG,KAAJ,EAAW;AACT2C,UAAAA,GAAG,GAAGA,GAAG,CAAC8H,KAAJ,CAAU,IAAV,EAAgB0F,GAAhB,CAAoB,UAASY,IAAT,EAAe;AACvC,mBAAO,OAAOA,IAAd;AACD,WAFK,EAEHnW,IAFG,CAEE,IAFF,EAEQ6J,MAFR,CAEe,CAFf,CAAN;AAGD,SAJD,MAIO;AACL9B,UAAAA,GAAG,GAAG,OAAOA,GAAG,CAAC8H,KAAJ,CAAU,IAAV,EAAgB0F,GAAhB,CAAoB,UAASY,IAAT,EAAe;AAC9C,mBAAO,QAAQA,IAAf;AACD,WAFY,EAEVnW,IAFU,CAEL,IAFK,CAAb;AAGD;AACF;AACF,KAjBD,MAiBO;AACL+H,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,YAAZ,EAA0B,SAA1B,CAAN;AACD;AACF;;AACD,MAAId,WAAW,CAACnC,IAAD,CAAf,EAAuB;AACrB,QAAIlL,KAAK,IAAIoQ,GAAG,CAACQ,KAAJ,CAAU,OAAV,CAAb,EAAiC;AAC/B,aAAOjO,GAAP;AACD;;AACDuI,IAAAA,IAAI,GAAG2B,IAAI,CAACC,SAAL,CAAe,KAAKsD,GAApB,CAAP;;AACA,QAAIlF,IAAI,CAAC0F,KAAL,CAAW,8BAAX,CAAJ,EAAgD;AAC9C1F,MAAAA,IAAI,GAAGA,IAAI,CAACzG,MAAL,CAAY,CAAZ,EAAeyG,IAAI,CAAC3R,MAAL,GAAc,CAA7B,CAAP;AACA2R,MAAAA,IAAI,GAAG+C,GAAG,CAACE,OAAJ,CAAYjD,IAAZ,EAAkB,MAAlB,CAAP;AACD,KAHD,MAGO;AACLA,MAAAA,IAAI,GAAGA,IAAI,CAACpI,OAAL,CAAa,IAAb,EAAmB,KAAnB,EACKA,OADL,CACa,MADb,EACqB,GADrB,EAEKA,OAFL,CAEa,UAFb,EAEyB,GAFzB,CAAP;AAGAoI,MAAAA,IAAI,GAAG+C,GAAG,CAACE,OAAJ,CAAYjD,IAAZ,EAAkB,QAAlB,CAAP;AACD;AACF;;AAED,SAAOA,IAAI,GAAG,IAAP,GAAcvI,GAArB;AACD;;AAGD,SAAS4N,oBAAT,CAA8B7V,MAA9B,EAAsCqV,IAAtC,EAA4CC,MAA5C,EAAoD;AAElD,MAAIzW,MAAM,GAAGmB,MAAM,CAACsW,MAAP,CAAc,UAASC,IAAT,EAAeC,GAAf,EAAoB;AAE7C,QAAIA,GAAG,CAACrX,OAAJ,CAAY,IAAZ,KAAqB,CAAzB,EAA4BsX;AAC5B,WAAOF,IAAI,GAAGC,GAAG,CAACpO,OAAJ,CAAY,iBAAZ,EAA+B,EAA/B,EAAmCvJ,MAA1C,GAAmD,CAA1D;AACD,GAJY,EAIV,CAJU,CAAb;;AAMA,MAAIA,MAAM,GAAG,EAAb,EAAiB;AACf,WAAOyW,MAAM,CAAC,CAAD,CAAN,IACCD,IAAI,KAAK,EAAT,GAAc,EAAd,GAAmBA,IAAI,GAAG,KAD3B,IAEA,GAFA,GAGArV,MAAM,CAACE,IAAP,CAAY,OAAZ,CAHA,GAIA,GAJA,GAKAoV,MAAM,CAAC,CAAD,CALb;AAMD;;AAED,SAAOA,MAAM,CAAC,CAAD,CAAN,GAAYD,IAAZ,GAAmB,GAAnB,GAAyBrV,MAAM,CAACE,IAAP,CAAY,IAAZ,CAAzB,GAA6C,GAA7C,GAAmDoV,MAAM,CAAC,CAAD,CAAhE;AACD;AAID;;;AACO,SAAS1P,OAAT,CAAiB8Q,EAAjB,EAAqB;AAC1B,SAAOjY,KAAK,CAACmH,OAAN,CAAc8Q,EAAd,CAAP;AACD;AAEM,SAAS7C,SAAT,CAAmBrQ,GAAnB,EAAwB;AAC7B,SAAO,OAAOA,GAAP,KAAe,SAAtB;AACD;AAEM,SAAS8O,MAAT,CAAgB9O,GAAhB,EAAqB;AAC1B,SAAOA,GAAG,KAAK,IAAf;AACD;AAEM,SAASmT,iBAAT,CAA2BnT,GAA3B,EAAgC;AACrC,SAAOA,GAAG,IAAI,IAAd;AACD;AAEM,SAASuS,QAAT,CAAkBvS,GAAlB,EAAuB;AAC5B,SAAO,OAAOA,GAAP,KAAe,QAAtB;AACD;AAEM,SAASwO,QAAT,CAAkBxO,GAAlB,EAAuB;AAC5B,SAAO,OAAOA,GAAP,KAAe,QAAtB;AACD;AAEM,SAASoT,QAAT,CAAkBpT,GAAlB,EAAuB;AAC5B,SAAO,uBAAOA,GAAP,MAAe,QAAtB;AACD;AAEM,SAASmP,WAAT,CAAqBnP,GAArB,EAA0B;AAC/B,SAAOA,GAAG,KAAK,KAAK,CAApB;AACD;AAEM,SAAS2R,QAAT,CAAkB0B,EAAlB,EAAsB;AAC3B,SAAOtE,QAAQ,CAACsE,EAAD,CAAR,IAAgBC,cAAc,CAACD,EAAD,CAAd,KAAuB,iBAA9C;AACD;AAEM,SAAStE,QAAT,CAAkB/O,GAAlB,EAAuB;AAC5B,SAAO,uBAAOA,GAAP,MAAe,QAAf,IAA2BA,GAAG,KAAK,IAA1C;AACD;AAEM,SAAS4R,MAAT,CAAgBlU,CAAhB,EAAmB;AACxB,SAAOqR,QAAQ,CAACrR,CAAD,CAAR,IAAe4V,cAAc,CAAC5V,CAAD,CAAd,KAAsB,eAA5C;AACD;AAEM,SAAS+T,OAAT,CAAiBrU,CAAjB,EAAoB;AACzB,SAAO2R,QAAQ,CAAC3R,CAAD,CAAR,KACFkW,cAAc,CAAClW,CAAD,CAAd,KAAsB,gBAAtB,IAA0CA,CAAC,YAAY3B,KADrD,CAAP;AAED;AAEM,SAAS0V,UAAT,CAAoBnR,GAApB,EAAyB;AAC9B,SAAO,OAAOA,GAAP,KAAe,UAAtB;AACD;AAEM,SAASuT,WAAT,CAAqBvT,GAArB,EAA0B;AAC/B,SAAOA,GAAG,KAAK,IAAR,IACA,OAAOA,GAAP,KAAe,SADf,IAEA,OAAOA,GAAP,KAAe,QAFf,IAGA,OAAOA,GAAP,KAAe,QAHf,IAIA,uBAAOA,GAAP,MAAe,QAJf;AAKA,SAAOA,GAAP,KAAe,WALtB;AAMD;AAEM,SAASN,QAAT,CAAkB8T,QAAlB,EAA4B;AACjC,SAAO7U,MAAM,CAACe,QAAP,CAAgB8T,QAAhB,CAAP;AACD;;AAED,SAASF,cAAT,CAAwBG,CAAxB,EAA2B;AACzB,SAAOrU,MAAM,CAACE,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BsQ,CAA/B,CAAP;AACD;;AAGD,SAASC,GAAT,CAAazP,CAAb,EAAgB;AACd,SAAOA,CAAC,GAAG,EAAJ,GAAS,MAAMA,CAAC,CAAC3B,QAAF,CAAW,EAAX,CAAf,GAAgC2B,CAAC,CAAC3B,QAAF,CAAW,EAAX,CAAvC;AACD;;AAGD,IAAIqR,MAAM,GAAG,CAAC,KAAD,EAAQ,KAAR,EAAe,KAAf,EAAsB,KAAtB,EAA6B,KAA7B,EAAoC,KAApC,EAA2C,KAA3C,EAAkD,KAAlD,EAAyD,KAAzD,EACC,KADD,EACQ,KADR,EACe,KADf,CAAb;;AAIA,SAASC,WAAT,GAAqB;AACnB,MAAIlW,CAAC,GAAG,IAAIiQ,IAAJ,EAAR;AACA,MAAIkG,IAAI,GAAG,CAACH,GAAG,CAAChW,CAAC,CAACoW,QAAF,EAAD,CAAJ,EACCJ,GAAG,CAAChW,CAAC,CAACqW,UAAF,EAAD,CADJ,EAECL,GAAG,CAAChW,CAAC,CAACsW,UAAF,EAAD,CAFJ,EAEsBtX,IAFtB,CAE2B,GAF3B,CAAX;AAGA,SAAO,CAACgB,CAAC,CAACuW,OAAF,EAAD,EAAcN,MAAM,CAACjW,CAAC,CAACwW,QAAF,EAAD,CAApB,EAAoCL,IAApC,EAA0CnX,IAA1C,CAA+C,GAA/C,CAAP;AACD;;;AAIM,SAAS4B,GAAT,GAAe;AACpBU,EAAAA,OAAO,CAACV,GAAR,CAAY,SAAZ,EAAuBsV,WAAS,EAAhC,EAAoCtF,MAAM,CAACjK,KAAP,CAAa,IAAb,EAAmBhB,SAAnB,CAApC;AACD;AAmBM,SAASkN,OAAT,CAAiB4D,MAAjB,EAAyBC,GAAzB,EAA8B;AACnC;AACA,MAAI,CAACA,GAAD,IAAQ,CAACrF,QAAQ,CAACqF,GAAD,CAArB,EAA4B,OAAOD,MAAP;AAE5B,MAAI7C,IAAI,GAAGlS,MAAM,CAACkS,IAAP,CAAY8C,GAAZ,CAAX;AACA,MAAIjZ,CAAC,GAAGmW,IAAI,CAACjW,MAAb;;AACA,SAAOF,CAAC,EAAR,EAAY;AACVgZ,IAAAA,MAAM,CAAC7C,IAAI,CAACnW,CAAD,CAAL,CAAN,GAAkBiZ,GAAG,CAAC9C,IAAI,CAACnW,CAAD,CAAL,CAArB;AACD;;AACD,SAAOgZ,MAAP;AACD;;AAED,SAAS1B,cAAT,CAAwBxQ,GAAxB,EAA6BoS,IAA7B,EAAmC;AACjC,SAAOjV,MAAM,CAACE,SAAP,CAAiBmT,cAAjB,CAAgCtP,IAAhC,CAAqClB,GAArC,EAA0CoS,IAA1C,CAAP;AACD;;AAED,iBAAe;AACbxG,EAAAA,QAAQ,EAAEA,UADG;AAEb0C,EAAAA,OAAO,EAAEA,OAFI;AAGbjS,EAAAA,GAAG,EAAEA,GAHQ;AAIboB,EAAAA,QAAQ,EAAEA,QAJG;AAKb6T,EAAAA,WAAW,EAAEA,WALA;AAMbpC,EAAAA,UAAU,EAAEA,UANC;AAObM,EAAAA,OAAO,EAAEA,OAPI;AAQbG,EAAAA,MAAM,EAAEA,MARK;AASb7C,EAAAA,QAAQ,EAAEA,QATG;AAUb4C,EAAAA,QAAQ,EAAEA,QAVG;AAWbxC,EAAAA,WAAW,EAAEA,WAXA;AAYbiE,EAAAA,QAAQ,EAAEA,QAZG;AAab5E,EAAAA,QAAQ,EAAEA,QAbG;AAcb+D,EAAAA,QAAQ,EAAEA,QAdG;AAebY,EAAAA,iBAAiB,EAAEA,iBAfN;AAgBbrE,EAAAA,MAAM,EAAEA,MAhBK;AAiBbuB,EAAAA,SAAS,EAAEA,SAjBE;AAkBbjO,EAAAA,OAAO,EAAEA,OAlBI;AAmBboC,EAAAA,OAAO,EAAEA,OAnBI;AAoBbwK,EAAAA,SAAS,EAAEA,SApBE;AAqBbV,EAAAA,MAAM,EAAEA,MArBK;AAsBbmB,EAAAA,QAAQ,EAAEA;AAtBG,CAAf;;;;;AC9jBgC;AAIhC;;;;AAIA,SAAgB,wBAAwB,CAAC,EAAY;IACnD,OAAO,EAAE,CAAC,QAAQ,EAAE,CAAC,OAAO,CAAC,WAAW,EAAE,YAAY,CAAC,CAAC;AAC1D,CAAC;AAFD,4DAEC;AAED,IAAM,aAAa,GACjB,OAAOpC,cAAM,CAAC,SAAS,KAAK,QAAQ,IAAIA,cAAM,CAAC,SAAS,CAAC,OAAO,KAAK,aAAa,CAAC;AAErF,IAAM,eAAe,GAAG,aAAa;MACjC,0IAA0I;MAC1I,+GAA+G,CAAC;AAEpH,IAAM,mBAAmB,GAAwB,SAAS,mBAAmB,CAAC,IAAY;IACxF,OAAO,CAAC,IAAI,CAAC,eAAe,CAAC,CAAC;IAE9B,IAAM,MAAM,GAAGiH,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;IAClC,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,EAAE,EAAE,CAAC;QAAE,MAAM,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,MAAM,EAAE,GAAG,GAAG,CAAC,CAAC;IAC3E,OAAO,MAAM,CAAC;AAChB,CAAC,CAAC;AAUF,IAAM,iBAAiB,GAAG;IACxB,IAAI,OAAO,MAAM,KAAK,WAAW,EAAE;;QAEjC,IAAM,QAAM,GAAG,MAAM,CAAC,MAAM,IAAI,MAAM,CAAC,QAAQ,CAAC;QAChD,IAAI,QAAM,IAAI,QAAM,CAAC,eAAe,EAAE;YACpC,OAAO,UAAA,IAAI,IAAI,OAAA,QAAM,CAAC,eAAe,CAACA,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,GAAA,CAAC;SAC3D;KACF;IAED,IAAI,OAAOjH,cAAM,KAAK,WAAW,IAAIA,cAAM,CAAC,MAAM,IAAIA,cAAM,CAAC,MAAM,CAAC,eAAe,EAAE;;QAEnF,OAAO,UAAA,IAAI,IAAI,OAAAA,cAAM,CAAC,MAAM,CAAC,eAAe,CAACiH,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,GAAA,CAAC;KAClE;IAED,IAAI,mBAA2D,CAAC;IAChE,IAAI;;QAEF,mBAAmB,GAAG,UAAiB,CAAC,WAAW,CAAC;KACrD;IAAC,OAAO,CAAC,EAAE;;KAEX;;IAID,OAAO,mBAAmB,IAAI,mBAAmB,CAAC;AACpD,CAAC,CAAC;AAEW,mBAAW,GAAG,iBAAiB,EAAE,CAAC;AAE/C,SAAgB,gBAAgB,CAAC,KAAc;IAC7C,OAAO,CAAC,sBAAsB,EAAE,4BAA4B,CAAC,CAAC,QAAQ,CACpE,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CACtC,CAAC;AACJ,CAAC;AAJD,4CAIC;AAED,SAAgB,YAAY,CAAC,KAAc;IACzC,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,qBAAqB,CAAC;AACzE,CAAC;AAFD,oCAEC;AAED,SAAgB,eAAe,CAAC,KAAc;IAC5C,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,wBAAwB,CAAC;AAC5E,CAAC;AAFD,0CAEC;AAED,SAAgB,gBAAgB,CAAC,KAAc;IAC7C,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,yBAAyB,CAAC;AAC7E,CAAC;AAFD,4CAEC;AAED,SAAgB,QAAQ,CAAC,CAAU;IACjC,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,iBAAiB,CAAC;AACjE,CAAC;AAFD,4BAEC;AAED,SAAgB,KAAK,CAAC,CAAU;IAC9B,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,cAAc,CAAC;AAC9D,CAAC;AAFD,sBAEC;AAED;AACA,SAAgB,UAAU;IACxB,OAAO,OAAOjH,cAAM,KAAK,WAAW,IAAI,OAAOA,cAAM,CAAC,MAAM,KAAK,WAAW,CAAC;AAC/E,CAAC;AAFD,gCAEC;AAED;AACA,SAAgB,MAAM,CAAC,CAAU;IAC/B,OAAO,YAAY,CAAC,CAAC,CAAC,IAAI,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,eAAe,CAAC;AAClF,CAAC;AAFD,wBAEC;AAED;;;;;AAKA,SAAgB,YAAY,CAAC,SAAkB;IAC7C,OAAO,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,KAAK,IAAI,CAAC;AAC7D,CAAC;AAFD,oCAEC;AAGD,SAAgB,SAAS,CAAqB,EAAK,EAAE,OAAe;IAClE,IAAI,OAAO,MAAM,KAAK,WAAW,IAAI,OAAO,IAAI,KAAK,WAAW,EAAE;;QAEhE,OAAO,UAAe,CAAC,SAAS,CAAC,EAAE,EAAE,OAAO,CAAC,CAAC;KAC/C;IACD,IAAI,MAAM,GAAG,KAAK,CAAC;IACnB,SAAS,UAAU;QAAgB,cAAkB;aAAlB,UAAkB,EAAlB,qBAAkB,EAAlB,IAAkB;YAAlB,yBAAkB;;QACnD,IAAI,CAAC,MAAM,EAAE;YACX,OAAO,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC;YACtB,MAAM,GAAG,IAAI,CAAC;SACf;QACD,OAAO,EAAE,CAAC,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;KAC7B;IACD,OAAQ,UAA2B,CAAC;AACtC,CAAC;AAdD,8BAcC;;;;;;;;;;;;;;;;;;;;;AC5H+B;AACkB;AAElD;;;;;;;;AAQA,SAAgB,YAAY,CAAC,eAAuD;IAClF,IAAI,WAAW,CAAC,MAAM,CAAC,eAAe,CAAC,EAAE;QACvC,OAAOiH,aAAM,CAAC,IAAI,CAChB,eAAe,CAAC,MAAM,EACtB,eAAe,CAAC,UAAU,EAC1B,eAAe,CAAC,UAAU,CAC3B,CAAC;KACH;IAED,IAAIC,sBAAgB,CAAC,eAAe,CAAC,EAAE;QACrC,OAAOD,aAAM,CAAC,IAAI,CAAC,eAAe,CAAC,CAAC;KACrC;IAED,MAAM,IAAI,SAAS,CAAC,sCAAsC,CAAC,CAAC;AAC9D,CAAC;AAdD,oCAcC;;;;;;;;;;ACzB+B;AAEhC;AACA,IAAM,gBAAgB,GAAG,uHAAuH,CAAC;AAE1I,IAAM,kBAAkB,GAAG,UAAC,GAAW;IAC5C,OAAA,OAAO,GAAG,KAAK,QAAQ,IAAI,gBAAgB,CAAC,IAAI,CAAC,GAAG,CAAC;AAArD,CAAqD,CAAC;AAD3C,0BAAkB,sBACyB;AAEjD,IAAM,qBAAqB,GAAG,UAAC,SAAiB;IACrD,IAAI,CAAC,0BAAkB,CAAC,SAAS,CAAC,EAAE;QAClC,MAAM,IAAI,SAAS,CACjB,uLAAuL,CACxL,CAAC;KACH;IAED,IAAM,kBAAkB,GAAG,SAAS,CAAC,OAAO,CAAC,IAAI,EAAE,EAAE,CAAC,CAAC;IACvD,OAAOA,aAAM,CAAC,IAAI,CAAC,kBAAkB,EAAE,KAAK,CAAC,CAAC;AAChD,CAAC,CAAC;AATW,6BAAqB,yBAShC;AAEK,IAAM,qBAAqB,GAAG,UAAC,MAAc,EAAE,aAAoB;IAApB,8BAAA,EAAA,oBAAoB;IACxE,OAAA,aAAa;UACT,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;YAC5B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;YAC5B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;YAC5B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,EAAE,CAAC;YAC7B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,EAAE,EAAE,EAAE,CAAC;UAC9B,MAAM,CAAC,QAAQ,CAAC,KAAK,CAAC;AAV1B,CAU0B,CAAC;AAXhB,6BAAqB,yBAWL;;;;;;;;;;;;AC9BG;AACe;AACb;AAC8D;AACrC;AAO3D,IAAM,WAAW,GAAG,EAAE,CAAC;AAEvB,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;AAEzB;;;;AAIA;;;;;;IAgBE,cAAY,KAA8B;QACxC,IAAI,OAAO,KAAK,KAAK,WAAW,EAAE;;YAEhC,IAAI,CAAC,EAAE,GAAG,IAAI,CAAC,QAAQ,EAAE,CAAC;SAC3B;aAAM,IAAI,KAAK,YAAY,IAAI,EAAE;YAChC,IAAI,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;YAClC,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,CAAC;SACxB;aAAM,IAAI,WAAW,CAAC,MAAM,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,UAAU,KAAK,WAAW,EAAE;YACxE,IAAI,CAAC,EAAE,GAAGE,0BAAY,CAAC,KAAK,CAAC,CAAC;SAC/B;aAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YACpC,IAAI,CAAC,EAAE,GAAGC,gCAAqB,CAAC,KAAK,CAAC,CAAC;SACxC;aAAM;YACL,MAAM,IAAI,SAAS,CACjB,gLAAgL,CACjL,CAAC;SACH;KACF;IAMD,sBAAI,oBAAE;;;;;aAAN;YACE,OAAO,IAAI,CAAC,GAAG,CAAC,CAAC;SAClB;aAED,UAAO,KAAa;YAClB,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;YAElB,IAAI,IAAI,CAAC,cAAc,EAAE;gBACvB,IAAI,CAAC,IAAI,GAAGA,gCAAqB,CAAC,KAAK,CAAC,CAAC;aAC1C;SACF;;;OARA;;;;;;;;IAkBD,0BAAW,GAAX,UAAY,aAAoB;QAApB,8BAAA,EAAA,oBAAoB;QAC9B,IAAI,IAAI,CAAC,cAAc,IAAI,IAAI,CAAC,IAAI,EAAE;YACpC,OAAO,IAAI,CAAC,IAAI,CAAC;SAClB;QAED,IAAM,aAAa,GAAGA,gCAAqB,CAAC,IAAI,CAAC,EAAE,EAAE,aAAa,CAAC,CAAC;QAEpE,IAAI,IAAI,CAAC,cAAc,EAAE;YACvB,IAAI,CAAC,IAAI,GAAG,aAAa,CAAC;SAC3B;QAED,OAAO,aAAa,CAAC;KACtB;;;;;IAMD,uBAAQ,GAAR,UAAS,QAAiB;QACxB,OAAO,QAAQ,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,QAAQ,CAAC,GAAG,IAAI,CAAC,WAAW,EAAE,CAAC;KACnE;;;;;IAMD,qBAAM,GAAN;QACE,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;KAC3B;;;;;;IAOD,qBAAM,GAAN,UAAO,OAA+B;QACpC,IAAI,CAAC,OAAO,EAAE;YACZ,OAAO,KAAK,CAAC;SACd;QAED,IAAI,OAAO,YAAY,IAAI,EAAE;YAC3B,OAAO,OAAO,CAAC,EAAE,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;SACnC;QAED,IAAI;YACF,OAAO,IAAI,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;SAC7C;QAAC,WAAM;YACN,OAAO,KAAK,CAAC;SACd;KACF;;;;IAKD,uBAAQ,GAAR;QACE,OAAO,IAAIC,aAAM,CAAC,IAAI,CAAC,EAAE,EAAEA,aAAM,CAAC,YAAY,CAAC,CAAC;KACjD;;;;IAKM,aAAQ,GAAf;QACE,IAAM,KAAK,GAAGH,iBAAW,CAAC,WAAW,CAAC,CAAC;;;QAIvC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,IAAI,IAAI,CAAC;QACpC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,IAAI,IAAI,CAAC;QAEpC,OAAOD,aAAM,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;KAC3B;;;;;IAMM,YAAO,GAAd,UAAe,KAA6B;QAC1C,IAAI,CAAC,KAAK,EAAE;YACV,OAAO,KAAK,CAAC;SACd;QAED,IAAI,KAAK,YAAY,IAAI,EAAE;YACzB,OAAO,IAAI,CAAC;SACb;QAED,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YAC7B,OAAOG,6BAAkB,CAAC,KAAK,CAAC,CAAC;SAClC;QAED,IAAIF,kBAAY,CAAC,KAAK,CAAC,EAAE;;YAEvB,IAAI,KAAK,CAAC,MAAM,KAAK,WAAW,EAAE;gBAChC,OAAO,KAAK,CAAC;aACd;YAED,IAAI;;;gBAGF,OAAO,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,QAAQ,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,KAAKG,aAAM,CAAC,YAAY,CAAC;aACvE;YAAC,WAAM;gBACN,OAAO,KAAK,CAAC;aACd;SACF;QAED,OAAO,KAAK,CAAC;KACd;;;;;IAMM,wBAAmB,GAA1B,UAA2B,SAAiB;QAC1C,IAAM,MAAM,GAAGD,gCAAqB,CAAC,SAAS,CAAC,CAAC;QAChD,OAAO,IAAI,IAAI,CAAC,MAAM,CAAC,CAAC;KACzB;;;;;;;IAQD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,sBAAO,GAAP;QACE,OAAO,gBAAa,IAAI,CAAC,WAAW,EAAE,QAAI,CAAC;KAC5C;IACH,WAAC;AAAD,CAAC,IAAA;AA3LY,oBAAI;AA6LjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;AChNtC;AACe;AACM;AACT;AAoB5C;;;;AAIA;;;;;IAkCE,gBAAY1X,QAAgC,EAAE,OAAgB;QAC5D,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,CAACA,QAAM,EAAE,OAAO,CAAC,CAAC;QAElE,IACE,EAAEA,QAAM,IAAI,IAAI,CAAC;YACjB,EAAE,OAAOA,QAAM,KAAK,QAAQ,CAAC;YAC7B,CAAC,WAAW,CAAC,MAAM,CAACA,QAAM,CAAC;YAC3B,EAAEA,QAAM,YAAY,WAAW,CAAC;YAChC,CAAC,KAAK,CAAC,OAAO,CAACA,QAAM,CAAC,EACtB;YACA,MAAM,IAAI,SAAS,CACjB,kFAAkF,CACnF,CAAC;SACH;QAED,IAAI,CAAC,QAAQ,GAAG,OAAO,aAAP,OAAO,cAAP,OAAO,GAAI,MAAM,CAAC,2BAA2B,CAAC;QAE9D,IAAIA,QAAM,IAAI,IAAI,EAAE;;YAElB,IAAI,CAAC,MAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,MAAM,CAAC,WAAW,CAAC,CAAC;YAC/C,IAAI,CAAC,QAAQ,GAAG,CAAC,CAAC;SACnB;aAAM;YACL,IAAI,OAAOvX,QAAM,KAAK,QAAQ,EAAE;;gBAE9B,IAAI,CAAC,MAAM,GAAGuX,aAAM,CAAC,IAAI,CAACvX,QAAM,EAAE,QAAQ,CAAC,CAAC;aAC7C;iBAAM,IAAI,KAAK,CAAC,OAAO,CAACA,QAAM,CAAC,EAAE;;gBAEhC,IAAI,CAAC,MAAM,GAAGuX,aAAM,CAAC,IAAI,CAACvX,QAAM,CAAC,CAAC;aACnC;iBAAM;;gBAEL,IAAI,CAAC,MAAM,GAAGyX,0BAAY,CAACzX,QAAM,CAAC,CAAC;aACpC;YAED,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;SACxC;KACF;;;;;;IAOD,oBAAG,GAAH,UAAI,SAA2D;;QAE7D,IAAI,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,CAAC,MAAM,KAAK,CAAC,EAAE;YAC3D,MAAM,IAAI,SAAS,CAAC,sCAAsC,CAAC,CAAC;SAC7D;aAAM,IAAI,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,CAAC,MAAM,KAAK,CAAC;YAChE,MAAM,IAAI,SAAS,CAAC,mDAAmD,CAAC,CAAC;;QAG3E,IAAI,WAAmB,CAAC;QACxB,IAAI,OAAO,SAAS,KAAK,QAAQ,EAAE;YACjC,WAAW,GAAG,SAAS,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;SACvC;aAAM,IAAI,OAAO,SAAS,KAAK,QAAQ,EAAE;YACxC,WAAW,GAAG,SAAS,CAAC;SACzB;aAAM;YACL,WAAW,GAAG,SAAS,CAAC,CAAC,CAAC,CAAC;SAC5B;QAED,IAAI,WAAW,GAAG,CAAC,IAAI,WAAW,GAAG,GAAG,EAAE;YACxC,MAAM,IAAI,SAAS,CAAC,0DAA0D,CAAC,CAAC;SACjF;QAED,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,EAAE;YACtC,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,GAAG,WAAW,CAAC;SAC5C;aAAM;YACL,IAAMA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,MAAM,CAAC,WAAW,GAAG,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;;YAErE,IAAI,CAAC,MAAM,CAAC,IAAI,CAACvX,QAAM,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;YACnD,IAAI,CAAC,MAAM,GAAGA,QAAM,CAAC;YACrB,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,GAAG,WAAW,CAAC;SAC5C;KACF;;;;;;;IAQD,sBAAK,GAAL,UAAM,QAAiC,EAAE,MAAc;QACrD,MAAM,GAAG,OAAO,MAAM,KAAK,QAAQ,GAAG,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;;QAG7D,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,EAAE;YACjD,IAAMA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,QAAQ,CAAC,MAAM,CAAC,CAAC;YAClE,IAAI,CAAC,MAAM,CAAC,IAAI,CAACvX,QAAM,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;;YAGnD,IAAI,CAAC,MAAM,GAAGA,QAAM,CAAC;SACtB;QAED,IAAI,WAAW,CAAC,MAAM,CAAC,QAAQ,CAAC,EAAE;YAChC,IAAI,CAAC,MAAM,CAAC,GAAG,CAACyX,0BAAY,CAAC,QAAQ,CAAC,EAAE,MAAM,CAAC,CAAC;YAChD,IAAI,CAAC,QAAQ;gBACX,MAAM,GAAG,QAAQ,CAAC,UAAU,GAAG,IAAI,CAAC,QAAQ,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;SAC3F;aAAM,IAAI,OAAO,QAAQ,KAAK,QAAQ,EAAE;YACvC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,QAAQ,EAAE,MAAM,EAAE,QAAQ,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;YAC/D,IAAI,CAAC,QAAQ;gBACX,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;SACvF;KACF;;;;;;;IAQD,qBAAI,GAAJ,UAAK,QAAgB,EAAE,MAAc;QACnC,MAAM,GAAG,MAAM,IAAI,MAAM,GAAG,CAAC,GAAG,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;;QAGvD,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,QAAQ,EAAE,QAAQ,GAAG,MAAM,CAAC,CAAC;KACvD;;;;;;;IAQD,sBAAK,GAAL,UAAM,KAAe;QACnB,KAAK,GAAG,CAAC,CAAC,KAAK,CAAC;;QAGhB,IAAI,KAAK,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,KAAK,IAAI,CAAC,QAAQ,EAAE;YACjD,OAAO,IAAI,CAAC,MAAM,CAAC;SACpB;;QAGD,IAAI,KAAK,EAAE;YACT,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC5C;QACD,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KACzD;;IAGD,uBAAM,GAAN;QACE,OAAO,IAAI,CAAC,QAAQ,CAAC;KACtB;;IAGD,uBAAM,GAAN;QACE,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;KACvC;;IAGD,yBAAQ,GAAR,UAAS,MAAc;QACrB,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC;KACrC;;IAGD,+BAAc,GAAd,UAAe,OAAsB;QACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAM,YAAY,GAAG,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;QAEpD,IAAM,OAAO,GAAG,MAAM,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,QAAQ,CAAC,EAAE,CAAC,CAAC;QACnD,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO;gBACL,OAAO,EAAE,YAAY;gBACrB,KAAK,EAAE,OAAO,CAAC,MAAM,KAAK,CAAC,GAAG,GAAG,GAAG,OAAO,GAAG,OAAO;aACtD,CAAC;SACH;QACD,OAAO;YACL,OAAO,EAAE;gBACP,MAAM,EAAE,YAAY;gBACpB,OAAO,EAAE,OAAO,CAAC,MAAM,KAAK,CAAC,GAAG,GAAG,GAAG,OAAO,GAAG,OAAO;aACxD;SACF,CAAC;KACH;;IAGD,uBAAM,GAAN;QACE,IAAI,IAAI,CAAC,QAAQ,KAAK,MAAM,CAAC,YAAY,EAAE;YACzC,OAAO,IAAIG,SAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC;SACtD;QAED,MAAM,IAAI,KAAK,CACb,uBAAoB,IAAI,CAAC,QAAQ,2DAAoD,MAAM,CAAC,YAAY,+BAA2B,CACpI,CAAC;KACH;;IAGM,uBAAgB,GAAvB,UACE,GAAyD,EACzD,OAAsB;QAEtB,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAI,IAAwB,CAAC;QAC7B,IAAI,IAAI,CAAC;QACT,IAAI,SAAS,IAAI,GAAG,EAAE;YACpB,IAAI,OAAO,CAAC,MAAM,IAAI,OAAO,GAAG,CAAC,OAAO,KAAK,QAAQ,IAAI,OAAO,IAAI,GAAG,EAAE;gBACvE,IAAI,GAAG,GAAG,CAAC,KAAK,GAAG,QAAQ,CAAC,GAAG,CAAC,KAAK,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;gBAC/C,IAAI,GAAGL,aAAM,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;aAC3C;iBAAM;gBACL,IAAI,OAAO,GAAG,CAAC,OAAO,KAAK,QAAQ,EAAE;oBACnC,IAAI,GAAG,GAAG,CAAC,OAAO,CAAC,OAAO,GAAG,QAAQ,CAAC,GAAG,CAAC,OAAO,CAAC,OAAO,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;oBACnE,IAAI,GAAGA,aAAM,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;iBAClD;aACF;SACF;aAAM,IAAI,OAAO,IAAI,GAAG,EAAE;YACzB,IAAI,GAAG,CAAC,CAAC;YACT,IAAI,GAAGG,gCAAqB,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC;SACzC;QACD,IAAI,CAAC,IAAI,EAAE;YACT,MAAM,IAAI,SAAS,CAAC,4CAA0C,IAAI,CAAC,SAAS,CAAC,GAAG,CAAG,CAAC,CAAC;SACtF;QACD,OAAO,IAAI,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;KAC/B;;IAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,wBAAO,GAAP;QACE,IAAM,QAAQ,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;QAClC,OAAO,8BAA2B,QAAQ,CAAC,QAAQ,CAAC,KAAK,CAAC,sBAAc,IAAI,CAAC,QAAQ,MAAG,CAAC;KAC1F;;;;;IAtPuB,kCAA2B,GAAG,CAAC,CAAC;;IAGxC,kBAAW,GAAG,GAAG,CAAC;;IAElB,sBAAe,GAAG,CAAC,CAAC;;IAEpB,uBAAgB,GAAG,CAAC,CAAC;;IAErB,yBAAkB,GAAG,CAAC,CAAC;;IAEvB,uBAAgB,GAAG,CAAC,CAAC;;IAErB,mBAAY,GAAG,CAAC,CAAC;;IAEjB,kBAAW,GAAG,CAAC,CAAC;;IAEhB,2BAAoB,GAAG,GAAG,CAAC;IAsO7C,aAAC;CA9PD,IA8PC;AA9PY,wBAAM;AAgQnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;ACnR1E;;;;AAIA;;;;;IASE,cAAY,IAAuB,EAAE,KAAgB;QACnD,IAAI,EAAE,IAAI,YAAY,IAAI,CAAC;YAAE,OAAO,IAAI,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;QAE1D,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC;QACjB,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;KACpB;;IAGD,qBAAM,GAAN;QACE,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,IAAI,EAAE,KAAK,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;KAC/C;;IAGD,6BAAc,GAAd;QACE,IAAI,IAAI,CAAC,KAAK,EAAE;YACd,OAAO,EAAE,KAAK,EAAE,IAAI,CAAC,IAAI,EAAE,MAAM,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;SACjD;QAED,OAAO,EAAE,KAAK,EAAE,IAAI,CAAC,IAAI,EAAE,CAAC;KAC7B;;IAGM,qBAAgB,GAAvB,UAAwB,GAAiB;QACvC,OAAO,IAAI,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,MAAM,CAAC,CAAC;KACxC;;IAGD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,sBAAO,GAAP;QACE,IAAM,QAAQ,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC;QAC/B,OAAO,gBAAa,QAAQ,CAAC,IAAI,WAC/B,QAAQ,CAAC,KAAK,GAAG,OAAK,IAAI,CAAC,SAAS,CAAC,QAAQ,CAAC,KAAK,CAAG,GAAG,EAAE,OAC1D,CAAC;KACL;IACH,WAAC;AAAD,CAAC,IAAA;AA9CY,oBAAI;AAgDjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;ACzDxB;AAS9C;AACA,SAAgB,WAAW,CAAC,KAAc;IACxC,QACEF,kBAAY,CAAC,KAAK,CAAC;QACnB,KAAK,CAAC,GAAG,IAAI,IAAI;QACjB,OAAO,KAAK,CAAC,IAAI,KAAK,QAAQ;SAC7B,KAAK,CAAC,GAAG,IAAI,IAAI,IAAI,OAAO,KAAK,CAAC,GAAG,KAAK,QAAQ,CAAC,EACpD;AACJ,CAAC;AAPD,kCAOC;AAED;;;;AAIA;;;;;;IAaE,eAAY,UAAkB,EAAE,GAAa,EAAE,EAAW,EAAE,MAAiB;QAC3E,IAAI,EAAE,IAAI,YAAY,KAAK,CAAC;YAAE,OAAO,IAAI,KAAK,CAAC,UAAU,EAAE,GAAG,EAAE,EAAE,EAAE,MAAM,CAAC,CAAC;;QAG5E,IAAM,KAAK,GAAG,UAAU,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;QACpC,IAAI,KAAK,CAAC,MAAM,KAAK,CAAC,EAAE;YACtB,EAAE,GAAG,KAAK,CAAC,KAAK,EAAE,CAAC;;YAEnB,UAAU,GAAG,KAAK,CAAC,KAAK,EAAG,CAAC;SAC7B;QAED,IAAI,CAAC,UAAU,GAAG,UAAU,CAAC;QAC7B,IAAI,CAAC,GAAG,GAAG,GAAG,CAAC;QACf,IAAI,CAAC,EAAE,GAAG,EAAE,CAAC;QACb,IAAI,CAAC,MAAM,GAAG,MAAM,IAAI,EAAE,CAAC;KAC5B;IAMD,sBAAI,4BAAS;;;;aAAb;YACE,OAAO,IAAI,CAAC,UAAU,CAAC;SACxB;aAED,UAAc,KAAa;YACzB,IAAI,CAAC,UAAU,GAAG,KAAK,CAAC;SACzB;;;OAJA;;IAOD,sBAAM,GAAN;QACE,IAAM,CAAC,GAAG,MAAM,CAAC,MAAM,CACrB;YACE,IAAI,EAAE,IAAI,CAAC,UAAU;YACrB,GAAG,EAAE,IAAI,CAAC,GAAG;SACd,EACD,IAAI,CAAC,MAAM,CACZ,CAAC;QAEF,IAAI,IAAI,CAAC,EAAE,IAAI,IAAI;YAAE,CAAC,CAAC,GAAG,GAAG,IAAI,CAAC,EAAE,CAAC;QACrC,OAAO,CAAC,CAAC;KACV;;IAGD,8BAAc,GAAd,UAAe,OAAsB;QACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAI,CAAC,GAAc;YACjB,IAAI,EAAE,IAAI,CAAC,UAAU;YACrB,GAAG,EAAE,IAAI,CAAC,GAAG;SACd,CAAC;QAEF,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO,CAAC,CAAC;SACV;QAED,IAAI,IAAI,CAAC,EAAE;YAAE,CAAC,CAAC,GAAG,GAAG,IAAI,CAAC,EAAE,CAAC;QAC7B,CAAC,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,CAAC;QAClC,OAAO,CAAC,CAAC;KACV;;IAGM,sBAAgB,GAAvB,UAAwB,GAAc;QACpC,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,GAAG,CAAuB,CAAC;QAC1D,OAAO,IAAI,CAAC,IAAI,CAAC;QACjB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAI,KAAK,CAAC,GAAG,CAAC,IAAI,EAAE,GAAG,CAAC,GAAG,EAAE,GAAG,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;KACpD;;IAGD,gBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,uBAAO,GAAP;;QAEE,IAAM,GAAG,GACP,IAAI,CAAC,GAAG,KAAK,SAAS,IAAI,IAAI,CAAC,GAAG,CAAC,QAAQ,KAAK,SAAS,GAAG,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,QAAQ,EAAE,CAAC;QAC7F,OAAO,iBAAc,IAAI,CAAC,SAAS,2BAAoB,GAAG,YACxD,IAAI,CAAC,EAAE,GAAG,SAAM,IAAI,CAAC,EAAE,OAAG,GAAG,EAAE,OAC9B,CAAC;KACL;IACH,YAAC;AAAD,CAAC,IAAA;AA/FY,sBAAK;AAiGlB,MAAM,CAAC,cAAc,CAAC,KAAK,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,OAAO,EAAE,CAAC,CAAC;;;;;;;;;;;AC1H1B;AAkB9C;;;AAGA,IAAI,IAAI,GAAgC,SAAS,CAAC;AAMlD,IAAI;IACF,IAAI,GAAI,IAAI,WAAW,CAAC,QAAQ,CAC9B,IAAI,WAAW,CAAC,MAAM;;IAEpB,IAAI,UAAU,CAAC,CAAC,CAAC,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,CAAC,CAAC,CAC/oC,EACD,EAAE,CACH,CAAC,OAAsC,CAAC;CAC1C;AAAC,WAAM;;CAEP;AAED,IAAM,cAAc,GAAG,CAAC,IAAI,EAAE,CAAC;AAC/B,IAAM,cAAc,GAAG,CAAC,IAAI,EAAE,CAAC;AAC/B,IAAM,cAAc,GAAG,cAAc,GAAG,cAAc,CAAC;AACvD,IAAM,cAAc,GAAG,cAAc,GAAG,cAAc,CAAC;AACvD,IAAM,cAAc,GAAG,cAAc,GAAG,CAAC,CAAC;AAE1C;AACA,IAAM,SAAS,GAA4B,EAAE,CAAC;AAE9C;AACA,IAAM,UAAU,GAA4B,EAAE,CAAC;AAO/C;;;;;;;;;;;;;;;;;;AAkBA;;;;;;;;;;;;;;IAkCE,cAAY,GAAiC,EAAE,IAAuB,EAAE,QAAkB;QAA9E,oBAAA,EAAA,OAAiC;QAC3C,IAAI,EAAE,IAAI,YAAY,IAAI,CAAC;YAAE,OAAO,IAAI,IAAI,CAAC,GAAG,EAAE,IAAI,EAAE,QAAQ,CAAC,CAAC;QAElE,IAAI,OAAO,GAAG,KAAK,QAAQ,EAAE;YAC3B,MAAM,CAAC,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;SACnD;aAAM,IAAI,OAAO,GAAG,KAAK,QAAQ,EAAE;YAClC,MAAM,CAAC,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;SACnD;aAAM;YACL,IAAI,CAAC,GAAG,GAAG,GAAG,GAAG,CAAC,CAAC;YACnB,IAAI,CAAC,IAAI,GAAI,IAAe,GAAG,CAAC,CAAC;YACjC,IAAI,CAAC,QAAQ,GAAG,CAAC,CAAC,QAAQ,CAAC;SAC5B;QAED,MAAM,CAAC,cAAc,CAAC,IAAI,EAAE,YAAY,EAAE;YACxC,KAAK,EAAE,IAAI;YACX,YAAY,EAAE,KAAK;YACnB,QAAQ,EAAE,KAAK;YACf,UAAU,EAAE,KAAK;SAClB,CAAC,CAAC;KACJ;;;;;;;;;IA6BM,aAAQ,GAAf,UAAgB,OAAe,EAAE,QAAgB,EAAE,QAAkB;QACnE,OAAO,IAAI,IAAI,CAAC,OAAO,EAAE,QAAQ,EAAE,QAAQ,CAAC,CAAC;KAC9C;;;;;;;IAQM,YAAO,GAAd,UAAe,KAAa,EAAE,QAAkB;QAC9C,IAAI,GAAG,EAAE,SAAS,EAAE,KAAK,CAAC;QAC1B,IAAI,QAAQ,EAAE;YACZ,KAAK,MAAM,CAAC,CAAC;YACb,KAAK,KAAK,GAAG,CAAC,IAAI,KAAK,IAAI,KAAK,GAAG,GAAG,GAAG;gBACvC,SAAS,GAAG,UAAU,CAAC,KAAK,CAAC,CAAC;gBAC9B,IAAI,SAAS;oBAAE,OAAO,SAAS,CAAC;aACjC;YACD,GAAG,GAAG,IAAI,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,KAAK,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,EAAE,IAAI,CAAC,CAAC;YAC3D,IAAI,KAAK;gBAAE,UAAU,CAAC,KAAK,CAAC,GAAG,GAAG,CAAC;YACnC,OAAO,GAAG,CAAC;SACZ;aAAM;YACL,KAAK,IAAI,CAAC,CAAC;YACX,KAAK,KAAK,GAAG,CAAC,GAAG,IAAI,KAAK,IAAI,KAAK,GAAG,GAAG,GAAG;gBAC1C,SAAS,GAAG,SAAS,CAAC,KAAK,CAAC,CAAC;gBAC7B,IAAI,SAAS;oBAAE,OAAO,SAAS,CAAC;aACjC;YACD,GAAG,GAAG,IAAI,CAAC,QAAQ,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;YACtD,IAAI,KAAK;gBAAE,SAAS,CAAC,KAAK,CAAC,GAAG,GAAG,CAAC;YAClC,OAAO,GAAG,CAAC;SACZ;KACF;;;;;;;IAQM,eAAU,GAAjB,UAAkB,KAAa,EAAE,QAAkB;QACjD,IAAI,KAAK,CAAC,KAAK,CAAC;YAAE,OAAO,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;QAC3D,IAAI,QAAQ,EAAE;YACZ,IAAI,KAAK,GAAG,CAAC;gBAAE,OAAO,IAAI,CAAC,KAAK,CAAC;YACjC,IAAI,KAAK,IAAI,cAAc;gBAAE,OAAO,IAAI,CAAC,kBAAkB,CAAC;SAC7D;aAAM;YACL,IAAI,KAAK,IAAI,CAAC,cAAc;gBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;YACpD,IAAI,KAAK,GAAG,CAAC,IAAI,cAAc;gBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;SACxD;QACD,IAAI,KAAK,GAAG,CAAC;YAAE,OAAO,IAAI,CAAC,UAAU,CAAC,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC,GAAG,EAAE,CAAC;QAC9D,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,GAAG,cAAc,GAAG,CAAC,EAAE,CAAC,KAAK,GAAG,cAAc,IAAI,CAAC,EAAE,QAAQ,CAAC,CAAC;KAC1F;;;;;;;IAQM,eAAU,GAAjB,UAAkB,KAAa,EAAE,QAAkB;QACjD,OAAO,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,QAAQ,EAAE,EAAE,QAAQ,CAAC,CAAC;KACpD;;;;;;;;IASM,eAAU,GAAjB,UAAkB,GAAW,EAAE,QAAkB,EAAE,KAAc;QAC/D,IAAI,GAAG,CAAC,MAAM,KAAK,CAAC;YAAE,MAAM,KAAK,CAAC,cAAc,CAAC,CAAC;QAClD,IAAI,GAAG,KAAK,KAAK,IAAI,GAAG,KAAK,UAAU,IAAI,GAAG,KAAK,WAAW,IAAI,GAAG,KAAK,WAAW;YACnF,OAAO,IAAI,CAAC,IAAI,CAAC;QACnB,IAAI,OAAO,QAAQ,KAAK,QAAQ,EAAE;;YAEhC,CAAC,KAAK,GAAG,QAAQ,IAAI,QAAQ,GAAG,KAAK,CAAC,CAAC;SACxC;aAAM;YACL,QAAQ,GAAG,CAAC,CAAC,QAAQ,CAAC;SACvB;QACD,KAAK,GAAG,KAAK,IAAI,EAAE,CAAC;QACpB,IAAI,KAAK,GAAG,CAAC,IAAI,EAAE,GAAG,KAAK;YAAE,MAAM,UAAU,CAAC,OAAO,CAAC,CAAC;QAEvD,IAAI,CAAC,CAAC;QACN,IAAI,CAAC,CAAC,GAAG,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,CAAC;YAAE,MAAM,KAAK,CAAC,iBAAiB,CAAC,CAAC;aAC1D,IAAI,CAAC,KAAK,CAAC,EAAE;YAChB,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,CAAC,EAAE,QAAQ,EAAE,KAAK,CAAC,CAAC,GAAG,EAAE,CAAC;SACjE;;;QAID,IAAM,YAAY,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC,CAAC;QAEzD,IAAI,MAAM,GAAG,IAAI,CAAC,IAAI,CAAC;QACvB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,GAAG,CAAC,MAAM,EAAE,CAAC,IAAI,CAAC,EAAE;YACtC,IAAM,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,GAAG,CAAC,MAAM,GAAG,CAAC,CAAC,EACtC,KAAK,GAAG,QAAQ,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,EAAE,KAAK,CAAC,CAAC;YACtD,IAAI,IAAI,GAAG,CAAC,EAAE;gBACZ,IAAM,KAAK,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;gBACrD,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,CAAC;aACxD;iBAAM;gBACL,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC;gBAClC,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,CAAC;aAC7C;SACF;QACD,MAAM,CAAC,QAAQ,GAAG,QAAQ,CAAC;QAC3B,OAAO,MAAM,CAAC;KACf;;;;;;;;IASM,cAAS,GAAhB,UAAiB,KAAe,EAAE,QAAkB,EAAE,EAAY;QAChE,OAAO,EAAE,GAAG,IAAI,CAAC,WAAW,CAAC,KAAK,EAAE,QAAQ,CAAC,GAAG,IAAI,CAAC,WAAW,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC;KACnF;;;;;;;IAQM,gBAAW,GAAlB,UAAmB,KAAe,EAAE,QAAkB;QACpD,OAAO,IAAI,IAAI,CACb,KAAK,CAAC,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,EAChE,KAAK,CAAC,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,EAChE,QAAQ,CACT,CAAC;KACH;;;;;;;IAQM,gBAAW,GAAlB,UAAmB,KAAe,EAAE,QAAkB;QACpD,OAAO,IAAI,IAAI,CACb,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,KAAK,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,EAChE,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,KAAK,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,EAChE,QAAQ,CACT,CAAC;KACH;;;;;IAMM,WAAM,GAAb,UAAc,KAAU;QACtB,OAAOA,kBAAY,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,YAAY,CAAC,KAAK,IAAI,CAAC;KAC5D;;;;;IAMM,cAAS,GAAhB,UACE,GAAwE,EACxE,QAAkB;QAElB,IAAI,OAAO,GAAG,KAAK,QAAQ;YAAE,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;QACnE,IAAI,OAAO,GAAG,KAAK,QAAQ;YAAE,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;;QAEnE,OAAO,IAAI,CAAC,QAAQ,CAClB,GAAG,CAAC,GAAG,EACP,GAAG,CAAC,IAAI,EACR,OAAO,QAAQ,KAAK,SAAS,GAAG,QAAQ,GAAG,GAAG,CAAC,QAAQ,CACxD,CAAC;KACH;;IAGD,kBAAG,GAAH,UAAI,MAA0C;QAC5C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC;YAAE,MAAM,GAAG,IAAI,CAAC,SAAS,CAAC,MAAM,CAAC,CAAC;;QAI1D,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,EAAE,CAAC;QAC7B,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,GAAG,MAAM,CAAC;QAC/B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC;QAC5B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,GAAG,MAAM,CAAC;QAE9B,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,KAAK,EAAE,CAAC;QAC/B,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,GAAG,MAAM,CAAC;QACjC,IAAM,GAAG,GAAG,MAAM,CAAC,GAAG,KAAK,EAAE,CAAC;QAC9B,IAAM,GAAG,GAAG,MAAM,CAAC,GAAG,GAAG,MAAM,CAAC;QAEhC,IAAI,GAAG,GAAG,CAAC,EACT,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,CAAC;QACV,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,MAAM,CAAC;QACd,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KAC3E;;;;;IAMD,kBAAG,GAAH,UAAI,KAAyC;QAC3C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KACnF;;;;;IAMD,sBAAO,GAAP,UAAQ,KAAyC;QAC/C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,IAAI,IAAI,CAAC,EAAE,CAAC,KAAK,CAAC;YAAE,OAAO,CAAC,CAAC;QAC7B,IAAM,OAAO,GAAG,IAAI,CAAC,UAAU,EAAE,EAC/B,QAAQ,GAAG,KAAK,CAAC,UAAU,EAAE,CAAC;QAChC,IAAI,OAAO,IAAI,CAAC,QAAQ;YAAE,OAAO,CAAC,CAAC,CAAC;QACpC,IAAI,CAAC,OAAO,IAAI,QAAQ;YAAE,OAAO,CAAC,CAAC;;QAEnC,IAAI,CAAC,IAAI,CAAC,QAAQ;YAAE,OAAO,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,UAAU,EAAE,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;QAEjE,OAAO,KAAK,CAAC,IAAI,KAAK,CAAC,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC;aACtC,KAAK,CAAC,IAAI,KAAK,IAAI,CAAC,IAAI,IAAI,KAAK,CAAC,GAAG,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;cAC5D,CAAC,CAAC;cACF,CAAC,CAAC;KACP;;IAGD,mBAAI,GAAJ,UAAK,KAAyC;QAC5C,OAAO,IAAI,CAAC,OAAO,CAAC,KAAK,CAAC,CAAC;KAC5B;;;;;IAMD,qBAAM,GAAN,UAAO,OAA2C;QAChD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;QAC7D,IAAI,OAAO,CAAC,MAAM,EAAE;YAAE,MAAM,KAAK,CAAC,kBAAkB,CAAC,CAAC;;QAGtD,IAAI,IAAI,EAAE;;;;YAIR,IACE,CAAC,IAAI,CAAC,QAAQ;gBACd,IAAI,CAAC,IAAI,KAAK,CAAC,UAAU;gBACzB,OAAO,CAAC,GAAG,KAAK,CAAC,CAAC;gBAClB,OAAO,CAAC,IAAI,KAAK,CAAC,CAAC,EACnB;;gBAEA,OAAO,IAAI,CAAC;aACb;YACD,IAAM,GAAG,GAAG,CAAC,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,KAAK,EAClD,IAAI,CAAC,GAAG,EACR,IAAI,CAAC,IAAI,EACT,OAAO,CAAC,GAAG,EACX,OAAO,CAAC,IAAI,CACb,CAAC;YACF,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC3D;QAED,IAAI,IAAI,CAAC,MAAM,EAAE;YAAE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;QACjE,IAAI,MAAM,EAAE,GAAG,EAAE,GAAG,CAAC;QACrB,IAAI,CAAC,IAAI,CAAC,QAAQ,EAAE;;;YAGlB,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;gBAC3B,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,GAAG,CAAC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,OAAO,CAAC;oBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;;qBAEvE,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;oBAAE,OAAO,IAAI,CAAC,GAAG,CAAC;qBAChD;;oBAEH,IAAM,QAAQ,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;oBAC7B,MAAM,GAAG,QAAQ,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;oBACtC,IAAI,MAAM,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,EAAE;wBACxB,OAAO,OAAO,CAAC,UAAU,EAAE,GAAG,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC,OAAO,CAAC;qBACvD;yBAAM;wBACL,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,MAAM,CAAC,CAAC,CAAC;wBACpC,GAAG,GAAG,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,CAAC;wBACnC,OAAO,GAAG,CAAC;qBACZ;iBACF;aACF;iBAAM,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;gBAAE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;YACrF,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;gBACrB,IAAI,OAAO,CAAC,UAAU,EAAE;oBAAE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC;gBAC/D,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,EAAE,CAAC;aACtC;iBAAM,IAAI,OAAO,CAAC,UAAU,EAAE;gBAAE,OAAO,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC;YACtE,GAAG,GAAG,IAAI,CAAC,IAAI,CAAC;SACjB;aAAM;;;YAGL,IAAI,CAAC,OAAO,CAAC,QAAQ;gBAAE,OAAO,GAAG,OAAO,CAAC,UAAU,EAAE,CAAC;YACtD,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC;gBAAE,OAAO,IAAI,CAAC,KAAK,CAAC;YACxC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;;gBAE1B,OAAO,IAAI,CAAC,IAAI,CAAC;YACnB,GAAG,GAAG,IAAI,CAAC,KAAK,CAAC;SAClB;;;;;;QAOD,GAAG,GAAG,IAAI,CAAC;QACX,OAAO,GAAG,CAAC,GAAG,CAAC,OAAO,CAAC,EAAE;;;YAGvB,MAAM,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,QAAQ,EAAE,GAAG,OAAO,CAAC,QAAQ,EAAE,CAAC,CAAC,CAAC;;;YAItE,IAAM,IAAI,GAAG,IAAI,CAAC,IAAI,CAAC,IAAI,CAAC,GAAG,CAAC,MAAM,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC;YACpD,IAAM,KAAK,GAAG,IAAI,IAAI,EAAE,GAAG,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,GAAG,EAAE,CAAC,CAAC;;;YAGtD,IAAI,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,MAAM,CAAC,CAAC;YACxC,IAAI,SAAS,GAAG,SAAS,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;YACvC,OAAO,SAAS,CAAC,UAAU,EAAE,IAAI,SAAS,CAAC,EAAE,CAAC,GAAG,CAAC,EAAE;gBAClD,MAAM,IAAI,KAAK,CAAC;gBAChB,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,MAAM,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;gBACnD,SAAS,GAAG,SAAS,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;aACpC;;;YAID,IAAI,SAAS,CAAC,MAAM,EAAE;gBAAE,SAAS,GAAG,IAAI,CAAC,GAAG,CAAC;YAE7C,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;YACzB,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;SAC1B;QACD,OAAO,GAAG,CAAC;KACZ;;IAGD,kBAAG,GAAH,UAAI,OAA2C;QAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;KAC7B;;;;;IAMD,qBAAM,GAAN,UAAO,KAAyC;QAC9C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,IAAI,IAAI,CAAC,QAAQ,KAAK,KAAK,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,KAAK,EAAE,KAAK,CAAC,IAAI,KAAK,CAAC,IAAI,KAAK,EAAE,KAAK,CAAC;YACvF,OAAO,KAAK,CAAC;QACf,OAAO,IAAI,CAAC,IAAI,KAAK,KAAK,CAAC,IAAI,IAAI,IAAI,CAAC,GAAG,KAAK,KAAK,CAAC,GAAG,CAAC;KAC3D;;IAGD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC;KAC3B;;IAGD,0BAAW,GAAX;QACE,OAAO,IAAI,CAAC,IAAI,CAAC;KAClB;;IAGD,kCAAmB,GAAnB;QACE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC;KACxB;;IAGD,yBAAU,GAAV;QACE,OAAO,IAAI,CAAC,GAAG,CAAC;KACjB;;IAGD,iCAAkB,GAAlB;QACE,OAAO,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;KACvB;;IAGD,4BAAa,GAAb;QACE,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;;YAErB,OAAO,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,GAAG,EAAE,GAAG,IAAI,CAAC,GAAG,EAAE,CAAC,aAAa,EAAE,CAAC;SAClE;QACD,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC,GAAG,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC;QACnD,IAAI,GAAW,CAAC;QAChB,KAAK,GAAG,GAAG,EAAE,EAAE,GAAG,GAAG,CAAC,EAAE,GAAG,EAAE;YAAE,IAAI,CAAC,GAAG,IAAI,CAAC,IAAI,GAAG,CAAC,MAAM,CAAC;gBAAE,MAAM;QACnE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,GAAG,GAAG,GAAG,EAAE,GAAG,GAAG,GAAG,CAAC,CAAC;KAC7C;;IAGD,0BAAW,GAAX,UAAY,KAAyC;QACnD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;KAC7B;;IAGD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,WAAW,CAAC,KAAK,CAAC,CAAC;KAChC;;IAGD,iCAAkB,GAAlB,UAAmB,KAAyC;QAC1D,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;KAC9B;;IAGD,kBAAG,GAAH,UAAI,KAAyC;QAC3C,OAAO,IAAI,CAAC,kBAAkB,CAAC,KAAK,CAAC,CAAC;KACvC;;IAED,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,kBAAkB,CAAC,KAAK,CAAC,CAAC;KACvC;;IAGD,qBAAM,GAAN;QACE,OAAO,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC,MAAM,CAAC,CAAC;KAC7B;;IAGD,yBAAU,GAAV;QACE,OAAO,CAAC,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,GAAG,CAAC,CAAC;KACxC;;IAGD,oBAAK,GAAL;QACE,OAAO,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC,MAAM,CAAC,CAAC;KAC7B;;IAGD,yBAAU,GAAV;QACE,OAAO,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC,CAAC;KACxC;;IAGD,qBAAM,GAAN;QACE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;KAC1C;;IAGD,uBAAQ,GAAR,UAAS,KAAyC;QAChD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;KAC7B;;IAGD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;KAC7B;;IAGD,8BAAe,GAAf,UAAgB,KAAyC;QACvD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;KAC9B;;IAGD,kBAAG,GAAH,UAAI,KAAyC;QAC3C,OAAO,IAAI,CAAC,eAAe,CAAC,KAAK,CAAC,CAAC;KACpC;;IAGD,qBAAM,GAAN,UAAO,OAA2C;QAChD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;;QAG7D,IAAI,IAAI,EAAE;YACR,IAAM,GAAG,GAAG,CAAC,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,KAAK,EAClD,IAAI,CAAC,GAAG,EACR,IAAI,CAAC,IAAI,EACT,OAAO,CAAC,GAAG,EACX,OAAO,CAAC,IAAI,CACb,CAAC;YACF,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC3D;QAED,OAAO,IAAI,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,CAAC;KACjD;;IAGD,kBAAG,GAAH,UAAI,OAA2C;QAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;KAC7B;;IAED,kBAAG,GAAH,UAAI,OAA2C;QAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;KAC7B;;;;;;IAOD,uBAAQ,GAAR,UAAS,UAA8C;QACrD,IAAI,IAAI,CAAC,MAAM,EAAE;YAAE,OAAO,IAAI,CAAC,IAAI,CAAC;QACpC,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;YAAE,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;;QAGtE,IAAI,IAAI,EAAE;YACR,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,UAAU,CAAC,GAAG,EAAE,UAAU,CAAC,IAAI,CAAC,CAAC;YAC3E,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC3D;QAED,IAAI,UAAU,CAAC,MAAM,EAAE;YAAE,OAAO,IAAI,CAAC,IAAI,CAAC;QAC1C,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;YAAE,OAAO,UAAU,CAAC,KAAK,EAAE,GAAG,IAAI,CAAC,SAAS,GAAG,IAAI,CAAC,IAAI,CAAC;QACpF,IAAI,UAAU,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;YAAE,OAAO,IAAI,CAAC,KAAK,EAAE,GAAG,IAAI,CAAC,SAAS,GAAG,IAAI,CAAC,IAAI,CAAC;QAEpF,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;YACrB,IAAI,UAAU,CAAC,UAAU,EAAE;gBAAE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC;;gBAChE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC,GAAG,EAAE,CAAC;SAC9C;aAAM,IAAI,UAAU,CAAC,UAAU,EAAE;YAAE,OAAO,IAAI,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC;;QAG5E,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,UAAU,CAAC,IAAI,UAAU,CAAC,EAAE,CAAC,IAAI,CAAC,UAAU,CAAC;YAC5D,OAAO,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,QAAQ,EAAE,GAAG,UAAU,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;;QAKjF,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,EAAE,CAAC;QAC7B,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,GAAG,MAAM,CAAC;QAC/B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC;QAC5B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,GAAG,MAAM,CAAC;QAE9B,IAAM,GAAG,GAAG,UAAU,CAAC,IAAI,KAAK,EAAE,CAAC;QACnC,IAAM,GAAG,GAAG,UAAU,CAAC,IAAI,GAAG,MAAM,CAAC;QACrC,IAAM,GAAG,GAAG,UAAU,CAAC,GAAG,KAAK,EAAE,CAAC;QAClC,IAAM,GAAG,GAAG,UAAU,CAAC,GAAG,GAAG,MAAM,CAAC;QAEpC,IAAI,GAAG,GAAG,CAAC,EACT,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,CAAC;QACV,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,CAAC;QACrD,GAAG,IAAI,MAAM,CAAC;QACd,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KAC3E;;IAGD,kBAAG,GAAH,UAAI,UAA8C;QAChD,OAAO,IAAI,CAAC,QAAQ,CAAC,UAAU,CAAC,CAAC;KAClC;;IAGD,qBAAM,GAAN;QACE,IAAI,CAAC,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;YAAE,OAAO,IAAI,CAAC,SAAS,CAAC;QACrE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;KACjC;;IAGD,kBAAG,GAAH;QACE,OAAO,IAAI,CAAC,MAAM,EAAE,CAAC;KACtB;;IAGD,kBAAG,GAAH;QACE,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KAC5D;;IAGD,wBAAS,GAAT,UAAU,KAAyC;QACjD,OAAO,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC;KAC5B;;IAGD,kBAAG,GAAH,UAAI,KAAyC;QAC3C,OAAO,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;KAC9B;;IAED,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;KAC9B;;;;IAKD,iBAAE,GAAF,UAAG,KAA6B;QAC9B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KACnF;;;;;;IAOD,wBAAS,GAAT,UAAU,OAAsB;QAC9B,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;QACpD,IAAI,CAAC,OAAO,IAAI,EAAE,MAAM,CAAC;YAAE,OAAO,IAAI,CAAC;aAClC,IAAI,OAAO,GAAG,EAAE;YACnB,OAAO,IAAI,CAAC,QAAQ,CAClB,IAAI,CAAC,GAAG,IAAI,OAAO,EACnB,CAAC,IAAI,CAAC,IAAI,IAAI,OAAO,KAAK,IAAI,CAAC,GAAG,MAAM,EAAE,GAAG,OAAO,CAAC,CAAC,EACtD,IAAI,CAAC,QAAQ,CACd,CAAC;;YACC,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,EAAE,IAAI,CAAC,GAAG,KAAK,OAAO,GAAG,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KACzE;;IAGD,kBAAG,GAAH,UAAI,OAAsB;QACxB,OAAO,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;KAChC;;;;;;IAOD,yBAAU,GAAV,UAAW,OAAsB;QAC/B,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;QACpD,IAAI,CAAC,OAAO,IAAI,EAAE,MAAM,CAAC;YAAE,OAAO,IAAI,CAAC;aAClC,IAAI,OAAO,GAAG,EAAE;YACnB,OAAO,IAAI,CAAC,QAAQ,CAClB,CAAC,IAAI,CAAC,GAAG,KAAK,OAAO,KAAK,IAAI,CAAC,IAAI,KAAK,EAAE,GAAG,OAAO,CAAC,CAAC,EACtD,IAAI,CAAC,IAAI,IAAI,OAAO,EACpB,IAAI,CAAC,QAAQ,CACd,CAAC;;YACC,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,IAAI,KAAK,OAAO,GAAG,EAAE,CAAC,EAAE,IAAI,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KAChG;;IAGD,kBAAG,GAAH,UAAI,OAAsB;QACxB,OAAO,IAAI,CAAC,UAAU,CAAC,OAAO,CAAC,CAAC;KACjC;;;;;;IAOD,iCAAkB,GAAlB,UAAmB,OAAsB;QACvC,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;QACpD,OAAO,IAAI,EAAE,CAAC;QACd,IAAI,OAAO,KAAK,CAAC;YAAE,OAAO,IAAI,CAAC;aAC1B;YACH,IAAM,IAAI,GAAG,IAAI,CAAC,IAAI,CAAC;YACvB,IAAI,OAAO,GAAG,EAAE,EAAE;gBAChB,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC;gBACrB,OAAO,IAAI,CAAC,QAAQ,CAClB,CAAC,GAAG,KAAK,OAAO,KAAK,IAAI,KAAK,EAAE,GAAG,OAAO,CAAC,CAAC,EAC5C,IAAI,KAAK,OAAO,EAChB,IAAI,CAAC,QAAQ,CACd,CAAC;aACH;iBAAM,IAAI,OAAO,KAAK,EAAE;gBAAE,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;gBACnE,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,MAAM,OAAO,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SACtE;KACF;;IAGD,oBAAK,GAAL,UAAM,OAAsB;QAC1B,OAAO,IAAI,CAAC,kBAAkB,CAAC,OAAO,CAAC,CAAC;KACzC;;IAED,mBAAI,GAAJ,UAAK,OAAsB;QACzB,OAAO,IAAI,CAAC,kBAAkB,CAAC,OAAO,CAAC,CAAC;KACzC;;;;;;IAOD,uBAAQ,GAAR,UAAS,UAA8C;QACrD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;YAAE,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;QACtE,OAAO,IAAI,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC;KACnC;;IAGD,kBAAG,GAAH,UAAI,UAA8C;QAChD,OAAO,IAAI,CAAC,QAAQ,CAAC,UAAU,CAAC,CAAC;KAClC;;IAGD,oBAAK,GAAL;QACE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC;KAClD;;IAGD,uBAAQ,GAAR;QACE,IAAI,IAAI,CAAC,QAAQ;YAAE,OAAO,CAAC,IAAI,CAAC,IAAI,KAAK,CAAC,IAAI,cAAc,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC;QAChF,OAAO,IAAI,CAAC,IAAI,GAAG,cAAc,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC;KACtD;;IAGD,uBAAQ,GAAR;QACE,OAAO,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,CAAC;KAChC;;;;;;IAOD,sBAAO,GAAP,UAAQ,EAAY;QAClB,OAAO,EAAE,GAAG,IAAI,CAAC,SAAS,EAAE,GAAG,IAAI,CAAC,SAAS,EAAE,CAAC;KACjD;;;;;IAMD,wBAAS,GAAT;QACE,IAAM,EAAE,GAAG,IAAI,CAAC,IAAI,EAClB,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO;YACL,EAAE,GAAG,IAAI;YACT,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;YACjB,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;YAClB,EAAE,KAAK,EAAE;YACT,EAAE,GAAG,IAAI;YACT,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;YACjB,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;YAClB,EAAE,KAAK,EAAE;SACV,CAAC;KACH;;;;;IAMD,wBAAS,GAAT;QACE,IAAM,EAAE,GAAG,IAAI,CAAC,IAAI,EAClB,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO;YACL,EAAE,KAAK,EAAE;YACT,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;YAClB,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;YACjB,EAAE,GAAG,IAAI;YACT,EAAE,KAAK,EAAE;YACT,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;YAClB,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;YACjB,EAAE,GAAG,IAAI;SACV,CAAC;KACH;;;;IAKD,uBAAQ,GAAR;QACE,IAAI,CAAC,IAAI,CAAC,QAAQ;YAAE,OAAO,IAAI,CAAC;QAChC,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;KAClD;;;;;;IAOD,uBAAQ,GAAR,UAAS,KAAc;QACrB,KAAK,GAAG,KAAK,IAAI,EAAE,CAAC;QACpB,IAAI,KAAK,GAAG,CAAC,IAAI,EAAE,GAAG,KAAK;YAAE,MAAM,UAAU,CAAC,OAAO,CAAC,CAAC;QACvD,IAAI,IAAI,CAAC,MAAM,EAAE;YAAE,OAAO,GAAG,CAAC;QAC9B,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;;YAErB,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;;;gBAG3B,IAAM,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,EACtC,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,SAAS,CAAC,EACzB,IAAI,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;gBACtC,OAAO,GAAG,CAAC,QAAQ,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC,KAAK,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aAC3D;;gBAAM,OAAO,GAAG,GAAG,IAAI,CAAC,GAAG,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;SAChD;;;QAID,IAAM,YAAY,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;QAExE,IAAI,GAAG,GAAS,IAAI,CAAC;QACrB,IAAI,MAAM,GAAG,EAAE,CAAC;;QAEhB,OAAO,IAAI,EAAE;YACX,IAAM,MAAM,GAAG,GAAG,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC;YACrC,IAAM,MAAM,GAAG,GAAG,CAAC,GAAG,CAAC,MAAM,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC,CAAC,KAAK,EAAE,KAAK,CAAC,CAAC;YAC/D,IAAI,MAAM,GAAG,MAAM,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;YACpC,GAAG,GAAG,MAAM,CAAC;YACb,IAAI,GAAG,CAAC,MAAM,EAAE,EAAE;gBAChB,OAAO,MAAM,GAAG,MAAM,CAAC;aACxB;iBAAM;gBACL,OAAO,MAAM,CAAC,MAAM,GAAG,CAAC;oBAAE,MAAM,GAAG,GAAG,GAAG,MAAM,CAAC;gBAChD,MAAM,GAAG,EAAE,GAAG,MAAM,GAAG,MAAM,CAAC;aAC/B;SACF;KACF;;IAGD,yBAAU,GAAV;QACE,IAAI,IAAI,CAAC,QAAQ;YAAE,OAAO,IAAI,CAAC;QAC/B,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;KACjD;;IAGD,kBAAG,GAAH,UAAI,KAA6B;QAC/B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KACnF;;IAGD,kBAAG,GAAH;QACE,OAAO,IAAI,CAAC,MAAM,EAAE,CAAC;KACtB;;IAGD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,eAAe,CAAC,KAAK,CAAC,CAAC;KACpC;;;;;;IAOD,6BAAc,GAAd,UAAe,OAAsB;QACnC,IAAI,OAAO,IAAI,OAAO,CAAC,OAAO;YAAE,OAAO,IAAI,CAAC,QAAQ,EAAE,CAAC;QACvD,OAAO,EAAE,WAAW,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;KACzC;IACM,qBAAgB,GAAvB,UAAwB,GAA4B,EAAE,OAAsB;QAC1E,IAAM,MAAM,GAAG,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,WAAW,CAAC,CAAC;QAChD,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,MAAM,CAAC,QAAQ,EAAE,GAAG,MAAM,CAAC;KAChE;;IAGD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,sBAAO,GAAP;QACE,OAAO,gBAAa,IAAI,CAAC,QAAQ,EAAE,WAAI,IAAI,CAAC,QAAQ,GAAG,QAAQ,GAAG,EAAE,OAAG,CAAC;KACzE;IA/2BM,eAAU,GAAG,IAAI,CAAC,OAAO,CAAC,cAAc,CAAC,CAAC;;IAG1C,uBAAkB,GAAG,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,IAAI,CAAC,CAAC;;IAEzE,SAAI,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;;IAEvB,UAAK,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;;IAE9B,QAAG,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;;IAEtB,SAAI,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;;IAE7B,YAAO,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC,CAAC;;IAE3B,cAAS,GAAG,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;;IAEjE,cAAS,GAAG,IAAI,CAAC,QAAQ,CAAC,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;IA+1B7D,WAAC;CAv6BD,IAu6BC;AAv6BY,oBAAI;AAy6BjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,YAAY,EAAE,EAAE,KAAK,EAAE,IAAI,EAAE,CAAC,CAAC;AACrE,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;ACr/BtC;AACF;AAE9B,IAAM,mBAAmB,GAAG,+CAA+C,CAAC;AAC5E,IAAM,gBAAgB,GAAG,0BAA0B,CAAC;AACpD,IAAM,gBAAgB,GAAG,eAAe,CAAC;AAEzC,IAAM,YAAY,GAAG,IAAI,CAAC;AAC1B,IAAM,YAAY,GAAG,CAAC,IAAI,CAAC;AAC3B,IAAM,aAAa,GAAG,IAAI,CAAC;AAC3B,IAAM,UAAU,GAAG,EAAE,CAAC;AAEtB;AACA,IAAM,UAAU,GAAG;IACjB,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;CACL,CAAC,OAAO,EAAE,CAAC;AACZ;AACA,IAAM,mBAAmB,GAAG;IAC1B,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;CACL,CAAC,OAAO,EAAE,CAAC;AACZ,IAAM,mBAAmB,GAAG;IAC1B,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;CACL,CAAC,OAAO,EAAE,CAAC;AAEZ,IAAM,cAAc,GAAG,iBAAiB,CAAC;AAEzC;AACA,IAAM,gBAAgB,GAAG,IAAI,CAAC;AAC9B;AACA,IAAM,aAAa,GAAG,MAAM,CAAC;AAC7B;AACA,IAAM,oBAAoB,GAAG,EAAE,CAAC;AAChC;AACA,IAAM,eAAe,GAAG,EAAE,CAAC;AAE3B;AACA,SAAS,OAAO,CAAC,KAAa;IAC5B,OAAO,CAAC,KAAK,CAAC,QAAQ,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,CAAC;AACrC,CAAC;AAED;AACA,SAAS,UAAU,CAAC,KAAkD;IACpE,IAAM,OAAO,GAAG,WAAI,CAAC,UAAU,CAAC,IAAI,GAAG,IAAI,GAAG,IAAI,CAAC,CAAC;IACpD,IAAI,IAAI,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;IAE9B,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE;QAC5E,OAAO,EAAE,QAAQ,EAAE,KAAK,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;KACvC;IAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;;QAE3B,IAAI,GAAG,IAAI,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC;;QAE1B,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC,IAAI,WAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;QAC7C,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC;QACvC,IAAI,GAAG,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;KAC7B;IAED,OAAO,EAAE,QAAQ,EAAE,KAAK,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;AACxC,CAAC;AAED;AACA,SAAS,YAAY,CAAC,IAAU,EAAE,KAAW;IAC3C,IAAI,CAAC,IAAI,IAAI,CAAC,KAAK,EAAE;QACnB,OAAO,EAAE,IAAI,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,GAAG,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,CAAC;KAC9D;IAED,IAAM,QAAQ,GAAG,IAAI,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC;IAC7C,IAAM,OAAO,GAAG,IAAI,WAAI,CAAC,IAAI,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC;IAC/C,IAAM,SAAS,GAAG,KAAK,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC;IAC/C,IAAM,QAAQ,GAAG,IAAI,WAAI,CAAC,KAAK,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC;IAEjD,IAAI,WAAW,GAAG,QAAQ,CAAC,QAAQ,CAAC,SAAS,CAAC,CAAC;IAC/C,IAAI,UAAU,GAAG,QAAQ,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;IAC7C,IAAM,WAAW,GAAG,OAAO,CAAC,QAAQ,CAAC,SAAS,CAAC,CAAC;IAChD,IAAI,UAAU,GAAG,OAAO,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;IAE5C,WAAW,GAAG,WAAW,CAAC,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;IACjE,UAAU,GAAG,IAAI,WAAI,CAAC,UAAU,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC;SAC9C,GAAG,CAAC,WAAW,CAAC;SAChB,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;IAE1C,WAAW,GAAG,WAAW,CAAC,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;IACjE,UAAU,GAAG,UAAU,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,GAAG,CAAC,IAAI,WAAI,CAAC,UAAU,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC,CAAC;;IAGhF,OAAO,EAAE,IAAI,EAAE,WAAW,EAAE,GAAG,EAAE,UAAU,EAAE,CAAC;AAChD,CAAC;AAED,SAAS,QAAQ,CAAC,IAAU,EAAE,KAAW;;IAEvC,IAAM,MAAM,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC;IAC/B,IAAM,OAAO,GAAG,KAAK,CAAC,IAAI,KAAK,CAAC,CAAC;;IAGjC,IAAI,MAAM,GAAG,OAAO,EAAE;QACpB,OAAO,IAAI,CAAC;KACb;SAAM,IAAI,MAAM,KAAK,OAAO,EAAE;QAC7B,IAAM,MAAM,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;QAC9B,IAAM,OAAO,GAAG,KAAK,CAAC,GAAG,KAAK,CAAC,CAAC;QAChC,IAAI,MAAM,GAAG,OAAO;YAAE,OAAO,IAAI,CAAC;KACnC;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,UAAU,CAAC,MAAc,EAAE,OAAe;IACjD,MAAM,IAAI,SAAS,CAAC,OAAI,MAAM,8CAAwC,OAAS,CAAC,CAAC;AACnF,CAAC;AAOD;;;;AAIA;;;;;IASE,oBAAY,KAAsB;QAChC,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;YAAE,OAAO,IAAI,UAAU,CAAC,KAAK,CAAC,CAAC;QAEhE,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YAC7B,IAAI,CAAC,KAAK,GAAG,UAAU,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,KAAK,CAAC;SACjD;aAAM;YACL,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;SACpB;KACF;;;;;;IAOM,qBAAU,GAAjB,UAAkB,cAAsB;;QAEtC,IAAI,UAAU,GAAG,KAAK,CAAC;QACvB,IAAI,QAAQ,GAAG,KAAK,CAAC;QACrB,IAAI,YAAY,GAAG,KAAK,CAAC;;QAGzB,IAAI,iBAAiB,GAAG,CAAC,CAAC;;QAE1B,IAAI,WAAW,GAAG,CAAC,CAAC;;QAEpB,IAAI,OAAO,GAAG,CAAC,CAAC;;QAEhB,IAAI,aAAa,GAAG,CAAC,CAAC;;QAEtB,IAAI,YAAY,GAAG,CAAC,CAAC;;QAGrB,IAAM,MAAM,GAAG,CAAC,CAAC,CAAC,CAAC;;QAEnB,IAAI,aAAa,GAAG,CAAC,CAAC;;QAEtB,IAAI,YAAY,GAAG,CAAC,CAAC;;QAErB,IAAI,UAAU,GAAG,CAAC,CAAC;;QAEnB,IAAI,SAAS,GAAG,CAAC,CAAC;;QAGlB,IAAI,QAAQ,GAAG,CAAC,CAAC;;QAEjB,IAAI,CAAC,GAAG,CAAC,CAAC;;QAEV,IAAI,eAAe,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;;QAErC,IAAI,cAAc,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;;QAEpC,IAAI,cAAc,GAAG,CAAC,CAAC;;QAGvB,IAAI,KAAK,GAAG,CAAC,CAAC;;;;QAKd,IAAI,cAAc,CAAC,MAAM,IAAI,IAAI,EAAE;YACjC,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;SAC7E;;QAGD,IAAM,WAAW,GAAG,cAAc,CAAC,KAAK,CAAC,mBAAmB,CAAC,CAAC;QAC9D,IAAM,QAAQ,GAAG,cAAc,CAAC,KAAK,CAAC,gBAAgB,CAAC,CAAC;QACxD,IAAM,QAAQ,GAAG,cAAc,CAAC,KAAK,CAAC,gBAAgB,CAAC,CAAC;;QAGxD,IAAI,CAAC,CAAC,WAAW,IAAI,CAAC,QAAQ,IAAI,CAAC,QAAQ,KAAK,cAAc,CAAC,MAAM,KAAK,CAAC,EAAE;YAC3E,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;SAC7E;QAED,IAAI,WAAW,EAAE;;;YAIf,IAAM,cAAc,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;;;YAItC,IAAM,CAAC,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;YACzB,IAAM,OAAO,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;YAC/B,IAAM,SAAS,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;;YAGjC,IAAI,CAAC,IAAI,SAAS,KAAK,SAAS;gBAAE,UAAU,CAAC,cAAc,EAAE,wBAAwB,CAAC,CAAC;;YAGvF,IAAI,CAAC,IAAI,cAAc,KAAK,SAAS;gBAAE,UAAU,CAAC,cAAc,EAAE,uBAAuB,CAAC,CAAC;YAE3F,IAAI,CAAC,KAAK,SAAS,KAAK,OAAO,IAAI,SAAS,CAAC,EAAE;gBAC7C,UAAU,CAAC,cAAc,EAAE,2BAA2B,CAAC,CAAC;aACzD;SACF;;QAGD,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;YAClE,UAAU,GAAG,cAAc,CAAC,KAAK,EAAE,CAAC,KAAK,GAAG,CAAC;SAC9C;;QAGD,IAAI,CAAC,OAAO,CAAC,cAAc,CAAC,KAAK,CAAC,CAAC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;YACpE,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;gBAClE,OAAO,IAAI,UAAU,CAACD,aAAM,CAAC,IAAI,CAAC,UAAU,GAAG,mBAAmB,GAAG,mBAAmB,CAAC,CAAC,CAAC;aAC5F;iBAAM,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;gBACxC,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;aAChD;SACF;;QAGD,OAAO,OAAO,CAAC,cAAc,CAAC,KAAK,CAAC,CAAC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;YACtE,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;gBACjC,IAAI,QAAQ;oBAAE,UAAU,CAAC,cAAc,EAAE,2BAA2B,CAAC,CAAC;gBAEtE,QAAQ,GAAG,IAAI,CAAC;gBAChB,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;gBAClB,SAAS;aACV;YAED,IAAI,aAAa,GAAG,EAAE,EAAE;gBACtB,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,YAAY,EAAE;oBACjD,IAAI,CAAC,YAAY,EAAE;wBACjB,YAAY,GAAG,WAAW,CAAC;qBAC5B;oBAED,YAAY,GAAG,IAAI,CAAC;;oBAGpB,MAAM,CAAC,YAAY,EAAE,CAAC,GAAG,QAAQ,CAAC,cAAc,CAAC,KAAK,CAAC,EAAE,EAAE,CAAC,CAAC;oBAC7D,aAAa,GAAG,aAAa,GAAG,CAAC,CAAC;iBACnC;aACF;YAED,IAAI,YAAY;gBAAE,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC;YACxC,IAAI,QAAQ;gBAAE,aAAa,GAAG,aAAa,GAAG,CAAC,CAAC;YAEhD,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;YAC9B,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;SACnB;QAED,IAAI,QAAQ,IAAI,CAAC,WAAW;YAC1B,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;;QAG9E,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;;YAElE,IAAM,KAAK,GAAG,cAAc,CAAC,MAAM,CAAC,EAAE,KAAK,CAAC,CAAC,KAAK,CAAC,cAAc,CAAC,CAAC;;YAGnE,IAAI,CAAC,KAAK,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC;gBAAE,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;;YAGxE,QAAQ,GAAG,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;;YAGlC,KAAK,GAAG,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC;SACjC;;QAGD,IAAI,cAAc,CAAC,KAAK,CAAC;YAAE,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;;;QAI1E,UAAU,GAAG,CAAC,CAAC;QAEf,IAAI,CAAC,aAAa,EAAE;YAClB,UAAU,GAAG,CAAC,CAAC;YACf,SAAS,GAAG,CAAC,CAAC;YACd,MAAM,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;YACd,OAAO,GAAG,CAAC,CAAC;YACZ,aAAa,GAAG,CAAC,CAAC;YAClB,iBAAiB,GAAG,CAAC,CAAC;SACvB;aAAM;YACL,SAAS,GAAG,aAAa,GAAG,CAAC,CAAC;YAC9B,iBAAiB,GAAG,OAAO,CAAC;YAC5B,IAAI,iBAAiB,KAAK,CAAC,EAAE;gBAC3B,OAAO,cAAc,CAAC,YAAY,GAAG,iBAAiB,GAAG,CAAC,CAAC,KAAK,GAAG,EAAE;oBACnE,iBAAiB,GAAG,iBAAiB,GAAG,CAAC,CAAC;iBAC3C;aACF;SACF;;;;;QAOD,IAAI,QAAQ,IAAI,aAAa,IAAI,aAAa,GAAG,QAAQ,GAAG,CAAC,IAAI,EAAE,EAAE;YACnE,QAAQ,GAAG,YAAY,CAAC;SACzB;aAAM;YACL,QAAQ,GAAG,QAAQ,GAAG,aAAa,CAAC;SACrC;;QAGD,OAAO,QAAQ,GAAG,YAAY,EAAE;;YAE9B,SAAS,GAAG,SAAS,GAAG,CAAC,CAAC;YAE1B,IAAI,SAAS,GAAG,UAAU,GAAG,UAAU,EAAE;;gBAEvC,IAAM,YAAY,GAAG,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;gBACrC,IAAI,YAAY,CAAC,KAAK,CAAC,MAAM,CAAC,EAAE;oBAC9B,QAAQ,GAAG,YAAY,CAAC;oBACxB,MAAM;iBACP;gBAED,UAAU,CAAC,cAAc,EAAE,UAAU,CAAC,CAAC;aACxC;YACD,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;SACzB;QAED,OAAO,QAAQ,GAAG,YAAY,IAAI,aAAa,GAAG,OAAO,EAAE;;YAEzD,IAAI,SAAS,KAAK,CAAC,IAAI,iBAAiB,GAAG,aAAa,EAAE;gBACxD,QAAQ,GAAG,YAAY,CAAC;gBACxB,iBAAiB,GAAG,CAAC,CAAC;gBACtB,MAAM;aACP;YAED,IAAI,aAAa,GAAG,OAAO,EAAE;;gBAE3B,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC;aACvB;iBAAM;;gBAEL,SAAS,GAAG,SAAS,GAAG,CAAC,CAAC;aAC3B;YAED,IAAI,QAAQ,GAAG,YAAY,EAAE;gBAC3B,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;aACzB;iBAAM;;gBAEL,IAAM,YAAY,GAAG,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;gBACrC,IAAI,YAAY,CAAC,KAAK,CAAC,MAAM,CAAC,EAAE;oBAC9B,QAAQ,GAAG,YAAY,CAAC;oBACxB,MAAM;iBACP;gBACD,UAAU,CAAC,cAAc,EAAE,UAAU,CAAC,CAAC;aACxC;SACF;;;QAID,IAAI,SAAS,GAAG,UAAU,GAAG,CAAC,GAAG,iBAAiB,EAAE;YAClD,IAAI,WAAW,GAAG,WAAW,CAAC;;;;YAK9B,IAAI,QAAQ,EAAE;gBACZ,YAAY,GAAG,YAAY,GAAG,CAAC,CAAC;gBAChC,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;aAC/B;;YAED,IAAI,UAAU,EAAE;gBACd,YAAY,GAAG,YAAY,GAAG,CAAC,CAAC;gBAChC,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;aAC/B;YAED,IAAM,UAAU,GAAG,QAAQ,CAAC,cAAc,CAAC,YAAY,GAAG,SAAS,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;YAC9E,IAAI,QAAQ,GAAG,CAAC,CAAC;YAEjB,IAAI,UAAU,IAAI,CAAC,EAAE;gBACnB,QAAQ,GAAG,CAAC,CAAC;gBACb,IAAI,UAAU,KAAK,CAAC,EAAE;oBACpB,QAAQ,GAAG,MAAM,CAAC,SAAS,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;oBAC/C,KAAK,CAAC,GAAG,YAAY,GAAG,SAAS,GAAG,CAAC,EAAE,CAAC,GAAG,WAAW,EAAE,CAAC,EAAE,EAAE;wBAC3D,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE;4BACnC,QAAQ,GAAG,CAAC,CAAC;4BACb,MAAM;yBACP;qBACF;iBACF;aACF;YAED,IAAI,QAAQ,EAAE;gBACZ,IAAI,IAAI,GAAG,SAAS,CAAC;gBAErB,OAAO,IAAI,IAAI,CAAC,EAAE,IAAI,EAAE,EAAE;oBACxB,IAAI,EAAE,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,EAAE;wBACtB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;wBAGjB,IAAI,IAAI,KAAK,CAAC,EAAE;4BACd,IAAI,QAAQ,GAAG,YAAY,EAAE;gCAC3B,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;gCACxB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;6BAClB;iCAAM;gCACL,OAAO,IAAI,UAAU,CACnBA,aAAM,CAAC,IAAI,CAAC,UAAU,GAAG,mBAAmB,GAAG,mBAAmB,CAAC,CACpE,CAAC;6BACH;yBACF;qBACF;iBACF;aACF;SACF;;;QAID,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;;QAErC,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;;QAGpC,IAAI,iBAAiB,KAAK,CAAC,EAAE;YAC3B,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;YACrC,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;SACrC;aAAM,IAAI,SAAS,GAAG,UAAU,GAAG,EAAE,EAAE;YACtC,IAAI,IAAI,GAAG,UAAU,CAAC;YACtB,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;YACjD,eAAe,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;YAEjC,OAAO,IAAI,IAAI,SAAS,EAAE,IAAI,EAAE,EAAE;gBAChC,cAAc,GAAG,cAAc,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;gBAC9D,cAAc,GAAG,cAAc,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;aACpE;SACF;aAAM;YACL,IAAI,IAAI,GAAG,UAAU,CAAC;YACtB,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;YAElD,OAAO,IAAI,IAAI,SAAS,GAAG,EAAE,EAAE,IAAI,EAAE,EAAE;gBACrC,eAAe,GAAG,eAAe,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;gBAChE,eAAe,GAAG,eAAe,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;aACtE;YAED,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;YAEjD,OAAO,IAAI,IAAI,SAAS,EAAE,IAAI,EAAE,EAAE;gBAChC,cAAc,GAAG,cAAc,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;gBAC9D,cAAc,GAAG,cAAc,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;aACpE;SACF;QAED,IAAM,WAAW,GAAG,YAAY,CAAC,eAAe,EAAE,WAAI,CAAC,UAAU,CAAC,oBAAoB,CAAC,CAAC,CAAC;QACzF,WAAW,CAAC,GAAG,GAAG,WAAW,CAAC,GAAG,CAAC,GAAG,CAAC,cAAc,CAAC,CAAC;QAEtD,IAAI,QAAQ,CAAC,WAAW,CAAC,GAAG,EAAE,cAAc,CAAC,EAAE;YAC7C,WAAW,CAAC,IAAI,GAAG,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC;SAC7D;;QAGD,cAAc,GAAG,QAAQ,GAAG,aAAa,CAAC;QAC1C,IAAM,GAAG,GAAG,EAAE,GAAG,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,IAAI,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,CAAC;;QAGlE,IACE,WAAW,CAAC,IAAI,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,EAC1F;;YAEA,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,GAAG,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAAC;YAC3D,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CACpB,WAAI,CAAC,UAAU,CAAC,cAAc,CAAC,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAC3E,CAAC;YACF,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,cAAc,CAAC,CAAC,CAAC,CAAC;SAC/E;aAAM;YACL,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,cAAc,GAAG,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAAC;YAC/E,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,eAAe,CAAC,CAAC,CAAC,CAAC;SAChF;QAED,GAAG,CAAC,GAAG,GAAG,WAAW,CAAC,GAAG,CAAC;;QAG1B,IAAI,UAAU,EAAE;YACd,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,qBAAqB,CAAC,CAAC,CAAC;SAChE;;QAGD,IAAMvX,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;QAChC,KAAK,GAAG,CAAC,CAAC;;;QAIVvX,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,GAAG,CAAC,GAAG,GAAG,IAAI,CAAC;QACrCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;QAC5CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;QAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;;QAE7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,GAAG,CAAC,IAAI,GAAG,IAAI,CAAC;QACtCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;QAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;QAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;;QAI9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC;QACtCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;QAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;QAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;;QAE9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC;QACvCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;QAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;QAC/CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;QAG/C,OAAO,IAAI,UAAU,CAACA,QAAM,CAAC,CAAC;KAC/B;;IAGD,6BAAQ,GAAR;;;;QAKE,IAAI,eAAe,CAAC;;QAEpB,IAAI,kBAAkB,GAAG,CAAC,CAAC;;QAE3B,IAAM,WAAW,GAAG,IAAI,KAAK,CAAS,EAAE,CAAC,CAAC;QAC1C,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,WAAW,CAAC,MAAM,EAAE,CAAC,EAAE;YAAE,WAAW,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;;QAEhE,IAAI,KAAK,GAAG,CAAC,CAAC;;QAGd,IAAI,OAAO,GAAG,KAAK,CAAC;;QAGpB,IAAI,eAAe,CAAC;;QAEpB,IAAI,cAAc,GAAgD,EAAE,KAAK,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,EAAE,CAAC;;QAE1F,IAAI,CAAC,EAAE,CAAC,CAAC;;QAGT,IAAM,MAAM,GAAa,EAAE,CAAC;;QAG5B,KAAK,GAAG,CAAC,CAAC;;QAGV,IAAM,MAAM,GAAG,IAAI,CAAC,KAAK,CAAC;;;QAI1B,IAAM,GAAG,GACP,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;QAE/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;;QAI/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;QAE/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;QAG/F,KAAK,GAAG,CAAC,CAAC;;QAGV,IAAM,GAAG,GAAG;YACV,GAAG,EAAE,IAAI,WAAI,CAAC,GAAG,EAAE,IAAI,CAAC;YACxB,IAAI,EAAE,IAAI,WAAI,CAAC,IAAI,EAAE,IAAI,CAAC;SAC3B,CAAC;QAEF,IAAI,GAAG,CAAC,IAAI,CAAC,QAAQ,CAAC,WAAI,CAAC,IAAI,CAAC,EAAE;YAChC,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;SAClB;;;QAID,IAAM,WAAW,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,gBAAgB,CAAC;QAEpD,IAAI,WAAW,IAAI,CAAC,KAAK,CAAC,EAAE;;YAE1B,IAAI,WAAW,KAAK,oBAAoB,EAAE;gBACxC,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,GAAG,UAAU,CAAC;aACrC;iBAAM,IAAI,WAAW,KAAK,eAAe,EAAE;gBAC1C,OAAO,KAAK,CAAC;aACd;iBAAM;gBACL,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,aAAa,CAAC;gBAC/C,eAAe,GAAG,IAAI,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC,CAAC;aAChD;SACF;aAAM;YACL,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;YACtC,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,aAAa,CAAC;SAChD;;QAGD,IAAM,QAAQ,GAAG,eAAe,GAAG,aAAa,CAAC;;;;;QAOjD,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,IAAI,GAAG,MAAM,KAAK,CAAC,eAAe,GAAG,GAAG,KAAK,EAAE,CAAC,CAAC;QAC5E,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC;QAC/B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC;QAC/B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;QAE9B,IACE,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;YAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;YAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;YAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC,EAC7B;YACA,OAAO,GAAG,IAAI,CAAC;SAChB;aAAM;YACL,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;gBACvB,IAAI,YAAY,GAAG,CAAC,CAAC;;gBAErB,IAAM,MAAM,GAAG,UAAU,CAAC,cAAc,CAAC,CAAC;gBAC1C,cAAc,GAAG,MAAM,CAAC,QAAQ,CAAC;gBACjC,YAAY,GAAG,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;;;gBAI9B,IAAI,CAAC,YAAY;oBAAE,SAAS;gBAE5B,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;;oBAEvB,WAAW,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,YAAY,GAAG,EAAE,CAAC;;oBAE3C,YAAY,GAAG,IAAI,CAAC,KAAK,CAAC,YAAY,GAAG,EAAE,CAAC,CAAC;iBAC9C;aACF;SACF;;;;QAMD,IAAI,OAAO,EAAE;YACX,kBAAkB,GAAG,CAAC,CAAC;YACvB,WAAW,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;SACxB;aAAM;YACL,kBAAkB,GAAG,EAAE,CAAC;YACxB,OAAO,CAAC,WAAW,CAAC,KAAK,CAAC,EAAE;gBAC1B,kBAAkB,GAAG,kBAAkB,GAAG,CAAC,CAAC;gBAC5C,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;aACnB;SACF;;QAGD,IAAM,mBAAmB,GAAG,kBAAkB,GAAG,CAAC,GAAG,QAAQ,CAAC;;;;;;;;QAS9D,IAAI,mBAAmB,IAAI,EAAE,IAAI,mBAAmB,IAAI,CAAC,CAAC,IAAI,QAAQ,GAAG,CAAC,EAAE;;;;;YAM1E,IAAI,kBAAkB,GAAG,EAAE,EAAE;gBAC3B,MAAM,CAAC,IAAI,CAAC,KAAG,CAAG,CAAC,CAAC;gBACpB,IAAI,QAAQ,GAAG,CAAC;oBAAE,MAAM,CAAC,IAAI,CAAC,IAAI,GAAG,QAAQ,CAAC,CAAC;qBAC1C,IAAI,QAAQ,GAAG,CAAC;oBAAE,MAAM,CAAC,IAAI,CAAC,GAAG,GAAG,QAAQ,CAAC,CAAC;gBACnD,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;aACxB;YAED,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;YACvC,kBAAkB,GAAG,kBAAkB,GAAG,CAAC,CAAC;YAE5C,IAAI,kBAAkB,EAAE;gBACtB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;aAClB;YAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,EAAE,CAAC,EAAE,EAAE;gBAC3C,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;aACxC;;YAGD,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;YACjB,IAAI,mBAAmB,GAAG,CAAC,EAAE;gBAC3B,MAAM,CAAC,IAAI,CAAC,GAAG,GAAG,mBAAmB,CAAC,CAAC;aACxC;iBAAM;gBACL,MAAM,CAAC,IAAI,CAAC,KAAG,mBAAqB,CAAC,CAAC;aACvC;SACF;aAAM;;YAEL,IAAI,QAAQ,IAAI,CAAC,EAAE;gBACjB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,EAAE,CAAC,EAAE,EAAE;oBAC3C,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;iBACxC;aACF;iBAAM;gBACL,IAAI,cAAc,GAAG,kBAAkB,GAAG,QAAQ,CAAC;;gBAGnD,IAAI,cAAc,GAAG,CAAC,EAAE;oBACtB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,cAAc,EAAE,CAAC,EAAE,EAAE;wBACvC,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;qBACxC;iBACF;qBAAM;oBACL,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;iBAClB;gBAED,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;gBAEjB,OAAO,cAAc,EAAE,GAAG,CAAC,EAAE;oBAC3B,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;iBAClB;gBAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,GAAG,IAAI,CAAC,GAAG,CAAC,cAAc,GAAG,CAAC,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,EAAE;oBAC7E,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;iBACxC;aACF;SACF;QAED,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;KACxB;IAED,2BAAM,GAAN;QACE,OAAO,EAAE,cAAc,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;KAC5C;;IAGD,mCAAc,GAAd;QACE,OAAO,EAAE,cAAc,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;KAC5C;;IAGM,2BAAgB,GAAvB,UAAwB,GAAuB;QAC7C,OAAO,UAAU,CAAC,UAAU,CAAC,GAAG,CAAC,cAAc,CAAC,CAAC;KAClD;;IAGD,qBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,4BAAO,GAAP;QACE,OAAO,sBAAmB,IAAI,CAAC,QAAQ,EAAE,QAAI,CAAC;KAC/C;IACH,iBAAC;AAAD,CAAC,IAAA;AAnoBY,gCAAU;AAqoBvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,YAAY,EAAE,CAAC,CAAC;;;;;;;;;;AClyBlF;;;;AAIA;;;;;;IASE,gBAAY,KAAa;QACvB,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,CAAC,KAAK,CAAC,CAAC;QAExD,IAAK,KAAiB,YAAY,MAAM,EAAE;YACxC,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;SACzB;QAED,IAAI,CAAC,KAAK,GAAG,CAAC,KAAK,CAAC;KACrB;;;;;;IAOD,wBAAO,GAAP;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,uBAAM,GAAN;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,+BAAc,GAAd,UAAe,OAAsB;QACnC,IAAI,OAAO,KAAK,OAAO,CAAC,MAAM,KAAK,OAAO,CAAC,OAAO,IAAI,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE;YAC5E,OAAO,IAAI,CAAC,KAAK,CAAC;SACnB;;;QAID,IAAI,MAAM,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,CAAC,EAAE;YACxC,OAAO,EAAE,aAAa,EAAE,MAAI,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAG,EAAE,CAAC;SACvD;QAED,IAAI,aAAqB,CAAC;QAC1B,IAAI,MAAM,CAAC,SAAS,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE;YAChC,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;YACtC,IAAI,aAAa,CAAC,MAAM,IAAI,EAAE,EAAE;gBAC9B,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,aAAa,CAAC,EAAE,CAAC,CAAC,WAAW,EAAE,CAAC;aAC5D;SACF;aAAM;YACL,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,QAAQ,EAAE,CAAC;SACvC;QAED,OAAO,EAAE,aAAa,eAAA,EAAE,CAAC;KAC1B;;IAGM,uBAAgB,GAAvB,UAAwB,GAAmB,EAAE,OAAsB;QACjE,IAAM,WAAW,GAAG,UAAU,CAAC,GAAG,CAAC,aAAa,CAAC,CAAC;QAClD,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,WAAW,GAAG,IAAI,MAAM,CAAC,WAAW,CAAC,CAAC;KAC3E;;IAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,wBAAO,GAAP;QACE,IAAM,KAAK,GAAG,IAAI,CAAC,cAAc,EAAoB,CAAC;QACtD,OAAO,gBAAc,KAAK,CAAC,aAAa,MAAG,CAAC;KAC7C;IACH,aAAC;AAAD,CAAC,IAAA;AAzEY,wBAAM;AA2EnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;AC/E1E;;;;AAIA;;;;;;IASE,eAAY,KAAsB;QAChC,IAAI,EAAE,IAAI,YAAY,KAAK,CAAC;YAAE,OAAO,IAAI,KAAK,CAAC,KAAK,CAAC,CAAC;QAEtD,IAAK,KAAiB,YAAY,MAAM,EAAE;YACxC,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;SACzB;QAED,IAAI,CAAC,KAAK,GAAG,CAAC,KAAK,CAAC;KACrB;;;;;;IAOD,uBAAO,GAAP;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,sBAAM,GAAN;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,8BAAc,GAAd,UAAe,OAAsB;QACnC,IAAI,OAAO,KAAK,OAAO,CAAC,OAAO,IAAI,OAAO,CAAC,MAAM,CAAC;YAAE,OAAO,IAAI,CAAC,KAAK,CAAC;QACtE,OAAO,EAAE,UAAU,EAAE,IAAI,CAAC,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;KAC9C;;IAGM,sBAAgB,GAAvB,UAAwB,GAAkB,EAAE,OAAsB;QAChE,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,QAAQ,CAAC,GAAG,CAAC,UAAU,EAAE,EAAE,CAAC,GAAG,IAAI,KAAK,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC;KAC9F;;IAGD,gBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,uBAAO,GAAP;QACE,OAAO,eAAa,IAAI,CAAC,OAAO,EAAE,MAAG,CAAC;KACvC;IACH,YAAC;AAAD,CAAC,IAAA;AApDY,sBAAK;AAsDlB,MAAM,CAAC,cAAc,CAAC,KAAK,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,OAAO,EAAE,CAAC,CAAC;;;;;;;;;;AC5DxE;;;;AAIA;IAGE;QACE,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,EAAE,CAAC;KACpD;;IAGD,+BAAc,GAAd;QACE,OAAO,EAAE,OAAO,EAAE,CAAC,EAAE,CAAC;KACvB;;IAGM,uBAAgB,GAAvB;QACE,OAAO,IAAI,MAAM,EAAE,CAAC;KACrB;;IAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,wBAAO,GAAP;QACE,OAAO,cAAc,CAAC;KACvB;IACH,aAAC;AAAD,CAAC,IAAA;AAzBY,wBAAM;AA2BnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;AC/B1E;;;;AAIA;IAGE;QACE,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,EAAE,CAAC;KACpD;;IAGD,+BAAc,GAAd;QACE,OAAO,EAAE,OAAO,EAAE,CAAC,EAAE,CAAC;KACvB;;IAGM,uBAAgB,GAAvB;QACE,OAAO,IAAI,MAAM,EAAE,CAAC;KACrB;;IAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,wBAAO,GAAP;QACE,OAAO,cAAc,CAAC;KACvB;IACH,aAAC;AAAD,CAAC,IAAA;AAzBY,wBAAM;AA2BnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;ACpC1C;AACe;AACuB;AAEtE;AACA,IAAM,iBAAiB,GAAG,IAAI,MAAM,CAAC,mBAAmB,CAAC,CAAC;AAE1D;AACA,IAAI,cAAc,GAAsB,IAAI,CAAC;AAc7C,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;AAEzB;;;;AAIA;;;;;;IAkBE,kBAAY,EAAuD;QACjE,IAAI,EAAE,IAAI,YAAY,QAAQ,CAAC;YAAE,OAAO,IAAI,QAAQ,CAAC,EAAE,CAAC,CAAC;;QAGzD,IAAI,EAAE,YAAY,QAAQ,EAAE;YAC1B,IAAI,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,CAAC;YAClB,IAAI,CAAC,IAAI,GAAG,EAAE,CAAC,IAAI,CAAC;SACrB;QAED,IAAI,OAAO,EAAE,KAAK,QAAQ,IAAI,EAAE,IAAI,IAAI,IAAI,EAAE,EAAE;YAC9C,IAAI,aAAa,IAAI,EAAE,IAAI,OAAO,EAAE,CAAC,WAAW,KAAK,UAAU,EAAE;gBAC/D,IAAI,CAAC,GAAG,CAAC,GAAGuX,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,EAAE,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM;gBACL,IAAI,CAAC,GAAG,CAAC,GAAG,OAAO,EAAE,CAAC,EAAE,KAAK,QAAQ,GAAGA,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,EAAE,CAAC,EAAE,CAAC;aACpE;SACF;;QAGD,IAAI,EAAE,IAAI,IAAI,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;;YAExC,IAAI,CAAC,GAAG,CAAC,GAAG,QAAQ,CAAC,QAAQ,CAAC,OAAO,EAAE,KAAK,QAAQ,GAAG,EAAE,GAAG,SAAS,CAAC,CAAC;;YAEvE,IAAI,QAAQ,CAAC,cAAc,EAAE;gBAC3B,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aACrC;SACF;QAED,IAAI,WAAW,CAAC,MAAM,CAAC,EAAE,CAAC,IAAI,EAAE,CAAC,UAAU,KAAK,EAAE,EAAE;YAClD,IAAI,CAAC,GAAG,CAAC,GAAGE,0BAAY,CAAC,EAAE,CAAC,CAAC;SAC9B;QAED,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;YAC1B,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,EAAE;gBACpB,IAAM,KAAK,GAAGF,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;gBAC9B,IAAI,KAAK,CAAC,UAAU,KAAK,EAAE,EAAE;oBAC3B,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;iBACnB;aACF;iBAAM,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE;gBACzD,IAAI,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,IAAI,CAAC,EAAE,EAAE,KAAK,CAAC,CAAC;aACpC;iBAAM;gBACL,MAAM,IAAI,SAAS,CACjB,4FAA4F,CAC7F,CAAC;aACH;SACF;QAED,IAAI,QAAQ,CAAC,cAAc,EAAE;YAC3B,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;SACrC;KACF;IAMD,sBAAI,wBAAE;;;;;aAAN;YACE,OAAO,IAAI,CAAC,GAAG,CAAC,CAAC;SAClB;aAED,UAAO,KAAa;YAClB,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;YAClB,IAAI,QAAQ,CAAC,cAAc,EAAE;gBAC3B,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aACnC;SACF;;;OAPA;IAaD,sBAAI,oCAAc;;;;;aAAlB;YACE,OAAO,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,CAAC,CAAC,CAAC;SAC/B;aAED,UAAmB,KAAa;;YAE9B,IAAI,CAAC,EAAE,CAAC,aAAa,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC;SACjC;;;OALA;;IAQD,8BAAW,GAAX;QACE,IAAI,QAAQ,CAAC,cAAc,IAAI,IAAI,CAAC,IAAI,EAAE;YACxC,OAAO,IAAI,CAAC,IAAI,CAAC;SAClB;QAED,IAAM,SAAS,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;QAE1C,IAAI,QAAQ,CAAC,cAAc,IAAI,CAAC,IAAI,CAAC,IAAI,EAAE;YACzC,IAAI,CAAC,IAAI,GAAG,SAAS,CAAC;SACvB;QAED,OAAO,SAAS,CAAC;KAClB;;;;;;;IAQM,eAAM,GAAb;QACE,QAAQ,QAAQ,CAAC,KAAK,GAAG,CAAC,QAAQ,CAAC,KAAK,GAAG,CAAC,IAAI,QAAQ,EAAE;KAC3D;;;;;;IAOM,iBAAQ,GAAf,UAAgB,IAAa;QAC3B,IAAI,QAAQ,KAAK,OAAO,IAAI,EAAE;YAC5B,IAAI,GAAG,CAAC,EAAE,IAAI,CAAC,GAAG,EAAE,GAAG,IAAI,CAAC,CAAC;SAC9B;QAED,IAAM,GAAG,GAAG,QAAQ,CAAC,MAAM,EAAE,CAAC;QAC9B,IAAMvX,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;;QAGhCvX,QAAM,CAAC,aAAa,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;;QAG9B,IAAI,cAAc,KAAK,IAAI,EAAE;YAC3B,cAAc,GAAGwX,iBAAW,CAAC,CAAC,CAAC,CAAC;SACjC;;QAGDxX,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;;QAG9BA,QAAM,CAAC,EAAE,CAAC,GAAG,GAAG,GAAG,IAAI,CAAC;QACxBA,QAAM,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;QAC/BA,QAAM,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;QAE/B,OAAOA,QAAM,CAAC;KACf;;;;;;;IAQD,2BAAQ,GAAR,UAAS,MAAe;;QAEtB,IAAI,MAAM;YAAE,OAAO,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC;QAC5C,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;KAC3B;;;;;IAMD,yBAAM,GAAN;QACE,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;KAC3B;;;;;;IAOD,yBAAM,GAAN,UAAO,OAAyC;QAC9C,IAAI,OAAO,KAAK,SAAS,IAAI,OAAO,KAAK,IAAI,EAAE;YAC7C,OAAO,KAAK,CAAC;SACd;QAED,IAAI,OAAO,YAAY,QAAQ,EAAE;YAC/B,OAAO,IAAI,CAAC,QAAQ,EAAE,KAAK,OAAO,CAAC,QAAQ,EAAE,CAAC;SAC/C;QAED,IACE,OAAO,OAAO,KAAK,QAAQ;YAC3B,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC;YACzB,OAAO,CAAC,MAAM,KAAK,EAAE;YACrBwX,kBAAY,CAAC,IAAI,CAAC,EAAE,CAAC,EACrB;YACA,OAAO,OAAO,KAAKD,aAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,IAAI,CAAC,EAAE,EAAE,QAAQ,CAAC,CAAC;SACtE;QAED,IAAI,OAAO,OAAO,KAAK,QAAQ,IAAI,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC,IAAI,OAAO,CAAC,MAAM,KAAK,EAAE,EAAE;YACrF,OAAO,OAAO,CAAC,WAAW,EAAE,KAAK,IAAI,CAAC,WAAW,EAAE,CAAC;SACrD;QAED,IAAI,OAAO,OAAO,KAAK,QAAQ,IAAI,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC,IAAI,OAAO,CAAC,MAAM,KAAK,EAAE,EAAE;YACrF,OAAOA,aAAM,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;SAC7C;QAED,IACE,OAAO,OAAO,KAAK,QAAQ;YAC3B,aAAa,IAAI,OAAO;YACxB,OAAO,OAAO,CAAC,WAAW,KAAK,UAAU,EACzC;YACA,OAAO,OAAO,CAAC,WAAW,EAAE,KAAK,IAAI,CAAC,WAAW,EAAE,CAAC;SACrD;QAED,OAAO,KAAK,CAAC;KACd;;IAGD,+BAAY,GAAZ;QACE,IAAM,SAAS,GAAG,IAAI,IAAI,EAAE,CAAC;QAC7B,IAAM,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,YAAY,CAAC,CAAC,CAAC,CAAC;QACrC,SAAS,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,CAAC;QAC3C,OAAO,SAAS,CAAC;KAClB;;IAGM,iBAAQ,GAAf;QACE,OAAO,IAAI,QAAQ,EAAE,CAAC;KACvB;;;;;;IAOM,uBAAc,GAArB,UAAsB,IAAY;QAChC,IAAMvX,QAAM,GAAGuX,aAAM,CAAC,IAAI,CAAC,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;;QAEjEvX,QAAM,CAAC,aAAa,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;;QAE9B,OAAO,IAAI,QAAQ,CAACA,QAAM,CAAC,CAAC;KAC7B;;;;;;IAOM,4BAAmB,GAA1B,UAA2B,SAAiB;;QAE1C,IAAI,OAAO,SAAS,KAAK,WAAW,KAAK,SAAS,IAAI,IAAI,IAAI,SAAS,CAAC,MAAM,KAAK,EAAE,CAAC,EAAE;YACtF,MAAM,IAAI,SAAS,CACjB,yFAAyF,CAC1F,CAAC;SACH;QAED,OAAO,IAAI,QAAQ,CAACuX,aAAM,CAAC,IAAI,CAAC,SAAS,EAAE,KAAK,CAAC,CAAC,CAAC;KACpD;;;;;;IAOM,gBAAO,GAAd,UAAe,EAA0D;QACvE,IAAI,EAAE,IAAI,IAAI;YAAE,OAAO,KAAK,CAAC;QAE7B,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;YAC1B,OAAO,IAAI,CAAC;SACb;QAED,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;YAC1B,OAAO,EAAE,CAAC,MAAM,KAAK,EAAE,KAAK,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC;SAC7E;QAED,IAAI,EAAE,YAAY,QAAQ,EAAE;YAC1B,OAAO,IAAI,CAAC;SACb;QAED,IAAIC,kBAAY,CAAC,EAAE,CAAC,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,EAAE;YACxC,OAAO,IAAI,CAAC;SACb;;QAGD,IAAI,OAAO,EAAE,KAAK,QAAQ,IAAI,aAAa,IAAI,EAAE,IAAI,OAAO,EAAE,CAAC,WAAW,KAAK,UAAU,EAAE;YACzF,IAAI,OAAO,EAAE,CAAC,EAAE,KAAK,QAAQ,EAAE;gBAC7B,OAAO,EAAE,CAAC,EAAE,CAAC,MAAM,KAAK,EAAE,CAAC;aAC5B;YACD,OAAO,EAAE,CAAC,WAAW,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC;SACxF;QAED,OAAO,KAAK,CAAC;KACd;;IAGD,iCAAc,GAAd;QACE,IAAI,IAAI,CAAC,WAAW;YAAE,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,WAAW,EAAE,EAAE,CAAC;QAC1D,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,EAAE,CAAC;KACvC;;IAGM,yBAAgB,GAAvB,UAAwB,GAAqB;QAC3C,OAAO,IAAI,QAAQ,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;KAC/B;;;;;;;IAQD,mBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,0BAAO,GAAP;QACE,OAAO,oBAAiB,IAAI,CAAC,WAAW,EAAE,QAAI,CAAC;KAChD;;IA5TM,cAAK,GAAG,CAAC,EAAE,IAAI,CAAC,MAAM,EAAE,GAAG,QAAQ,CAAC,CAAC;IA6T9C,eAAC;CAjUD,IAiUC;AAjUY,4BAAQ;AAmUrB;AACA,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,UAAU,EAAE;IACpD,KAAK,EAAEA,eAAS,CACd,UAAC,IAAY,IAAK,OAAA,QAAQ,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAA,EACzC,yDAAyD,CAC1D;CACF,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,QAAQ,EAAE;IAClD,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;CAC/F,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,SAAS,EAAE;IACnD,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;CAC/F,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,EAAE,SAAS,EAAE;IACzC,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;CAC/F,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,UAAU,EAAE,CAAC,CAAC;;;;;;;;;;ACjX9E,SAAS,WAAW,CAAC,GAAW;IAC9B,OAAO,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;AACvC,CAAC;AAgBD;;;;AAIA;;;;;IASE,oBAAY,OAAe,EAAE,OAAgB;QAC3C,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;YAAE,OAAO,IAAI,UAAU,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;QAE3E,IAAI,CAAC,OAAO,GAAG,OAAO,CAAC;QACvB,IAAI,CAAC,OAAO,GAAG,WAAW,CAAC,OAAO,aAAP,OAAO,cAAP,OAAO,GAAI,EAAE,CAAC,CAAC;;QAG1C,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,OAAO,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;YAC5C,IACE,EACE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG,CACxB,EACD;gBACA,MAAM,IAAI,KAAK,CAAC,oCAAkC,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,uBAAoB,CAAC,CAAC;aACxF;SACF;KACF;IAEM,uBAAY,GAAnB,UAAoB,OAAgB;QAClC,OAAO,OAAO,GAAG,OAAO,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,GAAG,EAAE,CAAC;KACzD;;IAGD,mCAAc,GAAd,UAAe,OAAsB;QACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO,EAAE,MAAM,EAAE,IAAI,CAAC,OAAO,EAAE,QAAQ,EAAE,IAAI,CAAC,OAAO,EAAE,CAAC;SACzD;QACD,OAAO,EAAE,kBAAkB,EAAE,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,EAAE,CAAC;KACjF;;IAGM,2BAAgB,GAAvB,UAAwB,GAAkD;QACxE,IAAI,QAAQ,IAAI,GAAG,EAAE;YACnB,IAAI,OAAO,GAAG,CAAC,MAAM,KAAK,QAAQ,EAAE;;gBAElC,IAAI,GAAG,CAAC,MAAM,CAAC,SAAS,KAAK,YAAY,EAAE;oBACzC,OAAQ,GAA6B,CAAC;iBACvC;aACF;iBAAM;gBACL,OAAO,IAAI,UAAU,CAAC,GAAG,CAAC,MAAM,EAAE,UAAU,CAAC,YAAY,CAAC,GAAG,CAAC,QAAQ,CAAC,CAAC,CAAC;aAC1E;SACF;QACD,IAAI,oBAAoB,IAAI,GAAG,EAAE;YAC/B,OAAO,IAAI,UAAU,CACnB,GAAG,CAAC,kBAAkB,CAAC,OAAO,EAC9B,UAAU,CAAC,YAAY,CAAC,GAAG,CAAC,kBAAkB,CAAC,OAAO,CAAC,CACxD,CAAC;SACH;QACD,MAAM,IAAI,SAAS,CAAC,8CAA4C,IAAI,CAAC,SAAS,CAAC,GAAG,CAAG,CAAC,CAAC;KACxF;IACH,iBAAC;AAAD,CAAC,IAAA;AAjEY,gCAAU;AAmEvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,YAAY,EAAE,CAAC,CAAC;;;;;;;;;;ACtFlF;;;;AAIA;;;;IAOE,oBAAY,KAAa;QACvB,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;YAAE,OAAO,IAAI,UAAU,CAAC,KAAK,CAAC,CAAC;QAEhE,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;KACpB;;IAGD,4BAAO,GAAP;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,6BAAQ,GAAR;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,4BAAO,GAAP;QACE,OAAO,sBAAmB,IAAI,CAAC,KAAK,QAAI,CAAC;KAC1C;;IAGD,2BAAM,GAAN;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,mCAAc,GAAd;QACE,OAAO,EAAE,OAAO,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;KAChC;;IAGM,2BAAgB,GAAvB,UAAwB,GAAuB;QAC7C,OAAO,IAAI,UAAU,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;KACpC;;IAGD,qBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IACH,iBAAC;AAAD,CAAC,IAAA;AA/CY,gCAAU;AAiDvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;AC1D9E;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;AACA;AAEA,IAAIK,cAAa,GAAG,uBAASlX,CAAT,EAAYoD,CAAZ,EAAe;AAC/B8T,EAAAA,cAAa,GAAGxV,MAAM,CAACC,cAAP,IACX;AAAEwV,IAAAA,SAAS,EAAE;AAAb,eAA6B5Z,KAA7B,IAAsC,UAAUyC,CAAV,EAAaoD,CAAb,EAAgB;AAAEpD,IAAAA,CAAC,CAACmX,SAAF,GAAc/T,CAAd;AAAkB,GAD/D,IAEZ,UAAUpD,CAAV,EAAaoD,CAAb,EAAgB;AAAE,SAAK,IAAIgU,CAAT,IAAchU,CAAd;AAAiB,UAAIA,CAAC,CAAC2R,cAAF,CAAiBqC,CAAjB,CAAJ,EAAyBpX,CAAC,CAACoX,CAAD,CAAD,GAAOhU,CAAC,CAACgU,CAAD,CAAR;AAA1C;AAAwD,GAF9E;;AAGA,SAAOF,cAAa,CAAClX,CAAD,EAAIoD,CAAJ,CAApB;AACH,CALD;;AAOO,SAASiU,SAAT,CAAmBrX,CAAnB,EAAsBoD,CAAtB,EAAyB;AAC5B8T,EAAAA,cAAa,CAAClX,CAAD,EAAIoD,CAAJ,CAAb;;AACA,WAASkU,EAAT,GAAc;AAAE,SAAKjI,WAAL,GAAmBrP,CAAnB;AAAuB;;AACvCA,EAAAA,CAAC,CAAC4B,SAAF,GAAcwB,CAAC,KAAK,IAAN,GAAa1B,MAAM,CAAC0O,MAAP,CAAchN,CAAd,CAAb,IAAiCkU,EAAE,CAAC1V,SAAH,GAAewB,CAAC,CAACxB,SAAjB,EAA4B,IAAI0V,EAAJ,EAA7D,CAAd;AACH;;AAEM,IAAIC,OAAQ,GAAG,oBAAW;AAC7BA,EAAAA,OAAQ,GAAG7V,MAAM,CAAC8V,MAAP,IAAiB,SAASD,QAAT,CAAkBE,CAAlB,EAAqB;AAC7C,SAAK,IAAIxX,CAAJ,EAAOxC,CAAC,GAAG,CAAX,EAAc8I,CAAC,GAAGZ,SAAS,CAAChI,MAAjC,EAAyCF,CAAC,GAAG8I,CAA7C,EAAgD9I,CAAC,EAAjD,EAAqD;AACjDwC,MAAAA,CAAC,GAAG0F,SAAS,CAAClI,CAAD,CAAb;;AACA,WAAK,IAAI2Z,CAAT,IAAcnX,CAAd;AAAiB,YAAIyB,MAAM,CAACE,SAAP,CAAiBmT,cAAjB,CAAgCtP,IAAhC,CAAqCxF,CAArC,EAAwCmX,CAAxC,CAAJ,EAAgDK,CAAC,CAACL,CAAD,CAAD,GAAOnX,CAAC,CAACmX,CAAD,CAAR;AAAjE;AACH;;AACD,WAAOK,CAAP;AACH,GAND;;AAOA,SAAOF,OAAQ,CAAC5Q,KAAT,CAAe,IAAf,EAAqBhB,SAArB,CAAP;AACH,CATM;AAWA,SAAS+R,MAAT,CAAgBzX,CAAhB,EAAmBP,CAAnB,EAAsB;AACzB,MAAI+X,CAAC,GAAG,EAAR;;AACA,OAAK,IAAIL,CAAT,IAAcnX,CAAd;AAAiB,QAAIyB,MAAM,CAACE,SAAP,CAAiBmT,cAAjB,CAAgCtP,IAAhC,CAAqCxF,CAArC,EAAwCmX,CAAxC,KAA8C1X,CAAC,CAACzB,OAAF,CAAUmZ,CAAV,IAAe,CAAjE,EACbK,CAAC,CAACL,CAAD,CAAD,GAAOnX,CAAC,CAACmX,CAAD,CAAR;AADJ;;AAEA,MAAInX,CAAC,IAAI,IAAL,IAAa,OAAOyB,MAAM,CAACiW,qBAAd,KAAwC,UAAzD,EACI,KAAK,IAAIla,CAAC,GAAG,CAAR,EAAW2Z,CAAC,GAAG1V,MAAM,CAACiW,qBAAP,CAA6B1X,CAA7B,CAApB,EAAqDxC,CAAC,GAAG2Z,CAAC,CAACzZ,MAA3D,EAAmEF,CAAC,EAApE,EAAwE;AACpE,QAAIiC,CAAC,CAACzB,OAAF,CAAUmZ,CAAC,CAAC3Z,CAAD,CAAX,IAAkB,CAAlB,IAAuBiE,MAAM,CAACE,SAAP,CAAiBgW,oBAAjB,CAAsCnS,IAAtC,CAA2CxF,CAA3C,EAA8CmX,CAAC,CAAC3Z,CAAD,CAA/C,CAA3B,EACIga,CAAC,CAACL,CAAC,CAAC3Z,CAAD,CAAF,CAAD,GAAUwC,CAAC,CAACmX,CAAC,CAAC3Z,CAAD,CAAF,CAAX;AACP;AACL,SAAOga,CAAP;AACH;AAEM,SAASI,UAAT,CAAoBC,UAApB,EAAgC1Q,MAAhC,EAAwCoN,GAAxC,EAA6CS,IAA7C,EAAmD;AACtD,MAAI1U,CAAC,GAAGoF,SAAS,CAAChI,MAAlB;AAAA,MAA0Boa,CAAC,GAAGxX,CAAC,GAAG,CAAJ,GAAQ6G,MAAR,GAAiB6N,IAAI,KAAK,IAAT,GAAgBA,IAAI,GAAGvT,MAAM,CAACwT,wBAAP,CAAgC9N,MAAhC,EAAwCoN,GAAxC,CAAvB,GAAsES,IAArH;AAAA,MAA2HjV,CAA3H;AACA,MAAI,QAAOgY,OAAP,wDAAOA,OAAP,OAAmB,QAAnB,IAA+B,OAAOA,OAAO,CAACC,QAAf,KAA4B,UAA/D,EAA2EF,CAAC,GAAGC,OAAO,CAACC,QAAR,CAAiBH,UAAjB,EAA6B1Q,MAA7B,EAAqCoN,GAArC,EAA0CS,IAA1C,CAAJ,CAA3E,KACK,KAAK,IAAIxX,CAAC,GAAGqa,UAAU,CAACna,MAAX,GAAoB,CAAjC,EAAoCF,CAAC,IAAI,CAAzC,EAA4CA,CAAC,EAA7C;AAAiD,QAAIuC,CAAC,GAAG8X,UAAU,CAACra,CAAD,CAAlB,EAAuBsa,CAAC,GAAG,CAACxX,CAAC,GAAG,CAAJ,GAAQP,CAAC,CAAC+X,CAAD,CAAT,GAAexX,CAAC,GAAG,CAAJ,GAAQP,CAAC,CAACoH,MAAD,EAASoN,GAAT,EAAcuD,CAAd,CAAT,GAA4B/X,CAAC,CAACoH,MAAD,EAASoN,GAAT,CAA7C,KAA+DuD,CAAnE;AAAxE;AACL,SAAOxX,CAAC,GAAG,CAAJ,IAASwX,CAAT,IAAcrW,MAAM,CAACG,cAAP,CAAsBuF,MAAtB,EAA8BoN,GAA9B,EAAmCuD,CAAnC,CAAd,EAAqDA,CAA5D;AACH;AAEM,SAASG,OAAT,CAAiBC,UAAjB,EAA6BC,SAA7B,EAAwC;AAC3C,SAAO,UAAUhR,MAAV,EAAkBoN,GAAlB,EAAuB;AAAE4D,IAAAA,SAAS,CAAChR,MAAD,EAASoN,GAAT,EAAc2D,UAAd,CAAT;AAAqC,GAArE;AACH;AAEM,SAASE,UAAT,CAAoBC,WAApB,EAAiCC,aAAjC,EAAgD;AACnD,MAAI,QAAOP,OAAP,wDAAOA,OAAP,OAAmB,QAAnB,IAA+B,OAAOA,OAAO,CAACQ,QAAf,KAA4B,UAA/D,EAA2E,OAAOR,OAAO,CAACQ,QAAR,CAAiBF,WAAjB,EAA8BC,aAA9B,CAAP;AAC9E;AAEM,SAASE,SAAT,CAAmBC,OAAnB,EAA4BC,UAA5B,EAAwCC,CAAxC,EAA2CC,SAA3C,EAAsD;AACzD,WAASC,KAAT,CAAexY,KAAf,EAAsB;AAAE,WAAOA,KAAK,YAAYsY,CAAjB,GAAqBtY,KAArB,GAA6B,IAAIsY,CAAJ,CAAM,UAAUG,OAAV,EAAmB;AAAEA,MAAAA,OAAO,CAACzY,KAAD,CAAP;AAAiB,KAA5C,CAApC;AAAoF;;AAC5G,SAAO,KAAKsY,CAAC,KAAKA,CAAC,GAAGI,OAAT,CAAN,EAAyB,UAAUD,OAAV,EAAmBE,MAAnB,EAA2B;AACvD,aAASC,SAAT,CAAmB5Y,KAAnB,EAA0B;AAAE,UAAI;AAAE6Y,QAAAA,IAAI,CAACN,SAAS,CAACO,IAAV,CAAe9Y,KAAf,CAAD,CAAJ;AAA8B,OAApC,CAAqC,OAAOZ,CAAP,EAAU;AAAEuZ,QAAAA,MAAM,CAACvZ,CAAD,CAAN;AAAY;AAAE;;AAC3F,aAAS2Z,QAAT,CAAkB/Y,KAAlB,EAAyB;AAAE,UAAI;AAAE6Y,QAAAA,IAAI,CAACN,SAAS,CAAC,OAAD,CAAT,CAAmBvY,KAAnB,CAAD,CAAJ;AAAkC,OAAxC,CAAyC,OAAOZ,CAAP,EAAU;AAAEuZ,QAAAA,MAAM,CAACvZ,CAAD,CAAN;AAAY;AAAE;;AAC9F,aAASyZ,IAAT,CAAcG,MAAd,EAAsB;AAAEA,MAAAA,MAAM,CAACC,IAAP,GAAcR,OAAO,CAACO,MAAM,CAAChZ,KAAR,CAArB,GAAsCwY,KAAK,CAACQ,MAAM,CAAChZ,KAAR,CAAL,CAAoBkZ,IAApB,CAAyBN,SAAzB,EAAoCG,QAApC,CAAtC;AAAsF;;AAC9GF,IAAAA,IAAI,CAAC,CAACN,SAAS,GAAGA,SAAS,CAAClS,KAAV,CAAgB+R,OAAhB,EAAyBC,UAAU,IAAI,EAAvC,CAAb,EAAyDS,IAAzD,EAAD,CAAJ;AACH,GALM,CAAP;AAMH;AAEM,SAASK,WAAT,CAAqBf,OAArB,EAA8BgB,IAA9B,EAAoC;AACvC,MAAIvI,CAAC,GAAG;AAAEwI,IAAAA,KAAK,EAAE,CAAT;AAAYC,IAAAA,IAAI,EAAE,gBAAW;AAAE,UAAInC,CAAC,CAAC,CAAD,CAAD,GAAO,CAAX,EAAc,MAAMA,CAAC,CAAC,CAAD,CAAP;AAAY,aAAOA,CAAC,CAAC,CAAD,CAAR;AAAc,KAAvE;AAAyEoC,IAAAA,IAAI,EAAE,EAA/E;AAAmFC,IAAAA,GAAG,EAAE;AAAxF,GAAR;AAAA,MAAsGjJ,CAAtG;AAAA,MAAyG5L,CAAzG;AAAA,MAA4GwS,CAA5G;AAAA,MAA+GsC,CAA/G;AACA,SAAOA,CAAC,GAAG;AAAEX,IAAAA,IAAI,EAAEY,IAAI,CAAC,CAAD,CAAZ;AAAiB,aAASA,IAAI,CAAC,CAAD,CAA9B;AAAmC,cAAUA,IAAI,CAAC,CAAD;AAAjD,GAAJ,EAA4D,OAAOjZ,MAAP,KAAkB,UAAlB,KAAiCgZ,CAAC,CAAChZ,MAAM,CAACkZ,QAAR,CAAD,GAAqB,YAAW;AAAE,WAAO,IAAP;AAAc,GAAjF,CAA5D,EAAgJF,CAAvJ;;AACA,WAASC,IAAT,CAAczT,CAAd,EAAiB;AAAE,WAAO,UAAU2T,CAAV,EAAa;AAAE,aAAOf,IAAI,CAAC,CAAC5S,CAAD,EAAI2T,CAAJ,CAAD,CAAX;AAAsB,KAA5C;AAA+C;;AAClE,WAASf,IAAT,CAAcgB,EAAd,EAAkB;AACd,QAAItJ,CAAJ,EAAO,MAAM,IAAIrO,SAAJ,CAAc,iCAAd,CAAN;;AACP,WAAO2O,CAAP;AAAU,UAAI;AACV,YAAIN,CAAC,GAAG,CAAJ,EAAO5L,CAAC,KAAKwS,CAAC,GAAG0C,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAR,GAAYlV,CAAC,CAAC,QAAD,CAAb,GAA0BkV,EAAE,CAAC,CAAD,CAAF,GAAQlV,CAAC,CAAC,OAAD,CAAD,KAAe,CAACwS,CAAC,GAAGxS,CAAC,CAAC,QAAD,CAAN,KAAqBwS,CAAC,CAAChS,IAAF,CAAOR,CAAP,CAArB,EAAgC,CAA/C,CAAR,GAA4DA,CAAC,CAACmU,IAAjG,CAAD,IAA2G,CAAC,CAAC3B,CAAC,GAAGA,CAAC,CAAChS,IAAF,CAAOR,CAAP,EAAUkV,EAAE,CAAC,CAAD,CAAZ,CAAL,EAAuBZ,IAA9I,EAAoJ,OAAO9B,CAAP;AACpJ,YAAIxS,CAAC,GAAG,CAAJ,EAAOwS,CAAX,EAAc0C,EAAE,GAAG,CAACA,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAT,EAAY1C,CAAC,CAACnX,KAAd,CAAL;;AACd,gBAAQ6Z,EAAE,CAAC,CAAD,CAAV;AACI,eAAK,CAAL;AAAQ,eAAK,CAAL;AAAQ1C,YAAAA,CAAC,GAAG0C,EAAJ;AAAQ;;AACxB,eAAK,CAAL;AAAQhJ,YAAAA,CAAC,CAACwI,KAAF;AAAW,mBAAO;AAAErZ,cAAAA,KAAK,EAAE6Z,EAAE,CAAC,CAAD,CAAX;AAAgBZ,cAAAA,IAAI,EAAE;AAAtB,aAAP;;AACnB,eAAK,CAAL;AAAQpI,YAAAA,CAAC,CAACwI,KAAF;AAAW1U,YAAAA,CAAC,GAAGkV,EAAE,CAAC,CAAD,CAAN;AAAWA,YAAAA,EAAE,GAAG,CAAC,CAAD,CAAL;AAAU;;AACxC,eAAK,CAAL;AAAQA,YAAAA,EAAE,GAAGhJ,CAAC,CAAC2I,GAAF,CAAMpF,GAAN,EAAL;;AAAkBvD,YAAAA,CAAC,CAAC0I,IAAF,CAAOnF,GAAP;;AAAc;;AACxC;AACI,gBAAI,EAAE+C,CAAC,GAAGtG,CAAC,CAAC0I,IAAN,EAAYpC,CAAC,GAAGA,CAAC,CAAC9Z,MAAF,GAAW,CAAX,IAAgB8Z,CAAC,CAACA,CAAC,CAAC9Z,MAAF,GAAW,CAAZ,CAAnC,MAAuDwc,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,IAAeA,EAAE,CAAC,CAAD,CAAF,KAAU,CAAhF,CAAJ,EAAwF;AAAEhJ,cAAAA,CAAC,GAAG,CAAJ;AAAO;AAAW;;AAC5G,gBAAIgJ,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,KAAgB,CAAC1C,CAAD,IAAO0C,EAAE,CAAC,CAAD,CAAF,GAAQ1C,CAAC,CAAC,CAAD,CAAT,IAAgB0C,EAAE,CAAC,CAAD,CAAF,GAAQ1C,CAAC,CAAC,CAAD,CAAhD,CAAJ,EAA2D;AAAEtG,cAAAA,CAAC,CAACwI,KAAF,GAAUQ,EAAE,CAAC,CAAD,CAAZ;AAAiB;AAAQ;;AACtF,gBAAIA,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,IAAehJ,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAA9B,EAAmC;AAAEtG,cAAAA,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAAX;AAAgBA,cAAAA,CAAC,GAAG0C,EAAJ;AAAQ;AAAQ;;AACrE,gBAAI1C,CAAC,IAAItG,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAApB,EAAyB;AAAEtG,cAAAA,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAAX;;AAAgBtG,cAAAA,CAAC,CAAC2I,GAAF,CAAM/a,IAAN,CAAWob,EAAX;;AAAgB;AAAQ;;AACnE,gBAAI1C,CAAC,CAAC,CAAD,CAAL,EAAUtG,CAAC,CAAC2I,GAAF,CAAMpF,GAAN;;AACVvD,YAAAA,CAAC,CAAC0I,IAAF,CAAOnF,GAAP;;AAAc;AAXtB;;AAaAyF,QAAAA,EAAE,GAAGT,IAAI,CAACjU,IAAL,CAAUiT,OAAV,EAAmBvH,CAAnB,CAAL;AACH,OAjBS,CAiBR,OAAOzR,CAAP,EAAU;AAAEya,QAAAA,EAAE,GAAG,CAAC,CAAD,EAAIza,CAAJ,CAAL;AAAauF,QAAAA,CAAC,GAAG,CAAJ;AAAQ,OAjBzB,SAiBkC;AAAE4L,QAAAA,CAAC,GAAG4G,CAAC,GAAG,CAAR;AAAY;AAjB1D;;AAkBA,QAAI0C,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAZ,EAAe,MAAMA,EAAE,CAAC,CAAD,CAAR;AAAa,WAAO;AAAE7Z,MAAAA,KAAK,EAAE6Z,EAAE,CAAC,CAAD,CAAF,GAAQA,EAAE,CAAC,CAAD,CAAV,GAAgB,KAAK,CAA9B;AAAiCZ,MAAAA,IAAI,EAAE;AAAvC,KAAP;AAC/B;AACJ;AAEM,SAASa,eAAT,CAAyBrE,CAAzB,EAA4BpW,CAA5B,EAA+B0a,CAA/B,EAAkCC,EAAlC,EAAsC;AACzC,MAAIA,EAAE,KAAKrY,SAAX,EAAsBqY,EAAE,GAAGD,CAAL;AACtBtE,EAAAA,CAAC,CAACuE,EAAD,CAAD,GAAQ3a,CAAC,CAAC0a,CAAD,CAAT;AACH;AAEM,SAASE,YAAT,CAAsB5a,CAAtB,EAAyBqB,OAAzB,EAAkC;AACrC,OAAK,IAAIoW,CAAT,IAAczX,CAAd;AAAiB,QAAIyX,CAAC,KAAK,SAAN,IAAmB,CAACpW,OAAO,CAAC+T,cAAR,CAAuBqC,CAAvB,CAAxB,EAAmDpW,OAAO,CAACoW,CAAD,CAAP,GAAazX,CAAC,CAACyX,CAAD,CAAd;AAApE;AACH;AAEM,SAASoD,QAAT,CAAkBzE,CAAlB,EAAqB;AACxB,MAAI9V,CAAC,GAAG,OAAOc,MAAP,KAAkB,UAAlB,IAAgCA,MAAM,CAACkZ,QAA/C;AAAA,MAAyDta,CAAC,GAAGM,CAAC,IAAI8V,CAAC,CAAC9V,CAAD,CAAnE;AAAA,MAAwExC,CAAC,GAAG,CAA5E;AACA,MAAIkC,CAAJ,EAAO,OAAOA,CAAC,CAAC8F,IAAF,CAAOsQ,CAAP,CAAP;AACP,MAAIA,CAAC,IAAI,OAAOA,CAAC,CAACpY,MAAT,KAAoB,QAA7B,EAAuC,OAAO;AAC1Cyb,IAAAA,IAAI,EAAE,gBAAY;AACd,UAAIrD,CAAC,IAAItY,CAAC,IAAIsY,CAAC,CAACpY,MAAhB,EAAwBoY,CAAC,GAAG,KAAK,CAAT;AACxB,aAAO;AAAEzV,QAAAA,KAAK,EAAEyV,CAAC,IAAIA,CAAC,CAACtY,CAAC,EAAF,CAAf;AAAsB8b,QAAAA,IAAI,EAAE,CAACxD;AAA7B,OAAP;AACH;AAJyC,GAAP;AAMvC,QAAM,IAAIvT,SAAJ,CAAcvC,CAAC,GAAG,yBAAH,GAA+B,iCAA9C,CAAN;AACH;AAEM,SAASwa,MAAT,CAAgB1E,CAAhB,EAAmBxP,CAAnB,EAAsB;AACzB,MAAI5G,CAAC,GAAG,OAAOoB,MAAP,KAAkB,UAAlB,IAAgCgV,CAAC,CAAChV,MAAM,CAACkZ,QAAR,CAAzC;AACA,MAAI,CAACta,CAAL,EAAQ,OAAOoW,CAAP;AACR,MAAItY,CAAC,GAAGkC,CAAC,CAAC8F,IAAF,CAAOsQ,CAAP,CAAR;AAAA,MAAmBgC,CAAnB;AAAA,MAAsBvC,EAAE,GAAG,EAA3B;AAAA,MAA+B9V,CAA/B;;AACA,MAAI;AACA,WAAO,CAAC6G,CAAC,KAAK,KAAK,CAAX,IAAgBA,CAAC,KAAK,CAAvB,KAA6B,CAAC,CAACwR,CAAC,GAAGta,CAAC,CAAC2b,IAAF,EAAL,EAAeG,IAApD;AAA0D/D,MAAAA,EAAE,CAACzW,IAAH,CAAQgZ,CAAC,CAACzX,KAAV;AAA1D;AACH,GAFD,CAGA,OAAOiB,KAAP,EAAc;AAAE7B,IAAAA,CAAC,GAAG;AAAE6B,MAAAA,KAAK,EAAEA;AAAT,KAAJ;AAAuB,GAHvC,SAIQ;AACJ,QAAI;AACA,UAAIwW,CAAC,IAAI,CAACA,CAAC,CAACwB,IAAR,KAAiB5Z,CAAC,GAAGlC,CAAC,CAAC,QAAD,CAAtB,CAAJ,EAAuCkC,CAAC,CAAC8F,IAAF,CAAOhI,CAAP;AAC1C,KAFD,SAGQ;AAAE,UAAIiC,CAAJ,EAAO,MAAMA,CAAC,CAAC6B,KAAR;AAAgB;AACpC;;AACD,SAAOiU,EAAP;AACH;AAEM,SAASkF,QAAT,GAAoB;AACvB,OAAK,IAAIlF,EAAE,GAAG,EAAT,EAAa/X,CAAC,GAAG,CAAtB,EAAyBA,CAAC,GAAGkI,SAAS,CAAChI,MAAvC,EAA+CF,CAAC,EAAhD;AACI+X,IAAAA,EAAE,GAAGA,EAAE,CAACnQ,MAAH,CAAUoV,MAAM,CAAC9U,SAAS,CAAClI,CAAD,CAAV,CAAhB,CAAL;AADJ;;AAEA,SAAO+X,EAAP;AACH;AAEM,SAASmF,cAAT,GAA0B;AAC7B,OAAK,IAAI1a,CAAC,GAAG,CAAR,EAAWxC,CAAC,GAAG,CAAf,EAAkBmd,EAAE,GAAGjV,SAAS,CAAChI,MAAtC,EAA8CF,CAAC,GAAGmd,EAAlD,EAAsDnd,CAAC,EAAvD;AAA2DwC,IAAAA,CAAC,IAAI0F,SAAS,CAAClI,CAAD,CAAT,CAAaE,MAAlB;AAA3D;;AACA,OAAK,IAAIoa,CAAC,GAAGxa,KAAK,CAAC0C,CAAD,CAAb,EAAkBoa,CAAC,GAAG,CAAtB,EAAyB5c,CAAC,GAAG,CAAlC,EAAqCA,CAAC,GAAGmd,EAAzC,EAA6Cnd,CAAC,EAA9C;AACI,SAAK,IAAIsH,CAAC,GAAGY,SAAS,CAAClI,CAAD,CAAjB,EAAsB4K,CAAC,GAAG,CAA1B,EAA6BwS,EAAE,GAAG9V,CAAC,CAACpH,MAAzC,EAAiD0K,CAAC,GAAGwS,EAArD,EAAyDxS,CAAC,IAAIgS,CAAC,EAA/D;AACItC,MAAAA,CAAC,CAACsC,CAAD,CAAD,GAAOtV,CAAC,CAACsD,CAAD,CAAR;AADJ;AADJ;;AAGA,SAAO0P,CAAP;AACH;AAEM,SAAS+C,OAAT,CAAiBZ,CAAjB,EAAoB;AACvB,SAAO,gBAAgBY,OAAhB,IAA2B,KAAKZ,CAAL,GAASA,CAAT,EAAY,IAAvC,IAA+C,IAAIY,OAAJ,CAAYZ,CAAZ,CAAtD;AACH;AAEM,SAASa,gBAAT,CAA0BrC,OAA1B,EAAmCC,UAAnC,EAA+CE,SAA/C,EAA0D;AAC7D,MAAI,CAAC9X,MAAM,CAACia,aAAZ,EAA2B,MAAM,IAAIxY,SAAJ,CAAc,sCAAd,CAAN;AAC3B,MAAIuX,CAAC,GAAGlB,SAAS,CAAClS,KAAV,CAAgB+R,OAAhB,EAAyBC,UAAU,IAAI,EAAvC,CAAR;AAAA,MAAoDlb,CAApD;AAAA,MAAuDwd,CAAC,GAAG,EAA3D;AACA,SAAOxd,CAAC,GAAG,EAAJ,EAAQuc,IAAI,CAAC,MAAD,CAAZ,EAAsBA,IAAI,CAAC,OAAD,CAA1B,EAAqCA,IAAI,CAAC,QAAD,CAAzC,EAAqDvc,CAAC,CAACsD,MAAM,CAACia,aAAR,CAAD,GAA0B,YAAY;AAAE,WAAO,IAAP;AAAc,GAA3G,EAA6Gvd,CAApH;;AACA,WAASuc,IAAT,CAAczT,CAAd,EAAiB;AAAE,QAAIwT,CAAC,CAACxT,CAAD,CAAL,EAAU9I,CAAC,CAAC8I,CAAD,CAAD,GAAO,UAAU2T,CAAV,EAAa;AAAE,aAAO,IAAIlB,OAAJ,CAAY,UAAUjU,CAAV,EAAa3B,CAAb,EAAgB;AAAE6X,QAAAA,CAAC,CAAClc,IAAF,CAAO,CAACwH,CAAD,EAAI2T,CAAJ,EAAOnV,CAAP,EAAU3B,CAAV,CAAP,IAAuB,CAAvB,IAA4B8X,MAAM,CAAC3U,CAAD,EAAI2T,CAAJ,CAAlC;AAA2C,OAAzE,CAAP;AAAoF,KAA1G;AAA6G;;AAC1I,WAASgB,MAAT,CAAgB3U,CAAhB,EAAmB2T,CAAnB,EAAsB;AAAE,QAAI;AAAEf,MAAAA,IAAI,CAACY,CAAC,CAACxT,CAAD,CAAD,CAAK2T,CAAL,CAAD,CAAJ;AAAgB,KAAtB,CAAuB,OAAOxa,CAAP,EAAU;AAAEyb,MAAAA,MAAM,CAACF,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUvb,CAAV,CAAN;AAAqB;AAAE;;AAClF,WAASyZ,IAAT,CAAcpB,CAAd,EAAiB;AAAEA,IAAAA,CAAC,CAACzX,KAAF,YAAmBwa,OAAnB,GAA6B9B,OAAO,CAACD,OAAR,CAAgBhB,CAAC,CAACzX,KAAF,CAAQ4Z,CAAxB,EAA2BV,IAA3B,CAAgC4B,OAAhC,EAAyCnC,MAAzC,CAA7B,GAAgFkC,MAAM,CAACF,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUlD,CAAV,CAAtF;AAAqG;;AACxH,WAASqD,OAAT,CAAiB9a,KAAjB,EAAwB;AAAE4a,IAAAA,MAAM,CAAC,MAAD,EAAS5a,KAAT,CAAN;AAAwB;;AAClD,WAAS2Y,MAAT,CAAgB3Y,KAAhB,EAAuB;AAAE4a,IAAAA,MAAM,CAAC,OAAD,EAAU5a,KAAV,CAAN;AAAyB;;AAClD,WAAS6a,MAAT,CAAgBtK,CAAhB,EAAmBqJ,CAAnB,EAAsB;AAAE,QAAIrJ,CAAC,CAACqJ,CAAD,CAAD,EAAMe,CAAC,CAACI,KAAF,EAAN,EAAiBJ,CAAC,CAACtd,MAAvB,EAA+Bud,MAAM,CAACD,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUA,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAV,CAAN;AAA2B;AACrF;AAEM,SAASK,gBAAT,CAA0BvF,CAA1B,EAA6B;AAChC,MAAItY,CAAJ,EAAO2Z,CAAP;AACA,SAAO3Z,CAAC,GAAG,EAAJ,EAAQuc,IAAI,CAAC,MAAD,CAAZ,EAAsBA,IAAI,CAAC,OAAD,EAAU,UAAUta,CAAV,EAAa;AAAE,UAAMA,CAAN;AAAU,GAAnC,CAA1B,EAAgEsa,IAAI,CAAC,QAAD,CAApE,EAAgFvc,CAAC,CAACsD,MAAM,CAACkZ,QAAR,CAAD,GAAqB,YAAY;AAAE,WAAO,IAAP;AAAc,GAAjI,EAAmIxc,CAA1I;;AACA,WAASuc,IAAT,CAAczT,CAAd,EAAiBsK,CAAjB,EAAoB;AAAEpT,IAAAA,CAAC,CAAC8I,CAAD,CAAD,GAAOwP,CAAC,CAACxP,CAAD,CAAD,GAAO,UAAU2T,CAAV,EAAa;AAAE,aAAO,CAAC9C,CAAC,GAAG,CAACA,CAAN,IAAW;AAAE9W,QAAAA,KAAK,EAAEwa,OAAO,CAAC/E,CAAC,CAACxP,CAAD,CAAD,CAAK2T,CAAL,CAAD,CAAhB;AAA2BX,QAAAA,IAAI,EAAEhT,CAAC,KAAK;AAAvC,OAAX,GAA+DsK,CAAC,GAAGA,CAAC,CAACqJ,CAAD,CAAJ,GAAUA,CAAjF;AAAqF,KAA3G,GAA8GrJ,CAArH;AAAyH;AAClJ;AAEM,SAAS0K,aAAT,CAAuBxF,CAAvB,EAA0B;AAC7B,MAAI,CAAChV,MAAM,CAACia,aAAZ,EAA2B,MAAM,IAAIxY,SAAJ,CAAc,sCAAd,CAAN;AAC3B,MAAI7C,CAAC,GAAGoW,CAAC,CAAChV,MAAM,CAACia,aAAR,CAAT;AAAA,MAAiCvd,CAAjC;AACA,SAAOkC,CAAC,GAAGA,CAAC,CAAC8F,IAAF,CAAOsQ,CAAP,CAAH,IAAgBA,CAAC,GAAG,OAAOyE,QAAP,KAAoB,UAApB,GAAiCA,QAAQ,CAACzE,CAAD,CAAzC,GAA+CA,CAAC,CAAChV,MAAM,CAACkZ,QAAR,CAAD,EAAnD,EAAyExc,CAAC,GAAG,EAA7E,EAAiFuc,IAAI,CAAC,MAAD,CAArF,EAA+FA,IAAI,CAAC,OAAD,CAAnG,EAA8GA,IAAI,CAAC,QAAD,CAAlH,EAA8Hvc,CAAC,CAACsD,MAAM,CAACia,aAAR,CAAD,GAA0B,YAAY;AAAE,WAAO,IAAP;AAAc,GAApL,EAAsLvd,CAAtM,CAAR;;AACA,WAASuc,IAAT,CAAczT,CAAd,EAAiB;AAAE9I,IAAAA,CAAC,CAAC8I,CAAD,CAAD,GAAOwP,CAAC,CAACxP,CAAD,CAAD,IAAQ,UAAU2T,CAAV,EAAa;AAAE,aAAO,IAAIlB,OAAJ,CAAY,UAAUD,OAAV,EAAmBE,MAAnB,EAA2B;AAAEiB,QAAAA,CAAC,GAAGnE,CAAC,CAACxP,CAAD,CAAD,CAAK2T,CAAL,CAAJ,EAAaiB,MAAM,CAACpC,OAAD,EAAUE,MAAV,EAAkBiB,CAAC,CAACX,IAApB,EAA0BW,CAAC,CAAC5Z,KAA5B,CAAnB;AAAwD,OAAjG,CAAP;AAA4G,KAA1I;AAA6I;;AAChK,WAAS6a,MAAT,CAAgBpC,OAAhB,EAAyBE,MAAzB,EAAiCjZ,CAAjC,EAAoCka,CAApC,EAAuC;AAAElB,IAAAA,OAAO,CAACD,OAAR,CAAgBmB,CAAhB,EAAmBV,IAAnB,CAAwB,UAASU,CAAT,EAAY;AAAEnB,MAAAA,OAAO,CAAC;AAAEzY,QAAAA,KAAK,EAAE4Z,CAAT;AAAYX,QAAAA,IAAI,EAAEvZ;AAAlB,OAAD,CAAP;AAAiC,KAAvE,EAAyEiZ,MAAzE;AAAmF;AAC/H;AAEM,SAASuC,oBAAT,CAA8BC,MAA9B,EAAsCC,GAAtC,EAA2C;AAC9C,MAAIha,MAAM,CAACG,cAAX,EAA2B;AAAEH,IAAAA,MAAM,CAACG,cAAP,CAAsB4Z,MAAtB,EAA8B,KAA9B,EAAqC;AAAEnb,MAAAA,KAAK,EAAEob;AAAT,KAArC;AAAuD,GAApF,MAA0F;AAAED,IAAAA,MAAM,CAACC,GAAP,GAAaA,GAAb;AAAmB;;AAC/G,SAAOD,MAAP;AACH;AAEM,SAASE,YAAT,CAAsBC,GAAtB,EAA2B;AAC9B,MAAIA,GAAG,IAAIA,GAAG,CAACC,UAAf,EAA2B,OAAOD,GAAP;AAC3B,MAAItC,MAAM,GAAG,EAAb;AACA,MAAIsC,GAAG,IAAI,IAAX,EAAiB,KAAK,IAAIvB,CAAT,IAAcuB,GAAd;AAAmB,QAAIla,MAAM,CAACqT,cAAP,CAAsBtP,IAAtB,CAA2BmW,GAA3B,EAAgCvB,CAAhC,CAAJ,EAAwCf,MAAM,CAACe,CAAD,CAAN,GAAYuB,GAAG,CAACvB,CAAD,CAAf;AAA3D;AACjBf,EAAAA,MAAM,WAAN,GAAiBsC,GAAjB;AACA,SAAOtC,MAAP;AACH;AAEM,SAASwC,eAAT,CAAyBF,GAAzB,EAA8B;AACjC,SAAQA,GAAG,IAAIA,GAAG,CAACC,UAAZ,GAA0BD,GAA1B,GAAgC;AAAE,eAASA;AAAX,GAAvC;AACH;AAEM,SAASG,sBAAT,CAAgCC,QAAhC,EAA0CC,UAA1C,EAAsD;AACzD,MAAI,CAACA,UAAU,CAACC,GAAX,CAAeF,QAAf,CAAL,EAA+B;AAC3B,UAAM,IAAIxZ,SAAJ,CAAc,gDAAd,CAAN;AACH;;AACD,SAAOyZ,UAAU,CAACla,GAAX,CAAeia,QAAf,CAAP;AACH;AAEM,SAASG,sBAAT,CAAgCH,QAAhC,EAA0CC,UAA1C,EAAsD3b,KAAtD,EAA6D;AAChE,MAAI,CAAC2b,UAAU,CAACC,GAAX,CAAeF,QAAf,CAAL,EAA+B;AAC3B,UAAM,IAAIxZ,SAAJ,CAAc,gDAAd,CAAN;AACH;;AACDyZ,EAAAA,UAAU,CAACzW,GAAX,CAAewW,QAAf,EAAyB1b,KAAzB;AACA,SAAOA,KAAP;AACH;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;ACzN6B;AAQ9B;AACa,iCAAyB,GAA0B,WAAwC,CAAC;AAUzG;AACA;IAA+B8b,uCAAyB;IAetD,mBAAY,GAAkB,EAAE,IAAa;QAA7C,iBAgBC;;;QAbC,IAAI,EAAE,KAAI,YAAY,SAAS,CAAC;YAAE,OAAO,IAAI,SAAS,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;QAElE,IAAI,WAAI,CAAC,MAAM,CAAC,GAAG,CAAC,EAAE;YACpB,QAAA,kBAAM,GAAG,CAAC,GAAG,EAAE,GAAG,CAAC,IAAI,EAAE,IAAI,CAAC,SAAC;SAChC;aAAM;YACL,QAAA,kBAAM,GAAG,EAAE,IAAI,EAAE,IAAI,CAAC,SAAC;SACxB;QACD,MAAM,CAAC,cAAc,CAAC,KAAI,EAAE,WAAW,EAAE;YACvC,KAAK,EAAE,WAAW;YAClB,QAAQ,EAAE,KAAK;YACf,YAAY,EAAE,KAAK;YACnB,UAAU,EAAE,KAAK;SAClB,CAAC,CAAC;;KACJ;IAED,0BAAM,GAAN;QACE,OAAO;YACL,UAAU,EAAE,IAAI,CAAC,QAAQ,EAAE;SAC5B,CAAC;KACH;;IAGM,iBAAO,GAAd,UAAe,KAAa;QAC1B,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,OAAO,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;KACjD;;IAGM,oBAAU,GAAjB,UAAkB,KAAa;QAC7B,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,UAAU,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;KACpD;;;;;;;IAQM,kBAAQ,GAAf,UAAgB,OAAe,EAAE,QAAgB;QAC/C,OAAO,IAAI,SAAS,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;KACzC;;;;;;;IAQM,oBAAU,GAAjB,UAAkB,GAAW,EAAE,QAAgB;QAC7C,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,UAAU,CAAC,GAAG,EAAE,IAAI,EAAE,QAAQ,CAAC,CAAC,CAAC;KAC5D;;IAGD,kCAAc,GAAd;QACE,OAAO,EAAE,UAAU,EAAE,EAAE,CAAC,EAAE,IAAI,CAAC,IAAI,KAAK,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,GAAG,KAAK,CAAC,EAAE,EAAE,CAAC;KAClE;;IAGM,0BAAgB,GAAvB,UAAwB,GAAsB;QAC5C,OAAO,IAAI,SAAS,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC,EAAE,GAAG,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;KAC1D;;IAGD,oBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,2BAAO,GAAP;QACE,OAAO,mBAAiB,IAAI,CAAC,UAAU,EAAE,CAAC,QAAQ,EAAE,UAAK,IAAI,CAAC,WAAW,EAAE,CAAC,QAAQ,EAAE,MAAG,CAAC;KAC3F;IAnFe,mBAAS,GAAG,WAAI,CAAC,kBAAkB,CAAC;IAoFtD,gBAAC;CAAA,CAvF8B,iCAAyB,GAuFvD;AAvFY,8BAAS;;;;;;;;;;;ACpBY;AAEJ;AACgB;AACJ;AACR;AACD;AACH;AACK;AACA;AACG;AAC0B;AAC1B;AACA;AACE;AAqBxC,SAAgB,UAAU,CAAC,KAAc;IACvC,QACEvF,kBAAY,CAAC,KAAK,CAAC,IAAI,OAAO,CAAC,GAAG,CAAC,KAAK,EAAE,WAAW,CAAC,IAAI,OAAO,KAAK,CAAC,SAAS,KAAK,QAAQ,EAC7F;AACJ,CAAC;AAJD,gCAIC;AAED;AACA,IAAM,cAAc,GAAG,UAAU,CAAC;AAClC,IAAM,cAAc,GAAG,CAAC,UAAU,CAAC;AACnC;AACA,IAAM,cAAc,GAAG,kBAAkB,CAAC;AAC1C,IAAM,cAAc,GAAG,CAAC,kBAAkB,CAAC;AAE3C;AACA;AACA,IAAM,YAAY,GAAG;IACnB,IAAI,EAAEwF,iBAAQ;IACd,OAAO,EAAErF,aAAM;IACf,KAAK,EAAEA,aAAM;IACb,OAAO,EAAEsF,iBAAU;IACnB,UAAU,EAAEC,YAAK;IACjB,cAAc,EAAEC,qBAAU;IAC1B,aAAa,EAAE,eAAM;IACrB,WAAW,EAAE,WAAI;IACjB,OAAO,EAAEC,cAAM;IACf,OAAO,EAAEC,cAAM;IACf,MAAM,EAAEC,iBAAU;IAClB,kBAAkB,EAAEA,iBAAU;IAC9B,UAAU,EAAEC,mBAAS;CACb,CAAC;AAEX;AACA,SAAS,gBAAgB,CAAC,KAAU,EAAE,OAA2B;IAA3B,wBAAA,EAAA,YAA2B;IAC/D,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;QAC7B,IAAI,OAAO,CAAC,OAAO,IAAI,OAAO,CAAC,MAAM,EAAE;YACrC,OAAO,KAAK,CAAC;SACd;;;QAID,IAAI,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK,EAAE;YAC/B,IAAI,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc;gBAAE,OAAO,IAAIL,YAAK,CAAC,KAAK,CAAC,CAAC;YAChF,IAAI,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc;gBAAE,OAAO,WAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC;SACvF;;QAGD,OAAO,IAAI,eAAM,CAAC,KAAK,CAAC,CAAC;KAC1B;;IAGD,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ;QAAE,OAAO,KAAK,CAAC;;IAG7D,IAAI,KAAK,CAAC,UAAU;QAAE,OAAO,IAAI,CAAC;IAElC,IAAM,IAAI,GAAG,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,MAAM,CACpC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,IAAI,GAAA,CACV,CAAC;IACnC,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;QACpC,IAAM,CAAC,GAAG,YAAY,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,CAAC;QAChC,IAAI,CAAC;YAAE,OAAO,CAAC,CAAC,gBAAgB,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;KAClD;IAED,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,EAAE;QACvB,IAAM,CAAC,GAAG,KAAK,CAAC,KAAK,CAAC;QACtB,IAAM,IAAI,GAAG,IAAI,IAAI,EAAE,CAAC;QAExB,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,IAAI,OAAO,CAAC,KAAK,QAAQ;gBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;iBACtC,IAAI,OAAO,CAAC,KAAK,QAAQ;gBAAE,IAAI,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;SAC7D;aAAM;YACL,IAAI,OAAO,CAAC,KAAK,QAAQ;gBAAE,IAAI,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;iBAClD,IAAI,WAAI,CAAC,MAAM,CAAC,CAAC,CAAC;gBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,QAAQ,EAAE,CAAC,CAAC;iBAC/C,IAAI,OAAO,CAAC,KAAK,QAAQ,IAAI,OAAO,CAAC,OAAO;gBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;SACpE;QACD,OAAO,IAAI,CAAC;KACb;IAED,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,EAAE;QACvB,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,KAAK,CAAC,CAAC;QACtC,IAAI,KAAK,CAAC,MAAM,EAAE;YAChB,IAAI,CAAC,MAAM,GAAG,gBAAgB,CAAC,KAAK,CAAC,MAAM,CAAC,CAAC;SAC9C;QAED,OAAOM,SAAI,CAAC,gBAAgB,CAAC,KAAK,CAAC,CAAC;KACrC;IAED,IAAIC,kBAAW,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,UAAU,EAAE;QAC1C,IAAM,CAAC,GAAG,KAAK,CAAC,IAAI,GAAG,KAAK,GAAG,KAAK,CAAC,UAAU,CAAC;;;QAIhD,IAAI,CAAC,YAAYA,YAAK;YAAE,OAAO,CAAC,CAAC;QAEjC,IAAM,UAAU,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,GAAA,CAAC,CAAC;QACjE,IAAI,OAAK,GAAG,IAAI,CAAC;QACjB,UAAU,CAAC,OAAO,CAAC,UAAA,CAAC;YAClB,IAAI,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC;gBAAE,OAAK,GAAG,KAAK,CAAC;SAC7D,CAAC,CAAC;;QAGH,IAAI,OAAK;YAAE,OAAOA,YAAK,CAAC,gBAAgB,CAAC,CAAC,CAAC,CAAC;KAC7C;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAMD;AACA,SAAS,cAAc,CAAC,KAAY,EAAE,OAA8B;IAClE,OAAO,KAAK,CAAC,GAAG,CAAC,UAAC,CAAU,EAAE,KAAa;QACzC,OAAO,CAAC,WAAW,CAAC,IAAI,CAAC,EAAE,YAAY,EAAE,WAAS,KAAO,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC,CAAC;QACxE,IAAI;YACF,OAAO,cAAc,CAAC,CAAC,EAAE,OAAO,CAAC,CAAC;SACnC;gBAAS;YACR,OAAO,CAAC,WAAW,CAAC,GAAG,EAAE,CAAC;SAC3B;KACF,CAAC,CAAC;AACL,CAAC;AAED,SAAS,YAAY,CAAC,IAAU;IAC9B,IAAM,MAAM,GAAG,IAAI,CAAC,WAAW,EAAE,CAAC;;IAElC,OAAO,IAAI,CAAC,kBAAkB,EAAE,KAAK,CAAC,GAAG,MAAM,GAAG,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;AAC9E,CAAC;AAED;AACA,SAAS,cAAc,CAAC,KAAU,EAAE,OAA8B;IAChE,IAAI,CAAC,OAAO,KAAK,KAAK,QAAQ,IAAI,OAAO,KAAK,KAAK,UAAU,KAAK,KAAK,KAAK,IAAI,EAAE;QAChF,IAAM,KAAK,GAAG,OAAO,CAAC,WAAW,CAAC,SAAS,CAAC,UAAA,KAAK,IAAI,OAAA,KAAK,CAAC,GAAG,KAAK,KAAK,GAAA,CAAC,CAAC;QAC1E,IAAI,KAAK,KAAK,CAAC,CAAC,EAAE;YAChB,IAAM,KAAK,GAAG,OAAO,CAAC,WAAW,CAAC,GAAG,CAAC,UAAA,KAAK,IAAI,OAAA,KAAK,CAAC,YAAY,GAAA,CAAC,CAAC;YACnE,IAAM,WAAW,GAAG,KAAK;iBACtB,KAAK,CAAC,CAAC,EAAE,KAAK,CAAC;iBACf,GAAG,CAAC,UAAA,IAAI,IAAI,OAAG,IAAI,SAAM,GAAA,CAAC;iBAC1B,IAAI,CAAC,EAAE,CAAC,CAAC;YACZ,IAAM,WAAW,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC;YACjC,IAAM,YAAY,GAChB,MAAM;gBACN,KAAK;qBACF,KAAK,CAAC,KAAK,GAAG,CAAC,EAAE,KAAK,CAAC,MAAM,GAAG,CAAC,CAAC;qBAClC,GAAG,CAAC,UAAA,IAAI,IAAI,OAAG,IAAI,SAAM,GAAA,CAAC;qBAC1B,IAAI,CAAC,EAAE,CAAC,CAAC;YACd,IAAM,OAAO,GAAG,KAAK,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;YACxC,IAAM,YAAY,GAAG,GAAG,CAAC,MAAM,CAAC,WAAW,CAAC,MAAM,GAAG,WAAW,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;YAC7E,IAAM,MAAM,GAAG,GAAG,CAAC,MAAM,CACvB,YAAY,CAAC,MAAM,GAAG,CAAC,WAAW,CAAC,MAAM,GAAG,OAAO,CAAC,MAAM,IAAI,CAAC,GAAG,CAAC,CACpE,CAAC;YAEF,MAAM,IAAI,SAAS,CACjB,2CAA2C;iBACzC,SAAO,WAAW,GAAG,WAAW,GAAG,YAAY,GAAG,OAAO,OAAI,CAAA;iBAC7D,SAAO,YAAY,UAAK,MAAM,MAAG,CAAA,CACpC,CAAC;SACH;QACD,OAAO,CAAC,WAAW,CAAC,OAAO,CAAC,WAAW,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC,GAAG,GAAG,KAAK,CAAC;KACjE;IAED,IAAI,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC;QAAE,OAAO,cAAc,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;IAEhE,IAAI,KAAK,KAAK,SAAS;QAAE,OAAO,IAAI,CAAC;IAErC,IAAI,KAAK,YAAY,IAAI,IAAIjG,YAAM,CAAC,KAAK,CAAC,EAAE;QAC1C,IAAM,OAAO,GAAG,KAAK,CAAC,OAAO,EAAE;;QAE7B,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC,IAAI,OAAO,GAAG,eAAe,CAAC;QAEtD,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO,OAAO,CAAC,OAAO,IAAI,OAAO;kBAC7B,EAAE,KAAK,EAAE,KAAK,CAAC,OAAO,EAAE,EAAE;kBAC1B,EAAE,KAAK,EAAE,YAAY,CAAC,KAAK,CAAC,EAAE,CAAC;SACpC;QACD,OAAO,OAAO,CAAC,OAAO,IAAI,OAAO;cAC7B,EAAE,KAAK,EAAE,YAAY,CAAC,KAAK,CAAC,EAAE;cAC9B,EAAE,KAAK,EAAE,EAAE,WAAW,EAAE,KAAK,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE,EAAE,EAAE,CAAC;KAC5D;IAED,IAAI,OAAO,KAAK,KAAK,QAAQ,KAAK,CAAC,OAAO,CAAC,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC,EAAE;;QAEvE,IAAI,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK,EAAE;YAC/B,IAAM,UAAU,GAAG,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc,EACnE,UAAU,GAAG,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc,CAAC;;YAGlE,IAAI,UAAU;gBAAE,OAAO,EAAE,UAAU,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;YACxD,IAAI,UAAU;gBAAE,OAAO,EAAE,WAAW,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;SAC1D;QACD,OAAO,EAAE,aAAa,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;KAC5C;IAED,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;QAC9C,IAAI,KAAK,GAAG,KAAK,CAAC,KAAK,CAAC;QACxB,IAAI,KAAK,KAAK,SAAS,EAAE;YACvB,IAAM,KAAK,GAAG,KAAK,CAAC,QAAQ,EAAE,CAAC,KAAK,CAAC,WAAW,CAAC,CAAC;YAClD,IAAI,KAAK,EAAE;gBACT,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;aAClB;SACF;QAED,IAAM,EAAE,GAAG,IAAI8F,iBAAU,CAAC,KAAK,CAAC,MAAM,EAAE,KAAK,CAAC,CAAC;QAC/C,OAAO,EAAE,CAAC,cAAc,CAAC,OAAO,CAAC,CAAC;KACnC;IAED,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ;QAAE,OAAO,iBAAiB,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;IACzF,OAAO,KAAK,CAAC;AACf,CAAC;AAED,IAAM,kBAAkB,GAAG;IACzB,MAAM,EAAE,UAAC,CAAS,IAAK,OAAA,IAAI3F,aAAM,CAAC,CAAC,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,QAAQ,CAAC,GAAA;IACxD,IAAI,EAAE,UAAC,CAAO,IAAK,OAAA,IAAI6F,SAAI,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC,KAAK,CAAC,GAAA;IAC5C,KAAK,EAAE,UAAC,CAAQ,IAAK,OAAA,IAAIC,YAAK,CAAC,CAAC,CAAC,UAAU,IAAI,CAAC,CAAC,SAAS,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,MAAM,CAAC,GAAA;IAClF,UAAU,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIN,qBAAU,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;IACtD,MAAM,EAAE,UAAC,CAAS,IAAK,OAAA,IAAI,eAAM,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;IAC1C,KAAK,EAAE,UAAC,CAAQ,IAAK,OAAA,IAAID,YAAK,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;IACvC,IAAI,EAAE,UACJ,CAIC;QAED,OAAA,WAAI,CAAC,QAAQ;;QAEX,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,GAAG,GAAG,CAAC,CAAC,IAAI,EAC9B,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,CAAC,KAAK,EAChC,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,QAAQ,GAAG,CAAC,CAAC,SAAS,CACzC;KAAA;IACH,MAAM,EAAE,cAAM,OAAA,IAAIG,cAAM,EAAE,GAAA;IAC1B,MAAM,EAAE,cAAM,OAAA,IAAID,cAAM,EAAE,GAAA;IAC1B,QAAQ,EAAE,UAAC,CAAW,IAAK,OAAA,IAAIJ,iBAAQ,CAAC,CAAC,CAAC,GAAA;IAC1C,QAAQ,EAAE,UAAC,CAAW,IAAK,OAAA,IAAIA,iBAAQ,CAAC,CAAC,CAAC,GAAA;IAC1C,UAAU,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIM,iBAAU,CAAC,CAAC,CAAC,OAAO,EAAE,CAAC,CAAC,OAAO,CAAC,GAAA;IACnE,MAAM,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIL,iBAAU,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;IAClD,SAAS,EAAE,UAAC,CAAY,IAAK,OAAAM,mBAAS,CAAC,QAAQ,CAAC,CAAC,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,GAAA;CACtD,CAAC;AAEX;AACA,SAAS,iBAAiB,CAAC,GAAQ,EAAE,OAA8B;IACjE,IAAI,GAAG,IAAI,IAAI,IAAI,OAAO,GAAG,KAAK,QAAQ;QAAE,MAAM,IAAI,KAAK,CAAC,wBAAwB,CAAC,CAAC;IAEtF,IAAM,QAAQ,GAA0B,GAAG,CAAC,SAAS,CAAC;IACtD,IAAI,OAAO,QAAQ,KAAK,WAAW,EAAE;;QAEnC,IAAM,IAAI,GAAa,EAAE,CAAC;QAC1B,KAAK,IAAM,IAAI,IAAI,GAAG,EAAE;YACtB,OAAO,CAAC,WAAW,CAAC,IAAI,CAAC,EAAE,YAAY,EAAE,IAAI,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC,CAAC;YAC5D,IAAI;gBACF,IAAI,CAAC,IAAI,CAAC,GAAG,cAAc,CAAC,GAAG,CAAC,IAAI,CAAC,EAAE,OAAO,CAAC,CAAC;aACjD;oBAAS;gBACR,OAAO,CAAC,WAAW,CAAC,GAAG,EAAE,CAAC;aAC3B;SACF;QACD,OAAO,IAAI,CAAC;KACb;SAAM,IAAI,UAAU,CAAC,GAAG,CAAC,EAAE;;;QAG1B,IAAI,MAAM,GAAQ,GAAG,CAAC;QACtB,IAAI,OAAO,MAAM,CAAC,cAAc,KAAK,UAAU,EAAE;;;;;YAK/C,IAAM,MAAM,GAAG,kBAAkB,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;YACjD,IAAI,CAAC,MAAM,EAAE;gBACX,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,GAAG,CAAC,SAAS,CAAC,CAAC;aAC5E;YACD,MAAM,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC;SACzB;;QAGD,IAAI,QAAQ,KAAK,MAAM,IAAI,MAAM,CAAC,KAAK,EAAE;YACvC,MAAM,GAAG,IAAIC,SAAI,CAAC,MAAM,CAAC,IAAI,EAAE,cAAc,CAAC,MAAM,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC,CAAC;SACvE;aAAM,IAAI,QAAQ,KAAK,OAAO,IAAI,MAAM,CAAC,GAAG,EAAE;YAC7C,MAAM,GAAG,IAAIC,YAAK,CAChB,cAAc,CAAC,MAAM,CAAC,UAAU,EAAE,OAAO,CAAC,EAC1C,cAAc,CAAC,MAAM,CAAC,GAAG,EAAE,OAAO,CAAC,EACnC,cAAc,CAAC,MAAM,CAAC,EAAE,EAAE,OAAO,CAAC,EAClC,cAAc,CAAC,MAAM,CAAC,MAAM,EAAE,OAAO,CAAC,CACvC,CAAC;SACH;QAED,OAAO,MAAM,CAAC,cAAc,CAAC,OAAO,CAAC,CAAC;KACvC;SAAM;QACL,MAAM,IAAI,KAAK,CAAC,uCAAuC,GAAG,OAAO,QAAQ,CAAC,CAAC;KAC5E;AACH,CAAC;AASD,WAAiB,KAAK;;;;;;;;;;;;;;;;;IA6BpB,SAAgB,KAAK,CAAC,IAAY,EAAE,OAAuB;QACzD,IAAM,YAAY,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,EAAE,OAAO,EAAE,IAAI,EAAE,MAAM,EAAE,KAAK,EAAE,EAAE,OAAO,CAAC,CAAC;;QAGlF,IAAI,OAAO,YAAY,CAAC,OAAO,KAAK,SAAS;YAAE,YAAY,CAAC,MAAM,GAAG,CAAC,YAAY,CAAC,OAAO,CAAC;QAC3F,IAAI,OAAO,YAAY,CAAC,MAAM,KAAK,SAAS;YAAE,YAAY,CAAC,OAAO,GAAG,CAAC,YAAY,CAAC,MAAM,CAAC;QAE1F,OAAO,IAAI,CAAC,KAAK,CAAC,IAAI,EAAE,UAAC,IAAI,EAAE,KAAK,IAAK,OAAA,gBAAgB,CAAC,KAAK,EAAE,YAAY,CAAC,GAAA,CAAC,CAAC;KACjF;IARe,WAAK,QAQpB,CAAA;;;;;;;;;;;;;;;;;;;;;;;;IA4BD,SAAgB,SAAS,CACvB,KAAwB;;IAExB,QAA8F,EAC9F,KAAuB,EACvB,OAAuB;QAEvB,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YAC9C,OAAO,GAAG,KAAK,CAAC;YAChB,KAAK,GAAG,CAAC,CAAC;SACX;QACD,IAAI,QAAQ,IAAI,IAAI,IAAI,OAAO,QAAQ,KAAK,QAAQ,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,QAAQ,CAAC,EAAE;YAChF,OAAO,GAAG,QAAQ,CAAC;YACnB,QAAQ,GAAG,SAAS,CAAC;YACrB,KAAK,GAAG,CAAC,CAAC;SACX;QACD,IAAM,gBAAgB,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,OAAO,EAAE,IAAI,EAAE,MAAM,EAAE,KAAK,EAAE,EAAE,OAAO,EAAE;YAChF,WAAW,EAAE,CAAC,EAAE,YAAY,EAAE,QAAQ,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;SACrD,CAAC,CAAC;QAEH,IAAM,GAAG,GAAG,cAAc,CAAC,KAAK,EAAE,gBAAgB,CAAC,CAAC;QACpD,OAAO,IAAI,CAAC,SAAS,CAAC,GAAG,EAAE,QAA4C,EAAE,KAAK,CAAC,CAAC;KACjF;IAtBe,eAAS,YAsBxB,CAAA;;;;;;;IAQD,SAAgB,SAAS,CAAC,KAAwB,EAAE,OAAuB;QACzE,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,OAAO,IAAI,CAAC,KAAK,CAAC,SAAS,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC,CAAC;KAC9C;IAHe,eAAS,YAGxB,CAAA;;;;;;;IAQD,SAAgB,WAAW,CAAC,KAAe,EAAE,OAAuB;QAClE,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,OAAO,KAAK,CAAC,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,EAAE,OAAO,CAAC,CAAC;KAC9C;IAHe,iBAAW,cAG1B,CAAA;AACH,CAAC,EA9GgB,aAAK,KAAL,aAAK,QA8GrB;;;;;;;;;AC1bD;AACA;;;AAOA;AACA,IAAI,OAAuB,CAAC;AAiIR,sBAAG;AA/HvB,IAAM,KAAK,GAAG,UAAU,eAAoB;;IAE1C,OAAO,eAAe,IAAI,eAAe,CAAC,IAAI,IAAI,IAAI,IAAI,eAAe,CAAC;AAC5E,CAAC,CAAC;AAEF;AACA,SAAS,SAAS;;IAEhB,QACE,KAAK,CAAC,OAAO,UAAU,KAAK,QAAQ,IAAI,UAAU,CAAC;QACnD,KAAK,CAAC,OAAO,MAAM,KAAK,QAAQ,IAAI,MAAM,CAAC;QAC3C,KAAK,CAAC,OAAO,IAAI,KAAK,QAAQ,IAAI,IAAI,CAAC;QACvC,KAAK,CAAC,OAAOnN,cAAM,KAAK,QAAQ,IAAIA,cAAM,CAAC;QAC3C,QAAQ,CAAC,aAAa,CAAC,EAAE,EACzB;AACJ,CAAC;AAED,IAAM,UAAU,GAAG,SAAS,EAAE,CAAC;AAC/B,IAAI,MAAM,CAAC,SAAS,CAAC,cAAc,CAAC,IAAI,CAAC,UAAU,EAAE,KAAK,CAAC,EAAE;IAC3D,cAAA,OAAO,GAAG,UAAU,CAAC,GAAG,CAAC;CAC1B;KAAM;;IAEL,cAAA,OAAO;QAGL,aAAY,KAA2B;YAA3B,sBAAA,EAAA,UAA2B;YACrC,IAAI,CAAC,KAAK,GAAG,EAAE,CAAC;YAChB,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC;YAElB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;gBACrC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,IAAI;oBAAE,SAAS;gBAC/B,IAAM,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;gBACvB,IAAM,GAAG,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;gBACrB,IAAM,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;;gBAEvB,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;;gBAGrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,EAAE,CAAC;aAC5D;SACF;QACD,mBAAK,GAAL;YACE,IAAI,CAAC,KAAK,GAAG,EAAE,CAAC;YAChB,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC;SACnB;QACD,oBAAM,GAAN,UAAO,GAAW;YAChB,IAAM,KAAK,GAAG,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC;YAChC,IAAI,KAAK,IAAI,IAAI;gBAAE,OAAO,KAAK,CAAC;;YAEhC,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC;;YAEzB,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;YAC9B,OAAO,IAAI,CAAC;SACb;QACD,qBAAO,GAAP;YAAA,iBAYC;YAXC,IAAI,KAAK,GAAG,CAAC,CAAC;YAEd,OAAO;gBACL,IAAI,EAAE;oBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;oBAChC,OAAO;wBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,CAAC,GAAG,EAAE,KAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,GAAG,SAAS;wBACjE,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;qBACvC,CAAC;iBACH;aACF,CAAC;SACH;QACD,qBAAO,GAAP,UAAQ,QAAmE,EAAE,IAAW;YACtF,IAAI,GAAG,IAAI,IAAI,IAAI,CAAC;YAEpB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;gBAC1C,IAAM,GAAG,GAAG,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;;gBAE1B,QAAQ,CAAC,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,EAAE,GAAG,EAAE,IAAI,CAAC,CAAC;aACrD;SACF;QACD,iBAAG,GAAH,UAAI,GAAW;YACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,SAAS,CAAC;SAC5D;QACD,iBAAG,GAAH,UAAI,GAAW;YACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC;SAClC;QACD,kBAAI,GAAJ;YAAA,iBAYC;YAXC,IAAI,KAAK,GAAG,CAAC,CAAC;YAEd,OAAO;gBACL,IAAI,EAAE;oBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;oBAChC,OAAO;wBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,GAAG,GAAG,SAAS;wBAC1C,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;qBACvC,CAAC;iBACH;aACF,CAAC;SACH;QACD,iBAAG,GAAH,UAAI,GAAW,EAAE,KAAU;YACzB,IAAI,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;gBACrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,KAAK,CAAC;gBAC5B,OAAO,IAAI,CAAC;aACb;;YAGD,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;;YAGrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,EAAE,CAAC;YAC3D,OAAO,IAAI,CAAC;SACb;QACD,oBAAM,GAAN;YAAA,iBAYC;YAXC,IAAI,KAAK,GAAG,CAAC,CAAC;YAEd,OAAO;gBACL,IAAI,EAAE;oBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;oBAChC,OAAO;wBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,KAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,SAAS;wBAC1D,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;qBACvC,CAAC;iBACH;aACF,CAAC;SACH;QACD,sBAAI,qBAAI;iBAAR;gBACE,OAAO,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC;aAC1B;;;WAAA;QACH,UAAC;KAtGU,GAsGoB,CAAC;CACjC;;;;;;;;;;ACxID;AACa,sBAAc,GAAG,UAAU,CAAC;AACzC;AACa,sBAAc,GAAG,CAAC,UAAU,CAAC;AAC1C;AACa,sBAAc,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;AAClD;AACa,sBAAc,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;AAE/C;;;;AAIa,kBAAU,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;AAE1C;;;;AAIa,kBAAU,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;AAE3C;AACa,wBAAgB,GAAG,CAAC,CAAC;AAElC;AACa,wBAAgB,GAAG,CAAC,CAAC;AAElC;AACa,wBAAgB,GAAG,CAAC,CAAC;AAElC;AACa,uBAAe,GAAG,CAAC,CAAC;AAEjC;AACa,wBAAgB,GAAG,CAAC,CAAC;AAElC;AACa,2BAAmB,GAAG,CAAC,CAAC;AAErC;AACa,qBAAa,GAAG,CAAC,CAAC;AAE/B;AACa,yBAAiB,GAAG,CAAC,CAAC;AAEnC;AACa,sBAAc,GAAG,CAAC,CAAC;AAEhC;AACa,sBAAc,GAAG,EAAE,CAAC;AAEjC;AACa,wBAAgB,GAAG,EAAE,CAAC;AAEnC;AACa,2BAAmB,GAAG,EAAE,CAAC;AAEtC;AACa,sBAAc,GAAG,EAAE,CAAC;AAEjC;AACa,wBAAgB,GAAG,EAAE,CAAC;AAEnC;AACa,8BAAsB,GAAG,EAAE,CAAC;AAEzC;AACa,qBAAa,GAAG,EAAE,CAAC;AAEhC;AACa,2BAAmB,GAAG,EAAE,CAAC;AAEtC;AACa,sBAAc,GAAG,EAAE,CAAC;AAEjC;AACa,4BAAoB,GAAG,EAAE,CAAC;AAEvC;AACa,yBAAiB,GAAG,IAAI,CAAC;AAEtC;AACa,yBAAiB,GAAG,IAAI,CAAC;AAEtC;AACa,mCAA2B,GAAG,CAAC,CAAC;AAE7C;AACa,oCAA4B,GAAG,CAAC,CAAC;AAE9C;AACa,sCAA8B,GAAG,CAAC,CAAC;AAEhD;AACa,gCAAwB,GAAG,CAAC,CAAC;AAE1C;AACa,oCAA4B,GAAG,CAAC,CAAC;AAE9C;AACa,+BAAuB,GAAG,CAAC,CAAC;AAEzC;AACa,wCAAgC,GAAG,GAAG,CAAC;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;ACvGpB;AACG;AAEO;AAC6C;AAEvF,SAAgB,mBAAmB,CACjC,MAAgB,EAChB,kBAA4B,EAC5B,eAAyB;IAEzB,IAAI,WAAW,GAAG,CAAC,GAAG,CAAC,CAAC;IAExB,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;QACzB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;YACtC,WAAW,IAAI,gBAAgB,CAC7B,CAAC,CAAC,QAAQ,EAAE,EACZ,MAAM,CAAC,CAAC,CAAC,EACT,kBAAkB,EAClB,IAAI,EACJ,eAAe,CAChB,CAAC;SACH;KACF;SAAM;;QAGL,IAAI,MAAM,CAAC,MAAM,EAAE;YACjB,MAAM,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC;SAC1B;;QAGD,KAAK,IAAM,GAAG,IAAI,MAAM,EAAE;YACxB,WAAW,IAAI,gBAAgB,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,CAAC,EAAE,kBAAkB,EAAE,KAAK,EAAE,eAAe,CAAC,CAAC;SAC/F;KACF;IAED,OAAO,WAAW,CAAC;AACrB,CAAC;AA/BD,kDA+BC;AAED;AACA,SAAS,gBAAgB,CACvB,IAAY;AACZ;AACA,KAAU,EACV,kBAA0B,EAC1B,OAAe,EACf,eAAuB;IAFvB,mCAAA,EAAA,0BAA0B;IAC1B,wBAAA,EAAA,eAAe;IACf,gCAAA,EAAA,uBAAuB;;IAGvB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;QACzB,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;KACxB;IAED,QAAQ,OAAO,KAAK;QAClB,KAAK,QAAQ;YACX,OAAO,CAAC,GAAGiH,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,UAAU,CAAC,KAAK,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;QAC5F,KAAK,QAAQ;YACX,IACE,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK;gBAC3B,KAAK,IAAI,SAAS,CAAC,UAAU;gBAC7B,KAAK,IAAI,SAAS,CAAC,UAAU,EAC7B;gBACA,IAAI,KAAK,IAAI,SAAS,CAAC,cAAc,IAAI,KAAK,IAAI,SAAS,CAAC,cAAc,EAAE;;oBAE1E,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;iBAC3E;qBAAM;oBACL,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;iBAC3E;aACF;iBAAM;;gBAEL,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;aAC3E;QACH,KAAK,WAAW;YACd,IAAI,OAAO,IAAI,CAAC,eAAe;gBAC7B,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;YACtE,OAAO,CAAC,CAAC;QACX,KAAK,SAAS;YACZ,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;QAC5E,KAAK,QAAQ;YACX,IAAI,KAAK,IAAI,IAAI,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBACvF,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;aACrE;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,CAAC,CAAC;aAC5E;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAIC,YAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;aAC3E;iBAAM,IACL,WAAW,CAAC,MAAM,CAAC,KAAK,CAAC;gBACzB,KAAK,YAAY,WAAW;gBAC5BC,sBAAgB,CAAC,KAAK,CAAC,EACvB;gBACA,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,KAAK,CAAC,UAAU,EACzF;aACH;iBAAM,IACL,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM;gBAC7B,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ;gBAC/B,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAClC;gBACA,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;aAC3E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,CAAC,CAAC;aAC5E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;;gBAExC,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,MAAM,GAAG,CAAC,EAAE;oBAC9D,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;wBACvD,CAAC;wBACD,CAAC;wBACD,CAAC;wBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,EAAE,MAAM,CAAC;wBAChD,CAAC;wBACD,mBAAmB,CAAC,KAAK,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACrE;iBACH;qBAAM;oBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;wBACvD,CAAC;wBACD,CAAC;wBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,EAAE,MAAM,CAAC;wBAChD,CAAC,EACD;iBACH;aACF;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;;gBAE1C,IAAI,KAAK,CAAC,QAAQ,KAAKI,aAAM,CAAC,kBAAkB,EAAE;oBAChD,QACE,CAAC,IAAI,IAAI,IAAI,GAAGJ,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;yBACtD,KAAK,CAAC,QAAQ,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EAChC;iBACH;qBAAM;oBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,KAAK,CAAC,QAAQ,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EACvF;iBACH;aACF;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,KAAK,EAAE,MAAM,CAAC;oBACtC,CAAC;oBACD,CAAC;oBACD,CAAC,EACD;aACH;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;;gBAEzC,IAAM,cAAc,GAAG,MAAM,CAAC,MAAM,CAClC;oBACE,IAAI,EAAE,KAAK,CAAC,UAAU;oBACtB,GAAG,EAAE,KAAK,CAAC,GAAG;iBACf,EACD,KAAK,CAAC,MAAM,CACb,CAAC;;gBAGF,IAAI,KAAK,CAAC,EAAE,IAAI,IAAI,EAAE;oBACpB,cAAc,CAAC,KAAK,CAAC,GAAG,KAAK,CAAC,EAAE,CAAC;iBAClC;gBAED,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvD,CAAC;oBACD,mBAAmB,CAAC,cAAc,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACxE;aACH;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAIC,cAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvD,CAAC;oBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,MAAM,EAAE,MAAM,CAAC;oBACvC,CAAC;qBACA,KAAK,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;qBACrB,KAAK,CAAC,UAAU,GAAG,CAAC,GAAG,CAAC,CAAC;qBACzB,KAAK,CAAC,SAAS,GAAG,CAAC,GAAG,CAAC,CAAC;oBACzB,CAAC,EACD;aACH;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvD,CAAC;oBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,MAAM,CAAC;oBACxC,CAAC;oBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,MAAM,CAAC;oBACxC,CAAC,EACD;aACH;iBAAM;gBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvD,mBAAmB,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC;oBAC/D,CAAC,EACD;aACH;QACH,KAAK,UAAU;;YAEb,IAAI,KAAK,YAAY,MAAM,IAAIC,cAAQ,CAAC,KAAK,CAAC,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,iBAAiB,EAAE;gBAC1F,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvD,CAAC;oBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,MAAM,EAAE,MAAM,CAAC;oBACvC,CAAC;qBACA,KAAK,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;qBACrB,KAAK,CAAC,UAAU,GAAG,CAAC,GAAG,CAAC,CAAC;qBACzB,KAAK,CAAC,SAAS,GAAG,CAAC,GAAG,CAAC,CAAC;oBACzB,CAAC,EACD;aACH;iBAAM;gBACL,IAAI,kBAAkB,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,MAAM,GAAG,CAAC,EAAE;oBACpF,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;wBACvD,CAAC;wBACD,CAAC;wBACD,CAAC;wBACDA,aAAM,CAAC,UAAU,CAACC,8BAAwB,CAAC,KAAK,CAAC,EAAE,MAAM,CAAC;wBAC1D,CAAC;wBACD,mBAAmB,CAAC,KAAK,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACrE;iBACH;qBAAM,IAAI,kBAAkB,EAAE;oBAC7B,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;wBACvD,CAAC;wBACD,CAAC;wBACDA,aAAM,CAAC,UAAU,CAACC,8BAAwB,CAAC,KAAK,CAAC,EAAE,MAAM,CAAC;wBAC1D,CAAC,EACD;iBACH;aACF;KACJ;IAED,OAAO,CAAC,CAAC;AACX,CAAC;;;;;;;;;;AClOD,IAAM,SAAS,GAAG,IAAI,CAAC;AACvB,IAAM,cAAc,GAAG,IAAI,CAAC;AAC5B,IAAM,gBAAgB,GAAG,IAAI,CAAC;AAC9B,IAAM,eAAe,GAAG,IAAI,CAAC;AAC7B,IAAM,eAAe,GAAG,IAAI,CAAC;AAE7B,IAAM,YAAY,GAAG,IAAI,CAAC;AAC1B,IAAM,cAAc,GAAG,IAAI,CAAC;AAC5B,IAAM,aAAa,GAAG,IAAI,CAAC;AAC3B,IAAM,eAAe,GAAG,IAAI,CAAC;AAE7B;;;;;;AAMA,SAAgB,YAAY,CAC1B,KAAkC,EAClC,KAAa,EACb,GAAW;IAEX,IAAI,YAAY,GAAG,CAAC,CAAC;IAErB,KAAK,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC,GAAG,GAAG,EAAE,CAAC,IAAI,CAAC,EAAE;QACnC,IAAM,IAAI,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;QAEtB,IAAI,YAAY,EAAE;YAChB,IAAI,CAAC,IAAI,GAAG,cAAc,MAAM,eAAe,EAAE;gBAC/C,OAAO,KAAK,CAAC;aACd;YACD,YAAY,IAAI,CAAC,CAAC;SACnB;aAAM,IAAI,IAAI,GAAG,SAAS,EAAE;YAC3B,IAAI,CAAC,IAAI,GAAG,gBAAgB,MAAM,YAAY,EAAE;gBAC9C,YAAY,GAAG,CAAC,CAAC;aAClB;iBAAM,IAAI,CAAC,IAAI,GAAG,eAAe,MAAM,cAAc,EAAE;gBACtD,YAAY,GAAG,CAAC,CAAC;aAClB;iBAAM,IAAI,CAAC,IAAI,GAAG,eAAe,MAAM,aAAa,EAAE;gBACrD,YAAY,GAAG,CAAC,CAAC;aAClB;iBAAM;gBACL,OAAO,KAAK,CAAC;aACd;SACF;KACF;IAED,OAAO,CAAC,YAAY,CAAC;AACvB,CAAC;AA7BD,oCA6BC;;;;;;;;;;AC9C+B;AACG;AAEJ;AACW;AACgB;AACf;AACR;AACD;AACH;AACK;AACA;AACG;AACA;AACA;AACE;AACO;AAgChD;AACA,IAAM,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;AAC9D,IAAM,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;AAE9D,IAAM,aAAa,GAAiC,EAAE,CAAC;AAEvD,SAAgB,WAAW,CACzB,MAAc,EACd,OAA2B,EAC3B,OAAiB;IAEjB,OAAO,GAAG,OAAO,IAAI,IAAI,GAAG,EAAE,GAAG,OAAO,CAAC;IACzC,IAAM,KAAK,GAAG,OAAO,IAAI,OAAO,CAAC,KAAK,GAAG,OAAO,CAAC,KAAK,GAAG,CAAC,CAAC;;IAE3D,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,CAAC;SACZ,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;SACvB,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;SACxB,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;IAE5B,IAAI,IAAI,GAAG,CAAC,EAAE;QACZ,MAAM,IAAI,KAAK,CAAC,gCAA8B,IAAM,CAAC,CAAC;KACvD;IAED,IAAI,OAAO,CAAC,gCAAgC,IAAI,MAAM,CAAC,MAAM,GAAG,IAAI,EAAE;QACpE,MAAM,IAAI,KAAK,CAAC,mBAAiB,MAAM,CAAC,MAAM,8BAAyB,IAAM,CAAC,CAAC;KAChF;IAED,IAAI,CAAC,OAAO,CAAC,gCAAgC,IAAI,MAAM,CAAC,MAAM,KAAK,IAAI,EAAE;QACvE,MAAM,IAAI,KAAK,CAAC,mBAAiB,MAAM,CAAC,MAAM,4BAAuB,IAAM,CAAC,CAAC;KAC9E;IAED,IAAI,IAAI,GAAG,KAAK,GAAG,MAAM,CAAC,UAAU,EAAE;QACpC,MAAM,IAAI,KAAK,CACb,gBAAc,IAAI,yBAAoB,KAAK,kCAA6B,MAAM,CAAC,UAAU,MAAG,CAC7F,CAAC;KACH;;IAGD,IAAI,MAAM,CAAC,KAAK,GAAG,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC,EAAE;QAClC,MAAM,IAAI,KAAK,CAAC,6EAA6E,CAAC,CAAC;KAChG;;IAGD,OAAO,iBAAiB,CAAC,MAAM,EAAE,KAAK,EAAE,OAAO,EAAE,OAAO,CAAC,CAAC;AAC5D,CAAC;AAvCD,kCAuCC;AAED,SAAS,iBAAiB,CACxBxX,QAAc,EACd,KAAa,EACb,OAA2B,EAC3B,OAAe;IAAf,wBAAA,EAAA,eAAe;IAEf,IAAM,aAAa,GAAG,OAAO,CAAC,eAAe,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,eAAe,CAAC,CAAC;IAC1F,IAAM,cAAc,GAAG,OAAO,CAAC,gBAAgB,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,gBAAgB,CAAC,CAAC;IAE7F,IAAM,WAAW,GAAG,OAAO,CAAC,aAAa,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,aAAa,CAAC,CAAC;;IAGnF,IAAM,GAAG,GAAG,OAAO,CAAC,KAAK,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,KAAK,CAAC,CAAC;;IAG5D,IAAM,UAAU,GAAG,OAAO,OAAO,CAAC,YAAY,CAAC,KAAK,SAAS,GAAG,OAAO,CAAC,YAAY,CAAC,GAAG,KAAK,CAAC;;IAG9F,IAAM,cAAc,GAAG,OAAO,CAAC,gBAAgB,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,gBAAgB,CAAC,CAAC;IAC7F,IAAM,YAAY,GAAG,OAAO,CAAC,cAAc,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,cAAc,CAAC,CAAC;IACtF,IAAM,aAAa,GAAG,OAAO,CAAC,eAAe,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,eAAe,CAAC,CAAC;;IAGzF,IAAM,UAAU,GAAG,KAAK,CAAC;;IAGzB,IAAIA,QAAM,CAAC,MAAM,GAAG,CAAC;QAAE,MAAM,IAAI,KAAK,CAAC,qCAAqC,CAAC,CAAC;;IAG9E,IAAM,IAAI,GACRA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;IAG/F,IAAI,IAAI,GAAG,CAAC,IAAI,IAAI,GAAGA,QAAM,CAAC,MAAM;QAAE,MAAM,IAAI,KAAK,CAAC,sBAAsB,CAAC,CAAC;;IAG9E,IAAM,MAAM,GAAa,OAAO,GAAG,EAAE,GAAG,EAAE,CAAC;;IAE3C,IAAI,UAAU,GAAG,CAAC,CAAC;IACnB,IAAM,IAAI,GAAG,KAAK,CAAC;;IAGnB,OAAO,CAAC,IAAI,EAAE;;QAEZ,IAAM,WAAW,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,CAAC;;QAGpC,IAAI,WAAW,KAAK,CAAC;YAAE,MAAM;;QAG7B,IAAI,CAAC,GAAG,KAAK,CAAC;;QAEd,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;YAC9C,CAAC,EAAE,CAAC;SACL;;QAGD,IAAI,CAAC,IAAIA,QAAM,CAAC,UAAU;YAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;QAClF,IAAM,IAAI,GAAG,OAAO,GAAG,UAAU,EAAE,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;QACxE,IAAI,KAAK,SAAA,CAAC;QAEV,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;QAEd,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YAC9C,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAE/C,IAAI,CAAC0d,0BAAY,CAAC1d,QAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,EAAE;gBACxD,MAAM,IAAI,KAAK,CAAC,uCAAuC,CAAC,CAAC;aAC1D;YAED,KAAK,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;YAE/D,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,EAAE;YAClD,IAAM,GAAG,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;YAC7BvX,QAAM,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;YACvC,KAAK,GAAG,IAAIgd,iBAAQ,CAAC,GAAG,CAAC,CAAC;YAC1B,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;SACpB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,IAAI,aAAa,KAAK,KAAK,EAAE;YAC7E,KAAK,GAAG,IAAIE,YAAK,CACfld,QAAM,CAAC,KAAK,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAC7F,CAAC;SACH;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,EAAE;YAClD,KAAK;gBACHA,QAAM,CAAC,KAAK,EAAE,CAAC;qBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;qBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;qBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;SAC3B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,aAAa,KAAK,KAAK,EAAE;YAChF,KAAK,GAAG,IAAI,eAAM,CAACA,QAAM,CAAC,YAAY,CAAC,KAAK,CAAC,CAAC,CAAC;YAC/C,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;SACnB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,KAAK,GAAGA,QAAM,CAAC,YAAY,CAAC,KAAK,CAAC,CAAC;YACnC,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;SACnB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;YACnD,IAAM,OAAO,GACXA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,KAAK,GAAG,IAAI,IAAI,CAAC,IAAI,WAAI,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC,QAAQ,EAAE,CAAC,CAAC;SAC1D;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;YACtD,IAAIA,QAAM,CAAC,KAAK,CAAC,KAAK,CAAC,IAAIA,QAAM,CAAC,KAAK,CAAC,KAAK,CAAC;gBAAE,MAAM,IAAI,KAAK,CAAC,4BAA4B,CAAC,CAAC;YAC9F,KAAK,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,KAAK,CAAC,CAAC;SAC/B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,IAAM,MAAM,GAAG,KAAK,CAAC;YACrB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;iBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;iBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;iBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;YAC5B,IAAI,UAAU,IAAI,CAAC,IAAI,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBACvD,MAAM,IAAI,KAAK,CAAC,sCAAsC,CAAC,CAAC;;YAG1D,IAAI,GAAG,EAAE;gBACP,KAAK,GAAGA,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,CAAC;aACjD;iBAAM;gBACL,KAAK,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC;aAC3D;YAED,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,eAAe,EAAE;YACpD,IAAM,MAAM,GAAG,KAAK,CAAC;YACrB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;iBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;iBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;iBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;YAC5B,IAAI,YAAY,GAAG,OAAO,CAAC;;YAG3B,IAAM,SAAS,GAAG,KAAK,GAAG,UAAU,CAAC;;YAGrC,IAAI,WAAW,IAAI,WAAW,CAAC,IAAI,CAAC,EAAE;gBACpC,YAAY,GAAG,EAAE,CAAC;gBAClB,KAAK,IAAM,CAAC,IAAI,OAAO,EAAE;oBACtB,YAEC,CAAC,CAAC,CAAC,GAAG,OAAO,CAAC,CAA6B,CAAC,CAAC;iBAChD;gBACD,YAAY,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC;aAC5B;YAED,KAAK,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,YAAY,EAAE,IAAI,CAAC,CAAC;YAC9D,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;YAE3B,IAAIA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,KAAK,CAAC;gBAAE,MAAM,IAAI,KAAK,CAAC,+BAA+B,CAAC,CAAC;YAC9E,IAAI,KAAK,KAAK,SAAS;gBAAE,MAAM,IAAI,KAAK,CAAC,sBAAsB,CAAC,CAAC;SAClE;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;YACxD,KAAK,GAAG,SAAS,CAAC;SACnB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;YACnD,KAAK,GAAG,IAAI,CAAC;SACd;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;;YAEnD,IAAM,OAAO,GACXA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,IAAI,GAAG,IAAI,WAAI,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;;YAEzC,IAAI,YAAY,IAAI,aAAa,KAAK,IAAI,EAAE;gBAC1C,KAAK;oBACH,IAAI,CAAC,eAAe,CAAC,eAAe,CAAC,IAAI,IAAI,CAAC,kBAAkB,CAAC,eAAe,CAAC;0BAC7E,IAAI,CAAC,QAAQ,EAAE;0BACf,IAAI,CAAC;aACZ;iBAAM;gBACL,KAAK,GAAG,IAAI,CAAC;aACd;SACF;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,oBAAoB,EAAE;;YAEzD,IAAM,KAAK,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;;YAE/BvX,QAAM,CAAC,IAAI,CAAC,KAAK,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;;YAEzC,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;;YAEnB,IAAM2d,YAAU,GAAG,IAAIR,qBAAU,CAAC,KAAK,CAAyC,CAAC;;YAEjF,IAAI,UAAU,IAAIQ,YAAU,IAAI,OAAOA,YAAU,CAAC,QAAQ,KAAK,UAAU,EAAE;gBACzE,KAAK,GAAGA,YAAU,CAAC,QAAQ,EAAE,CAAC;aAC/B;iBAAM;gBACL,KAAK,GAAGA,YAAU,CAAC;aACpB;SACF;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,IAAI,UAAU,GACZ3d,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,eAAe,GAAG,UAAU,CAAC;YACnC,IAAM,OAAO,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,CAAC;;YAGhC,IAAI,UAAU,GAAG,CAAC;gBAAE,MAAM,IAAI,KAAK,CAAC,yCAAyC,CAAC,CAAC;;YAG/E,IAAI,UAAU,GAAGA,QAAM,CAAC,UAAU;gBAChC,MAAM,IAAI,KAAK,CAAC,4CAA4C,CAAC,CAAC;;YAGhE,IAAIA,QAAM,CAAC,OAAO,CAAC,IAAI,IAAI,EAAE;;gBAE3B,IAAI,OAAO,KAAK2X,aAAM,CAAC,kBAAkB,EAAE;oBACzC,UAAU;wBACR3X,QAAM,CAAC,KAAK,EAAE,CAAC;6BACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;6BACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;6BACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;oBAC1B,IAAI,UAAU,GAAG,CAAC;wBAChB,MAAM,IAAI,KAAK,CAAC,0DAA0D,CAAC,CAAC;oBAC9E,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,6DAA6D,CAAC,CAAC;oBACjF,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,8DAA8D,CAAC,CAAC;iBACnF;gBAED,IAAI,cAAc,IAAI,aAAa,EAAE;oBACnC,KAAK,GAAGA,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,CAAC;iBACjD;qBAAM;oBACL,KAAK,GAAG,IAAI2X,aAAM,CAAC3X,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,EAAE,OAAO,CAAC,CAAC;iBACtE;aACF;iBAAM;gBACL,IAAM,OAAO,GAAGuX,aAAM,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC;;gBAEzC,IAAI,OAAO,KAAKI,aAAM,CAAC,kBAAkB,EAAE;oBACzC,UAAU;wBACR3X,QAAM,CAAC,KAAK,EAAE,CAAC;6BACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;6BACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;6BACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;oBAC1B,IAAI,UAAU,GAAG,CAAC;wBAChB,MAAM,IAAI,KAAK,CAAC,0DAA0D,CAAC,CAAC;oBAC9E,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,6DAA6D,CAAC,CAAC;oBACjF,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,8DAA8D,CAAC,CAAC;iBACnF;;gBAGD,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,UAAU,EAAE,CAAC,EAAE,EAAE;oBAC/B,OAAO,CAAC,CAAC,CAAC,GAAGA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,CAAC;iBAChC;gBAED,IAAI,cAAc,IAAI,aAAa,EAAE;oBACnC,KAAK,GAAG,OAAO,CAAC;iBACjB;qBAAM;oBACL,KAAK,GAAG,IAAI2X,aAAM,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;iBACtC;aACF;;YAGD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,UAAU,KAAK,KAAK,EAAE;;YAE7E,CAAC,GAAG,KAAK,CAAC;;YAEV,OAAO3X,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;;YAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;YAE9E,IAAM,MAAM,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;;YAEjD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;YAGd,CAAC,GAAG,KAAK,CAAC;;YAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;;YAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;YAE9E,IAAM,aAAa,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;YACxD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;YAGd,IAAM,YAAY,GAAG,IAAI,KAAK,CAAC,aAAa,CAAC,MAAM,CAAC,CAAC;;YAGrD,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,aAAa,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;gBACzC,QAAQ,aAAa,CAAC,CAAC,CAAC;oBACtB,KAAK,GAAG;wBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;wBACtB,MAAM;oBACR,KAAK,GAAG;wBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;wBACtB,MAAM;oBACR,KAAK,GAAG;wBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;wBACtB,MAAM;iBACT;aACF;YAED,KAAK,GAAG,IAAI,MAAM,CAAC,MAAM,EAAE,YAAY,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC;SACnD;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,UAAU,KAAK,IAAI,EAAE;;YAE5E,CAAC,GAAG,KAAK,CAAC;;YAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;;YAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;YAE9E,IAAM,MAAM,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;YACjD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;YAGd,CAAC,GAAG,KAAK,CAAC;;YAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;;YAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;YAE9E,IAAM,aAAa,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;YACxD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;YAGd,KAAK,GAAG,IAAIsd,iBAAU,CAAC,MAAM,EAAE,aAAa,CAAC,CAAC;SAC/C;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,IAAM,UAAU,GACdtd,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAC/C,IAAM4d,QAAM,GAAG5d,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;YACtE,KAAK,GAAG,aAAa,GAAG4d,QAAM,GAAG,IAAIX,iBAAU,CAACW,QAAM,CAAC,CAAC;YACxD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;YACxD,IAAM,OAAO,GACX5d,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAE1B,KAAK,GAAG,IAAIud,mBAAS,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;SAC1C;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;YACtD,KAAK,GAAG,IAAIH,cAAM,EAAE,CAAC;SACtB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;YACtD,KAAK,GAAG,IAAIC,cAAM,EAAE,CAAC;SACtB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;YACnD,IAAM,UAAU,GACdrd,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAC/C,IAAM,cAAc,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;YAG9E,IAAI,aAAa,EAAE;;gBAEjB,IAAI,cAAc,EAAE;;oBAElB,KAAK,GAAG,WAAW,CAAC,cAAc,EAAE,aAAa,EAAE,MAAM,CAAC,CAAC;iBAC5D;qBAAM;oBACL,KAAK,GAAG,WAAW,CAAC,cAAc,CAAC,CAAC;iBACrC;aACF;iBAAM;gBACL,KAAK,GAAG,IAAIwd,SAAI,CAAC,cAAc,CAAC,CAAC;aAClC;;YAGD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,sBAAsB,EAAE;YAC3D,IAAM,SAAS,GACbxd,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;YAG1B,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;gBAC7B,MAAM,IAAI,KAAK,CAAC,yDAAyD,CAAC,CAAC;aAC5E;;YAGD,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;YAE1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;;YAG/C,IAAM,cAAc,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;YAE9E,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;YAE3B,IAAM,MAAM,GAAG,KAAK,CAAC;;YAErB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;iBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;iBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;iBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;;YAE5B,IAAM,WAAW,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC;;YAEtE,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;YAG3B,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,UAAU,GAAG,UAAU,EAAE;gBAC/C,MAAM,IAAI,KAAK,CAAC,wDAAwD,CAAC,CAAC;aAC3E;;YAGD,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,UAAU,GAAG,UAAU,EAAE;gBAC/C,MAAM,IAAI,KAAK,CAAC,2DAA2D,CAAC,CAAC;aAC9E;;YAGD,IAAI,aAAa,EAAE;;gBAEjB,IAAI,cAAc,EAAE;;oBAElB,KAAK,GAAG,WAAW,CAAC,cAAc,EAAE,aAAa,EAAE,MAAM,CAAC,CAAC;iBAC5D;qBAAM;oBACL,KAAK,GAAG,WAAW,CAAC,cAAc,CAAC,CAAC;iBACrC;gBAED,KAAK,CAAC,KAAK,GAAG,WAAW,CAAC;aAC3B;iBAAM;gBACL,KAAK,GAAG,IAAIwd,SAAI,CAAC,cAAc,EAAE,WAAW,CAAC,CAAC;aAC/C;SACF;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;;YAExD,IAAM,UAAU,GACdxd,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;YAE1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;;YAE/C,IAAI,CAAC0d,0BAAY,CAAC1d,QAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,EAAE;gBACxD,MAAM,IAAI,KAAK,CAAC,uCAAuC,CAAC,CAAC;aAC1D;YACD,IAAM,SAAS,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;YAEzE,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;YAG3B,IAAM,SAAS,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;YACnCvX,QAAM,CAAC,IAAI,CAAC,SAAS,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;YAC7C,IAAM,GAAG,GAAG,IAAIgd,iBAAQ,CAAC,SAAS,CAAC,CAAC;;YAGpC,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;;YAGnB,KAAK,GAAG,IAAIS,YAAK,CAAC,SAAS,EAAE,GAAG,CAAC,CAAC;SACnC;aAAM;YACL,MAAM,IAAI,KAAK,CACb,6BAA6B,GAAG,WAAW,CAAC,QAAQ,CAAC,EAAE,CAAC,GAAG,kBAAkB,GAAG,IAAI,GAAG,GAAG,CAC3F,CAAC;SACH;QACD,IAAI,IAAI,KAAK,WAAW,EAAE;YACxB,MAAM,CAAC,cAAc,CAAC,MAAM,EAAE,IAAI,EAAE;gBAClC,KAAK,OAAA;gBACL,QAAQ,EAAE,IAAI;gBACd,UAAU,EAAE,IAAI;gBAChB,YAAY,EAAE,IAAI;aACnB,CAAC,CAAC;SACJ;aAAM;YACL,MAAM,CAAC,IAAI,CAAC,GAAG,KAAK,CAAC;SACtB;KACF;;IAGD,IAAI,IAAI,KAAK,KAAK,GAAG,UAAU,EAAE;QAC/B,IAAI,OAAO;YAAE,MAAM,IAAI,KAAK,CAAC,oBAAoB,CAAC,CAAC;QACnD,MAAM,IAAI,KAAK,CAAC,qBAAqB,CAAC,CAAC;KACxC;;IAGD,IAAM,UAAU,GAAG,MAAM,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,MAAM,CAAC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,GAAA,CAAC,CAAC;IACtE,IAAI,KAAK,GAAG,IAAI,CAAC;IACjB,UAAU,CAAC,OAAO,CAAC,UAAA,CAAC;QAClB,IAAI,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC;YAAE,KAAK,GAAG,KAAK,CAAC;KAC7D,CAAC,CAAC;;IAGH,IAAI,CAAC,KAAK;QAAE,OAAO,MAAM,CAAC;IAE1B,IAAIA,kBAAW,CAAC,MAAM,CAAC,EAAE;QACvB,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,MAAM,CAAuB,CAAC;QAC7D,OAAO,IAAI,CAAC,IAAI,CAAC;QACjB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAIA,YAAK,CAAC,MAAM,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;KAC7D;IAED,OAAO,MAAM,CAAC;AAChB,CAAC;AAED;;;;;AAKA,SAAS,WAAW,CAClB,cAAsB,EACtB,aAA4C,EAC5C,MAAiB;IAEjB,IAAI,CAAC,aAAa;QAAE,OAAO,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC;;IAExD,IAAI,aAAa,CAAC,cAAc,CAAC,IAAI,IAAI,EAAE;QACzC,aAAa,CAAC,cAAc,CAAC,GAAG,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC;KAC9D;;IAGD,OAAO,aAAa,CAAC,cAAc,CAAC,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;AACpD,CAAC;;;;;;;;ACxpBD;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;;AAIA,SAAgB,WAAW,CACzB,MAAyB,EACzB,MAAc,EACd,MAAwB,EACxB,IAAY,EACZ,MAAc;IAEd,IAAI,CAAS,CAAC;IACd,IAAI,CAAS,CAAC;IACd,IAAM,GAAG,GAAG,MAAM,KAAK,KAAK,CAAC;IAC7B,IAAM,IAAI,GAAG,MAAM,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;IACnC,IAAM,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;IAC7B,IAAM,KAAK,GAAG,IAAI,IAAI,CAAC,CAAC;IACxB,IAAI,KAAK,GAAG,CAAC,CAAC,CAAC;IACf,IAAI,CAAC,GAAG,GAAG,GAAG,CAAC,GAAG,MAAM,GAAG,CAAC,CAAC;IAC7B,IAAM,CAAC,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,CAAC,CAAC;IACvB,IAAI,CAAC,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;IAE3B,CAAC,IAAI,CAAC,CAAC;IAEP,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC,IAAI,CAAC,KAAK,IAAI,CAAC,CAAC,CAAC;IAC5B,CAAC,KAAK,CAAC,KAAK,CAAC;IACb,KAAK,IAAI,IAAI,CAAC;IACd,OAAO,KAAK,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,KAAK,IAAI,CAAC;QAAC,CAAC;IAExE,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC,IAAI,CAAC,KAAK,IAAI,CAAC,CAAC,CAAC;IAC5B,CAAC,KAAK,CAAC,KAAK,CAAC;IACb,KAAK,IAAI,IAAI,CAAC;IACd,OAAO,KAAK,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,KAAK,IAAI,CAAC;QAAC,CAAC;IAExE,IAAI,CAAC,KAAK,CAAC,EAAE;QACX,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;KACf;SAAM,IAAI,CAAC,KAAK,IAAI,EAAE;QACrB,OAAO,CAAC,GAAG,GAAG,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,QAAQ,CAAC;KAC1C;SAAM;QACL,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;QAC1B,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;KACf;IACD,OAAO,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,CAAC;AAClD,CAAC;AAvCD,kCAuCC;AAED,SAAgB,YAAY,CAC1B,MAAyB,EACzB,KAAa,EACb,MAAc,EACd,MAAwB,EACxB,IAAY,EACZ,MAAc;IAEd,IAAI,CAAS,CAAC;IACd,IAAI,CAAS,CAAC;IACd,IAAI,CAAS,CAAC;IACd,IAAM,GAAG,GAAG,MAAM,KAAK,KAAK,CAAC;IAC7B,IAAI,IAAI,GAAG,MAAM,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;IACjC,IAAM,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;IAC7B,IAAM,KAAK,GAAG,IAAI,IAAI,CAAC,CAAC;IACxB,IAAM,EAAE,GAAG,IAAI,KAAK,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,CAAC,CAAC;IACjE,IAAI,CAAC,GAAG,GAAG,GAAG,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;IAC7B,IAAM,CAAC,GAAG,GAAG,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;IACvB,IAAM,CAAC,GAAG,KAAK,GAAG,CAAC,KAAK,KAAK,KAAK,CAAC,IAAI,CAAC,GAAG,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;IAE9D,KAAK,GAAG,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC;IAExB,IAAI,KAAK,CAAC,KAAK,CAAC,IAAI,KAAK,KAAK,QAAQ,EAAE;QACtC,CAAC,GAAG,KAAK,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;QACzB,CAAC,GAAG,IAAI,CAAC;KACV;SAAM;QACL,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC;QAC3C,IAAI,KAAK,IAAI,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,EAAE;YACrC,CAAC,EAAE,CAAC;YACJ,CAAC,IAAI,CAAC,CAAC;SACR;QACD,IAAI,CAAC,GAAG,KAAK,IAAI,CAAC,EAAE;YAClB,KAAK,IAAI,EAAE,GAAG,CAAC,CAAC;SACjB;aAAM;YACL,KAAK,IAAI,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,GAAG,KAAK,CAAC,CAAC;SACtC;QACD,IAAI,KAAK,GAAG,CAAC,IAAI,CAAC,EAAE;YAClB,CAAC,EAAE,CAAC;YACJ,CAAC,IAAI,CAAC,CAAC;SACR;QAED,IAAI,CAAC,GAAG,KAAK,IAAI,IAAI,EAAE;YACrB,CAAC,GAAG,CAAC,CAAC;YACN,CAAC,GAAG,IAAI,CAAC;SACV;aAAM,IAAI,CAAC,GAAG,KAAK,IAAI,CAAC,EAAE;YACzB,CAAC,GAAG,CAAC,KAAK,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;YACxC,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;SACf;aAAM;YACL,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,KAAK,GAAG,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;YACvD,CAAC,GAAG,CAAC,CAAC;SACP;KACF;IAED,IAAI,KAAK,CAAC,KAAK,CAAC;QAAE,CAAC,GAAG,CAAC,CAAC;IAExB,OAAO,IAAI,IAAI,CAAC,EAAE;QAChB,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC;QAC9B,CAAC,IAAI,CAAC,CAAC;QACP,CAAC,IAAI,GAAG,CAAC;QACT,IAAI,IAAI,CAAC,CAAC;KACX;IAED,CAAC,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;IAEpB,IAAI,KAAK,CAAC,KAAK,CAAC;QAAE,CAAC,IAAI,CAAC,CAAC;IAEzB,IAAI,IAAI,IAAI,CAAC;IAEb,OAAO,IAAI,GAAG,CAAC,EAAE;QACf,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC;QAC9B,CAAC,IAAI,CAAC,CAAC;QACP,CAAC,IAAI,GAAG,CAAC;QACT,IAAI,IAAI,CAAC,CAAC;KACX;IAED,MAAM,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC;AACpC,CAAC;AA5ED,oCA4EC;;;;;;;;;;;ACtJkC;AAGO;AAIM;AACF;AACC;AAEhB;AACF;AAYZ;AAgBjB,IAAM,MAAM,GAAG,MAAM,CAAC;AACtB,IAAM,UAAU,GAAG,IAAI,GAAG,CAAC,CAAC,KAAK,EAAE,MAAM,EAAE,KAAK,EAAE,cAAc,CAAC,CAAC,CAAC;AAEnE;;;;;AAMA,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,GAAG,CAAC,CAAC;IACzC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;IAEtB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;IAE/D,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,EAAE,IAAI,IAAI,CAAC;IAC9C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,EAAE,IAAI,IAAI,CAAC;IAC9C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,CAAC,IAAI,IAAI,CAAC;IAC7C,MAAM,CAAC,KAAK,CAAC,GAAG,CAAC,IAAI,GAAG,CAAC,IAAI,IAAI,CAAC;;IAElC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,CAAC;;IAEzB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;;IAIjB,IACE,MAAM,CAAC,SAAS,CAAC,KAAK,CAAC;QACvB,KAAK,IAAI,SAAS,CAAC,cAAc;QACjC,KAAK,IAAI,SAAS,CAAC,cAAc,EACjC;;;QAGA,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;QAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;cACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;cAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;QAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;QAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC;QAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,CAAC,IAAI,IAAI,CAAC;QACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;QACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;KACxC;SAAM;;QAEL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;QAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;cACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;cAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;QAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;QAEpBI,yBAAY,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,EAAE,QAAQ,EAAE,EAAE,EAAE,CAAC,CAAC,CAAC;;QAEpD,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;KACnB;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,CAAU,EAAE,KAAa,EAAE,OAAiB;;IAE9F,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;IAG3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,gBAAgB,CACvB,MAAc,EACd,GAAW,EACX,KAAc,EACd,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;;IAE9C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;IAChC,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,KAAW,EAAE,KAAa,EAAE,OAAiB;;IAE/F,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;IAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAGpB,IAAM,WAAW,GAAG,WAAI,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,CAAC,CAAC;IACrD,IAAM,OAAO,GAAG,WAAW,CAAC,UAAU,EAAE,CAAC;IACzC,IAAM,QAAQ,GAAG,WAAW,CAAC,WAAW,EAAE,CAAC;;IAE3C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,OAAO,GAAG,IAAI,CAAC;IACjC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,CAAC,IAAI,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;;IAEzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;IAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;IAC1C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;IAC1C,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,IAAI,KAAK,CAAC,MAAM,IAAI,KAAK,CAAC,MAAM,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;QACtD,MAAM,KAAK,CAAC,QAAQ,GAAG,KAAK,CAAC,MAAM,GAAG,8BAA8B,CAAC,CAAC;KACvE;;IAED,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,MAAM,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;IAErE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;IAEvB,IAAI,KAAK,CAAC,UAAU;QAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IAC7C,IAAI,KAAK,CAAC,MAAM;QAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACzC,IAAI,KAAK,CAAC,SAAS;QAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;IAG5C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,mBAAmB,CAC1B,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAGpB,IAAI,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;QAGvC,MAAM,KAAK,CAAC,UAAU,GAAG,KAAK,CAAC,OAAO,GAAG,8BAA8B,CAAC,CAAC;KAC1E;;IAGD,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,OAAO,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;IAEtE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;IAEvB,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;IAEhG,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAsB,EACtB,KAAa,EACb,OAAiB;;IAGjB,IAAI,KAAK,KAAK,IAAI,EAAE;QAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;KAC5C;SAAM,IAAI,KAAK,CAAC,SAAS,KAAK,QAAQ,EAAE;QACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;KAC/C;SAAM;QACL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;KAC/C;;IAGD,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,iBAAiB,CACxB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;IAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAGpB,IAAI,OAAO,KAAK,CAAC,EAAE,KAAK,QAAQ,EAAE;QAChC,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,EAAE,EAAE,KAAK,EAAE,SAAS,EAAE,QAAQ,CAAC,CAAC;KACpD;SAAM,IAAIrG,kBAAY,CAAC,KAAK,CAAC,EAAE,CAAC,EAAE;;;QAGjC,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,QAAQ,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE,KAAK,CAAC,CAAC;KAC7C;SAAM;QACL,MAAM,IAAI,SAAS,CAAC,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,GAAG,2BAA2B,CAAC,CAAC;KACvF;;IAGD,OAAO,KAAK,GAAG,EAAE,CAAC;AACpB,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAA0B,EAC1B,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,IAAM,IAAI,GAAG,KAAK,CAAC,MAAM,CAAC;;IAE1B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;IAEtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,2BAA2B,CAAC;;IAExD,MAAM,CAAC,GAAG,CAACC,0BAAY,CAAC,KAAK,CAAC,EAAE,KAAK,CAAC,CAAC;;IAEvC,KAAK,GAAG,KAAK,GAAG,IAAI,CAAC;IACrB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,SAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,OAAe,EACf,IAAqB;IALrB,0BAAA,EAAA,iBAAiB;IACjB,sBAAA,EAAA,SAAS;IACT,mCAAA,EAAA,0BAA0B;IAC1B,gCAAA,EAAA,sBAAsB;IACtB,wBAAA,EAAA,eAAe;IACf,qBAAA,EAAA,SAAqB;IAErB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;QACpC,IAAI,IAAI,CAAC,CAAC,CAAC,KAAK,KAAK;YAAE,MAAM,IAAI,KAAK,CAAC,4BAA4B,CAAC,CAAC;KACtE;;IAGD,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;;IAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,GAAG,SAAS,CAAC,eAAe,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAEhG,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,IAAM,QAAQ,GAAG,aAAa,CAC5B,MAAM,EACN,KAAK,EACL,SAAS,EACT,KAAK,EACL,KAAK,GAAG,CAAC,EACT,kBAAkB,EAClB,eAAe,EACf,IAAI,CACL,CAAC;;IAEF,IAAI,CAAC,GAAG,EAAE,CAAC;IACX,OAAO,QAAQ,CAAC;AAClB,CAAC;AAED,SAAS,mBAAmB,CAC1B,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;IAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,oBAAoB,CAAC;;IAEjD,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;;;IAIpB,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,KAAK,CAAC,QAAQ,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE,KAAK,CAAC,CAAC;IAC/C,OAAO,KAAK,GAAG,EAAE,CAAC;AACpB,CAAC;AAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,KAAW,EAAE,KAAa,EAAE,OAAiB;;IAE/F,MAAM,CAAC,KAAK,EAAE,CAAC;QACb,KAAK,CAAC,SAAS,KAAK,MAAM,GAAG,SAAS,CAAC,cAAc,GAAG,SAAS,CAAC,mBAAmB,CAAC;;IAExF,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,IAAM,OAAO,GAAG,KAAK,CAAC,UAAU,EAAE,CAAC;IACnC,IAAM,QAAQ,GAAG,KAAK,CAAC,WAAW,EAAE,CAAC;;IAErC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,OAAO,GAAG,IAAI,CAAC;IACjC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,CAAC,IAAI,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;;IAEzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;IAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;IAC1C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;IAC1C,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,cAAc,CACrB,MAAc,EACd,GAAW,EACX,KAAqB,EACrB,KAAa,EACb,OAAiB;IAEjB,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;;IAExB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;IAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC;IAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,CAAC,IAAI,IAAI,CAAC;IACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;IACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;IACvC,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAG7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAGpBoG,yBAAY,CAAC,MAAM,EAAE,KAAK,CAAC,KAAK,EAAE,KAAK,EAAE,QAAQ,EAAE,EAAE,EAAE,CAAC,CAAC,CAAC;;IAG1D,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;IAClB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,iBAAiB,CACxB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,UAAkB,EAClB,MAAU,EACV,OAAiB;IAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;IAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,IAAM,cAAc,GAAGrG,8BAAwB,CAAC,KAAK,CAAC,CAAC;;IAGvD,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;IAE5E,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;IAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;IAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,aAAa,CACpB,MAAc,EACd,GAAW,EACX,KAAW,EACX,KAAa,EACb,SAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,OAAe;IAJf,0BAAA,EAAA,iBAAiB;IACjB,sBAAA,EAAA,SAAS;IACT,mCAAA,EAAA,0BAA0B;IAC1B,gCAAA,EAAA,sBAAsB;IACtB,wBAAA,EAAA,eAAe;IAEf,IAAI,KAAK,CAAC,KAAK,IAAI,OAAO,KAAK,CAAC,KAAK,KAAK,QAAQ,EAAE;;QAElD,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,sBAAsB,CAAC;;QAEnD,IAAM,oBAAoB,GAAG,CAAC,OAAO;cACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;cAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;QAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;QAGpB,IAAI,UAAU,GAAG,KAAK,CAAC;;;QAIvB,IAAM,cAAc,GAAG,OAAO,KAAK,CAAC,IAAI,KAAK,QAAQ,GAAG,KAAK,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC;;QAE3F,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;;QAElB,IAAM,QAAQ,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;QAEhF,MAAM,CAAC,KAAK,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;QAChC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;QAC3C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;QAC5C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;;QAE5C,MAAM,CAAC,KAAK,GAAG,CAAC,GAAG,QAAQ,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;QAErC,KAAK,GAAG,KAAK,GAAG,QAAQ,GAAG,CAAC,CAAC;;;QAI7B,IAAM,QAAQ,GAAG,aAAa,CAC5B,MAAM,EACN,KAAK,CAAC,KAAK,EACX,SAAS,EACT,KAAK,EACL,KAAK,GAAG,CAAC,EACT,kBAAkB,EAClB,eAAe,CAChB,CAAC;QACF,KAAK,GAAG,QAAQ,GAAG,CAAC,CAAC;;QAGrB,IAAM,SAAS,GAAG,QAAQ,GAAG,UAAU,CAAC;;QAGxC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,SAAS,GAAG,IAAI,CAAC;QACxC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,CAAC,IAAI,IAAI,CAAC;QAC/C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,EAAE,IAAI,IAAI,CAAC;QAChD,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,EAAE,IAAI,IAAI,CAAC;;QAEhD,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACrB;SAAM;QACL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;QAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;cACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;cAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;QAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;QAEpB,IAAM,cAAc,GAAG,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC;;QAE7C,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;QAE5E,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;QAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;QACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;QACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;QAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;QAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACrB;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,IAAM,IAAI,GAAG,KAAK,CAAC,KAAK,CAAC,IAAI,CAAwB,CAAC;;IAEtD,IAAI,IAAI,GAAG,KAAK,CAAC,QAAQ,CAAC;;IAE1B,IAAI,KAAK,CAAC,QAAQ,KAAKG,aAAM,CAAC,kBAAkB;QAAE,IAAI,GAAG,IAAI,GAAG,CAAC,CAAC;;IAElE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;IAEtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,QAAQ,CAAC;;IAGjC,IAAI,KAAK,CAAC,QAAQ,KAAKA,aAAM,CAAC,kBAAkB,EAAE;QAChD,IAAI,GAAG,IAAI,GAAG,CAAC,CAAC;QAChB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;QAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;QACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KACvC;;IAGD,MAAM,CAAC,GAAG,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;;IAExB,KAAK,GAAG,KAAK,GAAG,KAAK,CAAC,QAAQ,CAAC;IAC/B,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;IAEzE,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;IAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;IAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,cAAc,CACrB,MAAc,EACd,GAAW,EACX,KAAY,EACZ,KAAa,EACb,KAAa,EACb,kBAA2B,EAC3B,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IAEpB,IAAI,UAAU,GAAG,KAAK,CAAC;IACvB,IAAI,MAAM,GAAc;QACtB,IAAI,EAAE,KAAK,CAAC,UAAU,IAAI,KAAK,CAAC,SAAS;QACzC,GAAG,EAAE,KAAK,CAAC,GAAG;KACf,CAAC;IAEF,IAAI,KAAK,CAAC,EAAE,IAAI,IAAI,EAAE;QACpB,MAAM,CAAC,GAAG,GAAG,KAAK,CAAC,EAAE,CAAC;KACvB;IAED,MAAM,GAAG,MAAM,CAAC,MAAM,CAAC,MAAM,EAAE,KAAK,CAAC,MAAM,CAAC,CAAC;IAC7C,IAAM,QAAQ,GAAG,aAAa,CAAC,MAAM,EAAE,MAAM,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,kBAAkB,CAAC,CAAC;;IAG5F,IAAM,IAAI,GAAG,QAAQ,GAAG,UAAU,CAAC;;IAEnC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IACnC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IAC1C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IAC3C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;IAE3C,OAAO,QAAQ,CAAC;AAClB,CAAC;AAED,SAAgB,aAAa,CAC3B,MAAc,EACd,MAAgB,EAChB,SAAiB,EACjB,aAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,IAAqB;IALrB,0BAAA,EAAA,iBAAiB;IACjB,8BAAA,EAAA,iBAAiB;IACjB,sBAAA,EAAA,SAAS;IACT,mCAAA,EAAA,0BAA0B;IAC1B,gCAAA,EAAA,sBAAsB;IACtB,qBAAA,EAAA,SAAqB;IAErB,aAAa,GAAG,aAAa,IAAI,CAAC,CAAC;IACnC,IAAI,GAAG,IAAI,IAAI,EAAE,CAAC;;IAGlB,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;;IAGlB,IAAI,KAAK,GAAG,aAAa,GAAG,CAAC,CAAC;;IAG9B,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;;QAEzB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;YACtC,IAAM,GAAG,GAAG,EAAE,GAAG,CAAC,CAAC;YACnB,IAAI,KAAK,GAAG,MAAM,CAAC,CAAC,CAAC,CAAC;;YAGtB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;gBACzB,IAAI,OAAO,KAAK,CAAC,MAAM,KAAK,UAAU;oBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;gBACxF,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;aACxB;YAED,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;gBAC7B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;gBACpC,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;gBACpC,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;aACvE;iBAAM,IAAI,OAAO,KAAK,KAAK,SAAS,EAAE;gBACrC,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC3D;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAIH,YAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,KAAK,SAAS,EAAE;gBAC9B,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,KAAK,IAAI,EAAE;gBACzB,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC5D;iBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;gBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;gBAClE,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,IAAI,EACJ,IAAI,CACL,CAAC;aACH;iBAAM,IACL,OAAO,KAAK,KAAK,QAAQ;gBACzBsG,wBAAU,CAAC,KAAK,CAAC;gBACjB,KAAK,CAAC,SAAS,KAAK,YAAY,EAChC;gBACA,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC9D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;gBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC9E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;gBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,IAAI,CACL,CAAC;aACH;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,EAAE,IAAI,CAAC,CAAC;aACpF;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC9D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACzD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;aACjF;SACF;KACF;SAAM,IAAI,MAAM,YAAYC,OAAG,IAAIvG,WAAK,CAAC,MAAM,CAAC,EAAE;QACjD,IAAM,QAAQ,GAAG,MAAM,CAAC,OAAO,EAAE,CAAC;QAClC,IAAI,IAAI,GAAG,KAAK,CAAC;QAEjB,OAAO,CAAC,IAAI,EAAE;;YAEZ,IAAM,KAAK,GAAG,QAAQ,CAAC,IAAI,EAAE,CAAC;YAC9B,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC,IAAI,CAAC;;YAEpB,IAAI,IAAI;gBAAE,SAAS;;YAGnB,IAAM,GAAG,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;YAC3B,IAAM,KAAK,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;;YAG7B,IAAM,IAAI,GAAG,OAAO,KAAK,CAAC;;YAG1B,IAAI,OAAO,GAAG,KAAK,QAAQ,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;gBACnD,IAAI,GAAG,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;oBAG7B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,8BAA8B,CAAC,CAAC;iBAC5D;gBAED,IAAI,SAAS,EAAE;oBACb,IAAI,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,EAAE;wBAClB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,0BAA0B,CAAC,CAAC;qBACxD;yBAAM,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;wBAC5B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,uBAAuB,CAAC,CAAC;qBACrD;iBACF;aACF;YAED,IAAI,IAAI,KAAK,QAAQ,EAAE;gBACrB,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;gBAC5B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAIA,qBAAe,CAAC,KAAK,CAAC,IAAIA,sBAAgB,CAAC,KAAK,CAAC,EAAE;gBACjF,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;aACvE;iBAAM,IAAI,IAAI,KAAK,SAAS,EAAE;gBAC7B,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACrD;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAIA,YAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,KAAK,IAAI,KAAK,KAAK,KAAK,SAAS,IAAI,eAAe,KAAK,KAAK,CAAC,EAAE;gBAC/E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACtD;iBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;gBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;gBAC1D,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,KAAK,EACL,IAAI,CACL,CAAC;aACH;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBACnE,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;gBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,CAChB,CAAC;aACH;iBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;gBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC5F;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC9E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACnD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;aACjF;SACF;KACF;SAAM;;QAEL,IAAI,MAAM,CAAC,MAAM,EAAE;YACjB,IAAI,OAAO,MAAM,CAAC,MAAM,KAAK,UAAU;gBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;YACzF,MAAM,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC;YACzB,IAAI,MAAM,IAAI,IAAI,IAAI,OAAO,MAAM,KAAK,QAAQ;gBAC9C,MAAM,IAAI,SAAS,CAAC,0CAA0C,CAAC,CAAC;SACnE;;QAGD,KAAK,IAAM,GAAG,IAAI,MAAM,EAAE;YACxB,IAAI,KAAK,GAAG,MAAM,CAAC,GAAG,CAAC,CAAC;;YAExB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;gBACzB,IAAI,OAAO,KAAK,CAAC,MAAM,KAAK,UAAU;oBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;gBACxF,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;aACxB;;YAGD,IAAM,IAAI,GAAG,OAAO,KAAK,CAAC;;YAG1B,IAAI,OAAO,GAAG,KAAK,QAAQ,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;gBACnD,IAAI,GAAG,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;oBAG7B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,8BAA8B,CAAC,CAAC;iBAC5D;gBAED,IAAI,SAAS,EAAE;oBACb,IAAI,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,EAAE;wBAClB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,0BAA0B,CAAC,CAAC;qBACxD;yBAAM,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;wBAC5B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,uBAAuB,CAAC,CAAC;qBACrD;iBACF;aACF;YAED,IAAI,IAAI,KAAK,QAAQ,EAAE;gBACrB,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;gBAC5B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;gBAC5B,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;aACvE;iBAAM,IAAI,IAAI,KAAK,SAAS,EAAE;gBAC7B,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACrD;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAIA,YAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,KAAK,SAAS,EAAE;gBAC9B,IAAI,eAAe,KAAK,KAAK;oBAAE,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACjF;iBAAM,IAAI,KAAK,KAAK,IAAI,EAAE;gBACzB,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACtD;iBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;gBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;gBAC1D,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,KAAK,EACL,IAAI,CACL,CAAC;aACH;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBACnE,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;gBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,CAChB,CAAC;aACH;iBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;gBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC5F;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC9E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACnD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;aACjF;SACF;KACF;;IAGD,IAAI,CAAC,GAAG,EAAE,CAAC;;IAGX,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;IAGvB,IAAM,IAAI,GAAG,KAAK,GAAG,aAAa,CAAC;;IAEnC,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IACtC,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IAC7C,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IAC9C,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IAC9C,OAAO,KAAK,CAAC;AACf,CAAC;AAxUD,sCAwUC;;;;;;;;;;;AC7iC+B;AACE;AAiFhC,uFAjFOG,aAAM,OAiFP;AAhFsB;AA4E5B,qFA5EO6F,SAAI,OA4EP;AA3E2B;AA8E/B,sFA9EOC,YAAK,OA8EP;AA7EmC;AAwFxC,2FAxFON,qBAAU,OAwFP;AAvFsB;AAkFhC,uFAlFO,eAAM,OAkFP;AAjFuC;AACP;AACP;AAgF/B,sFAhFOD,YAAK,OAgFP;AA/EuB;AA4E5B,qFA5EO,WAAI,OA4EP;AA3EsB;AAqE1B,oFArEOa,OAAG,OAqEP;AApE8B;AA+EjC,uFA/EOV,cAAM,OA+EP;AA9E2B;AA6EjC,uFA7EOD,cAAM,OA6EP;AA5E8B;AAsEpC,yFAtEOJ,iBAAQ,OAsEP;AAaI,yFAnFLA,iBAAQ,OAmFK;AAlFuE;AAC7F;AAC+F;AACJ;AACrD;AAyEpC,2FAzEOM,iBAAU,OAyEP;AAxE0B;AA6DpC,2FA7DOL,iBAAU,OA6DP;AA5D4B;AAkEtC,0FAlEOM,mBAAS,OAkEP;AAjEmB;AA+D5B,qFA/DO3F,SAAI,OA+DP;AA3Be;AAhCnB,yHAAA,8BAA8B,OAAA;AAC9B,sHAAA,2BAA2B,OAAA;AAC3B,uHAAA,4BAA4B,OAAA;AAC5B,kHAAA,uBAAuB,OAAA;AACvB,2HAAA,gCAAgC,OAAA;AAChC,mHAAA,wBAAwB,OAAA;AACxB,uHAAA,4BAA4B,OAAA;AAC5B,0GAAA,eAAe,OAAA;AACf,2GAAA,gBAAgB,OAAA;AAChB,4GAAA,iBAAiB,OAAA;AACjB,yGAAA,cAAc,OAAA;AACd,iHAAA,sBAAsB,OAAA;AACtB,yGAAA,cAAc,OAAA;AACd,8GAAA,mBAAmB,OAAA;AACnB,+GAAA,oBAAoB,OAAA;AACpB,wGAAA,aAAa,OAAA;AACb,yGAAA,cAAc,OAAA;AACd,4GAAA,iBAAiB,OAAA;AACjB,4GAAA,iBAAiB,OAAA;AACjB,yGAAA,cAAc,OAAA;AACd,2GAAA,gBAAgB,OAAA;AAChB,2GAAA,gBAAgB,OAAA;AAChB,wGAAA,aAAa,OAAA;AACb,2GAAA,gBAAgB,OAAA;AAChB,2GAAA,gBAAgB,OAAA;AAChB,2GAAA,gBAAgB,OAAA;AAChB,8GAAA,mBAAmB,OAAA;AACnB,8GAAA,mBAAmB,OAAA;AACnB,yGAAA,cAAc,OAAA;AACd,yGAAA,cAAc,OAAA;AACd,yGAAA,cAAc,OAAA;AACd,yGAAA,cAAc,OAAA;AAKhB,oCAAsD;AAA7C,sGAAA,KAAK,OAAA;AAQd,4BAKqB;AAHnB,sHAAA,yBAAyB,OAAA;AAkC3B;AACA;AACA,IAAM,OAAO,GAAG,IAAI,GAAG,IAAI,GAAG,EAAE,CAAC;AAEjC;AACA,IAAI5X,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC;AAEnC;;;;;;AAMA,SAAgB,qBAAqB,CAAC,IAAY;;IAEhD,IAAIvX,QAAM,CAAC,MAAM,GAAG,IAAI,EAAE;QACxBA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;KAC7B;AACH,CAAC;AALD,sDAKC;AAED;;;;;;;AAOA,SAAgB,SAAS,CAAC,MAAgB,EAAE,OAA8B;IAA9B,wBAAA,EAAA,YAA8B;;IAExE,IAAM,SAAS,GAAG,OAAO,OAAO,CAAC,SAAS,KAAK,SAAS,GAAG,OAAO,CAAC,SAAS,GAAG,KAAK,CAAC;IACrF,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;IACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;IAChF,IAAM,qBAAqB,GACzB,OAAO,OAAO,CAAC,qBAAqB,KAAK,QAAQ,GAAG,OAAO,CAAC,qBAAqB,GAAG,OAAO,CAAC;;IAG9F,IAAIvX,QAAM,CAAC,MAAM,GAAG,qBAAqB,EAAE;QACzCA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,qBAAqB,CAAC,CAAC;KAC9C;;IAGD,IAAM,kBAAkB,GAAGyG,wBAAiB,CAC1Che,QAAM,EACN,MAAM,EACN,SAAS,EACT,CAAC,EACD,CAAC,EACD,kBAAkB,EAClB,eAAe,EACf,EAAE,CACH,CAAC;;IAGF,IAAM,cAAc,GAAGuX,aAAM,CAAC,KAAK,CAAC,kBAAkB,CAAC,CAAC;;IAGxDvX,QAAM,CAAC,IAAI,CAAC,cAAc,EAAE,CAAC,EAAE,CAAC,EAAE,cAAc,CAAC,MAAM,CAAC,CAAC;;IAGzD,OAAO,cAAc,CAAC;AACxB,CAAC;AAnCD,8BAmCC;AAED;;;;;;;;;AASA,SAAgB,2BAA2B,CACzC,MAAgB,EAChB,WAAmB,EACnB,OAA8B;IAA9B,wBAAA,EAAA,YAA8B;;IAG9B,IAAM,SAAS,GAAG,OAAO,OAAO,CAAC,SAAS,KAAK,SAAS,GAAG,OAAO,CAAC,SAAS,GAAG,KAAK,CAAC;IACrF,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;IACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;IAChF,IAAM,UAAU,GAAG,OAAO,OAAO,CAAC,KAAK,KAAK,QAAQ,GAAG,OAAO,CAAC,KAAK,GAAG,CAAC,CAAC;;IAGzE,IAAM,kBAAkB,GAAGge,wBAAiB,CAC1Che,QAAM,EACN,MAAM,EACN,SAAS,EACT,CAAC,EACD,CAAC,EACD,kBAAkB,EAClB,eAAe,CAChB,CAAC;IACFA,QAAM,CAAC,IAAI,CAAC,WAAW,EAAE,UAAU,EAAE,CAAC,EAAE,kBAAkB,CAAC,CAAC;;IAG5D,OAAO,UAAU,GAAG,kBAAkB,GAAG,CAAC,CAAC;AAC7C,CAAC;AA3BD,kEA2BC;AAED;;;;;;;AAOA,SAAgB,WAAW,CACzB,MAA8C,EAC9C,OAAgC;IAAhC,wBAAA,EAAA,YAAgC;IAEhC,OAAOie,wBAAmB,CAACxG,0BAAY,CAAC,MAAM,CAAC,EAAE,OAAO,CAAC,CAAC;AAC5D,CAAC;AALD,kCAKC;AAQD;;;;;;;AAOA,SAAgB,mBAAmB,CACjC,MAAgB,EAChB,OAAwC;IAAxC,wBAAA,EAAA,YAAwC;IAExC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;IAExB,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;IACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;IAEhF,OAAOyG,kCAA2B,CAAC,MAAM,EAAE,kBAAkB,EAAE,eAAe,CAAC,CAAC;AAClF,CAAC;AAZD,kDAYC;AAED;;;;;;;;;;;;AAYA,SAAgB,iBAAiB,CAC/B,IAA4C,EAC5C,UAAkB,EAClB,iBAAyB,EACzB,SAAqB,EACrB,aAAqB,EACrB,OAA2B;IAE3B,IAAM,eAAe,GAAG,MAAM,CAAC,MAAM,CACnC,EAAE,gCAAgC,EAAE,IAAI,EAAE,KAAK,EAAE,CAAC,EAAE,EACpD,OAAO,CACR,CAAC;IACF,IAAM,UAAU,GAAGzG,0BAAY,CAAC,IAAI,CAAC,CAAC;IAEtC,IAAI,KAAK,GAAG,UAAU,CAAC;;IAEvB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,iBAAiB,EAAE,CAAC,EAAE,EAAE;;QAE1C,IAAM,IAAI,GACR,UAAU,CAAC,KAAK,CAAC;aAChB,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;aAC3B,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;aAC5B,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;;QAEhC,eAAe,CAAC,KAAK,GAAG,KAAK,CAAC;;QAE9B,SAAS,CAAC,aAAa,GAAG,CAAC,CAAC,GAAGwG,wBAAmB,CAAC,UAAU,EAAE,eAAe,CAAC,CAAC;;QAEhF,KAAK,GAAG,KAAK,GAAG,IAAI,CAAC;KACtB;;IAGD,OAAO,KAAK,CAAC;AACf,CAAC;AAjCD,8CAiCC;AAED;;;;;;;;AAQA,IAAM,IAAI,GAAG;IACX,MAAM,eAAA;IACN,IAAI,WAAA;IACJ,KAAK,cAAA;IACL,UAAU,uBAAA;IACV,MAAM,iBAAA;IACN,KAAK,cAAA;IACL,IAAI,aAAA;IACJ,IAAI,WAAA;IACJ,GAAG,SAAA;IACH,MAAM,gBAAA;IACN,MAAM,gBAAA;IACN,QAAQ,mBAAA;IACR,QAAQ,EAAEjB,iBAAQ;IAClB,UAAU,mBAAA;IACV,UAAU,mBAAA;IACV,SAAS,qBAAA;IACT,KAAK,qBAAA;IACL,qBAAqB,uBAAA;IACrB,SAAS,WAAA;IACT,2BAA2B,6BAAA;IAC3B,WAAW,aAAA;IACX,mBAAmB,qBAAA;IACnB,iBAAiB,mBAAA;CAClB,CAAC;AACF,kBAAe,IAAI,CAAC;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;"}
\ No newline at end of file
diff --git a/node_modules/bson/dist/bson.browser.umd.js b/node_modules/bson/dist/bson.browser.umd.js
new file mode 100644
index 0000000..eafb947
--- /dev/null
+++ b/node_modules/bson/dist/bson.browser.umd.js
@@ -0,0 +1,9017 @@
+(function (global, factory) {
+ typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) :
+ typeof define === 'function' && define.amd ? define(['exports'], factory) :
+ (global = typeof globalThis !== 'undefined' ? globalThis : global || self, factory(global.BSON = {}));
+}(this, (function (exports) { 'use strict';
+
+ var commonjsGlobal = typeof globalThis !== 'undefined' ? globalThis : typeof window !== 'undefined' ? window : typeof global !== 'undefined' ? global : typeof self !== 'undefined' ? self : {};
+
+ function unwrapExports (x) {
+ return x && x.__esModule && Object.prototype.hasOwnProperty.call(x, 'default') ? x['default'] : x;
+ }
+
+ function createCommonjsModule(fn, module) {
+ return module = { exports: {} }, fn(module, module.exports), module.exports;
+ }
+
+ var byteLength_1 = byteLength;
+ var toByteArray_1 = toByteArray;
+ var fromByteArray_1 = fromByteArray;
+ var lookup = [];
+ var revLookup = [];
+ var Arr = typeof Uint8Array !== 'undefined' ? Uint8Array : Array;
+ var code$1 = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/';
+
+ for (var i = 0, len = code$1.length; i < len; ++i) {
+ lookup[i] = code$1[i];
+ revLookup[code$1.charCodeAt(i)] = i;
+ } // Support decoding URL-safe base64 strings, as Node.js does.
+ // See: https://en.wikipedia.org/wiki/Base64#URL_applications
+
+
+ revLookup['-'.charCodeAt(0)] = 62;
+ revLookup['_'.charCodeAt(0)] = 63;
+
+ function getLens(b64) {
+ var len = b64.length;
+
+ if (len % 4 > 0) {
+ throw new Error('Invalid string. Length must be a multiple of 4');
+ } // Trim off extra bytes after placeholder bytes are found
+ // See: https://github.com/beatgammit/base64-js/issues/42
+
+
+ var validLen = b64.indexOf('=');
+ if (validLen === -1) validLen = len;
+ var placeHoldersLen = validLen === len ? 0 : 4 - validLen % 4;
+ return [validLen, placeHoldersLen];
+ } // base64 is 4/3 + up to two characters of the original data
+
+
+ function byteLength(b64) {
+ var lens = getLens(b64);
+ var validLen = lens[0];
+ var placeHoldersLen = lens[1];
+ return (validLen + placeHoldersLen) * 3 / 4 - placeHoldersLen;
+ }
+
+ function _byteLength(b64, validLen, placeHoldersLen) {
+ return (validLen + placeHoldersLen) * 3 / 4 - placeHoldersLen;
+ }
+
+ function toByteArray(b64) {
+ var tmp;
+ var lens = getLens(b64);
+ var validLen = lens[0];
+ var placeHoldersLen = lens[1];
+ var arr = new Arr(_byteLength(b64, validLen, placeHoldersLen));
+ var curByte = 0; // if there are placeholders, only get up to the last complete 4 chars
+
+ var len = placeHoldersLen > 0 ? validLen - 4 : validLen;
+ var i;
+
+ for (i = 0; i < len; i += 4) {
+ tmp = revLookup[b64.charCodeAt(i)] << 18 | revLookup[b64.charCodeAt(i + 1)] << 12 | revLookup[b64.charCodeAt(i + 2)] << 6 | revLookup[b64.charCodeAt(i + 3)];
+ arr[curByte++] = tmp >> 16 & 0xFF;
+ arr[curByte++] = tmp >> 8 & 0xFF;
+ arr[curByte++] = tmp & 0xFF;
+ }
+
+ if (placeHoldersLen === 2) {
+ tmp = revLookup[b64.charCodeAt(i)] << 2 | revLookup[b64.charCodeAt(i + 1)] >> 4;
+ arr[curByte++] = tmp & 0xFF;
+ }
+
+ if (placeHoldersLen === 1) {
+ tmp = revLookup[b64.charCodeAt(i)] << 10 | revLookup[b64.charCodeAt(i + 1)] << 4 | revLookup[b64.charCodeAt(i + 2)] >> 2;
+ arr[curByte++] = tmp >> 8 & 0xFF;
+ arr[curByte++] = tmp & 0xFF;
+ }
+
+ return arr;
+ }
+
+ function tripletToBase64(num) {
+ return lookup[num >> 18 & 0x3F] + lookup[num >> 12 & 0x3F] + lookup[num >> 6 & 0x3F] + lookup[num & 0x3F];
+ }
+
+ function encodeChunk(uint8, start, end) {
+ var tmp;
+ var output = [];
+
+ for (var i = start; i < end; i += 3) {
+ tmp = (uint8[i] << 16 & 0xFF0000) + (uint8[i + 1] << 8 & 0xFF00) + (uint8[i + 2] & 0xFF);
+ output.push(tripletToBase64(tmp));
+ }
+
+ return output.join('');
+ }
+
+ function fromByteArray(uint8) {
+ var tmp;
+ var len = uint8.length;
+ var extraBytes = len % 3; // if we have 1 byte left, pad 2 bytes
+
+ var parts = [];
+ var maxChunkLength = 16383; // must be multiple of 3
+ // go through the array every three bytes, we'll deal with trailing stuff later
+
+ for (var i = 0, len2 = len - extraBytes; i < len2; i += maxChunkLength) {
+ parts.push(encodeChunk(uint8, i, i + maxChunkLength > len2 ? len2 : i + maxChunkLength));
+ } // pad the end with zeros, but make sure to not forget the extra bytes
+
+
+ if (extraBytes === 1) {
+ tmp = uint8[len - 1];
+ parts.push(lookup[tmp >> 2] + lookup[tmp << 4 & 0x3F] + '==');
+ } else if (extraBytes === 2) {
+ tmp = (uint8[len - 2] << 8) + uint8[len - 1];
+ parts.push(lookup[tmp >> 10] + lookup[tmp >> 4 & 0x3F] + lookup[tmp << 2 & 0x3F] + '=');
+ }
+
+ return parts.join('');
+ }
+
+ var base64Js = {
+ byteLength: byteLength_1,
+ toByteArray: toByteArray_1,
+ fromByteArray: fromByteArray_1
+ };
+
+ /*! ieee754. BSD-3-Clause License. Feross Aboukhadijeh */
+ var read = function read(buffer, offset, isLE, mLen, nBytes) {
+ var e, m;
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var nBits = -7;
+ var i = isLE ? nBytes - 1 : 0;
+ var d = isLE ? -1 : 1;
+ var s = buffer[offset + i];
+ i += d;
+ e = s & (1 << -nBits) - 1;
+ s >>= -nBits;
+ nBits += eLen;
+
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8) {}
+
+ m = e & (1 << -nBits) - 1;
+ e >>= -nBits;
+ nBits += mLen;
+
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8) {}
+
+ if (e === 0) {
+ e = 1 - eBias;
+ } else if (e === eMax) {
+ return m ? NaN : (s ? -1 : 1) * Infinity;
+ } else {
+ m = m + Math.pow(2, mLen);
+ e = e - eBias;
+ }
+
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
+ };
+
+ var write = function write(buffer, value, offset, isLE, mLen, nBytes) {
+ var e, m, c;
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0;
+ var i = isLE ? 0 : nBytes - 1;
+ var d = isLE ? 1 : -1;
+ var s = value < 0 || value === 0 && 1 / value < 0 ? 1 : 0;
+ value = Math.abs(value);
+
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0;
+ e = eMax;
+ } else {
+ e = Math.floor(Math.log(value) / Math.LN2);
+
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--;
+ c *= 2;
+ }
+
+ if (e + eBias >= 1) {
+ value += rt / c;
+ } else {
+ value += rt * Math.pow(2, 1 - eBias);
+ }
+
+ if (value * c >= 2) {
+ e++;
+ c /= 2;
+ }
+
+ if (e + eBias >= eMax) {
+ m = 0;
+ e = eMax;
+ } else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen);
+ e = e + eBias;
+ } else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
+ e = 0;
+ }
+ }
+
+ for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}
+
+ e = e << mLen | m;
+ eLen += mLen;
+
+ for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}
+
+ buffer[offset + i - d] |= s * 128;
+ };
+
+ var ieee754 = {
+ read: read,
+ write: write
+ };
+
+ var buffer = createCommonjsModule(function (module, exports) {
+
+ var customInspectSymbol = typeof Symbol === 'function' && typeof Symbol['for'] === 'function' ? // eslint-disable-line dot-notation
+ Symbol['for']('nodejs.util.inspect.custom') // eslint-disable-line dot-notation
+ : null;
+ exports.Buffer = Buffer;
+ exports.SlowBuffer = SlowBuffer;
+ exports.INSPECT_MAX_BYTES = 50;
+ var K_MAX_LENGTH = 0x7fffffff;
+ exports.kMaxLength = K_MAX_LENGTH;
+ /**
+ * If `Buffer.TYPED_ARRAY_SUPPORT`:
+ * === true Use Uint8Array implementation (fastest)
+ * === false Print warning and recommend using `buffer` v4.x which has an Object
+ * implementation (most compatible, even IE6)
+ *
+ * Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,
+ * Opera 11.6+, iOS 4.2+.
+ *
+ * We report that the browser does not support typed arrays if the are not subclassable
+ * using __proto__. Firefox 4-29 lacks support for adding new properties to `Uint8Array`
+ * (See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438). IE 10 lacks support
+ * for __proto__ and has a buggy typed array implementation.
+ */
+
+ Buffer.TYPED_ARRAY_SUPPORT = typedArraySupport();
+
+ if (!Buffer.TYPED_ARRAY_SUPPORT && typeof console !== 'undefined' && typeof console.error === 'function') {
+ console.error('This browser lacks typed array (Uint8Array) support which is required by ' + '`buffer` v5.x. Use `buffer` v4.x if you require old browser support.');
+ }
+
+ function typedArraySupport() {
+ // Can typed array instances can be augmented?
+ try {
+ var arr = new Uint8Array(1);
+ var proto = {
+ foo: function foo() {
+ return 42;
+ }
+ };
+ Object.setPrototypeOf(proto, Uint8Array.prototype);
+ Object.setPrototypeOf(arr, proto);
+ return arr.foo() === 42;
+ } catch (e) {
+ return false;
+ }
+ }
+
+ Object.defineProperty(Buffer.prototype, 'parent', {
+ enumerable: true,
+ get: function get() {
+ if (!Buffer.isBuffer(this)) return undefined;
+ return this.buffer;
+ }
+ });
+ Object.defineProperty(Buffer.prototype, 'offset', {
+ enumerable: true,
+ get: function get() {
+ if (!Buffer.isBuffer(this)) return undefined;
+ return this.byteOffset;
+ }
+ });
+
+ function createBuffer(length) {
+ if (length > K_MAX_LENGTH) {
+ throw new RangeError('The value "' + length + '" is invalid for option "size"');
+ } // Return an augmented `Uint8Array` instance
+
+
+ var buf = new Uint8Array(length);
+ Object.setPrototypeOf(buf, Buffer.prototype);
+ return buf;
+ }
+ /**
+ * The Buffer constructor returns instances of `Uint8Array` that have their
+ * prototype changed to `Buffer.prototype`. Furthermore, `Buffer` is a subclass of
+ * `Uint8Array`, so the returned instances will have all the node `Buffer` methods
+ * and the `Uint8Array` methods. Square bracket notation works as expected -- it
+ * returns a single octet.
+ *
+ * The `Uint8Array` prototype remains unmodified.
+ */
+
+
+ function Buffer(arg, encodingOrOffset, length) {
+ // Common case.
+ if (typeof arg === 'number') {
+ if (typeof encodingOrOffset === 'string') {
+ throw new TypeError('The "string" argument must be of type string. Received type number');
+ }
+
+ return allocUnsafe(arg);
+ }
+
+ return from(arg, encodingOrOffset, length);
+ }
+
+ Buffer.poolSize = 8192; // not used by this implementation
+
+ function from(value, encodingOrOffset, length) {
+ if (typeof value === 'string') {
+ return fromString(value, encodingOrOffset);
+ }
+
+ if (ArrayBuffer.isView(value)) {
+ return fromArrayView(value);
+ }
+
+ if (value == null) {
+ throw new TypeError('The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' + 'or Array-like Object. Received type ' + babelHelpers["typeof"](value));
+ }
+
+ if (isInstance(value, ArrayBuffer) || value && isInstance(value.buffer, ArrayBuffer)) {
+ return fromArrayBuffer(value, encodingOrOffset, length);
+ }
+
+ if (typeof SharedArrayBuffer !== 'undefined' && (isInstance(value, SharedArrayBuffer) || value && isInstance(value.buffer, SharedArrayBuffer))) {
+ return fromArrayBuffer(value, encodingOrOffset, length);
+ }
+
+ if (typeof value === 'number') {
+ throw new TypeError('The "value" argument must not be of type number. Received type number');
+ }
+
+ var valueOf = value.valueOf && value.valueOf();
+
+ if (valueOf != null && valueOf !== value) {
+ return Buffer.from(valueOf, encodingOrOffset, length);
+ }
+
+ var b = fromObject(value);
+ if (b) return b;
+
+ if (typeof Symbol !== 'undefined' && Symbol.toPrimitive != null && typeof value[Symbol.toPrimitive] === 'function') {
+ return Buffer.from(value[Symbol.toPrimitive]('string'), encodingOrOffset, length);
+ }
+
+ throw new TypeError('The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' + 'or Array-like Object. Received type ' + babelHelpers["typeof"](value));
+ }
+ /**
+ * Functionally equivalent to Buffer(arg, encoding) but throws a TypeError
+ * if value is a number.
+ * Buffer.from(str[, encoding])
+ * Buffer.from(array)
+ * Buffer.from(buffer)
+ * Buffer.from(arrayBuffer[, byteOffset[, length]])
+ **/
+
+
+ Buffer.from = function (value, encodingOrOffset, length) {
+ return from(value, encodingOrOffset, length);
+ }; // Note: Change prototype *after* Buffer.from is defined to workaround Chrome bug:
+ // https://github.com/feross/buffer/pull/148
+
+
+ Object.setPrototypeOf(Buffer.prototype, Uint8Array.prototype);
+ Object.setPrototypeOf(Buffer, Uint8Array);
+
+ function assertSize(size) {
+ if (typeof size !== 'number') {
+ throw new TypeError('"size" argument must be of type number');
+ } else if (size < 0) {
+ throw new RangeError('The value "' + size + '" is invalid for option "size"');
+ }
+ }
+
+ function alloc(size, fill, encoding) {
+ assertSize(size);
+
+ if (size <= 0) {
+ return createBuffer(size);
+ }
+
+ if (fill !== undefined) {
+ // Only pay attention to encoding if it's a string. This
+ // prevents accidentally sending in a number that would
+ // be interpreted as a start offset.
+ return typeof encoding === 'string' ? createBuffer(size).fill(fill, encoding) : createBuffer(size).fill(fill);
+ }
+
+ return createBuffer(size);
+ }
+ /**
+ * Creates a new filled Buffer instance.
+ * alloc(size[, fill[, encoding]])
+ **/
+
+
+ Buffer.alloc = function (size, fill, encoding) {
+ return alloc(size, fill, encoding);
+ };
+
+ function allocUnsafe(size) {
+ assertSize(size);
+ return createBuffer(size < 0 ? 0 : checked(size) | 0);
+ }
+ /**
+ * Equivalent to Buffer(num), by default creates a non-zero-filled Buffer instance.
+ * */
+
+
+ Buffer.allocUnsafe = function (size) {
+ return allocUnsafe(size);
+ };
+ /**
+ * Equivalent to SlowBuffer(num), by default creates a non-zero-filled Buffer instance.
+ */
+
+
+ Buffer.allocUnsafeSlow = function (size) {
+ return allocUnsafe(size);
+ };
+
+ function fromString(string, encoding) {
+ if (typeof encoding !== 'string' || encoding === '') {
+ encoding = 'utf8';
+ }
+
+ if (!Buffer.isEncoding(encoding)) {
+ throw new TypeError('Unknown encoding: ' + encoding);
+ }
+
+ var length = byteLength(string, encoding) | 0;
+ var buf = createBuffer(length);
+ var actual = buf.write(string, encoding);
+
+ if (actual !== length) {
+ // Writing a hex string, for example, that contains invalid characters will
+ // cause everything after the first invalid character to be ignored. (e.g.
+ // 'abxxcd' will be treated as 'ab')
+ buf = buf.slice(0, actual);
+ }
+
+ return buf;
+ }
+
+ function fromArrayLike(array) {
+ var length = array.length < 0 ? 0 : checked(array.length) | 0;
+ var buf = createBuffer(length);
+
+ for (var i = 0; i < length; i += 1) {
+ buf[i] = array[i] & 255;
+ }
+
+ return buf;
+ }
+
+ function fromArrayView(arrayView) {
+ if (isInstance(arrayView, Uint8Array)) {
+ var copy = new Uint8Array(arrayView);
+ return fromArrayBuffer(copy.buffer, copy.byteOffset, copy.byteLength);
+ }
+
+ return fromArrayLike(arrayView);
+ }
+
+ function fromArrayBuffer(array, byteOffset, length) {
+ if (byteOffset < 0 || array.byteLength < byteOffset) {
+ throw new RangeError('"offset" is outside of buffer bounds');
+ }
+
+ if (array.byteLength < byteOffset + (length || 0)) {
+ throw new RangeError('"length" is outside of buffer bounds');
+ }
+
+ var buf;
+
+ if (byteOffset === undefined && length === undefined) {
+ buf = new Uint8Array(array);
+ } else if (length === undefined) {
+ buf = new Uint8Array(array, byteOffset);
+ } else {
+ buf = new Uint8Array(array, byteOffset, length);
+ } // Return an augmented `Uint8Array` instance
+
+
+ Object.setPrototypeOf(buf, Buffer.prototype);
+ return buf;
+ }
+
+ function fromObject(obj) {
+ if (Buffer.isBuffer(obj)) {
+ var len = checked(obj.length) | 0;
+ var buf = createBuffer(len);
+
+ if (buf.length === 0) {
+ return buf;
+ }
+
+ obj.copy(buf, 0, 0, len);
+ return buf;
+ }
+
+ if (obj.length !== undefined) {
+ if (typeof obj.length !== 'number' || numberIsNaN(obj.length)) {
+ return createBuffer(0);
+ }
+
+ return fromArrayLike(obj);
+ }
+
+ if (obj.type === 'Buffer' && Array.isArray(obj.data)) {
+ return fromArrayLike(obj.data);
+ }
+ }
+
+ function checked(length) {
+ // Note: cannot use `length < K_MAX_LENGTH` here because that fails when
+ // length is NaN (which is otherwise coerced to zero.)
+ if (length >= K_MAX_LENGTH) {
+ throw new RangeError('Attempt to allocate Buffer larger than maximum ' + 'size: 0x' + K_MAX_LENGTH.toString(16) + ' bytes');
+ }
+
+ return length | 0;
+ }
+
+ function SlowBuffer(length) {
+ if (+length != length) {
+ // eslint-disable-line eqeqeq
+ length = 0;
+ }
+
+ return Buffer.alloc(+length);
+ }
+
+ Buffer.isBuffer = function isBuffer(b) {
+ return b != null && b._isBuffer === true && b !== Buffer.prototype; // so Buffer.isBuffer(Buffer.prototype) will be false
+ };
+
+ Buffer.compare = function compare(a, b) {
+ if (isInstance(a, Uint8Array)) a = Buffer.from(a, a.offset, a.byteLength);
+ if (isInstance(b, Uint8Array)) b = Buffer.from(b, b.offset, b.byteLength);
+
+ if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {
+ throw new TypeError('The "buf1", "buf2" arguments must be one of type Buffer or Uint8Array');
+ }
+
+ if (a === b) return 0;
+ var x = a.length;
+ var y = b.length;
+
+ for (var i = 0, len = Math.min(x, y); i < len; ++i) {
+ if (a[i] !== b[i]) {
+ x = a[i];
+ y = b[i];
+ break;
+ }
+ }
+
+ if (x < y) return -1;
+ if (y < x) return 1;
+ return 0;
+ };
+
+ Buffer.isEncoding = function isEncoding(encoding) {
+ switch (String(encoding).toLowerCase()) {
+ case 'hex':
+ case 'utf8':
+ case 'utf-8':
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ case 'base64':
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return true;
+
+ default:
+ return false;
+ }
+ };
+
+ Buffer.concat = function concat(list, length) {
+ if (!Array.isArray(list)) {
+ throw new TypeError('"list" argument must be an Array of Buffers');
+ }
+
+ if (list.length === 0) {
+ return Buffer.alloc(0);
+ }
+
+ var i;
+
+ if (length === undefined) {
+ length = 0;
+
+ for (i = 0; i < list.length; ++i) {
+ length += list[i].length;
+ }
+ }
+
+ var buffer = Buffer.allocUnsafe(length);
+ var pos = 0;
+
+ for (i = 0; i < list.length; ++i) {
+ var buf = list[i];
+
+ if (isInstance(buf, Uint8Array)) {
+ if (pos + buf.length > buffer.length) {
+ Buffer.from(buf).copy(buffer, pos);
+ } else {
+ Uint8Array.prototype.set.call(buffer, buf, pos);
+ }
+ } else if (!Buffer.isBuffer(buf)) {
+ throw new TypeError('"list" argument must be an Array of Buffers');
+ } else {
+ buf.copy(buffer, pos);
+ }
+
+ pos += buf.length;
+ }
+
+ return buffer;
+ };
+
+ function byteLength(string, encoding) {
+ if (Buffer.isBuffer(string)) {
+ return string.length;
+ }
+
+ if (ArrayBuffer.isView(string) || isInstance(string, ArrayBuffer)) {
+ return string.byteLength;
+ }
+
+ if (typeof string !== 'string') {
+ throw new TypeError('The "string" argument must be one of type string, Buffer, or ArrayBuffer. ' + 'Received type ' + babelHelpers["typeof"](string));
+ }
+
+ var len = string.length;
+ var mustMatch = arguments.length > 2 && arguments[2] === true;
+ if (!mustMatch && len === 0) return 0; // Use a for loop to avoid recursion
+
+ var loweredCase = false;
+
+ for (;;) {
+ switch (encoding) {
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ return len;
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8ToBytes(string).length;
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return len * 2;
+
+ case 'hex':
+ return len >>> 1;
+
+ case 'base64':
+ return base64ToBytes(string).length;
+
+ default:
+ if (loweredCase) {
+ return mustMatch ? -1 : utf8ToBytes(string).length; // assume utf8
+ }
+
+ encoding = ('' + encoding).toLowerCase();
+ loweredCase = true;
+ }
+ }
+ }
+
+ Buffer.byteLength = byteLength;
+
+ function slowToString(encoding, start, end) {
+ var loweredCase = false; // No need to verify that "this.length <= MAX_UINT32" since it's a read-only
+ // property of a typed array.
+ // This behaves neither like String nor Uint8Array in that we set start/end
+ // to their upper/lower bounds if the value passed is out of range.
+ // undefined is handled specially as per ECMA-262 6th Edition,
+ // Section 13.3.3.7 Runtime Semantics: KeyedBindingInitialization.
+
+ if (start === undefined || start < 0) {
+ start = 0;
+ } // Return early if start > this.length. Done here to prevent potential uint32
+ // coercion fail below.
+
+
+ if (start > this.length) {
+ return '';
+ }
+
+ if (end === undefined || end > this.length) {
+ end = this.length;
+ }
+
+ if (end <= 0) {
+ return '';
+ } // Force coercion to uint32. This will also coerce falsey/NaN values to 0.
+
+
+ end >>>= 0;
+ start >>>= 0;
+
+ if (end <= start) {
+ return '';
+ }
+
+ if (!encoding) encoding = 'utf8';
+
+ while (true) {
+ switch (encoding) {
+ case 'hex':
+ return hexSlice(this, start, end);
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Slice(this, start, end);
+
+ case 'ascii':
+ return asciiSlice(this, start, end);
+
+ case 'latin1':
+ case 'binary':
+ return latin1Slice(this, start, end);
+
+ case 'base64':
+ return base64Slice(this, start, end);
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return utf16leSlice(this, start, end);
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding);
+ encoding = (encoding + '').toLowerCase();
+ loweredCase = true;
+ }
+ }
+ } // This property is used by `Buffer.isBuffer` (and the `is-buffer` npm package)
+ // to detect a Buffer instance. It's not possible to use `instanceof Buffer`
+ // reliably in a browserify context because there could be multiple different
+ // copies of the 'buffer' package in use. This method works even for Buffer
+ // instances that were created from another copy of the `buffer` package.
+ // See: https://github.com/feross/buffer/issues/154
+
+
+ Buffer.prototype._isBuffer = true;
+
+ function swap(b, n, m) {
+ var i = b[n];
+ b[n] = b[m];
+ b[m] = i;
+ }
+
+ Buffer.prototype.swap16 = function swap16() {
+ var len = this.length;
+
+ if (len % 2 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 16-bits');
+ }
+
+ for (var i = 0; i < len; i += 2) {
+ swap(this, i, i + 1);
+ }
+
+ return this;
+ };
+
+ Buffer.prototype.swap32 = function swap32() {
+ var len = this.length;
+
+ if (len % 4 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 32-bits');
+ }
+
+ for (var i = 0; i < len; i += 4) {
+ swap(this, i, i + 3);
+ swap(this, i + 1, i + 2);
+ }
+
+ return this;
+ };
+
+ Buffer.prototype.swap64 = function swap64() {
+ var len = this.length;
+
+ if (len % 8 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 64-bits');
+ }
+
+ for (var i = 0; i < len; i += 8) {
+ swap(this, i, i + 7);
+ swap(this, i + 1, i + 6);
+ swap(this, i + 2, i + 5);
+ swap(this, i + 3, i + 4);
+ }
+
+ return this;
+ };
+
+ Buffer.prototype.toString = function toString() {
+ var length = this.length;
+ if (length === 0) return '';
+ if (arguments.length === 0) return utf8Slice(this, 0, length);
+ return slowToString.apply(this, arguments);
+ };
+
+ Buffer.prototype.toLocaleString = Buffer.prototype.toString;
+
+ Buffer.prototype.equals = function equals(b) {
+ if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer');
+ if (this === b) return true;
+ return Buffer.compare(this, b) === 0;
+ };
+
+ Buffer.prototype.inspect = function inspect() {
+ var str = '';
+ var max = exports.INSPECT_MAX_BYTES;
+ str = this.toString('hex', 0, max).replace(/(.{2})/g, '$1 ').trim();
+ if (this.length > max) str += ' ... ';
+ return '';
+ };
+
+ if (customInspectSymbol) {
+ Buffer.prototype[customInspectSymbol] = Buffer.prototype.inspect;
+ }
+
+ Buffer.prototype.compare = function compare(target, start, end, thisStart, thisEnd) {
+ if (isInstance(target, Uint8Array)) {
+ target = Buffer.from(target, target.offset, target.byteLength);
+ }
+
+ if (!Buffer.isBuffer(target)) {
+ throw new TypeError('The "target" argument must be one of type Buffer or Uint8Array. ' + 'Received type ' + babelHelpers["typeof"](target));
+ }
+
+ if (start === undefined) {
+ start = 0;
+ }
+
+ if (end === undefined) {
+ end = target ? target.length : 0;
+ }
+
+ if (thisStart === undefined) {
+ thisStart = 0;
+ }
+
+ if (thisEnd === undefined) {
+ thisEnd = this.length;
+ }
+
+ if (start < 0 || end > target.length || thisStart < 0 || thisEnd > this.length) {
+ throw new RangeError('out of range index');
+ }
+
+ if (thisStart >= thisEnd && start >= end) {
+ return 0;
+ }
+
+ if (thisStart >= thisEnd) {
+ return -1;
+ }
+
+ if (start >= end) {
+ return 1;
+ }
+
+ start >>>= 0;
+ end >>>= 0;
+ thisStart >>>= 0;
+ thisEnd >>>= 0;
+ if (this === target) return 0;
+ var x = thisEnd - thisStart;
+ var y = end - start;
+ var len = Math.min(x, y);
+ var thisCopy = this.slice(thisStart, thisEnd);
+ var targetCopy = target.slice(start, end);
+
+ for (var i = 0; i < len; ++i) {
+ if (thisCopy[i] !== targetCopy[i]) {
+ x = thisCopy[i];
+ y = targetCopy[i];
+ break;
+ }
+ }
+
+ if (x < y) return -1;
+ if (y < x) return 1;
+ return 0;
+ }; // Finds either the first index of `val` in `buffer` at offset >= `byteOffset`,
+ // OR the last index of `val` in `buffer` at offset <= `byteOffset`.
+ //
+ // Arguments:
+ // - buffer - a Buffer to search
+ // - val - a string, Buffer, or number
+ // - byteOffset - an index into `buffer`; will be clamped to an int32
+ // - encoding - an optional encoding, relevant is val is a string
+ // - dir - true for indexOf, false for lastIndexOf
+
+
+ function bidirectionalIndexOf(buffer, val, byteOffset, encoding, dir) {
+ // Empty buffer means no match
+ if (buffer.length === 0) return -1; // Normalize byteOffset
+
+ if (typeof byteOffset === 'string') {
+ encoding = byteOffset;
+ byteOffset = 0;
+ } else if (byteOffset > 0x7fffffff) {
+ byteOffset = 0x7fffffff;
+ } else if (byteOffset < -0x80000000) {
+ byteOffset = -0x80000000;
+ }
+
+ byteOffset = +byteOffset; // Coerce to Number.
+
+ if (numberIsNaN(byteOffset)) {
+ // byteOffset: it it's undefined, null, NaN, "foo", etc, search whole buffer
+ byteOffset = dir ? 0 : buffer.length - 1;
+ } // Normalize byteOffset: negative offsets start from the end of the buffer
+
+
+ if (byteOffset < 0) byteOffset = buffer.length + byteOffset;
+
+ if (byteOffset >= buffer.length) {
+ if (dir) return -1;else byteOffset = buffer.length - 1;
+ } else if (byteOffset < 0) {
+ if (dir) byteOffset = 0;else return -1;
+ } // Normalize val
+
+
+ if (typeof val === 'string') {
+ val = Buffer.from(val, encoding);
+ } // Finally, search either indexOf (if dir is true) or lastIndexOf
+
+
+ if (Buffer.isBuffer(val)) {
+ // Special case: looking for empty string/buffer always fails
+ if (val.length === 0) {
+ return -1;
+ }
+
+ return arrayIndexOf(buffer, val, byteOffset, encoding, dir);
+ } else if (typeof val === 'number') {
+ val = val & 0xFF; // Search for a byte value [0-255]
+
+ if (typeof Uint8Array.prototype.indexOf === 'function') {
+ if (dir) {
+ return Uint8Array.prototype.indexOf.call(buffer, val, byteOffset);
+ } else {
+ return Uint8Array.prototype.lastIndexOf.call(buffer, val, byteOffset);
+ }
+ }
+
+ return arrayIndexOf(buffer, [val], byteOffset, encoding, dir);
+ }
+
+ throw new TypeError('val must be string, number or Buffer');
+ }
+
+ function arrayIndexOf(arr, val, byteOffset, encoding, dir) {
+ var indexSize = 1;
+ var arrLength = arr.length;
+ var valLength = val.length;
+
+ if (encoding !== undefined) {
+ encoding = String(encoding).toLowerCase();
+
+ if (encoding === 'ucs2' || encoding === 'ucs-2' || encoding === 'utf16le' || encoding === 'utf-16le') {
+ if (arr.length < 2 || val.length < 2) {
+ return -1;
+ }
+
+ indexSize = 2;
+ arrLength /= 2;
+ valLength /= 2;
+ byteOffset /= 2;
+ }
+ }
+
+ function read(buf, i) {
+ if (indexSize === 1) {
+ return buf[i];
+ } else {
+ return buf.readUInt16BE(i * indexSize);
+ }
+ }
+
+ var i;
+
+ if (dir) {
+ var foundIndex = -1;
+
+ for (i = byteOffset; i < arrLength; i++) {
+ if (read(arr, i) === read(val, foundIndex === -1 ? 0 : i - foundIndex)) {
+ if (foundIndex === -1) foundIndex = i;
+ if (i - foundIndex + 1 === valLength) return foundIndex * indexSize;
+ } else {
+ if (foundIndex !== -1) i -= i - foundIndex;
+ foundIndex = -1;
+ }
+ }
+ } else {
+ if (byteOffset + valLength > arrLength) byteOffset = arrLength - valLength;
+
+ for (i = byteOffset; i >= 0; i--) {
+ var found = true;
+
+ for (var j = 0; j < valLength; j++) {
+ if (read(arr, i + j) !== read(val, j)) {
+ found = false;
+ break;
+ }
+ }
+
+ if (found) return i;
+ }
+ }
+
+ return -1;
+ }
+
+ Buffer.prototype.includes = function includes(val, byteOffset, encoding) {
+ return this.indexOf(val, byteOffset, encoding) !== -1;
+ };
+
+ Buffer.prototype.indexOf = function indexOf(val, byteOffset, encoding) {
+ return bidirectionalIndexOf(this, val, byteOffset, encoding, true);
+ };
+
+ Buffer.prototype.lastIndexOf = function lastIndexOf(val, byteOffset, encoding) {
+ return bidirectionalIndexOf(this, val, byteOffset, encoding, false);
+ };
+
+ function hexWrite(buf, string, offset, length) {
+ offset = Number(offset) || 0;
+ var remaining = buf.length - offset;
+
+ if (!length) {
+ length = remaining;
+ } else {
+ length = Number(length);
+
+ if (length > remaining) {
+ length = remaining;
+ }
+ }
+
+ var strLen = string.length;
+
+ if (length > strLen / 2) {
+ length = strLen / 2;
+ }
+
+ for (var i = 0; i < length; ++i) {
+ var parsed = parseInt(string.substr(i * 2, 2), 16);
+ if (numberIsNaN(parsed)) return i;
+ buf[offset + i] = parsed;
+ }
+
+ return i;
+ }
+
+ function utf8Write(buf, string, offset, length) {
+ return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length);
+ }
+
+ function asciiWrite(buf, string, offset, length) {
+ return blitBuffer(asciiToBytes(string), buf, offset, length);
+ }
+
+ function base64Write(buf, string, offset, length) {
+ return blitBuffer(base64ToBytes(string), buf, offset, length);
+ }
+
+ function ucs2Write(buf, string, offset, length) {
+ return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length);
+ }
+
+ Buffer.prototype.write = function write(string, offset, length, encoding) {
+ // Buffer#write(string)
+ if (offset === undefined) {
+ encoding = 'utf8';
+ length = this.length;
+ offset = 0; // Buffer#write(string, encoding)
+ } else if (length === undefined && typeof offset === 'string') {
+ encoding = offset;
+ length = this.length;
+ offset = 0; // Buffer#write(string, offset[, length][, encoding])
+ } else if (isFinite(offset)) {
+ offset = offset >>> 0;
+
+ if (isFinite(length)) {
+ length = length >>> 0;
+ if (encoding === undefined) encoding = 'utf8';
+ } else {
+ encoding = length;
+ length = undefined;
+ }
+ } else {
+ throw new Error('Buffer.write(string, encoding, offset[, length]) is no longer supported');
+ }
+
+ var remaining = this.length - offset;
+ if (length === undefined || length > remaining) length = remaining;
+
+ if (string.length > 0 && (length < 0 || offset < 0) || offset > this.length) {
+ throw new RangeError('Attempt to write outside buffer bounds');
+ }
+
+ if (!encoding) encoding = 'utf8';
+ var loweredCase = false;
+
+ for (;;) {
+ switch (encoding) {
+ case 'hex':
+ return hexWrite(this, string, offset, length);
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Write(this, string, offset, length);
+
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ return asciiWrite(this, string, offset, length);
+
+ case 'base64':
+ // Warning: maxLength not taken into account in base64Write
+ return base64Write(this, string, offset, length);
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return ucs2Write(this, string, offset, length);
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding);
+ encoding = ('' + encoding).toLowerCase();
+ loweredCase = true;
+ }
+ }
+ };
+
+ Buffer.prototype.toJSON = function toJSON() {
+ return {
+ type: 'Buffer',
+ data: Array.prototype.slice.call(this._arr || this, 0)
+ };
+ };
+
+ function base64Slice(buf, start, end) {
+ if (start === 0 && end === buf.length) {
+ return base64Js.fromByteArray(buf);
+ } else {
+ return base64Js.fromByteArray(buf.slice(start, end));
+ }
+ }
+
+ function utf8Slice(buf, start, end) {
+ end = Math.min(buf.length, end);
+ var res = [];
+ var i = start;
+
+ while (i < end) {
+ var firstByte = buf[i];
+ var codePoint = null;
+ var bytesPerSequence = firstByte > 0xEF ? 4 : firstByte > 0xDF ? 3 : firstByte > 0xBF ? 2 : 1;
+
+ if (i + bytesPerSequence <= end) {
+ var secondByte, thirdByte, fourthByte, tempCodePoint;
+
+ switch (bytesPerSequence) {
+ case 1:
+ if (firstByte < 0x80) {
+ codePoint = firstByte;
+ }
+
+ break;
+
+ case 2:
+ secondByte = buf[i + 1];
+
+ if ((secondByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0x1F) << 0x6 | secondByte & 0x3F;
+
+ if (tempCodePoint > 0x7F) {
+ codePoint = tempCodePoint;
+ }
+ }
+
+ break;
+
+ case 3:
+ secondByte = buf[i + 1];
+ thirdByte = buf[i + 2];
+
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | thirdByte & 0x3F;
+
+ if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {
+ codePoint = tempCodePoint;
+ }
+ }
+
+ break;
+
+ case 4:
+ secondByte = buf[i + 1];
+ thirdByte = buf[i + 2];
+ fourthByte = buf[i + 3];
+
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | fourthByte & 0x3F;
+
+ if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {
+ codePoint = tempCodePoint;
+ }
+ }
+
+ }
+ }
+
+ if (codePoint === null) {
+ // we did not generate a valid codePoint so insert a
+ // replacement char (U+FFFD) and advance only 1 byte
+ codePoint = 0xFFFD;
+ bytesPerSequence = 1;
+ } else if (codePoint > 0xFFFF) {
+ // encode to utf16 (surrogate pair dance)
+ codePoint -= 0x10000;
+ res.push(codePoint >>> 10 & 0x3FF | 0xD800);
+ codePoint = 0xDC00 | codePoint & 0x3FF;
+ }
+
+ res.push(codePoint);
+ i += bytesPerSequence;
+ }
+
+ return decodeCodePointsArray(res);
+ } // Based on http://stackoverflow.com/a/22747272/680742, the browser with
+ // the lowest limit is Chrome, with 0x10000 args.
+ // We go 1 magnitude less, for safety
+
+
+ var MAX_ARGUMENTS_LENGTH = 0x1000;
+
+ function decodeCodePointsArray(codePoints) {
+ var len = codePoints.length;
+
+ if (len <= MAX_ARGUMENTS_LENGTH) {
+ return String.fromCharCode.apply(String, codePoints); // avoid extra slice()
+ } // Decode in chunks to avoid "call stack size exceeded".
+
+
+ var res = '';
+ var i = 0;
+
+ while (i < len) {
+ res += String.fromCharCode.apply(String, codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH));
+ }
+
+ return res;
+ }
+
+ function asciiSlice(buf, start, end) {
+ var ret = '';
+ end = Math.min(buf.length, end);
+
+ for (var i = start; i < end; ++i) {
+ ret += String.fromCharCode(buf[i] & 0x7F);
+ }
+
+ return ret;
+ }
+
+ function latin1Slice(buf, start, end) {
+ var ret = '';
+ end = Math.min(buf.length, end);
+
+ for (var i = start; i < end; ++i) {
+ ret += String.fromCharCode(buf[i]);
+ }
+
+ return ret;
+ }
+
+ function hexSlice(buf, start, end) {
+ var len = buf.length;
+ if (!start || start < 0) start = 0;
+ if (!end || end < 0 || end > len) end = len;
+ var out = '';
+
+ for (var i = start; i < end; ++i) {
+ out += hexSliceLookupTable[buf[i]];
+ }
+
+ return out;
+ }
+
+ function utf16leSlice(buf, start, end) {
+ var bytes = buf.slice(start, end);
+ var res = ''; // If bytes.length is odd, the last 8 bits must be ignored (same as node.js)
+
+ for (var i = 0; i < bytes.length - 1; i += 2) {
+ res += String.fromCharCode(bytes[i] + bytes[i + 1] * 256);
+ }
+
+ return res;
+ }
+
+ Buffer.prototype.slice = function slice(start, end) {
+ var len = this.length;
+ start = ~~start;
+ end = end === undefined ? len : ~~end;
+
+ if (start < 0) {
+ start += len;
+ if (start < 0) start = 0;
+ } else if (start > len) {
+ start = len;
+ }
+
+ if (end < 0) {
+ end += len;
+ if (end < 0) end = 0;
+ } else if (end > len) {
+ end = len;
+ }
+
+ if (end < start) end = start;
+ var newBuf = this.subarray(start, end); // Return an augmented `Uint8Array` instance
+
+ Object.setPrototypeOf(newBuf, Buffer.prototype);
+ return newBuf;
+ };
+ /*
+ * Need to make sure that buffer isn't trying to write out of bounds.
+ */
+
+
+ function checkOffset(offset, ext, length) {
+ if (offset % 1 !== 0 || offset < 0) throw new RangeError('offset is not uint');
+ if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length');
+ }
+
+ Buffer.prototype.readUintLE = Buffer.prototype.readUIntLE = function readUIntLE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+ if (!noAssert) checkOffset(offset, byteLength, this.length);
+ var val = this[offset];
+ var mul = 1;
+ var i = 0;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul;
+ }
+
+ return val;
+ };
+
+ Buffer.prototype.readUintBE = Buffer.prototype.readUIntBE = function readUIntBE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+
+ if (!noAssert) {
+ checkOffset(offset, byteLength, this.length);
+ }
+
+ var val = this[offset + --byteLength];
+ var mul = 1;
+
+ while (byteLength > 0 && (mul *= 0x100)) {
+ val += this[offset + --byteLength] * mul;
+ }
+
+ return val;
+ };
+
+ Buffer.prototype.readUint8 = Buffer.prototype.readUInt8 = function readUInt8(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 1, this.length);
+ return this[offset];
+ };
+
+ Buffer.prototype.readUint16LE = Buffer.prototype.readUInt16LE = function readUInt16LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ return this[offset] | this[offset + 1] << 8;
+ };
+
+ Buffer.prototype.readUint16BE = Buffer.prototype.readUInt16BE = function readUInt16BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ return this[offset] << 8 | this[offset + 1];
+ };
+
+ Buffer.prototype.readUint32LE = Buffer.prototype.readUInt32LE = function readUInt32LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return (this[offset] | this[offset + 1] << 8 | this[offset + 2] << 16) + this[offset + 3] * 0x1000000;
+ };
+
+ Buffer.prototype.readUint32BE = Buffer.prototype.readUInt32BE = function readUInt32BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return this[offset] * 0x1000000 + (this[offset + 1] << 16 | this[offset + 2] << 8 | this[offset + 3]);
+ };
+
+ Buffer.prototype.readIntLE = function readIntLE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+ if (!noAssert) checkOffset(offset, byteLength, this.length);
+ var val = this[offset];
+ var mul = 1;
+ var i = 0;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul;
+ }
+
+ mul *= 0x80;
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength);
+ return val;
+ };
+
+ Buffer.prototype.readIntBE = function readIntBE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+ if (!noAssert) checkOffset(offset, byteLength, this.length);
+ var i = byteLength;
+ var mul = 1;
+ var val = this[offset + --i];
+
+ while (i > 0 && (mul *= 0x100)) {
+ val += this[offset + --i] * mul;
+ }
+
+ mul *= 0x80;
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength);
+ return val;
+ };
+
+ Buffer.prototype.readInt8 = function readInt8(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 1, this.length);
+ if (!(this[offset] & 0x80)) return this[offset];
+ return (0xff - this[offset] + 1) * -1;
+ };
+
+ Buffer.prototype.readInt16LE = function readInt16LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ var val = this[offset] | this[offset + 1] << 8;
+ return val & 0x8000 ? val | 0xFFFF0000 : val;
+ };
+
+ Buffer.prototype.readInt16BE = function readInt16BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ var val = this[offset + 1] | this[offset] << 8;
+ return val & 0x8000 ? val | 0xFFFF0000 : val;
+ };
+
+ Buffer.prototype.readInt32LE = function readInt32LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return this[offset] | this[offset + 1] << 8 | this[offset + 2] << 16 | this[offset + 3] << 24;
+ };
+
+ Buffer.prototype.readInt32BE = function readInt32BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return this[offset] << 24 | this[offset + 1] << 16 | this[offset + 2] << 8 | this[offset + 3];
+ };
+
+ Buffer.prototype.readFloatLE = function readFloatLE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return ieee754.read(this, offset, true, 23, 4);
+ };
+
+ Buffer.prototype.readFloatBE = function readFloatBE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return ieee754.read(this, offset, false, 23, 4);
+ };
+
+ Buffer.prototype.readDoubleLE = function readDoubleLE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 8, this.length);
+ return ieee754.read(this, offset, true, 52, 8);
+ };
+
+ Buffer.prototype.readDoubleBE = function readDoubleBE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 8, this.length);
+ return ieee754.read(this, offset, false, 52, 8);
+ };
+
+ function checkInt(buf, value, offset, ext, max, min) {
+ if (!Buffer.isBuffer(buf)) throw new TypeError('"buffer" argument must be a Buffer instance');
+ if (value > max || value < min) throw new RangeError('"value" argument is out of bounds');
+ if (offset + ext > buf.length) throw new RangeError('Index out of range');
+ }
+
+ Buffer.prototype.writeUintLE = Buffer.prototype.writeUIntLE = function writeUIntLE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+
+ if (!noAssert) {
+ var maxBytes = Math.pow(2, 8 * byteLength) - 1;
+ checkInt(this, value, offset, byteLength, maxBytes, 0);
+ }
+
+ var mul = 1;
+ var i = 0;
+ this[offset] = value & 0xFF;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ this[offset + i] = value / mul & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeUintBE = Buffer.prototype.writeUIntBE = function writeUIntBE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+
+ if (!noAssert) {
+ var maxBytes = Math.pow(2, 8 * byteLength) - 1;
+ checkInt(this, value, offset, byteLength, maxBytes, 0);
+ }
+
+ var i = byteLength - 1;
+ var mul = 1;
+ this[offset + i] = value & 0xFF;
+
+ while (--i >= 0 && (mul *= 0x100)) {
+ this[offset + i] = value / mul & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeUint8 = Buffer.prototype.writeUInt8 = function writeUInt8(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0);
+ this[offset] = value & 0xff;
+ return offset + 1;
+ };
+
+ Buffer.prototype.writeUint16LE = Buffer.prototype.writeUInt16LE = function writeUInt16LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0);
+ this[offset] = value & 0xff;
+ this[offset + 1] = value >>> 8;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeUint16BE = Buffer.prototype.writeUInt16BE = function writeUInt16BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0);
+ this[offset] = value >>> 8;
+ this[offset + 1] = value & 0xff;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeUint32LE = Buffer.prototype.writeUInt32LE = function writeUInt32LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0);
+ this[offset + 3] = value >>> 24;
+ this[offset + 2] = value >>> 16;
+ this[offset + 1] = value >>> 8;
+ this[offset] = value & 0xff;
+ return offset + 4;
+ };
+
+ Buffer.prototype.writeUint32BE = Buffer.prototype.writeUInt32BE = function writeUInt32BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0);
+ this[offset] = value >>> 24;
+ this[offset + 1] = value >>> 16;
+ this[offset + 2] = value >>> 8;
+ this[offset + 3] = value & 0xff;
+ return offset + 4;
+ };
+
+ Buffer.prototype.writeIntLE = function writeIntLE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ var limit = Math.pow(2, 8 * byteLength - 1);
+ checkInt(this, value, offset, byteLength, limit - 1, -limit);
+ }
+
+ var i = 0;
+ var mul = 1;
+ var sub = 0;
+ this[offset] = value & 0xFF;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ if (value < 0 && sub === 0 && this[offset + i - 1] !== 0) {
+ sub = 1;
+ }
+
+ this[offset + i] = (value / mul >> 0) - sub & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeIntBE = function writeIntBE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ var limit = Math.pow(2, 8 * byteLength - 1);
+ checkInt(this, value, offset, byteLength, limit - 1, -limit);
+ }
+
+ var i = byteLength - 1;
+ var mul = 1;
+ var sub = 0;
+ this[offset + i] = value & 0xFF;
+
+ while (--i >= 0 && (mul *= 0x100)) {
+ if (value < 0 && sub === 0 && this[offset + i + 1] !== 0) {
+ sub = 1;
+ }
+
+ this[offset + i] = (value / mul >> 0) - sub & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeInt8 = function writeInt8(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80);
+ if (value < 0) value = 0xff + value + 1;
+ this[offset] = value & 0xff;
+ return offset + 1;
+ };
+
+ Buffer.prototype.writeInt16LE = function writeInt16LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000);
+ this[offset] = value & 0xff;
+ this[offset + 1] = value >>> 8;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeInt16BE = function writeInt16BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000);
+ this[offset] = value >>> 8;
+ this[offset + 1] = value & 0xff;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeInt32LE = function writeInt32LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000);
+ this[offset] = value & 0xff;
+ this[offset + 1] = value >>> 8;
+ this[offset + 2] = value >>> 16;
+ this[offset + 3] = value >>> 24;
+ return offset + 4;
+ };
+
+ Buffer.prototype.writeInt32BE = function writeInt32BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000);
+ if (value < 0) value = 0xffffffff + value + 1;
+ this[offset] = value >>> 24;
+ this[offset + 1] = value >>> 16;
+ this[offset + 2] = value >>> 8;
+ this[offset + 3] = value & 0xff;
+ return offset + 4;
+ };
+
+ function checkIEEE754(buf, value, offset, ext, max, min) {
+ if (offset + ext > buf.length) throw new RangeError('Index out of range');
+ if (offset < 0) throw new RangeError('Index out of range');
+ }
+
+ function writeFloat(buf, value, offset, littleEndian, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 4);
+ }
+
+ ieee754.write(buf, value, offset, littleEndian, 23, 4);
+ return offset + 4;
+ }
+
+ Buffer.prototype.writeFloatLE = function writeFloatLE(value, offset, noAssert) {
+ return writeFloat(this, value, offset, true, noAssert);
+ };
+
+ Buffer.prototype.writeFloatBE = function writeFloatBE(value, offset, noAssert) {
+ return writeFloat(this, value, offset, false, noAssert);
+ };
+
+ function writeDouble(buf, value, offset, littleEndian, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 8);
+ }
+
+ ieee754.write(buf, value, offset, littleEndian, 52, 8);
+ return offset + 8;
+ }
+
+ Buffer.prototype.writeDoubleLE = function writeDoubleLE(value, offset, noAssert) {
+ return writeDouble(this, value, offset, true, noAssert);
+ };
+
+ Buffer.prototype.writeDoubleBE = function writeDoubleBE(value, offset, noAssert) {
+ return writeDouble(this, value, offset, false, noAssert);
+ }; // copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)
+
+
+ Buffer.prototype.copy = function copy(target, targetStart, start, end) {
+ if (!Buffer.isBuffer(target)) throw new TypeError('argument should be a Buffer');
+ if (!start) start = 0;
+ if (!end && end !== 0) end = this.length;
+ if (targetStart >= target.length) targetStart = target.length;
+ if (!targetStart) targetStart = 0;
+ if (end > 0 && end < start) end = start; // Copy 0 bytes; we're done
+
+ if (end === start) return 0;
+ if (target.length === 0 || this.length === 0) return 0; // Fatal error conditions
+
+ if (targetStart < 0) {
+ throw new RangeError('targetStart out of bounds');
+ }
+
+ if (start < 0 || start >= this.length) throw new RangeError('Index out of range');
+ if (end < 0) throw new RangeError('sourceEnd out of bounds'); // Are we oob?
+
+ if (end > this.length) end = this.length;
+
+ if (target.length - targetStart < end - start) {
+ end = target.length - targetStart + start;
+ }
+
+ var len = end - start;
+
+ if (this === target && typeof Uint8Array.prototype.copyWithin === 'function') {
+ // Use built-in when available, missing from IE11
+ this.copyWithin(targetStart, start, end);
+ } else {
+ Uint8Array.prototype.set.call(target, this.subarray(start, end), targetStart);
+ }
+
+ return len;
+ }; // Usage:
+ // buffer.fill(number[, offset[, end]])
+ // buffer.fill(buffer[, offset[, end]])
+ // buffer.fill(string[, offset[, end]][, encoding])
+
+
+ Buffer.prototype.fill = function fill(val, start, end, encoding) {
+ // Handle string cases:
+ if (typeof val === 'string') {
+ if (typeof start === 'string') {
+ encoding = start;
+ start = 0;
+ end = this.length;
+ } else if (typeof end === 'string') {
+ encoding = end;
+ end = this.length;
+ }
+
+ if (encoding !== undefined && typeof encoding !== 'string') {
+ throw new TypeError('encoding must be a string');
+ }
+
+ if (typeof encoding === 'string' && !Buffer.isEncoding(encoding)) {
+ throw new TypeError('Unknown encoding: ' + encoding);
+ }
+
+ if (val.length === 1) {
+ var code = val.charCodeAt(0);
+
+ if (encoding === 'utf8' && code < 128 || encoding === 'latin1') {
+ // Fast path: If `val` fits into a single byte, use that numeric value.
+ val = code;
+ }
+ }
+ } else if (typeof val === 'number') {
+ val = val & 255;
+ } else if (typeof val === 'boolean') {
+ val = Number(val);
+ } // Invalid ranges are not set to a default, so can range check early.
+
+
+ if (start < 0 || this.length < start || this.length < end) {
+ throw new RangeError('Out of range index');
+ }
+
+ if (end <= start) {
+ return this;
+ }
+
+ start = start >>> 0;
+ end = end === undefined ? this.length : end >>> 0;
+ if (!val) val = 0;
+ var i;
+
+ if (typeof val === 'number') {
+ for (i = start; i < end; ++i) {
+ this[i] = val;
+ }
+ } else {
+ var bytes = Buffer.isBuffer(val) ? val : Buffer.from(val, encoding);
+ var len = bytes.length;
+
+ if (len === 0) {
+ throw new TypeError('The value "' + val + '" is invalid for argument "value"');
+ }
+
+ for (i = 0; i < end - start; ++i) {
+ this[i + start] = bytes[i % len];
+ }
+ }
+
+ return this;
+ }; // HELPER FUNCTIONS
+ // ================
+
+
+ var INVALID_BASE64_RE = /[^+/0-9A-Za-z-_]/g;
+
+ function base64clean(str) {
+ // Node takes equal signs as end of the Base64 encoding
+ str = str.split('=')[0]; // Node strips out invalid characters like \n and \t from the string, base64-js does not
+
+ str = str.trim().replace(INVALID_BASE64_RE, ''); // Node converts strings with length < 2 to ''
+
+ if (str.length < 2) return ''; // Node allows for non-padded base64 strings (missing trailing ===), base64-js does not
+
+ while (str.length % 4 !== 0) {
+ str = str + '=';
+ }
+
+ return str;
+ }
+
+ function utf8ToBytes(string, units) {
+ units = units || Infinity;
+ var codePoint;
+ var length = string.length;
+ var leadSurrogate = null;
+ var bytes = [];
+
+ for (var i = 0; i < length; ++i) {
+ codePoint = string.charCodeAt(i); // is surrogate component
+
+ if (codePoint > 0xD7FF && codePoint < 0xE000) {
+ // last char was a lead
+ if (!leadSurrogate) {
+ // no lead yet
+ if (codePoint > 0xDBFF) {
+ // unexpected trail
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ continue;
+ } else if (i + 1 === length) {
+ // unpaired lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ continue;
+ } // valid lead
+
+
+ leadSurrogate = codePoint;
+ continue;
+ } // 2 leads in a row
+
+
+ if (codePoint < 0xDC00) {
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ leadSurrogate = codePoint;
+ continue;
+ } // valid surrogate pair
+
+
+ codePoint = (leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00) + 0x10000;
+ } else if (leadSurrogate) {
+ // valid bmp char, but last char was a lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ }
+
+ leadSurrogate = null; // encode utf8
+
+ if (codePoint < 0x80) {
+ if ((units -= 1) < 0) break;
+ bytes.push(codePoint);
+ } else if (codePoint < 0x800) {
+ if ((units -= 2) < 0) break;
+ bytes.push(codePoint >> 0x6 | 0xC0, codePoint & 0x3F | 0x80);
+ } else if (codePoint < 0x10000) {
+ if ((units -= 3) < 0) break;
+ bytes.push(codePoint >> 0xC | 0xE0, codePoint >> 0x6 & 0x3F | 0x80, codePoint & 0x3F | 0x80);
+ } else if (codePoint < 0x110000) {
+ if ((units -= 4) < 0) break;
+ bytes.push(codePoint >> 0x12 | 0xF0, codePoint >> 0xC & 0x3F | 0x80, codePoint >> 0x6 & 0x3F | 0x80, codePoint & 0x3F | 0x80);
+ } else {
+ throw new Error('Invalid code point');
+ }
+ }
+
+ return bytes;
+ }
+
+ function asciiToBytes(str) {
+ var byteArray = [];
+
+ for (var i = 0; i < str.length; ++i) {
+ // Node's code seems to be doing this and not & 0x7F..
+ byteArray.push(str.charCodeAt(i) & 0xFF);
+ }
+
+ return byteArray;
+ }
+
+ function utf16leToBytes(str, units) {
+ var c, hi, lo;
+ var byteArray = [];
+
+ for (var i = 0; i < str.length; ++i) {
+ if ((units -= 2) < 0) break;
+ c = str.charCodeAt(i);
+ hi = c >> 8;
+ lo = c % 256;
+ byteArray.push(lo);
+ byteArray.push(hi);
+ }
+
+ return byteArray;
+ }
+
+ function base64ToBytes(str) {
+ return base64Js.toByteArray(base64clean(str));
+ }
+
+ function blitBuffer(src, dst, offset, length) {
+ for (var i = 0; i < length; ++i) {
+ if (i + offset >= dst.length || i >= src.length) break;
+ dst[i + offset] = src[i];
+ }
+
+ return i;
+ } // ArrayBuffer or Uint8Array objects from other contexts (i.e. iframes) do not pass
+ // the `instanceof` check but they should be treated as of that type.
+ // See: https://github.com/feross/buffer/issues/166
+
+
+ function isInstance(obj, type) {
+ return obj instanceof type || obj != null && obj.constructor != null && obj.constructor.name != null && obj.constructor.name === type.name;
+ }
+
+ function numberIsNaN(obj) {
+ // For IE11 support
+ return obj !== obj; // eslint-disable-line no-self-compare
+ } // Create lookup table for `toString('hex')`
+ // See: https://github.com/feross/buffer/issues/219
+
+
+ var hexSliceLookupTable = function () {
+ var alphabet = '0123456789abcdef';
+ var table = new Array(256);
+
+ for (var i = 0; i < 16; ++i) {
+ var i16 = i * 16;
+
+ for (var j = 0; j < 16; ++j) {
+ table[i16 + j] = alphabet[i] + alphabet[j];
+ }
+ }
+
+ return table;
+ }();
+ });
+ buffer.Buffer;
+ buffer.SlowBuffer;
+ buffer.INSPECT_MAX_BYTES;
+ buffer.kMaxLength;
+
+ var require$$0 = {};
+
+ // shim for using process in browser
+
+ var performance = global.performance || {};
+
+ performance.now || performance.mozNow || performance.msNow || performance.oNow || performance.webkitNow || function () {
+ return new Date().getTime();
+ }; // generate timestamp or delta
+
+ var inherits;
+
+ if (typeof Object.create === 'function') {
+ inherits = function inherits(ctor, superCtor) {
+ // implementation from standard node.js 'util' module
+ ctor.super_ = superCtor;
+ ctor.prototype = Object.create(superCtor.prototype, {
+ constructor: {
+ value: ctor,
+ enumerable: false,
+ writable: true,
+ configurable: true
+ }
+ });
+ };
+ } else {
+ inherits = function inherits(ctor, superCtor) {
+ ctor.super_ = superCtor;
+
+ var TempCtor = function TempCtor() {};
+
+ TempCtor.prototype = superCtor.prototype;
+ ctor.prototype = new TempCtor();
+ ctor.prototype.constructor = ctor;
+ };
+ }
+
+ var inherits$1 = inherits;
+
+ // Copyright Joyent, Inc. and other Node contributors.
+ var formatRegExp = /%[sdj%]/g;
+ function format(f) {
+ if (!isString(f)) {
+ var objects = [];
+
+ for (var i = 0; i < arguments.length; i++) {
+ objects.push(inspect(arguments[i]));
+ }
+
+ return objects.join(' ');
+ }
+
+ var i = 1;
+ var args = arguments;
+ var len = args.length;
+ var str = String(f).replace(formatRegExp, function (x) {
+ if (x === '%%') return '%';
+ if (i >= len) return x;
+
+ switch (x) {
+ case '%s':
+ return String(args[i++]);
+
+ case '%d':
+ return Number(args[i++]);
+
+ case '%j':
+ try {
+ return JSON.stringify(args[i++]);
+ } catch (_) {
+ return '[Circular]';
+ }
+
+ default:
+ return x;
+ }
+ });
+
+ for (var x = args[i]; i < len; x = args[++i]) {
+ if (isNull(x) || !isObject(x)) {
+ str += ' ' + x;
+ } else {
+ str += ' ' + inspect(x);
+ }
+ }
+
+ return str;
+ }
+ // Returns a modified function which warns once by default.
+ // If --no-deprecation is set, then it is a no-op.
+
+ function deprecate(fn, msg) {
+ // Allow for deprecating things in the process of starting up.
+ if (isUndefined(global.process)) {
+ return function () {
+ return deprecate(fn, msg).apply(this, arguments);
+ };
+ }
+
+ var warned = false;
+
+ function deprecated() {
+ if (!warned) {
+ {
+ console.error(msg);
+ }
+
+ warned = true;
+ }
+
+ return fn.apply(this, arguments);
+ }
+
+ return deprecated;
+ }
+ var debugs = {};
+ var debugEnviron;
+ function debuglog(set) {
+ if (isUndefined(debugEnviron)) debugEnviron = '';
+ set = set.toUpperCase();
+
+ if (!debugs[set]) {
+ if (new RegExp('\\b' + set + '\\b', 'i').test(debugEnviron)) {
+ var pid = 0;
+
+ debugs[set] = function () {
+ var msg = format.apply(null, arguments);
+ console.error('%s %d: %s', set, pid, msg);
+ };
+ } else {
+ debugs[set] = function () {};
+ }
+ }
+
+ return debugs[set];
+ }
+ /**
+ * Echos the value of a value. Trys to print the value out
+ * in the best way possible given the different types.
+ *
+ * @param {Object} obj The object to print out.
+ * @param {Object} opts Optional options object that alters the output.
+ */
+
+ /* legacy: obj, showHidden, depth, colors*/
+
+ function inspect(obj, opts) {
+ // default options
+ var ctx = {
+ seen: [],
+ stylize: stylizeNoColor
+ }; // legacy...
+
+ if (arguments.length >= 3) ctx.depth = arguments[2];
+ if (arguments.length >= 4) ctx.colors = arguments[3];
+
+ if (isBoolean(opts)) {
+ // legacy...
+ ctx.showHidden = opts;
+ } else if (opts) {
+ // got an "options" object
+ _extend(ctx, opts);
+ } // set default options
+
+
+ if (isUndefined(ctx.showHidden)) ctx.showHidden = false;
+ if (isUndefined(ctx.depth)) ctx.depth = 2;
+ if (isUndefined(ctx.colors)) ctx.colors = false;
+ if (isUndefined(ctx.customInspect)) ctx.customInspect = true;
+ if (ctx.colors) ctx.stylize = stylizeWithColor;
+ return formatValue(ctx, obj, ctx.depth);
+ } // http://en.wikipedia.org/wiki/ANSI_escape_code#graphics
+
+ inspect.colors = {
+ 'bold': [1, 22],
+ 'italic': [3, 23],
+ 'underline': [4, 24],
+ 'inverse': [7, 27],
+ 'white': [37, 39],
+ 'grey': [90, 39],
+ 'black': [30, 39],
+ 'blue': [34, 39],
+ 'cyan': [36, 39],
+ 'green': [32, 39],
+ 'magenta': [35, 39],
+ 'red': [31, 39],
+ 'yellow': [33, 39]
+ }; // Don't use 'blue' not visible on cmd.exe
+
+ inspect.styles = {
+ 'special': 'cyan',
+ 'number': 'yellow',
+ 'boolean': 'yellow',
+ 'undefined': 'grey',
+ 'null': 'bold',
+ 'string': 'green',
+ 'date': 'magenta',
+ // "name": intentionally not styling
+ 'regexp': 'red'
+ };
+
+ function stylizeWithColor(str, styleType) {
+ var style = inspect.styles[styleType];
+
+ if (style) {
+ return "\x1B[" + inspect.colors[style][0] + 'm' + str + "\x1B[" + inspect.colors[style][1] + 'm';
+ } else {
+ return str;
+ }
+ }
+
+ function stylizeNoColor(str, styleType) {
+ return str;
+ }
+
+ function arrayToHash(array) {
+ var hash = {};
+ array.forEach(function (val, idx) {
+ hash[val] = true;
+ });
+ return hash;
+ }
+
+ function formatValue(ctx, value, recurseTimes) {
+ // Provide a hook for user-specified inspect functions.
+ // Check that value is an object with an inspect function on it
+ if (ctx.customInspect && value && isFunction(value.inspect) && // Filter out the util module, it's inspect function is special
+ value.inspect !== inspect && // Also filter out any prototype objects using the circular check.
+ !(value.constructor && value.constructor.prototype === value)) {
+ var ret = value.inspect(recurseTimes, ctx);
+
+ if (!isString(ret)) {
+ ret = formatValue(ctx, ret, recurseTimes);
+ }
+
+ return ret;
+ } // Primitive types cannot have properties
+
+
+ var primitive = formatPrimitive(ctx, value);
+
+ if (primitive) {
+ return primitive;
+ } // Look up the keys of the object.
+
+
+ var keys = Object.keys(value);
+ var visibleKeys = arrayToHash(keys);
+
+ if (ctx.showHidden) {
+ keys = Object.getOwnPropertyNames(value);
+ } // IE doesn't make error fields non-enumerable
+ // http://msdn.microsoft.com/en-us/library/ie/dww52sbt(v=vs.94).aspx
+
+
+ if (isError(value) && (keys.indexOf('message') >= 0 || keys.indexOf('description') >= 0)) {
+ return formatError(value);
+ } // Some type of object without properties can be shortcutted.
+
+
+ if (keys.length === 0) {
+ if (isFunction(value)) {
+ var name = value.name ? ': ' + value.name : '';
+ return ctx.stylize('[Function' + name + ']', 'special');
+ }
+
+ if (isRegExp(value)) {
+ return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
+ }
+
+ if (isDate(value)) {
+ return ctx.stylize(Date.prototype.toString.call(value), 'date');
+ }
+
+ if (isError(value)) {
+ return formatError(value);
+ }
+ }
+
+ var base = '',
+ array = false,
+ braces = ['{', '}']; // Make Array say that they are Array
+
+ if (isArray(value)) {
+ array = true;
+ braces = ['[', ']'];
+ } // Make functions say that they are functions
+
+
+ if (isFunction(value)) {
+ var n = value.name ? ': ' + value.name : '';
+ base = ' [Function' + n + ']';
+ } // Make RegExps say that they are RegExps
+
+
+ if (isRegExp(value)) {
+ base = ' ' + RegExp.prototype.toString.call(value);
+ } // Make dates with properties first say the date
+
+
+ if (isDate(value)) {
+ base = ' ' + Date.prototype.toUTCString.call(value);
+ } // Make error with message first say the error
+
+
+ if (isError(value)) {
+ base = ' ' + formatError(value);
+ }
+
+ if (keys.length === 0 && (!array || value.length == 0)) {
+ return braces[0] + base + braces[1];
+ }
+
+ if (recurseTimes < 0) {
+ if (isRegExp(value)) {
+ return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
+ } else {
+ return ctx.stylize('[Object]', 'special');
+ }
+ }
+
+ ctx.seen.push(value);
+ var output;
+
+ if (array) {
+ output = formatArray(ctx, value, recurseTimes, visibleKeys, keys);
+ } else {
+ output = keys.map(function (key) {
+ return formatProperty(ctx, value, recurseTimes, visibleKeys, key, array);
+ });
+ }
+
+ ctx.seen.pop();
+ return reduceToSingleString(output, base, braces);
+ }
+
+ function formatPrimitive(ctx, value) {
+ if (isUndefined(value)) return ctx.stylize('undefined', 'undefined');
+
+ if (isString(value)) {
+ var simple = '\'' + JSON.stringify(value).replace(/^"|"$/g, '').replace(/'/g, "\\'").replace(/\\"/g, '"') + '\'';
+ return ctx.stylize(simple, 'string');
+ }
+
+ if (isNumber(value)) return ctx.stylize('' + value, 'number');
+ if (isBoolean(value)) return ctx.stylize('' + value, 'boolean'); // For some reason typeof null is "object", so special case here.
+
+ if (isNull(value)) return ctx.stylize('null', 'null');
+ }
+
+ function formatError(value) {
+ return '[' + Error.prototype.toString.call(value) + ']';
+ }
+
+ function formatArray(ctx, value, recurseTimes, visibleKeys, keys) {
+ var output = [];
+
+ for (var i = 0, l = value.length; i < l; ++i) {
+ if (hasOwnProperty(value, String(i))) {
+ output.push(formatProperty(ctx, value, recurseTimes, visibleKeys, String(i), true));
+ } else {
+ output.push('');
+ }
+ }
+
+ keys.forEach(function (key) {
+ if (!key.match(/^\d+$/)) {
+ output.push(formatProperty(ctx, value, recurseTimes, visibleKeys, key, true));
+ }
+ });
+ return output;
+ }
+
+ function formatProperty(ctx, value, recurseTimes, visibleKeys, key, array) {
+ var name, str, desc;
+ desc = Object.getOwnPropertyDescriptor(value, key) || {
+ value: value[key]
+ };
+
+ if (desc.get) {
+ if (desc.set) {
+ str = ctx.stylize('[Getter/Setter]', 'special');
+ } else {
+ str = ctx.stylize('[Getter]', 'special');
+ }
+ } else {
+ if (desc.set) {
+ str = ctx.stylize('[Setter]', 'special');
+ }
+ }
+
+ if (!hasOwnProperty(visibleKeys, key)) {
+ name = '[' + key + ']';
+ }
+
+ if (!str) {
+ if (ctx.seen.indexOf(desc.value) < 0) {
+ if (isNull(recurseTimes)) {
+ str = formatValue(ctx, desc.value, null);
+ } else {
+ str = formatValue(ctx, desc.value, recurseTimes - 1);
+ }
+
+ if (str.indexOf('\n') > -1) {
+ if (array) {
+ str = str.split('\n').map(function (line) {
+ return ' ' + line;
+ }).join('\n').substr(2);
+ } else {
+ str = '\n' + str.split('\n').map(function (line) {
+ return ' ' + line;
+ }).join('\n');
+ }
+ }
+ } else {
+ str = ctx.stylize('[Circular]', 'special');
+ }
+ }
+
+ if (isUndefined(name)) {
+ if (array && key.match(/^\d+$/)) {
+ return str;
+ }
+
+ name = JSON.stringify('' + key);
+
+ if (name.match(/^"([a-zA-Z_][a-zA-Z_0-9]*)"$/)) {
+ name = name.substr(1, name.length - 2);
+ name = ctx.stylize(name, 'name');
+ } else {
+ name = name.replace(/'/g, "\\'").replace(/\\"/g, '"').replace(/(^"|"$)/g, "'");
+ name = ctx.stylize(name, 'string');
+ }
+ }
+
+ return name + ': ' + str;
+ }
+
+ function reduceToSingleString(output, base, braces) {
+ var length = output.reduce(function (prev, cur) {
+ if (cur.indexOf('\n') >= 0) ;
+ return prev + cur.replace(/\u001b\[\d\d?m/g, '').length + 1;
+ }, 0);
+
+ if (length > 60) {
+ return braces[0] + (base === '' ? '' : base + '\n ') + ' ' + output.join(',\n ') + ' ' + braces[1];
+ }
+
+ return braces[0] + base + ' ' + output.join(', ') + ' ' + braces[1];
+ } // NOTE: These type checking functions intentionally don't use `instanceof`
+ // because it is fragile and can be easily faked with `Object.create()`.
+
+
+ function isArray(ar) {
+ return Array.isArray(ar);
+ }
+ function isBoolean(arg) {
+ return typeof arg === 'boolean';
+ }
+ function isNull(arg) {
+ return arg === null;
+ }
+ function isNullOrUndefined(arg) {
+ return arg == null;
+ }
+ function isNumber(arg) {
+ return typeof arg === 'number';
+ }
+ function isString(arg) {
+ return typeof arg === 'string';
+ }
+ function isSymbol(arg) {
+ return babelHelpers["typeof"](arg) === 'symbol';
+ }
+ function isUndefined(arg) {
+ return arg === void 0;
+ }
+ function isRegExp(re) {
+ return isObject(re) && objectToString(re) === '[object RegExp]';
+ }
+ function isObject(arg) {
+ return babelHelpers["typeof"](arg) === 'object' && arg !== null;
+ }
+ function isDate(d) {
+ return isObject(d) && objectToString(d) === '[object Date]';
+ }
+ function isError(e) {
+ return isObject(e) && (objectToString(e) === '[object Error]' || e instanceof Error);
+ }
+ function isFunction(arg) {
+ return typeof arg === 'function';
+ }
+ function isPrimitive(arg) {
+ return arg === null || typeof arg === 'boolean' || typeof arg === 'number' || typeof arg === 'string' || babelHelpers["typeof"](arg) === 'symbol' || // ES6 symbol
+ typeof arg === 'undefined';
+ }
+ function isBuffer(maybeBuf) {
+ return Buffer.isBuffer(maybeBuf);
+ }
+
+ function objectToString(o) {
+ return Object.prototype.toString.call(o);
+ }
+
+ function pad(n) {
+ return n < 10 ? '0' + n.toString(10) : n.toString(10);
+ }
+
+ var months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']; // 26 Feb 16:19:34
+
+ function timestamp$1() {
+ var d = new Date();
+ var time = [pad(d.getHours()), pad(d.getMinutes()), pad(d.getSeconds())].join(':');
+ return [d.getDate(), months[d.getMonth()], time].join(' ');
+ } // log is just a thin wrapper to console.log that prepends a timestamp
+
+
+ function log() {
+ console.log('%s - %s', timestamp$1(), format.apply(null, arguments));
+ }
+ function _extend(origin, add) {
+ // Don't do anything if add isn't an object
+ if (!add || !isObject(add)) return origin;
+ var keys = Object.keys(add);
+ var i = keys.length;
+
+ while (i--) {
+ origin[keys[i]] = add[keys[i]];
+ }
+
+ return origin;
+ }
+
+ function hasOwnProperty(obj, prop) {
+ return Object.prototype.hasOwnProperty.call(obj, prop);
+ }
+
+ var require$$1 = {
+ inherits: inherits$1,
+ _extend: _extend,
+ log: log,
+ isBuffer: isBuffer,
+ isPrimitive: isPrimitive,
+ isFunction: isFunction,
+ isError: isError,
+ isDate: isDate,
+ isObject: isObject,
+ isRegExp: isRegExp,
+ isUndefined: isUndefined,
+ isSymbol: isSymbol,
+ isString: isString,
+ isNumber: isNumber,
+ isNullOrUndefined: isNullOrUndefined,
+ isNull: isNull,
+ isBoolean: isBoolean,
+ isArray: isArray,
+ inspect: inspect,
+ deprecate: deprecate,
+ format: format,
+ debuglog: debuglog
+ };
+
+ var utils = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.deprecate = exports.isObjectLike = exports.isDate = exports.haveBuffer = exports.isMap = exports.isRegExp = exports.isBigUInt64Array = exports.isBigInt64Array = exports.isUint8Array = exports.isAnyArrayBuffer = exports.randomBytes = exports.normalizedFunctionString = void 0;
+
+ /**
+ * Normalizes our expected stringified form of a function across versions of node
+ * @param fn - The function to stringify
+ */
+ function normalizedFunctionString(fn) {
+ return fn.toString().replace('function(', 'function (');
+ }
+ exports.normalizedFunctionString = normalizedFunctionString;
+ var isReactNative = typeof commonjsGlobal.navigator === 'object' && commonjsGlobal.navigator.product === 'ReactNative';
+ var insecureWarning = isReactNative
+ ? 'BSON: For React Native please polyfill crypto.getRandomValues, e.g. using: https://www.npmjs.com/package/react-native-get-random-values.'
+ : 'BSON: No cryptographic implementation for random bytes present, falling back to a less secure implementation.';
+ var insecureRandomBytes = function insecureRandomBytes(size) {
+ console.warn(insecureWarning);
+ var result = buffer.Buffer.alloc(size);
+ for (var i = 0; i < size; ++i)
+ result[i] = Math.floor(Math.random() * 256);
+ return result;
+ };
+ var detectRandomBytes = function () {
+ if (typeof window !== 'undefined') {
+ // browser crypto implementation(s)
+ var target_1 = window.crypto || window.msCrypto; // allow for IE11
+ if (target_1 && target_1.getRandomValues) {
+ return function (size) { return target_1.getRandomValues(buffer.Buffer.alloc(size)); };
+ }
+ }
+ if (typeof commonjsGlobal !== 'undefined' && commonjsGlobal.crypto && commonjsGlobal.crypto.getRandomValues) {
+ // allow for RN packages such as https://www.npmjs.com/package/react-native-get-random-values to populate global
+ return function (size) { return commonjsGlobal.crypto.getRandomValues(buffer.Buffer.alloc(size)); };
+ }
+ var requiredRandomBytes;
+ try {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ requiredRandomBytes = require$$0.randomBytes;
+ }
+ catch (e) {
+ // keep the fallback
+ }
+ // NOTE: in transpiled cases the above require might return null/undefined
+ return requiredRandomBytes || insecureRandomBytes;
+ };
+ exports.randomBytes = detectRandomBytes();
+ function isAnyArrayBuffer(value) {
+ return ['[object ArrayBuffer]', '[object SharedArrayBuffer]'].includes(Object.prototype.toString.call(value));
+ }
+ exports.isAnyArrayBuffer = isAnyArrayBuffer;
+ function isUint8Array(value) {
+ return Object.prototype.toString.call(value) === '[object Uint8Array]';
+ }
+ exports.isUint8Array = isUint8Array;
+ function isBigInt64Array(value) {
+ return Object.prototype.toString.call(value) === '[object BigInt64Array]';
+ }
+ exports.isBigInt64Array = isBigInt64Array;
+ function isBigUInt64Array(value) {
+ return Object.prototype.toString.call(value) === '[object BigUint64Array]';
+ }
+ exports.isBigUInt64Array = isBigUInt64Array;
+ function isRegExp(d) {
+ return Object.prototype.toString.call(d) === '[object RegExp]';
+ }
+ exports.isRegExp = isRegExp;
+ function isMap(d) {
+ return Object.prototype.toString.call(d) === '[object Map]';
+ }
+ exports.isMap = isMap;
+ /** Call to check if your environment has `Buffer` */
+ function haveBuffer() {
+ return typeof commonjsGlobal !== 'undefined' && typeof commonjsGlobal.Buffer !== 'undefined';
+ }
+ exports.haveBuffer = haveBuffer;
+ // To ensure that 0.4 of node works correctly
+ function isDate(d) {
+ return isObjectLike(d) && Object.prototype.toString.call(d) === '[object Date]';
+ }
+ exports.isDate = isDate;
+ /**
+ * @internal
+ * this is to solve the `'someKey' in x` problem where x is unknown.
+ * https://github.com/typescript-eslint/typescript-eslint/issues/1071#issuecomment-541955753
+ */
+ function isObjectLike(candidate) {
+ return typeof candidate === 'object' && candidate !== null;
+ }
+ exports.isObjectLike = isObjectLike;
+ function deprecate(fn, message) {
+ if (typeof window === 'undefined' && typeof self === 'undefined') {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ return require$$1.deprecate(fn, message);
+ }
+ var warned = false;
+ function deprecated() {
+ var args = [];
+ for (var _i = 0; _i < arguments.length; _i++) {
+ args[_i] = arguments[_i];
+ }
+ if (!warned) {
+ console.warn(message);
+ warned = true;
+ }
+ return fn.apply(this, args);
+ }
+ return deprecated;
+ }
+ exports.deprecate = deprecate;
+
+ });
+
+ unwrapExports(utils);
+ utils.deprecate;
+ utils.isObjectLike;
+ utils.isDate;
+ utils.haveBuffer;
+ utils.isMap;
+ utils.isRegExp;
+ utils.isBigUInt64Array;
+ utils.isBigInt64Array;
+ utils.isUint8Array;
+ utils.isAnyArrayBuffer;
+ utils.randomBytes;
+ utils.normalizedFunctionString;
+
+ var ensure_buffer = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.ensureBuffer = void 0;
+
+
+ /**
+ * Makes sure that, if a Uint8Array is passed in, it is wrapped in a Buffer.
+ *
+ * @param potentialBuffer - The potential buffer
+ * @returns Buffer the input if potentialBuffer is a buffer, or a buffer that
+ * wraps a passed in Uint8Array
+ * @throws TypeError If anything other than a Buffer or Uint8Array is passed in
+ */
+ function ensureBuffer(potentialBuffer) {
+ if (ArrayBuffer.isView(potentialBuffer)) {
+ return buffer.Buffer.from(potentialBuffer.buffer, potentialBuffer.byteOffset, potentialBuffer.byteLength);
+ }
+ if (utils.isAnyArrayBuffer(potentialBuffer)) {
+ return buffer.Buffer.from(potentialBuffer);
+ }
+ throw new TypeError('Must use either Buffer or TypedArray');
+ }
+ exports.ensureBuffer = ensureBuffer;
+
+ });
+
+ unwrapExports(ensure_buffer);
+ ensure_buffer.ensureBuffer;
+
+ var uuid_utils = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.bufferToUuidHexString = exports.uuidHexStringToBuffer = exports.uuidValidateString = void 0;
+
+ // Validation regex for v4 uuid (validates with or without dashes)
+ var VALIDATION_REGEX = /^(?:[0-9a-f]{8}-[0-9a-f]{4}-4[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}|[0-9a-f]{12}4[0-9a-f]{3}[89ab][0-9a-f]{15})$/i;
+ var uuidValidateString = function (str) {
+ return typeof str === 'string' && VALIDATION_REGEX.test(str);
+ };
+ exports.uuidValidateString = uuidValidateString;
+ var uuidHexStringToBuffer = function (hexString) {
+ if (!exports.uuidValidateString(hexString)) {
+ throw new TypeError('UUID string representations must be a 32 or 36 character hex string (dashes excluded/included). Format: "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx" or "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx".');
+ }
+ var sanitizedHexString = hexString.replace(/-/g, '');
+ return buffer.Buffer.from(sanitizedHexString, 'hex');
+ };
+ exports.uuidHexStringToBuffer = uuidHexStringToBuffer;
+ var bufferToUuidHexString = function (buffer, includeDashes) {
+ if (includeDashes === void 0) { includeDashes = true; }
+ return includeDashes
+ ? buffer.toString('hex', 0, 4) +
+ '-' +
+ buffer.toString('hex', 4, 6) +
+ '-' +
+ buffer.toString('hex', 6, 8) +
+ '-' +
+ buffer.toString('hex', 8, 10) +
+ '-' +
+ buffer.toString('hex', 10, 16)
+ : buffer.toString('hex');
+ };
+ exports.bufferToUuidHexString = bufferToUuidHexString;
+
+ });
+
+ unwrapExports(uuid_utils);
+ uuid_utils.bufferToUuidHexString;
+ uuid_utils.uuidHexStringToBuffer;
+ uuid_utils.uuidValidateString;
+
+ var uuid = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.UUID = void 0;
+
+
+
+
+
+ var BYTE_LENGTH = 16;
+ var kId = Symbol('id');
+ /**
+ * A class representation of the BSON UUID type.
+ * @public
+ */
+ var UUID = /** @class */ (function () {
+ /**
+ * Create an UUID type
+ *
+ * @param input - Can be a 32 or 36 character hex string (dashes excluded/included) or a 16 byte binary Buffer.
+ */
+ function UUID(input) {
+ if (typeof input === 'undefined') {
+ // The most common use case (blank id, new UUID() instance)
+ this.id = UUID.generate();
+ }
+ else if (input instanceof UUID) {
+ this[kId] = buffer.Buffer.from(input.id);
+ this.__id = input.__id;
+ }
+ else if (ArrayBuffer.isView(input) && input.byteLength === BYTE_LENGTH) {
+ this.id = ensure_buffer.ensureBuffer(input);
+ }
+ else if (typeof input === 'string') {
+ this.id = uuid_utils.uuidHexStringToBuffer(input);
+ }
+ else {
+ throw new TypeError('Argument passed in UUID constructor must be a UUID, a 16 byte Buffer or a 32/36 character hex string (dashes excluded/included, format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx).');
+ }
+ }
+ Object.defineProperty(UUID.prototype, "id", {
+ /**
+ * The UUID bytes
+ * @readonly
+ */
+ get: function () {
+ return this[kId];
+ },
+ set: function (value) {
+ this[kId] = value;
+ if (UUID.cacheHexString) {
+ this.__id = uuid_utils.bufferToUuidHexString(value);
+ }
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /**
+ * Generate a 16 byte uuid v4 buffer used in UUIDs
+ */
+ /**
+ * Returns the UUID id as a 32 or 36 character hex string representation, excluding/including dashes (defaults to 36 character dash separated)
+ * @param includeDashes - should the string exclude dash-separators.
+ * */
+ UUID.prototype.toHexString = function (includeDashes) {
+ if (includeDashes === void 0) { includeDashes = true; }
+ if (UUID.cacheHexString && this.__id) {
+ return this.__id;
+ }
+ var uuidHexString = uuid_utils.bufferToUuidHexString(this.id, includeDashes);
+ if (UUID.cacheHexString) {
+ this.__id = uuidHexString;
+ }
+ return uuidHexString;
+ };
+ /**
+ * Converts the id into a 36 character (dashes included) hex string, unless a encoding is specified.
+ * @internal
+ */
+ UUID.prototype.toString = function (encoding) {
+ return encoding ? this.id.toString(encoding) : this.toHexString();
+ };
+ /**
+ * Converts the id into its JSON string representation. A 36 character (dashes included) hex string in the format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx
+ * @internal
+ */
+ UUID.prototype.toJSON = function () {
+ return this.toHexString();
+ };
+ /**
+ * Compares the equality of this UUID with `otherID`.
+ *
+ * @param otherId - UUID instance to compare against.
+ */
+ UUID.prototype.equals = function (otherId) {
+ if (!otherId) {
+ return false;
+ }
+ if (otherId instanceof UUID) {
+ return otherId.id.equals(this.id);
+ }
+ try {
+ return new UUID(otherId).id.equals(this.id);
+ }
+ catch (_a) {
+ return false;
+ }
+ };
+ /**
+ * Creates a Binary instance from the current UUID.
+ */
+ UUID.prototype.toBinary = function () {
+ return new binary.Binary(this.id, binary.Binary.SUBTYPE_UUID);
+ };
+ /**
+ * Generates a populated buffer containing a v4 uuid
+ */
+ UUID.generate = function () {
+ var bytes = utils.randomBytes(BYTE_LENGTH);
+ // Per 4.4, set bits for version and `clock_seq_hi_and_reserved`
+ // Kindly borrowed from https://github.com/uuidjs/uuid/blob/master/src/v4.js
+ bytes[6] = (bytes[6] & 0x0f) | 0x40;
+ bytes[8] = (bytes[8] & 0x3f) | 0x80;
+ return buffer.Buffer.from(bytes);
+ };
+ /**
+ * Checks if a value is a valid bson UUID
+ * @param input - UUID, string or Buffer to validate.
+ */
+ UUID.isValid = function (input) {
+ if (!input) {
+ return false;
+ }
+ if (input instanceof UUID) {
+ return true;
+ }
+ if (typeof input === 'string') {
+ return uuid_utils.uuidValidateString(input);
+ }
+ if (utils.isUint8Array(input)) {
+ // check for length & uuid version (https://tools.ietf.org/html/rfc4122#section-4.1.3)
+ if (input.length !== BYTE_LENGTH) {
+ return false;
+ }
+ try {
+ // get this byte as hex: xxxxxxxx-xxxx-XXxx-xxxx-xxxxxxxxxxxx
+ // check first part as uuid version: xxxxxxxx-xxxx-Xxxx-xxxx-xxxxxxxxxxxx
+ return parseInt(input[6].toString(16)[0], 10) === binary.Binary.SUBTYPE_UUID;
+ }
+ catch (_a) {
+ return false;
+ }
+ }
+ return false;
+ };
+ /**
+ * Creates an UUID from a hex string representation of an UUID.
+ * @param hexString - 32 or 36 character hex string (dashes excluded/included).
+ */
+ UUID.createFromHexString = function (hexString) {
+ var buffer = uuid_utils.uuidHexStringToBuffer(hexString);
+ return new UUID(buffer);
+ };
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 36 character hex string representation.
+ * @internal
+ */
+ UUID.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ UUID.prototype.inspect = function () {
+ return "new UUID(\"" + this.toHexString() + "\")";
+ };
+ return UUID;
+ }());
+ exports.UUID = UUID;
+ Object.defineProperty(UUID.prototype, '_bsontype', { value: 'UUID' });
+
+ });
+
+ unwrapExports(uuid);
+ uuid.UUID;
+
+ var binary = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Binary = void 0;
+
+
+
+
+ /**
+ * A class representation of the BSON Binary type.
+ * @public
+ */
+ var Binary = /** @class */ (function () {
+ /**
+ * @param buffer - a buffer object containing the binary data.
+ * @param subType - the option binary type.
+ */
+ function Binary(buffer$1, subType) {
+ if (!(this instanceof Binary))
+ return new Binary(buffer$1, subType);
+ if (!(buffer$1 == null) &&
+ !(typeof buffer$1 === 'string') &&
+ !ArrayBuffer.isView(buffer$1) &&
+ !(buffer$1 instanceof ArrayBuffer) &&
+ !Array.isArray(buffer$1)) {
+ throw new TypeError('Binary can only be constructed from string, Buffer, TypedArray, or Array');
+ }
+ this.sub_type = subType !== null && subType !== void 0 ? subType : Binary.BSON_BINARY_SUBTYPE_DEFAULT;
+ if (buffer$1 == null) {
+ // create an empty binary buffer
+ this.buffer = buffer.Buffer.alloc(Binary.BUFFER_SIZE);
+ this.position = 0;
+ }
+ else {
+ if (typeof buffer$1 === 'string') {
+ // string
+ this.buffer = buffer.Buffer.from(buffer$1, 'binary');
+ }
+ else if (Array.isArray(buffer$1)) {
+ // number[]
+ this.buffer = buffer.Buffer.from(buffer$1);
+ }
+ else {
+ // Buffer | TypedArray | ArrayBuffer
+ this.buffer = ensure_buffer.ensureBuffer(buffer$1);
+ }
+ this.position = this.buffer.byteLength;
+ }
+ }
+ /**
+ * Updates this binary with byte_value.
+ *
+ * @param byteValue - a single byte we wish to write.
+ */
+ Binary.prototype.put = function (byteValue) {
+ // If it's a string and a has more than one character throw an error
+ if (typeof byteValue === 'string' && byteValue.length !== 1) {
+ throw new TypeError('only accepts single character String');
+ }
+ else if (typeof byteValue !== 'number' && byteValue.length !== 1)
+ throw new TypeError('only accepts single character Uint8Array or Array');
+ // Decode the byte value once
+ var decodedByte;
+ if (typeof byteValue === 'string') {
+ decodedByte = byteValue.charCodeAt(0);
+ }
+ else if (typeof byteValue === 'number') {
+ decodedByte = byteValue;
+ }
+ else {
+ decodedByte = byteValue[0];
+ }
+ if (decodedByte < 0 || decodedByte > 255) {
+ throw new TypeError('only accepts number in a valid unsigned byte range 0-255');
+ }
+ if (this.buffer.length > this.position) {
+ this.buffer[this.position++] = decodedByte;
+ }
+ else {
+ var buffer$1 = buffer.Buffer.alloc(Binary.BUFFER_SIZE + this.buffer.length);
+ // Combine the two buffers together
+ this.buffer.copy(buffer$1, 0, 0, this.buffer.length);
+ this.buffer = buffer$1;
+ this.buffer[this.position++] = decodedByte;
+ }
+ };
+ /**
+ * Writes a buffer or string to the binary.
+ *
+ * @param sequence - a string or buffer to be written to the Binary BSON object.
+ * @param offset - specify the binary of where to write the content.
+ */
+ Binary.prototype.write = function (sequence, offset) {
+ offset = typeof offset === 'number' ? offset : this.position;
+ // If the buffer is to small let's extend the buffer
+ if (this.buffer.length < offset + sequence.length) {
+ var buffer$1 = buffer.Buffer.alloc(this.buffer.length + sequence.length);
+ this.buffer.copy(buffer$1, 0, 0, this.buffer.length);
+ // Assign the new buffer
+ this.buffer = buffer$1;
+ }
+ if (ArrayBuffer.isView(sequence)) {
+ this.buffer.set(ensure_buffer.ensureBuffer(sequence), offset);
+ this.position =
+ offset + sequence.byteLength > this.position ? offset + sequence.length : this.position;
+ }
+ else if (typeof sequence === 'string') {
+ this.buffer.write(sequence, offset, sequence.length, 'binary');
+ this.position =
+ offset + sequence.length > this.position ? offset + sequence.length : this.position;
+ }
+ };
+ /**
+ * Reads **length** bytes starting at **position**.
+ *
+ * @param position - read from the given position in the Binary.
+ * @param length - the number of bytes to read.
+ */
+ Binary.prototype.read = function (position, length) {
+ length = length && length > 0 ? length : this.position;
+ // Let's return the data based on the type we have
+ return this.buffer.slice(position, position + length);
+ };
+ /**
+ * Returns the value of this binary as a string.
+ * @param asRaw - Will skip converting to a string
+ * @remarks
+ * This is handy when calling this function conditionally for some key value pairs and not others
+ */
+ Binary.prototype.value = function (asRaw) {
+ asRaw = !!asRaw;
+ // Optimize to serialize for the situation where the data == size of buffer
+ if (asRaw && this.buffer.length === this.position) {
+ return this.buffer;
+ }
+ // If it's a node.js buffer object
+ if (asRaw) {
+ return this.buffer.slice(0, this.position);
+ }
+ return this.buffer.toString('binary', 0, this.position);
+ };
+ /** the length of the binary sequence */
+ Binary.prototype.length = function () {
+ return this.position;
+ };
+ /** @internal */
+ Binary.prototype.toJSON = function () {
+ return this.buffer.toString('base64');
+ };
+ /** @internal */
+ Binary.prototype.toString = function (format) {
+ return this.buffer.toString(format);
+ };
+ /** @internal */
+ Binary.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ var base64String = this.buffer.toString('base64');
+ var subType = Number(this.sub_type).toString(16);
+ if (options.legacy) {
+ return {
+ $binary: base64String,
+ $type: subType.length === 1 ? '0' + subType : subType
+ };
+ }
+ return {
+ $binary: {
+ base64: base64String,
+ subType: subType.length === 1 ? '0' + subType : subType
+ }
+ };
+ };
+ /** @internal */
+ Binary.prototype.toUUID = function () {
+ if (this.sub_type === Binary.SUBTYPE_UUID) {
+ return new uuid.UUID(this.buffer.slice(0, this.position));
+ }
+ throw new Error("Binary sub_type \"" + this.sub_type + "\" is not supported for converting to UUID. Only \"" + Binary.SUBTYPE_UUID + "\" is currently supported.");
+ };
+ /** @internal */
+ Binary.fromExtendedJSON = function (doc, options) {
+ options = options || {};
+ var data;
+ var type;
+ if ('$binary' in doc) {
+ if (options.legacy && typeof doc.$binary === 'string' && '$type' in doc) {
+ type = doc.$type ? parseInt(doc.$type, 16) : 0;
+ data = buffer.Buffer.from(doc.$binary, 'base64');
+ }
+ else {
+ if (typeof doc.$binary !== 'string') {
+ type = doc.$binary.subType ? parseInt(doc.$binary.subType, 16) : 0;
+ data = buffer.Buffer.from(doc.$binary.base64, 'base64');
+ }
+ }
+ }
+ else if ('$uuid' in doc) {
+ type = 4;
+ data = uuid_utils.uuidHexStringToBuffer(doc.$uuid);
+ }
+ if (!data) {
+ throw new TypeError("Unexpected Binary Extended JSON format " + JSON.stringify(doc));
+ }
+ return new Binary(data, type);
+ };
+ /** @internal */
+ Binary.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Binary.prototype.inspect = function () {
+ var asBuffer = this.value(true);
+ return "new Binary(Buffer.from(\"" + asBuffer.toString('hex') + "\", \"hex\"), " + this.sub_type + ")";
+ };
+ /**
+ * Binary default subtype
+ * @internal
+ */
+ Binary.BSON_BINARY_SUBTYPE_DEFAULT = 0;
+ /** Initial buffer default size */
+ Binary.BUFFER_SIZE = 256;
+ /** Default BSON type */
+ Binary.SUBTYPE_DEFAULT = 0;
+ /** Function BSON type */
+ Binary.SUBTYPE_FUNCTION = 1;
+ /** Byte Array BSON type */
+ Binary.SUBTYPE_BYTE_ARRAY = 2;
+ /** Deprecated UUID BSON type @deprecated Please use SUBTYPE_UUID */
+ Binary.SUBTYPE_UUID_OLD = 3;
+ /** UUID BSON type */
+ Binary.SUBTYPE_UUID = 4;
+ /** MD5 BSON type */
+ Binary.SUBTYPE_MD5 = 5;
+ /** User BSON type */
+ Binary.SUBTYPE_USER_DEFINED = 128;
+ return Binary;
+ }());
+ exports.Binary = Binary;
+ Object.defineProperty(Binary.prototype, '_bsontype', { value: 'Binary' });
+
+ });
+
+ unwrapExports(binary);
+ binary.Binary;
+
+ var code = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Code = void 0;
+ /**
+ * A class representation of the BSON Code type.
+ * @public
+ */
+ var Code = /** @class */ (function () {
+ /**
+ * @param code - a string or function.
+ * @param scope - an optional scope for the function.
+ */
+ function Code(code, scope) {
+ if (!(this instanceof Code))
+ return new Code(code, scope);
+ this.code = code;
+ this.scope = scope;
+ }
+ /** @internal */
+ Code.prototype.toJSON = function () {
+ return { code: this.code, scope: this.scope };
+ };
+ /** @internal */
+ Code.prototype.toExtendedJSON = function () {
+ if (this.scope) {
+ return { $code: this.code, $scope: this.scope };
+ }
+ return { $code: this.code };
+ };
+ /** @internal */
+ Code.fromExtendedJSON = function (doc) {
+ return new Code(doc.$code, doc.$scope);
+ };
+ /** @internal */
+ Code.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Code.prototype.inspect = function () {
+ var codeJson = this.toJSON();
+ return "new Code(\"" + codeJson.code + "\"" + (codeJson.scope ? ", " + JSON.stringify(codeJson.scope) : '') + ")";
+ };
+ return Code;
+ }());
+ exports.Code = Code;
+ Object.defineProperty(Code.prototype, '_bsontype', { value: 'Code' });
+
+ });
+
+ unwrapExports(code);
+ code.Code;
+
+ var db_ref = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.DBRef = exports.isDBRefLike = void 0;
+
+ /** @internal */
+ function isDBRefLike(value) {
+ return (utils.isObjectLike(value) &&
+ value.$id != null &&
+ typeof value.$ref === 'string' &&
+ (value.$db == null || typeof value.$db === 'string'));
+ }
+ exports.isDBRefLike = isDBRefLike;
+ /**
+ * A class representation of the BSON DBRef type.
+ * @public
+ */
+ var DBRef = /** @class */ (function () {
+ /**
+ * @param collection - the collection name.
+ * @param oid - the reference ObjectId.
+ * @param db - optional db name, if omitted the reference is local to the current db.
+ */
+ function DBRef(collection, oid, db, fields) {
+ if (!(this instanceof DBRef))
+ return new DBRef(collection, oid, db, fields);
+ // check if namespace has been provided
+ var parts = collection.split('.');
+ if (parts.length === 2) {
+ db = parts.shift();
+ // eslint-disable-next-line @typescript-eslint/no-non-null-assertion
+ collection = parts.shift();
+ }
+ this.collection = collection;
+ this.oid = oid;
+ this.db = db;
+ this.fields = fields || {};
+ }
+ Object.defineProperty(DBRef.prototype, "namespace", {
+ // Property provided for compatibility with the 1.x parser
+ // the 1.x parser used a "namespace" property, while 4.x uses "collection"
+ /** @internal */
+ get: function () {
+ return this.collection;
+ },
+ set: function (value) {
+ this.collection = value;
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /** @internal */
+ DBRef.prototype.toJSON = function () {
+ var o = Object.assign({
+ $ref: this.collection,
+ $id: this.oid
+ }, this.fields);
+ if (this.db != null)
+ o.$db = this.db;
+ return o;
+ };
+ /** @internal */
+ DBRef.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ var o = {
+ $ref: this.collection,
+ $id: this.oid
+ };
+ if (options.legacy) {
+ return o;
+ }
+ if (this.db)
+ o.$db = this.db;
+ o = Object.assign(o, this.fields);
+ return o;
+ };
+ /** @internal */
+ DBRef.fromExtendedJSON = function (doc) {
+ var copy = Object.assign({}, doc);
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new DBRef(doc.$ref, doc.$id, doc.$db, copy);
+ };
+ /** @internal */
+ DBRef.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ DBRef.prototype.inspect = function () {
+ // NOTE: if OID is an ObjectId class it will just print the oid string.
+ var oid = this.oid === undefined || this.oid.toString === undefined ? this.oid : this.oid.toString();
+ return "new DBRef(\"" + this.namespace + "\", new ObjectId(\"" + oid + "\")" + (this.db ? ", \"" + this.db + "\"" : '') + ")";
+ };
+ return DBRef;
+ }());
+ exports.DBRef = DBRef;
+ Object.defineProperty(DBRef.prototype, '_bsontype', { value: 'DBRef' });
+
+ });
+
+ unwrapExports(db_ref);
+ db_ref.DBRef;
+ db_ref.isDBRefLike;
+
+ var long_1 = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Long = void 0;
+
+ /**
+ * wasm optimizations, to do native i64 multiplication and divide
+ */
+ var wasm = undefined;
+ try {
+ wasm = new WebAssembly.Instance(new WebAssembly.Module(
+ // prettier-ignore
+ new Uint8Array([0, 97, 115, 109, 1, 0, 0, 0, 1, 13, 2, 96, 0, 1, 127, 96, 4, 127, 127, 127, 127, 1, 127, 3, 7, 6, 0, 1, 1, 1, 1, 1, 6, 6, 1, 127, 1, 65, 0, 11, 7, 50, 6, 3, 109, 117, 108, 0, 1, 5, 100, 105, 118, 95, 115, 0, 2, 5, 100, 105, 118, 95, 117, 0, 3, 5, 114, 101, 109, 95, 115, 0, 4, 5, 114, 101, 109, 95, 117, 0, 5, 8, 103, 101, 116, 95, 104, 105, 103, 104, 0, 0, 10, 191, 1, 6, 4, 0, 35, 0, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 126, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 127, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 128, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 129, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 130, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11])), {}).exports;
+ }
+ catch (_a) {
+ // no wasm support
+ }
+ var TWO_PWR_16_DBL = 1 << 16;
+ var TWO_PWR_24_DBL = 1 << 24;
+ var TWO_PWR_32_DBL = TWO_PWR_16_DBL * TWO_PWR_16_DBL;
+ var TWO_PWR_64_DBL = TWO_PWR_32_DBL * TWO_PWR_32_DBL;
+ var TWO_PWR_63_DBL = TWO_PWR_64_DBL / 2;
+ /** A cache of the Long representations of small integer values. */
+ var INT_CACHE = {};
+ /** A cache of the Long representations of small unsigned integer values. */
+ var UINT_CACHE = {};
+ /**
+ * A class representing a 64-bit integer
+ * @public
+ * @remarks
+ * The internal representation of a long is the two given signed, 32-bit values.
+ * We use 32-bit pieces because these are the size of integers on which
+ * Javascript performs bit-operations. For operations like addition and
+ * multiplication, we split each number into 16 bit pieces, which can easily be
+ * multiplied within Javascript's floating-point representation without overflow
+ * or change in sign.
+ * In the algorithms below, we frequently reduce the negative case to the
+ * positive case by negating the input(s) and then post-processing the result.
+ * Note that we must ALWAYS check specially whether those values are MIN_VALUE
+ * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
+ * a positive number, it overflows back into a negative). Not handling this
+ * case would often result in infinite recursion.
+ * Common constant values ZERO, ONE, NEG_ONE, etc. are found as static properties on this class.
+ */
+ var Long = /** @class */ (function () {
+ /**
+ * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers.
+ * See the from* functions below for more convenient ways of constructing Longs.
+ *
+ * Acceptable signatures are:
+ * - Long(low, high, unsigned?)
+ * - Long(bigint, unsigned?)
+ * - Long(string, unsigned?)
+ *
+ * @param low - The low (signed) 32 bits of the long
+ * @param high - The high (signed) 32 bits of the long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ function Long(low, high, unsigned) {
+ if (low === void 0) { low = 0; }
+ if (!(this instanceof Long))
+ return new Long(low, high, unsigned);
+ if (typeof low === 'bigint') {
+ Object.assign(this, Long.fromBigInt(low, !!high));
+ }
+ else if (typeof low === 'string') {
+ Object.assign(this, Long.fromString(low, !!high));
+ }
+ else {
+ this.low = low | 0;
+ this.high = high | 0;
+ this.unsigned = !!unsigned;
+ }
+ Object.defineProperty(this, '__isLong__', {
+ value: true,
+ configurable: false,
+ writable: false,
+ enumerable: false
+ });
+ }
+ /**
+ * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits.
+ * Each is assumed to use 32 bits.
+ * @param lowBits - The low 32 bits
+ * @param highBits - The high 32 bits
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBits = function (lowBits, highBits, unsigned) {
+ return new Long(lowBits, highBits, unsigned);
+ };
+ /**
+ * Returns a Long representing the given 32 bit integer value.
+ * @param value - The 32 bit integer in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromInt = function (value, unsigned) {
+ var obj, cachedObj, cache;
+ if (unsigned) {
+ value >>>= 0;
+ if ((cache = 0 <= value && value < 256)) {
+ cachedObj = UINT_CACHE[value];
+ if (cachedObj)
+ return cachedObj;
+ }
+ obj = Long.fromBits(value, (value | 0) < 0 ? -1 : 0, true);
+ if (cache)
+ UINT_CACHE[value] = obj;
+ return obj;
+ }
+ else {
+ value |= 0;
+ if ((cache = -128 <= value && value < 128)) {
+ cachedObj = INT_CACHE[value];
+ if (cachedObj)
+ return cachedObj;
+ }
+ obj = Long.fromBits(value, value < 0 ? -1 : 0, false);
+ if (cache)
+ INT_CACHE[value] = obj;
+ return obj;
+ }
+ };
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromNumber = function (value, unsigned) {
+ if (isNaN(value))
+ return unsigned ? Long.UZERO : Long.ZERO;
+ if (unsigned) {
+ if (value < 0)
+ return Long.UZERO;
+ if (value >= TWO_PWR_64_DBL)
+ return Long.MAX_UNSIGNED_VALUE;
+ }
+ else {
+ if (value <= -TWO_PWR_63_DBL)
+ return Long.MIN_VALUE;
+ if (value + 1 >= TWO_PWR_63_DBL)
+ return Long.MAX_VALUE;
+ }
+ if (value < 0)
+ return Long.fromNumber(-value, unsigned).neg();
+ return Long.fromBits(value % TWO_PWR_32_DBL | 0, (value / TWO_PWR_32_DBL) | 0, unsigned);
+ };
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBigInt = function (value, unsigned) {
+ return Long.fromString(value.toString(), unsigned);
+ };
+ /**
+ * Returns a Long representation of the given string, written using the specified radix.
+ * @param str - The textual representation of the Long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param radix - The radix in which the text is written (2-36), defaults to 10
+ * @returns The corresponding Long value
+ */
+ Long.fromString = function (str, unsigned, radix) {
+ if (str.length === 0)
+ throw Error('empty string');
+ if (str === 'NaN' || str === 'Infinity' || str === '+Infinity' || str === '-Infinity')
+ return Long.ZERO;
+ if (typeof unsigned === 'number') {
+ // For goog.math.long compatibility
+ (radix = unsigned), (unsigned = false);
+ }
+ else {
+ unsigned = !!unsigned;
+ }
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix)
+ throw RangeError('radix');
+ var p;
+ if ((p = str.indexOf('-')) > 0)
+ throw Error('interior hyphen');
+ else if (p === 0) {
+ return Long.fromString(str.substring(1), unsigned, radix).neg();
+ }
+ // Do several (8) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ var radixToPower = Long.fromNumber(Math.pow(radix, 8));
+ var result = Long.ZERO;
+ for (var i = 0; i < str.length; i += 8) {
+ var size = Math.min(8, str.length - i), value = parseInt(str.substring(i, i + size), radix);
+ if (size < 8) {
+ var power = Long.fromNumber(Math.pow(radix, size));
+ result = result.mul(power).add(Long.fromNumber(value));
+ }
+ else {
+ result = result.mul(radixToPower);
+ result = result.add(Long.fromNumber(value));
+ }
+ }
+ result.unsigned = unsigned;
+ return result;
+ };
+ /**
+ * Creates a Long from its byte representation.
+ * @param bytes - Byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns The corresponding Long value
+ */
+ Long.fromBytes = function (bytes, unsigned, le) {
+ return le ? Long.fromBytesLE(bytes, unsigned) : Long.fromBytesBE(bytes, unsigned);
+ };
+ /**
+ * Creates a Long from its little endian byte representation.
+ * @param bytes - Little endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBytesLE = function (bytes, unsigned) {
+ return new Long(bytes[0] | (bytes[1] << 8) | (bytes[2] << 16) | (bytes[3] << 24), bytes[4] | (bytes[5] << 8) | (bytes[6] << 16) | (bytes[7] << 24), unsigned);
+ };
+ /**
+ * Creates a Long from its big endian byte representation.
+ * @param bytes - Big endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBytesBE = function (bytes, unsigned) {
+ return new Long((bytes[4] << 24) | (bytes[5] << 16) | (bytes[6] << 8) | bytes[7], (bytes[0] << 24) | (bytes[1] << 16) | (bytes[2] << 8) | bytes[3], unsigned);
+ };
+ /**
+ * Tests if the specified object is a Long.
+ */
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any, @typescript-eslint/explicit-module-boundary-types
+ Long.isLong = function (value) {
+ return utils.isObjectLike(value) && value['__isLong__'] === true;
+ };
+ /**
+ * Converts the specified value to a Long.
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ Long.fromValue = function (val, unsigned) {
+ if (typeof val === 'number')
+ return Long.fromNumber(val, unsigned);
+ if (typeof val === 'string')
+ return Long.fromString(val, unsigned);
+ // Throws for non-objects, converts non-instanceof Long:
+ return Long.fromBits(val.low, val.high, typeof unsigned === 'boolean' ? unsigned : val.unsigned);
+ };
+ /** Returns the sum of this and the specified Long. */
+ Long.prototype.add = function (addend) {
+ if (!Long.isLong(addend))
+ addend = Long.fromValue(addend);
+ // Divide each number into 4 chunks of 16 bits, and then sum the chunks.
+ var a48 = this.high >>> 16;
+ var a32 = this.high & 0xffff;
+ var a16 = this.low >>> 16;
+ var a00 = this.low & 0xffff;
+ var b48 = addend.high >>> 16;
+ var b32 = addend.high & 0xffff;
+ var b16 = addend.low >>> 16;
+ var b00 = addend.low & 0xffff;
+ var c48 = 0, c32 = 0, c16 = 0, c00 = 0;
+ c00 += a00 + b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 + b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 + b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 + b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ };
+ /**
+ * Returns the sum of this and the specified Long.
+ * @returns Sum
+ */
+ Long.prototype.and = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low & other.low, this.high & other.high, this.unsigned);
+ };
+ /**
+ * Compares this Long's value with the specified's.
+ * @returns 0 if they are the same, 1 if the this is greater and -1 if the given one is greater
+ */
+ Long.prototype.compare = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ if (this.eq(other))
+ return 0;
+ var thisNeg = this.isNegative(), otherNeg = other.isNegative();
+ if (thisNeg && !otherNeg)
+ return -1;
+ if (!thisNeg && otherNeg)
+ return 1;
+ // At this point the sign bits are the same
+ if (!this.unsigned)
+ return this.sub(other).isNegative() ? -1 : 1;
+ // Both are positive if at least one is unsigned
+ return other.high >>> 0 > this.high >>> 0 ||
+ (other.high === this.high && other.low >>> 0 > this.low >>> 0)
+ ? -1
+ : 1;
+ };
+ /** This is an alias of {@link Long.compare} */
+ Long.prototype.comp = function (other) {
+ return this.compare(other);
+ };
+ /**
+ * Returns this Long divided by the specified. The result is signed if this Long is signed or unsigned if this Long is unsigned.
+ * @returns Quotient
+ */
+ Long.prototype.divide = function (divisor) {
+ if (!Long.isLong(divisor))
+ divisor = Long.fromValue(divisor);
+ if (divisor.isZero())
+ throw Error('division by zero');
+ // use wasm support if present
+ if (wasm) {
+ // guard against signed division overflow: the largest
+ // negative number / -1 would be 1 larger than the largest
+ // positive number, due to two's complement.
+ if (!this.unsigned &&
+ this.high === -0x80000000 &&
+ divisor.low === -1 &&
+ divisor.high === -1) {
+ // be consistent with non-wasm code path
+ return this;
+ }
+ var low = (this.unsigned ? wasm.div_u : wasm.div_s)(this.low, this.high, divisor.low, divisor.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ if (this.isZero())
+ return this.unsigned ? Long.UZERO : Long.ZERO;
+ var approx, rem, res;
+ if (!this.unsigned) {
+ // This section is only relevant for signed longs and is derived from the
+ // closure library as a whole.
+ if (this.eq(Long.MIN_VALUE)) {
+ if (divisor.eq(Long.ONE) || divisor.eq(Long.NEG_ONE))
+ return Long.MIN_VALUE;
+ // recall that -MIN_VALUE == MIN_VALUE
+ else if (divisor.eq(Long.MIN_VALUE))
+ return Long.ONE;
+ else {
+ // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.
+ var halfThis = this.shr(1);
+ approx = halfThis.div(divisor).shl(1);
+ if (approx.eq(Long.ZERO)) {
+ return divisor.isNegative() ? Long.ONE : Long.NEG_ONE;
+ }
+ else {
+ rem = this.sub(divisor.mul(approx));
+ res = approx.add(rem.div(divisor));
+ return res;
+ }
+ }
+ }
+ else if (divisor.eq(Long.MIN_VALUE))
+ return this.unsigned ? Long.UZERO : Long.ZERO;
+ if (this.isNegative()) {
+ if (divisor.isNegative())
+ return this.neg().div(divisor.neg());
+ return this.neg().div(divisor).neg();
+ }
+ else if (divisor.isNegative())
+ return this.div(divisor.neg()).neg();
+ res = Long.ZERO;
+ }
+ else {
+ // The algorithm below has not been made for unsigned longs. It's therefore
+ // required to take special care of the MSB prior to running it.
+ if (!divisor.unsigned)
+ divisor = divisor.toUnsigned();
+ if (divisor.gt(this))
+ return Long.UZERO;
+ if (divisor.gt(this.shru(1)))
+ // 15 >>> 1 = 7 ; with divisor = 8 ; true
+ return Long.UONE;
+ res = Long.UZERO;
+ }
+ // Repeat the following until the remainder is less than other: find a
+ // floating-point that approximates remainder / other *from below*, add this
+ // into the result, and subtract it from the remainder. It is critical that
+ // the approximate value is less than or equal to the real value so that the
+ // remainder never becomes negative.
+ rem = this;
+ while (rem.gte(divisor)) {
+ // Approximate the result of division. This may be a little greater or
+ // smaller than the actual value.
+ approx = Math.max(1, Math.floor(rem.toNumber() / divisor.toNumber()));
+ // We will tweak the approximate result by changing it in the 48-th digit or
+ // the smallest non-fractional digit, whichever is larger.
+ var log2 = Math.ceil(Math.log(approx) / Math.LN2);
+ var delta = log2 <= 48 ? 1 : Math.pow(2, log2 - 48);
+ // Decrease the approximation until it is smaller than the remainder. Note
+ // that if it is too large, the product overflows and is negative.
+ var approxRes = Long.fromNumber(approx);
+ var approxRem = approxRes.mul(divisor);
+ while (approxRem.isNegative() || approxRem.gt(rem)) {
+ approx -= delta;
+ approxRes = Long.fromNumber(approx, this.unsigned);
+ approxRem = approxRes.mul(divisor);
+ }
+ // We know the answer can't be zero... and actually, zero would cause
+ // infinite recursion since we would make no progress.
+ if (approxRes.isZero())
+ approxRes = Long.ONE;
+ res = res.add(approxRes);
+ rem = rem.sub(approxRem);
+ }
+ return res;
+ };
+ /**This is an alias of {@link Long.divide} */
+ Long.prototype.div = function (divisor) {
+ return this.divide(divisor);
+ };
+ /**
+ * Tests if this Long's value equals the specified's.
+ * @param other - Other value
+ */
+ Long.prototype.equals = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ if (this.unsigned !== other.unsigned && this.high >>> 31 === 1 && other.high >>> 31 === 1)
+ return false;
+ return this.high === other.high && this.low === other.low;
+ };
+ /** This is an alias of {@link Long.equals} */
+ Long.prototype.eq = function (other) {
+ return this.equals(other);
+ };
+ /** Gets the high 32 bits as a signed integer. */
+ Long.prototype.getHighBits = function () {
+ return this.high;
+ };
+ /** Gets the high 32 bits as an unsigned integer. */
+ Long.prototype.getHighBitsUnsigned = function () {
+ return this.high >>> 0;
+ };
+ /** Gets the low 32 bits as a signed integer. */
+ Long.prototype.getLowBits = function () {
+ return this.low;
+ };
+ /** Gets the low 32 bits as an unsigned integer. */
+ Long.prototype.getLowBitsUnsigned = function () {
+ return this.low >>> 0;
+ };
+ /** Gets the number of bits needed to represent the absolute value of this Long. */
+ Long.prototype.getNumBitsAbs = function () {
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ return this.eq(Long.MIN_VALUE) ? 64 : this.neg().getNumBitsAbs();
+ }
+ var val = this.high !== 0 ? this.high : this.low;
+ var bit;
+ for (bit = 31; bit > 0; bit--)
+ if ((val & (1 << bit)) !== 0)
+ break;
+ return this.high !== 0 ? bit + 33 : bit + 1;
+ };
+ /** Tests if this Long's value is greater than the specified's. */
+ Long.prototype.greaterThan = function (other) {
+ return this.comp(other) > 0;
+ };
+ /** This is an alias of {@link Long.greaterThan} */
+ Long.prototype.gt = function (other) {
+ return this.greaterThan(other);
+ };
+ /** Tests if this Long's value is greater than or equal the specified's. */
+ Long.prototype.greaterThanOrEqual = function (other) {
+ return this.comp(other) >= 0;
+ };
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ Long.prototype.gte = function (other) {
+ return this.greaterThanOrEqual(other);
+ };
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ Long.prototype.ge = function (other) {
+ return this.greaterThanOrEqual(other);
+ };
+ /** Tests if this Long's value is even. */
+ Long.prototype.isEven = function () {
+ return (this.low & 1) === 0;
+ };
+ /** Tests if this Long's value is negative. */
+ Long.prototype.isNegative = function () {
+ return !this.unsigned && this.high < 0;
+ };
+ /** Tests if this Long's value is odd. */
+ Long.prototype.isOdd = function () {
+ return (this.low & 1) === 1;
+ };
+ /** Tests if this Long's value is positive. */
+ Long.prototype.isPositive = function () {
+ return this.unsigned || this.high >= 0;
+ };
+ /** Tests if this Long's value equals zero. */
+ Long.prototype.isZero = function () {
+ return this.high === 0 && this.low === 0;
+ };
+ /** Tests if this Long's value is less than the specified's. */
+ Long.prototype.lessThan = function (other) {
+ return this.comp(other) < 0;
+ };
+ /** This is an alias of {@link Long#lessThan}. */
+ Long.prototype.lt = function (other) {
+ return this.lessThan(other);
+ };
+ /** Tests if this Long's value is less than or equal the specified's. */
+ Long.prototype.lessThanOrEqual = function (other) {
+ return this.comp(other) <= 0;
+ };
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ Long.prototype.lte = function (other) {
+ return this.lessThanOrEqual(other);
+ };
+ /** Returns this Long modulo the specified. */
+ Long.prototype.modulo = function (divisor) {
+ if (!Long.isLong(divisor))
+ divisor = Long.fromValue(divisor);
+ // use wasm support if present
+ if (wasm) {
+ var low = (this.unsigned ? wasm.rem_u : wasm.rem_s)(this.low, this.high, divisor.low, divisor.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ return this.sub(this.div(divisor).mul(divisor));
+ };
+ /** This is an alias of {@link Long.modulo} */
+ Long.prototype.mod = function (divisor) {
+ return this.modulo(divisor);
+ };
+ /** This is an alias of {@link Long.modulo} */
+ Long.prototype.rem = function (divisor) {
+ return this.modulo(divisor);
+ };
+ /**
+ * Returns the product of this and the specified Long.
+ * @param multiplier - Multiplier
+ * @returns Product
+ */
+ Long.prototype.multiply = function (multiplier) {
+ if (this.isZero())
+ return Long.ZERO;
+ if (!Long.isLong(multiplier))
+ multiplier = Long.fromValue(multiplier);
+ // use wasm support if present
+ if (wasm) {
+ var low = wasm.mul(this.low, this.high, multiplier.low, multiplier.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ if (multiplier.isZero())
+ return Long.ZERO;
+ if (this.eq(Long.MIN_VALUE))
+ return multiplier.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (multiplier.eq(Long.MIN_VALUE))
+ return this.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (this.isNegative()) {
+ if (multiplier.isNegative())
+ return this.neg().mul(multiplier.neg());
+ else
+ return this.neg().mul(multiplier).neg();
+ }
+ else if (multiplier.isNegative())
+ return this.mul(multiplier.neg()).neg();
+ // If both longs are small, use float multiplication
+ if (this.lt(Long.TWO_PWR_24) && multiplier.lt(Long.TWO_PWR_24))
+ return Long.fromNumber(this.toNumber() * multiplier.toNumber(), this.unsigned);
+ // Divide each long into 4 chunks of 16 bits, and then add up 4x4 products.
+ // We can skip products that would overflow.
+ var a48 = this.high >>> 16;
+ var a32 = this.high & 0xffff;
+ var a16 = this.low >>> 16;
+ var a00 = this.low & 0xffff;
+ var b48 = multiplier.high >>> 16;
+ var b32 = multiplier.high & 0xffff;
+ var b16 = multiplier.low >>> 16;
+ var b00 = multiplier.low & 0xffff;
+ var c48 = 0, c32 = 0, c16 = 0, c00 = 0;
+ c00 += a00 * b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 * b00;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c16 += a00 * b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 * b00;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a16 * b16;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a00 * b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ };
+ /** This is an alias of {@link Long.multiply} */
+ Long.prototype.mul = function (multiplier) {
+ return this.multiply(multiplier);
+ };
+ /** Returns the Negation of this Long's value. */
+ Long.prototype.negate = function () {
+ if (!this.unsigned && this.eq(Long.MIN_VALUE))
+ return Long.MIN_VALUE;
+ return this.not().add(Long.ONE);
+ };
+ /** This is an alias of {@link Long.negate} */
+ Long.prototype.neg = function () {
+ return this.negate();
+ };
+ /** Returns the bitwise NOT of this Long. */
+ Long.prototype.not = function () {
+ return Long.fromBits(~this.low, ~this.high, this.unsigned);
+ };
+ /** Tests if this Long's value differs from the specified's. */
+ Long.prototype.notEquals = function (other) {
+ return !this.equals(other);
+ };
+ /** This is an alias of {@link Long.notEquals} */
+ Long.prototype.neq = function (other) {
+ return this.notEquals(other);
+ };
+ /** This is an alias of {@link Long.notEquals} */
+ Long.prototype.ne = function (other) {
+ return this.notEquals(other);
+ };
+ /**
+ * Returns the bitwise OR of this Long and the specified.
+ */
+ Long.prototype.or = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low | other.low, this.high | other.high, this.unsigned);
+ };
+ /**
+ * Returns this Long with bits shifted to the left by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftLeft = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ if ((numBits &= 63) === 0)
+ return this;
+ else if (numBits < 32)
+ return Long.fromBits(this.low << numBits, (this.high << numBits) | (this.low >>> (32 - numBits)), this.unsigned);
+ else
+ return Long.fromBits(0, this.low << (numBits - 32), this.unsigned);
+ };
+ /** This is an alias of {@link Long.shiftLeft} */
+ Long.prototype.shl = function (numBits) {
+ return this.shiftLeft(numBits);
+ };
+ /**
+ * Returns this Long with bits arithmetically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftRight = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ if ((numBits &= 63) === 0)
+ return this;
+ else if (numBits < 32)
+ return Long.fromBits((this.low >>> numBits) | (this.high << (32 - numBits)), this.high >> numBits, this.unsigned);
+ else
+ return Long.fromBits(this.high >> (numBits - 32), this.high >= 0 ? 0 : -1, this.unsigned);
+ };
+ /** This is an alias of {@link Long.shiftRight} */
+ Long.prototype.shr = function (numBits) {
+ return this.shiftRight(numBits);
+ };
+ /**
+ * Returns this Long with bits logically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftRightUnsigned = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ numBits &= 63;
+ if (numBits === 0)
+ return this;
+ else {
+ var high = this.high;
+ if (numBits < 32) {
+ var low = this.low;
+ return Long.fromBits((low >>> numBits) | (high << (32 - numBits)), high >>> numBits, this.unsigned);
+ }
+ else if (numBits === 32)
+ return Long.fromBits(high, 0, this.unsigned);
+ else
+ return Long.fromBits(high >>> (numBits - 32), 0, this.unsigned);
+ }
+ };
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ Long.prototype.shr_u = function (numBits) {
+ return this.shiftRightUnsigned(numBits);
+ };
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ Long.prototype.shru = function (numBits) {
+ return this.shiftRightUnsigned(numBits);
+ };
+ /**
+ * Returns the difference of this and the specified Long.
+ * @param subtrahend - Subtrahend
+ * @returns Difference
+ */
+ Long.prototype.subtract = function (subtrahend) {
+ if (!Long.isLong(subtrahend))
+ subtrahend = Long.fromValue(subtrahend);
+ return this.add(subtrahend.neg());
+ };
+ /** This is an alias of {@link Long.subtract} */
+ Long.prototype.sub = function (subtrahend) {
+ return this.subtract(subtrahend);
+ };
+ /** Converts the Long to a 32 bit integer, assuming it is a 32 bit integer. */
+ Long.prototype.toInt = function () {
+ return this.unsigned ? this.low >>> 0 : this.low;
+ };
+ /** Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa). */
+ Long.prototype.toNumber = function () {
+ if (this.unsigned)
+ return (this.high >>> 0) * TWO_PWR_32_DBL + (this.low >>> 0);
+ return this.high * TWO_PWR_32_DBL + (this.low >>> 0);
+ };
+ /** Converts the Long to a BigInt (arbitrary precision). */
+ Long.prototype.toBigInt = function () {
+ return BigInt(this.toString());
+ };
+ /**
+ * Converts this Long to its byte representation.
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns Byte representation
+ */
+ Long.prototype.toBytes = function (le) {
+ return le ? this.toBytesLE() : this.toBytesBE();
+ };
+ /**
+ * Converts this Long to its little endian byte representation.
+ * @returns Little endian byte representation
+ */
+ Long.prototype.toBytesLE = function () {
+ var hi = this.high, lo = this.low;
+ return [
+ lo & 0xff,
+ (lo >>> 8) & 0xff,
+ (lo >>> 16) & 0xff,
+ lo >>> 24,
+ hi & 0xff,
+ (hi >>> 8) & 0xff,
+ (hi >>> 16) & 0xff,
+ hi >>> 24
+ ];
+ };
+ /**
+ * Converts this Long to its big endian byte representation.
+ * @returns Big endian byte representation
+ */
+ Long.prototype.toBytesBE = function () {
+ var hi = this.high, lo = this.low;
+ return [
+ hi >>> 24,
+ (hi >>> 16) & 0xff,
+ (hi >>> 8) & 0xff,
+ hi & 0xff,
+ lo >>> 24,
+ (lo >>> 16) & 0xff,
+ (lo >>> 8) & 0xff,
+ lo & 0xff
+ ];
+ };
+ /**
+ * Converts this Long to signed.
+ */
+ Long.prototype.toSigned = function () {
+ if (!this.unsigned)
+ return this;
+ return Long.fromBits(this.low, this.high, false);
+ };
+ /**
+ * Converts the Long to a string written in the specified radix.
+ * @param radix - Radix (2-36), defaults to 10
+ * @throws RangeError If `radix` is out of range
+ */
+ Long.prototype.toString = function (radix) {
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix)
+ throw RangeError('radix');
+ if (this.isZero())
+ return '0';
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ if (this.eq(Long.MIN_VALUE)) {
+ // We need to change the Long value before it can be negated, so we remove
+ // the bottom-most digit in this base and then recurse to do the rest.
+ var radixLong = Long.fromNumber(radix), div = this.div(radixLong), rem1 = div.mul(radixLong).sub(this);
+ return div.toString(radix) + rem1.toInt().toString(radix);
+ }
+ else
+ return '-' + this.neg().toString(radix);
+ }
+ // Do several (6) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ var radixToPower = Long.fromNumber(Math.pow(radix, 6), this.unsigned);
+ // eslint-disable-next-line @typescript-eslint/no-this-alias
+ var rem = this;
+ var result = '';
+ // eslint-disable-next-line no-constant-condition
+ while (true) {
+ var remDiv = rem.div(radixToPower);
+ var intval = rem.sub(remDiv.mul(radixToPower)).toInt() >>> 0;
+ var digits = intval.toString(radix);
+ rem = remDiv;
+ if (rem.isZero()) {
+ return digits + result;
+ }
+ else {
+ while (digits.length < 6)
+ digits = '0' + digits;
+ result = '' + digits + result;
+ }
+ }
+ };
+ /** Converts this Long to unsigned. */
+ Long.prototype.toUnsigned = function () {
+ if (this.unsigned)
+ return this;
+ return Long.fromBits(this.low, this.high, true);
+ };
+ /** Returns the bitwise XOR of this Long and the given one. */
+ Long.prototype.xor = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low ^ other.low, this.high ^ other.high, this.unsigned);
+ };
+ /** This is an alias of {@link Long.isZero} */
+ Long.prototype.eqz = function () {
+ return this.isZero();
+ };
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ Long.prototype.le = function (other) {
+ return this.lessThanOrEqual(other);
+ };
+ /*
+ ****************************************************************
+ * BSON SPECIFIC ADDITIONS *
+ ****************************************************************
+ */
+ Long.prototype.toExtendedJSON = function (options) {
+ if (options && options.relaxed)
+ return this.toNumber();
+ return { $numberLong: this.toString() };
+ };
+ Long.fromExtendedJSON = function (doc, options) {
+ var result = Long.fromString(doc.$numberLong);
+ return options && options.relaxed ? result.toNumber() : result;
+ };
+ /** @internal */
+ Long.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Long.prototype.inspect = function () {
+ return "new Long(\"" + this.toString() + "\"" + (this.unsigned ? ', true' : '') + ")";
+ };
+ Long.TWO_PWR_24 = Long.fromInt(TWO_PWR_24_DBL);
+ /** Maximum unsigned value. */
+ Long.MAX_UNSIGNED_VALUE = Long.fromBits(0xffffffff | 0, 0xffffffff | 0, true);
+ /** Signed zero */
+ Long.ZERO = Long.fromInt(0);
+ /** Unsigned zero. */
+ Long.UZERO = Long.fromInt(0, true);
+ /** Signed one. */
+ Long.ONE = Long.fromInt(1);
+ /** Unsigned one. */
+ Long.UONE = Long.fromInt(1, true);
+ /** Signed negative one. */
+ Long.NEG_ONE = Long.fromInt(-1);
+ /** Maximum signed value. */
+ Long.MAX_VALUE = Long.fromBits(0xffffffff | 0, 0x7fffffff | 0, false);
+ /** Minimum signed value. */
+ Long.MIN_VALUE = Long.fromBits(0, 0x80000000 | 0, false);
+ return Long;
+ }());
+ exports.Long = Long;
+ Object.defineProperty(Long.prototype, '__isLong__', { value: true });
+ Object.defineProperty(Long.prototype, '_bsontype', { value: 'Long' });
+
+ });
+
+ unwrapExports(long_1);
+ long_1.Long;
+
+ var decimal128 = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Decimal128 = void 0;
+
+
+ var PARSE_STRING_REGEXP = /^(\+|-)?(\d+|(\d*\.\d*))?(E|e)?([-+])?(\d+)?$/;
+ var PARSE_INF_REGEXP = /^(\+|-)?(Infinity|inf)$/i;
+ var PARSE_NAN_REGEXP = /^(\+|-)?NaN$/i;
+ var EXPONENT_MAX = 6111;
+ var EXPONENT_MIN = -6176;
+ var EXPONENT_BIAS = 6176;
+ var MAX_DIGITS = 34;
+ // Nan value bits as 32 bit values (due to lack of longs)
+ var NAN_BUFFER = [
+ 0x7c,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+ ].reverse();
+ // Infinity value bits 32 bit values (due to lack of longs)
+ var INF_NEGATIVE_BUFFER = [
+ 0xf8,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+ ].reverse();
+ var INF_POSITIVE_BUFFER = [
+ 0x78,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+ ].reverse();
+ var EXPONENT_REGEX = /^([-+])?(\d+)?$/;
+ // Extract least significant 5 bits
+ var COMBINATION_MASK = 0x1f;
+ // Extract least significant 14 bits
+ var EXPONENT_MASK = 0x3fff;
+ // Value of combination field for Inf
+ var COMBINATION_INFINITY = 30;
+ // Value of combination field for NaN
+ var COMBINATION_NAN = 31;
+ // Detect if the value is a digit
+ function isDigit(value) {
+ return !isNaN(parseInt(value, 10));
+ }
+ // Divide two uint128 values
+ function divideu128(value) {
+ var DIVISOR = long_1.Long.fromNumber(1000 * 1000 * 1000);
+ var _rem = long_1.Long.fromNumber(0);
+ if (!value.parts[0] && !value.parts[1] && !value.parts[2] && !value.parts[3]) {
+ return { quotient: value, rem: _rem };
+ }
+ for (var i = 0; i <= 3; i++) {
+ // Adjust remainder to match value of next dividend
+ _rem = _rem.shiftLeft(32);
+ // Add the divided to _rem
+ _rem = _rem.add(new long_1.Long(value.parts[i], 0));
+ value.parts[i] = _rem.div(DIVISOR).low;
+ _rem = _rem.modulo(DIVISOR);
+ }
+ return { quotient: value, rem: _rem };
+ }
+ // Multiply two Long values and return the 128 bit value
+ function multiply64x2(left, right) {
+ if (!left && !right) {
+ return { high: long_1.Long.fromNumber(0), low: long_1.Long.fromNumber(0) };
+ }
+ var leftHigh = left.shiftRightUnsigned(32);
+ var leftLow = new long_1.Long(left.getLowBits(), 0);
+ var rightHigh = right.shiftRightUnsigned(32);
+ var rightLow = new long_1.Long(right.getLowBits(), 0);
+ var productHigh = leftHigh.multiply(rightHigh);
+ var productMid = leftHigh.multiply(rightLow);
+ var productMid2 = leftLow.multiply(rightHigh);
+ var productLow = leftLow.multiply(rightLow);
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productMid = new long_1.Long(productMid.getLowBits(), 0)
+ .add(productMid2)
+ .add(productLow.shiftRightUnsigned(32));
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productLow = productMid.shiftLeft(32).add(new long_1.Long(productLow.getLowBits(), 0));
+ // Return the 128 bit result
+ return { high: productHigh, low: productLow };
+ }
+ function lessThan(left, right) {
+ // Make values unsigned
+ var uhleft = left.high >>> 0;
+ var uhright = right.high >>> 0;
+ // Compare high bits first
+ if (uhleft < uhright) {
+ return true;
+ }
+ else if (uhleft === uhright) {
+ var ulleft = left.low >>> 0;
+ var ulright = right.low >>> 0;
+ if (ulleft < ulright)
+ return true;
+ }
+ return false;
+ }
+ function invalidErr(string, message) {
+ throw new TypeError("\"" + string + "\" is not a valid Decimal128 string - " + message);
+ }
+ /**
+ * A class representation of the BSON Decimal128 type.
+ * @public
+ */
+ var Decimal128 = /** @class */ (function () {
+ /**
+ * @param bytes - a buffer containing the raw Decimal128 bytes in little endian order,
+ * or a string representation as returned by .toString()
+ */
+ function Decimal128(bytes) {
+ if (!(this instanceof Decimal128))
+ return new Decimal128(bytes);
+ if (typeof bytes === 'string') {
+ this.bytes = Decimal128.fromString(bytes).bytes;
+ }
+ else {
+ this.bytes = bytes;
+ }
+ }
+ /**
+ * Create a Decimal128 instance from a string representation
+ *
+ * @param representation - a numeric string representation.
+ */
+ Decimal128.fromString = function (representation) {
+ // Parse state tracking
+ var isNegative = false;
+ var sawRadix = false;
+ var foundNonZero = false;
+ // Total number of significant digits (no leading or trailing zero)
+ var significantDigits = 0;
+ // Total number of significand digits read
+ var nDigitsRead = 0;
+ // Total number of digits (no leading zeros)
+ var nDigits = 0;
+ // The number of the digits after radix
+ var radixPosition = 0;
+ // The index of the first non-zero in *str*
+ var firstNonZero = 0;
+ // Digits Array
+ var digits = [0];
+ // The number of digits in digits
+ var nDigitsStored = 0;
+ // Insertion pointer for digits
+ var digitsInsert = 0;
+ // The index of the first non-zero digit
+ var firstDigit = 0;
+ // The index of the last digit
+ var lastDigit = 0;
+ // Exponent
+ var exponent = 0;
+ // loop index over array
+ var i = 0;
+ // The high 17 digits of the significand
+ var significandHigh = new long_1.Long(0, 0);
+ // The low 17 digits of the significand
+ var significandLow = new long_1.Long(0, 0);
+ // The biased exponent
+ var biasedExponent = 0;
+ // Read index
+ var index = 0;
+ // Naively prevent against REDOS attacks.
+ // TODO: implementing a custom parsing for this, or refactoring the regex would yield
+ // further gains.
+ if (representation.length >= 7000) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+ // Results
+ var stringMatch = representation.match(PARSE_STRING_REGEXP);
+ var infMatch = representation.match(PARSE_INF_REGEXP);
+ var nanMatch = representation.match(PARSE_NAN_REGEXP);
+ // Validate the string
+ if ((!stringMatch && !infMatch && !nanMatch) || representation.length === 0) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+ if (stringMatch) {
+ // full_match = stringMatch[0]
+ // sign = stringMatch[1]
+ var unsignedNumber = stringMatch[2];
+ // stringMatch[3] is undefined if a whole number (ex "1", 12")
+ // but defined if a number w/ decimal in it (ex "1.0, 12.2")
+ var e = stringMatch[4];
+ var expSign = stringMatch[5];
+ var expNumber = stringMatch[6];
+ // they provided e, but didn't give an exponent number. for ex "1e"
+ if (e && expNumber === undefined)
+ invalidErr(representation, 'missing exponent power');
+ // they provided e, but didn't give a number before it. for ex "e1"
+ if (e && unsignedNumber === undefined)
+ invalidErr(representation, 'missing exponent base');
+ if (e === undefined && (expSign || expNumber)) {
+ invalidErr(representation, 'missing e before exponent');
+ }
+ }
+ // Get the negative or positive sign
+ if (representation[index] === '+' || representation[index] === '-') {
+ isNegative = representation[index++] === '-';
+ }
+ // Check if user passed Infinity or NaN
+ if (!isDigit(representation[index]) && representation[index] !== '.') {
+ if (representation[index] === 'i' || representation[index] === 'I') {
+ return new Decimal128(buffer.Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ }
+ else if (representation[index] === 'N') {
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ }
+ }
+ // Read all the digits
+ while (isDigit(representation[index]) || representation[index] === '.') {
+ if (representation[index] === '.') {
+ if (sawRadix)
+ invalidErr(representation, 'contains multiple periods');
+ sawRadix = true;
+ index = index + 1;
+ continue;
+ }
+ if (nDigitsStored < 34) {
+ if (representation[index] !== '0' || foundNonZero) {
+ if (!foundNonZero) {
+ firstNonZero = nDigitsRead;
+ }
+ foundNonZero = true;
+ // Only store 34 digits
+ digits[digitsInsert++] = parseInt(representation[index], 10);
+ nDigitsStored = nDigitsStored + 1;
+ }
+ }
+ if (foundNonZero)
+ nDigits = nDigits + 1;
+ if (sawRadix)
+ radixPosition = radixPosition + 1;
+ nDigitsRead = nDigitsRead + 1;
+ index = index + 1;
+ }
+ if (sawRadix && !nDigitsRead)
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ // Read exponent if exists
+ if (representation[index] === 'e' || representation[index] === 'E') {
+ // Read exponent digits
+ var match = representation.substr(++index).match(EXPONENT_REGEX);
+ // No digits read
+ if (!match || !match[2])
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ // Get exponent
+ exponent = parseInt(match[0], 10);
+ // Adjust the index
+ index = index + match[0].length;
+ }
+ // Return not a number
+ if (representation[index])
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ // Done reading input
+ // Find first non-zero digit in digits
+ firstDigit = 0;
+ if (!nDigitsStored) {
+ firstDigit = 0;
+ lastDigit = 0;
+ digits[0] = 0;
+ nDigits = 1;
+ nDigitsStored = 1;
+ significantDigits = 0;
+ }
+ else {
+ lastDigit = nDigitsStored - 1;
+ significantDigits = nDigits;
+ if (significantDigits !== 1) {
+ while (representation[firstNonZero + significantDigits - 1] === '0') {
+ significantDigits = significantDigits - 1;
+ }
+ }
+ }
+ // Normalization of exponent
+ // Correct exponent based on radix position, and shift significand as needed
+ // to represent user input
+ // Overflow prevention
+ if (exponent <= radixPosition && radixPosition - exponent > 1 << 14) {
+ exponent = EXPONENT_MIN;
+ }
+ else {
+ exponent = exponent - radixPosition;
+ }
+ // Attempt to normalize the exponent
+ while (exponent > EXPONENT_MAX) {
+ // Shift exponent to significand and decrease
+ lastDigit = lastDigit + 1;
+ if (lastDigit - firstDigit > MAX_DIGITS) {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ var digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ exponent = exponent - 1;
+ }
+ while (exponent < EXPONENT_MIN || nDigitsStored < nDigits) {
+ // Shift last digit. can only do this if < significant digits than # stored.
+ if (lastDigit === 0 && significantDigits < nDigitsStored) {
+ exponent = EXPONENT_MIN;
+ significantDigits = 0;
+ break;
+ }
+ if (nDigitsStored < nDigits) {
+ // adjust to match digits not stored
+ nDigits = nDigits - 1;
+ }
+ else {
+ // adjust to round
+ lastDigit = lastDigit - 1;
+ }
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ }
+ else {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ var digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ }
+ // Round
+ // We've normalized the exponent, but might still need to round.
+ if (lastDigit - firstDigit + 1 < significantDigits) {
+ var endOfString = nDigitsRead;
+ // If we have seen a radix point, 'string' is 1 longer than we have
+ // documented with ndigits_read, so inc the position of the first nonzero
+ // digit and the position that digits are read to.
+ if (sawRadix) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ // if negative, we need to increment again to account for - sign at start.
+ if (isNegative) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ var roundDigit = parseInt(representation[firstNonZero + lastDigit + 1], 10);
+ var roundBit = 0;
+ if (roundDigit >= 5) {
+ roundBit = 1;
+ if (roundDigit === 5) {
+ roundBit = digits[lastDigit] % 2 === 1 ? 1 : 0;
+ for (i = firstNonZero + lastDigit + 2; i < endOfString; i++) {
+ if (parseInt(representation[i], 10)) {
+ roundBit = 1;
+ break;
+ }
+ }
+ }
+ }
+ if (roundBit) {
+ var dIdx = lastDigit;
+ for (; dIdx >= 0; dIdx--) {
+ if (++digits[dIdx] > 9) {
+ digits[dIdx] = 0;
+ // overflowed most significant digit
+ if (dIdx === 0) {
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ digits[dIdx] = 1;
+ }
+ else {
+ return new Decimal128(buffer.Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ }
+ }
+ }
+ }
+ }
+ }
+ // Encode significand
+ // The high 17 digits of the significand
+ significandHigh = long_1.Long.fromNumber(0);
+ // The low 17 digits of the significand
+ significandLow = long_1.Long.fromNumber(0);
+ // read a zero
+ if (significantDigits === 0) {
+ significandHigh = long_1.Long.fromNumber(0);
+ significandLow = long_1.Long.fromNumber(0);
+ }
+ else if (lastDigit - firstDigit < 17) {
+ var dIdx = firstDigit;
+ significandLow = long_1.Long.fromNumber(digits[dIdx++]);
+ significandHigh = new long_1.Long(0, 0);
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(long_1.Long.fromNumber(10));
+ significandLow = significandLow.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ }
+ else {
+ var dIdx = firstDigit;
+ significandHigh = long_1.Long.fromNumber(digits[dIdx++]);
+ for (; dIdx <= lastDigit - 17; dIdx++) {
+ significandHigh = significandHigh.multiply(long_1.Long.fromNumber(10));
+ significandHigh = significandHigh.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ significandLow = long_1.Long.fromNumber(digits[dIdx++]);
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(long_1.Long.fromNumber(10));
+ significandLow = significandLow.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ }
+ var significand = multiply64x2(significandHigh, long_1.Long.fromString('100000000000000000'));
+ significand.low = significand.low.add(significandLow);
+ if (lessThan(significand.low, significandLow)) {
+ significand.high = significand.high.add(long_1.Long.fromNumber(1));
+ }
+ // Biased exponent
+ biasedExponent = exponent + EXPONENT_BIAS;
+ var dec = { low: long_1.Long.fromNumber(0), high: long_1.Long.fromNumber(0) };
+ // Encode combination, exponent, and significand.
+ if (significand.high.shiftRightUnsigned(49).and(long_1.Long.fromNumber(1)).equals(long_1.Long.fromNumber(1))) {
+ // Encode '11' into bits 1 to 3
+ dec.high = dec.high.or(long_1.Long.fromNumber(0x3).shiftLeft(61));
+ dec.high = dec.high.or(long_1.Long.fromNumber(biasedExponent).and(long_1.Long.fromNumber(0x3fff).shiftLeft(47)));
+ dec.high = dec.high.or(significand.high.and(long_1.Long.fromNumber(0x7fffffffffff)));
+ }
+ else {
+ dec.high = dec.high.or(long_1.Long.fromNumber(biasedExponent & 0x3fff).shiftLeft(49));
+ dec.high = dec.high.or(significand.high.and(long_1.Long.fromNumber(0x1ffffffffffff)));
+ }
+ dec.low = significand.low;
+ // Encode sign
+ if (isNegative) {
+ dec.high = dec.high.or(long_1.Long.fromString('9223372036854775808'));
+ }
+ // Encode into a buffer
+ var buffer$1 = buffer.Buffer.alloc(16);
+ index = 0;
+ // Encode the low 64 bits of the decimal
+ // Encode low bits
+ buffer$1[index++] = dec.low.low & 0xff;
+ buffer$1[index++] = (dec.low.low >> 8) & 0xff;
+ buffer$1[index++] = (dec.low.low >> 16) & 0xff;
+ buffer$1[index++] = (dec.low.low >> 24) & 0xff;
+ // Encode high bits
+ buffer$1[index++] = dec.low.high & 0xff;
+ buffer$1[index++] = (dec.low.high >> 8) & 0xff;
+ buffer$1[index++] = (dec.low.high >> 16) & 0xff;
+ buffer$1[index++] = (dec.low.high >> 24) & 0xff;
+ // Encode the high 64 bits of the decimal
+ // Encode low bits
+ buffer$1[index++] = dec.high.low & 0xff;
+ buffer$1[index++] = (dec.high.low >> 8) & 0xff;
+ buffer$1[index++] = (dec.high.low >> 16) & 0xff;
+ buffer$1[index++] = (dec.high.low >> 24) & 0xff;
+ // Encode high bits
+ buffer$1[index++] = dec.high.high & 0xff;
+ buffer$1[index++] = (dec.high.high >> 8) & 0xff;
+ buffer$1[index++] = (dec.high.high >> 16) & 0xff;
+ buffer$1[index++] = (dec.high.high >> 24) & 0xff;
+ // Return the new Decimal128
+ return new Decimal128(buffer$1);
+ };
+ /** Create a string representation of the raw Decimal128 value */
+ Decimal128.prototype.toString = function () {
+ // Note: bits in this routine are referred to starting at 0,
+ // from the sign bit, towards the coefficient.
+ // decoded biased exponent (14 bits)
+ var biased_exponent;
+ // the number of significand digits
+ var significand_digits = 0;
+ // the base-10 digits in the significand
+ var significand = new Array(36);
+ for (var i = 0; i < significand.length; i++)
+ significand[i] = 0;
+ // read pointer into significand
+ var index = 0;
+ // true if the number is zero
+ var is_zero = false;
+ // the most significant significand bits (50-46)
+ var significand_msb;
+ // temporary storage for significand decoding
+ var significand128 = { parts: [0, 0, 0, 0] };
+ // indexing variables
+ var j, k;
+ // Output string
+ var string = [];
+ // Unpack index
+ index = 0;
+ // Buffer reference
+ var buffer = this.bytes;
+ // Unpack the low 64bits into a long
+ // bits 96 - 127
+ var low = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 64 - 95
+ var midl = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Unpack the high 64bits into a long
+ // bits 32 - 63
+ var midh = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 0 - 31
+ var high = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Unpack index
+ index = 0;
+ // Create the state of the decimal
+ var dec = {
+ low: new long_1.Long(low, midl),
+ high: new long_1.Long(midh, high)
+ };
+ if (dec.high.lessThan(long_1.Long.ZERO)) {
+ string.push('-');
+ }
+ // Decode combination field and exponent
+ // bits 1 - 5
+ var combination = (high >> 26) & COMBINATION_MASK;
+ if (combination >> 3 === 3) {
+ // Check for 'special' values
+ if (combination === COMBINATION_INFINITY) {
+ return string.join('') + 'Infinity';
+ }
+ else if (combination === COMBINATION_NAN) {
+ return 'NaN';
+ }
+ else {
+ biased_exponent = (high >> 15) & EXPONENT_MASK;
+ significand_msb = 0x08 + ((high >> 14) & 0x01);
+ }
+ }
+ else {
+ significand_msb = (high >> 14) & 0x07;
+ biased_exponent = (high >> 17) & EXPONENT_MASK;
+ }
+ // unbiased exponent
+ var exponent = biased_exponent - EXPONENT_BIAS;
+ // Create string of significand digits
+ // Convert the 114-bit binary number represented by
+ // (significand_high, significand_low) to at most 34 decimal
+ // digits through modulo and division.
+ significand128.parts[0] = (high & 0x3fff) + ((significand_msb & 0xf) << 14);
+ significand128.parts[1] = midh;
+ significand128.parts[2] = midl;
+ significand128.parts[3] = low;
+ if (significand128.parts[0] === 0 &&
+ significand128.parts[1] === 0 &&
+ significand128.parts[2] === 0 &&
+ significand128.parts[3] === 0) {
+ is_zero = true;
+ }
+ else {
+ for (k = 3; k >= 0; k--) {
+ var least_digits = 0;
+ // Perform the divide
+ var result = divideu128(significand128);
+ significand128 = result.quotient;
+ least_digits = result.rem.low;
+ // We now have the 9 least significant digits (in base 2).
+ // Convert and output to string.
+ if (!least_digits)
+ continue;
+ for (j = 8; j >= 0; j--) {
+ // significand[k * 9 + j] = Math.round(least_digits % 10);
+ significand[k * 9 + j] = least_digits % 10;
+ // least_digits = Math.round(least_digits / 10);
+ least_digits = Math.floor(least_digits / 10);
+ }
+ }
+ }
+ // Output format options:
+ // Scientific - [-]d.dddE(+/-)dd or [-]dE(+/-)dd
+ // Regular - ddd.ddd
+ if (is_zero) {
+ significand_digits = 1;
+ significand[index] = 0;
+ }
+ else {
+ significand_digits = 36;
+ while (!significand[index]) {
+ significand_digits = significand_digits - 1;
+ index = index + 1;
+ }
+ }
+ // the exponent if scientific notation is used
+ var scientific_exponent = significand_digits - 1 + exponent;
+ // The scientific exponent checks are dictated by the string conversion
+ // specification and are somewhat arbitrary cutoffs.
+ //
+ // We must check exponent > 0, because if this is the case, the number
+ // has trailing zeros. However, we *cannot* output these trailing zeros,
+ // because doing so would change the precision of the value, and would
+ // change stored data if the string converted number is round tripped.
+ if (scientific_exponent >= 34 || scientific_exponent <= -7 || exponent > 0) {
+ // Scientific format
+ // if there are too many significant digits, we should just be treating numbers
+ // as + or - 0 and using the non-scientific exponent (this is for the "invalid
+ // representation should be treated as 0/-0" spec cases in decimal128-1.json)
+ if (significand_digits > 34) {
+ string.push("" + 0);
+ if (exponent > 0)
+ string.push('E+' + exponent);
+ else if (exponent < 0)
+ string.push('E' + exponent);
+ return string.join('');
+ }
+ string.push("" + significand[index++]);
+ significand_digits = significand_digits - 1;
+ if (significand_digits) {
+ string.push('.');
+ }
+ for (var i = 0; i < significand_digits; i++) {
+ string.push("" + significand[index++]);
+ }
+ // Exponent
+ string.push('E');
+ if (scientific_exponent > 0) {
+ string.push('+' + scientific_exponent);
+ }
+ else {
+ string.push("" + scientific_exponent);
+ }
+ }
+ else {
+ // Regular format with no decimal place
+ if (exponent >= 0) {
+ for (var i = 0; i < significand_digits; i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ else {
+ var radix_position = significand_digits + exponent;
+ // non-zero digits before radix
+ if (radix_position > 0) {
+ for (var i = 0; i < radix_position; i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ else {
+ string.push('0');
+ }
+ string.push('.');
+ // add leading zeros after radix
+ while (radix_position++ < 0) {
+ string.push('0');
+ }
+ for (var i = 0; i < significand_digits - Math.max(radix_position - 1, 0); i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ }
+ return string.join('');
+ };
+ Decimal128.prototype.toJSON = function () {
+ return { $numberDecimal: this.toString() };
+ };
+ /** @internal */
+ Decimal128.prototype.toExtendedJSON = function () {
+ return { $numberDecimal: this.toString() };
+ };
+ /** @internal */
+ Decimal128.fromExtendedJSON = function (doc) {
+ return Decimal128.fromString(doc.$numberDecimal);
+ };
+ /** @internal */
+ Decimal128.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Decimal128.prototype.inspect = function () {
+ return "new Decimal128(\"" + this.toString() + "\")";
+ };
+ return Decimal128;
+ }());
+ exports.Decimal128 = Decimal128;
+ Object.defineProperty(Decimal128.prototype, '_bsontype', { value: 'Decimal128' });
+
+ });
+
+ unwrapExports(decimal128);
+ decimal128.Decimal128;
+
+ var double_1 = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Double = void 0;
+ /**
+ * A class representation of the BSON Double type.
+ * @public
+ */
+ var Double = /** @class */ (function () {
+ /**
+ * Create a Double type
+ *
+ * @param value - the number we want to represent as a double.
+ */
+ function Double(value) {
+ if (!(this instanceof Double))
+ return new Double(value);
+ if (value instanceof Number) {
+ value = value.valueOf();
+ }
+ this.value = +value;
+ }
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped double number.
+ */
+ Double.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ Double.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ Double.prototype.toExtendedJSON = function (options) {
+ if (options && (options.legacy || (options.relaxed && isFinite(this.value)))) {
+ return this.value;
+ }
+ // NOTE: JavaScript has +0 and -0, apparently to model limit calculations. If a user
+ // explicitly provided `-0` then we need to ensure the sign makes it into the output
+ if (Object.is(Math.sign(this.value), -0)) {
+ return { $numberDouble: "-" + this.value.toFixed(1) };
+ }
+ var $numberDouble;
+ if (Number.isInteger(this.value)) {
+ $numberDouble = this.value.toFixed(1);
+ if ($numberDouble.length >= 13) {
+ $numberDouble = this.value.toExponential(13).toUpperCase();
+ }
+ }
+ else {
+ $numberDouble = this.value.toString();
+ }
+ return { $numberDouble: $numberDouble };
+ };
+ /** @internal */
+ Double.fromExtendedJSON = function (doc, options) {
+ var doubleValue = parseFloat(doc.$numberDouble);
+ return options && options.relaxed ? doubleValue : new Double(doubleValue);
+ };
+ /** @internal */
+ Double.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Double.prototype.inspect = function () {
+ var eJSON = this.toExtendedJSON();
+ return "new Double(" + eJSON.$numberDouble + ")";
+ };
+ return Double;
+ }());
+ exports.Double = Double;
+ Object.defineProperty(Double.prototype, '_bsontype', { value: 'Double' });
+
+ });
+
+ unwrapExports(double_1);
+ double_1.Double;
+
+ var int_32 = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Int32 = void 0;
+ /**
+ * A class representation of a BSON Int32 type.
+ * @public
+ */
+ var Int32 = /** @class */ (function () {
+ /**
+ * Create an Int32 type
+ *
+ * @param value - the number we want to represent as an int32.
+ */
+ function Int32(value) {
+ if (!(this instanceof Int32))
+ return new Int32(value);
+ if (value instanceof Number) {
+ value = value.valueOf();
+ }
+ this.value = +value;
+ }
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped int32 number.
+ */
+ Int32.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ Int32.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ Int32.prototype.toExtendedJSON = function (options) {
+ if (options && (options.relaxed || options.legacy))
+ return this.value;
+ return { $numberInt: this.value.toString() };
+ };
+ /** @internal */
+ Int32.fromExtendedJSON = function (doc, options) {
+ return options && options.relaxed ? parseInt(doc.$numberInt, 10) : new Int32(doc.$numberInt);
+ };
+ /** @internal */
+ Int32.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Int32.prototype.inspect = function () {
+ return "new Int32(" + this.valueOf() + ")";
+ };
+ return Int32;
+ }());
+ exports.Int32 = Int32;
+ Object.defineProperty(Int32.prototype, '_bsontype', { value: 'Int32' });
+
+ });
+
+ unwrapExports(int_32);
+ int_32.Int32;
+
+ var max_key = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.MaxKey = void 0;
+ /**
+ * A class representation of the BSON MaxKey type.
+ * @public
+ */
+ var MaxKey = /** @class */ (function () {
+ function MaxKey() {
+ if (!(this instanceof MaxKey))
+ return new MaxKey();
+ }
+ /** @internal */
+ MaxKey.prototype.toExtendedJSON = function () {
+ return { $maxKey: 1 };
+ };
+ /** @internal */
+ MaxKey.fromExtendedJSON = function () {
+ return new MaxKey();
+ };
+ /** @internal */
+ MaxKey.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ MaxKey.prototype.inspect = function () {
+ return 'new MaxKey()';
+ };
+ return MaxKey;
+ }());
+ exports.MaxKey = MaxKey;
+ Object.defineProperty(MaxKey.prototype, '_bsontype', { value: 'MaxKey' });
+
+ });
+
+ unwrapExports(max_key);
+ max_key.MaxKey;
+
+ var min_key = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.MinKey = void 0;
+ /**
+ * A class representation of the BSON MinKey type.
+ * @public
+ */
+ var MinKey = /** @class */ (function () {
+ function MinKey() {
+ if (!(this instanceof MinKey))
+ return new MinKey();
+ }
+ /** @internal */
+ MinKey.prototype.toExtendedJSON = function () {
+ return { $minKey: 1 };
+ };
+ /** @internal */
+ MinKey.fromExtendedJSON = function () {
+ return new MinKey();
+ };
+ /** @internal */
+ MinKey.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ MinKey.prototype.inspect = function () {
+ return 'new MinKey()';
+ };
+ return MinKey;
+ }());
+ exports.MinKey = MinKey;
+ Object.defineProperty(MinKey.prototype, '_bsontype', { value: 'MinKey' });
+
+ });
+
+ unwrapExports(min_key);
+ min_key.MinKey;
+
+ var objectid = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.ObjectId = void 0;
+
+
+
+ // Regular expression that checks for hex value
+ var checkForHexRegExp = new RegExp('^[0-9a-fA-F]{24}$');
+ // Unique sequence for the current process (initialized on first use)
+ var PROCESS_UNIQUE = null;
+ var kId = Symbol('id');
+ /**
+ * A class representation of the BSON ObjectId type.
+ * @public
+ */
+ var ObjectId = /** @class */ (function () {
+ /**
+ * Create an ObjectId type
+ *
+ * @param id - Can be a 24 character hex string, 12 byte binary Buffer, or a number.
+ */
+ function ObjectId(id) {
+ if (!(this instanceof ObjectId))
+ return new ObjectId(id);
+ // Duck-typing to support ObjectId from different npm packages
+ if (id instanceof ObjectId) {
+ this[kId] = id.id;
+ this.__id = id.__id;
+ }
+ if (typeof id === 'object' && id && 'id' in id) {
+ if ('toHexString' in id && typeof id.toHexString === 'function') {
+ this[kId] = buffer.Buffer.from(id.toHexString(), 'hex');
+ }
+ else {
+ this[kId] = typeof id.id === 'string' ? buffer.Buffer.from(id.id) : id.id;
+ }
+ }
+ // The most common use case (blank id, new objectId instance)
+ if (id == null || typeof id === 'number') {
+ // Generate a new id
+ this[kId] = ObjectId.generate(typeof id === 'number' ? id : undefined);
+ // If we are caching the hex string
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+ if (ArrayBuffer.isView(id) && id.byteLength === 12) {
+ this[kId] = ensure_buffer.ensureBuffer(id);
+ }
+ if (typeof id === 'string') {
+ if (id.length === 12) {
+ var bytes = buffer.Buffer.from(id);
+ if (bytes.byteLength === 12) {
+ this[kId] = bytes;
+ }
+ }
+ else if (id.length === 24 && checkForHexRegExp.test(id)) {
+ this[kId] = buffer.Buffer.from(id, 'hex');
+ }
+ else {
+ throw new TypeError('Argument passed in must be a Buffer or string of 12 bytes or a string of 24 hex characters');
+ }
+ }
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+ Object.defineProperty(ObjectId.prototype, "id", {
+ /**
+ * The ObjectId bytes
+ * @readonly
+ */
+ get: function () {
+ return this[kId];
+ },
+ set: function (value) {
+ this[kId] = value;
+ if (ObjectId.cacheHexString) {
+ this.__id = value.toString('hex');
+ }
+ },
+ enumerable: false,
+ configurable: true
+ });
+ Object.defineProperty(ObjectId.prototype, "generationTime", {
+ /**
+ * The generation time of this ObjectId instance
+ * @deprecated Please use getTimestamp / createFromTime which returns an int32 epoch
+ */
+ get: function () {
+ return this.id.readInt32BE(0);
+ },
+ set: function (value) {
+ // Encode time into first 4 bytes
+ this.id.writeUInt32BE(value, 0);
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /** Returns the ObjectId id as a 24 character hex string representation */
+ ObjectId.prototype.toHexString = function () {
+ if (ObjectId.cacheHexString && this.__id) {
+ return this.__id;
+ }
+ var hexString = this.id.toString('hex');
+ if (ObjectId.cacheHexString && !this.__id) {
+ this.__id = hexString;
+ }
+ return hexString;
+ };
+ /**
+ * Update the ObjectId index
+ * @privateRemarks
+ * Used in generating new ObjectId's on the driver
+ * @internal
+ */
+ ObjectId.getInc = function () {
+ return (ObjectId.index = (ObjectId.index + 1) % 0xffffff);
+ };
+ /**
+ * Generate a 12 byte id buffer used in ObjectId's
+ *
+ * @param time - pass in a second based timestamp.
+ */
+ ObjectId.generate = function (time) {
+ if ('number' !== typeof time) {
+ time = ~~(Date.now() / 1000);
+ }
+ var inc = ObjectId.getInc();
+ var buffer$1 = buffer.Buffer.alloc(12);
+ // 4-byte timestamp
+ buffer$1.writeUInt32BE(time, 0);
+ // set PROCESS_UNIQUE if yet not initialized
+ if (PROCESS_UNIQUE === null) {
+ PROCESS_UNIQUE = utils.randomBytes(5);
+ }
+ // 5-byte process unique
+ buffer$1[4] = PROCESS_UNIQUE[0];
+ buffer$1[5] = PROCESS_UNIQUE[1];
+ buffer$1[6] = PROCESS_UNIQUE[2];
+ buffer$1[7] = PROCESS_UNIQUE[3];
+ buffer$1[8] = PROCESS_UNIQUE[4];
+ // 3-byte counter
+ buffer$1[11] = inc & 0xff;
+ buffer$1[10] = (inc >> 8) & 0xff;
+ buffer$1[9] = (inc >> 16) & 0xff;
+ return buffer$1;
+ };
+ /**
+ * Converts the id into a 24 character hex string for printing
+ *
+ * @param format - The Buffer toString format parameter.
+ * @internal
+ */
+ ObjectId.prototype.toString = function (format) {
+ // Is the id a buffer then use the buffer toString method to return the format
+ if (format)
+ return this.id.toString(format);
+ return this.toHexString();
+ };
+ /**
+ * Converts to its JSON the 24 character hex string representation.
+ * @internal
+ */
+ ObjectId.prototype.toJSON = function () {
+ return this.toHexString();
+ };
+ /**
+ * Compares the equality of this ObjectId with `otherID`.
+ *
+ * @param otherId - ObjectId instance to compare against.
+ */
+ ObjectId.prototype.equals = function (otherId) {
+ if (otherId === undefined || otherId === null) {
+ return false;
+ }
+ if (otherId instanceof ObjectId) {
+ return this.toString() === otherId.toString();
+ }
+ if (typeof otherId === 'string' &&
+ ObjectId.isValid(otherId) &&
+ otherId.length === 12 &&
+ utils.isUint8Array(this.id)) {
+ return otherId === buffer.Buffer.prototype.toString.call(this.id, 'latin1');
+ }
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 24) {
+ return otherId.toLowerCase() === this.toHexString();
+ }
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 12) {
+ return buffer.Buffer.from(otherId).equals(this.id);
+ }
+ if (typeof otherId === 'object' &&
+ 'toHexString' in otherId &&
+ typeof otherId.toHexString === 'function') {
+ return otherId.toHexString() === this.toHexString();
+ }
+ return false;
+ };
+ /** Returns the generation date (accurate up to the second) that this ID was generated. */
+ ObjectId.prototype.getTimestamp = function () {
+ var timestamp = new Date();
+ var time = this.id.readUInt32BE(0);
+ timestamp.setTime(Math.floor(time) * 1000);
+ return timestamp;
+ };
+ /** @internal */
+ ObjectId.createPk = function () {
+ return new ObjectId();
+ };
+ /**
+ * Creates an ObjectId from a second based number, with the rest of the ObjectId zeroed out. Used for comparisons or sorting the ObjectId.
+ *
+ * @param time - an integer number representing a number of seconds.
+ */
+ ObjectId.createFromTime = function (time) {
+ var buffer$1 = buffer.Buffer.from([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]);
+ // Encode time into first 4 bytes
+ buffer$1.writeUInt32BE(time, 0);
+ // Return the new objectId
+ return new ObjectId(buffer$1);
+ };
+ /**
+ * Creates an ObjectId from a hex string representation of an ObjectId.
+ *
+ * @param hexString - create a ObjectId from a passed in 24 character hexstring.
+ */
+ ObjectId.createFromHexString = function (hexString) {
+ // Throw an error if it's not a valid setup
+ if (typeof hexString === 'undefined' || (hexString != null && hexString.length !== 24)) {
+ throw new TypeError('Argument passed in must be a single String of 12 bytes or a string of 24 hex characters');
+ }
+ return new ObjectId(buffer.Buffer.from(hexString, 'hex'));
+ };
+ /**
+ * Checks if a value is a valid bson ObjectId
+ *
+ * @param id - ObjectId instance to validate.
+ */
+ ObjectId.isValid = function (id) {
+ if (id == null)
+ return false;
+ if (typeof id === 'number') {
+ return true;
+ }
+ if (typeof id === 'string') {
+ return id.length === 12 || (id.length === 24 && checkForHexRegExp.test(id));
+ }
+ if (id instanceof ObjectId) {
+ return true;
+ }
+ if (utils.isUint8Array(id) && id.length === 12) {
+ return true;
+ }
+ // Duck-Typing detection of ObjectId like objects
+ if (typeof id === 'object' && 'toHexString' in id && typeof id.toHexString === 'function') {
+ if (typeof id.id === 'string') {
+ return id.id.length === 12;
+ }
+ return id.toHexString().length === 24 && checkForHexRegExp.test(id.id.toString('hex'));
+ }
+ return false;
+ };
+ /** @internal */
+ ObjectId.prototype.toExtendedJSON = function () {
+ if (this.toHexString)
+ return { $oid: this.toHexString() };
+ return { $oid: this.toString('hex') };
+ };
+ /** @internal */
+ ObjectId.fromExtendedJSON = function (doc) {
+ return new ObjectId(doc.$oid);
+ };
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 24 character hex string representation.
+ * @internal
+ */
+ ObjectId.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ ObjectId.prototype.inspect = function () {
+ return "new ObjectId(\"" + this.toHexString() + "\")";
+ };
+ /** @internal */
+ ObjectId.index = ~~(Math.random() * 0xffffff);
+ return ObjectId;
+ }());
+ exports.ObjectId = ObjectId;
+ // Deprecated methods
+ Object.defineProperty(ObjectId.prototype, 'generate', {
+ value: utils.deprecate(function (time) { return ObjectId.generate(time); }, 'Please use the static `ObjectId.generate(time)` instead')
+ });
+ Object.defineProperty(ObjectId.prototype, 'getInc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+ });
+ Object.defineProperty(ObjectId.prototype, 'get_inc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+ });
+ Object.defineProperty(ObjectId, 'get_inc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+ });
+ Object.defineProperty(ObjectId.prototype, '_bsontype', { value: 'ObjectID' });
+
+ });
+
+ unwrapExports(objectid);
+ objectid.ObjectId;
+
+ var regexp = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.BSONRegExp = void 0;
+ function alphabetize(str) {
+ return str.split('').sort().join('');
+ }
+ /**
+ * A class representation of the BSON RegExp type.
+ * @public
+ */
+ var BSONRegExp = /** @class */ (function () {
+ /**
+ * @param pattern - The regular expression pattern to match
+ * @param options - The regular expression options
+ */
+ function BSONRegExp(pattern, options) {
+ if (!(this instanceof BSONRegExp))
+ return new BSONRegExp(pattern, options);
+ this.pattern = pattern;
+ this.options = alphabetize(options !== null && options !== void 0 ? options : '');
+ // Validate options
+ for (var i = 0; i < this.options.length; i++) {
+ if (!(this.options[i] === 'i' ||
+ this.options[i] === 'm' ||
+ this.options[i] === 'x' ||
+ this.options[i] === 'l' ||
+ this.options[i] === 's' ||
+ this.options[i] === 'u')) {
+ throw new Error("The regular expression option [" + this.options[i] + "] is not supported");
+ }
+ }
+ }
+ BSONRegExp.parseOptions = function (options) {
+ return options ? options.split('').sort().join('') : '';
+ };
+ /** @internal */
+ BSONRegExp.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ if (options.legacy) {
+ return { $regex: this.pattern, $options: this.options };
+ }
+ return { $regularExpression: { pattern: this.pattern, options: this.options } };
+ };
+ /** @internal */
+ BSONRegExp.fromExtendedJSON = function (doc) {
+ if ('$regex' in doc) {
+ if (typeof doc.$regex !== 'string') {
+ // This is for $regex query operators that have extended json values.
+ if (doc.$regex._bsontype === 'BSONRegExp') {
+ return doc;
+ }
+ }
+ else {
+ return new BSONRegExp(doc.$regex, BSONRegExp.parseOptions(doc.$options));
+ }
+ }
+ if ('$regularExpression' in doc) {
+ return new BSONRegExp(doc.$regularExpression.pattern, BSONRegExp.parseOptions(doc.$regularExpression.options));
+ }
+ throw new TypeError("Unexpected BSONRegExp EJSON object form: " + JSON.stringify(doc));
+ };
+ return BSONRegExp;
+ }());
+ exports.BSONRegExp = BSONRegExp;
+ Object.defineProperty(BSONRegExp.prototype, '_bsontype', { value: 'BSONRegExp' });
+
+ });
+
+ unwrapExports(regexp);
+ regexp.BSONRegExp;
+
+ var symbol = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.BSONSymbol = void 0;
+ /**
+ * A class representation of the BSON Symbol type.
+ * @public
+ */
+ var BSONSymbol = /** @class */ (function () {
+ /**
+ * @param value - the string representing the symbol.
+ */
+ function BSONSymbol(value) {
+ if (!(this instanceof BSONSymbol))
+ return new BSONSymbol(value);
+ this.value = value;
+ }
+ /** Access the wrapped string value. */
+ BSONSymbol.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.toString = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.inspect = function () {
+ return "new BSONSymbol(\"" + this.value + "\")";
+ };
+ /** @internal */
+ BSONSymbol.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.toExtendedJSON = function () {
+ return { $symbol: this.value };
+ };
+ /** @internal */
+ BSONSymbol.fromExtendedJSON = function (doc) {
+ return new BSONSymbol(doc.$symbol);
+ };
+ /** @internal */
+ BSONSymbol.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ return BSONSymbol;
+ }());
+ exports.BSONSymbol = BSONSymbol;
+ Object.defineProperty(BSONSymbol.prototype, '_bsontype', { value: 'Symbol' });
+
+ });
+
+ unwrapExports(symbol);
+ symbol.BSONSymbol;
+
+ /*! *****************************************************************************
+ Copyright (c) Microsoft Corporation.
+
+ Permission to use, copy, modify, and/or distribute this software for any
+ purpose with or without fee is hereby granted.
+
+ THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH
+ REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY
+ AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,
+ INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM
+ LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR
+ OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR
+ PERFORMANCE OF THIS SOFTWARE.
+ ***************************************************************************** */
+
+ /* global Reflect, Promise */
+ var _extendStatics = function extendStatics(d, b) {
+ _extendStatics = Object.setPrototypeOf || {
+ __proto__: []
+ } instanceof Array && function (d, b) {
+ d.__proto__ = b;
+ } || function (d, b) {
+ for (var p in b) {
+ if (b.hasOwnProperty(p)) d[p] = b[p];
+ }
+ };
+
+ return _extendStatics(d, b);
+ };
+
+ function __extends(d, b) {
+ _extendStatics(d, b);
+
+ function __() {
+ this.constructor = d;
+ }
+
+ d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
+ }
+
+ var _assign = function __assign() {
+ _assign = Object.assign || function __assign(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+
+ for (var p in s) {
+ if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p];
+ }
+ }
+
+ return t;
+ };
+
+ return _assign.apply(this, arguments);
+ };
+ function __rest(s, e) {
+ var t = {};
+
+ for (var p in s) {
+ if (Object.prototype.hasOwnProperty.call(s, p) && e.indexOf(p) < 0) t[p] = s[p];
+ }
+
+ if (s != null && typeof Object.getOwnPropertySymbols === "function") for (var i = 0, p = Object.getOwnPropertySymbols(s); i < p.length; i++) {
+ if (e.indexOf(p[i]) < 0 && Object.prototype.propertyIsEnumerable.call(s, p[i])) t[p[i]] = s[p[i]];
+ }
+ return t;
+ }
+ function __decorate(decorators, target, key, desc) {
+ var c = arguments.length,
+ r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc,
+ d;
+ if ((typeof Reflect === "undefined" ? "undefined" : babelHelpers["typeof"](Reflect)) === "object" && typeof Reflect.decorate === "function") r = Reflect.decorate(decorators, target, key, desc);else for (var i = decorators.length - 1; i >= 0; i--) {
+ if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;
+ }
+ return c > 3 && r && Object.defineProperty(target, key, r), r;
+ }
+ function __param(paramIndex, decorator) {
+ return function (target, key) {
+ decorator(target, key, paramIndex);
+ };
+ }
+ function __metadata(metadataKey, metadataValue) {
+ if ((typeof Reflect === "undefined" ? "undefined" : babelHelpers["typeof"](Reflect)) === "object" && typeof Reflect.metadata === "function") return Reflect.metadata(metadataKey, metadataValue);
+ }
+ function __awaiter(thisArg, _arguments, P, generator) {
+ function adopt(value) {
+ return value instanceof P ? value : new P(function (resolve) {
+ resolve(value);
+ });
+ }
+
+ return new (P || (P = Promise))(function (resolve, reject) {
+ function fulfilled(value) {
+ try {
+ step(generator.next(value));
+ } catch (e) {
+ reject(e);
+ }
+ }
+
+ function rejected(value) {
+ try {
+ step(generator["throw"](value));
+ } catch (e) {
+ reject(e);
+ }
+ }
+
+ function step(result) {
+ result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected);
+ }
+
+ step((generator = generator.apply(thisArg, _arguments || [])).next());
+ });
+ }
+ function __generator(thisArg, body) {
+ var _ = {
+ label: 0,
+ sent: function sent() {
+ if (t[0] & 1) throw t[1];
+ return t[1];
+ },
+ trys: [],
+ ops: []
+ },
+ f,
+ y,
+ t,
+ g;
+ return g = {
+ next: verb(0),
+ "throw": verb(1),
+ "return": verb(2)
+ }, typeof Symbol === "function" && (g[Symbol.iterator] = function () {
+ return this;
+ }), g;
+
+ function verb(n) {
+ return function (v) {
+ return step([n, v]);
+ };
+ }
+
+ function step(op) {
+ if (f) throw new TypeError("Generator is already executing.");
+
+ while (_) {
+ try {
+ if (f = 1, y && (t = op[0] & 2 ? y["return"] : op[0] ? y["throw"] || ((t = y["return"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done) return t;
+ if (y = 0, t) op = [op[0] & 2, t.value];
+
+ switch (op[0]) {
+ case 0:
+ case 1:
+ t = op;
+ break;
+
+ case 4:
+ _.label++;
+ return {
+ value: op[1],
+ done: false
+ };
+
+ case 5:
+ _.label++;
+ y = op[1];
+ op = [0];
+ continue;
+
+ case 7:
+ op = _.ops.pop();
+
+ _.trys.pop();
+
+ continue;
+
+ default:
+ if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) {
+ _ = 0;
+ continue;
+ }
+
+ if (op[0] === 3 && (!t || op[1] > t[0] && op[1] < t[3])) {
+ _.label = op[1];
+ break;
+ }
+
+ if (op[0] === 6 && _.label < t[1]) {
+ _.label = t[1];
+ t = op;
+ break;
+ }
+
+ if (t && _.label < t[2]) {
+ _.label = t[2];
+
+ _.ops.push(op);
+
+ break;
+ }
+
+ if (t[2]) _.ops.pop();
+
+ _.trys.pop();
+
+ continue;
+ }
+
+ op = body.call(thisArg, _);
+ } catch (e) {
+ op = [6, e];
+ y = 0;
+ } finally {
+ f = t = 0;
+ }
+ }
+
+ if (op[0] & 5) throw op[1];
+ return {
+ value: op[0] ? op[1] : void 0,
+ done: true
+ };
+ }
+ }
+ function __createBinding(o, m, k, k2) {
+ if (k2 === undefined) k2 = k;
+ o[k2] = m[k];
+ }
+ function __exportStar(m, exports) {
+ for (var p in m) {
+ if (p !== "default" && !exports.hasOwnProperty(p)) exports[p] = m[p];
+ }
+ }
+ function __values(o) {
+ var s = typeof Symbol === "function" && Symbol.iterator,
+ m = s && o[s],
+ i = 0;
+ if (m) return m.call(o);
+ if (o && typeof o.length === "number") return {
+ next: function next() {
+ if (o && i >= o.length) o = void 0;
+ return {
+ value: o && o[i++],
+ done: !o
+ };
+ }
+ };
+ throw new TypeError(s ? "Object is not iterable." : "Symbol.iterator is not defined.");
+ }
+ function __read(o, n) {
+ var m = typeof Symbol === "function" && o[Symbol.iterator];
+ if (!m) return o;
+ var i = m.call(o),
+ r,
+ ar = [],
+ e;
+
+ try {
+ while ((n === void 0 || n-- > 0) && !(r = i.next()).done) {
+ ar.push(r.value);
+ }
+ } catch (error) {
+ e = {
+ error: error
+ };
+ } finally {
+ try {
+ if (r && !r.done && (m = i["return"])) m.call(i);
+ } finally {
+ if (e) throw e.error;
+ }
+ }
+
+ return ar;
+ }
+ function __spread() {
+ for (var ar = [], i = 0; i < arguments.length; i++) {
+ ar = ar.concat(__read(arguments[i]));
+ }
+
+ return ar;
+ }
+ function __spreadArrays() {
+ for (var s = 0, i = 0, il = arguments.length; i < il; i++) {
+ s += arguments[i].length;
+ }
+
+ for (var r = Array(s), k = 0, i = 0; i < il; i++) {
+ for (var a = arguments[i], j = 0, jl = a.length; j < jl; j++, k++) {
+ r[k] = a[j];
+ }
+ }
+
+ return r;
+ }
+ function __await(v) {
+ return this instanceof __await ? (this.v = v, this) : new __await(v);
+ }
+ function __asyncGenerator(thisArg, _arguments, generator) {
+ if (!Symbol.asyncIterator) throw new TypeError("Symbol.asyncIterator is not defined.");
+ var g = generator.apply(thisArg, _arguments || []),
+ i,
+ q = [];
+ return i = {}, verb("next"), verb("throw"), verb("return"), i[Symbol.asyncIterator] = function () {
+ return this;
+ }, i;
+
+ function verb(n) {
+ if (g[n]) i[n] = function (v) {
+ return new Promise(function (a, b) {
+ q.push([n, v, a, b]) > 1 || resume(n, v);
+ });
+ };
+ }
+
+ function resume(n, v) {
+ try {
+ step(g[n](v));
+ } catch (e) {
+ settle(q[0][3], e);
+ }
+ }
+
+ function step(r) {
+ r.value instanceof __await ? Promise.resolve(r.value.v).then(fulfill, reject) : settle(q[0][2], r);
+ }
+
+ function fulfill(value) {
+ resume("next", value);
+ }
+
+ function reject(value) {
+ resume("throw", value);
+ }
+
+ function settle(f, v) {
+ if (f(v), q.shift(), q.length) resume(q[0][0], q[0][1]);
+ }
+ }
+ function __asyncDelegator(o) {
+ var i, p;
+ return i = {}, verb("next"), verb("throw", function (e) {
+ throw e;
+ }), verb("return"), i[Symbol.iterator] = function () {
+ return this;
+ }, i;
+
+ function verb(n, f) {
+ i[n] = o[n] ? function (v) {
+ return (p = !p) ? {
+ value: __await(o[n](v)),
+ done: n === "return"
+ } : f ? f(v) : v;
+ } : f;
+ }
+ }
+ function __asyncValues(o) {
+ if (!Symbol.asyncIterator) throw new TypeError("Symbol.asyncIterator is not defined.");
+ var m = o[Symbol.asyncIterator],
+ i;
+ return m ? m.call(o) : (o = typeof __values === "function" ? __values(o) : o[Symbol.iterator](), i = {}, verb("next"), verb("throw"), verb("return"), i[Symbol.asyncIterator] = function () {
+ return this;
+ }, i);
+
+ function verb(n) {
+ i[n] = o[n] && function (v) {
+ return new Promise(function (resolve, reject) {
+ v = o[n](v), settle(resolve, reject, v.done, v.value);
+ });
+ };
+ }
+
+ function settle(resolve, reject, d, v) {
+ Promise.resolve(v).then(function (v) {
+ resolve({
+ value: v,
+ done: d
+ });
+ }, reject);
+ }
+ }
+ function __makeTemplateObject(cooked, raw) {
+ if (Object.defineProperty) {
+ Object.defineProperty(cooked, "raw", {
+ value: raw
+ });
+ } else {
+ cooked.raw = raw;
+ }
+
+ return cooked;
+ }
+ function __importStar(mod) {
+ if (mod && mod.__esModule) return mod;
+ var result = {};
+ if (mod != null) for (var k in mod) {
+ if (Object.hasOwnProperty.call(mod, k)) result[k] = mod[k];
+ }
+ result["default"] = mod;
+ return result;
+ }
+ function __importDefault(mod) {
+ return mod && mod.__esModule ? mod : {
+ "default": mod
+ };
+ }
+ function __classPrivateFieldGet(receiver, privateMap) {
+ if (!privateMap.has(receiver)) {
+ throw new TypeError("attempted to get private field on non-instance");
+ }
+
+ return privateMap.get(receiver);
+ }
+ function __classPrivateFieldSet(receiver, privateMap, value) {
+ if (!privateMap.has(receiver)) {
+ throw new TypeError("attempted to set private field on non-instance");
+ }
+
+ privateMap.set(receiver, value);
+ return value;
+ }
+
+ var tslib_es6 = /*#__PURE__*/Object.freeze({
+ __proto__: null,
+ __extends: __extends,
+ get __assign () { return _assign; },
+ __rest: __rest,
+ __decorate: __decorate,
+ __param: __param,
+ __metadata: __metadata,
+ __awaiter: __awaiter,
+ __generator: __generator,
+ __createBinding: __createBinding,
+ __exportStar: __exportStar,
+ __values: __values,
+ __read: __read,
+ __spread: __spread,
+ __spreadArrays: __spreadArrays,
+ __await: __await,
+ __asyncGenerator: __asyncGenerator,
+ __asyncDelegator: __asyncDelegator,
+ __asyncValues: __asyncValues,
+ __makeTemplateObject: __makeTemplateObject,
+ __importStar: __importStar,
+ __importDefault: __importDefault,
+ __classPrivateFieldGet: __classPrivateFieldGet,
+ __classPrivateFieldSet: __classPrivateFieldSet
+ });
+
+ var timestamp = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Timestamp = exports.LongWithoutOverridesClass = void 0;
+
+
+ /** @public */
+ exports.LongWithoutOverridesClass = long_1.Long;
+ /** @public */
+ var Timestamp = /** @class */ (function (_super) {
+ tslib_es6.__extends(Timestamp, _super);
+ function Timestamp(low, high) {
+ var _this = this;
+ // eslint-disable-next-line @typescript-eslint/ban-ts-comment
+ ///@ts-expect-error
+ if (!(_this instanceof Timestamp))
+ return new Timestamp(low, high);
+ if (long_1.Long.isLong(low)) {
+ _this = _super.call(this, low.low, low.high, true) || this;
+ }
+ else {
+ _this = _super.call(this, low, high, true) || this;
+ }
+ Object.defineProperty(_this, '_bsontype', {
+ value: 'Timestamp',
+ writable: false,
+ configurable: false,
+ enumerable: false
+ });
+ return _this;
+ }
+ Timestamp.prototype.toJSON = function () {
+ return {
+ $timestamp: this.toString()
+ };
+ };
+ /** Returns a Timestamp represented by the given (32-bit) integer value. */
+ Timestamp.fromInt = function (value) {
+ return new Timestamp(long_1.Long.fromInt(value, true));
+ };
+ /** Returns a Timestamp representing the given number value, provided that it is a finite number. Otherwise, zero is returned. */
+ Timestamp.fromNumber = function (value) {
+ return new Timestamp(long_1.Long.fromNumber(value, true));
+ };
+ /**
+ * Returns a Timestamp for the given high and low bits. Each is assumed to use 32 bits.
+ *
+ * @param lowBits - the low 32-bits.
+ * @param highBits - the high 32-bits.
+ */
+ Timestamp.fromBits = function (lowBits, highBits) {
+ return new Timestamp(lowBits, highBits);
+ };
+ /**
+ * Returns a Timestamp from the given string, optionally using the given radix.
+ *
+ * @param str - the textual representation of the Timestamp.
+ * @param optRadix - the radix in which the text is written.
+ */
+ Timestamp.fromString = function (str, optRadix) {
+ return new Timestamp(long_1.Long.fromString(str, true, optRadix));
+ };
+ /** @internal */
+ Timestamp.prototype.toExtendedJSON = function () {
+ return { $timestamp: { t: this.high >>> 0, i: this.low >>> 0 } };
+ };
+ /** @internal */
+ Timestamp.fromExtendedJSON = function (doc) {
+ return new Timestamp(doc.$timestamp.i, doc.$timestamp.t);
+ };
+ /** @internal */
+ Timestamp.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Timestamp.prototype.inspect = function () {
+ return "new Timestamp(" + this.getLowBits().toString() + ", " + this.getHighBits().toString() + ")";
+ };
+ Timestamp.MAX_VALUE = long_1.Long.MAX_UNSIGNED_VALUE;
+ return Timestamp;
+ }(exports.LongWithoutOverridesClass));
+ exports.Timestamp = Timestamp;
+
+ });
+
+ unwrapExports(timestamp);
+ timestamp.Timestamp;
+ timestamp.LongWithoutOverridesClass;
+
+ var extended_json = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.EJSON = exports.isBSONType = void 0;
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ function isBSONType(value) {
+ return (utils.isObjectLike(value) && Reflect.has(value, '_bsontype') && typeof value._bsontype === 'string');
+ }
+ exports.isBSONType = isBSONType;
+ // INT32 boundaries
+ var BSON_INT32_MAX = 0x7fffffff;
+ var BSON_INT32_MIN = -0x80000000;
+ // INT64 boundaries
+ var BSON_INT64_MAX = 0x7fffffffffffffff;
+ var BSON_INT64_MIN = -0x8000000000000000;
+ // all the types where we don't need to do any special processing and can just pass the EJSON
+ //straight to type.fromExtendedJSON
+ var keysToCodecs = {
+ $oid: objectid.ObjectId,
+ $binary: binary.Binary,
+ $uuid: binary.Binary,
+ $symbol: symbol.BSONSymbol,
+ $numberInt: int_32.Int32,
+ $numberDecimal: decimal128.Decimal128,
+ $numberDouble: double_1.Double,
+ $numberLong: long_1.Long,
+ $minKey: min_key.MinKey,
+ $maxKey: max_key.MaxKey,
+ $regex: regexp.BSONRegExp,
+ $regularExpression: regexp.BSONRegExp,
+ $timestamp: timestamp.Timestamp
+ };
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ function deserializeValue(value, options) {
+ if (options === void 0) { options = {}; }
+ if (typeof value === 'number') {
+ if (options.relaxed || options.legacy) {
+ return value;
+ }
+ // if it's an integer, should interpret as smallest BSON integer
+ // that can represent it exactly. (if out of range, interpret as double.)
+ if (Math.floor(value) === value) {
+ if (value >= BSON_INT32_MIN && value <= BSON_INT32_MAX)
+ return new int_32.Int32(value);
+ if (value >= BSON_INT64_MIN && value <= BSON_INT64_MAX)
+ return long_1.Long.fromNumber(value);
+ }
+ // If the number is a non-integer or out of integer range, should interpret as BSON Double.
+ return new double_1.Double(value);
+ }
+ // from here on out we're looking for bson types, so bail if its not an object
+ if (value == null || typeof value !== 'object')
+ return value;
+ // upgrade deprecated undefined to null
+ if (value.$undefined)
+ return null;
+ var keys = Object.keys(value).filter(function (k) { return k.startsWith('$') && value[k] != null; });
+ for (var i = 0; i < keys.length; i++) {
+ var c = keysToCodecs[keys[i]];
+ if (c)
+ return c.fromExtendedJSON(value, options);
+ }
+ if (value.$date != null) {
+ var d = value.$date;
+ var date = new Date();
+ if (options.legacy) {
+ if (typeof d === 'number')
+ date.setTime(d);
+ else if (typeof d === 'string')
+ date.setTime(Date.parse(d));
+ }
+ else {
+ if (typeof d === 'string')
+ date.setTime(Date.parse(d));
+ else if (long_1.Long.isLong(d))
+ date.setTime(d.toNumber());
+ else if (typeof d === 'number' && options.relaxed)
+ date.setTime(d);
+ }
+ return date;
+ }
+ if (value.$code != null) {
+ var copy = Object.assign({}, value);
+ if (value.$scope) {
+ copy.$scope = deserializeValue(value.$scope);
+ }
+ return code.Code.fromExtendedJSON(value);
+ }
+ if (db_ref.isDBRefLike(value) || value.$dbPointer) {
+ var v = value.$ref ? value : value.$dbPointer;
+ // we run into this in a "degenerate EJSON" case (with $id and $ref order flipped)
+ // because of the order JSON.parse goes through the document
+ if (v instanceof db_ref.DBRef)
+ return v;
+ var dollarKeys = Object.keys(v).filter(function (k) { return k.startsWith('$'); });
+ var valid_1 = true;
+ dollarKeys.forEach(function (k) {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1)
+ valid_1 = false;
+ });
+ // only make DBRef if $ keys are all valid
+ if (valid_1)
+ return db_ref.DBRef.fromExtendedJSON(v);
+ }
+ return value;
+ }
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ function serializeArray(array, options) {
+ return array.map(function (v, index) {
+ options.seenObjects.push({ propertyName: "index " + index, obj: null });
+ try {
+ return serializeValue(v, options);
+ }
+ finally {
+ options.seenObjects.pop();
+ }
+ });
+ }
+ function getISOString(date) {
+ var isoStr = date.toISOString();
+ // we should only show milliseconds in timestamp if they're non-zero
+ return date.getUTCMilliseconds() !== 0 ? isoStr : isoStr.slice(0, -5) + 'Z';
+ }
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ function serializeValue(value, options) {
+ if ((typeof value === 'object' || typeof value === 'function') && value !== null) {
+ var index = options.seenObjects.findIndex(function (entry) { return entry.obj === value; });
+ if (index !== -1) {
+ var props = options.seenObjects.map(function (entry) { return entry.propertyName; });
+ var leadingPart = props
+ .slice(0, index)
+ .map(function (prop) { return prop + " -> "; })
+ .join('');
+ var alreadySeen = props[index];
+ var circularPart = ' -> ' +
+ props
+ .slice(index + 1, props.length - 1)
+ .map(function (prop) { return prop + " -> "; })
+ .join('');
+ var current = props[props.length - 1];
+ var leadingSpace = ' '.repeat(leadingPart.length + alreadySeen.length / 2);
+ var dashes = '-'.repeat(circularPart.length + (alreadySeen.length + current.length) / 2 - 1);
+ throw new TypeError('Converting circular structure to EJSON:\n' +
+ (" " + leadingPart + alreadySeen + circularPart + current + "\n") +
+ (" " + leadingSpace + "\\" + dashes + "/"));
+ }
+ options.seenObjects[options.seenObjects.length - 1].obj = value;
+ }
+ if (Array.isArray(value))
+ return serializeArray(value, options);
+ if (value === undefined)
+ return null;
+ if (value instanceof Date || utils.isDate(value)) {
+ var dateNum = value.getTime(),
+ // is it in year range 1970-9999?
+ inRange = dateNum > -1 && dateNum < 253402318800000;
+ if (options.legacy) {
+ return options.relaxed && inRange
+ ? { $date: value.getTime() }
+ : { $date: getISOString(value) };
+ }
+ return options.relaxed && inRange
+ ? { $date: getISOString(value) }
+ : { $date: { $numberLong: value.getTime().toString() } };
+ }
+ if (typeof value === 'number' && (!options.relaxed || !isFinite(value))) {
+ // it's an integer
+ if (Math.floor(value) === value) {
+ var int32Range = value >= BSON_INT32_MIN && value <= BSON_INT32_MAX, int64Range = value >= BSON_INT64_MIN && value <= BSON_INT64_MAX;
+ // interpret as being of the smallest BSON integer type that can represent the number exactly
+ if (int32Range)
+ return { $numberInt: value.toString() };
+ if (int64Range)
+ return { $numberLong: value.toString() };
+ }
+ return { $numberDouble: value.toString() };
+ }
+ if (value instanceof RegExp || utils.isRegExp(value)) {
+ var flags = value.flags;
+ if (flags === undefined) {
+ var match = value.toString().match(/[gimuy]*$/);
+ if (match) {
+ flags = match[0];
+ }
+ }
+ var rx = new regexp.BSONRegExp(value.source, flags);
+ return rx.toExtendedJSON(options);
+ }
+ if (value != null && typeof value === 'object')
+ return serializeDocument(value, options);
+ return value;
+ }
+ var BSON_TYPE_MAPPINGS = {
+ Binary: function (o) { return new binary.Binary(o.value(), o.sub_type); },
+ Code: function (o) { return new code.Code(o.code, o.scope); },
+ DBRef: function (o) { return new db_ref.DBRef(o.collection || o.namespace, o.oid, o.db, o.fields); },
+ Decimal128: function (o) { return new decimal128.Decimal128(o.bytes); },
+ Double: function (o) { return new double_1.Double(o.value); },
+ Int32: function (o) { return new int_32.Int32(o.value); },
+ Long: function (o) {
+ return long_1.Long.fromBits(
+ // underscore variants for 1.x backwards compatibility
+ o.low != null ? o.low : o.low_, o.low != null ? o.high : o.high_, o.low != null ? o.unsigned : o.unsigned_);
+ },
+ MaxKey: function () { return new max_key.MaxKey(); },
+ MinKey: function () { return new min_key.MinKey(); },
+ ObjectID: function (o) { return new objectid.ObjectId(o); },
+ ObjectId: function (o) { return new objectid.ObjectId(o); },
+ BSONRegExp: function (o) { return new regexp.BSONRegExp(o.pattern, o.options); },
+ Symbol: function (o) { return new symbol.BSONSymbol(o.value); },
+ Timestamp: function (o) { return timestamp.Timestamp.fromBits(o.low, o.high); }
+ };
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ function serializeDocument(doc, options) {
+ if (doc == null || typeof doc !== 'object')
+ throw new Error('not an object instance');
+ var bsontype = doc._bsontype;
+ if (typeof bsontype === 'undefined') {
+ // It's a regular object. Recursively serialize its property values.
+ var _doc = {};
+ for (var name in doc) {
+ options.seenObjects.push({ propertyName: name, obj: null });
+ try {
+ _doc[name] = serializeValue(doc[name], options);
+ }
+ finally {
+ options.seenObjects.pop();
+ }
+ }
+ return _doc;
+ }
+ else if (isBSONType(doc)) {
+ // the "document" is really just a BSON type object
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ var outDoc = doc;
+ if (typeof outDoc.toExtendedJSON !== 'function') {
+ // There's no EJSON serialization function on the object. It's probably an
+ // object created by a previous version of this library (or another library)
+ // that's duck-typing objects to look like they were generated by this library).
+ // Copy the object into this library's version of that type.
+ var mapper = BSON_TYPE_MAPPINGS[doc._bsontype];
+ if (!mapper) {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + doc._bsontype);
+ }
+ outDoc = mapper(outDoc);
+ }
+ // Two BSON types may have nested objects that may need to be serialized too
+ if (bsontype === 'Code' && outDoc.scope) {
+ outDoc = new code.Code(outDoc.code, serializeValue(outDoc.scope, options));
+ }
+ else if (bsontype === 'DBRef' && outDoc.oid) {
+ outDoc = new db_ref.DBRef(serializeValue(outDoc.collection, options), serializeValue(outDoc.oid, options), serializeValue(outDoc.db, options), serializeValue(outDoc.fields, options));
+ }
+ return outDoc.toExtendedJSON(options);
+ }
+ else {
+ throw new Error('_bsontype must be a string, but was: ' + typeof bsontype);
+ }
+ }
+ (function (EJSON) {
+ /**
+ * Parse an Extended JSON string, constructing the JavaScript value or object described by that
+ * string.
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const text = '{ "int32": { "$numberInt": "10" } }';
+ *
+ * // prints { int32: { [String: '10'] _bsontype: 'Int32', value: '10' } }
+ * console.log(EJSON.parse(text, { relaxed: false }));
+ *
+ * // prints { int32: 10 }
+ * console.log(EJSON.parse(text));
+ * ```
+ */
+ function parse(text, options) {
+ var finalOptions = Object.assign({}, { relaxed: true, legacy: false }, options);
+ // relaxed implies not strict
+ if (typeof finalOptions.relaxed === 'boolean')
+ finalOptions.strict = !finalOptions.relaxed;
+ if (typeof finalOptions.strict === 'boolean')
+ finalOptions.relaxed = !finalOptions.strict;
+ return JSON.parse(text, function (_key, value) { return deserializeValue(value, finalOptions); });
+ }
+ EJSON.parse = parse;
+ /**
+ * Converts a BSON document to an Extended JSON string, optionally replacing values if a replacer
+ * function is specified or optionally including only the specified properties if a replacer array
+ * is specified.
+ *
+ * @param value - The value to convert to extended JSON
+ * @param replacer - A function that alters the behavior of the stringification process, or an array of String and Number objects that serve as a whitelist for selecting/filtering the properties of the value object to be included in the JSON string. If this value is null or not provided, all properties of the object are included in the resulting JSON string
+ * @param space - A String or Number object that's used to insert white space into the output JSON string for readability purposes.
+ * @param options - Optional settings
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const Int32 = require('mongodb').Int32;
+ * const doc = { int32: new Int32(10) };
+ *
+ * // prints '{"int32":{"$numberInt":"10"}}'
+ * console.log(EJSON.stringify(doc, { relaxed: false }));
+ *
+ * // prints '{"int32":10}'
+ * console.log(EJSON.stringify(doc));
+ * ```
+ */
+ function stringify(value,
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ replacer, space, options) {
+ if (space != null && typeof space === 'object') {
+ options = space;
+ space = 0;
+ }
+ if (replacer != null && typeof replacer === 'object' && !Array.isArray(replacer)) {
+ options = replacer;
+ replacer = undefined;
+ space = 0;
+ }
+ var serializeOptions = Object.assign({ relaxed: true, legacy: false }, options, {
+ seenObjects: [{ propertyName: '(root)', obj: null }]
+ });
+ var doc = serializeValue(value, serializeOptions);
+ return JSON.stringify(doc, replacer, space);
+ }
+ EJSON.stringify = stringify;
+ /**
+ * Serializes an object to an Extended JSON string, and reparse it as a JavaScript object.
+ *
+ * @param value - The object to serialize
+ * @param options - Optional settings passed to the `stringify` function
+ */
+ function serialize(value, options) {
+ options = options || {};
+ return JSON.parse(stringify(value, options));
+ }
+ EJSON.serialize = serialize;
+ /**
+ * Deserializes an Extended JSON object into a plain JavaScript object with native/BSON types
+ *
+ * @param ejson - The Extended JSON object to deserialize
+ * @param options - Optional settings passed to the parse method
+ */
+ function deserialize(ejson, options) {
+ options = options || {};
+ return parse(JSON.stringify(ejson), options);
+ }
+ EJSON.deserialize = deserialize;
+ })(exports.EJSON || (exports.EJSON = {}));
+
+ });
+
+ unwrapExports(extended_json);
+ extended_json.EJSON;
+ extended_json.isBSONType;
+
+ var map = createCommonjsModule(function (module, exports) {
+ /* eslint-disable @typescript-eslint/no-explicit-any */
+ // We have an ES6 Map available, return the native instance
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Map = void 0;
+ /** @public */
+ var bsonMap;
+ exports.Map = bsonMap;
+ var check = function (potentialGlobal) {
+ // eslint-disable-next-line eqeqeq
+ return potentialGlobal && potentialGlobal.Math == Math && potentialGlobal;
+ };
+ // https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+ function getGlobal() {
+ // eslint-disable-next-line no-undef
+ return (check(typeof globalThis === 'object' && globalThis) ||
+ check(typeof window === 'object' && window) ||
+ check(typeof self === 'object' && self) ||
+ check(typeof commonjsGlobal === 'object' && commonjsGlobal) ||
+ Function('return this')());
+ }
+ var bsonGlobal = getGlobal();
+ if (Object.prototype.hasOwnProperty.call(bsonGlobal, 'Map')) {
+ exports.Map = bsonMap = bsonGlobal.Map;
+ }
+ else {
+ // We will return a polyfill
+ exports.Map = bsonMap = /** @class */ (function () {
+ function Map(array) {
+ if (array === void 0) { array = []; }
+ this._keys = [];
+ this._values = {};
+ for (var i = 0; i < array.length; i++) {
+ if (array[i] == null)
+ continue; // skip null and undefined
+ var entry = array[i];
+ var key = entry[0];
+ var value = entry[1];
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ }
+ }
+ Map.prototype.clear = function () {
+ this._keys = [];
+ this._values = {};
+ };
+ Map.prototype.delete = function (key) {
+ var value = this._values[key];
+ if (value == null)
+ return false;
+ // Delete entry
+ delete this._values[key];
+ // Remove the key from the ordered keys list
+ this._keys.splice(value.i, 1);
+ return true;
+ };
+ Map.prototype.entries = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? [key, _this._values[key].v] : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Map.prototype.forEach = function (callback, self) {
+ self = self || this;
+ for (var i = 0; i < this._keys.length; i++) {
+ var key = this._keys[i];
+ // Call the forEach callback
+ callback.call(self, this._values[key].v, key, self);
+ }
+ };
+ Map.prototype.get = function (key) {
+ return this._values[key] ? this._values[key].v : undefined;
+ };
+ Map.prototype.has = function (key) {
+ return this._values[key] != null;
+ };
+ Map.prototype.keys = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? key : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Map.prototype.set = function (key, value) {
+ if (this._values[key]) {
+ this._values[key].v = value;
+ return this;
+ }
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ return this;
+ };
+ Map.prototype.values = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? _this._values[key].v : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Object.defineProperty(Map.prototype, "size", {
+ get: function () {
+ return this._keys.length;
+ },
+ enumerable: false,
+ configurable: true
+ });
+ return Map;
+ }());
+ }
+
+ });
+
+ unwrapExports(map);
+ map.Map;
+
+ var constants = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.BSON_BINARY_SUBTYPE_USER_DEFINED = exports.BSON_BINARY_SUBTYPE_MD5 = exports.BSON_BINARY_SUBTYPE_UUID_NEW = exports.BSON_BINARY_SUBTYPE_UUID = exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = exports.BSON_BINARY_SUBTYPE_FUNCTION = exports.BSON_BINARY_SUBTYPE_DEFAULT = exports.BSON_DATA_MAX_KEY = exports.BSON_DATA_MIN_KEY = exports.BSON_DATA_DECIMAL128 = exports.BSON_DATA_LONG = exports.BSON_DATA_TIMESTAMP = exports.BSON_DATA_INT = exports.BSON_DATA_CODE_W_SCOPE = exports.BSON_DATA_SYMBOL = exports.BSON_DATA_CODE = exports.BSON_DATA_DBPOINTER = exports.BSON_DATA_REGEXP = exports.BSON_DATA_NULL = exports.BSON_DATA_DATE = exports.BSON_DATA_BOOLEAN = exports.BSON_DATA_OID = exports.BSON_DATA_UNDEFINED = exports.BSON_DATA_BINARY = exports.BSON_DATA_ARRAY = exports.BSON_DATA_OBJECT = exports.BSON_DATA_STRING = exports.BSON_DATA_NUMBER = exports.JS_INT_MIN = exports.JS_INT_MAX = exports.BSON_INT64_MIN = exports.BSON_INT64_MAX = exports.BSON_INT32_MIN = exports.BSON_INT32_MAX = void 0;
+ /** @internal */
+ exports.BSON_INT32_MAX = 0x7fffffff;
+ /** @internal */
+ exports.BSON_INT32_MIN = -0x80000000;
+ /** @internal */
+ exports.BSON_INT64_MAX = Math.pow(2, 63) - 1;
+ /** @internal */
+ exports.BSON_INT64_MIN = -Math.pow(2, 63);
+ /**
+ * Any integer up to 2^53 can be precisely represented by a double.
+ * @internal
+ */
+ exports.JS_INT_MAX = Math.pow(2, 53);
+ /**
+ * Any integer down to -2^53 can be precisely represented by a double.
+ * @internal
+ */
+ exports.JS_INT_MIN = -Math.pow(2, 53);
+ /** Number BSON Type @internal */
+ exports.BSON_DATA_NUMBER = 1;
+ /** String BSON Type @internal */
+ exports.BSON_DATA_STRING = 2;
+ /** Object BSON Type @internal */
+ exports.BSON_DATA_OBJECT = 3;
+ /** Array BSON Type @internal */
+ exports.BSON_DATA_ARRAY = 4;
+ /** Binary BSON Type @internal */
+ exports.BSON_DATA_BINARY = 5;
+ /** Binary BSON Type @internal */
+ exports.BSON_DATA_UNDEFINED = 6;
+ /** ObjectId BSON Type @internal */
+ exports.BSON_DATA_OID = 7;
+ /** Boolean BSON Type @internal */
+ exports.BSON_DATA_BOOLEAN = 8;
+ /** Date BSON Type @internal */
+ exports.BSON_DATA_DATE = 9;
+ /** null BSON Type @internal */
+ exports.BSON_DATA_NULL = 10;
+ /** RegExp BSON Type @internal */
+ exports.BSON_DATA_REGEXP = 11;
+ /** Code BSON Type @internal */
+ exports.BSON_DATA_DBPOINTER = 12;
+ /** Code BSON Type @internal */
+ exports.BSON_DATA_CODE = 13;
+ /** Symbol BSON Type @internal */
+ exports.BSON_DATA_SYMBOL = 14;
+ /** Code with Scope BSON Type @internal */
+ exports.BSON_DATA_CODE_W_SCOPE = 15;
+ /** 32 bit Integer BSON Type @internal */
+ exports.BSON_DATA_INT = 16;
+ /** Timestamp BSON Type @internal */
+ exports.BSON_DATA_TIMESTAMP = 17;
+ /** Long BSON Type @internal */
+ exports.BSON_DATA_LONG = 18;
+ /** Decimal128 BSON Type @internal */
+ exports.BSON_DATA_DECIMAL128 = 19;
+ /** MinKey BSON Type @internal */
+ exports.BSON_DATA_MIN_KEY = 0xff;
+ /** MaxKey BSON Type @internal */
+ exports.BSON_DATA_MAX_KEY = 0x7f;
+ /** Binary Default Type @internal */
+ exports.BSON_BINARY_SUBTYPE_DEFAULT = 0;
+ /** Binary Function Type @internal */
+ exports.BSON_BINARY_SUBTYPE_FUNCTION = 1;
+ /** Binary Byte Array Type @internal */
+ exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
+ /** Binary Deprecated UUID Type @deprecated Please use BSON_BINARY_SUBTYPE_UUID_NEW @internal */
+ exports.BSON_BINARY_SUBTYPE_UUID = 3;
+ /** Binary UUID Type @internal */
+ exports.BSON_BINARY_SUBTYPE_UUID_NEW = 4;
+ /** Binary MD5 Type @internal */
+ exports.BSON_BINARY_SUBTYPE_MD5 = 5;
+ /** Binary User Defined Type @internal */
+ exports.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
+
+ });
+
+ unwrapExports(constants);
+ constants.BSON_BINARY_SUBTYPE_USER_DEFINED;
+ constants.BSON_BINARY_SUBTYPE_MD5;
+ constants.BSON_BINARY_SUBTYPE_UUID_NEW;
+ constants.BSON_BINARY_SUBTYPE_UUID;
+ constants.BSON_BINARY_SUBTYPE_BYTE_ARRAY;
+ constants.BSON_BINARY_SUBTYPE_FUNCTION;
+ constants.BSON_BINARY_SUBTYPE_DEFAULT;
+ constants.BSON_DATA_MAX_KEY;
+ constants.BSON_DATA_MIN_KEY;
+ constants.BSON_DATA_DECIMAL128;
+ constants.BSON_DATA_LONG;
+ constants.BSON_DATA_TIMESTAMP;
+ constants.BSON_DATA_INT;
+ constants.BSON_DATA_CODE_W_SCOPE;
+ constants.BSON_DATA_SYMBOL;
+ constants.BSON_DATA_CODE;
+ constants.BSON_DATA_DBPOINTER;
+ constants.BSON_DATA_REGEXP;
+ constants.BSON_DATA_NULL;
+ constants.BSON_DATA_DATE;
+ constants.BSON_DATA_BOOLEAN;
+ constants.BSON_DATA_OID;
+ constants.BSON_DATA_UNDEFINED;
+ constants.BSON_DATA_BINARY;
+ constants.BSON_DATA_ARRAY;
+ constants.BSON_DATA_OBJECT;
+ constants.BSON_DATA_STRING;
+ constants.BSON_DATA_NUMBER;
+ constants.JS_INT_MIN;
+ constants.JS_INT_MAX;
+ constants.BSON_INT64_MIN;
+ constants.BSON_INT64_MAX;
+ constants.BSON_INT32_MIN;
+ constants.BSON_INT32_MAX;
+
+ var calculate_size = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.calculateObjectSize = void 0;
+
+
+
+
+ function calculateObjectSize(object, serializeFunctions, ignoreUndefined) {
+ var totalLength = 4 + 1;
+ if (Array.isArray(object)) {
+ for (var i = 0; i < object.length; i++) {
+ totalLength += calculateElement(i.toString(), object[i], serializeFunctions, true, ignoreUndefined);
+ }
+ }
+ else {
+ // If we have toBSON defined, override the current object
+ if (object.toBSON) {
+ object = object.toBSON();
+ }
+ // Calculate size
+ for (var key in object) {
+ totalLength += calculateElement(key, object[key], serializeFunctions, false, ignoreUndefined);
+ }
+ }
+ return totalLength;
+ }
+ exports.calculateObjectSize = calculateObjectSize;
+ /** @internal */
+ function calculateElement(name,
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ value, serializeFunctions, isArray, ignoreUndefined) {
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (isArray === void 0) { isArray = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = false; }
+ // If we have toBSON defined, override the current object
+ if (value && value.toBSON) {
+ value = value.toBSON();
+ }
+ switch (typeof value) {
+ case 'string':
+ return 1 + buffer.Buffer.byteLength(name, 'utf8') + 1 + 4 + buffer.Buffer.byteLength(value, 'utf8') + 1;
+ case 'number':
+ if (Math.floor(value) === value &&
+ value >= constants.JS_INT_MIN &&
+ value <= constants.JS_INT_MAX) {
+ if (value >= constants.BSON_INT32_MIN && value <= constants.BSON_INT32_MAX) {
+ // 32 bit
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (4 + 1);
+ }
+ else {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ }
+ else {
+ // 64 bit
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ case 'undefined':
+ if (isArray || !ignoreUndefined)
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ return 0;
+ case 'boolean':
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 1);
+ case 'object':
+ if (value == null || value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (12 + 1);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ else if (ArrayBuffer.isView(value) ||
+ value instanceof ArrayBuffer ||
+ utils.isAnyArrayBuffer(value)) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 4 + 1) + value.byteLength);
+ }
+ else if (value['_bsontype'] === 'Long' ||
+ value['_bsontype'] === 'Double' ||
+ value['_bsontype'] === 'Timestamp') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ else if (value['_bsontype'] === 'Decimal128') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (16 + 1);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ // Calculate size depending on the availability of a scope
+ if (value.scope != null && Object.keys(value.scope).length > 0) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ buffer.Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined));
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ buffer.Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1);
+ }
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ // Check what kind of subtype we have
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ (value.position + 1 + 4 + 1 + 4));
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (value.position + 1 + 4 + 1));
+ }
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ buffer.Buffer.byteLength(value.value, 'utf8') +
+ 4 +
+ 1 +
+ 1);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ // Set up correct object for serialization
+ var ordered_values = Object.assign({
+ $ref: value.collection,
+ $id: value.oid
+ }, value.fields);
+ // Add db reference if it exists
+ if (value.db != null) {
+ ordered_values['$db'] = value.db;
+ }
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ calculateObjectSize(ordered_values, serializeFunctions, ignoreUndefined));
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.pattern, 'utf8') +
+ 1 +
+ buffer.Buffer.byteLength(value.options, 'utf8') +
+ 1);
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ calculateObjectSize(value, serializeFunctions, ignoreUndefined) +
+ 1);
+ }
+ case 'function':
+ // WTF for 0.4.X where typeof /someregexp/ === 'function'
+ if (value instanceof RegExp || utils.isRegExp(value) || String.call(value) === '[object RegExp]') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1);
+ }
+ else {
+ if (serializeFunctions && value.scope != null && Object.keys(value.scope).length > 0) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ buffer.Buffer.byteLength(utils.normalizedFunctionString(value), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined));
+ }
+ else if (serializeFunctions) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ buffer.Buffer.byteLength(utils.normalizedFunctionString(value), 'utf8') +
+ 1);
+ }
+ }
+ }
+ return 0;
+ }
+
+ });
+
+ unwrapExports(calculate_size);
+ calculate_size.calculateObjectSize;
+
+ var validate_utf8 = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.validateUtf8 = void 0;
+ var FIRST_BIT = 0x80;
+ var FIRST_TWO_BITS = 0xc0;
+ var FIRST_THREE_BITS = 0xe0;
+ var FIRST_FOUR_BITS = 0xf0;
+ var FIRST_FIVE_BITS = 0xf8;
+ var TWO_BIT_CHAR = 0xc0;
+ var THREE_BIT_CHAR = 0xe0;
+ var FOUR_BIT_CHAR = 0xf0;
+ var CONTINUING_CHAR = 0x80;
+ /**
+ * Determines if the passed in bytes are valid utf8
+ * @param bytes - An array of 8-bit bytes. Must be indexable and have length property
+ * @param start - The index to start validating
+ * @param end - The index to end validating
+ */
+ function validateUtf8(bytes, start, end) {
+ var continuation = 0;
+ for (var i = start; i < end; i += 1) {
+ var byte = bytes[i];
+ if (continuation) {
+ if ((byte & FIRST_TWO_BITS) !== CONTINUING_CHAR) {
+ return false;
+ }
+ continuation -= 1;
+ }
+ else if (byte & FIRST_BIT) {
+ if ((byte & FIRST_THREE_BITS) === TWO_BIT_CHAR) {
+ continuation = 1;
+ }
+ else if ((byte & FIRST_FOUR_BITS) === THREE_BIT_CHAR) {
+ continuation = 2;
+ }
+ else if ((byte & FIRST_FIVE_BITS) === FOUR_BIT_CHAR) {
+ continuation = 3;
+ }
+ else {
+ return false;
+ }
+ }
+ }
+ return !continuation;
+ }
+ exports.validateUtf8 = validateUtf8;
+
+ });
+
+ unwrapExports(validate_utf8);
+ validate_utf8.validateUtf8;
+
+ var deserializer = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.deserialize = void 0;
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ // Internal long versions
+ var JS_INT_MAX_LONG = long_1.Long.fromNumber(constants.JS_INT_MAX);
+ var JS_INT_MIN_LONG = long_1.Long.fromNumber(constants.JS_INT_MIN);
+ var functionCache = {};
+ function deserialize(buffer, options, isArray) {
+ options = options == null ? {} : options;
+ var index = options && options.index ? options.index : 0;
+ // Read the document size
+ var size = buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ if (size < 5) {
+ throw new Error("bson size must be >= 5, is " + size);
+ }
+ if (options.allowObjectSmallerThanBufferSize && buffer.length < size) {
+ throw new Error("buffer length " + buffer.length + " must be >= bson size " + size);
+ }
+ if (!options.allowObjectSmallerThanBufferSize && buffer.length !== size) {
+ throw new Error("buffer length " + buffer.length + " must === bson size " + size);
+ }
+ if (size + index > buffer.byteLength) {
+ throw new Error("(bson size " + size + " + options.index " + index + " must be <= buffer length " + buffer.byteLength + ")");
+ }
+ // Illegal end value
+ if (buffer[index + size - 1] !== 0) {
+ throw new Error("One object, sized correctly, with a spot for an EOO, but the EOO isn't 0x00");
+ }
+ // Start deserializtion
+ return deserializeObject(buffer, index, options, isArray);
+ }
+ exports.deserialize = deserialize;
+ function deserializeObject(buffer$1, index, options, isArray) {
+ if (isArray === void 0) { isArray = false; }
+ var evalFunctions = options['evalFunctions'] == null ? false : options['evalFunctions'];
+ var cacheFunctions = options['cacheFunctions'] == null ? false : options['cacheFunctions'];
+ var fieldsAsRaw = options['fieldsAsRaw'] == null ? null : options['fieldsAsRaw'];
+ // Return raw bson buffer instead of parsing it
+ var raw = options['raw'] == null ? false : options['raw'];
+ // Return BSONRegExp objects instead of native regular expressions
+ var bsonRegExp = typeof options['bsonRegExp'] === 'boolean' ? options['bsonRegExp'] : false;
+ // Controls the promotion of values vs wrapper classes
+ var promoteBuffers = options['promoteBuffers'] == null ? false : options['promoteBuffers'];
+ var promoteLongs = options['promoteLongs'] == null ? true : options['promoteLongs'];
+ var promoteValues = options['promoteValues'] == null ? true : options['promoteValues'];
+ // Set the start index
+ var startIndex = index;
+ // Validate that we have at least 4 bytes of buffer
+ if (buffer$1.length < 5)
+ throw new Error('corrupt bson message < 5 bytes long');
+ // Read the document size
+ var size = buffer$1[index++] | (buffer$1[index++] << 8) | (buffer$1[index++] << 16) | (buffer$1[index++] << 24);
+ // Ensure buffer is valid size
+ if (size < 5 || size > buffer$1.length)
+ throw new Error('corrupt bson message');
+ // Create holding object
+ var object = isArray ? [] : {};
+ // Used for arrays to skip having to perform utf8 decoding
+ var arrayIndex = 0;
+ var done = false;
+ // While we have more left data left keep parsing
+ while (!done) {
+ // Read the type
+ var elementType = buffer$1[index++];
+ // If we get a zero it's the last byte, exit
+ if (elementType === 0)
+ break;
+ // Get the start search index
+ var i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.byteLength)
+ throw new Error('Bad BSON Document: illegal CString');
+ var name = isArray ? arrayIndex++ : buffer$1.toString('utf8', index, i);
+ var value = void 0;
+ index = i + 1;
+ if (elementType === constants.BSON_DATA_STRING) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ if (!validate_utf8.validateUtf8(buffer$1, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ value = buffer$1.toString('utf8', index, index + stringSize - 1);
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_OID) {
+ var oid = buffer.Buffer.alloc(12);
+ buffer$1.copy(oid, 0, index, index + 12);
+ value = new objectid.ObjectId(oid);
+ index = index + 12;
+ }
+ else if (elementType === constants.BSON_DATA_INT && promoteValues === false) {
+ value = new int_32.Int32(buffer$1[index++] | (buffer$1[index++] << 8) | (buffer$1[index++] << 16) | (buffer$1[index++] << 24));
+ }
+ else if (elementType === constants.BSON_DATA_INT) {
+ value =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ }
+ else if (elementType === constants.BSON_DATA_NUMBER && promoteValues === false) {
+ value = new double_1.Double(buffer$1.readDoubleLE(index));
+ index = index + 8;
+ }
+ else if (elementType === constants.BSON_DATA_NUMBER) {
+ value = buffer$1.readDoubleLE(index);
+ index = index + 8;
+ }
+ else if (elementType === constants.BSON_DATA_DATE) {
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ value = new Date(new long_1.Long(lowBits, highBits).toNumber());
+ }
+ else if (elementType === constants.BSON_DATA_BOOLEAN) {
+ if (buffer$1[index] !== 0 && buffer$1[index] !== 1)
+ throw new Error('illegal boolean type value');
+ value = buffer$1[index++] === 1;
+ }
+ else if (elementType === constants.BSON_DATA_OBJECT) {
+ var _index = index;
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ if (objectSize <= 0 || objectSize > buffer$1.length - index)
+ throw new Error('bad embedded document length in bson');
+ // We have a raw value
+ if (raw) {
+ value = buffer$1.slice(index, index + objectSize);
+ }
+ else {
+ value = deserializeObject(buffer$1, _index, options, false);
+ }
+ index = index + objectSize;
+ }
+ else if (elementType === constants.BSON_DATA_ARRAY) {
+ var _index = index;
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ var arrayOptions = options;
+ // Stop index
+ var stopIndex = index + objectSize;
+ // All elements of array to be returned as raw bson
+ if (fieldsAsRaw && fieldsAsRaw[name]) {
+ arrayOptions = {};
+ for (var n in options) {
+ arrayOptions[n] = options[n];
+ }
+ arrayOptions['raw'] = true;
+ }
+ value = deserializeObject(buffer$1, _index, arrayOptions, true);
+ index = index + objectSize;
+ if (buffer$1[index - 1] !== 0)
+ throw new Error('invalid array terminator byte');
+ if (index !== stopIndex)
+ throw new Error('corrupted array bson');
+ }
+ else if (elementType === constants.BSON_DATA_UNDEFINED) {
+ value = undefined;
+ }
+ else if (elementType === constants.BSON_DATA_NULL) {
+ value = null;
+ }
+ else if (elementType === constants.BSON_DATA_LONG) {
+ // Unpack the low and high bits
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var long = new long_1.Long(lowBits, highBits);
+ // Promote the long if possible
+ if (promoteLongs && promoteValues === true) {
+ value =
+ long.lessThanOrEqual(JS_INT_MAX_LONG) && long.greaterThanOrEqual(JS_INT_MIN_LONG)
+ ? long.toNumber()
+ : long;
+ }
+ else {
+ value = long;
+ }
+ }
+ else if (elementType === constants.BSON_DATA_DECIMAL128) {
+ // Buffer to contain the decimal bytes
+ var bytes = buffer.Buffer.alloc(16);
+ // Copy the next 16 bytes into the bytes buffer
+ buffer$1.copy(bytes, 0, index, index + 16);
+ // Update index
+ index = index + 16;
+ // Assign the new Decimal128 value
+ var decimal128$1 = new decimal128.Decimal128(bytes);
+ // If we have an alternative mapper use that
+ if ('toObject' in decimal128$1 && typeof decimal128$1.toObject === 'function') {
+ value = decimal128$1.toObject();
+ }
+ else {
+ value = decimal128$1;
+ }
+ }
+ else if (elementType === constants.BSON_DATA_BINARY) {
+ var binarySize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var totalBinarySize = binarySize;
+ var subType = buffer$1[index++];
+ // Did we have a negative binary size, throw
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found');
+ // Is the length longer than the document
+ if (binarySize > buffer$1.byteLength)
+ throw new Error('Binary type size larger than document size');
+ // Decode as raw Buffer object if options specifies it
+ if (buffer$1['slice'] != null) {
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+ if (promoteBuffers && promoteValues) {
+ value = buffer$1.slice(index, index + binarySize);
+ }
+ else {
+ value = new binary.Binary(buffer$1.slice(index, index + binarySize), subType);
+ }
+ }
+ else {
+ var _buffer = buffer.Buffer.alloc(binarySize);
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+ // Copy the data
+ for (i = 0; i < binarySize; i++) {
+ _buffer[i] = buffer$1[index + i];
+ }
+ if (promoteBuffers && promoteValues) {
+ value = _buffer;
+ }
+ else {
+ value = new binary.Binary(_buffer, subType);
+ }
+ }
+ // Update the index
+ index = index + binarySize;
+ }
+ else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === false) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var source = buffer$1.toString('utf8', index, i);
+ // Create the regexp
+ index = i + 1;
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var regExpOptions = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // For each option add the corresponding one for javascript
+ var optionsArray = new Array(regExpOptions.length);
+ // Parse options
+ for (i = 0; i < regExpOptions.length; i++) {
+ switch (regExpOptions[i]) {
+ case 'm':
+ optionsArray[i] = 'm';
+ break;
+ case 's':
+ optionsArray[i] = 'g';
+ break;
+ case 'i':
+ optionsArray[i] = 'i';
+ break;
+ }
+ }
+ value = new RegExp(source, optionsArray.join(''));
+ }
+ else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === true) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var source = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var regExpOptions = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // Set the object
+ value = new regexp.BSONRegExp(source, regExpOptions);
+ }
+ else if (elementType === constants.BSON_DATA_SYMBOL) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ var symbol$1 = buffer$1.toString('utf8', index, index + stringSize - 1);
+ value = promoteValues ? symbol$1 : new symbol.BSONSymbol(symbol$1);
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_TIMESTAMP) {
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ value = new timestamp.Timestamp(lowBits, highBits);
+ }
+ else if (elementType === constants.BSON_DATA_MIN_KEY) {
+ value = new min_key.MinKey();
+ }
+ else if (elementType === constants.BSON_DATA_MAX_KEY) {
+ value = new max_key.MaxKey();
+ }
+ else if (elementType === constants.BSON_DATA_CODE) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ var functionString = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ }
+ else {
+ value = isolateEval(functionString);
+ }
+ }
+ else {
+ value = new code.Code(functionString);
+ }
+ // Update parse index position
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_CODE_W_SCOPE) {
+ var totalSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Element cannot be shorter than totalSize + stringSize + documentSize + terminator
+ if (totalSize < 4 + 4 + 4 + 1) {
+ throw new Error('code_w_scope total size shorter minimum expected length');
+ }
+ // Get the code string size
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Check if we have a valid string
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ // Javascript function
+ var functionString = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Parse the element
+ var _index = index;
+ // Decode the size of the object document
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ // Decode the scope object
+ var scopeObject = deserializeObject(buffer$1, _index, options, false);
+ // Adjust the index
+ index = index + objectSize;
+ // Check if field length is too short
+ if (totalSize < 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too short, truncating scope');
+ }
+ // Check if totalSize field is too long
+ if (totalSize > 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too long, clips outer document');
+ }
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ }
+ else {
+ value = isolateEval(functionString);
+ }
+ value.scope = scopeObject;
+ }
+ else {
+ value = new code.Code(functionString, scopeObject);
+ }
+ }
+ else if (elementType === constants.BSON_DATA_DBPOINTER) {
+ // Get the code string size
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Check if we have a valid string
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ // Namespace
+ if (!validate_utf8.validateUtf8(buffer$1, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ var namespace = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Read the oid
+ var oidBuffer = buffer.Buffer.alloc(12);
+ buffer$1.copy(oidBuffer, 0, index, index + 12);
+ var oid = new objectid.ObjectId(oidBuffer);
+ // Update the index
+ index = index + 12;
+ // Upgrade to DBRef type
+ value = new db_ref.DBRef(namespace, oid);
+ }
+ else {
+ throw new Error('Detected unknown BSON type ' + elementType.toString(16) + ' for fieldname "' + name + '"');
+ }
+ if (name === '__proto__') {
+ Object.defineProperty(object, name, {
+ value: value,
+ writable: true,
+ enumerable: true,
+ configurable: true
+ });
+ }
+ else {
+ object[name] = value;
+ }
+ }
+ // Check if the deserialization was against a valid array/object
+ if (size !== index - startIndex) {
+ if (isArray)
+ throw new Error('corrupt array bson');
+ throw new Error('corrupt object bson');
+ }
+ // check if object's $ keys are those of a DBRef
+ var dollarKeys = Object.keys(object).filter(function (k) { return k.startsWith('$'); });
+ var valid = true;
+ dollarKeys.forEach(function (k) {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1)
+ valid = false;
+ });
+ // if a $key not in "$ref", "$id", "$db", don't make a DBRef
+ if (!valid)
+ return object;
+ if (db_ref.isDBRefLike(object)) {
+ var copy = Object.assign({}, object);
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new db_ref.DBRef(object.$ref, object.$id, object.$db, copy);
+ }
+ return object;
+ }
+ /**
+ * Ensure eval is isolated, store the result in functionCache.
+ *
+ * @internal
+ */
+ function isolateEval(functionString, functionCache, object) {
+ if (!functionCache)
+ return new Function(functionString);
+ // Check for cache hit, eval if missing and return cached function
+ if (functionCache[functionString] == null) {
+ functionCache[functionString] = new Function(functionString);
+ }
+ // Set the object
+ return functionCache[functionString].bind(object);
+ }
+
+ });
+
+ unwrapExports(deserializer);
+ deserializer.deserialize;
+
+ var float_parser = createCommonjsModule(function (module, exports) {
+ // Copyright (c) 2008, Fair Oaks Labs, Inc.
+ // All rights reserved.
+ //
+ // Redistribution and use in source and binary forms, with or without
+ // modification, are permitted provided that the following conditions are met:
+ //
+ // * Redistributions of source code must retain the above copyright notice,
+ // this list of conditions and the following disclaimer.
+ //
+ // * Redistributions in binary form must reproduce the above copyright notice,
+ // this list of conditions and the following disclaimer in the documentation
+ // and/or other materials provided with the distribution.
+ //
+ // * Neither the name of Fair Oaks Labs, Inc. nor the names of its contributors
+ // may be used to endorse or promote products derived from this software
+ // without specific prior written permission.
+ //
+ // THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+ // AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+ // IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
+ // ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
+ // LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
+ // CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
+ // SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
+ // INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
+ // CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
+ // ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
+ // POSSIBILITY OF SUCH DAMAGE.
+ //
+ //
+ // Modifications to writeIEEE754 to support negative zeroes made by Brian White
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.writeIEEE754 = exports.readIEEE754 = void 0;
+ function readIEEE754(buffer, offset, endian, mLen, nBytes) {
+ var e;
+ var m;
+ var bBE = endian === 'big';
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var nBits = -7;
+ var i = bBE ? 0 : nBytes - 1;
+ var d = bBE ? 1 : -1;
+ var s = buffer[offset + i];
+ i += d;
+ e = s & ((1 << -nBits) - 1);
+ s >>= -nBits;
+ nBits += eLen;
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8)
+ ;
+ m = e & ((1 << -nBits) - 1);
+ e >>= -nBits;
+ nBits += mLen;
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8)
+ ;
+ if (e === 0) {
+ e = 1 - eBias;
+ }
+ else if (e === eMax) {
+ return m ? NaN : (s ? -1 : 1) * Infinity;
+ }
+ else {
+ m = m + Math.pow(2, mLen);
+ e = e - eBias;
+ }
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
+ }
+ exports.readIEEE754 = readIEEE754;
+ function writeIEEE754(buffer, value, offset, endian, mLen, nBytes) {
+ var e;
+ var m;
+ var c;
+ var bBE = endian === 'big';
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0;
+ var i = bBE ? nBytes - 1 : 0;
+ var d = bBE ? -1 : 1;
+ var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0;
+ value = Math.abs(value);
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0;
+ e = eMax;
+ }
+ else {
+ e = Math.floor(Math.log(value) / Math.LN2);
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--;
+ c *= 2;
+ }
+ if (e + eBias >= 1) {
+ value += rt / c;
+ }
+ else {
+ value += rt * Math.pow(2, 1 - eBias);
+ }
+ if (value * c >= 2) {
+ e++;
+ c /= 2;
+ }
+ if (e + eBias >= eMax) {
+ m = 0;
+ e = eMax;
+ }
+ else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen);
+ e = e + eBias;
+ }
+ else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
+ e = 0;
+ }
+ }
+ if (isNaN(value))
+ m = 0;
+ while (mLen >= 8) {
+ buffer[offset + i] = m & 0xff;
+ i += d;
+ m /= 256;
+ mLen -= 8;
+ }
+ e = (e << mLen) | m;
+ if (isNaN(value))
+ e += 8;
+ eLen += mLen;
+ while (eLen > 0) {
+ buffer[offset + i] = e & 0xff;
+ i += d;
+ e /= 256;
+ eLen -= 8;
+ }
+ buffer[offset + i - d] |= s * 128;
+ }
+ exports.writeIEEE754 = writeIEEE754;
+
+ });
+
+ unwrapExports(float_parser);
+ float_parser.writeIEEE754;
+ float_parser.readIEEE754;
+
+ var serializer = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.serializeInto = void 0;
+
+
+
+
+
+
+
+
+ var regexp = /\x00/; // eslint-disable-line no-control-regex
+ var ignoreKeys = new Set(['$db', '$ref', '$id', '$clusterTime']);
+ /*
+ * isArray indicates if we are writing to a BSON array (type 0x04)
+ * which forces the "key" which really an array index as a string to be written as ascii
+ * This will catch any errors in index as a string generation
+ */
+ function serializeString(buffer, key, value, index, isArray) {
+ // Encode String type
+ buffer[index++] = constants.BSON_DATA_STRING;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes + 1;
+ buffer[index - 1] = 0;
+ // Write the string
+ var size = buffer.write(value, index + 4, undefined, 'utf8');
+ // Write the size of the string to buffer
+ buffer[index + 3] = ((size + 1) >> 24) & 0xff;
+ buffer[index + 2] = ((size + 1) >> 16) & 0xff;
+ buffer[index + 1] = ((size + 1) >> 8) & 0xff;
+ buffer[index] = (size + 1) & 0xff;
+ // Update index
+ index = index + 4 + size;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+ }
+ function serializeNumber(buffer, key, value, index, isArray) {
+ // We have an integer value
+ // TODO(NODE-2529): Add support for big int
+ if (Number.isInteger(value) &&
+ value >= constants.BSON_INT32_MIN &&
+ value <= constants.BSON_INT32_MAX) {
+ // If the value fits in 32 bits encode as int32
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ }
+ else {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ float_parser.writeIEEE754(buffer, value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ }
+ return index;
+ }
+ function serializeNull(buffer, key, _, index, isArray) {
+ // Set long type
+ buffer[index++] = constants.BSON_DATA_NULL;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+ }
+ function serializeBoolean(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BOOLEAN;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Encode the boolean value
+ buffer[index++] = value ? 1 : 0;
+ return index;
+ }
+ function serializeDate(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_DATE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ var dateInMilis = long_1.Long.fromNumber(value.getTime());
+ var lowBits = dateInMilis.getLowBits();
+ var highBits = dateInMilis.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+ }
+ function serializeRegExp(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ if (value.source && value.source.match(regexp) != null) {
+ throw Error('value ' + value.source + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.source, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the parameters
+ if (value.ignoreCase)
+ buffer[index++] = 0x69; // i
+ if (value.global)
+ buffer[index++] = 0x73; // s
+ if (value.multiline)
+ buffer[index++] = 0x6d; // m
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+ }
+ function serializeBSONRegExp(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Check the pattern for 0 bytes
+ if (value.pattern.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('pattern ' + value.pattern + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.pattern, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the options
+ index = index + buffer.write(value.options.split('').sort().join(''), index, undefined, 'utf8');
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+ }
+ function serializeMinMax(buffer, key, value, index, isArray) {
+ // Write the type of either min or max key
+ if (value === null) {
+ buffer[index++] = constants.BSON_DATA_NULL;
+ }
+ else if (value._bsontype === 'MinKey') {
+ buffer[index++] = constants.BSON_DATA_MIN_KEY;
+ }
+ else {
+ buffer[index++] = constants.BSON_DATA_MAX_KEY;
+ }
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+ }
+ function serializeObjectId(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OID;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the objectId into the shared buffer
+ if (typeof value.id === 'string') {
+ buffer.write(value.id, index, undefined, 'binary');
+ }
+ else if (utils.isUint8Array(value.id)) {
+ // Use the standard JS methods here because buffer.copy() is buggy with the
+ // browser polyfill
+ buffer.set(value.id.subarray(0, 12), index);
+ }
+ else {
+ throw new TypeError('object [' + JSON.stringify(value) + '] is not a valid ObjectId');
+ }
+ // Adjust index
+ return index + 12;
+ }
+ function serializeBuffer(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Get size of the buffer (current write point)
+ var size = value.length;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the default subtype
+ buffer[index++] = constants.BSON_BINARY_SUBTYPE_DEFAULT;
+ // Copy the content form the binary field to the buffer
+ buffer.set(ensure_buffer.ensureBuffer(value), index);
+ // Adjust the index
+ index = index + size;
+ return index;
+ }
+ function serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray, path) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (isArray === void 0) { isArray = false; }
+ if (path === void 0) { path = []; }
+ for (var i = 0; i < path.length; i++) {
+ if (path[i] === value)
+ throw new Error('cyclic dependency detected');
+ }
+ // Push value to stack
+ path.push(value);
+ // Write the type
+ buffer[index++] = Array.isArray(value) ? constants.BSON_DATA_ARRAY : constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ var endIndex = serializeInto(buffer, value, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined, path);
+ // Pop stack
+ path.pop();
+ return endIndex;
+ }
+ function serializeDecimal128(buffer, key, value, index, isArray) {
+ buffer[index++] = constants.BSON_DATA_DECIMAL128;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the data from the value
+ // Prefer the standard JS methods because their typechecking is not buggy,
+ // unlike the `buffer` polyfill's.
+ buffer.set(value.bytes.subarray(0, 16), index);
+ return index + 16;
+ }
+ function serializeLong(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] =
+ value._bsontype === 'Long' ? constants.BSON_DATA_LONG : constants.BSON_DATA_TIMESTAMP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ var lowBits = value.getLowBits();
+ var highBits = value.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+ }
+ function serializeInt32(buffer, key, value, index, isArray) {
+ value = value.valueOf();
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ return index;
+ }
+ function serializeDouble(buffer, key, value, index, isArray) {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ float_parser.writeIEEE754(buffer, value.value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ return index;
+ }
+ function serializeFunction(buffer, key, value, index, _checkKeys, _depth, isArray) {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ var functionString = utils.normalizedFunctionString(value);
+ // Write the string
+ var size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+ }
+ function serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (isArray === void 0) { isArray = false; }
+ if (value.scope && typeof value.scope === 'object') {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_CODE_W_SCOPE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Starting index
+ var startIndex = index;
+ // Serialize the function
+ // Get the function string
+ var functionString = typeof value.code === 'string' ? value.code : value.code.toString();
+ // Index adjustment
+ index = index + 4;
+ // Write string into buffer
+ var codeSize = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = codeSize & 0xff;
+ buffer[index + 1] = (codeSize >> 8) & 0xff;
+ buffer[index + 2] = (codeSize >> 16) & 0xff;
+ buffer[index + 3] = (codeSize >> 24) & 0xff;
+ // Write end 0
+ buffer[index + 4 + codeSize - 1] = 0;
+ // Write the
+ index = index + codeSize + 4;
+ //
+ // Serialize the scope value
+ var endIndex = serializeInto(buffer, value.scope, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined);
+ index = endIndex - 1;
+ // Writ the total
+ var totalSize = endIndex - startIndex;
+ // Write the total size of the object
+ buffer[startIndex++] = totalSize & 0xff;
+ buffer[startIndex++] = (totalSize >> 8) & 0xff;
+ buffer[startIndex++] = (totalSize >> 16) & 0xff;
+ buffer[startIndex++] = (totalSize >> 24) & 0xff;
+ // Write trailing zero
+ buffer[index++] = 0;
+ }
+ else {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ var functionString = value.code.toString();
+ // Write the string
+ var size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ }
+ return index;
+ }
+ function serializeBinary(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Extract the buffer
+ var data = value.value(true);
+ // Calculate size
+ var size = value.position;
+ // Add the deprecated 02 type 4 bytes of size to total
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY)
+ size = size + 4;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the subtype to the buffer
+ buffer[index++] = value.sub_type;
+ // If we have binary type 2 the 4 first bytes are the size
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ size = size - 4;
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ }
+ // Write the data to the object
+ buffer.set(data, index);
+ // Adjust the index
+ index = index + value.position;
+ return index;
+ }
+ function serializeSymbol(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_SYMBOL;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the string
+ var size = buffer.write(value.value, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0x00;
+ return index;
+ }
+ function serializeDBRef(buffer, key, value, index, depth, serializeFunctions, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ var startIndex = index;
+ var output = {
+ $ref: value.collection || value.namespace,
+ $id: value.oid
+ };
+ if (value.db != null) {
+ output.$db = value.db;
+ }
+ output = Object.assign(output, value.fields);
+ var endIndex = serializeInto(buffer, output, false, index, depth + 1, serializeFunctions);
+ // Calculate object size
+ var size = endIndex - startIndex;
+ // Write the size
+ buffer[startIndex++] = size & 0xff;
+ buffer[startIndex++] = (size >> 8) & 0xff;
+ buffer[startIndex++] = (size >> 16) & 0xff;
+ buffer[startIndex++] = (size >> 24) & 0xff;
+ // Set index
+ return endIndex;
+ }
+ function serializeInto(buffer, object, checkKeys, startingIndex, depth, serializeFunctions, ignoreUndefined, path) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (startingIndex === void 0) { startingIndex = 0; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (path === void 0) { path = []; }
+ startingIndex = startingIndex || 0;
+ path = path || [];
+ // Push the object to the path
+ path.push(object);
+ // Start place to serialize into
+ var index = startingIndex + 4;
+ // Special case isArray
+ if (Array.isArray(object)) {
+ // Get object keys
+ for (var i = 0; i < object.length; i++) {
+ var key = '' + i;
+ var value = object[i];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+ if (typeof value === 'string') {
+ index = serializeString(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'number') {
+ index = serializeNumber(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (typeof value === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index, true);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index, true);
+ }
+ else if (value === undefined) {
+ index = serializeNull(buffer, key, value, index, true);
+ }
+ else if (value === null) {
+ index = serializeNull(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index, true);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index, true);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true, path);
+ }
+ else if (typeof value === 'object' &&
+ extended_json.isBSONType(value) &&
+ value._bsontype === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, true);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions, true);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index, true);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ else if (object instanceof map.Map || utils.isMap(object)) {
+ var iterator = object.entries();
+ var done = false;
+ while (!done) {
+ // Unpack the next entry
+ var entry = iterator.next();
+ done = !!entry.done;
+ // Are we done, then skip and terminate
+ if (done)
+ continue;
+ // Get the entry values
+ var key = entry.value[0];
+ var value = entry.value[1];
+ // Check the type of the value
+ var type = typeof value;
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ }
+ else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ }
+ else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ }
+ else if (type === 'bigint' || utils.isBigInt64Array(value) || utils.isBigUInt64Array(value)) {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ }
+ else if (value === null || (value === undefined && ignoreUndefined === false)) {
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ }
+ else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
+ }
+ else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ else {
+ // Did we provide a custom serialization method
+ if (object.toBSON) {
+ if (typeof object.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ object = object.toBSON();
+ if (object != null && typeof object !== 'object')
+ throw new TypeError('toBSON function did not return an object');
+ }
+ // Iterate over all the keys
+ for (var key in object) {
+ var value = object[key];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+ // Check the type of the value
+ var type = typeof value;
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ }
+ else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ }
+ else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ }
+ else if (type === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ }
+ else if (value === undefined) {
+ if (ignoreUndefined === false)
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value === null) {
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ }
+ else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
+ }
+ else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ // Remove the path
+ path.pop();
+ // Final padding byte for object
+ buffer[index++] = 0x00;
+ // Final size
+ var size = index - startingIndex;
+ // Write the size of the object
+ buffer[startingIndex++] = size & 0xff;
+ buffer[startingIndex++] = (size >> 8) & 0xff;
+ buffer[startingIndex++] = (size >> 16) & 0xff;
+ buffer[startingIndex++] = (size >> 24) & 0xff;
+ return index;
+ }
+ exports.serializeInto = serializeInto;
+
+ });
+
+ unwrapExports(serializer);
+ serializer.serializeInto;
+
+ var bson = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.ObjectID = exports.Decimal128 = exports.BSONRegExp = exports.MaxKey = exports.MinKey = exports.Int32 = exports.Double = exports.Timestamp = exports.Long = exports.UUID = exports.ObjectId = exports.Binary = exports.DBRef = exports.BSONSymbol = exports.Map = exports.Code = exports.LongWithoutOverridesClass = exports.EJSON = exports.BSON_INT64_MIN = exports.BSON_INT64_MAX = exports.BSON_INT32_MIN = exports.BSON_INT32_MAX = exports.BSON_DATA_UNDEFINED = exports.BSON_DATA_TIMESTAMP = exports.BSON_DATA_SYMBOL = exports.BSON_DATA_STRING = exports.BSON_DATA_REGEXP = exports.BSON_DATA_OID = exports.BSON_DATA_OBJECT = exports.BSON_DATA_NUMBER = exports.BSON_DATA_NULL = exports.BSON_DATA_MIN_KEY = exports.BSON_DATA_MAX_KEY = exports.BSON_DATA_LONG = exports.BSON_DATA_INT = exports.BSON_DATA_DECIMAL128 = exports.BSON_DATA_DBPOINTER = exports.BSON_DATA_DATE = exports.BSON_DATA_CODE_W_SCOPE = exports.BSON_DATA_CODE = exports.BSON_DATA_BOOLEAN = exports.BSON_DATA_BINARY = exports.BSON_DATA_ARRAY = exports.BSON_BINARY_SUBTYPE_UUID_NEW = exports.BSON_BINARY_SUBTYPE_UUID = exports.BSON_BINARY_SUBTYPE_USER_DEFINED = exports.BSON_BINARY_SUBTYPE_MD5 = exports.BSON_BINARY_SUBTYPE_FUNCTION = exports.BSON_BINARY_SUBTYPE_DEFAULT = exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = void 0;
+ exports.deserializeStream = exports.calculateObjectSize = exports.deserialize = exports.serializeWithBufferAndIndex = exports.serialize = exports.setInternalBufferSize = void 0;
+
+
+ Object.defineProperty(exports, "Binary", { enumerable: true, get: function () { return binary.Binary; } });
+
+ Object.defineProperty(exports, "Code", { enumerable: true, get: function () { return code.Code; } });
+
+ Object.defineProperty(exports, "DBRef", { enumerable: true, get: function () { return db_ref.DBRef; } });
+
+ Object.defineProperty(exports, "Decimal128", { enumerable: true, get: function () { return decimal128.Decimal128; } });
+
+ Object.defineProperty(exports, "Double", { enumerable: true, get: function () { return double_1.Double; } });
+
+
+
+ Object.defineProperty(exports, "Int32", { enumerable: true, get: function () { return int_32.Int32; } });
+
+ Object.defineProperty(exports, "Long", { enumerable: true, get: function () { return long_1.Long; } });
+
+ Object.defineProperty(exports, "Map", { enumerable: true, get: function () { return map.Map; } });
+
+ Object.defineProperty(exports, "MaxKey", { enumerable: true, get: function () { return max_key.MaxKey; } });
+
+ Object.defineProperty(exports, "MinKey", { enumerable: true, get: function () { return min_key.MinKey; } });
+
+ Object.defineProperty(exports, "ObjectId", { enumerable: true, get: function () { return objectid.ObjectId; } });
+ Object.defineProperty(exports, "ObjectID", { enumerable: true, get: function () { return objectid.ObjectId; } });
+
+ // Parts of the parser
+
+
+
+ Object.defineProperty(exports, "BSONRegExp", { enumerable: true, get: function () { return regexp.BSONRegExp; } });
+
+ Object.defineProperty(exports, "BSONSymbol", { enumerable: true, get: function () { return symbol.BSONSymbol; } });
+
+ Object.defineProperty(exports, "Timestamp", { enumerable: true, get: function () { return timestamp.Timestamp; } });
+
+ Object.defineProperty(exports, "UUID", { enumerable: true, get: function () { return uuid.UUID; } });
+
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_BYTE_ARRAY", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_BYTE_ARRAY; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_DEFAULT", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_DEFAULT; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_FUNCTION", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_FUNCTION; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_MD5", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_MD5; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_USER_DEFINED", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_USER_DEFINED; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_UUID", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_UUID; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_UUID_NEW", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_UUID_NEW; } });
+ Object.defineProperty(exports, "BSON_DATA_ARRAY", { enumerable: true, get: function () { return constants.BSON_DATA_ARRAY; } });
+ Object.defineProperty(exports, "BSON_DATA_BINARY", { enumerable: true, get: function () { return constants.BSON_DATA_BINARY; } });
+ Object.defineProperty(exports, "BSON_DATA_BOOLEAN", { enumerable: true, get: function () { return constants.BSON_DATA_BOOLEAN; } });
+ Object.defineProperty(exports, "BSON_DATA_CODE", { enumerable: true, get: function () { return constants.BSON_DATA_CODE; } });
+ Object.defineProperty(exports, "BSON_DATA_CODE_W_SCOPE", { enumerable: true, get: function () { return constants.BSON_DATA_CODE_W_SCOPE; } });
+ Object.defineProperty(exports, "BSON_DATA_DATE", { enumerable: true, get: function () { return constants.BSON_DATA_DATE; } });
+ Object.defineProperty(exports, "BSON_DATA_DBPOINTER", { enumerable: true, get: function () { return constants.BSON_DATA_DBPOINTER; } });
+ Object.defineProperty(exports, "BSON_DATA_DECIMAL128", { enumerable: true, get: function () { return constants.BSON_DATA_DECIMAL128; } });
+ Object.defineProperty(exports, "BSON_DATA_INT", { enumerable: true, get: function () { return constants.BSON_DATA_INT; } });
+ Object.defineProperty(exports, "BSON_DATA_LONG", { enumerable: true, get: function () { return constants.BSON_DATA_LONG; } });
+ Object.defineProperty(exports, "BSON_DATA_MAX_KEY", { enumerable: true, get: function () { return constants.BSON_DATA_MAX_KEY; } });
+ Object.defineProperty(exports, "BSON_DATA_MIN_KEY", { enumerable: true, get: function () { return constants.BSON_DATA_MIN_KEY; } });
+ Object.defineProperty(exports, "BSON_DATA_NULL", { enumerable: true, get: function () { return constants.BSON_DATA_NULL; } });
+ Object.defineProperty(exports, "BSON_DATA_NUMBER", { enumerable: true, get: function () { return constants.BSON_DATA_NUMBER; } });
+ Object.defineProperty(exports, "BSON_DATA_OBJECT", { enumerable: true, get: function () { return constants.BSON_DATA_OBJECT; } });
+ Object.defineProperty(exports, "BSON_DATA_OID", { enumerable: true, get: function () { return constants.BSON_DATA_OID; } });
+ Object.defineProperty(exports, "BSON_DATA_REGEXP", { enumerable: true, get: function () { return constants.BSON_DATA_REGEXP; } });
+ Object.defineProperty(exports, "BSON_DATA_STRING", { enumerable: true, get: function () { return constants.BSON_DATA_STRING; } });
+ Object.defineProperty(exports, "BSON_DATA_SYMBOL", { enumerable: true, get: function () { return constants.BSON_DATA_SYMBOL; } });
+ Object.defineProperty(exports, "BSON_DATA_TIMESTAMP", { enumerable: true, get: function () { return constants.BSON_DATA_TIMESTAMP; } });
+ Object.defineProperty(exports, "BSON_DATA_UNDEFINED", { enumerable: true, get: function () { return constants.BSON_DATA_UNDEFINED; } });
+ Object.defineProperty(exports, "BSON_INT32_MAX", { enumerable: true, get: function () { return constants.BSON_INT32_MAX; } });
+ Object.defineProperty(exports, "BSON_INT32_MIN", { enumerable: true, get: function () { return constants.BSON_INT32_MIN; } });
+ Object.defineProperty(exports, "BSON_INT64_MAX", { enumerable: true, get: function () { return constants.BSON_INT64_MAX; } });
+ Object.defineProperty(exports, "BSON_INT64_MIN", { enumerable: true, get: function () { return constants.BSON_INT64_MIN; } });
+ var extended_json_2 = extended_json;
+ Object.defineProperty(exports, "EJSON", { enumerable: true, get: function () { return extended_json_2.EJSON; } });
+ var timestamp_2 = timestamp;
+ Object.defineProperty(exports, "LongWithoutOverridesClass", { enumerable: true, get: function () { return timestamp_2.LongWithoutOverridesClass; } });
+ /** @internal */
+ // Default Max Size
+ var MAXSIZE = 1024 * 1024 * 17;
+ // Current Internal Temporary Serialization Buffer
+ var buffer$1 = buffer.Buffer.alloc(MAXSIZE);
+ /**
+ * Sets the size of the internal serialization buffer.
+ *
+ * @param size - The desired size for the internal serialization buffer
+ * @public
+ */
+ function setInternalBufferSize(size) {
+ // Resize the internal serialization buffer if needed
+ if (buffer$1.length < size) {
+ buffer$1 = buffer.Buffer.alloc(size);
+ }
+ }
+ exports.setInternalBufferSize = setInternalBufferSize;
+ /**
+ * Serialize a Javascript object.
+ *
+ * @param object - the Javascript object to serialize.
+ * @returns Buffer object containing the serialized object.
+ * @public
+ */
+ function serialize(object, options) {
+ if (options === void 0) { options = {}; }
+ // Unpack the options
+ var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ var minInternalBufferSize = typeof options.minInternalBufferSize === 'number' ? options.minInternalBufferSize : MAXSIZE;
+ // Resize the internal serialization buffer if needed
+ if (buffer$1.length < minInternalBufferSize) {
+ buffer$1 = buffer.Buffer.alloc(minInternalBufferSize);
+ }
+ // Attempt to serialize
+ var serializationIndex = serializer.serializeInto(buffer$1, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined, []);
+ // Create the final buffer
+ var finishedBuffer = buffer.Buffer.alloc(serializationIndex);
+ // Copy into the finished buffer
+ buffer$1.copy(finishedBuffer, 0, 0, finishedBuffer.length);
+ // Return the buffer
+ return finishedBuffer;
+ }
+ exports.serialize = serialize;
+ /**
+ * Serialize a Javascript object using a predefined Buffer and index into the buffer,
+ * useful when pre-allocating the space for serialization.
+ *
+ * @param object - the Javascript object to serialize.
+ * @param finalBuffer - the Buffer you pre-allocated to store the serialized BSON object.
+ * @returns the index pointing to the last written byte in the buffer.
+ * @public
+ */
+ function serializeWithBufferAndIndex(object, finalBuffer, options) {
+ if (options === void 0) { options = {}; }
+ // Unpack the options
+ var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ var startIndex = typeof options.index === 'number' ? options.index : 0;
+ // Attempt to serialize
+ var serializationIndex = serializer.serializeInto(buffer$1, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined);
+ buffer$1.copy(finalBuffer, startIndex, 0, serializationIndex);
+ // Return the index
+ return startIndex + serializationIndex - 1;
+ }
+ exports.serializeWithBufferAndIndex = serializeWithBufferAndIndex;
+ /**
+ * Deserialize data as BSON.
+ *
+ * @param buffer - the buffer containing the serialized set of BSON documents.
+ * @returns returns the deserialized Javascript Object.
+ * @public
+ */
+ function deserialize(buffer, options) {
+ if (options === void 0) { options = {}; }
+ return deserializer.deserialize(ensure_buffer.ensureBuffer(buffer), options);
+ }
+ exports.deserialize = deserialize;
+ /**
+ * Calculate the bson size for a passed in Javascript object.
+ *
+ * @param object - the Javascript object to calculate the BSON byte size for
+ * @returns size of BSON object in bytes
+ * @public
+ */
+ function calculateObjectSize(object, options) {
+ if (options === void 0) { options = {}; }
+ options = options || {};
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ return calculate_size.calculateObjectSize(object, serializeFunctions, ignoreUndefined);
+ }
+ exports.calculateObjectSize = calculateObjectSize;
+ /**
+ * Deserialize stream data as BSON documents.
+ *
+ * @param data - the buffer containing the serialized set of BSON documents.
+ * @param startIndex - the start index in the data Buffer where the deserialization is to start.
+ * @param numberOfDocuments - number of documents to deserialize.
+ * @param documents - an array where to store the deserialized documents.
+ * @param docStartIndex - the index in the documents array from where to start inserting documents.
+ * @param options - additional options used for the deserialization.
+ * @returns next index in the buffer after deserialization **x** numbers of documents.
+ * @public
+ */
+ function deserializeStream(data, startIndex, numberOfDocuments, documents, docStartIndex, options) {
+ var internalOptions = Object.assign({ allowObjectSmallerThanBufferSize: true, index: 0 }, options);
+ var bufferData = ensure_buffer.ensureBuffer(data);
+ var index = startIndex;
+ // Loop over all documents
+ for (var i = 0; i < numberOfDocuments; i++) {
+ // Find size of the document
+ var size = bufferData[index] |
+ (bufferData[index + 1] << 8) |
+ (bufferData[index + 2] << 16) |
+ (bufferData[index + 3] << 24);
+ // Update options with index
+ internalOptions.index = index;
+ // Parse the document at this point
+ documents[docStartIndex + i] = deserializer.deserialize(bufferData, internalOptions);
+ // Adjust index by the document size
+ index = index + size;
+ }
+ // Return object containing end index of parsing and list of documents
+ return index;
+ }
+ exports.deserializeStream = deserializeStream;
+ /**
+ * BSON default export
+ * @deprecated Please use named exports
+ * @privateRemarks
+ * We want to someday deprecate the default export,
+ * so none of the new TS types are being exported on the default
+ * @public
+ */
+ var BSON = {
+ Binary: binary.Binary,
+ Code: code.Code,
+ DBRef: db_ref.DBRef,
+ Decimal128: decimal128.Decimal128,
+ Double: double_1.Double,
+ Int32: int_32.Int32,
+ Long: long_1.Long,
+ UUID: uuid.UUID,
+ Map: map.Map,
+ MaxKey: max_key.MaxKey,
+ MinKey: min_key.MinKey,
+ ObjectId: objectid.ObjectId,
+ ObjectID: objectid.ObjectId,
+ BSONRegExp: regexp.BSONRegExp,
+ BSONSymbol: symbol.BSONSymbol,
+ Timestamp: timestamp.Timestamp,
+ EJSON: extended_json.EJSON,
+ setInternalBufferSize: setInternalBufferSize,
+ serialize: serialize,
+ serializeWithBufferAndIndex: serializeWithBufferAndIndex,
+ deserialize: deserialize,
+ calculateObjectSize: calculateObjectSize,
+ deserializeStream: deserializeStream
+ };
+ exports.default = BSON;
+
+ });
+
+ var bson$1 = unwrapExports(bson);
+ var bson_1 = bson.ObjectID;
+ var bson_2 = bson.Decimal128;
+ var bson_3 = bson.BSONRegExp;
+ var bson_4 = bson.MaxKey;
+ var bson_5 = bson.MinKey;
+ var bson_6 = bson.Int32;
+ var bson_7 = bson.Double;
+ var bson_8 = bson.Timestamp;
+ var bson_9 = bson.Long;
+ var bson_10 = bson.UUID;
+ var bson_11 = bson.ObjectId;
+ var bson_12 = bson.Binary;
+ var bson_13 = bson.DBRef;
+ var bson_14 = bson.BSONSymbol;
+ var bson_15 = bson.Map;
+ var bson_16 = bson.Code;
+ var bson_17 = bson.LongWithoutOverridesClass;
+ var bson_18 = bson.EJSON;
+ var bson_19 = bson.BSON_INT64_MIN;
+ var bson_20 = bson.BSON_INT64_MAX;
+ var bson_21 = bson.BSON_INT32_MIN;
+ var bson_22 = bson.BSON_INT32_MAX;
+ var bson_23 = bson.BSON_DATA_UNDEFINED;
+ var bson_24 = bson.BSON_DATA_TIMESTAMP;
+ var bson_25 = bson.BSON_DATA_SYMBOL;
+ var bson_26 = bson.BSON_DATA_STRING;
+ var bson_27 = bson.BSON_DATA_REGEXP;
+ var bson_28 = bson.BSON_DATA_OID;
+ var bson_29 = bson.BSON_DATA_OBJECT;
+ var bson_30 = bson.BSON_DATA_NUMBER;
+ var bson_31 = bson.BSON_DATA_NULL;
+ var bson_32 = bson.BSON_DATA_MIN_KEY;
+ var bson_33 = bson.BSON_DATA_MAX_KEY;
+ var bson_34 = bson.BSON_DATA_LONG;
+ var bson_35 = bson.BSON_DATA_INT;
+ var bson_36 = bson.BSON_DATA_DECIMAL128;
+ var bson_37 = bson.BSON_DATA_DBPOINTER;
+ var bson_38 = bson.BSON_DATA_DATE;
+ var bson_39 = bson.BSON_DATA_CODE_W_SCOPE;
+ var bson_40 = bson.BSON_DATA_CODE;
+ var bson_41 = bson.BSON_DATA_BOOLEAN;
+ var bson_42 = bson.BSON_DATA_BINARY;
+ var bson_43 = bson.BSON_DATA_ARRAY;
+ var bson_44 = bson.BSON_BINARY_SUBTYPE_UUID_NEW;
+ var bson_45 = bson.BSON_BINARY_SUBTYPE_UUID;
+ var bson_46 = bson.BSON_BINARY_SUBTYPE_USER_DEFINED;
+ var bson_47 = bson.BSON_BINARY_SUBTYPE_MD5;
+ var bson_48 = bson.BSON_BINARY_SUBTYPE_FUNCTION;
+ var bson_49 = bson.BSON_BINARY_SUBTYPE_DEFAULT;
+ var bson_50 = bson.BSON_BINARY_SUBTYPE_BYTE_ARRAY;
+ var bson_51 = bson.deserializeStream;
+ var bson_52 = bson.calculateObjectSize;
+ var bson_53 = bson.deserialize;
+ var bson_54 = bson.serializeWithBufferAndIndex;
+ var bson_55 = bson.serialize;
+ var bson_56 = bson.setInternalBufferSize;
+
+ exports.BSONRegExp = bson_3;
+ exports.BSONSymbol = bson_14;
+ exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = bson_50;
+ exports.BSON_BINARY_SUBTYPE_DEFAULT = bson_49;
+ exports.BSON_BINARY_SUBTYPE_FUNCTION = bson_48;
+ exports.BSON_BINARY_SUBTYPE_MD5 = bson_47;
+ exports.BSON_BINARY_SUBTYPE_USER_DEFINED = bson_46;
+ exports.BSON_BINARY_SUBTYPE_UUID = bson_45;
+ exports.BSON_BINARY_SUBTYPE_UUID_NEW = bson_44;
+ exports.BSON_DATA_ARRAY = bson_43;
+ exports.BSON_DATA_BINARY = bson_42;
+ exports.BSON_DATA_BOOLEAN = bson_41;
+ exports.BSON_DATA_CODE = bson_40;
+ exports.BSON_DATA_CODE_W_SCOPE = bson_39;
+ exports.BSON_DATA_DATE = bson_38;
+ exports.BSON_DATA_DBPOINTER = bson_37;
+ exports.BSON_DATA_DECIMAL128 = bson_36;
+ exports.BSON_DATA_INT = bson_35;
+ exports.BSON_DATA_LONG = bson_34;
+ exports.BSON_DATA_MAX_KEY = bson_33;
+ exports.BSON_DATA_MIN_KEY = bson_32;
+ exports.BSON_DATA_NULL = bson_31;
+ exports.BSON_DATA_NUMBER = bson_30;
+ exports.BSON_DATA_OBJECT = bson_29;
+ exports.BSON_DATA_OID = bson_28;
+ exports.BSON_DATA_REGEXP = bson_27;
+ exports.BSON_DATA_STRING = bson_26;
+ exports.BSON_DATA_SYMBOL = bson_25;
+ exports.BSON_DATA_TIMESTAMP = bson_24;
+ exports.BSON_DATA_UNDEFINED = bson_23;
+ exports.BSON_INT32_MAX = bson_22;
+ exports.BSON_INT32_MIN = bson_21;
+ exports.BSON_INT64_MAX = bson_20;
+ exports.BSON_INT64_MIN = bson_19;
+ exports.Binary = bson_12;
+ exports.Code = bson_16;
+ exports.DBRef = bson_13;
+ exports.Decimal128 = bson_2;
+ exports.Double = bson_7;
+ exports.EJSON = bson_18;
+ exports.Int32 = bson_6;
+ exports.Long = bson_9;
+ exports.LongWithoutOverridesClass = bson_17;
+ exports.Map = bson_15;
+ exports.MaxKey = bson_4;
+ exports.MinKey = bson_5;
+ exports.ObjectID = bson_1;
+ exports.ObjectId = bson_11;
+ exports.Timestamp = bson_8;
+ exports.UUID = bson_10;
+ exports.calculateObjectSize = bson_52;
+ exports.default = bson$1;
+ exports.deserialize = bson_53;
+ exports.deserializeStream = bson_51;
+ exports.serialize = bson_55;
+ exports.serializeWithBufferAndIndex = bson_54;
+ exports.setInternalBufferSize = bson_56;
+
+ Object.defineProperty(exports, '__esModule', { value: true });
+
+})));
+//# sourceMappingURL=bson.browser.umd.js.map
diff --git a/node_modules/bson/dist/bson.browser.umd.js.map b/node_modules/bson/dist/bson.browser.umd.js.map
new file mode 100644
index 0000000..4e153db
--- /dev/null
+++ b/node_modules/bson/dist/bson.browser.umd.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"bson.browser.umd.js","sources":["../node_modules/base64-js/index.js","../node_modules/ieee754/index.js","../node_modules/buffer/index.js","../node_modules/rollup-plugin-node-builtins/src/es6/empty.js","../node_modules/process-es6/browser.js","../node_modules/rollup-plugin-node-builtins/src/es6/inherits.js","../node_modules/rollup-plugin-node-builtins/src/es6/util.js","../src/parser/utils.ts","../src/ensure_buffer.ts","../src/uuid_utils.ts","../src/uuid.ts","../src/binary.ts","../src/code.ts","../src/db_ref.ts","../src/long.ts","../src/decimal128.ts","../src/double.ts","../src/int_32.ts","../src/max_key.ts","../src/min_key.ts","../src/objectid.ts","../src/regexp.ts","../src/symbol.ts","../node_modules/tslib/tslib.es6.js","../src/timestamp.ts","../src/extended_json.ts","../src/map.ts","../src/constants.ts","../src/parser/calculate_size.ts","../src/validate_utf8.ts","../src/parser/deserializer.ts","../src/float_parser.ts","../src/parser/serializer.ts","../src/bson.ts"],"sourcesContent":["'use strict'\n\nexports.byteLength = byteLength\nexports.toByteArray = toByteArray\nexports.fromByteArray = fromByteArray\n\nvar lookup = []\nvar revLookup = []\nvar Arr = typeof Uint8Array !== 'undefined' ? Uint8Array : Array\n\nvar code = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/'\nfor (var i = 0, len = code.length; i < len; ++i) {\n lookup[i] = code[i]\n revLookup[code.charCodeAt(i)] = i\n}\n\n// Support decoding URL-safe base64 strings, as Node.js does.\n// See: https://en.wikipedia.org/wiki/Base64#URL_applications\nrevLookup['-'.charCodeAt(0)] = 62\nrevLookup['_'.charCodeAt(0)] = 63\n\nfunction getLens (b64) {\n var len = b64.length\n\n if (len % 4 > 0) {\n throw new Error('Invalid string. Length must be a multiple of 4')\n }\n\n // Trim off extra bytes after placeholder bytes are found\n // See: https://github.com/beatgammit/base64-js/issues/42\n var validLen = b64.indexOf('=')\n if (validLen === -1) validLen = len\n\n var placeHoldersLen = validLen === len\n ? 0\n : 4 - (validLen % 4)\n\n return [validLen, placeHoldersLen]\n}\n\n// base64 is 4/3 + up to two characters of the original data\nfunction byteLength (b64) {\n var lens = getLens(b64)\n var validLen = lens[0]\n var placeHoldersLen = lens[1]\n return ((validLen + placeHoldersLen) * 3 / 4) - placeHoldersLen\n}\n\nfunction _byteLength (b64, validLen, placeHoldersLen) {\n return ((validLen + placeHoldersLen) * 3 / 4) - placeHoldersLen\n}\n\nfunction toByteArray (b64) {\n var tmp\n var lens = getLens(b64)\n var validLen = lens[0]\n var placeHoldersLen = lens[1]\n\n var arr = new Arr(_byteLength(b64, validLen, placeHoldersLen))\n\n var curByte = 0\n\n // if there are placeholders, only get up to the last complete 4 chars\n var len = placeHoldersLen > 0\n ? validLen - 4\n : validLen\n\n var i\n for (i = 0; i < len; i += 4) {\n tmp =\n (revLookup[b64.charCodeAt(i)] << 18) |\n (revLookup[b64.charCodeAt(i + 1)] << 12) |\n (revLookup[b64.charCodeAt(i + 2)] << 6) |\n revLookup[b64.charCodeAt(i + 3)]\n arr[curByte++] = (tmp >> 16) & 0xFF\n arr[curByte++] = (tmp >> 8) & 0xFF\n arr[curByte++] = tmp & 0xFF\n }\n\n if (placeHoldersLen === 2) {\n tmp =\n (revLookup[b64.charCodeAt(i)] << 2) |\n (revLookup[b64.charCodeAt(i + 1)] >> 4)\n arr[curByte++] = tmp & 0xFF\n }\n\n if (placeHoldersLen === 1) {\n tmp =\n (revLookup[b64.charCodeAt(i)] << 10) |\n (revLookup[b64.charCodeAt(i + 1)] << 4) |\n (revLookup[b64.charCodeAt(i + 2)] >> 2)\n arr[curByte++] = (tmp >> 8) & 0xFF\n arr[curByte++] = tmp & 0xFF\n }\n\n return arr\n}\n\nfunction tripletToBase64 (num) {\n return lookup[num >> 18 & 0x3F] +\n lookup[num >> 12 & 0x3F] +\n lookup[num >> 6 & 0x3F] +\n lookup[num & 0x3F]\n}\n\nfunction encodeChunk (uint8, start, end) {\n var tmp\n var output = []\n for (var i = start; i < end; i += 3) {\n tmp =\n ((uint8[i] << 16) & 0xFF0000) +\n ((uint8[i + 1] << 8) & 0xFF00) +\n (uint8[i + 2] & 0xFF)\n output.push(tripletToBase64(tmp))\n }\n return output.join('')\n}\n\nfunction fromByteArray (uint8) {\n var tmp\n var len = uint8.length\n var extraBytes = len % 3 // if we have 1 byte left, pad 2 bytes\n var parts = []\n var maxChunkLength = 16383 // must be multiple of 3\n\n // go through the array every three bytes, we'll deal with trailing stuff later\n for (var i = 0, len2 = len - extraBytes; i < len2; i += maxChunkLength) {\n parts.push(encodeChunk(uint8, i, (i + maxChunkLength) > len2 ? len2 : (i + maxChunkLength)))\n }\n\n // pad the end with zeros, but make sure to not forget the extra bytes\n if (extraBytes === 1) {\n tmp = uint8[len - 1]\n parts.push(\n lookup[tmp >> 2] +\n lookup[(tmp << 4) & 0x3F] +\n '=='\n )\n } else if (extraBytes === 2) {\n tmp = (uint8[len - 2] << 8) + uint8[len - 1]\n parts.push(\n lookup[tmp >> 10] +\n lookup[(tmp >> 4) & 0x3F] +\n lookup[(tmp << 2) & 0x3F] +\n '='\n )\n }\n\n return parts.join('')\n}\n","/*! ieee754. BSD-3-Clause License. Feross Aboukhadijeh */\nexports.read = function (buffer, offset, isLE, mLen, nBytes) {\n var e, m\n var eLen = (nBytes * 8) - mLen - 1\n var eMax = (1 << eLen) - 1\n var eBias = eMax >> 1\n var nBits = -7\n var i = isLE ? (nBytes - 1) : 0\n var d = isLE ? -1 : 1\n var s = buffer[offset + i]\n\n i += d\n\n e = s & ((1 << (-nBits)) - 1)\n s >>= (-nBits)\n nBits += eLen\n for (; nBits > 0; e = (e * 256) + buffer[offset + i], i += d, nBits -= 8) {}\n\n m = e & ((1 << (-nBits)) - 1)\n e >>= (-nBits)\n nBits += mLen\n for (; nBits > 0; m = (m * 256) + buffer[offset + i], i += d, nBits -= 8) {}\n\n if (e === 0) {\n e = 1 - eBias\n } else if (e === eMax) {\n return m ? NaN : ((s ? -1 : 1) * Infinity)\n } else {\n m = m + Math.pow(2, mLen)\n e = e - eBias\n }\n return (s ? -1 : 1) * m * Math.pow(2, e - mLen)\n}\n\nexports.write = function (buffer, value, offset, isLE, mLen, nBytes) {\n var e, m, c\n var eLen = (nBytes * 8) - mLen - 1\n var eMax = (1 << eLen) - 1\n var eBias = eMax >> 1\n var rt = (mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0)\n var i = isLE ? 0 : (nBytes - 1)\n var d = isLE ? 1 : -1\n var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0\n\n value = Math.abs(value)\n\n if (isNaN(value) || value === Infinity) {\n m = isNaN(value) ? 1 : 0\n e = eMax\n } else {\n e = Math.floor(Math.log(value) / Math.LN2)\n if (value * (c = Math.pow(2, -e)) < 1) {\n e--\n c *= 2\n }\n if (e + eBias >= 1) {\n value += rt / c\n } else {\n value += rt * Math.pow(2, 1 - eBias)\n }\n if (value * c >= 2) {\n e++\n c /= 2\n }\n\n if (e + eBias >= eMax) {\n m = 0\n e = eMax\n } else if (e + eBias >= 1) {\n m = ((value * c) - 1) * Math.pow(2, mLen)\n e = e + eBias\n } else {\n m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen)\n e = 0\n }\n }\n\n for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}\n\n e = (e << mLen) | m\n eLen += mLen\n for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}\n\n buffer[offset + i - d] |= s * 128\n}\n","/*!\n * The buffer module from node.js, for the browser.\n *\n * @author Feross Aboukhadijeh \n * @license MIT\n */\n/* eslint-disable no-proto */\n\n'use strict'\n\nvar base64 = require('base64-js')\nvar ieee754 = require('ieee754')\nvar customInspectSymbol =\n (typeof Symbol === 'function' && typeof Symbol['for'] === 'function') // eslint-disable-line dot-notation\n ? Symbol['for']('nodejs.util.inspect.custom') // eslint-disable-line dot-notation\n : null\n\nexports.Buffer = Buffer\nexports.SlowBuffer = SlowBuffer\nexports.INSPECT_MAX_BYTES = 50\n\nvar K_MAX_LENGTH = 0x7fffffff\nexports.kMaxLength = K_MAX_LENGTH\n\n/**\n * If `Buffer.TYPED_ARRAY_SUPPORT`:\n * === true Use Uint8Array implementation (fastest)\n * === false Print warning and recommend using `buffer` v4.x which has an Object\n * implementation (most compatible, even IE6)\n *\n * Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,\n * Opera 11.6+, iOS 4.2+.\n *\n * We report that the browser does not support typed arrays if the are not subclassable\n * using __proto__. Firefox 4-29 lacks support for adding new properties to `Uint8Array`\n * (See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438). IE 10 lacks support\n * for __proto__ and has a buggy typed array implementation.\n */\nBuffer.TYPED_ARRAY_SUPPORT = typedArraySupport()\n\nif (!Buffer.TYPED_ARRAY_SUPPORT && typeof console !== 'undefined' &&\n typeof console.error === 'function') {\n console.error(\n 'This browser lacks typed array (Uint8Array) support which is required by ' +\n '`buffer` v5.x. Use `buffer` v4.x if you require old browser support.'\n )\n}\n\nfunction typedArraySupport () {\n // Can typed array instances can be augmented?\n try {\n var arr = new Uint8Array(1)\n var proto = { foo: function () { return 42 } }\n Object.setPrototypeOf(proto, Uint8Array.prototype)\n Object.setPrototypeOf(arr, proto)\n return arr.foo() === 42\n } catch (e) {\n return false\n }\n}\n\nObject.defineProperty(Buffer.prototype, 'parent', {\n enumerable: true,\n get: function () {\n if (!Buffer.isBuffer(this)) return undefined\n return this.buffer\n }\n})\n\nObject.defineProperty(Buffer.prototype, 'offset', {\n enumerable: true,\n get: function () {\n if (!Buffer.isBuffer(this)) return undefined\n return this.byteOffset\n }\n})\n\nfunction createBuffer (length) {\n if (length > K_MAX_LENGTH) {\n throw new RangeError('The value \"' + length + '\" is invalid for option \"size\"')\n }\n // Return an augmented `Uint8Array` instance\n var buf = new Uint8Array(length)\n Object.setPrototypeOf(buf, Buffer.prototype)\n return buf\n}\n\n/**\n * The Buffer constructor returns instances of `Uint8Array` that have their\n * prototype changed to `Buffer.prototype`. Furthermore, `Buffer` is a subclass of\n * `Uint8Array`, so the returned instances will have all the node `Buffer` methods\n * and the `Uint8Array` methods. Square bracket notation works as expected -- it\n * returns a single octet.\n *\n * The `Uint8Array` prototype remains unmodified.\n */\n\nfunction Buffer (arg, encodingOrOffset, length) {\n // Common case.\n if (typeof arg === 'number') {\n if (typeof encodingOrOffset === 'string') {\n throw new TypeError(\n 'The \"string\" argument must be of type string. Received type number'\n )\n }\n return allocUnsafe(arg)\n }\n return from(arg, encodingOrOffset, length)\n}\n\nBuffer.poolSize = 8192 // not used by this implementation\n\nfunction from (value, encodingOrOffset, length) {\n if (typeof value === 'string') {\n return fromString(value, encodingOrOffset)\n }\n\n if (ArrayBuffer.isView(value)) {\n return fromArrayView(value)\n }\n\n if (value == null) {\n throw new TypeError(\n 'The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' +\n 'or Array-like Object. Received type ' + (typeof value)\n )\n }\n\n if (isInstance(value, ArrayBuffer) ||\n (value && isInstance(value.buffer, ArrayBuffer))) {\n return fromArrayBuffer(value, encodingOrOffset, length)\n }\n\n if (typeof SharedArrayBuffer !== 'undefined' &&\n (isInstance(value, SharedArrayBuffer) ||\n (value && isInstance(value.buffer, SharedArrayBuffer)))) {\n return fromArrayBuffer(value, encodingOrOffset, length)\n }\n\n if (typeof value === 'number') {\n throw new TypeError(\n 'The \"value\" argument must not be of type number. Received type number'\n )\n }\n\n var valueOf = value.valueOf && value.valueOf()\n if (valueOf != null && valueOf !== value) {\n return Buffer.from(valueOf, encodingOrOffset, length)\n }\n\n var b = fromObject(value)\n if (b) return b\n\n if (typeof Symbol !== 'undefined' && Symbol.toPrimitive != null &&\n typeof value[Symbol.toPrimitive] === 'function') {\n return Buffer.from(\n value[Symbol.toPrimitive]('string'), encodingOrOffset, length\n )\n }\n\n throw new TypeError(\n 'The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' +\n 'or Array-like Object. Received type ' + (typeof value)\n )\n}\n\n/**\n * Functionally equivalent to Buffer(arg, encoding) but throws a TypeError\n * if value is a number.\n * Buffer.from(str[, encoding])\n * Buffer.from(array)\n * Buffer.from(buffer)\n * Buffer.from(arrayBuffer[, byteOffset[, length]])\n **/\nBuffer.from = function (value, encodingOrOffset, length) {\n return from(value, encodingOrOffset, length)\n}\n\n// Note: Change prototype *after* Buffer.from is defined to workaround Chrome bug:\n// https://github.com/feross/buffer/pull/148\nObject.setPrototypeOf(Buffer.prototype, Uint8Array.prototype)\nObject.setPrototypeOf(Buffer, Uint8Array)\n\nfunction assertSize (size) {\n if (typeof size !== 'number') {\n throw new TypeError('\"size\" argument must be of type number')\n } else if (size < 0) {\n throw new RangeError('The value \"' + size + '\" is invalid for option \"size\"')\n }\n}\n\nfunction alloc (size, fill, encoding) {\n assertSize(size)\n if (size <= 0) {\n return createBuffer(size)\n }\n if (fill !== undefined) {\n // Only pay attention to encoding if it's a string. This\n // prevents accidentally sending in a number that would\n // be interpreted as a start offset.\n return typeof encoding === 'string'\n ? createBuffer(size).fill(fill, encoding)\n : createBuffer(size).fill(fill)\n }\n return createBuffer(size)\n}\n\n/**\n * Creates a new filled Buffer instance.\n * alloc(size[, fill[, encoding]])\n **/\nBuffer.alloc = function (size, fill, encoding) {\n return alloc(size, fill, encoding)\n}\n\nfunction allocUnsafe (size) {\n assertSize(size)\n return createBuffer(size < 0 ? 0 : checked(size) | 0)\n}\n\n/**\n * Equivalent to Buffer(num), by default creates a non-zero-filled Buffer instance.\n * */\nBuffer.allocUnsafe = function (size) {\n return allocUnsafe(size)\n}\n/**\n * Equivalent to SlowBuffer(num), by default creates a non-zero-filled Buffer instance.\n */\nBuffer.allocUnsafeSlow = function (size) {\n return allocUnsafe(size)\n}\n\nfunction fromString (string, encoding) {\n if (typeof encoding !== 'string' || encoding === '') {\n encoding = 'utf8'\n }\n\n if (!Buffer.isEncoding(encoding)) {\n throw new TypeError('Unknown encoding: ' + encoding)\n }\n\n var length = byteLength(string, encoding) | 0\n var buf = createBuffer(length)\n\n var actual = buf.write(string, encoding)\n\n if (actual !== length) {\n // Writing a hex string, for example, that contains invalid characters will\n // cause everything after the first invalid character to be ignored. (e.g.\n // 'abxxcd' will be treated as 'ab')\n buf = buf.slice(0, actual)\n }\n\n return buf\n}\n\nfunction fromArrayLike (array) {\n var length = array.length < 0 ? 0 : checked(array.length) | 0\n var buf = createBuffer(length)\n for (var i = 0; i < length; i += 1) {\n buf[i] = array[i] & 255\n }\n return buf\n}\n\nfunction fromArrayView (arrayView) {\n if (isInstance(arrayView, Uint8Array)) {\n var copy = new Uint8Array(arrayView)\n return fromArrayBuffer(copy.buffer, copy.byteOffset, copy.byteLength)\n }\n return fromArrayLike(arrayView)\n}\n\nfunction fromArrayBuffer (array, byteOffset, length) {\n if (byteOffset < 0 || array.byteLength < byteOffset) {\n throw new RangeError('\"offset\" is outside of buffer bounds')\n }\n\n if (array.byteLength < byteOffset + (length || 0)) {\n throw new RangeError('\"length\" is outside of buffer bounds')\n }\n\n var buf\n if (byteOffset === undefined && length === undefined) {\n buf = new Uint8Array(array)\n } else if (length === undefined) {\n buf = new Uint8Array(array, byteOffset)\n } else {\n buf = new Uint8Array(array, byteOffset, length)\n }\n\n // Return an augmented `Uint8Array` instance\n Object.setPrototypeOf(buf, Buffer.prototype)\n\n return buf\n}\n\nfunction fromObject (obj) {\n if (Buffer.isBuffer(obj)) {\n var len = checked(obj.length) | 0\n var buf = createBuffer(len)\n\n if (buf.length === 0) {\n return buf\n }\n\n obj.copy(buf, 0, 0, len)\n return buf\n }\n\n if (obj.length !== undefined) {\n if (typeof obj.length !== 'number' || numberIsNaN(obj.length)) {\n return createBuffer(0)\n }\n return fromArrayLike(obj)\n }\n\n if (obj.type === 'Buffer' && Array.isArray(obj.data)) {\n return fromArrayLike(obj.data)\n }\n}\n\nfunction checked (length) {\n // Note: cannot use `length < K_MAX_LENGTH` here because that fails when\n // length is NaN (which is otherwise coerced to zero.)\n if (length >= K_MAX_LENGTH) {\n throw new RangeError('Attempt to allocate Buffer larger than maximum ' +\n 'size: 0x' + K_MAX_LENGTH.toString(16) + ' bytes')\n }\n return length | 0\n}\n\nfunction SlowBuffer (length) {\n if (+length != length) { // eslint-disable-line eqeqeq\n length = 0\n }\n return Buffer.alloc(+length)\n}\n\nBuffer.isBuffer = function isBuffer (b) {\n return b != null && b._isBuffer === true &&\n b !== Buffer.prototype // so Buffer.isBuffer(Buffer.prototype) will be false\n}\n\nBuffer.compare = function compare (a, b) {\n if (isInstance(a, Uint8Array)) a = Buffer.from(a, a.offset, a.byteLength)\n if (isInstance(b, Uint8Array)) b = Buffer.from(b, b.offset, b.byteLength)\n if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {\n throw new TypeError(\n 'The \"buf1\", \"buf2\" arguments must be one of type Buffer or Uint8Array'\n )\n }\n\n if (a === b) return 0\n\n var x = a.length\n var y = b.length\n\n for (var i = 0, len = Math.min(x, y); i < len; ++i) {\n if (a[i] !== b[i]) {\n x = a[i]\n y = b[i]\n break\n }\n }\n\n if (x < y) return -1\n if (y < x) return 1\n return 0\n}\n\nBuffer.isEncoding = function isEncoding (encoding) {\n switch (String(encoding).toLowerCase()) {\n case 'hex':\n case 'utf8':\n case 'utf-8':\n case 'ascii':\n case 'latin1':\n case 'binary':\n case 'base64':\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return true\n default:\n return false\n }\n}\n\nBuffer.concat = function concat (list, length) {\n if (!Array.isArray(list)) {\n throw new TypeError('\"list\" argument must be an Array of Buffers')\n }\n\n if (list.length === 0) {\n return Buffer.alloc(0)\n }\n\n var i\n if (length === undefined) {\n length = 0\n for (i = 0; i < list.length; ++i) {\n length += list[i].length\n }\n }\n\n var buffer = Buffer.allocUnsafe(length)\n var pos = 0\n for (i = 0; i < list.length; ++i) {\n var buf = list[i]\n if (isInstance(buf, Uint8Array)) {\n if (pos + buf.length > buffer.length) {\n Buffer.from(buf).copy(buffer, pos)\n } else {\n Uint8Array.prototype.set.call(\n buffer,\n buf,\n pos\n )\n }\n } else if (!Buffer.isBuffer(buf)) {\n throw new TypeError('\"list\" argument must be an Array of Buffers')\n } else {\n buf.copy(buffer, pos)\n }\n pos += buf.length\n }\n return buffer\n}\n\nfunction byteLength (string, encoding) {\n if (Buffer.isBuffer(string)) {\n return string.length\n }\n if (ArrayBuffer.isView(string) || isInstance(string, ArrayBuffer)) {\n return string.byteLength\n }\n if (typeof string !== 'string') {\n throw new TypeError(\n 'The \"string\" argument must be one of type string, Buffer, or ArrayBuffer. ' +\n 'Received type ' + typeof string\n )\n }\n\n var len = string.length\n var mustMatch = (arguments.length > 2 && arguments[2] === true)\n if (!mustMatch && len === 0) return 0\n\n // Use a for loop to avoid recursion\n var loweredCase = false\n for (;;) {\n switch (encoding) {\n case 'ascii':\n case 'latin1':\n case 'binary':\n return len\n case 'utf8':\n case 'utf-8':\n return utf8ToBytes(string).length\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return len * 2\n case 'hex':\n return len >>> 1\n case 'base64':\n return base64ToBytes(string).length\n default:\n if (loweredCase) {\n return mustMatch ? -1 : utf8ToBytes(string).length // assume utf8\n }\n encoding = ('' + encoding).toLowerCase()\n loweredCase = true\n }\n }\n}\nBuffer.byteLength = byteLength\n\nfunction slowToString (encoding, start, end) {\n var loweredCase = false\n\n // No need to verify that \"this.length <= MAX_UINT32\" since it's a read-only\n // property of a typed array.\n\n // This behaves neither like String nor Uint8Array in that we set start/end\n // to their upper/lower bounds if the value passed is out of range.\n // undefined is handled specially as per ECMA-262 6th Edition,\n // Section 13.3.3.7 Runtime Semantics: KeyedBindingInitialization.\n if (start === undefined || start < 0) {\n start = 0\n }\n // Return early if start > this.length. Done here to prevent potential uint32\n // coercion fail below.\n if (start > this.length) {\n return ''\n }\n\n if (end === undefined || end > this.length) {\n end = this.length\n }\n\n if (end <= 0) {\n return ''\n }\n\n // Force coercion to uint32. This will also coerce falsey/NaN values to 0.\n end >>>= 0\n start >>>= 0\n\n if (end <= start) {\n return ''\n }\n\n if (!encoding) encoding = 'utf8'\n\n while (true) {\n switch (encoding) {\n case 'hex':\n return hexSlice(this, start, end)\n\n case 'utf8':\n case 'utf-8':\n return utf8Slice(this, start, end)\n\n case 'ascii':\n return asciiSlice(this, start, end)\n\n case 'latin1':\n case 'binary':\n return latin1Slice(this, start, end)\n\n case 'base64':\n return base64Slice(this, start, end)\n\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return utf16leSlice(this, start, end)\n\n default:\n if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)\n encoding = (encoding + '').toLowerCase()\n loweredCase = true\n }\n }\n}\n\n// This property is used by `Buffer.isBuffer` (and the `is-buffer` npm package)\n// to detect a Buffer instance. It's not possible to use `instanceof Buffer`\n// reliably in a browserify context because there could be multiple different\n// copies of the 'buffer' package in use. This method works even for Buffer\n// instances that were created from another copy of the `buffer` package.\n// See: https://github.com/feross/buffer/issues/154\nBuffer.prototype._isBuffer = true\n\nfunction swap (b, n, m) {\n var i = b[n]\n b[n] = b[m]\n b[m] = i\n}\n\nBuffer.prototype.swap16 = function swap16 () {\n var len = this.length\n if (len % 2 !== 0) {\n throw new RangeError('Buffer size must be a multiple of 16-bits')\n }\n for (var i = 0; i < len; i += 2) {\n swap(this, i, i + 1)\n }\n return this\n}\n\nBuffer.prototype.swap32 = function swap32 () {\n var len = this.length\n if (len % 4 !== 0) {\n throw new RangeError('Buffer size must be a multiple of 32-bits')\n }\n for (var i = 0; i < len; i += 4) {\n swap(this, i, i + 3)\n swap(this, i + 1, i + 2)\n }\n return this\n}\n\nBuffer.prototype.swap64 = function swap64 () {\n var len = this.length\n if (len % 8 !== 0) {\n throw new RangeError('Buffer size must be a multiple of 64-bits')\n }\n for (var i = 0; i < len; i += 8) {\n swap(this, i, i + 7)\n swap(this, i + 1, i + 6)\n swap(this, i + 2, i + 5)\n swap(this, i + 3, i + 4)\n }\n return this\n}\n\nBuffer.prototype.toString = function toString () {\n var length = this.length\n if (length === 0) return ''\n if (arguments.length === 0) return utf8Slice(this, 0, length)\n return slowToString.apply(this, arguments)\n}\n\nBuffer.prototype.toLocaleString = Buffer.prototype.toString\n\nBuffer.prototype.equals = function equals (b) {\n if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer')\n if (this === b) return true\n return Buffer.compare(this, b) === 0\n}\n\nBuffer.prototype.inspect = function inspect () {\n var str = ''\n var max = exports.INSPECT_MAX_BYTES\n str = this.toString('hex', 0, max).replace(/(.{2})/g, '$1 ').trim()\n if (this.length > max) str += ' ... '\n return ''\n}\nif (customInspectSymbol) {\n Buffer.prototype[customInspectSymbol] = Buffer.prototype.inspect\n}\n\nBuffer.prototype.compare = function compare (target, start, end, thisStart, thisEnd) {\n if (isInstance(target, Uint8Array)) {\n target = Buffer.from(target, target.offset, target.byteLength)\n }\n if (!Buffer.isBuffer(target)) {\n throw new TypeError(\n 'The \"target\" argument must be one of type Buffer or Uint8Array. ' +\n 'Received type ' + (typeof target)\n )\n }\n\n if (start === undefined) {\n start = 0\n }\n if (end === undefined) {\n end = target ? target.length : 0\n }\n if (thisStart === undefined) {\n thisStart = 0\n }\n if (thisEnd === undefined) {\n thisEnd = this.length\n }\n\n if (start < 0 || end > target.length || thisStart < 0 || thisEnd > this.length) {\n throw new RangeError('out of range index')\n }\n\n if (thisStart >= thisEnd && start >= end) {\n return 0\n }\n if (thisStart >= thisEnd) {\n return -1\n }\n if (start >= end) {\n return 1\n }\n\n start >>>= 0\n end >>>= 0\n thisStart >>>= 0\n thisEnd >>>= 0\n\n if (this === target) return 0\n\n var x = thisEnd - thisStart\n var y = end - start\n var len = Math.min(x, y)\n\n var thisCopy = this.slice(thisStart, thisEnd)\n var targetCopy = target.slice(start, end)\n\n for (var i = 0; i < len; ++i) {\n if (thisCopy[i] !== targetCopy[i]) {\n x = thisCopy[i]\n y = targetCopy[i]\n break\n }\n }\n\n if (x < y) return -1\n if (y < x) return 1\n return 0\n}\n\n// Finds either the first index of `val` in `buffer` at offset >= `byteOffset`,\n// OR the last index of `val` in `buffer` at offset <= `byteOffset`.\n//\n// Arguments:\n// - buffer - a Buffer to search\n// - val - a string, Buffer, or number\n// - byteOffset - an index into `buffer`; will be clamped to an int32\n// - encoding - an optional encoding, relevant is val is a string\n// - dir - true for indexOf, false for lastIndexOf\nfunction bidirectionalIndexOf (buffer, val, byteOffset, encoding, dir) {\n // Empty buffer means no match\n if (buffer.length === 0) return -1\n\n // Normalize byteOffset\n if (typeof byteOffset === 'string') {\n encoding = byteOffset\n byteOffset = 0\n } else if (byteOffset > 0x7fffffff) {\n byteOffset = 0x7fffffff\n } else if (byteOffset < -0x80000000) {\n byteOffset = -0x80000000\n }\n byteOffset = +byteOffset // Coerce to Number.\n if (numberIsNaN(byteOffset)) {\n // byteOffset: it it's undefined, null, NaN, \"foo\", etc, search whole buffer\n byteOffset = dir ? 0 : (buffer.length - 1)\n }\n\n // Normalize byteOffset: negative offsets start from the end of the buffer\n if (byteOffset < 0) byteOffset = buffer.length + byteOffset\n if (byteOffset >= buffer.length) {\n if (dir) return -1\n else byteOffset = buffer.length - 1\n } else if (byteOffset < 0) {\n if (dir) byteOffset = 0\n else return -1\n }\n\n // Normalize val\n if (typeof val === 'string') {\n val = Buffer.from(val, encoding)\n }\n\n // Finally, search either indexOf (if dir is true) or lastIndexOf\n if (Buffer.isBuffer(val)) {\n // Special case: looking for empty string/buffer always fails\n if (val.length === 0) {\n return -1\n }\n return arrayIndexOf(buffer, val, byteOffset, encoding, dir)\n } else if (typeof val === 'number') {\n val = val & 0xFF // Search for a byte value [0-255]\n if (typeof Uint8Array.prototype.indexOf === 'function') {\n if (dir) {\n return Uint8Array.prototype.indexOf.call(buffer, val, byteOffset)\n } else {\n return Uint8Array.prototype.lastIndexOf.call(buffer, val, byteOffset)\n }\n }\n return arrayIndexOf(buffer, [val], byteOffset, encoding, dir)\n }\n\n throw new TypeError('val must be string, number or Buffer')\n}\n\nfunction arrayIndexOf (arr, val, byteOffset, encoding, dir) {\n var indexSize = 1\n var arrLength = arr.length\n var valLength = val.length\n\n if (encoding !== undefined) {\n encoding = String(encoding).toLowerCase()\n if (encoding === 'ucs2' || encoding === 'ucs-2' ||\n encoding === 'utf16le' || encoding === 'utf-16le') {\n if (arr.length < 2 || val.length < 2) {\n return -1\n }\n indexSize = 2\n arrLength /= 2\n valLength /= 2\n byteOffset /= 2\n }\n }\n\n function read (buf, i) {\n if (indexSize === 1) {\n return buf[i]\n } else {\n return buf.readUInt16BE(i * indexSize)\n }\n }\n\n var i\n if (dir) {\n var foundIndex = -1\n for (i = byteOffset; i < arrLength; i++) {\n if (read(arr, i) === read(val, foundIndex === -1 ? 0 : i - foundIndex)) {\n if (foundIndex === -1) foundIndex = i\n if (i - foundIndex + 1 === valLength) return foundIndex * indexSize\n } else {\n if (foundIndex !== -1) i -= i - foundIndex\n foundIndex = -1\n }\n }\n } else {\n if (byteOffset + valLength > arrLength) byteOffset = arrLength - valLength\n for (i = byteOffset; i >= 0; i--) {\n var found = true\n for (var j = 0; j < valLength; j++) {\n if (read(arr, i + j) !== read(val, j)) {\n found = false\n break\n }\n }\n if (found) return i\n }\n }\n\n return -1\n}\n\nBuffer.prototype.includes = function includes (val, byteOffset, encoding) {\n return this.indexOf(val, byteOffset, encoding) !== -1\n}\n\nBuffer.prototype.indexOf = function indexOf (val, byteOffset, encoding) {\n return bidirectionalIndexOf(this, val, byteOffset, encoding, true)\n}\n\nBuffer.prototype.lastIndexOf = function lastIndexOf (val, byteOffset, encoding) {\n return bidirectionalIndexOf(this, val, byteOffset, encoding, false)\n}\n\nfunction hexWrite (buf, string, offset, length) {\n offset = Number(offset) || 0\n var remaining = buf.length - offset\n if (!length) {\n length = remaining\n } else {\n length = Number(length)\n if (length > remaining) {\n length = remaining\n }\n }\n\n var strLen = string.length\n\n if (length > strLen / 2) {\n length = strLen / 2\n }\n for (var i = 0; i < length; ++i) {\n var parsed = parseInt(string.substr(i * 2, 2), 16)\n if (numberIsNaN(parsed)) return i\n buf[offset + i] = parsed\n }\n return i\n}\n\nfunction utf8Write (buf, string, offset, length) {\n return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length)\n}\n\nfunction asciiWrite (buf, string, offset, length) {\n return blitBuffer(asciiToBytes(string), buf, offset, length)\n}\n\nfunction base64Write (buf, string, offset, length) {\n return blitBuffer(base64ToBytes(string), buf, offset, length)\n}\n\nfunction ucs2Write (buf, string, offset, length) {\n return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length)\n}\n\nBuffer.prototype.write = function write (string, offset, length, encoding) {\n // Buffer#write(string)\n if (offset === undefined) {\n encoding = 'utf8'\n length = this.length\n offset = 0\n // Buffer#write(string, encoding)\n } else if (length === undefined && typeof offset === 'string') {\n encoding = offset\n length = this.length\n offset = 0\n // Buffer#write(string, offset[, length][, encoding])\n } else if (isFinite(offset)) {\n offset = offset >>> 0\n if (isFinite(length)) {\n length = length >>> 0\n if (encoding === undefined) encoding = 'utf8'\n } else {\n encoding = length\n length = undefined\n }\n } else {\n throw new Error(\n 'Buffer.write(string, encoding, offset[, length]) is no longer supported'\n )\n }\n\n var remaining = this.length - offset\n if (length === undefined || length > remaining) length = remaining\n\n if ((string.length > 0 && (length < 0 || offset < 0)) || offset > this.length) {\n throw new RangeError('Attempt to write outside buffer bounds')\n }\n\n if (!encoding) encoding = 'utf8'\n\n var loweredCase = false\n for (;;) {\n switch (encoding) {\n case 'hex':\n return hexWrite(this, string, offset, length)\n\n case 'utf8':\n case 'utf-8':\n return utf8Write(this, string, offset, length)\n\n case 'ascii':\n case 'latin1':\n case 'binary':\n return asciiWrite(this, string, offset, length)\n\n case 'base64':\n // Warning: maxLength not taken into account in base64Write\n return base64Write(this, string, offset, length)\n\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return ucs2Write(this, string, offset, length)\n\n default:\n if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)\n encoding = ('' + encoding).toLowerCase()\n loweredCase = true\n }\n }\n}\n\nBuffer.prototype.toJSON = function toJSON () {\n return {\n type: 'Buffer',\n data: Array.prototype.slice.call(this._arr || this, 0)\n }\n}\n\nfunction base64Slice (buf, start, end) {\n if (start === 0 && end === buf.length) {\n return base64.fromByteArray(buf)\n } else {\n return base64.fromByteArray(buf.slice(start, end))\n }\n}\n\nfunction utf8Slice (buf, start, end) {\n end = Math.min(buf.length, end)\n var res = []\n\n var i = start\n while (i < end) {\n var firstByte = buf[i]\n var codePoint = null\n var bytesPerSequence = (firstByte > 0xEF)\n ? 4\n : (firstByte > 0xDF)\n ? 3\n : (firstByte > 0xBF)\n ? 2\n : 1\n\n if (i + bytesPerSequence <= end) {\n var secondByte, thirdByte, fourthByte, tempCodePoint\n\n switch (bytesPerSequence) {\n case 1:\n if (firstByte < 0x80) {\n codePoint = firstByte\n }\n break\n case 2:\n secondByte = buf[i + 1]\n if ((secondByte & 0xC0) === 0x80) {\n tempCodePoint = (firstByte & 0x1F) << 0x6 | (secondByte & 0x3F)\n if (tempCodePoint > 0x7F) {\n codePoint = tempCodePoint\n }\n }\n break\n case 3:\n secondByte = buf[i + 1]\n thirdByte = buf[i + 2]\n if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {\n tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | (thirdByte & 0x3F)\n if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {\n codePoint = tempCodePoint\n }\n }\n break\n case 4:\n secondByte = buf[i + 1]\n thirdByte = buf[i + 2]\n fourthByte = buf[i + 3]\n if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {\n tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | (fourthByte & 0x3F)\n if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {\n codePoint = tempCodePoint\n }\n }\n }\n }\n\n if (codePoint === null) {\n // we did not generate a valid codePoint so insert a\n // replacement char (U+FFFD) and advance only 1 byte\n codePoint = 0xFFFD\n bytesPerSequence = 1\n } else if (codePoint > 0xFFFF) {\n // encode to utf16 (surrogate pair dance)\n codePoint -= 0x10000\n res.push(codePoint >>> 10 & 0x3FF | 0xD800)\n codePoint = 0xDC00 | codePoint & 0x3FF\n }\n\n res.push(codePoint)\n i += bytesPerSequence\n }\n\n return decodeCodePointsArray(res)\n}\n\n// Based on http://stackoverflow.com/a/22747272/680742, the browser with\n// the lowest limit is Chrome, with 0x10000 args.\n// We go 1 magnitude less, for safety\nvar MAX_ARGUMENTS_LENGTH = 0x1000\n\nfunction decodeCodePointsArray (codePoints) {\n var len = codePoints.length\n if (len <= MAX_ARGUMENTS_LENGTH) {\n return String.fromCharCode.apply(String, codePoints) // avoid extra slice()\n }\n\n // Decode in chunks to avoid \"call stack size exceeded\".\n var res = ''\n var i = 0\n while (i < len) {\n res += String.fromCharCode.apply(\n String,\n codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH)\n )\n }\n return res\n}\n\nfunction asciiSlice (buf, start, end) {\n var ret = ''\n end = Math.min(buf.length, end)\n\n for (var i = start; i < end; ++i) {\n ret += String.fromCharCode(buf[i] & 0x7F)\n }\n return ret\n}\n\nfunction latin1Slice (buf, start, end) {\n var ret = ''\n end = Math.min(buf.length, end)\n\n for (var i = start; i < end; ++i) {\n ret += String.fromCharCode(buf[i])\n }\n return ret\n}\n\nfunction hexSlice (buf, start, end) {\n var len = buf.length\n\n if (!start || start < 0) start = 0\n if (!end || end < 0 || end > len) end = len\n\n var out = ''\n for (var i = start; i < end; ++i) {\n out += hexSliceLookupTable[buf[i]]\n }\n return out\n}\n\nfunction utf16leSlice (buf, start, end) {\n var bytes = buf.slice(start, end)\n var res = ''\n // If bytes.length is odd, the last 8 bits must be ignored (same as node.js)\n for (var i = 0; i < bytes.length - 1; i += 2) {\n res += String.fromCharCode(bytes[i] + (bytes[i + 1] * 256))\n }\n return res\n}\n\nBuffer.prototype.slice = function slice (start, end) {\n var len = this.length\n start = ~~start\n end = end === undefined ? len : ~~end\n\n if (start < 0) {\n start += len\n if (start < 0) start = 0\n } else if (start > len) {\n start = len\n }\n\n if (end < 0) {\n end += len\n if (end < 0) end = 0\n } else if (end > len) {\n end = len\n }\n\n if (end < start) end = start\n\n var newBuf = this.subarray(start, end)\n // Return an augmented `Uint8Array` instance\n Object.setPrototypeOf(newBuf, Buffer.prototype)\n\n return newBuf\n}\n\n/*\n * Need to make sure that buffer isn't trying to write out of bounds.\n */\nfunction checkOffset (offset, ext, length) {\n if ((offset % 1) !== 0 || offset < 0) throw new RangeError('offset is not uint')\n if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length')\n}\n\nBuffer.prototype.readUintLE =\nBuffer.prototype.readUIntLE = function readUIntLE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) checkOffset(offset, byteLength, this.length)\n\n var val = this[offset]\n var mul = 1\n var i = 0\n while (++i < byteLength && (mul *= 0x100)) {\n val += this[offset + i] * mul\n }\n\n return val\n}\n\nBuffer.prototype.readUintBE =\nBuffer.prototype.readUIntBE = function readUIntBE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) {\n checkOffset(offset, byteLength, this.length)\n }\n\n var val = this[offset + --byteLength]\n var mul = 1\n while (byteLength > 0 && (mul *= 0x100)) {\n val += this[offset + --byteLength] * mul\n }\n\n return val\n}\n\nBuffer.prototype.readUint8 =\nBuffer.prototype.readUInt8 = function readUInt8 (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 1, this.length)\n return this[offset]\n}\n\nBuffer.prototype.readUint16LE =\nBuffer.prototype.readUInt16LE = function readUInt16LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n return this[offset] | (this[offset + 1] << 8)\n}\n\nBuffer.prototype.readUint16BE =\nBuffer.prototype.readUInt16BE = function readUInt16BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n return (this[offset] << 8) | this[offset + 1]\n}\n\nBuffer.prototype.readUint32LE =\nBuffer.prototype.readUInt32LE = function readUInt32LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return ((this[offset]) |\n (this[offset + 1] << 8) |\n (this[offset + 2] << 16)) +\n (this[offset + 3] * 0x1000000)\n}\n\nBuffer.prototype.readUint32BE =\nBuffer.prototype.readUInt32BE = function readUInt32BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return (this[offset] * 0x1000000) +\n ((this[offset + 1] << 16) |\n (this[offset + 2] << 8) |\n this[offset + 3])\n}\n\nBuffer.prototype.readIntLE = function readIntLE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) checkOffset(offset, byteLength, this.length)\n\n var val = this[offset]\n var mul = 1\n var i = 0\n while (++i < byteLength && (mul *= 0x100)) {\n val += this[offset + i] * mul\n }\n mul *= 0x80\n\n if (val >= mul) val -= Math.pow(2, 8 * byteLength)\n\n return val\n}\n\nBuffer.prototype.readIntBE = function readIntBE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) checkOffset(offset, byteLength, this.length)\n\n var i = byteLength\n var mul = 1\n var val = this[offset + --i]\n while (i > 0 && (mul *= 0x100)) {\n val += this[offset + --i] * mul\n }\n mul *= 0x80\n\n if (val >= mul) val -= Math.pow(2, 8 * byteLength)\n\n return val\n}\n\nBuffer.prototype.readInt8 = function readInt8 (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 1, this.length)\n if (!(this[offset] & 0x80)) return (this[offset])\n return ((0xff - this[offset] + 1) * -1)\n}\n\nBuffer.prototype.readInt16LE = function readInt16LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n var val = this[offset] | (this[offset + 1] << 8)\n return (val & 0x8000) ? val | 0xFFFF0000 : val\n}\n\nBuffer.prototype.readInt16BE = function readInt16BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n var val = this[offset + 1] | (this[offset] << 8)\n return (val & 0x8000) ? val | 0xFFFF0000 : val\n}\n\nBuffer.prototype.readInt32LE = function readInt32LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return (this[offset]) |\n (this[offset + 1] << 8) |\n (this[offset + 2] << 16) |\n (this[offset + 3] << 24)\n}\n\nBuffer.prototype.readInt32BE = function readInt32BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return (this[offset] << 24) |\n (this[offset + 1] << 16) |\n (this[offset + 2] << 8) |\n (this[offset + 3])\n}\n\nBuffer.prototype.readFloatLE = function readFloatLE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n return ieee754.read(this, offset, true, 23, 4)\n}\n\nBuffer.prototype.readFloatBE = function readFloatBE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n return ieee754.read(this, offset, false, 23, 4)\n}\n\nBuffer.prototype.readDoubleLE = function readDoubleLE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 8, this.length)\n return ieee754.read(this, offset, true, 52, 8)\n}\n\nBuffer.prototype.readDoubleBE = function readDoubleBE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 8, this.length)\n return ieee754.read(this, offset, false, 52, 8)\n}\n\nfunction checkInt (buf, value, offset, ext, max, min) {\n if (!Buffer.isBuffer(buf)) throw new TypeError('\"buffer\" argument must be a Buffer instance')\n if (value > max || value < min) throw new RangeError('\"value\" argument is out of bounds')\n if (offset + ext > buf.length) throw new RangeError('Index out of range')\n}\n\nBuffer.prototype.writeUintLE =\nBuffer.prototype.writeUIntLE = function writeUIntLE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) {\n var maxBytes = Math.pow(2, 8 * byteLength) - 1\n checkInt(this, value, offset, byteLength, maxBytes, 0)\n }\n\n var mul = 1\n var i = 0\n this[offset] = value & 0xFF\n while (++i < byteLength && (mul *= 0x100)) {\n this[offset + i] = (value / mul) & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeUintBE =\nBuffer.prototype.writeUIntBE = function writeUIntBE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) {\n var maxBytes = Math.pow(2, 8 * byteLength) - 1\n checkInt(this, value, offset, byteLength, maxBytes, 0)\n }\n\n var i = byteLength - 1\n var mul = 1\n this[offset + i] = value & 0xFF\n while (--i >= 0 && (mul *= 0x100)) {\n this[offset + i] = (value / mul) & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeUint8 =\nBuffer.prototype.writeUInt8 = function writeUInt8 (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0)\n this[offset] = (value & 0xff)\n return offset + 1\n}\n\nBuffer.prototype.writeUint16LE =\nBuffer.prototype.writeUInt16LE = function writeUInt16LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)\n this[offset] = (value & 0xff)\n this[offset + 1] = (value >>> 8)\n return offset + 2\n}\n\nBuffer.prototype.writeUint16BE =\nBuffer.prototype.writeUInt16BE = function writeUInt16BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)\n this[offset] = (value >>> 8)\n this[offset + 1] = (value & 0xff)\n return offset + 2\n}\n\nBuffer.prototype.writeUint32LE =\nBuffer.prototype.writeUInt32LE = function writeUInt32LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)\n this[offset + 3] = (value >>> 24)\n this[offset + 2] = (value >>> 16)\n this[offset + 1] = (value >>> 8)\n this[offset] = (value & 0xff)\n return offset + 4\n}\n\nBuffer.prototype.writeUint32BE =\nBuffer.prototype.writeUInt32BE = function writeUInt32BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)\n this[offset] = (value >>> 24)\n this[offset + 1] = (value >>> 16)\n this[offset + 2] = (value >>> 8)\n this[offset + 3] = (value & 0xff)\n return offset + 4\n}\n\nBuffer.prototype.writeIntLE = function writeIntLE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n var limit = Math.pow(2, (8 * byteLength) - 1)\n\n checkInt(this, value, offset, byteLength, limit - 1, -limit)\n }\n\n var i = 0\n var mul = 1\n var sub = 0\n this[offset] = value & 0xFF\n while (++i < byteLength && (mul *= 0x100)) {\n if (value < 0 && sub === 0 && this[offset + i - 1] !== 0) {\n sub = 1\n }\n this[offset + i] = ((value / mul) >> 0) - sub & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeIntBE = function writeIntBE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n var limit = Math.pow(2, (8 * byteLength) - 1)\n\n checkInt(this, value, offset, byteLength, limit - 1, -limit)\n }\n\n var i = byteLength - 1\n var mul = 1\n var sub = 0\n this[offset + i] = value & 0xFF\n while (--i >= 0 && (mul *= 0x100)) {\n if (value < 0 && sub === 0 && this[offset + i + 1] !== 0) {\n sub = 1\n }\n this[offset + i] = ((value / mul) >> 0) - sub & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeInt8 = function writeInt8 (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80)\n if (value < 0) value = 0xff + value + 1\n this[offset] = (value & 0xff)\n return offset + 1\n}\n\nBuffer.prototype.writeInt16LE = function writeInt16LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)\n this[offset] = (value & 0xff)\n this[offset + 1] = (value >>> 8)\n return offset + 2\n}\n\nBuffer.prototype.writeInt16BE = function writeInt16BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)\n this[offset] = (value >>> 8)\n this[offset + 1] = (value & 0xff)\n return offset + 2\n}\n\nBuffer.prototype.writeInt32LE = function writeInt32LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)\n this[offset] = (value & 0xff)\n this[offset + 1] = (value >>> 8)\n this[offset + 2] = (value >>> 16)\n this[offset + 3] = (value >>> 24)\n return offset + 4\n}\n\nBuffer.prototype.writeInt32BE = function writeInt32BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)\n if (value < 0) value = 0xffffffff + value + 1\n this[offset] = (value >>> 24)\n this[offset + 1] = (value >>> 16)\n this[offset + 2] = (value >>> 8)\n this[offset + 3] = (value & 0xff)\n return offset + 4\n}\n\nfunction checkIEEE754 (buf, value, offset, ext, max, min) {\n if (offset + ext > buf.length) throw new RangeError('Index out of range')\n if (offset < 0) throw new RangeError('Index out of range')\n}\n\nfunction writeFloat (buf, value, offset, littleEndian, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n checkIEEE754(buf, value, offset, 4, 3.4028234663852886e+38, -3.4028234663852886e+38)\n }\n ieee754.write(buf, value, offset, littleEndian, 23, 4)\n return offset + 4\n}\n\nBuffer.prototype.writeFloatLE = function writeFloatLE (value, offset, noAssert) {\n return writeFloat(this, value, offset, true, noAssert)\n}\n\nBuffer.prototype.writeFloatBE = function writeFloatBE (value, offset, noAssert) {\n return writeFloat(this, value, offset, false, noAssert)\n}\n\nfunction writeDouble (buf, value, offset, littleEndian, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n checkIEEE754(buf, value, offset, 8, 1.7976931348623157E+308, -1.7976931348623157E+308)\n }\n ieee754.write(buf, value, offset, littleEndian, 52, 8)\n return offset + 8\n}\n\nBuffer.prototype.writeDoubleLE = function writeDoubleLE (value, offset, noAssert) {\n return writeDouble(this, value, offset, true, noAssert)\n}\n\nBuffer.prototype.writeDoubleBE = function writeDoubleBE (value, offset, noAssert) {\n return writeDouble(this, value, offset, false, noAssert)\n}\n\n// copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)\nBuffer.prototype.copy = function copy (target, targetStart, start, end) {\n if (!Buffer.isBuffer(target)) throw new TypeError('argument should be a Buffer')\n if (!start) start = 0\n if (!end && end !== 0) end = this.length\n if (targetStart >= target.length) targetStart = target.length\n if (!targetStart) targetStart = 0\n if (end > 0 && end < start) end = start\n\n // Copy 0 bytes; we're done\n if (end === start) return 0\n if (target.length === 0 || this.length === 0) return 0\n\n // Fatal error conditions\n if (targetStart < 0) {\n throw new RangeError('targetStart out of bounds')\n }\n if (start < 0 || start >= this.length) throw new RangeError('Index out of range')\n if (end < 0) throw new RangeError('sourceEnd out of bounds')\n\n // Are we oob?\n if (end > this.length) end = this.length\n if (target.length - targetStart < end - start) {\n end = target.length - targetStart + start\n }\n\n var len = end - start\n\n if (this === target && typeof Uint8Array.prototype.copyWithin === 'function') {\n // Use built-in when available, missing from IE11\n this.copyWithin(targetStart, start, end)\n } else {\n Uint8Array.prototype.set.call(\n target,\n this.subarray(start, end),\n targetStart\n )\n }\n\n return len\n}\n\n// Usage:\n// buffer.fill(number[, offset[, end]])\n// buffer.fill(buffer[, offset[, end]])\n// buffer.fill(string[, offset[, end]][, encoding])\nBuffer.prototype.fill = function fill (val, start, end, encoding) {\n // Handle string cases:\n if (typeof val === 'string') {\n if (typeof start === 'string') {\n encoding = start\n start = 0\n end = this.length\n } else if (typeof end === 'string') {\n encoding = end\n end = this.length\n }\n if (encoding !== undefined && typeof encoding !== 'string') {\n throw new TypeError('encoding must be a string')\n }\n if (typeof encoding === 'string' && !Buffer.isEncoding(encoding)) {\n throw new TypeError('Unknown encoding: ' + encoding)\n }\n if (val.length === 1) {\n var code = val.charCodeAt(0)\n if ((encoding === 'utf8' && code < 128) ||\n encoding === 'latin1') {\n // Fast path: If `val` fits into a single byte, use that numeric value.\n val = code\n }\n }\n } else if (typeof val === 'number') {\n val = val & 255\n } else if (typeof val === 'boolean') {\n val = Number(val)\n }\n\n // Invalid ranges are not set to a default, so can range check early.\n if (start < 0 || this.length < start || this.length < end) {\n throw new RangeError('Out of range index')\n }\n\n if (end <= start) {\n return this\n }\n\n start = start >>> 0\n end = end === undefined ? this.length : end >>> 0\n\n if (!val) val = 0\n\n var i\n if (typeof val === 'number') {\n for (i = start; i < end; ++i) {\n this[i] = val\n }\n } else {\n var bytes = Buffer.isBuffer(val)\n ? val\n : Buffer.from(val, encoding)\n var len = bytes.length\n if (len === 0) {\n throw new TypeError('The value \"' + val +\n '\" is invalid for argument \"value\"')\n }\n for (i = 0; i < end - start; ++i) {\n this[i + start] = bytes[i % len]\n }\n }\n\n return this\n}\n\n// HELPER FUNCTIONS\n// ================\n\nvar INVALID_BASE64_RE = /[^+/0-9A-Za-z-_]/g\n\nfunction base64clean (str) {\n // Node takes equal signs as end of the Base64 encoding\n str = str.split('=')[0]\n // Node strips out invalid characters like \\n and \\t from the string, base64-js does not\n str = str.trim().replace(INVALID_BASE64_RE, '')\n // Node converts strings with length < 2 to ''\n if (str.length < 2) return ''\n // Node allows for non-padded base64 strings (missing trailing ===), base64-js does not\n while (str.length % 4 !== 0) {\n str = str + '='\n }\n return str\n}\n\nfunction utf8ToBytes (string, units) {\n units = units || Infinity\n var codePoint\n var length = string.length\n var leadSurrogate = null\n var bytes = []\n\n for (var i = 0; i < length; ++i) {\n codePoint = string.charCodeAt(i)\n\n // is surrogate component\n if (codePoint > 0xD7FF && codePoint < 0xE000) {\n // last char was a lead\n if (!leadSurrogate) {\n // no lead yet\n if (codePoint > 0xDBFF) {\n // unexpected trail\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n continue\n } else if (i + 1 === length) {\n // unpaired lead\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n continue\n }\n\n // valid lead\n leadSurrogate = codePoint\n\n continue\n }\n\n // 2 leads in a row\n if (codePoint < 0xDC00) {\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n leadSurrogate = codePoint\n continue\n }\n\n // valid surrogate pair\n codePoint = (leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00) + 0x10000\n } else if (leadSurrogate) {\n // valid bmp char, but last char was a lead\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n }\n\n leadSurrogate = null\n\n // encode utf8\n if (codePoint < 0x80) {\n if ((units -= 1) < 0) break\n bytes.push(codePoint)\n } else if (codePoint < 0x800) {\n if ((units -= 2) < 0) break\n bytes.push(\n codePoint >> 0x6 | 0xC0,\n codePoint & 0x3F | 0x80\n )\n } else if (codePoint < 0x10000) {\n if ((units -= 3) < 0) break\n bytes.push(\n codePoint >> 0xC | 0xE0,\n codePoint >> 0x6 & 0x3F | 0x80,\n codePoint & 0x3F | 0x80\n )\n } else if (codePoint < 0x110000) {\n if ((units -= 4) < 0) break\n bytes.push(\n codePoint >> 0x12 | 0xF0,\n codePoint >> 0xC & 0x3F | 0x80,\n codePoint >> 0x6 & 0x3F | 0x80,\n codePoint & 0x3F | 0x80\n )\n } else {\n throw new Error('Invalid code point')\n }\n }\n\n return bytes\n}\n\nfunction asciiToBytes (str) {\n var byteArray = []\n for (var i = 0; i < str.length; ++i) {\n // Node's code seems to be doing this and not & 0x7F..\n byteArray.push(str.charCodeAt(i) & 0xFF)\n }\n return byteArray\n}\n\nfunction utf16leToBytes (str, units) {\n var c, hi, lo\n var byteArray = []\n for (var i = 0; i < str.length; ++i) {\n if ((units -= 2) < 0) break\n\n c = str.charCodeAt(i)\n hi = c >> 8\n lo = c % 256\n byteArray.push(lo)\n byteArray.push(hi)\n }\n\n return byteArray\n}\n\nfunction base64ToBytes (str) {\n return base64.toByteArray(base64clean(str))\n}\n\nfunction blitBuffer (src, dst, offset, length) {\n for (var i = 0; i < length; ++i) {\n if ((i + offset >= dst.length) || (i >= src.length)) break\n dst[i + offset] = src[i]\n }\n return i\n}\n\n// ArrayBuffer or Uint8Array objects from other contexts (i.e. iframes) do not pass\n// the `instanceof` check but they should be treated as of that type.\n// See: https://github.com/feross/buffer/issues/166\nfunction isInstance (obj, type) {\n return obj instanceof type ||\n (obj != null && obj.constructor != null && obj.constructor.name != null &&\n obj.constructor.name === type.name)\n}\nfunction numberIsNaN (obj) {\n // For IE11 support\n return obj !== obj // eslint-disable-line no-self-compare\n}\n\n// Create lookup table for `toString('hex')`\n// See: https://github.com/feross/buffer/issues/219\nvar hexSliceLookupTable = (function () {\n var alphabet = '0123456789abcdef'\n var table = new Array(256)\n for (var i = 0; i < 16; ++i) {\n var i16 = i * 16\n for (var j = 0; j < 16; ++j) {\n table[i16 + j] = alphabet[i] + alphabet[j]\n }\n }\n return table\n})()\n","export default {};\n","// shim for using process in browser\n// based off https://github.com/defunctzombie/node-process/blob/master/browser.js\n\nfunction defaultSetTimout() {\n throw new Error('setTimeout has not been defined');\n}\nfunction defaultClearTimeout () {\n throw new Error('clearTimeout has not been defined');\n}\nvar cachedSetTimeout = defaultSetTimout;\nvar cachedClearTimeout = defaultClearTimeout;\nif (typeof global.setTimeout === 'function') {\n cachedSetTimeout = setTimeout;\n}\nif (typeof global.clearTimeout === 'function') {\n cachedClearTimeout = clearTimeout;\n}\n\nfunction runTimeout(fun) {\n if (cachedSetTimeout === setTimeout) {\n //normal enviroments in sane situations\n return setTimeout(fun, 0);\n }\n // if setTimeout wasn't available but was latter defined\n if ((cachedSetTimeout === defaultSetTimout || !cachedSetTimeout) && setTimeout) {\n cachedSetTimeout = setTimeout;\n return setTimeout(fun, 0);\n }\n try {\n // when when somebody has screwed with setTimeout but no I.E. maddness\n return cachedSetTimeout(fun, 0);\n } catch(e){\n try {\n // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally\n return cachedSetTimeout.call(null, fun, 0);\n } catch(e){\n // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error\n return cachedSetTimeout.call(this, fun, 0);\n }\n }\n\n\n}\nfunction runClearTimeout(marker) {\n if (cachedClearTimeout === clearTimeout) {\n //normal enviroments in sane situations\n return clearTimeout(marker);\n }\n // if clearTimeout wasn't available but was latter defined\n if ((cachedClearTimeout === defaultClearTimeout || !cachedClearTimeout) && clearTimeout) {\n cachedClearTimeout = clearTimeout;\n return clearTimeout(marker);\n }\n try {\n // when when somebody has screwed with setTimeout but no I.E. maddness\n return cachedClearTimeout(marker);\n } catch (e){\n try {\n // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally\n return cachedClearTimeout.call(null, marker);\n } catch (e){\n // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error.\n // Some versions of I.E. have different rules for clearTimeout vs setTimeout\n return cachedClearTimeout.call(this, marker);\n }\n }\n\n\n\n}\nvar queue = [];\nvar draining = false;\nvar currentQueue;\nvar queueIndex = -1;\n\nfunction cleanUpNextTick() {\n if (!draining || !currentQueue) {\n return;\n }\n draining = false;\n if (currentQueue.length) {\n queue = currentQueue.concat(queue);\n } else {\n queueIndex = -1;\n }\n if (queue.length) {\n drainQueue();\n }\n}\n\nfunction drainQueue() {\n if (draining) {\n return;\n }\n var timeout = runTimeout(cleanUpNextTick);\n draining = true;\n\n var len = queue.length;\n while(len) {\n currentQueue = queue;\n queue = [];\n while (++queueIndex < len) {\n if (currentQueue) {\n currentQueue[queueIndex].run();\n }\n }\n queueIndex = -1;\n len = queue.length;\n }\n currentQueue = null;\n draining = false;\n runClearTimeout(timeout);\n}\nexport function nextTick(fun) {\n var args = new Array(arguments.length - 1);\n if (arguments.length > 1) {\n for (var i = 1; i < arguments.length; i++) {\n args[i - 1] = arguments[i];\n }\n }\n queue.push(new Item(fun, args));\n if (queue.length === 1 && !draining) {\n runTimeout(drainQueue);\n }\n}\n// v8 likes predictible objects\nfunction Item(fun, array) {\n this.fun = fun;\n this.array = array;\n}\nItem.prototype.run = function () {\n this.fun.apply(null, this.array);\n};\nexport var title = 'browser';\nexport var platform = 'browser';\nexport var browser = true;\nexport var env = {};\nexport var argv = [];\nexport var version = ''; // empty string to avoid regexp issues\nexport var versions = {};\nexport var release = {};\nexport var config = {};\n\nfunction noop() {}\n\nexport var on = noop;\nexport var addListener = noop;\nexport var once = noop;\nexport var off = noop;\nexport var removeListener = noop;\nexport var removeAllListeners = noop;\nexport var emit = noop;\n\nexport function binding(name) {\n throw new Error('process.binding is not supported');\n}\n\nexport function cwd () { return '/' }\nexport function chdir (dir) {\n throw new Error('process.chdir is not supported');\n};\nexport function umask() { return 0; }\n\n// from https://github.com/kumavis/browser-process-hrtime/blob/master/index.js\nvar performance = global.performance || {}\nvar performanceNow =\n performance.now ||\n performance.mozNow ||\n performance.msNow ||\n performance.oNow ||\n performance.webkitNow ||\n function(){ return (new Date()).getTime() }\n\n// generate timestamp or delta\n// see http://nodejs.org/api/process.html#process_process_hrtime\nexport function hrtime(previousTimestamp){\n var clocktime = performanceNow.call(performance)*1e-3\n var seconds = Math.floor(clocktime)\n var nanoseconds = Math.floor((clocktime%1)*1e9)\n if (previousTimestamp) {\n seconds = seconds - previousTimestamp[0]\n nanoseconds = nanoseconds - previousTimestamp[1]\n if (nanoseconds<0) {\n seconds--\n nanoseconds += 1e9\n }\n }\n return [seconds,nanoseconds]\n}\n\nvar startTime = new Date();\nexport function uptime() {\n var currentTime = new Date();\n var dif = currentTime - startTime;\n return dif / 1000;\n}\n\nexport default {\n nextTick: nextTick,\n title: title,\n browser: browser,\n env: env,\n argv: argv,\n version: version,\n versions: versions,\n on: on,\n addListener: addListener,\n once: once,\n off: off,\n removeListener: removeListener,\n removeAllListeners: removeAllListeners,\n emit: emit,\n binding: binding,\n cwd: cwd,\n chdir: chdir,\n umask: umask,\n hrtime: hrtime,\n platform: platform,\n release: release,\n config: config,\n uptime: uptime\n};\n","\nvar inherits;\nif (typeof Object.create === 'function'){\n inherits = function inherits(ctor, superCtor) {\n // implementation from standard node.js 'util' module\n ctor.super_ = superCtor\n ctor.prototype = Object.create(superCtor.prototype, {\n constructor: {\n value: ctor,\n enumerable: false,\n writable: true,\n configurable: true\n }\n });\n };\n} else {\n inherits = function inherits(ctor, superCtor) {\n ctor.super_ = superCtor\n var TempCtor = function () {}\n TempCtor.prototype = superCtor.prototype\n ctor.prototype = new TempCtor()\n ctor.prototype.constructor = ctor\n }\n}\nexport default inherits;\n","// Copyright Joyent, Inc. and other Node contributors.\n//\n// Permission is hereby granted, free of charge, to any person obtaining a\n// copy of this software and associated documentation files (the\n// \"Software\"), to deal in the Software without restriction, including\n// without limitation the rights to use, copy, modify, merge, publish,\n// distribute, sublicense, and/or sell copies of the Software, and to permit\n// persons to whom the Software is furnished to do so, subject to the\n// following conditions:\n//\n// The above copyright notice and this permission notice shall be included\n// in all copies or substantial portions of the Software.\n//\n// THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS\n// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF\n// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN\n// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,\n// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR\n// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE\n// USE OR OTHER DEALINGS IN THE SOFTWARE.\nimport process from 'process';\nvar formatRegExp = /%[sdj%]/g;\nexport function format(f) {\n if (!isString(f)) {\n var objects = [];\n for (var i = 0; i < arguments.length; i++) {\n objects.push(inspect(arguments[i]));\n }\n return objects.join(' ');\n }\n\n var i = 1;\n var args = arguments;\n var len = args.length;\n var str = String(f).replace(formatRegExp, function(x) {\n if (x === '%%') return '%';\n if (i >= len) return x;\n switch (x) {\n case '%s': return String(args[i++]);\n case '%d': return Number(args[i++]);\n case '%j':\n try {\n return JSON.stringify(args[i++]);\n } catch (_) {\n return '[Circular]';\n }\n default:\n return x;\n }\n });\n for (var x = args[i]; i < len; x = args[++i]) {\n if (isNull(x) || !isObject(x)) {\n str += ' ' + x;\n } else {\n str += ' ' + inspect(x);\n }\n }\n return str;\n};\n\n\n// Mark that a method should not be used.\n// Returns a modified function which warns once by default.\n// If --no-deprecation is set, then it is a no-op.\nexport function deprecate(fn, msg) {\n // Allow for deprecating things in the process of starting up.\n if (isUndefined(global.process)) {\n return function() {\n return deprecate(fn, msg).apply(this, arguments);\n };\n }\n\n if (process.noDeprecation === true) {\n return fn;\n }\n\n var warned = false;\n function deprecated() {\n if (!warned) {\n if (process.throwDeprecation) {\n throw new Error(msg);\n } else if (process.traceDeprecation) {\n console.trace(msg);\n } else {\n console.error(msg);\n }\n warned = true;\n }\n return fn.apply(this, arguments);\n }\n\n return deprecated;\n};\n\n\nvar debugs = {};\nvar debugEnviron;\nexport function debuglog(set) {\n if (isUndefined(debugEnviron))\n debugEnviron = process.env.NODE_DEBUG || '';\n set = set.toUpperCase();\n if (!debugs[set]) {\n if (new RegExp('\\\\b' + set + '\\\\b', 'i').test(debugEnviron)) {\n var pid = 0;\n debugs[set] = function() {\n var msg = format.apply(null, arguments);\n console.error('%s %d: %s', set, pid, msg);\n };\n } else {\n debugs[set] = function() {};\n }\n }\n return debugs[set];\n};\n\n\n/**\n * Echos the value of a value. Trys to print the value out\n * in the best way possible given the different types.\n *\n * @param {Object} obj The object to print out.\n * @param {Object} opts Optional options object that alters the output.\n */\n/* legacy: obj, showHidden, depth, colors*/\nexport function inspect(obj, opts) {\n // default options\n var ctx = {\n seen: [],\n stylize: stylizeNoColor\n };\n // legacy...\n if (arguments.length >= 3) ctx.depth = arguments[2];\n if (arguments.length >= 4) ctx.colors = arguments[3];\n if (isBoolean(opts)) {\n // legacy...\n ctx.showHidden = opts;\n } else if (opts) {\n // got an \"options\" object\n _extend(ctx, opts);\n }\n // set default options\n if (isUndefined(ctx.showHidden)) ctx.showHidden = false;\n if (isUndefined(ctx.depth)) ctx.depth = 2;\n if (isUndefined(ctx.colors)) ctx.colors = false;\n if (isUndefined(ctx.customInspect)) ctx.customInspect = true;\n if (ctx.colors) ctx.stylize = stylizeWithColor;\n return formatValue(ctx, obj, ctx.depth);\n}\n\n// http://en.wikipedia.org/wiki/ANSI_escape_code#graphics\ninspect.colors = {\n 'bold' : [1, 22],\n 'italic' : [3, 23],\n 'underline' : [4, 24],\n 'inverse' : [7, 27],\n 'white' : [37, 39],\n 'grey' : [90, 39],\n 'black' : [30, 39],\n 'blue' : [34, 39],\n 'cyan' : [36, 39],\n 'green' : [32, 39],\n 'magenta' : [35, 39],\n 'red' : [31, 39],\n 'yellow' : [33, 39]\n};\n\n// Don't use 'blue' not visible on cmd.exe\ninspect.styles = {\n 'special': 'cyan',\n 'number': 'yellow',\n 'boolean': 'yellow',\n 'undefined': 'grey',\n 'null': 'bold',\n 'string': 'green',\n 'date': 'magenta',\n // \"name\": intentionally not styling\n 'regexp': 'red'\n};\n\n\nfunction stylizeWithColor(str, styleType) {\n var style = inspect.styles[styleType];\n\n if (style) {\n return '\\u001b[' + inspect.colors[style][0] + 'm' + str +\n '\\u001b[' + inspect.colors[style][1] + 'm';\n } else {\n return str;\n }\n}\n\n\nfunction stylizeNoColor(str, styleType) {\n return str;\n}\n\n\nfunction arrayToHash(array) {\n var hash = {};\n\n array.forEach(function(val, idx) {\n hash[val] = true;\n });\n\n return hash;\n}\n\n\nfunction formatValue(ctx, value, recurseTimes) {\n // Provide a hook for user-specified inspect functions.\n // Check that value is an object with an inspect function on it\n if (ctx.customInspect &&\n value &&\n isFunction(value.inspect) &&\n // Filter out the util module, it's inspect function is special\n value.inspect !== inspect &&\n // Also filter out any prototype objects using the circular check.\n !(value.constructor && value.constructor.prototype === value)) {\n var ret = value.inspect(recurseTimes, ctx);\n if (!isString(ret)) {\n ret = formatValue(ctx, ret, recurseTimes);\n }\n return ret;\n }\n\n // Primitive types cannot have properties\n var primitive = formatPrimitive(ctx, value);\n if (primitive) {\n return primitive;\n }\n\n // Look up the keys of the object.\n var keys = Object.keys(value);\n var visibleKeys = arrayToHash(keys);\n\n if (ctx.showHidden) {\n keys = Object.getOwnPropertyNames(value);\n }\n\n // IE doesn't make error fields non-enumerable\n // http://msdn.microsoft.com/en-us/library/ie/dww52sbt(v=vs.94).aspx\n if (isError(value)\n && (keys.indexOf('message') >= 0 || keys.indexOf('description') >= 0)) {\n return formatError(value);\n }\n\n // Some type of object without properties can be shortcutted.\n if (keys.length === 0) {\n if (isFunction(value)) {\n var name = value.name ? ': ' + value.name : '';\n return ctx.stylize('[Function' + name + ']', 'special');\n }\n if (isRegExp(value)) {\n return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');\n }\n if (isDate(value)) {\n return ctx.stylize(Date.prototype.toString.call(value), 'date');\n }\n if (isError(value)) {\n return formatError(value);\n }\n }\n\n var base = '', array = false, braces = ['{', '}'];\n\n // Make Array say that they are Array\n if (isArray(value)) {\n array = true;\n braces = ['[', ']'];\n }\n\n // Make functions say that they are functions\n if (isFunction(value)) {\n var n = value.name ? ': ' + value.name : '';\n base = ' [Function' + n + ']';\n }\n\n // Make RegExps say that they are RegExps\n if (isRegExp(value)) {\n base = ' ' + RegExp.prototype.toString.call(value);\n }\n\n // Make dates with properties first say the date\n if (isDate(value)) {\n base = ' ' + Date.prototype.toUTCString.call(value);\n }\n\n // Make error with message first say the error\n if (isError(value)) {\n base = ' ' + formatError(value);\n }\n\n if (keys.length === 0 && (!array || value.length == 0)) {\n return braces[0] + base + braces[1];\n }\n\n if (recurseTimes < 0) {\n if (isRegExp(value)) {\n return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');\n } else {\n return ctx.stylize('[Object]', 'special');\n }\n }\n\n ctx.seen.push(value);\n\n var output;\n if (array) {\n output = formatArray(ctx, value, recurseTimes, visibleKeys, keys);\n } else {\n output = keys.map(function(key) {\n return formatProperty(ctx, value, recurseTimes, visibleKeys, key, array);\n });\n }\n\n ctx.seen.pop();\n\n return reduceToSingleString(output, base, braces);\n}\n\n\nfunction formatPrimitive(ctx, value) {\n if (isUndefined(value))\n return ctx.stylize('undefined', 'undefined');\n if (isString(value)) {\n var simple = '\\'' + JSON.stringify(value).replace(/^\"|\"$/g, '')\n .replace(/'/g, \"\\\\'\")\n .replace(/\\\\\"/g, '\"') + '\\'';\n return ctx.stylize(simple, 'string');\n }\n if (isNumber(value))\n return ctx.stylize('' + value, 'number');\n if (isBoolean(value))\n return ctx.stylize('' + value, 'boolean');\n // For some reason typeof null is \"object\", so special case here.\n if (isNull(value))\n return ctx.stylize('null', 'null');\n}\n\n\nfunction formatError(value) {\n return '[' + Error.prototype.toString.call(value) + ']';\n}\n\n\nfunction formatArray(ctx, value, recurseTimes, visibleKeys, keys) {\n var output = [];\n for (var i = 0, l = value.length; i < l; ++i) {\n if (hasOwnProperty(value, String(i))) {\n output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,\n String(i), true));\n } else {\n output.push('');\n }\n }\n keys.forEach(function(key) {\n if (!key.match(/^\\d+$/)) {\n output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,\n key, true));\n }\n });\n return output;\n}\n\n\nfunction formatProperty(ctx, value, recurseTimes, visibleKeys, key, array) {\n var name, str, desc;\n desc = Object.getOwnPropertyDescriptor(value, key) || { value: value[key] };\n if (desc.get) {\n if (desc.set) {\n str = ctx.stylize('[Getter/Setter]', 'special');\n } else {\n str = ctx.stylize('[Getter]', 'special');\n }\n } else {\n if (desc.set) {\n str = ctx.stylize('[Setter]', 'special');\n }\n }\n if (!hasOwnProperty(visibleKeys, key)) {\n name = '[' + key + ']';\n }\n if (!str) {\n if (ctx.seen.indexOf(desc.value) < 0) {\n if (isNull(recurseTimes)) {\n str = formatValue(ctx, desc.value, null);\n } else {\n str = formatValue(ctx, desc.value, recurseTimes - 1);\n }\n if (str.indexOf('\\n') > -1) {\n if (array) {\n str = str.split('\\n').map(function(line) {\n return ' ' + line;\n }).join('\\n').substr(2);\n } else {\n str = '\\n' + str.split('\\n').map(function(line) {\n return ' ' + line;\n }).join('\\n');\n }\n }\n } else {\n str = ctx.stylize('[Circular]', 'special');\n }\n }\n if (isUndefined(name)) {\n if (array && key.match(/^\\d+$/)) {\n return str;\n }\n name = JSON.stringify('' + key);\n if (name.match(/^\"([a-zA-Z_][a-zA-Z_0-9]*)\"$/)) {\n name = name.substr(1, name.length - 2);\n name = ctx.stylize(name, 'name');\n } else {\n name = name.replace(/'/g, \"\\\\'\")\n .replace(/\\\\\"/g, '\"')\n .replace(/(^\"|\"$)/g, \"'\");\n name = ctx.stylize(name, 'string');\n }\n }\n\n return name + ': ' + str;\n}\n\n\nfunction reduceToSingleString(output, base, braces) {\n var numLinesEst = 0;\n var length = output.reduce(function(prev, cur) {\n numLinesEst++;\n if (cur.indexOf('\\n') >= 0) numLinesEst++;\n return prev + cur.replace(/\\u001b\\[\\d\\d?m/g, '').length + 1;\n }, 0);\n\n if (length > 60) {\n return braces[0] +\n (base === '' ? '' : base + '\\n ') +\n ' ' +\n output.join(',\\n ') +\n ' ' +\n braces[1];\n }\n\n return braces[0] + base + ' ' + output.join(', ') + ' ' + braces[1];\n}\n\n\n// NOTE: These type checking functions intentionally don't use `instanceof`\n// because it is fragile and can be easily faked with `Object.create()`.\nexport function isArray(ar) {\n return Array.isArray(ar);\n}\n\nexport function isBoolean(arg) {\n return typeof arg === 'boolean';\n}\n\nexport function isNull(arg) {\n return arg === null;\n}\n\nexport function isNullOrUndefined(arg) {\n return arg == null;\n}\n\nexport function isNumber(arg) {\n return typeof arg === 'number';\n}\n\nexport function isString(arg) {\n return typeof arg === 'string';\n}\n\nexport function isSymbol(arg) {\n return typeof arg === 'symbol';\n}\n\nexport function isUndefined(arg) {\n return arg === void 0;\n}\n\nexport function isRegExp(re) {\n return isObject(re) && objectToString(re) === '[object RegExp]';\n}\n\nexport function isObject(arg) {\n return typeof arg === 'object' && arg !== null;\n}\n\nexport function isDate(d) {\n return isObject(d) && objectToString(d) === '[object Date]';\n}\n\nexport function isError(e) {\n return isObject(e) &&\n (objectToString(e) === '[object Error]' || e instanceof Error);\n}\n\nexport function isFunction(arg) {\n return typeof arg === 'function';\n}\n\nexport function isPrimitive(arg) {\n return arg === null ||\n typeof arg === 'boolean' ||\n typeof arg === 'number' ||\n typeof arg === 'string' ||\n typeof arg === 'symbol' || // ES6 symbol\n typeof arg === 'undefined';\n}\n\nexport function isBuffer(maybeBuf) {\n return Buffer.isBuffer(maybeBuf);\n}\n\nfunction objectToString(o) {\n return Object.prototype.toString.call(o);\n}\n\n\nfunction pad(n) {\n return n < 10 ? '0' + n.toString(10) : n.toString(10);\n}\n\n\nvar months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep',\n 'Oct', 'Nov', 'Dec'];\n\n// 26 Feb 16:19:34\nfunction timestamp() {\n var d = new Date();\n var time = [pad(d.getHours()),\n pad(d.getMinutes()),\n pad(d.getSeconds())].join(':');\n return [d.getDate(), months[d.getMonth()], time].join(' ');\n}\n\n\n// log is just a thin wrapper to console.log that prepends a timestamp\nexport function log() {\n console.log('%s - %s', timestamp(), format.apply(null, arguments));\n}\n\n\n/**\n * Inherit the prototype methods from one constructor into another.\n *\n * The Function.prototype.inherits from lang.js rewritten as a standalone\n * function (not on Function.prototype). NOTE: If this file is to be loaded\n * during bootstrapping this function needs to be rewritten using some native\n * functions as prototype setup using normal JavaScript does not work as\n * expected during bootstrapping (see mirror.js in r114903).\n *\n * @param {function} ctor Constructor function which needs to inherit the\n * prototype.\n * @param {function} superCtor Constructor function to inherit prototype from.\n */\nimport inherits from './inherits';\nexport {inherits}\n\nexport function _extend(origin, add) {\n // Don't do anything if add isn't an object\n if (!add || !isObject(add)) return origin;\n\n var keys = Object.keys(add);\n var i = keys.length;\n while (i--) {\n origin[keys[i]] = add[keys[i]];\n }\n return origin;\n};\n\nfunction hasOwnProperty(obj, prop) {\n return Object.prototype.hasOwnProperty.call(obj, prop);\n}\n\nexport default {\n inherits: inherits,\n _extend: _extend,\n log: log,\n isBuffer: isBuffer,\n isPrimitive: isPrimitive,\n isFunction: isFunction,\n isError: isError,\n isDate: isDate,\n isObject: isObject,\n isRegExp: isRegExp,\n isUndefined: isUndefined,\n isSymbol: isSymbol,\n isString: isString,\n isNumber: isNumber,\n isNullOrUndefined: isNullOrUndefined,\n isNull: isNull,\n isBoolean: isBoolean,\n isArray: isArray,\n inspect: inspect,\n deprecate: deprecate,\n format: format,\n debuglog: debuglog\n}\n",null,null,null,null,null,null,null,null,null,null,null,null,null,null,null,null,"/*! *****************************************************************************\r\nCopyright (c) Microsoft Corporation.\r\n\r\nPermission to use, copy, modify, and/or distribute this software for any\r\npurpose with or without fee is hereby granted.\r\n\r\nTHE SOFTWARE IS PROVIDED \"AS IS\" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH\r\nREGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY\r\nAND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,\r\nINDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM\r\nLOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR\r\nOTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR\r\nPERFORMANCE OF THIS SOFTWARE.\r\n***************************************************************************** */\r\n/* global Reflect, Promise */\r\n\r\nvar extendStatics = function(d, b) {\r\n extendStatics = Object.setPrototypeOf ||\r\n ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||\r\n function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; };\r\n return extendStatics(d, b);\r\n};\r\n\r\nexport function __extends(d, b) {\r\n extendStatics(d, b);\r\n function __() { this.constructor = d; }\r\n d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());\r\n}\r\n\r\nexport var __assign = function() {\r\n __assign = Object.assign || function __assign(t) {\r\n for (var s, i = 1, n = arguments.length; i < n; i++) {\r\n s = arguments[i];\r\n for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p];\r\n }\r\n return t;\r\n }\r\n return __assign.apply(this, arguments);\r\n}\r\n\r\nexport function __rest(s, e) {\r\n var t = {};\r\n for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p) && e.indexOf(p) < 0)\r\n t[p] = s[p];\r\n if (s != null && typeof Object.getOwnPropertySymbols === \"function\")\r\n for (var i = 0, p = Object.getOwnPropertySymbols(s); i < p.length; i++) {\r\n if (e.indexOf(p[i]) < 0 && Object.prototype.propertyIsEnumerable.call(s, p[i]))\r\n t[p[i]] = s[p[i]];\r\n }\r\n return t;\r\n}\r\n\r\nexport function __decorate(decorators, target, key, desc) {\r\n var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;\r\n if (typeof Reflect === \"object\" && typeof Reflect.decorate === \"function\") r = Reflect.decorate(decorators, target, key, desc);\r\n else for (var i = decorators.length - 1; i >= 0; i--) if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;\r\n return c > 3 && r && Object.defineProperty(target, key, r), r;\r\n}\r\n\r\nexport function __param(paramIndex, decorator) {\r\n return function (target, key) { decorator(target, key, paramIndex); }\r\n}\r\n\r\nexport function __metadata(metadataKey, metadataValue) {\r\n if (typeof Reflect === \"object\" && typeof Reflect.metadata === \"function\") return Reflect.metadata(metadataKey, metadataValue);\r\n}\r\n\r\nexport function __awaiter(thisArg, _arguments, P, generator) {\r\n function adopt(value) { return value instanceof P ? value : new P(function (resolve) { resolve(value); }); }\r\n return new (P || (P = Promise))(function (resolve, reject) {\r\n function fulfilled(value) { try { step(generator.next(value)); } catch (e) { reject(e); } }\r\n function rejected(value) { try { step(generator[\"throw\"](value)); } catch (e) { reject(e); } }\r\n function step(result) { result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected); }\r\n step((generator = generator.apply(thisArg, _arguments || [])).next());\r\n });\r\n}\r\n\r\nexport function __generator(thisArg, body) {\r\n var _ = { label: 0, sent: function() { if (t[0] & 1) throw t[1]; return t[1]; }, trys: [], ops: [] }, f, y, t, g;\r\n return g = { next: verb(0), \"throw\": verb(1), \"return\": verb(2) }, typeof Symbol === \"function\" && (g[Symbol.iterator] = function() { return this; }), g;\r\n function verb(n) { return function (v) { return step([n, v]); }; }\r\n function step(op) {\r\n if (f) throw new TypeError(\"Generator is already executing.\");\r\n while (_) try {\r\n if (f = 1, y && (t = op[0] & 2 ? y[\"return\"] : op[0] ? y[\"throw\"] || ((t = y[\"return\"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done) return t;\r\n if (y = 0, t) op = [op[0] & 2, t.value];\r\n switch (op[0]) {\r\n case 0: case 1: t = op; break;\r\n case 4: _.label++; return { value: op[1], done: false };\r\n case 5: _.label++; y = op[1]; op = [0]; continue;\r\n case 7: op = _.ops.pop(); _.trys.pop(); continue;\r\n default:\r\n if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) { _ = 0; continue; }\r\n if (op[0] === 3 && (!t || (op[1] > t[0] && op[1] < t[3]))) { _.label = op[1]; break; }\r\n if (op[0] === 6 && _.label < t[1]) { _.label = t[1]; t = op; break; }\r\n if (t && _.label < t[2]) { _.label = t[2]; _.ops.push(op); break; }\r\n if (t[2]) _.ops.pop();\r\n _.trys.pop(); continue;\r\n }\r\n op = body.call(thisArg, _);\r\n } catch (e) { op = [6, e]; y = 0; } finally { f = t = 0; }\r\n if (op[0] & 5) throw op[1]; return { value: op[0] ? op[1] : void 0, done: true };\r\n }\r\n}\r\n\r\nexport function __createBinding(o, m, k, k2) {\r\n if (k2 === undefined) k2 = k;\r\n o[k2] = m[k];\r\n}\r\n\r\nexport function __exportStar(m, exports) {\r\n for (var p in m) if (p !== \"default\" && !exports.hasOwnProperty(p)) exports[p] = m[p];\r\n}\r\n\r\nexport function __values(o) {\r\n var s = typeof Symbol === \"function\" && Symbol.iterator, m = s && o[s], i = 0;\r\n if (m) return m.call(o);\r\n if (o && typeof o.length === \"number\") return {\r\n next: function () {\r\n if (o && i >= o.length) o = void 0;\r\n return { value: o && o[i++], done: !o };\r\n }\r\n };\r\n throw new TypeError(s ? \"Object is not iterable.\" : \"Symbol.iterator is not defined.\");\r\n}\r\n\r\nexport function __read(o, n) {\r\n var m = typeof Symbol === \"function\" && o[Symbol.iterator];\r\n if (!m) return o;\r\n var i = m.call(o), r, ar = [], e;\r\n try {\r\n while ((n === void 0 || n-- > 0) && !(r = i.next()).done) ar.push(r.value);\r\n }\r\n catch (error) { e = { error: error }; }\r\n finally {\r\n try {\r\n if (r && !r.done && (m = i[\"return\"])) m.call(i);\r\n }\r\n finally { if (e) throw e.error; }\r\n }\r\n return ar;\r\n}\r\n\r\nexport function __spread() {\r\n for (var ar = [], i = 0; i < arguments.length; i++)\r\n ar = ar.concat(__read(arguments[i]));\r\n return ar;\r\n}\r\n\r\nexport function __spreadArrays() {\r\n for (var s = 0, i = 0, il = arguments.length; i < il; i++) s += arguments[i].length;\r\n for (var r = Array(s), k = 0, i = 0; i < il; i++)\r\n for (var a = arguments[i], j = 0, jl = a.length; j < jl; j++, k++)\r\n r[k] = a[j];\r\n return r;\r\n};\r\n\r\nexport function __await(v) {\r\n return this instanceof __await ? (this.v = v, this) : new __await(v);\r\n}\r\n\r\nexport function __asyncGenerator(thisArg, _arguments, generator) {\r\n if (!Symbol.asyncIterator) throw new TypeError(\"Symbol.asyncIterator is not defined.\");\r\n var g = generator.apply(thisArg, _arguments || []), i, q = [];\r\n return i = {}, verb(\"next\"), verb(\"throw\"), verb(\"return\"), i[Symbol.asyncIterator] = function () { return this; }, i;\r\n function verb(n) { if (g[n]) i[n] = function (v) { return new Promise(function (a, b) { q.push([n, v, a, b]) > 1 || resume(n, v); }); }; }\r\n function resume(n, v) { try { step(g[n](v)); } catch (e) { settle(q[0][3], e); } }\r\n function step(r) { r.value instanceof __await ? Promise.resolve(r.value.v).then(fulfill, reject) : settle(q[0][2], r); }\r\n function fulfill(value) { resume(\"next\", value); }\r\n function reject(value) { resume(\"throw\", value); }\r\n function settle(f, v) { if (f(v), q.shift(), q.length) resume(q[0][0], q[0][1]); }\r\n}\r\n\r\nexport function __asyncDelegator(o) {\r\n var i, p;\r\n return i = {}, verb(\"next\"), verb(\"throw\", function (e) { throw e; }), verb(\"return\"), i[Symbol.iterator] = function () { return this; }, i;\r\n function verb(n, f) { i[n] = o[n] ? function (v) { return (p = !p) ? { value: __await(o[n](v)), done: n === \"return\" } : f ? f(v) : v; } : f; }\r\n}\r\n\r\nexport function __asyncValues(o) {\r\n if (!Symbol.asyncIterator) throw new TypeError(\"Symbol.asyncIterator is not defined.\");\r\n var m = o[Symbol.asyncIterator], i;\r\n return m ? m.call(o) : (o = typeof __values === \"function\" ? __values(o) : o[Symbol.iterator](), i = {}, verb(\"next\"), verb(\"throw\"), verb(\"return\"), i[Symbol.asyncIterator] = function () { return this; }, i);\r\n function verb(n) { i[n] = o[n] && function (v) { return new Promise(function (resolve, reject) { v = o[n](v), settle(resolve, reject, v.done, v.value); }); }; }\r\n function settle(resolve, reject, d, v) { Promise.resolve(v).then(function(v) { resolve({ value: v, done: d }); }, reject); }\r\n}\r\n\r\nexport function __makeTemplateObject(cooked, raw) {\r\n if (Object.defineProperty) { Object.defineProperty(cooked, \"raw\", { value: raw }); } else { cooked.raw = raw; }\r\n return cooked;\r\n};\r\n\r\nexport function __importStar(mod) {\r\n if (mod && mod.__esModule) return mod;\r\n var result = {};\r\n if (mod != null) for (var k in mod) if (Object.hasOwnProperty.call(mod, k)) result[k] = mod[k];\r\n result.default = mod;\r\n return result;\r\n}\r\n\r\nexport function __importDefault(mod) {\r\n return (mod && mod.__esModule) ? mod : { default: mod };\r\n}\r\n\r\nexport function __classPrivateFieldGet(receiver, privateMap) {\r\n if (!privateMap.has(receiver)) {\r\n throw new TypeError(\"attempted to get private field on non-instance\");\r\n }\r\n return privateMap.get(receiver);\r\n}\r\n\r\nexport function __classPrivateFieldSet(receiver, privateMap, value) {\r\n if (!privateMap.has(receiver)) {\r\n throw new TypeError(\"attempted to set private field on non-instance\");\r\n }\r\n privateMap.set(receiver, value);\r\n return value;\r\n}\r\n",null,null,null,null,null,null,null,null,null,null],"names":["byteLength","toByteArray","fromByteArray","lookup","revLookup","Arr","Uint8Array","Array","code","i","len","length","charCodeAt","getLens","b64","Error","validLen","indexOf","placeHoldersLen","lens","_byteLength","tmp","arr","curByte","tripletToBase64","num","encodeChunk","uint8","start","end","output","push","join","extraBytes","parts","maxChunkLength","len2","buffer","offset","isLE","mLen","nBytes","e","m","eLen","eMax","eBias","nBits","d","s","NaN","Infinity","Math","pow","value","c","rt","abs","isNaN","floor","log","LN2","customInspectSymbol","Symbol","exports","Buffer","SlowBuffer","K_MAX_LENGTH","TYPED_ARRAY_SUPPORT","typedArraySupport","console","error","proto","foo","Object","setPrototypeOf","prototype","defineProperty","enumerable","get","isBuffer","undefined","byteOffset","createBuffer","RangeError","buf","arg","encodingOrOffset","TypeError","allocUnsafe","from","poolSize","fromString","ArrayBuffer","isView","fromArrayView","isInstance","fromArrayBuffer","SharedArrayBuffer","valueOf","b","fromObject","toPrimitive","assertSize","size","alloc","fill","encoding","checked","allocUnsafeSlow","string","isEncoding","actual","write","slice","fromArrayLike","array","arrayView","copy","obj","numberIsNaN","type","isArray","data","toString","_isBuffer","compare","a","x","y","min","String","toLowerCase","concat","list","pos","set","call","mustMatch","arguments","loweredCase","utf8ToBytes","base64ToBytes","slowToString","hexSlice","utf8Slice","asciiSlice","latin1Slice","base64Slice","utf16leSlice","swap","n","swap16","swap32","swap64","apply","toLocaleString","equals","inspect","str","max","INSPECT_MAX_BYTES","replace","trim","target","thisStart","thisEnd","thisCopy","targetCopy","bidirectionalIndexOf","val","dir","arrayIndexOf","lastIndexOf","indexSize","arrLength","valLength","read","readUInt16BE","foundIndex","found","j","includes","hexWrite","Number","remaining","strLen","parsed","parseInt","substr","utf8Write","blitBuffer","asciiWrite","asciiToBytes","base64Write","ucs2Write","utf16leToBytes","isFinite","toJSON","_arr","base64","res","firstByte","codePoint","bytesPerSequence","secondByte","thirdByte","fourthByte","tempCodePoint","decodeCodePointsArray","MAX_ARGUMENTS_LENGTH","codePoints","fromCharCode","ret","out","hexSliceLookupTable","bytes","newBuf","subarray","checkOffset","ext","readUintLE","readUIntLE","noAssert","mul","readUintBE","readUIntBE","readUint8","readUInt8","readUint16LE","readUInt16LE","readUint16BE","readUint32LE","readUInt32LE","readUint32BE","readUInt32BE","readIntLE","readIntBE","readInt8","readInt16LE","readInt16BE","readInt32LE","readInt32BE","readFloatLE","ieee754","readFloatBE","readDoubleLE","readDoubleBE","checkInt","writeUintLE","writeUIntLE","maxBytes","writeUintBE","writeUIntBE","writeUint8","writeUInt8","writeUint16LE","writeUInt16LE","writeUint16BE","writeUInt16BE","writeUint32LE","writeUInt32LE","writeUint32BE","writeUInt32BE","writeIntLE","limit","sub","writeIntBE","writeInt8","writeInt16LE","writeInt16BE","writeInt32LE","writeInt32BE","checkIEEE754","writeFloat","littleEndian","writeFloatLE","writeFloatBE","writeDouble","writeDoubleLE","writeDoubleBE","targetStart","copyWithin","INVALID_BASE64_RE","base64clean","split","units","leadSurrogate","byteArray","hi","lo","src","dst","constructor","name","alphabet","table","i16","performance","global","now","mozNow","msNow","oNow","webkitNow","Date","getTime","inherits","create","ctor","superCtor","super_","writable","configurable","TempCtor","formatRegExp","format","f","isString","objects","args","JSON","stringify","_","isNull","isObject","deprecate","fn","msg","isUndefined","process","warned","deprecated","debugs","debugEnviron","debuglog","toUpperCase","RegExp","test","pid","opts","ctx","seen","stylize","stylizeNoColor","depth","colors","isBoolean","showHidden","_extend","customInspect","stylizeWithColor","formatValue","styles","styleType","style","arrayToHash","hash","forEach","idx","recurseTimes","isFunction","primitive","formatPrimitive","keys","visibleKeys","getOwnPropertyNames","isError","formatError","isRegExp","isDate","base","braces","toUTCString","formatArray","map","key","formatProperty","pop","reduceToSingleString","simple","isNumber","l","hasOwnProperty","match","desc","getOwnPropertyDescriptor","line","reduce","prev","cur","numLinesEst","ar","isNullOrUndefined","isSymbol","re","objectToString","isPrimitive","maybeBuf","o","pad","months","timestamp","time","getHours","getMinutes","getSeconds","getDate","getMonth","origin","add","prop","buffer_1","utils_1","ensure_buffer_1","uuid_utils_1","binary_1","uuid_1","extendStatics","__proto__","p","__extends","__","__assign","assign","t","__rest","getOwnPropertySymbols","propertyIsEnumerable","__decorate","decorators","r","Reflect","decorate","__param","paramIndex","decorator","__metadata","metadataKey","metadataValue","metadata","__awaiter","thisArg","_arguments","P","generator","adopt","resolve","Promise","reject","fulfilled","step","next","rejected","result","done","then","__generator","body","label","sent","trys","ops","g","verb","iterator","v","op","__createBinding","k","k2","__exportStar","__values","__read","__spread","__spreadArrays","il","jl","__await","__asyncGenerator","asyncIterator","q","resume","settle","fulfill","shift","__asyncDelegator","__asyncValues","__makeTemplateObject","cooked","raw","__importStar","mod","__esModule","__importDefault","__classPrivateFieldGet","receiver","privateMap","has","__classPrivateFieldSet","tslib_1","objectid_1","symbol_1","int_32_1","decimal128_1","min_key_1","max_key_1","regexp_1","timestamp_1","code_1","db_ref_1","validate_utf8_1","decimal128","symbol","float_parser_1","extended_json_1","map_1","serializer_1","deserializer_1","calculate_size_1"],"mappings":";;;;;;;;;;;;;;;;CAEA,gBAAkB,GAAGA,UAArB;CACA,iBAAmB,GAAGC,WAAtB;CACA,mBAAqB,GAAGC,aAAxB;CAEA,IAAIC,MAAM,GAAG,EAAb;CACA,IAAIC,SAAS,GAAG,EAAhB;CACA,IAAIC,GAAG,GAAG,OAAOC,UAAP,KAAsB,WAAtB,GAAoCA,UAApC,GAAiDC,KAA3D;CAEA,IAAIC,MAAI,GAAG,kEAAX;;CACA,KAAK,IAAIC,CAAC,GAAG,CAAR,EAAWC,GAAG,GAAGF,MAAI,CAACG,MAA3B,EAAmCF,CAAC,GAAGC,GAAvC,EAA4C,EAAED,CAA9C,EAAiD;CAC/CN,EAAAA,MAAM,CAACM,CAAD,CAAN,GAAYD,MAAI,CAACC,CAAD,CAAhB;CACAL,EAAAA,SAAS,CAACI,MAAI,CAACI,UAAL,CAAgBH,CAAhB,CAAD,CAAT,GAAgCA,CAAhC;CACD;CAGD;;;CACAL,SAAS,CAAC,IAAIQ,UAAJ,CAAe,CAAf,CAAD,CAAT,GAA+B,EAA/B;CACAR,SAAS,CAAC,IAAIQ,UAAJ,CAAe,CAAf,CAAD,CAAT,GAA+B,EAA/B;;CAEA,SAASC,OAAT,CAAkBC,GAAlB,EAAuB;CACrB,MAAIJ,GAAG,GAAGI,GAAG,CAACH,MAAd;;CAEA,MAAID,GAAG,GAAG,CAAN,GAAU,CAAd,EAAiB;CACf,UAAM,IAAIK,KAAJ,CAAU,gDAAV,CAAN;CACD,GALoB;;;;CASrB,MAAIC,QAAQ,GAAGF,GAAG,CAACG,OAAJ,CAAY,GAAZ,CAAf;CACA,MAAID,QAAQ,KAAK,CAAC,CAAlB,EAAqBA,QAAQ,GAAGN,GAAX;CAErB,MAAIQ,eAAe,GAAGF,QAAQ,KAAKN,GAAb,GAClB,CADkB,GAElB,IAAKM,QAAQ,GAAG,CAFpB;CAIA,SAAO,CAACA,QAAD,EAAWE,eAAX,CAAP;CACD;;;CAGD,SAASlB,UAAT,CAAqBc,GAArB,EAA0B;CACxB,MAAIK,IAAI,GAAGN,OAAO,CAACC,GAAD,CAAlB;CACA,MAAIE,QAAQ,GAAGG,IAAI,CAAC,CAAD,CAAnB;CACA,MAAID,eAAe,GAAGC,IAAI,CAAC,CAAD,CAA1B;CACA,SAAQ,CAACH,QAAQ,GAAGE,eAAZ,IAA+B,CAA/B,GAAmC,CAApC,GAAyCA,eAAhD;CACD;;CAED,SAASE,WAAT,CAAsBN,GAAtB,EAA2BE,QAA3B,EAAqCE,eAArC,EAAsD;CACpD,SAAQ,CAACF,QAAQ,GAAGE,eAAZ,IAA+B,CAA/B,GAAmC,CAApC,GAAyCA,eAAhD;CACD;;CAED,SAASjB,WAAT,CAAsBa,GAAtB,EAA2B;CACzB,MAAIO,GAAJ;CACA,MAAIF,IAAI,GAAGN,OAAO,CAACC,GAAD,CAAlB;CACA,MAAIE,QAAQ,GAAGG,IAAI,CAAC,CAAD,CAAnB;CACA,MAAID,eAAe,GAAGC,IAAI,CAAC,CAAD,CAA1B;CAEA,MAAIG,GAAG,GAAG,IAAIjB,GAAJ,CAAQe,WAAW,CAACN,GAAD,EAAME,QAAN,EAAgBE,eAAhB,CAAnB,CAAV;CAEA,MAAIK,OAAO,GAAG,CAAd,CARyB;;CAWzB,MAAIb,GAAG,GAAGQ,eAAe,GAAG,CAAlB,GACNF,QAAQ,GAAG,CADL,GAENA,QAFJ;CAIA,MAAIP,CAAJ;;CACA,OAAKA,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAGC,GAAhB,EAAqBD,CAAC,IAAI,CAA1B,EAA6B;CAC3BY,IAAAA,GAAG,GACAjB,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAf,CAAD,CAAT,IAAgC,EAAjC,GACCL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,EADrC,GAECL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CAFrC,GAGAL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAJX;CAKAa,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAkBF,GAAG,IAAI,EAAR,GAAc,IAA/B;CACAC,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAkBF,GAAG,IAAI,CAAR,GAAa,IAA9B;CACAC,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAiBF,GAAG,GAAG,IAAvB;CACD;;CAED,MAAIH,eAAe,KAAK,CAAxB,EAA2B;CACzBG,IAAAA,GAAG,GACAjB,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAf,CAAD,CAAT,IAAgC,CAAjC,GACCL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CAFvC;CAGAa,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAiBF,GAAG,GAAG,IAAvB;CACD;;CAED,MAAIH,eAAe,KAAK,CAAxB,EAA2B;CACzBG,IAAAA,GAAG,GACAjB,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAf,CAAD,CAAT,IAAgC,EAAjC,GACCL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CADrC,GAECL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CAHvC;CAIAa,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAkBF,GAAG,IAAI,CAAR,GAAa,IAA9B;CACAC,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAiBF,GAAG,GAAG,IAAvB;CACD;;CAED,SAAOC,GAAP;CACD;;CAED,SAASE,eAAT,CAA0BC,GAA1B,EAA+B;CAC7B,SAAOtB,MAAM,CAACsB,GAAG,IAAI,EAAP,GAAY,IAAb,CAAN,GACLtB,MAAM,CAACsB,GAAG,IAAI,EAAP,GAAY,IAAb,CADD,GAELtB,MAAM,CAACsB,GAAG,IAAI,CAAP,GAAW,IAAZ,CAFD,GAGLtB,MAAM,CAACsB,GAAG,GAAG,IAAP,CAHR;CAID;;CAED,SAASC,WAAT,CAAsBC,KAAtB,EAA6BC,KAA7B,EAAoCC,GAApC,EAAyC;CACvC,MAAIR,GAAJ;CACA,MAAIS,MAAM,GAAG,EAAb;;CACA,OAAK,IAAIrB,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6BpB,CAAC,IAAI,CAAlC,EAAqC;CACnCY,IAAAA,GAAG,GACD,CAAEM,KAAK,CAAClB,CAAD,CAAL,IAAY,EAAb,GAAmB,QAApB,KACEkB,KAAK,CAAClB,CAAC,GAAG,CAAL,CAAL,IAAgB,CAAjB,GAAsB,MADvB,KAECkB,KAAK,CAAClB,CAAC,GAAG,CAAL,CAAL,GAAe,IAFhB,CADF;CAIAqB,IAAAA,MAAM,CAACC,IAAP,CAAYP,eAAe,CAACH,GAAD,CAA3B;CACD;;CACD,SAAOS,MAAM,CAACE,IAAP,CAAY,EAAZ,CAAP;CACD;;CAED,SAAS9B,aAAT,CAAwByB,KAAxB,EAA+B;CAC7B,MAAIN,GAAJ;CACA,MAAIX,GAAG,GAAGiB,KAAK,CAAChB,MAAhB;CACA,MAAIsB,UAAU,GAAGvB,GAAG,GAAG,CAAvB,CAH6B;;CAI7B,MAAIwB,KAAK,GAAG,EAAZ;CACA,MAAIC,cAAc,GAAG,KAArB,CAL6B;;;CAQ7B,OAAK,IAAI1B,CAAC,GAAG,CAAR,EAAW2B,IAAI,GAAG1B,GAAG,GAAGuB,UAA7B,EAAyCxB,CAAC,GAAG2B,IAA7C,EAAmD3B,CAAC,IAAI0B,cAAxD,EAAwE;CACtED,IAAAA,KAAK,CAACH,IAAN,CAAWL,WAAW,CAACC,KAAD,EAAQlB,CAAR,EAAYA,CAAC,GAAG0B,cAAL,GAAuBC,IAAvB,GAA8BA,IAA9B,GAAsC3B,CAAC,GAAG0B,cAArD,CAAtB;CACD,GAV4B;;;CAa7B,MAAIF,UAAU,KAAK,CAAnB,EAAsB;CACpBZ,IAAAA,GAAG,GAAGM,KAAK,CAACjB,GAAG,GAAG,CAAP,CAAX;CACAwB,IAAAA,KAAK,CAACH,IAAN,CACE5B,MAAM,CAACkB,GAAG,IAAI,CAAR,CAAN,GACAlB,MAAM,CAAEkB,GAAG,IAAI,CAAR,GAAa,IAAd,CADN,GAEA,IAHF;CAKD,GAPD,MAOO,IAAIY,UAAU,KAAK,CAAnB,EAAsB;CAC3BZ,IAAAA,GAAG,GAAG,CAACM,KAAK,CAACjB,GAAG,GAAG,CAAP,CAAL,IAAkB,CAAnB,IAAwBiB,KAAK,CAACjB,GAAG,GAAG,CAAP,CAAnC;CACAwB,IAAAA,KAAK,CAACH,IAAN,CACE5B,MAAM,CAACkB,GAAG,IAAI,EAAR,CAAN,GACAlB,MAAM,CAAEkB,GAAG,IAAI,CAAR,GAAa,IAAd,CADN,GAEAlB,MAAM,CAAEkB,GAAG,IAAI,CAAR,GAAa,IAAd,CAFN,GAGA,GAJF;CAMD;;CAED,SAAOa,KAAK,CAACF,IAAN,CAAW,EAAX,CAAP;;;;;;;;;CCpJF;CACA,QAAY,GAAG,aAAA,CAAUK,MAAV,EAAkBC,MAAlB,EAA0BC,IAA1B,EAAgCC,IAAhC,EAAsCC,MAAtC,EAA8C;CAC3D,MAAIC,CAAJ,EAAOC,CAAP;CACA,MAAIC,IAAI,GAAIH,MAAM,GAAG,CAAV,GAAeD,IAAf,GAAsB,CAAjC;CACA,MAAIK,IAAI,GAAG,CAAC,KAAKD,IAAN,IAAc,CAAzB;CACA,MAAIE,KAAK,GAAGD,IAAI,IAAI,CAApB;CACA,MAAIE,KAAK,GAAG,CAAC,CAAb;CACA,MAAItC,CAAC,GAAG8B,IAAI,GAAIE,MAAM,GAAG,CAAb,GAAkB,CAA9B;CACA,MAAIO,CAAC,GAAGT,IAAI,GAAG,CAAC,CAAJ,GAAQ,CAApB;CACA,MAAIU,CAAC,GAAGZ,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAd;CAEAA,EAAAA,CAAC,IAAIuC,CAAL;CAEAN,EAAAA,CAAC,GAAGO,CAAC,GAAI,CAAC,KAAM,CAACF,KAAR,IAAkB,CAA3B;CACAE,EAAAA,CAAC,KAAM,CAACF,KAAR;CACAA,EAAAA,KAAK,IAAIH,IAAT;;CACA,SAAOG,KAAK,GAAG,CAAf,EAAkBL,CAAC,GAAIA,CAAC,GAAG,GAAL,GAAYL,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAtB,EAAoCA,CAAC,IAAIuC,CAAzC,EAA4CD,KAAK,IAAI,CAAvE,EAA0E;;CAE1EJ,EAAAA,CAAC,GAAGD,CAAC,GAAI,CAAC,KAAM,CAACK,KAAR,IAAkB,CAA3B;CACAL,EAAAA,CAAC,KAAM,CAACK,KAAR;CACAA,EAAAA,KAAK,IAAIP,IAAT;;CACA,SAAOO,KAAK,GAAG,CAAf,EAAkBJ,CAAC,GAAIA,CAAC,GAAG,GAAL,GAAYN,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAtB,EAAoCA,CAAC,IAAIuC,CAAzC,EAA4CD,KAAK,IAAI,CAAvE,EAA0E;;CAE1E,MAAIL,CAAC,KAAK,CAAV,EAAa;CACXA,IAAAA,CAAC,GAAG,IAAII,KAAR;CACD,GAFD,MAEO,IAAIJ,CAAC,KAAKG,IAAV,EAAgB;CACrB,WAAOF,CAAC,GAAGO,GAAH,GAAU,CAACD,CAAC,GAAG,CAAC,CAAJ,GAAQ,CAAV,IAAeE,QAAjC;CACD,GAFM,MAEA;CACLR,IAAAA,CAAC,GAAGA,CAAC,GAAGS,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYb,IAAZ,CAAR;CACAE,IAAAA,CAAC,GAAGA,CAAC,GAAGI,KAAR;CACD;;CACD,SAAO,CAACG,CAAC,GAAG,CAAC,CAAJ,GAAQ,CAAV,IAAeN,CAAf,GAAmBS,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYX,CAAC,GAAGF,IAAhB,CAA1B;CACD,CA/BD;;CAiCA,SAAa,GAAG,cAAA,CAAUH,MAAV,EAAkBiB,KAAlB,EAAyBhB,MAAzB,EAAiCC,IAAjC,EAAuCC,IAAvC,EAA6CC,MAA7C,EAAqD;CACnE,MAAIC,CAAJ,EAAOC,CAAP,EAAUY,CAAV;CACA,MAAIX,IAAI,GAAIH,MAAM,GAAG,CAAV,GAAeD,IAAf,GAAsB,CAAjC;CACA,MAAIK,IAAI,GAAG,CAAC,KAAKD,IAAN,IAAc,CAAzB;CACA,MAAIE,KAAK,GAAGD,IAAI,IAAI,CAApB;CACA,MAAIW,EAAE,GAAIhB,IAAI,KAAK,EAAT,GAAcY,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,CAAC,EAAb,IAAmBD,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,CAAC,EAAb,CAAjC,GAAoD,CAA9D;CACA,MAAI5C,CAAC,GAAG8B,IAAI,GAAG,CAAH,GAAQE,MAAM,GAAG,CAA7B;CACA,MAAIO,CAAC,GAAGT,IAAI,GAAG,CAAH,GAAO,CAAC,CAApB;CACA,MAAIU,CAAC,GAAGK,KAAK,GAAG,CAAR,IAAcA,KAAK,KAAK,CAAV,IAAe,IAAIA,KAAJ,GAAY,CAAzC,GAA8C,CAA9C,GAAkD,CAA1D;CAEAA,EAAAA,KAAK,GAAGF,IAAI,CAACK,GAAL,CAASH,KAAT,CAAR;;CAEA,MAAII,KAAK,CAACJ,KAAD,CAAL,IAAgBA,KAAK,KAAKH,QAA9B,EAAwC;CACtCR,IAAAA,CAAC,GAAGe,KAAK,CAACJ,KAAD,CAAL,GAAe,CAAf,GAAmB,CAAvB;CACAZ,IAAAA,CAAC,GAAGG,IAAJ;CACD,GAHD,MAGO;CACLH,IAAAA,CAAC,GAAGU,IAAI,CAACO,KAAL,CAAWP,IAAI,CAACQ,GAAL,CAASN,KAAT,IAAkBF,IAAI,CAACS,GAAlC,CAAJ;;CACA,QAAIP,KAAK,IAAIC,CAAC,GAAGH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,CAACX,CAAb,CAAR,CAAL,GAAgC,CAApC,EAAuC;CACrCA,MAAAA,CAAC;CACDa,MAAAA,CAAC,IAAI,CAAL;CACD;;CACD,QAAIb,CAAC,GAAGI,KAAJ,IAAa,CAAjB,EAAoB;CAClBQ,MAAAA,KAAK,IAAIE,EAAE,GAAGD,CAAd;CACD,KAFD,MAEO;CACLD,MAAAA,KAAK,IAAIE,EAAE,GAAGJ,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIP,KAAhB,CAAd;CACD;;CACD,QAAIQ,KAAK,GAAGC,CAAR,IAAa,CAAjB,EAAoB;CAClBb,MAAAA,CAAC;CACDa,MAAAA,CAAC,IAAI,CAAL;CACD;;CAED,QAAIb,CAAC,GAAGI,KAAJ,IAAaD,IAAjB,EAAuB;CACrBF,MAAAA,CAAC,GAAG,CAAJ;CACAD,MAAAA,CAAC,GAAGG,IAAJ;CACD,KAHD,MAGO,IAAIH,CAAC,GAAGI,KAAJ,IAAa,CAAjB,EAAoB;CACzBH,MAAAA,CAAC,GAAG,CAAEW,KAAK,GAAGC,CAAT,GAAc,CAAf,IAAoBH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYb,IAAZ,CAAxB;CACAE,MAAAA,CAAC,GAAGA,CAAC,GAAGI,KAAR;CACD,KAHM,MAGA;CACLH,MAAAA,CAAC,GAAGW,KAAK,GAAGF,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYP,KAAK,GAAG,CAApB,CAAR,GAAiCM,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYb,IAAZ,CAArC;CACAE,MAAAA,CAAC,GAAG,CAAJ;CACD;CACF;;CAED,SAAOF,IAAI,IAAI,CAAf,EAAkBH,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAN,GAAqBkC,CAAC,GAAG,IAAzB,EAA+BlC,CAAC,IAAIuC,CAApC,EAAuCL,CAAC,IAAI,GAA5C,EAAiDH,IAAI,IAAI,CAA3E,EAA8E;;CAE9EE,EAAAA,CAAC,GAAIA,CAAC,IAAIF,IAAN,GAAcG,CAAlB;CACAC,EAAAA,IAAI,IAAIJ,IAAR;;CACA,SAAOI,IAAI,GAAG,CAAd,EAAiBP,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAN,GAAqBiC,CAAC,GAAG,IAAzB,EAA+BjC,CAAC,IAAIuC,CAApC,EAAuCN,CAAC,IAAI,GAA5C,EAAiDE,IAAI,IAAI,CAA1E,EAA6E;;CAE7EP,EAAAA,MAAM,CAACC,MAAM,GAAG7B,CAAT,GAAauC,CAAd,CAAN,IAA0BC,CAAC,GAAG,GAA9B;EAjDF;;;;;;;;;CCtBA,MAAIa,mBAAmB,GACpB,OAAOC,MAAP,KAAkB,UAAlB,IAAgC,OAAOA,MAAM,CAAC,KAAD,CAAb,KAAyB,UAA1D;CACIA,EAAAA,MAAM,CAAC,KAAD,CAAN,CAAc,4BAAd,CADJ;CAAA,IAEI,IAHN;CAKAC,EAAAA,cAAA,GAAiBC,MAAjB;CACAD,EAAAA,kBAAA,GAAqBE,UAArB;CACAF,EAAAA,yBAAA,GAA4B,EAA5B;CAEA,MAAIG,YAAY,GAAG,UAAnB;CACAH,EAAAA,kBAAA,GAAqBG,YAArB;CAEA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;CACAF,EAAAA,MAAM,CAACG,mBAAP,GAA6BC,iBAAiB,EAA9C;;CAEA,MAAI,CAACJ,MAAM,CAACG,mBAAR,IAA+B,OAAOE,OAAP,KAAmB,WAAlD,IACA,OAAOA,OAAO,CAACC,KAAf,KAAyB,UAD7B,EACyC;CACvCD,IAAAA,OAAO,CAACC,KAAR,CACE,8EACA,sEAFF;CAID;;CAED,WAASF,iBAAT,GAA8B;;CAE5B,QAAI;CACF,UAAI/C,GAAG,GAAG,IAAIhB,UAAJ,CAAe,CAAf,CAAV;CACA,UAAIkE,KAAK,GAAG;CAAEC,QAAAA,GAAG,EAAE,eAAY;CAAE,iBAAO,EAAP;CAAW;CAAhC,OAAZ;CACAC,MAAAA,MAAM,CAACC,cAAP,CAAsBH,KAAtB,EAA6BlE,UAAU,CAACsE,SAAxC;CACAF,MAAAA,MAAM,CAACC,cAAP,CAAsBrD,GAAtB,EAA2BkD,KAA3B;CACA,aAAOlD,GAAG,CAACmD,GAAJ,OAAc,EAArB;CACD,KAND,CAME,OAAO/B,CAAP,EAAU;CACV,aAAO,KAAP;CACD;CACF;;CAEDgC,EAAAA,MAAM,CAACG,cAAP,CAAsBZ,MAAM,CAACW,SAA7B,EAAwC,QAAxC,EAAkD;CAChDE,IAAAA,UAAU,EAAE,IADoC;CAEhDC,IAAAA,GAAG,EAAE,eAAY;CACf,UAAI,CAACd,MAAM,CAACe,QAAP,CAAgB,IAAhB,CAAL,EAA4B,OAAOC,SAAP;CAC5B,aAAO,KAAK5C,MAAZ;CACD;CAL+C,GAAlD;CAQAqC,EAAAA,MAAM,CAACG,cAAP,CAAsBZ,MAAM,CAACW,SAA7B,EAAwC,QAAxC,EAAkD;CAChDE,IAAAA,UAAU,EAAE,IADoC;CAEhDC,IAAAA,GAAG,EAAE,eAAY;CACf,UAAI,CAACd,MAAM,CAACe,QAAP,CAAgB,IAAhB,CAAL,EAA4B,OAAOC,SAAP;CAC5B,aAAO,KAAKC,UAAZ;CACD;CAL+C,GAAlD;;CAQA,WAASC,YAAT,CAAuBxE,MAAvB,EAA+B;CAC7B,QAAIA,MAAM,GAAGwD,YAAb,EAA2B;CACzB,YAAM,IAAIiB,UAAJ,CAAe,gBAAgBzE,MAAhB,GAAyB,gCAAxC,CAAN;CACD,KAH4B;;;CAK7B,QAAI0E,GAAG,GAAG,IAAI/E,UAAJ,CAAeK,MAAf,CAAV;CACA+D,IAAAA,MAAM,CAACC,cAAP,CAAsBU,GAAtB,EAA2BpB,MAAM,CAACW,SAAlC;CACA,WAAOS,GAAP;CACD;CAED;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;;CAEA,WAASpB,MAAT,CAAiBqB,GAAjB,EAAsBC,gBAAtB,EAAwC5E,MAAxC,EAAgD;;CAE9C,QAAI,OAAO2E,GAAP,KAAe,QAAnB,EAA6B;CAC3B,UAAI,OAAOC,gBAAP,KAA4B,QAAhC,EAA0C;CACxC,cAAM,IAAIC,SAAJ,CACJ,oEADI,CAAN;CAGD;;CACD,aAAOC,WAAW,CAACH,GAAD,CAAlB;CACD;;CACD,WAAOI,IAAI,CAACJ,GAAD,EAAMC,gBAAN,EAAwB5E,MAAxB,CAAX;CACD;;CAEDsD,EAAAA,MAAM,CAAC0B,QAAP,GAAkB,IAAlB;;CAEA,WAASD,IAAT,CAAepC,KAAf,EAAsBiC,gBAAtB,EAAwC5E,MAAxC,EAAgD;CAC9C,QAAI,OAAO2C,KAAP,KAAiB,QAArB,EAA+B;CAC7B,aAAOsC,UAAU,CAACtC,KAAD,EAAQiC,gBAAR,CAAjB;CACD;;CAED,QAAIM,WAAW,CAACC,MAAZ,CAAmBxC,KAAnB,CAAJ,EAA+B;CAC7B,aAAOyC,aAAa,CAACzC,KAAD,CAApB;CACD;;CAED,QAAIA,KAAK,IAAI,IAAb,EAAmB;CACjB,YAAM,IAAIkC,SAAJ,CACJ,gFACA,sCADA,0BACiDlC,KADjD,CADI,CAAN;CAID;;CAED,QAAI0C,UAAU,CAAC1C,KAAD,EAAQuC,WAAR,CAAV,IACCvC,KAAK,IAAI0C,UAAU,CAAC1C,KAAK,CAACjB,MAAP,EAAewD,WAAf,CADxB,EACsD;CACpD,aAAOI,eAAe,CAAC3C,KAAD,EAAQiC,gBAAR,EAA0B5E,MAA1B,CAAtB;CACD;;CAED,QAAI,OAAOuF,iBAAP,KAA6B,WAA7B,KACCF,UAAU,CAAC1C,KAAD,EAAQ4C,iBAAR,CAAV,IACA5C,KAAK,IAAI0C,UAAU,CAAC1C,KAAK,CAACjB,MAAP,EAAe6D,iBAAf,CAFpB,CAAJ,EAE6D;CAC3D,aAAOD,eAAe,CAAC3C,KAAD,EAAQiC,gBAAR,EAA0B5E,MAA1B,CAAtB;CACD;;CAED,QAAI,OAAO2C,KAAP,KAAiB,QAArB,EAA+B;CAC7B,YAAM,IAAIkC,SAAJ,CACJ,uEADI,CAAN;CAGD;;CAED,QAAIW,OAAO,GAAG7C,KAAK,CAAC6C,OAAN,IAAiB7C,KAAK,CAAC6C,OAAN,EAA/B;;CACA,QAAIA,OAAO,IAAI,IAAX,IAAmBA,OAAO,KAAK7C,KAAnC,EAA0C;CACxC,aAAOW,MAAM,CAACyB,IAAP,CAAYS,OAAZ,EAAqBZ,gBAArB,EAAuC5E,MAAvC,CAAP;CACD;;CAED,QAAIyF,CAAC,GAAGC,UAAU,CAAC/C,KAAD,CAAlB;CACA,QAAI8C,CAAJ,EAAO,OAAOA,CAAP;;CAEP,QAAI,OAAOrC,MAAP,KAAkB,WAAlB,IAAiCA,MAAM,CAACuC,WAAP,IAAsB,IAAvD,IACA,OAAOhD,KAAK,CAACS,MAAM,CAACuC,WAAR,CAAZ,KAAqC,UADzC,EACqD;CACnD,aAAOrC,MAAM,CAACyB,IAAP,CACLpC,KAAK,CAACS,MAAM,CAACuC,WAAR,CAAL,CAA0B,QAA1B,CADK,EACgCf,gBADhC,EACkD5E,MADlD,CAAP;CAGD;;CAED,UAAM,IAAI6E,SAAJ,CACJ,gFACA,sCADA,0BACiDlC,KADjD,CADI,CAAN;CAID;CAED;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;;CACAW,EAAAA,MAAM,CAACyB,IAAP,GAAc,UAAUpC,KAAV,EAAiBiC,gBAAjB,EAAmC5E,MAAnC,EAA2C;CACvD,WAAO+E,IAAI,CAACpC,KAAD,EAAQiC,gBAAR,EAA0B5E,MAA1B,CAAX;CACD,GAFD;CAKA;;;CACA+D,EAAAA,MAAM,CAACC,cAAP,CAAsBV,MAAM,CAACW,SAA7B,EAAwCtE,UAAU,CAACsE,SAAnD;CACAF,EAAAA,MAAM,CAACC,cAAP,CAAsBV,MAAtB,EAA8B3D,UAA9B;;CAEA,WAASiG,UAAT,CAAqBC,IAArB,EAA2B;CACzB,QAAI,OAAOA,IAAP,KAAgB,QAApB,EAA8B;CAC5B,YAAM,IAAIhB,SAAJ,CAAc,wCAAd,CAAN;CACD,KAFD,MAEO,IAAIgB,IAAI,GAAG,CAAX,EAAc;CACnB,YAAM,IAAIpB,UAAJ,CAAe,gBAAgBoB,IAAhB,GAAuB,gCAAtC,CAAN;CACD;CACF;;CAED,WAASC,KAAT,CAAgBD,IAAhB,EAAsBE,IAAtB,EAA4BC,QAA5B,EAAsC;CACpCJ,IAAAA,UAAU,CAACC,IAAD,CAAV;;CACA,QAAIA,IAAI,IAAI,CAAZ,EAAe;CACb,aAAOrB,YAAY,CAACqB,IAAD,CAAnB;CACD;;CACD,QAAIE,IAAI,KAAKzB,SAAb,EAAwB;;;;CAItB,aAAO,OAAO0B,QAAP,KAAoB,QAApB,GACHxB,YAAY,CAACqB,IAAD,CAAZ,CAAmBE,IAAnB,CAAwBA,IAAxB,EAA8BC,QAA9B,CADG,GAEHxB,YAAY,CAACqB,IAAD,CAAZ,CAAmBE,IAAnB,CAAwBA,IAAxB,CAFJ;CAGD;;CACD,WAAOvB,YAAY,CAACqB,IAAD,CAAnB;CACD;CAED;CACA;CACA;CACA;;;CACAvC,EAAAA,MAAM,CAACwC,KAAP,GAAe,UAAUD,IAAV,EAAgBE,IAAhB,EAAsBC,QAAtB,EAAgC;CAC7C,WAAOF,KAAK,CAACD,IAAD,EAAOE,IAAP,EAAaC,QAAb,CAAZ;CACD,GAFD;;CAIA,WAASlB,WAAT,CAAsBe,IAAtB,EAA4B;CAC1BD,IAAAA,UAAU,CAACC,IAAD,CAAV;CACA,WAAOrB,YAAY,CAACqB,IAAI,GAAG,CAAP,GAAW,CAAX,GAAeI,OAAO,CAACJ,IAAD,CAAP,GAAgB,CAAhC,CAAnB;CACD;CAED;CACA;CACA;;;CACAvC,EAAAA,MAAM,CAACwB,WAAP,GAAqB,UAAUe,IAAV,EAAgB;CACnC,WAAOf,WAAW,CAACe,IAAD,CAAlB;CACD,GAFD;CAGA;CACA;CACA;;;CACAvC,EAAAA,MAAM,CAAC4C,eAAP,GAAyB,UAAUL,IAAV,EAAgB;CACvC,WAAOf,WAAW,CAACe,IAAD,CAAlB;CACD,GAFD;;CAIA,WAASZ,UAAT,CAAqBkB,MAArB,EAA6BH,QAA7B,EAAuC;CACrC,QAAI,OAAOA,QAAP,KAAoB,QAApB,IAAgCA,QAAQ,KAAK,EAAjD,EAAqD;CACnDA,MAAAA,QAAQ,GAAG,MAAX;CACD;;CAED,QAAI,CAAC1C,MAAM,CAAC8C,UAAP,CAAkBJ,QAAlB,CAAL,EAAkC;CAChC,YAAM,IAAInB,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;CACD;;CAED,QAAIhG,MAAM,GAAGX,UAAU,CAAC8G,MAAD,EAASH,QAAT,CAAV,GAA+B,CAA5C;CACA,QAAItB,GAAG,GAAGF,YAAY,CAACxE,MAAD,CAAtB;CAEA,QAAIqG,MAAM,GAAG3B,GAAG,CAAC4B,KAAJ,CAAUH,MAAV,EAAkBH,QAAlB,CAAb;;CAEA,QAAIK,MAAM,KAAKrG,MAAf,EAAuB;;;;CAIrB0E,MAAAA,GAAG,GAAGA,GAAG,CAAC6B,KAAJ,CAAU,CAAV,EAAaF,MAAb,CAAN;CACD;;CAED,WAAO3B,GAAP;CACD;;CAED,WAAS8B,aAAT,CAAwBC,KAAxB,EAA+B;CAC7B,QAAIzG,MAAM,GAAGyG,KAAK,CAACzG,MAAN,GAAe,CAAf,GAAmB,CAAnB,GAAuBiG,OAAO,CAACQ,KAAK,CAACzG,MAAP,CAAP,GAAwB,CAA5D;CACA,QAAI0E,GAAG,GAAGF,YAAY,CAACxE,MAAD,CAAtB;;CACA,SAAK,IAAIF,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4BF,CAAC,IAAI,CAAjC,EAAoC;CAClC4E,MAAAA,GAAG,CAAC5E,CAAD,CAAH,GAAS2G,KAAK,CAAC3G,CAAD,CAAL,GAAW,GAApB;CACD;;CACD,WAAO4E,GAAP;CACD;;CAED,WAASU,aAAT,CAAwBsB,SAAxB,EAAmC;CACjC,QAAIrB,UAAU,CAACqB,SAAD,EAAY/G,UAAZ,CAAd,EAAuC;CACrC,UAAIgH,IAAI,GAAG,IAAIhH,UAAJ,CAAe+G,SAAf,CAAX;CACA,aAAOpB,eAAe,CAACqB,IAAI,CAACjF,MAAN,EAAciF,IAAI,CAACpC,UAAnB,EAA+BoC,IAAI,CAACtH,UAApC,CAAtB;CACD;;CACD,WAAOmH,aAAa,CAACE,SAAD,CAApB;CACD;;CAED,WAASpB,eAAT,CAA0BmB,KAA1B,EAAiClC,UAAjC,EAA6CvE,MAA7C,EAAqD;CACnD,QAAIuE,UAAU,GAAG,CAAb,IAAkBkC,KAAK,CAACpH,UAAN,GAAmBkF,UAAzC,EAAqD;CACnD,YAAM,IAAIE,UAAJ,CAAe,sCAAf,CAAN;CACD;;CAED,QAAIgC,KAAK,CAACpH,UAAN,GAAmBkF,UAAU,IAAIvE,MAAM,IAAI,CAAd,CAAjC,EAAmD;CACjD,YAAM,IAAIyE,UAAJ,CAAe,sCAAf,CAAN;CACD;;CAED,QAAIC,GAAJ;;CACA,QAAIH,UAAU,KAAKD,SAAf,IAA4BtE,MAAM,KAAKsE,SAA3C,EAAsD;CACpDI,MAAAA,GAAG,GAAG,IAAI/E,UAAJ,CAAe8G,KAAf,CAAN;CACD,KAFD,MAEO,IAAIzG,MAAM,KAAKsE,SAAf,EAA0B;CAC/BI,MAAAA,GAAG,GAAG,IAAI/E,UAAJ,CAAe8G,KAAf,EAAsBlC,UAAtB,CAAN;CACD,KAFM,MAEA;CACLG,MAAAA,GAAG,GAAG,IAAI/E,UAAJ,CAAe8G,KAAf,EAAsBlC,UAAtB,EAAkCvE,MAAlC,CAAN;CACD,KAhBkD;;;CAmBnD+D,IAAAA,MAAM,CAACC,cAAP,CAAsBU,GAAtB,EAA2BpB,MAAM,CAACW,SAAlC;CAEA,WAAOS,GAAP;CACD;;CAED,WAASgB,UAAT,CAAqBkB,GAArB,EAA0B;CACxB,QAAItD,MAAM,CAACe,QAAP,CAAgBuC,GAAhB,CAAJ,EAA0B;CACxB,UAAI7G,GAAG,GAAGkG,OAAO,CAACW,GAAG,CAAC5G,MAAL,CAAP,GAAsB,CAAhC;CACA,UAAI0E,GAAG,GAAGF,YAAY,CAACzE,GAAD,CAAtB;;CAEA,UAAI2E,GAAG,CAAC1E,MAAJ,KAAe,CAAnB,EAAsB;CACpB,eAAO0E,GAAP;CACD;;CAEDkC,MAAAA,GAAG,CAACD,IAAJ,CAASjC,GAAT,EAAc,CAAd,EAAiB,CAAjB,EAAoB3E,GAApB;CACA,aAAO2E,GAAP;CACD;;CAED,QAAIkC,GAAG,CAAC5G,MAAJ,KAAesE,SAAnB,EAA8B;CAC5B,UAAI,OAAOsC,GAAG,CAAC5G,MAAX,KAAsB,QAAtB,IAAkC6G,WAAW,CAACD,GAAG,CAAC5G,MAAL,CAAjD,EAA+D;CAC7D,eAAOwE,YAAY,CAAC,CAAD,CAAnB;CACD;;CACD,aAAOgC,aAAa,CAACI,GAAD,CAApB;CACD;;CAED,QAAIA,GAAG,CAACE,IAAJ,KAAa,QAAb,IAAyBlH,KAAK,CAACmH,OAAN,CAAcH,GAAG,CAACI,IAAlB,CAA7B,EAAsD;CACpD,aAAOR,aAAa,CAACI,GAAG,CAACI,IAAL,CAApB;CACD;CACF;;CAED,WAASf,OAAT,CAAkBjG,MAAlB,EAA0B;;;CAGxB,QAAIA,MAAM,IAAIwD,YAAd,EAA4B;CAC1B,YAAM,IAAIiB,UAAJ,CAAe,oDACA,UADA,GACajB,YAAY,CAACyD,QAAb,CAAsB,EAAtB,CADb,GACyC,QADxD,CAAN;CAED;;CACD,WAAOjH,MAAM,GAAG,CAAhB;CACD;;CAED,WAASuD,UAAT,CAAqBvD,MAArB,EAA6B;CAC3B,QAAI,CAACA,MAAD,IAAWA,MAAf,EAAuB;;CACrBA,MAAAA,MAAM,GAAG,CAAT;CACD;;CACD,WAAOsD,MAAM,CAACwC,KAAP,CAAa,CAAC9F,MAAd,CAAP;CACD;;CAEDsD,EAAAA,MAAM,CAACe,QAAP,GAAkB,SAASA,QAAT,CAAmBoB,CAAnB,EAAsB;CACtC,WAAOA,CAAC,IAAI,IAAL,IAAaA,CAAC,CAACyB,SAAF,KAAgB,IAA7B,IACLzB,CAAC,KAAKnC,MAAM,CAACW,SADf,CADsC;CAGvC,GAHD;;CAKAX,EAAAA,MAAM,CAAC6D,OAAP,GAAiB,SAASA,OAAT,CAAkBC,CAAlB,EAAqB3B,CAArB,EAAwB;CACvC,QAAIJ,UAAU,CAAC+B,CAAD,EAAIzH,UAAJ,CAAd,EAA+ByH,CAAC,GAAG9D,MAAM,CAACyB,IAAP,CAAYqC,CAAZ,EAAeA,CAAC,CAACzF,MAAjB,EAAyByF,CAAC,CAAC/H,UAA3B,CAAJ;CAC/B,QAAIgG,UAAU,CAACI,CAAD,EAAI9F,UAAJ,CAAd,EAA+B8F,CAAC,GAAGnC,MAAM,CAACyB,IAAP,CAAYU,CAAZ,EAAeA,CAAC,CAAC9D,MAAjB,EAAyB8D,CAAC,CAACpG,UAA3B,CAAJ;;CAC/B,QAAI,CAACiE,MAAM,CAACe,QAAP,CAAgB+C,CAAhB,CAAD,IAAuB,CAAC9D,MAAM,CAACe,QAAP,CAAgBoB,CAAhB,CAA5B,EAAgD;CAC9C,YAAM,IAAIZ,SAAJ,CACJ,uEADI,CAAN;CAGD;;CAED,QAAIuC,CAAC,KAAK3B,CAAV,EAAa,OAAO,CAAP;CAEb,QAAI4B,CAAC,GAAGD,CAAC,CAACpH,MAAV;CACA,QAAIsH,CAAC,GAAG7B,CAAC,CAACzF,MAAV;;CAEA,SAAK,IAAIF,CAAC,GAAG,CAAR,EAAWC,GAAG,GAAG0C,IAAI,CAAC8E,GAAL,CAASF,CAAT,EAAYC,CAAZ,CAAtB,EAAsCxH,CAAC,GAAGC,GAA1C,EAA+C,EAAED,CAAjD,EAAoD;CAClD,UAAIsH,CAAC,CAACtH,CAAD,CAAD,KAAS2F,CAAC,CAAC3F,CAAD,CAAd,EAAmB;CACjBuH,QAAAA,CAAC,GAAGD,CAAC,CAACtH,CAAD,CAAL;CACAwH,QAAAA,CAAC,GAAG7B,CAAC,CAAC3F,CAAD,CAAL;CACA;CACD;CACF;;CAED,QAAIuH,CAAC,GAAGC,CAAR,EAAW,OAAO,CAAC,CAAR;CACX,QAAIA,CAAC,GAAGD,CAAR,EAAW,OAAO,CAAP;CACX,WAAO,CAAP;CACD,GAzBD;;CA2BA/D,EAAAA,MAAM,CAAC8C,UAAP,GAAoB,SAASA,UAAT,CAAqBJ,QAArB,EAA+B;CACjD,YAAQwB,MAAM,CAACxB,QAAD,CAAN,CAAiByB,WAAjB,EAAR;CACE,WAAK,KAAL;CACA,WAAK,MAAL;CACA,WAAK,OAAL;CACA,WAAK,OAAL;CACA,WAAK,QAAL;CACA,WAAK,QAAL;CACA,WAAK,QAAL;CACA,WAAK,MAAL;CACA,WAAK,OAAL;CACA,WAAK,SAAL;CACA,WAAK,UAAL;CACE,eAAO,IAAP;;CACF;CACE,eAAO,KAAP;CAdJ;CAgBD,GAjBD;;CAmBAnE,EAAAA,MAAM,CAACoE,MAAP,GAAgB,SAASA,MAAT,CAAiBC,IAAjB,EAAuB3H,MAAvB,EAA+B;CAC7C,QAAI,CAACJ,KAAK,CAACmH,OAAN,CAAcY,IAAd,CAAL,EAA0B;CACxB,YAAM,IAAI9C,SAAJ,CAAc,6CAAd,CAAN;CACD;;CAED,QAAI8C,IAAI,CAAC3H,MAAL,KAAgB,CAApB,EAAuB;CACrB,aAAOsD,MAAM,CAACwC,KAAP,CAAa,CAAb,CAAP;CACD;;CAED,QAAIhG,CAAJ;;CACA,QAAIE,MAAM,KAAKsE,SAAf,EAA0B;CACxBtE,MAAAA,MAAM,GAAG,CAAT;;CACA,WAAKF,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAG6H,IAAI,CAAC3H,MAArB,EAA6B,EAAEF,CAA/B,EAAkC;CAChCE,QAAAA,MAAM,IAAI2H,IAAI,CAAC7H,CAAD,CAAJ,CAAQE,MAAlB;CACD;CACF;;CAED,QAAI0B,MAAM,GAAG4B,MAAM,CAACwB,WAAP,CAAmB9E,MAAnB,CAAb;CACA,QAAI4H,GAAG,GAAG,CAAV;;CACA,SAAK9H,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAG6H,IAAI,CAAC3H,MAArB,EAA6B,EAAEF,CAA/B,EAAkC;CAChC,UAAI4E,GAAG,GAAGiD,IAAI,CAAC7H,CAAD,CAAd;;CACA,UAAIuF,UAAU,CAACX,GAAD,EAAM/E,UAAN,CAAd,EAAiC;CAC/B,YAAIiI,GAAG,GAAGlD,GAAG,CAAC1E,MAAV,GAAmB0B,MAAM,CAAC1B,MAA9B,EAAsC;CACpCsD,UAAAA,MAAM,CAACyB,IAAP,CAAYL,GAAZ,EAAiBiC,IAAjB,CAAsBjF,MAAtB,EAA8BkG,GAA9B;CACD,SAFD,MAEO;CACLjI,UAAAA,UAAU,CAACsE,SAAX,CAAqB4D,GAArB,CAAyBC,IAAzB,CACEpG,MADF,EAEEgD,GAFF,EAGEkD,GAHF;CAKD;CACF,OAVD,MAUO,IAAI,CAACtE,MAAM,CAACe,QAAP,CAAgBK,GAAhB,CAAL,EAA2B;CAChC,cAAM,IAAIG,SAAJ,CAAc,6CAAd,CAAN;CACD,OAFM,MAEA;CACLH,QAAAA,GAAG,CAACiC,IAAJ,CAASjF,MAAT,EAAiBkG,GAAjB;CACD;;CACDA,MAAAA,GAAG,IAAIlD,GAAG,CAAC1E,MAAX;CACD;;CACD,WAAO0B,MAAP;CACD,GAvCD;;CAyCA,WAASrC,UAAT,CAAqB8G,MAArB,EAA6BH,QAA7B,EAAuC;CACrC,QAAI1C,MAAM,CAACe,QAAP,CAAgB8B,MAAhB,CAAJ,EAA6B;CAC3B,aAAOA,MAAM,CAACnG,MAAd;CACD;;CACD,QAAIkF,WAAW,CAACC,MAAZ,CAAmBgB,MAAnB,KAA8Bd,UAAU,CAACc,MAAD,EAASjB,WAAT,CAA5C,EAAmE;CACjE,aAAOiB,MAAM,CAAC9G,UAAd;CACD;;CACD,QAAI,OAAO8G,MAAP,KAAkB,QAAtB,EAAgC;CAC9B,YAAM,IAAItB,SAAJ,CACJ,+EACA,gBADA,0BAC0BsB,MAD1B,CADI,CAAN;CAID;;CAED,QAAIpG,GAAG,GAAGoG,MAAM,CAACnG,MAAjB;CACA,QAAI+H,SAAS,GAAIC,SAAS,CAAChI,MAAV,GAAmB,CAAnB,IAAwBgI,SAAS,CAAC,CAAD,CAAT,KAAiB,IAA1D;CACA,QAAI,CAACD,SAAD,IAAchI,GAAG,KAAK,CAA1B,EAA6B,OAAO,CAAP,CAhBQ;;CAmBrC,QAAIkI,WAAW,GAAG,KAAlB;;CACA,aAAS;CACP,cAAQjC,QAAR;CACE,aAAK,OAAL;CACA,aAAK,QAAL;CACA,aAAK,QAAL;CACE,iBAAOjG,GAAP;;CACF,aAAK,MAAL;CACA,aAAK,OAAL;CACE,iBAAOmI,WAAW,CAAC/B,MAAD,CAAX,CAAoBnG,MAA3B;;CACF,aAAK,MAAL;CACA,aAAK,OAAL;CACA,aAAK,SAAL;CACA,aAAK,UAAL;CACE,iBAAOD,GAAG,GAAG,CAAb;;CACF,aAAK,KAAL;CACE,iBAAOA,GAAG,KAAK,CAAf;;CACF,aAAK,QAAL;CACE,iBAAOoI,aAAa,CAAChC,MAAD,CAAb,CAAsBnG,MAA7B;;CACF;CACE,cAAIiI,WAAJ,EAAiB;CACf,mBAAOF,SAAS,GAAG,CAAC,CAAJ,GAAQG,WAAW,CAAC/B,MAAD,CAAX,CAAoBnG,MAA5C,CADe;CAEhB;;CACDgG,UAAAA,QAAQ,GAAG,CAAC,KAAKA,QAAN,EAAgByB,WAAhB,EAAX;CACAQ,UAAAA,WAAW,GAAG,IAAd;CAtBJ;CAwBD;CACF;;CACD3E,EAAAA,MAAM,CAACjE,UAAP,GAAoBA,UAApB;;CAEA,WAAS+I,YAAT,CAAuBpC,QAAvB,EAAiC/E,KAAjC,EAAwCC,GAAxC,EAA6C;CAC3C,QAAI+G,WAAW,GAAG,KAAlB,CAD2C;;;;;;;CAU3C,QAAIhH,KAAK,KAAKqD,SAAV,IAAuBrD,KAAK,GAAG,CAAnC,EAAsC;CACpCA,MAAAA,KAAK,GAAG,CAAR;CACD,KAZ0C;;;;CAe3C,QAAIA,KAAK,GAAG,KAAKjB,MAAjB,EAAyB;CACvB,aAAO,EAAP;CACD;;CAED,QAAIkB,GAAG,KAAKoD,SAAR,IAAqBpD,GAAG,GAAG,KAAKlB,MAApC,EAA4C;CAC1CkB,MAAAA,GAAG,GAAG,KAAKlB,MAAX;CACD;;CAED,QAAIkB,GAAG,IAAI,CAAX,EAAc;CACZ,aAAO,EAAP;CACD,KAzB0C;;;CA4B3CA,IAAAA,GAAG,MAAM,CAAT;CACAD,IAAAA,KAAK,MAAM,CAAX;;CAEA,QAAIC,GAAG,IAAID,KAAX,EAAkB;CAChB,aAAO,EAAP;CACD;;CAED,QAAI,CAAC+E,QAAL,EAAeA,QAAQ,GAAG,MAAX;;CAEf,WAAO,IAAP,EAAa;CACX,cAAQA,QAAR;CACE,aAAK,KAAL;CACE,iBAAOqC,QAAQ,CAAC,IAAD,EAAOpH,KAAP,EAAcC,GAAd,CAAf;;CAEF,aAAK,MAAL;CACA,aAAK,OAAL;CACE,iBAAOoH,SAAS,CAAC,IAAD,EAAOrH,KAAP,EAAcC,GAAd,CAAhB;;CAEF,aAAK,OAAL;CACE,iBAAOqH,UAAU,CAAC,IAAD,EAAOtH,KAAP,EAAcC,GAAd,CAAjB;;CAEF,aAAK,QAAL;CACA,aAAK,QAAL;CACE,iBAAOsH,WAAW,CAAC,IAAD,EAAOvH,KAAP,EAAcC,GAAd,CAAlB;;CAEF,aAAK,QAAL;CACE,iBAAOuH,WAAW,CAAC,IAAD,EAAOxH,KAAP,EAAcC,GAAd,CAAlB;;CAEF,aAAK,MAAL;CACA,aAAK,OAAL;CACA,aAAK,SAAL;CACA,aAAK,UAAL;CACE,iBAAOwH,YAAY,CAAC,IAAD,EAAOzH,KAAP,EAAcC,GAAd,CAAnB;;CAEF;CACE,cAAI+G,WAAJ,EAAiB,MAAM,IAAIpD,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;CACjBA,UAAAA,QAAQ,GAAG,CAACA,QAAQ,GAAG,EAAZ,EAAgByB,WAAhB,EAAX;CACAQ,UAAAA,WAAW,GAAG,IAAd;CA3BJ;CA6BD;CACF;CAGD;CACA;CACA;CACA;CACA;;;CACA3E,EAAAA,MAAM,CAACW,SAAP,CAAiBiD,SAAjB,GAA6B,IAA7B;;CAEA,WAASyB,IAAT,CAAelD,CAAf,EAAkBmD,CAAlB,EAAqB5G,CAArB,EAAwB;CACtB,QAAIlC,CAAC,GAAG2F,CAAC,CAACmD,CAAD,CAAT;CACAnD,IAAAA,CAAC,CAACmD,CAAD,CAAD,GAAOnD,CAAC,CAACzD,CAAD,CAAR;CACAyD,IAAAA,CAAC,CAACzD,CAAD,CAAD,GAAOlC,CAAP;CACD;;CAEDwD,EAAAA,MAAM,CAACW,SAAP,CAAiB4E,MAAjB,GAA0B,SAASA,MAAT,GAAmB;CAC3C,QAAI9I,GAAG,GAAG,KAAKC,MAAf;;CACA,QAAID,GAAG,GAAG,CAAN,KAAY,CAAhB,EAAmB;CACjB,YAAM,IAAI0E,UAAJ,CAAe,2CAAf,CAAN;CACD;;CACD,SAAK,IAAI3E,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyBD,CAAC,IAAI,CAA9B,EAAiC;CAC/B6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAP,EAAUA,CAAC,GAAG,CAAd,CAAJ;CACD;;CACD,WAAO,IAAP;CACD,GATD;;CAWAwD,EAAAA,MAAM,CAACW,SAAP,CAAiB6E,MAAjB,GAA0B,SAASA,MAAT,GAAmB;CAC3C,QAAI/I,GAAG,GAAG,KAAKC,MAAf;;CACA,QAAID,GAAG,GAAG,CAAN,KAAY,CAAhB,EAAmB;CACjB,YAAM,IAAI0E,UAAJ,CAAe,2CAAf,CAAN;CACD;;CACD,SAAK,IAAI3E,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyBD,CAAC,IAAI,CAA9B,EAAiC;CAC/B6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAP,EAAUA,CAAC,GAAG,CAAd,CAAJ;CACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;CACD;;CACD,WAAO,IAAP;CACD,GAVD;;CAYAwD,EAAAA,MAAM,CAACW,SAAP,CAAiB8E,MAAjB,GAA0B,SAASA,MAAT,GAAmB;CAC3C,QAAIhJ,GAAG,GAAG,KAAKC,MAAf;;CACA,QAAID,GAAG,GAAG,CAAN,KAAY,CAAhB,EAAmB;CACjB,YAAM,IAAI0E,UAAJ,CAAe,2CAAf,CAAN;CACD;;CACD,SAAK,IAAI3E,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyBD,CAAC,IAAI,CAA9B,EAAiC;CAC/B6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAP,EAAUA,CAAC,GAAG,CAAd,CAAJ;CACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;CACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;CACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;CACD;;CACD,WAAO,IAAP;CACD,GAZD;;CAcAwD,EAAAA,MAAM,CAACW,SAAP,CAAiBgD,QAAjB,GAA4B,SAASA,QAAT,GAAqB;CAC/C,QAAIjH,MAAM,GAAG,KAAKA,MAAlB;CACA,QAAIA,MAAM,KAAK,CAAf,EAAkB,OAAO,EAAP;CAClB,QAAIgI,SAAS,CAAChI,MAAV,KAAqB,CAAzB,EAA4B,OAAOsI,SAAS,CAAC,IAAD,EAAO,CAAP,EAAUtI,MAAV,CAAhB;CAC5B,WAAOoI,YAAY,CAACY,KAAb,CAAmB,IAAnB,EAAyBhB,SAAzB,CAAP;CACD,GALD;;CAOA1E,EAAAA,MAAM,CAACW,SAAP,CAAiBgF,cAAjB,GAAkC3F,MAAM,CAACW,SAAP,CAAiBgD,QAAnD;;CAEA3D,EAAAA,MAAM,CAACW,SAAP,CAAiBiF,MAAjB,GAA0B,SAASA,MAAT,CAAiBzD,CAAjB,EAAoB;CAC5C,QAAI,CAACnC,MAAM,CAACe,QAAP,CAAgBoB,CAAhB,CAAL,EAAyB,MAAM,IAAIZ,SAAJ,CAAc,2BAAd,CAAN;CACzB,QAAI,SAASY,CAAb,EAAgB,OAAO,IAAP;CAChB,WAAOnC,MAAM,CAAC6D,OAAP,CAAe,IAAf,EAAqB1B,CAArB,MAA4B,CAAnC;CACD,GAJD;;CAMAnC,EAAAA,MAAM,CAACW,SAAP,CAAiBkF,OAAjB,GAA2B,SAASA,OAAT,GAAoB;CAC7C,QAAIC,GAAG,GAAG,EAAV;CACA,QAAIC,GAAG,GAAGhG,OAAO,CAACiG,iBAAlB;CACAF,IAAAA,GAAG,GAAG,KAAKnC,QAAL,CAAc,KAAd,EAAqB,CAArB,EAAwBoC,GAAxB,EAA6BE,OAA7B,CAAqC,SAArC,EAAgD,KAAhD,EAAuDC,IAAvD,EAAN;CACA,QAAI,KAAKxJ,MAAL,GAAcqJ,GAAlB,EAAuBD,GAAG,IAAI,OAAP;CACvB,WAAO,aAAaA,GAAb,GAAmB,GAA1B;CACD,GAND;;CAOA,MAAIjG,mBAAJ,EAAyB;CACvBG,IAAAA,MAAM,CAACW,SAAP,CAAiBd,mBAAjB,IAAwCG,MAAM,CAACW,SAAP,CAAiBkF,OAAzD;CACD;;CAED7F,EAAAA,MAAM,CAACW,SAAP,CAAiBkD,OAAjB,GAA2B,SAASA,OAAT,CAAkBsC,MAAlB,EAA0BxI,KAA1B,EAAiCC,GAAjC,EAAsCwI,SAAtC,EAAiDC,OAAjD,EAA0D;CACnF,QAAItE,UAAU,CAACoE,MAAD,EAAS9J,UAAT,CAAd,EAAoC;CAClC8J,MAAAA,MAAM,GAAGnG,MAAM,CAACyB,IAAP,CAAY0E,MAAZ,EAAoBA,MAAM,CAAC9H,MAA3B,EAAmC8H,MAAM,CAACpK,UAA1C,CAAT;CACD;;CACD,QAAI,CAACiE,MAAM,CAACe,QAAP,CAAgBoF,MAAhB,CAAL,EAA8B;CAC5B,YAAM,IAAI5E,SAAJ,CACJ,qEACA,gBADA,0BAC2B4E,MAD3B,CADI,CAAN;CAID;;CAED,QAAIxI,KAAK,KAAKqD,SAAd,EAAyB;CACvBrD,MAAAA,KAAK,GAAG,CAAR;CACD;;CACD,QAAIC,GAAG,KAAKoD,SAAZ,EAAuB;CACrBpD,MAAAA,GAAG,GAAGuI,MAAM,GAAGA,MAAM,CAACzJ,MAAV,GAAmB,CAA/B;CACD;;CACD,QAAI0J,SAAS,KAAKpF,SAAlB,EAA6B;CAC3BoF,MAAAA,SAAS,GAAG,CAAZ;CACD;;CACD,QAAIC,OAAO,KAAKrF,SAAhB,EAA2B;CACzBqF,MAAAA,OAAO,GAAG,KAAK3J,MAAf;CACD;;CAED,QAAIiB,KAAK,GAAG,CAAR,IAAaC,GAAG,GAAGuI,MAAM,CAACzJ,MAA1B,IAAoC0J,SAAS,GAAG,CAAhD,IAAqDC,OAAO,GAAG,KAAK3J,MAAxE,EAAgF;CAC9E,YAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;CACD;;CAED,QAAIiF,SAAS,IAAIC,OAAb,IAAwB1I,KAAK,IAAIC,GAArC,EAA0C;CACxC,aAAO,CAAP;CACD;;CACD,QAAIwI,SAAS,IAAIC,OAAjB,EAA0B;CACxB,aAAO,CAAC,CAAR;CACD;;CACD,QAAI1I,KAAK,IAAIC,GAAb,EAAkB;CAChB,aAAO,CAAP;CACD;;CAEDD,IAAAA,KAAK,MAAM,CAAX;CACAC,IAAAA,GAAG,MAAM,CAAT;CACAwI,IAAAA,SAAS,MAAM,CAAf;CACAC,IAAAA,OAAO,MAAM,CAAb;CAEA,QAAI,SAASF,MAAb,EAAqB,OAAO,CAAP;CAErB,QAAIpC,CAAC,GAAGsC,OAAO,GAAGD,SAAlB;CACA,QAAIpC,CAAC,GAAGpG,GAAG,GAAGD,KAAd;CACA,QAAIlB,GAAG,GAAG0C,IAAI,CAAC8E,GAAL,CAASF,CAAT,EAAYC,CAAZ,CAAV;CAEA,QAAIsC,QAAQ,GAAG,KAAKrD,KAAL,CAAWmD,SAAX,EAAsBC,OAAtB,CAAf;CACA,QAAIE,UAAU,GAAGJ,MAAM,CAAClD,KAAP,CAAatF,KAAb,EAAoBC,GAApB,CAAjB;;CAEA,SAAK,IAAIpB,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyB,EAAED,CAA3B,EAA8B;CAC5B,UAAI8J,QAAQ,CAAC9J,CAAD,CAAR,KAAgB+J,UAAU,CAAC/J,CAAD,CAA9B,EAAmC;CACjCuH,QAAAA,CAAC,GAAGuC,QAAQ,CAAC9J,CAAD,CAAZ;CACAwH,QAAAA,CAAC,GAAGuC,UAAU,CAAC/J,CAAD,CAAd;CACA;CACD;CACF;;CAED,QAAIuH,CAAC,GAAGC,CAAR,EAAW,OAAO,CAAC,CAAR;CACX,QAAIA,CAAC,GAAGD,CAAR,EAAW,OAAO,CAAP;CACX,WAAO,CAAP;CACD,GA/DD;CAkEA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;;CACA,WAASyC,oBAAT,CAA+BpI,MAA/B,EAAuCqI,GAAvC,EAA4CxF,UAA5C,EAAwDyB,QAAxD,EAAkEgE,GAAlE,EAAuE;;CAErE,QAAItI,MAAM,CAAC1B,MAAP,KAAkB,CAAtB,EAAyB,OAAO,CAAC,CAAR,CAF4C;;CAKrE,QAAI,OAAOuE,UAAP,KAAsB,QAA1B,EAAoC;CAClCyB,MAAAA,QAAQ,GAAGzB,UAAX;CACAA,MAAAA,UAAU,GAAG,CAAb;CACD,KAHD,MAGO,IAAIA,UAAU,GAAG,UAAjB,EAA6B;CAClCA,MAAAA,UAAU,GAAG,UAAb;CACD,KAFM,MAEA,IAAIA,UAAU,GAAG,CAAC,UAAlB,EAA8B;CACnCA,MAAAA,UAAU,GAAG,CAAC,UAAd;CACD;;CACDA,IAAAA,UAAU,GAAG,CAACA,UAAd,CAbqE;;CAcrE,QAAIsC,WAAW,CAACtC,UAAD,CAAf,EAA6B;;CAE3BA,MAAAA,UAAU,GAAGyF,GAAG,GAAG,CAAH,GAAQtI,MAAM,CAAC1B,MAAP,GAAgB,CAAxC;CACD,KAjBoE;;;CAoBrE,QAAIuE,UAAU,GAAG,CAAjB,EAAoBA,UAAU,GAAG7C,MAAM,CAAC1B,MAAP,GAAgBuE,UAA7B;;CACpB,QAAIA,UAAU,IAAI7C,MAAM,CAAC1B,MAAzB,EAAiC;CAC/B,UAAIgK,GAAJ,EAAS,OAAO,CAAC,CAAR,CAAT,KACKzF,UAAU,GAAG7C,MAAM,CAAC1B,MAAP,GAAgB,CAA7B;CACN,KAHD,MAGO,IAAIuE,UAAU,GAAG,CAAjB,EAAoB;CACzB,UAAIyF,GAAJ,EAASzF,UAAU,GAAG,CAAb,CAAT,KACK,OAAO,CAAC,CAAR;CACN,KA3BoE;;;CA8BrE,QAAI,OAAOwF,GAAP,KAAe,QAAnB,EAA6B;CAC3BA,MAAAA,GAAG,GAAGzG,MAAM,CAACyB,IAAP,CAAYgF,GAAZ,EAAiB/D,QAAjB,CAAN;CACD,KAhCoE;;;CAmCrE,QAAI1C,MAAM,CAACe,QAAP,CAAgB0F,GAAhB,CAAJ,EAA0B;;CAExB,UAAIA,GAAG,CAAC/J,MAAJ,KAAe,CAAnB,EAAsB;CACpB,eAAO,CAAC,CAAR;CACD;;CACD,aAAOiK,YAAY,CAACvI,MAAD,EAASqI,GAAT,EAAcxF,UAAd,EAA0ByB,QAA1B,EAAoCgE,GAApC,CAAnB;CACD,KAND,MAMO,IAAI,OAAOD,GAAP,KAAe,QAAnB,EAA6B;CAClCA,MAAAA,GAAG,GAAGA,GAAG,GAAG,IAAZ,CADkC;;CAElC,UAAI,OAAOpK,UAAU,CAACsE,SAAX,CAAqB3D,OAA5B,KAAwC,UAA5C,EAAwD;CACtD,YAAI0J,GAAJ,EAAS;CACP,iBAAOrK,UAAU,CAACsE,SAAX,CAAqB3D,OAArB,CAA6BwH,IAA7B,CAAkCpG,MAAlC,EAA0CqI,GAA1C,EAA+CxF,UAA/C,CAAP;CACD,SAFD,MAEO;CACL,iBAAO5E,UAAU,CAACsE,SAAX,CAAqBiG,WAArB,CAAiCpC,IAAjC,CAAsCpG,MAAtC,EAA8CqI,GAA9C,EAAmDxF,UAAnD,CAAP;CACD;CACF;;CACD,aAAO0F,YAAY,CAACvI,MAAD,EAAS,CAACqI,GAAD,CAAT,EAAgBxF,UAAhB,EAA4ByB,QAA5B,EAAsCgE,GAAtC,CAAnB;CACD;;CAED,UAAM,IAAInF,SAAJ,CAAc,sCAAd,CAAN;CACD;;CAED,WAASoF,YAAT,CAAuBtJ,GAAvB,EAA4BoJ,GAA5B,EAAiCxF,UAAjC,EAA6CyB,QAA7C,EAAuDgE,GAAvD,EAA4D;CAC1D,QAAIG,SAAS,GAAG,CAAhB;CACA,QAAIC,SAAS,GAAGzJ,GAAG,CAACX,MAApB;CACA,QAAIqK,SAAS,GAAGN,GAAG,CAAC/J,MAApB;;CAEA,QAAIgG,QAAQ,KAAK1B,SAAjB,EAA4B;CAC1B0B,MAAAA,QAAQ,GAAGwB,MAAM,CAACxB,QAAD,CAAN,CAAiByB,WAAjB,EAAX;;CACA,UAAIzB,QAAQ,KAAK,MAAb,IAAuBA,QAAQ,KAAK,OAApC,IACAA,QAAQ,KAAK,SADb,IAC0BA,QAAQ,KAAK,UAD3C,EACuD;CACrD,YAAIrF,GAAG,CAACX,MAAJ,GAAa,CAAb,IAAkB+J,GAAG,CAAC/J,MAAJ,GAAa,CAAnC,EAAsC;CACpC,iBAAO,CAAC,CAAR;CACD;;CACDmK,QAAAA,SAAS,GAAG,CAAZ;CACAC,QAAAA,SAAS,IAAI,CAAb;CACAC,QAAAA,SAAS,IAAI,CAAb;CACA9F,QAAAA,UAAU,IAAI,CAAd;CACD;CACF;;CAED,aAAS+F,IAAT,CAAe5F,GAAf,EAAoB5E,CAApB,EAAuB;CACrB,UAAIqK,SAAS,KAAK,CAAlB,EAAqB;CACnB,eAAOzF,GAAG,CAAC5E,CAAD,CAAV;CACD,OAFD,MAEO;CACL,eAAO4E,GAAG,CAAC6F,YAAJ,CAAiBzK,CAAC,GAAGqK,SAArB,CAAP;CACD;CACF;;CAED,QAAIrK,CAAJ;;CACA,QAAIkK,GAAJ,EAAS;CACP,UAAIQ,UAAU,GAAG,CAAC,CAAlB;;CACA,WAAK1K,CAAC,GAAGyE,UAAT,EAAqBzE,CAAC,GAAGsK,SAAzB,EAAoCtK,CAAC,EAArC,EAAyC;CACvC,YAAIwK,IAAI,CAAC3J,GAAD,EAAMb,CAAN,CAAJ,KAAiBwK,IAAI,CAACP,GAAD,EAAMS,UAAU,KAAK,CAAC,CAAhB,GAAoB,CAApB,GAAwB1K,CAAC,GAAG0K,UAAlC,CAAzB,EAAwE;CACtE,cAAIA,UAAU,KAAK,CAAC,CAApB,EAAuBA,UAAU,GAAG1K,CAAb;CACvB,cAAIA,CAAC,GAAG0K,UAAJ,GAAiB,CAAjB,KAAuBH,SAA3B,EAAsC,OAAOG,UAAU,GAAGL,SAApB;CACvC,SAHD,MAGO;CACL,cAAIK,UAAU,KAAK,CAAC,CAApB,EAAuB1K,CAAC,IAAIA,CAAC,GAAG0K,UAAT;CACvBA,UAAAA,UAAU,GAAG,CAAC,CAAd;CACD;CACF;CACF,KAXD,MAWO;CACL,UAAIjG,UAAU,GAAG8F,SAAb,GAAyBD,SAA7B,EAAwC7F,UAAU,GAAG6F,SAAS,GAAGC,SAAzB;;CACxC,WAAKvK,CAAC,GAAGyE,UAAT,EAAqBzE,CAAC,IAAI,CAA1B,EAA6BA,CAAC,EAA9B,EAAkC;CAChC,YAAI2K,KAAK,GAAG,IAAZ;;CACA,aAAK,IAAIC,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGL,SAApB,EAA+BK,CAAC,EAAhC,EAAoC;CAClC,cAAIJ,IAAI,CAAC3J,GAAD,EAAMb,CAAC,GAAG4K,CAAV,CAAJ,KAAqBJ,IAAI,CAACP,GAAD,EAAMW,CAAN,CAA7B,EAAuC;CACrCD,YAAAA,KAAK,GAAG,KAAR;CACA;CACD;CACF;;CACD,YAAIA,KAAJ,EAAW,OAAO3K,CAAP;CACZ;CACF;;CAED,WAAO,CAAC,CAAR;CACD;;CAEDwD,EAAAA,MAAM,CAACW,SAAP,CAAiB0G,QAAjB,GAA4B,SAASA,QAAT,CAAmBZ,GAAnB,EAAwBxF,UAAxB,EAAoCyB,QAApC,EAA8C;CACxE,WAAO,KAAK1F,OAAL,CAAayJ,GAAb,EAAkBxF,UAAlB,EAA8ByB,QAA9B,MAA4C,CAAC,CAApD;CACD,GAFD;;CAIA1C,EAAAA,MAAM,CAACW,SAAP,CAAiB3D,OAAjB,GAA2B,SAASA,OAAT,CAAkByJ,GAAlB,EAAuBxF,UAAvB,EAAmCyB,QAAnC,EAA6C;CACtE,WAAO8D,oBAAoB,CAAC,IAAD,EAAOC,GAAP,EAAYxF,UAAZ,EAAwByB,QAAxB,EAAkC,IAAlC,CAA3B;CACD,GAFD;;CAIA1C,EAAAA,MAAM,CAACW,SAAP,CAAiBiG,WAAjB,GAA+B,SAASA,WAAT,CAAsBH,GAAtB,EAA2BxF,UAA3B,EAAuCyB,QAAvC,EAAiD;CAC9E,WAAO8D,oBAAoB,CAAC,IAAD,EAAOC,GAAP,EAAYxF,UAAZ,EAAwByB,QAAxB,EAAkC,KAAlC,CAA3B;CACD,GAFD;;CAIA,WAAS4E,QAAT,CAAmBlG,GAAnB,EAAwByB,MAAxB,EAAgCxE,MAAhC,EAAwC3B,MAAxC,EAAgD;CAC9C2B,IAAAA,MAAM,GAAGkJ,MAAM,CAAClJ,MAAD,CAAN,IAAkB,CAA3B;CACA,QAAImJ,SAAS,GAAGpG,GAAG,CAAC1E,MAAJ,GAAa2B,MAA7B;;CACA,QAAI,CAAC3B,MAAL,EAAa;CACXA,MAAAA,MAAM,GAAG8K,SAAT;CACD,KAFD,MAEO;CACL9K,MAAAA,MAAM,GAAG6K,MAAM,CAAC7K,MAAD,CAAf;;CACA,UAAIA,MAAM,GAAG8K,SAAb,EAAwB;CACtB9K,QAAAA,MAAM,GAAG8K,SAAT;CACD;CACF;;CAED,QAAIC,MAAM,GAAG5E,MAAM,CAACnG,MAApB;;CAEA,QAAIA,MAAM,GAAG+K,MAAM,GAAG,CAAtB,EAAyB;CACvB/K,MAAAA,MAAM,GAAG+K,MAAM,GAAG,CAAlB;CACD;;CACD,SAAK,IAAIjL,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4B,EAAEF,CAA9B,EAAiC;CAC/B,UAAIkL,MAAM,GAAGC,QAAQ,CAAC9E,MAAM,CAAC+E,MAAP,CAAcpL,CAAC,GAAG,CAAlB,EAAqB,CAArB,CAAD,EAA0B,EAA1B,CAArB;CACA,UAAI+G,WAAW,CAACmE,MAAD,CAAf,EAAyB,OAAOlL,CAAP;CACzB4E,MAAAA,GAAG,CAAC/C,MAAM,GAAG7B,CAAV,CAAH,GAAkBkL,MAAlB;CACD;;CACD,WAAOlL,CAAP;CACD;;CAED,WAASqL,SAAT,CAAoBzG,GAApB,EAAyByB,MAAzB,EAAiCxE,MAAjC,EAAyC3B,MAAzC,EAAiD;CAC/C,WAAOoL,UAAU,CAAClD,WAAW,CAAC/B,MAAD,EAASzB,GAAG,CAAC1E,MAAJ,GAAa2B,MAAtB,CAAZ,EAA2C+C,GAA3C,EAAgD/C,MAAhD,EAAwD3B,MAAxD,CAAjB;CACD;;CAED,WAASqL,UAAT,CAAqB3G,GAArB,EAA0ByB,MAA1B,EAAkCxE,MAAlC,EAA0C3B,MAA1C,EAAkD;CAChD,WAAOoL,UAAU,CAACE,YAAY,CAACnF,MAAD,CAAb,EAAuBzB,GAAvB,EAA4B/C,MAA5B,EAAoC3B,MAApC,CAAjB;CACD;;CAED,WAASuL,WAAT,CAAsB7G,GAAtB,EAA2ByB,MAA3B,EAAmCxE,MAAnC,EAA2C3B,MAA3C,EAAmD;CACjD,WAAOoL,UAAU,CAACjD,aAAa,CAAChC,MAAD,CAAd,EAAwBzB,GAAxB,EAA6B/C,MAA7B,EAAqC3B,MAArC,CAAjB;CACD;;CAED,WAASwL,SAAT,CAAoB9G,GAApB,EAAyByB,MAAzB,EAAiCxE,MAAjC,EAAyC3B,MAAzC,EAAiD;CAC/C,WAAOoL,UAAU,CAACK,cAAc,CAACtF,MAAD,EAASzB,GAAG,CAAC1E,MAAJ,GAAa2B,MAAtB,CAAf,EAA8C+C,GAA9C,EAAmD/C,MAAnD,EAA2D3B,MAA3D,CAAjB;CACD;;CAEDsD,EAAAA,MAAM,CAACW,SAAP,CAAiBqC,KAAjB,GAAyB,SAASA,KAAT,CAAgBH,MAAhB,EAAwBxE,MAAxB,EAAgC3B,MAAhC,EAAwCgG,QAAxC,EAAkD;;CAEzE,QAAIrE,MAAM,KAAK2C,SAAf,EAA0B;CACxB0B,MAAAA,QAAQ,GAAG,MAAX;CACAhG,MAAAA,MAAM,GAAG,KAAKA,MAAd;CACA2B,MAAAA,MAAM,GAAG,CAAT,CAHwB;CAKzB,KALD,MAKO,IAAI3B,MAAM,KAAKsE,SAAX,IAAwB,OAAO3C,MAAP,KAAkB,QAA9C,EAAwD;CAC7DqE,MAAAA,QAAQ,GAAGrE,MAAX;CACA3B,MAAAA,MAAM,GAAG,KAAKA,MAAd;CACA2B,MAAAA,MAAM,GAAG,CAAT,CAH6D;CAK9D,KALM,MAKA,IAAI+J,QAAQ,CAAC/J,MAAD,CAAZ,EAAsB;CAC3BA,MAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;CACA,UAAI+J,QAAQ,CAAC1L,MAAD,CAAZ,EAAsB;CACpBA,QAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,YAAIgG,QAAQ,KAAK1B,SAAjB,EAA4B0B,QAAQ,GAAG,MAAX;CAC7B,OAHD,MAGO;CACLA,QAAAA,QAAQ,GAAGhG,MAAX;CACAA,QAAAA,MAAM,GAAGsE,SAAT;CACD;CACF,KATM,MASA;CACL,YAAM,IAAIlE,KAAJ,CACJ,yEADI,CAAN;CAGD;;CAED,QAAI0K,SAAS,GAAG,KAAK9K,MAAL,GAAc2B,MAA9B;CACA,QAAI3B,MAAM,KAAKsE,SAAX,IAAwBtE,MAAM,GAAG8K,SAArC,EAAgD9K,MAAM,GAAG8K,SAAT;;CAEhD,QAAK3E,MAAM,CAACnG,MAAP,GAAgB,CAAhB,KAAsBA,MAAM,GAAG,CAAT,IAAc2B,MAAM,GAAG,CAA7C,CAAD,IAAqDA,MAAM,GAAG,KAAK3B,MAAvE,EAA+E;CAC7E,YAAM,IAAIyE,UAAJ,CAAe,wCAAf,CAAN;CACD;;CAED,QAAI,CAACuB,QAAL,EAAeA,QAAQ,GAAG,MAAX;CAEf,QAAIiC,WAAW,GAAG,KAAlB;;CACA,aAAS;CACP,cAAQjC,QAAR;CACE,aAAK,KAAL;CACE,iBAAO4E,QAAQ,CAAC,IAAD,EAAOzE,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAf;;CAEF,aAAK,MAAL;CACA,aAAK,OAAL;CACE,iBAAOmL,SAAS,CAAC,IAAD,EAAOhF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAhB;;CAEF,aAAK,OAAL;CACA,aAAK,QAAL;CACA,aAAK,QAAL;CACE,iBAAOqL,UAAU,CAAC,IAAD,EAAOlF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAjB;;CAEF,aAAK,QAAL;;CAEE,iBAAOuL,WAAW,CAAC,IAAD,EAAOpF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAlB;;CAEF,aAAK,MAAL;CACA,aAAK,OAAL;CACA,aAAK,SAAL;CACA,aAAK,UAAL;CACE,iBAAOwL,SAAS,CAAC,IAAD,EAAOrF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAhB;;CAEF;CACE,cAAIiI,WAAJ,EAAiB,MAAM,IAAIpD,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;CACjBA,UAAAA,QAAQ,GAAG,CAAC,KAAKA,QAAN,EAAgByB,WAAhB,EAAX;CACAQ,UAAAA,WAAW,GAAG,IAAd;CA1BJ;CA4BD;CACF,GAnED;;CAqEA3E,EAAAA,MAAM,CAACW,SAAP,CAAiB0H,MAAjB,GAA0B,SAASA,MAAT,GAAmB;CAC3C,WAAO;CACL7E,MAAAA,IAAI,EAAE,QADD;CAELE,MAAAA,IAAI,EAAEpH,KAAK,CAACqE,SAAN,CAAgBsC,KAAhB,CAAsBuB,IAAtB,CAA2B,KAAK8D,IAAL,IAAa,IAAxC,EAA8C,CAA9C;CAFD,KAAP;CAID,GALD;;CAOA,WAASnD,WAAT,CAAsB/D,GAAtB,EAA2BzD,KAA3B,EAAkCC,GAAlC,EAAuC;CACrC,QAAID,KAAK,KAAK,CAAV,IAAeC,GAAG,KAAKwD,GAAG,CAAC1E,MAA/B,EAAuC;CACrC,aAAO6L,QAAM,CAACtM,aAAP,CAAqBmF,GAArB,CAAP;CACD,KAFD,MAEO;CACL,aAAOmH,QAAM,CAACtM,aAAP,CAAqBmF,GAAG,CAAC6B,KAAJ,CAAUtF,KAAV,EAAiBC,GAAjB,CAArB,CAAP;CACD;CACF;;CAED,WAASoH,SAAT,CAAoB5D,GAApB,EAAyBzD,KAAzB,EAAgCC,GAAhC,EAAqC;CACnCA,IAAAA,GAAG,GAAGuB,IAAI,CAAC8E,GAAL,CAAS7C,GAAG,CAAC1E,MAAb,EAAqBkB,GAArB,CAAN;CACA,QAAI4K,GAAG,GAAG,EAAV;CAEA,QAAIhM,CAAC,GAAGmB,KAAR;;CACA,WAAOnB,CAAC,GAAGoB,GAAX,EAAgB;CACd,UAAI6K,SAAS,GAAGrH,GAAG,CAAC5E,CAAD,CAAnB;CACA,UAAIkM,SAAS,GAAG,IAAhB;CACA,UAAIC,gBAAgB,GAAIF,SAAS,GAAG,IAAb,GACnB,CADmB,GAElBA,SAAS,GAAG,IAAb,GACI,CADJ,GAEKA,SAAS,GAAG,IAAb,GACI,CADJ,GAEI,CANZ;;CAQA,UAAIjM,CAAC,GAAGmM,gBAAJ,IAAwB/K,GAA5B,EAAiC;CAC/B,YAAIgL,UAAJ,EAAgBC,SAAhB,EAA2BC,UAA3B,EAAuCC,aAAvC;;CAEA,gBAAQJ,gBAAR;CACE,eAAK,CAAL;CACE,gBAAIF,SAAS,GAAG,IAAhB,EAAsB;CACpBC,cAAAA,SAAS,GAAGD,SAAZ;CACD;;CACD;;CACF,eAAK,CAAL;CACEG,YAAAA,UAAU,GAAGxH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;;CACA,gBAAI,CAACoM,UAAU,GAAG,IAAd,MAAwB,IAA5B,EAAkC;CAChCG,cAAAA,aAAa,GAAG,CAACN,SAAS,GAAG,IAAb,KAAsB,GAAtB,GAA6BG,UAAU,GAAG,IAA1D;;CACA,kBAAIG,aAAa,GAAG,IAApB,EAA0B;CACxBL,gBAAAA,SAAS,GAAGK,aAAZ;CACD;CACF;;CACD;;CACF,eAAK,CAAL;CACEH,YAAAA,UAAU,GAAGxH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;CACAqM,YAAAA,SAAS,GAAGzH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAf;;CACA,gBAAI,CAACoM,UAAU,GAAG,IAAd,MAAwB,IAAxB,IAAgC,CAACC,SAAS,GAAG,IAAb,MAAuB,IAA3D,EAAiE;CAC/DE,cAAAA,aAAa,GAAG,CAACN,SAAS,GAAG,GAAb,KAAqB,GAArB,GAA2B,CAACG,UAAU,GAAG,IAAd,KAAuB,GAAlD,GAAyDC,SAAS,GAAG,IAArF;;CACA,kBAAIE,aAAa,GAAG,KAAhB,KAA0BA,aAAa,GAAG,MAAhB,IAA0BA,aAAa,GAAG,MAApE,CAAJ,EAAiF;CAC/EL,gBAAAA,SAAS,GAAGK,aAAZ;CACD;CACF;;CACD;;CACF,eAAK,CAAL;CACEH,YAAAA,UAAU,GAAGxH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;CACAqM,YAAAA,SAAS,GAAGzH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAf;CACAsM,YAAAA,UAAU,GAAG1H,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;;CACA,gBAAI,CAACoM,UAAU,GAAG,IAAd,MAAwB,IAAxB,IAAgC,CAACC,SAAS,GAAG,IAAb,MAAuB,IAAvD,IAA+D,CAACC,UAAU,GAAG,IAAd,MAAwB,IAA3F,EAAiG;CAC/FC,cAAAA,aAAa,GAAG,CAACN,SAAS,GAAG,GAAb,KAAqB,IAArB,GAA4B,CAACG,UAAU,GAAG,IAAd,KAAuB,GAAnD,GAAyD,CAACC,SAAS,GAAG,IAAb,KAAsB,GAA/E,GAAsFC,UAAU,GAAG,IAAnH;;CACA,kBAAIC,aAAa,GAAG,MAAhB,IAA0BA,aAAa,GAAG,QAA9C,EAAwD;CACtDL,gBAAAA,SAAS,GAAGK,aAAZ;CACD;CACF;;CAlCL;CAoCD;;CAED,UAAIL,SAAS,KAAK,IAAlB,EAAwB;;;CAGtBA,QAAAA,SAAS,GAAG,MAAZ;CACAC,QAAAA,gBAAgB,GAAG,CAAnB;CACD,OALD,MAKO,IAAID,SAAS,GAAG,MAAhB,EAAwB;;CAE7BA,QAAAA,SAAS,IAAI,OAAb;CACAF,QAAAA,GAAG,CAAC1K,IAAJ,CAAS4K,SAAS,KAAK,EAAd,GAAmB,KAAnB,GAA2B,MAApC;CACAA,QAAAA,SAAS,GAAG,SAASA,SAAS,GAAG,KAAjC;CACD;;CAEDF,MAAAA,GAAG,CAAC1K,IAAJ,CAAS4K,SAAT;CACAlM,MAAAA,CAAC,IAAImM,gBAAL;CACD;;CAED,WAAOK,qBAAqB,CAACR,GAAD,CAA5B;CACD;CAGD;CACA;;;CACA,MAAIS,oBAAoB,GAAG,MAA3B;;CAEA,WAASD,qBAAT,CAAgCE,UAAhC,EAA4C;CAC1C,QAAIzM,GAAG,GAAGyM,UAAU,CAACxM,MAArB;;CACA,QAAID,GAAG,IAAIwM,oBAAX,EAAiC;CAC/B,aAAO/E,MAAM,CAACiF,YAAP,CAAoBzD,KAApB,CAA0BxB,MAA1B,EAAkCgF,UAAlC,CAAP,CAD+B;CAEhC,KAJyC;;;CAO1C,QAAIV,GAAG,GAAG,EAAV;CACA,QAAIhM,CAAC,GAAG,CAAR;;CACA,WAAOA,CAAC,GAAGC,GAAX,EAAgB;CACd+L,MAAAA,GAAG,IAAItE,MAAM,CAACiF,YAAP,CAAoBzD,KAApB,CACLxB,MADK,EAELgF,UAAU,CAACjG,KAAX,CAAiBzG,CAAjB,EAAoBA,CAAC,IAAIyM,oBAAzB,CAFK,CAAP;CAID;;CACD,WAAOT,GAAP;CACD;;CAED,WAASvD,UAAT,CAAqB7D,GAArB,EAA0BzD,KAA1B,EAAiCC,GAAjC,EAAsC;CACpC,QAAIwL,GAAG,GAAG,EAAV;CACAxL,IAAAA,GAAG,GAAGuB,IAAI,CAAC8E,GAAL,CAAS7C,GAAG,CAAC1E,MAAb,EAAqBkB,GAArB,CAAN;;CAEA,SAAK,IAAIpB,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6B,EAAEpB,CAA/B,EAAkC;CAChC4M,MAAAA,GAAG,IAAIlF,MAAM,CAACiF,YAAP,CAAoB/H,GAAG,CAAC5E,CAAD,CAAH,GAAS,IAA7B,CAAP;CACD;;CACD,WAAO4M,GAAP;CACD;;CAED,WAASlE,WAAT,CAAsB9D,GAAtB,EAA2BzD,KAA3B,EAAkCC,GAAlC,EAAuC;CACrC,QAAIwL,GAAG,GAAG,EAAV;CACAxL,IAAAA,GAAG,GAAGuB,IAAI,CAAC8E,GAAL,CAAS7C,GAAG,CAAC1E,MAAb,EAAqBkB,GAArB,CAAN;;CAEA,SAAK,IAAIpB,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6B,EAAEpB,CAA/B,EAAkC;CAChC4M,MAAAA,GAAG,IAAIlF,MAAM,CAACiF,YAAP,CAAoB/H,GAAG,CAAC5E,CAAD,CAAvB,CAAP;CACD;;CACD,WAAO4M,GAAP;CACD;;CAED,WAASrE,QAAT,CAAmB3D,GAAnB,EAAwBzD,KAAxB,EAA+BC,GAA/B,EAAoC;CAClC,QAAInB,GAAG,GAAG2E,GAAG,CAAC1E,MAAd;CAEA,QAAI,CAACiB,KAAD,IAAUA,KAAK,GAAG,CAAtB,EAAyBA,KAAK,GAAG,CAAR;CACzB,QAAI,CAACC,GAAD,IAAQA,GAAG,GAAG,CAAd,IAAmBA,GAAG,GAAGnB,GAA7B,EAAkCmB,GAAG,GAAGnB,GAAN;CAElC,QAAI4M,GAAG,GAAG,EAAV;;CACA,SAAK,IAAI7M,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6B,EAAEpB,CAA/B,EAAkC;CAChC6M,MAAAA,GAAG,IAAIC,mBAAmB,CAAClI,GAAG,CAAC5E,CAAD,CAAJ,CAA1B;CACD;;CACD,WAAO6M,GAAP;CACD;;CAED,WAASjE,YAAT,CAAuBhE,GAAvB,EAA4BzD,KAA5B,EAAmCC,GAAnC,EAAwC;CACtC,QAAI2L,KAAK,GAAGnI,GAAG,CAAC6B,KAAJ,CAAUtF,KAAV,EAAiBC,GAAjB,CAAZ;CACA,QAAI4K,GAAG,GAAG,EAAV,CAFsC;;CAItC,SAAK,IAAIhM,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAG+M,KAAK,CAAC7M,MAAN,GAAe,CAAnC,EAAsCF,CAAC,IAAI,CAA3C,EAA8C;CAC5CgM,MAAAA,GAAG,IAAItE,MAAM,CAACiF,YAAP,CAAoBI,KAAK,CAAC/M,CAAD,CAAL,GAAY+M,KAAK,CAAC/M,CAAC,GAAG,CAAL,CAAL,GAAe,GAA/C,CAAP;CACD;;CACD,WAAOgM,GAAP;CACD;;CAEDxI,EAAAA,MAAM,CAACW,SAAP,CAAiBsC,KAAjB,GAAyB,SAASA,KAAT,CAAgBtF,KAAhB,EAAuBC,GAAvB,EAA4B;CACnD,QAAInB,GAAG,GAAG,KAAKC,MAAf;CACAiB,IAAAA,KAAK,GAAG,CAAC,CAACA,KAAV;CACAC,IAAAA,GAAG,GAAGA,GAAG,KAAKoD,SAAR,GAAoBvE,GAApB,GAA0B,CAAC,CAACmB,GAAlC;;CAEA,QAAID,KAAK,GAAG,CAAZ,EAAe;CACbA,MAAAA,KAAK,IAAIlB,GAAT;CACA,UAAIkB,KAAK,GAAG,CAAZ,EAAeA,KAAK,GAAG,CAAR;CAChB,KAHD,MAGO,IAAIA,KAAK,GAAGlB,GAAZ,EAAiB;CACtBkB,MAAAA,KAAK,GAAGlB,GAAR;CACD;;CAED,QAAImB,GAAG,GAAG,CAAV,EAAa;CACXA,MAAAA,GAAG,IAAInB,GAAP;CACA,UAAImB,GAAG,GAAG,CAAV,EAAaA,GAAG,GAAG,CAAN;CACd,KAHD,MAGO,IAAIA,GAAG,GAAGnB,GAAV,EAAe;CACpBmB,MAAAA,GAAG,GAAGnB,GAAN;CACD;;CAED,QAAImB,GAAG,GAAGD,KAAV,EAAiBC,GAAG,GAAGD,KAAN;CAEjB,QAAI6L,MAAM,GAAG,KAAKC,QAAL,CAAc9L,KAAd,EAAqBC,GAArB,CAAb,CArBmD;;CAuBnD6C,IAAAA,MAAM,CAACC,cAAP,CAAsB8I,MAAtB,EAA8BxJ,MAAM,CAACW,SAArC;CAEA,WAAO6I,MAAP;CACD,GA1BD;CA4BA;CACA;CACA;;;CACA,WAASE,WAAT,CAAsBrL,MAAtB,EAA8BsL,GAA9B,EAAmCjN,MAAnC,EAA2C;CACzC,QAAK2B,MAAM,GAAG,CAAV,KAAiB,CAAjB,IAAsBA,MAAM,GAAG,CAAnC,EAAsC,MAAM,IAAI8C,UAAJ,CAAe,oBAAf,CAAN;CACtC,QAAI9C,MAAM,GAAGsL,GAAT,GAAejN,MAAnB,EAA2B,MAAM,IAAIyE,UAAJ,CAAe,uCAAf,CAAN;CAC5B;;CAEDnB,EAAAA,MAAM,CAACW,SAAP,CAAiBiJ,UAAjB,GACA5J,MAAM,CAACW,SAAP,CAAiBkJ,UAAjB,GAA8B,SAASA,UAAT,CAAqBxL,MAArB,EAA6BtC,UAA7B,EAAyC+N,QAAzC,EAAmD;CAC/EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;CACA,QAAI,CAAC+N,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;CAEf,QAAI+J,GAAG,GAAG,KAAKpI,MAAL,CAAV;CACA,QAAI0L,GAAG,GAAG,CAAV;CACA,QAAIvN,CAAC,GAAG,CAAR;;CACA,WAAO,EAAEA,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;CACzCtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG7B,CAAd,IAAmBuN,GAA1B;CACD;;CAED,WAAOtD,GAAP;CACD,GAdD;;CAgBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBqJ,UAAjB,GACAhK,MAAM,CAACW,SAAP,CAAiBsJ,UAAjB,GAA8B,SAASA,UAAT,CAAqB5L,MAArB,EAA6BtC,UAA7B,EAAyC+N,QAAzC,EAAmD;CAC/EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;;CACA,QAAI,CAAC+N,QAAL,EAAe;CACbJ,MAAAA,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;CACD;;CAED,QAAI+J,GAAG,GAAG,KAAKpI,MAAM,GAAG,EAAEtC,UAAhB,CAAV;CACA,QAAIgO,GAAG,GAAG,CAAV;;CACA,WAAOhO,UAAU,GAAG,CAAb,KAAmBgO,GAAG,IAAI,KAA1B,CAAP,EAAyC;CACvCtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG,EAAEtC,UAAhB,IAA8BgO,GAArC;CACD;;CAED,WAAOtD,GAAP;CACD,GAfD;;CAiBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBuJ,SAAjB,GACAlK,MAAM,CAACW,SAAP,CAAiBwJ,SAAjB,GAA6B,SAASA,SAAT,CAAoB9L,MAApB,EAA4ByL,QAA5B,EAAsC;CACjEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAO,KAAK2B,MAAL,CAAP;CACD,GALD;;CAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiByJ,YAAjB,GACApK,MAAM,CAACW,SAAP,CAAiB0J,YAAjB,GAAgC,SAASA,YAAT,CAAuBhM,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAO,KAAK2B,MAAL,IAAgB,KAAKA,MAAM,GAAG,CAAd,KAAoB,CAA3C;CACD,GALD;;CAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB2J,YAAjB,GACAtK,MAAM,CAACW,SAAP,CAAiBsG,YAAjB,GAAgC,SAASA,YAAT,CAAuB5I,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAQ,KAAK2B,MAAL,KAAgB,CAAjB,GAAsB,KAAKA,MAAM,GAAG,CAAd,CAA7B;CACD,GALD;;CAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB4J,YAAjB,GACAvK,MAAM,CAACW,SAAP,CAAiB6J,YAAjB,GAAgC,SAASA,YAAT,CAAuBnM,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CAEf,WAAO,CAAE,KAAK2B,MAAL,CAAD,GACH,KAAKA,MAAM,GAAG,CAAd,KAAoB,CADjB,GAEH,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAFlB,IAGF,KAAKA,MAAM,GAAG,CAAd,IAAmB,SAHxB;CAID,GATD;;CAWA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB8J,YAAjB,GACAzK,MAAM,CAACW,SAAP,CAAiB+J,YAAjB,GAAgC,SAASA,YAAT,CAAuBrM,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CAEf,WAAQ,KAAK2B,MAAL,IAAe,SAAhB,IACH,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAArB,GACA,KAAKA,MAAM,GAAG,CAAd,KAAoB,CADpB,GAED,KAAKA,MAAM,GAAG,CAAd,CAHK,CAAP;CAID,GATD;;CAWA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBgK,SAAjB,GAA6B,SAASA,SAAT,CAAoBtM,MAApB,EAA4BtC,UAA5B,EAAwC+N,QAAxC,EAAkD;CAC7EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;CACA,QAAI,CAAC+N,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;CAEf,QAAI+J,GAAG,GAAG,KAAKpI,MAAL,CAAV;CACA,QAAI0L,GAAG,GAAG,CAAV;CACA,QAAIvN,CAAC,GAAG,CAAR;;CACA,WAAO,EAAEA,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;CACzCtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG7B,CAAd,IAAmBuN,GAA1B;CACD;;CACDA,IAAAA,GAAG,IAAI,IAAP;CAEA,QAAItD,GAAG,IAAIsD,GAAX,EAAgBtD,GAAG,IAAItH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,CAAP;CAEhB,WAAO0K,GAAP;CACD,GAhBD;;CAkBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBiK,SAAjB,GAA6B,SAASA,SAAT,CAAoBvM,MAApB,EAA4BtC,UAA5B,EAAwC+N,QAAxC,EAAkD;CAC7EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;CACA,QAAI,CAAC+N,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;CAEf,QAAIF,CAAC,GAAGT,UAAR;CACA,QAAIgO,GAAG,GAAG,CAAV;CACA,QAAItD,GAAG,GAAG,KAAKpI,MAAM,GAAG,EAAE7B,CAAhB,CAAV;;CACA,WAAOA,CAAC,GAAG,CAAJ,KAAUuN,GAAG,IAAI,KAAjB,CAAP,EAAgC;CAC9BtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG,EAAE7B,CAAhB,IAAqBuN,GAA5B;CACD;;CACDA,IAAAA,GAAG,IAAI,IAAP;CAEA,QAAItD,GAAG,IAAIsD,GAAX,EAAgBtD,GAAG,IAAItH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,CAAP;CAEhB,WAAO0K,GAAP;CACD,GAhBD;;CAkBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBkK,QAAjB,GAA4B,SAASA,QAAT,CAAmBxM,MAAnB,EAA2ByL,QAA3B,EAAqC;CAC/DzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,QAAI,EAAE,KAAK2B,MAAL,IAAe,IAAjB,CAAJ,EAA4B,OAAQ,KAAKA,MAAL,CAAR;CAC5B,WAAQ,CAAC,OAAO,KAAKA,MAAL,CAAP,GAAsB,CAAvB,IAA4B,CAAC,CAArC;CACD,GALD;;CAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBmK,WAAjB,GAA+B,SAASA,WAAT,CAAsBzM,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,QAAI+J,GAAG,GAAG,KAAKpI,MAAL,IAAgB,KAAKA,MAAM,GAAG,CAAd,KAAoB,CAA9C;CACA,WAAQoI,GAAG,GAAG,MAAP,GAAiBA,GAAG,GAAG,UAAvB,GAAoCA,GAA3C;CACD,GALD;;CAOAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBoK,WAAjB,GAA+B,SAASA,WAAT,CAAsB1M,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,QAAI+J,GAAG,GAAG,KAAKpI,MAAM,GAAG,CAAd,IAAoB,KAAKA,MAAL,KAAgB,CAA9C;CACA,WAAQoI,GAAG,GAAG,MAAP,GAAiBA,GAAG,GAAG,UAAvB,GAAoCA,GAA3C;CACD,GALD;;CAOAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBqK,WAAjB,GAA+B,SAASA,WAAT,CAAsB3M,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CAEf,WAAQ,KAAK2B,MAAL,CAAD,GACJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,CADhB,GAEJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAFhB,GAGJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAHvB;CAID,GARD;;CAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBsK,WAAjB,GAA+B,SAASA,WAAT,CAAsB5M,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CAEf,WAAQ,KAAK2B,MAAL,KAAgB,EAAjB,GACJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,EADhB,GAEJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,CAFhB,GAGJ,KAAKA,MAAM,GAAG,CAAd,CAHH;CAID,GARD;;CAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBuK,WAAjB,GAA+B,SAASA,WAAT,CAAsB7M,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,IAA3B,EAAiC,EAAjC,EAAqC,CAArC,CAAP;CACD,GAJD;;CAMA2B,EAAAA,MAAM,CAACW,SAAP,CAAiByK,WAAjB,GAA+B,SAASA,WAAT,CAAsB/M,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,KAA3B,EAAkC,EAAlC,EAAsC,CAAtC,CAAP;CACD,GAJD;;CAMA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB0K,YAAjB,GAAgC,SAASA,YAAT,CAAuBhN,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,IAA3B,EAAiC,EAAjC,EAAqC,CAArC,CAAP;CACD,GAJD;;CAMA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB2K,YAAjB,GAAgC,SAASA,YAAT,CAAuBjN,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,KAA3B,EAAkC,EAAlC,EAAsC,CAAtC,CAAP;CACD,GAJD;;CAMA,WAASkN,QAAT,CAAmBnK,GAAnB,EAAwB/B,KAAxB,EAA+BhB,MAA/B,EAAuCsL,GAAvC,EAA4C5D,GAA5C,EAAiD9B,GAAjD,EAAsD;CACpD,QAAI,CAACjE,MAAM,CAACe,QAAP,CAAgBK,GAAhB,CAAL,EAA2B,MAAM,IAAIG,SAAJ,CAAc,6CAAd,CAAN;CAC3B,QAAIlC,KAAK,GAAG0G,GAAR,IAAe1G,KAAK,GAAG4E,GAA3B,EAAgC,MAAM,IAAI9C,UAAJ,CAAe,mCAAf,CAAN;CAChC,QAAI9C,MAAM,GAAGsL,GAAT,GAAevI,GAAG,CAAC1E,MAAvB,EAA+B,MAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;CAChC;;CAEDnB,EAAAA,MAAM,CAACW,SAAP,CAAiB6K,WAAjB,GACAxL,MAAM,CAACW,SAAP,CAAiB8K,WAAjB,GAA+B,SAASA,WAAT,CAAsBpM,KAAtB,EAA6BhB,MAA7B,EAAqCtC,UAArC,EAAiD+N,QAAjD,EAA2D;CACxFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;;CACA,QAAI,CAAC+N,QAAL,EAAe;CACb,UAAI4B,QAAQ,GAAGvM,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,IAA8B,CAA7C;CACAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkC2P,QAAlC,EAA4C,CAA5C,CAAR;CACD;;CAED,QAAI3B,GAAG,GAAG,CAAV;CACA,QAAIvN,CAAC,GAAG,CAAR;CACA,SAAK6B,MAAL,IAAegB,KAAK,GAAG,IAAvB;;CACA,WAAO,EAAE7C,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;CACzC,WAAK1L,MAAM,GAAG7B,CAAd,IAAoB6C,KAAK,GAAG0K,GAAT,GAAgB,IAAnC;CACD;;CAED,WAAO1L,MAAM,GAAGtC,UAAhB;CACD,GAlBD;;CAoBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiBgL,WAAjB,GACA3L,MAAM,CAACW,SAAP,CAAiBiL,WAAjB,GAA+B,SAASA,WAAT,CAAsBvM,KAAtB,EAA6BhB,MAA7B,EAAqCtC,UAArC,EAAiD+N,QAAjD,EAA2D;CACxFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;;CACA,QAAI,CAAC+N,QAAL,EAAe;CACb,UAAI4B,QAAQ,GAAGvM,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,IAA8B,CAA7C;CACAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkC2P,QAAlC,EAA4C,CAA5C,CAAR;CACD;;CAED,QAAIlP,CAAC,GAAGT,UAAU,GAAG,CAArB;CACA,QAAIgO,GAAG,GAAG,CAAV;CACA,SAAK1L,MAAM,GAAG7B,CAAd,IAAmB6C,KAAK,GAAG,IAA3B;;CACA,WAAO,EAAE7C,CAAF,IAAO,CAAP,KAAauN,GAAG,IAAI,KAApB,CAAP,EAAmC;CACjC,WAAK1L,MAAM,GAAG7B,CAAd,IAAoB6C,KAAK,GAAG0K,GAAT,GAAgB,IAAnC;CACD;;CAED,WAAO1L,MAAM,GAAGtC,UAAhB;CACD,GAlBD;;CAoBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiBkL,UAAjB,GACA7L,MAAM,CAACW,SAAP,CAAiBmL,UAAjB,GAA8B,SAASA,UAAT,CAAqBzM,KAArB,EAA4BhB,MAA5B,EAAoCyL,QAApC,EAA8C;CAC1EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,IAAzB,EAA+B,CAA/B,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAPD;;CASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBoL,aAAjB,GACA/L,MAAM,CAACW,SAAP,CAAiBqL,aAAjB,GAAiC,SAASA,aAAT,CAAwB3M,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAjC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GARD;;CAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBsL,aAAjB,GACAjM,MAAM,CAACW,SAAP,CAAiBuL,aAAjB,GAAiC,SAASA,aAAT,CAAwB7M,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAjC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,KAAK,CAA1B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GARD;;CAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBwL,aAAjB,GACAnM,MAAM,CAACW,SAAP,CAAiByL,aAAjB,GAAiC,SAASA,aAAT,CAAwB/M,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAArC,CAAR;CACf,SAAKA,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,SAAKhB,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAVD;;CAYA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB0L,aAAjB,GACArM,MAAM,CAACW,SAAP,CAAiB2L,aAAjB,GAAiC,SAASA,aAAT,CAAwBjN,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAArC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,KAAK,EAA1B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAVD;;CAYA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB4L,UAAjB,GAA8B,SAASA,UAAT,CAAqBlN,KAArB,EAA4BhB,MAA5B,EAAoCtC,UAApC,EAAgD+N,QAAhD,EAA0D;CACtFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;CACA,QAAI,CAACyL,QAAL,EAAe;CACb,UAAI0C,KAAK,GAAGrN,IAAI,CAACC,GAAL,CAAS,CAAT,EAAa,IAAIrD,UAAL,GAAmB,CAA/B,CAAZ;CAEAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkCyQ,KAAK,GAAG,CAA1C,EAA6C,CAACA,KAA9C,CAAR;CACD;;CAED,QAAIhQ,CAAC,GAAG,CAAR;CACA,QAAIuN,GAAG,GAAG,CAAV;CACA,QAAI0C,GAAG,GAAG,CAAV;CACA,SAAKpO,MAAL,IAAegB,KAAK,GAAG,IAAvB;;CACA,WAAO,EAAE7C,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;CACzC,UAAI1K,KAAK,GAAG,CAAR,IAAaoN,GAAG,KAAK,CAArB,IAA0B,KAAKpO,MAAM,GAAG7B,CAAT,GAAa,CAAlB,MAAyB,CAAvD,EAA0D;CACxDiQ,QAAAA,GAAG,GAAG,CAAN;CACD;;CACD,WAAKpO,MAAM,GAAG7B,CAAd,IAAmB,CAAE6C,KAAK,GAAG0K,GAAT,IAAiB,CAAlB,IAAuB0C,GAAvB,GAA6B,IAAhD;CACD;;CAED,WAAOpO,MAAM,GAAGtC,UAAhB;CACD,GArBD;;CAuBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiB+L,UAAjB,GAA8B,SAASA,UAAT,CAAqBrN,KAArB,EAA4BhB,MAA5B,EAAoCtC,UAApC,EAAgD+N,QAAhD,EAA0D;CACtFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;CACA,QAAI,CAACyL,QAAL,EAAe;CACb,UAAI0C,KAAK,GAAGrN,IAAI,CAACC,GAAL,CAAS,CAAT,EAAa,IAAIrD,UAAL,GAAmB,CAA/B,CAAZ;CAEAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkCyQ,KAAK,GAAG,CAA1C,EAA6C,CAACA,KAA9C,CAAR;CACD;;CAED,QAAIhQ,CAAC,GAAGT,UAAU,GAAG,CAArB;CACA,QAAIgO,GAAG,GAAG,CAAV;CACA,QAAI0C,GAAG,GAAG,CAAV;CACA,SAAKpO,MAAM,GAAG7B,CAAd,IAAmB6C,KAAK,GAAG,IAA3B;;CACA,WAAO,EAAE7C,CAAF,IAAO,CAAP,KAAauN,GAAG,IAAI,KAApB,CAAP,EAAmC;CACjC,UAAI1K,KAAK,GAAG,CAAR,IAAaoN,GAAG,KAAK,CAArB,IAA0B,KAAKpO,MAAM,GAAG7B,CAAT,GAAa,CAAlB,MAAyB,CAAvD,EAA0D;CACxDiQ,QAAAA,GAAG,GAAG,CAAN;CACD;;CACD,WAAKpO,MAAM,GAAG7B,CAAd,IAAmB,CAAE6C,KAAK,GAAG0K,GAAT,IAAiB,CAAlB,IAAuB0C,GAAvB,GAA6B,IAAhD;CACD;;CAED,WAAOpO,MAAM,GAAGtC,UAAhB;CACD,GArBD;;CAuBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiBgM,SAAjB,GAA6B,SAASA,SAAT,CAAoBtN,KAApB,EAA2BhB,MAA3B,EAAmCyL,QAAnC,EAA6C;CACxEzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,IAAzB,EAA+B,CAAC,IAAhC,CAAR;CACf,QAAIgB,KAAK,GAAG,CAAZ,EAAeA,KAAK,GAAG,OAAOA,KAAP,GAAe,CAAvB;CACf,SAAKhB,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAPD;;CASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBiM,YAAjB,GAAgC,SAASA,YAAT,CAAuBvN,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAC,MAAlC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAPD;;CASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBkM,YAAjB,GAAgC,SAASA,YAAT,CAAuBxN,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAC,MAAlC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,KAAK,CAA1B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAPD;;CASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBmM,YAAjB,GAAgC,SAASA,YAAT,CAAuBzN,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAAC,UAAtC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GATD;;CAWA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBoM,YAAjB,GAAgC,SAASA,YAAT,CAAuB1N,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAAC,UAAtC,CAAR;CACf,QAAIgB,KAAK,GAAG,CAAZ,EAAeA,KAAK,GAAG,aAAaA,KAAb,GAAqB,CAA7B;CACf,SAAKhB,MAAL,IAAgBgB,KAAK,KAAK,EAA1B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAVD;;CAYA,WAAS2O,YAAT,CAAuB5L,GAAvB,EAA4B/B,KAA5B,EAAmChB,MAAnC,EAA2CsL,GAA3C,EAAgD5D,GAAhD,EAAqD9B,GAArD,EAA0D;CACxD,QAAI5F,MAAM,GAAGsL,GAAT,GAAevI,GAAG,CAAC1E,MAAvB,EAA+B,MAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;CAC/B,QAAI9C,MAAM,GAAG,CAAb,EAAgB,MAAM,IAAI8C,UAAJ,CAAe,oBAAf,CAAN;CACjB;;CAED,WAAS8L,UAAT,CAAqB7L,GAArB,EAA0B/B,KAA1B,EAAiChB,MAAjC,EAAyC6O,YAAzC,EAAuDpD,QAAvD,EAAiE;CAC/DzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;CACA,QAAI,CAACyL,QAAL,EAAe;CACbkD,MAAAA,YAAY,CAAC5L,GAAD,EAAM/B,KAAN,EAAahB,MAAb,EAAqB,CAArB,CAAZ;CACD;;CACD8M,IAAAA,OAAO,CAACnI,KAAR,CAAc5B,GAAd,EAAmB/B,KAAnB,EAA0BhB,MAA1B,EAAkC6O,YAAlC,EAAgD,EAAhD,EAAoD,CAApD;CACA,WAAO7O,MAAM,GAAG,CAAhB;CACD;;CAED2B,EAAAA,MAAM,CAACW,SAAP,CAAiBwM,YAAjB,GAAgC,SAASA,YAAT,CAAuB9N,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9E,WAAOmD,UAAU,CAAC,IAAD,EAAO5N,KAAP,EAAchB,MAAd,EAAsB,IAAtB,EAA4ByL,QAA5B,CAAjB;CACD,GAFD;;CAIA9J,EAAAA,MAAM,CAACW,SAAP,CAAiByM,YAAjB,GAAgC,SAASA,YAAT,CAAuB/N,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9E,WAAOmD,UAAU,CAAC,IAAD,EAAO5N,KAAP,EAAchB,MAAd,EAAsB,KAAtB,EAA6ByL,QAA7B,CAAjB;CACD,GAFD;;CAIA,WAASuD,WAAT,CAAsBjM,GAAtB,EAA2B/B,KAA3B,EAAkChB,MAAlC,EAA0C6O,YAA1C,EAAwDpD,QAAxD,EAAkE;CAChEzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;CACA,QAAI,CAACyL,QAAL,EAAe;CACbkD,MAAAA,YAAY,CAAC5L,GAAD,EAAM/B,KAAN,EAAahB,MAAb,EAAqB,CAArB,CAAZ;CACD;;CACD8M,IAAAA,OAAO,CAACnI,KAAR,CAAc5B,GAAd,EAAmB/B,KAAnB,EAA0BhB,MAA1B,EAAkC6O,YAAlC,EAAgD,EAAhD,EAAoD,CAApD;CACA,WAAO7O,MAAM,GAAG,CAAhB;CACD;;CAED2B,EAAAA,MAAM,CAACW,SAAP,CAAiB2M,aAAjB,GAAiC,SAASA,aAAT,CAAwBjO,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChF,WAAOuD,WAAW,CAAC,IAAD,EAAOhO,KAAP,EAAchB,MAAd,EAAsB,IAAtB,EAA4ByL,QAA5B,CAAlB;CACD,GAFD;;CAIA9J,EAAAA,MAAM,CAACW,SAAP,CAAiB4M,aAAjB,GAAiC,SAASA,aAAT,CAAwBlO,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChF,WAAOuD,WAAW,CAAC,IAAD,EAAOhO,KAAP,EAAchB,MAAd,EAAsB,KAAtB,EAA6ByL,QAA7B,CAAlB;CACD,GAFD;;;CAKA9J,EAAAA,MAAM,CAACW,SAAP,CAAiB0C,IAAjB,GAAwB,SAASA,IAAT,CAAe8C,MAAf,EAAuBqH,WAAvB,EAAoC7P,KAApC,EAA2CC,GAA3C,EAAgD;CACtE,QAAI,CAACoC,MAAM,CAACe,QAAP,CAAgBoF,MAAhB,CAAL,EAA8B,MAAM,IAAI5E,SAAJ,CAAc,6BAAd,CAAN;CAC9B,QAAI,CAAC5D,KAAL,EAAYA,KAAK,GAAG,CAAR;CACZ,QAAI,CAACC,GAAD,IAAQA,GAAG,KAAK,CAApB,EAAuBA,GAAG,GAAG,KAAKlB,MAAX;CACvB,QAAI8Q,WAAW,IAAIrH,MAAM,CAACzJ,MAA1B,EAAkC8Q,WAAW,GAAGrH,MAAM,CAACzJ,MAArB;CAClC,QAAI,CAAC8Q,WAAL,EAAkBA,WAAW,GAAG,CAAd;CAClB,QAAI5P,GAAG,GAAG,CAAN,IAAWA,GAAG,GAAGD,KAArB,EAA4BC,GAAG,GAAGD,KAAN,CAN0C;;CAStE,QAAIC,GAAG,KAAKD,KAAZ,EAAmB,OAAO,CAAP;CACnB,QAAIwI,MAAM,CAACzJ,MAAP,KAAkB,CAAlB,IAAuB,KAAKA,MAAL,KAAgB,CAA3C,EAA8C,OAAO,CAAP,CAVwB;;CAatE,QAAI8Q,WAAW,GAAG,CAAlB,EAAqB;CACnB,YAAM,IAAIrM,UAAJ,CAAe,2BAAf,CAAN;CACD;;CACD,QAAIxD,KAAK,GAAG,CAAR,IAAaA,KAAK,IAAI,KAAKjB,MAA/B,EAAuC,MAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;CACvC,QAAIvD,GAAG,GAAG,CAAV,EAAa,MAAM,IAAIuD,UAAJ,CAAe,yBAAf,CAAN,CAjByD;;CAoBtE,QAAIvD,GAAG,GAAG,KAAKlB,MAAf,EAAuBkB,GAAG,GAAG,KAAKlB,MAAX;;CACvB,QAAIyJ,MAAM,CAACzJ,MAAP,GAAgB8Q,WAAhB,GAA8B5P,GAAG,GAAGD,KAAxC,EAA+C;CAC7CC,MAAAA,GAAG,GAAGuI,MAAM,CAACzJ,MAAP,GAAgB8Q,WAAhB,GAA8B7P,KAApC;CACD;;CAED,QAAIlB,GAAG,GAAGmB,GAAG,GAAGD,KAAhB;;CAEA,QAAI,SAASwI,MAAT,IAAmB,OAAO9J,UAAU,CAACsE,SAAX,CAAqB8M,UAA5B,KAA2C,UAAlE,EAA8E;;CAE5E,WAAKA,UAAL,CAAgBD,WAAhB,EAA6B7P,KAA7B,EAAoCC,GAApC;CACD,KAHD,MAGO;CACLvB,MAAAA,UAAU,CAACsE,SAAX,CAAqB4D,GAArB,CAAyBC,IAAzB,CACE2B,MADF,EAEE,KAAKsD,QAAL,CAAc9L,KAAd,EAAqBC,GAArB,CAFF,EAGE4P,WAHF;CAKD;;CAED,WAAO/Q,GAAP;CACD,GAvCD;CA0CA;CACA;CACA;;;CACAuD,EAAAA,MAAM,CAACW,SAAP,CAAiB8B,IAAjB,GAAwB,SAASA,IAAT,CAAegE,GAAf,EAAoB9I,KAApB,EAA2BC,GAA3B,EAAgC8E,QAAhC,EAA0C;;CAEhE,QAAI,OAAO+D,GAAP,KAAe,QAAnB,EAA6B;CAC3B,UAAI,OAAO9I,KAAP,KAAiB,QAArB,EAA+B;CAC7B+E,QAAAA,QAAQ,GAAG/E,KAAX;CACAA,QAAAA,KAAK,GAAG,CAAR;CACAC,QAAAA,GAAG,GAAG,KAAKlB,MAAX;CACD,OAJD,MAIO,IAAI,OAAOkB,GAAP,KAAe,QAAnB,EAA6B;CAClC8E,QAAAA,QAAQ,GAAG9E,GAAX;CACAA,QAAAA,GAAG,GAAG,KAAKlB,MAAX;CACD;;CACD,UAAIgG,QAAQ,KAAK1B,SAAb,IAA0B,OAAO0B,QAAP,KAAoB,QAAlD,EAA4D;CAC1D,cAAM,IAAInB,SAAJ,CAAc,2BAAd,CAAN;CACD;;CACD,UAAI,OAAOmB,QAAP,KAAoB,QAApB,IAAgC,CAAC1C,MAAM,CAAC8C,UAAP,CAAkBJ,QAAlB,CAArC,EAAkE;CAChE,cAAM,IAAInB,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;CACD;;CACD,UAAI+D,GAAG,CAAC/J,MAAJ,KAAe,CAAnB,EAAsB;CACpB,YAAIH,IAAI,GAAGkK,GAAG,CAAC9J,UAAJ,CAAe,CAAf,CAAX;;CACA,YAAK+F,QAAQ,KAAK,MAAb,IAAuBnG,IAAI,GAAG,GAA/B,IACAmG,QAAQ,KAAK,QADjB,EAC2B;;CAEzB+D,UAAAA,GAAG,GAAGlK,IAAN;CACD;CACF;CACF,KAvBD,MAuBO,IAAI,OAAOkK,GAAP,KAAe,QAAnB,EAA6B;CAClCA,MAAAA,GAAG,GAAGA,GAAG,GAAG,GAAZ;CACD,KAFM,MAEA,IAAI,OAAOA,GAAP,KAAe,SAAnB,EAA8B;CACnCA,MAAAA,GAAG,GAAGc,MAAM,CAACd,GAAD,CAAZ;CACD,KA7B+D;;;CAgChE,QAAI9I,KAAK,GAAG,CAAR,IAAa,KAAKjB,MAAL,GAAciB,KAA3B,IAAoC,KAAKjB,MAAL,GAAckB,GAAtD,EAA2D;CACzD,YAAM,IAAIuD,UAAJ,CAAe,oBAAf,CAAN;CACD;;CAED,QAAIvD,GAAG,IAAID,KAAX,EAAkB;CAChB,aAAO,IAAP;CACD;;CAEDA,IAAAA,KAAK,GAAGA,KAAK,KAAK,CAAlB;CACAC,IAAAA,GAAG,GAAGA,GAAG,KAAKoD,SAAR,GAAoB,KAAKtE,MAAzB,GAAkCkB,GAAG,KAAK,CAAhD;CAEA,QAAI,CAAC6I,GAAL,EAAUA,GAAG,GAAG,CAAN;CAEV,QAAIjK,CAAJ;;CACA,QAAI,OAAOiK,GAAP,KAAe,QAAnB,EAA6B;CAC3B,WAAKjK,CAAC,GAAGmB,KAAT,EAAgBnB,CAAC,GAAGoB,GAApB,EAAyB,EAAEpB,CAA3B,EAA8B;CAC5B,aAAKA,CAAL,IAAUiK,GAAV;CACD;CACF,KAJD,MAIO;CACL,UAAI8C,KAAK,GAAGvJ,MAAM,CAACe,QAAP,CAAgB0F,GAAhB,IACRA,GADQ,GAERzG,MAAM,CAACyB,IAAP,CAAYgF,GAAZ,EAAiB/D,QAAjB,CAFJ;CAGA,UAAIjG,GAAG,GAAG8M,KAAK,CAAC7M,MAAhB;;CACA,UAAID,GAAG,KAAK,CAAZ,EAAe;CACb,cAAM,IAAI8E,SAAJ,CAAc,gBAAgBkF,GAAhB,GAClB,mCADI,CAAN;CAED;;CACD,WAAKjK,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAGoB,GAAG,GAAGD,KAAtB,EAA6B,EAAEnB,CAA/B,EAAkC;CAChC,aAAKA,CAAC,GAAGmB,KAAT,IAAkB4L,KAAK,CAAC/M,CAAC,GAAGC,GAAL,CAAvB;CACD;CACF;;CAED,WAAO,IAAP;CACD,GAjED;CAoEA;;;CAEA,MAAIiR,iBAAiB,GAAG,mBAAxB;;CAEA,WAASC,WAAT,CAAsB7H,GAAtB,EAA2B;;CAEzBA,IAAAA,GAAG,GAAGA,GAAG,CAAC8H,KAAJ,CAAU,GAAV,EAAe,CAAf,CAAN,CAFyB;;CAIzB9H,IAAAA,GAAG,GAAGA,GAAG,CAACI,IAAJ,GAAWD,OAAX,CAAmByH,iBAAnB,EAAsC,EAAtC,CAAN,CAJyB;;CAMzB,QAAI5H,GAAG,CAACpJ,MAAJ,GAAa,CAAjB,EAAoB,OAAO,EAAP,CANK;;CAQzB,WAAOoJ,GAAG,CAACpJ,MAAJ,GAAa,CAAb,KAAmB,CAA1B,EAA6B;CAC3BoJ,MAAAA,GAAG,GAAGA,GAAG,GAAG,GAAZ;CACD;;CACD,WAAOA,GAAP;CACD;;CAED,WAASlB,WAAT,CAAsB/B,MAAtB,EAA8BgL,KAA9B,EAAqC;CACnCA,IAAAA,KAAK,GAAGA,KAAK,IAAI3O,QAAjB;CACA,QAAIwJ,SAAJ;CACA,QAAIhM,MAAM,GAAGmG,MAAM,CAACnG,MAApB;CACA,QAAIoR,aAAa,GAAG,IAApB;CACA,QAAIvE,KAAK,GAAG,EAAZ;;CAEA,SAAK,IAAI/M,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4B,EAAEF,CAA9B,EAAiC;CAC/BkM,MAAAA,SAAS,GAAG7F,MAAM,CAAClG,UAAP,CAAkBH,CAAlB,CAAZ,CAD+B;;CAI/B,UAAIkM,SAAS,GAAG,MAAZ,IAAsBA,SAAS,GAAG,MAAtC,EAA8C;;CAE5C,YAAI,CAACoF,aAAL,EAAoB;;CAElB,cAAIpF,SAAS,GAAG,MAAhB,EAAwB;;CAEtB,gBAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;CACvB;CACD,WAJD,MAIO,IAAItB,CAAC,GAAG,CAAJ,KAAUE,MAAd,EAAsB;;CAE3B,gBAAI,CAACmR,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;CACvB;CACD,WAViB;;;CAalBgQ,UAAAA,aAAa,GAAGpF,SAAhB;CAEA;CACD,SAlB2C;;;CAqB5C,YAAIA,SAAS,GAAG,MAAhB,EAAwB;CACtB,cAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;CACvBgQ,UAAAA,aAAa,GAAGpF,SAAhB;CACA;CACD,SAzB2C;;;CA4B5CA,QAAAA,SAAS,GAAG,CAACoF,aAAa,GAAG,MAAhB,IAA0B,EAA1B,GAA+BpF,SAAS,GAAG,MAA5C,IAAsD,OAAlE;CACD,OA7BD,MA6BO,IAAIoF,aAAJ,EAAmB;;CAExB,YAAI,CAACD,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;CACxB;;CAEDgQ,MAAAA,aAAa,GAAG,IAAhB,CAtC+B;;CAyC/B,UAAIpF,SAAS,GAAG,IAAhB,EAAsB;CACpB,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;CACtBtE,QAAAA,KAAK,CAACzL,IAAN,CAAW4K,SAAX;CACD,OAHD,MAGO,IAAIA,SAAS,GAAG,KAAhB,EAAuB;CAC5B,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;CACtBtE,QAAAA,KAAK,CAACzL,IAAN,CACE4K,SAAS,IAAI,GAAb,GAAmB,IADrB,EAEEA,SAAS,GAAG,IAAZ,GAAmB,IAFrB;CAID,OANM,MAMA,IAAIA,SAAS,GAAG,OAAhB,EAAyB;CAC9B,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;CACtBtE,QAAAA,KAAK,CAACzL,IAAN,CACE4K,SAAS,IAAI,GAAb,GAAmB,IADrB,EAEEA,SAAS,IAAI,GAAb,GAAmB,IAAnB,GAA0B,IAF5B,EAGEA,SAAS,GAAG,IAAZ,GAAmB,IAHrB;CAKD,OAPM,MAOA,IAAIA,SAAS,GAAG,QAAhB,EAA0B;CAC/B,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;CACtBtE,QAAAA,KAAK,CAACzL,IAAN,CACE4K,SAAS,IAAI,IAAb,GAAoB,IADtB,EAEEA,SAAS,IAAI,GAAb,GAAmB,IAAnB,GAA0B,IAF5B,EAGEA,SAAS,IAAI,GAAb,GAAmB,IAAnB,GAA0B,IAH5B,EAIEA,SAAS,GAAG,IAAZ,GAAmB,IAJrB;CAMD,OARM,MAQA;CACL,cAAM,IAAI5L,KAAJ,CAAU,oBAAV,CAAN;CACD;CACF;;CAED,WAAOyM,KAAP;CACD;;CAED,WAASvB,YAAT,CAAuBlC,GAAvB,EAA4B;CAC1B,QAAIiI,SAAS,GAAG,EAAhB;;CACA,SAAK,IAAIvR,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGsJ,GAAG,CAACpJ,MAAxB,EAAgC,EAAEF,CAAlC,EAAqC;;CAEnCuR,MAAAA,SAAS,CAACjQ,IAAV,CAAegI,GAAG,CAACnJ,UAAJ,CAAeH,CAAf,IAAoB,IAAnC;CACD;;CACD,WAAOuR,SAAP;CACD;;CAED,WAAS5F,cAAT,CAAyBrC,GAAzB,EAA8B+H,KAA9B,EAAqC;CACnC,QAAIvO,CAAJ,EAAO0O,EAAP,EAAWC,EAAX;CACA,QAAIF,SAAS,GAAG,EAAhB;;CACA,SAAK,IAAIvR,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGsJ,GAAG,CAACpJ,MAAxB,EAAgC,EAAEF,CAAlC,EAAqC;CACnC,UAAI,CAACqR,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;CAEtBvO,MAAAA,CAAC,GAAGwG,GAAG,CAACnJ,UAAJ,CAAeH,CAAf,CAAJ;CACAwR,MAAAA,EAAE,GAAG1O,CAAC,IAAI,CAAV;CACA2O,MAAAA,EAAE,GAAG3O,CAAC,GAAG,GAAT;CACAyO,MAAAA,SAAS,CAACjQ,IAAV,CAAemQ,EAAf;CACAF,MAAAA,SAAS,CAACjQ,IAAV,CAAekQ,EAAf;CACD;;CAED,WAAOD,SAAP;CACD;;CAED,WAASlJ,aAAT,CAAwBiB,GAAxB,EAA6B;CAC3B,WAAOyC,QAAM,CAACvM,WAAP,CAAmB2R,WAAW,CAAC7H,GAAD,CAA9B,CAAP;CACD;;CAED,WAASgC,UAAT,CAAqBoG,GAArB,EAA0BC,GAA1B,EAA+B9P,MAA/B,EAAuC3B,MAAvC,EAA+C;CAC7C,SAAK,IAAIF,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4B,EAAEF,CAA9B,EAAiC;CAC/B,UAAKA,CAAC,GAAG6B,MAAJ,IAAc8P,GAAG,CAACzR,MAAnB,IAA+BF,CAAC,IAAI0R,GAAG,CAACxR,MAA5C,EAAqD;CACrDyR,MAAAA,GAAG,CAAC3R,CAAC,GAAG6B,MAAL,CAAH,GAAkB6P,GAAG,CAAC1R,CAAD,CAArB;CACD;;CACD,WAAOA,CAAP;CACD;CAGD;CACA;;;CACA,WAASuF,UAAT,CAAqBuB,GAArB,EAA0BE,IAA1B,EAAgC;CAC9B,WAAOF,GAAG,YAAYE,IAAf,IACJF,GAAG,IAAI,IAAP,IAAeA,GAAG,CAAC8K,WAAJ,IAAmB,IAAlC,IAA0C9K,GAAG,CAAC8K,WAAJ,CAAgBC,IAAhB,IAAwB,IAAlE,IACC/K,GAAG,CAAC8K,WAAJ,CAAgBC,IAAhB,KAAyB7K,IAAI,CAAC6K,IAFlC;CAGD;;CACD,WAAS9K,WAAT,CAAsBD,GAAtB,EAA2B;;CAEzB,WAAOA,GAAG,KAAKA,GAAf,CAFyB;CAG1B;CAGD;;;CACA,MAAIgG,mBAAmB,GAAI,YAAY;CACrC,QAAIgF,QAAQ,GAAG,kBAAf;CACA,QAAIC,KAAK,GAAG,IAAIjS,KAAJ,CAAU,GAAV,CAAZ;;CACA,SAAK,IAAIE,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAG,EAApB,EAAwB,EAAEA,CAA1B,EAA6B;CAC3B,UAAIgS,GAAG,GAAGhS,CAAC,GAAG,EAAd;;CACA,WAAK,IAAI4K,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAG,EAApB,EAAwB,EAAEA,CAA1B,EAA6B;CAC3BmH,QAAAA,KAAK,CAACC,GAAG,GAAGpH,CAAP,CAAL,GAAiBkH,QAAQ,CAAC9R,CAAD,CAAR,GAAc8R,QAAQ,CAAClH,CAAD,CAAvC;CACD;CACF;;CACD,WAAOmH,KAAP;CACD,GAVyB,EAA1B;;;;;;;AC9wDA,kBAAe,EAAf;;CCAA;;CAoKA,IAAIE,WAAW,GAAGC,MAAM,CAACD,WAAP,IAAsB,EAAxC;;CAEEA,WAAW,CAACE,GAAZ,IACAF,WAAW,CAACG,MADZ,IAEAH,WAAW,CAACI,KAFZ,IAGAJ,WAAW,CAACK,IAHZ,IAIAL,WAAW,CAACM,SAJZ,IAKA,YAAU;CAAE,SAAQ,IAAIC,IAAJ,EAAD,CAAaC,OAAb,EAAP;CAA+B;;CC1K7C,IAAIC,QAAJ;;CACA,IAAI,OAAOzO,MAAM,CAAC0O,MAAd,KAAyB,UAA7B,EAAwC;CACtCD,EAAAA,QAAQ,GAAG,SAASA,QAAT,CAAkBE,IAAlB,EAAwBC,SAAxB,EAAmC;CAC5C;CACAD,IAAAA,IAAI,CAACE,MAAL,GAAcD,SAAd;CACAD,IAAAA,IAAI,CAACzO,SAAL,GAAiBF,MAAM,CAAC0O,MAAP,CAAcE,SAAS,CAAC1O,SAAxB,EAAmC;CAClDyN,MAAAA,WAAW,EAAE;CACX/O,QAAAA,KAAK,EAAE+P,IADI;CAEXvO,QAAAA,UAAU,EAAE,KAFD;CAGX0O,QAAAA,QAAQ,EAAE,IAHC;CAIXC,QAAAA,YAAY,EAAE;CAJH;CADqC,KAAnC,CAAjB;CAQD,GAXD;CAYD,CAbD,MAaO;CACLN,EAAAA,QAAQ,GAAG,SAASA,QAAT,CAAkBE,IAAlB,EAAwBC,SAAxB,EAAmC;CAC5CD,IAAAA,IAAI,CAACE,MAAL,GAAcD,SAAd;;CACA,QAAII,QAAQ,GAAG,SAAXA,QAAW,GAAY,EAA3B;;CACAA,IAAAA,QAAQ,CAAC9O,SAAT,GAAqB0O,SAAS,CAAC1O,SAA/B;CACAyO,IAAAA,IAAI,CAACzO,SAAL,GAAiB,IAAI8O,QAAJ,EAAjB;CACAL,IAAAA,IAAI,CAACzO,SAAL,CAAeyN,WAAf,GAA6BgB,IAA7B;CACD,GAND;CAOD;;AACD,kBAAeF,QAAf;;CCxBA;CAqBA,IAAIQ,YAAY,GAAG,UAAnB;CACO,SAASC,MAAT,CAAgBC,CAAhB,EAAmB;CACxB,MAAI,CAACC,QAAQ,CAACD,CAAD,CAAb,EAAkB;CAChB,QAAIE,OAAO,GAAG,EAAd;;CACA,SAAK,IAAItT,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGkI,SAAS,CAAChI,MAA9B,EAAsCF,CAAC,EAAvC,EAA2C;CACzCsT,MAAAA,OAAO,CAAChS,IAAR,CAAa+H,OAAO,CAACnB,SAAS,CAAClI,CAAD,CAAV,CAApB;CACD;;CACD,WAAOsT,OAAO,CAAC/R,IAAR,CAAa,GAAb,CAAP;CACD;;CAED,MAAIvB,CAAC,GAAG,CAAR;CACA,MAAIuT,IAAI,GAAGrL,SAAX;CACA,MAAIjI,GAAG,GAAGsT,IAAI,CAACrT,MAAf;CACA,MAAIoJ,GAAG,GAAG5B,MAAM,CAAC0L,CAAD,CAAN,CAAU3J,OAAV,CAAkByJ,YAAlB,EAAgC,UAAS3L,CAAT,EAAY;CACpD,QAAIA,CAAC,KAAK,IAAV,EAAgB,OAAO,GAAP;CAChB,QAAIvH,CAAC,IAAIC,GAAT,EAAc,OAAOsH,CAAP;;CACd,YAAQA,CAAR;CACE,WAAK,IAAL;CAAW,eAAOG,MAAM,CAAC6L,IAAI,CAACvT,CAAC,EAAF,CAAL,CAAb;;CACX,WAAK,IAAL;CAAW,eAAO+K,MAAM,CAACwI,IAAI,CAACvT,CAAC,EAAF,CAAL,CAAb;;CACX,WAAK,IAAL;CACE,YAAI;CACF,iBAAOwT,IAAI,CAACC,SAAL,CAAeF,IAAI,CAACvT,CAAC,EAAF,CAAnB,CAAP;CACD,SAFD,CAEE,OAAO0T,CAAP,EAAU;CACV,iBAAO,YAAP;CACD;;CACH;CACE,eAAOnM,CAAP;CAVJ;CAYD,GAfS,CAAV;;CAgBA,OAAK,IAAIA,CAAC,GAAGgM,IAAI,CAACvT,CAAD,CAAjB,EAAsBA,CAAC,GAAGC,GAA1B,EAA+BsH,CAAC,GAAGgM,IAAI,CAAC,EAAEvT,CAAH,CAAvC,EAA8C;CAC5C,QAAI2T,MAAM,CAACpM,CAAD,CAAN,IAAa,CAACqM,QAAQ,CAACrM,CAAD,CAA1B,EAA+B;CAC7B+B,MAAAA,GAAG,IAAI,MAAM/B,CAAb;CACD,KAFD,MAEO;CACL+B,MAAAA,GAAG,IAAI,MAAMD,OAAO,CAAC9B,CAAD,CAApB;CACD;CACF;;CACD,SAAO+B,GAAP;CACD;CAID;CACA;;CACO,SAASuK,SAAT,CAAmBC,EAAnB,EAAuBC,GAAvB,EAA4B;CACjC;CACA,MAAIC,WAAW,CAAC9B,MAAM,CAAC+B,OAAR,CAAf,EAAiC;CAC/B,WAAO,YAAW;CAChB,aAAOJ,SAAS,CAACC,EAAD,EAAKC,GAAL,CAAT,CAAmB7K,KAAnB,CAAyB,IAAzB,EAA+BhB,SAA/B,CAAP;CACD,KAFD;CAGD;;CAMD,MAAIgM,MAAM,GAAG,KAAb;;CACA,WAASC,UAAT,GAAsB;CACpB,QAAI,CAACD,MAAL,EAAa;CACX,MAIO;CACLrQ,QAAAA,OAAO,CAACC,KAAR,CAAciQ,GAAd;CACD;;CACDG,MAAAA,MAAM,GAAG,IAAT;CACD;;CACD,WAAOJ,EAAE,CAAC5K,KAAH,CAAS,IAAT,EAAehB,SAAf,CAAP;CACD;;CAED,SAAOiM,UAAP;CACD;CAGD,IAAIC,MAAM,GAAG,EAAb;CACA,IAAIC,YAAJ;CACO,SAASC,QAAT,CAAkBvM,GAAlB,EAAuB;CAC5B,MAAIiM,WAAW,CAACK,YAAD,CAAf,EACEA,YAAY,GAA6B,EAAzC;CACFtM,EAAAA,GAAG,GAAGA,GAAG,CAACwM,WAAJ,EAAN;;CACA,MAAI,CAACH,MAAM,CAACrM,GAAD,CAAX,EAAkB;CAChB,QAAI,IAAIyM,MAAJ,CAAW,QAAQzM,GAAR,GAAc,KAAzB,EAAgC,GAAhC,EAAqC0M,IAArC,CAA0CJ,YAA1C,CAAJ,EAA6D;CAC3D,UAAIK,GAAG,GAAG,CAAV;;CACAN,MAAAA,MAAM,CAACrM,GAAD,CAAN,GAAc,YAAW;CACvB,YAAIgM,GAAG,GAAGZ,MAAM,CAACjK,KAAP,CAAa,IAAb,EAAmBhB,SAAnB,CAAV;CACArE,QAAAA,OAAO,CAACC,KAAR,CAAc,WAAd,EAA2BiE,GAA3B,EAAgC2M,GAAhC,EAAqCX,GAArC;CACD,OAHD;CAID,KAND,MAMO;CACLK,MAAAA,MAAM,CAACrM,GAAD,CAAN,GAAc,YAAW,EAAzB;CACD;CACF;;CACD,SAAOqM,MAAM,CAACrM,GAAD,CAAb;CACD;CAGD;CACA;CACA;CACA;CACA;CACA;CACA;;CACA;;CACO,SAASsB,OAAT,CAAiBvC,GAAjB,EAAsB6N,IAAtB,EAA4B;CACjC;CACA,MAAIC,GAAG,GAAG;CACRC,IAAAA,IAAI,EAAE,EADE;CAERC,IAAAA,OAAO,EAAEC;CAFD,GAAV,CAFiC;;CAOjC,MAAI7M,SAAS,CAAChI,MAAV,IAAoB,CAAxB,EAA2B0U,GAAG,CAACI,KAAJ,GAAY9M,SAAS,CAAC,CAAD,CAArB;CAC3B,MAAIA,SAAS,CAAChI,MAAV,IAAoB,CAAxB,EAA2B0U,GAAG,CAACK,MAAJ,GAAa/M,SAAS,CAAC,CAAD,CAAtB;;CAC3B,MAAIgN,SAAS,CAACP,IAAD,CAAb,EAAqB;CACnB;CACAC,IAAAA,GAAG,CAACO,UAAJ,GAAiBR,IAAjB;CACD,GAHD,MAGO,IAAIA,IAAJ,EAAU;CACf;CACAS,IAAAA,OAAO,CAACR,GAAD,EAAMD,IAAN,CAAP;CACD,GAfgC;;;CAiBjC,MAAIX,WAAW,CAACY,GAAG,CAACO,UAAL,CAAf,EAAiCP,GAAG,CAACO,UAAJ,GAAiB,KAAjB;CACjC,MAAInB,WAAW,CAACY,GAAG,CAACI,KAAL,CAAf,EAA4BJ,GAAG,CAACI,KAAJ,GAAY,CAAZ;CAC5B,MAAIhB,WAAW,CAACY,GAAG,CAACK,MAAL,CAAf,EAA6BL,GAAG,CAACK,MAAJ,GAAa,KAAb;CAC7B,MAAIjB,WAAW,CAACY,GAAG,CAACS,aAAL,CAAf,EAAoCT,GAAG,CAACS,aAAJ,GAAoB,IAApB;CACpC,MAAIT,GAAG,CAACK,MAAR,EAAgBL,GAAG,CAACE,OAAJ,GAAcQ,gBAAd;CAChB,SAAOC,WAAW,CAACX,GAAD,EAAM9N,GAAN,EAAW8N,GAAG,CAACI,KAAf,CAAlB;CACD;;CAGD3L,OAAO,CAAC4L,MAAR,GAAiB;CACf,UAAS,CAAC,CAAD,EAAI,EAAJ,CADM;CAEf,YAAW,CAAC,CAAD,EAAI,EAAJ,CAFI;CAGf,eAAc,CAAC,CAAD,EAAI,EAAJ,CAHC;CAIf,aAAY,CAAC,CAAD,EAAI,EAAJ,CAJG;CAKf,WAAU,CAAC,EAAD,EAAK,EAAL,CALK;CAMf,UAAS,CAAC,EAAD,EAAK,EAAL,CANM;CAOf,WAAU,CAAC,EAAD,EAAK,EAAL,CAPK;CAQf,UAAS,CAAC,EAAD,EAAK,EAAL,CARM;CASf,UAAS,CAAC,EAAD,EAAK,EAAL,CATM;CAUf,WAAU,CAAC,EAAD,EAAK,EAAL,CAVK;CAWf,aAAY,CAAC,EAAD,EAAK,EAAL,CAXG;CAYf,SAAQ,CAAC,EAAD,EAAK,EAAL,CAZO;CAaf,YAAW,CAAC,EAAD,EAAK,EAAL;CAbI,CAAjB;;CAiBA5L,OAAO,CAACmM,MAAR,GAAiB;CACf,aAAW,MADI;CAEf,YAAU,QAFK;CAGf,aAAW,QAHI;CAIf,eAAa,MAJE;CAKf,UAAQ,MALO;CAMf,YAAU,OANK;CAOf,UAAQ,SAPO;CAQf;CACA,YAAU;CATK,CAAjB;;CAaA,SAASF,gBAAT,CAA0BhM,GAA1B,EAA+BmM,SAA/B,EAA0C;CACxC,MAAIC,KAAK,GAAGrM,OAAO,CAACmM,MAAR,CAAeC,SAAf,CAAZ;;CAEA,MAAIC,KAAJ,EAAW;CACT,WAAO,UAAYrM,OAAO,CAAC4L,MAAR,CAAeS,KAAf,EAAsB,CAAtB,CAAZ,GAAuC,GAAvC,GAA6CpM,GAA7C,GACA,OADA,GACYD,OAAO,CAAC4L,MAAR,CAAeS,KAAf,EAAsB,CAAtB,CADZ,GACuC,GAD9C;CAED,GAHD,MAGO;CACL,WAAOpM,GAAP;CACD;CACF;;CAGD,SAASyL,cAAT,CAAwBzL,GAAxB,EAA6BmM,SAA7B,EAAwC;CACtC,SAAOnM,GAAP;CACD;;CAGD,SAASqM,WAAT,CAAqBhP,KAArB,EAA4B;CAC1B,MAAIiP,IAAI,GAAG,EAAX;CAEAjP,EAAAA,KAAK,CAACkP,OAAN,CAAc,UAAS5L,GAAT,EAAc6L,GAAd,EAAmB;CAC/BF,IAAAA,IAAI,CAAC3L,GAAD,CAAJ,GAAY,IAAZ;CACD,GAFD;CAIA,SAAO2L,IAAP;CACD;;CAGD,SAASL,WAAT,CAAqBX,GAArB,EAA0B/R,KAA1B,EAAiCkT,YAAjC,EAA+C;CAC7C;CACA;CACA,MAAInB,GAAG,CAACS,aAAJ,IACAxS,KADA,IAEAmT,UAAU,CAACnT,KAAK,CAACwG,OAAP,CAFV;CAIAxG,EAAAA,KAAK,CAACwG,OAAN,KAAkBA,OAJlB;CAMA,IAAExG,KAAK,CAAC+O,WAAN,IAAqB/O,KAAK,CAAC+O,WAAN,CAAkBzN,SAAlB,KAAgCtB,KAAvD,CANJ,EAMmE;CACjE,QAAI+J,GAAG,GAAG/J,KAAK,CAACwG,OAAN,CAAc0M,YAAd,EAA4BnB,GAA5B,CAAV;;CACA,QAAI,CAACvB,QAAQ,CAACzG,GAAD,CAAb,EAAoB;CAClBA,MAAAA,GAAG,GAAG2I,WAAW,CAACX,GAAD,EAAMhI,GAAN,EAAWmJ,YAAX,CAAjB;CACD;;CACD,WAAOnJ,GAAP;CACD,GAf4C;;;CAkB7C,MAAIqJ,SAAS,GAAGC,eAAe,CAACtB,GAAD,EAAM/R,KAAN,CAA/B;;CACA,MAAIoT,SAAJ,EAAe;CACb,WAAOA,SAAP;CACD,GArB4C;;;CAwB7C,MAAIE,IAAI,GAAGlS,MAAM,CAACkS,IAAP,CAAYtT,KAAZ,CAAX;CACA,MAAIuT,WAAW,GAAGT,WAAW,CAACQ,IAAD,CAA7B;;CAEA,MAAIvB,GAAG,CAACO,UAAR,EAAoB;CAClBgB,IAAAA,IAAI,GAAGlS,MAAM,CAACoS,mBAAP,CAA2BxT,KAA3B,CAAP;CACD,GA7B4C;CAgC7C;;;CACA,MAAIyT,OAAO,CAACzT,KAAD,CAAP,KACIsT,IAAI,CAAC3V,OAAL,CAAa,SAAb,KAA2B,CAA3B,IAAgC2V,IAAI,CAAC3V,OAAL,CAAa,aAAb,KAA+B,CADnE,CAAJ,EAC2E;CACzE,WAAO+V,WAAW,CAAC1T,KAAD,CAAlB;CACD,GApC4C;;;CAuC7C,MAAIsT,IAAI,CAACjW,MAAL,KAAgB,CAApB,EAAuB;CACrB,QAAI8V,UAAU,CAACnT,KAAD,CAAd,EAAuB;CACrB,UAAIgP,IAAI,GAAGhP,KAAK,CAACgP,IAAN,GAAa,OAAOhP,KAAK,CAACgP,IAA1B,GAAiC,EAA5C;CACA,aAAO+C,GAAG,CAACE,OAAJ,CAAY,cAAcjD,IAAd,GAAqB,GAAjC,EAAsC,SAAtC,CAAP;CACD;;CACD,QAAI2E,QAAQ,CAAC3T,KAAD,CAAZ,EAAqB;CACnB,aAAO+R,GAAG,CAACE,OAAJ,CAAYN,MAAM,CAACrQ,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BnF,KAA/B,CAAZ,EAAmD,QAAnD,CAAP;CACD;;CACD,QAAI4T,MAAM,CAAC5T,KAAD,CAAV,EAAmB;CACjB,aAAO+R,GAAG,CAACE,OAAJ,CAAYtC,IAAI,CAACrO,SAAL,CAAegD,QAAf,CAAwBa,IAAxB,CAA6BnF,KAA7B,CAAZ,EAAiD,MAAjD,CAAP;CACD;;CACD,QAAIyT,OAAO,CAACzT,KAAD,CAAX,EAAoB;CAClB,aAAO0T,WAAW,CAAC1T,KAAD,CAAlB;CACD;CACF;;CAED,MAAI6T,IAAI,GAAG,EAAX;CAAA,MAAe/P,KAAK,GAAG,KAAvB;CAAA,MAA8BgQ,MAAM,GAAG,CAAC,GAAD,EAAM,GAAN,CAAvC,CAvD6C;;CA0D7C,MAAI1P,OAAO,CAACpE,KAAD,CAAX,EAAoB;CAClB8D,IAAAA,KAAK,GAAG,IAAR;CACAgQ,IAAAA,MAAM,GAAG,CAAC,GAAD,EAAM,GAAN,CAAT;CACD,GA7D4C;;;CAgE7C,MAAIX,UAAU,CAACnT,KAAD,CAAd,EAAuB;CACrB,QAAIiG,CAAC,GAAGjG,KAAK,CAACgP,IAAN,GAAa,OAAOhP,KAAK,CAACgP,IAA1B,GAAiC,EAAzC;CACA6E,IAAAA,IAAI,GAAG,eAAe5N,CAAf,GAAmB,GAA1B;CACD,GAnE4C;;;CAsE7C,MAAI0N,QAAQ,CAAC3T,KAAD,CAAZ,EAAqB;CACnB6T,IAAAA,IAAI,GAAG,MAAMlC,MAAM,CAACrQ,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BnF,KAA/B,CAAb;CACD,GAxE4C;;;CA2E7C,MAAI4T,MAAM,CAAC5T,KAAD,CAAV,EAAmB;CACjB6T,IAAAA,IAAI,GAAG,MAAMlE,IAAI,CAACrO,SAAL,CAAeyS,WAAf,CAA2B5O,IAA3B,CAAgCnF,KAAhC,CAAb;CACD,GA7E4C;;;CAgF7C,MAAIyT,OAAO,CAACzT,KAAD,CAAX,EAAoB;CAClB6T,IAAAA,IAAI,GAAG,MAAMH,WAAW,CAAC1T,KAAD,CAAxB;CACD;;CAED,MAAIsT,IAAI,CAACjW,MAAL,KAAgB,CAAhB,KAAsB,CAACyG,KAAD,IAAU9D,KAAK,CAAC3C,MAAN,IAAgB,CAAhD,CAAJ,EAAwD;CACtD,WAAOyW,MAAM,CAAC,CAAD,CAAN,GAAYD,IAAZ,GAAmBC,MAAM,CAAC,CAAD,CAAhC;CACD;;CAED,MAAIZ,YAAY,GAAG,CAAnB,EAAsB;CACpB,QAAIS,QAAQ,CAAC3T,KAAD,CAAZ,EAAqB;CACnB,aAAO+R,GAAG,CAACE,OAAJ,CAAYN,MAAM,CAACrQ,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BnF,KAA/B,CAAZ,EAAmD,QAAnD,CAAP;CACD,KAFD,MAEO;CACL,aAAO+R,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAP;CACD;CACF;;CAEDF,EAAAA,GAAG,CAACC,IAAJ,CAASvT,IAAT,CAAcuB,KAAd;CAEA,MAAIxB,MAAJ;;CACA,MAAIsF,KAAJ,EAAW;CACTtF,IAAAA,MAAM,GAAGwV,WAAW,CAACjC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EAAwCD,IAAxC,CAApB;CACD,GAFD,MAEO;CACL9U,IAAAA,MAAM,GAAG8U,IAAI,CAACW,GAAL,CAAS,UAASC,GAAT,EAAc;CAC9B,aAAOC,cAAc,CAACpC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EAAwCW,GAAxC,EAA6CpQ,KAA7C,CAArB;CACD,KAFQ,CAAT;CAGD;;CAEDiO,EAAAA,GAAG,CAACC,IAAJ,CAASoC,GAAT;CAEA,SAAOC,oBAAoB,CAAC7V,MAAD,EAASqV,IAAT,EAAeC,MAAf,CAA3B;CACD;;CAGD,SAAST,eAAT,CAAyBtB,GAAzB,EAA8B/R,KAA9B,EAAqC;CACnC,MAAImR,WAAW,CAACnR,KAAD,CAAf,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,WAAZ,EAAyB,WAAzB,CAAP;;CACF,MAAIzB,QAAQ,CAACxQ,KAAD,CAAZ,EAAqB;CACnB,QAAIsU,MAAM,GAAG,OAAO3D,IAAI,CAACC,SAAL,CAAe5Q,KAAf,EAAsB4G,OAAtB,CAA8B,QAA9B,EAAwC,EAAxC,EACsBA,OADtB,CAC8B,IAD9B,EACoC,KADpC,EAEsBA,OAFtB,CAE8B,MAF9B,EAEsC,GAFtC,CAAP,GAEoD,IAFjE;CAGA,WAAOmL,GAAG,CAACE,OAAJ,CAAYqC,MAAZ,EAAoB,QAApB,CAAP;CACD;;CACD,MAAIC,QAAQ,CAACvU,KAAD,CAAZ,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,KAAKjS,KAAjB,EAAwB,QAAxB,CAAP;CACF,MAAIqS,SAAS,CAACrS,KAAD,CAAb,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,KAAKjS,KAAjB,EAAwB,SAAxB,CAAP,CAZiC;;CAcnC,MAAI8Q,MAAM,CAAC9Q,KAAD,CAAV,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,MAAZ,EAAoB,MAApB,CAAP;CACH;;CAGD,SAASyB,WAAT,CAAqB1T,KAArB,EAA4B;CAC1B,SAAO,MAAMvC,KAAK,CAAC6D,SAAN,CAAgBgD,QAAhB,CAAyBa,IAAzB,CAA8BnF,KAA9B,CAAN,GAA6C,GAApD;CACD;;CAGD,SAASgU,WAAT,CAAqBjC,GAArB,EAA0B/R,KAA1B,EAAiCkT,YAAjC,EAA+CK,WAA/C,EAA4DD,IAA5D,EAAkE;CAChE,MAAI9U,MAAM,GAAG,EAAb;;CACA,OAAK,IAAIrB,CAAC,GAAG,CAAR,EAAWqX,CAAC,GAAGxU,KAAK,CAAC3C,MAA1B,EAAkCF,CAAC,GAAGqX,CAAtC,EAAyC,EAAErX,CAA3C,EAA8C;CAC5C,QAAIsX,cAAc,CAACzU,KAAD,EAAQ6E,MAAM,CAAC1H,CAAD,CAAd,CAAlB,EAAsC;CACpCqB,MAAAA,MAAM,CAACC,IAAP,CAAY0V,cAAc,CAACpC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EACtB1O,MAAM,CAAC1H,CAAD,CADgB,EACX,IADW,CAA1B;CAED,KAHD,MAGO;CACLqB,MAAAA,MAAM,CAACC,IAAP,CAAY,EAAZ;CACD;CACF;;CACD6U,EAAAA,IAAI,CAACN,OAAL,CAAa,UAASkB,GAAT,EAAc;CACzB,QAAI,CAACA,GAAG,CAACQ,KAAJ,CAAU,OAAV,CAAL,EAAyB;CACvBlW,MAAAA,MAAM,CAACC,IAAP,CAAY0V,cAAc,CAACpC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EACtBW,GADsB,EACjB,IADiB,CAA1B;CAED;CACF,GALD;CAMA,SAAO1V,MAAP;CACD;;CAGD,SAAS2V,cAAT,CAAwBpC,GAAxB,EAA6B/R,KAA7B,EAAoCkT,YAApC,EAAkDK,WAAlD,EAA+DW,GAA/D,EAAoEpQ,KAApE,EAA2E;CACzE,MAAIkL,IAAJ,EAAUvI,GAAV,EAAekO,IAAf;CACAA,EAAAA,IAAI,GAAGvT,MAAM,CAACwT,wBAAP,CAAgC5U,KAAhC,EAAuCkU,GAAvC,KAA+C;CAAElU,IAAAA,KAAK,EAAEA,KAAK,CAACkU,GAAD;CAAd,GAAtD;;CACA,MAAIS,IAAI,CAAClT,GAAT,EAAc;CACZ,QAAIkT,IAAI,CAACzP,GAAT,EAAc;CACZuB,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,iBAAZ,EAA+B,SAA/B,CAAN;CACD,KAFD,MAEO;CACLxL,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAN;CACD;CACF,GAND,MAMO;CACL,QAAI0C,IAAI,CAACzP,GAAT,EAAc;CACZuB,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAN;CACD;CACF;;CACD,MAAI,CAACwC,cAAc,CAAClB,WAAD,EAAcW,GAAd,CAAnB,EAAuC;CACrClF,IAAAA,IAAI,GAAG,MAAMkF,GAAN,GAAY,GAAnB;CACD;;CACD,MAAI,CAACzN,GAAL,EAAU;CACR,QAAIsL,GAAG,CAACC,IAAJ,CAASrU,OAAT,CAAiBgX,IAAI,CAAC3U,KAAtB,IAA+B,CAAnC,EAAsC;CACpC,UAAI8Q,MAAM,CAACoC,YAAD,CAAV,EAA0B;CACxBzM,QAAAA,GAAG,GAAGiM,WAAW,CAACX,GAAD,EAAM4C,IAAI,CAAC3U,KAAX,EAAkB,IAAlB,CAAjB;CACD,OAFD,MAEO;CACLyG,QAAAA,GAAG,GAAGiM,WAAW,CAACX,GAAD,EAAM4C,IAAI,CAAC3U,KAAX,EAAkBkT,YAAY,GAAG,CAAjC,CAAjB;CACD;;CACD,UAAIzM,GAAG,CAAC9I,OAAJ,CAAY,IAAZ,IAAoB,CAAC,CAAzB,EAA4B;CAC1B,YAAImG,KAAJ,EAAW;CACT2C,UAAAA,GAAG,GAAGA,GAAG,CAAC8H,KAAJ,CAAU,IAAV,EAAgB0F,GAAhB,CAAoB,UAASY,IAAT,EAAe;CACvC,mBAAO,OAAOA,IAAd;CACD,WAFK,EAEHnW,IAFG,CAEE,IAFF,EAEQ6J,MAFR,CAEe,CAFf,CAAN;CAGD,SAJD,MAIO;CACL9B,UAAAA,GAAG,GAAG,OAAOA,GAAG,CAAC8H,KAAJ,CAAU,IAAV,EAAgB0F,GAAhB,CAAoB,UAASY,IAAT,EAAe;CAC9C,mBAAO,QAAQA,IAAf;CACD,WAFY,EAEVnW,IAFU,CAEL,IAFK,CAAb;CAGD;CACF;CACF,KAjBD,MAiBO;CACL+H,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,YAAZ,EAA0B,SAA1B,CAAN;CACD;CACF;;CACD,MAAId,WAAW,CAACnC,IAAD,CAAf,EAAuB;CACrB,QAAIlL,KAAK,IAAIoQ,GAAG,CAACQ,KAAJ,CAAU,OAAV,CAAb,EAAiC;CAC/B,aAAOjO,GAAP;CACD;;CACDuI,IAAAA,IAAI,GAAG2B,IAAI,CAACC,SAAL,CAAe,KAAKsD,GAApB,CAAP;;CACA,QAAIlF,IAAI,CAAC0F,KAAL,CAAW,8BAAX,CAAJ,EAAgD;CAC9C1F,MAAAA,IAAI,GAAGA,IAAI,CAACzG,MAAL,CAAY,CAAZ,EAAeyG,IAAI,CAAC3R,MAAL,GAAc,CAA7B,CAAP;CACA2R,MAAAA,IAAI,GAAG+C,GAAG,CAACE,OAAJ,CAAYjD,IAAZ,EAAkB,MAAlB,CAAP;CACD,KAHD,MAGO;CACLA,MAAAA,IAAI,GAAGA,IAAI,CAACpI,OAAL,CAAa,IAAb,EAAmB,KAAnB,EACKA,OADL,CACa,MADb,EACqB,GADrB,EAEKA,OAFL,CAEa,UAFb,EAEyB,GAFzB,CAAP;CAGAoI,MAAAA,IAAI,GAAG+C,GAAG,CAACE,OAAJ,CAAYjD,IAAZ,EAAkB,QAAlB,CAAP;CACD;CACF;;CAED,SAAOA,IAAI,GAAG,IAAP,GAAcvI,GAArB;CACD;;CAGD,SAAS4N,oBAAT,CAA8B7V,MAA9B,EAAsCqV,IAAtC,EAA4CC,MAA5C,EAAoD;CAElD,MAAIzW,MAAM,GAAGmB,MAAM,CAACsW,MAAP,CAAc,UAASC,IAAT,EAAeC,GAAf,EAAoB;CAE7C,QAAIA,GAAG,CAACrX,OAAJ,CAAY,IAAZ,KAAqB,CAAzB,EAA4BsX;CAC5B,WAAOF,IAAI,GAAGC,GAAG,CAACpO,OAAJ,CAAY,iBAAZ,EAA+B,EAA/B,EAAmCvJ,MAA1C,GAAmD,CAA1D;CACD,GAJY,EAIV,CAJU,CAAb;;CAMA,MAAIA,MAAM,GAAG,EAAb,EAAiB;CACf,WAAOyW,MAAM,CAAC,CAAD,CAAN,IACCD,IAAI,KAAK,EAAT,GAAc,EAAd,GAAmBA,IAAI,GAAG,KAD3B,IAEA,GAFA,GAGArV,MAAM,CAACE,IAAP,CAAY,OAAZ,CAHA,GAIA,GAJA,GAKAoV,MAAM,CAAC,CAAD,CALb;CAMD;;CAED,SAAOA,MAAM,CAAC,CAAD,CAAN,GAAYD,IAAZ,GAAmB,GAAnB,GAAyBrV,MAAM,CAACE,IAAP,CAAY,IAAZ,CAAzB,GAA6C,GAA7C,GAAmDoV,MAAM,CAAC,CAAD,CAAhE;CACD;CAID;;;CACO,SAAS1P,OAAT,CAAiB8Q,EAAjB,EAAqB;CAC1B,SAAOjY,KAAK,CAACmH,OAAN,CAAc8Q,EAAd,CAAP;CACD;CAEM,SAAS7C,SAAT,CAAmBrQ,GAAnB,EAAwB;CAC7B,SAAO,OAAOA,GAAP,KAAe,SAAtB;CACD;CAEM,SAAS8O,MAAT,CAAgB9O,GAAhB,EAAqB;CAC1B,SAAOA,GAAG,KAAK,IAAf;CACD;CAEM,SAASmT,iBAAT,CAA2BnT,GAA3B,EAAgC;CACrC,SAAOA,GAAG,IAAI,IAAd;CACD;CAEM,SAASuS,QAAT,CAAkBvS,GAAlB,EAAuB;CAC5B,SAAO,OAAOA,GAAP,KAAe,QAAtB;CACD;CAEM,SAASwO,QAAT,CAAkBxO,GAAlB,EAAuB;CAC5B,SAAO,OAAOA,GAAP,KAAe,QAAtB;CACD;CAEM,SAASoT,QAAT,CAAkBpT,GAAlB,EAAuB;CAC5B,SAAO,uBAAOA,GAAP,MAAe,QAAtB;CACD;CAEM,SAASmP,WAAT,CAAqBnP,GAArB,EAA0B;CAC/B,SAAOA,GAAG,KAAK,KAAK,CAApB;CACD;CAEM,SAAS2R,QAAT,CAAkB0B,EAAlB,EAAsB;CAC3B,SAAOtE,QAAQ,CAACsE,EAAD,CAAR,IAAgBC,cAAc,CAACD,EAAD,CAAd,KAAuB,iBAA9C;CACD;CAEM,SAAStE,QAAT,CAAkB/O,GAAlB,EAAuB;CAC5B,SAAO,uBAAOA,GAAP,MAAe,QAAf,IAA2BA,GAAG,KAAK,IAA1C;CACD;CAEM,SAAS4R,MAAT,CAAgBlU,CAAhB,EAAmB;CACxB,SAAOqR,QAAQ,CAACrR,CAAD,CAAR,IAAe4V,cAAc,CAAC5V,CAAD,CAAd,KAAsB,eAA5C;CACD;CAEM,SAAS+T,OAAT,CAAiBrU,CAAjB,EAAoB;CACzB,SAAO2R,QAAQ,CAAC3R,CAAD,CAAR,KACFkW,cAAc,CAAClW,CAAD,CAAd,KAAsB,gBAAtB,IAA0CA,CAAC,YAAY3B,KADrD,CAAP;CAED;CAEM,SAAS0V,UAAT,CAAoBnR,GAApB,EAAyB;CAC9B,SAAO,OAAOA,GAAP,KAAe,UAAtB;CACD;CAEM,SAASuT,WAAT,CAAqBvT,GAArB,EAA0B;CAC/B,SAAOA,GAAG,KAAK,IAAR,IACA,OAAOA,GAAP,KAAe,SADf,IAEA,OAAOA,GAAP,KAAe,QAFf,IAGA,OAAOA,GAAP,KAAe,QAHf,IAIA,uBAAOA,GAAP,MAAe,QAJf;CAKA,SAAOA,GAAP,KAAe,WALtB;CAMD;CAEM,SAASN,QAAT,CAAkB8T,QAAlB,EAA4B;CACjC,SAAO7U,MAAM,CAACe,QAAP,CAAgB8T,QAAhB,CAAP;CACD;;CAED,SAASF,cAAT,CAAwBG,CAAxB,EAA2B;CACzB,SAAOrU,MAAM,CAACE,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BsQ,CAA/B,CAAP;CACD;;CAGD,SAASC,GAAT,CAAazP,CAAb,EAAgB;CACd,SAAOA,CAAC,GAAG,EAAJ,GAAS,MAAMA,CAAC,CAAC3B,QAAF,CAAW,EAAX,CAAf,GAAgC2B,CAAC,CAAC3B,QAAF,CAAW,EAAX,CAAvC;CACD;;CAGD,IAAIqR,MAAM,GAAG,CAAC,KAAD,EAAQ,KAAR,EAAe,KAAf,EAAsB,KAAtB,EAA6B,KAA7B,EAAoC,KAApC,EAA2C,KAA3C,EAAkD,KAAlD,EAAyD,KAAzD,EACC,KADD,EACQ,KADR,EACe,KADf,CAAb;;CAIA,SAASC,WAAT,GAAqB;CACnB,MAAIlW,CAAC,GAAG,IAAIiQ,IAAJ,EAAR;CACA,MAAIkG,IAAI,GAAG,CAACH,GAAG,CAAChW,CAAC,CAACoW,QAAF,EAAD,CAAJ,EACCJ,GAAG,CAAChW,CAAC,CAACqW,UAAF,EAAD,CADJ,EAECL,GAAG,CAAChW,CAAC,CAACsW,UAAF,EAAD,CAFJ,EAEsBtX,IAFtB,CAE2B,GAF3B,CAAX;CAGA,SAAO,CAACgB,CAAC,CAACuW,OAAF,EAAD,EAAcN,MAAM,CAACjW,CAAC,CAACwW,QAAF,EAAD,CAApB,EAAoCL,IAApC,EAA0CnX,IAA1C,CAA+C,GAA/C,CAAP;CACD;;;CAIM,SAAS4B,GAAT,GAAe;CACpBU,EAAAA,OAAO,CAACV,GAAR,CAAY,SAAZ,EAAuBsV,WAAS,EAAhC,EAAoCtF,MAAM,CAACjK,KAAP,CAAa,IAAb,EAAmBhB,SAAnB,CAApC;CACD;CAmBM,SAASkN,OAAT,CAAiB4D,MAAjB,EAAyBC,GAAzB,EAA8B;CACnC;CACA,MAAI,CAACA,GAAD,IAAQ,CAACrF,QAAQ,CAACqF,GAAD,CAArB,EAA4B,OAAOD,MAAP;CAE5B,MAAI7C,IAAI,GAAGlS,MAAM,CAACkS,IAAP,CAAY8C,GAAZ,CAAX;CACA,MAAIjZ,CAAC,GAAGmW,IAAI,CAACjW,MAAb;;CACA,SAAOF,CAAC,EAAR,EAAY;CACVgZ,IAAAA,MAAM,CAAC7C,IAAI,CAACnW,CAAD,CAAL,CAAN,GAAkBiZ,GAAG,CAAC9C,IAAI,CAACnW,CAAD,CAAL,CAArB;CACD;;CACD,SAAOgZ,MAAP;CACD;;CAED,SAAS1B,cAAT,CAAwBxQ,GAAxB,EAA6BoS,IAA7B,EAAmC;CACjC,SAAOjV,MAAM,CAACE,SAAP,CAAiBmT,cAAjB,CAAgCtP,IAAhC,CAAqClB,GAArC,EAA0CoS,IAA1C,CAAP;CACD;;AAED,kBAAe;CACbxG,EAAAA,QAAQ,EAAEA,UADG;CAEb0C,EAAAA,OAAO,EAAEA,OAFI;CAGbjS,EAAAA,GAAG,EAAEA,GAHQ;CAIboB,EAAAA,QAAQ,EAAEA,QAJG;CAKb6T,EAAAA,WAAW,EAAEA,WALA;CAMbpC,EAAAA,UAAU,EAAEA,UANC;CAObM,EAAAA,OAAO,EAAEA,OAPI;CAQbG,EAAAA,MAAM,EAAEA,MARK;CASb7C,EAAAA,QAAQ,EAAEA,QATG;CAUb4C,EAAAA,QAAQ,EAAEA,QAVG;CAWbxC,EAAAA,WAAW,EAAEA,WAXA;CAYbiE,EAAAA,QAAQ,EAAEA,QAZG;CAab5E,EAAAA,QAAQ,EAAEA,QAbG;CAcb+D,EAAAA,QAAQ,EAAEA,QAdG;CAebY,EAAAA,iBAAiB,EAAEA,iBAfN;CAgBbrE,EAAAA,MAAM,EAAEA,MAhBK;CAiBbuB,EAAAA,SAAS,EAAEA,SAjBE;CAkBbjO,EAAAA,OAAO,EAAEA,OAlBI;CAmBboC,EAAAA,OAAO,EAAEA,OAnBI;CAoBbwK,EAAAA,SAAS,EAAEA,SApBE;CAqBbV,EAAAA,MAAM,EAAEA,MArBK;CAsBbmB,EAAAA,QAAQ,EAAEA;CAtBG,CAAf;;;;;AC9jBgC;CAIhC;;;;CAIA,SAAgB,wBAAwB,CAAC,EAAY;KACnD,OAAO,EAAE,CAAC,QAAQ,EAAE,CAAC,OAAO,CAAC,WAAW,EAAE,YAAY,CAAC,CAAC;CAC1D,CAAC;CAFD,4DAEC;CAED,IAAM,aAAa,GACjB,OAAOpC,cAAM,CAAC,SAAS,KAAK,QAAQ,IAAIA,cAAM,CAAC,SAAS,CAAC,OAAO,KAAK,aAAa,CAAC;CAErF,IAAM,eAAe,GAAG,aAAa;OACjC,0IAA0I;OAC1I,+GAA+G,CAAC;CAEpH,IAAM,mBAAmB,GAAwB,SAAS,mBAAmB,CAAC,IAAY;KACxF,OAAO,CAAC,IAAI,CAAC,eAAe,CAAC,CAAC;KAE9B,IAAM,MAAM,GAAGiH,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;KAClC,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,EAAE,EAAE,CAAC;SAAE,MAAM,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,MAAM,EAAE,GAAG,GAAG,CAAC,CAAC;KAC3E,OAAO,MAAM,CAAC;CAChB,CAAC,CAAC;CAUF,IAAM,iBAAiB,GAAG;KACxB,IAAI,OAAO,MAAM,KAAK,WAAW,EAAE;;SAEjC,IAAM,QAAM,GAAG,MAAM,CAAC,MAAM,IAAI,MAAM,CAAC,QAAQ,CAAC;SAChD,IAAI,QAAM,IAAI,QAAM,CAAC,eAAe,EAAE;aACpC,OAAO,UAAA,IAAI,IAAI,OAAA,QAAM,CAAC,eAAe,CAACA,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,GAAA,CAAC;UAC3D;MACF;KAED,IAAI,OAAOjH,cAAM,KAAK,WAAW,IAAIA,cAAM,CAAC,MAAM,IAAIA,cAAM,CAAC,MAAM,CAAC,eAAe,EAAE;;SAEnF,OAAO,UAAA,IAAI,IAAI,OAAAA,cAAM,CAAC,MAAM,CAAC,eAAe,CAACiH,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,GAAA,CAAC;MAClE;KAED,IAAI,mBAA2D,CAAC;KAChE,IAAI;;SAEF,mBAAmB,GAAG,UAAiB,CAAC,WAAW,CAAC;MACrD;KAAC,OAAO,CAAC,EAAE;;MAEX;;KAID,OAAO,mBAAmB,IAAI,mBAAmB,CAAC;CACpD,CAAC,CAAC;CAEW,mBAAW,GAAG,iBAAiB,EAAE,CAAC;CAE/C,SAAgB,gBAAgB,CAAC,KAAc;KAC7C,OAAO,CAAC,sBAAsB,EAAE,4BAA4B,CAAC,CAAC,QAAQ,CACpE,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CACtC,CAAC;CACJ,CAAC;CAJD,4CAIC;CAED,SAAgB,YAAY,CAAC,KAAc;KACzC,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,qBAAqB,CAAC;CACzE,CAAC;CAFD,oCAEC;CAED,SAAgB,eAAe,CAAC,KAAc;KAC5C,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,wBAAwB,CAAC;CAC5E,CAAC;CAFD,0CAEC;CAED,SAAgB,gBAAgB,CAAC,KAAc;KAC7C,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,yBAAyB,CAAC;CAC7E,CAAC;CAFD,4CAEC;CAED,SAAgB,QAAQ,CAAC,CAAU;KACjC,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,iBAAiB,CAAC;CACjE,CAAC;CAFD,4BAEC;CAED,SAAgB,KAAK,CAAC,CAAU;KAC9B,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,cAAc,CAAC;CAC9D,CAAC;CAFD,sBAEC;CAED;CACA,SAAgB,UAAU;KACxB,OAAO,OAAOjH,cAAM,KAAK,WAAW,IAAI,OAAOA,cAAM,CAAC,MAAM,KAAK,WAAW,CAAC;CAC/E,CAAC;CAFD,gCAEC;CAED;CACA,SAAgB,MAAM,CAAC,CAAU;KAC/B,OAAO,YAAY,CAAC,CAAC,CAAC,IAAI,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,eAAe,CAAC;CAClF,CAAC;CAFD,wBAEC;CAED;;;;;CAKA,SAAgB,YAAY,CAAC,SAAkB;KAC7C,OAAO,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,KAAK,IAAI,CAAC;CAC7D,CAAC;CAFD,oCAEC;CAGD,SAAgB,SAAS,CAAqB,EAAK,EAAE,OAAe;KAClE,IAAI,OAAO,MAAM,KAAK,WAAW,IAAI,OAAO,IAAI,KAAK,WAAW,EAAE;;SAEhE,OAAO,UAAe,CAAC,SAAS,CAAC,EAAE,EAAE,OAAO,CAAC,CAAC;MAC/C;KACD,IAAI,MAAM,GAAG,KAAK,CAAC;KACnB,SAAS,UAAU;SAAgB,cAAkB;cAAlB,UAAkB,EAAlB,qBAAkB,EAAlB,IAAkB;aAAlB,yBAAkB;;SACnD,IAAI,CAAC,MAAM,EAAE;aACX,OAAO,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC;aACtB,MAAM,GAAG,IAAI,CAAC;UACf;SACD,OAAO,EAAE,CAAC,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;MAC7B;KACD,OAAQ,UAA2B,CAAC;CACtC,CAAC;CAdD,8BAcC;;;;;;;;;;;;;;;;;;;;;AC5H+B;AACkB;CAElD;;;;;;;;CAQA,SAAgB,YAAY,CAAC,eAAuD;KAClF,IAAI,WAAW,CAAC,MAAM,CAAC,eAAe,CAAC,EAAE;SACvC,OAAOiH,aAAM,CAAC,IAAI,CAChB,eAAe,CAAC,MAAM,EACtB,eAAe,CAAC,UAAU,EAC1B,eAAe,CAAC,UAAU,CAC3B,CAAC;MACH;KAED,IAAIC,sBAAgB,CAAC,eAAe,CAAC,EAAE;SACrC,OAAOD,aAAM,CAAC,IAAI,CAAC,eAAe,CAAC,CAAC;MACrC;KAED,MAAM,IAAI,SAAS,CAAC,sCAAsC,CAAC,CAAC;CAC9D,CAAC;CAdD,oCAcC;;;;;;;;;;ACzB+B;CAEhC;CACA,IAAM,gBAAgB,GAAG,uHAAuH,CAAC;CAE1I,IAAM,kBAAkB,GAAG,UAAC,GAAW;KAC5C,OAAA,OAAO,GAAG,KAAK,QAAQ,IAAI,gBAAgB,CAAC,IAAI,CAAC,GAAG,CAAC;CAArD,CAAqD,CAAC;CAD3C,0BAAkB,sBACyB;CAEjD,IAAM,qBAAqB,GAAG,UAAC,SAAiB;KACrD,IAAI,CAAC,0BAAkB,CAAC,SAAS,CAAC,EAAE;SAClC,MAAM,IAAI,SAAS,CACjB,uLAAuL,CACxL,CAAC;MACH;KAED,IAAM,kBAAkB,GAAG,SAAS,CAAC,OAAO,CAAC,IAAI,EAAE,EAAE,CAAC,CAAC;KACvD,OAAOA,aAAM,CAAC,IAAI,CAAC,kBAAkB,EAAE,KAAK,CAAC,CAAC;CAChD,CAAC,CAAC;CATW,6BAAqB,yBAShC;CAEK,IAAM,qBAAqB,GAAG,UAAC,MAAc,EAAE,aAAoB;KAApB,8BAAA,EAAA,oBAAoB;KACxE,OAAA,aAAa;WACT,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;aAC5B,GAAG;aACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;aAC5B,GAAG;aACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;aAC5B,GAAG;aACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,EAAE,CAAC;aAC7B,GAAG;aACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,EAAE,EAAE,EAAE,CAAC;WAC9B,MAAM,CAAC,QAAQ,CAAC,KAAK,CAAC;CAV1B,CAU0B,CAAC;CAXhB,6BAAqB,yBAWL;;;;;;;;;;;;AC9BG;AACe;AACb;AAC8D;AACrC;CAO3D,IAAM,WAAW,GAAG,EAAE,CAAC;CAEvB,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;CAEzB;;;;CAIA;;;;;;KAgBE,cAAY,KAA8B;SACxC,IAAI,OAAO,KAAK,KAAK,WAAW,EAAE;;aAEhC,IAAI,CAAC,EAAE,GAAG,IAAI,CAAC,QAAQ,EAAE,CAAC;UAC3B;cAAM,IAAI,KAAK,YAAY,IAAI,EAAE;aAChC,IAAI,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;aAClC,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,CAAC;UACxB;cAAM,IAAI,WAAW,CAAC,MAAM,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,UAAU,KAAK,WAAW,EAAE;aACxE,IAAI,CAAC,EAAE,GAAGE,0BAAY,CAAC,KAAK,CAAC,CAAC;UAC/B;cAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;aACpC,IAAI,CAAC,EAAE,GAAGC,gCAAqB,CAAC,KAAK,CAAC,CAAC;UACxC;cAAM;aACL,MAAM,IAAI,SAAS,CACjB,gLAAgL,CACjL,CAAC;UACH;MACF;KAMD,sBAAI,oBAAE;;;;;cAAN;aACE,OAAO,IAAI,CAAC,GAAG,CAAC,CAAC;UAClB;cAED,UAAO,KAAa;aAClB,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;aAElB,IAAI,IAAI,CAAC,cAAc,EAAE;iBACvB,IAAI,CAAC,IAAI,GAAGA,gCAAqB,CAAC,KAAK,CAAC,CAAC;cAC1C;UACF;;;QARA;;;;;;;;KAkBD,0BAAW,GAAX,UAAY,aAAoB;SAApB,8BAAA,EAAA,oBAAoB;SAC9B,IAAI,IAAI,CAAC,cAAc,IAAI,IAAI,CAAC,IAAI,EAAE;aACpC,OAAO,IAAI,CAAC,IAAI,CAAC;UAClB;SAED,IAAM,aAAa,GAAGA,gCAAqB,CAAC,IAAI,CAAC,EAAE,EAAE,aAAa,CAAC,CAAC;SAEpE,IAAI,IAAI,CAAC,cAAc,EAAE;aACvB,IAAI,CAAC,IAAI,GAAG,aAAa,CAAC;UAC3B;SAED,OAAO,aAAa,CAAC;MACtB;;;;;KAMD,uBAAQ,GAAR,UAAS,QAAiB;SACxB,OAAO,QAAQ,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,QAAQ,CAAC,GAAG,IAAI,CAAC,WAAW,EAAE,CAAC;MACnE;;;;;KAMD,qBAAM,GAAN;SACE,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;MAC3B;;;;;;KAOD,qBAAM,GAAN,UAAO,OAA+B;SACpC,IAAI,CAAC,OAAO,EAAE;aACZ,OAAO,KAAK,CAAC;UACd;SAED,IAAI,OAAO,YAAY,IAAI,EAAE;aAC3B,OAAO,OAAO,CAAC,EAAE,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;UACnC;SAED,IAAI;aACF,OAAO,IAAI,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;UAC7C;SAAC,WAAM;aACN,OAAO,KAAK,CAAC;UACd;MACF;;;;KAKD,uBAAQ,GAAR;SACE,OAAO,IAAIC,aAAM,CAAC,IAAI,CAAC,EAAE,EAAEA,aAAM,CAAC,YAAY,CAAC,CAAC;MACjD;;;;KAKM,aAAQ,GAAf;SACE,IAAM,KAAK,GAAGH,iBAAW,CAAC,WAAW,CAAC,CAAC;;;SAIvC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,IAAI,IAAI,CAAC;SACpC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,IAAI,IAAI,CAAC;SAEpC,OAAOD,aAAM,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;MAC3B;;;;;KAMM,YAAO,GAAd,UAAe,KAA6B;SAC1C,IAAI,CAAC,KAAK,EAAE;aACV,OAAO,KAAK,CAAC;UACd;SAED,IAAI,KAAK,YAAY,IAAI,EAAE;aACzB,OAAO,IAAI,CAAC;UACb;SAED,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;aAC7B,OAAOG,6BAAkB,CAAC,KAAK,CAAC,CAAC;UAClC;SAED,IAAIF,kBAAY,CAAC,KAAK,CAAC,EAAE;;aAEvB,IAAI,KAAK,CAAC,MAAM,KAAK,WAAW,EAAE;iBAChC,OAAO,KAAK,CAAC;cACd;aAED,IAAI;;;iBAGF,OAAO,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,QAAQ,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,KAAKG,aAAM,CAAC,YAAY,CAAC;cACvE;aAAC,WAAM;iBACN,OAAO,KAAK,CAAC;cACd;UACF;SAED,OAAO,KAAK,CAAC;MACd;;;;;KAMM,wBAAmB,GAA1B,UAA2B,SAAiB;SAC1C,IAAM,MAAM,GAAGD,gCAAqB,CAAC,SAAS,CAAC,CAAC;SAChD,OAAO,IAAI,IAAI,CAAC,MAAM,CAAC,CAAC;MACzB;;;;;;;KAQD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,sBAAO,GAAP;SACE,OAAO,gBAAa,IAAI,CAAC,WAAW,EAAE,QAAI,CAAC;MAC5C;KACH,WAAC;CAAD,CAAC,IAAA;CA3LY,oBAAI;CA6LjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;AChNtC;AACe;AACM;AACT;CAoB5C;;;;CAIA;;;;;KAkCE,gBAAY1X,QAAgC,EAAE,OAAgB;SAC5D,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;aAAE,OAAO,IAAI,MAAM,CAACA,QAAM,EAAE,OAAO,CAAC,CAAC;SAElE,IACE,EAAEA,QAAM,IAAI,IAAI,CAAC;aACjB,EAAE,OAAOA,QAAM,KAAK,QAAQ,CAAC;aAC7B,CAAC,WAAW,CAAC,MAAM,CAACA,QAAM,CAAC;aAC3B,EAAEA,QAAM,YAAY,WAAW,CAAC;aAChC,CAAC,KAAK,CAAC,OAAO,CAACA,QAAM,CAAC,EACtB;aACA,MAAM,IAAI,SAAS,CACjB,kFAAkF,CACnF,CAAC;UACH;SAED,IAAI,CAAC,QAAQ,GAAG,OAAO,aAAP,OAAO,cAAP,OAAO,GAAI,MAAM,CAAC,2BAA2B,CAAC;SAE9D,IAAIA,QAAM,IAAI,IAAI,EAAE;;aAElB,IAAI,CAAC,MAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,MAAM,CAAC,WAAW,CAAC,CAAC;aAC/C,IAAI,CAAC,QAAQ,GAAG,CAAC,CAAC;UACnB;cAAM;aACL,IAAI,OAAOvX,QAAM,KAAK,QAAQ,EAAE;;iBAE9B,IAAI,CAAC,MAAM,GAAGuX,aAAM,CAAC,IAAI,CAACvX,QAAM,EAAE,QAAQ,CAAC,CAAC;cAC7C;kBAAM,IAAI,KAAK,CAAC,OAAO,CAACA,QAAM,CAAC,EAAE;;iBAEhC,IAAI,CAAC,MAAM,GAAGuX,aAAM,CAAC,IAAI,CAACvX,QAAM,CAAC,CAAC;cACnC;kBAAM;;iBAEL,IAAI,CAAC,MAAM,GAAGyX,0BAAY,CAACzX,QAAM,CAAC,CAAC;cACpC;aAED,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;UACxC;MACF;;;;;;KAOD,oBAAG,GAAH,UAAI,SAA2D;;SAE7D,IAAI,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,CAAC,MAAM,KAAK,CAAC,EAAE;aAC3D,MAAM,IAAI,SAAS,CAAC,sCAAsC,CAAC,CAAC;UAC7D;cAAM,IAAI,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,CAAC,MAAM,KAAK,CAAC;aAChE,MAAM,IAAI,SAAS,CAAC,mDAAmD,CAAC,CAAC;;SAG3E,IAAI,WAAmB,CAAC;SACxB,IAAI,OAAO,SAAS,KAAK,QAAQ,EAAE;aACjC,WAAW,GAAG,SAAS,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;UACvC;cAAM,IAAI,OAAO,SAAS,KAAK,QAAQ,EAAE;aACxC,WAAW,GAAG,SAAS,CAAC;UACzB;cAAM;aACL,WAAW,GAAG,SAAS,CAAC,CAAC,CAAC,CAAC;UAC5B;SAED,IAAI,WAAW,GAAG,CAAC,IAAI,WAAW,GAAG,GAAG,EAAE;aACxC,MAAM,IAAI,SAAS,CAAC,0DAA0D,CAAC,CAAC;UACjF;SAED,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,EAAE;aACtC,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,GAAG,WAAW,CAAC;UAC5C;cAAM;aACL,IAAMA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,MAAM,CAAC,WAAW,GAAG,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;;aAErE,IAAI,CAAC,MAAM,CAAC,IAAI,CAACvX,QAAM,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;aACnD,IAAI,CAAC,MAAM,GAAGA,QAAM,CAAC;aACrB,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,GAAG,WAAW,CAAC;UAC5C;MACF;;;;;;;KAQD,sBAAK,GAAL,UAAM,QAAiC,EAAE,MAAc;SACrD,MAAM,GAAG,OAAO,MAAM,KAAK,QAAQ,GAAG,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;;SAG7D,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,EAAE;aACjD,IAAMA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,QAAQ,CAAC,MAAM,CAAC,CAAC;aAClE,IAAI,CAAC,MAAM,CAAC,IAAI,CAACvX,QAAM,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;;aAGnD,IAAI,CAAC,MAAM,GAAGA,QAAM,CAAC;UACtB;SAED,IAAI,WAAW,CAAC,MAAM,CAAC,QAAQ,CAAC,EAAE;aAChC,IAAI,CAAC,MAAM,CAAC,GAAG,CAACyX,0BAAY,CAAC,QAAQ,CAAC,EAAE,MAAM,CAAC,CAAC;aAChD,IAAI,CAAC,QAAQ;iBACX,MAAM,GAAG,QAAQ,CAAC,UAAU,GAAG,IAAI,CAAC,QAAQ,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;UAC3F;cAAM,IAAI,OAAO,QAAQ,KAAK,QAAQ,EAAE;aACvC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,QAAQ,EAAE,MAAM,EAAE,QAAQ,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;aAC/D,IAAI,CAAC,QAAQ;iBACX,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;UACvF;MACF;;;;;;;KAQD,qBAAI,GAAJ,UAAK,QAAgB,EAAE,MAAc;SACnC,MAAM,GAAG,MAAM,IAAI,MAAM,GAAG,CAAC,GAAG,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;;SAGvD,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,QAAQ,EAAE,QAAQ,GAAG,MAAM,CAAC,CAAC;MACvD;;;;;;;KAQD,sBAAK,GAAL,UAAM,KAAe;SACnB,KAAK,GAAG,CAAC,CAAC,KAAK,CAAC;;SAGhB,IAAI,KAAK,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,KAAK,IAAI,CAAC,QAAQ,EAAE;aACjD,OAAO,IAAI,CAAC,MAAM,CAAC;UACpB;;SAGD,IAAI,KAAK,EAAE;aACT,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;UAC5C;SACD,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MACzD;;KAGD,uBAAM,GAAN;SACE,OAAO,IAAI,CAAC,QAAQ,CAAC;MACtB;;KAGD,uBAAM,GAAN;SACE,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;MACvC;;KAGD,yBAAQ,GAAR,UAAS,MAAc;SACrB,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC;MACrC;;KAGD,+BAAc,GAAd,UAAe,OAAsB;SACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,IAAM,YAAY,GAAG,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;SAEpD,IAAM,OAAO,GAAG,MAAM,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,QAAQ,CAAC,EAAE,CAAC,CAAC;SACnD,IAAI,OAAO,CAAC,MAAM,EAAE;aAClB,OAAO;iBACL,OAAO,EAAE,YAAY;iBACrB,KAAK,EAAE,OAAO,CAAC,MAAM,KAAK,CAAC,GAAG,GAAG,GAAG,OAAO,GAAG,OAAO;cACtD,CAAC;UACH;SACD,OAAO;aACL,OAAO,EAAE;iBACP,MAAM,EAAE,YAAY;iBACpB,OAAO,EAAE,OAAO,CAAC,MAAM,KAAK,CAAC,GAAG,GAAG,GAAG,OAAO,GAAG,OAAO;cACxD;UACF,CAAC;MACH;;KAGD,uBAAM,GAAN;SACE,IAAI,IAAI,CAAC,QAAQ,KAAK,MAAM,CAAC,YAAY,EAAE;aACzC,OAAO,IAAIG,SAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC;UACtD;SAED,MAAM,IAAI,KAAK,CACb,uBAAoB,IAAI,CAAC,QAAQ,2DAAoD,MAAM,CAAC,YAAY,+BAA2B,CACpI,CAAC;MACH;;KAGM,uBAAgB,GAAvB,UACE,GAAyD,EACzD,OAAsB;SAEtB,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,IAAI,IAAwB,CAAC;SAC7B,IAAI,IAAI,CAAC;SACT,IAAI,SAAS,IAAI,GAAG,EAAE;aACpB,IAAI,OAAO,CAAC,MAAM,IAAI,OAAO,GAAG,CAAC,OAAO,KAAK,QAAQ,IAAI,OAAO,IAAI,GAAG,EAAE;iBACvE,IAAI,GAAG,GAAG,CAAC,KAAK,GAAG,QAAQ,CAAC,GAAG,CAAC,KAAK,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;iBAC/C,IAAI,GAAGL,aAAM,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;cAC3C;kBAAM;iBACL,IAAI,OAAO,GAAG,CAAC,OAAO,KAAK,QAAQ,EAAE;qBACnC,IAAI,GAAG,GAAG,CAAC,OAAO,CAAC,OAAO,GAAG,QAAQ,CAAC,GAAG,CAAC,OAAO,CAAC,OAAO,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;qBACnE,IAAI,GAAGA,aAAM,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;kBAClD;cACF;UACF;cAAM,IAAI,OAAO,IAAI,GAAG,EAAE;aACzB,IAAI,GAAG,CAAC,CAAC;aACT,IAAI,GAAGG,gCAAqB,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC;UACzC;SACD,IAAI,CAAC,IAAI,EAAE;aACT,MAAM,IAAI,SAAS,CAAC,4CAA0C,IAAI,CAAC,SAAS,CAAC,GAAG,CAAG,CAAC,CAAC;UACtF;SACD,OAAO,IAAI,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;MAC/B;;KAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,wBAAO,GAAP;SACE,IAAM,QAAQ,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;SAClC,OAAO,8BAA2B,QAAQ,CAAC,QAAQ,CAAC,KAAK,CAAC,sBAAc,IAAI,CAAC,QAAQ,MAAG,CAAC;MAC1F;;;;;KAtPuB,kCAA2B,GAAG,CAAC,CAAC;;KAGxC,kBAAW,GAAG,GAAG,CAAC;;KAElB,sBAAe,GAAG,CAAC,CAAC;;KAEpB,uBAAgB,GAAG,CAAC,CAAC;;KAErB,yBAAkB,GAAG,CAAC,CAAC;;KAEvB,uBAAgB,GAAG,CAAC,CAAC;;KAErB,mBAAY,GAAG,CAAC,CAAC;;KAEjB,kBAAW,GAAG,CAAC,CAAC;;KAEhB,2BAAoB,GAAG,GAAG,CAAC;KAsO7C,aAAC;EA9PD,IA8PC;CA9PY,wBAAM;CAgQnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;CCnR1E;;;;CAIA;;;;;KASE,cAAY,IAAuB,EAAE,KAAgB;SACnD,IAAI,EAAE,IAAI,YAAY,IAAI,CAAC;aAAE,OAAO,IAAI,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;SAE1D,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC;SACjB,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;MACpB;;KAGD,qBAAM,GAAN;SACE,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,IAAI,EAAE,KAAK,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;MAC/C;;KAGD,6BAAc,GAAd;SACE,IAAI,IAAI,CAAC,KAAK,EAAE;aACd,OAAO,EAAE,KAAK,EAAE,IAAI,CAAC,IAAI,EAAE,MAAM,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;UACjD;SAED,OAAO,EAAE,KAAK,EAAE,IAAI,CAAC,IAAI,EAAE,CAAC;MAC7B;;KAGM,qBAAgB,GAAvB,UAAwB,GAAiB;SACvC,OAAO,IAAI,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,MAAM,CAAC,CAAC;MACxC;;KAGD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,sBAAO,GAAP;SACE,IAAM,QAAQ,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC;SAC/B,OAAO,gBAAa,QAAQ,CAAC,IAAI,WAC/B,QAAQ,CAAC,KAAK,GAAG,OAAK,IAAI,CAAC,SAAS,CAAC,QAAQ,CAAC,KAAK,CAAG,GAAG,EAAE,OAC1D,CAAC;MACL;KACH,WAAC;CAAD,CAAC,IAAA;CA9CY,oBAAI;CAgDjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;ACzDxB;CAS9C;CACA,SAAgB,WAAW,CAAC,KAAc;KACxC,QACEF,kBAAY,CAAC,KAAK,CAAC;SACnB,KAAK,CAAC,GAAG,IAAI,IAAI;SACjB,OAAO,KAAK,CAAC,IAAI,KAAK,QAAQ;UAC7B,KAAK,CAAC,GAAG,IAAI,IAAI,IAAI,OAAO,KAAK,CAAC,GAAG,KAAK,QAAQ,CAAC,EACpD;CACJ,CAAC;CAPD,kCAOC;CAED;;;;CAIA;;;;;;KAaE,eAAY,UAAkB,EAAE,GAAa,EAAE,EAAW,EAAE,MAAiB;SAC3E,IAAI,EAAE,IAAI,YAAY,KAAK,CAAC;aAAE,OAAO,IAAI,KAAK,CAAC,UAAU,EAAE,GAAG,EAAE,EAAE,EAAE,MAAM,CAAC,CAAC;;SAG5E,IAAM,KAAK,GAAG,UAAU,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;SACpC,IAAI,KAAK,CAAC,MAAM,KAAK,CAAC,EAAE;aACtB,EAAE,GAAG,KAAK,CAAC,KAAK,EAAE,CAAC;;aAEnB,UAAU,GAAG,KAAK,CAAC,KAAK,EAAG,CAAC;UAC7B;SAED,IAAI,CAAC,UAAU,GAAG,UAAU,CAAC;SAC7B,IAAI,CAAC,GAAG,GAAG,GAAG,CAAC;SACf,IAAI,CAAC,EAAE,GAAG,EAAE,CAAC;SACb,IAAI,CAAC,MAAM,GAAG,MAAM,IAAI,EAAE,CAAC;MAC5B;KAMD,sBAAI,4BAAS;;;;cAAb;aACE,OAAO,IAAI,CAAC,UAAU,CAAC;UACxB;cAED,UAAc,KAAa;aACzB,IAAI,CAAC,UAAU,GAAG,KAAK,CAAC;UACzB;;;QAJA;;KAOD,sBAAM,GAAN;SACE,IAAM,CAAC,GAAG,MAAM,CAAC,MAAM,CACrB;aACE,IAAI,EAAE,IAAI,CAAC,UAAU;aACrB,GAAG,EAAE,IAAI,CAAC,GAAG;UACd,EACD,IAAI,CAAC,MAAM,CACZ,CAAC;SAEF,IAAI,IAAI,CAAC,EAAE,IAAI,IAAI;aAAE,CAAC,CAAC,GAAG,GAAG,IAAI,CAAC,EAAE,CAAC;SACrC,OAAO,CAAC,CAAC;MACV;;KAGD,8BAAc,GAAd,UAAe,OAAsB;SACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,IAAI,CAAC,GAAc;aACjB,IAAI,EAAE,IAAI,CAAC,UAAU;aACrB,GAAG,EAAE,IAAI,CAAC,GAAG;UACd,CAAC;SAEF,IAAI,OAAO,CAAC,MAAM,EAAE;aAClB,OAAO,CAAC,CAAC;UACV;SAED,IAAI,IAAI,CAAC,EAAE;aAAE,CAAC,CAAC,GAAG,GAAG,IAAI,CAAC,EAAE,CAAC;SAC7B,CAAC,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,CAAC;SAClC,OAAO,CAAC,CAAC;MACV;;KAGM,sBAAgB,GAAvB,UAAwB,GAAc;SACpC,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,GAAG,CAAuB,CAAC;SAC1D,OAAO,IAAI,CAAC,IAAI,CAAC;SACjB,OAAO,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO,IAAI,KAAK,CAAC,GAAG,CAAC,IAAI,EAAE,GAAG,CAAC,GAAG,EAAE,GAAG,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;MACpD;;KAGD,gBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,uBAAO,GAAP;;SAEE,IAAM,GAAG,GACP,IAAI,CAAC,GAAG,KAAK,SAAS,IAAI,IAAI,CAAC,GAAG,CAAC,QAAQ,KAAK,SAAS,GAAG,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,QAAQ,EAAE,CAAC;SAC7F,OAAO,iBAAc,IAAI,CAAC,SAAS,2BAAoB,GAAG,YACxD,IAAI,CAAC,EAAE,GAAG,SAAM,IAAI,CAAC,EAAE,OAAG,GAAG,EAAE,OAC9B,CAAC;MACL;KACH,YAAC;CAAD,CAAC,IAAA;CA/FY,sBAAK;CAiGlB,MAAM,CAAC,cAAc,CAAC,KAAK,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,OAAO,EAAE,CAAC,CAAC;;;;;;;;;;;AC1H1B;CAkB9C;;;CAGA,IAAI,IAAI,GAAgC,SAAS,CAAC;CAMlD,IAAI;KACF,IAAI,GAAI,IAAI,WAAW,CAAC,QAAQ,CAC9B,IAAI,WAAW,CAAC,MAAM;;KAEpB,IAAI,UAAU,CAAC,CAAC,CAAC,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,CAAC,CAAC,CAC/oC,EACD,EAAE,CACH,CAAC,OAAsC,CAAC;EAC1C;CAAC,WAAM;;EAEP;CAED,IAAM,cAAc,GAAG,CAAC,IAAI,EAAE,CAAC;CAC/B,IAAM,cAAc,GAAG,CAAC,IAAI,EAAE,CAAC;CAC/B,IAAM,cAAc,GAAG,cAAc,GAAG,cAAc,CAAC;CACvD,IAAM,cAAc,GAAG,cAAc,GAAG,cAAc,CAAC;CACvD,IAAM,cAAc,GAAG,cAAc,GAAG,CAAC,CAAC;CAE1C;CACA,IAAM,SAAS,GAA4B,EAAE,CAAC;CAE9C;CACA,IAAM,UAAU,GAA4B,EAAE,CAAC;CAO/C;;;;;;;;;;;;;;;;;;CAkBA;;;;;;;;;;;;;;KAkCE,cAAY,GAAiC,EAAE,IAAuB,EAAE,QAAkB;SAA9E,oBAAA,EAAA,OAAiC;SAC3C,IAAI,EAAE,IAAI,YAAY,IAAI,CAAC;aAAE,OAAO,IAAI,IAAI,CAAC,GAAG,EAAE,IAAI,EAAE,QAAQ,CAAC,CAAC;SAElE,IAAI,OAAO,GAAG,KAAK,QAAQ,EAAE;aAC3B,MAAM,CAAC,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;UACnD;cAAM,IAAI,OAAO,GAAG,KAAK,QAAQ,EAAE;aAClC,MAAM,CAAC,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;UACnD;cAAM;aACL,IAAI,CAAC,GAAG,GAAG,GAAG,GAAG,CAAC,CAAC;aACnB,IAAI,CAAC,IAAI,GAAI,IAAe,GAAG,CAAC,CAAC;aACjC,IAAI,CAAC,QAAQ,GAAG,CAAC,CAAC,QAAQ,CAAC;UAC5B;SAED,MAAM,CAAC,cAAc,CAAC,IAAI,EAAE,YAAY,EAAE;aACxC,KAAK,EAAE,IAAI;aACX,YAAY,EAAE,KAAK;aACnB,QAAQ,EAAE,KAAK;aACf,UAAU,EAAE,KAAK;UAClB,CAAC,CAAC;MACJ;;;;;;;;;KA6BM,aAAQ,GAAf,UAAgB,OAAe,EAAE,QAAgB,EAAE,QAAkB;SACnE,OAAO,IAAI,IAAI,CAAC,OAAO,EAAE,QAAQ,EAAE,QAAQ,CAAC,CAAC;MAC9C;;;;;;;KAQM,YAAO,GAAd,UAAe,KAAa,EAAE,QAAkB;SAC9C,IAAI,GAAG,EAAE,SAAS,EAAE,KAAK,CAAC;SAC1B,IAAI,QAAQ,EAAE;aACZ,KAAK,MAAM,CAAC,CAAC;aACb,KAAK,KAAK,GAAG,CAAC,IAAI,KAAK,IAAI,KAAK,GAAG,GAAG,GAAG;iBACvC,SAAS,GAAG,UAAU,CAAC,KAAK,CAAC,CAAC;iBAC9B,IAAI,SAAS;qBAAE,OAAO,SAAS,CAAC;cACjC;aACD,GAAG,GAAG,IAAI,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,KAAK,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,EAAE,IAAI,CAAC,CAAC;aAC3D,IAAI,KAAK;iBAAE,UAAU,CAAC,KAAK,CAAC,GAAG,GAAG,CAAC;aACnC,OAAO,GAAG,CAAC;UACZ;cAAM;aACL,KAAK,IAAI,CAAC,CAAC;aACX,KAAK,KAAK,GAAG,CAAC,GAAG,IAAI,KAAK,IAAI,KAAK,GAAG,GAAG,GAAG;iBAC1C,SAAS,GAAG,SAAS,CAAC,KAAK,CAAC,CAAC;iBAC7B,IAAI,SAAS;qBAAE,OAAO,SAAS,CAAC;cACjC;aACD,GAAG,GAAG,IAAI,CAAC,QAAQ,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;aACtD,IAAI,KAAK;iBAAE,SAAS,CAAC,KAAK,CAAC,GAAG,GAAG,CAAC;aAClC,OAAO,GAAG,CAAC;UACZ;MACF;;;;;;;KAQM,eAAU,GAAjB,UAAkB,KAAa,EAAE,QAAkB;SACjD,IAAI,KAAK,CAAC,KAAK,CAAC;aAAE,OAAO,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;SAC3D,IAAI,QAAQ,EAAE;aACZ,IAAI,KAAK,GAAG,CAAC;iBAAE,OAAO,IAAI,CAAC,KAAK,CAAC;aACjC,IAAI,KAAK,IAAI,cAAc;iBAAE,OAAO,IAAI,CAAC,kBAAkB,CAAC;UAC7D;cAAM;aACL,IAAI,KAAK,IAAI,CAAC,cAAc;iBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;aACpD,IAAI,KAAK,GAAG,CAAC,IAAI,cAAc;iBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;UACxD;SACD,IAAI,KAAK,GAAG,CAAC;aAAE,OAAO,IAAI,CAAC,UAAU,CAAC,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC,GAAG,EAAE,CAAC;SAC9D,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,GAAG,cAAc,GAAG,CAAC,EAAE,CAAC,KAAK,GAAG,cAAc,IAAI,CAAC,EAAE,QAAQ,CAAC,CAAC;MAC1F;;;;;;;KAQM,eAAU,GAAjB,UAAkB,KAAa,EAAE,QAAkB;SACjD,OAAO,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,QAAQ,EAAE,EAAE,QAAQ,CAAC,CAAC;MACpD;;;;;;;;KASM,eAAU,GAAjB,UAAkB,GAAW,EAAE,QAAkB,EAAE,KAAc;SAC/D,IAAI,GAAG,CAAC,MAAM,KAAK,CAAC;aAAE,MAAM,KAAK,CAAC,cAAc,CAAC,CAAC;SAClD,IAAI,GAAG,KAAK,KAAK,IAAI,GAAG,KAAK,UAAU,IAAI,GAAG,KAAK,WAAW,IAAI,GAAG,KAAK,WAAW;aACnF,OAAO,IAAI,CAAC,IAAI,CAAC;SACnB,IAAI,OAAO,QAAQ,KAAK,QAAQ,EAAE;;aAEhC,CAAC,KAAK,GAAG,QAAQ,IAAI,QAAQ,GAAG,KAAK,CAAC,CAAC;UACxC;cAAM;aACL,QAAQ,GAAG,CAAC,CAAC,QAAQ,CAAC;UACvB;SACD,KAAK,GAAG,KAAK,IAAI,EAAE,CAAC;SACpB,IAAI,KAAK,GAAG,CAAC,IAAI,EAAE,GAAG,KAAK;aAAE,MAAM,UAAU,CAAC,OAAO,CAAC,CAAC;SAEvD,IAAI,CAAC,CAAC;SACN,IAAI,CAAC,CAAC,GAAG,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,CAAC;aAAE,MAAM,KAAK,CAAC,iBAAiB,CAAC,CAAC;cAC1D,IAAI,CAAC,KAAK,CAAC,EAAE;aAChB,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,CAAC,EAAE,QAAQ,EAAE,KAAK,CAAC,CAAC,GAAG,EAAE,CAAC;UACjE;;;SAID,IAAM,YAAY,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC,CAAC;SAEzD,IAAI,MAAM,GAAG,IAAI,CAAC,IAAI,CAAC;SACvB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,GAAG,CAAC,MAAM,EAAE,CAAC,IAAI,CAAC,EAAE;aACtC,IAAM,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,GAAG,CAAC,MAAM,GAAG,CAAC,CAAC,EACtC,KAAK,GAAG,QAAQ,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,EAAE,KAAK,CAAC,CAAC;aACtD,IAAI,IAAI,GAAG,CAAC,EAAE;iBACZ,IAAM,KAAK,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;iBACrD,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,CAAC;cACxD;kBAAM;iBACL,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC;iBAClC,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,CAAC;cAC7C;UACF;SACD,MAAM,CAAC,QAAQ,GAAG,QAAQ,CAAC;SAC3B,OAAO,MAAM,CAAC;MACf;;;;;;;;KASM,cAAS,GAAhB,UAAiB,KAAe,EAAE,QAAkB,EAAE,EAAY;SAChE,OAAO,EAAE,GAAG,IAAI,CAAC,WAAW,CAAC,KAAK,EAAE,QAAQ,CAAC,GAAG,IAAI,CAAC,WAAW,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC;MACnF;;;;;;;KAQM,gBAAW,GAAlB,UAAmB,KAAe,EAAE,QAAkB;SACpD,OAAO,IAAI,IAAI,CACb,KAAK,CAAC,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,EAChE,KAAK,CAAC,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,EAChE,QAAQ,CACT,CAAC;MACH;;;;;;;KAQM,gBAAW,GAAlB,UAAmB,KAAe,EAAE,QAAkB;SACpD,OAAO,IAAI,IAAI,CACb,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,KAAK,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,EAChE,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,KAAK,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,EAChE,QAAQ,CACT,CAAC;MACH;;;;;KAMM,WAAM,GAAb,UAAc,KAAU;SACtB,OAAOA,kBAAY,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,YAAY,CAAC,KAAK,IAAI,CAAC;MAC5D;;;;;KAMM,cAAS,GAAhB,UACE,GAAwE,EACxE,QAAkB;SAElB,IAAI,OAAO,GAAG,KAAK,QAAQ;aAAE,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;SACnE,IAAI,OAAO,GAAG,KAAK,QAAQ;aAAE,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;;SAEnE,OAAO,IAAI,CAAC,QAAQ,CAClB,GAAG,CAAC,GAAG,EACP,GAAG,CAAC,IAAI,EACR,OAAO,QAAQ,KAAK,SAAS,GAAG,QAAQ,GAAG,GAAG,CAAC,QAAQ,CACxD,CAAC;MACH;;KAGD,kBAAG,GAAH,UAAI,MAA0C;SAC5C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC;aAAE,MAAM,GAAG,IAAI,CAAC,SAAS,CAAC,MAAM,CAAC,CAAC;;SAI1D,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,EAAE,CAAC;SAC7B,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,GAAG,MAAM,CAAC;SAC/B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC;SAC5B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,GAAG,MAAM,CAAC;SAE9B,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,KAAK,EAAE,CAAC;SAC/B,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,GAAG,MAAM,CAAC;SACjC,IAAM,GAAG,GAAG,MAAM,CAAC,GAAG,KAAK,EAAE,CAAC;SAC9B,IAAM,GAAG,GAAG,MAAM,CAAC,GAAG,GAAG,MAAM,CAAC;SAEhC,IAAI,GAAG,GAAG,CAAC,EACT,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,CAAC;SACV,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,MAAM,CAAC;SACd,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MAC3E;;;;;KAMD,kBAAG,GAAH,UAAI,KAAyC;SAC3C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;aAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;SACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MACnF;;;;;KAMD,sBAAO,GAAP,UAAQ,KAAyC;SAC/C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;aAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;SACvD,IAAI,IAAI,CAAC,EAAE,CAAC,KAAK,CAAC;aAAE,OAAO,CAAC,CAAC;SAC7B,IAAM,OAAO,GAAG,IAAI,CAAC,UAAU,EAAE,EAC/B,QAAQ,GAAG,KAAK,CAAC,UAAU,EAAE,CAAC;SAChC,IAAI,OAAO,IAAI,CAAC,QAAQ;aAAE,OAAO,CAAC,CAAC,CAAC;SACpC,IAAI,CAAC,OAAO,IAAI,QAAQ;aAAE,OAAO,CAAC,CAAC;;SAEnC,IAAI,CAAC,IAAI,CAAC,QAAQ;aAAE,OAAO,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,UAAU,EAAE,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;SAEjE,OAAO,KAAK,CAAC,IAAI,KAAK,CAAC,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC;cACtC,KAAK,CAAC,IAAI,KAAK,IAAI,CAAC,IAAI,IAAI,KAAK,CAAC,GAAG,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;eAC5D,CAAC,CAAC;eACF,CAAC,CAAC;MACP;;KAGD,mBAAI,GAAJ,UAAK,KAAyC;SAC5C,OAAO,IAAI,CAAC,OAAO,CAAC,KAAK,CAAC,CAAC;MAC5B;;;;;KAMD,qBAAM,GAAN,UAAO,OAA2C;SAChD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;aAAE,OAAO,GAAG,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;SAC7D,IAAI,OAAO,CAAC,MAAM,EAAE;aAAE,MAAM,KAAK,CAAC,kBAAkB,CAAC,CAAC;;SAGtD,IAAI,IAAI,EAAE;;;;aAIR,IACE,CAAC,IAAI,CAAC,QAAQ;iBACd,IAAI,CAAC,IAAI,KAAK,CAAC,UAAU;iBACzB,OAAO,CAAC,GAAG,KAAK,CAAC,CAAC;iBAClB,OAAO,CAAC,IAAI,KAAK,CAAC,CAAC,EACnB;;iBAEA,OAAO,IAAI,CAAC;cACb;aACD,IAAM,GAAG,GAAG,CAAC,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,KAAK,EAClD,IAAI,CAAC,GAAG,EACR,IAAI,CAAC,IAAI,EACT,OAAO,CAAC,GAAG,EACX,OAAO,CAAC,IAAI,CACb,CAAC;aACF,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;UAC3D;SAED,IAAI,IAAI,CAAC,MAAM,EAAE;aAAE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;SACjE,IAAI,MAAM,EAAE,GAAG,EAAE,GAAG,CAAC;SACrB,IAAI,CAAC,IAAI,CAAC,QAAQ,EAAE;;;aAGlB,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;iBAC3B,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,GAAG,CAAC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,OAAO,CAAC;qBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;;sBAEvE,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;qBAAE,OAAO,IAAI,CAAC,GAAG,CAAC;sBAChD;;qBAEH,IAAM,QAAQ,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;qBAC7B,MAAM,GAAG,QAAQ,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;qBACtC,IAAI,MAAM,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,EAAE;yBACxB,OAAO,OAAO,CAAC,UAAU,EAAE,GAAG,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC,OAAO,CAAC;sBACvD;0BAAM;yBACL,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,MAAM,CAAC,CAAC,CAAC;yBACpC,GAAG,GAAG,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,CAAC;yBACnC,OAAO,GAAG,CAAC;sBACZ;kBACF;cACF;kBAAM,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;iBAAE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;aACrF,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;iBACrB,IAAI,OAAO,CAAC,UAAU,EAAE;qBAAE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC;iBAC/D,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,EAAE,CAAC;cACtC;kBAAM,IAAI,OAAO,CAAC,UAAU,EAAE;iBAAE,OAAO,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC;aACtE,GAAG,GAAG,IAAI,CAAC,IAAI,CAAC;UACjB;cAAM;;;aAGL,IAAI,CAAC,OAAO,CAAC,QAAQ;iBAAE,OAAO,GAAG,OAAO,CAAC,UAAU,EAAE,CAAC;aACtD,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC;iBAAE,OAAO,IAAI,CAAC,KAAK,CAAC;aACxC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;;iBAE1B,OAAO,IAAI,CAAC,IAAI,CAAC;aACnB,GAAG,GAAG,IAAI,CAAC,KAAK,CAAC;UAClB;;;;;;SAOD,GAAG,GAAG,IAAI,CAAC;SACX,OAAO,GAAG,CAAC,GAAG,CAAC,OAAO,CAAC,EAAE;;;aAGvB,MAAM,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,QAAQ,EAAE,GAAG,OAAO,CAAC,QAAQ,EAAE,CAAC,CAAC,CAAC;;;aAItE,IAAM,IAAI,GAAG,IAAI,CAAC,IAAI,CAAC,IAAI,CAAC,GAAG,CAAC,MAAM,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC;aACpD,IAAM,KAAK,GAAG,IAAI,IAAI,EAAE,GAAG,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,GAAG,EAAE,CAAC,CAAC;;;aAGtD,IAAI,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,MAAM,CAAC,CAAC;aACxC,IAAI,SAAS,GAAG,SAAS,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;aACvC,OAAO,SAAS,CAAC,UAAU,EAAE,IAAI,SAAS,CAAC,EAAE,CAAC,GAAG,CAAC,EAAE;iBAClD,MAAM,IAAI,KAAK,CAAC;iBAChB,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,MAAM,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;iBACnD,SAAS,GAAG,SAAS,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;cACpC;;;aAID,IAAI,SAAS,CAAC,MAAM,EAAE;iBAAE,SAAS,GAAG,IAAI,CAAC,GAAG,CAAC;aAE7C,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;aACzB,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;UAC1B;SACD,OAAO,GAAG,CAAC;MACZ;;KAGD,kBAAG,GAAH,UAAI,OAA2C;SAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;MAC7B;;;;;KAMD,qBAAM,GAAN,UAAO,KAAyC;SAC9C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;aAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;SACvD,IAAI,IAAI,CAAC,QAAQ,KAAK,KAAK,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,KAAK,EAAE,KAAK,CAAC,IAAI,KAAK,CAAC,IAAI,KAAK,EAAE,KAAK,CAAC;aACvF,OAAO,KAAK,CAAC;SACf,OAAO,IAAI,CAAC,IAAI,KAAK,KAAK,CAAC,IAAI,IAAI,IAAI,CAAC,GAAG,KAAK,KAAK,CAAC,GAAG,CAAC;MAC3D;;KAGD,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC;MAC3B;;KAGD,0BAAW,GAAX;SACE,OAAO,IAAI,CAAC,IAAI,CAAC;MAClB;;KAGD,kCAAmB,GAAnB;SACE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC;MACxB;;KAGD,yBAAU,GAAV;SACE,OAAO,IAAI,CAAC,GAAG,CAAC;MACjB;;KAGD,iCAAkB,GAAlB;SACE,OAAO,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;MACvB;;KAGD,4BAAa,GAAb;SACE,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;;aAErB,OAAO,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,GAAG,EAAE,GAAG,IAAI,CAAC,GAAG,EAAE,CAAC,aAAa,EAAE,CAAC;UAClE;SACD,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC,GAAG,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC;SACnD,IAAI,GAAW,CAAC;SAChB,KAAK,GAAG,GAAG,EAAE,EAAE,GAAG,GAAG,CAAC,EAAE,GAAG,EAAE;aAAE,IAAI,CAAC,GAAG,IAAI,CAAC,IAAI,GAAG,CAAC,MAAM,CAAC;iBAAE,MAAM;SACnE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,GAAG,GAAG,GAAG,EAAE,GAAG,GAAG,GAAG,CAAC,CAAC;MAC7C;;KAGD,0BAAW,GAAX,UAAY,KAAyC;SACnD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;MAC7B;;KAGD,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,WAAW,CAAC,KAAK,CAAC,CAAC;MAChC;;KAGD,iCAAkB,GAAlB,UAAmB,KAAyC;SAC1D,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;MAC9B;;KAGD,kBAAG,GAAH,UAAI,KAAyC;SAC3C,OAAO,IAAI,CAAC,kBAAkB,CAAC,KAAK,CAAC,CAAC;MACvC;;KAED,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,kBAAkB,CAAC,KAAK,CAAC,CAAC;MACvC;;KAGD,qBAAM,GAAN;SACE,OAAO,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC,MAAM,CAAC,CAAC;MAC7B;;KAGD,yBAAU,GAAV;SACE,OAAO,CAAC,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,GAAG,CAAC,CAAC;MACxC;;KAGD,oBAAK,GAAL;SACE,OAAO,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC,MAAM,CAAC,CAAC;MAC7B;;KAGD,yBAAU,GAAV;SACE,OAAO,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC,CAAC;MACxC;;KAGD,qBAAM,GAAN;SACE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;MAC1C;;KAGD,uBAAQ,GAAR,UAAS,KAAyC;SAChD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;MAC7B;;KAGD,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;MAC7B;;KAGD,8BAAe,GAAf,UAAgB,KAAyC;SACvD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;MAC9B;;KAGD,kBAAG,GAAH,UAAI,KAAyC;SAC3C,OAAO,IAAI,CAAC,eAAe,CAAC,KAAK,CAAC,CAAC;MACpC;;KAGD,qBAAM,GAAN,UAAO,OAA2C;SAChD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;aAAE,OAAO,GAAG,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;;SAG7D,IAAI,IAAI,EAAE;aACR,IAAM,GAAG,GAAG,CAAC,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,KAAK,EAClD,IAAI,CAAC,GAAG,EACR,IAAI,CAAC,IAAI,EACT,OAAO,CAAC,GAAG,EACX,OAAO,CAAC,IAAI,CACb,CAAC;aACF,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;UAC3D;SAED,OAAO,IAAI,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,CAAC;MACjD;;KAGD,kBAAG,GAAH,UAAI,OAA2C;SAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;MAC7B;;KAED,kBAAG,GAAH,UAAI,OAA2C;SAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;MAC7B;;;;;;KAOD,uBAAQ,GAAR,UAAS,UAA8C;SACrD,IAAI,IAAI,CAAC,MAAM,EAAE;aAAE,OAAO,IAAI,CAAC,IAAI,CAAC;SACpC,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;aAAE,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;;SAGtE,IAAI,IAAI,EAAE;aACR,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,UAAU,CAAC,GAAG,EAAE,UAAU,CAAC,IAAI,CAAC,CAAC;aAC3E,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;UAC3D;SAED,IAAI,UAAU,CAAC,MAAM,EAAE;aAAE,OAAO,IAAI,CAAC,IAAI,CAAC;SAC1C,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;aAAE,OAAO,UAAU,CAAC,KAAK,EAAE,GAAG,IAAI,CAAC,SAAS,GAAG,IAAI,CAAC,IAAI,CAAC;SACpF,IAAI,UAAU,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;aAAE,OAAO,IAAI,CAAC,KAAK,EAAE,GAAG,IAAI,CAAC,SAAS,GAAG,IAAI,CAAC,IAAI,CAAC;SAEpF,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;aACrB,IAAI,UAAU,CAAC,UAAU,EAAE;iBAAE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC;;iBAChE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC,GAAG,EAAE,CAAC;UAC9C;cAAM,IAAI,UAAU,CAAC,UAAU,EAAE;aAAE,OAAO,IAAI,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC;;SAG5E,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,UAAU,CAAC,IAAI,UAAU,CAAC,EAAE,CAAC,IAAI,CAAC,UAAU,CAAC;aAC5D,OAAO,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,QAAQ,EAAE,GAAG,UAAU,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;;SAKjF,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,EAAE,CAAC;SAC7B,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,GAAG,MAAM,CAAC;SAC/B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC;SAC5B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,GAAG,MAAM,CAAC;SAE9B,IAAM,GAAG,GAAG,UAAU,CAAC,IAAI,KAAK,EAAE,CAAC;SACnC,IAAM,GAAG,GAAG,UAAU,CAAC,IAAI,GAAG,MAAM,CAAC;SACrC,IAAM,GAAG,GAAG,UAAU,CAAC,GAAG,KAAK,EAAE,CAAC;SAClC,IAAM,GAAG,GAAG,UAAU,CAAC,GAAG,GAAG,MAAM,CAAC;SAEpC,IAAI,GAAG,GAAG,CAAC,EACT,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,CAAC;SACV,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,CAAC;SACrD,GAAG,IAAI,MAAM,CAAC;SACd,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MAC3E;;KAGD,kBAAG,GAAH,UAAI,UAA8C;SAChD,OAAO,IAAI,CAAC,QAAQ,CAAC,UAAU,CAAC,CAAC;MAClC;;KAGD,qBAAM,GAAN;SACE,IAAI,CAAC,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;aAAE,OAAO,IAAI,CAAC,SAAS,CAAC;SACrE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;MACjC;;KAGD,kBAAG,GAAH;SACE,OAAO,IAAI,CAAC,MAAM,EAAE,CAAC;MACtB;;KAGD,kBAAG,GAAH;SACE,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MAC5D;;KAGD,wBAAS,GAAT,UAAU,KAAyC;SACjD,OAAO,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC;MAC5B;;KAGD,kBAAG,GAAH,UAAI,KAAyC;SAC3C,OAAO,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;MAC9B;;KAED,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;MAC9B;;;;KAKD,iBAAE,GAAF,UAAG,KAA6B;SAC9B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;aAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;SACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MACnF;;;;;;KAOD,wBAAS,GAAT,UAAU,OAAsB;SAC9B,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;aAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;SACpD,IAAI,CAAC,OAAO,IAAI,EAAE,MAAM,CAAC;aAAE,OAAO,IAAI,CAAC;cAClC,IAAI,OAAO,GAAG,EAAE;aACnB,OAAO,IAAI,CAAC,QAAQ,CAClB,IAAI,CAAC,GAAG,IAAI,OAAO,EACnB,CAAC,IAAI,CAAC,IAAI,IAAI,OAAO,KAAK,IAAI,CAAC,GAAG,MAAM,EAAE,GAAG,OAAO,CAAC,CAAC,EACtD,IAAI,CAAC,QAAQ,CACd,CAAC;;aACC,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,EAAE,IAAI,CAAC,GAAG,KAAK,OAAO,GAAG,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MACzE;;KAGD,kBAAG,GAAH,UAAI,OAAsB;SACxB,OAAO,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;MAChC;;;;;;KAOD,yBAAU,GAAV,UAAW,OAAsB;SAC/B,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;aAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;SACpD,IAAI,CAAC,OAAO,IAAI,EAAE,MAAM,CAAC;aAAE,OAAO,IAAI,CAAC;cAClC,IAAI,OAAO,GAAG,EAAE;aACnB,OAAO,IAAI,CAAC,QAAQ,CAClB,CAAC,IAAI,CAAC,GAAG,KAAK,OAAO,KAAK,IAAI,CAAC,IAAI,KAAK,EAAE,GAAG,OAAO,CAAC,CAAC,EACtD,IAAI,CAAC,IAAI,IAAI,OAAO,EACpB,IAAI,CAAC,QAAQ,CACd,CAAC;;aACC,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,IAAI,KAAK,OAAO,GAAG,EAAE,CAAC,EAAE,IAAI,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MAChG;;KAGD,kBAAG,GAAH,UAAI,OAAsB;SACxB,OAAO,IAAI,CAAC,UAAU,CAAC,OAAO,CAAC,CAAC;MACjC;;;;;;KAOD,iCAAkB,GAAlB,UAAmB,OAAsB;SACvC,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;aAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;SACpD,OAAO,IAAI,EAAE,CAAC;SACd,IAAI,OAAO,KAAK,CAAC;aAAE,OAAO,IAAI,CAAC;cAC1B;aACH,IAAM,IAAI,GAAG,IAAI,CAAC,IAAI,CAAC;aACvB,IAAI,OAAO,GAAG,EAAE,EAAE;iBAChB,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC;iBACrB,OAAO,IAAI,CAAC,QAAQ,CAClB,CAAC,GAAG,KAAK,OAAO,KAAK,IAAI,KAAK,EAAE,GAAG,OAAO,CAAC,CAAC,EAC5C,IAAI,KAAK,OAAO,EAChB,IAAI,CAAC,QAAQ,CACd,CAAC;cACH;kBAAM,IAAI,OAAO,KAAK,EAAE;iBAAE,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;iBACnE,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,MAAM,OAAO,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;UACtE;MACF;;KAGD,oBAAK,GAAL,UAAM,OAAsB;SAC1B,OAAO,IAAI,CAAC,kBAAkB,CAAC,OAAO,CAAC,CAAC;MACzC;;KAED,mBAAI,GAAJ,UAAK,OAAsB;SACzB,OAAO,IAAI,CAAC,kBAAkB,CAAC,OAAO,CAAC,CAAC;MACzC;;;;;;KAOD,uBAAQ,GAAR,UAAS,UAA8C;SACrD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;aAAE,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;SACtE,OAAO,IAAI,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC;MACnC;;KAGD,kBAAG,GAAH,UAAI,UAA8C;SAChD,OAAO,IAAI,CAAC,QAAQ,CAAC,UAAU,CAAC,CAAC;MAClC;;KAGD,oBAAK,GAAL;SACE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC;MAClD;;KAGD,uBAAQ,GAAR;SACE,IAAI,IAAI,CAAC,QAAQ;aAAE,OAAO,CAAC,IAAI,CAAC,IAAI,KAAK,CAAC,IAAI,cAAc,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC;SAChF,OAAO,IAAI,CAAC,IAAI,GAAG,cAAc,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC;MACtD;;KAGD,uBAAQ,GAAR;SACE,OAAO,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,CAAC;MAChC;;;;;;KAOD,sBAAO,GAAP,UAAQ,EAAY;SAClB,OAAO,EAAE,GAAG,IAAI,CAAC,SAAS,EAAE,GAAG,IAAI,CAAC,SAAS,EAAE,CAAC;MACjD;;;;;KAMD,wBAAS,GAAT;SACE,IAAM,EAAE,GAAG,IAAI,CAAC,IAAI,EAClB,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO;aACL,EAAE,GAAG,IAAI;aACT,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;aACjB,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;aAClB,EAAE,KAAK,EAAE;aACT,EAAE,GAAG,IAAI;aACT,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;aACjB,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;aAClB,EAAE,KAAK,EAAE;UACV,CAAC;MACH;;;;;KAMD,wBAAS,GAAT;SACE,IAAM,EAAE,GAAG,IAAI,CAAC,IAAI,EAClB,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO;aACL,EAAE,KAAK,EAAE;aACT,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;aAClB,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;aACjB,EAAE,GAAG,IAAI;aACT,EAAE,KAAK,EAAE;aACT,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;aAClB,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;aACjB,EAAE,GAAG,IAAI;UACV,CAAC;MACH;;;;KAKD,uBAAQ,GAAR;SACE,IAAI,CAAC,IAAI,CAAC,QAAQ;aAAE,OAAO,IAAI,CAAC;SAChC,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;MAClD;;;;;;KAOD,uBAAQ,GAAR,UAAS,KAAc;SACrB,KAAK,GAAG,KAAK,IAAI,EAAE,CAAC;SACpB,IAAI,KAAK,GAAG,CAAC,IAAI,EAAE,GAAG,KAAK;aAAE,MAAM,UAAU,CAAC,OAAO,CAAC,CAAC;SACvD,IAAI,IAAI,CAAC,MAAM,EAAE;aAAE,OAAO,GAAG,CAAC;SAC9B,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;;aAErB,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;;;iBAG3B,IAAM,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,EACtC,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,SAAS,CAAC,EACzB,IAAI,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;iBACtC,OAAO,GAAG,CAAC,QAAQ,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC,KAAK,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;cAC3D;;iBAAM,OAAO,GAAG,GAAG,IAAI,CAAC,GAAG,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;UAChD;;;SAID,IAAM,YAAY,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;SAExE,IAAI,GAAG,GAAS,IAAI,CAAC;SACrB,IAAI,MAAM,GAAG,EAAE,CAAC;;SAEhB,OAAO,IAAI,EAAE;aACX,IAAM,MAAM,GAAG,GAAG,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC;aACrC,IAAM,MAAM,GAAG,GAAG,CAAC,GAAG,CAAC,MAAM,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC,CAAC,KAAK,EAAE,KAAK,CAAC,CAAC;aAC/D,IAAI,MAAM,GAAG,MAAM,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aACpC,GAAG,GAAG,MAAM,CAAC;aACb,IAAI,GAAG,CAAC,MAAM,EAAE,EAAE;iBAChB,OAAO,MAAM,GAAG,MAAM,CAAC;cACxB;kBAAM;iBACL,OAAO,MAAM,CAAC,MAAM,GAAG,CAAC;qBAAE,MAAM,GAAG,GAAG,GAAG,MAAM,CAAC;iBAChD,MAAM,GAAG,EAAE,GAAG,MAAM,GAAG,MAAM,CAAC;cAC/B;UACF;MACF;;KAGD,yBAAU,GAAV;SACE,IAAI,IAAI,CAAC,QAAQ;aAAE,OAAO,IAAI,CAAC;SAC/B,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;MACjD;;KAGD,kBAAG,GAAH,UAAI,KAA6B;SAC/B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;aAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;SACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MACnF;;KAGD,kBAAG,GAAH;SACE,OAAO,IAAI,CAAC,MAAM,EAAE,CAAC;MACtB;;KAGD,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,eAAe,CAAC,KAAK,CAAC,CAAC;MACpC;;;;;;KAOD,6BAAc,GAAd,UAAe,OAAsB;SACnC,IAAI,OAAO,IAAI,OAAO,CAAC,OAAO;aAAE,OAAO,IAAI,CAAC,QAAQ,EAAE,CAAC;SACvD,OAAO,EAAE,WAAW,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;MACzC;KACM,qBAAgB,GAAvB,UAAwB,GAA4B,EAAE,OAAsB;SAC1E,IAAM,MAAM,GAAG,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,WAAW,CAAC,CAAC;SAChD,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,MAAM,CAAC,QAAQ,EAAE,GAAG,MAAM,CAAC;MAChE;;KAGD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,sBAAO,GAAP;SACE,OAAO,gBAAa,IAAI,CAAC,QAAQ,EAAE,WAAI,IAAI,CAAC,QAAQ,GAAG,QAAQ,GAAG,EAAE,OAAG,CAAC;MACzE;KA/2BM,eAAU,GAAG,IAAI,CAAC,OAAO,CAAC,cAAc,CAAC,CAAC;;KAG1C,uBAAkB,GAAG,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,IAAI,CAAC,CAAC;;KAEzE,SAAI,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;;KAEvB,UAAK,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;;KAE9B,QAAG,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;;KAEtB,SAAI,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;;KAE7B,YAAO,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC,CAAC;;KAE3B,cAAS,GAAG,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;;KAEjE,cAAS,GAAG,IAAI,CAAC,QAAQ,CAAC,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;KA+1B7D,WAAC;EAv6BD,IAu6BC;CAv6BY,oBAAI;CAy6BjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,YAAY,EAAE,EAAE,KAAK,EAAE,IAAI,EAAE,CAAC,CAAC;CACrE,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;ACr/BtC;AACF;CAE9B,IAAM,mBAAmB,GAAG,+CAA+C,CAAC;CAC5E,IAAM,gBAAgB,GAAG,0BAA0B,CAAC;CACpD,IAAM,gBAAgB,GAAG,eAAe,CAAC;CAEzC,IAAM,YAAY,GAAG,IAAI,CAAC;CAC1B,IAAM,YAAY,GAAG,CAAC,IAAI,CAAC;CAC3B,IAAM,aAAa,GAAG,IAAI,CAAC;CAC3B,IAAM,UAAU,GAAG,EAAE,CAAC;CAEtB;CACA,IAAM,UAAU,GAAG;KACjB,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;EACL,CAAC,OAAO,EAAE,CAAC;CACZ;CACA,IAAM,mBAAmB,GAAG;KAC1B,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;EACL,CAAC,OAAO,EAAE,CAAC;CACZ,IAAM,mBAAmB,GAAG;KAC1B,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;EACL,CAAC,OAAO,EAAE,CAAC;CAEZ,IAAM,cAAc,GAAG,iBAAiB,CAAC;CAEzC;CACA,IAAM,gBAAgB,GAAG,IAAI,CAAC;CAC9B;CACA,IAAM,aAAa,GAAG,MAAM,CAAC;CAC7B;CACA,IAAM,oBAAoB,GAAG,EAAE,CAAC;CAChC;CACA,IAAM,eAAe,GAAG,EAAE,CAAC;CAE3B;CACA,SAAS,OAAO,CAAC,KAAa;KAC5B,OAAO,CAAC,KAAK,CAAC,QAAQ,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,CAAC;CACrC,CAAC;CAED;CACA,SAAS,UAAU,CAAC,KAAkD;KACpE,IAAM,OAAO,GAAG,WAAI,CAAC,UAAU,CAAC,IAAI,GAAG,IAAI,GAAG,IAAI,CAAC,CAAC;KACpD,IAAI,IAAI,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;KAE9B,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE;SAC5E,OAAO,EAAE,QAAQ,EAAE,KAAK,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;MACvC;KAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;;SAE3B,IAAI,GAAG,IAAI,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC;;SAE1B,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC,IAAI,WAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;SAC7C,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC;SACvC,IAAI,GAAG,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;MAC7B;KAED,OAAO,EAAE,QAAQ,EAAE,KAAK,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;CACxC,CAAC;CAED;CACA,SAAS,YAAY,CAAC,IAAU,EAAE,KAAW;KAC3C,IAAI,CAAC,IAAI,IAAI,CAAC,KAAK,EAAE;SACnB,OAAO,EAAE,IAAI,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,GAAG,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,CAAC;MAC9D;KAED,IAAM,QAAQ,GAAG,IAAI,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC;KAC7C,IAAM,OAAO,GAAG,IAAI,WAAI,CAAC,IAAI,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC;KAC/C,IAAM,SAAS,GAAG,KAAK,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC;KAC/C,IAAM,QAAQ,GAAG,IAAI,WAAI,CAAC,KAAK,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC;KAEjD,IAAI,WAAW,GAAG,QAAQ,CAAC,QAAQ,CAAC,SAAS,CAAC,CAAC;KAC/C,IAAI,UAAU,GAAG,QAAQ,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;KAC7C,IAAM,WAAW,GAAG,OAAO,CAAC,QAAQ,CAAC,SAAS,CAAC,CAAC;KAChD,IAAI,UAAU,GAAG,OAAO,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;KAE5C,WAAW,GAAG,WAAW,CAAC,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;KACjE,UAAU,GAAG,IAAI,WAAI,CAAC,UAAU,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC;UAC9C,GAAG,CAAC,WAAW,CAAC;UAChB,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;KAE1C,WAAW,GAAG,WAAW,CAAC,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;KACjE,UAAU,GAAG,UAAU,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,GAAG,CAAC,IAAI,WAAI,CAAC,UAAU,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC,CAAC;;KAGhF,OAAO,EAAE,IAAI,EAAE,WAAW,EAAE,GAAG,EAAE,UAAU,EAAE,CAAC;CAChD,CAAC;CAED,SAAS,QAAQ,CAAC,IAAU,EAAE,KAAW;;KAEvC,IAAM,MAAM,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC;KAC/B,IAAM,OAAO,GAAG,KAAK,CAAC,IAAI,KAAK,CAAC,CAAC;;KAGjC,IAAI,MAAM,GAAG,OAAO,EAAE;SACpB,OAAO,IAAI,CAAC;MACb;UAAM,IAAI,MAAM,KAAK,OAAO,EAAE;SAC7B,IAAM,MAAM,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;SAC9B,IAAM,OAAO,GAAG,KAAK,CAAC,GAAG,KAAK,CAAC,CAAC;SAChC,IAAI,MAAM,GAAG,OAAO;aAAE,OAAO,IAAI,CAAC;MACnC;KAED,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,UAAU,CAAC,MAAc,EAAE,OAAe;KACjD,MAAM,IAAI,SAAS,CAAC,OAAI,MAAM,8CAAwC,OAAS,CAAC,CAAC;CACnF,CAAC;CAOD;;;;CAIA;;;;;KASE,oBAAY,KAAsB;SAChC,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;aAAE,OAAO,IAAI,UAAU,CAAC,KAAK,CAAC,CAAC;SAEhE,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;aAC7B,IAAI,CAAC,KAAK,GAAG,UAAU,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,KAAK,CAAC;UACjD;cAAM;aACL,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;UACpB;MACF;;;;;;KAOM,qBAAU,GAAjB,UAAkB,cAAsB;;SAEtC,IAAI,UAAU,GAAG,KAAK,CAAC;SACvB,IAAI,QAAQ,GAAG,KAAK,CAAC;SACrB,IAAI,YAAY,GAAG,KAAK,CAAC;;SAGzB,IAAI,iBAAiB,GAAG,CAAC,CAAC;;SAE1B,IAAI,WAAW,GAAG,CAAC,CAAC;;SAEpB,IAAI,OAAO,GAAG,CAAC,CAAC;;SAEhB,IAAI,aAAa,GAAG,CAAC,CAAC;;SAEtB,IAAI,YAAY,GAAG,CAAC,CAAC;;SAGrB,IAAM,MAAM,GAAG,CAAC,CAAC,CAAC,CAAC;;SAEnB,IAAI,aAAa,GAAG,CAAC,CAAC;;SAEtB,IAAI,YAAY,GAAG,CAAC,CAAC;;SAErB,IAAI,UAAU,GAAG,CAAC,CAAC;;SAEnB,IAAI,SAAS,GAAG,CAAC,CAAC;;SAGlB,IAAI,QAAQ,GAAG,CAAC,CAAC;;SAEjB,IAAI,CAAC,GAAG,CAAC,CAAC;;SAEV,IAAI,eAAe,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;;SAErC,IAAI,cAAc,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;;SAEpC,IAAI,cAAc,GAAG,CAAC,CAAC;;SAGvB,IAAI,KAAK,GAAG,CAAC,CAAC;;;;SAKd,IAAI,cAAc,CAAC,MAAM,IAAI,IAAI,EAAE;aACjC,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;UAC7E;;SAGD,IAAM,WAAW,GAAG,cAAc,CAAC,KAAK,CAAC,mBAAmB,CAAC,CAAC;SAC9D,IAAM,QAAQ,GAAG,cAAc,CAAC,KAAK,CAAC,gBAAgB,CAAC,CAAC;SACxD,IAAM,QAAQ,GAAG,cAAc,CAAC,KAAK,CAAC,gBAAgB,CAAC,CAAC;;SAGxD,IAAI,CAAC,CAAC,WAAW,IAAI,CAAC,QAAQ,IAAI,CAAC,QAAQ,KAAK,cAAc,CAAC,MAAM,KAAK,CAAC,EAAE;aAC3E,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;UAC7E;SAED,IAAI,WAAW,EAAE;;;aAIf,IAAM,cAAc,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;;;aAItC,IAAM,CAAC,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;aACzB,IAAM,OAAO,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;aAC/B,IAAM,SAAS,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;;aAGjC,IAAI,CAAC,IAAI,SAAS,KAAK,SAAS;iBAAE,UAAU,CAAC,cAAc,EAAE,wBAAwB,CAAC,CAAC;;aAGvF,IAAI,CAAC,IAAI,cAAc,KAAK,SAAS;iBAAE,UAAU,CAAC,cAAc,EAAE,uBAAuB,CAAC,CAAC;aAE3F,IAAI,CAAC,KAAK,SAAS,KAAK,OAAO,IAAI,SAAS,CAAC,EAAE;iBAC7C,UAAU,CAAC,cAAc,EAAE,2BAA2B,CAAC,CAAC;cACzD;UACF;;SAGD,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;aAClE,UAAU,GAAG,cAAc,CAAC,KAAK,EAAE,CAAC,KAAK,GAAG,CAAC;UAC9C;;SAGD,IAAI,CAAC,OAAO,CAAC,cAAc,CAAC,KAAK,CAAC,CAAC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;aACpE,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;iBAClE,OAAO,IAAI,UAAU,CAACD,aAAM,CAAC,IAAI,CAAC,UAAU,GAAG,mBAAmB,GAAG,mBAAmB,CAAC,CAAC,CAAC;cAC5F;kBAAM,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;iBACxC,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;cAChD;UACF;;SAGD,OAAO,OAAO,CAAC,cAAc,CAAC,KAAK,CAAC,CAAC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;aACtE,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;iBACjC,IAAI,QAAQ;qBAAE,UAAU,CAAC,cAAc,EAAE,2BAA2B,CAAC,CAAC;iBAEtE,QAAQ,GAAG,IAAI,CAAC;iBAChB,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;iBAClB,SAAS;cACV;aAED,IAAI,aAAa,GAAG,EAAE,EAAE;iBACtB,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,YAAY,EAAE;qBACjD,IAAI,CAAC,YAAY,EAAE;yBACjB,YAAY,GAAG,WAAW,CAAC;sBAC5B;qBAED,YAAY,GAAG,IAAI,CAAC;;qBAGpB,MAAM,CAAC,YAAY,EAAE,CAAC,GAAG,QAAQ,CAAC,cAAc,CAAC,KAAK,CAAC,EAAE,EAAE,CAAC,CAAC;qBAC7D,aAAa,GAAG,aAAa,GAAG,CAAC,CAAC;kBACnC;cACF;aAED,IAAI,YAAY;iBAAE,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC;aACxC,IAAI,QAAQ;iBAAE,aAAa,GAAG,aAAa,GAAG,CAAC,CAAC;aAEhD,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;aAC9B,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;UACnB;SAED,IAAI,QAAQ,IAAI,CAAC,WAAW;aAC1B,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;;SAG9E,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;;aAElE,IAAM,KAAK,GAAG,cAAc,CAAC,MAAM,CAAC,EAAE,KAAK,CAAC,CAAC,KAAK,CAAC,cAAc,CAAC,CAAC;;aAGnE,IAAI,CAAC,KAAK,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC;iBAAE,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;;aAGxE,QAAQ,GAAG,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;;aAGlC,KAAK,GAAG,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC;UACjC;;SAGD,IAAI,cAAc,CAAC,KAAK,CAAC;aAAE,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;;;SAI1E,UAAU,GAAG,CAAC,CAAC;SAEf,IAAI,CAAC,aAAa,EAAE;aAClB,UAAU,GAAG,CAAC,CAAC;aACf,SAAS,GAAG,CAAC,CAAC;aACd,MAAM,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;aACd,OAAO,GAAG,CAAC,CAAC;aACZ,aAAa,GAAG,CAAC,CAAC;aAClB,iBAAiB,GAAG,CAAC,CAAC;UACvB;cAAM;aACL,SAAS,GAAG,aAAa,GAAG,CAAC,CAAC;aAC9B,iBAAiB,GAAG,OAAO,CAAC;aAC5B,IAAI,iBAAiB,KAAK,CAAC,EAAE;iBAC3B,OAAO,cAAc,CAAC,YAAY,GAAG,iBAAiB,GAAG,CAAC,CAAC,KAAK,GAAG,EAAE;qBACnE,iBAAiB,GAAG,iBAAiB,GAAG,CAAC,CAAC;kBAC3C;cACF;UACF;;;;;SAOD,IAAI,QAAQ,IAAI,aAAa,IAAI,aAAa,GAAG,QAAQ,GAAG,CAAC,IAAI,EAAE,EAAE;aACnE,QAAQ,GAAG,YAAY,CAAC;UACzB;cAAM;aACL,QAAQ,GAAG,QAAQ,GAAG,aAAa,CAAC;UACrC;;SAGD,OAAO,QAAQ,GAAG,YAAY,EAAE;;aAE9B,SAAS,GAAG,SAAS,GAAG,CAAC,CAAC;aAE1B,IAAI,SAAS,GAAG,UAAU,GAAG,UAAU,EAAE;;iBAEvC,IAAM,YAAY,GAAG,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;iBACrC,IAAI,YAAY,CAAC,KAAK,CAAC,MAAM,CAAC,EAAE;qBAC9B,QAAQ,GAAG,YAAY,CAAC;qBACxB,MAAM;kBACP;iBAED,UAAU,CAAC,cAAc,EAAE,UAAU,CAAC,CAAC;cACxC;aACD,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;UACzB;SAED,OAAO,QAAQ,GAAG,YAAY,IAAI,aAAa,GAAG,OAAO,EAAE;;aAEzD,IAAI,SAAS,KAAK,CAAC,IAAI,iBAAiB,GAAG,aAAa,EAAE;iBACxD,QAAQ,GAAG,YAAY,CAAC;iBACxB,iBAAiB,GAAG,CAAC,CAAC;iBACtB,MAAM;cACP;aAED,IAAI,aAAa,GAAG,OAAO,EAAE;;iBAE3B,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC;cACvB;kBAAM;;iBAEL,SAAS,GAAG,SAAS,GAAG,CAAC,CAAC;cAC3B;aAED,IAAI,QAAQ,GAAG,YAAY,EAAE;iBAC3B,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;cACzB;kBAAM;;iBAEL,IAAM,YAAY,GAAG,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;iBACrC,IAAI,YAAY,CAAC,KAAK,CAAC,MAAM,CAAC,EAAE;qBAC9B,QAAQ,GAAG,YAAY,CAAC;qBACxB,MAAM;kBACP;iBACD,UAAU,CAAC,cAAc,EAAE,UAAU,CAAC,CAAC;cACxC;UACF;;;SAID,IAAI,SAAS,GAAG,UAAU,GAAG,CAAC,GAAG,iBAAiB,EAAE;aAClD,IAAI,WAAW,GAAG,WAAW,CAAC;;;;aAK9B,IAAI,QAAQ,EAAE;iBACZ,YAAY,GAAG,YAAY,GAAG,CAAC,CAAC;iBAChC,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;cAC/B;;aAED,IAAI,UAAU,EAAE;iBACd,YAAY,GAAG,YAAY,GAAG,CAAC,CAAC;iBAChC,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;cAC/B;aAED,IAAM,UAAU,GAAG,QAAQ,CAAC,cAAc,CAAC,YAAY,GAAG,SAAS,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;aAC9E,IAAI,QAAQ,GAAG,CAAC,CAAC;aAEjB,IAAI,UAAU,IAAI,CAAC,EAAE;iBACnB,QAAQ,GAAG,CAAC,CAAC;iBACb,IAAI,UAAU,KAAK,CAAC,EAAE;qBACpB,QAAQ,GAAG,MAAM,CAAC,SAAS,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;qBAC/C,KAAK,CAAC,GAAG,YAAY,GAAG,SAAS,GAAG,CAAC,EAAE,CAAC,GAAG,WAAW,EAAE,CAAC,EAAE,EAAE;yBAC3D,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE;6BACnC,QAAQ,GAAG,CAAC,CAAC;6BACb,MAAM;0BACP;sBACF;kBACF;cACF;aAED,IAAI,QAAQ,EAAE;iBACZ,IAAI,IAAI,GAAG,SAAS,CAAC;iBAErB,OAAO,IAAI,IAAI,CAAC,EAAE,IAAI,EAAE,EAAE;qBACxB,IAAI,EAAE,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,EAAE;yBACtB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;yBAGjB,IAAI,IAAI,KAAK,CAAC,EAAE;6BACd,IAAI,QAAQ,GAAG,YAAY,EAAE;iCAC3B,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;iCACxB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;8BAClB;kCAAM;iCACL,OAAO,IAAI,UAAU,CACnBA,aAAM,CAAC,IAAI,CAAC,UAAU,GAAG,mBAAmB,GAAG,mBAAmB,CAAC,CACpE,CAAC;8BACH;0BACF;sBACF;kBACF;cACF;UACF;;;SAID,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;;SAErC,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;;SAGpC,IAAI,iBAAiB,KAAK,CAAC,EAAE;aAC3B,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;aACrC,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;UACrC;cAAM,IAAI,SAAS,GAAG,UAAU,GAAG,EAAE,EAAE;aACtC,IAAI,IAAI,GAAG,UAAU,CAAC;aACtB,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;aACjD,eAAe,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;aAEjC,OAAO,IAAI,IAAI,SAAS,EAAE,IAAI,EAAE,EAAE;iBAChC,cAAc,GAAG,cAAc,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;iBAC9D,cAAc,GAAG,cAAc,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;cACpE;UACF;cAAM;aACL,IAAI,IAAI,GAAG,UAAU,CAAC;aACtB,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;aAElD,OAAO,IAAI,IAAI,SAAS,GAAG,EAAE,EAAE,IAAI,EAAE,EAAE;iBACrC,eAAe,GAAG,eAAe,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;iBAChE,eAAe,GAAG,eAAe,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;cACtE;aAED,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;aAEjD,OAAO,IAAI,IAAI,SAAS,EAAE,IAAI,EAAE,EAAE;iBAChC,cAAc,GAAG,cAAc,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;iBAC9D,cAAc,GAAG,cAAc,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;cACpE;UACF;SAED,IAAM,WAAW,GAAG,YAAY,CAAC,eAAe,EAAE,WAAI,CAAC,UAAU,CAAC,oBAAoB,CAAC,CAAC,CAAC;SACzF,WAAW,CAAC,GAAG,GAAG,WAAW,CAAC,GAAG,CAAC,GAAG,CAAC,cAAc,CAAC,CAAC;SAEtD,IAAI,QAAQ,CAAC,WAAW,CAAC,GAAG,EAAE,cAAc,CAAC,EAAE;aAC7C,WAAW,CAAC,IAAI,GAAG,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC;UAC7D;;SAGD,cAAc,GAAG,QAAQ,GAAG,aAAa,CAAC;SAC1C,IAAM,GAAG,GAAG,EAAE,GAAG,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,IAAI,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,CAAC;;SAGlE,IACE,WAAW,CAAC,IAAI,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,EAC1F;;aAEA,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,GAAG,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAAC;aAC3D,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CACpB,WAAI,CAAC,UAAU,CAAC,cAAc,CAAC,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAC3E,CAAC;aACF,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,cAAc,CAAC,CAAC,CAAC,CAAC;UAC/E;cAAM;aACL,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,cAAc,GAAG,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAAC;aAC/E,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,eAAe,CAAC,CAAC,CAAC,CAAC;UAChF;SAED,GAAG,CAAC,GAAG,GAAG,WAAW,CAAC,GAAG,CAAC;;SAG1B,IAAI,UAAU,EAAE;aACd,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,qBAAqB,CAAC,CAAC,CAAC;UAChE;;SAGD,IAAMvX,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;SAChC,KAAK,GAAG,CAAC,CAAC;;;SAIVvX,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,GAAG,CAAC,GAAG,GAAG,IAAI,CAAC;SACrCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;SAC5CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;SAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;;SAE7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,GAAG,CAAC,IAAI,GAAG,IAAI,CAAC;SACtCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;SAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;SAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;;SAI9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC;SACtCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;SAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;SAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;;SAE9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC;SACvCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;SAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;SAC/CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;SAG/C,OAAO,IAAI,UAAU,CAACA,QAAM,CAAC,CAAC;MAC/B;;KAGD,6BAAQ,GAAR;;;;SAKE,IAAI,eAAe,CAAC;;SAEpB,IAAI,kBAAkB,GAAG,CAAC,CAAC;;SAE3B,IAAM,WAAW,GAAG,IAAI,KAAK,CAAS,EAAE,CAAC,CAAC;SAC1C,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,WAAW,CAAC,MAAM,EAAE,CAAC,EAAE;aAAE,WAAW,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;;SAEhE,IAAI,KAAK,GAAG,CAAC,CAAC;;SAGd,IAAI,OAAO,GAAG,KAAK,CAAC;;SAGpB,IAAI,eAAe,CAAC;;SAEpB,IAAI,cAAc,GAAgD,EAAE,KAAK,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,EAAE,CAAC;;SAE1F,IAAI,CAAC,EAAE,CAAC,CAAC;;SAGT,IAAM,MAAM,GAAa,EAAE,CAAC;;SAG5B,KAAK,GAAG,CAAC,CAAC;;SAGV,IAAM,MAAM,GAAG,IAAI,CAAC,KAAK,CAAC;;;SAI1B,IAAM,GAAG,GACP,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;SAE/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;;SAI/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;SAE/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;SAG/F,KAAK,GAAG,CAAC,CAAC;;SAGV,IAAM,GAAG,GAAG;aACV,GAAG,EAAE,IAAI,WAAI,CAAC,GAAG,EAAE,IAAI,CAAC;aACxB,IAAI,EAAE,IAAI,WAAI,CAAC,IAAI,EAAE,IAAI,CAAC;UAC3B,CAAC;SAEF,IAAI,GAAG,CAAC,IAAI,CAAC,QAAQ,CAAC,WAAI,CAAC,IAAI,CAAC,EAAE;aAChC,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;UAClB;;;SAID,IAAM,WAAW,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,gBAAgB,CAAC;SAEpD,IAAI,WAAW,IAAI,CAAC,KAAK,CAAC,EAAE;;aAE1B,IAAI,WAAW,KAAK,oBAAoB,EAAE;iBACxC,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,GAAG,UAAU,CAAC;cACrC;kBAAM,IAAI,WAAW,KAAK,eAAe,EAAE;iBAC1C,OAAO,KAAK,CAAC;cACd;kBAAM;iBACL,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,aAAa,CAAC;iBAC/C,eAAe,GAAG,IAAI,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC,CAAC;cAChD;UACF;cAAM;aACL,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;aACtC,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,aAAa,CAAC;UAChD;;SAGD,IAAM,QAAQ,GAAG,eAAe,GAAG,aAAa,CAAC;;;;;SAOjD,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,IAAI,GAAG,MAAM,KAAK,CAAC,eAAe,GAAG,GAAG,KAAK,EAAE,CAAC,CAAC;SAC5E,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC;SAC/B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC;SAC/B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;SAE9B,IACE,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;aAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;aAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;aAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC,EAC7B;aACA,OAAO,GAAG,IAAI,CAAC;UAChB;cAAM;aACL,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;iBACvB,IAAI,YAAY,GAAG,CAAC,CAAC;;iBAErB,IAAM,MAAM,GAAG,UAAU,CAAC,cAAc,CAAC,CAAC;iBAC1C,cAAc,GAAG,MAAM,CAAC,QAAQ,CAAC;iBACjC,YAAY,GAAG,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;;;iBAI9B,IAAI,CAAC,YAAY;qBAAE,SAAS;iBAE5B,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;;qBAEvB,WAAW,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,YAAY,GAAG,EAAE,CAAC;;qBAE3C,YAAY,GAAG,IAAI,CAAC,KAAK,CAAC,YAAY,GAAG,EAAE,CAAC,CAAC;kBAC9C;cACF;UACF;;;;SAMD,IAAI,OAAO,EAAE;aACX,kBAAkB,GAAG,CAAC,CAAC;aACvB,WAAW,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;UACxB;cAAM;aACL,kBAAkB,GAAG,EAAE,CAAC;aACxB,OAAO,CAAC,WAAW,CAAC,KAAK,CAAC,EAAE;iBAC1B,kBAAkB,GAAG,kBAAkB,GAAG,CAAC,CAAC;iBAC5C,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;cACnB;UACF;;SAGD,IAAM,mBAAmB,GAAG,kBAAkB,GAAG,CAAC,GAAG,QAAQ,CAAC;;;;;;;;SAS9D,IAAI,mBAAmB,IAAI,EAAE,IAAI,mBAAmB,IAAI,CAAC,CAAC,IAAI,QAAQ,GAAG,CAAC,EAAE;;;;;aAM1E,IAAI,kBAAkB,GAAG,EAAE,EAAE;iBAC3B,MAAM,CAAC,IAAI,CAAC,KAAG,CAAG,CAAC,CAAC;iBACpB,IAAI,QAAQ,GAAG,CAAC;qBAAE,MAAM,CAAC,IAAI,CAAC,IAAI,GAAG,QAAQ,CAAC,CAAC;sBAC1C,IAAI,QAAQ,GAAG,CAAC;qBAAE,MAAM,CAAC,IAAI,CAAC,GAAG,GAAG,QAAQ,CAAC,CAAC;iBACnD,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;cACxB;aAED,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;aACvC,kBAAkB,GAAG,kBAAkB,GAAG,CAAC,CAAC;aAE5C,IAAI,kBAAkB,EAAE;iBACtB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;cAClB;aAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,EAAE,CAAC,EAAE,EAAE;iBAC3C,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;cACxC;;aAGD,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;aACjB,IAAI,mBAAmB,GAAG,CAAC,EAAE;iBAC3B,MAAM,CAAC,IAAI,CAAC,GAAG,GAAG,mBAAmB,CAAC,CAAC;cACxC;kBAAM;iBACL,MAAM,CAAC,IAAI,CAAC,KAAG,mBAAqB,CAAC,CAAC;cACvC;UACF;cAAM;;aAEL,IAAI,QAAQ,IAAI,CAAC,EAAE;iBACjB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,EAAE,CAAC,EAAE,EAAE;qBAC3C,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;kBACxC;cACF;kBAAM;iBACL,IAAI,cAAc,GAAG,kBAAkB,GAAG,QAAQ,CAAC;;iBAGnD,IAAI,cAAc,GAAG,CAAC,EAAE;qBACtB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,cAAc,EAAE,CAAC,EAAE,EAAE;yBACvC,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;sBACxC;kBACF;sBAAM;qBACL,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;kBAClB;iBAED,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;iBAEjB,OAAO,cAAc,EAAE,GAAG,CAAC,EAAE;qBAC3B,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;kBAClB;iBAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,GAAG,IAAI,CAAC,GAAG,CAAC,cAAc,GAAG,CAAC,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,EAAE;qBAC7E,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;kBACxC;cACF;UACF;SAED,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;MACxB;KAED,2BAAM,GAAN;SACE,OAAO,EAAE,cAAc,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;MAC5C;;KAGD,mCAAc,GAAd;SACE,OAAO,EAAE,cAAc,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;MAC5C;;KAGM,2BAAgB,GAAvB,UAAwB,GAAuB;SAC7C,OAAO,UAAU,CAAC,UAAU,CAAC,GAAG,CAAC,cAAc,CAAC,CAAC;MAClD;;KAGD,qBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,4BAAO,GAAP;SACE,OAAO,sBAAmB,IAAI,CAAC,QAAQ,EAAE,QAAI,CAAC;MAC/C;KACH,iBAAC;CAAD,CAAC,IAAA;CAnoBY,gCAAU;CAqoBvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,YAAY,EAAE,CAAC,CAAC;;;;;;;;;;CClyBlF;;;;CAIA;;;;;;KASE,gBAAY,KAAa;SACvB,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;aAAE,OAAO,IAAI,MAAM,CAAC,KAAK,CAAC,CAAC;SAExD,IAAK,KAAiB,YAAY,MAAM,EAAE;aACxC,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;UACzB;SAED,IAAI,CAAC,KAAK,GAAG,CAAC,KAAK,CAAC;MACrB;;;;;;KAOD,wBAAO,GAAP;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,uBAAM,GAAN;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,+BAAc,GAAd,UAAe,OAAsB;SACnC,IAAI,OAAO,KAAK,OAAO,CAAC,MAAM,KAAK,OAAO,CAAC,OAAO,IAAI,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE;aAC5E,OAAO,IAAI,CAAC,KAAK,CAAC;UACnB;;;SAID,IAAI,MAAM,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,CAAC,EAAE;aACxC,OAAO,EAAE,aAAa,EAAE,MAAI,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAG,EAAE,CAAC;UACvD;SAED,IAAI,aAAqB,CAAC;SAC1B,IAAI,MAAM,CAAC,SAAS,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE;aAChC,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;aACtC,IAAI,aAAa,CAAC,MAAM,IAAI,EAAE,EAAE;iBAC9B,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,aAAa,CAAC,EAAE,CAAC,CAAC,WAAW,EAAE,CAAC;cAC5D;UACF;cAAM;aACL,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,QAAQ,EAAE,CAAC;UACvC;SAED,OAAO,EAAE,aAAa,eAAA,EAAE,CAAC;MAC1B;;KAGM,uBAAgB,GAAvB,UAAwB,GAAmB,EAAE,OAAsB;SACjE,IAAM,WAAW,GAAG,UAAU,CAAC,GAAG,CAAC,aAAa,CAAC,CAAC;SAClD,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,WAAW,GAAG,IAAI,MAAM,CAAC,WAAW,CAAC,CAAC;MAC3E;;KAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,wBAAO,GAAP;SACE,IAAM,KAAK,GAAG,IAAI,CAAC,cAAc,EAAoB,CAAC;SACtD,OAAO,gBAAc,KAAK,CAAC,aAAa,MAAG,CAAC;MAC7C;KACH,aAAC;CAAD,CAAC,IAAA;CAzEY,wBAAM;CA2EnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;CC/E1E;;;;CAIA;;;;;;KASE,eAAY,KAAsB;SAChC,IAAI,EAAE,IAAI,YAAY,KAAK,CAAC;aAAE,OAAO,IAAI,KAAK,CAAC,KAAK,CAAC,CAAC;SAEtD,IAAK,KAAiB,YAAY,MAAM,EAAE;aACxC,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;UACzB;SAED,IAAI,CAAC,KAAK,GAAG,CAAC,KAAK,CAAC;MACrB;;;;;;KAOD,uBAAO,GAAP;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,sBAAM,GAAN;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,8BAAc,GAAd,UAAe,OAAsB;SACnC,IAAI,OAAO,KAAK,OAAO,CAAC,OAAO,IAAI,OAAO,CAAC,MAAM,CAAC;aAAE,OAAO,IAAI,CAAC,KAAK,CAAC;SACtE,OAAO,EAAE,UAAU,EAAE,IAAI,CAAC,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;MAC9C;;KAGM,sBAAgB,GAAvB,UAAwB,GAAkB,EAAE,OAAsB;SAChE,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,QAAQ,CAAC,GAAG,CAAC,UAAU,EAAE,EAAE,CAAC,GAAG,IAAI,KAAK,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC;MAC9F;;KAGD,gBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,uBAAO,GAAP;SACE,OAAO,eAAa,IAAI,CAAC,OAAO,EAAE,MAAG,CAAC;MACvC;KACH,YAAC;CAAD,CAAC,IAAA;CApDY,sBAAK;CAsDlB,MAAM,CAAC,cAAc,CAAC,KAAK,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,OAAO,EAAE,CAAC,CAAC;;;;;;;;;;CC5DxE;;;;CAIA;KAGE;SACE,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;aAAE,OAAO,IAAI,MAAM,EAAE,CAAC;MACpD;;KAGD,+BAAc,GAAd;SACE,OAAO,EAAE,OAAO,EAAE,CAAC,EAAE,CAAC;MACvB;;KAGM,uBAAgB,GAAvB;SACE,OAAO,IAAI,MAAM,EAAE,CAAC;MACrB;;KAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,wBAAO,GAAP;SACE,OAAO,cAAc,CAAC;MACvB;KACH,aAAC;CAAD,CAAC,IAAA;CAzBY,wBAAM;CA2BnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;CC/B1E;;;;CAIA;KAGE;SACE,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;aAAE,OAAO,IAAI,MAAM,EAAE,CAAC;MACpD;;KAGD,+BAAc,GAAd;SACE,OAAO,EAAE,OAAO,EAAE,CAAC,EAAE,CAAC;MACvB;;KAGM,uBAAgB,GAAvB;SACE,OAAO,IAAI,MAAM,EAAE,CAAC;MACrB;;KAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,wBAAO,GAAP;SACE,OAAO,cAAc,CAAC;MACvB;KACH,aAAC;CAAD,CAAC,IAAA;CAzBY,wBAAM;CA2BnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;ACpC1C;AACe;AACuB;CAEtE;CACA,IAAM,iBAAiB,GAAG,IAAI,MAAM,CAAC,mBAAmB,CAAC,CAAC;CAE1D;CACA,IAAI,cAAc,GAAsB,IAAI,CAAC;CAc7C,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;CAEzB;;;;CAIA;;;;;;KAkBE,kBAAY,EAAuD;SACjE,IAAI,EAAE,IAAI,YAAY,QAAQ,CAAC;aAAE,OAAO,IAAI,QAAQ,CAAC,EAAE,CAAC,CAAC;;SAGzD,IAAI,EAAE,YAAY,QAAQ,EAAE;aAC1B,IAAI,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,CAAC;aAClB,IAAI,CAAC,IAAI,GAAG,EAAE,CAAC,IAAI,CAAC;UACrB;SAED,IAAI,OAAO,EAAE,KAAK,QAAQ,IAAI,EAAE,IAAI,IAAI,IAAI,EAAE,EAAE;aAC9C,IAAI,aAAa,IAAI,EAAE,IAAI,OAAO,EAAE,CAAC,WAAW,KAAK,UAAU,EAAE;iBAC/D,IAAI,CAAC,GAAG,CAAC,GAAGuX,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,EAAE,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM;iBACL,IAAI,CAAC,GAAG,CAAC,GAAG,OAAO,EAAE,CAAC,EAAE,KAAK,QAAQ,GAAGA,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,EAAE,CAAC,EAAE,CAAC;cACpE;UACF;;SAGD,IAAI,EAAE,IAAI,IAAI,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;;aAExC,IAAI,CAAC,GAAG,CAAC,GAAG,QAAQ,CAAC,QAAQ,CAAC,OAAO,EAAE,KAAK,QAAQ,GAAG,EAAE,GAAG,SAAS,CAAC,CAAC;;aAEvE,IAAI,QAAQ,CAAC,cAAc,EAAE;iBAC3B,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;cACrC;UACF;SAED,IAAI,WAAW,CAAC,MAAM,CAAC,EAAE,CAAC,IAAI,EAAE,CAAC,UAAU,KAAK,EAAE,EAAE;aAClD,IAAI,CAAC,GAAG,CAAC,GAAGE,0BAAY,CAAC,EAAE,CAAC,CAAC;UAC9B;SAED,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;aAC1B,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,EAAE;iBACpB,IAAM,KAAK,GAAGF,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;iBAC9B,IAAI,KAAK,CAAC,UAAU,KAAK,EAAE,EAAE;qBAC3B,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;kBACnB;cACF;kBAAM,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE;iBACzD,IAAI,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,IAAI,CAAC,EAAE,EAAE,KAAK,CAAC,CAAC;cACpC;kBAAM;iBACL,MAAM,IAAI,SAAS,CACjB,4FAA4F,CAC7F,CAAC;cACH;UACF;SAED,IAAI,QAAQ,CAAC,cAAc,EAAE;aAC3B,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;UACrC;MACF;KAMD,sBAAI,wBAAE;;;;;cAAN;aACE,OAAO,IAAI,CAAC,GAAG,CAAC,CAAC;UAClB;cAED,UAAO,KAAa;aAClB,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;aAClB,IAAI,QAAQ,CAAC,cAAc,EAAE;iBAC3B,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;cACnC;UACF;;;QAPA;KAaD,sBAAI,oCAAc;;;;;cAAlB;aACE,OAAO,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,CAAC,CAAC,CAAC;UAC/B;cAED,UAAmB,KAAa;;aAE9B,IAAI,CAAC,EAAE,CAAC,aAAa,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC;UACjC;;;QALA;;KAQD,8BAAW,GAAX;SACE,IAAI,QAAQ,CAAC,cAAc,IAAI,IAAI,CAAC,IAAI,EAAE;aACxC,OAAO,IAAI,CAAC,IAAI,CAAC;UAClB;SAED,IAAM,SAAS,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;SAE1C,IAAI,QAAQ,CAAC,cAAc,IAAI,CAAC,IAAI,CAAC,IAAI,EAAE;aACzC,IAAI,CAAC,IAAI,GAAG,SAAS,CAAC;UACvB;SAED,OAAO,SAAS,CAAC;MAClB;;;;;;;KAQM,eAAM,GAAb;SACE,QAAQ,QAAQ,CAAC,KAAK,GAAG,CAAC,QAAQ,CAAC,KAAK,GAAG,CAAC,IAAI,QAAQ,EAAE;MAC3D;;;;;;KAOM,iBAAQ,GAAf,UAAgB,IAAa;SAC3B,IAAI,QAAQ,KAAK,OAAO,IAAI,EAAE;aAC5B,IAAI,GAAG,CAAC,EAAE,IAAI,CAAC,GAAG,EAAE,GAAG,IAAI,CAAC,CAAC;UAC9B;SAED,IAAM,GAAG,GAAG,QAAQ,CAAC,MAAM,EAAE,CAAC;SAC9B,IAAMvX,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;;SAGhCvX,QAAM,CAAC,aAAa,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;;SAG9B,IAAI,cAAc,KAAK,IAAI,EAAE;aAC3B,cAAc,GAAGwX,iBAAW,CAAC,CAAC,CAAC,CAAC;UACjC;;SAGDxX,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;SAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;SAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;SAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;SAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;;SAG9BA,QAAM,CAAC,EAAE,CAAC,GAAG,GAAG,GAAG,IAAI,CAAC;SACxBA,QAAM,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;SAC/BA,QAAM,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;SAE/B,OAAOA,QAAM,CAAC;MACf;;;;;;;KAQD,2BAAQ,GAAR,UAAS,MAAe;;SAEtB,IAAI,MAAM;aAAE,OAAO,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC;SAC5C,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;MAC3B;;;;;KAMD,yBAAM,GAAN;SACE,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;MAC3B;;;;;;KAOD,yBAAM,GAAN,UAAO,OAAyC;SAC9C,IAAI,OAAO,KAAK,SAAS,IAAI,OAAO,KAAK,IAAI,EAAE;aAC7C,OAAO,KAAK,CAAC;UACd;SAED,IAAI,OAAO,YAAY,QAAQ,EAAE;aAC/B,OAAO,IAAI,CAAC,QAAQ,EAAE,KAAK,OAAO,CAAC,QAAQ,EAAE,CAAC;UAC/C;SAED,IACE,OAAO,OAAO,KAAK,QAAQ;aAC3B,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC;aACzB,OAAO,CAAC,MAAM,KAAK,EAAE;aACrBwX,kBAAY,CAAC,IAAI,CAAC,EAAE,CAAC,EACrB;aACA,OAAO,OAAO,KAAKD,aAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,IAAI,CAAC,EAAE,EAAE,QAAQ,CAAC,CAAC;UACtE;SAED,IAAI,OAAO,OAAO,KAAK,QAAQ,IAAI,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC,IAAI,OAAO,CAAC,MAAM,KAAK,EAAE,EAAE;aACrF,OAAO,OAAO,CAAC,WAAW,EAAE,KAAK,IAAI,CAAC,WAAW,EAAE,CAAC;UACrD;SAED,IAAI,OAAO,OAAO,KAAK,QAAQ,IAAI,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC,IAAI,OAAO,CAAC,MAAM,KAAK,EAAE,EAAE;aACrF,OAAOA,aAAM,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;UAC7C;SAED,IACE,OAAO,OAAO,KAAK,QAAQ;aAC3B,aAAa,IAAI,OAAO;aACxB,OAAO,OAAO,CAAC,WAAW,KAAK,UAAU,EACzC;aACA,OAAO,OAAO,CAAC,WAAW,EAAE,KAAK,IAAI,CAAC,WAAW,EAAE,CAAC;UACrD;SAED,OAAO,KAAK,CAAC;MACd;;KAGD,+BAAY,GAAZ;SACE,IAAM,SAAS,GAAG,IAAI,IAAI,EAAE,CAAC;SAC7B,IAAM,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,YAAY,CAAC,CAAC,CAAC,CAAC;SACrC,SAAS,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,CAAC;SAC3C,OAAO,SAAS,CAAC;MAClB;;KAGM,iBAAQ,GAAf;SACE,OAAO,IAAI,QAAQ,EAAE,CAAC;MACvB;;;;;;KAOM,uBAAc,GAArB,UAAsB,IAAY;SAChC,IAAMvX,QAAM,GAAGuX,aAAM,CAAC,IAAI,CAAC,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;;SAEjEvX,QAAM,CAAC,aAAa,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;;SAE9B,OAAO,IAAI,QAAQ,CAACA,QAAM,CAAC,CAAC;MAC7B;;;;;;KAOM,4BAAmB,GAA1B,UAA2B,SAAiB;;SAE1C,IAAI,OAAO,SAAS,KAAK,WAAW,KAAK,SAAS,IAAI,IAAI,IAAI,SAAS,CAAC,MAAM,KAAK,EAAE,CAAC,EAAE;aACtF,MAAM,IAAI,SAAS,CACjB,yFAAyF,CAC1F,CAAC;UACH;SAED,OAAO,IAAI,QAAQ,CAACuX,aAAM,CAAC,IAAI,CAAC,SAAS,EAAE,KAAK,CAAC,CAAC,CAAC;MACpD;;;;;;KAOM,gBAAO,GAAd,UAAe,EAA0D;SACvE,IAAI,EAAE,IAAI,IAAI;aAAE,OAAO,KAAK,CAAC;SAE7B,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;aAC1B,OAAO,IAAI,CAAC;UACb;SAED,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;aAC1B,OAAO,EAAE,CAAC,MAAM,KAAK,EAAE,KAAK,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC;UAC7E;SAED,IAAI,EAAE,YAAY,QAAQ,EAAE;aAC1B,OAAO,IAAI,CAAC;UACb;SAED,IAAIC,kBAAY,CAAC,EAAE,CAAC,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,EAAE;aACxC,OAAO,IAAI,CAAC;UACb;;SAGD,IAAI,OAAO,EAAE,KAAK,QAAQ,IAAI,aAAa,IAAI,EAAE,IAAI,OAAO,EAAE,CAAC,WAAW,KAAK,UAAU,EAAE;aACzF,IAAI,OAAO,EAAE,CAAC,EAAE,KAAK,QAAQ,EAAE;iBAC7B,OAAO,EAAE,CAAC,EAAE,CAAC,MAAM,KAAK,EAAE,CAAC;cAC5B;aACD,OAAO,EAAE,CAAC,WAAW,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC;UACxF;SAED,OAAO,KAAK,CAAC;MACd;;KAGD,iCAAc,GAAd;SACE,IAAI,IAAI,CAAC,WAAW;aAAE,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,WAAW,EAAE,EAAE,CAAC;SAC1D,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,EAAE,CAAC;MACvC;;KAGM,yBAAgB,GAAvB,UAAwB,GAAqB;SAC3C,OAAO,IAAI,QAAQ,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;MAC/B;;;;;;;KAQD,mBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,0BAAO,GAAP;SACE,OAAO,oBAAiB,IAAI,CAAC,WAAW,EAAE,QAAI,CAAC;MAChD;;KA5TM,cAAK,GAAG,CAAC,EAAE,IAAI,CAAC,MAAM,EAAE,GAAG,QAAQ,CAAC,CAAC;KA6T9C,eAAC;EAjUD,IAiUC;CAjUY,4BAAQ;CAmUrB;CACA,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,UAAU,EAAE;KACpD,KAAK,EAAEA,eAAS,CACd,UAAC,IAAY,IAAK,OAAA,QAAQ,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAA,EACzC,yDAAyD,CAC1D;EACF,CAAC,CAAC;CAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,QAAQ,EAAE;KAClD,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;EAC/F,CAAC,CAAC;CAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,SAAS,EAAE;KACnD,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;EAC/F,CAAC,CAAC;CAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,EAAE,SAAS,EAAE;KACzC,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;EAC/F,CAAC,CAAC;CAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,UAAU,EAAE,CAAC,CAAC;;;;;;;;;;CCjX9E,SAAS,WAAW,CAAC,GAAW;KAC9B,OAAO,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;CACvC,CAAC;CAgBD;;;;CAIA;;;;;KASE,oBAAY,OAAe,EAAE,OAAgB;SAC3C,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;aAAE,OAAO,IAAI,UAAU,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;SAE3E,IAAI,CAAC,OAAO,GAAG,OAAO,CAAC;SACvB,IAAI,CAAC,OAAO,GAAG,WAAW,CAAC,OAAO,aAAP,OAAO,cAAP,OAAO,GAAI,EAAE,CAAC,CAAC;;SAG1C,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,OAAO,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;aAC5C,IACE,EACE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;iBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;iBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;iBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;iBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;iBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG,CACxB,EACD;iBACA,MAAM,IAAI,KAAK,CAAC,oCAAkC,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,uBAAoB,CAAC,CAAC;cACxF;UACF;MACF;KAEM,uBAAY,GAAnB,UAAoB,OAAgB;SAClC,OAAO,OAAO,GAAG,OAAO,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,GAAG,EAAE,CAAC;MACzD;;KAGD,mCAAc,GAAd,UAAe,OAAsB;SACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,IAAI,OAAO,CAAC,MAAM,EAAE;aAClB,OAAO,EAAE,MAAM,EAAE,IAAI,CAAC,OAAO,EAAE,QAAQ,EAAE,IAAI,CAAC,OAAO,EAAE,CAAC;UACzD;SACD,OAAO,EAAE,kBAAkB,EAAE,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,EAAE,CAAC;MACjF;;KAGM,2BAAgB,GAAvB,UAAwB,GAAkD;SACxE,IAAI,QAAQ,IAAI,GAAG,EAAE;aACnB,IAAI,OAAO,GAAG,CAAC,MAAM,KAAK,QAAQ,EAAE;;iBAElC,IAAI,GAAG,CAAC,MAAM,CAAC,SAAS,KAAK,YAAY,EAAE;qBACzC,OAAQ,GAA6B,CAAC;kBACvC;cACF;kBAAM;iBACL,OAAO,IAAI,UAAU,CAAC,GAAG,CAAC,MAAM,EAAE,UAAU,CAAC,YAAY,CAAC,GAAG,CAAC,QAAQ,CAAC,CAAC,CAAC;cAC1E;UACF;SACD,IAAI,oBAAoB,IAAI,GAAG,EAAE;aAC/B,OAAO,IAAI,UAAU,CACnB,GAAG,CAAC,kBAAkB,CAAC,OAAO,EAC9B,UAAU,CAAC,YAAY,CAAC,GAAG,CAAC,kBAAkB,CAAC,OAAO,CAAC,CACxD,CAAC;UACH;SACD,MAAM,IAAI,SAAS,CAAC,8CAA4C,IAAI,CAAC,SAAS,CAAC,GAAG,CAAG,CAAC,CAAC;MACxF;KACH,iBAAC;CAAD,CAAC,IAAA;CAjEY,gCAAU;CAmEvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,YAAY,EAAE,CAAC,CAAC;;;;;;;;;;CCtFlF;;;;CAIA;;;;KAOE,oBAAY,KAAa;SACvB,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;aAAE,OAAO,IAAI,UAAU,CAAC,KAAK,CAAC,CAAC;SAEhE,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;MACpB;;KAGD,4BAAO,GAAP;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,6BAAQ,GAAR;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,4BAAO,GAAP;SACE,OAAO,sBAAmB,IAAI,CAAC,KAAK,QAAI,CAAC;MAC1C;;KAGD,2BAAM,GAAN;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,mCAAc,GAAd;SACE,OAAO,EAAE,OAAO,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;MAChC;;KAGM,2BAAgB,GAAvB,UAAwB,GAAuB;SAC7C,OAAO,IAAI,UAAU,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;MACpC;;KAGD,qBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KACH,iBAAC;CAAD,CAAC,IAAA;CA/CY,gCAAU;CAiDvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;CC1D9E;CACA;AACA;CACA;CACA;AACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;CACA;CAEA,IAAIK,cAAa,GAAG,uBAASlX,CAAT,EAAYoD,CAAZ,EAAe;CAC/B8T,EAAAA,cAAa,GAAGxV,MAAM,CAACC,cAAP,IACX;CAAEwV,IAAAA,SAAS,EAAE;CAAb,eAA6B5Z,KAA7B,IAAsC,UAAUyC,CAAV,EAAaoD,CAAb,EAAgB;CAAEpD,IAAAA,CAAC,CAACmX,SAAF,GAAc/T,CAAd;CAAkB,GAD/D,IAEZ,UAAUpD,CAAV,EAAaoD,CAAb,EAAgB;CAAE,SAAK,IAAIgU,CAAT,IAAchU,CAAd;CAAiB,UAAIA,CAAC,CAAC2R,cAAF,CAAiBqC,CAAjB,CAAJ,EAAyBpX,CAAC,CAACoX,CAAD,CAAD,GAAOhU,CAAC,CAACgU,CAAD,CAAR;CAA1C;CAAwD,GAF9E;;CAGA,SAAOF,cAAa,CAAClX,CAAD,EAAIoD,CAAJ,CAApB;CACH,CALD;;CAOO,SAASiU,SAAT,CAAmBrX,CAAnB,EAAsBoD,CAAtB,EAAyB;CAC5B8T,EAAAA,cAAa,CAAClX,CAAD,EAAIoD,CAAJ,CAAb;;CACA,WAASkU,EAAT,GAAc;CAAE,SAAKjI,WAAL,GAAmBrP,CAAnB;CAAuB;;CACvCA,EAAAA,CAAC,CAAC4B,SAAF,GAAcwB,CAAC,KAAK,IAAN,GAAa1B,MAAM,CAAC0O,MAAP,CAAchN,CAAd,CAAb,IAAiCkU,EAAE,CAAC1V,SAAH,GAAewB,CAAC,CAACxB,SAAjB,EAA4B,IAAI0V,EAAJ,EAA7D,CAAd;CACH;;CAEM,IAAIC,OAAQ,GAAG,oBAAW;CAC7BA,EAAAA,OAAQ,GAAG7V,MAAM,CAAC8V,MAAP,IAAiB,SAASD,QAAT,CAAkBE,CAAlB,EAAqB;CAC7C,SAAK,IAAIxX,CAAJ,EAAOxC,CAAC,GAAG,CAAX,EAAc8I,CAAC,GAAGZ,SAAS,CAAChI,MAAjC,EAAyCF,CAAC,GAAG8I,CAA7C,EAAgD9I,CAAC,EAAjD,EAAqD;CACjDwC,MAAAA,CAAC,GAAG0F,SAAS,CAAClI,CAAD,CAAb;;CACA,WAAK,IAAI2Z,CAAT,IAAcnX,CAAd;CAAiB,YAAIyB,MAAM,CAACE,SAAP,CAAiBmT,cAAjB,CAAgCtP,IAAhC,CAAqCxF,CAArC,EAAwCmX,CAAxC,CAAJ,EAAgDK,CAAC,CAACL,CAAD,CAAD,GAAOnX,CAAC,CAACmX,CAAD,CAAR;CAAjE;CACH;;CACD,WAAOK,CAAP;CACH,GAND;;CAOA,SAAOF,OAAQ,CAAC5Q,KAAT,CAAe,IAAf,EAAqBhB,SAArB,CAAP;CACH,CATM;CAWA,SAAS+R,MAAT,CAAgBzX,CAAhB,EAAmBP,CAAnB,EAAsB;CACzB,MAAI+X,CAAC,GAAG,EAAR;;CACA,OAAK,IAAIL,CAAT,IAAcnX,CAAd;CAAiB,QAAIyB,MAAM,CAACE,SAAP,CAAiBmT,cAAjB,CAAgCtP,IAAhC,CAAqCxF,CAArC,EAAwCmX,CAAxC,KAA8C1X,CAAC,CAACzB,OAAF,CAAUmZ,CAAV,IAAe,CAAjE,EACbK,CAAC,CAACL,CAAD,CAAD,GAAOnX,CAAC,CAACmX,CAAD,CAAR;CADJ;;CAEA,MAAInX,CAAC,IAAI,IAAL,IAAa,OAAOyB,MAAM,CAACiW,qBAAd,KAAwC,UAAzD,EACI,KAAK,IAAIla,CAAC,GAAG,CAAR,EAAW2Z,CAAC,GAAG1V,MAAM,CAACiW,qBAAP,CAA6B1X,CAA7B,CAApB,EAAqDxC,CAAC,GAAG2Z,CAAC,CAACzZ,MAA3D,EAAmEF,CAAC,EAApE,EAAwE;CACpE,QAAIiC,CAAC,CAACzB,OAAF,CAAUmZ,CAAC,CAAC3Z,CAAD,CAAX,IAAkB,CAAlB,IAAuBiE,MAAM,CAACE,SAAP,CAAiBgW,oBAAjB,CAAsCnS,IAAtC,CAA2CxF,CAA3C,EAA8CmX,CAAC,CAAC3Z,CAAD,CAA/C,CAA3B,EACIga,CAAC,CAACL,CAAC,CAAC3Z,CAAD,CAAF,CAAD,GAAUwC,CAAC,CAACmX,CAAC,CAAC3Z,CAAD,CAAF,CAAX;CACP;CACL,SAAOga,CAAP;CACH;CAEM,SAASI,UAAT,CAAoBC,UAApB,EAAgC1Q,MAAhC,EAAwCoN,GAAxC,EAA6CS,IAA7C,EAAmD;CACtD,MAAI1U,CAAC,GAAGoF,SAAS,CAAChI,MAAlB;CAAA,MAA0Boa,CAAC,GAAGxX,CAAC,GAAG,CAAJ,GAAQ6G,MAAR,GAAiB6N,IAAI,KAAK,IAAT,GAAgBA,IAAI,GAAGvT,MAAM,CAACwT,wBAAP,CAAgC9N,MAAhC,EAAwCoN,GAAxC,CAAvB,GAAsES,IAArH;CAAA,MAA2HjV,CAA3H;CACA,MAAI,QAAOgY,OAAP,wDAAOA,OAAP,OAAmB,QAAnB,IAA+B,OAAOA,OAAO,CAACC,QAAf,KAA4B,UAA/D,EAA2EF,CAAC,GAAGC,OAAO,CAACC,QAAR,CAAiBH,UAAjB,EAA6B1Q,MAA7B,EAAqCoN,GAArC,EAA0CS,IAA1C,CAAJ,CAA3E,KACK,KAAK,IAAIxX,CAAC,GAAGqa,UAAU,CAACna,MAAX,GAAoB,CAAjC,EAAoCF,CAAC,IAAI,CAAzC,EAA4CA,CAAC,EAA7C;CAAiD,QAAIuC,CAAC,GAAG8X,UAAU,CAACra,CAAD,CAAlB,EAAuBsa,CAAC,GAAG,CAACxX,CAAC,GAAG,CAAJ,GAAQP,CAAC,CAAC+X,CAAD,CAAT,GAAexX,CAAC,GAAG,CAAJ,GAAQP,CAAC,CAACoH,MAAD,EAASoN,GAAT,EAAcuD,CAAd,CAAT,GAA4B/X,CAAC,CAACoH,MAAD,EAASoN,GAAT,CAA7C,KAA+DuD,CAAnE;CAAxE;CACL,SAAOxX,CAAC,GAAG,CAAJ,IAASwX,CAAT,IAAcrW,MAAM,CAACG,cAAP,CAAsBuF,MAAtB,EAA8BoN,GAA9B,EAAmCuD,CAAnC,CAAd,EAAqDA,CAA5D;CACH;CAEM,SAASG,OAAT,CAAiBC,UAAjB,EAA6BC,SAA7B,EAAwC;CAC3C,SAAO,UAAUhR,MAAV,EAAkBoN,GAAlB,EAAuB;CAAE4D,IAAAA,SAAS,CAAChR,MAAD,EAASoN,GAAT,EAAc2D,UAAd,CAAT;CAAqC,GAArE;CACH;CAEM,SAASE,UAAT,CAAoBC,WAApB,EAAiCC,aAAjC,EAAgD;CACnD,MAAI,QAAOP,OAAP,wDAAOA,OAAP,OAAmB,QAAnB,IAA+B,OAAOA,OAAO,CAACQ,QAAf,KAA4B,UAA/D,EAA2E,OAAOR,OAAO,CAACQ,QAAR,CAAiBF,WAAjB,EAA8BC,aAA9B,CAAP;CAC9E;CAEM,SAASE,SAAT,CAAmBC,OAAnB,EAA4BC,UAA5B,EAAwCC,CAAxC,EAA2CC,SAA3C,EAAsD;CACzD,WAASC,KAAT,CAAexY,KAAf,EAAsB;CAAE,WAAOA,KAAK,YAAYsY,CAAjB,GAAqBtY,KAArB,GAA6B,IAAIsY,CAAJ,CAAM,UAAUG,OAAV,EAAmB;CAAEA,MAAAA,OAAO,CAACzY,KAAD,CAAP;CAAiB,KAA5C,CAApC;CAAoF;;CAC5G,SAAO,KAAKsY,CAAC,KAAKA,CAAC,GAAGI,OAAT,CAAN,EAAyB,UAAUD,OAAV,EAAmBE,MAAnB,EAA2B;CACvD,aAASC,SAAT,CAAmB5Y,KAAnB,EAA0B;CAAE,UAAI;CAAE6Y,QAAAA,IAAI,CAACN,SAAS,CAACO,IAAV,CAAe9Y,KAAf,CAAD,CAAJ;CAA8B,OAApC,CAAqC,OAAOZ,CAAP,EAAU;CAAEuZ,QAAAA,MAAM,CAACvZ,CAAD,CAAN;CAAY;CAAE;;CAC3F,aAAS2Z,QAAT,CAAkB/Y,KAAlB,EAAyB;CAAE,UAAI;CAAE6Y,QAAAA,IAAI,CAACN,SAAS,CAAC,OAAD,CAAT,CAAmBvY,KAAnB,CAAD,CAAJ;CAAkC,OAAxC,CAAyC,OAAOZ,CAAP,EAAU;CAAEuZ,QAAAA,MAAM,CAACvZ,CAAD,CAAN;CAAY;CAAE;;CAC9F,aAASyZ,IAAT,CAAcG,MAAd,EAAsB;CAAEA,MAAAA,MAAM,CAACC,IAAP,GAAcR,OAAO,CAACO,MAAM,CAAChZ,KAAR,CAArB,GAAsCwY,KAAK,CAACQ,MAAM,CAAChZ,KAAR,CAAL,CAAoBkZ,IAApB,CAAyBN,SAAzB,EAAoCG,QAApC,CAAtC;CAAsF;;CAC9GF,IAAAA,IAAI,CAAC,CAACN,SAAS,GAAGA,SAAS,CAAClS,KAAV,CAAgB+R,OAAhB,EAAyBC,UAAU,IAAI,EAAvC,CAAb,EAAyDS,IAAzD,EAAD,CAAJ;CACH,GALM,CAAP;CAMH;CAEM,SAASK,WAAT,CAAqBf,OAArB,EAA8BgB,IAA9B,EAAoC;CACvC,MAAIvI,CAAC,GAAG;CAAEwI,IAAAA,KAAK,EAAE,CAAT;CAAYC,IAAAA,IAAI,EAAE,gBAAW;CAAE,UAAInC,CAAC,CAAC,CAAD,CAAD,GAAO,CAAX,EAAc,MAAMA,CAAC,CAAC,CAAD,CAAP;CAAY,aAAOA,CAAC,CAAC,CAAD,CAAR;CAAc,KAAvE;CAAyEoC,IAAAA,IAAI,EAAE,EAA/E;CAAmFC,IAAAA,GAAG,EAAE;CAAxF,GAAR;CAAA,MAAsGjJ,CAAtG;CAAA,MAAyG5L,CAAzG;CAAA,MAA4GwS,CAA5G;CAAA,MAA+GsC,CAA/G;CACA,SAAOA,CAAC,GAAG;CAAEX,IAAAA,IAAI,EAAEY,IAAI,CAAC,CAAD,CAAZ;CAAiB,aAASA,IAAI,CAAC,CAAD,CAA9B;CAAmC,cAAUA,IAAI,CAAC,CAAD;CAAjD,GAAJ,EAA4D,OAAOjZ,MAAP,KAAkB,UAAlB,KAAiCgZ,CAAC,CAAChZ,MAAM,CAACkZ,QAAR,CAAD,GAAqB,YAAW;CAAE,WAAO,IAAP;CAAc,GAAjF,CAA5D,EAAgJF,CAAvJ;;CACA,WAASC,IAAT,CAAczT,CAAd,EAAiB;CAAE,WAAO,UAAU2T,CAAV,EAAa;CAAE,aAAOf,IAAI,CAAC,CAAC5S,CAAD,EAAI2T,CAAJ,CAAD,CAAX;CAAsB,KAA5C;CAA+C;;CAClE,WAASf,IAAT,CAAcgB,EAAd,EAAkB;CACd,QAAItJ,CAAJ,EAAO,MAAM,IAAIrO,SAAJ,CAAc,iCAAd,CAAN;;CACP,WAAO2O,CAAP;CAAU,UAAI;CACV,YAAIN,CAAC,GAAG,CAAJ,EAAO5L,CAAC,KAAKwS,CAAC,GAAG0C,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAR,GAAYlV,CAAC,CAAC,QAAD,CAAb,GAA0BkV,EAAE,CAAC,CAAD,CAAF,GAAQlV,CAAC,CAAC,OAAD,CAAD,KAAe,CAACwS,CAAC,GAAGxS,CAAC,CAAC,QAAD,CAAN,KAAqBwS,CAAC,CAAChS,IAAF,CAAOR,CAAP,CAArB,EAAgC,CAA/C,CAAR,GAA4DA,CAAC,CAACmU,IAAjG,CAAD,IAA2G,CAAC,CAAC3B,CAAC,GAAGA,CAAC,CAAChS,IAAF,CAAOR,CAAP,EAAUkV,EAAE,CAAC,CAAD,CAAZ,CAAL,EAAuBZ,IAA9I,EAAoJ,OAAO9B,CAAP;CACpJ,YAAIxS,CAAC,GAAG,CAAJ,EAAOwS,CAAX,EAAc0C,EAAE,GAAG,CAACA,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAT,EAAY1C,CAAC,CAACnX,KAAd,CAAL;;CACd,gBAAQ6Z,EAAE,CAAC,CAAD,CAAV;CACI,eAAK,CAAL;CAAQ,eAAK,CAAL;CAAQ1C,YAAAA,CAAC,GAAG0C,EAAJ;CAAQ;;CACxB,eAAK,CAAL;CAAQhJ,YAAAA,CAAC,CAACwI,KAAF;CAAW,mBAAO;CAAErZ,cAAAA,KAAK,EAAE6Z,EAAE,CAAC,CAAD,CAAX;CAAgBZ,cAAAA,IAAI,EAAE;CAAtB,aAAP;;CACnB,eAAK,CAAL;CAAQpI,YAAAA,CAAC,CAACwI,KAAF;CAAW1U,YAAAA,CAAC,GAAGkV,EAAE,CAAC,CAAD,CAAN;CAAWA,YAAAA,EAAE,GAAG,CAAC,CAAD,CAAL;CAAU;;CACxC,eAAK,CAAL;CAAQA,YAAAA,EAAE,GAAGhJ,CAAC,CAAC2I,GAAF,CAAMpF,GAAN,EAAL;;CAAkBvD,YAAAA,CAAC,CAAC0I,IAAF,CAAOnF,GAAP;;CAAc;;CACxC;CACI,gBAAI,EAAE+C,CAAC,GAAGtG,CAAC,CAAC0I,IAAN,EAAYpC,CAAC,GAAGA,CAAC,CAAC9Z,MAAF,GAAW,CAAX,IAAgB8Z,CAAC,CAACA,CAAC,CAAC9Z,MAAF,GAAW,CAAZ,CAAnC,MAAuDwc,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,IAAeA,EAAE,CAAC,CAAD,CAAF,KAAU,CAAhF,CAAJ,EAAwF;CAAEhJ,cAAAA,CAAC,GAAG,CAAJ;CAAO;CAAW;;CAC5G,gBAAIgJ,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,KAAgB,CAAC1C,CAAD,IAAO0C,EAAE,CAAC,CAAD,CAAF,GAAQ1C,CAAC,CAAC,CAAD,CAAT,IAAgB0C,EAAE,CAAC,CAAD,CAAF,GAAQ1C,CAAC,CAAC,CAAD,CAAhD,CAAJ,EAA2D;CAAEtG,cAAAA,CAAC,CAACwI,KAAF,GAAUQ,EAAE,CAAC,CAAD,CAAZ;CAAiB;CAAQ;;CACtF,gBAAIA,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,IAAehJ,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAA9B,EAAmC;CAAEtG,cAAAA,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAAX;CAAgBA,cAAAA,CAAC,GAAG0C,EAAJ;CAAQ;CAAQ;;CACrE,gBAAI1C,CAAC,IAAItG,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAApB,EAAyB;CAAEtG,cAAAA,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAAX;;CAAgBtG,cAAAA,CAAC,CAAC2I,GAAF,CAAM/a,IAAN,CAAWob,EAAX;;CAAgB;CAAQ;;CACnE,gBAAI1C,CAAC,CAAC,CAAD,CAAL,EAAUtG,CAAC,CAAC2I,GAAF,CAAMpF,GAAN;;CACVvD,YAAAA,CAAC,CAAC0I,IAAF,CAAOnF,GAAP;;CAAc;CAXtB;;CAaAyF,QAAAA,EAAE,GAAGT,IAAI,CAACjU,IAAL,CAAUiT,OAAV,EAAmBvH,CAAnB,CAAL;CACH,OAjBS,CAiBR,OAAOzR,CAAP,EAAU;CAAEya,QAAAA,EAAE,GAAG,CAAC,CAAD,EAAIza,CAAJ,CAAL;CAAauF,QAAAA,CAAC,GAAG,CAAJ;CAAQ,OAjBzB,SAiBkC;CAAE4L,QAAAA,CAAC,GAAG4G,CAAC,GAAG,CAAR;CAAY;CAjB1D;;CAkBA,QAAI0C,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAZ,EAAe,MAAMA,EAAE,CAAC,CAAD,CAAR;CAAa,WAAO;CAAE7Z,MAAAA,KAAK,EAAE6Z,EAAE,CAAC,CAAD,CAAF,GAAQA,EAAE,CAAC,CAAD,CAAV,GAAgB,KAAK,CAA9B;CAAiCZ,MAAAA,IAAI,EAAE;CAAvC,KAAP;CAC/B;CACJ;CAEM,SAASa,eAAT,CAAyBrE,CAAzB,EAA4BpW,CAA5B,EAA+B0a,CAA/B,EAAkCC,EAAlC,EAAsC;CACzC,MAAIA,EAAE,KAAKrY,SAAX,EAAsBqY,EAAE,GAAGD,CAAL;CACtBtE,EAAAA,CAAC,CAACuE,EAAD,CAAD,GAAQ3a,CAAC,CAAC0a,CAAD,CAAT;CACH;CAEM,SAASE,YAAT,CAAsB5a,CAAtB,EAAyBqB,OAAzB,EAAkC;CACrC,OAAK,IAAIoW,CAAT,IAAczX,CAAd;CAAiB,QAAIyX,CAAC,KAAK,SAAN,IAAmB,CAACpW,OAAO,CAAC+T,cAAR,CAAuBqC,CAAvB,CAAxB,EAAmDpW,OAAO,CAACoW,CAAD,CAAP,GAAazX,CAAC,CAACyX,CAAD,CAAd;CAApE;CACH;CAEM,SAASoD,QAAT,CAAkBzE,CAAlB,EAAqB;CACxB,MAAI9V,CAAC,GAAG,OAAOc,MAAP,KAAkB,UAAlB,IAAgCA,MAAM,CAACkZ,QAA/C;CAAA,MAAyDta,CAAC,GAAGM,CAAC,IAAI8V,CAAC,CAAC9V,CAAD,CAAnE;CAAA,MAAwExC,CAAC,GAAG,CAA5E;CACA,MAAIkC,CAAJ,EAAO,OAAOA,CAAC,CAAC8F,IAAF,CAAOsQ,CAAP,CAAP;CACP,MAAIA,CAAC,IAAI,OAAOA,CAAC,CAACpY,MAAT,KAAoB,QAA7B,EAAuC,OAAO;CAC1Cyb,IAAAA,IAAI,EAAE,gBAAY;CACd,UAAIrD,CAAC,IAAItY,CAAC,IAAIsY,CAAC,CAACpY,MAAhB,EAAwBoY,CAAC,GAAG,KAAK,CAAT;CACxB,aAAO;CAAEzV,QAAAA,KAAK,EAAEyV,CAAC,IAAIA,CAAC,CAACtY,CAAC,EAAF,CAAf;CAAsB8b,QAAAA,IAAI,EAAE,CAACxD;CAA7B,OAAP;CACH;CAJyC,GAAP;CAMvC,QAAM,IAAIvT,SAAJ,CAAcvC,CAAC,GAAG,yBAAH,GAA+B,iCAA9C,CAAN;CACH;CAEM,SAASwa,MAAT,CAAgB1E,CAAhB,EAAmBxP,CAAnB,EAAsB;CACzB,MAAI5G,CAAC,GAAG,OAAOoB,MAAP,KAAkB,UAAlB,IAAgCgV,CAAC,CAAChV,MAAM,CAACkZ,QAAR,CAAzC;CACA,MAAI,CAACta,CAAL,EAAQ,OAAOoW,CAAP;CACR,MAAItY,CAAC,GAAGkC,CAAC,CAAC8F,IAAF,CAAOsQ,CAAP,CAAR;CAAA,MAAmBgC,CAAnB;CAAA,MAAsBvC,EAAE,GAAG,EAA3B;CAAA,MAA+B9V,CAA/B;;CACA,MAAI;CACA,WAAO,CAAC6G,CAAC,KAAK,KAAK,CAAX,IAAgBA,CAAC,KAAK,CAAvB,KAA6B,CAAC,CAACwR,CAAC,GAAGta,CAAC,CAAC2b,IAAF,EAAL,EAAeG,IAApD;CAA0D/D,MAAAA,EAAE,CAACzW,IAAH,CAAQgZ,CAAC,CAACzX,KAAV;CAA1D;CACH,GAFD,CAGA,OAAOiB,KAAP,EAAc;CAAE7B,IAAAA,CAAC,GAAG;CAAE6B,MAAAA,KAAK,EAAEA;CAAT,KAAJ;CAAuB,GAHvC,SAIQ;CACJ,QAAI;CACA,UAAIwW,CAAC,IAAI,CAACA,CAAC,CAACwB,IAAR,KAAiB5Z,CAAC,GAAGlC,CAAC,CAAC,QAAD,CAAtB,CAAJ,EAAuCkC,CAAC,CAAC8F,IAAF,CAAOhI,CAAP;CAC1C,KAFD,SAGQ;CAAE,UAAIiC,CAAJ,EAAO,MAAMA,CAAC,CAAC6B,KAAR;CAAgB;CACpC;;CACD,SAAOiU,EAAP;CACH;CAEM,SAASkF,QAAT,GAAoB;CACvB,OAAK,IAAIlF,EAAE,GAAG,EAAT,EAAa/X,CAAC,GAAG,CAAtB,EAAyBA,CAAC,GAAGkI,SAAS,CAAChI,MAAvC,EAA+CF,CAAC,EAAhD;CACI+X,IAAAA,EAAE,GAAGA,EAAE,CAACnQ,MAAH,CAAUoV,MAAM,CAAC9U,SAAS,CAAClI,CAAD,CAAV,CAAhB,CAAL;CADJ;;CAEA,SAAO+X,EAAP;CACH;CAEM,SAASmF,cAAT,GAA0B;CAC7B,OAAK,IAAI1a,CAAC,GAAG,CAAR,EAAWxC,CAAC,GAAG,CAAf,EAAkBmd,EAAE,GAAGjV,SAAS,CAAChI,MAAtC,EAA8CF,CAAC,GAAGmd,EAAlD,EAAsDnd,CAAC,EAAvD;CAA2DwC,IAAAA,CAAC,IAAI0F,SAAS,CAAClI,CAAD,CAAT,CAAaE,MAAlB;CAA3D;;CACA,OAAK,IAAIoa,CAAC,GAAGxa,KAAK,CAAC0C,CAAD,CAAb,EAAkBoa,CAAC,GAAG,CAAtB,EAAyB5c,CAAC,GAAG,CAAlC,EAAqCA,CAAC,GAAGmd,EAAzC,EAA6Cnd,CAAC,EAA9C;CACI,SAAK,IAAIsH,CAAC,GAAGY,SAAS,CAAClI,CAAD,CAAjB,EAAsB4K,CAAC,GAAG,CAA1B,EAA6BwS,EAAE,GAAG9V,CAAC,CAACpH,MAAzC,EAAiD0K,CAAC,GAAGwS,EAArD,EAAyDxS,CAAC,IAAIgS,CAAC,EAA/D;CACItC,MAAAA,CAAC,CAACsC,CAAD,CAAD,GAAOtV,CAAC,CAACsD,CAAD,CAAR;CADJ;CADJ;;CAGA,SAAO0P,CAAP;CACH;CAEM,SAAS+C,OAAT,CAAiBZ,CAAjB,EAAoB;CACvB,SAAO,gBAAgBY,OAAhB,IAA2B,KAAKZ,CAAL,GAASA,CAAT,EAAY,IAAvC,IAA+C,IAAIY,OAAJ,CAAYZ,CAAZ,CAAtD;CACH;CAEM,SAASa,gBAAT,CAA0BrC,OAA1B,EAAmCC,UAAnC,EAA+CE,SAA/C,EAA0D;CAC7D,MAAI,CAAC9X,MAAM,CAACia,aAAZ,EAA2B,MAAM,IAAIxY,SAAJ,CAAc,sCAAd,CAAN;CAC3B,MAAIuX,CAAC,GAAGlB,SAAS,CAAClS,KAAV,CAAgB+R,OAAhB,EAAyBC,UAAU,IAAI,EAAvC,CAAR;CAAA,MAAoDlb,CAApD;CAAA,MAAuDwd,CAAC,GAAG,EAA3D;CACA,SAAOxd,CAAC,GAAG,EAAJ,EAAQuc,IAAI,CAAC,MAAD,CAAZ,EAAsBA,IAAI,CAAC,OAAD,CAA1B,EAAqCA,IAAI,CAAC,QAAD,CAAzC,EAAqDvc,CAAC,CAACsD,MAAM,CAACia,aAAR,CAAD,GAA0B,YAAY;CAAE,WAAO,IAAP;CAAc,GAA3G,EAA6Gvd,CAApH;;CACA,WAASuc,IAAT,CAAczT,CAAd,EAAiB;CAAE,QAAIwT,CAAC,CAACxT,CAAD,CAAL,EAAU9I,CAAC,CAAC8I,CAAD,CAAD,GAAO,UAAU2T,CAAV,EAAa;CAAE,aAAO,IAAIlB,OAAJ,CAAY,UAAUjU,CAAV,EAAa3B,CAAb,EAAgB;CAAE6X,QAAAA,CAAC,CAAClc,IAAF,CAAO,CAACwH,CAAD,EAAI2T,CAAJ,EAAOnV,CAAP,EAAU3B,CAAV,CAAP,IAAuB,CAAvB,IAA4B8X,MAAM,CAAC3U,CAAD,EAAI2T,CAAJ,CAAlC;CAA2C,OAAzE,CAAP;CAAoF,KAA1G;CAA6G;;CAC1I,WAASgB,MAAT,CAAgB3U,CAAhB,EAAmB2T,CAAnB,EAAsB;CAAE,QAAI;CAAEf,MAAAA,IAAI,CAACY,CAAC,CAACxT,CAAD,CAAD,CAAK2T,CAAL,CAAD,CAAJ;CAAgB,KAAtB,CAAuB,OAAOxa,CAAP,EAAU;CAAEyb,MAAAA,MAAM,CAACF,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUvb,CAAV,CAAN;CAAqB;CAAE;;CAClF,WAASyZ,IAAT,CAAcpB,CAAd,EAAiB;CAAEA,IAAAA,CAAC,CAACzX,KAAF,YAAmBwa,OAAnB,GAA6B9B,OAAO,CAACD,OAAR,CAAgBhB,CAAC,CAACzX,KAAF,CAAQ4Z,CAAxB,EAA2BV,IAA3B,CAAgC4B,OAAhC,EAAyCnC,MAAzC,CAA7B,GAAgFkC,MAAM,CAACF,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUlD,CAAV,CAAtF;CAAqG;;CACxH,WAASqD,OAAT,CAAiB9a,KAAjB,EAAwB;CAAE4a,IAAAA,MAAM,CAAC,MAAD,EAAS5a,KAAT,CAAN;CAAwB;;CAClD,WAAS2Y,MAAT,CAAgB3Y,KAAhB,EAAuB;CAAE4a,IAAAA,MAAM,CAAC,OAAD,EAAU5a,KAAV,CAAN;CAAyB;;CAClD,WAAS6a,MAAT,CAAgBtK,CAAhB,EAAmBqJ,CAAnB,EAAsB;CAAE,QAAIrJ,CAAC,CAACqJ,CAAD,CAAD,EAAMe,CAAC,CAACI,KAAF,EAAN,EAAiBJ,CAAC,CAACtd,MAAvB,EAA+Bud,MAAM,CAACD,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUA,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAV,CAAN;CAA2B;CACrF;CAEM,SAASK,gBAAT,CAA0BvF,CAA1B,EAA6B;CAChC,MAAItY,CAAJ,EAAO2Z,CAAP;CACA,SAAO3Z,CAAC,GAAG,EAAJ,EAAQuc,IAAI,CAAC,MAAD,CAAZ,EAAsBA,IAAI,CAAC,OAAD,EAAU,UAAUta,CAAV,EAAa;CAAE,UAAMA,CAAN;CAAU,GAAnC,CAA1B,EAAgEsa,IAAI,CAAC,QAAD,CAApE,EAAgFvc,CAAC,CAACsD,MAAM,CAACkZ,QAAR,CAAD,GAAqB,YAAY;CAAE,WAAO,IAAP;CAAc,GAAjI,EAAmIxc,CAA1I;;CACA,WAASuc,IAAT,CAAczT,CAAd,EAAiBsK,CAAjB,EAAoB;CAAEpT,IAAAA,CAAC,CAAC8I,CAAD,CAAD,GAAOwP,CAAC,CAACxP,CAAD,CAAD,GAAO,UAAU2T,CAAV,EAAa;CAAE,aAAO,CAAC9C,CAAC,GAAG,CAACA,CAAN,IAAW;CAAE9W,QAAAA,KAAK,EAAEwa,OAAO,CAAC/E,CAAC,CAACxP,CAAD,CAAD,CAAK2T,CAAL,CAAD,CAAhB;CAA2BX,QAAAA,IAAI,EAAEhT,CAAC,KAAK;CAAvC,OAAX,GAA+DsK,CAAC,GAAGA,CAAC,CAACqJ,CAAD,CAAJ,GAAUA,CAAjF;CAAqF,KAA3G,GAA8GrJ,CAArH;CAAyH;CAClJ;CAEM,SAAS0K,aAAT,CAAuBxF,CAAvB,EAA0B;CAC7B,MAAI,CAAChV,MAAM,CAACia,aAAZ,EAA2B,MAAM,IAAIxY,SAAJ,CAAc,sCAAd,CAAN;CAC3B,MAAI7C,CAAC,GAAGoW,CAAC,CAAChV,MAAM,CAACia,aAAR,CAAT;CAAA,MAAiCvd,CAAjC;CACA,SAAOkC,CAAC,GAAGA,CAAC,CAAC8F,IAAF,CAAOsQ,CAAP,CAAH,IAAgBA,CAAC,GAAG,OAAOyE,QAAP,KAAoB,UAApB,GAAiCA,QAAQ,CAACzE,CAAD,CAAzC,GAA+CA,CAAC,CAAChV,MAAM,CAACkZ,QAAR,CAAD,EAAnD,EAAyExc,CAAC,GAAG,EAA7E,EAAiFuc,IAAI,CAAC,MAAD,CAArF,EAA+FA,IAAI,CAAC,OAAD,CAAnG,EAA8GA,IAAI,CAAC,QAAD,CAAlH,EAA8Hvc,CAAC,CAACsD,MAAM,CAACia,aAAR,CAAD,GAA0B,YAAY;CAAE,WAAO,IAAP;CAAc,GAApL,EAAsLvd,CAAtM,CAAR;;CACA,WAASuc,IAAT,CAAczT,CAAd,EAAiB;CAAE9I,IAAAA,CAAC,CAAC8I,CAAD,CAAD,GAAOwP,CAAC,CAACxP,CAAD,CAAD,IAAQ,UAAU2T,CAAV,EAAa;CAAE,aAAO,IAAIlB,OAAJ,CAAY,UAAUD,OAAV,EAAmBE,MAAnB,EAA2B;CAAEiB,QAAAA,CAAC,GAAGnE,CAAC,CAACxP,CAAD,CAAD,CAAK2T,CAAL,CAAJ,EAAaiB,MAAM,CAACpC,OAAD,EAAUE,MAAV,EAAkBiB,CAAC,CAACX,IAApB,EAA0BW,CAAC,CAAC5Z,KAA5B,CAAnB;CAAwD,OAAjG,CAAP;CAA4G,KAA1I;CAA6I;;CAChK,WAAS6a,MAAT,CAAgBpC,OAAhB,EAAyBE,MAAzB,EAAiCjZ,CAAjC,EAAoCka,CAApC,EAAuC;CAAElB,IAAAA,OAAO,CAACD,OAAR,CAAgBmB,CAAhB,EAAmBV,IAAnB,CAAwB,UAASU,CAAT,EAAY;CAAEnB,MAAAA,OAAO,CAAC;CAAEzY,QAAAA,KAAK,EAAE4Z,CAAT;CAAYX,QAAAA,IAAI,EAAEvZ;CAAlB,OAAD,CAAP;CAAiC,KAAvE,EAAyEiZ,MAAzE;CAAmF;CAC/H;CAEM,SAASuC,oBAAT,CAA8BC,MAA9B,EAAsCC,GAAtC,EAA2C;CAC9C,MAAIha,MAAM,CAACG,cAAX,EAA2B;CAAEH,IAAAA,MAAM,CAACG,cAAP,CAAsB4Z,MAAtB,EAA8B,KAA9B,EAAqC;CAAEnb,MAAAA,KAAK,EAAEob;CAAT,KAArC;CAAuD,GAApF,MAA0F;CAAED,IAAAA,MAAM,CAACC,GAAP,GAAaA,GAAb;CAAmB;;CAC/G,SAAOD,MAAP;CACH;CAEM,SAASE,YAAT,CAAsBC,GAAtB,EAA2B;CAC9B,MAAIA,GAAG,IAAIA,GAAG,CAACC,UAAf,EAA2B,OAAOD,GAAP;CAC3B,MAAItC,MAAM,GAAG,EAAb;CACA,MAAIsC,GAAG,IAAI,IAAX,EAAiB,KAAK,IAAIvB,CAAT,IAAcuB,GAAd;CAAmB,QAAIla,MAAM,CAACqT,cAAP,CAAsBtP,IAAtB,CAA2BmW,GAA3B,EAAgCvB,CAAhC,CAAJ,EAAwCf,MAAM,CAACe,CAAD,CAAN,GAAYuB,GAAG,CAACvB,CAAD,CAAf;CAA3D;CACjBf,EAAAA,MAAM,WAAN,GAAiBsC,GAAjB;CACA,SAAOtC,MAAP;CACH;CAEM,SAASwC,eAAT,CAAyBF,GAAzB,EAA8B;CACjC,SAAQA,GAAG,IAAIA,GAAG,CAACC,UAAZ,GAA0BD,GAA1B,GAAgC;CAAE,eAASA;CAAX,GAAvC;CACH;CAEM,SAASG,sBAAT,CAAgCC,QAAhC,EAA0CC,UAA1C,EAAsD;CACzD,MAAI,CAACA,UAAU,CAACC,GAAX,CAAeF,QAAf,CAAL,EAA+B;CAC3B,UAAM,IAAIxZ,SAAJ,CAAc,gDAAd,CAAN;CACH;;CACD,SAAOyZ,UAAU,CAACla,GAAX,CAAeia,QAAf,CAAP;CACH;CAEM,SAASG,sBAAT,CAAgCH,QAAhC,EAA0CC,UAA1C,EAAsD3b,KAAtD,EAA6D;CAChE,MAAI,CAAC2b,UAAU,CAACC,GAAX,CAAeF,QAAf,CAAL,EAA+B;CAC3B,UAAM,IAAIxZ,SAAJ,CAAc,gDAAd,CAAN;CACH;;CACDyZ,EAAAA,UAAU,CAACzW,GAAX,CAAewW,QAAf,EAAyB1b,KAAzB;CACA,SAAOA,KAAP;CACH;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;ACzN6B;CAQ9B;CACa,iCAAyB,GAA0B,WAAwC,CAAC;CAUzG;CACA;KAA+B8b,uCAAyB;KAetD,mBAAY,GAAkB,EAAE,IAAa;SAA7C,iBAgBC;;;SAbC,IAAI,EAAE,KAAI,YAAY,SAAS,CAAC;aAAE,OAAO,IAAI,SAAS,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;SAElE,IAAI,WAAI,CAAC,MAAM,CAAC,GAAG,CAAC,EAAE;aACpB,QAAA,kBAAM,GAAG,CAAC,GAAG,EAAE,GAAG,CAAC,IAAI,EAAE,IAAI,CAAC,SAAC;UAChC;cAAM;aACL,QAAA,kBAAM,GAAG,EAAE,IAAI,EAAE,IAAI,CAAC,SAAC;UACxB;SACD,MAAM,CAAC,cAAc,CAAC,KAAI,EAAE,WAAW,EAAE;aACvC,KAAK,EAAE,WAAW;aAClB,QAAQ,EAAE,KAAK;aACf,YAAY,EAAE,KAAK;aACnB,UAAU,EAAE,KAAK;UAClB,CAAC,CAAC;;MACJ;KAED,0BAAM,GAAN;SACE,OAAO;aACL,UAAU,EAAE,IAAI,CAAC,QAAQ,EAAE;UAC5B,CAAC;MACH;;KAGM,iBAAO,GAAd,UAAe,KAAa;SAC1B,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,OAAO,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;MACjD;;KAGM,oBAAU,GAAjB,UAAkB,KAAa;SAC7B,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,UAAU,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;MACpD;;;;;;;KAQM,kBAAQ,GAAf,UAAgB,OAAe,EAAE,QAAgB;SAC/C,OAAO,IAAI,SAAS,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;MACzC;;;;;;;KAQM,oBAAU,GAAjB,UAAkB,GAAW,EAAE,QAAgB;SAC7C,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,UAAU,CAAC,GAAG,EAAE,IAAI,EAAE,QAAQ,CAAC,CAAC,CAAC;MAC5D;;KAGD,kCAAc,GAAd;SACE,OAAO,EAAE,UAAU,EAAE,EAAE,CAAC,EAAE,IAAI,CAAC,IAAI,KAAK,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,GAAG,KAAK,CAAC,EAAE,EAAE,CAAC;MAClE;;KAGM,0BAAgB,GAAvB,UAAwB,GAAsB;SAC5C,OAAO,IAAI,SAAS,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC,EAAE,GAAG,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;MAC1D;;KAGD,oBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,2BAAO,GAAP;SACE,OAAO,mBAAiB,IAAI,CAAC,UAAU,EAAE,CAAC,QAAQ,EAAE,UAAK,IAAI,CAAC,WAAW,EAAE,CAAC,QAAQ,EAAE,MAAG,CAAC;MAC3F;KAnFe,mBAAS,GAAG,WAAI,CAAC,kBAAkB,CAAC;KAoFtD,gBAAC;EAAA,CAvF8B,iCAAyB,GAuFvD;CAvFY,8BAAS;;;;;;;;;;;ACpBY;AAEJ;AACgB;AACJ;AACR;AACD;AACH;AACK;AACA;AACG;AAC0B;AAC1B;AACA;AACE;CAqBxC,SAAgB,UAAU,CAAC,KAAc;KACvC,QACEvF,kBAAY,CAAC,KAAK,CAAC,IAAI,OAAO,CAAC,GAAG,CAAC,KAAK,EAAE,WAAW,CAAC,IAAI,OAAO,KAAK,CAAC,SAAS,KAAK,QAAQ,EAC7F;CACJ,CAAC;CAJD,gCAIC;CAED;CACA,IAAM,cAAc,GAAG,UAAU,CAAC;CAClC,IAAM,cAAc,GAAG,CAAC,UAAU,CAAC;CACnC;CACA,IAAM,cAAc,GAAG,kBAAkB,CAAC;CAC1C,IAAM,cAAc,GAAG,CAAC,kBAAkB,CAAC;CAE3C;CACA;CACA,IAAM,YAAY,GAAG;KACnB,IAAI,EAAEwF,iBAAQ;KACd,OAAO,EAAErF,aAAM;KACf,KAAK,EAAEA,aAAM;KACb,OAAO,EAAEsF,iBAAU;KACnB,UAAU,EAAEC,YAAK;KACjB,cAAc,EAAEC,qBAAU;KAC1B,aAAa,EAAE,eAAM;KACrB,WAAW,EAAE,WAAI;KACjB,OAAO,EAAEC,cAAM;KACf,OAAO,EAAEC,cAAM;KACf,MAAM,EAAEC,iBAAU;KAClB,kBAAkB,EAAEA,iBAAU;KAC9B,UAAU,EAAEC,mBAAS;EACb,CAAC;CAEX;CACA,SAAS,gBAAgB,CAAC,KAAU,EAAE,OAA2B;KAA3B,wBAAA,EAAA,YAA2B;KAC/D,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;SAC7B,IAAI,OAAO,CAAC,OAAO,IAAI,OAAO,CAAC,MAAM,EAAE;aACrC,OAAO,KAAK,CAAC;UACd;;;SAID,IAAI,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK,EAAE;aAC/B,IAAI,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc;iBAAE,OAAO,IAAIL,YAAK,CAAC,KAAK,CAAC,CAAC;aAChF,IAAI,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc;iBAAE,OAAO,WAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC;UACvF;;SAGD,OAAO,IAAI,eAAM,CAAC,KAAK,CAAC,CAAC;MAC1B;;KAGD,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ;SAAE,OAAO,KAAK,CAAC;;KAG7D,IAAI,KAAK,CAAC,UAAU;SAAE,OAAO,IAAI,CAAC;KAElC,IAAM,IAAI,GAAG,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,MAAM,CACpC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,IAAI,GAAA,CACV,CAAC;KACnC,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;SACpC,IAAM,CAAC,GAAG,YAAY,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,CAAC;SAChC,IAAI,CAAC;aAAE,OAAO,CAAC,CAAC,gBAAgB,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;MAClD;KAED,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,EAAE;SACvB,IAAM,CAAC,GAAG,KAAK,CAAC,KAAK,CAAC;SACtB,IAAM,IAAI,GAAG,IAAI,IAAI,EAAE,CAAC;SAExB,IAAI,OAAO,CAAC,MAAM,EAAE;aAClB,IAAI,OAAO,CAAC,KAAK,QAAQ;iBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;kBACtC,IAAI,OAAO,CAAC,KAAK,QAAQ;iBAAE,IAAI,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;UAC7D;cAAM;aACL,IAAI,OAAO,CAAC,KAAK,QAAQ;iBAAE,IAAI,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;kBAClD,IAAI,WAAI,CAAC,MAAM,CAAC,CAAC,CAAC;iBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,QAAQ,EAAE,CAAC,CAAC;kBAC/C,IAAI,OAAO,CAAC,KAAK,QAAQ,IAAI,OAAO,CAAC,OAAO;iBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;UACpE;SACD,OAAO,IAAI,CAAC;MACb;KAED,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,EAAE;SACvB,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,KAAK,CAAC,CAAC;SACtC,IAAI,KAAK,CAAC,MAAM,EAAE;aAChB,IAAI,CAAC,MAAM,GAAG,gBAAgB,CAAC,KAAK,CAAC,MAAM,CAAC,CAAC;UAC9C;SAED,OAAOM,SAAI,CAAC,gBAAgB,CAAC,KAAK,CAAC,CAAC;MACrC;KAED,IAAIC,kBAAW,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,UAAU,EAAE;SAC1C,IAAM,CAAC,GAAG,KAAK,CAAC,IAAI,GAAG,KAAK,GAAG,KAAK,CAAC,UAAU,CAAC;;;SAIhD,IAAI,CAAC,YAAYA,YAAK;aAAE,OAAO,CAAC,CAAC;SAEjC,IAAM,UAAU,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,GAAA,CAAC,CAAC;SACjE,IAAI,OAAK,GAAG,IAAI,CAAC;SACjB,UAAU,CAAC,OAAO,CAAC,UAAA,CAAC;aAClB,IAAI,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC;iBAAE,OAAK,GAAG,KAAK,CAAC;UAC7D,CAAC,CAAC;;SAGH,IAAI,OAAK;aAAE,OAAOA,YAAK,CAAC,gBAAgB,CAAC,CAAC,CAAC,CAAC;MAC7C;KAED,OAAO,KAAK,CAAC;CACf,CAAC;CAMD;CACA,SAAS,cAAc,CAAC,KAAY,EAAE,OAA8B;KAClE,OAAO,KAAK,CAAC,GAAG,CAAC,UAAC,CAAU,EAAE,KAAa;SACzC,OAAO,CAAC,WAAW,CAAC,IAAI,CAAC,EAAE,YAAY,EAAE,WAAS,KAAO,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC,CAAC;SACxE,IAAI;aACF,OAAO,cAAc,CAAC,CAAC,EAAE,OAAO,CAAC,CAAC;UACnC;iBAAS;aACR,OAAO,CAAC,WAAW,CAAC,GAAG,EAAE,CAAC;UAC3B;MACF,CAAC,CAAC;CACL,CAAC;CAED,SAAS,YAAY,CAAC,IAAU;KAC9B,IAAM,MAAM,GAAG,IAAI,CAAC,WAAW,EAAE,CAAC;;KAElC,OAAO,IAAI,CAAC,kBAAkB,EAAE,KAAK,CAAC,GAAG,MAAM,GAAG,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;CAC9E,CAAC;CAED;CACA,SAAS,cAAc,CAAC,KAAU,EAAE,OAA8B;KAChE,IAAI,CAAC,OAAO,KAAK,KAAK,QAAQ,IAAI,OAAO,KAAK,KAAK,UAAU,KAAK,KAAK,KAAK,IAAI,EAAE;SAChF,IAAM,KAAK,GAAG,OAAO,CAAC,WAAW,CAAC,SAAS,CAAC,UAAA,KAAK,IAAI,OAAA,KAAK,CAAC,GAAG,KAAK,KAAK,GAAA,CAAC,CAAC;SAC1E,IAAI,KAAK,KAAK,CAAC,CAAC,EAAE;aAChB,IAAM,KAAK,GAAG,OAAO,CAAC,WAAW,CAAC,GAAG,CAAC,UAAA,KAAK,IAAI,OAAA,KAAK,CAAC,YAAY,GAAA,CAAC,CAAC;aACnE,IAAM,WAAW,GAAG,KAAK;kBACtB,KAAK,CAAC,CAAC,EAAE,KAAK,CAAC;kBACf,GAAG,CAAC,UAAA,IAAI,IAAI,OAAG,IAAI,SAAM,GAAA,CAAC;kBAC1B,IAAI,CAAC,EAAE,CAAC,CAAC;aACZ,IAAM,WAAW,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC;aACjC,IAAM,YAAY,GAChB,MAAM;iBACN,KAAK;sBACF,KAAK,CAAC,KAAK,GAAG,CAAC,EAAE,KAAK,CAAC,MAAM,GAAG,CAAC,CAAC;sBAClC,GAAG,CAAC,UAAA,IAAI,IAAI,OAAG,IAAI,SAAM,GAAA,CAAC;sBAC1B,IAAI,CAAC,EAAE,CAAC,CAAC;aACd,IAAM,OAAO,GAAG,KAAK,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;aACxC,IAAM,YAAY,GAAG,GAAG,CAAC,MAAM,CAAC,WAAW,CAAC,MAAM,GAAG,WAAW,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;aAC7E,IAAM,MAAM,GAAG,GAAG,CAAC,MAAM,CACvB,YAAY,CAAC,MAAM,GAAG,CAAC,WAAW,CAAC,MAAM,GAAG,OAAO,CAAC,MAAM,IAAI,CAAC,GAAG,CAAC,CACpE,CAAC;aAEF,MAAM,IAAI,SAAS,CACjB,2CAA2C;kBACzC,SAAO,WAAW,GAAG,WAAW,GAAG,YAAY,GAAG,OAAO,OAAI,CAAA;kBAC7D,SAAO,YAAY,UAAK,MAAM,MAAG,CAAA,CACpC,CAAC;UACH;SACD,OAAO,CAAC,WAAW,CAAC,OAAO,CAAC,WAAW,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC,GAAG,GAAG,KAAK,CAAC;MACjE;KAED,IAAI,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC;SAAE,OAAO,cAAc,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;KAEhE,IAAI,KAAK,KAAK,SAAS;SAAE,OAAO,IAAI,CAAC;KAErC,IAAI,KAAK,YAAY,IAAI,IAAIjG,YAAM,CAAC,KAAK,CAAC,EAAE;SAC1C,IAAM,OAAO,GAAG,KAAK,CAAC,OAAO,EAAE;;SAE7B,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC,IAAI,OAAO,GAAG,eAAe,CAAC;SAEtD,IAAI,OAAO,CAAC,MAAM,EAAE;aAClB,OAAO,OAAO,CAAC,OAAO,IAAI,OAAO;mBAC7B,EAAE,KAAK,EAAE,KAAK,CAAC,OAAO,EAAE,EAAE;mBAC1B,EAAE,KAAK,EAAE,YAAY,CAAC,KAAK,CAAC,EAAE,CAAC;UACpC;SACD,OAAO,OAAO,CAAC,OAAO,IAAI,OAAO;eAC7B,EAAE,KAAK,EAAE,YAAY,CAAC,KAAK,CAAC,EAAE;eAC9B,EAAE,KAAK,EAAE,EAAE,WAAW,EAAE,KAAK,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE,EAAE,EAAE,CAAC;MAC5D;KAED,IAAI,OAAO,KAAK,KAAK,QAAQ,KAAK,CAAC,OAAO,CAAC,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC,EAAE;;SAEvE,IAAI,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK,EAAE;aAC/B,IAAM,UAAU,GAAG,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc,EACnE,UAAU,GAAG,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc,CAAC;;aAGlE,IAAI,UAAU;iBAAE,OAAO,EAAE,UAAU,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;aACxD,IAAI,UAAU;iBAAE,OAAO,EAAE,WAAW,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;UAC1D;SACD,OAAO,EAAE,aAAa,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;MAC5C;KAED,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;SAC9C,IAAI,KAAK,GAAG,KAAK,CAAC,KAAK,CAAC;SACxB,IAAI,KAAK,KAAK,SAAS,EAAE;aACvB,IAAM,KAAK,GAAG,KAAK,CAAC,QAAQ,EAAE,CAAC,KAAK,CAAC,WAAW,CAAC,CAAC;aAClD,IAAI,KAAK,EAAE;iBACT,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;cAClB;UACF;SAED,IAAM,EAAE,GAAG,IAAI8F,iBAAU,CAAC,KAAK,CAAC,MAAM,EAAE,KAAK,CAAC,CAAC;SAC/C,OAAO,EAAE,CAAC,cAAc,CAAC,OAAO,CAAC,CAAC;MACnC;KAED,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ;SAAE,OAAO,iBAAiB,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;KACzF,OAAO,KAAK,CAAC;CACf,CAAC;CAED,IAAM,kBAAkB,GAAG;KACzB,MAAM,EAAE,UAAC,CAAS,IAAK,OAAA,IAAI3F,aAAM,CAAC,CAAC,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,QAAQ,CAAC,GAAA;KACxD,IAAI,EAAE,UAAC,CAAO,IAAK,OAAA,IAAI6F,SAAI,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC,KAAK,CAAC,GAAA;KAC5C,KAAK,EAAE,UAAC,CAAQ,IAAK,OAAA,IAAIC,YAAK,CAAC,CAAC,CAAC,UAAU,IAAI,CAAC,CAAC,SAAS,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,MAAM,CAAC,GAAA;KAClF,UAAU,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIN,qBAAU,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;KACtD,MAAM,EAAE,UAAC,CAAS,IAAK,OAAA,IAAI,eAAM,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;KAC1C,KAAK,EAAE,UAAC,CAAQ,IAAK,OAAA,IAAID,YAAK,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;KACvC,IAAI,EAAE,UACJ,CAIC;SAED,OAAA,WAAI,CAAC,QAAQ;;SAEX,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,GAAG,GAAG,CAAC,CAAC,IAAI,EAC9B,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,CAAC,KAAK,EAChC,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,QAAQ,GAAG,CAAC,CAAC,SAAS,CACzC;MAAA;KACH,MAAM,EAAE,cAAM,OAAA,IAAIG,cAAM,EAAE,GAAA;KAC1B,MAAM,EAAE,cAAM,OAAA,IAAID,cAAM,EAAE,GAAA;KAC1B,QAAQ,EAAE,UAAC,CAAW,IAAK,OAAA,IAAIJ,iBAAQ,CAAC,CAAC,CAAC,GAAA;KAC1C,QAAQ,EAAE,UAAC,CAAW,IAAK,OAAA,IAAIA,iBAAQ,CAAC,CAAC,CAAC,GAAA;KAC1C,UAAU,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIM,iBAAU,CAAC,CAAC,CAAC,OAAO,EAAE,CAAC,CAAC,OAAO,CAAC,GAAA;KACnE,MAAM,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIL,iBAAU,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;KAClD,SAAS,EAAE,UAAC,CAAY,IAAK,OAAAM,mBAAS,CAAC,QAAQ,CAAC,CAAC,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,GAAA;EACtD,CAAC;CAEX;CACA,SAAS,iBAAiB,CAAC,GAAQ,EAAE,OAA8B;KACjE,IAAI,GAAG,IAAI,IAAI,IAAI,OAAO,GAAG,KAAK,QAAQ;SAAE,MAAM,IAAI,KAAK,CAAC,wBAAwB,CAAC,CAAC;KAEtF,IAAM,QAAQ,GAA0B,GAAG,CAAC,SAAS,CAAC;KACtD,IAAI,OAAO,QAAQ,KAAK,WAAW,EAAE;;SAEnC,IAAM,IAAI,GAAa,EAAE,CAAC;SAC1B,KAAK,IAAM,IAAI,IAAI,GAAG,EAAE;aACtB,OAAO,CAAC,WAAW,CAAC,IAAI,CAAC,EAAE,YAAY,EAAE,IAAI,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC,CAAC;aAC5D,IAAI;iBACF,IAAI,CAAC,IAAI,CAAC,GAAG,cAAc,CAAC,GAAG,CAAC,IAAI,CAAC,EAAE,OAAO,CAAC,CAAC;cACjD;qBAAS;iBACR,OAAO,CAAC,WAAW,CAAC,GAAG,EAAE,CAAC;cAC3B;UACF;SACD,OAAO,IAAI,CAAC;MACb;UAAM,IAAI,UAAU,CAAC,GAAG,CAAC,EAAE;;;SAG1B,IAAI,MAAM,GAAQ,GAAG,CAAC;SACtB,IAAI,OAAO,MAAM,CAAC,cAAc,KAAK,UAAU,EAAE;;;;;aAK/C,IAAM,MAAM,GAAG,kBAAkB,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;aACjD,IAAI,CAAC,MAAM,EAAE;iBACX,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,GAAG,CAAC,SAAS,CAAC,CAAC;cAC5E;aACD,MAAM,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC;UACzB;;SAGD,IAAI,QAAQ,KAAK,MAAM,IAAI,MAAM,CAAC,KAAK,EAAE;aACvC,MAAM,GAAG,IAAIC,SAAI,CAAC,MAAM,CAAC,IAAI,EAAE,cAAc,CAAC,MAAM,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC,CAAC;UACvE;cAAM,IAAI,QAAQ,KAAK,OAAO,IAAI,MAAM,CAAC,GAAG,EAAE;aAC7C,MAAM,GAAG,IAAIC,YAAK,CAChB,cAAc,CAAC,MAAM,CAAC,UAAU,EAAE,OAAO,CAAC,EAC1C,cAAc,CAAC,MAAM,CAAC,GAAG,EAAE,OAAO,CAAC,EACnC,cAAc,CAAC,MAAM,CAAC,EAAE,EAAE,OAAO,CAAC,EAClC,cAAc,CAAC,MAAM,CAAC,MAAM,EAAE,OAAO,CAAC,CACvC,CAAC;UACH;SAED,OAAO,MAAM,CAAC,cAAc,CAAC,OAAO,CAAC,CAAC;MACvC;UAAM;SACL,MAAM,IAAI,KAAK,CAAC,uCAAuC,GAAG,OAAO,QAAQ,CAAC,CAAC;MAC5E;CACH,CAAC;CASD,WAAiB,KAAK;;;;;;;;;;;;;;;;;KA6BpB,SAAgB,KAAK,CAAC,IAAY,EAAE,OAAuB;SACzD,IAAM,YAAY,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,EAAE,OAAO,EAAE,IAAI,EAAE,MAAM,EAAE,KAAK,EAAE,EAAE,OAAO,CAAC,CAAC;;SAGlF,IAAI,OAAO,YAAY,CAAC,OAAO,KAAK,SAAS;aAAE,YAAY,CAAC,MAAM,GAAG,CAAC,YAAY,CAAC,OAAO,CAAC;SAC3F,IAAI,OAAO,YAAY,CAAC,MAAM,KAAK,SAAS;aAAE,YAAY,CAAC,OAAO,GAAG,CAAC,YAAY,CAAC,MAAM,CAAC;SAE1F,OAAO,IAAI,CAAC,KAAK,CAAC,IAAI,EAAE,UAAC,IAAI,EAAE,KAAK,IAAK,OAAA,gBAAgB,CAAC,KAAK,EAAE,YAAY,CAAC,GAAA,CAAC,CAAC;MACjF;KARe,WAAK,QAQpB,CAAA;;;;;;;;;;;;;;;;;;;;;;;;KA4BD,SAAgB,SAAS,CACvB,KAAwB;;KAExB,QAA8F,EAC9F,KAAuB,EACvB,OAAuB;SAEvB,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;aAC9C,OAAO,GAAG,KAAK,CAAC;aAChB,KAAK,GAAG,CAAC,CAAC;UACX;SACD,IAAI,QAAQ,IAAI,IAAI,IAAI,OAAO,QAAQ,KAAK,QAAQ,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,QAAQ,CAAC,EAAE;aAChF,OAAO,GAAG,QAAQ,CAAC;aACnB,QAAQ,GAAG,SAAS,CAAC;aACrB,KAAK,GAAG,CAAC,CAAC;UACX;SACD,IAAM,gBAAgB,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,OAAO,EAAE,IAAI,EAAE,MAAM,EAAE,KAAK,EAAE,EAAE,OAAO,EAAE;aAChF,WAAW,EAAE,CAAC,EAAE,YAAY,EAAE,QAAQ,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;UACrD,CAAC,CAAC;SAEH,IAAM,GAAG,GAAG,cAAc,CAAC,KAAK,EAAE,gBAAgB,CAAC,CAAC;SACpD,OAAO,IAAI,CAAC,SAAS,CAAC,GAAG,EAAE,QAA4C,EAAE,KAAK,CAAC,CAAC;MACjF;KAtBe,eAAS,YAsBxB,CAAA;;;;;;;KAQD,SAAgB,SAAS,CAAC,KAAwB,EAAE,OAAuB;SACzE,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,OAAO,IAAI,CAAC,KAAK,CAAC,SAAS,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC,CAAC;MAC9C;KAHe,eAAS,YAGxB,CAAA;;;;;;;KAQD,SAAgB,WAAW,CAAC,KAAe,EAAE,OAAuB;SAClE,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,OAAO,KAAK,CAAC,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,EAAE,OAAO,CAAC,CAAC;MAC9C;KAHe,iBAAW,cAG1B,CAAA;CACH,CAAC,EA9GgB,aAAK,KAAL,aAAK,QA8GrB;;;;;;;;;CC1bD;CACA;;;CAOA;CACA,IAAI,OAAuB,CAAC;CAiIR,sBAAG;CA/HvB,IAAM,KAAK,GAAG,UAAU,eAAoB;;KAE1C,OAAO,eAAe,IAAI,eAAe,CAAC,IAAI,IAAI,IAAI,IAAI,eAAe,CAAC;CAC5E,CAAC,CAAC;CAEF;CACA,SAAS,SAAS;;KAEhB,QACE,KAAK,CAAC,OAAO,UAAU,KAAK,QAAQ,IAAI,UAAU,CAAC;SACnD,KAAK,CAAC,OAAO,MAAM,KAAK,QAAQ,IAAI,MAAM,CAAC;SAC3C,KAAK,CAAC,OAAO,IAAI,KAAK,QAAQ,IAAI,IAAI,CAAC;SACvC,KAAK,CAAC,OAAOnN,cAAM,KAAK,QAAQ,IAAIA,cAAM,CAAC;SAC3C,QAAQ,CAAC,aAAa,CAAC,EAAE,EACzB;CACJ,CAAC;CAED,IAAM,UAAU,GAAG,SAAS,EAAE,CAAC;CAC/B,IAAI,MAAM,CAAC,SAAS,CAAC,cAAc,CAAC,IAAI,CAAC,UAAU,EAAE,KAAK,CAAC,EAAE;KAC3D,cAAA,OAAO,GAAG,UAAU,CAAC,GAAG,CAAC;EAC1B;MAAM;;KAEL,cAAA,OAAO;SAGL,aAAY,KAA2B;aAA3B,sBAAA,EAAA,UAA2B;aACrC,IAAI,CAAC,KAAK,GAAG,EAAE,CAAC;aAChB,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC;aAElB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;iBACrC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,IAAI;qBAAE,SAAS;iBAC/B,IAAM,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;iBACvB,IAAM,GAAG,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;iBACrB,IAAM,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;;iBAEvB,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;;iBAGrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,EAAE,CAAC;cAC5D;UACF;SACD,mBAAK,GAAL;aACE,IAAI,CAAC,KAAK,GAAG,EAAE,CAAC;aAChB,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC;UACnB;SACD,oBAAM,GAAN,UAAO,GAAW;aAChB,IAAM,KAAK,GAAG,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC;aAChC,IAAI,KAAK,IAAI,IAAI;iBAAE,OAAO,KAAK,CAAC;;aAEhC,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC;;aAEzB,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;aAC9B,OAAO,IAAI,CAAC;UACb;SACD,qBAAO,GAAP;aAAA,iBAYC;aAXC,IAAI,KAAK,GAAG,CAAC,CAAC;aAEd,OAAO;iBACL,IAAI,EAAE;qBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;qBAChC,OAAO;yBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,CAAC,GAAG,EAAE,KAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,GAAG,SAAS;yBACjE,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;sBACvC,CAAC;kBACH;cACF,CAAC;UACH;SACD,qBAAO,GAAP,UAAQ,QAAmE,EAAE,IAAW;aACtF,IAAI,GAAG,IAAI,IAAI,IAAI,CAAC;aAEpB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;iBAC1C,IAAM,GAAG,GAAG,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;;iBAE1B,QAAQ,CAAC,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,EAAE,GAAG,EAAE,IAAI,CAAC,CAAC;cACrD;UACF;SACD,iBAAG,GAAH,UAAI,GAAW;aACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,SAAS,CAAC;UAC5D;SACD,iBAAG,GAAH,UAAI,GAAW;aACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC;UAClC;SACD,kBAAI,GAAJ;aAAA,iBAYC;aAXC,IAAI,KAAK,GAAG,CAAC,CAAC;aAEd,OAAO;iBACL,IAAI,EAAE;qBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;qBAChC,OAAO;yBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,GAAG,GAAG,SAAS;yBAC1C,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;sBACvC,CAAC;kBACH;cACF,CAAC;UACH;SACD,iBAAG,GAAH,UAAI,GAAW,EAAE,KAAU;aACzB,IAAI,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;iBACrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,KAAK,CAAC;iBAC5B,OAAO,IAAI,CAAC;cACb;;aAGD,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;;aAGrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,EAAE,CAAC;aAC3D,OAAO,IAAI,CAAC;UACb;SACD,oBAAM,GAAN;aAAA,iBAYC;aAXC,IAAI,KAAK,GAAG,CAAC,CAAC;aAEd,OAAO;iBACL,IAAI,EAAE;qBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;qBAChC,OAAO;yBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,KAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,SAAS;yBAC1D,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;sBACvC,CAAC;kBACH;cACF,CAAC;UACH;SACD,sBAAI,qBAAI;kBAAR;iBACE,OAAO,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC;cAC1B;;;YAAA;SACH,UAAC;MAtGU,GAsGoB,CAAC;EACjC;;;;;;;;;;CCxID;CACa,sBAAc,GAAG,UAAU,CAAC;CACzC;CACa,sBAAc,GAAG,CAAC,UAAU,CAAC;CAC1C;CACa,sBAAc,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;CAClD;CACa,sBAAc,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;CAE/C;;;;CAIa,kBAAU,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;CAE1C;;;;CAIa,kBAAU,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;CAE3C;CACa,wBAAgB,GAAG,CAAC,CAAC;CAElC;CACa,wBAAgB,GAAG,CAAC,CAAC;CAElC;CACa,wBAAgB,GAAG,CAAC,CAAC;CAElC;CACa,uBAAe,GAAG,CAAC,CAAC;CAEjC;CACa,wBAAgB,GAAG,CAAC,CAAC;CAElC;CACa,2BAAmB,GAAG,CAAC,CAAC;CAErC;CACa,qBAAa,GAAG,CAAC,CAAC;CAE/B;CACa,yBAAiB,GAAG,CAAC,CAAC;CAEnC;CACa,sBAAc,GAAG,CAAC,CAAC;CAEhC;CACa,sBAAc,GAAG,EAAE,CAAC;CAEjC;CACa,wBAAgB,GAAG,EAAE,CAAC;CAEnC;CACa,2BAAmB,GAAG,EAAE,CAAC;CAEtC;CACa,sBAAc,GAAG,EAAE,CAAC;CAEjC;CACa,wBAAgB,GAAG,EAAE,CAAC;CAEnC;CACa,8BAAsB,GAAG,EAAE,CAAC;CAEzC;CACa,qBAAa,GAAG,EAAE,CAAC;CAEhC;CACa,2BAAmB,GAAG,EAAE,CAAC;CAEtC;CACa,sBAAc,GAAG,EAAE,CAAC;CAEjC;CACa,4BAAoB,GAAG,EAAE,CAAC;CAEvC;CACa,yBAAiB,GAAG,IAAI,CAAC;CAEtC;CACa,yBAAiB,GAAG,IAAI,CAAC;CAEtC;CACa,mCAA2B,GAAG,CAAC,CAAC;CAE7C;CACa,oCAA4B,GAAG,CAAC,CAAC;CAE9C;CACa,sCAA8B,GAAG,CAAC,CAAC;CAEhD;CACa,gCAAwB,GAAG,CAAC,CAAC;CAE1C;CACa,oCAA4B,GAAG,CAAC,CAAC;CAE9C;CACa,+BAAuB,GAAG,CAAC,CAAC;CAEzC;CACa,wCAAgC,GAAG,GAAG,CAAC;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;ACvGpB;AACG;AAEO;AAC6C;CAEvF,SAAgB,mBAAmB,CACjC,MAAgB,EAChB,kBAA4B,EAC5B,eAAyB;KAEzB,IAAI,WAAW,GAAG,CAAC,GAAG,CAAC,CAAC;KAExB,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;SACzB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;aACtC,WAAW,IAAI,gBAAgB,CAC7B,CAAC,CAAC,QAAQ,EAAE,EACZ,MAAM,CAAC,CAAC,CAAC,EACT,kBAAkB,EAClB,IAAI,EACJ,eAAe,CAChB,CAAC;UACH;MACF;UAAM;;SAGL,IAAI,MAAM,CAAC,MAAM,EAAE;aACjB,MAAM,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC;UAC1B;;SAGD,KAAK,IAAM,GAAG,IAAI,MAAM,EAAE;aACxB,WAAW,IAAI,gBAAgB,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,CAAC,EAAE,kBAAkB,EAAE,KAAK,EAAE,eAAe,CAAC,CAAC;UAC/F;MACF;KAED,OAAO,WAAW,CAAC;CACrB,CAAC;CA/BD,kDA+BC;CAED;CACA,SAAS,gBAAgB,CACvB,IAAY;CACZ;CACA,KAAU,EACV,kBAA0B,EAC1B,OAAe,EACf,eAAuB;KAFvB,mCAAA,EAAA,0BAA0B;KAC1B,wBAAA,EAAA,eAAe;KACf,gCAAA,EAAA,uBAAuB;;KAGvB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;SACzB,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;MACxB;KAED,QAAQ,OAAO,KAAK;SAClB,KAAK,QAAQ;aACX,OAAO,CAAC,GAAGiH,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,UAAU,CAAC,KAAK,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;SAC5F,KAAK,QAAQ;aACX,IACE,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK;iBAC3B,KAAK,IAAI,SAAS,CAAC,UAAU;iBAC7B,KAAK,IAAI,SAAS,CAAC,UAAU,EAC7B;iBACA,IAAI,KAAK,IAAI,SAAS,CAAC,cAAc,IAAI,KAAK,IAAI,SAAS,CAAC,cAAc,EAAE;;qBAE1E,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;kBAC3E;sBAAM;qBACL,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;kBAC3E;cACF;kBAAM;;iBAEL,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;cAC3E;SACH,KAAK,WAAW;aACd,IAAI,OAAO,IAAI,CAAC,eAAe;iBAC7B,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;aACtE,OAAO,CAAC,CAAC;SACX,KAAK,SAAS;aACZ,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;SAC5E,KAAK,QAAQ;aACX,IAAI,KAAK,IAAI,IAAI,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBACvF,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;cACrE;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;iBACjF,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,CAAC,CAAC;cAC5E;kBAAM,IAAI,KAAK,YAAY,IAAI,IAAIC,YAAM,CAAC,KAAK,CAAC,EAAE;iBACjD,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;cAC3E;kBAAM,IACL,WAAW,CAAC,MAAM,CAAC,KAAK,CAAC;iBACzB,KAAK,YAAY,WAAW;iBAC5BC,sBAAgB,CAAC,KAAK,CAAC,EACvB;iBACA,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,KAAK,CAAC,UAAU,EACzF;cACH;kBAAM,IACL,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM;iBAC7B,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ;iBAC/B,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAClC;iBACA,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;cAC3E;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBAC9C,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,CAAC,CAAC;cAC5E;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;;iBAExC,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,MAAM,GAAG,CAAC,EAAE;qBAC9D,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;yBACvD,CAAC;yBACD,CAAC;yBACD,CAAC;yBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,EAAE,MAAM,CAAC;yBAChD,CAAC;yBACD,mBAAmB,CAAC,KAAK,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACrE;kBACH;sBAAM;qBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;yBACvD,CAAC;yBACD,CAAC;yBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,EAAE,MAAM,CAAC;yBAChD,CAAC,EACD;kBACH;cACF;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;;iBAE1C,IAAI,KAAK,CAAC,QAAQ,KAAKI,aAAM,CAAC,kBAAkB,EAAE;qBAChD,QACE,CAAC,IAAI,IAAI,IAAI,GAAGJ,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;0BACtD,KAAK,CAAC,QAAQ,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EAChC;kBACH;sBAAM;qBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,KAAK,CAAC,QAAQ,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EACvF;kBACH;cACF;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,KAAK,EAAE,MAAM,CAAC;qBACtC,CAAC;qBACD,CAAC;qBACD,CAAC,EACD;cACH;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;;iBAEzC,IAAM,cAAc,GAAG,MAAM,CAAC,MAAM,CAClC;qBACE,IAAI,EAAE,KAAK,CAAC,UAAU;qBACtB,GAAG,EAAE,KAAK,CAAC,GAAG;kBACf,EACD,KAAK,CAAC,MAAM,CACb,CAAC;;iBAGF,IAAI,KAAK,CAAC,EAAE,IAAI,IAAI,EAAE;qBACpB,cAAc,CAAC,KAAK,CAAC,GAAG,KAAK,CAAC,EAAE,CAAC;kBAClC;iBAED,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvD,CAAC;qBACD,mBAAmB,CAAC,cAAc,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACxE;cACH;kBAAM,IAAI,KAAK,YAAY,MAAM,IAAIC,cAAQ,CAAC,KAAK,CAAC,EAAE;iBACrD,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvD,CAAC;qBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,MAAM,EAAE,MAAM,CAAC;qBACvC,CAAC;sBACA,KAAK,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;sBACrB,KAAK,CAAC,UAAU,GAAG,CAAC,GAAG,CAAC,CAAC;sBACzB,KAAK,CAAC,SAAS,GAAG,CAAC,GAAG,CAAC,CAAC;qBACzB,CAAC,EACD;cACH;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBAC9C,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvD,CAAC;qBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,MAAM,CAAC;qBACxC,CAAC;qBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,MAAM,CAAC;qBACxC,CAAC,EACD;cACH;kBAAM;iBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvD,mBAAmB,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC;qBAC/D,CAAC,EACD;cACH;SACH,KAAK,UAAU;;aAEb,IAAI,KAAK,YAAY,MAAM,IAAIC,cAAQ,CAAC,KAAK,CAAC,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,iBAAiB,EAAE;iBAC1F,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvD,CAAC;qBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,MAAM,EAAE,MAAM,CAAC;qBACvC,CAAC;sBACA,KAAK,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;sBACrB,KAAK,CAAC,UAAU,GAAG,CAAC,GAAG,CAAC,CAAC;sBACzB,KAAK,CAAC,SAAS,GAAG,CAAC,GAAG,CAAC,CAAC;qBACzB,CAAC,EACD;cACH;kBAAM;iBACL,IAAI,kBAAkB,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,MAAM,GAAG,CAAC,EAAE;qBACpF,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;yBACvD,CAAC;yBACD,CAAC;yBACD,CAAC;yBACDA,aAAM,CAAC,UAAU,CAACC,8BAAwB,CAAC,KAAK,CAAC,EAAE,MAAM,CAAC;yBAC1D,CAAC;yBACD,mBAAmB,CAAC,KAAK,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACrE;kBACH;sBAAM,IAAI,kBAAkB,EAAE;qBAC7B,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;yBACvD,CAAC;yBACD,CAAC;yBACDA,aAAM,CAAC,UAAU,CAACC,8BAAwB,CAAC,KAAK,CAAC,EAAE,MAAM,CAAC;yBAC1D,CAAC,EACD;kBACH;cACF;MACJ;KAED,OAAO,CAAC,CAAC;CACX,CAAC;;;;;;;;;;CClOD,IAAM,SAAS,GAAG,IAAI,CAAC;CACvB,IAAM,cAAc,GAAG,IAAI,CAAC;CAC5B,IAAM,gBAAgB,GAAG,IAAI,CAAC;CAC9B,IAAM,eAAe,GAAG,IAAI,CAAC;CAC7B,IAAM,eAAe,GAAG,IAAI,CAAC;CAE7B,IAAM,YAAY,GAAG,IAAI,CAAC;CAC1B,IAAM,cAAc,GAAG,IAAI,CAAC;CAC5B,IAAM,aAAa,GAAG,IAAI,CAAC;CAC3B,IAAM,eAAe,GAAG,IAAI,CAAC;CAE7B;;;;;;CAMA,SAAgB,YAAY,CAC1B,KAAkC,EAClC,KAAa,EACb,GAAW;KAEX,IAAI,YAAY,GAAG,CAAC,CAAC;KAErB,KAAK,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC,GAAG,GAAG,EAAE,CAAC,IAAI,CAAC,EAAE;SACnC,IAAM,IAAI,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;SAEtB,IAAI,YAAY,EAAE;aAChB,IAAI,CAAC,IAAI,GAAG,cAAc,MAAM,eAAe,EAAE;iBAC/C,OAAO,KAAK,CAAC;cACd;aACD,YAAY,IAAI,CAAC,CAAC;UACnB;cAAM,IAAI,IAAI,GAAG,SAAS,EAAE;aAC3B,IAAI,CAAC,IAAI,GAAG,gBAAgB,MAAM,YAAY,EAAE;iBAC9C,YAAY,GAAG,CAAC,CAAC;cAClB;kBAAM,IAAI,CAAC,IAAI,GAAG,eAAe,MAAM,cAAc,EAAE;iBACtD,YAAY,GAAG,CAAC,CAAC;cAClB;kBAAM,IAAI,CAAC,IAAI,GAAG,eAAe,MAAM,aAAa,EAAE;iBACrD,YAAY,GAAG,CAAC,CAAC;cAClB;kBAAM;iBACL,OAAO,KAAK,CAAC;cACd;UACF;MACF;KAED,OAAO,CAAC,YAAY,CAAC;CACvB,CAAC;CA7BD,oCA6BC;;;;;;;;;;AC9C+B;AACG;AAEJ;AACW;AACgB;AACf;AACR;AACD;AACH;AACK;AACA;AACG;AACA;AACA;AACE;AACO;CAgChD;CACA,IAAM,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;CAC9D,IAAM,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;CAE9D,IAAM,aAAa,GAAiC,EAAE,CAAC;CAEvD,SAAgB,WAAW,CACzB,MAAc,EACd,OAA2B,EAC3B,OAAiB;KAEjB,OAAO,GAAG,OAAO,IAAI,IAAI,GAAG,EAAE,GAAG,OAAO,CAAC;KACzC,IAAM,KAAK,GAAG,OAAO,IAAI,OAAO,CAAC,KAAK,GAAG,OAAO,CAAC,KAAK,GAAG,CAAC,CAAC;;KAE3D,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,CAAC;UACZ,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;UACvB,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;UACxB,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;KAE5B,IAAI,IAAI,GAAG,CAAC,EAAE;SACZ,MAAM,IAAI,KAAK,CAAC,gCAA8B,IAAM,CAAC,CAAC;MACvD;KAED,IAAI,OAAO,CAAC,gCAAgC,IAAI,MAAM,CAAC,MAAM,GAAG,IAAI,EAAE;SACpE,MAAM,IAAI,KAAK,CAAC,mBAAiB,MAAM,CAAC,MAAM,8BAAyB,IAAM,CAAC,CAAC;MAChF;KAED,IAAI,CAAC,OAAO,CAAC,gCAAgC,IAAI,MAAM,CAAC,MAAM,KAAK,IAAI,EAAE;SACvE,MAAM,IAAI,KAAK,CAAC,mBAAiB,MAAM,CAAC,MAAM,4BAAuB,IAAM,CAAC,CAAC;MAC9E;KAED,IAAI,IAAI,GAAG,KAAK,GAAG,MAAM,CAAC,UAAU,EAAE;SACpC,MAAM,IAAI,KAAK,CACb,gBAAc,IAAI,yBAAoB,KAAK,kCAA6B,MAAM,CAAC,UAAU,MAAG,CAC7F,CAAC;MACH;;KAGD,IAAI,MAAM,CAAC,KAAK,GAAG,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC,EAAE;SAClC,MAAM,IAAI,KAAK,CAAC,6EAA6E,CAAC,CAAC;MAChG;;KAGD,OAAO,iBAAiB,CAAC,MAAM,EAAE,KAAK,EAAE,OAAO,EAAE,OAAO,CAAC,CAAC;CAC5D,CAAC;CAvCD,kCAuCC;CAED,SAAS,iBAAiB,CACxBxX,QAAc,EACd,KAAa,EACb,OAA2B,EAC3B,OAAe;KAAf,wBAAA,EAAA,eAAe;KAEf,IAAM,aAAa,GAAG,OAAO,CAAC,eAAe,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,eAAe,CAAC,CAAC;KAC1F,IAAM,cAAc,GAAG,OAAO,CAAC,gBAAgB,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,gBAAgB,CAAC,CAAC;KAE7F,IAAM,WAAW,GAAG,OAAO,CAAC,aAAa,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,aAAa,CAAC,CAAC;;KAGnF,IAAM,GAAG,GAAG,OAAO,CAAC,KAAK,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,KAAK,CAAC,CAAC;;KAG5D,IAAM,UAAU,GAAG,OAAO,OAAO,CAAC,YAAY,CAAC,KAAK,SAAS,GAAG,OAAO,CAAC,YAAY,CAAC,GAAG,KAAK,CAAC;;KAG9F,IAAM,cAAc,GAAG,OAAO,CAAC,gBAAgB,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,gBAAgB,CAAC,CAAC;KAC7F,IAAM,YAAY,GAAG,OAAO,CAAC,cAAc,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,cAAc,CAAC,CAAC;KACtF,IAAM,aAAa,GAAG,OAAO,CAAC,eAAe,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,eAAe,CAAC,CAAC;;KAGzF,IAAM,UAAU,GAAG,KAAK,CAAC;;KAGzB,IAAIA,QAAM,CAAC,MAAM,GAAG,CAAC;SAAE,MAAM,IAAI,KAAK,CAAC,qCAAqC,CAAC,CAAC;;KAG9E,IAAM,IAAI,GACRA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;KAG/F,IAAI,IAAI,GAAG,CAAC,IAAI,IAAI,GAAGA,QAAM,CAAC,MAAM;SAAE,MAAM,IAAI,KAAK,CAAC,sBAAsB,CAAC,CAAC;;KAG9E,IAAM,MAAM,GAAa,OAAO,GAAG,EAAE,GAAG,EAAE,CAAC;;KAE3C,IAAI,UAAU,GAAG,CAAC,CAAC;KACnB,IAAM,IAAI,GAAG,KAAK,CAAC;;KAGnB,OAAO,CAAC,IAAI,EAAE;;SAEZ,IAAM,WAAW,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,CAAC;;SAGpC,IAAI,WAAW,KAAK,CAAC;aAAE,MAAM;;SAG7B,IAAI,CAAC,GAAG,KAAK,CAAC;;SAEd,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;aAC9C,CAAC,EAAE,CAAC;UACL;;SAGD,IAAI,CAAC,IAAIA,QAAM,CAAC,UAAU;aAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;SAClF,IAAM,IAAI,GAAG,OAAO,GAAG,UAAU,EAAE,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;SACxE,IAAI,KAAK,SAAA,CAAC;SAEV,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;SAEd,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;aAC9C,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IACE,UAAU,IAAI,CAAC;iBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;iBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;aAE/C,IAAI,CAAC0d,0BAAY,CAAC1d,QAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,EAAE;iBACxD,MAAM,IAAI,KAAK,CAAC,uCAAuC,CAAC,CAAC;cAC1D;aAED,KAAK,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;aAE/D,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;UAC5B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,EAAE;aAClD,IAAM,GAAG,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;aAC7BvX,QAAM,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;aACvC,KAAK,GAAG,IAAIgd,iBAAQ,CAAC,GAAG,CAAC,CAAC;aAC1B,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;UACpB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,IAAI,aAAa,KAAK,KAAK,EAAE;aAC7E,KAAK,GAAG,IAAIE,YAAK,CACfld,QAAM,CAAC,KAAK,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAC7F,CAAC;UACH;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,EAAE;aAClD,KAAK;iBACHA,QAAM,CAAC,KAAK,EAAE,CAAC;sBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;sBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;sBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;UAC3B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,aAAa,KAAK,KAAK,EAAE;aAChF,KAAK,GAAG,IAAI,eAAM,CAACA,QAAM,CAAC,YAAY,CAAC,KAAK,CAAC,CAAC,CAAC;aAC/C,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;UACnB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;aACrD,KAAK,GAAGA,QAAM,CAAC,YAAY,CAAC,KAAK,CAAC,CAAC;aACnC,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;UACnB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;aACnD,IAAM,OAAO,GACXA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,KAAK,GAAG,IAAI,IAAI,CAAC,IAAI,WAAI,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC,QAAQ,EAAE,CAAC,CAAC;UAC1D;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;aACtD,IAAIA,QAAM,CAAC,KAAK,CAAC,KAAK,CAAC,IAAIA,QAAM,CAAC,KAAK,CAAC,KAAK,CAAC;iBAAE,MAAM,IAAI,KAAK,CAAC,4BAA4B,CAAC,CAAC;aAC9F,KAAK,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,KAAK,CAAC,CAAC;UAC/B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;aACrD,IAAM,MAAM,GAAG,KAAK,CAAC;aACrB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;kBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;kBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;kBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;aAC5B,IAAI,UAAU,IAAI,CAAC,IAAI,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBACvD,MAAM,IAAI,KAAK,CAAC,sCAAsC,CAAC,CAAC;;aAG1D,IAAI,GAAG,EAAE;iBACP,KAAK,GAAGA,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,CAAC;cACjD;kBAAM;iBACL,KAAK,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC;cAC3D;aAED,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;UAC5B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,eAAe,EAAE;aACpD,IAAM,MAAM,GAAG,KAAK,CAAC;aACrB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;kBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;kBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;kBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;aAC5B,IAAI,YAAY,GAAG,OAAO,CAAC;;aAG3B,IAAM,SAAS,GAAG,KAAK,GAAG,UAAU,CAAC;;aAGrC,IAAI,WAAW,IAAI,WAAW,CAAC,IAAI,CAAC,EAAE;iBACpC,YAAY,GAAG,EAAE,CAAC;iBAClB,KAAK,IAAM,CAAC,IAAI,OAAO,EAAE;qBACtB,YAEC,CAAC,CAAC,CAAC,GAAG,OAAO,CAAC,CAA6B,CAAC,CAAC;kBAChD;iBACD,YAAY,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC;cAC5B;aAED,KAAK,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,YAAY,EAAE,IAAI,CAAC,CAAC;aAC9D,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;aAE3B,IAAIA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,KAAK,CAAC;iBAAE,MAAM,IAAI,KAAK,CAAC,+BAA+B,CAAC,CAAC;aAC9E,IAAI,KAAK,KAAK,SAAS;iBAAE,MAAM,IAAI,KAAK,CAAC,sBAAsB,CAAC,CAAC;UAClE;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;aACxD,KAAK,GAAG,SAAS,CAAC;UACnB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;aACnD,KAAK,GAAG,IAAI,CAAC;UACd;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;;aAEnD,IAAM,OAAO,GACXA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IAAM,IAAI,GAAG,IAAI,WAAI,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;;aAEzC,IAAI,YAAY,IAAI,aAAa,KAAK,IAAI,EAAE;iBAC1C,KAAK;qBACH,IAAI,CAAC,eAAe,CAAC,eAAe,CAAC,IAAI,IAAI,CAAC,kBAAkB,CAAC,eAAe,CAAC;2BAC7E,IAAI,CAAC,QAAQ,EAAE;2BACf,IAAI,CAAC;cACZ;kBAAM;iBACL,KAAK,GAAG,IAAI,CAAC;cACd;UACF;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,oBAAoB,EAAE;;aAEzD,IAAM,KAAK,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;;aAE/BvX,QAAM,CAAC,IAAI,CAAC,KAAK,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;;aAEzC,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;;aAEnB,IAAM2d,YAAU,GAAG,IAAIR,qBAAU,CAAC,KAAK,CAAyC,CAAC;;aAEjF,IAAI,UAAU,IAAIQ,YAAU,IAAI,OAAOA,YAAU,CAAC,QAAQ,KAAK,UAAU,EAAE;iBACzE,KAAK,GAAGA,YAAU,CAAC,QAAQ,EAAE,CAAC;cAC/B;kBAAM;iBACL,KAAK,GAAGA,YAAU,CAAC;cACpB;UACF;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;aACrD,IAAI,UAAU,GACZ3d,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IAAM,eAAe,GAAG,UAAU,CAAC;aACnC,IAAM,OAAO,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,CAAC;;aAGhC,IAAI,UAAU,GAAG,CAAC;iBAAE,MAAM,IAAI,KAAK,CAAC,yCAAyC,CAAC,CAAC;;aAG/E,IAAI,UAAU,GAAGA,QAAM,CAAC,UAAU;iBAChC,MAAM,IAAI,KAAK,CAAC,4CAA4C,CAAC,CAAC;;aAGhE,IAAIA,QAAM,CAAC,OAAO,CAAC,IAAI,IAAI,EAAE;;iBAE3B,IAAI,OAAO,KAAK2X,aAAM,CAAC,kBAAkB,EAAE;qBACzC,UAAU;yBACR3X,QAAM,CAAC,KAAK,EAAE,CAAC;8BACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;8BACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;8BACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;qBAC1B,IAAI,UAAU,GAAG,CAAC;yBAChB,MAAM,IAAI,KAAK,CAAC,0DAA0D,CAAC,CAAC;qBAC9E,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;yBAClC,MAAM,IAAI,KAAK,CAAC,6DAA6D,CAAC,CAAC;qBACjF,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;yBAClC,MAAM,IAAI,KAAK,CAAC,8DAA8D,CAAC,CAAC;kBACnF;iBAED,IAAI,cAAc,IAAI,aAAa,EAAE;qBACnC,KAAK,GAAGA,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,CAAC;kBACjD;sBAAM;qBACL,KAAK,GAAG,IAAI2X,aAAM,CAAC3X,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,EAAE,OAAO,CAAC,CAAC;kBACtE;cACF;kBAAM;iBACL,IAAM,OAAO,GAAGuX,aAAM,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC;;iBAEzC,IAAI,OAAO,KAAKI,aAAM,CAAC,kBAAkB,EAAE;qBACzC,UAAU;yBACR3X,QAAM,CAAC,KAAK,EAAE,CAAC;8BACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;8BACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;8BACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;qBAC1B,IAAI,UAAU,GAAG,CAAC;yBAChB,MAAM,IAAI,KAAK,CAAC,0DAA0D,CAAC,CAAC;qBAC9E,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;yBAClC,MAAM,IAAI,KAAK,CAAC,6DAA6D,CAAC,CAAC;qBACjF,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;yBAClC,MAAM,IAAI,KAAK,CAAC,8DAA8D,CAAC,CAAC;kBACnF;;iBAGD,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,UAAU,EAAE,CAAC,EAAE,EAAE;qBAC/B,OAAO,CAAC,CAAC,CAAC,GAAGA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,CAAC;kBAChC;iBAED,IAAI,cAAc,IAAI,aAAa,EAAE;qBACnC,KAAK,GAAG,OAAO,CAAC;kBACjB;sBAAM;qBACL,KAAK,GAAG,IAAI2X,aAAM,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;kBACtC;cACF;;aAGD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;UAC5B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,UAAU,KAAK,KAAK,EAAE;;aAE7E,CAAC,GAAG,KAAK,CAAC;;aAEV,OAAO3X,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;iBAC9C,CAAC,EAAE,CAAC;cACL;;aAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;iBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;aAE9E,IAAM,MAAM,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;;aAEjD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;aAGd,CAAC,GAAG,KAAK,CAAC;;aAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;iBAC9C,CAAC,EAAE,CAAC;cACL;;aAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;iBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;aAE9E,IAAM,aAAa,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;aACxD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;aAGd,IAAM,YAAY,GAAG,IAAI,KAAK,CAAC,aAAa,CAAC,MAAM,CAAC,CAAC;;aAGrD,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,aAAa,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;iBACzC,QAAQ,aAAa,CAAC,CAAC,CAAC;qBACtB,KAAK,GAAG;yBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;yBACtB,MAAM;qBACR,KAAK,GAAG;yBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;yBACtB,MAAM;qBACR,KAAK,GAAG;yBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;yBACtB,MAAM;kBACT;cACF;aAED,KAAK,GAAG,IAAI,MAAM,CAAC,MAAM,EAAE,YAAY,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC;UACnD;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,UAAU,KAAK,IAAI,EAAE;;aAE5E,CAAC,GAAG,KAAK,CAAC;;aAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;iBAC9C,CAAC,EAAE,CAAC;cACL;;aAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;iBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;aAE9E,IAAM,MAAM,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;aACjD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;aAGd,CAAC,GAAG,KAAK,CAAC;;aAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;iBAC9C,CAAC,EAAE,CAAC;cACL;;aAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;iBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;aAE9E,IAAM,aAAa,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;aACxD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;aAGd,KAAK,GAAG,IAAIsd,iBAAU,CAAC,MAAM,EAAE,aAAa,CAAC,CAAC;UAC/C;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;aACrD,IAAM,UAAU,GACdtd,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IACE,UAAU,IAAI,CAAC;iBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;iBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;aAC/C,IAAM4d,QAAM,GAAG5d,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;aACtE,KAAK,GAAG,aAAa,GAAG4d,QAAM,GAAG,IAAIX,iBAAU,CAACW,QAAM,CAAC,CAAC;aACxD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;UAC5B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;aACxD,IAAM,OAAO,GACX5d,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAE1B,KAAK,GAAG,IAAIud,mBAAS,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;UAC1C;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;aACtD,KAAK,GAAG,IAAIH,cAAM,EAAE,CAAC;UACtB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;aACtD,KAAK,GAAG,IAAIC,cAAM,EAAE,CAAC;UACtB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;aACnD,IAAM,UAAU,GACdrd,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IACE,UAAU,IAAI,CAAC;iBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;iBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;aAC/C,IAAM,cAAc,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;aAG9E,IAAI,aAAa,EAAE;;iBAEjB,IAAI,cAAc,EAAE;;qBAElB,KAAK,GAAG,WAAW,CAAC,cAAc,EAAE,aAAa,EAAE,MAAM,CAAC,CAAC;kBAC5D;sBAAM;qBACL,KAAK,GAAG,WAAW,CAAC,cAAc,CAAC,CAAC;kBACrC;cACF;kBAAM;iBACL,KAAK,GAAG,IAAIwd,SAAI,CAAC,cAAc,CAAC,CAAC;cAClC;;aAGD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;UAC5B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,sBAAsB,EAAE;aAC3D,IAAM,SAAS,GACbxd,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;aAG1B,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;iBAC7B,MAAM,IAAI,KAAK,CAAC,yDAAyD,CAAC,CAAC;cAC5E;;aAGD,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;aAE1B,IACE,UAAU,IAAI,CAAC;iBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;iBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;;aAG/C,IAAM,cAAc,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;aAE9E,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;aAE3B,IAAM,MAAM,GAAG,KAAK,CAAC;;aAErB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;kBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;kBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;kBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;;aAE5B,IAAM,WAAW,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC;;aAEtE,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;aAG3B,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,UAAU,GAAG,UAAU,EAAE;iBAC/C,MAAM,IAAI,KAAK,CAAC,wDAAwD,CAAC,CAAC;cAC3E;;aAGD,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,UAAU,GAAG,UAAU,EAAE;iBAC/C,MAAM,IAAI,KAAK,CAAC,2DAA2D,CAAC,CAAC;cAC9E;;aAGD,IAAI,aAAa,EAAE;;iBAEjB,IAAI,cAAc,EAAE;;qBAElB,KAAK,GAAG,WAAW,CAAC,cAAc,EAAE,aAAa,EAAE,MAAM,CAAC,CAAC;kBAC5D;sBAAM;qBACL,KAAK,GAAG,WAAW,CAAC,cAAc,CAAC,CAAC;kBACrC;iBAED,KAAK,CAAC,KAAK,GAAG,WAAW,CAAC;cAC3B;kBAAM;iBACL,KAAK,GAAG,IAAIwd,SAAI,CAAC,cAAc,EAAE,WAAW,CAAC,CAAC;cAC/C;UACF;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;;aAExD,IAAM,UAAU,GACdxd,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;aAE1B,IACE,UAAU,IAAI,CAAC;iBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;iBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;;aAE/C,IAAI,CAAC0d,0BAAY,CAAC1d,QAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,EAAE;iBACxD,MAAM,IAAI,KAAK,CAAC,uCAAuC,CAAC,CAAC;cAC1D;aACD,IAAM,SAAS,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;aAEzE,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;aAG3B,IAAM,SAAS,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;aACnCvX,QAAM,CAAC,IAAI,CAAC,SAAS,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;aAC7C,IAAM,GAAG,GAAG,IAAIgd,iBAAQ,CAAC,SAAS,CAAC,CAAC;;aAGpC,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;;aAGnB,KAAK,GAAG,IAAIS,YAAK,CAAC,SAAS,EAAE,GAAG,CAAC,CAAC;UACnC;cAAM;aACL,MAAM,IAAI,KAAK,CACb,6BAA6B,GAAG,WAAW,CAAC,QAAQ,CAAC,EAAE,CAAC,GAAG,kBAAkB,GAAG,IAAI,GAAG,GAAG,CAC3F,CAAC;UACH;SACD,IAAI,IAAI,KAAK,WAAW,EAAE;aACxB,MAAM,CAAC,cAAc,CAAC,MAAM,EAAE,IAAI,EAAE;iBAClC,KAAK,OAAA;iBACL,QAAQ,EAAE,IAAI;iBACd,UAAU,EAAE,IAAI;iBAChB,YAAY,EAAE,IAAI;cACnB,CAAC,CAAC;UACJ;cAAM;aACL,MAAM,CAAC,IAAI,CAAC,GAAG,KAAK,CAAC;UACtB;MACF;;KAGD,IAAI,IAAI,KAAK,KAAK,GAAG,UAAU,EAAE;SAC/B,IAAI,OAAO;aAAE,MAAM,IAAI,KAAK,CAAC,oBAAoB,CAAC,CAAC;SACnD,MAAM,IAAI,KAAK,CAAC,qBAAqB,CAAC,CAAC;MACxC;;KAGD,IAAM,UAAU,GAAG,MAAM,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,MAAM,CAAC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,GAAA,CAAC,CAAC;KACtE,IAAI,KAAK,GAAG,IAAI,CAAC;KACjB,UAAU,CAAC,OAAO,CAAC,UAAA,CAAC;SAClB,IAAI,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC;aAAE,KAAK,GAAG,KAAK,CAAC;MAC7D,CAAC,CAAC;;KAGH,IAAI,CAAC,KAAK;SAAE,OAAO,MAAM,CAAC;KAE1B,IAAIA,kBAAW,CAAC,MAAM,CAAC,EAAE;SACvB,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,MAAM,CAAuB,CAAC;SAC7D,OAAO,IAAI,CAAC,IAAI,CAAC;SACjB,OAAO,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO,IAAIA,YAAK,CAAC,MAAM,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;MAC7D;KAED,OAAO,MAAM,CAAC;CAChB,CAAC;CAED;;;;;CAKA,SAAS,WAAW,CAClB,cAAsB,EACtB,aAA4C,EAC5C,MAAiB;KAEjB,IAAI,CAAC,aAAa;SAAE,OAAO,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC;;KAExD,IAAI,aAAa,CAAC,cAAc,CAAC,IAAI,IAAI,EAAE;SACzC,aAAa,CAAC,cAAc,CAAC,GAAG,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC;MAC9D;;KAGD,OAAO,aAAa,CAAC,cAAc,CAAC,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;CACpD,CAAC;;;;;;;;CCxpBD;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;;CAIA,SAAgB,WAAW,CACzB,MAAyB,EACzB,MAAc,EACd,MAAwB,EACxB,IAAY,EACZ,MAAc;KAEd,IAAI,CAAS,CAAC;KACd,IAAI,CAAS,CAAC;KACd,IAAM,GAAG,GAAG,MAAM,KAAK,KAAK,CAAC;KAC7B,IAAM,IAAI,GAAG,MAAM,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;KACnC,IAAM,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;KAC7B,IAAM,KAAK,GAAG,IAAI,IAAI,CAAC,CAAC;KACxB,IAAI,KAAK,GAAG,CAAC,CAAC,CAAC;KACf,IAAI,CAAC,GAAG,GAAG,GAAG,CAAC,GAAG,MAAM,GAAG,CAAC,CAAC;KAC7B,IAAM,CAAC,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,CAAC,CAAC;KACvB,IAAI,CAAC,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;KAE3B,CAAC,IAAI,CAAC,CAAC;KAEP,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC,IAAI,CAAC,KAAK,IAAI,CAAC,CAAC,CAAC;KAC5B,CAAC,KAAK,CAAC,KAAK,CAAC;KACb,KAAK,IAAI,IAAI,CAAC;KACd,OAAO,KAAK,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,KAAK,IAAI,CAAC;SAAC,CAAC;KAExE,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC,IAAI,CAAC,KAAK,IAAI,CAAC,CAAC,CAAC;KAC5B,CAAC,KAAK,CAAC,KAAK,CAAC;KACb,KAAK,IAAI,IAAI,CAAC;KACd,OAAO,KAAK,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,KAAK,IAAI,CAAC;SAAC,CAAC;KAExE,IAAI,CAAC,KAAK,CAAC,EAAE;SACX,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;MACf;UAAM,IAAI,CAAC,KAAK,IAAI,EAAE;SACrB,OAAO,CAAC,GAAG,GAAG,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,QAAQ,CAAC;MAC1C;UAAM;SACL,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;SAC1B,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;MACf;KACD,OAAO,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,CAAC;CAClD,CAAC;CAvCD,kCAuCC;CAED,SAAgB,YAAY,CAC1B,MAAyB,EACzB,KAAa,EACb,MAAc,EACd,MAAwB,EACxB,IAAY,EACZ,MAAc;KAEd,IAAI,CAAS,CAAC;KACd,IAAI,CAAS,CAAC;KACd,IAAI,CAAS,CAAC;KACd,IAAM,GAAG,GAAG,MAAM,KAAK,KAAK,CAAC;KAC7B,IAAI,IAAI,GAAG,MAAM,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;KACjC,IAAM,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;KAC7B,IAAM,KAAK,GAAG,IAAI,IAAI,CAAC,CAAC;KACxB,IAAM,EAAE,GAAG,IAAI,KAAK,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,CAAC,CAAC;KACjE,IAAI,CAAC,GAAG,GAAG,GAAG,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;KAC7B,IAAM,CAAC,GAAG,GAAG,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;KACvB,IAAM,CAAC,GAAG,KAAK,GAAG,CAAC,KAAK,KAAK,KAAK,CAAC,IAAI,CAAC,GAAG,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;KAE9D,KAAK,GAAG,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC;KAExB,IAAI,KAAK,CAAC,KAAK,CAAC,IAAI,KAAK,KAAK,QAAQ,EAAE;SACtC,CAAC,GAAG,KAAK,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;SACzB,CAAC,GAAG,IAAI,CAAC;MACV;UAAM;SACL,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC;SAC3C,IAAI,KAAK,IAAI,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,EAAE;aACrC,CAAC,EAAE,CAAC;aACJ,CAAC,IAAI,CAAC,CAAC;UACR;SACD,IAAI,CAAC,GAAG,KAAK,IAAI,CAAC,EAAE;aAClB,KAAK,IAAI,EAAE,GAAG,CAAC,CAAC;UACjB;cAAM;aACL,KAAK,IAAI,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,GAAG,KAAK,CAAC,CAAC;UACtC;SACD,IAAI,KAAK,GAAG,CAAC,IAAI,CAAC,EAAE;aAClB,CAAC,EAAE,CAAC;aACJ,CAAC,IAAI,CAAC,CAAC;UACR;SAED,IAAI,CAAC,GAAG,KAAK,IAAI,IAAI,EAAE;aACrB,CAAC,GAAG,CAAC,CAAC;aACN,CAAC,GAAG,IAAI,CAAC;UACV;cAAM,IAAI,CAAC,GAAG,KAAK,IAAI,CAAC,EAAE;aACzB,CAAC,GAAG,CAAC,KAAK,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;aACxC,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;UACf;cAAM;aACL,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,KAAK,GAAG,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;aACvD,CAAC,GAAG,CAAC,CAAC;UACP;MACF;KAED,IAAI,KAAK,CAAC,KAAK,CAAC;SAAE,CAAC,GAAG,CAAC,CAAC;KAExB,OAAO,IAAI,IAAI,CAAC,EAAE;SAChB,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC;SAC9B,CAAC,IAAI,CAAC,CAAC;SACP,CAAC,IAAI,GAAG,CAAC;SACT,IAAI,IAAI,CAAC,CAAC;MACX;KAED,CAAC,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;KAEpB,IAAI,KAAK,CAAC,KAAK,CAAC;SAAE,CAAC,IAAI,CAAC,CAAC;KAEzB,IAAI,IAAI,IAAI,CAAC;KAEb,OAAO,IAAI,GAAG,CAAC,EAAE;SACf,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC;SAC9B,CAAC,IAAI,CAAC,CAAC;SACP,CAAC,IAAI,GAAG,CAAC;SACT,IAAI,IAAI,CAAC,CAAC;MACX;KAED,MAAM,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC;CACpC,CAAC;CA5ED,oCA4EC;;;;;;;;;;;ACtJkC;AAGO;AAIM;AACF;AACC;AAEhB;AACF;AAYZ;CAgBjB,IAAM,MAAM,GAAG,MAAM,CAAC;CACtB,IAAM,UAAU,GAAG,IAAI,GAAG,CAAC,CAAC,KAAK,EAAE,MAAM,EAAE,KAAK,EAAE,cAAc,CAAC,CAAC,CAAC;CAEnE;;;;;CAMA,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,GAAG,CAAC,CAAC;KACzC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;KAEtB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;KAE/D,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,EAAE,IAAI,IAAI,CAAC;KAC9C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,EAAE,IAAI,IAAI,CAAC;KAC9C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,CAAC,IAAI,IAAI,CAAC;KAC7C,MAAM,CAAC,KAAK,CAAC,GAAG,CAAC,IAAI,GAAG,CAAC,IAAI,IAAI,CAAC;;KAElC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,CAAC;;KAEzB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;;KAIjB,IACE,MAAM,CAAC,SAAS,CAAC,KAAK,CAAC;SACvB,KAAK,IAAI,SAAS,CAAC,cAAc;SACjC,KAAK,IAAI,SAAS,CAAC,cAAc,EACjC;;;SAGA,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;SAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;eACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;eAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;SAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;SACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;SAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC;SAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,CAAC,IAAI,IAAI,CAAC;SACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;SACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;MACxC;UAAM;;SAEL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;SAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;eACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;eAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;SAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;SACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;SAEpBI,yBAAY,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,EAAE,QAAQ,EAAE,EAAE,EAAE,CAAC,CAAC,CAAC;;SAEpD,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;MACnB;KAED,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,CAAU,EAAE,KAAa,EAAE,OAAiB;;KAE9F,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;KAG3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,gBAAgB,CACvB,MAAc,EACd,GAAW,EACX,KAAc,EACd,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;;KAE9C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;KAChC,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,KAAW,EAAE,KAAa,EAAE,OAAiB;;KAE/F,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;KAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAGpB,IAAM,WAAW,GAAG,WAAI,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,CAAC,CAAC;KACrD,IAAM,OAAO,GAAG,WAAW,CAAC,UAAU,EAAE,CAAC;KACzC,IAAM,QAAQ,GAAG,WAAW,CAAC,WAAW,EAAE,CAAC;;KAE3C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,OAAO,GAAG,IAAI,CAAC;KACjC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,CAAC,IAAI,IAAI,CAAC;KACxC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;KACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;;KAEzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;KAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;KACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;KAC1C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;KAC1C,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,IAAI,KAAK,CAAC,MAAM,IAAI,KAAK,CAAC,MAAM,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;SACtD,MAAM,KAAK,CAAC,QAAQ,GAAG,KAAK,CAAC,MAAM,GAAG,8BAA8B,CAAC,CAAC;MACvE;;KAED,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,MAAM,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;KAErE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;KAEvB,IAAI,KAAK,CAAC,UAAU;SAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;KAC7C,IAAI,KAAK,CAAC,MAAM;SAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;KACzC,IAAI,KAAK,CAAC,SAAS;SAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;KAG5C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;KACvB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,mBAAmB,CAC1B,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAGpB,IAAI,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;SAGvC,MAAM,KAAK,CAAC,UAAU,GAAG,KAAK,CAAC,OAAO,GAAG,8BAA8B,CAAC,CAAC;MAC1E;;KAGD,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,OAAO,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;KAEtE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;KAEvB,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;KAEhG,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;KACvB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAsB,EACtB,KAAa,EACb,OAAiB;;KAGjB,IAAI,KAAK,KAAK,IAAI,EAAE;SAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;MAC5C;UAAM,IAAI,KAAK,CAAC,SAAS,KAAK,QAAQ,EAAE;SACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;MAC/C;UAAM;SACL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;MAC/C;;KAGD,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,iBAAiB,CACxB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;KAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAGpB,IAAI,OAAO,KAAK,CAAC,EAAE,KAAK,QAAQ,EAAE;SAChC,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,EAAE,EAAE,KAAK,EAAE,SAAS,EAAE,QAAQ,CAAC,CAAC;MACpD;UAAM,IAAIrG,kBAAY,CAAC,KAAK,CAAC,EAAE,CAAC,EAAE;;;SAGjC,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,QAAQ,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE,KAAK,CAAC,CAAC;MAC7C;UAAM;SACL,MAAM,IAAI,SAAS,CAAC,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,GAAG,2BAA2B,CAAC,CAAC;MACvF;;KAGD,OAAO,KAAK,GAAG,EAAE,CAAC;CACpB,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAA0B,EAC1B,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,IAAM,IAAI,GAAG,KAAK,CAAC,MAAM,CAAC;;KAE1B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;KAEtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,2BAA2B,CAAC;;KAExD,MAAM,CAAC,GAAG,CAACC,0BAAY,CAAC,KAAK,CAAC,EAAE,KAAK,CAAC,CAAC;;KAEvC,KAAK,GAAG,KAAK,GAAG,IAAI,CAAC;KACrB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,SAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,OAAe,EACf,IAAqB;KALrB,0BAAA,EAAA,iBAAiB;KACjB,sBAAA,EAAA,SAAS;KACT,mCAAA,EAAA,0BAA0B;KAC1B,gCAAA,EAAA,sBAAsB;KACtB,wBAAA,EAAA,eAAe;KACf,qBAAA,EAAA,SAAqB;KAErB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;SACpC,IAAI,IAAI,CAAC,CAAC,CAAC,KAAK,KAAK;aAAE,MAAM,IAAI,KAAK,CAAC,4BAA4B,CAAC,CAAC;MACtE;;KAGD,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;;KAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,GAAG,SAAS,CAAC,eAAe,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAEhG,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,IAAM,QAAQ,GAAG,aAAa,CAC5B,MAAM,EACN,KAAK,EACL,SAAS,EACT,KAAK,EACL,KAAK,GAAG,CAAC,EACT,kBAAkB,EAClB,eAAe,EACf,IAAI,CACL,CAAC;;KAEF,IAAI,CAAC,GAAG,EAAE,CAAC;KACX,OAAO,QAAQ,CAAC;CAClB,CAAC;CAED,SAAS,mBAAmB,CAC1B,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;KAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,oBAAoB,CAAC;;KAEjD,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;;;KAIpB,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,KAAK,CAAC,QAAQ,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE,KAAK,CAAC,CAAC;KAC/C,OAAO,KAAK,GAAG,EAAE,CAAC;CACpB,CAAC;CAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,KAAW,EAAE,KAAa,EAAE,OAAiB;;KAE/F,MAAM,CAAC,KAAK,EAAE,CAAC;SACb,KAAK,CAAC,SAAS,KAAK,MAAM,GAAG,SAAS,CAAC,cAAc,GAAG,SAAS,CAAC,mBAAmB,CAAC;;KAExF,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,IAAM,OAAO,GAAG,KAAK,CAAC,UAAU,EAAE,CAAC;KACnC,IAAM,QAAQ,GAAG,KAAK,CAAC,WAAW,EAAE,CAAC;;KAErC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,OAAO,GAAG,IAAI,CAAC;KACjC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,CAAC,IAAI,IAAI,CAAC;KACxC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;KACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;;KAEzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;KAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;KACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;KAC1C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;KAC1C,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,cAAc,CACrB,MAAc,EACd,GAAW,EACX,KAAqB,EACrB,KAAa,EACb,OAAiB;KAEjB,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;;KAExB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;KAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC;KAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,CAAC,IAAI,IAAI,CAAC;KACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;KACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;KACvC,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAG7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAGpBoG,yBAAY,CAAC,MAAM,EAAE,KAAK,CAAC,KAAK,EAAE,KAAK,EAAE,QAAQ,EAAE,EAAE,EAAE,CAAC,CAAC,CAAC;;KAG1D,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;KAClB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,iBAAiB,CACxB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,UAAkB,EAClB,MAAU,EACV,OAAiB;KAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;KAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,IAAM,cAAc,GAAGrG,8BAAwB,CAAC,KAAK,CAAC,CAAC;;KAGvD,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;KAE5E,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;KAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;KAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,aAAa,CACpB,MAAc,EACd,GAAW,EACX,KAAW,EACX,KAAa,EACb,SAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,OAAe;KAJf,0BAAA,EAAA,iBAAiB;KACjB,sBAAA,EAAA,SAAS;KACT,mCAAA,EAAA,0BAA0B;KAC1B,gCAAA,EAAA,sBAAsB;KACtB,wBAAA,EAAA,eAAe;KAEf,IAAI,KAAK,CAAC,KAAK,IAAI,OAAO,KAAK,CAAC,KAAK,KAAK,QAAQ,EAAE;;SAElD,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,sBAAsB,CAAC;;SAEnD,IAAM,oBAAoB,GAAG,CAAC,OAAO;eACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;eAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;SAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;SACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;SAGpB,IAAI,UAAU,GAAG,KAAK,CAAC;;;SAIvB,IAAM,cAAc,GAAG,OAAO,KAAK,CAAC,IAAI,KAAK,QAAQ,GAAG,KAAK,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC;;SAE3F,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;;SAElB,IAAM,QAAQ,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;SAEhF,MAAM,CAAC,KAAK,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;SAChC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;SAC3C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;SAC5C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;;SAE5C,MAAM,CAAC,KAAK,GAAG,CAAC,GAAG,QAAQ,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;SAErC,KAAK,GAAG,KAAK,GAAG,QAAQ,GAAG,CAAC,CAAC;;;SAI7B,IAAM,QAAQ,GAAG,aAAa,CAC5B,MAAM,EACN,KAAK,CAAC,KAAK,EACX,SAAS,EACT,KAAK,EACL,KAAK,GAAG,CAAC,EACT,kBAAkB,EAClB,eAAe,CAChB,CAAC;SACF,KAAK,GAAG,QAAQ,GAAG,CAAC,CAAC;;SAGrB,IAAM,SAAS,GAAG,QAAQ,GAAG,UAAU,CAAC;;SAGxC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,SAAS,GAAG,IAAI,CAAC;SACxC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,CAAC,IAAI,IAAI,CAAC;SAC/C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,EAAE,IAAI,IAAI,CAAC;SAChD,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,EAAE,IAAI,IAAI,CAAC;;SAEhD,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;MACrB;UAAM;SACL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;SAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;eACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;eAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;SAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;SACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;SAEpB,IAAM,cAAc,GAAG,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC;;SAE7C,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;SAE5E,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;SAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;SACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;SACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;SAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;SAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;MACrB;KAED,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,IAAM,IAAI,GAAG,KAAK,CAAC,KAAK,CAAC,IAAI,CAAwB,CAAC;;KAEtD,IAAI,IAAI,GAAG,KAAK,CAAC,QAAQ,CAAC;;KAE1B,IAAI,KAAK,CAAC,QAAQ,KAAKG,aAAM,CAAC,kBAAkB;SAAE,IAAI,GAAG,IAAI,GAAG,CAAC,CAAC;;KAElE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;KAEtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,QAAQ,CAAC;;KAGjC,IAAI,KAAK,CAAC,QAAQ,KAAKA,aAAM,CAAC,kBAAkB,EAAE;SAChD,IAAI,GAAG,IAAI,GAAG,CAAC,CAAC;SAChB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;SAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;SACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;SACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;MACvC;;KAGD,MAAM,CAAC,GAAG,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;;KAExB,KAAK,GAAG,KAAK,GAAG,KAAK,CAAC,QAAQ,CAAC;KAC/B,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;KAEzE,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;KAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;KAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;KACvB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,cAAc,CACrB,MAAc,EACd,GAAW,EACX,KAAY,EACZ,KAAa,EACb,KAAa,EACb,kBAA2B,EAC3B,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KAEpB,IAAI,UAAU,GAAG,KAAK,CAAC;KACvB,IAAI,MAAM,GAAc;SACtB,IAAI,EAAE,KAAK,CAAC,UAAU,IAAI,KAAK,CAAC,SAAS;SACzC,GAAG,EAAE,KAAK,CAAC,GAAG;MACf,CAAC;KAEF,IAAI,KAAK,CAAC,EAAE,IAAI,IAAI,EAAE;SACpB,MAAM,CAAC,GAAG,GAAG,KAAK,CAAC,EAAE,CAAC;MACvB;KAED,MAAM,GAAG,MAAM,CAAC,MAAM,CAAC,MAAM,EAAE,KAAK,CAAC,MAAM,CAAC,CAAC;KAC7C,IAAM,QAAQ,GAAG,aAAa,CAAC,MAAM,EAAE,MAAM,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,kBAAkB,CAAC,CAAC;;KAG5F,IAAM,IAAI,GAAG,QAAQ,GAAG,UAAU,CAAC;;KAEnC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KACnC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KAC1C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KAC3C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;KAE3C,OAAO,QAAQ,CAAC;CAClB,CAAC;CAED,SAAgB,aAAa,CAC3B,MAAc,EACd,MAAgB,EAChB,SAAiB,EACjB,aAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,IAAqB;KALrB,0BAAA,EAAA,iBAAiB;KACjB,8BAAA,EAAA,iBAAiB;KACjB,sBAAA,EAAA,SAAS;KACT,mCAAA,EAAA,0BAA0B;KAC1B,gCAAA,EAAA,sBAAsB;KACtB,qBAAA,EAAA,SAAqB;KAErB,aAAa,GAAG,aAAa,IAAI,CAAC,CAAC;KACnC,IAAI,GAAG,IAAI,IAAI,EAAE,CAAC;;KAGlB,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;;KAGlB,IAAI,KAAK,GAAG,aAAa,GAAG,CAAC,CAAC;;KAG9B,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;;SAEzB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;aACtC,IAAM,GAAG,GAAG,EAAE,GAAG,CAAC,CAAC;aACnB,IAAI,KAAK,GAAG,MAAM,CAAC,CAAC,CAAC,CAAC;;aAGtB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;iBACzB,IAAI,OAAO,KAAK,CAAC,MAAM,KAAK,UAAU;qBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;iBACxF,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;cACxB;aAED,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;iBAC7B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;iBACpC,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;iBACpC,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;cACvE;kBAAM,IAAI,OAAO,KAAK,KAAK,SAAS,EAAE;iBACrC,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC3D;kBAAM,IAAI,KAAK,YAAY,IAAI,IAAIH,YAAM,CAAC,KAAK,CAAC,EAAE;iBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,KAAK,SAAS,EAAE;iBAC9B,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,KAAK,IAAI,EAAE;iBACzB,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;iBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC5D;kBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;iBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;iBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;iBAClE,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,IAAI,EACJ,IAAI,CACL,CAAC;cACH;kBAAM,IACL,OAAO,KAAK,KAAK,QAAQ;iBACzBsG,wBAAU,CAAC,KAAK,CAAC;iBACjB,KAAK,CAAC,SAAS,KAAK,YAAY,EAChC;iBACA,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC9D;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;iBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC9E;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;iBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,IAAI,CACL,CAAC;cACH;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,EAAE,IAAI,CAAC,CAAC;cACpF;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC9D;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cACzD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;cACjF;UACF;MACF;UAAM,IAAI,MAAM,YAAYC,OAAG,IAAIvG,WAAK,CAAC,MAAM,CAAC,EAAE;SACjD,IAAM,QAAQ,GAAG,MAAM,CAAC,OAAO,EAAE,CAAC;SAClC,IAAI,IAAI,GAAG,KAAK,CAAC;SAEjB,OAAO,CAAC,IAAI,EAAE;;aAEZ,IAAM,KAAK,GAAG,QAAQ,CAAC,IAAI,EAAE,CAAC;aAC9B,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC,IAAI,CAAC;;aAEpB,IAAI,IAAI;iBAAE,SAAS;;aAGnB,IAAM,GAAG,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;aAC3B,IAAM,KAAK,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;;aAG7B,IAAM,IAAI,GAAG,OAAO,KAAK,CAAC;;aAG1B,IAAI,OAAO,GAAG,KAAK,QAAQ,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;iBACnD,IAAI,GAAG,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;qBAG7B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,8BAA8B,CAAC,CAAC;kBAC5D;iBAED,IAAI,SAAS,EAAE;qBACb,IAAI,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,EAAE;yBAClB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,0BAA0B,CAAC,CAAC;sBACxD;0BAAM,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;yBAC5B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,uBAAuB,CAAC,CAAC;sBACrD;kBACF;cACF;aAED,IAAI,IAAI,KAAK,QAAQ,EAAE;iBACrB,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;iBAC5B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,IAAIA,qBAAe,CAAC,KAAK,CAAC,IAAIA,sBAAgB,CAAC,KAAK,CAAC,EAAE;iBACjF,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;cACvE;kBAAM,IAAI,IAAI,KAAK,SAAS,EAAE;iBAC7B,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACrD;kBAAM,IAAI,KAAK,YAAY,IAAI,IAAIA,YAAM,CAAC,KAAK,CAAC,EAAE;iBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,KAAK,IAAI,KAAK,KAAK,KAAK,SAAS,IAAI,eAAe,KAAK,KAAK,CAAC,EAAE;iBAC/E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;iBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACtD;kBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;iBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;iBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;iBAC1D,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,KAAK,EACL,IAAI,CACL,CAAC;cACH;kBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBACnE,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;iBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,CAChB,CAAC;cACH;kBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;iBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;cAC5F;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;cAC9E;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACnD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;cACjF;UACF;MACF;UAAM;;SAEL,IAAI,MAAM,CAAC,MAAM,EAAE;aACjB,IAAI,OAAO,MAAM,CAAC,MAAM,KAAK,UAAU;iBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;aACzF,MAAM,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC;aACzB,IAAI,MAAM,IAAI,IAAI,IAAI,OAAO,MAAM,KAAK,QAAQ;iBAC9C,MAAM,IAAI,SAAS,CAAC,0CAA0C,CAAC,CAAC;UACnE;;SAGD,KAAK,IAAM,GAAG,IAAI,MAAM,EAAE;aACxB,IAAI,KAAK,GAAG,MAAM,CAAC,GAAG,CAAC,CAAC;;aAExB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;iBACzB,IAAI,OAAO,KAAK,CAAC,MAAM,KAAK,UAAU;qBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;iBACxF,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;cACxB;;aAGD,IAAM,IAAI,GAAG,OAAO,KAAK,CAAC;;aAG1B,IAAI,OAAO,GAAG,KAAK,QAAQ,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;iBACnD,IAAI,GAAG,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;qBAG7B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,8BAA8B,CAAC,CAAC;kBAC5D;iBAED,IAAI,SAAS,EAAE;qBACb,IAAI,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,EAAE;yBAClB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,0BAA0B,CAAC,CAAC;sBACxD;0BAAM,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;yBAC5B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,uBAAuB,CAAC,CAAC;sBACrD;kBACF;cACF;aAED,IAAI,IAAI,KAAK,QAAQ,EAAE;iBACrB,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;iBAC5B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;iBAC5B,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;cACvE;kBAAM,IAAI,IAAI,KAAK,SAAS,EAAE;iBAC7B,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACrD;kBAAM,IAAI,KAAK,YAAY,IAAI,IAAIA,YAAM,CAAC,KAAK,CAAC,EAAE;iBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,KAAK,SAAS,EAAE;iBAC9B,IAAI,eAAe,KAAK,KAAK;qBAAE,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACjF;kBAAM,IAAI,KAAK,KAAK,IAAI,EAAE;iBACzB,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;iBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACtD;kBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;iBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;iBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;iBAC1D,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,KAAK,EACL,IAAI,CACL,CAAC;cACH;kBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBACnE,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;iBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,CAChB,CAAC;cACH;kBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;iBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;cAC5F;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;cAC9E;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACnD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;cACjF;UACF;MACF;;KAGD,IAAI,CAAC,GAAG,EAAE,CAAC;;KAGX,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;KAGvB,IAAM,IAAI,GAAG,KAAK,GAAG,aAAa,CAAC;;KAEnC,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KACtC,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KAC7C,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KAC9C,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KAC9C,OAAO,KAAK,CAAC;CACf,CAAC;CAxUD,sCAwUC;;;;;;;;;;;AC7iC+B;AACE;CAiFhC,uFAjFOG,aAAM,OAiFP;AAhFsB;CA4E5B,qFA5EO6F,SAAI,OA4EP;AA3E2B;CA8E/B,sFA9EOC,YAAK,OA8EP;AA7EmC;CAwFxC,2FAxFON,qBAAU,OAwFP;AAvFsB;CAkFhC,uFAlFO,eAAM,OAkFP;AAjFuC;AACP;AACP;CAgF/B,sFAhFOD,YAAK,OAgFP;AA/EuB;CA4E5B,qFA5EO,WAAI,OA4EP;AA3EsB;CAqE1B,oFArEOa,OAAG,OAqEP;AApE8B;CA+EjC,uFA/EOV,cAAM,OA+EP;AA9E2B;CA6EjC,uFA7EOD,cAAM,OA6EP;AA5E8B;CAsEpC,yFAtEOJ,iBAAQ,OAsEP;CAaI,yFAnFLA,iBAAQ,OAmFK;AAlFuE;CAC7F;AAC+F;AACJ;AACrD;CAyEpC,2FAzEOM,iBAAU,OAyEP;AAxE0B;CA6DpC,2FA7DOL,iBAAU,OA6DP;AA5D4B;CAkEtC,0FAlEOM,mBAAS,OAkEP;AAjEmB;CA+D5B,qFA/DO3F,SAAI,OA+DP;AA3Be;CAhCnB,yHAAA,8BAA8B,OAAA;CAC9B,sHAAA,2BAA2B,OAAA;CAC3B,uHAAA,4BAA4B,OAAA;CAC5B,kHAAA,uBAAuB,OAAA;CACvB,2HAAA,gCAAgC,OAAA;CAChC,mHAAA,wBAAwB,OAAA;CACxB,uHAAA,4BAA4B,OAAA;CAC5B,0GAAA,eAAe,OAAA;CACf,2GAAA,gBAAgB,OAAA;CAChB,4GAAA,iBAAiB,OAAA;CACjB,yGAAA,cAAc,OAAA;CACd,iHAAA,sBAAsB,OAAA;CACtB,yGAAA,cAAc,OAAA;CACd,8GAAA,mBAAmB,OAAA;CACnB,+GAAA,oBAAoB,OAAA;CACpB,wGAAA,aAAa,OAAA;CACb,yGAAA,cAAc,OAAA;CACd,4GAAA,iBAAiB,OAAA;CACjB,4GAAA,iBAAiB,OAAA;CACjB,yGAAA,cAAc,OAAA;CACd,2GAAA,gBAAgB,OAAA;CAChB,2GAAA,gBAAgB,OAAA;CAChB,wGAAA,aAAa,OAAA;CACb,2GAAA,gBAAgB,OAAA;CAChB,2GAAA,gBAAgB,OAAA;CAChB,2GAAA,gBAAgB,OAAA;CAChB,8GAAA,mBAAmB,OAAA;CACnB,8GAAA,mBAAmB,OAAA;CACnB,yGAAA,cAAc,OAAA;CACd,yGAAA,cAAc,OAAA;CACd,yGAAA,cAAc,OAAA;CACd,yGAAA,cAAc,OAAA;CAKhB,oCAAsD;CAA7C,sGAAA,KAAK,OAAA;CAQd,4BAKqB;CAHnB,sHAAA,yBAAyB,OAAA;CAkC3B;CACA;CACA,IAAM,OAAO,GAAG,IAAI,GAAG,IAAI,GAAG,EAAE,CAAC;CAEjC;CACA,IAAI5X,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC;CAEnC;;;;;;CAMA,SAAgB,qBAAqB,CAAC,IAAY;;KAEhD,IAAIvX,QAAM,CAAC,MAAM,GAAG,IAAI,EAAE;SACxBA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;MAC7B;CACH,CAAC;CALD,sDAKC;CAED;;;;;;;CAOA,SAAgB,SAAS,CAAC,MAAgB,EAAE,OAA8B;KAA9B,wBAAA,EAAA,YAA8B;;KAExE,IAAM,SAAS,GAAG,OAAO,OAAO,CAAC,SAAS,KAAK,SAAS,GAAG,OAAO,CAAC,SAAS,GAAG,KAAK,CAAC;KACrF,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;KACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;KAChF,IAAM,qBAAqB,GACzB,OAAO,OAAO,CAAC,qBAAqB,KAAK,QAAQ,GAAG,OAAO,CAAC,qBAAqB,GAAG,OAAO,CAAC;;KAG9F,IAAIvX,QAAM,CAAC,MAAM,GAAG,qBAAqB,EAAE;SACzCA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,qBAAqB,CAAC,CAAC;MAC9C;;KAGD,IAAM,kBAAkB,GAAGyG,wBAAiB,CAC1Che,QAAM,EACN,MAAM,EACN,SAAS,EACT,CAAC,EACD,CAAC,EACD,kBAAkB,EAClB,eAAe,EACf,EAAE,CACH,CAAC;;KAGF,IAAM,cAAc,GAAGuX,aAAM,CAAC,KAAK,CAAC,kBAAkB,CAAC,CAAC;;KAGxDvX,QAAM,CAAC,IAAI,CAAC,cAAc,EAAE,CAAC,EAAE,CAAC,EAAE,cAAc,CAAC,MAAM,CAAC,CAAC;;KAGzD,OAAO,cAAc,CAAC;CACxB,CAAC;CAnCD,8BAmCC;CAED;;;;;;;;;CASA,SAAgB,2BAA2B,CACzC,MAAgB,EAChB,WAAmB,EACnB,OAA8B;KAA9B,wBAAA,EAAA,YAA8B;;KAG9B,IAAM,SAAS,GAAG,OAAO,OAAO,CAAC,SAAS,KAAK,SAAS,GAAG,OAAO,CAAC,SAAS,GAAG,KAAK,CAAC;KACrF,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;KACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;KAChF,IAAM,UAAU,GAAG,OAAO,OAAO,CAAC,KAAK,KAAK,QAAQ,GAAG,OAAO,CAAC,KAAK,GAAG,CAAC,CAAC;;KAGzE,IAAM,kBAAkB,GAAGge,wBAAiB,CAC1Che,QAAM,EACN,MAAM,EACN,SAAS,EACT,CAAC,EACD,CAAC,EACD,kBAAkB,EAClB,eAAe,CAChB,CAAC;KACFA,QAAM,CAAC,IAAI,CAAC,WAAW,EAAE,UAAU,EAAE,CAAC,EAAE,kBAAkB,CAAC,CAAC;;KAG5D,OAAO,UAAU,GAAG,kBAAkB,GAAG,CAAC,CAAC;CAC7C,CAAC;CA3BD,kEA2BC;CAED;;;;;;;CAOA,SAAgB,WAAW,CACzB,MAA8C,EAC9C,OAAgC;KAAhC,wBAAA,EAAA,YAAgC;KAEhC,OAAOie,wBAAmB,CAACxG,0BAAY,CAAC,MAAM,CAAC,EAAE,OAAO,CAAC,CAAC;CAC5D,CAAC;CALD,kCAKC;CAQD;;;;;;;CAOA,SAAgB,mBAAmB,CACjC,MAAgB,EAChB,OAAwC;KAAxC,wBAAA,EAAA,YAAwC;KAExC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;KAExB,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;KACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;KAEhF,OAAOyG,kCAA2B,CAAC,MAAM,EAAE,kBAAkB,EAAE,eAAe,CAAC,CAAC;CAClF,CAAC;CAZD,kDAYC;CAED;;;;;;;;;;;;CAYA,SAAgB,iBAAiB,CAC/B,IAA4C,EAC5C,UAAkB,EAClB,iBAAyB,EACzB,SAAqB,EACrB,aAAqB,EACrB,OAA2B;KAE3B,IAAM,eAAe,GAAG,MAAM,CAAC,MAAM,CACnC,EAAE,gCAAgC,EAAE,IAAI,EAAE,KAAK,EAAE,CAAC,EAAE,EACpD,OAAO,CACR,CAAC;KACF,IAAM,UAAU,GAAGzG,0BAAY,CAAC,IAAI,CAAC,CAAC;KAEtC,IAAI,KAAK,GAAG,UAAU,CAAC;;KAEvB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,iBAAiB,EAAE,CAAC,EAAE,EAAE;;SAE1C,IAAM,IAAI,GACR,UAAU,CAAC,KAAK,CAAC;cAChB,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;cAC3B,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;cAC5B,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;;SAEhC,eAAe,CAAC,KAAK,GAAG,KAAK,CAAC;;SAE9B,SAAS,CAAC,aAAa,GAAG,CAAC,CAAC,GAAGwG,wBAAmB,CAAC,UAAU,EAAE,eAAe,CAAC,CAAC;;SAEhF,KAAK,GAAG,KAAK,GAAG,IAAI,CAAC;MACtB;;KAGD,OAAO,KAAK,CAAC;CACf,CAAC;CAjCD,8CAiCC;CAED;;;;;;;;CAQA,IAAM,IAAI,GAAG;KACX,MAAM,eAAA;KACN,IAAI,WAAA;KACJ,KAAK,cAAA;KACL,UAAU,uBAAA;KACV,MAAM,iBAAA;KACN,KAAK,cAAA;KACL,IAAI,aAAA;KACJ,IAAI,WAAA;KACJ,GAAG,SAAA;KACH,MAAM,gBAAA;KACN,MAAM,gBAAA;KACN,QAAQ,mBAAA;KACR,QAAQ,EAAEjB,iBAAQ;KAClB,UAAU,mBAAA;KACV,UAAU,mBAAA;KACV,SAAS,qBAAA;KACT,KAAK,qBAAA;KACL,qBAAqB,uBAAA;KACrB,SAAS,WAAA;KACT,2BAA2B,6BAAA;KAC3B,WAAW,aAAA;KACX,mBAAmB,qBAAA;KACnB,iBAAiB,mBAAA;EAClB,CAAC;CACF,kBAAe,IAAI,CAAC;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;"}
\ No newline at end of file
diff --git a/node_modules/bson/dist/bson.bundle.js b/node_modules/bson/dist/bson.bundle.js
new file mode 100644
index 0000000..314e682
--- /dev/null
+++ b/node_modules/bson/dist/bson.bundle.js
@@ -0,0 +1,9016 @@
+var BSON = (function (exports) {
+ 'use strict';
+
+ var commonjsGlobal = typeof globalThis !== 'undefined' ? globalThis : typeof window !== 'undefined' ? window : typeof global !== 'undefined' ? global : typeof self !== 'undefined' ? self : {};
+
+ function unwrapExports (x) {
+ return x && x.__esModule && Object.prototype.hasOwnProperty.call(x, 'default') ? x['default'] : x;
+ }
+
+ function createCommonjsModule(fn, module) {
+ return module = { exports: {} }, fn(module, module.exports), module.exports;
+ }
+
+ var byteLength_1 = byteLength;
+ var toByteArray_1 = toByteArray;
+ var fromByteArray_1 = fromByteArray;
+ var lookup = [];
+ var revLookup = [];
+ var Arr = typeof Uint8Array !== 'undefined' ? Uint8Array : Array;
+ var code$1 = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/';
+
+ for (var i = 0, len = code$1.length; i < len; ++i) {
+ lookup[i] = code$1[i];
+ revLookup[code$1.charCodeAt(i)] = i;
+ } // Support decoding URL-safe base64 strings, as Node.js does.
+ // See: https://en.wikipedia.org/wiki/Base64#URL_applications
+
+
+ revLookup['-'.charCodeAt(0)] = 62;
+ revLookup['_'.charCodeAt(0)] = 63;
+
+ function getLens(b64) {
+ var len = b64.length;
+
+ if (len % 4 > 0) {
+ throw new Error('Invalid string. Length must be a multiple of 4');
+ } // Trim off extra bytes after placeholder bytes are found
+ // See: https://github.com/beatgammit/base64-js/issues/42
+
+
+ var validLen = b64.indexOf('=');
+ if (validLen === -1) validLen = len;
+ var placeHoldersLen = validLen === len ? 0 : 4 - validLen % 4;
+ return [validLen, placeHoldersLen];
+ } // base64 is 4/3 + up to two characters of the original data
+
+
+ function byteLength(b64) {
+ var lens = getLens(b64);
+ var validLen = lens[0];
+ var placeHoldersLen = lens[1];
+ return (validLen + placeHoldersLen) * 3 / 4 - placeHoldersLen;
+ }
+
+ function _byteLength(b64, validLen, placeHoldersLen) {
+ return (validLen + placeHoldersLen) * 3 / 4 - placeHoldersLen;
+ }
+
+ function toByteArray(b64) {
+ var tmp;
+ var lens = getLens(b64);
+ var validLen = lens[0];
+ var placeHoldersLen = lens[1];
+ var arr = new Arr(_byteLength(b64, validLen, placeHoldersLen));
+ var curByte = 0; // if there are placeholders, only get up to the last complete 4 chars
+
+ var len = placeHoldersLen > 0 ? validLen - 4 : validLen;
+ var i;
+
+ for (i = 0; i < len; i += 4) {
+ tmp = revLookup[b64.charCodeAt(i)] << 18 | revLookup[b64.charCodeAt(i + 1)] << 12 | revLookup[b64.charCodeAt(i + 2)] << 6 | revLookup[b64.charCodeAt(i + 3)];
+ arr[curByte++] = tmp >> 16 & 0xFF;
+ arr[curByte++] = tmp >> 8 & 0xFF;
+ arr[curByte++] = tmp & 0xFF;
+ }
+
+ if (placeHoldersLen === 2) {
+ tmp = revLookup[b64.charCodeAt(i)] << 2 | revLookup[b64.charCodeAt(i + 1)] >> 4;
+ arr[curByte++] = tmp & 0xFF;
+ }
+
+ if (placeHoldersLen === 1) {
+ tmp = revLookup[b64.charCodeAt(i)] << 10 | revLookup[b64.charCodeAt(i + 1)] << 4 | revLookup[b64.charCodeAt(i + 2)] >> 2;
+ arr[curByte++] = tmp >> 8 & 0xFF;
+ arr[curByte++] = tmp & 0xFF;
+ }
+
+ return arr;
+ }
+
+ function tripletToBase64(num) {
+ return lookup[num >> 18 & 0x3F] + lookup[num >> 12 & 0x3F] + lookup[num >> 6 & 0x3F] + lookup[num & 0x3F];
+ }
+
+ function encodeChunk(uint8, start, end) {
+ var tmp;
+ var output = [];
+
+ for (var i = start; i < end; i += 3) {
+ tmp = (uint8[i] << 16 & 0xFF0000) + (uint8[i + 1] << 8 & 0xFF00) + (uint8[i + 2] & 0xFF);
+ output.push(tripletToBase64(tmp));
+ }
+
+ return output.join('');
+ }
+
+ function fromByteArray(uint8) {
+ var tmp;
+ var len = uint8.length;
+ var extraBytes = len % 3; // if we have 1 byte left, pad 2 bytes
+
+ var parts = [];
+ var maxChunkLength = 16383; // must be multiple of 3
+ // go through the array every three bytes, we'll deal with trailing stuff later
+
+ for (var i = 0, len2 = len - extraBytes; i < len2; i += maxChunkLength) {
+ parts.push(encodeChunk(uint8, i, i + maxChunkLength > len2 ? len2 : i + maxChunkLength));
+ } // pad the end with zeros, but make sure to not forget the extra bytes
+
+
+ if (extraBytes === 1) {
+ tmp = uint8[len - 1];
+ parts.push(lookup[tmp >> 2] + lookup[tmp << 4 & 0x3F] + '==');
+ } else if (extraBytes === 2) {
+ tmp = (uint8[len - 2] << 8) + uint8[len - 1];
+ parts.push(lookup[tmp >> 10] + lookup[tmp >> 4 & 0x3F] + lookup[tmp << 2 & 0x3F] + '=');
+ }
+
+ return parts.join('');
+ }
+
+ var base64Js = {
+ byteLength: byteLength_1,
+ toByteArray: toByteArray_1,
+ fromByteArray: fromByteArray_1
+ };
+
+ /*! ieee754. BSD-3-Clause License. Feross Aboukhadijeh */
+ var read = function read(buffer, offset, isLE, mLen, nBytes) {
+ var e, m;
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var nBits = -7;
+ var i = isLE ? nBytes - 1 : 0;
+ var d = isLE ? -1 : 1;
+ var s = buffer[offset + i];
+ i += d;
+ e = s & (1 << -nBits) - 1;
+ s >>= -nBits;
+ nBits += eLen;
+
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8) {}
+
+ m = e & (1 << -nBits) - 1;
+ e >>= -nBits;
+ nBits += mLen;
+
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8) {}
+
+ if (e === 0) {
+ e = 1 - eBias;
+ } else if (e === eMax) {
+ return m ? NaN : (s ? -1 : 1) * Infinity;
+ } else {
+ m = m + Math.pow(2, mLen);
+ e = e - eBias;
+ }
+
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
+ };
+
+ var write = function write(buffer, value, offset, isLE, mLen, nBytes) {
+ var e, m, c;
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0;
+ var i = isLE ? 0 : nBytes - 1;
+ var d = isLE ? 1 : -1;
+ var s = value < 0 || value === 0 && 1 / value < 0 ? 1 : 0;
+ value = Math.abs(value);
+
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0;
+ e = eMax;
+ } else {
+ e = Math.floor(Math.log(value) / Math.LN2);
+
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--;
+ c *= 2;
+ }
+
+ if (e + eBias >= 1) {
+ value += rt / c;
+ } else {
+ value += rt * Math.pow(2, 1 - eBias);
+ }
+
+ if (value * c >= 2) {
+ e++;
+ c /= 2;
+ }
+
+ if (e + eBias >= eMax) {
+ m = 0;
+ e = eMax;
+ } else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen);
+ e = e + eBias;
+ } else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
+ e = 0;
+ }
+ }
+
+ for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}
+
+ e = e << mLen | m;
+ eLen += mLen;
+
+ for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}
+
+ buffer[offset + i - d] |= s * 128;
+ };
+
+ var ieee754 = {
+ read: read,
+ write: write
+ };
+
+ var buffer = createCommonjsModule(function (module, exports) {
+
+ var customInspectSymbol = typeof Symbol === 'function' && typeof Symbol['for'] === 'function' ? // eslint-disable-line dot-notation
+ Symbol['for']('nodejs.util.inspect.custom') // eslint-disable-line dot-notation
+ : null;
+ exports.Buffer = Buffer;
+ exports.SlowBuffer = SlowBuffer;
+ exports.INSPECT_MAX_BYTES = 50;
+ var K_MAX_LENGTH = 0x7fffffff;
+ exports.kMaxLength = K_MAX_LENGTH;
+ /**
+ * If `Buffer.TYPED_ARRAY_SUPPORT`:
+ * === true Use Uint8Array implementation (fastest)
+ * === false Print warning and recommend using `buffer` v4.x which has an Object
+ * implementation (most compatible, even IE6)
+ *
+ * Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,
+ * Opera 11.6+, iOS 4.2+.
+ *
+ * We report that the browser does not support typed arrays if the are not subclassable
+ * using __proto__. Firefox 4-29 lacks support for adding new properties to `Uint8Array`
+ * (See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438). IE 10 lacks support
+ * for __proto__ and has a buggy typed array implementation.
+ */
+
+ Buffer.TYPED_ARRAY_SUPPORT = typedArraySupport();
+
+ if (!Buffer.TYPED_ARRAY_SUPPORT && typeof console !== 'undefined' && typeof console.error === 'function') {
+ console.error('This browser lacks typed array (Uint8Array) support which is required by ' + '`buffer` v5.x. Use `buffer` v4.x if you require old browser support.');
+ }
+
+ function typedArraySupport() {
+ // Can typed array instances can be augmented?
+ try {
+ var arr = new Uint8Array(1);
+ var proto = {
+ foo: function foo() {
+ return 42;
+ }
+ };
+ Object.setPrototypeOf(proto, Uint8Array.prototype);
+ Object.setPrototypeOf(arr, proto);
+ return arr.foo() === 42;
+ } catch (e) {
+ return false;
+ }
+ }
+
+ Object.defineProperty(Buffer.prototype, 'parent', {
+ enumerable: true,
+ get: function get() {
+ if (!Buffer.isBuffer(this)) return undefined;
+ return this.buffer;
+ }
+ });
+ Object.defineProperty(Buffer.prototype, 'offset', {
+ enumerable: true,
+ get: function get() {
+ if (!Buffer.isBuffer(this)) return undefined;
+ return this.byteOffset;
+ }
+ });
+
+ function createBuffer(length) {
+ if (length > K_MAX_LENGTH) {
+ throw new RangeError('The value "' + length + '" is invalid for option "size"');
+ } // Return an augmented `Uint8Array` instance
+
+
+ var buf = new Uint8Array(length);
+ Object.setPrototypeOf(buf, Buffer.prototype);
+ return buf;
+ }
+ /**
+ * The Buffer constructor returns instances of `Uint8Array` that have their
+ * prototype changed to `Buffer.prototype`. Furthermore, `Buffer` is a subclass of
+ * `Uint8Array`, so the returned instances will have all the node `Buffer` methods
+ * and the `Uint8Array` methods. Square bracket notation works as expected -- it
+ * returns a single octet.
+ *
+ * The `Uint8Array` prototype remains unmodified.
+ */
+
+
+ function Buffer(arg, encodingOrOffset, length) {
+ // Common case.
+ if (typeof arg === 'number') {
+ if (typeof encodingOrOffset === 'string') {
+ throw new TypeError('The "string" argument must be of type string. Received type number');
+ }
+
+ return allocUnsafe(arg);
+ }
+
+ return from(arg, encodingOrOffset, length);
+ }
+
+ Buffer.poolSize = 8192; // not used by this implementation
+
+ function from(value, encodingOrOffset, length) {
+ if (typeof value === 'string') {
+ return fromString(value, encodingOrOffset);
+ }
+
+ if (ArrayBuffer.isView(value)) {
+ return fromArrayView(value);
+ }
+
+ if (value == null) {
+ throw new TypeError('The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' + 'or Array-like Object. Received type ' + babelHelpers["typeof"](value));
+ }
+
+ if (isInstance(value, ArrayBuffer) || value && isInstance(value.buffer, ArrayBuffer)) {
+ return fromArrayBuffer(value, encodingOrOffset, length);
+ }
+
+ if (typeof SharedArrayBuffer !== 'undefined' && (isInstance(value, SharedArrayBuffer) || value && isInstance(value.buffer, SharedArrayBuffer))) {
+ return fromArrayBuffer(value, encodingOrOffset, length);
+ }
+
+ if (typeof value === 'number') {
+ throw new TypeError('The "value" argument must not be of type number. Received type number');
+ }
+
+ var valueOf = value.valueOf && value.valueOf();
+
+ if (valueOf != null && valueOf !== value) {
+ return Buffer.from(valueOf, encodingOrOffset, length);
+ }
+
+ var b = fromObject(value);
+ if (b) return b;
+
+ if (typeof Symbol !== 'undefined' && Symbol.toPrimitive != null && typeof value[Symbol.toPrimitive] === 'function') {
+ return Buffer.from(value[Symbol.toPrimitive]('string'), encodingOrOffset, length);
+ }
+
+ throw new TypeError('The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' + 'or Array-like Object. Received type ' + babelHelpers["typeof"](value));
+ }
+ /**
+ * Functionally equivalent to Buffer(arg, encoding) but throws a TypeError
+ * if value is a number.
+ * Buffer.from(str[, encoding])
+ * Buffer.from(array)
+ * Buffer.from(buffer)
+ * Buffer.from(arrayBuffer[, byteOffset[, length]])
+ **/
+
+
+ Buffer.from = function (value, encodingOrOffset, length) {
+ return from(value, encodingOrOffset, length);
+ }; // Note: Change prototype *after* Buffer.from is defined to workaround Chrome bug:
+ // https://github.com/feross/buffer/pull/148
+
+
+ Object.setPrototypeOf(Buffer.prototype, Uint8Array.prototype);
+ Object.setPrototypeOf(Buffer, Uint8Array);
+
+ function assertSize(size) {
+ if (typeof size !== 'number') {
+ throw new TypeError('"size" argument must be of type number');
+ } else if (size < 0) {
+ throw new RangeError('The value "' + size + '" is invalid for option "size"');
+ }
+ }
+
+ function alloc(size, fill, encoding) {
+ assertSize(size);
+
+ if (size <= 0) {
+ return createBuffer(size);
+ }
+
+ if (fill !== undefined) {
+ // Only pay attention to encoding if it's a string. This
+ // prevents accidentally sending in a number that would
+ // be interpreted as a start offset.
+ return typeof encoding === 'string' ? createBuffer(size).fill(fill, encoding) : createBuffer(size).fill(fill);
+ }
+
+ return createBuffer(size);
+ }
+ /**
+ * Creates a new filled Buffer instance.
+ * alloc(size[, fill[, encoding]])
+ **/
+
+
+ Buffer.alloc = function (size, fill, encoding) {
+ return alloc(size, fill, encoding);
+ };
+
+ function allocUnsafe(size) {
+ assertSize(size);
+ return createBuffer(size < 0 ? 0 : checked(size) | 0);
+ }
+ /**
+ * Equivalent to Buffer(num), by default creates a non-zero-filled Buffer instance.
+ * */
+
+
+ Buffer.allocUnsafe = function (size) {
+ return allocUnsafe(size);
+ };
+ /**
+ * Equivalent to SlowBuffer(num), by default creates a non-zero-filled Buffer instance.
+ */
+
+
+ Buffer.allocUnsafeSlow = function (size) {
+ return allocUnsafe(size);
+ };
+
+ function fromString(string, encoding) {
+ if (typeof encoding !== 'string' || encoding === '') {
+ encoding = 'utf8';
+ }
+
+ if (!Buffer.isEncoding(encoding)) {
+ throw new TypeError('Unknown encoding: ' + encoding);
+ }
+
+ var length = byteLength(string, encoding) | 0;
+ var buf = createBuffer(length);
+ var actual = buf.write(string, encoding);
+
+ if (actual !== length) {
+ // Writing a hex string, for example, that contains invalid characters will
+ // cause everything after the first invalid character to be ignored. (e.g.
+ // 'abxxcd' will be treated as 'ab')
+ buf = buf.slice(0, actual);
+ }
+
+ return buf;
+ }
+
+ function fromArrayLike(array) {
+ var length = array.length < 0 ? 0 : checked(array.length) | 0;
+ var buf = createBuffer(length);
+
+ for (var i = 0; i < length; i += 1) {
+ buf[i] = array[i] & 255;
+ }
+
+ return buf;
+ }
+
+ function fromArrayView(arrayView) {
+ if (isInstance(arrayView, Uint8Array)) {
+ var copy = new Uint8Array(arrayView);
+ return fromArrayBuffer(copy.buffer, copy.byteOffset, copy.byteLength);
+ }
+
+ return fromArrayLike(arrayView);
+ }
+
+ function fromArrayBuffer(array, byteOffset, length) {
+ if (byteOffset < 0 || array.byteLength < byteOffset) {
+ throw new RangeError('"offset" is outside of buffer bounds');
+ }
+
+ if (array.byteLength < byteOffset + (length || 0)) {
+ throw new RangeError('"length" is outside of buffer bounds');
+ }
+
+ var buf;
+
+ if (byteOffset === undefined && length === undefined) {
+ buf = new Uint8Array(array);
+ } else if (length === undefined) {
+ buf = new Uint8Array(array, byteOffset);
+ } else {
+ buf = new Uint8Array(array, byteOffset, length);
+ } // Return an augmented `Uint8Array` instance
+
+
+ Object.setPrototypeOf(buf, Buffer.prototype);
+ return buf;
+ }
+
+ function fromObject(obj) {
+ if (Buffer.isBuffer(obj)) {
+ var len = checked(obj.length) | 0;
+ var buf = createBuffer(len);
+
+ if (buf.length === 0) {
+ return buf;
+ }
+
+ obj.copy(buf, 0, 0, len);
+ return buf;
+ }
+
+ if (obj.length !== undefined) {
+ if (typeof obj.length !== 'number' || numberIsNaN(obj.length)) {
+ return createBuffer(0);
+ }
+
+ return fromArrayLike(obj);
+ }
+
+ if (obj.type === 'Buffer' && Array.isArray(obj.data)) {
+ return fromArrayLike(obj.data);
+ }
+ }
+
+ function checked(length) {
+ // Note: cannot use `length < K_MAX_LENGTH` here because that fails when
+ // length is NaN (which is otherwise coerced to zero.)
+ if (length >= K_MAX_LENGTH) {
+ throw new RangeError('Attempt to allocate Buffer larger than maximum ' + 'size: 0x' + K_MAX_LENGTH.toString(16) + ' bytes');
+ }
+
+ return length | 0;
+ }
+
+ function SlowBuffer(length) {
+ if (+length != length) {
+ // eslint-disable-line eqeqeq
+ length = 0;
+ }
+
+ return Buffer.alloc(+length);
+ }
+
+ Buffer.isBuffer = function isBuffer(b) {
+ return b != null && b._isBuffer === true && b !== Buffer.prototype; // so Buffer.isBuffer(Buffer.prototype) will be false
+ };
+
+ Buffer.compare = function compare(a, b) {
+ if (isInstance(a, Uint8Array)) a = Buffer.from(a, a.offset, a.byteLength);
+ if (isInstance(b, Uint8Array)) b = Buffer.from(b, b.offset, b.byteLength);
+
+ if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {
+ throw new TypeError('The "buf1", "buf2" arguments must be one of type Buffer or Uint8Array');
+ }
+
+ if (a === b) return 0;
+ var x = a.length;
+ var y = b.length;
+
+ for (var i = 0, len = Math.min(x, y); i < len; ++i) {
+ if (a[i] !== b[i]) {
+ x = a[i];
+ y = b[i];
+ break;
+ }
+ }
+
+ if (x < y) return -1;
+ if (y < x) return 1;
+ return 0;
+ };
+
+ Buffer.isEncoding = function isEncoding(encoding) {
+ switch (String(encoding).toLowerCase()) {
+ case 'hex':
+ case 'utf8':
+ case 'utf-8':
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ case 'base64':
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return true;
+
+ default:
+ return false;
+ }
+ };
+
+ Buffer.concat = function concat(list, length) {
+ if (!Array.isArray(list)) {
+ throw new TypeError('"list" argument must be an Array of Buffers');
+ }
+
+ if (list.length === 0) {
+ return Buffer.alloc(0);
+ }
+
+ var i;
+
+ if (length === undefined) {
+ length = 0;
+
+ for (i = 0; i < list.length; ++i) {
+ length += list[i].length;
+ }
+ }
+
+ var buffer = Buffer.allocUnsafe(length);
+ var pos = 0;
+
+ for (i = 0; i < list.length; ++i) {
+ var buf = list[i];
+
+ if (isInstance(buf, Uint8Array)) {
+ if (pos + buf.length > buffer.length) {
+ Buffer.from(buf).copy(buffer, pos);
+ } else {
+ Uint8Array.prototype.set.call(buffer, buf, pos);
+ }
+ } else if (!Buffer.isBuffer(buf)) {
+ throw new TypeError('"list" argument must be an Array of Buffers');
+ } else {
+ buf.copy(buffer, pos);
+ }
+
+ pos += buf.length;
+ }
+
+ return buffer;
+ };
+
+ function byteLength(string, encoding) {
+ if (Buffer.isBuffer(string)) {
+ return string.length;
+ }
+
+ if (ArrayBuffer.isView(string) || isInstance(string, ArrayBuffer)) {
+ return string.byteLength;
+ }
+
+ if (typeof string !== 'string') {
+ throw new TypeError('The "string" argument must be one of type string, Buffer, or ArrayBuffer. ' + 'Received type ' + babelHelpers["typeof"](string));
+ }
+
+ var len = string.length;
+ var mustMatch = arguments.length > 2 && arguments[2] === true;
+ if (!mustMatch && len === 0) return 0; // Use a for loop to avoid recursion
+
+ var loweredCase = false;
+
+ for (;;) {
+ switch (encoding) {
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ return len;
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8ToBytes(string).length;
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return len * 2;
+
+ case 'hex':
+ return len >>> 1;
+
+ case 'base64':
+ return base64ToBytes(string).length;
+
+ default:
+ if (loweredCase) {
+ return mustMatch ? -1 : utf8ToBytes(string).length; // assume utf8
+ }
+
+ encoding = ('' + encoding).toLowerCase();
+ loweredCase = true;
+ }
+ }
+ }
+
+ Buffer.byteLength = byteLength;
+
+ function slowToString(encoding, start, end) {
+ var loweredCase = false; // No need to verify that "this.length <= MAX_UINT32" since it's a read-only
+ // property of a typed array.
+ // This behaves neither like String nor Uint8Array in that we set start/end
+ // to their upper/lower bounds if the value passed is out of range.
+ // undefined is handled specially as per ECMA-262 6th Edition,
+ // Section 13.3.3.7 Runtime Semantics: KeyedBindingInitialization.
+
+ if (start === undefined || start < 0) {
+ start = 0;
+ } // Return early if start > this.length. Done here to prevent potential uint32
+ // coercion fail below.
+
+
+ if (start > this.length) {
+ return '';
+ }
+
+ if (end === undefined || end > this.length) {
+ end = this.length;
+ }
+
+ if (end <= 0) {
+ return '';
+ } // Force coercion to uint32. This will also coerce falsey/NaN values to 0.
+
+
+ end >>>= 0;
+ start >>>= 0;
+
+ if (end <= start) {
+ return '';
+ }
+
+ if (!encoding) encoding = 'utf8';
+
+ while (true) {
+ switch (encoding) {
+ case 'hex':
+ return hexSlice(this, start, end);
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Slice(this, start, end);
+
+ case 'ascii':
+ return asciiSlice(this, start, end);
+
+ case 'latin1':
+ case 'binary':
+ return latin1Slice(this, start, end);
+
+ case 'base64':
+ return base64Slice(this, start, end);
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return utf16leSlice(this, start, end);
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding);
+ encoding = (encoding + '').toLowerCase();
+ loweredCase = true;
+ }
+ }
+ } // This property is used by `Buffer.isBuffer` (and the `is-buffer` npm package)
+ // to detect a Buffer instance. It's not possible to use `instanceof Buffer`
+ // reliably in a browserify context because there could be multiple different
+ // copies of the 'buffer' package in use. This method works even for Buffer
+ // instances that were created from another copy of the `buffer` package.
+ // See: https://github.com/feross/buffer/issues/154
+
+
+ Buffer.prototype._isBuffer = true;
+
+ function swap(b, n, m) {
+ var i = b[n];
+ b[n] = b[m];
+ b[m] = i;
+ }
+
+ Buffer.prototype.swap16 = function swap16() {
+ var len = this.length;
+
+ if (len % 2 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 16-bits');
+ }
+
+ for (var i = 0; i < len; i += 2) {
+ swap(this, i, i + 1);
+ }
+
+ return this;
+ };
+
+ Buffer.prototype.swap32 = function swap32() {
+ var len = this.length;
+
+ if (len % 4 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 32-bits');
+ }
+
+ for (var i = 0; i < len; i += 4) {
+ swap(this, i, i + 3);
+ swap(this, i + 1, i + 2);
+ }
+
+ return this;
+ };
+
+ Buffer.prototype.swap64 = function swap64() {
+ var len = this.length;
+
+ if (len % 8 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 64-bits');
+ }
+
+ for (var i = 0; i < len; i += 8) {
+ swap(this, i, i + 7);
+ swap(this, i + 1, i + 6);
+ swap(this, i + 2, i + 5);
+ swap(this, i + 3, i + 4);
+ }
+
+ return this;
+ };
+
+ Buffer.prototype.toString = function toString() {
+ var length = this.length;
+ if (length === 0) return '';
+ if (arguments.length === 0) return utf8Slice(this, 0, length);
+ return slowToString.apply(this, arguments);
+ };
+
+ Buffer.prototype.toLocaleString = Buffer.prototype.toString;
+
+ Buffer.prototype.equals = function equals(b) {
+ if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer');
+ if (this === b) return true;
+ return Buffer.compare(this, b) === 0;
+ };
+
+ Buffer.prototype.inspect = function inspect() {
+ var str = '';
+ var max = exports.INSPECT_MAX_BYTES;
+ str = this.toString('hex', 0, max).replace(/(.{2})/g, '$1 ').trim();
+ if (this.length > max) str += ' ... ';
+ return '';
+ };
+
+ if (customInspectSymbol) {
+ Buffer.prototype[customInspectSymbol] = Buffer.prototype.inspect;
+ }
+
+ Buffer.prototype.compare = function compare(target, start, end, thisStart, thisEnd) {
+ if (isInstance(target, Uint8Array)) {
+ target = Buffer.from(target, target.offset, target.byteLength);
+ }
+
+ if (!Buffer.isBuffer(target)) {
+ throw new TypeError('The "target" argument must be one of type Buffer or Uint8Array. ' + 'Received type ' + babelHelpers["typeof"](target));
+ }
+
+ if (start === undefined) {
+ start = 0;
+ }
+
+ if (end === undefined) {
+ end = target ? target.length : 0;
+ }
+
+ if (thisStart === undefined) {
+ thisStart = 0;
+ }
+
+ if (thisEnd === undefined) {
+ thisEnd = this.length;
+ }
+
+ if (start < 0 || end > target.length || thisStart < 0 || thisEnd > this.length) {
+ throw new RangeError('out of range index');
+ }
+
+ if (thisStart >= thisEnd && start >= end) {
+ return 0;
+ }
+
+ if (thisStart >= thisEnd) {
+ return -1;
+ }
+
+ if (start >= end) {
+ return 1;
+ }
+
+ start >>>= 0;
+ end >>>= 0;
+ thisStart >>>= 0;
+ thisEnd >>>= 0;
+ if (this === target) return 0;
+ var x = thisEnd - thisStart;
+ var y = end - start;
+ var len = Math.min(x, y);
+ var thisCopy = this.slice(thisStart, thisEnd);
+ var targetCopy = target.slice(start, end);
+
+ for (var i = 0; i < len; ++i) {
+ if (thisCopy[i] !== targetCopy[i]) {
+ x = thisCopy[i];
+ y = targetCopy[i];
+ break;
+ }
+ }
+
+ if (x < y) return -1;
+ if (y < x) return 1;
+ return 0;
+ }; // Finds either the first index of `val` in `buffer` at offset >= `byteOffset`,
+ // OR the last index of `val` in `buffer` at offset <= `byteOffset`.
+ //
+ // Arguments:
+ // - buffer - a Buffer to search
+ // - val - a string, Buffer, or number
+ // - byteOffset - an index into `buffer`; will be clamped to an int32
+ // - encoding - an optional encoding, relevant is val is a string
+ // - dir - true for indexOf, false for lastIndexOf
+
+
+ function bidirectionalIndexOf(buffer, val, byteOffset, encoding, dir) {
+ // Empty buffer means no match
+ if (buffer.length === 0) return -1; // Normalize byteOffset
+
+ if (typeof byteOffset === 'string') {
+ encoding = byteOffset;
+ byteOffset = 0;
+ } else if (byteOffset > 0x7fffffff) {
+ byteOffset = 0x7fffffff;
+ } else if (byteOffset < -0x80000000) {
+ byteOffset = -0x80000000;
+ }
+
+ byteOffset = +byteOffset; // Coerce to Number.
+
+ if (numberIsNaN(byteOffset)) {
+ // byteOffset: it it's undefined, null, NaN, "foo", etc, search whole buffer
+ byteOffset = dir ? 0 : buffer.length - 1;
+ } // Normalize byteOffset: negative offsets start from the end of the buffer
+
+
+ if (byteOffset < 0) byteOffset = buffer.length + byteOffset;
+
+ if (byteOffset >= buffer.length) {
+ if (dir) return -1;else byteOffset = buffer.length - 1;
+ } else if (byteOffset < 0) {
+ if (dir) byteOffset = 0;else return -1;
+ } // Normalize val
+
+
+ if (typeof val === 'string') {
+ val = Buffer.from(val, encoding);
+ } // Finally, search either indexOf (if dir is true) or lastIndexOf
+
+
+ if (Buffer.isBuffer(val)) {
+ // Special case: looking for empty string/buffer always fails
+ if (val.length === 0) {
+ return -1;
+ }
+
+ return arrayIndexOf(buffer, val, byteOffset, encoding, dir);
+ } else if (typeof val === 'number') {
+ val = val & 0xFF; // Search for a byte value [0-255]
+
+ if (typeof Uint8Array.prototype.indexOf === 'function') {
+ if (dir) {
+ return Uint8Array.prototype.indexOf.call(buffer, val, byteOffset);
+ } else {
+ return Uint8Array.prototype.lastIndexOf.call(buffer, val, byteOffset);
+ }
+ }
+
+ return arrayIndexOf(buffer, [val], byteOffset, encoding, dir);
+ }
+
+ throw new TypeError('val must be string, number or Buffer');
+ }
+
+ function arrayIndexOf(arr, val, byteOffset, encoding, dir) {
+ var indexSize = 1;
+ var arrLength = arr.length;
+ var valLength = val.length;
+
+ if (encoding !== undefined) {
+ encoding = String(encoding).toLowerCase();
+
+ if (encoding === 'ucs2' || encoding === 'ucs-2' || encoding === 'utf16le' || encoding === 'utf-16le') {
+ if (arr.length < 2 || val.length < 2) {
+ return -1;
+ }
+
+ indexSize = 2;
+ arrLength /= 2;
+ valLength /= 2;
+ byteOffset /= 2;
+ }
+ }
+
+ function read(buf, i) {
+ if (indexSize === 1) {
+ return buf[i];
+ } else {
+ return buf.readUInt16BE(i * indexSize);
+ }
+ }
+
+ var i;
+
+ if (dir) {
+ var foundIndex = -1;
+
+ for (i = byteOffset; i < arrLength; i++) {
+ if (read(arr, i) === read(val, foundIndex === -1 ? 0 : i - foundIndex)) {
+ if (foundIndex === -1) foundIndex = i;
+ if (i - foundIndex + 1 === valLength) return foundIndex * indexSize;
+ } else {
+ if (foundIndex !== -1) i -= i - foundIndex;
+ foundIndex = -1;
+ }
+ }
+ } else {
+ if (byteOffset + valLength > arrLength) byteOffset = arrLength - valLength;
+
+ for (i = byteOffset; i >= 0; i--) {
+ var found = true;
+
+ for (var j = 0; j < valLength; j++) {
+ if (read(arr, i + j) !== read(val, j)) {
+ found = false;
+ break;
+ }
+ }
+
+ if (found) return i;
+ }
+ }
+
+ return -1;
+ }
+
+ Buffer.prototype.includes = function includes(val, byteOffset, encoding) {
+ return this.indexOf(val, byteOffset, encoding) !== -1;
+ };
+
+ Buffer.prototype.indexOf = function indexOf(val, byteOffset, encoding) {
+ return bidirectionalIndexOf(this, val, byteOffset, encoding, true);
+ };
+
+ Buffer.prototype.lastIndexOf = function lastIndexOf(val, byteOffset, encoding) {
+ return bidirectionalIndexOf(this, val, byteOffset, encoding, false);
+ };
+
+ function hexWrite(buf, string, offset, length) {
+ offset = Number(offset) || 0;
+ var remaining = buf.length - offset;
+
+ if (!length) {
+ length = remaining;
+ } else {
+ length = Number(length);
+
+ if (length > remaining) {
+ length = remaining;
+ }
+ }
+
+ var strLen = string.length;
+
+ if (length > strLen / 2) {
+ length = strLen / 2;
+ }
+
+ for (var i = 0; i < length; ++i) {
+ var parsed = parseInt(string.substr(i * 2, 2), 16);
+ if (numberIsNaN(parsed)) return i;
+ buf[offset + i] = parsed;
+ }
+
+ return i;
+ }
+
+ function utf8Write(buf, string, offset, length) {
+ return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length);
+ }
+
+ function asciiWrite(buf, string, offset, length) {
+ return blitBuffer(asciiToBytes(string), buf, offset, length);
+ }
+
+ function base64Write(buf, string, offset, length) {
+ return blitBuffer(base64ToBytes(string), buf, offset, length);
+ }
+
+ function ucs2Write(buf, string, offset, length) {
+ return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length);
+ }
+
+ Buffer.prototype.write = function write(string, offset, length, encoding) {
+ // Buffer#write(string)
+ if (offset === undefined) {
+ encoding = 'utf8';
+ length = this.length;
+ offset = 0; // Buffer#write(string, encoding)
+ } else if (length === undefined && typeof offset === 'string') {
+ encoding = offset;
+ length = this.length;
+ offset = 0; // Buffer#write(string, offset[, length][, encoding])
+ } else if (isFinite(offset)) {
+ offset = offset >>> 0;
+
+ if (isFinite(length)) {
+ length = length >>> 0;
+ if (encoding === undefined) encoding = 'utf8';
+ } else {
+ encoding = length;
+ length = undefined;
+ }
+ } else {
+ throw new Error('Buffer.write(string, encoding, offset[, length]) is no longer supported');
+ }
+
+ var remaining = this.length - offset;
+ if (length === undefined || length > remaining) length = remaining;
+
+ if (string.length > 0 && (length < 0 || offset < 0) || offset > this.length) {
+ throw new RangeError('Attempt to write outside buffer bounds');
+ }
+
+ if (!encoding) encoding = 'utf8';
+ var loweredCase = false;
+
+ for (;;) {
+ switch (encoding) {
+ case 'hex':
+ return hexWrite(this, string, offset, length);
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Write(this, string, offset, length);
+
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ return asciiWrite(this, string, offset, length);
+
+ case 'base64':
+ // Warning: maxLength not taken into account in base64Write
+ return base64Write(this, string, offset, length);
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return ucs2Write(this, string, offset, length);
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding);
+ encoding = ('' + encoding).toLowerCase();
+ loweredCase = true;
+ }
+ }
+ };
+
+ Buffer.prototype.toJSON = function toJSON() {
+ return {
+ type: 'Buffer',
+ data: Array.prototype.slice.call(this._arr || this, 0)
+ };
+ };
+
+ function base64Slice(buf, start, end) {
+ if (start === 0 && end === buf.length) {
+ return base64Js.fromByteArray(buf);
+ } else {
+ return base64Js.fromByteArray(buf.slice(start, end));
+ }
+ }
+
+ function utf8Slice(buf, start, end) {
+ end = Math.min(buf.length, end);
+ var res = [];
+ var i = start;
+
+ while (i < end) {
+ var firstByte = buf[i];
+ var codePoint = null;
+ var bytesPerSequence = firstByte > 0xEF ? 4 : firstByte > 0xDF ? 3 : firstByte > 0xBF ? 2 : 1;
+
+ if (i + bytesPerSequence <= end) {
+ var secondByte, thirdByte, fourthByte, tempCodePoint;
+
+ switch (bytesPerSequence) {
+ case 1:
+ if (firstByte < 0x80) {
+ codePoint = firstByte;
+ }
+
+ break;
+
+ case 2:
+ secondByte = buf[i + 1];
+
+ if ((secondByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0x1F) << 0x6 | secondByte & 0x3F;
+
+ if (tempCodePoint > 0x7F) {
+ codePoint = tempCodePoint;
+ }
+ }
+
+ break;
+
+ case 3:
+ secondByte = buf[i + 1];
+ thirdByte = buf[i + 2];
+
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | thirdByte & 0x3F;
+
+ if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {
+ codePoint = tempCodePoint;
+ }
+ }
+
+ break;
+
+ case 4:
+ secondByte = buf[i + 1];
+ thirdByte = buf[i + 2];
+ fourthByte = buf[i + 3];
+
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | fourthByte & 0x3F;
+
+ if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {
+ codePoint = tempCodePoint;
+ }
+ }
+
+ }
+ }
+
+ if (codePoint === null) {
+ // we did not generate a valid codePoint so insert a
+ // replacement char (U+FFFD) and advance only 1 byte
+ codePoint = 0xFFFD;
+ bytesPerSequence = 1;
+ } else if (codePoint > 0xFFFF) {
+ // encode to utf16 (surrogate pair dance)
+ codePoint -= 0x10000;
+ res.push(codePoint >>> 10 & 0x3FF | 0xD800);
+ codePoint = 0xDC00 | codePoint & 0x3FF;
+ }
+
+ res.push(codePoint);
+ i += bytesPerSequence;
+ }
+
+ return decodeCodePointsArray(res);
+ } // Based on http://stackoverflow.com/a/22747272/680742, the browser with
+ // the lowest limit is Chrome, with 0x10000 args.
+ // We go 1 magnitude less, for safety
+
+
+ var MAX_ARGUMENTS_LENGTH = 0x1000;
+
+ function decodeCodePointsArray(codePoints) {
+ var len = codePoints.length;
+
+ if (len <= MAX_ARGUMENTS_LENGTH) {
+ return String.fromCharCode.apply(String, codePoints); // avoid extra slice()
+ } // Decode in chunks to avoid "call stack size exceeded".
+
+
+ var res = '';
+ var i = 0;
+
+ while (i < len) {
+ res += String.fromCharCode.apply(String, codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH));
+ }
+
+ return res;
+ }
+
+ function asciiSlice(buf, start, end) {
+ var ret = '';
+ end = Math.min(buf.length, end);
+
+ for (var i = start; i < end; ++i) {
+ ret += String.fromCharCode(buf[i] & 0x7F);
+ }
+
+ return ret;
+ }
+
+ function latin1Slice(buf, start, end) {
+ var ret = '';
+ end = Math.min(buf.length, end);
+
+ for (var i = start; i < end; ++i) {
+ ret += String.fromCharCode(buf[i]);
+ }
+
+ return ret;
+ }
+
+ function hexSlice(buf, start, end) {
+ var len = buf.length;
+ if (!start || start < 0) start = 0;
+ if (!end || end < 0 || end > len) end = len;
+ var out = '';
+
+ for (var i = start; i < end; ++i) {
+ out += hexSliceLookupTable[buf[i]];
+ }
+
+ return out;
+ }
+
+ function utf16leSlice(buf, start, end) {
+ var bytes = buf.slice(start, end);
+ var res = ''; // If bytes.length is odd, the last 8 bits must be ignored (same as node.js)
+
+ for (var i = 0; i < bytes.length - 1; i += 2) {
+ res += String.fromCharCode(bytes[i] + bytes[i + 1] * 256);
+ }
+
+ return res;
+ }
+
+ Buffer.prototype.slice = function slice(start, end) {
+ var len = this.length;
+ start = ~~start;
+ end = end === undefined ? len : ~~end;
+
+ if (start < 0) {
+ start += len;
+ if (start < 0) start = 0;
+ } else if (start > len) {
+ start = len;
+ }
+
+ if (end < 0) {
+ end += len;
+ if (end < 0) end = 0;
+ } else if (end > len) {
+ end = len;
+ }
+
+ if (end < start) end = start;
+ var newBuf = this.subarray(start, end); // Return an augmented `Uint8Array` instance
+
+ Object.setPrototypeOf(newBuf, Buffer.prototype);
+ return newBuf;
+ };
+ /*
+ * Need to make sure that buffer isn't trying to write out of bounds.
+ */
+
+
+ function checkOffset(offset, ext, length) {
+ if (offset % 1 !== 0 || offset < 0) throw new RangeError('offset is not uint');
+ if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length');
+ }
+
+ Buffer.prototype.readUintLE = Buffer.prototype.readUIntLE = function readUIntLE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+ if (!noAssert) checkOffset(offset, byteLength, this.length);
+ var val = this[offset];
+ var mul = 1;
+ var i = 0;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul;
+ }
+
+ return val;
+ };
+
+ Buffer.prototype.readUintBE = Buffer.prototype.readUIntBE = function readUIntBE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+
+ if (!noAssert) {
+ checkOffset(offset, byteLength, this.length);
+ }
+
+ var val = this[offset + --byteLength];
+ var mul = 1;
+
+ while (byteLength > 0 && (mul *= 0x100)) {
+ val += this[offset + --byteLength] * mul;
+ }
+
+ return val;
+ };
+
+ Buffer.prototype.readUint8 = Buffer.prototype.readUInt8 = function readUInt8(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 1, this.length);
+ return this[offset];
+ };
+
+ Buffer.prototype.readUint16LE = Buffer.prototype.readUInt16LE = function readUInt16LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ return this[offset] | this[offset + 1] << 8;
+ };
+
+ Buffer.prototype.readUint16BE = Buffer.prototype.readUInt16BE = function readUInt16BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ return this[offset] << 8 | this[offset + 1];
+ };
+
+ Buffer.prototype.readUint32LE = Buffer.prototype.readUInt32LE = function readUInt32LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return (this[offset] | this[offset + 1] << 8 | this[offset + 2] << 16) + this[offset + 3] * 0x1000000;
+ };
+
+ Buffer.prototype.readUint32BE = Buffer.prototype.readUInt32BE = function readUInt32BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return this[offset] * 0x1000000 + (this[offset + 1] << 16 | this[offset + 2] << 8 | this[offset + 3]);
+ };
+
+ Buffer.prototype.readIntLE = function readIntLE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+ if (!noAssert) checkOffset(offset, byteLength, this.length);
+ var val = this[offset];
+ var mul = 1;
+ var i = 0;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul;
+ }
+
+ mul *= 0x80;
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength);
+ return val;
+ };
+
+ Buffer.prototype.readIntBE = function readIntBE(offset, byteLength, noAssert) {
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+ if (!noAssert) checkOffset(offset, byteLength, this.length);
+ var i = byteLength;
+ var mul = 1;
+ var val = this[offset + --i];
+
+ while (i > 0 && (mul *= 0x100)) {
+ val += this[offset + --i] * mul;
+ }
+
+ mul *= 0x80;
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength);
+ return val;
+ };
+
+ Buffer.prototype.readInt8 = function readInt8(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 1, this.length);
+ if (!(this[offset] & 0x80)) return this[offset];
+ return (0xff - this[offset] + 1) * -1;
+ };
+
+ Buffer.prototype.readInt16LE = function readInt16LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ var val = this[offset] | this[offset + 1] << 8;
+ return val & 0x8000 ? val | 0xFFFF0000 : val;
+ };
+
+ Buffer.prototype.readInt16BE = function readInt16BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 2, this.length);
+ var val = this[offset + 1] | this[offset] << 8;
+ return val & 0x8000 ? val | 0xFFFF0000 : val;
+ };
+
+ Buffer.prototype.readInt32LE = function readInt32LE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return this[offset] | this[offset + 1] << 8 | this[offset + 2] << 16 | this[offset + 3] << 24;
+ };
+
+ Buffer.prototype.readInt32BE = function readInt32BE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return this[offset] << 24 | this[offset + 1] << 16 | this[offset + 2] << 8 | this[offset + 3];
+ };
+
+ Buffer.prototype.readFloatLE = function readFloatLE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return ieee754.read(this, offset, true, 23, 4);
+ };
+
+ Buffer.prototype.readFloatBE = function readFloatBE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 4, this.length);
+ return ieee754.read(this, offset, false, 23, 4);
+ };
+
+ Buffer.prototype.readDoubleLE = function readDoubleLE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 8, this.length);
+ return ieee754.read(this, offset, true, 52, 8);
+ };
+
+ Buffer.prototype.readDoubleBE = function readDoubleBE(offset, noAssert) {
+ offset = offset >>> 0;
+ if (!noAssert) checkOffset(offset, 8, this.length);
+ return ieee754.read(this, offset, false, 52, 8);
+ };
+
+ function checkInt(buf, value, offset, ext, max, min) {
+ if (!Buffer.isBuffer(buf)) throw new TypeError('"buffer" argument must be a Buffer instance');
+ if (value > max || value < min) throw new RangeError('"value" argument is out of bounds');
+ if (offset + ext > buf.length) throw new RangeError('Index out of range');
+ }
+
+ Buffer.prototype.writeUintLE = Buffer.prototype.writeUIntLE = function writeUIntLE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+
+ if (!noAssert) {
+ var maxBytes = Math.pow(2, 8 * byteLength) - 1;
+ checkInt(this, value, offset, byteLength, maxBytes, 0);
+ }
+
+ var mul = 1;
+ var i = 0;
+ this[offset] = value & 0xFF;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ this[offset + i] = value / mul & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeUintBE = Buffer.prototype.writeUIntBE = function writeUIntBE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ byteLength = byteLength >>> 0;
+
+ if (!noAssert) {
+ var maxBytes = Math.pow(2, 8 * byteLength) - 1;
+ checkInt(this, value, offset, byteLength, maxBytes, 0);
+ }
+
+ var i = byteLength - 1;
+ var mul = 1;
+ this[offset + i] = value & 0xFF;
+
+ while (--i >= 0 && (mul *= 0x100)) {
+ this[offset + i] = value / mul & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeUint8 = Buffer.prototype.writeUInt8 = function writeUInt8(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0);
+ this[offset] = value & 0xff;
+ return offset + 1;
+ };
+
+ Buffer.prototype.writeUint16LE = Buffer.prototype.writeUInt16LE = function writeUInt16LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0);
+ this[offset] = value & 0xff;
+ this[offset + 1] = value >>> 8;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeUint16BE = Buffer.prototype.writeUInt16BE = function writeUInt16BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0);
+ this[offset] = value >>> 8;
+ this[offset + 1] = value & 0xff;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeUint32LE = Buffer.prototype.writeUInt32LE = function writeUInt32LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0);
+ this[offset + 3] = value >>> 24;
+ this[offset + 2] = value >>> 16;
+ this[offset + 1] = value >>> 8;
+ this[offset] = value & 0xff;
+ return offset + 4;
+ };
+
+ Buffer.prototype.writeUint32BE = Buffer.prototype.writeUInt32BE = function writeUInt32BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0);
+ this[offset] = value >>> 24;
+ this[offset + 1] = value >>> 16;
+ this[offset + 2] = value >>> 8;
+ this[offset + 3] = value & 0xff;
+ return offset + 4;
+ };
+
+ Buffer.prototype.writeIntLE = function writeIntLE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ var limit = Math.pow(2, 8 * byteLength - 1);
+ checkInt(this, value, offset, byteLength, limit - 1, -limit);
+ }
+
+ var i = 0;
+ var mul = 1;
+ var sub = 0;
+ this[offset] = value & 0xFF;
+
+ while (++i < byteLength && (mul *= 0x100)) {
+ if (value < 0 && sub === 0 && this[offset + i - 1] !== 0) {
+ sub = 1;
+ }
+
+ this[offset + i] = (value / mul >> 0) - sub & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeIntBE = function writeIntBE(value, offset, byteLength, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ var limit = Math.pow(2, 8 * byteLength - 1);
+ checkInt(this, value, offset, byteLength, limit - 1, -limit);
+ }
+
+ var i = byteLength - 1;
+ var mul = 1;
+ var sub = 0;
+ this[offset + i] = value & 0xFF;
+
+ while (--i >= 0 && (mul *= 0x100)) {
+ if (value < 0 && sub === 0 && this[offset + i + 1] !== 0) {
+ sub = 1;
+ }
+
+ this[offset + i] = (value / mul >> 0) - sub & 0xFF;
+ }
+
+ return offset + byteLength;
+ };
+
+ Buffer.prototype.writeInt8 = function writeInt8(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80);
+ if (value < 0) value = 0xff + value + 1;
+ this[offset] = value & 0xff;
+ return offset + 1;
+ };
+
+ Buffer.prototype.writeInt16LE = function writeInt16LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000);
+ this[offset] = value & 0xff;
+ this[offset + 1] = value >>> 8;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeInt16BE = function writeInt16BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000);
+ this[offset] = value >>> 8;
+ this[offset + 1] = value & 0xff;
+ return offset + 2;
+ };
+
+ Buffer.prototype.writeInt32LE = function writeInt32LE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000);
+ this[offset] = value & 0xff;
+ this[offset + 1] = value >>> 8;
+ this[offset + 2] = value >>> 16;
+ this[offset + 3] = value >>> 24;
+ return offset + 4;
+ };
+
+ Buffer.prototype.writeInt32BE = function writeInt32BE(value, offset, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000);
+ if (value < 0) value = 0xffffffff + value + 1;
+ this[offset] = value >>> 24;
+ this[offset + 1] = value >>> 16;
+ this[offset + 2] = value >>> 8;
+ this[offset + 3] = value & 0xff;
+ return offset + 4;
+ };
+
+ function checkIEEE754(buf, value, offset, ext, max, min) {
+ if (offset + ext > buf.length) throw new RangeError('Index out of range');
+ if (offset < 0) throw new RangeError('Index out of range');
+ }
+
+ function writeFloat(buf, value, offset, littleEndian, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 4);
+ }
+
+ ieee754.write(buf, value, offset, littleEndian, 23, 4);
+ return offset + 4;
+ }
+
+ Buffer.prototype.writeFloatLE = function writeFloatLE(value, offset, noAssert) {
+ return writeFloat(this, value, offset, true, noAssert);
+ };
+
+ Buffer.prototype.writeFloatBE = function writeFloatBE(value, offset, noAssert) {
+ return writeFloat(this, value, offset, false, noAssert);
+ };
+
+ function writeDouble(buf, value, offset, littleEndian, noAssert) {
+ value = +value;
+ offset = offset >>> 0;
+
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 8);
+ }
+
+ ieee754.write(buf, value, offset, littleEndian, 52, 8);
+ return offset + 8;
+ }
+
+ Buffer.prototype.writeDoubleLE = function writeDoubleLE(value, offset, noAssert) {
+ return writeDouble(this, value, offset, true, noAssert);
+ };
+
+ Buffer.prototype.writeDoubleBE = function writeDoubleBE(value, offset, noAssert) {
+ return writeDouble(this, value, offset, false, noAssert);
+ }; // copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)
+
+
+ Buffer.prototype.copy = function copy(target, targetStart, start, end) {
+ if (!Buffer.isBuffer(target)) throw new TypeError('argument should be a Buffer');
+ if (!start) start = 0;
+ if (!end && end !== 0) end = this.length;
+ if (targetStart >= target.length) targetStart = target.length;
+ if (!targetStart) targetStart = 0;
+ if (end > 0 && end < start) end = start; // Copy 0 bytes; we're done
+
+ if (end === start) return 0;
+ if (target.length === 0 || this.length === 0) return 0; // Fatal error conditions
+
+ if (targetStart < 0) {
+ throw new RangeError('targetStart out of bounds');
+ }
+
+ if (start < 0 || start >= this.length) throw new RangeError('Index out of range');
+ if (end < 0) throw new RangeError('sourceEnd out of bounds'); // Are we oob?
+
+ if (end > this.length) end = this.length;
+
+ if (target.length - targetStart < end - start) {
+ end = target.length - targetStart + start;
+ }
+
+ var len = end - start;
+
+ if (this === target && typeof Uint8Array.prototype.copyWithin === 'function') {
+ // Use built-in when available, missing from IE11
+ this.copyWithin(targetStart, start, end);
+ } else {
+ Uint8Array.prototype.set.call(target, this.subarray(start, end), targetStart);
+ }
+
+ return len;
+ }; // Usage:
+ // buffer.fill(number[, offset[, end]])
+ // buffer.fill(buffer[, offset[, end]])
+ // buffer.fill(string[, offset[, end]][, encoding])
+
+
+ Buffer.prototype.fill = function fill(val, start, end, encoding) {
+ // Handle string cases:
+ if (typeof val === 'string') {
+ if (typeof start === 'string') {
+ encoding = start;
+ start = 0;
+ end = this.length;
+ } else if (typeof end === 'string') {
+ encoding = end;
+ end = this.length;
+ }
+
+ if (encoding !== undefined && typeof encoding !== 'string') {
+ throw new TypeError('encoding must be a string');
+ }
+
+ if (typeof encoding === 'string' && !Buffer.isEncoding(encoding)) {
+ throw new TypeError('Unknown encoding: ' + encoding);
+ }
+
+ if (val.length === 1) {
+ var code = val.charCodeAt(0);
+
+ if (encoding === 'utf8' && code < 128 || encoding === 'latin1') {
+ // Fast path: If `val` fits into a single byte, use that numeric value.
+ val = code;
+ }
+ }
+ } else if (typeof val === 'number') {
+ val = val & 255;
+ } else if (typeof val === 'boolean') {
+ val = Number(val);
+ } // Invalid ranges are not set to a default, so can range check early.
+
+
+ if (start < 0 || this.length < start || this.length < end) {
+ throw new RangeError('Out of range index');
+ }
+
+ if (end <= start) {
+ return this;
+ }
+
+ start = start >>> 0;
+ end = end === undefined ? this.length : end >>> 0;
+ if (!val) val = 0;
+ var i;
+
+ if (typeof val === 'number') {
+ for (i = start; i < end; ++i) {
+ this[i] = val;
+ }
+ } else {
+ var bytes = Buffer.isBuffer(val) ? val : Buffer.from(val, encoding);
+ var len = bytes.length;
+
+ if (len === 0) {
+ throw new TypeError('The value "' + val + '" is invalid for argument "value"');
+ }
+
+ for (i = 0; i < end - start; ++i) {
+ this[i + start] = bytes[i % len];
+ }
+ }
+
+ return this;
+ }; // HELPER FUNCTIONS
+ // ================
+
+
+ var INVALID_BASE64_RE = /[^+/0-9A-Za-z-_]/g;
+
+ function base64clean(str) {
+ // Node takes equal signs as end of the Base64 encoding
+ str = str.split('=')[0]; // Node strips out invalid characters like \n and \t from the string, base64-js does not
+
+ str = str.trim().replace(INVALID_BASE64_RE, ''); // Node converts strings with length < 2 to ''
+
+ if (str.length < 2) return ''; // Node allows for non-padded base64 strings (missing trailing ===), base64-js does not
+
+ while (str.length % 4 !== 0) {
+ str = str + '=';
+ }
+
+ return str;
+ }
+
+ function utf8ToBytes(string, units) {
+ units = units || Infinity;
+ var codePoint;
+ var length = string.length;
+ var leadSurrogate = null;
+ var bytes = [];
+
+ for (var i = 0; i < length; ++i) {
+ codePoint = string.charCodeAt(i); // is surrogate component
+
+ if (codePoint > 0xD7FF && codePoint < 0xE000) {
+ // last char was a lead
+ if (!leadSurrogate) {
+ // no lead yet
+ if (codePoint > 0xDBFF) {
+ // unexpected trail
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ continue;
+ } else if (i + 1 === length) {
+ // unpaired lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ continue;
+ } // valid lead
+
+
+ leadSurrogate = codePoint;
+ continue;
+ } // 2 leads in a row
+
+
+ if (codePoint < 0xDC00) {
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ leadSurrogate = codePoint;
+ continue;
+ } // valid surrogate pair
+
+
+ codePoint = (leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00) + 0x10000;
+ } else if (leadSurrogate) {
+ // valid bmp char, but last char was a lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD);
+ }
+
+ leadSurrogate = null; // encode utf8
+
+ if (codePoint < 0x80) {
+ if ((units -= 1) < 0) break;
+ bytes.push(codePoint);
+ } else if (codePoint < 0x800) {
+ if ((units -= 2) < 0) break;
+ bytes.push(codePoint >> 0x6 | 0xC0, codePoint & 0x3F | 0x80);
+ } else if (codePoint < 0x10000) {
+ if ((units -= 3) < 0) break;
+ bytes.push(codePoint >> 0xC | 0xE0, codePoint >> 0x6 & 0x3F | 0x80, codePoint & 0x3F | 0x80);
+ } else if (codePoint < 0x110000) {
+ if ((units -= 4) < 0) break;
+ bytes.push(codePoint >> 0x12 | 0xF0, codePoint >> 0xC & 0x3F | 0x80, codePoint >> 0x6 & 0x3F | 0x80, codePoint & 0x3F | 0x80);
+ } else {
+ throw new Error('Invalid code point');
+ }
+ }
+
+ return bytes;
+ }
+
+ function asciiToBytes(str) {
+ var byteArray = [];
+
+ for (var i = 0; i < str.length; ++i) {
+ // Node's code seems to be doing this and not & 0x7F..
+ byteArray.push(str.charCodeAt(i) & 0xFF);
+ }
+
+ return byteArray;
+ }
+
+ function utf16leToBytes(str, units) {
+ var c, hi, lo;
+ var byteArray = [];
+
+ for (var i = 0; i < str.length; ++i) {
+ if ((units -= 2) < 0) break;
+ c = str.charCodeAt(i);
+ hi = c >> 8;
+ lo = c % 256;
+ byteArray.push(lo);
+ byteArray.push(hi);
+ }
+
+ return byteArray;
+ }
+
+ function base64ToBytes(str) {
+ return base64Js.toByteArray(base64clean(str));
+ }
+
+ function blitBuffer(src, dst, offset, length) {
+ for (var i = 0; i < length; ++i) {
+ if (i + offset >= dst.length || i >= src.length) break;
+ dst[i + offset] = src[i];
+ }
+
+ return i;
+ } // ArrayBuffer or Uint8Array objects from other contexts (i.e. iframes) do not pass
+ // the `instanceof` check but they should be treated as of that type.
+ // See: https://github.com/feross/buffer/issues/166
+
+
+ function isInstance(obj, type) {
+ return obj instanceof type || obj != null && obj.constructor != null && obj.constructor.name != null && obj.constructor.name === type.name;
+ }
+
+ function numberIsNaN(obj) {
+ // For IE11 support
+ return obj !== obj; // eslint-disable-line no-self-compare
+ } // Create lookup table for `toString('hex')`
+ // See: https://github.com/feross/buffer/issues/219
+
+
+ var hexSliceLookupTable = function () {
+ var alphabet = '0123456789abcdef';
+ var table = new Array(256);
+
+ for (var i = 0; i < 16; ++i) {
+ var i16 = i * 16;
+
+ for (var j = 0; j < 16; ++j) {
+ table[i16 + j] = alphabet[i] + alphabet[j];
+ }
+ }
+
+ return table;
+ }();
+ });
+ buffer.Buffer;
+ buffer.SlowBuffer;
+ buffer.INSPECT_MAX_BYTES;
+ buffer.kMaxLength;
+
+ var require$$0 = {};
+
+ // shim for using process in browser
+
+ var performance = global.performance || {};
+
+ performance.now || performance.mozNow || performance.msNow || performance.oNow || performance.webkitNow || function () {
+ return new Date().getTime();
+ }; // generate timestamp or delta
+
+ var inherits;
+
+ if (typeof Object.create === 'function') {
+ inherits = function inherits(ctor, superCtor) {
+ // implementation from standard node.js 'util' module
+ ctor.super_ = superCtor;
+ ctor.prototype = Object.create(superCtor.prototype, {
+ constructor: {
+ value: ctor,
+ enumerable: false,
+ writable: true,
+ configurable: true
+ }
+ });
+ };
+ } else {
+ inherits = function inherits(ctor, superCtor) {
+ ctor.super_ = superCtor;
+
+ var TempCtor = function TempCtor() {};
+
+ TempCtor.prototype = superCtor.prototype;
+ ctor.prototype = new TempCtor();
+ ctor.prototype.constructor = ctor;
+ };
+ }
+
+ var inherits$1 = inherits;
+
+ // Copyright Joyent, Inc. and other Node contributors.
+ var formatRegExp = /%[sdj%]/g;
+ function format(f) {
+ if (!isString(f)) {
+ var objects = [];
+
+ for (var i = 0; i < arguments.length; i++) {
+ objects.push(inspect(arguments[i]));
+ }
+
+ return objects.join(' ');
+ }
+
+ var i = 1;
+ var args = arguments;
+ var len = args.length;
+ var str = String(f).replace(formatRegExp, function (x) {
+ if (x === '%%') return '%';
+ if (i >= len) return x;
+
+ switch (x) {
+ case '%s':
+ return String(args[i++]);
+
+ case '%d':
+ return Number(args[i++]);
+
+ case '%j':
+ try {
+ return JSON.stringify(args[i++]);
+ } catch (_) {
+ return '[Circular]';
+ }
+
+ default:
+ return x;
+ }
+ });
+
+ for (var x = args[i]; i < len; x = args[++i]) {
+ if (isNull(x) || !isObject(x)) {
+ str += ' ' + x;
+ } else {
+ str += ' ' + inspect(x);
+ }
+ }
+
+ return str;
+ }
+ // Returns a modified function which warns once by default.
+ // If --no-deprecation is set, then it is a no-op.
+
+ function deprecate(fn, msg) {
+ // Allow for deprecating things in the process of starting up.
+ if (isUndefined(global.process)) {
+ return function () {
+ return deprecate(fn, msg).apply(this, arguments);
+ };
+ }
+
+ var warned = false;
+
+ function deprecated() {
+ if (!warned) {
+ {
+ console.error(msg);
+ }
+
+ warned = true;
+ }
+
+ return fn.apply(this, arguments);
+ }
+
+ return deprecated;
+ }
+ var debugs = {};
+ var debugEnviron;
+ function debuglog(set) {
+ if (isUndefined(debugEnviron)) debugEnviron = '';
+ set = set.toUpperCase();
+
+ if (!debugs[set]) {
+ if (new RegExp('\\b' + set + '\\b', 'i').test(debugEnviron)) {
+ var pid = 0;
+
+ debugs[set] = function () {
+ var msg = format.apply(null, arguments);
+ console.error('%s %d: %s', set, pid, msg);
+ };
+ } else {
+ debugs[set] = function () {};
+ }
+ }
+
+ return debugs[set];
+ }
+ /**
+ * Echos the value of a value. Trys to print the value out
+ * in the best way possible given the different types.
+ *
+ * @param {Object} obj The object to print out.
+ * @param {Object} opts Optional options object that alters the output.
+ */
+
+ /* legacy: obj, showHidden, depth, colors*/
+
+ function inspect(obj, opts) {
+ // default options
+ var ctx = {
+ seen: [],
+ stylize: stylizeNoColor
+ }; // legacy...
+
+ if (arguments.length >= 3) ctx.depth = arguments[2];
+ if (arguments.length >= 4) ctx.colors = arguments[3];
+
+ if (isBoolean(opts)) {
+ // legacy...
+ ctx.showHidden = opts;
+ } else if (opts) {
+ // got an "options" object
+ _extend(ctx, opts);
+ } // set default options
+
+
+ if (isUndefined(ctx.showHidden)) ctx.showHidden = false;
+ if (isUndefined(ctx.depth)) ctx.depth = 2;
+ if (isUndefined(ctx.colors)) ctx.colors = false;
+ if (isUndefined(ctx.customInspect)) ctx.customInspect = true;
+ if (ctx.colors) ctx.stylize = stylizeWithColor;
+ return formatValue(ctx, obj, ctx.depth);
+ } // http://en.wikipedia.org/wiki/ANSI_escape_code#graphics
+
+ inspect.colors = {
+ 'bold': [1, 22],
+ 'italic': [3, 23],
+ 'underline': [4, 24],
+ 'inverse': [7, 27],
+ 'white': [37, 39],
+ 'grey': [90, 39],
+ 'black': [30, 39],
+ 'blue': [34, 39],
+ 'cyan': [36, 39],
+ 'green': [32, 39],
+ 'magenta': [35, 39],
+ 'red': [31, 39],
+ 'yellow': [33, 39]
+ }; // Don't use 'blue' not visible on cmd.exe
+
+ inspect.styles = {
+ 'special': 'cyan',
+ 'number': 'yellow',
+ 'boolean': 'yellow',
+ 'undefined': 'grey',
+ 'null': 'bold',
+ 'string': 'green',
+ 'date': 'magenta',
+ // "name": intentionally not styling
+ 'regexp': 'red'
+ };
+
+ function stylizeWithColor(str, styleType) {
+ var style = inspect.styles[styleType];
+
+ if (style) {
+ return "\x1B[" + inspect.colors[style][0] + 'm' + str + "\x1B[" + inspect.colors[style][1] + 'm';
+ } else {
+ return str;
+ }
+ }
+
+ function stylizeNoColor(str, styleType) {
+ return str;
+ }
+
+ function arrayToHash(array) {
+ var hash = {};
+ array.forEach(function (val, idx) {
+ hash[val] = true;
+ });
+ return hash;
+ }
+
+ function formatValue(ctx, value, recurseTimes) {
+ // Provide a hook for user-specified inspect functions.
+ // Check that value is an object with an inspect function on it
+ if (ctx.customInspect && value && isFunction(value.inspect) && // Filter out the util module, it's inspect function is special
+ value.inspect !== inspect && // Also filter out any prototype objects using the circular check.
+ !(value.constructor && value.constructor.prototype === value)) {
+ var ret = value.inspect(recurseTimes, ctx);
+
+ if (!isString(ret)) {
+ ret = formatValue(ctx, ret, recurseTimes);
+ }
+
+ return ret;
+ } // Primitive types cannot have properties
+
+
+ var primitive = formatPrimitive(ctx, value);
+
+ if (primitive) {
+ return primitive;
+ } // Look up the keys of the object.
+
+
+ var keys = Object.keys(value);
+ var visibleKeys = arrayToHash(keys);
+
+ if (ctx.showHidden) {
+ keys = Object.getOwnPropertyNames(value);
+ } // IE doesn't make error fields non-enumerable
+ // http://msdn.microsoft.com/en-us/library/ie/dww52sbt(v=vs.94).aspx
+
+
+ if (isError(value) && (keys.indexOf('message') >= 0 || keys.indexOf('description') >= 0)) {
+ return formatError(value);
+ } // Some type of object without properties can be shortcutted.
+
+
+ if (keys.length === 0) {
+ if (isFunction(value)) {
+ var name = value.name ? ': ' + value.name : '';
+ return ctx.stylize('[Function' + name + ']', 'special');
+ }
+
+ if (isRegExp(value)) {
+ return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
+ }
+
+ if (isDate(value)) {
+ return ctx.stylize(Date.prototype.toString.call(value), 'date');
+ }
+
+ if (isError(value)) {
+ return formatError(value);
+ }
+ }
+
+ var base = '',
+ array = false,
+ braces = ['{', '}']; // Make Array say that they are Array
+
+ if (isArray(value)) {
+ array = true;
+ braces = ['[', ']'];
+ } // Make functions say that they are functions
+
+
+ if (isFunction(value)) {
+ var n = value.name ? ': ' + value.name : '';
+ base = ' [Function' + n + ']';
+ } // Make RegExps say that they are RegExps
+
+
+ if (isRegExp(value)) {
+ base = ' ' + RegExp.prototype.toString.call(value);
+ } // Make dates with properties first say the date
+
+
+ if (isDate(value)) {
+ base = ' ' + Date.prototype.toUTCString.call(value);
+ } // Make error with message first say the error
+
+
+ if (isError(value)) {
+ base = ' ' + formatError(value);
+ }
+
+ if (keys.length === 0 && (!array || value.length == 0)) {
+ return braces[0] + base + braces[1];
+ }
+
+ if (recurseTimes < 0) {
+ if (isRegExp(value)) {
+ return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
+ } else {
+ return ctx.stylize('[Object]', 'special');
+ }
+ }
+
+ ctx.seen.push(value);
+ var output;
+
+ if (array) {
+ output = formatArray(ctx, value, recurseTimes, visibleKeys, keys);
+ } else {
+ output = keys.map(function (key) {
+ return formatProperty(ctx, value, recurseTimes, visibleKeys, key, array);
+ });
+ }
+
+ ctx.seen.pop();
+ return reduceToSingleString(output, base, braces);
+ }
+
+ function formatPrimitive(ctx, value) {
+ if (isUndefined(value)) return ctx.stylize('undefined', 'undefined');
+
+ if (isString(value)) {
+ var simple = '\'' + JSON.stringify(value).replace(/^"|"$/g, '').replace(/'/g, "\\'").replace(/\\"/g, '"') + '\'';
+ return ctx.stylize(simple, 'string');
+ }
+
+ if (isNumber(value)) return ctx.stylize('' + value, 'number');
+ if (isBoolean(value)) return ctx.stylize('' + value, 'boolean'); // For some reason typeof null is "object", so special case here.
+
+ if (isNull(value)) return ctx.stylize('null', 'null');
+ }
+
+ function formatError(value) {
+ return '[' + Error.prototype.toString.call(value) + ']';
+ }
+
+ function formatArray(ctx, value, recurseTimes, visibleKeys, keys) {
+ var output = [];
+
+ for (var i = 0, l = value.length; i < l; ++i) {
+ if (hasOwnProperty(value, String(i))) {
+ output.push(formatProperty(ctx, value, recurseTimes, visibleKeys, String(i), true));
+ } else {
+ output.push('');
+ }
+ }
+
+ keys.forEach(function (key) {
+ if (!key.match(/^\d+$/)) {
+ output.push(formatProperty(ctx, value, recurseTimes, visibleKeys, key, true));
+ }
+ });
+ return output;
+ }
+
+ function formatProperty(ctx, value, recurseTimes, visibleKeys, key, array) {
+ var name, str, desc;
+ desc = Object.getOwnPropertyDescriptor(value, key) || {
+ value: value[key]
+ };
+
+ if (desc.get) {
+ if (desc.set) {
+ str = ctx.stylize('[Getter/Setter]', 'special');
+ } else {
+ str = ctx.stylize('[Getter]', 'special');
+ }
+ } else {
+ if (desc.set) {
+ str = ctx.stylize('[Setter]', 'special');
+ }
+ }
+
+ if (!hasOwnProperty(visibleKeys, key)) {
+ name = '[' + key + ']';
+ }
+
+ if (!str) {
+ if (ctx.seen.indexOf(desc.value) < 0) {
+ if (isNull(recurseTimes)) {
+ str = formatValue(ctx, desc.value, null);
+ } else {
+ str = formatValue(ctx, desc.value, recurseTimes - 1);
+ }
+
+ if (str.indexOf('\n') > -1) {
+ if (array) {
+ str = str.split('\n').map(function (line) {
+ return ' ' + line;
+ }).join('\n').substr(2);
+ } else {
+ str = '\n' + str.split('\n').map(function (line) {
+ return ' ' + line;
+ }).join('\n');
+ }
+ }
+ } else {
+ str = ctx.stylize('[Circular]', 'special');
+ }
+ }
+
+ if (isUndefined(name)) {
+ if (array && key.match(/^\d+$/)) {
+ return str;
+ }
+
+ name = JSON.stringify('' + key);
+
+ if (name.match(/^"([a-zA-Z_][a-zA-Z_0-9]*)"$/)) {
+ name = name.substr(1, name.length - 2);
+ name = ctx.stylize(name, 'name');
+ } else {
+ name = name.replace(/'/g, "\\'").replace(/\\"/g, '"').replace(/(^"|"$)/g, "'");
+ name = ctx.stylize(name, 'string');
+ }
+ }
+
+ return name + ': ' + str;
+ }
+
+ function reduceToSingleString(output, base, braces) {
+ var length = output.reduce(function (prev, cur) {
+ if (cur.indexOf('\n') >= 0) ;
+ return prev + cur.replace(/\u001b\[\d\d?m/g, '').length + 1;
+ }, 0);
+
+ if (length > 60) {
+ return braces[0] + (base === '' ? '' : base + '\n ') + ' ' + output.join(',\n ') + ' ' + braces[1];
+ }
+
+ return braces[0] + base + ' ' + output.join(', ') + ' ' + braces[1];
+ } // NOTE: These type checking functions intentionally don't use `instanceof`
+ // because it is fragile and can be easily faked with `Object.create()`.
+
+
+ function isArray(ar) {
+ return Array.isArray(ar);
+ }
+ function isBoolean(arg) {
+ return typeof arg === 'boolean';
+ }
+ function isNull(arg) {
+ return arg === null;
+ }
+ function isNullOrUndefined(arg) {
+ return arg == null;
+ }
+ function isNumber(arg) {
+ return typeof arg === 'number';
+ }
+ function isString(arg) {
+ return typeof arg === 'string';
+ }
+ function isSymbol(arg) {
+ return babelHelpers["typeof"](arg) === 'symbol';
+ }
+ function isUndefined(arg) {
+ return arg === void 0;
+ }
+ function isRegExp(re) {
+ return isObject(re) && objectToString(re) === '[object RegExp]';
+ }
+ function isObject(arg) {
+ return babelHelpers["typeof"](arg) === 'object' && arg !== null;
+ }
+ function isDate(d) {
+ return isObject(d) && objectToString(d) === '[object Date]';
+ }
+ function isError(e) {
+ return isObject(e) && (objectToString(e) === '[object Error]' || e instanceof Error);
+ }
+ function isFunction(arg) {
+ return typeof arg === 'function';
+ }
+ function isPrimitive(arg) {
+ return arg === null || typeof arg === 'boolean' || typeof arg === 'number' || typeof arg === 'string' || babelHelpers["typeof"](arg) === 'symbol' || // ES6 symbol
+ typeof arg === 'undefined';
+ }
+ function isBuffer(maybeBuf) {
+ return Buffer.isBuffer(maybeBuf);
+ }
+
+ function objectToString(o) {
+ return Object.prototype.toString.call(o);
+ }
+
+ function pad(n) {
+ return n < 10 ? '0' + n.toString(10) : n.toString(10);
+ }
+
+ var months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']; // 26 Feb 16:19:34
+
+ function timestamp$1() {
+ var d = new Date();
+ var time = [pad(d.getHours()), pad(d.getMinutes()), pad(d.getSeconds())].join(':');
+ return [d.getDate(), months[d.getMonth()], time].join(' ');
+ } // log is just a thin wrapper to console.log that prepends a timestamp
+
+
+ function log() {
+ console.log('%s - %s', timestamp$1(), format.apply(null, arguments));
+ }
+ function _extend(origin, add) {
+ // Don't do anything if add isn't an object
+ if (!add || !isObject(add)) return origin;
+ var keys = Object.keys(add);
+ var i = keys.length;
+
+ while (i--) {
+ origin[keys[i]] = add[keys[i]];
+ }
+
+ return origin;
+ }
+
+ function hasOwnProperty(obj, prop) {
+ return Object.prototype.hasOwnProperty.call(obj, prop);
+ }
+
+ var require$$1 = {
+ inherits: inherits$1,
+ _extend: _extend,
+ log: log,
+ isBuffer: isBuffer,
+ isPrimitive: isPrimitive,
+ isFunction: isFunction,
+ isError: isError,
+ isDate: isDate,
+ isObject: isObject,
+ isRegExp: isRegExp,
+ isUndefined: isUndefined,
+ isSymbol: isSymbol,
+ isString: isString,
+ isNumber: isNumber,
+ isNullOrUndefined: isNullOrUndefined,
+ isNull: isNull,
+ isBoolean: isBoolean,
+ isArray: isArray,
+ inspect: inspect,
+ deprecate: deprecate,
+ format: format,
+ debuglog: debuglog
+ };
+
+ var utils = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.deprecate = exports.isObjectLike = exports.isDate = exports.haveBuffer = exports.isMap = exports.isRegExp = exports.isBigUInt64Array = exports.isBigInt64Array = exports.isUint8Array = exports.isAnyArrayBuffer = exports.randomBytes = exports.normalizedFunctionString = void 0;
+
+ /**
+ * Normalizes our expected stringified form of a function across versions of node
+ * @param fn - The function to stringify
+ */
+ function normalizedFunctionString(fn) {
+ return fn.toString().replace('function(', 'function (');
+ }
+ exports.normalizedFunctionString = normalizedFunctionString;
+ var isReactNative = typeof commonjsGlobal.navigator === 'object' && commonjsGlobal.navigator.product === 'ReactNative';
+ var insecureWarning = isReactNative
+ ? 'BSON: For React Native please polyfill crypto.getRandomValues, e.g. using: https://www.npmjs.com/package/react-native-get-random-values.'
+ : 'BSON: No cryptographic implementation for random bytes present, falling back to a less secure implementation.';
+ var insecureRandomBytes = function insecureRandomBytes(size) {
+ console.warn(insecureWarning);
+ var result = buffer.Buffer.alloc(size);
+ for (var i = 0; i < size; ++i)
+ result[i] = Math.floor(Math.random() * 256);
+ return result;
+ };
+ var detectRandomBytes = function () {
+ if (typeof window !== 'undefined') {
+ // browser crypto implementation(s)
+ var target_1 = window.crypto || window.msCrypto; // allow for IE11
+ if (target_1 && target_1.getRandomValues) {
+ return function (size) { return target_1.getRandomValues(buffer.Buffer.alloc(size)); };
+ }
+ }
+ if (typeof commonjsGlobal !== 'undefined' && commonjsGlobal.crypto && commonjsGlobal.crypto.getRandomValues) {
+ // allow for RN packages such as https://www.npmjs.com/package/react-native-get-random-values to populate global
+ return function (size) { return commonjsGlobal.crypto.getRandomValues(buffer.Buffer.alloc(size)); };
+ }
+ var requiredRandomBytes;
+ try {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ requiredRandomBytes = require$$0.randomBytes;
+ }
+ catch (e) {
+ // keep the fallback
+ }
+ // NOTE: in transpiled cases the above require might return null/undefined
+ return requiredRandomBytes || insecureRandomBytes;
+ };
+ exports.randomBytes = detectRandomBytes();
+ function isAnyArrayBuffer(value) {
+ return ['[object ArrayBuffer]', '[object SharedArrayBuffer]'].includes(Object.prototype.toString.call(value));
+ }
+ exports.isAnyArrayBuffer = isAnyArrayBuffer;
+ function isUint8Array(value) {
+ return Object.prototype.toString.call(value) === '[object Uint8Array]';
+ }
+ exports.isUint8Array = isUint8Array;
+ function isBigInt64Array(value) {
+ return Object.prototype.toString.call(value) === '[object BigInt64Array]';
+ }
+ exports.isBigInt64Array = isBigInt64Array;
+ function isBigUInt64Array(value) {
+ return Object.prototype.toString.call(value) === '[object BigUint64Array]';
+ }
+ exports.isBigUInt64Array = isBigUInt64Array;
+ function isRegExp(d) {
+ return Object.prototype.toString.call(d) === '[object RegExp]';
+ }
+ exports.isRegExp = isRegExp;
+ function isMap(d) {
+ return Object.prototype.toString.call(d) === '[object Map]';
+ }
+ exports.isMap = isMap;
+ /** Call to check if your environment has `Buffer` */
+ function haveBuffer() {
+ return typeof commonjsGlobal !== 'undefined' && typeof commonjsGlobal.Buffer !== 'undefined';
+ }
+ exports.haveBuffer = haveBuffer;
+ // To ensure that 0.4 of node works correctly
+ function isDate(d) {
+ return isObjectLike(d) && Object.prototype.toString.call(d) === '[object Date]';
+ }
+ exports.isDate = isDate;
+ /**
+ * @internal
+ * this is to solve the `'someKey' in x` problem where x is unknown.
+ * https://github.com/typescript-eslint/typescript-eslint/issues/1071#issuecomment-541955753
+ */
+ function isObjectLike(candidate) {
+ return typeof candidate === 'object' && candidate !== null;
+ }
+ exports.isObjectLike = isObjectLike;
+ function deprecate(fn, message) {
+ if (typeof window === 'undefined' && typeof self === 'undefined') {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ return require$$1.deprecate(fn, message);
+ }
+ var warned = false;
+ function deprecated() {
+ var args = [];
+ for (var _i = 0; _i < arguments.length; _i++) {
+ args[_i] = arguments[_i];
+ }
+ if (!warned) {
+ console.warn(message);
+ warned = true;
+ }
+ return fn.apply(this, args);
+ }
+ return deprecated;
+ }
+ exports.deprecate = deprecate;
+
+ });
+
+ unwrapExports(utils);
+ utils.deprecate;
+ utils.isObjectLike;
+ utils.isDate;
+ utils.haveBuffer;
+ utils.isMap;
+ utils.isRegExp;
+ utils.isBigUInt64Array;
+ utils.isBigInt64Array;
+ utils.isUint8Array;
+ utils.isAnyArrayBuffer;
+ utils.randomBytes;
+ utils.normalizedFunctionString;
+
+ var ensure_buffer = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.ensureBuffer = void 0;
+
+
+ /**
+ * Makes sure that, if a Uint8Array is passed in, it is wrapped in a Buffer.
+ *
+ * @param potentialBuffer - The potential buffer
+ * @returns Buffer the input if potentialBuffer is a buffer, or a buffer that
+ * wraps a passed in Uint8Array
+ * @throws TypeError If anything other than a Buffer or Uint8Array is passed in
+ */
+ function ensureBuffer(potentialBuffer) {
+ if (ArrayBuffer.isView(potentialBuffer)) {
+ return buffer.Buffer.from(potentialBuffer.buffer, potentialBuffer.byteOffset, potentialBuffer.byteLength);
+ }
+ if (utils.isAnyArrayBuffer(potentialBuffer)) {
+ return buffer.Buffer.from(potentialBuffer);
+ }
+ throw new TypeError('Must use either Buffer or TypedArray');
+ }
+ exports.ensureBuffer = ensureBuffer;
+
+ });
+
+ unwrapExports(ensure_buffer);
+ ensure_buffer.ensureBuffer;
+
+ var uuid_utils = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.bufferToUuidHexString = exports.uuidHexStringToBuffer = exports.uuidValidateString = void 0;
+
+ // Validation regex for v4 uuid (validates with or without dashes)
+ var VALIDATION_REGEX = /^(?:[0-9a-f]{8}-[0-9a-f]{4}-4[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}|[0-9a-f]{12}4[0-9a-f]{3}[89ab][0-9a-f]{15})$/i;
+ var uuidValidateString = function (str) {
+ return typeof str === 'string' && VALIDATION_REGEX.test(str);
+ };
+ exports.uuidValidateString = uuidValidateString;
+ var uuidHexStringToBuffer = function (hexString) {
+ if (!exports.uuidValidateString(hexString)) {
+ throw new TypeError('UUID string representations must be a 32 or 36 character hex string (dashes excluded/included). Format: "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx" or "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx".');
+ }
+ var sanitizedHexString = hexString.replace(/-/g, '');
+ return buffer.Buffer.from(sanitizedHexString, 'hex');
+ };
+ exports.uuidHexStringToBuffer = uuidHexStringToBuffer;
+ var bufferToUuidHexString = function (buffer, includeDashes) {
+ if (includeDashes === void 0) { includeDashes = true; }
+ return includeDashes
+ ? buffer.toString('hex', 0, 4) +
+ '-' +
+ buffer.toString('hex', 4, 6) +
+ '-' +
+ buffer.toString('hex', 6, 8) +
+ '-' +
+ buffer.toString('hex', 8, 10) +
+ '-' +
+ buffer.toString('hex', 10, 16)
+ : buffer.toString('hex');
+ };
+ exports.bufferToUuidHexString = bufferToUuidHexString;
+
+ });
+
+ unwrapExports(uuid_utils);
+ uuid_utils.bufferToUuidHexString;
+ uuid_utils.uuidHexStringToBuffer;
+ uuid_utils.uuidValidateString;
+
+ var uuid = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.UUID = void 0;
+
+
+
+
+
+ var BYTE_LENGTH = 16;
+ var kId = Symbol('id');
+ /**
+ * A class representation of the BSON UUID type.
+ * @public
+ */
+ var UUID = /** @class */ (function () {
+ /**
+ * Create an UUID type
+ *
+ * @param input - Can be a 32 or 36 character hex string (dashes excluded/included) or a 16 byte binary Buffer.
+ */
+ function UUID(input) {
+ if (typeof input === 'undefined') {
+ // The most common use case (blank id, new UUID() instance)
+ this.id = UUID.generate();
+ }
+ else if (input instanceof UUID) {
+ this[kId] = buffer.Buffer.from(input.id);
+ this.__id = input.__id;
+ }
+ else if (ArrayBuffer.isView(input) && input.byteLength === BYTE_LENGTH) {
+ this.id = ensure_buffer.ensureBuffer(input);
+ }
+ else if (typeof input === 'string') {
+ this.id = uuid_utils.uuidHexStringToBuffer(input);
+ }
+ else {
+ throw new TypeError('Argument passed in UUID constructor must be a UUID, a 16 byte Buffer or a 32/36 character hex string (dashes excluded/included, format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx).');
+ }
+ }
+ Object.defineProperty(UUID.prototype, "id", {
+ /**
+ * The UUID bytes
+ * @readonly
+ */
+ get: function () {
+ return this[kId];
+ },
+ set: function (value) {
+ this[kId] = value;
+ if (UUID.cacheHexString) {
+ this.__id = uuid_utils.bufferToUuidHexString(value);
+ }
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /**
+ * Generate a 16 byte uuid v4 buffer used in UUIDs
+ */
+ /**
+ * Returns the UUID id as a 32 or 36 character hex string representation, excluding/including dashes (defaults to 36 character dash separated)
+ * @param includeDashes - should the string exclude dash-separators.
+ * */
+ UUID.prototype.toHexString = function (includeDashes) {
+ if (includeDashes === void 0) { includeDashes = true; }
+ if (UUID.cacheHexString && this.__id) {
+ return this.__id;
+ }
+ var uuidHexString = uuid_utils.bufferToUuidHexString(this.id, includeDashes);
+ if (UUID.cacheHexString) {
+ this.__id = uuidHexString;
+ }
+ return uuidHexString;
+ };
+ /**
+ * Converts the id into a 36 character (dashes included) hex string, unless a encoding is specified.
+ * @internal
+ */
+ UUID.prototype.toString = function (encoding) {
+ return encoding ? this.id.toString(encoding) : this.toHexString();
+ };
+ /**
+ * Converts the id into its JSON string representation. A 36 character (dashes included) hex string in the format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx
+ * @internal
+ */
+ UUID.prototype.toJSON = function () {
+ return this.toHexString();
+ };
+ /**
+ * Compares the equality of this UUID with `otherID`.
+ *
+ * @param otherId - UUID instance to compare against.
+ */
+ UUID.prototype.equals = function (otherId) {
+ if (!otherId) {
+ return false;
+ }
+ if (otherId instanceof UUID) {
+ return otherId.id.equals(this.id);
+ }
+ try {
+ return new UUID(otherId).id.equals(this.id);
+ }
+ catch (_a) {
+ return false;
+ }
+ };
+ /**
+ * Creates a Binary instance from the current UUID.
+ */
+ UUID.prototype.toBinary = function () {
+ return new binary.Binary(this.id, binary.Binary.SUBTYPE_UUID);
+ };
+ /**
+ * Generates a populated buffer containing a v4 uuid
+ */
+ UUID.generate = function () {
+ var bytes = utils.randomBytes(BYTE_LENGTH);
+ // Per 4.4, set bits for version and `clock_seq_hi_and_reserved`
+ // Kindly borrowed from https://github.com/uuidjs/uuid/blob/master/src/v4.js
+ bytes[6] = (bytes[6] & 0x0f) | 0x40;
+ bytes[8] = (bytes[8] & 0x3f) | 0x80;
+ return buffer.Buffer.from(bytes);
+ };
+ /**
+ * Checks if a value is a valid bson UUID
+ * @param input - UUID, string or Buffer to validate.
+ */
+ UUID.isValid = function (input) {
+ if (!input) {
+ return false;
+ }
+ if (input instanceof UUID) {
+ return true;
+ }
+ if (typeof input === 'string') {
+ return uuid_utils.uuidValidateString(input);
+ }
+ if (utils.isUint8Array(input)) {
+ // check for length & uuid version (https://tools.ietf.org/html/rfc4122#section-4.1.3)
+ if (input.length !== BYTE_LENGTH) {
+ return false;
+ }
+ try {
+ // get this byte as hex: xxxxxxxx-xxxx-XXxx-xxxx-xxxxxxxxxxxx
+ // check first part as uuid version: xxxxxxxx-xxxx-Xxxx-xxxx-xxxxxxxxxxxx
+ return parseInt(input[6].toString(16)[0], 10) === binary.Binary.SUBTYPE_UUID;
+ }
+ catch (_a) {
+ return false;
+ }
+ }
+ return false;
+ };
+ /**
+ * Creates an UUID from a hex string representation of an UUID.
+ * @param hexString - 32 or 36 character hex string (dashes excluded/included).
+ */
+ UUID.createFromHexString = function (hexString) {
+ var buffer = uuid_utils.uuidHexStringToBuffer(hexString);
+ return new UUID(buffer);
+ };
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 36 character hex string representation.
+ * @internal
+ */
+ UUID.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ UUID.prototype.inspect = function () {
+ return "new UUID(\"" + this.toHexString() + "\")";
+ };
+ return UUID;
+ }());
+ exports.UUID = UUID;
+ Object.defineProperty(UUID.prototype, '_bsontype', { value: 'UUID' });
+
+ });
+
+ unwrapExports(uuid);
+ uuid.UUID;
+
+ var binary = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Binary = void 0;
+
+
+
+
+ /**
+ * A class representation of the BSON Binary type.
+ * @public
+ */
+ var Binary = /** @class */ (function () {
+ /**
+ * @param buffer - a buffer object containing the binary data.
+ * @param subType - the option binary type.
+ */
+ function Binary(buffer$1, subType) {
+ if (!(this instanceof Binary))
+ return new Binary(buffer$1, subType);
+ if (!(buffer$1 == null) &&
+ !(typeof buffer$1 === 'string') &&
+ !ArrayBuffer.isView(buffer$1) &&
+ !(buffer$1 instanceof ArrayBuffer) &&
+ !Array.isArray(buffer$1)) {
+ throw new TypeError('Binary can only be constructed from string, Buffer, TypedArray, or Array');
+ }
+ this.sub_type = subType !== null && subType !== void 0 ? subType : Binary.BSON_BINARY_SUBTYPE_DEFAULT;
+ if (buffer$1 == null) {
+ // create an empty binary buffer
+ this.buffer = buffer.Buffer.alloc(Binary.BUFFER_SIZE);
+ this.position = 0;
+ }
+ else {
+ if (typeof buffer$1 === 'string') {
+ // string
+ this.buffer = buffer.Buffer.from(buffer$1, 'binary');
+ }
+ else if (Array.isArray(buffer$1)) {
+ // number[]
+ this.buffer = buffer.Buffer.from(buffer$1);
+ }
+ else {
+ // Buffer | TypedArray | ArrayBuffer
+ this.buffer = ensure_buffer.ensureBuffer(buffer$1);
+ }
+ this.position = this.buffer.byteLength;
+ }
+ }
+ /**
+ * Updates this binary with byte_value.
+ *
+ * @param byteValue - a single byte we wish to write.
+ */
+ Binary.prototype.put = function (byteValue) {
+ // If it's a string and a has more than one character throw an error
+ if (typeof byteValue === 'string' && byteValue.length !== 1) {
+ throw new TypeError('only accepts single character String');
+ }
+ else if (typeof byteValue !== 'number' && byteValue.length !== 1)
+ throw new TypeError('only accepts single character Uint8Array or Array');
+ // Decode the byte value once
+ var decodedByte;
+ if (typeof byteValue === 'string') {
+ decodedByte = byteValue.charCodeAt(0);
+ }
+ else if (typeof byteValue === 'number') {
+ decodedByte = byteValue;
+ }
+ else {
+ decodedByte = byteValue[0];
+ }
+ if (decodedByte < 0 || decodedByte > 255) {
+ throw new TypeError('only accepts number in a valid unsigned byte range 0-255');
+ }
+ if (this.buffer.length > this.position) {
+ this.buffer[this.position++] = decodedByte;
+ }
+ else {
+ var buffer$1 = buffer.Buffer.alloc(Binary.BUFFER_SIZE + this.buffer.length);
+ // Combine the two buffers together
+ this.buffer.copy(buffer$1, 0, 0, this.buffer.length);
+ this.buffer = buffer$1;
+ this.buffer[this.position++] = decodedByte;
+ }
+ };
+ /**
+ * Writes a buffer or string to the binary.
+ *
+ * @param sequence - a string or buffer to be written to the Binary BSON object.
+ * @param offset - specify the binary of where to write the content.
+ */
+ Binary.prototype.write = function (sequence, offset) {
+ offset = typeof offset === 'number' ? offset : this.position;
+ // If the buffer is to small let's extend the buffer
+ if (this.buffer.length < offset + sequence.length) {
+ var buffer$1 = buffer.Buffer.alloc(this.buffer.length + sequence.length);
+ this.buffer.copy(buffer$1, 0, 0, this.buffer.length);
+ // Assign the new buffer
+ this.buffer = buffer$1;
+ }
+ if (ArrayBuffer.isView(sequence)) {
+ this.buffer.set(ensure_buffer.ensureBuffer(sequence), offset);
+ this.position =
+ offset + sequence.byteLength > this.position ? offset + sequence.length : this.position;
+ }
+ else if (typeof sequence === 'string') {
+ this.buffer.write(sequence, offset, sequence.length, 'binary');
+ this.position =
+ offset + sequence.length > this.position ? offset + sequence.length : this.position;
+ }
+ };
+ /**
+ * Reads **length** bytes starting at **position**.
+ *
+ * @param position - read from the given position in the Binary.
+ * @param length - the number of bytes to read.
+ */
+ Binary.prototype.read = function (position, length) {
+ length = length && length > 0 ? length : this.position;
+ // Let's return the data based on the type we have
+ return this.buffer.slice(position, position + length);
+ };
+ /**
+ * Returns the value of this binary as a string.
+ * @param asRaw - Will skip converting to a string
+ * @remarks
+ * This is handy when calling this function conditionally for some key value pairs and not others
+ */
+ Binary.prototype.value = function (asRaw) {
+ asRaw = !!asRaw;
+ // Optimize to serialize for the situation where the data == size of buffer
+ if (asRaw && this.buffer.length === this.position) {
+ return this.buffer;
+ }
+ // If it's a node.js buffer object
+ if (asRaw) {
+ return this.buffer.slice(0, this.position);
+ }
+ return this.buffer.toString('binary', 0, this.position);
+ };
+ /** the length of the binary sequence */
+ Binary.prototype.length = function () {
+ return this.position;
+ };
+ /** @internal */
+ Binary.prototype.toJSON = function () {
+ return this.buffer.toString('base64');
+ };
+ /** @internal */
+ Binary.prototype.toString = function (format) {
+ return this.buffer.toString(format);
+ };
+ /** @internal */
+ Binary.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ var base64String = this.buffer.toString('base64');
+ var subType = Number(this.sub_type).toString(16);
+ if (options.legacy) {
+ return {
+ $binary: base64String,
+ $type: subType.length === 1 ? '0' + subType : subType
+ };
+ }
+ return {
+ $binary: {
+ base64: base64String,
+ subType: subType.length === 1 ? '0' + subType : subType
+ }
+ };
+ };
+ /** @internal */
+ Binary.prototype.toUUID = function () {
+ if (this.sub_type === Binary.SUBTYPE_UUID) {
+ return new uuid.UUID(this.buffer.slice(0, this.position));
+ }
+ throw new Error("Binary sub_type \"" + this.sub_type + "\" is not supported for converting to UUID. Only \"" + Binary.SUBTYPE_UUID + "\" is currently supported.");
+ };
+ /** @internal */
+ Binary.fromExtendedJSON = function (doc, options) {
+ options = options || {};
+ var data;
+ var type;
+ if ('$binary' in doc) {
+ if (options.legacy && typeof doc.$binary === 'string' && '$type' in doc) {
+ type = doc.$type ? parseInt(doc.$type, 16) : 0;
+ data = buffer.Buffer.from(doc.$binary, 'base64');
+ }
+ else {
+ if (typeof doc.$binary !== 'string') {
+ type = doc.$binary.subType ? parseInt(doc.$binary.subType, 16) : 0;
+ data = buffer.Buffer.from(doc.$binary.base64, 'base64');
+ }
+ }
+ }
+ else if ('$uuid' in doc) {
+ type = 4;
+ data = uuid_utils.uuidHexStringToBuffer(doc.$uuid);
+ }
+ if (!data) {
+ throw new TypeError("Unexpected Binary Extended JSON format " + JSON.stringify(doc));
+ }
+ return new Binary(data, type);
+ };
+ /** @internal */
+ Binary.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Binary.prototype.inspect = function () {
+ var asBuffer = this.value(true);
+ return "new Binary(Buffer.from(\"" + asBuffer.toString('hex') + "\", \"hex\"), " + this.sub_type + ")";
+ };
+ /**
+ * Binary default subtype
+ * @internal
+ */
+ Binary.BSON_BINARY_SUBTYPE_DEFAULT = 0;
+ /** Initial buffer default size */
+ Binary.BUFFER_SIZE = 256;
+ /** Default BSON type */
+ Binary.SUBTYPE_DEFAULT = 0;
+ /** Function BSON type */
+ Binary.SUBTYPE_FUNCTION = 1;
+ /** Byte Array BSON type */
+ Binary.SUBTYPE_BYTE_ARRAY = 2;
+ /** Deprecated UUID BSON type @deprecated Please use SUBTYPE_UUID */
+ Binary.SUBTYPE_UUID_OLD = 3;
+ /** UUID BSON type */
+ Binary.SUBTYPE_UUID = 4;
+ /** MD5 BSON type */
+ Binary.SUBTYPE_MD5 = 5;
+ /** User BSON type */
+ Binary.SUBTYPE_USER_DEFINED = 128;
+ return Binary;
+ }());
+ exports.Binary = Binary;
+ Object.defineProperty(Binary.prototype, '_bsontype', { value: 'Binary' });
+
+ });
+
+ unwrapExports(binary);
+ binary.Binary;
+
+ var code = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Code = void 0;
+ /**
+ * A class representation of the BSON Code type.
+ * @public
+ */
+ var Code = /** @class */ (function () {
+ /**
+ * @param code - a string or function.
+ * @param scope - an optional scope for the function.
+ */
+ function Code(code, scope) {
+ if (!(this instanceof Code))
+ return new Code(code, scope);
+ this.code = code;
+ this.scope = scope;
+ }
+ /** @internal */
+ Code.prototype.toJSON = function () {
+ return { code: this.code, scope: this.scope };
+ };
+ /** @internal */
+ Code.prototype.toExtendedJSON = function () {
+ if (this.scope) {
+ return { $code: this.code, $scope: this.scope };
+ }
+ return { $code: this.code };
+ };
+ /** @internal */
+ Code.fromExtendedJSON = function (doc) {
+ return new Code(doc.$code, doc.$scope);
+ };
+ /** @internal */
+ Code.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Code.prototype.inspect = function () {
+ var codeJson = this.toJSON();
+ return "new Code(\"" + codeJson.code + "\"" + (codeJson.scope ? ", " + JSON.stringify(codeJson.scope) : '') + ")";
+ };
+ return Code;
+ }());
+ exports.Code = Code;
+ Object.defineProperty(Code.prototype, '_bsontype', { value: 'Code' });
+
+ });
+
+ unwrapExports(code);
+ code.Code;
+
+ var db_ref = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.DBRef = exports.isDBRefLike = void 0;
+
+ /** @internal */
+ function isDBRefLike(value) {
+ return (utils.isObjectLike(value) &&
+ value.$id != null &&
+ typeof value.$ref === 'string' &&
+ (value.$db == null || typeof value.$db === 'string'));
+ }
+ exports.isDBRefLike = isDBRefLike;
+ /**
+ * A class representation of the BSON DBRef type.
+ * @public
+ */
+ var DBRef = /** @class */ (function () {
+ /**
+ * @param collection - the collection name.
+ * @param oid - the reference ObjectId.
+ * @param db - optional db name, if omitted the reference is local to the current db.
+ */
+ function DBRef(collection, oid, db, fields) {
+ if (!(this instanceof DBRef))
+ return new DBRef(collection, oid, db, fields);
+ // check if namespace has been provided
+ var parts = collection.split('.');
+ if (parts.length === 2) {
+ db = parts.shift();
+ // eslint-disable-next-line @typescript-eslint/no-non-null-assertion
+ collection = parts.shift();
+ }
+ this.collection = collection;
+ this.oid = oid;
+ this.db = db;
+ this.fields = fields || {};
+ }
+ Object.defineProperty(DBRef.prototype, "namespace", {
+ // Property provided for compatibility with the 1.x parser
+ // the 1.x parser used a "namespace" property, while 4.x uses "collection"
+ /** @internal */
+ get: function () {
+ return this.collection;
+ },
+ set: function (value) {
+ this.collection = value;
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /** @internal */
+ DBRef.prototype.toJSON = function () {
+ var o = Object.assign({
+ $ref: this.collection,
+ $id: this.oid
+ }, this.fields);
+ if (this.db != null)
+ o.$db = this.db;
+ return o;
+ };
+ /** @internal */
+ DBRef.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ var o = {
+ $ref: this.collection,
+ $id: this.oid
+ };
+ if (options.legacy) {
+ return o;
+ }
+ if (this.db)
+ o.$db = this.db;
+ o = Object.assign(o, this.fields);
+ return o;
+ };
+ /** @internal */
+ DBRef.fromExtendedJSON = function (doc) {
+ var copy = Object.assign({}, doc);
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new DBRef(doc.$ref, doc.$id, doc.$db, copy);
+ };
+ /** @internal */
+ DBRef.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ DBRef.prototype.inspect = function () {
+ // NOTE: if OID is an ObjectId class it will just print the oid string.
+ var oid = this.oid === undefined || this.oid.toString === undefined ? this.oid : this.oid.toString();
+ return "new DBRef(\"" + this.namespace + "\", new ObjectId(\"" + oid + "\")" + (this.db ? ", \"" + this.db + "\"" : '') + ")";
+ };
+ return DBRef;
+ }());
+ exports.DBRef = DBRef;
+ Object.defineProperty(DBRef.prototype, '_bsontype', { value: 'DBRef' });
+
+ });
+
+ unwrapExports(db_ref);
+ db_ref.DBRef;
+ db_ref.isDBRefLike;
+
+ var long_1 = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Long = void 0;
+
+ /**
+ * wasm optimizations, to do native i64 multiplication and divide
+ */
+ var wasm = undefined;
+ try {
+ wasm = new WebAssembly.Instance(new WebAssembly.Module(
+ // prettier-ignore
+ new Uint8Array([0, 97, 115, 109, 1, 0, 0, 0, 1, 13, 2, 96, 0, 1, 127, 96, 4, 127, 127, 127, 127, 1, 127, 3, 7, 6, 0, 1, 1, 1, 1, 1, 6, 6, 1, 127, 1, 65, 0, 11, 7, 50, 6, 3, 109, 117, 108, 0, 1, 5, 100, 105, 118, 95, 115, 0, 2, 5, 100, 105, 118, 95, 117, 0, 3, 5, 114, 101, 109, 95, 115, 0, 4, 5, 114, 101, 109, 95, 117, 0, 5, 8, 103, 101, 116, 95, 104, 105, 103, 104, 0, 0, 10, 191, 1, 6, 4, 0, 35, 0, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 126, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 127, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 128, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 129, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 130, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11])), {}).exports;
+ }
+ catch (_a) {
+ // no wasm support
+ }
+ var TWO_PWR_16_DBL = 1 << 16;
+ var TWO_PWR_24_DBL = 1 << 24;
+ var TWO_PWR_32_DBL = TWO_PWR_16_DBL * TWO_PWR_16_DBL;
+ var TWO_PWR_64_DBL = TWO_PWR_32_DBL * TWO_PWR_32_DBL;
+ var TWO_PWR_63_DBL = TWO_PWR_64_DBL / 2;
+ /** A cache of the Long representations of small integer values. */
+ var INT_CACHE = {};
+ /** A cache of the Long representations of small unsigned integer values. */
+ var UINT_CACHE = {};
+ /**
+ * A class representing a 64-bit integer
+ * @public
+ * @remarks
+ * The internal representation of a long is the two given signed, 32-bit values.
+ * We use 32-bit pieces because these are the size of integers on which
+ * Javascript performs bit-operations. For operations like addition and
+ * multiplication, we split each number into 16 bit pieces, which can easily be
+ * multiplied within Javascript's floating-point representation without overflow
+ * or change in sign.
+ * In the algorithms below, we frequently reduce the negative case to the
+ * positive case by negating the input(s) and then post-processing the result.
+ * Note that we must ALWAYS check specially whether those values are MIN_VALUE
+ * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
+ * a positive number, it overflows back into a negative). Not handling this
+ * case would often result in infinite recursion.
+ * Common constant values ZERO, ONE, NEG_ONE, etc. are found as static properties on this class.
+ */
+ var Long = /** @class */ (function () {
+ /**
+ * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers.
+ * See the from* functions below for more convenient ways of constructing Longs.
+ *
+ * Acceptable signatures are:
+ * - Long(low, high, unsigned?)
+ * - Long(bigint, unsigned?)
+ * - Long(string, unsigned?)
+ *
+ * @param low - The low (signed) 32 bits of the long
+ * @param high - The high (signed) 32 bits of the long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ function Long(low, high, unsigned) {
+ if (low === void 0) { low = 0; }
+ if (!(this instanceof Long))
+ return new Long(low, high, unsigned);
+ if (typeof low === 'bigint') {
+ Object.assign(this, Long.fromBigInt(low, !!high));
+ }
+ else if (typeof low === 'string') {
+ Object.assign(this, Long.fromString(low, !!high));
+ }
+ else {
+ this.low = low | 0;
+ this.high = high | 0;
+ this.unsigned = !!unsigned;
+ }
+ Object.defineProperty(this, '__isLong__', {
+ value: true,
+ configurable: false,
+ writable: false,
+ enumerable: false
+ });
+ }
+ /**
+ * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits.
+ * Each is assumed to use 32 bits.
+ * @param lowBits - The low 32 bits
+ * @param highBits - The high 32 bits
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBits = function (lowBits, highBits, unsigned) {
+ return new Long(lowBits, highBits, unsigned);
+ };
+ /**
+ * Returns a Long representing the given 32 bit integer value.
+ * @param value - The 32 bit integer in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromInt = function (value, unsigned) {
+ var obj, cachedObj, cache;
+ if (unsigned) {
+ value >>>= 0;
+ if ((cache = 0 <= value && value < 256)) {
+ cachedObj = UINT_CACHE[value];
+ if (cachedObj)
+ return cachedObj;
+ }
+ obj = Long.fromBits(value, (value | 0) < 0 ? -1 : 0, true);
+ if (cache)
+ UINT_CACHE[value] = obj;
+ return obj;
+ }
+ else {
+ value |= 0;
+ if ((cache = -128 <= value && value < 128)) {
+ cachedObj = INT_CACHE[value];
+ if (cachedObj)
+ return cachedObj;
+ }
+ obj = Long.fromBits(value, value < 0 ? -1 : 0, false);
+ if (cache)
+ INT_CACHE[value] = obj;
+ return obj;
+ }
+ };
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromNumber = function (value, unsigned) {
+ if (isNaN(value))
+ return unsigned ? Long.UZERO : Long.ZERO;
+ if (unsigned) {
+ if (value < 0)
+ return Long.UZERO;
+ if (value >= TWO_PWR_64_DBL)
+ return Long.MAX_UNSIGNED_VALUE;
+ }
+ else {
+ if (value <= -TWO_PWR_63_DBL)
+ return Long.MIN_VALUE;
+ if (value + 1 >= TWO_PWR_63_DBL)
+ return Long.MAX_VALUE;
+ }
+ if (value < 0)
+ return Long.fromNumber(-value, unsigned).neg();
+ return Long.fromBits(value % TWO_PWR_32_DBL | 0, (value / TWO_PWR_32_DBL) | 0, unsigned);
+ };
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBigInt = function (value, unsigned) {
+ return Long.fromString(value.toString(), unsigned);
+ };
+ /**
+ * Returns a Long representation of the given string, written using the specified radix.
+ * @param str - The textual representation of the Long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param radix - The radix in which the text is written (2-36), defaults to 10
+ * @returns The corresponding Long value
+ */
+ Long.fromString = function (str, unsigned, radix) {
+ if (str.length === 0)
+ throw Error('empty string');
+ if (str === 'NaN' || str === 'Infinity' || str === '+Infinity' || str === '-Infinity')
+ return Long.ZERO;
+ if (typeof unsigned === 'number') {
+ // For goog.math.long compatibility
+ (radix = unsigned), (unsigned = false);
+ }
+ else {
+ unsigned = !!unsigned;
+ }
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix)
+ throw RangeError('radix');
+ var p;
+ if ((p = str.indexOf('-')) > 0)
+ throw Error('interior hyphen');
+ else if (p === 0) {
+ return Long.fromString(str.substring(1), unsigned, radix).neg();
+ }
+ // Do several (8) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ var radixToPower = Long.fromNumber(Math.pow(radix, 8));
+ var result = Long.ZERO;
+ for (var i = 0; i < str.length; i += 8) {
+ var size = Math.min(8, str.length - i), value = parseInt(str.substring(i, i + size), radix);
+ if (size < 8) {
+ var power = Long.fromNumber(Math.pow(radix, size));
+ result = result.mul(power).add(Long.fromNumber(value));
+ }
+ else {
+ result = result.mul(radixToPower);
+ result = result.add(Long.fromNumber(value));
+ }
+ }
+ result.unsigned = unsigned;
+ return result;
+ };
+ /**
+ * Creates a Long from its byte representation.
+ * @param bytes - Byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns The corresponding Long value
+ */
+ Long.fromBytes = function (bytes, unsigned, le) {
+ return le ? Long.fromBytesLE(bytes, unsigned) : Long.fromBytesBE(bytes, unsigned);
+ };
+ /**
+ * Creates a Long from its little endian byte representation.
+ * @param bytes - Little endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBytesLE = function (bytes, unsigned) {
+ return new Long(bytes[0] | (bytes[1] << 8) | (bytes[2] << 16) | (bytes[3] << 24), bytes[4] | (bytes[5] << 8) | (bytes[6] << 16) | (bytes[7] << 24), unsigned);
+ };
+ /**
+ * Creates a Long from its big endian byte representation.
+ * @param bytes - Big endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBytesBE = function (bytes, unsigned) {
+ return new Long((bytes[4] << 24) | (bytes[5] << 16) | (bytes[6] << 8) | bytes[7], (bytes[0] << 24) | (bytes[1] << 16) | (bytes[2] << 8) | bytes[3], unsigned);
+ };
+ /**
+ * Tests if the specified object is a Long.
+ */
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any, @typescript-eslint/explicit-module-boundary-types
+ Long.isLong = function (value) {
+ return utils.isObjectLike(value) && value['__isLong__'] === true;
+ };
+ /**
+ * Converts the specified value to a Long.
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ Long.fromValue = function (val, unsigned) {
+ if (typeof val === 'number')
+ return Long.fromNumber(val, unsigned);
+ if (typeof val === 'string')
+ return Long.fromString(val, unsigned);
+ // Throws for non-objects, converts non-instanceof Long:
+ return Long.fromBits(val.low, val.high, typeof unsigned === 'boolean' ? unsigned : val.unsigned);
+ };
+ /** Returns the sum of this and the specified Long. */
+ Long.prototype.add = function (addend) {
+ if (!Long.isLong(addend))
+ addend = Long.fromValue(addend);
+ // Divide each number into 4 chunks of 16 bits, and then sum the chunks.
+ var a48 = this.high >>> 16;
+ var a32 = this.high & 0xffff;
+ var a16 = this.low >>> 16;
+ var a00 = this.low & 0xffff;
+ var b48 = addend.high >>> 16;
+ var b32 = addend.high & 0xffff;
+ var b16 = addend.low >>> 16;
+ var b00 = addend.low & 0xffff;
+ var c48 = 0, c32 = 0, c16 = 0, c00 = 0;
+ c00 += a00 + b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 + b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 + b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 + b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ };
+ /**
+ * Returns the sum of this and the specified Long.
+ * @returns Sum
+ */
+ Long.prototype.and = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low & other.low, this.high & other.high, this.unsigned);
+ };
+ /**
+ * Compares this Long's value with the specified's.
+ * @returns 0 if they are the same, 1 if the this is greater and -1 if the given one is greater
+ */
+ Long.prototype.compare = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ if (this.eq(other))
+ return 0;
+ var thisNeg = this.isNegative(), otherNeg = other.isNegative();
+ if (thisNeg && !otherNeg)
+ return -1;
+ if (!thisNeg && otherNeg)
+ return 1;
+ // At this point the sign bits are the same
+ if (!this.unsigned)
+ return this.sub(other).isNegative() ? -1 : 1;
+ // Both are positive if at least one is unsigned
+ return other.high >>> 0 > this.high >>> 0 ||
+ (other.high === this.high && other.low >>> 0 > this.low >>> 0)
+ ? -1
+ : 1;
+ };
+ /** This is an alias of {@link Long.compare} */
+ Long.prototype.comp = function (other) {
+ return this.compare(other);
+ };
+ /**
+ * Returns this Long divided by the specified. The result is signed if this Long is signed or unsigned if this Long is unsigned.
+ * @returns Quotient
+ */
+ Long.prototype.divide = function (divisor) {
+ if (!Long.isLong(divisor))
+ divisor = Long.fromValue(divisor);
+ if (divisor.isZero())
+ throw Error('division by zero');
+ // use wasm support if present
+ if (wasm) {
+ // guard against signed division overflow: the largest
+ // negative number / -1 would be 1 larger than the largest
+ // positive number, due to two's complement.
+ if (!this.unsigned &&
+ this.high === -0x80000000 &&
+ divisor.low === -1 &&
+ divisor.high === -1) {
+ // be consistent with non-wasm code path
+ return this;
+ }
+ var low = (this.unsigned ? wasm.div_u : wasm.div_s)(this.low, this.high, divisor.low, divisor.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ if (this.isZero())
+ return this.unsigned ? Long.UZERO : Long.ZERO;
+ var approx, rem, res;
+ if (!this.unsigned) {
+ // This section is only relevant for signed longs and is derived from the
+ // closure library as a whole.
+ if (this.eq(Long.MIN_VALUE)) {
+ if (divisor.eq(Long.ONE) || divisor.eq(Long.NEG_ONE))
+ return Long.MIN_VALUE;
+ // recall that -MIN_VALUE == MIN_VALUE
+ else if (divisor.eq(Long.MIN_VALUE))
+ return Long.ONE;
+ else {
+ // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.
+ var halfThis = this.shr(1);
+ approx = halfThis.div(divisor).shl(1);
+ if (approx.eq(Long.ZERO)) {
+ return divisor.isNegative() ? Long.ONE : Long.NEG_ONE;
+ }
+ else {
+ rem = this.sub(divisor.mul(approx));
+ res = approx.add(rem.div(divisor));
+ return res;
+ }
+ }
+ }
+ else if (divisor.eq(Long.MIN_VALUE))
+ return this.unsigned ? Long.UZERO : Long.ZERO;
+ if (this.isNegative()) {
+ if (divisor.isNegative())
+ return this.neg().div(divisor.neg());
+ return this.neg().div(divisor).neg();
+ }
+ else if (divisor.isNegative())
+ return this.div(divisor.neg()).neg();
+ res = Long.ZERO;
+ }
+ else {
+ // The algorithm below has not been made for unsigned longs. It's therefore
+ // required to take special care of the MSB prior to running it.
+ if (!divisor.unsigned)
+ divisor = divisor.toUnsigned();
+ if (divisor.gt(this))
+ return Long.UZERO;
+ if (divisor.gt(this.shru(1)))
+ // 15 >>> 1 = 7 ; with divisor = 8 ; true
+ return Long.UONE;
+ res = Long.UZERO;
+ }
+ // Repeat the following until the remainder is less than other: find a
+ // floating-point that approximates remainder / other *from below*, add this
+ // into the result, and subtract it from the remainder. It is critical that
+ // the approximate value is less than or equal to the real value so that the
+ // remainder never becomes negative.
+ rem = this;
+ while (rem.gte(divisor)) {
+ // Approximate the result of division. This may be a little greater or
+ // smaller than the actual value.
+ approx = Math.max(1, Math.floor(rem.toNumber() / divisor.toNumber()));
+ // We will tweak the approximate result by changing it in the 48-th digit or
+ // the smallest non-fractional digit, whichever is larger.
+ var log2 = Math.ceil(Math.log(approx) / Math.LN2);
+ var delta = log2 <= 48 ? 1 : Math.pow(2, log2 - 48);
+ // Decrease the approximation until it is smaller than the remainder. Note
+ // that if it is too large, the product overflows and is negative.
+ var approxRes = Long.fromNumber(approx);
+ var approxRem = approxRes.mul(divisor);
+ while (approxRem.isNegative() || approxRem.gt(rem)) {
+ approx -= delta;
+ approxRes = Long.fromNumber(approx, this.unsigned);
+ approxRem = approxRes.mul(divisor);
+ }
+ // We know the answer can't be zero... and actually, zero would cause
+ // infinite recursion since we would make no progress.
+ if (approxRes.isZero())
+ approxRes = Long.ONE;
+ res = res.add(approxRes);
+ rem = rem.sub(approxRem);
+ }
+ return res;
+ };
+ /**This is an alias of {@link Long.divide} */
+ Long.prototype.div = function (divisor) {
+ return this.divide(divisor);
+ };
+ /**
+ * Tests if this Long's value equals the specified's.
+ * @param other - Other value
+ */
+ Long.prototype.equals = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ if (this.unsigned !== other.unsigned && this.high >>> 31 === 1 && other.high >>> 31 === 1)
+ return false;
+ return this.high === other.high && this.low === other.low;
+ };
+ /** This is an alias of {@link Long.equals} */
+ Long.prototype.eq = function (other) {
+ return this.equals(other);
+ };
+ /** Gets the high 32 bits as a signed integer. */
+ Long.prototype.getHighBits = function () {
+ return this.high;
+ };
+ /** Gets the high 32 bits as an unsigned integer. */
+ Long.prototype.getHighBitsUnsigned = function () {
+ return this.high >>> 0;
+ };
+ /** Gets the low 32 bits as a signed integer. */
+ Long.prototype.getLowBits = function () {
+ return this.low;
+ };
+ /** Gets the low 32 bits as an unsigned integer. */
+ Long.prototype.getLowBitsUnsigned = function () {
+ return this.low >>> 0;
+ };
+ /** Gets the number of bits needed to represent the absolute value of this Long. */
+ Long.prototype.getNumBitsAbs = function () {
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ return this.eq(Long.MIN_VALUE) ? 64 : this.neg().getNumBitsAbs();
+ }
+ var val = this.high !== 0 ? this.high : this.low;
+ var bit;
+ for (bit = 31; bit > 0; bit--)
+ if ((val & (1 << bit)) !== 0)
+ break;
+ return this.high !== 0 ? bit + 33 : bit + 1;
+ };
+ /** Tests if this Long's value is greater than the specified's. */
+ Long.prototype.greaterThan = function (other) {
+ return this.comp(other) > 0;
+ };
+ /** This is an alias of {@link Long.greaterThan} */
+ Long.prototype.gt = function (other) {
+ return this.greaterThan(other);
+ };
+ /** Tests if this Long's value is greater than or equal the specified's. */
+ Long.prototype.greaterThanOrEqual = function (other) {
+ return this.comp(other) >= 0;
+ };
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ Long.prototype.gte = function (other) {
+ return this.greaterThanOrEqual(other);
+ };
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ Long.prototype.ge = function (other) {
+ return this.greaterThanOrEqual(other);
+ };
+ /** Tests if this Long's value is even. */
+ Long.prototype.isEven = function () {
+ return (this.low & 1) === 0;
+ };
+ /** Tests if this Long's value is negative. */
+ Long.prototype.isNegative = function () {
+ return !this.unsigned && this.high < 0;
+ };
+ /** Tests if this Long's value is odd. */
+ Long.prototype.isOdd = function () {
+ return (this.low & 1) === 1;
+ };
+ /** Tests if this Long's value is positive. */
+ Long.prototype.isPositive = function () {
+ return this.unsigned || this.high >= 0;
+ };
+ /** Tests if this Long's value equals zero. */
+ Long.prototype.isZero = function () {
+ return this.high === 0 && this.low === 0;
+ };
+ /** Tests if this Long's value is less than the specified's. */
+ Long.prototype.lessThan = function (other) {
+ return this.comp(other) < 0;
+ };
+ /** This is an alias of {@link Long#lessThan}. */
+ Long.prototype.lt = function (other) {
+ return this.lessThan(other);
+ };
+ /** Tests if this Long's value is less than or equal the specified's. */
+ Long.prototype.lessThanOrEqual = function (other) {
+ return this.comp(other) <= 0;
+ };
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ Long.prototype.lte = function (other) {
+ return this.lessThanOrEqual(other);
+ };
+ /** Returns this Long modulo the specified. */
+ Long.prototype.modulo = function (divisor) {
+ if (!Long.isLong(divisor))
+ divisor = Long.fromValue(divisor);
+ // use wasm support if present
+ if (wasm) {
+ var low = (this.unsigned ? wasm.rem_u : wasm.rem_s)(this.low, this.high, divisor.low, divisor.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ return this.sub(this.div(divisor).mul(divisor));
+ };
+ /** This is an alias of {@link Long.modulo} */
+ Long.prototype.mod = function (divisor) {
+ return this.modulo(divisor);
+ };
+ /** This is an alias of {@link Long.modulo} */
+ Long.prototype.rem = function (divisor) {
+ return this.modulo(divisor);
+ };
+ /**
+ * Returns the product of this and the specified Long.
+ * @param multiplier - Multiplier
+ * @returns Product
+ */
+ Long.prototype.multiply = function (multiplier) {
+ if (this.isZero())
+ return Long.ZERO;
+ if (!Long.isLong(multiplier))
+ multiplier = Long.fromValue(multiplier);
+ // use wasm support if present
+ if (wasm) {
+ var low = wasm.mul(this.low, this.high, multiplier.low, multiplier.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ if (multiplier.isZero())
+ return Long.ZERO;
+ if (this.eq(Long.MIN_VALUE))
+ return multiplier.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (multiplier.eq(Long.MIN_VALUE))
+ return this.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (this.isNegative()) {
+ if (multiplier.isNegative())
+ return this.neg().mul(multiplier.neg());
+ else
+ return this.neg().mul(multiplier).neg();
+ }
+ else if (multiplier.isNegative())
+ return this.mul(multiplier.neg()).neg();
+ // If both longs are small, use float multiplication
+ if (this.lt(Long.TWO_PWR_24) && multiplier.lt(Long.TWO_PWR_24))
+ return Long.fromNumber(this.toNumber() * multiplier.toNumber(), this.unsigned);
+ // Divide each long into 4 chunks of 16 bits, and then add up 4x4 products.
+ // We can skip products that would overflow.
+ var a48 = this.high >>> 16;
+ var a32 = this.high & 0xffff;
+ var a16 = this.low >>> 16;
+ var a00 = this.low & 0xffff;
+ var b48 = multiplier.high >>> 16;
+ var b32 = multiplier.high & 0xffff;
+ var b16 = multiplier.low >>> 16;
+ var b00 = multiplier.low & 0xffff;
+ var c48 = 0, c32 = 0, c16 = 0, c00 = 0;
+ c00 += a00 * b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 * b00;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c16 += a00 * b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 * b00;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a16 * b16;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a00 * b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ };
+ /** This is an alias of {@link Long.multiply} */
+ Long.prototype.mul = function (multiplier) {
+ return this.multiply(multiplier);
+ };
+ /** Returns the Negation of this Long's value. */
+ Long.prototype.negate = function () {
+ if (!this.unsigned && this.eq(Long.MIN_VALUE))
+ return Long.MIN_VALUE;
+ return this.not().add(Long.ONE);
+ };
+ /** This is an alias of {@link Long.negate} */
+ Long.prototype.neg = function () {
+ return this.negate();
+ };
+ /** Returns the bitwise NOT of this Long. */
+ Long.prototype.not = function () {
+ return Long.fromBits(~this.low, ~this.high, this.unsigned);
+ };
+ /** Tests if this Long's value differs from the specified's. */
+ Long.prototype.notEquals = function (other) {
+ return !this.equals(other);
+ };
+ /** This is an alias of {@link Long.notEquals} */
+ Long.prototype.neq = function (other) {
+ return this.notEquals(other);
+ };
+ /** This is an alias of {@link Long.notEquals} */
+ Long.prototype.ne = function (other) {
+ return this.notEquals(other);
+ };
+ /**
+ * Returns the bitwise OR of this Long and the specified.
+ */
+ Long.prototype.or = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low | other.low, this.high | other.high, this.unsigned);
+ };
+ /**
+ * Returns this Long with bits shifted to the left by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftLeft = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ if ((numBits &= 63) === 0)
+ return this;
+ else if (numBits < 32)
+ return Long.fromBits(this.low << numBits, (this.high << numBits) | (this.low >>> (32 - numBits)), this.unsigned);
+ else
+ return Long.fromBits(0, this.low << (numBits - 32), this.unsigned);
+ };
+ /** This is an alias of {@link Long.shiftLeft} */
+ Long.prototype.shl = function (numBits) {
+ return this.shiftLeft(numBits);
+ };
+ /**
+ * Returns this Long with bits arithmetically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftRight = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ if ((numBits &= 63) === 0)
+ return this;
+ else if (numBits < 32)
+ return Long.fromBits((this.low >>> numBits) | (this.high << (32 - numBits)), this.high >> numBits, this.unsigned);
+ else
+ return Long.fromBits(this.high >> (numBits - 32), this.high >= 0 ? 0 : -1, this.unsigned);
+ };
+ /** This is an alias of {@link Long.shiftRight} */
+ Long.prototype.shr = function (numBits) {
+ return this.shiftRight(numBits);
+ };
+ /**
+ * Returns this Long with bits logically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftRightUnsigned = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ numBits &= 63;
+ if (numBits === 0)
+ return this;
+ else {
+ var high = this.high;
+ if (numBits < 32) {
+ var low = this.low;
+ return Long.fromBits((low >>> numBits) | (high << (32 - numBits)), high >>> numBits, this.unsigned);
+ }
+ else if (numBits === 32)
+ return Long.fromBits(high, 0, this.unsigned);
+ else
+ return Long.fromBits(high >>> (numBits - 32), 0, this.unsigned);
+ }
+ };
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ Long.prototype.shr_u = function (numBits) {
+ return this.shiftRightUnsigned(numBits);
+ };
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ Long.prototype.shru = function (numBits) {
+ return this.shiftRightUnsigned(numBits);
+ };
+ /**
+ * Returns the difference of this and the specified Long.
+ * @param subtrahend - Subtrahend
+ * @returns Difference
+ */
+ Long.prototype.subtract = function (subtrahend) {
+ if (!Long.isLong(subtrahend))
+ subtrahend = Long.fromValue(subtrahend);
+ return this.add(subtrahend.neg());
+ };
+ /** This is an alias of {@link Long.subtract} */
+ Long.prototype.sub = function (subtrahend) {
+ return this.subtract(subtrahend);
+ };
+ /** Converts the Long to a 32 bit integer, assuming it is a 32 bit integer. */
+ Long.prototype.toInt = function () {
+ return this.unsigned ? this.low >>> 0 : this.low;
+ };
+ /** Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa). */
+ Long.prototype.toNumber = function () {
+ if (this.unsigned)
+ return (this.high >>> 0) * TWO_PWR_32_DBL + (this.low >>> 0);
+ return this.high * TWO_PWR_32_DBL + (this.low >>> 0);
+ };
+ /** Converts the Long to a BigInt (arbitrary precision). */
+ Long.prototype.toBigInt = function () {
+ return BigInt(this.toString());
+ };
+ /**
+ * Converts this Long to its byte representation.
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns Byte representation
+ */
+ Long.prototype.toBytes = function (le) {
+ return le ? this.toBytesLE() : this.toBytesBE();
+ };
+ /**
+ * Converts this Long to its little endian byte representation.
+ * @returns Little endian byte representation
+ */
+ Long.prototype.toBytesLE = function () {
+ var hi = this.high, lo = this.low;
+ return [
+ lo & 0xff,
+ (lo >>> 8) & 0xff,
+ (lo >>> 16) & 0xff,
+ lo >>> 24,
+ hi & 0xff,
+ (hi >>> 8) & 0xff,
+ (hi >>> 16) & 0xff,
+ hi >>> 24
+ ];
+ };
+ /**
+ * Converts this Long to its big endian byte representation.
+ * @returns Big endian byte representation
+ */
+ Long.prototype.toBytesBE = function () {
+ var hi = this.high, lo = this.low;
+ return [
+ hi >>> 24,
+ (hi >>> 16) & 0xff,
+ (hi >>> 8) & 0xff,
+ hi & 0xff,
+ lo >>> 24,
+ (lo >>> 16) & 0xff,
+ (lo >>> 8) & 0xff,
+ lo & 0xff
+ ];
+ };
+ /**
+ * Converts this Long to signed.
+ */
+ Long.prototype.toSigned = function () {
+ if (!this.unsigned)
+ return this;
+ return Long.fromBits(this.low, this.high, false);
+ };
+ /**
+ * Converts the Long to a string written in the specified radix.
+ * @param radix - Radix (2-36), defaults to 10
+ * @throws RangeError If `radix` is out of range
+ */
+ Long.prototype.toString = function (radix) {
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix)
+ throw RangeError('radix');
+ if (this.isZero())
+ return '0';
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ if (this.eq(Long.MIN_VALUE)) {
+ // We need to change the Long value before it can be negated, so we remove
+ // the bottom-most digit in this base and then recurse to do the rest.
+ var radixLong = Long.fromNumber(radix), div = this.div(radixLong), rem1 = div.mul(radixLong).sub(this);
+ return div.toString(radix) + rem1.toInt().toString(radix);
+ }
+ else
+ return '-' + this.neg().toString(radix);
+ }
+ // Do several (6) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ var radixToPower = Long.fromNumber(Math.pow(radix, 6), this.unsigned);
+ // eslint-disable-next-line @typescript-eslint/no-this-alias
+ var rem = this;
+ var result = '';
+ // eslint-disable-next-line no-constant-condition
+ while (true) {
+ var remDiv = rem.div(radixToPower);
+ var intval = rem.sub(remDiv.mul(radixToPower)).toInt() >>> 0;
+ var digits = intval.toString(radix);
+ rem = remDiv;
+ if (rem.isZero()) {
+ return digits + result;
+ }
+ else {
+ while (digits.length < 6)
+ digits = '0' + digits;
+ result = '' + digits + result;
+ }
+ }
+ };
+ /** Converts this Long to unsigned. */
+ Long.prototype.toUnsigned = function () {
+ if (this.unsigned)
+ return this;
+ return Long.fromBits(this.low, this.high, true);
+ };
+ /** Returns the bitwise XOR of this Long and the given one. */
+ Long.prototype.xor = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low ^ other.low, this.high ^ other.high, this.unsigned);
+ };
+ /** This is an alias of {@link Long.isZero} */
+ Long.prototype.eqz = function () {
+ return this.isZero();
+ };
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ Long.prototype.le = function (other) {
+ return this.lessThanOrEqual(other);
+ };
+ /*
+ ****************************************************************
+ * BSON SPECIFIC ADDITIONS *
+ ****************************************************************
+ */
+ Long.prototype.toExtendedJSON = function (options) {
+ if (options && options.relaxed)
+ return this.toNumber();
+ return { $numberLong: this.toString() };
+ };
+ Long.fromExtendedJSON = function (doc, options) {
+ var result = Long.fromString(doc.$numberLong);
+ return options && options.relaxed ? result.toNumber() : result;
+ };
+ /** @internal */
+ Long.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Long.prototype.inspect = function () {
+ return "new Long(\"" + this.toString() + "\"" + (this.unsigned ? ', true' : '') + ")";
+ };
+ Long.TWO_PWR_24 = Long.fromInt(TWO_PWR_24_DBL);
+ /** Maximum unsigned value. */
+ Long.MAX_UNSIGNED_VALUE = Long.fromBits(0xffffffff | 0, 0xffffffff | 0, true);
+ /** Signed zero */
+ Long.ZERO = Long.fromInt(0);
+ /** Unsigned zero. */
+ Long.UZERO = Long.fromInt(0, true);
+ /** Signed one. */
+ Long.ONE = Long.fromInt(1);
+ /** Unsigned one. */
+ Long.UONE = Long.fromInt(1, true);
+ /** Signed negative one. */
+ Long.NEG_ONE = Long.fromInt(-1);
+ /** Maximum signed value. */
+ Long.MAX_VALUE = Long.fromBits(0xffffffff | 0, 0x7fffffff | 0, false);
+ /** Minimum signed value. */
+ Long.MIN_VALUE = Long.fromBits(0, 0x80000000 | 0, false);
+ return Long;
+ }());
+ exports.Long = Long;
+ Object.defineProperty(Long.prototype, '__isLong__', { value: true });
+ Object.defineProperty(Long.prototype, '_bsontype', { value: 'Long' });
+
+ });
+
+ unwrapExports(long_1);
+ long_1.Long;
+
+ var decimal128 = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Decimal128 = void 0;
+
+
+ var PARSE_STRING_REGEXP = /^(\+|-)?(\d+|(\d*\.\d*))?(E|e)?([-+])?(\d+)?$/;
+ var PARSE_INF_REGEXP = /^(\+|-)?(Infinity|inf)$/i;
+ var PARSE_NAN_REGEXP = /^(\+|-)?NaN$/i;
+ var EXPONENT_MAX = 6111;
+ var EXPONENT_MIN = -6176;
+ var EXPONENT_BIAS = 6176;
+ var MAX_DIGITS = 34;
+ // Nan value bits as 32 bit values (due to lack of longs)
+ var NAN_BUFFER = [
+ 0x7c,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+ ].reverse();
+ // Infinity value bits 32 bit values (due to lack of longs)
+ var INF_NEGATIVE_BUFFER = [
+ 0xf8,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+ ].reverse();
+ var INF_POSITIVE_BUFFER = [
+ 0x78,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+ ].reverse();
+ var EXPONENT_REGEX = /^([-+])?(\d+)?$/;
+ // Extract least significant 5 bits
+ var COMBINATION_MASK = 0x1f;
+ // Extract least significant 14 bits
+ var EXPONENT_MASK = 0x3fff;
+ // Value of combination field for Inf
+ var COMBINATION_INFINITY = 30;
+ // Value of combination field for NaN
+ var COMBINATION_NAN = 31;
+ // Detect if the value is a digit
+ function isDigit(value) {
+ return !isNaN(parseInt(value, 10));
+ }
+ // Divide two uint128 values
+ function divideu128(value) {
+ var DIVISOR = long_1.Long.fromNumber(1000 * 1000 * 1000);
+ var _rem = long_1.Long.fromNumber(0);
+ if (!value.parts[0] && !value.parts[1] && !value.parts[2] && !value.parts[3]) {
+ return { quotient: value, rem: _rem };
+ }
+ for (var i = 0; i <= 3; i++) {
+ // Adjust remainder to match value of next dividend
+ _rem = _rem.shiftLeft(32);
+ // Add the divided to _rem
+ _rem = _rem.add(new long_1.Long(value.parts[i], 0));
+ value.parts[i] = _rem.div(DIVISOR).low;
+ _rem = _rem.modulo(DIVISOR);
+ }
+ return { quotient: value, rem: _rem };
+ }
+ // Multiply two Long values and return the 128 bit value
+ function multiply64x2(left, right) {
+ if (!left && !right) {
+ return { high: long_1.Long.fromNumber(0), low: long_1.Long.fromNumber(0) };
+ }
+ var leftHigh = left.shiftRightUnsigned(32);
+ var leftLow = new long_1.Long(left.getLowBits(), 0);
+ var rightHigh = right.shiftRightUnsigned(32);
+ var rightLow = new long_1.Long(right.getLowBits(), 0);
+ var productHigh = leftHigh.multiply(rightHigh);
+ var productMid = leftHigh.multiply(rightLow);
+ var productMid2 = leftLow.multiply(rightHigh);
+ var productLow = leftLow.multiply(rightLow);
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productMid = new long_1.Long(productMid.getLowBits(), 0)
+ .add(productMid2)
+ .add(productLow.shiftRightUnsigned(32));
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productLow = productMid.shiftLeft(32).add(new long_1.Long(productLow.getLowBits(), 0));
+ // Return the 128 bit result
+ return { high: productHigh, low: productLow };
+ }
+ function lessThan(left, right) {
+ // Make values unsigned
+ var uhleft = left.high >>> 0;
+ var uhright = right.high >>> 0;
+ // Compare high bits first
+ if (uhleft < uhright) {
+ return true;
+ }
+ else if (uhleft === uhright) {
+ var ulleft = left.low >>> 0;
+ var ulright = right.low >>> 0;
+ if (ulleft < ulright)
+ return true;
+ }
+ return false;
+ }
+ function invalidErr(string, message) {
+ throw new TypeError("\"" + string + "\" is not a valid Decimal128 string - " + message);
+ }
+ /**
+ * A class representation of the BSON Decimal128 type.
+ * @public
+ */
+ var Decimal128 = /** @class */ (function () {
+ /**
+ * @param bytes - a buffer containing the raw Decimal128 bytes in little endian order,
+ * or a string representation as returned by .toString()
+ */
+ function Decimal128(bytes) {
+ if (!(this instanceof Decimal128))
+ return new Decimal128(bytes);
+ if (typeof bytes === 'string') {
+ this.bytes = Decimal128.fromString(bytes).bytes;
+ }
+ else {
+ this.bytes = bytes;
+ }
+ }
+ /**
+ * Create a Decimal128 instance from a string representation
+ *
+ * @param representation - a numeric string representation.
+ */
+ Decimal128.fromString = function (representation) {
+ // Parse state tracking
+ var isNegative = false;
+ var sawRadix = false;
+ var foundNonZero = false;
+ // Total number of significant digits (no leading or trailing zero)
+ var significantDigits = 0;
+ // Total number of significand digits read
+ var nDigitsRead = 0;
+ // Total number of digits (no leading zeros)
+ var nDigits = 0;
+ // The number of the digits after radix
+ var radixPosition = 0;
+ // The index of the first non-zero in *str*
+ var firstNonZero = 0;
+ // Digits Array
+ var digits = [0];
+ // The number of digits in digits
+ var nDigitsStored = 0;
+ // Insertion pointer for digits
+ var digitsInsert = 0;
+ // The index of the first non-zero digit
+ var firstDigit = 0;
+ // The index of the last digit
+ var lastDigit = 0;
+ // Exponent
+ var exponent = 0;
+ // loop index over array
+ var i = 0;
+ // The high 17 digits of the significand
+ var significandHigh = new long_1.Long(0, 0);
+ // The low 17 digits of the significand
+ var significandLow = new long_1.Long(0, 0);
+ // The biased exponent
+ var biasedExponent = 0;
+ // Read index
+ var index = 0;
+ // Naively prevent against REDOS attacks.
+ // TODO: implementing a custom parsing for this, or refactoring the regex would yield
+ // further gains.
+ if (representation.length >= 7000) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+ // Results
+ var stringMatch = representation.match(PARSE_STRING_REGEXP);
+ var infMatch = representation.match(PARSE_INF_REGEXP);
+ var nanMatch = representation.match(PARSE_NAN_REGEXP);
+ // Validate the string
+ if ((!stringMatch && !infMatch && !nanMatch) || representation.length === 0) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+ if (stringMatch) {
+ // full_match = stringMatch[0]
+ // sign = stringMatch[1]
+ var unsignedNumber = stringMatch[2];
+ // stringMatch[3] is undefined if a whole number (ex "1", 12")
+ // but defined if a number w/ decimal in it (ex "1.0, 12.2")
+ var e = stringMatch[4];
+ var expSign = stringMatch[5];
+ var expNumber = stringMatch[6];
+ // they provided e, but didn't give an exponent number. for ex "1e"
+ if (e && expNumber === undefined)
+ invalidErr(representation, 'missing exponent power');
+ // they provided e, but didn't give a number before it. for ex "e1"
+ if (e && unsignedNumber === undefined)
+ invalidErr(representation, 'missing exponent base');
+ if (e === undefined && (expSign || expNumber)) {
+ invalidErr(representation, 'missing e before exponent');
+ }
+ }
+ // Get the negative or positive sign
+ if (representation[index] === '+' || representation[index] === '-') {
+ isNegative = representation[index++] === '-';
+ }
+ // Check if user passed Infinity or NaN
+ if (!isDigit(representation[index]) && representation[index] !== '.') {
+ if (representation[index] === 'i' || representation[index] === 'I') {
+ return new Decimal128(buffer.Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ }
+ else if (representation[index] === 'N') {
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ }
+ }
+ // Read all the digits
+ while (isDigit(representation[index]) || representation[index] === '.') {
+ if (representation[index] === '.') {
+ if (sawRadix)
+ invalidErr(representation, 'contains multiple periods');
+ sawRadix = true;
+ index = index + 1;
+ continue;
+ }
+ if (nDigitsStored < 34) {
+ if (representation[index] !== '0' || foundNonZero) {
+ if (!foundNonZero) {
+ firstNonZero = nDigitsRead;
+ }
+ foundNonZero = true;
+ // Only store 34 digits
+ digits[digitsInsert++] = parseInt(representation[index], 10);
+ nDigitsStored = nDigitsStored + 1;
+ }
+ }
+ if (foundNonZero)
+ nDigits = nDigits + 1;
+ if (sawRadix)
+ radixPosition = radixPosition + 1;
+ nDigitsRead = nDigitsRead + 1;
+ index = index + 1;
+ }
+ if (sawRadix && !nDigitsRead)
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ // Read exponent if exists
+ if (representation[index] === 'e' || representation[index] === 'E') {
+ // Read exponent digits
+ var match = representation.substr(++index).match(EXPONENT_REGEX);
+ // No digits read
+ if (!match || !match[2])
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ // Get exponent
+ exponent = parseInt(match[0], 10);
+ // Adjust the index
+ index = index + match[0].length;
+ }
+ // Return not a number
+ if (representation[index])
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ // Done reading input
+ // Find first non-zero digit in digits
+ firstDigit = 0;
+ if (!nDigitsStored) {
+ firstDigit = 0;
+ lastDigit = 0;
+ digits[0] = 0;
+ nDigits = 1;
+ nDigitsStored = 1;
+ significantDigits = 0;
+ }
+ else {
+ lastDigit = nDigitsStored - 1;
+ significantDigits = nDigits;
+ if (significantDigits !== 1) {
+ while (representation[firstNonZero + significantDigits - 1] === '0') {
+ significantDigits = significantDigits - 1;
+ }
+ }
+ }
+ // Normalization of exponent
+ // Correct exponent based on radix position, and shift significand as needed
+ // to represent user input
+ // Overflow prevention
+ if (exponent <= radixPosition && radixPosition - exponent > 1 << 14) {
+ exponent = EXPONENT_MIN;
+ }
+ else {
+ exponent = exponent - radixPosition;
+ }
+ // Attempt to normalize the exponent
+ while (exponent > EXPONENT_MAX) {
+ // Shift exponent to significand and decrease
+ lastDigit = lastDigit + 1;
+ if (lastDigit - firstDigit > MAX_DIGITS) {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ var digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ exponent = exponent - 1;
+ }
+ while (exponent < EXPONENT_MIN || nDigitsStored < nDigits) {
+ // Shift last digit. can only do this if < significant digits than # stored.
+ if (lastDigit === 0 && significantDigits < nDigitsStored) {
+ exponent = EXPONENT_MIN;
+ significantDigits = 0;
+ break;
+ }
+ if (nDigitsStored < nDigits) {
+ // adjust to match digits not stored
+ nDigits = nDigits - 1;
+ }
+ else {
+ // adjust to round
+ lastDigit = lastDigit - 1;
+ }
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ }
+ else {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ var digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ }
+ // Round
+ // We've normalized the exponent, but might still need to round.
+ if (lastDigit - firstDigit + 1 < significantDigits) {
+ var endOfString = nDigitsRead;
+ // If we have seen a radix point, 'string' is 1 longer than we have
+ // documented with ndigits_read, so inc the position of the first nonzero
+ // digit and the position that digits are read to.
+ if (sawRadix) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ // if negative, we need to increment again to account for - sign at start.
+ if (isNegative) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ var roundDigit = parseInt(representation[firstNonZero + lastDigit + 1], 10);
+ var roundBit = 0;
+ if (roundDigit >= 5) {
+ roundBit = 1;
+ if (roundDigit === 5) {
+ roundBit = digits[lastDigit] % 2 === 1 ? 1 : 0;
+ for (i = firstNonZero + lastDigit + 2; i < endOfString; i++) {
+ if (parseInt(representation[i], 10)) {
+ roundBit = 1;
+ break;
+ }
+ }
+ }
+ }
+ if (roundBit) {
+ var dIdx = lastDigit;
+ for (; dIdx >= 0; dIdx--) {
+ if (++digits[dIdx] > 9) {
+ digits[dIdx] = 0;
+ // overflowed most significant digit
+ if (dIdx === 0) {
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ digits[dIdx] = 1;
+ }
+ else {
+ return new Decimal128(buffer.Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ }
+ }
+ }
+ }
+ }
+ }
+ // Encode significand
+ // The high 17 digits of the significand
+ significandHigh = long_1.Long.fromNumber(0);
+ // The low 17 digits of the significand
+ significandLow = long_1.Long.fromNumber(0);
+ // read a zero
+ if (significantDigits === 0) {
+ significandHigh = long_1.Long.fromNumber(0);
+ significandLow = long_1.Long.fromNumber(0);
+ }
+ else if (lastDigit - firstDigit < 17) {
+ var dIdx = firstDigit;
+ significandLow = long_1.Long.fromNumber(digits[dIdx++]);
+ significandHigh = new long_1.Long(0, 0);
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(long_1.Long.fromNumber(10));
+ significandLow = significandLow.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ }
+ else {
+ var dIdx = firstDigit;
+ significandHigh = long_1.Long.fromNumber(digits[dIdx++]);
+ for (; dIdx <= lastDigit - 17; dIdx++) {
+ significandHigh = significandHigh.multiply(long_1.Long.fromNumber(10));
+ significandHigh = significandHigh.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ significandLow = long_1.Long.fromNumber(digits[dIdx++]);
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(long_1.Long.fromNumber(10));
+ significandLow = significandLow.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ }
+ var significand = multiply64x2(significandHigh, long_1.Long.fromString('100000000000000000'));
+ significand.low = significand.low.add(significandLow);
+ if (lessThan(significand.low, significandLow)) {
+ significand.high = significand.high.add(long_1.Long.fromNumber(1));
+ }
+ // Biased exponent
+ biasedExponent = exponent + EXPONENT_BIAS;
+ var dec = { low: long_1.Long.fromNumber(0), high: long_1.Long.fromNumber(0) };
+ // Encode combination, exponent, and significand.
+ if (significand.high.shiftRightUnsigned(49).and(long_1.Long.fromNumber(1)).equals(long_1.Long.fromNumber(1))) {
+ // Encode '11' into bits 1 to 3
+ dec.high = dec.high.or(long_1.Long.fromNumber(0x3).shiftLeft(61));
+ dec.high = dec.high.or(long_1.Long.fromNumber(biasedExponent).and(long_1.Long.fromNumber(0x3fff).shiftLeft(47)));
+ dec.high = dec.high.or(significand.high.and(long_1.Long.fromNumber(0x7fffffffffff)));
+ }
+ else {
+ dec.high = dec.high.or(long_1.Long.fromNumber(biasedExponent & 0x3fff).shiftLeft(49));
+ dec.high = dec.high.or(significand.high.and(long_1.Long.fromNumber(0x1ffffffffffff)));
+ }
+ dec.low = significand.low;
+ // Encode sign
+ if (isNegative) {
+ dec.high = dec.high.or(long_1.Long.fromString('9223372036854775808'));
+ }
+ // Encode into a buffer
+ var buffer$1 = buffer.Buffer.alloc(16);
+ index = 0;
+ // Encode the low 64 bits of the decimal
+ // Encode low bits
+ buffer$1[index++] = dec.low.low & 0xff;
+ buffer$1[index++] = (dec.low.low >> 8) & 0xff;
+ buffer$1[index++] = (dec.low.low >> 16) & 0xff;
+ buffer$1[index++] = (dec.low.low >> 24) & 0xff;
+ // Encode high bits
+ buffer$1[index++] = dec.low.high & 0xff;
+ buffer$1[index++] = (dec.low.high >> 8) & 0xff;
+ buffer$1[index++] = (dec.low.high >> 16) & 0xff;
+ buffer$1[index++] = (dec.low.high >> 24) & 0xff;
+ // Encode the high 64 bits of the decimal
+ // Encode low bits
+ buffer$1[index++] = dec.high.low & 0xff;
+ buffer$1[index++] = (dec.high.low >> 8) & 0xff;
+ buffer$1[index++] = (dec.high.low >> 16) & 0xff;
+ buffer$1[index++] = (dec.high.low >> 24) & 0xff;
+ // Encode high bits
+ buffer$1[index++] = dec.high.high & 0xff;
+ buffer$1[index++] = (dec.high.high >> 8) & 0xff;
+ buffer$1[index++] = (dec.high.high >> 16) & 0xff;
+ buffer$1[index++] = (dec.high.high >> 24) & 0xff;
+ // Return the new Decimal128
+ return new Decimal128(buffer$1);
+ };
+ /** Create a string representation of the raw Decimal128 value */
+ Decimal128.prototype.toString = function () {
+ // Note: bits in this routine are referred to starting at 0,
+ // from the sign bit, towards the coefficient.
+ // decoded biased exponent (14 bits)
+ var biased_exponent;
+ // the number of significand digits
+ var significand_digits = 0;
+ // the base-10 digits in the significand
+ var significand = new Array(36);
+ for (var i = 0; i < significand.length; i++)
+ significand[i] = 0;
+ // read pointer into significand
+ var index = 0;
+ // true if the number is zero
+ var is_zero = false;
+ // the most significant significand bits (50-46)
+ var significand_msb;
+ // temporary storage for significand decoding
+ var significand128 = { parts: [0, 0, 0, 0] };
+ // indexing variables
+ var j, k;
+ // Output string
+ var string = [];
+ // Unpack index
+ index = 0;
+ // Buffer reference
+ var buffer = this.bytes;
+ // Unpack the low 64bits into a long
+ // bits 96 - 127
+ var low = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 64 - 95
+ var midl = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Unpack the high 64bits into a long
+ // bits 32 - 63
+ var midh = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 0 - 31
+ var high = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Unpack index
+ index = 0;
+ // Create the state of the decimal
+ var dec = {
+ low: new long_1.Long(low, midl),
+ high: new long_1.Long(midh, high)
+ };
+ if (dec.high.lessThan(long_1.Long.ZERO)) {
+ string.push('-');
+ }
+ // Decode combination field and exponent
+ // bits 1 - 5
+ var combination = (high >> 26) & COMBINATION_MASK;
+ if (combination >> 3 === 3) {
+ // Check for 'special' values
+ if (combination === COMBINATION_INFINITY) {
+ return string.join('') + 'Infinity';
+ }
+ else if (combination === COMBINATION_NAN) {
+ return 'NaN';
+ }
+ else {
+ biased_exponent = (high >> 15) & EXPONENT_MASK;
+ significand_msb = 0x08 + ((high >> 14) & 0x01);
+ }
+ }
+ else {
+ significand_msb = (high >> 14) & 0x07;
+ biased_exponent = (high >> 17) & EXPONENT_MASK;
+ }
+ // unbiased exponent
+ var exponent = biased_exponent - EXPONENT_BIAS;
+ // Create string of significand digits
+ // Convert the 114-bit binary number represented by
+ // (significand_high, significand_low) to at most 34 decimal
+ // digits through modulo and division.
+ significand128.parts[0] = (high & 0x3fff) + ((significand_msb & 0xf) << 14);
+ significand128.parts[1] = midh;
+ significand128.parts[2] = midl;
+ significand128.parts[3] = low;
+ if (significand128.parts[0] === 0 &&
+ significand128.parts[1] === 0 &&
+ significand128.parts[2] === 0 &&
+ significand128.parts[3] === 0) {
+ is_zero = true;
+ }
+ else {
+ for (k = 3; k >= 0; k--) {
+ var least_digits = 0;
+ // Perform the divide
+ var result = divideu128(significand128);
+ significand128 = result.quotient;
+ least_digits = result.rem.low;
+ // We now have the 9 least significant digits (in base 2).
+ // Convert and output to string.
+ if (!least_digits)
+ continue;
+ for (j = 8; j >= 0; j--) {
+ // significand[k * 9 + j] = Math.round(least_digits % 10);
+ significand[k * 9 + j] = least_digits % 10;
+ // least_digits = Math.round(least_digits / 10);
+ least_digits = Math.floor(least_digits / 10);
+ }
+ }
+ }
+ // Output format options:
+ // Scientific - [-]d.dddE(+/-)dd or [-]dE(+/-)dd
+ // Regular - ddd.ddd
+ if (is_zero) {
+ significand_digits = 1;
+ significand[index] = 0;
+ }
+ else {
+ significand_digits = 36;
+ while (!significand[index]) {
+ significand_digits = significand_digits - 1;
+ index = index + 1;
+ }
+ }
+ // the exponent if scientific notation is used
+ var scientific_exponent = significand_digits - 1 + exponent;
+ // The scientific exponent checks are dictated by the string conversion
+ // specification and are somewhat arbitrary cutoffs.
+ //
+ // We must check exponent > 0, because if this is the case, the number
+ // has trailing zeros. However, we *cannot* output these trailing zeros,
+ // because doing so would change the precision of the value, and would
+ // change stored data if the string converted number is round tripped.
+ if (scientific_exponent >= 34 || scientific_exponent <= -7 || exponent > 0) {
+ // Scientific format
+ // if there are too many significant digits, we should just be treating numbers
+ // as + or - 0 and using the non-scientific exponent (this is for the "invalid
+ // representation should be treated as 0/-0" spec cases in decimal128-1.json)
+ if (significand_digits > 34) {
+ string.push("" + 0);
+ if (exponent > 0)
+ string.push('E+' + exponent);
+ else if (exponent < 0)
+ string.push('E' + exponent);
+ return string.join('');
+ }
+ string.push("" + significand[index++]);
+ significand_digits = significand_digits - 1;
+ if (significand_digits) {
+ string.push('.');
+ }
+ for (var i = 0; i < significand_digits; i++) {
+ string.push("" + significand[index++]);
+ }
+ // Exponent
+ string.push('E');
+ if (scientific_exponent > 0) {
+ string.push('+' + scientific_exponent);
+ }
+ else {
+ string.push("" + scientific_exponent);
+ }
+ }
+ else {
+ // Regular format with no decimal place
+ if (exponent >= 0) {
+ for (var i = 0; i < significand_digits; i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ else {
+ var radix_position = significand_digits + exponent;
+ // non-zero digits before radix
+ if (radix_position > 0) {
+ for (var i = 0; i < radix_position; i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ else {
+ string.push('0');
+ }
+ string.push('.');
+ // add leading zeros after radix
+ while (radix_position++ < 0) {
+ string.push('0');
+ }
+ for (var i = 0; i < significand_digits - Math.max(radix_position - 1, 0); i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ }
+ return string.join('');
+ };
+ Decimal128.prototype.toJSON = function () {
+ return { $numberDecimal: this.toString() };
+ };
+ /** @internal */
+ Decimal128.prototype.toExtendedJSON = function () {
+ return { $numberDecimal: this.toString() };
+ };
+ /** @internal */
+ Decimal128.fromExtendedJSON = function (doc) {
+ return Decimal128.fromString(doc.$numberDecimal);
+ };
+ /** @internal */
+ Decimal128.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Decimal128.prototype.inspect = function () {
+ return "new Decimal128(\"" + this.toString() + "\")";
+ };
+ return Decimal128;
+ }());
+ exports.Decimal128 = Decimal128;
+ Object.defineProperty(Decimal128.prototype, '_bsontype', { value: 'Decimal128' });
+
+ });
+
+ unwrapExports(decimal128);
+ decimal128.Decimal128;
+
+ var double_1 = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Double = void 0;
+ /**
+ * A class representation of the BSON Double type.
+ * @public
+ */
+ var Double = /** @class */ (function () {
+ /**
+ * Create a Double type
+ *
+ * @param value - the number we want to represent as a double.
+ */
+ function Double(value) {
+ if (!(this instanceof Double))
+ return new Double(value);
+ if (value instanceof Number) {
+ value = value.valueOf();
+ }
+ this.value = +value;
+ }
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped double number.
+ */
+ Double.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ Double.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ Double.prototype.toExtendedJSON = function (options) {
+ if (options && (options.legacy || (options.relaxed && isFinite(this.value)))) {
+ return this.value;
+ }
+ // NOTE: JavaScript has +0 and -0, apparently to model limit calculations. If a user
+ // explicitly provided `-0` then we need to ensure the sign makes it into the output
+ if (Object.is(Math.sign(this.value), -0)) {
+ return { $numberDouble: "-" + this.value.toFixed(1) };
+ }
+ var $numberDouble;
+ if (Number.isInteger(this.value)) {
+ $numberDouble = this.value.toFixed(1);
+ if ($numberDouble.length >= 13) {
+ $numberDouble = this.value.toExponential(13).toUpperCase();
+ }
+ }
+ else {
+ $numberDouble = this.value.toString();
+ }
+ return { $numberDouble: $numberDouble };
+ };
+ /** @internal */
+ Double.fromExtendedJSON = function (doc, options) {
+ var doubleValue = parseFloat(doc.$numberDouble);
+ return options && options.relaxed ? doubleValue : new Double(doubleValue);
+ };
+ /** @internal */
+ Double.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Double.prototype.inspect = function () {
+ var eJSON = this.toExtendedJSON();
+ return "new Double(" + eJSON.$numberDouble + ")";
+ };
+ return Double;
+ }());
+ exports.Double = Double;
+ Object.defineProperty(Double.prototype, '_bsontype', { value: 'Double' });
+
+ });
+
+ unwrapExports(double_1);
+ double_1.Double;
+
+ var int_32 = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Int32 = void 0;
+ /**
+ * A class representation of a BSON Int32 type.
+ * @public
+ */
+ var Int32 = /** @class */ (function () {
+ /**
+ * Create an Int32 type
+ *
+ * @param value - the number we want to represent as an int32.
+ */
+ function Int32(value) {
+ if (!(this instanceof Int32))
+ return new Int32(value);
+ if (value instanceof Number) {
+ value = value.valueOf();
+ }
+ this.value = +value;
+ }
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped int32 number.
+ */
+ Int32.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ Int32.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ Int32.prototype.toExtendedJSON = function (options) {
+ if (options && (options.relaxed || options.legacy))
+ return this.value;
+ return { $numberInt: this.value.toString() };
+ };
+ /** @internal */
+ Int32.fromExtendedJSON = function (doc, options) {
+ return options && options.relaxed ? parseInt(doc.$numberInt, 10) : new Int32(doc.$numberInt);
+ };
+ /** @internal */
+ Int32.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Int32.prototype.inspect = function () {
+ return "new Int32(" + this.valueOf() + ")";
+ };
+ return Int32;
+ }());
+ exports.Int32 = Int32;
+ Object.defineProperty(Int32.prototype, '_bsontype', { value: 'Int32' });
+
+ });
+
+ unwrapExports(int_32);
+ int_32.Int32;
+
+ var max_key = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.MaxKey = void 0;
+ /**
+ * A class representation of the BSON MaxKey type.
+ * @public
+ */
+ var MaxKey = /** @class */ (function () {
+ function MaxKey() {
+ if (!(this instanceof MaxKey))
+ return new MaxKey();
+ }
+ /** @internal */
+ MaxKey.prototype.toExtendedJSON = function () {
+ return { $maxKey: 1 };
+ };
+ /** @internal */
+ MaxKey.fromExtendedJSON = function () {
+ return new MaxKey();
+ };
+ /** @internal */
+ MaxKey.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ MaxKey.prototype.inspect = function () {
+ return 'new MaxKey()';
+ };
+ return MaxKey;
+ }());
+ exports.MaxKey = MaxKey;
+ Object.defineProperty(MaxKey.prototype, '_bsontype', { value: 'MaxKey' });
+
+ });
+
+ unwrapExports(max_key);
+ max_key.MaxKey;
+
+ var min_key = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.MinKey = void 0;
+ /**
+ * A class representation of the BSON MinKey type.
+ * @public
+ */
+ var MinKey = /** @class */ (function () {
+ function MinKey() {
+ if (!(this instanceof MinKey))
+ return new MinKey();
+ }
+ /** @internal */
+ MinKey.prototype.toExtendedJSON = function () {
+ return { $minKey: 1 };
+ };
+ /** @internal */
+ MinKey.fromExtendedJSON = function () {
+ return new MinKey();
+ };
+ /** @internal */
+ MinKey.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ MinKey.prototype.inspect = function () {
+ return 'new MinKey()';
+ };
+ return MinKey;
+ }());
+ exports.MinKey = MinKey;
+ Object.defineProperty(MinKey.prototype, '_bsontype', { value: 'MinKey' });
+
+ });
+
+ unwrapExports(min_key);
+ min_key.MinKey;
+
+ var objectid = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.ObjectId = void 0;
+
+
+
+ // Regular expression that checks for hex value
+ var checkForHexRegExp = new RegExp('^[0-9a-fA-F]{24}$');
+ // Unique sequence for the current process (initialized on first use)
+ var PROCESS_UNIQUE = null;
+ var kId = Symbol('id');
+ /**
+ * A class representation of the BSON ObjectId type.
+ * @public
+ */
+ var ObjectId = /** @class */ (function () {
+ /**
+ * Create an ObjectId type
+ *
+ * @param id - Can be a 24 character hex string, 12 byte binary Buffer, or a number.
+ */
+ function ObjectId(id) {
+ if (!(this instanceof ObjectId))
+ return new ObjectId(id);
+ // Duck-typing to support ObjectId from different npm packages
+ if (id instanceof ObjectId) {
+ this[kId] = id.id;
+ this.__id = id.__id;
+ }
+ if (typeof id === 'object' && id && 'id' in id) {
+ if ('toHexString' in id && typeof id.toHexString === 'function') {
+ this[kId] = buffer.Buffer.from(id.toHexString(), 'hex');
+ }
+ else {
+ this[kId] = typeof id.id === 'string' ? buffer.Buffer.from(id.id) : id.id;
+ }
+ }
+ // The most common use case (blank id, new objectId instance)
+ if (id == null || typeof id === 'number') {
+ // Generate a new id
+ this[kId] = ObjectId.generate(typeof id === 'number' ? id : undefined);
+ // If we are caching the hex string
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+ if (ArrayBuffer.isView(id) && id.byteLength === 12) {
+ this[kId] = ensure_buffer.ensureBuffer(id);
+ }
+ if (typeof id === 'string') {
+ if (id.length === 12) {
+ var bytes = buffer.Buffer.from(id);
+ if (bytes.byteLength === 12) {
+ this[kId] = bytes;
+ }
+ }
+ else if (id.length === 24 && checkForHexRegExp.test(id)) {
+ this[kId] = buffer.Buffer.from(id, 'hex');
+ }
+ else {
+ throw new TypeError('Argument passed in must be a Buffer or string of 12 bytes or a string of 24 hex characters');
+ }
+ }
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+ Object.defineProperty(ObjectId.prototype, "id", {
+ /**
+ * The ObjectId bytes
+ * @readonly
+ */
+ get: function () {
+ return this[kId];
+ },
+ set: function (value) {
+ this[kId] = value;
+ if (ObjectId.cacheHexString) {
+ this.__id = value.toString('hex');
+ }
+ },
+ enumerable: false,
+ configurable: true
+ });
+ Object.defineProperty(ObjectId.prototype, "generationTime", {
+ /**
+ * The generation time of this ObjectId instance
+ * @deprecated Please use getTimestamp / createFromTime which returns an int32 epoch
+ */
+ get: function () {
+ return this.id.readInt32BE(0);
+ },
+ set: function (value) {
+ // Encode time into first 4 bytes
+ this.id.writeUInt32BE(value, 0);
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /** Returns the ObjectId id as a 24 character hex string representation */
+ ObjectId.prototype.toHexString = function () {
+ if (ObjectId.cacheHexString && this.__id) {
+ return this.__id;
+ }
+ var hexString = this.id.toString('hex');
+ if (ObjectId.cacheHexString && !this.__id) {
+ this.__id = hexString;
+ }
+ return hexString;
+ };
+ /**
+ * Update the ObjectId index
+ * @privateRemarks
+ * Used in generating new ObjectId's on the driver
+ * @internal
+ */
+ ObjectId.getInc = function () {
+ return (ObjectId.index = (ObjectId.index + 1) % 0xffffff);
+ };
+ /**
+ * Generate a 12 byte id buffer used in ObjectId's
+ *
+ * @param time - pass in a second based timestamp.
+ */
+ ObjectId.generate = function (time) {
+ if ('number' !== typeof time) {
+ time = ~~(Date.now() / 1000);
+ }
+ var inc = ObjectId.getInc();
+ var buffer$1 = buffer.Buffer.alloc(12);
+ // 4-byte timestamp
+ buffer$1.writeUInt32BE(time, 0);
+ // set PROCESS_UNIQUE if yet not initialized
+ if (PROCESS_UNIQUE === null) {
+ PROCESS_UNIQUE = utils.randomBytes(5);
+ }
+ // 5-byte process unique
+ buffer$1[4] = PROCESS_UNIQUE[0];
+ buffer$1[5] = PROCESS_UNIQUE[1];
+ buffer$1[6] = PROCESS_UNIQUE[2];
+ buffer$1[7] = PROCESS_UNIQUE[3];
+ buffer$1[8] = PROCESS_UNIQUE[4];
+ // 3-byte counter
+ buffer$1[11] = inc & 0xff;
+ buffer$1[10] = (inc >> 8) & 0xff;
+ buffer$1[9] = (inc >> 16) & 0xff;
+ return buffer$1;
+ };
+ /**
+ * Converts the id into a 24 character hex string for printing
+ *
+ * @param format - The Buffer toString format parameter.
+ * @internal
+ */
+ ObjectId.prototype.toString = function (format) {
+ // Is the id a buffer then use the buffer toString method to return the format
+ if (format)
+ return this.id.toString(format);
+ return this.toHexString();
+ };
+ /**
+ * Converts to its JSON the 24 character hex string representation.
+ * @internal
+ */
+ ObjectId.prototype.toJSON = function () {
+ return this.toHexString();
+ };
+ /**
+ * Compares the equality of this ObjectId with `otherID`.
+ *
+ * @param otherId - ObjectId instance to compare against.
+ */
+ ObjectId.prototype.equals = function (otherId) {
+ if (otherId === undefined || otherId === null) {
+ return false;
+ }
+ if (otherId instanceof ObjectId) {
+ return this.toString() === otherId.toString();
+ }
+ if (typeof otherId === 'string' &&
+ ObjectId.isValid(otherId) &&
+ otherId.length === 12 &&
+ utils.isUint8Array(this.id)) {
+ return otherId === buffer.Buffer.prototype.toString.call(this.id, 'latin1');
+ }
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 24) {
+ return otherId.toLowerCase() === this.toHexString();
+ }
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 12) {
+ return buffer.Buffer.from(otherId).equals(this.id);
+ }
+ if (typeof otherId === 'object' &&
+ 'toHexString' in otherId &&
+ typeof otherId.toHexString === 'function') {
+ return otherId.toHexString() === this.toHexString();
+ }
+ return false;
+ };
+ /** Returns the generation date (accurate up to the second) that this ID was generated. */
+ ObjectId.prototype.getTimestamp = function () {
+ var timestamp = new Date();
+ var time = this.id.readUInt32BE(0);
+ timestamp.setTime(Math.floor(time) * 1000);
+ return timestamp;
+ };
+ /** @internal */
+ ObjectId.createPk = function () {
+ return new ObjectId();
+ };
+ /**
+ * Creates an ObjectId from a second based number, with the rest of the ObjectId zeroed out. Used for comparisons or sorting the ObjectId.
+ *
+ * @param time - an integer number representing a number of seconds.
+ */
+ ObjectId.createFromTime = function (time) {
+ var buffer$1 = buffer.Buffer.from([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]);
+ // Encode time into first 4 bytes
+ buffer$1.writeUInt32BE(time, 0);
+ // Return the new objectId
+ return new ObjectId(buffer$1);
+ };
+ /**
+ * Creates an ObjectId from a hex string representation of an ObjectId.
+ *
+ * @param hexString - create a ObjectId from a passed in 24 character hexstring.
+ */
+ ObjectId.createFromHexString = function (hexString) {
+ // Throw an error if it's not a valid setup
+ if (typeof hexString === 'undefined' || (hexString != null && hexString.length !== 24)) {
+ throw new TypeError('Argument passed in must be a single String of 12 bytes or a string of 24 hex characters');
+ }
+ return new ObjectId(buffer.Buffer.from(hexString, 'hex'));
+ };
+ /**
+ * Checks if a value is a valid bson ObjectId
+ *
+ * @param id - ObjectId instance to validate.
+ */
+ ObjectId.isValid = function (id) {
+ if (id == null)
+ return false;
+ if (typeof id === 'number') {
+ return true;
+ }
+ if (typeof id === 'string') {
+ return id.length === 12 || (id.length === 24 && checkForHexRegExp.test(id));
+ }
+ if (id instanceof ObjectId) {
+ return true;
+ }
+ if (utils.isUint8Array(id) && id.length === 12) {
+ return true;
+ }
+ // Duck-Typing detection of ObjectId like objects
+ if (typeof id === 'object' && 'toHexString' in id && typeof id.toHexString === 'function') {
+ if (typeof id.id === 'string') {
+ return id.id.length === 12;
+ }
+ return id.toHexString().length === 24 && checkForHexRegExp.test(id.id.toString('hex'));
+ }
+ return false;
+ };
+ /** @internal */
+ ObjectId.prototype.toExtendedJSON = function () {
+ if (this.toHexString)
+ return { $oid: this.toHexString() };
+ return { $oid: this.toString('hex') };
+ };
+ /** @internal */
+ ObjectId.fromExtendedJSON = function (doc) {
+ return new ObjectId(doc.$oid);
+ };
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 24 character hex string representation.
+ * @internal
+ */
+ ObjectId.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ ObjectId.prototype.inspect = function () {
+ return "new ObjectId(\"" + this.toHexString() + "\")";
+ };
+ /** @internal */
+ ObjectId.index = ~~(Math.random() * 0xffffff);
+ return ObjectId;
+ }());
+ exports.ObjectId = ObjectId;
+ // Deprecated methods
+ Object.defineProperty(ObjectId.prototype, 'generate', {
+ value: utils.deprecate(function (time) { return ObjectId.generate(time); }, 'Please use the static `ObjectId.generate(time)` instead')
+ });
+ Object.defineProperty(ObjectId.prototype, 'getInc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+ });
+ Object.defineProperty(ObjectId.prototype, 'get_inc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+ });
+ Object.defineProperty(ObjectId, 'get_inc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+ });
+ Object.defineProperty(ObjectId.prototype, '_bsontype', { value: 'ObjectID' });
+
+ });
+
+ unwrapExports(objectid);
+ objectid.ObjectId;
+
+ var regexp = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.BSONRegExp = void 0;
+ function alphabetize(str) {
+ return str.split('').sort().join('');
+ }
+ /**
+ * A class representation of the BSON RegExp type.
+ * @public
+ */
+ var BSONRegExp = /** @class */ (function () {
+ /**
+ * @param pattern - The regular expression pattern to match
+ * @param options - The regular expression options
+ */
+ function BSONRegExp(pattern, options) {
+ if (!(this instanceof BSONRegExp))
+ return new BSONRegExp(pattern, options);
+ this.pattern = pattern;
+ this.options = alphabetize(options !== null && options !== void 0 ? options : '');
+ // Validate options
+ for (var i = 0; i < this.options.length; i++) {
+ if (!(this.options[i] === 'i' ||
+ this.options[i] === 'm' ||
+ this.options[i] === 'x' ||
+ this.options[i] === 'l' ||
+ this.options[i] === 's' ||
+ this.options[i] === 'u')) {
+ throw new Error("The regular expression option [" + this.options[i] + "] is not supported");
+ }
+ }
+ }
+ BSONRegExp.parseOptions = function (options) {
+ return options ? options.split('').sort().join('') : '';
+ };
+ /** @internal */
+ BSONRegExp.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ if (options.legacy) {
+ return { $regex: this.pattern, $options: this.options };
+ }
+ return { $regularExpression: { pattern: this.pattern, options: this.options } };
+ };
+ /** @internal */
+ BSONRegExp.fromExtendedJSON = function (doc) {
+ if ('$regex' in doc) {
+ if (typeof doc.$regex !== 'string') {
+ // This is for $regex query operators that have extended json values.
+ if (doc.$regex._bsontype === 'BSONRegExp') {
+ return doc;
+ }
+ }
+ else {
+ return new BSONRegExp(doc.$regex, BSONRegExp.parseOptions(doc.$options));
+ }
+ }
+ if ('$regularExpression' in doc) {
+ return new BSONRegExp(doc.$regularExpression.pattern, BSONRegExp.parseOptions(doc.$regularExpression.options));
+ }
+ throw new TypeError("Unexpected BSONRegExp EJSON object form: " + JSON.stringify(doc));
+ };
+ return BSONRegExp;
+ }());
+ exports.BSONRegExp = BSONRegExp;
+ Object.defineProperty(BSONRegExp.prototype, '_bsontype', { value: 'BSONRegExp' });
+
+ });
+
+ unwrapExports(regexp);
+ regexp.BSONRegExp;
+
+ var symbol = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.BSONSymbol = void 0;
+ /**
+ * A class representation of the BSON Symbol type.
+ * @public
+ */
+ var BSONSymbol = /** @class */ (function () {
+ /**
+ * @param value - the string representing the symbol.
+ */
+ function BSONSymbol(value) {
+ if (!(this instanceof BSONSymbol))
+ return new BSONSymbol(value);
+ this.value = value;
+ }
+ /** Access the wrapped string value. */
+ BSONSymbol.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.toString = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.inspect = function () {
+ return "new BSONSymbol(\"" + this.value + "\")";
+ };
+ /** @internal */
+ BSONSymbol.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.toExtendedJSON = function () {
+ return { $symbol: this.value };
+ };
+ /** @internal */
+ BSONSymbol.fromExtendedJSON = function (doc) {
+ return new BSONSymbol(doc.$symbol);
+ };
+ /** @internal */
+ BSONSymbol.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ return BSONSymbol;
+ }());
+ exports.BSONSymbol = BSONSymbol;
+ Object.defineProperty(BSONSymbol.prototype, '_bsontype', { value: 'Symbol' });
+
+ });
+
+ unwrapExports(symbol);
+ symbol.BSONSymbol;
+
+ /*! *****************************************************************************
+ Copyright (c) Microsoft Corporation.
+
+ Permission to use, copy, modify, and/or distribute this software for any
+ purpose with or without fee is hereby granted.
+
+ THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH
+ REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY
+ AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,
+ INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM
+ LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR
+ OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR
+ PERFORMANCE OF THIS SOFTWARE.
+ ***************************************************************************** */
+
+ /* global Reflect, Promise */
+ var _extendStatics = function extendStatics(d, b) {
+ _extendStatics = Object.setPrototypeOf || {
+ __proto__: []
+ } instanceof Array && function (d, b) {
+ d.__proto__ = b;
+ } || function (d, b) {
+ for (var p in b) {
+ if (b.hasOwnProperty(p)) d[p] = b[p];
+ }
+ };
+
+ return _extendStatics(d, b);
+ };
+
+ function __extends(d, b) {
+ _extendStatics(d, b);
+
+ function __() {
+ this.constructor = d;
+ }
+
+ d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
+ }
+
+ var _assign = function __assign() {
+ _assign = Object.assign || function __assign(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+
+ for (var p in s) {
+ if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p];
+ }
+ }
+
+ return t;
+ };
+
+ return _assign.apply(this, arguments);
+ };
+ function __rest(s, e) {
+ var t = {};
+
+ for (var p in s) {
+ if (Object.prototype.hasOwnProperty.call(s, p) && e.indexOf(p) < 0) t[p] = s[p];
+ }
+
+ if (s != null && typeof Object.getOwnPropertySymbols === "function") for (var i = 0, p = Object.getOwnPropertySymbols(s); i < p.length; i++) {
+ if (e.indexOf(p[i]) < 0 && Object.prototype.propertyIsEnumerable.call(s, p[i])) t[p[i]] = s[p[i]];
+ }
+ return t;
+ }
+ function __decorate(decorators, target, key, desc) {
+ var c = arguments.length,
+ r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc,
+ d;
+ if ((typeof Reflect === "undefined" ? "undefined" : babelHelpers["typeof"](Reflect)) === "object" && typeof Reflect.decorate === "function") r = Reflect.decorate(decorators, target, key, desc);else for (var i = decorators.length - 1; i >= 0; i--) {
+ if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;
+ }
+ return c > 3 && r && Object.defineProperty(target, key, r), r;
+ }
+ function __param(paramIndex, decorator) {
+ return function (target, key) {
+ decorator(target, key, paramIndex);
+ };
+ }
+ function __metadata(metadataKey, metadataValue) {
+ if ((typeof Reflect === "undefined" ? "undefined" : babelHelpers["typeof"](Reflect)) === "object" && typeof Reflect.metadata === "function") return Reflect.metadata(metadataKey, metadataValue);
+ }
+ function __awaiter(thisArg, _arguments, P, generator) {
+ function adopt(value) {
+ return value instanceof P ? value : new P(function (resolve) {
+ resolve(value);
+ });
+ }
+
+ return new (P || (P = Promise))(function (resolve, reject) {
+ function fulfilled(value) {
+ try {
+ step(generator.next(value));
+ } catch (e) {
+ reject(e);
+ }
+ }
+
+ function rejected(value) {
+ try {
+ step(generator["throw"](value));
+ } catch (e) {
+ reject(e);
+ }
+ }
+
+ function step(result) {
+ result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected);
+ }
+
+ step((generator = generator.apply(thisArg, _arguments || [])).next());
+ });
+ }
+ function __generator(thisArg, body) {
+ var _ = {
+ label: 0,
+ sent: function sent() {
+ if (t[0] & 1) throw t[1];
+ return t[1];
+ },
+ trys: [],
+ ops: []
+ },
+ f,
+ y,
+ t,
+ g;
+ return g = {
+ next: verb(0),
+ "throw": verb(1),
+ "return": verb(2)
+ }, typeof Symbol === "function" && (g[Symbol.iterator] = function () {
+ return this;
+ }), g;
+
+ function verb(n) {
+ return function (v) {
+ return step([n, v]);
+ };
+ }
+
+ function step(op) {
+ if (f) throw new TypeError("Generator is already executing.");
+
+ while (_) {
+ try {
+ if (f = 1, y && (t = op[0] & 2 ? y["return"] : op[0] ? y["throw"] || ((t = y["return"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done) return t;
+ if (y = 0, t) op = [op[0] & 2, t.value];
+
+ switch (op[0]) {
+ case 0:
+ case 1:
+ t = op;
+ break;
+
+ case 4:
+ _.label++;
+ return {
+ value: op[1],
+ done: false
+ };
+
+ case 5:
+ _.label++;
+ y = op[1];
+ op = [0];
+ continue;
+
+ case 7:
+ op = _.ops.pop();
+
+ _.trys.pop();
+
+ continue;
+
+ default:
+ if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) {
+ _ = 0;
+ continue;
+ }
+
+ if (op[0] === 3 && (!t || op[1] > t[0] && op[1] < t[3])) {
+ _.label = op[1];
+ break;
+ }
+
+ if (op[0] === 6 && _.label < t[1]) {
+ _.label = t[1];
+ t = op;
+ break;
+ }
+
+ if (t && _.label < t[2]) {
+ _.label = t[2];
+
+ _.ops.push(op);
+
+ break;
+ }
+
+ if (t[2]) _.ops.pop();
+
+ _.trys.pop();
+
+ continue;
+ }
+
+ op = body.call(thisArg, _);
+ } catch (e) {
+ op = [6, e];
+ y = 0;
+ } finally {
+ f = t = 0;
+ }
+ }
+
+ if (op[0] & 5) throw op[1];
+ return {
+ value: op[0] ? op[1] : void 0,
+ done: true
+ };
+ }
+ }
+ function __createBinding(o, m, k, k2) {
+ if (k2 === undefined) k2 = k;
+ o[k2] = m[k];
+ }
+ function __exportStar(m, exports) {
+ for (var p in m) {
+ if (p !== "default" && !exports.hasOwnProperty(p)) exports[p] = m[p];
+ }
+ }
+ function __values(o) {
+ var s = typeof Symbol === "function" && Symbol.iterator,
+ m = s && o[s],
+ i = 0;
+ if (m) return m.call(o);
+ if (o && typeof o.length === "number") return {
+ next: function next() {
+ if (o && i >= o.length) o = void 0;
+ return {
+ value: o && o[i++],
+ done: !o
+ };
+ }
+ };
+ throw new TypeError(s ? "Object is not iterable." : "Symbol.iterator is not defined.");
+ }
+ function __read(o, n) {
+ var m = typeof Symbol === "function" && o[Symbol.iterator];
+ if (!m) return o;
+ var i = m.call(o),
+ r,
+ ar = [],
+ e;
+
+ try {
+ while ((n === void 0 || n-- > 0) && !(r = i.next()).done) {
+ ar.push(r.value);
+ }
+ } catch (error) {
+ e = {
+ error: error
+ };
+ } finally {
+ try {
+ if (r && !r.done && (m = i["return"])) m.call(i);
+ } finally {
+ if (e) throw e.error;
+ }
+ }
+
+ return ar;
+ }
+ function __spread() {
+ for (var ar = [], i = 0; i < arguments.length; i++) {
+ ar = ar.concat(__read(arguments[i]));
+ }
+
+ return ar;
+ }
+ function __spreadArrays() {
+ for (var s = 0, i = 0, il = arguments.length; i < il; i++) {
+ s += arguments[i].length;
+ }
+
+ for (var r = Array(s), k = 0, i = 0; i < il; i++) {
+ for (var a = arguments[i], j = 0, jl = a.length; j < jl; j++, k++) {
+ r[k] = a[j];
+ }
+ }
+
+ return r;
+ }
+ function __await(v) {
+ return this instanceof __await ? (this.v = v, this) : new __await(v);
+ }
+ function __asyncGenerator(thisArg, _arguments, generator) {
+ if (!Symbol.asyncIterator) throw new TypeError("Symbol.asyncIterator is not defined.");
+ var g = generator.apply(thisArg, _arguments || []),
+ i,
+ q = [];
+ return i = {}, verb("next"), verb("throw"), verb("return"), i[Symbol.asyncIterator] = function () {
+ return this;
+ }, i;
+
+ function verb(n) {
+ if (g[n]) i[n] = function (v) {
+ return new Promise(function (a, b) {
+ q.push([n, v, a, b]) > 1 || resume(n, v);
+ });
+ };
+ }
+
+ function resume(n, v) {
+ try {
+ step(g[n](v));
+ } catch (e) {
+ settle(q[0][3], e);
+ }
+ }
+
+ function step(r) {
+ r.value instanceof __await ? Promise.resolve(r.value.v).then(fulfill, reject) : settle(q[0][2], r);
+ }
+
+ function fulfill(value) {
+ resume("next", value);
+ }
+
+ function reject(value) {
+ resume("throw", value);
+ }
+
+ function settle(f, v) {
+ if (f(v), q.shift(), q.length) resume(q[0][0], q[0][1]);
+ }
+ }
+ function __asyncDelegator(o) {
+ var i, p;
+ return i = {}, verb("next"), verb("throw", function (e) {
+ throw e;
+ }), verb("return"), i[Symbol.iterator] = function () {
+ return this;
+ }, i;
+
+ function verb(n, f) {
+ i[n] = o[n] ? function (v) {
+ return (p = !p) ? {
+ value: __await(o[n](v)),
+ done: n === "return"
+ } : f ? f(v) : v;
+ } : f;
+ }
+ }
+ function __asyncValues(o) {
+ if (!Symbol.asyncIterator) throw new TypeError("Symbol.asyncIterator is not defined.");
+ var m = o[Symbol.asyncIterator],
+ i;
+ return m ? m.call(o) : (o = typeof __values === "function" ? __values(o) : o[Symbol.iterator](), i = {}, verb("next"), verb("throw"), verb("return"), i[Symbol.asyncIterator] = function () {
+ return this;
+ }, i);
+
+ function verb(n) {
+ i[n] = o[n] && function (v) {
+ return new Promise(function (resolve, reject) {
+ v = o[n](v), settle(resolve, reject, v.done, v.value);
+ });
+ };
+ }
+
+ function settle(resolve, reject, d, v) {
+ Promise.resolve(v).then(function (v) {
+ resolve({
+ value: v,
+ done: d
+ });
+ }, reject);
+ }
+ }
+ function __makeTemplateObject(cooked, raw) {
+ if (Object.defineProperty) {
+ Object.defineProperty(cooked, "raw", {
+ value: raw
+ });
+ } else {
+ cooked.raw = raw;
+ }
+
+ return cooked;
+ }
+ function __importStar(mod) {
+ if (mod && mod.__esModule) return mod;
+ var result = {};
+ if (mod != null) for (var k in mod) {
+ if (Object.hasOwnProperty.call(mod, k)) result[k] = mod[k];
+ }
+ result["default"] = mod;
+ return result;
+ }
+ function __importDefault(mod) {
+ return mod && mod.__esModule ? mod : {
+ "default": mod
+ };
+ }
+ function __classPrivateFieldGet(receiver, privateMap) {
+ if (!privateMap.has(receiver)) {
+ throw new TypeError("attempted to get private field on non-instance");
+ }
+
+ return privateMap.get(receiver);
+ }
+ function __classPrivateFieldSet(receiver, privateMap, value) {
+ if (!privateMap.has(receiver)) {
+ throw new TypeError("attempted to set private field on non-instance");
+ }
+
+ privateMap.set(receiver, value);
+ return value;
+ }
+
+ var tslib_es6 = /*#__PURE__*/Object.freeze({
+ __proto__: null,
+ __extends: __extends,
+ get __assign () { return _assign; },
+ __rest: __rest,
+ __decorate: __decorate,
+ __param: __param,
+ __metadata: __metadata,
+ __awaiter: __awaiter,
+ __generator: __generator,
+ __createBinding: __createBinding,
+ __exportStar: __exportStar,
+ __values: __values,
+ __read: __read,
+ __spread: __spread,
+ __spreadArrays: __spreadArrays,
+ __await: __await,
+ __asyncGenerator: __asyncGenerator,
+ __asyncDelegator: __asyncDelegator,
+ __asyncValues: __asyncValues,
+ __makeTemplateObject: __makeTemplateObject,
+ __importStar: __importStar,
+ __importDefault: __importDefault,
+ __classPrivateFieldGet: __classPrivateFieldGet,
+ __classPrivateFieldSet: __classPrivateFieldSet
+ });
+
+ var timestamp = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Timestamp = exports.LongWithoutOverridesClass = void 0;
+
+
+ /** @public */
+ exports.LongWithoutOverridesClass = long_1.Long;
+ /** @public */
+ var Timestamp = /** @class */ (function (_super) {
+ tslib_es6.__extends(Timestamp, _super);
+ function Timestamp(low, high) {
+ var _this = this;
+ // eslint-disable-next-line @typescript-eslint/ban-ts-comment
+ ///@ts-expect-error
+ if (!(_this instanceof Timestamp))
+ return new Timestamp(low, high);
+ if (long_1.Long.isLong(low)) {
+ _this = _super.call(this, low.low, low.high, true) || this;
+ }
+ else {
+ _this = _super.call(this, low, high, true) || this;
+ }
+ Object.defineProperty(_this, '_bsontype', {
+ value: 'Timestamp',
+ writable: false,
+ configurable: false,
+ enumerable: false
+ });
+ return _this;
+ }
+ Timestamp.prototype.toJSON = function () {
+ return {
+ $timestamp: this.toString()
+ };
+ };
+ /** Returns a Timestamp represented by the given (32-bit) integer value. */
+ Timestamp.fromInt = function (value) {
+ return new Timestamp(long_1.Long.fromInt(value, true));
+ };
+ /** Returns a Timestamp representing the given number value, provided that it is a finite number. Otherwise, zero is returned. */
+ Timestamp.fromNumber = function (value) {
+ return new Timestamp(long_1.Long.fromNumber(value, true));
+ };
+ /**
+ * Returns a Timestamp for the given high and low bits. Each is assumed to use 32 bits.
+ *
+ * @param lowBits - the low 32-bits.
+ * @param highBits - the high 32-bits.
+ */
+ Timestamp.fromBits = function (lowBits, highBits) {
+ return new Timestamp(lowBits, highBits);
+ };
+ /**
+ * Returns a Timestamp from the given string, optionally using the given radix.
+ *
+ * @param str - the textual representation of the Timestamp.
+ * @param optRadix - the radix in which the text is written.
+ */
+ Timestamp.fromString = function (str, optRadix) {
+ return new Timestamp(long_1.Long.fromString(str, true, optRadix));
+ };
+ /** @internal */
+ Timestamp.prototype.toExtendedJSON = function () {
+ return { $timestamp: { t: this.high >>> 0, i: this.low >>> 0 } };
+ };
+ /** @internal */
+ Timestamp.fromExtendedJSON = function (doc) {
+ return new Timestamp(doc.$timestamp.i, doc.$timestamp.t);
+ };
+ /** @internal */
+ Timestamp.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Timestamp.prototype.inspect = function () {
+ return "new Timestamp(" + this.getLowBits().toString() + ", " + this.getHighBits().toString() + ")";
+ };
+ Timestamp.MAX_VALUE = long_1.Long.MAX_UNSIGNED_VALUE;
+ return Timestamp;
+ }(exports.LongWithoutOverridesClass));
+ exports.Timestamp = Timestamp;
+
+ });
+
+ unwrapExports(timestamp);
+ timestamp.Timestamp;
+ timestamp.LongWithoutOverridesClass;
+
+ var extended_json = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.EJSON = exports.isBSONType = void 0;
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ function isBSONType(value) {
+ return (utils.isObjectLike(value) && Reflect.has(value, '_bsontype') && typeof value._bsontype === 'string');
+ }
+ exports.isBSONType = isBSONType;
+ // INT32 boundaries
+ var BSON_INT32_MAX = 0x7fffffff;
+ var BSON_INT32_MIN = -0x80000000;
+ // INT64 boundaries
+ var BSON_INT64_MAX = 0x7fffffffffffffff;
+ var BSON_INT64_MIN = -0x8000000000000000;
+ // all the types where we don't need to do any special processing and can just pass the EJSON
+ //straight to type.fromExtendedJSON
+ var keysToCodecs = {
+ $oid: objectid.ObjectId,
+ $binary: binary.Binary,
+ $uuid: binary.Binary,
+ $symbol: symbol.BSONSymbol,
+ $numberInt: int_32.Int32,
+ $numberDecimal: decimal128.Decimal128,
+ $numberDouble: double_1.Double,
+ $numberLong: long_1.Long,
+ $minKey: min_key.MinKey,
+ $maxKey: max_key.MaxKey,
+ $regex: regexp.BSONRegExp,
+ $regularExpression: regexp.BSONRegExp,
+ $timestamp: timestamp.Timestamp
+ };
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ function deserializeValue(value, options) {
+ if (options === void 0) { options = {}; }
+ if (typeof value === 'number') {
+ if (options.relaxed || options.legacy) {
+ return value;
+ }
+ // if it's an integer, should interpret as smallest BSON integer
+ // that can represent it exactly. (if out of range, interpret as double.)
+ if (Math.floor(value) === value) {
+ if (value >= BSON_INT32_MIN && value <= BSON_INT32_MAX)
+ return new int_32.Int32(value);
+ if (value >= BSON_INT64_MIN && value <= BSON_INT64_MAX)
+ return long_1.Long.fromNumber(value);
+ }
+ // If the number is a non-integer or out of integer range, should interpret as BSON Double.
+ return new double_1.Double(value);
+ }
+ // from here on out we're looking for bson types, so bail if its not an object
+ if (value == null || typeof value !== 'object')
+ return value;
+ // upgrade deprecated undefined to null
+ if (value.$undefined)
+ return null;
+ var keys = Object.keys(value).filter(function (k) { return k.startsWith('$') && value[k] != null; });
+ for (var i = 0; i < keys.length; i++) {
+ var c = keysToCodecs[keys[i]];
+ if (c)
+ return c.fromExtendedJSON(value, options);
+ }
+ if (value.$date != null) {
+ var d = value.$date;
+ var date = new Date();
+ if (options.legacy) {
+ if (typeof d === 'number')
+ date.setTime(d);
+ else if (typeof d === 'string')
+ date.setTime(Date.parse(d));
+ }
+ else {
+ if (typeof d === 'string')
+ date.setTime(Date.parse(d));
+ else if (long_1.Long.isLong(d))
+ date.setTime(d.toNumber());
+ else if (typeof d === 'number' && options.relaxed)
+ date.setTime(d);
+ }
+ return date;
+ }
+ if (value.$code != null) {
+ var copy = Object.assign({}, value);
+ if (value.$scope) {
+ copy.$scope = deserializeValue(value.$scope);
+ }
+ return code.Code.fromExtendedJSON(value);
+ }
+ if (db_ref.isDBRefLike(value) || value.$dbPointer) {
+ var v = value.$ref ? value : value.$dbPointer;
+ // we run into this in a "degenerate EJSON" case (with $id and $ref order flipped)
+ // because of the order JSON.parse goes through the document
+ if (v instanceof db_ref.DBRef)
+ return v;
+ var dollarKeys = Object.keys(v).filter(function (k) { return k.startsWith('$'); });
+ var valid_1 = true;
+ dollarKeys.forEach(function (k) {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1)
+ valid_1 = false;
+ });
+ // only make DBRef if $ keys are all valid
+ if (valid_1)
+ return db_ref.DBRef.fromExtendedJSON(v);
+ }
+ return value;
+ }
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ function serializeArray(array, options) {
+ return array.map(function (v, index) {
+ options.seenObjects.push({ propertyName: "index " + index, obj: null });
+ try {
+ return serializeValue(v, options);
+ }
+ finally {
+ options.seenObjects.pop();
+ }
+ });
+ }
+ function getISOString(date) {
+ var isoStr = date.toISOString();
+ // we should only show milliseconds in timestamp if they're non-zero
+ return date.getUTCMilliseconds() !== 0 ? isoStr : isoStr.slice(0, -5) + 'Z';
+ }
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ function serializeValue(value, options) {
+ if ((typeof value === 'object' || typeof value === 'function') && value !== null) {
+ var index = options.seenObjects.findIndex(function (entry) { return entry.obj === value; });
+ if (index !== -1) {
+ var props = options.seenObjects.map(function (entry) { return entry.propertyName; });
+ var leadingPart = props
+ .slice(0, index)
+ .map(function (prop) { return prop + " -> "; })
+ .join('');
+ var alreadySeen = props[index];
+ var circularPart = ' -> ' +
+ props
+ .slice(index + 1, props.length - 1)
+ .map(function (prop) { return prop + " -> "; })
+ .join('');
+ var current = props[props.length - 1];
+ var leadingSpace = ' '.repeat(leadingPart.length + alreadySeen.length / 2);
+ var dashes = '-'.repeat(circularPart.length + (alreadySeen.length + current.length) / 2 - 1);
+ throw new TypeError('Converting circular structure to EJSON:\n' +
+ (" " + leadingPart + alreadySeen + circularPart + current + "\n") +
+ (" " + leadingSpace + "\\" + dashes + "/"));
+ }
+ options.seenObjects[options.seenObjects.length - 1].obj = value;
+ }
+ if (Array.isArray(value))
+ return serializeArray(value, options);
+ if (value === undefined)
+ return null;
+ if (value instanceof Date || utils.isDate(value)) {
+ var dateNum = value.getTime(),
+ // is it in year range 1970-9999?
+ inRange = dateNum > -1 && dateNum < 253402318800000;
+ if (options.legacy) {
+ return options.relaxed && inRange
+ ? { $date: value.getTime() }
+ : { $date: getISOString(value) };
+ }
+ return options.relaxed && inRange
+ ? { $date: getISOString(value) }
+ : { $date: { $numberLong: value.getTime().toString() } };
+ }
+ if (typeof value === 'number' && (!options.relaxed || !isFinite(value))) {
+ // it's an integer
+ if (Math.floor(value) === value) {
+ var int32Range = value >= BSON_INT32_MIN && value <= BSON_INT32_MAX, int64Range = value >= BSON_INT64_MIN && value <= BSON_INT64_MAX;
+ // interpret as being of the smallest BSON integer type that can represent the number exactly
+ if (int32Range)
+ return { $numberInt: value.toString() };
+ if (int64Range)
+ return { $numberLong: value.toString() };
+ }
+ return { $numberDouble: value.toString() };
+ }
+ if (value instanceof RegExp || utils.isRegExp(value)) {
+ var flags = value.flags;
+ if (flags === undefined) {
+ var match = value.toString().match(/[gimuy]*$/);
+ if (match) {
+ flags = match[0];
+ }
+ }
+ var rx = new regexp.BSONRegExp(value.source, flags);
+ return rx.toExtendedJSON(options);
+ }
+ if (value != null && typeof value === 'object')
+ return serializeDocument(value, options);
+ return value;
+ }
+ var BSON_TYPE_MAPPINGS = {
+ Binary: function (o) { return new binary.Binary(o.value(), o.sub_type); },
+ Code: function (o) { return new code.Code(o.code, o.scope); },
+ DBRef: function (o) { return new db_ref.DBRef(o.collection || o.namespace, o.oid, o.db, o.fields); },
+ Decimal128: function (o) { return new decimal128.Decimal128(o.bytes); },
+ Double: function (o) { return new double_1.Double(o.value); },
+ Int32: function (o) { return new int_32.Int32(o.value); },
+ Long: function (o) {
+ return long_1.Long.fromBits(
+ // underscore variants for 1.x backwards compatibility
+ o.low != null ? o.low : o.low_, o.low != null ? o.high : o.high_, o.low != null ? o.unsigned : o.unsigned_);
+ },
+ MaxKey: function () { return new max_key.MaxKey(); },
+ MinKey: function () { return new min_key.MinKey(); },
+ ObjectID: function (o) { return new objectid.ObjectId(o); },
+ ObjectId: function (o) { return new objectid.ObjectId(o); },
+ BSONRegExp: function (o) { return new regexp.BSONRegExp(o.pattern, o.options); },
+ Symbol: function (o) { return new symbol.BSONSymbol(o.value); },
+ Timestamp: function (o) { return timestamp.Timestamp.fromBits(o.low, o.high); }
+ };
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ function serializeDocument(doc, options) {
+ if (doc == null || typeof doc !== 'object')
+ throw new Error('not an object instance');
+ var bsontype = doc._bsontype;
+ if (typeof bsontype === 'undefined') {
+ // It's a regular object. Recursively serialize its property values.
+ var _doc = {};
+ for (var name in doc) {
+ options.seenObjects.push({ propertyName: name, obj: null });
+ try {
+ _doc[name] = serializeValue(doc[name], options);
+ }
+ finally {
+ options.seenObjects.pop();
+ }
+ }
+ return _doc;
+ }
+ else if (isBSONType(doc)) {
+ // the "document" is really just a BSON type object
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ var outDoc = doc;
+ if (typeof outDoc.toExtendedJSON !== 'function') {
+ // There's no EJSON serialization function on the object. It's probably an
+ // object created by a previous version of this library (or another library)
+ // that's duck-typing objects to look like they were generated by this library).
+ // Copy the object into this library's version of that type.
+ var mapper = BSON_TYPE_MAPPINGS[doc._bsontype];
+ if (!mapper) {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + doc._bsontype);
+ }
+ outDoc = mapper(outDoc);
+ }
+ // Two BSON types may have nested objects that may need to be serialized too
+ if (bsontype === 'Code' && outDoc.scope) {
+ outDoc = new code.Code(outDoc.code, serializeValue(outDoc.scope, options));
+ }
+ else if (bsontype === 'DBRef' && outDoc.oid) {
+ outDoc = new db_ref.DBRef(serializeValue(outDoc.collection, options), serializeValue(outDoc.oid, options), serializeValue(outDoc.db, options), serializeValue(outDoc.fields, options));
+ }
+ return outDoc.toExtendedJSON(options);
+ }
+ else {
+ throw new Error('_bsontype must be a string, but was: ' + typeof bsontype);
+ }
+ }
+ (function (EJSON) {
+ /**
+ * Parse an Extended JSON string, constructing the JavaScript value or object described by that
+ * string.
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const text = '{ "int32": { "$numberInt": "10" } }';
+ *
+ * // prints { int32: { [String: '10'] _bsontype: 'Int32', value: '10' } }
+ * console.log(EJSON.parse(text, { relaxed: false }));
+ *
+ * // prints { int32: 10 }
+ * console.log(EJSON.parse(text));
+ * ```
+ */
+ function parse(text, options) {
+ var finalOptions = Object.assign({}, { relaxed: true, legacy: false }, options);
+ // relaxed implies not strict
+ if (typeof finalOptions.relaxed === 'boolean')
+ finalOptions.strict = !finalOptions.relaxed;
+ if (typeof finalOptions.strict === 'boolean')
+ finalOptions.relaxed = !finalOptions.strict;
+ return JSON.parse(text, function (_key, value) { return deserializeValue(value, finalOptions); });
+ }
+ EJSON.parse = parse;
+ /**
+ * Converts a BSON document to an Extended JSON string, optionally replacing values if a replacer
+ * function is specified or optionally including only the specified properties if a replacer array
+ * is specified.
+ *
+ * @param value - The value to convert to extended JSON
+ * @param replacer - A function that alters the behavior of the stringification process, or an array of String and Number objects that serve as a whitelist for selecting/filtering the properties of the value object to be included in the JSON string. If this value is null or not provided, all properties of the object are included in the resulting JSON string
+ * @param space - A String or Number object that's used to insert white space into the output JSON string for readability purposes.
+ * @param options - Optional settings
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const Int32 = require('mongodb').Int32;
+ * const doc = { int32: new Int32(10) };
+ *
+ * // prints '{"int32":{"$numberInt":"10"}}'
+ * console.log(EJSON.stringify(doc, { relaxed: false }));
+ *
+ * // prints '{"int32":10}'
+ * console.log(EJSON.stringify(doc));
+ * ```
+ */
+ function stringify(value,
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ replacer, space, options) {
+ if (space != null && typeof space === 'object') {
+ options = space;
+ space = 0;
+ }
+ if (replacer != null && typeof replacer === 'object' && !Array.isArray(replacer)) {
+ options = replacer;
+ replacer = undefined;
+ space = 0;
+ }
+ var serializeOptions = Object.assign({ relaxed: true, legacy: false }, options, {
+ seenObjects: [{ propertyName: '(root)', obj: null }]
+ });
+ var doc = serializeValue(value, serializeOptions);
+ return JSON.stringify(doc, replacer, space);
+ }
+ EJSON.stringify = stringify;
+ /**
+ * Serializes an object to an Extended JSON string, and reparse it as a JavaScript object.
+ *
+ * @param value - The object to serialize
+ * @param options - Optional settings passed to the `stringify` function
+ */
+ function serialize(value, options) {
+ options = options || {};
+ return JSON.parse(stringify(value, options));
+ }
+ EJSON.serialize = serialize;
+ /**
+ * Deserializes an Extended JSON object into a plain JavaScript object with native/BSON types
+ *
+ * @param ejson - The Extended JSON object to deserialize
+ * @param options - Optional settings passed to the parse method
+ */
+ function deserialize(ejson, options) {
+ options = options || {};
+ return parse(JSON.stringify(ejson), options);
+ }
+ EJSON.deserialize = deserialize;
+ })(exports.EJSON || (exports.EJSON = {}));
+
+ });
+
+ unwrapExports(extended_json);
+ extended_json.EJSON;
+ extended_json.isBSONType;
+
+ var map = createCommonjsModule(function (module, exports) {
+ /* eslint-disable @typescript-eslint/no-explicit-any */
+ // We have an ES6 Map available, return the native instance
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.Map = void 0;
+ /** @public */
+ var bsonMap;
+ exports.Map = bsonMap;
+ var check = function (potentialGlobal) {
+ // eslint-disable-next-line eqeqeq
+ return potentialGlobal && potentialGlobal.Math == Math && potentialGlobal;
+ };
+ // https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+ function getGlobal() {
+ // eslint-disable-next-line no-undef
+ return (check(typeof globalThis === 'object' && globalThis) ||
+ check(typeof window === 'object' && window) ||
+ check(typeof self === 'object' && self) ||
+ check(typeof commonjsGlobal === 'object' && commonjsGlobal) ||
+ Function('return this')());
+ }
+ var bsonGlobal = getGlobal();
+ if (Object.prototype.hasOwnProperty.call(bsonGlobal, 'Map')) {
+ exports.Map = bsonMap = bsonGlobal.Map;
+ }
+ else {
+ // We will return a polyfill
+ exports.Map = bsonMap = /** @class */ (function () {
+ function Map(array) {
+ if (array === void 0) { array = []; }
+ this._keys = [];
+ this._values = {};
+ for (var i = 0; i < array.length; i++) {
+ if (array[i] == null)
+ continue; // skip null and undefined
+ var entry = array[i];
+ var key = entry[0];
+ var value = entry[1];
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ }
+ }
+ Map.prototype.clear = function () {
+ this._keys = [];
+ this._values = {};
+ };
+ Map.prototype.delete = function (key) {
+ var value = this._values[key];
+ if (value == null)
+ return false;
+ // Delete entry
+ delete this._values[key];
+ // Remove the key from the ordered keys list
+ this._keys.splice(value.i, 1);
+ return true;
+ };
+ Map.prototype.entries = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? [key, _this._values[key].v] : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Map.prototype.forEach = function (callback, self) {
+ self = self || this;
+ for (var i = 0; i < this._keys.length; i++) {
+ var key = this._keys[i];
+ // Call the forEach callback
+ callback.call(self, this._values[key].v, key, self);
+ }
+ };
+ Map.prototype.get = function (key) {
+ return this._values[key] ? this._values[key].v : undefined;
+ };
+ Map.prototype.has = function (key) {
+ return this._values[key] != null;
+ };
+ Map.prototype.keys = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? key : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Map.prototype.set = function (key, value) {
+ if (this._values[key]) {
+ this._values[key].v = value;
+ return this;
+ }
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ return this;
+ };
+ Map.prototype.values = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? _this._values[key].v : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Object.defineProperty(Map.prototype, "size", {
+ get: function () {
+ return this._keys.length;
+ },
+ enumerable: false,
+ configurable: true
+ });
+ return Map;
+ }());
+ }
+
+ });
+
+ unwrapExports(map);
+ map.Map;
+
+ var constants = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.BSON_BINARY_SUBTYPE_USER_DEFINED = exports.BSON_BINARY_SUBTYPE_MD5 = exports.BSON_BINARY_SUBTYPE_UUID_NEW = exports.BSON_BINARY_SUBTYPE_UUID = exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = exports.BSON_BINARY_SUBTYPE_FUNCTION = exports.BSON_BINARY_SUBTYPE_DEFAULT = exports.BSON_DATA_MAX_KEY = exports.BSON_DATA_MIN_KEY = exports.BSON_DATA_DECIMAL128 = exports.BSON_DATA_LONG = exports.BSON_DATA_TIMESTAMP = exports.BSON_DATA_INT = exports.BSON_DATA_CODE_W_SCOPE = exports.BSON_DATA_SYMBOL = exports.BSON_DATA_CODE = exports.BSON_DATA_DBPOINTER = exports.BSON_DATA_REGEXP = exports.BSON_DATA_NULL = exports.BSON_DATA_DATE = exports.BSON_DATA_BOOLEAN = exports.BSON_DATA_OID = exports.BSON_DATA_UNDEFINED = exports.BSON_DATA_BINARY = exports.BSON_DATA_ARRAY = exports.BSON_DATA_OBJECT = exports.BSON_DATA_STRING = exports.BSON_DATA_NUMBER = exports.JS_INT_MIN = exports.JS_INT_MAX = exports.BSON_INT64_MIN = exports.BSON_INT64_MAX = exports.BSON_INT32_MIN = exports.BSON_INT32_MAX = void 0;
+ /** @internal */
+ exports.BSON_INT32_MAX = 0x7fffffff;
+ /** @internal */
+ exports.BSON_INT32_MIN = -0x80000000;
+ /** @internal */
+ exports.BSON_INT64_MAX = Math.pow(2, 63) - 1;
+ /** @internal */
+ exports.BSON_INT64_MIN = -Math.pow(2, 63);
+ /**
+ * Any integer up to 2^53 can be precisely represented by a double.
+ * @internal
+ */
+ exports.JS_INT_MAX = Math.pow(2, 53);
+ /**
+ * Any integer down to -2^53 can be precisely represented by a double.
+ * @internal
+ */
+ exports.JS_INT_MIN = -Math.pow(2, 53);
+ /** Number BSON Type @internal */
+ exports.BSON_DATA_NUMBER = 1;
+ /** String BSON Type @internal */
+ exports.BSON_DATA_STRING = 2;
+ /** Object BSON Type @internal */
+ exports.BSON_DATA_OBJECT = 3;
+ /** Array BSON Type @internal */
+ exports.BSON_DATA_ARRAY = 4;
+ /** Binary BSON Type @internal */
+ exports.BSON_DATA_BINARY = 5;
+ /** Binary BSON Type @internal */
+ exports.BSON_DATA_UNDEFINED = 6;
+ /** ObjectId BSON Type @internal */
+ exports.BSON_DATA_OID = 7;
+ /** Boolean BSON Type @internal */
+ exports.BSON_DATA_BOOLEAN = 8;
+ /** Date BSON Type @internal */
+ exports.BSON_DATA_DATE = 9;
+ /** null BSON Type @internal */
+ exports.BSON_DATA_NULL = 10;
+ /** RegExp BSON Type @internal */
+ exports.BSON_DATA_REGEXP = 11;
+ /** Code BSON Type @internal */
+ exports.BSON_DATA_DBPOINTER = 12;
+ /** Code BSON Type @internal */
+ exports.BSON_DATA_CODE = 13;
+ /** Symbol BSON Type @internal */
+ exports.BSON_DATA_SYMBOL = 14;
+ /** Code with Scope BSON Type @internal */
+ exports.BSON_DATA_CODE_W_SCOPE = 15;
+ /** 32 bit Integer BSON Type @internal */
+ exports.BSON_DATA_INT = 16;
+ /** Timestamp BSON Type @internal */
+ exports.BSON_DATA_TIMESTAMP = 17;
+ /** Long BSON Type @internal */
+ exports.BSON_DATA_LONG = 18;
+ /** Decimal128 BSON Type @internal */
+ exports.BSON_DATA_DECIMAL128 = 19;
+ /** MinKey BSON Type @internal */
+ exports.BSON_DATA_MIN_KEY = 0xff;
+ /** MaxKey BSON Type @internal */
+ exports.BSON_DATA_MAX_KEY = 0x7f;
+ /** Binary Default Type @internal */
+ exports.BSON_BINARY_SUBTYPE_DEFAULT = 0;
+ /** Binary Function Type @internal */
+ exports.BSON_BINARY_SUBTYPE_FUNCTION = 1;
+ /** Binary Byte Array Type @internal */
+ exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
+ /** Binary Deprecated UUID Type @deprecated Please use BSON_BINARY_SUBTYPE_UUID_NEW @internal */
+ exports.BSON_BINARY_SUBTYPE_UUID = 3;
+ /** Binary UUID Type @internal */
+ exports.BSON_BINARY_SUBTYPE_UUID_NEW = 4;
+ /** Binary MD5 Type @internal */
+ exports.BSON_BINARY_SUBTYPE_MD5 = 5;
+ /** Binary User Defined Type @internal */
+ exports.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
+
+ });
+
+ unwrapExports(constants);
+ constants.BSON_BINARY_SUBTYPE_USER_DEFINED;
+ constants.BSON_BINARY_SUBTYPE_MD5;
+ constants.BSON_BINARY_SUBTYPE_UUID_NEW;
+ constants.BSON_BINARY_SUBTYPE_UUID;
+ constants.BSON_BINARY_SUBTYPE_BYTE_ARRAY;
+ constants.BSON_BINARY_SUBTYPE_FUNCTION;
+ constants.BSON_BINARY_SUBTYPE_DEFAULT;
+ constants.BSON_DATA_MAX_KEY;
+ constants.BSON_DATA_MIN_KEY;
+ constants.BSON_DATA_DECIMAL128;
+ constants.BSON_DATA_LONG;
+ constants.BSON_DATA_TIMESTAMP;
+ constants.BSON_DATA_INT;
+ constants.BSON_DATA_CODE_W_SCOPE;
+ constants.BSON_DATA_SYMBOL;
+ constants.BSON_DATA_CODE;
+ constants.BSON_DATA_DBPOINTER;
+ constants.BSON_DATA_REGEXP;
+ constants.BSON_DATA_NULL;
+ constants.BSON_DATA_DATE;
+ constants.BSON_DATA_BOOLEAN;
+ constants.BSON_DATA_OID;
+ constants.BSON_DATA_UNDEFINED;
+ constants.BSON_DATA_BINARY;
+ constants.BSON_DATA_ARRAY;
+ constants.BSON_DATA_OBJECT;
+ constants.BSON_DATA_STRING;
+ constants.BSON_DATA_NUMBER;
+ constants.JS_INT_MIN;
+ constants.JS_INT_MAX;
+ constants.BSON_INT64_MIN;
+ constants.BSON_INT64_MAX;
+ constants.BSON_INT32_MIN;
+ constants.BSON_INT32_MAX;
+
+ var calculate_size = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.calculateObjectSize = void 0;
+
+
+
+
+ function calculateObjectSize(object, serializeFunctions, ignoreUndefined) {
+ var totalLength = 4 + 1;
+ if (Array.isArray(object)) {
+ for (var i = 0; i < object.length; i++) {
+ totalLength += calculateElement(i.toString(), object[i], serializeFunctions, true, ignoreUndefined);
+ }
+ }
+ else {
+ // If we have toBSON defined, override the current object
+ if (object.toBSON) {
+ object = object.toBSON();
+ }
+ // Calculate size
+ for (var key in object) {
+ totalLength += calculateElement(key, object[key], serializeFunctions, false, ignoreUndefined);
+ }
+ }
+ return totalLength;
+ }
+ exports.calculateObjectSize = calculateObjectSize;
+ /** @internal */
+ function calculateElement(name,
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ value, serializeFunctions, isArray, ignoreUndefined) {
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (isArray === void 0) { isArray = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = false; }
+ // If we have toBSON defined, override the current object
+ if (value && value.toBSON) {
+ value = value.toBSON();
+ }
+ switch (typeof value) {
+ case 'string':
+ return 1 + buffer.Buffer.byteLength(name, 'utf8') + 1 + 4 + buffer.Buffer.byteLength(value, 'utf8') + 1;
+ case 'number':
+ if (Math.floor(value) === value &&
+ value >= constants.JS_INT_MIN &&
+ value <= constants.JS_INT_MAX) {
+ if (value >= constants.BSON_INT32_MIN && value <= constants.BSON_INT32_MAX) {
+ // 32 bit
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (4 + 1);
+ }
+ else {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ }
+ else {
+ // 64 bit
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ case 'undefined':
+ if (isArray || !ignoreUndefined)
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ return 0;
+ case 'boolean':
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 1);
+ case 'object':
+ if (value == null || value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (12 + 1);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ else if (ArrayBuffer.isView(value) ||
+ value instanceof ArrayBuffer ||
+ utils.isAnyArrayBuffer(value)) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 4 + 1) + value.byteLength);
+ }
+ else if (value['_bsontype'] === 'Long' ||
+ value['_bsontype'] === 'Double' ||
+ value['_bsontype'] === 'Timestamp') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ else if (value['_bsontype'] === 'Decimal128') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (16 + 1);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ // Calculate size depending on the availability of a scope
+ if (value.scope != null && Object.keys(value.scope).length > 0) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ buffer.Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined));
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ buffer.Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1);
+ }
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ // Check what kind of subtype we have
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ (value.position + 1 + 4 + 1 + 4));
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (value.position + 1 + 4 + 1));
+ }
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ buffer.Buffer.byteLength(value.value, 'utf8') +
+ 4 +
+ 1 +
+ 1);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ // Set up correct object for serialization
+ var ordered_values = Object.assign({
+ $ref: value.collection,
+ $id: value.oid
+ }, value.fields);
+ // Add db reference if it exists
+ if (value.db != null) {
+ ordered_values['$db'] = value.db;
+ }
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ calculateObjectSize(ordered_values, serializeFunctions, ignoreUndefined));
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.pattern, 'utf8') +
+ 1 +
+ buffer.Buffer.byteLength(value.options, 'utf8') +
+ 1);
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ calculateObjectSize(value, serializeFunctions, ignoreUndefined) +
+ 1);
+ }
+ case 'function':
+ // WTF for 0.4.X where typeof /someregexp/ === 'function'
+ if (value instanceof RegExp || utils.isRegExp(value) || String.call(value) === '[object RegExp]') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1);
+ }
+ else {
+ if (serializeFunctions && value.scope != null && Object.keys(value.scope).length > 0) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ buffer.Buffer.byteLength(utils.normalizedFunctionString(value), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined));
+ }
+ else if (serializeFunctions) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ buffer.Buffer.byteLength(utils.normalizedFunctionString(value), 'utf8') +
+ 1);
+ }
+ }
+ }
+ return 0;
+ }
+
+ });
+
+ unwrapExports(calculate_size);
+ calculate_size.calculateObjectSize;
+
+ var validate_utf8 = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.validateUtf8 = void 0;
+ var FIRST_BIT = 0x80;
+ var FIRST_TWO_BITS = 0xc0;
+ var FIRST_THREE_BITS = 0xe0;
+ var FIRST_FOUR_BITS = 0xf0;
+ var FIRST_FIVE_BITS = 0xf8;
+ var TWO_BIT_CHAR = 0xc0;
+ var THREE_BIT_CHAR = 0xe0;
+ var FOUR_BIT_CHAR = 0xf0;
+ var CONTINUING_CHAR = 0x80;
+ /**
+ * Determines if the passed in bytes are valid utf8
+ * @param bytes - An array of 8-bit bytes. Must be indexable and have length property
+ * @param start - The index to start validating
+ * @param end - The index to end validating
+ */
+ function validateUtf8(bytes, start, end) {
+ var continuation = 0;
+ for (var i = start; i < end; i += 1) {
+ var byte = bytes[i];
+ if (continuation) {
+ if ((byte & FIRST_TWO_BITS) !== CONTINUING_CHAR) {
+ return false;
+ }
+ continuation -= 1;
+ }
+ else if (byte & FIRST_BIT) {
+ if ((byte & FIRST_THREE_BITS) === TWO_BIT_CHAR) {
+ continuation = 1;
+ }
+ else if ((byte & FIRST_FOUR_BITS) === THREE_BIT_CHAR) {
+ continuation = 2;
+ }
+ else if ((byte & FIRST_FIVE_BITS) === FOUR_BIT_CHAR) {
+ continuation = 3;
+ }
+ else {
+ return false;
+ }
+ }
+ }
+ return !continuation;
+ }
+ exports.validateUtf8 = validateUtf8;
+
+ });
+
+ unwrapExports(validate_utf8);
+ validate_utf8.validateUtf8;
+
+ var deserializer = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.deserialize = void 0;
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ // Internal long versions
+ var JS_INT_MAX_LONG = long_1.Long.fromNumber(constants.JS_INT_MAX);
+ var JS_INT_MIN_LONG = long_1.Long.fromNumber(constants.JS_INT_MIN);
+ var functionCache = {};
+ function deserialize(buffer, options, isArray) {
+ options = options == null ? {} : options;
+ var index = options && options.index ? options.index : 0;
+ // Read the document size
+ var size = buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ if (size < 5) {
+ throw new Error("bson size must be >= 5, is " + size);
+ }
+ if (options.allowObjectSmallerThanBufferSize && buffer.length < size) {
+ throw new Error("buffer length " + buffer.length + " must be >= bson size " + size);
+ }
+ if (!options.allowObjectSmallerThanBufferSize && buffer.length !== size) {
+ throw new Error("buffer length " + buffer.length + " must === bson size " + size);
+ }
+ if (size + index > buffer.byteLength) {
+ throw new Error("(bson size " + size + " + options.index " + index + " must be <= buffer length " + buffer.byteLength + ")");
+ }
+ // Illegal end value
+ if (buffer[index + size - 1] !== 0) {
+ throw new Error("One object, sized correctly, with a spot for an EOO, but the EOO isn't 0x00");
+ }
+ // Start deserializtion
+ return deserializeObject(buffer, index, options, isArray);
+ }
+ exports.deserialize = deserialize;
+ function deserializeObject(buffer$1, index, options, isArray) {
+ if (isArray === void 0) { isArray = false; }
+ var evalFunctions = options['evalFunctions'] == null ? false : options['evalFunctions'];
+ var cacheFunctions = options['cacheFunctions'] == null ? false : options['cacheFunctions'];
+ var fieldsAsRaw = options['fieldsAsRaw'] == null ? null : options['fieldsAsRaw'];
+ // Return raw bson buffer instead of parsing it
+ var raw = options['raw'] == null ? false : options['raw'];
+ // Return BSONRegExp objects instead of native regular expressions
+ var bsonRegExp = typeof options['bsonRegExp'] === 'boolean' ? options['bsonRegExp'] : false;
+ // Controls the promotion of values vs wrapper classes
+ var promoteBuffers = options['promoteBuffers'] == null ? false : options['promoteBuffers'];
+ var promoteLongs = options['promoteLongs'] == null ? true : options['promoteLongs'];
+ var promoteValues = options['promoteValues'] == null ? true : options['promoteValues'];
+ // Set the start index
+ var startIndex = index;
+ // Validate that we have at least 4 bytes of buffer
+ if (buffer$1.length < 5)
+ throw new Error('corrupt bson message < 5 bytes long');
+ // Read the document size
+ var size = buffer$1[index++] | (buffer$1[index++] << 8) | (buffer$1[index++] << 16) | (buffer$1[index++] << 24);
+ // Ensure buffer is valid size
+ if (size < 5 || size > buffer$1.length)
+ throw new Error('corrupt bson message');
+ // Create holding object
+ var object = isArray ? [] : {};
+ // Used for arrays to skip having to perform utf8 decoding
+ var arrayIndex = 0;
+ var done = false;
+ // While we have more left data left keep parsing
+ while (!done) {
+ // Read the type
+ var elementType = buffer$1[index++];
+ // If we get a zero it's the last byte, exit
+ if (elementType === 0)
+ break;
+ // Get the start search index
+ var i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.byteLength)
+ throw new Error('Bad BSON Document: illegal CString');
+ var name = isArray ? arrayIndex++ : buffer$1.toString('utf8', index, i);
+ var value = void 0;
+ index = i + 1;
+ if (elementType === constants.BSON_DATA_STRING) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ if (!validate_utf8.validateUtf8(buffer$1, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ value = buffer$1.toString('utf8', index, index + stringSize - 1);
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_OID) {
+ var oid = buffer.Buffer.alloc(12);
+ buffer$1.copy(oid, 0, index, index + 12);
+ value = new objectid.ObjectId(oid);
+ index = index + 12;
+ }
+ else if (elementType === constants.BSON_DATA_INT && promoteValues === false) {
+ value = new int_32.Int32(buffer$1[index++] | (buffer$1[index++] << 8) | (buffer$1[index++] << 16) | (buffer$1[index++] << 24));
+ }
+ else if (elementType === constants.BSON_DATA_INT) {
+ value =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ }
+ else if (elementType === constants.BSON_DATA_NUMBER && promoteValues === false) {
+ value = new double_1.Double(buffer$1.readDoubleLE(index));
+ index = index + 8;
+ }
+ else if (elementType === constants.BSON_DATA_NUMBER) {
+ value = buffer$1.readDoubleLE(index);
+ index = index + 8;
+ }
+ else if (elementType === constants.BSON_DATA_DATE) {
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ value = new Date(new long_1.Long(lowBits, highBits).toNumber());
+ }
+ else if (elementType === constants.BSON_DATA_BOOLEAN) {
+ if (buffer$1[index] !== 0 && buffer$1[index] !== 1)
+ throw new Error('illegal boolean type value');
+ value = buffer$1[index++] === 1;
+ }
+ else if (elementType === constants.BSON_DATA_OBJECT) {
+ var _index = index;
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ if (objectSize <= 0 || objectSize > buffer$1.length - index)
+ throw new Error('bad embedded document length in bson');
+ // We have a raw value
+ if (raw) {
+ value = buffer$1.slice(index, index + objectSize);
+ }
+ else {
+ value = deserializeObject(buffer$1, _index, options, false);
+ }
+ index = index + objectSize;
+ }
+ else if (elementType === constants.BSON_DATA_ARRAY) {
+ var _index = index;
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ var arrayOptions = options;
+ // Stop index
+ var stopIndex = index + objectSize;
+ // All elements of array to be returned as raw bson
+ if (fieldsAsRaw && fieldsAsRaw[name]) {
+ arrayOptions = {};
+ for (var n in options) {
+ arrayOptions[n] = options[n];
+ }
+ arrayOptions['raw'] = true;
+ }
+ value = deserializeObject(buffer$1, _index, arrayOptions, true);
+ index = index + objectSize;
+ if (buffer$1[index - 1] !== 0)
+ throw new Error('invalid array terminator byte');
+ if (index !== stopIndex)
+ throw new Error('corrupted array bson');
+ }
+ else if (elementType === constants.BSON_DATA_UNDEFINED) {
+ value = undefined;
+ }
+ else if (elementType === constants.BSON_DATA_NULL) {
+ value = null;
+ }
+ else if (elementType === constants.BSON_DATA_LONG) {
+ // Unpack the low and high bits
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var long = new long_1.Long(lowBits, highBits);
+ // Promote the long if possible
+ if (promoteLongs && promoteValues === true) {
+ value =
+ long.lessThanOrEqual(JS_INT_MAX_LONG) && long.greaterThanOrEqual(JS_INT_MIN_LONG)
+ ? long.toNumber()
+ : long;
+ }
+ else {
+ value = long;
+ }
+ }
+ else if (elementType === constants.BSON_DATA_DECIMAL128) {
+ // Buffer to contain the decimal bytes
+ var bytes = buffer.Buffer.alloc(16);
+ // Copy the next 16 bytes into the bytes buffer
+ buffer$1.copy(bytes, 0, index, index + 16);
+ // Update index
+ index = index + 16;
+ // Assign the new Decimal128 value
+ var decimal128$1 = new decimal128.Decimal128(bytes);
+ // If we have an alternative mapper use that
+ if ('toObject' in decimal128$1 && typeof decimal128$1.toObject === 'function') {
+ value = decimal128$1.toObject();
+ }
+ else {
+ value = decimal128$1;
+ }
+ }
+ else if (elementType === constants.BSON_DATA_BINARY) {
+ var binarySize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var totalBinarySize = binarySize;
+ var subType = buffer$1[index++];
+ // Did we have a negative binary size, throw
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found');
+ // Is the length longer than the document
+ if (binarySize > buffer$1.byteLength)
+ throw new Error('Binary type size larger than document size');
+ // Decode as raw Buffer object if options specifies it
+ if (buffer$1['slice'] != null) {
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+ if (promoteBuffers && promoteValues) {
+ value = buffer$1.slice(index, index + binarySize);
+ }
+ else {
+ value = new binary.Binary(buffer$1.slice(index, index + binarySize), subType);
+ }
+ }
+ else {
+ var _buffer = buffer.Buffer.alloc(binarySize);
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+ // Copy the data
+ for (i = 0; i < binarySize; i++) {
+ _buffer[i] = buffer$1[index + i];
+ }
+ if (promoteBuffers && promoteValues) {
+ value = _buffer;
+ }
+ else {
+ value = new binary.Binary(_buffer, subType);
+ }
+ }
+ // Update the index
+ index = index + binarySize;
+ }
+ else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === false) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var source = buffer$1.toString('utf8', index, i);
+ // Create the regexp
+ index = i + 1;
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var regExpOptions = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // For each option add the corresponding one for javascript
+ var optionsArray = new Array(regExpOptions.length);
+ // Parse options
+ for (i = 0; i < regExpOptions.length; i++) {
+ switch (regExpOptions[i]) {
+ case 'm':
+ optionsArray[i] = 'm';
+ break;
+ case 's':
+ optionsArray[i] = 'g';
+ break;
+ case 'i':
+ optionsArray[i] = 'i';
+ break;
+ }
+ }
+ value = new RegExp(source, optionsArray.join(''));
+ }
+ else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === true) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var source = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var regExpOptions = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // Set the object
+ value = new regexp.BSONRegExp(source, regExpOptions);
+ }
+ else if (elementType === constants.BSON_DATA_SYMBOL) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ var symbol$1 = buffer$1.toString('utf8', index, index + stringSize - 1);
+ value = promoteValues ? symbol$1 : new symbol.BSONSymbol(symbol$1);
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_TIMESTAMP) {
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ value = new timestamp.Timestamp(lowBits, highBits);
+ }
+ else if (elementType === constants.BSON_DATA_MIN_KEY) {
+ value = new min_key.MinKey();
+ }
+ else if (elementType === constants.BSON_DATA_MAX_KEY) {
+ value = new max_key.MaxKey();
+ }
+ else if (elementType === constants.BSON_DATA_CODE) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ var functionString = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ }
+ else {
+ value = isolateEval(functionString);
+ }
+ }
+ else {
+ value = new code.Code(functionString);
+ }
+ // Update parse index position
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_CODE_W_SCOPE) {
+ var totalSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Element cannot be shorter than totalSize + stringSize + documentSize + terminator
+ if (totalSize < 4 + 4 + 4 + 1) {
+ throw new Error('code_w_scope total size shorter minimum expected length');
+ }
+ // Get the code string size
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Check if we have a valid string
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ // Javascript function
+ var functionString = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Parse the element
+ var _index = index;
+ // Decode the size of the object document
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ // Decode the scope object
+ var scopeObject = deserializeObject(buffer$1, _index, options, false);
+ // Adjust the index
+ index = index + objectSize;
+ // Check if field length is too short
+ if (totalSize < 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too short, truncating scope');
+ }
+ // Check if totalSize field is too long
+ if (totalSize > 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too long, clips outer document');
+ }
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ }
+ else {
+ value = isolateEval(functionString);
+ }
+ value.scope = scopeObject;
+ }
+ else {
+ value = new code.Code(functionString, scopeObject);
+ }
+ }
+ else if (elementType === constants.BSON_DATA_DBPOINTER) {
+ // Get the code string size
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Check if we have a valid string
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ // Namespace
+ if (!validate_utf8.validateUtf8(buffer$1, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ var namespace = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Read the oid
+ var oidBuffer = buffer.Buffer.alloc(12);
+ buffer$1.copy(oidBuffer, 0, index, index + 12);
+ var oid = new objectid.ObjectId(oidBuffer);
+ // Update the index
+ index = index + 12;
+ // Upgrade to DBRef type
+ value = new db_ref.DBRef(namespace, oid);
+ }
+ else {
+ throw new Error('Detected unknown BSON type ' + elementType.toString(16) + ' for fieldname "' + name + '"');
+ }
+ if (name === '__proto__') {
+ Object.defineProperty(object, name, {
+ value: value,
+ writable: true,
+ enumerable: true,
+ configurable: true
+ });
+ }
+ else {
+ object[name] = value;
+ }
+ }
+ // Check if the deserialization was against a valid array/object
+ if (size !== index - startIndex) {
+ if (isArray)
+ throw new Error('corrupt array bson');
+ throw new Error('corrupt object bson');
+ }
+ // check if object's $ keys are those of a DBRef
+ var dollarKeys = Object.keys(object).filter(function (k) { return k.startsWith('$'); });
+ var valid = true;
+ dollarKeys.forEach(function (k) {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1)
+ valid = false;
+ });
+ // if a $key not in "$ref", "$id", "$db", don't make a DBRef
+ if (!valid)
+ return object;
+ if (db_ref.isDBRefLike(object)) {
+ var copy = Object.assign({}, object);
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new db_ref.DBRef(object.$ref, object.$id, object.$db, copy);
+ }
+ return object;
+ }
+ /**
+ * Ensure eval is isolated, store the result in functionCache.
+ *
+ * @internal
+ */
+ function isolateEval(functionString, functionCache, object) {
+ if (!functionCache)
+ return new Function(functionString);
+ // Check for cache hit, eval if missing and return cached function
+ if (functionCache[functionString] == null) {
+ functionCache[functionString] = new Function(functionString);
+ }
+ // Set the object
+ return functionCache[functionString].bind(object);
+ }
+
+ });
+
+ unwrapExports(deserializer);
+ deserializer.deserialize;
+
+ var float_parser = createCommonjsModule(function (module, exports) {
+ // Copyright (c) 2008, Fair Oaks Labs, Inc.
+ // All rights reserved.
+ //
+ // Redistribution and use in source and binary forms, with or without
+ // modification, are permitted provided that the following conditions are met:
+ //
+ // * Redistributions of source code must retain the above copyright notice,
+ // this list of conditions and the following disclaimer.
+ //
+ // * Redistributions in binary form must reproduce the above copyright notice,
+ // this list of conditions and the following disclaimer in the documentation
+ // and/or other materials provided with the distribution.
+ //
+ // * Neither the name of Fair Oaks Labs, Inc. nor the names of its contributors
+ // may be used to endorse or promote products derived from this software
+ // without specific prior written permission.
+ //
+ // THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+ // AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+ // IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
+ // ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
+ // LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
+ // CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
+ // SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
+ // INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
+ // CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
+ // ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
+ // POSSIBILITY OF SUCH DAMAGE.
+ //
+ //
+ // Modifications to writeIEEE754 to support negative zeroes made by Brian White
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.writeIEEE754 = exports.readIEEE754 = void 0;
+ function readIEEE754(buffer, offset, endian, mLen, nBytes) {
+ var e;
+ var m;
+ var bBE = endian === 'big';
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var nBits = -7;
+ var i = bBE ? 0 : nBytes - 1;
+ var d = bBE ? 1 : -1;
+ var s = buffer[offset + i];
+ i += d;
+ e = s & ((1 << -nBits) - 1);
+ s >>= -nBits;
+ nBits += eLen;
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8)
+ ;
+ m = e & ((1 << -nBits) - 1);
+ e >>= -nBits;
+ nBits += mLen;
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8)
+ ;
+ if (e === 0) {
+ e = 1 - eBias;
+ }
+ else if (e === eMax) {
+ return m ? NaN : (s ? -1 : 1) * Infinity;
+ }
+ else {
+ m = m + Math.pow(2, mLen);
+ e = e - eBias;
+ }
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
+ }
+ exports.readIEEE754 = readIEEE754;
+ function writeIEEE754(buffer, value, offset, endian, mLen, nBytes) {
+ var e;
+ var m;
+ var c;
+ var bBE = endian === 'big';
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0;
+ var i = bBE ? nBytes - 1 : 0;
+ var d = bBE ? -1 : 1;
+ var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0;
+ value = Math.abs(value);
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0;
+ e = eMax;
+ }
+ else {
+ e = Math.floor(Math.log(value) / Math.LN2);
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--;
+ c *= 2;
+ }
+ if (e + eBias >= 1) {
+ value += rt / c;
+ }
+ else {
+ value += rt * Math.pow(2, 1 - eBias);
+ }
+ if (value * c >= 2) {
+ e++;
+ c /= 2;
+ }
+ if (e + eBias >= eMax) {
+ m = 0;
+ e = eMax;
+ }
+ else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen);
+ e = e + eBias;
+ }
+ else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
+ e = 0;
+ }
+ }
+ if (isNaN(value))
+ m = 0;
+ while (mLen >= 8) {
+ buffer[offset + i] = m & 0xff;
+ i += d;
+ m /= 256;
+ mLen -= 8;
+ }
+ e = (e << mLen) | m;
+ if (isNaN(value))
+ e += 8;
+ eLen += mLen;
+ while (eLen > 0) {
+ buffer[offset + i] = e & 0xff;
+ i += d;
+ e /= 256;
+ eLen -= 8;
+ }
+ buffer[offset + i - d] |= s * 128;
+ }
+ exports.writeIEEE754 = writeIEEE754;
+
+ });
+
+ unwrapExports(float_parser);
+ float_parser.writeIEEE754;
+ float_parser.readIEEE754;
+
+ var serializer = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.serializeInto = void 0;
+
+
+
+
+
+
+
+
+ var regexp = /\x00/; // eslint-disable-line no-control-regex
+ var ignoreKeys = new Set(['$db', '$ref', '$id', '$clusterTime']);
+ /*
+ * isArray indicates if we are writing to a BSON array (type 0x04)
+ * which forces the "key" which really an array index as a string to be written as ascii
+ * This will catch any errors in index as a string generation
+ */
+ function serializeString(buffer, key, value, index, isArray) {
+ // Encode String type
+ buffer[index++] = constants.BSON_DATA_STRING;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes + 1;
+ buffer[index - 1] = 0;
+ // Write the string
+ var size = buffer.write(value, index + 4, undefined, 'utf8');
+ // Write the size of the string to buffer
+ buffer[index + 3] = ((size + 1) >> 24) & 0xff;
+ buffer[index + 2] = ((size + 1) >> 16) & 0xff;
+ buffer[index + 1] = ((size + 1) >> 8) & 0xff;
+ buffer[index] = (size + 1) & 0xff;
+ // Update index
+ index = index + 4 + size;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+ }
+ function serializeNumber(buffer, key, value, index, isArray) {
+ // We have an integer value
+ // TODO(NODE-2529): Add support for big int
+ if (Number.isInteger(value) &&
+ value >= constants.BSON_INT32_MIN &&
+ value <= constants.BSON_INT32_MAX) {
+ // If the value fits in 32 bits encode as int32
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ }
+ else {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ float_parser.writeIEEE754(buffer, value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ }
+ return index;
+ }
+ function serializeNull(buffer, key, _, index, isArray) {
+ // Set long type
+ buffer[index++] = constants.BSON_DATA_NULL;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+ }
+ function serializeBoolean(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BOOLEAN;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Encode the boolean value
+ buffer[index++] = value ? 1 : 0;
+ return index;
+ }
+ function serializeDate(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_DATE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ var dateInMilis = long_1.Long.fromNumber(value.getTime());
+ var lowBits = dateInMilis.getLowBits();
+ var highBits = dateInMilis.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+ }
+ function serializeRegExp(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ if (value.source && value.source.match(regexp) != null) {
+ throw Error('value ' + value.source + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.source, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the parameters
+ if (value.ignoreCase)
+ buffer[index++] = 0x69; // i
+ if (value.global)
+ buffer[index++] = 0x73; // s
+ if (value.multiline)
+ buffer[index++] = 0x6d; // m
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+ }
+ function serializeBSONRegExp(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Check the pattern for 0 bytes
+ if (value.pattern.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('pattern ' + value.pattern + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.pattern, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the options
+ index = index + buffer.write(value.options.split('').sort().join(''), index, undefined, 'utf8');
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+ }
+ function serializeMinMax(buffer, key, value, index, isArray) {
+ // Write the type of either min or max key
+ if (value === null) {
+ buffer[index++] = constants.BSON_DATA_NULL;
+ }
+ else if (value._bsontype === 'MinKey') {
+ buffer[index++] = constants.BSON_DATA_MIN_KEY;
+ }
+ else {
+ buffer[index++] = constants.BSON_DATA_MAX_KEY;
+ }
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+ }
+ function serializeObjectId(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OID;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the objectId into the shared buffer
+ if (typeof value.id === 'string') {
+ buffer.write(value.id, index, undefined, 'binary');
+ }
+ else if (utils.isUint8Array(value.id)) {
+ // Use the standard JS methods here because buffer.copy() is buggy with the
+ // browser polyfill
+ buffer.set(value.id.subarray(0, 12), index);
+ }
+ else {
+ throw new TypeError('object [' + JSON.stringify(value) + '] is not a valid ObjectId');
+ }
+ // Adjust index
+ return index + 12;
+ }
+ function serializeBuffer(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Get size of the buffer (current write point)
+ var size = value.length;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the default subtype
+ buffer[index++] = constants.BSON_BINARY_SUBTYPE_DEFAULT;
+ // Copy the content form the binary field to the buffer
+ buffer.set(ensure_buffer.ensureBuffer(value), index);
+ // Adjust the index
+ index = index + size;
+ return index;
+ }
+ function serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray, path) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (isArray === void 0) { isArray = false; }
+ if (path === void 0) { path = []; }
+ for (var i = 0; i < path.length; i++) {
+ if (path[i] === value)
+ throw new Error('cyclic dependency detected');
+ }
+ // Push value to stack
+ path.push(value);
+ // Write the type
+ buffer[index++] = Array.isArray(value) ? constants.BSON_DATA_ARRAY : constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ var endIndex = serializeInto(buffer, value, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined, path);
+ // Pop stack
+ path.pop();
+ return endIndex;
+ }
+ function serializeDecimal128(buffer, key, value, index, isArray) {
+ buffer[index++] = constants.BSON_DATA_DECIMAL128;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the data from the value
+ // Prefer the standard JS methods because their typechecking is not buggy,
+ // unlike the `buffer` polyfill's.
+ buffer.set(value.bytes.subarray(0, 16), index);
+ return index + 16;
+ }
+ function serializeLong(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] =
+ value._bsontype === 'Long' ? constants.BSON_DATA_LONG : constants.BSON_DATA_TIMESTAMP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ var lowBits = value.getLowBits();
+ var highBits = value.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+ }
+ function serializeInt32(buffer, key, value, index, isArray) {
+ value = value.valueOf();
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ return index;
+ }
+ function serializeDouble(buffer, key, value, index, isArray) {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ float_parser.writeIEEE754(buffer, value.value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ return index;
+ }
+ function serializeFunction(buffer, key, value, index, _checkKeys, _depth, isArray) {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ var functionString = utils.normalizedFunctionString(value);
+ // Write the string
+ var size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+ }
+ function serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (isArray === void 0) { isArray = false; }
+ if (value.scope && typeof value.scope === 'object') {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_CODE_W_SCOPE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Starting index
+ var startIndex = index;
+ // Serialize the function
+ // Get the function string
+ var functionString = typeof value.code === 'string' ? value.code : value.code.toString();
+ // Index adjustment
+ index = index + 4;
+ // Write string into buffer
+ var codeSize = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = codeSize & 0xff;
+ buffer[index + 1] = (codeSize >> 8) & 0xff;
+ buffer[index + 2] = (codeSize >> 16) & 0xff;
+ buffer[index + 3] = (codeSize >> 24) & 0xff;
+ // Write end 0
+ buffer[index + 4 + codeSize - 1] = 0;
+ // Write the
+ index = index + codeSize + 4;
+ //
+ // Serialize the scope value
+ var endIndex = serializeInto(buffer, value.scope, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined);
+ index = endIndex - 1;
+ // Writ the total
+ var totalSize = endIndex - startIndex;
+ // Write the total size of the object
+ buffer[startIndex++] = totalSize & 0xff;
+ buffer[startIndex++] = (totalSize >> 8) & 0xff;
+ buffer[startIndex++] = (totalSize >> 16) & 0xff;
+ buffer[startIndex++] = (totalSize >> 24) & 0xff;
+ // Write trailing zero
+ buffer[index++] = 0;
+ }
+ else {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ var functionString = value.code.toString();
+ // Write the string
+ var size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ }
+ return index;
+ }
+ function serializeBinary(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Extract the buffer
+ var data = value.value(true);
+ // Calculate size
+ var size = value.position;
+ // Add the deprecated 02 type 4 bytes of size to total
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY)
+ size = size + 4;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the subtype to the buffer
+ buffer[index++] = value.sub_type;
+ // If we have binary type 2 the 4 first bytes are the size
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ size = size - 4;
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ }
+ // Write the data to the object
+ buffer.set(data, index);
+ // Adjust the index
+ index = index + value.position;
+ return index;
+ }
+ function serializeSymbol(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_SYMBOL;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the string
+ var size = buffer.write(value.value, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0x00;
+ return index;
+ }
+ function serializeDBRef(buffer, key, value, index, depth, serializeFunctions, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ var startIndex = index;
+ var output = {
+ $ref: value.collection || value.namespace,
+ $id: value.oid
+ };
+ if (value.db != null) {
+ output.$db = value.db;
+ }
+ output = Object.assign(output, value.fields);
+ var endIndex = serializeInto(buffer, output, false, index, depth + 1, serializeFunctions);
+ // Calculate object size
+ var size = endIndex - startIndex;
+ // Write the size
+ buffer[startIndex++] = size & 0xff;
+ buffer[startIndex++] = (size >> 8) & 0xff;
+ buffer[startIndex++] = (size >> 16) & 0xff;
+ buffer[startIndex++] = (size >> 24) & 0xff;
+ // Set index
+ return endIndex;
+ }
+ function serializeInto(buffer, object, checkKeys, startingIndex, depth, serializeFunctions, ignoreUndefined, path) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (startingIndex === void 0) { startingIndex = 0; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (path === void 0) { path = []; }
+ startingIndex = startingIndex || 0;
+ path = path || [];
+ // Push the object to the path
+ path.push(object);
+ // Start place to serialize into
+ var index = startingIndex + 4;
+ // Special case isArray
+ if (Array.isArray(object)) {
+ // Get object keys
+ for (var i = 0; i < object.length; i++) {
+ var key = '' + i;
+ var value = object[i];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+ if (typeof value === 'string') {
+ index = serializeString(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'number') {
+ index = serializeNumber(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (typeof value === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index, true);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index, true);
+ }
+ else if (value === undefined) {
+ index = serializeNull(buffer, key, value, index, true);
+ }
+ else if (value === null) {
+ index = serializeNull(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index, true);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index, true);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true, path);
+ }
+ else if (typeof value === 'object' &&
+ extended_json.isBSONType(value) &&
+ value._bsontype === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, true);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions, true);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index, true);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ else if (object instanceof map.Map || utils.isMap(object)) {
+ var iterator = object.entries();
+ var done = false;
+ while (!done) {
+ // Unpack the next entry
+ var entry = iterator.next();
+ done = !!entry.done;
+ // Are we done, then skip and terminate
+ if (done)
+ continue;
+ // Get the entry values
+ var key = entry.value[0];
+ var value = entry.value[1];
+ // Check the type of the value
+ var type = typeof value;
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ }
+ else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ }
+ else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ }
+ else if (type === 'bigint' || utils.isBigInt64Array(value) || utils.isBigUInt64Array(value)) {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ }
+ else if (value === null || (value === undefined && ignoreUndefined === false)) {
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ }
+ else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
+ }
+ else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ else {
+ // Did we provide a custom serialization method
+ if (object.toBSON) {
+ if (typeof object.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ object = object.toBSON();
+ if (object != null && typeof object !== 'object')
+ throw new TypeError('toBSON function did not return an object');
+ }
+ // Iterate over all the keys
+ for (var key in object) {
+ var value = object[key];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+ // Check the type of the value
+ var type = typeof value;
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ }
+ else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ }
+ else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ }
+ else if (type === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ }
+ else if (value === undefined) {
+ if (ignoreUndefined === false)
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value === null) {
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ }
+ else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
+ }
+ else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ // Remove the path
+ path.pop();
+ // Final padding byte for object
+ buffer[index++] = 0x00;
+ // Final size
+ var size = index - startingIndex;
+ // Write the size of the object
+ buffer[startingIndex++] = size & 0xff;
+ buffer[startingIndex++] = (size >> 8) & 0xff;
+ buffer[startingIndex++] = (size >> 16) & 0xff;
+ buffer[startingIndex++] = (size >> 24) & 0xff;
+ return index;
+ }
+ exports.serializeInto = serializeInto;
+
+ });
+
+ unwrapExports(serializer);
+ serializer.serializeInto;
+
+ var bson = createCommonjsModule(function (module, exports) {
+ Object.defineProperty(exports, "__esModule", { value: true });
+ exports.ObjectID = exports.Decimal128 = exports.BSONRegExp = exports.MaxKey = exports.MinKey = exports.Int32 = exports.Double = exports.Timestamp = exports.Long = exports.UUID = exports.ObjectId = exports.Binary = exports.DBRef = exports.BSONSymbol = exports.Map = exports.Code = exports.LongWithoutOverridesClass = exports.EJSON = exports.BSON_INT64_MIN = exports.BSON_INT64_MAX = exports.BSON_INT32_MIN = exports.BSON_INT32_MAX = exports.BSON_DATA_UNDEFINED = exports.BSON_DATA_TIMESTAMP = exports.BSON_DATA_SYMBOL = exports.BSON_DATA_STRING = exports.BSON_DATA_REGEXP = exports.BSON_DATA_OID = exports.BSON_DATA_OBJECT = exports.BSON_DATA_NUMBER = exports.BSON_DATA_NULL = exports.BSON_DATA_MIN_KEY = exports.BSON_DATA_MAX_KEY = exports.BSON_DATA_LONG = exports.BSON_DATA_INT = exports.BSON_DATA_DECIMAL128 = exports.BSON_DATA_DBPOINTER = exports.BSON_DATA_DATE = exports.BSON_DATA_CODE_W_SCOPE = exports.BSON_DATA_CODE = exports.BSON_DATA_BOOLEAN = exports.BSON_DATA_BINARY = exports.BSON_DATA_ARRAY = exports.BSON_BINARY_SUBTYPE_UUID_NEW = exports.BSON_BINARY_SUBTYPE_UUID = exports.BSON_BINARY_SUBTYPE_USER_DEFINED = exports.BSON_BINARY_SUBTYPE_MD5 = exports.BSON_BINARY_SUBTYPE_FUNCTION = exports.BSON_BINARY_SUBTYPE_DEFAULT = exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = void 0;
+ exports.deserializeStream = exports.calculateObjectSize = exports.deserialize = exports.serializeWithBufferAndIndex = exports.serialize = exports.setInternalBufferSize = void 0;
+
+
+ Object.defineProperty(exports, "Binary", { enumerable: true, get: function () { return binary.Binary; } });
+
+ Object.defineProperty(exports, "Code", { enumerable: true, get: function () { return code.Code; } });
+
+ Object.defineProperty(exports, "DBRef", { enumerable: true, get: function () { return db_ref.DBRef; } });
+
+ Object.defineProperty(exports, "Decimal128", { enumerable: true, get: function () { return decimal128.Decimal128; } });
+
+ Object.defineProperty(exports, "Double", { enumerable: true, get: function () { return double_1.Double; } });
+
+
+
+ Object.defineProperty(exports, "Int32", { enumerable: true, get: function () { return int_32.Int32; } });
+
+ Object.defineProperty(exports, "Long", { enumerable: true, get: function () { return long_1.Long; } });
+
+ Object.defineProperty(exports, "Map", { enumerable: true, get: function () { return map.Map; } });
+
+ Object.defineProperty(exports, "MaxKey", { enumerable: true, get: function () { return max_key.MaxKey; } });
+
+ Object.defineProperty(exports, "MinKey", { enumerable: true, get: function () { return min_key.MinKey; } });
+
+ Object.defineProperty(exports, "ObjectId", { enumerable: true, get: function () { return objectid.ObjectId; } });
+ Object.defineProperty(exports, "ObjectID", { enumerable: true, get: function () { return objectid.ObjectId; } });
+
+ // Parts of the parser
+
+
+
+ Object.defineProperty(exports, "BSONRegExp", { enumerable: true, get: function () { return regexp.BSONRegExp; } });
+
+ Object.defineProperty(exports, "BSONSymbol", { enumerable: true, get: function () { return symbol.BSONSymbol; } });
+
+ Object.defineProperty(exports, "Timestamp", { enumerable: true, get: function () { return timestamp.Timestamp; } });
+
+ Object.defineProperty(exports, "UUID", { enumerable: true, get: function () { return uuid.UUID; } });
+
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_BYTE_ARRAY", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_BYTE_ARRAY; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_DEFAULT", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_DEFAULT; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_FUNCTION", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_FUNCTION; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_MD5", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_MD5; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_USER_DEFINED", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_USER_DEFINED; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_UUID", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_UUID; } });
+ Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_UUID_NEW", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_UUID_NEW; } });
+ Object.defineProperty(exports, "BSON_DATA_ARRAY", { enumerable: true, get: function () { return constants.BSON_DATA_ARRAY; } });
+ Object.defineProperty(exports, "BSON_DATA_BINARY", { enumerable: true, get: function () { return constants.BSON_DATA_BINARY; } });
+ Object.defineProperty(exports, "BSON_DATA_BOOLEAN", { enumerable: true, get: function () { return constants.BSON_DATA_BOOLEAN; } });
+ Object.defineProperty(exports, "BSON_DATA_CODE", { enumerable: true, get: function () { return constants.BSON_DATA_CODE; } });
+ Object.defineProperty(exports, "BSON_DATA_CODE_W_SCOPE", { enumerable: true, get: function () { return constants.BSON_DATA_CODE_W_SCOPE; } });
+ Object.defineProperty(exports, "BSON_DATA_DATE", { enumerable: true, get: function () { return constants.BSON_DATA_DATE; } });
+ Object.defineProperty(exports, "BSON_DATA_DBPOINTER", { enumerable: true, get: function () { return constants.BSON_DATA_DBPOINTER; } });
+ Object.defineProperty(exports, "BSON_DATA_DECIMAL128", { enumerable: true, get: function () { return constants.BSON_DATA_DECIMAL128; } });
+ Object.defineProperty(exports, "BSON_DATA_INT", { enumerable: true, get: function () { return constants.BSON_DATA_INT; } });
+ Object.defineProperty(exports, "BSON_DATA_LONG", { enumerable: true, get: function () { return constants.BSON_DATA_LONG; } });
+ Object.defineProperty(exports, "BSON_DATA_MAX_KEY", { enumerable: true, get: function () { return constants.BSON_DATA_MAX_KEY; } });
+ Object.defineProperty(exports, "BSON_DATA_MIN_KEY", { enumerable: true, get: function () { return constants.BSON_DATA_MIN_KEY; } });
+ Object.defineProperty(exports, "BSON_DATA_NULL", { enumerable: true, get: function () { return constants.BSON_DATA_NULL; } });
+ Object.defineProperty(exports, "BSON_DATA_NUMBER", { enumerable: true, get: function () { return constants.BSON_DATA_NUMBER; } });
+ Object.defineProperty(exports, "BSON_DATA_OBJECT", { enumerable: true, get: function () { return constants.BSON_DATA_OBJECT; } });
+ Object.defineProperty(exports, "BSON_DATA_OID", { enumerable: true, get: function () { return constants.BSON_DATA_OID; } });
+ Object.defineProperty(exports, "BSON_DATA_REGEXP", { enumerable: true, get: function () { return constants.BSON_DATA_REGEXP; } });
+ Object.defineProperty(exports, "BSON_DATA_STRING", { enumerable: true, get: function () { return constants.BSON_DATA_STRING; } });
+ Object.defineProperty(exports, "BSON_DATA_SYMBOL", { enumerable: true, get: function () { return constants.BSON_DATA_SYMBOL; } });
+ Object.defineProperty(exports, "BSON_DATA_TIMESTAMP", { enumerable: true, get: function () { return constants.BSON_DATA_TIMESTAMP; } });
+ Object.defineProperty(exports, "BSON_DATA_UNDEFINED", { enumerable: true, get: function () { return constants.BSON_DATA_UNDEFINED; } });
+ Object.defineProperty(exports, "BSON_INT32_MAX", { enumerable: true, get: function () { return constants.BSON_INT32_MAX; } });
+ Object.defineProperty(exports, "BSON_INT32_MIN", { enumerable: true, get: function () { return constants.BSON_INT32_MIN; } });
+ Object.defineProperty(exports, "BSON_INT64_MAX", { enumerable: true, get: function () { return constants.BSON_INT64_MAX; } });
+ Object.defineProperty(exports, "BSON_INT64_MIN", { enumerable: true, get: function () { return constants.BSON_INT64_MIN; } });
+ var extended_json_2 = extended_json;
+ Object.defineProperty(exports, "EJSON", { enumerable: true, get: function () { return extended_json_2.EJSON; } });
+ var timestamp_2 = timestamp;
+ Object.defineProperty(exports, "LongWithoutOverridesClass", { enumerable: true, get: function () { return timestamp_2.LongWithoutOverridesClass; } });
+ /** @internal */
+ // Default Max Size
+ var MAXSIZE = 1024 * 1024 * 17;
+ // Current Internal Temporary Serialization Buffer
+ var buffer$1 = buffer.Buffer.alloc(MAXSIZE);
+ /**
+ * Sets the size of the internal serialization buffer.
+ *
+ * @param size - The desired size for the internal serialization buffer
+ * @public
+ */
+ function setInternalBufferSize(size) {
+ // Resize the internal serialization buffer if needed
+ if (buffer$1.length < size) {
+ buffer$1 = buffer.Buffer.alloc(size);
+ }
+ }
+ exports.setInternalBufferSize = setInternalBufferSize;
+ /**
+ * Serialize a Javascript object.
+ *
+ * @param object - the Javascript object to serialize.
+ * @returns Buffer object containing the serialized object.
+ * @public
+ */
+ function serialize(object, options) {
+ if (options === void 0) { options = {}; }
+ // Unpack the options
+ var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ var minInternalBufferSize = typeof options.minInternalBufferSize === 'number' ? options.minInternalBufferSize : MAXSIZE;
+ // Resize the internal serialization buffer if needed
+ if (buffer$1.length < minInternalBufferSize) {
+ buffer$1 = buffer.Buffer.alloc(minInternalBufferSize);
+ }
+ // Attempt to serialize
+ var serializationIndex = serializer.serializeInto(buffer$1, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined, []);
+ // Create the final buffer
+ var finishedBuffer = buffer.Buffer.alloc(serializationIndex);
+ // Copy into the finished buffer
+ buffer$1.copy(finishedBuffer, 0, 0, finishedBuffer.length);
+ // Return the buffer
+ return finishedBuffer;
+ }
+ exports.serialize = serialize;
+ /**
+ * Serialize a Javascript object using a predefined Buffer and index into the buffer,
+ * useful when pre-allocating the space for serialization.
+ *
+ * @param object - the Javascript object to serialize.
+ * @param finalBuffer - the Buffer you pre-allocated to store the serialized BSON object.
+ * @returns the index pointing to the last written byte in the buffer.
+ * @public
+ */
+ function serializeWithBufferAndIndex(object, finalBuffer, options) {
+ if (options === void 0) { options = {}; }
+ // Unpack the options
+ var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ var startIndex = typeof options.index === 'number' ? options.index : 0;
+ // Attempt to serialize
+ var serializationIndex = serializer.serializeInto(buffer$1, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined);
+ buffer$1.copy(finalBuffer, startIndex, 0, serializationIndex);
+ // Return the index
+ return startIndex + serializationIndex - 1;
+ }
+ exports.serializeWithBufferAndIndex = serializeWithBufferAndIndex;
+ /**
+ * Deserialize data as BSON.
+ *
+ * @param buffer - the buffer containing the serialized set of BSON documents.
+ * @returns returns the deserialized Javascript Object.
+ * @public
+ */
+ function deserialize(buffer, options) {
+ if (options === void 0) { options = {}; }
+ return deserializer.deserialize(ensure_buffer.ensureBuffer(buffer), options);
+ }
+ exports.deserialize = deserialize;
+ /**
+ * Calculate the bson size for a passed in Javascript object.
+ *
+ * @param object - the Javascript object to calculate the BSON byte size for
+ * @returns size of BSON object in bytes
+ * @public
+ */
+ function calculateObjectSize(object, options) {
+ if (options === void 0) { options = {}; }
+ options = options || {};
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ return calculate_size.calculateObjectSize(object, serializeFunctions, ignoreUndefined);
+ }
+ exports.calculateObjectSize = calculateObjectSize;
+ /**
+ * Deserialize stream data as BSON documents.
+ *
+ * @param data - the buffer containing the serialized set of BSON documents.
+ * @param startIndex - the start index in the data Buffer where the deserialization is to start.
+ * @param numberOfDocuments - number of documents to deserialize.
+ * @param documents - an array where to store the deserialized documents.
+ * @param docStartIndex - the index in the documents array from where to start inserting documents.
+ * @param options - additional options used for the deserialization.
+ * @returns next index in the buffer after deserialization **x** numbers of documents.
+ * @public
+ */
+ function deserializeStream(data, startIndex, numberOfDocuments, documents, docStartIndex, options) {
+ var internalOptions = Object.assign({ allowObjectSmallerThanBufferSize: true, index: 0 }, options);
+ var bufferData = ensure_buffer.ensureBuffer(data);
+ var index = startIndex;
+ // Loop over all documents
+ for (var i = 0; i < numberOfDocuments; i++) {
+ // Find size of the document
+ var size = bufferData[index] |
+ (bufferData[index + 1] << 8) |
+ (bufferData[index + 2] << 16) |
+ (bufferData[index + 3] << 24);
+ // Update options with index
+ internalOptions.index = index;
+ // Parse the document at this point
+ documents[docStartIndex + i] = deserializer.deserialize(bufferData, internalOptions);
+ // Adjust index by the document size
+ index = index + size;
+ }
+ // Return object containing end index of parsing and list of documents
+ return index;
+ }
+ exports.deserializeStream = deserializeStream;
+ /**
+ * BSON default export
+ * @deprecated Please use named exports
+ * @privateRemarks
+ * We want to someday deprecate the default export,
+ * so none of the new TS types are being exported on the default
+ * @public
+ */
+ var BSON = {
+ Binary: binary.Binary,
+ Code: code.Code,
+ DBRef: db_ref.DBRef,
+ Decimal128: decimal128.Decimal128,
+ Double: double_1.Double,
+ Int32: int_32.Int32,
+ Long: long_1.Long,
+ UUID: uuid.UUID,
+ Map: map.Map,
+ MaxKey: max_key.MaxKey,
+ MinKey: min_key.MinKey,
+ ObjectId: objectid.ObjectId,
+ ObjectID: objectid.ObjectId,
+ BSONRegExp: regexp.BSONRegExp,
+ BSONSymbol: symbol.BSONSymbol,
+ Timestamp: timestamp.Timestamp,
+ EJSON: extended_json.EJSON,
+ setInternalBufferSize: setInternalBufferSize,
+ serialize: serialize,
+ serializeWithBufferAndIndex: serializeWithBufferAndIndex,
+ deserialize: deserialize,
+ calculateObjectSize: calculateObjectSize,
+ deserializeStream: deserializeStream
+ };
+ exports.default = BSON;
+
+ });
+
+ var bson$1 = unwrapExports(bson);
+ var bson_1 = bson.ObjectID;
+ var bson_2 = bson.Decimal128;
+ var bson_3 = bson.BSONRegExp;
+ var bson_4 = bson.MaxKey;
+ var bson_5 = bson.MinKey;
+ var bson_6 = bson.Int32;
+ var bson_7 = bson.Double;
+ var bson_8 = bson.Timestamp;
+ var bson_9 = bson.Long;
+ var bson_10 = bson.UUID;
+ var bson_11 = bson.ObjectId;
+ var bson_12 = bson.Binary;
+ var bson_13 = bson.DBRef;
+ var bson_14 = bson.BSONSymbol;
+ var bson_15 = bson.Map;
+ var bson_16 = bson.Code;
+ var bson_17 = bson.LongWithoutOverridesClass;
+ var bson_18 = bson.EJSON;
+ var bson_19 = bson.BSON_INT64_MIN;
+ var bson_20 = bson.BSON_INT64_MAX;
+ var bson_21 = bson.BSON_INT32_MIN;
+ var bson_22 = bson.BSON_INT32_MAX;
+ var bson_23 = bson.BSON_DATA_UNDEFINED;
+ var bson_24 = bson.BSON_DATA_TIMESTAMP;
+ var bson_25 = bson.BSON_DATA_SYMBOL;
+ var bson_26 = bson.BSON_DATA_STRING;
+ var bson_27 = bson.BSON_DATA_REGEXP;
+ var bson_28 = bson.BSON_DATA_OID;
+ var bson_29 = bson.BSON_DATA_OBJECT;
+ var bson_30 = bson.BSON_DATA_NUMBER;
+ var bson_31 = bson.BSON_DATA_NULL;
+ var bson_32 = bson.BSON_DATA_MIN_KEY;
+ var bson_33 = bson.BSON_DATA_MAX_KEY;
+ var bson_34 = bson.BSON_DATA_LONG;
+ var bson_35 = bson.BSON_DATA_INT;
+ var bson_36 = bson.BSON_DATA_DECIMAL128;
+ var bson_37 = bson.BSON_DATA_DBPOINTER;
+ var bson_38 = bson.BSON_DATA_DATE;
+ var bson_39 = bson.BSON_DATA_CODE_W_SCOPE;
+ var bson_40 = bson.BSON_DATA_CODE;
+ var bson_41 = bson.BSON_DATA_BOOLEAN;
+ var bson_42 = bson.BSON_DATA_BINARY;
+ var bson_43 = bson.BSON_DATA_ARRAY;
+ var bson_44 = bson.BSON_BINARY_SUBTYPE_UUID_NEW;
+ var bson_45 = bson.BSON_BINARY_SUBTYPE_UUID;
+ var bson_46 = bson.BSON_BINARY_SUBTYPE_USER_DEFINED;
+ var bson_47 = bson.BSON_BINARY_SUBTYPE_MD5;
+ var bson_48 = bson.BSON_BINARY_SUBTYPE_FUNCTION;
+ var bson_49 = bson.BSON_BINARY_SUBTYPE_DEFAULT;
+ var bson_50 = bson.BSON_BINARY_SUBTYPE_BYTE_ARRAY;
+ var bson_51 = bson.deserializeStream;
+ var bson_52 = bson.calculateObjectSize;
+ var bson_53 = bson.deserialize;
+ var bson_54 = bson.serializeWithBufferAndIndex;
+ var bson_55 = bson.serialize;
+ var bson_56 = bson.setInternalBufferSize;
+
+ exports.BSONRegExp = bson_3;
+ exports.BSONSymbol = bson_14;
+ exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = bson_50;
+ exports.BSON_BINARY_SUBTYPE_DEFAULT = bson_49;
+ exports.BSON_BINARY_SUBTYPE_FUNCTION = bson_48;
+ exports.BSON_BINARY_SUBTYPE_MD5 = bson_47;
+ exports.BSON_BINARY_SUBTYPE_USER_DEFINED = bson_46;
+ exports.BSON_BINARY_SUBTYPE_UUID = bson_45;
+ exports.BSON_BINARY_SUBTYPE_UUID_NEW = bson_44;
+ exports.BSON_DATA_ARRAY = bson_43;
+ exports.BSON_DATA_BINARY = bson_42;
+ exports.BSON_DATA_BOOLEAN = bson_41;
+ exports.BSON_DATA_CODE = bson_40;
+ exports.BSON_DATA_CODE_W_SCOPE = bson_39;
+ exports.BSON_DATA_DATE = bson_38;
+ exports.BSON_DATA_DBPOINTER = bson_37;
+ exports.BSON_DATA_DECIMAL128 = bson_36;
+ exports.BSON_DATA_INT = bson_35;
+ exports.BSON_DATA_LONG = bson_34;
+ exports.BSON_DATA_MAX_KEY = bson_33;
+ exports.BSON_DATA_MIN_KEY = bson_32;
+ exports.BSON_DATA_NULL = bson_31;
+ exports.BSON_DATA_NUMBER = bson_30;
+ exports.BSON_DATA_OBJECT = bson_29;
+ exports.BSON_DATA_OID = bson_28;
+ exports.BSON_DATA_REGEXP = bson_27;
+ exports.BSON_DATA_STRING = bson_26;
+ exports.BSON_DATA_SYMBOL = bson_25;
+ exports.BSON_DATA_TIMESTAMP = bson_24;
+ exports.BSON_DATA_UNDEFINED = bson_23;
+ exports.BSON_INT32_MAX = bson_22;
+ exports.BSON_INT32_MIN = bson_21;
+ exports.BSON_INT64_MAX = bson_20;
+ exports.BSON_INT64_MIN = bson_19;
+ exports.Binary = bson_12;
+ exports.Code = bson_16;
+ exports.DBRef = bson_13;
+ exports.Decimal128 = bson_2;
+ exports.Double = bson_7;
+ exports.EJSON = bson_18;
+ exports.Int32 = bson_6;
+ exports.Long = bson_9;
+ exports.LongWithoutOverridesClass = bson_17;
+ exports.Map = bson_15;
+ exports.MaxKey = bson_4;
+ exports.MinKey = bson_5;
+ exports.ObjectID = bson_1;
+ exports.ObjectId = bson_11;
+ exports.Timestamp = bson_8;
+ exports.UUID = bson_10;
+ exports.calculateObjectSize = bson_52;
+ exports.default = bson$1;
+ exports.deserialize = bson_53;
+ exports.deserializeStream = bson_51;
+ exports.serialize = bson_55;
+ exports.serializeWithBufferAndIndex = bson_54;
+ exports.setInternalBufferSize = bson_56;
+
+ Object.defineProperty(exports, '__esModule', { value: true });
+
+ return exports;
+
+}({}));
+//# sourceMappingURL=bson.bundle.js.map
diff --git a/node_modules/bson/dist/bson.bundle.js.map b/node_modules/bson/dist/bson.bundle.js.map
new file mode 100644
index 0000000..3b2e142
--- /dev/null
+++ b/node_modules/bson/dist/bson.bundle.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"bson.bundle.js","sources":["../node_modules/base64-js/index.js","../node_modules/ieee754/index.js","../node_modules/buffer/index.js","../node_modules/rollup-plugin-node-builtins/src/es6/empty.js","../node_modules/process-es6/browser.js","../node_modules/rollup-plugin-node-builtins/src/es6/inherits.js","../node_modules/rollup-plugin-node-builtins/src/es6/util.js","../src/parser/utils.ts","../src/ensure_buffer.ts","../src/uuid_utils.ts","../src/uuid.ts","../src/binary.ts","../src/code.ts","../src/db_ref.ts","../src/long.ts","../src/decimal128.ts","../src/double.ts","../src/int_32.ts","../src/max_key.ts","../src/min_key.ts","../src/objectid.ts","../src/regexp.ts","../src/symbol.ts","../node_modules/tslib/tslib.es6.js","../src/timestamp.ts","../src/extended_json.ts","../src/map.ts","../src/constants.ts","../src/parser/calculate_size.ts","../src/validate_utf8.ts","../src/parser/deserializer.ts","../src/float_parser.ts","../src/parser/serializer.ts","../src/bson.ts"],"sourcesContent":["'use strict'\n\nexports.byteLength = byteLength\nexports.toByteArray = toByteArray\nexports.fromByteArray = fromByteArray\n\nvar lookup = []\nvar revLookup = []\nvar Arr = typeof Uint8Array !== 'undefined' ? Uint8Array : Array\n\nvar code = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/'\nfor (var i = 0, len = code.length; i < len; ++i) {\n lookup[i] = code[i]\n revLookup[code.charCodeAt(i)] = i\n}\n\n// Support decoding URL-safe base64 strings, as Node.js does.\n// See: https://en.wikipedia.org/wiki/Base64#URL_applications\nrevLookup['-'.charCodeAt(0)] = 62\nrevLookup['_'.charCodeAt(0)] = 63\n\nfunction getLens (b64) {\n var len = b64.length\n\n if (len % 4 > 0) {\n throw new Error('Invalid string. Length must be a multiple of 4')\n }\n\n // Trim off extra bytes after placeholder bytes are found\n // See: https://github.com/beatgammit/base64-js/issues/42\n var validLen = b64.indexOf('=')\n if (validLen === -1) validLen = len\n\n var placeHoldersLen = validLen === len\n ? 0\n : 4 - (validLen % 4)\n\n return [validLen, placeHoldersLen]\n}\n\n// base64 is 4/3 + up to two characters of the original data\nfunction byteLength (b64) {\n var lens = getLens(b64)\n var validLen = lens[0]\n var placeHoldersLen = lens[1]\n return ((validLen + placeHoldersLen) * 3 / 4) - placeHoldersLen\n}\n\nfunction _byteLength (b64, validLen, placeHoldersLen) {\n return ((validLen + placeHoldersLen) * 3 / 4) - placeHoldersLen\n}\n\nfunction toByteArray (b64) {\n var tmp\n var lens = getLens(b64)\n var validLen = lens[0]\n var placeHoldersLen = lens[1]\n\n var arr = new Arr(_byteLength(b64, validLen, placeHoldersLen))\n\n var curByte = 0\n\n // if there are placeholders, only get up to the last complete 4 chars\n var len = placeHoldersLen > 0\n ? validLen - 4\n : validLen\n\n var i\n for (i = 0; i < len; i += 4) {\n tmp =\n (revLookup[b64.charCodeAt(i)] << 18) |\n (revLookup[b64.charCodeAt(i + 1)] << 12) |\n (revLookup[b64.charCodeAt(i + 2)] << 6) |\n revLookup[b64.charCodeAt(i + 3)]\n arr[curByte++] = (tmp >> 16) & 0xFF\n arr[curByte++] = (tmp >> 8) & 0xFF\n arr[curByte++] = tmp & 0xFF\n }\n\n if (placeHoldersLen === 2) {\n tmp =\n (revLookup[b64.charCodeAt(i)] << 2) |\n (revLookup[b64.charCodeAt(i + 1)] >> 4)\n arr[curByte++] = tmp & 0xFF\n }\n\n if (placeHoldersLen === 1) {\n tmp =\n (revLookup[b64.charCodeAt(i)] << 10) |\n (revLookup[b64.charCodeAt(i + 1)] << 4) |\n (revLookup[b64.charCodeAt(i + 2)] >> 2)\n arr[curByte++] = (tmp >> 8) & 0xFF\n arr[curByte++] = tmp & 0xFF\n }\n\n return arr\n}\n\nfunction tripletToBase64 (num) {\n return lookup[num >> 18 & 0x3F] +\n lookup[num >> 12 & 0x3F] +\n lookup[num >> 6 & 0x3F] +\n lookup[num & 0x3F]\n}\n\nfunction encodeChunk (uint8, start, end) {\n var tmp\n var output = []\n for (var i = start; i < end; i += 3) {\n tmp =\n ((uint8[i] << 16) & 0xFF0000) +\n ((uint8[i + 1] << 8) & 0xFF00) +\n (uint8[i + 2] & 0xFF)\n output.push(tripletToBase64(tmp))\n }\n return output.join('')\n}\n\nfunction fromByteArray (uint8) {\n var tmp\n var len = uint8.length\n var extraBytes = len % 3 // if we have 1 byte left, pad 2 bytes\n var parts = []\n var maxChunkLength = 16383 // must be multiple of 3\n\n // go through the array every three bytes, we'll deal with trailing stuff later\n for (var i = 0, len2 = len - extraBytes; i < len2; i += maxChunkLength) {\n parts.push(encodeChunk(uint8, i, (i + maxChunkLength) > len2 ? len2 : (i + maxChunkLength)))\n }\n\n // pad the end with zeros, but make sure to not forget the extra bytes\n if (extraBytes === 1) {\n tmp = uint8[len - 1]\n parts.push(\n lookup[tmp >> 2] +\n lookup[(tmp << 4) & 0x3F] +\n '=='\n )\n } else if (extraBytes === 2) {\n tmp = (uint8[len - 2] << 8) + uint8[len - 1]\n parts.push(\n lookup[tmp >> 10] +\n lookup[(tmp >> 4) & 0x3F] +\n lookup[(tmp << 2) & 0x3F] +\n '='\n )\n }\n\n return parts.join('')\n}\n","/*! ieee754. BSD-3-Clause License. Feross Aboukhadijeh */\nexports.read = function (buffer, offset, isLE, mLen, nBytes) {\n var e, m\n var eLen = (nBytes * 8) - mLen - 1\n var eMax = (1 << eLen) - 1\n var eBias = eMax >> 1\n var nBits = -7\n var i = isLE ? (nBytes - 1) : 0\n var d = isLE ? -1 : 1\n var s = buffer[offset + i]\n\n i += d\n\n e = s & ((1 << (-nBits)) - 1)\n s >>= (-nBits)\n nBits += eLen\n for (; nBits > 0; e = (e * 256) + buffer[offset + i], i += d, nBits -= 8) {}\n\n m = e & ((1 << (-nBits)) - 1)\n e >>= (-nBits)\n nBits += mLen\n for (; nBits > 0; m = (m * 256) + buffer[offset + i], i += d, nBits -= 8) {}\n\n if (e === 0) {\n e = 1 - eBias\n } else if (e === eMax) {\n return m ? NaN : ((s ? -1 : 1) * Infinity)\n } else {\n m = m + Math.pow(2, mLen)\n e = e - eBias\n }\n return (s ? -1 : 1) * m * Math.pow(2, e - mLen)\n}\n\nexports.write = function (buffer, value, offset, isLE, mLen, nBytes) {\n var e, m, c\n var eLen = (nBytes * 8) - mLen - 1\n var eMax = (1 << eLen) - 1\n var eBias = eMax >> 1\n var rt = (mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0)\n var i = isLE ? 0 : (nBytes - 1)\n var d = isLE ? 1 : -1\n var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0\n\n value = Math.abs(value)\n\n if (isNaN(value) || value === Infinity) {\n m = isNaN(value) ? 1 : 0\n e = eMax\n } else {\n e = Math.floor(Math.log(value) / Math.LN2)\n if (value * (c = Math.pow(2, -e)) < 1) {\n e--\n c *= 2\n }\n if (e + eBias >= 1) {\n value += rt / c\n } else {\n value += rt * Math.pow(2, 1 - eBias)\n }\n if (value * c >= 2) {\n e++\n c /= 2\n }\n\n if (e + eBias >= eMax) {\n m = 0\n e = eMax\n } else if (e + eBias >= 1) {\n m = ((value * c) - 1) * Math.pow(2, mLen)\n e = e + eBias\n } else {\n m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen)\n e = 0\n }\n }\n\n for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}\n\n e = (e << mLen) | m\n eLen += mLen\n for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}\n\n buffer[offset + i - d] |= s * 128\n}\n","/*!\n * The buffer module from node.js, for the browser.\n *\n * @author Feross Aboukhadijeh \n * @license MIT\n */\n/* eslint-disable no-proto */\n\n'use strict'\n\nvar base64 = require('base64-js')\nvar ieee754 = require('ieee754')\nvar customInspectSymbol =\n (typeof Symbol === 'function' && typeof Symbol['for'] === 'function') // eslint-disable-line dot-notation\n ? Symbol['for']('nodejs.util.inspect.custom') // eslint-disable-line dot-notation\n : null\n\nexports.Buffer = Buffer\nexports.SlowBuffer = SlowBuffer\nexports.INSPECT_MAX_BYTES = 50\n\nvar K_MAX_LENGTH = 0x7fffffff\nexports.kMaxLength = K_MAX_LENGTH\n\n/**\n * If `Buffer.TYPED_ARRAY_SUPPORT`:\n * === true Use Uint8Array implementation (fastest)\n * === false Print warning and recommend using `buffer` v4.x which has an Object\n * implementation (most compatible, even IE6)\n *\n * Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,\n * Opera 11.6+, iOS 4.2+.\n *\n * We report that the browser does not support typed arrays if the are not subclassable\n * using __proto__. Firefox 4-29 lacks support for adding new properties to `Uint8Array`\n * (See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438). IE 10 lacks support\n * for __proto__ and has a buggy typed array implementation.\n */\nBuffer.TYPED_ARRAY_SUPPORT = typedArraySupport()\n\nif (!Buffer.TYPED_ARRAY_SUPPORT && typeof console !== 'undefined' &&\n typeof console.error === 'function') {\n console.error(\n 'This browser lacks typed array (Uint8Array) support which is required by ' +\n '`buffer` v5.x. Use `buffer` v4.x if you require old browser support.'\n )\n}\n\nfunction typedArraySupport () {\n // Can typed array instances can be augmented?\n try {\n var arr = new Uint8Array(1)\n var proto = { foo: function () { return 42 } }\n Object.setPrototypeOf(proto, Uint8Array.prototype)\n Object.setPrototypeOf(arr, proto)\n return arr.foo() === 42\n } catch (e) {\n return false\n }\n}\n\nObject.defineProperty(Buffer.prototype, 'parent', {\n enumerable: true,\n get: function () {\n if (!Buffer.isBuffer(this)) return undefined\n return this.buffer\n }\n})\n\nObject.defineProperty(Buffer.prototype, 'offset', {\n enumerable: true,\n get: function () {\n if (!Buffer.isBuffer(this)) return undefined\n return this.byteOffset\n }\n})\n\nfunction createBuffer (length) {\n if (length > K_MAX_LENGTH) {\n throw new RangeError('The value \"' + length + '\" is invalid for option \"size\"')\n }\n // Return an augmented `Uint8Array` instance\n var buf = new Uint8Array(length)\n Object.setPrototypeOf(buf, Buffer.prototype)\n return buf\n}\n\n/**\n * The Buffer constructor returns instances of `Uint8Array` that have their\n * prototype changed to `Buffer.prototype`. Furthermore, `Buffer` is a subclass of\n * `Uint8Array`, so the returned instances will have all the node `Buffer` methods\n * and the `Uint8Array` methods. Square bracket notation works as expected -- it\n * returns a single octet.\n *\n * The `Uint8Array` prototype remains unmodified.\n */\n\nfunction Buffer (arg, encodingOrOffset, length) {\n // Common case.\n if (typeof arg === 'number') {\n if (typeof encodingOrOffset === 'string') {\n throw new TypeError(\n 'The \"string\" argument must be of type string. Received type number'\n )\n }\n return allocUnsafe(arg)\n }\n return from(arg, encodingOrOffset, length)\n}\n\nBuffer.poolSize = 8192 // not used by this implementation\n\nfunction from (value, encodingOrOffset, length) {\n if (typeof value === 'string') {\n return fromString(value, encodingOrOffset)\n }\n\n if (ArrayBuffer.isView(value)) {\n return fromArrayView(value)\n }\n\n if (value == null) {\n throw new TypeError(\n 'The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' +\n 'or Array-like Object. Received type ' + (typeof value)\n )\n }\n\n if (isInstance(value, ArrayBuffer) ||\n (value && isInstance(value.buffer, ArrayBuffer))) {\n return fromArrayBuffer(value, encodingOrOffset, length)\n }\n\n if (typeof SharedArrayBuffer !== 'undefined' &&\n (isInstance(value, SharedArrayBuffer) ||\n (value && isInstance(value.buffer, SharedArrayBuffer)))) {\n return fromArrayBuffer(value, encodingOrOffset, length)\n }\n\n if (typeof value === 'number') {\n throw new TypeError(\n 'The \"value\" argument must not be of type number. Received type number'\n )\n }\n\n var valueOf = value.valueOf && value.valueOf()\n if (valueOf != null && valueOf !== value) {\n return Buffer.from(valueOf, encodingOrOffset, length)\n }\n\n var b = fromObject(value)\n if (b) return b\n\n if (typeof Symbol !== 'undefined' && Symbol.toPrimitive != null &&\n typeof value[Symbol.toPrimitive] === 'function') {\n return Buffer.from(\n value[Symbol.toPrimitive]('string'), encodingOrOffset, length\n )\n }\n\n throw new TypeError(\n 'The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' +\n 'or Array-like Object. Received type ' + (typeof value)\n )\n}\n\n/**\n * Functionally equivalent to Buffer(arg, encoding) but throws a TypeError\n * if value is a number.\n * Buffer.from(str[, encoding])\n * Buffer.from(array)\n * Buffer.from(buffer)\n * Buffer.from(arrayBuffer[, byteOffset[, length]])\n **/\nBuffer.from = function (value, encodingOrOffset, length) {\n return from(value, encodingOrOffset, length)\n}\n\n// Note: Change prototype *after* Buffer.from is defined to workaround Chrome bug:\n// https://github.com/feross/buffer/pull/148\nObject.setPrototypeOf(Buffer.prototype, Uint8Array.prototype)\nObject.setPrototypeOf(Buffer, Uint8Array)\n\nfunction assertSize (size) {\n if (typeof size !== 'number') {\n throw new TypeError('\"size\" argument must be of type number')\n } else if (size < 0) {\n throw new RangeError('The value \"' + size + '\" is invalid for option \"size\"')\n }\n}\n\nfunction alloc (size, fill, encoding) {\n assertSize(size)\n if (size <= 0) {\n return createBuffer(size)\n }\n if (fill !== undefined) {\n // Only pay attention to encoding if it's a string. This\n // prevents accidentally sending in a number that would\n // be interpreted as a start offset.\n return typeof encoding === 'string'\n ? createBuffer(size).fill(fill, encoding)\n : createBuffer(size).fill(fill)\n }\n return createBuffer(size)\n}\n\n/**\n * Creates a new filled Buffer instance.\n * alloc(size[, fill[, encoding]])\n **/\nBuffer.alloc = function (size, fill, encoding) {\n return alloc(size, fill, encoding)\n}\n\nfunction allocUnsafe (size) {\n assertSize(size)\n return createBuffer(size < 0 ? 0 : checked(size) | 0)\n}\n\n/**\n * Equivalent to Buffer(num), by default creates a non-zero-filled Buffer instance.\n * */\nBuffer.allocUnsafe = function (size) {\n return allocUnsafe(size)\n}\n/**\n * Equivalent to SlowBuffer(num), by default creates a non-zero-filled Buffer instance.\n */\nBuffer.allocUnsafeSlow = function (size) {\n return allocUnsafe(size)\n}\n\nfunction fromString (string, encoding) {\n if (typeof encoding !== 'string' || encoding === '') {\n encoding = 'utf8'\n }\n\n if (!Buffer.isEncoding(encoding)) {\n throw new TypeError('Unknown encoding: ' + encoding)\n }\n\n var length = byteLength(string, encoding) | 0\n var buf = createBuffer(length)\n\n var actual = buf.write(string, encoding)\n\n if (actual !== length) {\n // Writing a hex string, for example, that contains invalid characters will\n // cause everything after the first invalid character to be ignored. (e.g.\n // 'abxxcd' will be treated as 'ab')\n buf = buf.slice(0, actual)\n }\n\n return buf\n}\n\nfunction fromArrayLike (array) {\n var length = array.length < 0 ? 0 : checked(array.length) | 0\n var buf = createBuffer(length)\n for (var i = 0; i < length; i += 1) {\n buf[i] = array[i] & 255\n }\n return buf\n}\n\nfunction fromArrayView (arrayView) {\n if (isInstance(arrayView, Uint8Array)) {\n var copy = new Uint8Array(arrayView)\n return fromArrayBuffer(copy.buffer, copy.byteOffset, copy.byteLength)\n }\n return fromArrayLike(arrayView)\n}\n\nfunction fromArrayBuffer (array, byteOffset, length) {\n if (byteOffset < 0 || array.byteLength < byteOffset) {\n throw new RangeError('\"offset\" is outside of buffer bounds')\n }\n\n if (array.byteLength < byteOffset + (length || 0)) {\n throw new RangeError('\"length\" is outside of buffer bounds')\n }\n\n var buf\n if (byteOffset === undefined && length === undefined) {\n buf = new Uint8Array(array)\n } else if (length === undefined) {\n buf = new Uint8Array(array, byteOffset)\n } else {\n buf = new Uint8Array(array, byteOffset, length)\n }\n\n // Return an augmented `Uint8Array` instance\n Object.setPrototypeOf(buf, Buffer.prototype)\n\n return buf\n}\n\nfunction fromObject (obj) {\n if (Buffer.isBuffer(obj)) {\n var len = checked(obj.length) | 0\n var buf = createBuffer(len)\n\n if (buf.length === 0) {\n return buf\n }\n\n obj.copy(buf, 0, 0, len)\n return buf\n }\n\n if (obj.length !== undefined) {\n if (typeof obj.length !== 'number' || numberIsNaN(obj.length)) {\n return createBuffer(0)\n }\n return fromArrayLike(obj)\n }\n\n if (obj.type === 'Buffer' && Array.isArray(obj.data)) {\n return fromArrayLike(obj.data)\n }\n}\n\nfunction checked (length) {\n // Note: cannot use `length < K_MAX_LENGTH` here because that fails when\n // length is NaN (which is otherwise coerced to zero.)\n if (length >= K_MAX_LENGTH) {\n throw new RangeError('Attempt to allocate Buffer larger than maximum ' +\n 'size: 0x' + K_MAX_LENGTH.toString(16) + ' bytes')\n }\n return length | 0\n}\n\nfunction SlowBuffer (length) {\n if (+length != length) { // eslint-disable-line eqeqeq\n length = 0\n }\n return Buffer.alloc(+length)\n}\n\nBuffer.isBuffer = function isBuffer (b) {\n return b != null && b._isBuffer === true &&\n b !== Buffer.prototype // so Buffer.isBuffer(Buffer.prototype) will be false\n}\n\nBuffer.compare = function compare (a, b) {\n if (isInstance(a, Uint8Array)) a = Buffer.from(a, a.offset, a.byteLength)\n if (isInstance(b, Uint8Array)) b = Buffer.from(b, b.offset, b.byteLength)\n if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {\n throw new TypeError(\n 'The \"buf1\", \"buf2\" arguments must be one of type Buffer or Uint8Array'\n )\n }\n\n if (a === b) return 0\n\n var x = a.length\n var y = b.length\n\n for (var i = 0, len = Math.min(x, y); i < len; ++i) {\n if (a[i] !== b[i]) {\n x = a[i]\n y = b[i]\n break\n }\n }\n\n if (x < y) return -1\n if (y < x) return 1\n return 0\n}\n\nBuffer.isEncoding = function isEncoding (encoding) {\n switch (String(encoding).toLowerCase()) {\n case 'hex':\n case 'utf8':\n case 'utf-8':\n case 'ascii':\n case 'latin1':\n case 'binary':\n case 'base64':\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return true\n default:\n return false\n }\n}\n\nBuffer.concat = function concat (list, length) {\n if (!Array.isArray(list)) {\n throw new TypeError('\"list\" argument must be an Array of Buffers')\n }\n\n if (list.length === 0) {\n return Buffer.alloc(0)\n }\n\n var i\n if (length === undefined) {\n length = 0\n for (i = 0; i < list.length; ++i) {\n length += list[i].length\n }\n }\n\n var buffer = Buffer.allocUnsafe(length)\n var pos = 0\n for (i = 0; i < list.length; ++i) {\n var buf = list[i]\n if (isInstance(buf, Uint8Array)) {\n if (pos + buf.length > buffer.length) {\n Buffer.from(buf).copy(buffer, pos)\n } else {\n Uint8Array.prototype.set.call(\n buffer,\n buf,\n pos\n )\n }\n } else if (!Buffer.isBuffer(buf)) {\n throw new TypeError('\"list\" argument must be an Array of Buffers')\n } else {\n buf.copy(buffer, pos)\n }\n pos += buf.length\n }\n return buffer\n}\n\nfunction byteLength (string, encoding) {\n if (Buffer.isBuffer(string)) {\n return string.length\n }\n if (ArrayBuffer.isView(string) || isInstance(string, ArrayBuffer)) {\n return string.byteLength\n }\n if (typeof string !== 'string') {\n throw new TypeError(\n 'The \"string\" argument must be one of type string, Buffer, or ArrayBuffer. ' +\n 'Received type ' + typeof string\n )\n }\n\n var len = string.length\n var mustMatch = (arguments.length > 2 && arguments[2] === true)\n if (!mustMatch && len === 0) return 0\n\n // Use a for loop to avoid recursion\n var loweredCase = false\n for (;;) {\n switch (encoding) {\n case 'ascii':\n case 'latin1':\n case 'binary':\n return len\n case 'utf8':\n case 'utf-8':\n return utf8ToBytes(string).length\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return len * 2\n case 'hex':\n return len >>> 1\n case 'base64':\n return base64ToBytes(string).length\n default:\n if (loweredCase) {\n return mustMatch ? -1 : utf8ToBytes(string).length // assume utf8\n }\n encoding = ('' + encoding).toLowerCase()\n loweredCase = true\n }\n }\n}\nBuffer.byteLength = byteLength\n\nfunction slowToString (encoding, start, end) {\n var loweredCase = false\n\n // No need to verify that \"this.length <= MAX_UINT32\" since it's a read-only\n // property of a typed array.\n\n // This behaves neither like String nor Uint8Array in that we set start/end\n // to their upper/lower bounds if the value passed is out of range.\n // undefined is handled specially as per ECMA-262 6th Edition,\n // Section 13.3.3.7 Runtime Semantics: KeyedBindingInitialization.\n if (start === undefined || start < 0) {\n start = 0\n }\n // Return early if start > this.length. Done here to prevent potential uint32\n // coercion fail below.\n if (start > this.length) {\n return ''\n }\n\n if (end === undefined || end > this.length) {\n end = this.length\n }\n\n if (end <= 0) {\n return ''\n }\n\n // Force coercion to uint32. This will also coerce falsey/NaN values to 0.\n end >>>= 0\n start >>>= 0\n\n if (end <= start) {\n return ''\n }\n\n if (!encoding) encoding = 'utf8'\n\n while (true) {\n switch (encoding) {\n case 'hex':\n return hexSlice(this, start, end)\n\n case 'utf8':\n case 'utf-8':\n return utf8Slice(this, start, end)\n\n case 'ascii':\n return asciiSlice(this, start, end)\n\n case 'latin1':\n case 'binary':\n return latin1Slice(this, start, end)\n\n case 'base64':\n return base64Slice(this, start, end)\n\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return utf16leSlice(this, start, end)\n\n default:\n if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)\n encoding = (encoding + '').toLowerCase()\n loweredCase = true\n }\n }\n}\n\n// This property is used by `Buffer.isBuffer` (and the `is-buffer` npm package)\n// to detect a Buffer instance. It's not possible to use `instanceof Buffer`\n// reliably in a browserify context because there could be multiple different\n// copies of the 'buffer' package in use. This method works even for Buffer\n// instances that were created from another copy of the `buffer` package.\n// See: https://github.com/feross/buffer/issues/154\nBuffer.prototype._isBuffer = true\n\nfunction swap (b, n, m) {\n var i = b[n]\n b[n] = b[m]\n b[m] = i\n}\n\nBuffer.prototype.swap16 = function swap16 () {\n var len = this.length\n if (len % 2 !== 0) {\n throw new RangeError('Buffer size must be a multiple of 16-bits')\n }\n for (var i = 0; i < len; i += 2) {\n swap(this, i, i + 1)\n }\n return this\n}\n\nBuffer.prototype.swap32 = function swap32 () {\n var len = this.length\n if (len % 4 !== 0) {\n throw new RangeError('Buffer size must be a multiple of 32-bits')\n }\n for (var i = 0; i < len; i += 4) {\n swap(this, i, i + 3)\n swap(this, i + 1, i + 2)\n }\n return this\n}\n\nBuffer.prototype.swap64 = function swap64 () {\n var len = this.length\n if (len % 8 !== 0) {\n throw new RangeError('Buffer size must be a multiple of 64-bits')\n }\n for (var i = 0; i < len; i += 8) {\n swap(this, i, i + 7)\n swap(this, i + 1, i + 6)\n swap(this, i + 2, i + 5)\n swap(this, i + 3, i + 4)\n }\n return this\n}\n\nBuffer.prototype.toString = function toString () {\n var length = this.length\n if (length === 0) return ''\n if (arguments.length === 0) return utf8Slice(this, 0, length)\n return slowToString.apply(this, arguments)\n}\n\nBuffer.prototype.toLocaleString = Buffer.prototype.toString\n\nBuffer.prototype.equals = function equals (b) {\n if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer')\n if (this === b) return true\n return Buffer.compare(this, b) === 0\n}\n\nBuffer.prototype.inspect = function inspect () {\n var str = ''\n var max = exports.INSPECT_MAX_BYTES\n str = this.toString('hex', 0, max).replace(/(.{2})/g, '$1 ').trim()\n if (this.length > max) str += ' ... '\n return ''\n}\nif (customInspectSymbol) {\n Buffer.prototype[customInspectSymbol] = Buffer.prototype.inspect\n}\n\nBuffer.prototype.compare = function compare (target, start, end, thisStart, thisEnd) {\n if (isInstance(target, Uint8Array)) {\n target = Buffer.from(target, target.offset, target.byteLength)\n }\n if (!Buffer.isBuffer(target)) {\n throw new TypeError(\n 'The \"target\" argument must be one of type Buffer or Uint8Array. ' +\n 'Received type ' + (typeof target)\n )\n }\n\n if (start === undefined) {\n start = 0\n }\n if (end === undefined) {\n end = target ? target.length : 0\n }\n if (thisStart === undefined) {\n thisStart = 0\n }\n if (thisEnd === undefined) {\n thisEnd = this.length\n }\n\n if (start < 0 || end > target.length || thisStart < 0 || thisEnd > this.length) {\n throw new RangeError('out of range index')\n }\n\n if (thisStart >= thisEnd && start >= end) {\n return 0\n }\n if (thisStart >= thisEnd) {\n return -1\n }\n if (start >= end) {\n return 1\n }\n\n start >>>= 0\n end >>>= 0\n thisStart >>>= 0\n thisEnd >>>= 0\n\n if (this === target) return 0\n\n var x = thisEnd - thisStart\n var y = end - start\n var len = Math.min(x, y)\n\n var thisCopy = this.slice(thisStart, thisEnd)\n var targetCopy = target.slice(start, end)\n\n for (var i = 0; i < len; ++i) {\n if (thisCopy[i] !== targetCopy[i]) {\n x = thisCopy[i]\n y = targetCopy[i]\n break\n }\n }\n\n if (x < y) return -1\n if (y < x) return 1\n return 0\n}\n\n// Finds either the first index of `val` in `buffer` at offset >= `byteOffset`,\n// OR the last index of `val` in `buffer` at offset <= `byteOffset`.\n//\n// Arguments:\n// - buffer - a Buffer to search\n// - val - a string, Buffer, or number\n// - byteOffset - an index into `buffer`; will be clamped to an int32\n// - encoding - an optional encoding, relevant is val is a string\n// - dir - true for indexOf, false for lastIndexOf\nfunction bidirectionalIndexOf (buffer, val, byteOffset, encoding, dir) {\n // Empty buffer means no match\n if (buffer.length === 0) return -1\n\n // Normalize byteOffset\n if (typeof byteOffset === 'string') {\n encoding = byteOffset\n byteOffset = 0\n } else if (byteOffset > 0x7fffffff) {\n byteOffset = 0x7fffffff\n } else if (byteOffset < -0x80000000) {\n byteOffset = -0x80000000\n }\n byteOffset = +byteOffset // Coerce to Number.\n if (numberIsNaN(byteOffset)) {\n // byteOffset: it it's undefined, null, NaN, \"foo\", etc, search whole buffer\n byteOffset = dir ? 0 : (buffer.length - 1)\n }\n\n // Normalize byteOffset: negative offsets start from the end of the buffer\n if (byteOffset < 0) byteOffset = buffer.length + byteOffset\n if (byteOffset >= buffer.length) {\n if (dir) return -1\n else byteOffset = buffer.length - 1\n } else if (byteOffset < 0) {\n if (dir) byteOffset = 0\n else return -1\n }\n\n // Normalize val\n if (typeof val === 'string') {\n val = Buffer.from(val, encoding)\n }\n\n // Finally, search either indexOf (if dir is true) or lastIndexOf\n if (Buffer.isBuffer(val)) {\n // Special case: looking for empty string/buffer always fails\n if (val.length === 0) {\n return -1\n }\n return arrayIndexOf(buffer, val, byteOffset, encoding, dir)\n } else if (typeof val === 'number') {\n val = val & 0xFF // Search for a byte value [0-255]\n if (typeof Uint8Array.prototype.indexOf === 'function') {\n if (dir) {\n return Uint8Array.prototype.indexOf.call(buffer, val, byteOffset)\n } else {\n return Uint8Array.prototype.lastIndexOf.call(buffer, val, byteOffset)\n }\n }\n return arrayIndexOf(buffer, [val], byteOffset, encoding, dir)\n }\n\n throw new TypeError('val must be string, number or Buffer')\n}\n\nfunction arrayIndexOf (arr, val, byteOffset, encoding, dir) {\n var indexSize = 1\n var arrLength = arr.length\n var valLength = val.length\n\n if (encoding !== undefined) {\n encoding = String(encoding).toLowerCase()\n if (encoding === 'ucs2' || encoding === 'ucs-2' ||\n encoding === 'utf16le' || encoding === 'utf-16le') {\n if (arr.length < 2 || val.length < 2) {\n return -1\n }\n indexSize = 2\n arrLength /= 2\n valLength /= 2\n byteOffset /= 2\n }\n }\n\n function read (buf, i) {\n if (indexSize === 1) {\n return buf[i]\n } else {\n return buf.readUInt16BE(i * indexSize)\n }\n }\n\n var i\n if (dir) {\n var foundIndex = -1\n for (i = byteOffset; i < arrLength; i++) {\n if (read(arr, i) === read(val, foundIndex === -1 ? 0 : i - foundIndex)) {\n if (foundIndex === -1) foundIndex = i\n if (i - foundIndex + 1 === valLength) return foundIndex * indexSize\n } else {\n if (foundIndex !== -1) i -= i - foundIndex\n foundIndex = -1\n }\n }\n } else {\n if (byteOffset + valLength > arrLength) byteOffset = arrLength - valLength\n for (i = byteOffset; i >= 0; i--) {\n var found = true\n for (var j = 0; j < valLength; j++) {\n if (read(arr, i + j) !== read(val, j)) {\n found = false\n break\n }\n }\n if (found) return i\n }\n }\n\n return -1\n}\n\nBuffer.prototype.includes = function includes (val, byteOffset, encoding) {\n return this.indexOf(val, byteOffset, encoding) !== -1\n}\n\nBuffer.prototype.indexOf = function indexOf (val, byteOffset, encoding) {\n return bidirectionalIndexOf(this, val, byteOffset, encoding, true)\n}\n\nBuffer.prototype.lastIndexOf = function lastIndexOf (val, byteOffset, encoding) {\n return bidirectionalIndexOf(this, val, byteOffset, encoding, false)\n}\n\nfunction hexWrite (buf, string, offset, length) {\n offset = Number(offset) || 0\n var remaining = buf.length - offset\n if (!length) {\n length = remaining\n } else {\n length = Number(length)\n if (length > remaining) {\n length = remaining\n }\n }\n\n var strLen = string.length\n\n if (length > strLen / 2) {\n length = strLen / 2\n }\n for (var i = 0; i < length; ++i) {\n var parsed = parseInt(string.substr(i * 2, 2), 16)\n if (numberIsNaN(parsed)) return i\n buf[offset + i] = parsed\n }\n return i\n}\n\nfunction utf8Write (buf, string, offset, length) {\n return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length)\n}\n\nfunction asciiWrite (buf, string, offset, length) {\n return blitBuffer(asciiToBytes(string), buf, offset, length)\n}\n\nfunction base64Write (buf, string, offset, length) {\n return blitBuffer(base64ToBytes(string), buf, offset, length)\n}\n\nfunction ucs2Write (buf, string, offset, length) {\n return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length)\n}\n\nBuffer.prototype.write = function write (string, offset, length, encoding) {\n // Buffer#write(string)\n if (offset === undefined) {\n encoding = 'utf8'\n length = this.length\n offset = 0\n // Buffer#write(string, encoding)\n } else if (length === undefined && typeof offset === 'string') {\n encoding = offset\n length = this.length\n offset = 0\n // Buffer#write(string, offset[, length][, encoding])\n } else if (isFinite(offset)) {\n offset = offset >>> 0\n if (isFinite(length)) {\n length = length >>> 0\n if (encoding === undefined) encoding = 'utf8'\n } else {\n encoding = length\n length = undefined\n }\n } else {\n throw new Error(\n 'Buffer.write(string, encoding, offset[, length]) is no longer supported'\n )\n }\n\n var remaining = this.length - offset\n if (length === undefined || length > remaining) length = remaining\n\n if ((string.length > 0 && (length < 0 || offset < 0)) || offset > this.length) {\n throw new RangeError('Attempt to write outside buffer bounds')\n }\n\n if (!encoding) encoding = 'utf8'\n\n var loweredCase = false\n for (;;) {\n switch (encoding) {\n case 'hex':\n return hexWrite(this, string, offset, length)\n\n case 'utf8':\n case 'utf-8':\n return utf8Write(this, string, offset, length)\n\n case 'ascii':\n case 'latin1':\n case 'binary':\n return asciiWrite(this, string, offset, length)\n\n case 'base64':\n // Warning: maxLength not taken into account in base64Write\n return base64Write(this, string, offset, length)\n\n case 'ucs2':\n case 'ucs-2':\n case 'utf16le':\n case 'utf-16le':\n return ucs2Write(this, string, offset, length)\n\n default:\n if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)\n encoding = ('' + encoding).toLowerCase()\n loweredCase = true\n }\n }\n}\n\nBuffer.prototype.toJSON = function toJSON () {\n return {\n type: 'Buffer',\n data: Array.prototype.slice.call(this._arr || this, 0)\n }\n}\n\nfunction base64Slice (buf, start, end) {\n if (start === 0 && end === buf.length) {\n return base64.fromByteArray(buf)\n } else {\n return base64.fromByteArray(buf.slice(start, end))\n }\n}\n\nfunction utf8Slice (buf, start, end) {\n end = Math.min(buf.length, end)\n var res = []\n\n var i = start\n while (i < end) {\n var firstByte = buf[i]\n var codePoint = null\n var bytesPerSequence = (firstByte > 0xEF)\n ? 4\n : (firstByte > 0xDF)\n ? 3\n : (firstByte > 0xBF)\n ? 2\n : 1\n\n if (i + bytesPerSequence <= end) {\n var secondByte, thirdByte, fourthByte, tempCodePoint\n\n switch (bytesPerSequence) {\n case 1:\n if (firstByte < 0x80) {\n codePoint = firstByte\n }\n break\n case 2:\n secondByte = buf[i + 1]\n if ((secondByte & 0xC0) === 0x80) {\n tempCodePoint = (firstByte & 0x1F) << 0x6 | (secondByte & 0x3F)\n if (tempCodePoint > 0x7F) {\n codePoint = tempCodePoint\n }\n }\n break\n case 3:\n secondByte = buf[i + 1]\n thirdByte = buf[i + 2]\n if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {\n tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | (thirdByte & 0x3F)\n if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {\n codePoint = tempCodePoint\n }\n }\n break\n case 4:\n secondByte = buf[i + 1]\n thirdByte = buf[i + 2]\n fourthByte = buf[i + 3]\n if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {\n tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | (fourthByte & 0x3F)\n if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {\n codePoint = tempCodePoint\n }\n }\n }\n }\n\n if (codePoint === null) {\n // we did not generate a valid codePoint so insert a\n // replacement char (U+FFFD) and advance only 1 byte\n codePoint = 0xFFFD\n bytesPerSequence = 1\n } else if (codePoint > 0xFFFF) {\n // encode to utf16 (surrogate pair dance)\n codePoint -= 0x10000\n res.push(codePoint >>> 10 & 0x3FF | 0xD800)\n codePoint = 0xDC00 | codePoint & 0x3FF\n }\n\n res.push(codePoint)\n i += bytesPerSequence\n }\n\n return decodeCodePointsArray(res)\n}\n\n// Based on http://stackoverflow.com/a/22747272/680742, the browser with\n// the lowest limit is Chrome, with 0x10000 args.\n// We go 1 magnitude less, for safety\nvar MAX_ARGUMENTS_LENGTH = 0x1000\n\nfunction decodeCodePointsArray (codePoints) {\n var len = codePoints.length\n if (len <= MAX_ARGUMENTS_LENGTH) {\n return String.fromCharCode.apply(String, codePoints) // avoid extra slice()\n }\n\n // Decode in chunks to avoid \"call stack size exceeded\".\n var res = ''\n var i = 0\n while (i < len) {\n res += String.fromCharCode.apply(\n String,\n codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH)\n )\n }\n return res\n}\n\nfunction asciiSlice (buf, start, end) {\n var ret = ''\n end = Math.min(buf.length, end)\n\n for (var i = start; i < end; ++i) {\n ret += String.fromCharCode(buf[i] & 0x7F)\n }\n return ret\n}\n\nfunction latin1Slice (buf, start, end) {\n var ret = ''\n end = Math.min(buf.length, end)\n\n for (var i = start; i < end; ++i) {\n ret += String.fromCharCode(buf[i])\n }\n return ret\n}\n\nfunction hexSlice (buf, start, end) {\n var len = buf.length\n\n if (!start || start < 0) start = 0\n if (!end || end < 0 || end > len) end = len\n\n var out = ''\n for (var i = start; i < end; ++i) {\n out += hexSliceLookupTable[buf[i]]\n }\n return out\n}\n\nfunction utf16leSlice (buf, start, end) {\n var bytes = buf.slice(start, end)\n var res = ''\n // If bytes.length is odd, the last 8 bits must be ignored (same as node.js)\n for (var i = 0; i < bytes.length - 1; i += 2) {\n res += String.fromCharCode(bytes[i] + (bytes[i + 1] * 256))\n }\n return res\n}\n\nBuffer.prototype.slice = function slice (start, end) {\n var len = this.length\n start = ~~start\n end = end === undefined ? len : ~~end\n\n if (start < 0) {\n start += len\n if (start < 0) start = 0\n } else if (start > len) {\n start = len\n }\n\n if (end < 0) {\n end += len\n if (end < 0) end = 0\n } else if (end > len) {\n end = len\n }\n\n if (end < start) end = start\n\n var newBuf = this.subarray(start, end)\n // Return an augmented `Uint8Array` instance\n Object.setPrototypeOf(newBuf, Buffer.prototype)\n\n return newBuf\n}\n\n/*\n * Need to make sure that buffer isn't trying to write out of bounds.\n */\nfunction checkOffset (offset, ext, length) {\n if ((offset % 1) !== 0 || offset < 0) throw new RangeError('offset is not uint')\n if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length')\n}\n\nBuffer.prototype.readUintLE =\nBuffer.prototype.readUIntLE = function readUIntLE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) checkOffset(offset, byteLength, this.length)\n\n var val = this[offset]\n var mul = 1\n var i = 0\n while (++i < byteLength && (mul *= 0x100)) {\n val += this[offset + i] * mul\n }\n\n return val\n}\n\nBuffer.prototype.readUintBE =\nBuffer.prototype.readUIntBE = function readUIntBE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) {\n checkOffset(offset, byteLength, this.length)\n }\n\n var val = this[offset + --byteLength]\n var mul = 1\n while (byteLength > 0 && (mul *= 0x100)) {\n val += this[offset + --byteLength] * mul\n }\n\n return val\n}\n\nBuffer.prototype.readUint8 =\nBuffer.prototype.readUInt8 = function readUInt8 (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 1, this.length)\n return this[offset]\n}\n\nBuffer.prototype.readUint16LE =\nBuffer.prototype.readUInt16LE = function readUInt16LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n return this[offset] | (this[offset + 1] << 8)\n}\n\nBuffer.prototype.readUint16BE =\nBuffer.prototype.readUInt16BE = function readUInt16BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n return (this[offset] << 8) | this[offset + 1]\n}\n\nBuffer.prototype.readUint32LE =\nBuffer.prototype.readUInt32LE = function readUInt32LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return ((this[offset]) |\n (this[offset + 1] << 8) |\n (this[offset + 2] << 16)) +\n (this[offset + 3] * 0x1000000)\n}\n\nBuffer.prototype.readUint32BE =\nBuffer.prototype.readUInt32BE = function readUInt32BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return (this[offset] * 0x1000000) +\n ((this[offset + 1] << 16) |\n (this[offset + 2] << 8) |\n this[offset + 3])\n}\n\nBuffer.prototype.readIntLE = function readIntLE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) checkOffset(offset, byteLength, this.length)\n\n var val = this[offset]\n var mul = 1\n var i = 0\n while (++i < byteLength && (mul *= 0x100)) {\n val += this[offset + i] * mul\n }\n mul *= 0x80\n\n if (val >= mul) val -= Math.pow(2, 8 * byteLength)\n\n return val\n}\n\nBuffer.prototype.readIntBE = function readIntBE (offset, byteLength, noAssert) {\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) checkOffset(offset, byteLength, this.length)\n\n var i = byteLength\n var mul = 1\n var val = this[offset + --i]\n while (i > 0 && (mul *= 0x100)) {\n val += this[offset + --i] * mul\n }\n mul *= 0x80\n\n if (val >= mul) val -= Math.pow(2, 8 * byteLength)\n\n return val\n}\n\nBuffer.prototype.readInt8 = function readInt8 (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 1, this.length)\n if (!(this[offset] & 0x80)) return (this[offset])\n return ((0xff - this[offset] + 1) * -1)\n}\n\nBuffer.prototype.readInt16LE = function readInt16LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n var val = this[offset] | (this[offset + 1] << 8)\n return (val & 0x8000) ? val | 0xFFFF0000 : val\n}\n\nBuffer.prototype.readInt16BE = function readInt16BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 2, this.length)\n var val = this[offset + 1] | (this[offset] << 8)\n return (val & 0x8000) ? val | 0xFFFF0000 : val\n}\n\nBuffer.prototype.readInt32LE = function readInt32LE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return (this[offset]) |\n (this[offset + 1] << 8) |\n (this[offset + 2] << 16) |\n (this[offset + 3] << 24)\n}\n\nBuffer.prototype.readInt32BE = function readInt32BE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n\n return (this[offset] << 24) |\n (this[offset + 1] << 16) |\n (this[offset + 2] << 8) |\n (this[offset + 3])\n}\n\nBuffer.prototype.readFloatLE = function readFloatLE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n return ieee754.read(this, offset, true, 23, 4)\n}\n\nBuffer.prototype.readFloatBE = function readFloatBE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 4, this.length)\n return ieee754.read(this, offset, false, 23, 4)\n}\n\nBuffer.prototype.readDoubleLE = function readDoubleLE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 8, this.length)\n return ieee754.read(this, offset, true, 52, 8)\n}\n\nBuffer.prototype.readDoubleBE = function readDoubleBE (offset, noAssert) {\n offset = offset >>> 0\n if (!noAssert) checkOffset(offset, 8, this.length)\n return ieee754.read(this, offset, false, 52, 8)\n}\n\nfunction checkInt (buf, value, offset, ext, max, min) {\n if (!Buffer.isBuffer(buf)) throw new TypeError('\"buffer\" argument must be a Buffer instance')\n if (value > max || value < min) throw new RangeError('\"value\" argument is out of bounds')\n if (offset + ext > buf.length) throw new RangeError('Index out of range')\n}\n\nBuffer.prototype.writeUintLE =\nBuffer.prototype.writeUIntLE = function writeUIntLE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) {\n var maxBytes = Math.pow(2, 8 * byteLength) - 1\n checkInt(this, value, offset, byteLength, maxBytes, 0)\n }\n\n var mul = 1\n var i = 0\n this[offset] = value & 0xFF\n while (++i < byteLength && (mul *= 0x100)) {\n this[offset + i] = (value / mul) & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeUintBE =\nBuffer.prototype.writeUIntBE = function writeUIntBE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n byteLength = byteLength >>> 0\n if (!noAssert) {\n var maxBytes = Math.pow(2, 8 * byteLength) - 1\n checkInt(this, value, offset, byteLength, maxBytes, 0)\n }\n\n var i = byteLength - 1\n var mul = 1\n this[offset + i] = value & 0xFF\n while (--i >= 0 && (mul *= 0x100)) {\n this[offset + i] = (value / mul) & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeUint8 =\nBuffer.prototype.writeUInt8 = function writeUInt8 (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0)\n this[offset] = (value & 0xff)\n return offset + 1\n}\n\nBuffer.prototype.writeUint16LE =\nBuffer.prototype.writeUInt16LE = function writeUInt16LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)\n this[offset] = (value & 0xff)\n this[offset + 1] = (value >>> 8)\n return offset + 2\n}\n\nBuffer.prototype.writeUint16BE =\nBuffer.prototype.writeUInt16BE = function writeUInt16BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)\n this[offset] = (value >>> 8)\n this[offset + 1] = (value & 0xff)\n return offset + 2\n}\n\nBuffer.prototype.writeUint32LE =\nBuffer.prototype.writeUInt32LE = function writeUInt32LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)\n this[offset + 3] = (value >>> 24)\n this[offset + 2] = (value >>> 16)\n this[offset + 1] = (value >>> 8)\n this[offset] = (value & 0xff)\n return offset + 4\n}\n\nBuffer.prototype.writeUint32BE =\nBuffer.prototype.writeUInt32BE = function writeUInt32BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)\n this[offset] = (value >>> 24)\n this[offset + 1] = (value >>> 16)\n this[offset + 2] = (value >>> 8)\n this[offset + 3] = (value & 0xff)\n return offset + 4\n}\n\nBuffer.prototype.writeIntLE = function writeIntLE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n var limit = Math.pow(2, (8 * byteLength) - 1)\n\n checkInt(this, value, offset, byteLength, limit - 1, -limit)\n }\n\n var i = 0\n var mul = 1\n var sub = 0\n this[offset] = value & 0xFF\n while (++i < byteLength && (mul *= 0x100)) {\n if (value < 0 && sub === 0 && this[offset + i - 1] !== 0) {\n sub = 1\n }\n this[offset + i] = ((value / mul) >> 0) - sub & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeIntBE = function writeIntBE (value, offset, byteLength, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n var limit = Math.pow(2, (8 * byteLength) - 1)\n\n checkInt(this, value, offset, byteLength, limit - 1, -limit)\n }\n\n var i = byteLength - 1\n var mul = 1\n var sub = 0\n this[offset + i] = value & 0xFF\n while (--i >= 0 && (mul *= 0x100)) {\n if (value < 0 && sub === 0 && this[offset + i + 1] !== 0) {\n sub = 1\n }\n this[offset + i] = ((value / mul) >> 0) - sub & 0xFF\n }\n\n return offset + byteLength\n}\n\nBuffer.prototype.writeInt8 = function writeInt8 (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80)\n if (value < 0) value = 0xff + value + 1\n this[offset] = (value & 0xff)\n return offset + 1\n}\n\nBuffer.prototype.writeInt16LE = function writeInt16LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)\n this[offset] = (value & 0xff)\n this[offset + 1] = (value >>> 8)\n return offset + 2\n}\n\nBuffer.prototype.writeInt16BE = function writeInt16BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)\n this[offset] = (value >>> 8)\n this[offset + 1] = (value & 0xff)\n return offset + 2\n}\n\nBuffer.prototype.writeInt32LE = function writeInt32LE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)\n this[offset] = (value & 0xff)\n this[offset + 1] = (value >>> 8)\n this[offset + 2] = (value >>> 16)\n this[offset + 3] = (value >>> 24)\n return offset + 4\n}\n\nBuffer.prototype.writeInt32BE = function writeInt32BE (value, offset, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)\n if (value < 0) value = 0xffffffff + value + 1\n this[offset] = (value >>> 24)\n this[offset + 1] = (value >>> 16)\n this[offset + 2] = (value >>> 8)\n this[offset + 3] = (value & 0xff)\n return offset + 4\n}\n\nfunction checkIEEE754 (buf, value, offset, ext, max, min) {\n if (offset + ext > buf.length) throw new RangeError('Index out of range')\n if (offset < 0) throw new RangeError('Index out of range')\n}\n\nfunction writeFloat (buf, value, offset, littleEndian, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n checkIEEE754(buf, value, offset, 4, 3.4028234663852886e+38, -3.4028234663852886e+38)\n }\n ieee754.write(buf, value, offset, littleEndian, 23, 4)\n return offset + 4\n}\n\nBuffer.prototype.writeFloatLE = function writeFloatLE (value, offset, noAssert) {\n return writeFloat(this, value, offset, true, noAssert)\n}\n\nBuffer.prototype.writeFloatBE = function writeFloatBE (value, offset, noAssert) {\n return writeFloat(this, value, offset, false, noAssert)\n}\n\nfunction writeDouble (buf, value, offset, littleEndian, noAssert) {\n value = +value\n offset = offset >>> 0\n if (!noAssert) {\n checkIEEE754(buf, value, offset, 8, 1.7976931348623157E+308, -1.7976931348623157E+308)\n }\n ieee754.write(buf, value, offset, littleEndian, 52, 8)\n return offset + 8\n}\n\nBuffer.prototype.writeDoubleLE = function writeDoubleLE (value, offset, noAssert) {\n return writeDouble(this, value, offset, true, noAssert)\n}\n\nBuffer.prototype.writeDoubleBE = function writeDoubleBE (value, offset, noAssert) {\n return writeDouble(this, value, offset, false, noAssert)\n}\n\n// copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)\nBuffer.prototype.copy = function copy (target, targetStart, start, end) {\n if (!Buffer.isBuffer(target)) throw new TypeError('argument should be a Buffer')\n if (!start) start = 0\n if (!end && end !== 0) end = this.length\n if (targetStart >= target.length) targetStart = target.length\n if (!targetStart) targetStart = 0\n if (end > 0 && end < start) end = start\n\n // Copy 0 bytes; we're done\n if (end === start) return 0\n if (target.length === 0 || this.length === 0) return 0\n\n // Fatal error conditions\n if (targetStart < 0) {\n throw new RangeError('targetStart out of bounds')\n }\n if (start < 0 || start >= this.length) throw new RangeError('Index out of range')\n if (end < 0) throw new RangeError('sourceEnd out of bounds')\n\n // Are we oob?\n if (end > this.length) end = this.length\n if (target.length - targetStart < end - start) {\n end = target.length - targetStart + start\n }\n\n var len = end - start\n\n if (this === target && typeof Uint8Array.prototype.copyWithin === 'function') {\n // Use built-in when available, missing from IE11\n this.copyWithin(targetStart, start, end)\n } else {\n Uint8Array.prototype.set.call(\n target,\n this.subarray(start, end),\n targetStart\n )\n }\n\n return len\n}\n\n// Usage:\n// buffer.fill(number[, offset[, end]])\n// buffer.fill(buffer[, offset[, end]])\n// buffer.fill(string[, offset[, end]][, encoding])\nBuffer.prototype.fill = function fill (val, start, end, encoding) {\n // Handle string cases:\n if (typeof val === 'string') {\n if (typeof start === 'string') {\n encoding = start\n start = 0\n end = this.length\n } else if (typeof end === 'string') {\n encoding = end\n end = this.length\n }\n if (encoding !== undefined && typeof encoding !== 'string') {\n throw new TypeError('encoding must be a string')\n }\n if (typeof encoding === 'string' && !Buffer.isEncoding(encoding)) {\n throw new TypeError('Unknown encoding: ' + encoding)\n }\n if (val.length === 1) {\n var code = val.charCodeAt(0)\n if ((encoding === 'utf8' && code < 128) ||\n encoding === 'latin1') {\n // Fast path: If `val` fits into a single byte, use that numeric value.\n val = code\n }\n }\n } else if (typeof val === 'number') {\n val = val & 255\n } else if (typeof val === 'boolean') {\n val = Number(val)\n }\n\n // Invalid ranges are not set to a default, so can range check early.\n if (start < 0 || this.length < start || this.length < end) {\n throw new RangeError('Out of range index')\n }\n\n if (end <= start) {\n return this\n }\n\n start = start >>> 0\n end = end === undefined ? this.length : end >>> 0\n\n if (!val) val = 0\n\n var i\n if (typeof val === 'number') {\n for (i = start; i < end; ++i) {\n this[i] = val\n }\n } else {\n var bytes = Buffer.isBuffer(val)\n ? val\n : Buffer.from(val, encoding)\n var len = bytes.length\n if (len === 0) {\n throw new TypeError('The value \"' + val +\n '\" is invalid for argument \"value\"')\n }\n for (i = 0; i < end - start; ++i) {\n this[i + start] = bytes[i % len]\n }\n }\n\n return this\n}\n\n// HELPER FUNCTIONS\n// ================\n\nvar INVALID_BASE64_RE = /[^+/0-9A-Za-z-_]/g\n\nfunction base64clean (str) {\n // Node takes equal signs as end of the Base64 encoding\n str = str.split('=')[0]\n // Node strips out invalid characters like \\n and \\t from the string, base64-js does not\n str = str.trim().replace(INVALID_BASE64_RE, '')\n // Node converts strings with length < 2 to ''\n if (str.length < 2) return ''\n // Node allows for non-padded base64 strings (missing trailing ===), base64-js does not\n while (str.length % 4 !== 0) {\n str = str + '='\n }\n return str\n}\n\nfunction utf8ToBytes (string, units) {\n units = units || Infinity\n var codePoint\n var length = string.length\n var leadSurrogate = null\n var bytes = []\n\n for (var i = 0; i < length; ++i) {\n codePoint = string.charCodeAt(i)\n\n // is surrogate component\n if (codePoint > 0xD7FF && codePoint < 0xE000) {\n // last char was a lead\n if (!leadSurrogate) {\n // no lead yet\n if (codePoint > 0xDBFF) {\n // unexpected trail\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n continue\n } else if (i + 1 === length) {\n // unpaired lead\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n continue\n }\n\n // valid lead\n leadSurrogate = codePoint\n\n continue\n }\n\n // 2 leads in a row\n if (codePoint < 0xDC00) {\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n leadSurrogate = codePoint\n continue\n }\n\n // valid surrogate pair\n codePoint = (leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00) + 0x10000\n } else if (leadSurrogate) {\n // valid bmp char, but last char was a lead\n if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)\n }\n\n leadSurrogate = null\n\n // encode utf8\n if (codePoint < 0x80) {\n if ((units -= 1) < 0) break\n bytes.push(codePoint)\n } else if (codePoint < 0x800) {\n if ((units -= 2) < 0) break\n bytes.push(\n codePoint >> 0x6 | 0xC0,\n codePoint & 0x3F | 0x80\n )\n } else if (codePoint < 0x10000) {\n if ((units -= 3) < 0) break\n bytes.push(\n codePoint >> 0xC | 0xE0,\n codePoint >> 0x6 & 0x3F | 0x80,\n codePoint & 0x3F | 0x80\n )\n } else if (codePoint < 0x110000) {\n if ((units -= 4) < 0) break\n bytes.push(\n codePoint >> 0x12 | 0xF0,\n codePoint >> 0xC & 0x3F | 0x80,\n codePoint >> 0x6 & 0x3F | 0x80,\n codePoint & 0x3F | 0x80\n )\n } else {\n throw new Error('Invalid code point')\n }\n }\n\n return bytes\n}\n\nfunction asciiToBytes (str) {\n var byteArray = []\n for (var i = 0; i < str.length; ++i) {\n // Node's code seems to be doing this and not & 0x7F..\n byteArray.push(str.charCodeAt(i) & 0xFF)\n }\n return byteArray\n}\n\nfunction utf16leToBytes (str, units) {\n var c, hi, lo\n var byteArray = []\n for (var i = 0; i < str.length; ++i) {\n if ((units -= 2) < 0) break\n\n c = str.charCodeAt(i)\n hi = c >> 8\n lo = c % 256\n byteArray.push(lo)\n byteArray.push(hi)\n }\n\n return byteArray\n}\n\nfunction base64ToBytes (str) {\n return base64.toByteArray(base64clean(str))\n}\n\nfunction blitBuffer (src, dst, offset, length) {\n for (var i = 0; i < length; ++i) {\n if ((i + offset >= dst.length) || (i >= src.length)) break\n dst[i + offset] = src[i]\n }\n return i\n}\n\n// ArrayBuffer or Uint8Array objects from other contexts (i.e. iframes) do not pass\n// the `instanceof` check but they should be treated as of that type.\n// See: https://github.com/feross/buffer/issues/166\nfunction isInstance (obj, type) {\n return obj instanceof type ||\n (obj != null && obj.constructor != null && obj.constructor.name != null &&\n obj.constructor.name === type.name)\n}\nfunction numberIsNaN (obj) {\n // For IE11 support\n return obj !== obj // eslint-disable-line no-self-compare\n}\n\n// Create lookup table for `toString('hex')`\n// See: https://github.com/feross/buffer/issues/219\nvar hexSliceLookupTable = (function () {\n var alphabet = '0123456789abcdef'\n var table = new Array(256)\n for (var i = 0; i < 16; ++i) {\n var i16 = i * 16\n for (var j = 0; j < 16; ++j) {\n table[i16 + j] = alphabet[i] + alphabet[j]\n }\n }\n return table\n})()\n","export default {};\n","// shim for using process in browser\n// based off https://github.com/defunctzombie/node-process/blob/master/browser.js\n\nfunction defaultSetTimout() {\n throw new Error('setTimeout has not been defined');\n}\nfunction defaultClearTimeout () {\n throw new Error('clearTimeout has not been defined');\n}\nvar cachedSetTimeout = defaultSetTimout;\nvar cachedClearTimeout = defaultClearTimeout;\nif (typeof global.setTimeout === 'function') {\n cachedSetTimeout = setTimeout;\n}\nif (typeof global.clearTimeout === 'function') {\n cachedClearTimeout = clearTimeout;\n}\n\nfunction runTimeout(fun) {\n if (cachedSetTimeout === setTimeout) {\n //normal enviroments in sane situations\n return setTimeout(fun, 0);\n }\n // if setTimeout wasn't available but was latter defined\n if ((cachedSetTimeout === defaultSetTimout || !cachedSetTimeout) && setTimeout) {\n cachedSetTimeout = setTimeout;\n return setTimeout(fun, 0);\n }\n try {\n // when when somebody has screwed with setTimeout but no I.E. maddness\n return cachedSetTimeout(fun, 0);\n } catch(e){\n try {\n // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally\n return cachedSetTimeout.call(null, fun, 0);\n } catch(e){\n // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error\n return cachedSetTimeout.call(this, fun, 0);\n }\n }\n\n\n}\nfunction runClearTimeout(marker) {\n if (cachedClearTimeout === clearTimeout) {\n //normal enviroments in sane situations\n return clearTimeout(marker);\n }\n // if clearTimeout wasn't available but was latter defined\n if ((cachedClearTimeout === defaultClearTimeout || !cachedClearTimeout) && clearTimeout) {\n cachedClearTimeout = clearTimeout;\n return clearTimeout(marker);\n }\n try {\n // when when somebody has screwed with setTimeout but no I.E. maddness\n return cachedClearTimeout(marker);\n } catch (e){\n try {\n // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally\n return cachedClearTimeout.call(null, marker);\n } catch (e){\n // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error.\n // Some versions of I.E. have different rules for clearTimeout vs setTimeout\n return cachedClearTimeout.call(this, marker);\n }\n }\n\n\n\n}\nvar queue = [];\nvar draining = false;\nvar currentQueue;\nvar queueIndex = -1;\n\nfunction cleanUpNextTick() {\n if (!draining || !currentQueue) {\n return;\n }\n draining = false;\n if (currentQueue.length) {\n queue = currentQueue.concat(queue);\n } else {\n queueIndex = -1;\n }\n if (queue.length) {\n drainQueue();\n }\n}\n\nfunction drainQueue() {\n if (draining) {\n return;\n }\n var timeout = runTimeout(cleanUpNextTick);\n draining = true;\n\n var len = queue.length;\n while(len) {\n currentQueue = queue;\n queue = [];\n while (++queueIndex < len) {\n if (currentQueue) {\n currentQueue[queueIndex].run();\n }\n }\n queueIndex = -1;\n len = queue.length;\n }\n currentQueue = null;\n draining = false;\n runClearTimeout(timeout);\n}\nexport function nextTick(fun) {\n var args = new Array(arguments.length - 1);\n if (arguments.length > 1) {\n for (var i = 1; i < arguments.length; i++) {\n args[i - 1] = arguments[i];\n }\n }\n queue.push(new Item(fun, args));\n if (queue.length === 1 && !draining) {\n runTimeout(drainQueue);\n }\n}\n// v8 likes predictible objects\nfunction Item(fun, array) {\n this.fun = fun;\n this.array = array;\n}\nItem.prototype.run = function () {\n this.fun.apply(null, this.array);\n};\nexport var title = 'browser';\nexport var platform = 'browser';\nexport var browser = true;\nexport var env = {};\nexport var argv = [];\nexport var version = ''; // empty string to avoid regexp issues\nexport var versions = {};\nexport var release = {};\nexport var config = {};\n\nfunction noop() {}\n\nexport var on = noop;\nexport var addListener = noop;\nexport var once = noop;\nexport var off = noop;\nexport var removeListener = noop;\nexport var removeAllListeners = noop;\nexport var emit = noop;\n\nexport function binding(name) {\n throw new Error('process.binding is not supported');\n}\n\nexport function cwd () { return '/' }\nexport function chdir (dir) {\n throw new Error('process.chdir is not supported');\n};\nexport function umask() { return 0; }\n\n// from https://github.com/kumavis/browser-process-hrtime/blob/master/index.js\nvar performance = global.performance || {}\nvar performanceNow =\n performance.now ||\n performance.mozNow ||\n performance.msNow ||\n performance.oNow ||\n performance.webkitNow ||\n function(){ return (new Date()).getTime() }\n\n// generate timestamp or delta\n// see http://nodejs.org/api/process.html#process_process_hrtime\nexport function hrtime(previousTimestamp){\n var clocktime = performanceNow.call(performance)*1e-3\n var seconds = Math.floor(clocktime)\n var nanoseconds = Math.floor((clocktime%1)*1e9)\n if (previousTimestamp) {\n seconds = seconds - previousTimestamp[0]\n nanoseconds = nanoseconds - previousTimestamp[1]\n if (nanoseconds<0) {\n seconds--\n nanoseconds += 1e9\n }\n }\n return [seconds,nanoseconds]\n}\n\nvar startTime = new Date();\nexport function uptime() {\n var currentTime = new Date();\n var dif = currentTime - startTime;\n return dif / 1000;\n}\n\nexport default {\n nextTick: nextTick,\n title: title,\n browser: browser,\n env: env,\n argv: argv,\n version: version,\n versions: versions,\n on: on,\n addListener: addListener,\n once: once,\n off: off,\n removeListener: removeListener,\n removeAllListeners: removeAllListeners,\n emit: emit,\n binding: binding,\n cwd: cwd,\n chdir: chdir,\n umask: umask,\n hrtime: hrtime,\n platform: platform,\n release: release,\n config: config,\n uptime: uptime\n};\n","\nvar inherits;\nif (typeof Object.create === 'function'){\n inherits = function inherits(ctor, superCtor) {\n // implementation from standard node.js 'util' module\n ctor.super_ = superCtor\n ctor.prototype = Object.create(superCtor.prototype, {\n constructor: {\n value: ctor,\n enumerable: false,\n writable: true,\n configurable: true\n }\n });\n };\n} else {\n inherits = function inherits(ctor, superCtor) {\n ctor.super_ = superCtor\n var TempCtor = function () {}\n TempCtor.prototype = superCtor.prototype\n ctor.prototype = new TempCtor()\n ctor.prototype.constructor = ctor\n }\n}\nexport default inherits;\n","// Copyright Joyent, Inc. and other Node contributors.\n//\n// Permission is hereby granted, free of charge, to any person obtaining a\n// copy of this software and associated documentation files (the\n// \"Software\"), to deal in the Software without restriction, including\n// without limitation the rights to use, copy, modify, merge, publish,\n// distribute, sublicense, and/or sell copies of the Software, and to permit\n// persons to whom the Software is furnished to do so, subject to the\n// following conditions:\n//\n// The above copyright notice and this permission notice shall be included\n// in all copies or substantial portions of the Software.\n//\n// THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS\n// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF\n// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN\n// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,\n// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR\n// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE\n// USE OR OTHER DEALINGS IN THE SOFTWARE.\nimport process from 'process';\nvar formatRegExp = /%[sdj%]/g;\nexport function format(f) {\n if (!isString(f)) {\n var objects = [];\n for (var i = 0; i < arguments.length; i++) {\n objects.push(inspect(arguments[i]));\n }\n return objects.join(' ');\n }\n\n var i = 1;\n var args = arguments;\n var len = args.length;\n var str = String(f).replace(formatRegExp, function(x) {\n if (x === '%%') return '%';\n if (i >= len) return x;\n switch (x) {\n case '%s': return String(args[i++]);\n case '%d': return Number(args[i++]);\n case '%j':\n try {\n return JSON.stringify(args[i++]);\n } catch (_) {\n return '[Circular]';\n }\n default:\n return x;\n }\n });\n for (var x = args[i]; i < len; x = args[++i]) {\n if (isNull(x) || !isObject(x)) {\n str += ' ' + x;\n } else {\n str += ' ' + inspect(x);\n }\n }\n return str;\n};\n\n\n// Mark that a method should not be used.\n// Returns a modified function which warns once by default.\n// If --no-deprecation is set, then it is a no-op.\nexport function deprecate(fn, msg) {\n // Allow for deprecating things in the process of starting up.\n if (isUndefined(global.process)) {\n return function() {\n return deprecate(fn, msg).apply(this, arguments);\n };\n }\n\n if (process.noDeprecation === true) {\n return fn;\n }\n\n var warned = false;\n function deprecated() {\n if (!warned) {\n if (process.throwDeprecation) {\n throw new Error(msg);\n } else if (process.traceDeprecation) {\n console.trace(msg);\n } else {\n console.error(msg);\n }\n warned = true;\n }\n return fn.apply(this, arguments);\n }\n\n return deprecated;\n};\n\n\nvar debugs = {};\nvar debugEnviron;\nexport function debuglog(set) {\n if (isUndefined(debugEnviron))\n debugEnviron = process.env.NODE_DEBUG || '';\n set = set.toUpperCase();\n if (!debugs[set]) {\n if (new RegExp('\\\\b' + set + '\\\\b', 'i').test(debugEnviron)) {\n var pid = 0;\n debugs[set] = function() {\n var msg = format.apply(null, arguments);\n console.error('%s %d: %s', set, pid, msg);\n };\n } else {\n debugs[set] = function() {};\n }\n }\n return debugs[set];\n};\n\n\n/**\n * Echos the value of a value. Trys to print the value out\n * in the best way possible given the different types.\n *\n * @param {Object} obj The object to print out.\n * @param {Object} opts Optional options object that alters the output.\n */\n/* legacy: obj, showHidden, depth, colors*/\nexport function inspect(obj, opts) {\n // default options\n var ctx = {\n seen: [],\n stylize: stylizeNoColor\n };\n // legacy...\n if (arguments.length >= 3) ctx.depth = arguments[2];\n if (arguments.length >= 4) ctx.colors = arguments[3];\n if (isBoolean(opts)) {\n // legacy...\n ctx.showHidden = opts;\n } else if (opts) {\n // got an \"options\" object\n _extend(ctx, opts);\n }\n // set default options\n if (isUndefined(ctx.showHidden)) ctx.showHidden = false;\n if (isUndefined(ctx.depth)) ctx.depth = 2;\n if (isUndefined(ctx.colors)) ctx.colors = false;\n if (isUndefined(ctx.customInspect)) ctx.customInspect = true;\n if (ctx.colors) ctx.stylize = stylizeWithColor;\n return formatValue(ctx, obj, ctx.depth);\n}\n\n// http://en.wikipedia.org/wiki/ANSI_escape_code#graphics\ninspect.colors = {\n 'bold' : [1, 22],\n 'italic' : [3, 23],\n 'underline' : [4, 24],\n 'inverse' : [7, 27],\n 'white' : [37, 39],\n 'grey' : [90, 39],\n 'black' : [30, 39],\n 'blue' : [34, 39],\n 'cyan' : [36, 39],\n 'green' : [32, 39],\n 'magenta' : [35, 39],\n 'red' : [31, 39],\n 'yellow' : [33, 39]\n};\n\n// Don't use 'blue' not visible on cmd.exe\ninspect.styles = {\n 'special': 'cyan',\n 'number': 'yellow',\n 'boolean': 'yellow',\n 'undefined': 'grey',\n 'null': 'bold',\n 'string': 'green',\n 'date': 'magenta',\n // \"name\": intentionally not styling\n 'regexp': 'red'\n};\n\n\nfunction stylizeWithColor(str, styleType) {\n var style = inspect.styles[styleType];\n\n if (style) {\n return '\\u001b[' + inspect.colors[style][0] + 'm' + str +\n '\\u001b[' + inspect.colors[style][1] + 'm';\n } else {\n return str;\n }\n}\n\n\nfunction stylizeNoColor(str, styleType) {\n return str;\n}\n\n\nfunction arrayToHash(array) {\n var hash = {};\n\n array.forEach(function(val, idx) {\n hash[val] = true;\n });\n\n return hash;\n}\n\n\nfunction formatValue(ctx, value, recurseTimes) {\n // Provide a hook for user-specified inspect functions.\n // Check that value is an object with an inspect function on it\n if (ctx.customInspect &&\n value &&\n isFunction(value.inspect) &&\n // Filter out the util module, it's inspect function is special\n value.inspect !== inspect &&\n // Also filter out any prototype objects using the circular check.\n !(value.constructor && value.constructor.prototype === value)) {\n var ret = value.inspect(recurseTimes, ctx);\n if (!isString(ret)) {\n ret = formatValue(ctx, ret, recurseTimes);\n }\n return ret;\n }\n\n // Primitive types cannot have properties\n var primitive = formatPrimitive(ctx, value);\n if (primitive) {\n return primitive;\n }\n\n // Look up the keys of the object.\n var keys = Object.keys(value);\n var visibleKeys = arrayToHash(keys);\n\n if (ctx.showHidden) {\n keys = Object.getOwnPropertyNames(value);\n }\n\n // IE doesn't make error fields non-enumerable\n // http://msdn.microsoft.com/en-us/library/ie/dww52sbt(v=vs.94).aspx\n if (isError(value)\n && (keys.indexOf('message') >= 0 || keys.indexOf('description') >= 0)) {\n return formatError(value);\n }\n\n // Some type of object without properties can be shortcutted.\n if (keys.length === 0) {\n if (isFunction(value)) {\n var name = value.name ? ': ' + value.name : '';\n return ctx.stylize('[Function' + name + ']', 'special');\n }\n if (isRegExp(value)) {\n return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');\n }\n if (isDate(value)) {\n return ctx.stylize(Date.prototype.toString.call(value), 'date');\n }\n if (isError(value)) {\n return formatError(value);\n }\n }\n\n var base = '', array = false, braces = ['{', '}'];\n\n // Make Array say that they are Array\n if (isArray(value)) {\n array = true;\n braces = ['[', ']'];\n }\n\n // Make functions say that they are functions\n if (isFunction(value)) {\n var n = value.name ? ': ' + value.name : '';\n base = ' [Function' + n + ']';\n }\n\n // Make RegExps say that they are RegExps\n if (isRegExp(value)) {\n base = ' ' + RegExp.prototype.toString.call(value);\n }\n\n // Make dates with properties first say the date\n if (isDate(value)) {\n base = ' ' + Date.prototype.toUTCString.call(value);\n }\n\n // Make error with message first say the error\n if (isError(value)) {\n base = ' ' + formatError(value);\n }\n\n if (keys.length === 0 && (!array || value.length == 0)) {\n return braces[0] + base + braces[1];\n }\n\n if (recurseTimes < 0) {\n if (isRegExp(value)) {\n return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');\n } else {\n return ctx.stylize('[Object]', 'special');\n }\n }\n\n ctx.seen.push(value);\n\n var output;\n if (array) {\n output = formatArray(ctx, value, recurseTimes, visibleKeys, keys);\n } else {\n output = keys.map(function(key) {\n return formatProperty(ctx, value, recurseTimes, visibleKeys, key, array);\n });\n }\n\n ctx.seen.pop();\n\n return reduceToSingleString(output, base, braces);\n}\n\n\nfunction formatPrimitive(ctx, value) {\n if (isUndefined(value))\n return ctx.stylize('undefined', 'undefined');\n if (isString(value)) {\n var simple = '\\'' + JSON.stringify(value).replace(/^\"|\"$/g, '')\n .replace(/'/g, \"\\\\'\")\n .replace(/\\\\\"/g, '\"') + '\\'';\n return ctx.stylize(simple, 'string');\n }\n if (isNumber(value))\n return ctx.stylize('' + value, 'number');\n if (isBoolean(value))\n return ctx.stylize('' + value, 'boolean');\n // For some reason typeof null is \"object\", so special case here.\n if (isNull(value))\n return ctx.stylize('null', 'null');\n}\n\n\nfunction formatError(value) {\n return '[' + Error.prototype.toString.call(value) + ']';\n}\n\n\nfunction formatArray(ctx, value, recurseTimes, visibleKeys, keys) {\n var output = [];\n for (var i = 0, l = value.length; i < l; ++i) {\n if (hasOwnProperty(value, String(i))) {\n output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,\n String(i), true));\n } else {\n output.push('');\n }\n }\n keys.forEach(function(key) {\n if (!key.match(/^\\d+$/)) {\n output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,\n key, true));\n }\n });\n return output;\n}\n\n\nfunction formatProperty(ctx, value, recurseTimes, visibleKeys, key, array) {\n var name, str, desc;\n desc = Object.getOwnPropertyDescriptor(value, key) || { value: value[key] };\n if (desc.get) {\n if (desc.set) {\n str = ctx.stylize('[Getter/Setter]', 'special');\n } else {\n str = ctx.stylize('[Getter]', 'special');\n }\n } else {\n if (desc.set) {\n str = ctx.stylize('[Setter]', 'special');\n }\n }\n if (!hasOwnProperty(visibleKeys, key)) {\n name = '[' + key + ']';\n }\n if (!str) {\n if (ctx.seen.indexOf(desc.value) < 0) {\n if (isNull(recurseTimes)) {\n str = formatValue(ctx, desc.value, null);\n } else {\n str = formatValue(ctx, desc.value, recurseTimes - 1);\n }\n if (str.indexOf('\\n') > -1) {\n if (array) {\n str = str.split('\\n').map(function(line) {\n return ' ' + line;\n }).join('\\n').substr(2);\n } else {\n str = '\\n' + str.split('\\n').map(function(line) {\n return ' ' + line;\n }).join('\\n');\n }\n }\n } else {\n str = ctx.stylize('[Circular]', 'special');\n }\n }\n if (isUndefined(name)) {\n if (array && key.match(/^\\d+$/)) {\n return str;\n }\n name = JSON.stringify('' + key);\n if (name.match(/^\"([a-zA-Z_][a-zA-Z_0-9]*)\"$/)) {\n name = name.substr(1, name.length - 2);\n name = ctx.stylize(name, 'name');\n } else {\n name = name.replace(/'/g, \"\\\\'\")\n .replace(/\\\\\"/g, '\"')\n .replace(/(^\"|\"$)/g, \"'\");\n name = ctx.stylize(name, 'string');\n }\n }\n\n return name + ': ' + str;\n}\n\n\nfunction reduceToSingleString(output, base, braces) {\n var numLinesEst = 0;\n var length = output.reduce(function(prev, cur) {\n numLinesEst++;\n if (cur.indexOf('\\n') >= 0) numLinesEst++;\n return prev + cur.replace(/\\u001b\\[\\d\\d?m/g, '').length + 1;\n }, 0);\n\n if (length > 60) {\n return braces[0] +\n (base === '' ? '' : base + '\\n ') +\n ' ' +\n output.join(',\\n ') +\n ' ' +\n braces[1];\n }\n\n return braces[0] + base + ' ' + output.join(', ') + ' ' + braces[1];\n}\n\n\n// NOTE: These type checking functions intentionally don't use `instanceof`\n// because it is fragile and can be easily faked with `Object.create()`.\nexport function isArray(ar) {\n return Array.isArray(ar);\n}\n\nexport function isBoolean(arg) {\n return typeof arg === 'boolean';\n}\n\nexport function isNull(arg) {\n return arg === null;\n}\n\nexport function isNullOrUndefined(arg) {\n return arg == null;\n}\n\nexport function isNumber(arg) {\n return typeof arg === 'number';\n}\n\nexport function isString(arg) {\n return typeof arg === 'string';\n}\n\nexport function isSymbol(arg) {\n return typeof arg === 'symbol';\n}\n\nexport function isUndefined(arg) {\n return arg === void 0;\n}\n\nexport function isRegExp(re) {\n return isObject(re) && objectToString(re) === '[object RegExp]';\n}\n\nexport function isObject(arg) {\n return typeof arg === 'object' && arg !== null;\n}\n\nexport function isDate(d) {\n return isObject(d) && objectToString(d) === '[object Date]';\n}\n\nexport function isError(e) {\n return isObject(e) &&\n (objectToString(e) === '[object Error]' || e instanceof Error);\n}\n\nexport function isFunction(arg) {\n return typeof arg === 'function';\n}\n\nexport function isPrimitive(arg) {\n return arg === null ||\n typeof arg === 'boolean' ||\n typeof arg === 'number' ||\n typeof arg === 'string' ||\n typeof arg === 'symbol' || // ES6 symbol\n typeof arg === 'undefined';\n}\n\nexport function isBuffer(maybeBuf) {\n return Buffer.isBuffer(maybeBuf);\n}\n\nfunction objectToString(o) {\n return Object.prototype.toString.call(o);\n}\n\n\nfunction pad(n) {\n return n < 10 ? '0' + n.toString(10) : n.toString(10);\n}\n\n\nvar months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep',\n 'Oct', 'Nov', 'Dec'];\n\n// 26 Feb 16:19:34\nfunction timestamp() {\n var d = new Date();\n var time = [pad(d.getHours()),\n pad(d.getMinutes()),\n pad(d.getSeconds())].join(':');\n return [d.getDate(), months[d.getMonth()], time].join(' ');\n}\n\n\n// log is just a thin wrapper to console.log that prepends a timestamp\nexport function log() {\n console.log('%s - %s', timestamp(), format.apply(null, arguments));\n}\n\n\n/**\n * Inherit the prototype methods from one constructor into another.\n *\n * The Function.prototype.inherits from lang.js rewritten as a standalone\n * function (not on Function.prototype). NOTE: If this file is to be loaded\n * during bootstrapping this function needs to be rewritten using some native\n * functions as prototype setup using normal JavaScript does not work as\n * expected during bootstrapping (see mirror.js in r114903).\n *\n * @param {function} ctor Constructor function which needs to inherit the\n * prototype.\n * @param {function} superCtor Constructor function to inherit prototype from.\n */\nimport inherits from './inherits';\nexport {inherits}\n\nexport function _extend(origin, add) {\n // Don't do anything if add isn't an object\n if (!add || !isObject(add)) return origin;\n\n var keys = Object.keys(add);\n var i = keys.length;\n while (i--) {\n origin[keys[i]] = add[keys[i]];\n }\n return origin;\n};\n\nfunction hasOwnProperty(obj, prop) {\n return Object.prototype.hasOwnProperty.call(obj, prop);\n}\n\nexport default {\n inherits: inherits,\n _extend: _extend,\n log: log,\n isBuffer: isBuffer,\n isPrimitive: isPrimitive,\n isFunction: isFunction,\n isError: isError,\n isDate: isDate,\n isObject: isObject,\n isRegExp: isRegExp,\n isUndefined: isUndefined,\n isSymbol: isSymbol,\n isString: isString,\n isNumber: isNumber,\n isNullOrUndefined: isNullOrUndefined,\n isNull: isNull,\n isBoolean: isBoolean,\n isArray: isArray,\n inspect: inspect,\n deprecate: deprecate,\n format: format,\n debuglog: debuglog\n}\n",null,null,null,null,null,null,null,null,null,null,null,null,null,null,null,null,"/*! *****************************************************************************\r\nCopyright (c) Microsoft Corporation.\r\n\r\nPermission to use, copy, modify, and/or distribute this software for any\r\npurpose with or without fee is hereby granted.\r\n\r\nTHE SOFTWARE IS PROVIDED \"AS IS\" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH\r\nREGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY\r\nAND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,\r\nINDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM\r\nLOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR\r\nOTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR\r\nPERFORMANCE OF THIS SOFTWARE.\r\n***************************************************************************** */\r\n/* global Reflect, Promise */\r\n\r\nvar extendStatics = function(d, b) {\r\n extendStatics = Object.setPrototypeOf ||\r\n ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||\r\n function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; };\r\n return extendStatics(d, b);\r\n};\r\n\r\nexport function __extends(d, b) {\r\n extendStatics(d, b);\r\n function __() { this.constructor = d; }\r\n d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());\r\n}\r\n\r\nexport var __assign = function() {\r\n __assign = Object.assign || function __assign(t) {\r\n for (var s, i = 1, n = arguments.length; i < n; i++) {\r\n s = arguments[i];\r\n for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p];\r\n }\r\n return t;\r\n }\r\n return __assign.apply(this, arguments);\r\n}\r\n\r\nexport function __rest(s, e) {\r\n var t = {};\r\n for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p) && e.indexOf(p) < 0)\r\n t[p] = s[p];\r\n if (s != null && typeof Object.getOwnPropertySymbols === \"function\")\r\n for (var i = 0, p = Object.getOwnPropertySymbols(s); i < p.length; i++) {\r\n if (e.indexOf(p[i]) < 0 && Object.prototype.propertyIsEnumerable.call(s, p[i]))\r\n t[p[i]] = s[p[i]];\r\n }\r\n return t;\r\n}\r\n\r\nexport function __decorate(decorators, target, key, desc) {\r\n var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;\r\n if (typeof Reflect === \"object\" && typeof Reflect.decorate === \"function\") r = Reflect.decorate(decorators, target, key, desc);\r\n else for (var i = decorators.length - 1; i >= 0; i--) if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;\r\n return c > 3 && r && Object.defineProperty(target, key, r), r;\r\n}\r\n\r\nexport function __param(paramIndex, decorator) {\r\n return function (target, key) { decorator(target, key, paramIndex); }\r\n}\r\n\r\nexport function __metadata(metadataKey, metadataValue) {\r\n if (typeof Reflect === \"object\" && typeof Reflect.metadata === \"function\") return Reflect.metadata(metadataKey, metadataValue);\r\n}\r\n\r\nexport function __awaiter(thisArg, _arguments, P, generator) {\r\n function adopt(value) { return value instanceof P ? value : new P(function (resolve) { resolve(value); }); }\r\n return new (P || (P = Promise))(function (resolve, reject) {\r\n function fulfilled(value) { try { step(generator.next(value)); } catch (e) { reject(e); } }\r\n function rejected(value) { try { step(generator[\"throw\"](value)); } catch (e) { reject(e); } }\r\n function step(result) { result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected); }\r\n step((generator = generator.apply(thisArg, _arguments || [])).next());\r\n });\r\n}\r\n\r\nexport function __generator(thisArg, body) {\r\n var _ = { label: 0, sent: function() { if (t[0] & 1) throw t[1]; return t[1]; }, trys: [], ops: [] }, f, y, t, g;\r\n return g = { next: verb(0), \"throw\": verb(1), \"return\": verb(2) }, typeof Symbol === \"function\" && (g[Symbol.iterator] = function() { return this; }), g;\r\n function verb(n) { return function (v) { return step([n, v]); }; }\r\n function step(op) {\r\n if (f) throw new TypeError(\"Generator is already executing.\");\r\n while (_) try {\r\n if (f = 1, y && (t = op[0] & 2 ? y[\"return\"] : op[0] ? y[\"throw\"] || ((t = y[\"return\"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done) return t;\r\n if (y = 0, t) op = [op[0] & 2, t.value];\r\n switch (op[0]) {\r\n case 0: case 1: t = op; break;\r\n case 4: _.label++; return { value: op[1], done: false };\r\n case 5: _.label++; y = op[1]; op = [0]; continue;\r\n case 7: op = _.ops.pop(); _.trys.pop(); continue;\r\n default:\r\n if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) { _ = 0; continue; }\r\n if (op[0] === 3 && (!t || (op[1] > t[0] && op[1] < t[3]))) { _.label = op[1]; break; }\r\n if (op[0] === 6 && _.label < t[1]) { _.label = t[1]; t = op; break; }\r\n if (t && _.label < t[2]) { _.label = t[2]; _.ops.push(op); break; }\r\n if (t[2]) _.ops.pop();\r\n _.trys.pop(); continue;\r\n }\r\n op = body.call(thisArg, _);\r\n } catch (e) { op = [6, e]; y = 0; } finally { f = t = 0; }\r\n if (op[0] & 5) throw op[1]; return { value: op[0] ? op[1] : void 0, done: true };\r\n }\r\n}\r\n\r\nexport function __createBinding(o, m, k, k2) {\r\n if (k2 === undefined) k2 = k;\r\n o[k2] = m[k];\r\n}\r\n\r\nexport function __exportStar(m, exports) {\r\n for (var p in m) if (p !== \"default\" && !exports.hasOwnProperty(p)) exports[p] = m[p];\r\n}\r\n\r\nexport function __values(o) {\r\n var s = typeof Symbol === \"function\" && Symbol.iterator, m = s && o[s], i = 0;\r\n if (m) return m.call(o);\r\n if (o && typeof o.length === \"number\") return {\r\n next: function () {\r\n if (o && i >= o.length) o = void 0;\r\n return { value: o && o[i++], done: !o };\r\n }\r\n };\r\n throw new TypeError(s ? \"Object is not iterable.\" : \"Symbol.iterator is not defined.\");\r\n}\r\n\r\nexport function __read(o, n) {\r\n var m = typeof Symbol === \"function\" && o[Symbol.iterator];\r\n if (!m) return o;\r\n var i = m.call(o), r, ar = [], e;\r\n try {\r\n while ((n === void 0 || n-- > 0) && !(r = i.next()).done) ar.push(r.value);\r\n }\r\n catch (error) { e = { error: error }; }\r\n finally {\r\n try {\r\n if (r && !r.done && (m = i[\"return\"])) m.call(i);\r\n }\r\n finally { if (e) throw e.error; }\r\n }\r\n return ar;\r\n}\r\n\r\nexport function __spread() {\r\n for (var ar = [], i = 0; i < arguments.length; i++)\r\n ar = ar.concat(__read(arguments[i]));\r\n return ar;\r\n}\r\n\r\nexport function __spreadArrays() {\r\n for (var s = 0, i = 0, il = arguments.length; i < il; i++) s += arguments[i].length;\r\n for (var r = Array(s), k = 0, i = 0; i < il; i++)\r\n for (var a = arguments[i], j = 0, jl = a.length; j < jl; j++, k++)\r\n r[k] = a[j];\r\n return r;\r\n};\r\n\r\nexport function __await(v) {\r\n return this instanceof __await ? (this.v = v, this) : new __await(v);\r\n}\r\n\r\nexport function __asyncGenerator(thisArg, _arguments, generator) {\r\n if (!Symbol.asyncIterator) throw new TypeError(\"Symbol.asyncIterator is not defined.\");\r\n var g = generator.apply(thisArg, _arguments || []), i, q = [];\r\n return i = {}, verb(\"next\"), verb(\"throw\"), verb(\"return\"), i[Symbol.asyncIterator] = function () { return this; }, i;\r\n function verb(n) { if (g[n]) i[n] = function (v) { return new Promise(function (a, b) { q.push([n, v, a, b]) > 1 || resume(n, v); }); }; }\r\n function resume(n, v) { try { step(g[n](v)); } catch (e) { settle(q[0][3], e); } }\r\n function step(r) { r.value instanceof __await ? Promise.resolve(r.value.v).then(fulfill, reject) : settle(q[0][2], r); }\r\n function fulfill(value) { resume(\"next\", value); }\r\n function reject(value) { resume(\"throw\", value); }\r\n function settle(f, v) { if (f(v), q.shift(), q.length) resume(q[0][0], q[0][1]); }\r\n}\r\n\r\nexport function __asyncDelegator(o) {\r\n var i, p;\r\n return i = {}, verb(\"next\"), verb(\"throw\", function (e) { throw e; }), verb(\"return\"), i[Symbol.iterator] = function () { return this; }, i;\r\n function verb(n, f) { i[n] = o[n] ? function (v) { return (p = !p) ? { value: __await(o[n](v)), done: n === \"return\" } : f ? f(v) : v; } : f; }\r\n}\r\n\r\nexport function __asyncValues(o) {\r\n if (!Symbol.asyncIterator) throw new TypeError(\"Symbol.asyncIterator is not defined.\");\r\n var m = o[Symbol.asyncIterator], i;\r\n return m ? m.call(o) : (o = typeof __values === \"function\" ? __values(o) : o[Symbol.iterator](), i = {}, verb(\"next\"), verb(\"throw\"), verb(\"return\"), i[Symbol.asyncIterator] = function () { return this; }, i);\r\n function verb(n) { i[n] = o[n] && function (v) { return new Promise(function (resolve, reject) { v = o[n](v), settle(resolve, reject, v.done, v.value); }); }; }\r\n function settle(resolve, reject, d, v) { Promise.resolve(v).then(function(v) { resolve({ value: v, done: d }); }, reject); }\r\n}\r\n\r\nexport function __makeTemplateObject(cooked, raw) {\r\n if (Object.defineProperty) { Object.defineProperty(cooked, \"raw\", { value: raw }); } else { cooked.raw = raw; }\r\n return cooked;\r\n};\r\n\r\nexport function __importStar(mod) {\r\n if (mod && mod.__esModule) return mod;\r\n var result = {};\r\n if (mod != null) for (var k in mod) if (Object.hasOwnProperty.call(mod, k)) result[k] = mod[k];\r\n result.default = mod;\r\n return result;\r\n}\r\n\r\nexport function __importDefault(mod) {\r\n return (mod && mod.__esModule) ? mod : { default: mod };\r\n}\r\n\r\nexport function __classPrivateFieldGet(receiver, privateMap) {\r\n if (!privateMap.has(receiver)) {\r\n throw new TypeError(\"attempted to get private field on non-instance\");\r\n }\r\n return privateMap.get(receiver);\r\n}\r\n\r\nexport function __classPrivateFieldSet(receiver, privateMap, value) {\r\n if (!privateMap.has(receiver)) {\r\n throw new TypeError(\"attempted to set private field on non-instance\");\r\n }\r\n privateMap.set(receiver, value);\r\n return value;\r\n}\r\n",null,null,null,null,null,null,null,null,null,null],"names":["byteLength","toByteArray","fromByteArray","lookup","revLookup","Arr","Uint8Array","Array","code","i","len","length","charCodeAt","getLens","b64","Error","validLen","indexOf","placeHoldersLen","lens","_byteLength","tmp","arr","curByte","tripletToBase64","num","encodeChunk","uint8","start","end","output","push","join","extraBytes","parts","maxChunkLength","len2","buffer","offset","isLE","mLen","nBytes","e","m","eLen","eMax","eBias","nBits","d","s","NaN","Infinity","Math","pow","value","c","rt","abs","isNaN","floor","log","LN2","customInspectSymbol","Symbol","exports","Buffer","SlowBuffer","K_MAX_LENGTH","TYPED_ARRAY_SUPPORT","typedArraySupport","console","error","proto","foo","Object","setPrototypeOf","prototype","defineProperty","enumerable","get","isBuffer","undefined","byteOffset","createBuffer","RangeError","buf","arg","encodingOrOffset","TypeError","allocUnsafe","from","poolSize","fromString","ArrayBuffer","isView","fromArrayView","isInstance","fromArrayBuffer","SharedArrayBuffer","valueOf","b","fromObject","toPrimitive","assertSize","size","alloc","fill","encoding","checked","allocUnsafeSlow","string","isEncoding","actual","write","slice","fromArrayLike","array","arrayView","copy","obj","numberIsNaN","type","isArray","data","toString","_isBuffer","compare","a","x","y","min","String","toLowerCase","concat","list","pos","set","call","mustMatch","arguments","loweredCase","utf8ToBytes","base64ToBytes","slowToString","hexSlice","utf8Slice","asciiSlice","latin1Slice","base64Slice","utf16leSlice","swap","n","swap16","swap32","swap64","apply","toLocaleString","equals","inspect","str","max","INSPECT_MAX_BYTES","replace","trim","target","thisStart","thisEnd","thisCopy","targetCopy","bidirectionalIndexOf","val","dir","arrayIndexOf","lastIndexOf","indexSize","arrLength","valLength","read","readUInt16BE","foundIndex","found","j","includes","hexWrite","Number","remaining","strLen","parsed","parseInt","substr","utf8Write","blitBuffer","asciiWrite","asciiToBytes","base64Write","ucs2Write","utf16leToBytes","isFinite","toJSON","_arr","base64","res","firstByte","codePoint","bytesPerSequence","secondByte","thirdByte","fourthByte","tempCodePoint","decodeCodePointsArray","MAX_ARGUMENTS_LENGTH","codePoints","fromCharCode","ret","out","hexSliceLookupTable","bytes","newBuf","subarray","checkOffset","ext","readUintLE","readUIntLE","noAssert","mul","readUintBE","readUIntBE","readUint8","readUInt8","readUint16LE","readUInt16LE","readUint16BE","readUint32LE","readUInt32LE","readUint32BE","readUInt32BE","readIntLE","readIntBE","readInt8","readInt16LE","readInt16BE","readInt32LE","readInt32BE","readFloatLE","ieee754","readFloatBE","readDoubleLE","readDoubleBE","checkInt","writeUintLE","writeUIntLE","maxBytes","writeUintBE","writeUIntBE","writeUint8","writeUInt8","writeUint16LE","writeUInt16LE","writeUint16BE","writeUInt16BE","writeUint32LE","writeUInt32LE","writeUint32BE","writeUInt32BE","writeIntLE","limit","sub","writeIntBE","writeInt8","writeInt16LE","writeInt16BE","writeInt32LE","writeInt32BE","checkIEEE754","writeFloat","littleEndian","writeFloatLE","writeFloatBE","writeDouble","writeDoubleLE","writeDoubleBE","targetStart","copyWithin","INVALID_BASE64_RE","base64clean","split","units","leadSurrogate","byteArray","hi","lo","src","dst","constructor","name","alphabet","table","i16","performance","global","now","mozNow","msNow","oNow","webkitNow","Date","getTime","inherits","create","ctor","superCtor","super_","writable","configurable","TempCtor","formatRegExp","format","f","isString","objects","args","JSON","stringify","_","isNull","isObject","deprecate","fn","msg","isUndefined","process","warned","deprecated","debugs","debugEnviron","debuglog","toUpperCase","RegExp","test","pid","opts","ctx","seen","stylize","stylizeNoColor","depth","colors","isBoolean","showHidden","_extend","customInspect","stylizeWithColor","formatValue","styles","styleType","style","arrayToHash","hash","forEach","idx","recurseTimes","isFunction","primitive","formatPrimitive","keys","visibleKeys","getOwnPropertyNames","isError","formatError","isRegExp","isDate","base","braces","toUTCString","formatArray","map","key","formatProperty","pop","reduceToSingleString","simple","isNumber","l","hasOwnProperty","match","desc","getOwnPropertyDescriptor","line","reduce","prev","cur","numLinesEst","ar","isNullOrUndefined","isSymbol","re","objectToString","isPrimitive","maybeBuf","o","pad","months","timestamp","time","getHours","getMinutes","getSeconds","getDate","getMonth","origin","add","prop","buffer_1","utils_1","ensure_buffer_1","uuid_utils_1","binary_1","uuid_1","extendStatics","__proto__","p","__extends","__","__assign","assign","t","__rest","getOwnPropertySymbols","propertyIsEnumerable","__decorate","decorators","r","Reflect","decorate","__param","paramIndex","decorator","__metadata","metadataKey","metadataValue","metadata","__awaiter","thisArg","_arguments","P","generator","adopt","resolve","Promise","reject","fulfilled","step","next","rejected","result","done","then","__generator","body","label","sent","trys","ops","g","verb","iterator","v","op","__createBinding","k","k2","__exportStar","__values","__read","__spread","__spreadArrays","il","jl","__await","__asyncGenerator","asyncIterator","q","resume","settle","fulfill","shift","__asyncDelegator","__asyncValues","__makeTemplateObject","cooked","raw","__importStar","mod","__esModule","__importDefault","__classPrivateFieldGet","receiver","privateMap","has","__classPrivateFieldSet","tslib_1","objectid_1","symbol_1","int_32_1","decimal128_1","min_key_1","max_key_1","regexp_1","timestamp_1","code_1","db_ref_1","validate_utf8_1","decimal128","symbol","float_parser_1","extended_json_1","map_1","serializer_1","deserializer_1","calculate_size_1"],"mappings":";;;;;;;;;;;;;CAEA,gBAAkB,GAAGA,UAArB;CACA,iBAAmB,GAAGC,WAAtB;CACA,mBAAqB,GAAGC,aAAxB;CAEA,IAAIC,MAAM,GAAG,EAAb;CACA,IAAIC,SAAS,GAAG,EAAhB;CACA,IAAIC,GAAG,GAAG,OAAOC,UAAP,KAAsB,WAAtB,GAAoCA,UAApC,GAAiDC,KAA3D;CAEA,IAAIC,MAAI,GAAG,kEAAX;;CACA,KAAK,IAAIC,CAAC,GAAG,CAAR,EAAWC,GAAG,GAAGF,MAAI,CAACG,MAA3B,EAAmCF,CAAC,GAAGC,GAAvC,EAA4C,EAAED,CAA9C,EAAiD;CAC/CN,EAAAA,MAAM,CAACM,CAAD,CAAN,GAAYD,MAAI,CAACC,CAAD,CAAhB;CACAL,EAAAA,SAAS,CAACI,MAAI,CAACI,UAAL,CAAgBH,CAAhB,CAAD,CAAT,GAAgCA,CAAhC;CACD;CAGD;;;CACAL,SAAS,CAAC,IAAIQ,UAAJ,CAAe,CAAf,CAAD,CAAT,GAA+B,EAA/B;CACAR,SAAS,CAAC,IAAIQ,UAAJ,CAAe,CAAf,CAAD,CAAT,GAA+B,EAA/B;;CAEA,SAASC,OAAT,CAAkBC,GAAlB,EAAuB;CACrB,MAAIJ,GAAG,GAAGI,GAAG,CAACH,MAAd;;CAEA,MAAID,GAAG,GAAG,CAAN,GAAU,CAAd,EAAiB;CACf,UAAM,IAAIK,KAAJ,CAAU,gDAAV,CAAN;CACD,GALoB;;;;CASrB,MAAIC,QAAQ,GAAGF,GAAG,CAACG,OAAJ,CAAY,GAAZ,CAAf;CACA,MAAID,QAAQ,KAAK,CAAC,CAAlB,EAAqBA,QAAQ,GAAGN,GAAX;CAErB,MAAIQ,eAAe,GAAGF,QAAQ,KAAKN,GAAb,GAClB,CADkB,GAElB,IAAKM,QAAQ,GAAG,CAFpB;CAIA,SAAO,CAACA,QAAD,EAAWE,eAAX,CAAP;CACD;;;CAGD,SAASlB,UAAT,CAAqBc,GAArB,EAA0B;CACxB,MAAIK,IAAI,GAAGN,OAAO,CAACC,GAAD,CAAlB;CACA,MAAIE,QAAQ,GAAGG,IAAI,CAAC,CAAD,CAAnB;CACA,MAAID,eAAe,GAAGC,IAAI,CAAC,CAAD,CAA1B;CACA,SAAQ,CAACH,QAAQ,GAAGE,eAAZ,IAA+B,CAA/B,GAAmC,CAApC,GAAyCA,eAAhD;CACD;;CAED,SAASE,WAAT,CAAsBN,GAAtB,EAA2BE,QAA3B,EAAqCE,eAArC,EAAsD;CACpD,SAAQ,CAACF,QAAQ,GAAGE,eAAZ,IAA+B,CAA/B,GAAmC,CAApC,GAAyCA,eAAhD;CACD;;CAED,SAASjB,WAAT,CAAsBa,GAAtB,EAA2B;CACzB,MAAIO,GAAJ;CACA,MAAIF,IAAI,GAAGN,OAAO,CAACC,GAAD,CAAlB;CACA,MAAIE,QAAQ,GAAGG,IAAI,CAAC,CAAD,CAAnB;CACA,MAAID,eAAe,GAAGC,IAAI,CAAC,CAAD,CAA1B;CAEA,MAAIG,GAAG,GAAG,IAAIjB,GAAJ,CAAQe,WAAW,CAACN,GAAD,EAAME,QAAN,EAAgBE,eAAhB,CAAnB,CAAV;CAEA,MAAIK,OAAO,GAAG,CAAd,CARyB;;CAWzB,MAAIb,GAAG,GAAGQ,eAAe,GAAG,CAAlB,GACNF,QAAQ,GAAG,CADL,GAENA,QAFJ;CAIA,MAAIP,CAAJ;;CACA,OAAKA,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAGC,GAAhB,EAAqBD,CAAC,IAAI,CAA1B,EAA6B;CAC3BY,IAAAA,GAAG,GACAjB,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAf,CAAD,CAAT,IAAgC,EAAjC,GACCL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,EADrC,GAECL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CAFrC,GAGAL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAJX;CAKAa,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAkBF,GAAG,IAAI,EAAR,GAAc,IAA/B;CACAC,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAkBF,GAAG,IAAI,CAAR,GAAa,IAA9B;CACAC,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAiBF,GAAG,GAAG,IAAvB;CACD;;CAED,MAAIH,eAAe,KAAK,CAAxB,EAA2B;CACzBG,IAAAA,GAAG,GACAjB,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAf,CAAD,CAAT,IAAgC,CAAjC,GACCL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CAFvC;CAGAa,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAiBF,GAAG,GAAG,IAAvB;CACD;;CAED,MAAIH,eAAe,KAAK,CAAxB,EAA2B;CACzBG,IAAAA,GAAG,GACAjB,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAf,CAAD,CAAT,IAAgC,EAAjC,GACCL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CADrC,GAECL,SAAS,CAACU,GAAG,CAACF,UAAJ,CAAeH,CAAC,GAAG,CAAnB,CAAD,CAAT,IAAoC,CAHvC;CAIAa,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAkBF,GAAG,IAAI,CAAR,GAAa,IAA9B;CACAC,IAAAA,GAAG,CAACC,OAAO,EAAR,CAAH,GAAiBF,GAAG,GAAG,IAAvB;CACD;;CAED,SAAOC,GAAP;CACD;;CAED,SAASE,eAAT,CAA0BC,GAA1B,EAA+B;CAC7B,SAAOtB,MAAM,CAACsB,GAAG,IAAI,EAAP,GAAY,IAAb,CAAN,GACLtB,MAAM,CAACsB,GAAG,IAAI,EAAP,GAAY,IAAb,CADD,GAELtB,MAAM,CAACsB,GAAG,IAAI,CAAP,GAAW,IAAZ,CAFD,GAGLtB,MAAM,CAACsB,GAAG,GAAG,IAAP,CAHR;CAID;;CAED,SAASC,WAAT,CAAsBC,KAAtB,EAA6BC,KAA7B,EAAoCC,GAApC,EAAyC;CACvC,MAAIR,GAAJ;CACA,MAAIS,MAAM,GAAG,EAAb;;CACA,OAAK,IAAIrB,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6BpB,CAAC,IAAI,CAAlC,EAAqC;CACnCY,IAAAA,GAAG,GACD,CAAEM,KAAK,CAAClB,CAAD,CAAL,IAAY,EAAb,GAAmB,QAApB,KACEkB,KAAK,CAAClB,CAAC,GAAG,CAAL,CAAL,IAAgB,CAAjB,GAAsB,MADvB,KAECkB,KAAK,CAAClB,CAAC,GAAG,CAAL,CAAL,GAAe,IAFhB,CADF;CAIAqB,IAAAA,MAAM,CAACC,IAAP,CAAYP,eAAe,CAACH,GAAD,CAA3B;CACD;;CACD,SAAOS,MAAM,CAACE,IAAP,CAAY,EAAZ,CAAP;CACD;;CAED,SAAS9B,aAAT,CAAwByB,KAAxB,EAA+B;CAC7B,MAAIN,GAAJ;CACA,MAAIX,GAAG,GAAGiB,KAAK,CAAChB,MAAhB;CACA,MAAIsB,UAAU,GAAGvB,GAAG,GAAG,CAAvB,CAH6B;;CAI7B,MAAIwB,KAAK,GAAG,EAAZ;CACA,MAAIC,cAAc,GAAG,KAArB,CAL6B;;;CAQ7B,OAAK,IAAI1B,CAAC,GAAG,CAAR,EAAW2B,IAAI,GAAG1B,GAAG,GAAGuB,UAA7B,EAAyCxB,CAAC,GAAG2B,IAA7C,EAAmD3B,CAAC,IAAI0B,cAAxD,EAAwE;CACtED,IAAAA,KAAK,CAACH,IAAN,CAAWL,WAAW,CAACC,KAAD,EAAQlB,CAAR,EAAYA,CAAC,GAAG0B,cAAL,GAAuBC,IAAvB,GAA8BA,IAA9B,GAAsC3B,CAAC,GAAG0B,cAArD,CAAtB;CACD,GAV4B;;;CAa7B,MAAIF,UAAU,KAAK,CAAnB,EAAsB;CACpBZ,IAAAA,GAAG,GAAGM,KAAK,CAACjB,GAAG,GAAG,CAAP,CAAX;CACAwB,IAAAA,KAAK,CAACH,IAAN,CACE5B,MAAM,CAACkB,GAAG,IAAI,CAAR,CAAN,GACAlB,MAAM,CAAEkB,GAAG,IAAI,CAAR,GAAa,IAAd,CADN,GAEA,IAHF;CAKD,GAPD,MAOO,IAAIY,UAAU,KAAK,CAAnB,EAAsB;CAC3BZ,IAAAA,GAAG,GAAG,CAACM,KAAK,CAACjB,GAAG,GAAG,CAAP,CAAL,IAAkB,CAAnB,IAAwBiB,KAAK,CAACjB,GAAG,GAAG,CAAP,CAAnC;CACAwB,IAAAA,KAAK,CAACH,IAAN,CACE5B,MAAM,CAACkB,GAAG,IAAI,EAAR,CAAN,GACAlB,MAAM,CAAEkB,GAAG,IAAI,CAAR,GAAa,IAAd,CADN,GAEAlB,MAAM,CAAEkB,GAAG,IAAI,CAAR,GAAa,IAAd,CAFN,GAGA,GAJF;CAMD;;CAED,SAAOa,KAAK,CAACF,IAAN,CAAW,EAAX,CAAP;;;;;;;;;CCpJF;CACA,QAAY,GAAG,aAAA,CAAUK,MAAV,EAAkBC,MAAlB,EAA0BC,IAA1B,EAAgCC,IAAhC,EAAsCC,MAAtC,EAA8C;CAC3D,MAAIC,CAAJ,EAAOC,CAAP;CACA,MAAIC,IAAI,GAAIH,MAAM,GAAG,CAAV,GAAeD,IAAf,GAAsB,CAAjC;CACA,MAAIK,IAAI,GAAG,CAAC,KAAKD,IAAN,IAAc,CAAzB;CACA,MAAIE,KAAK,GAAGD,IAAI,IAAI,CAApB;CACA,MAAIE,KAAK,GAAG,CAAC,CAAb;CACA,MAAItC,CAAC,GAAG8B,IAAI,GAAIE,MAAM,GAAG,CAAb,GAAkB,CAA9B;CACA,MAAIO,CAAC,GAAGT,IAAI,GAAG,CAAC,CAAJ,GAAQ,CAApB;CACA,MAAIU,CAAC,GAAGZ,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAd;CAEAA,EAAAA,CAAC,IAAIuC,CAAL;CAEAN,EAAAA,CAAC,GAAGO,CAAC,GAAI,CAAC,KAAM,CAACF,KAAR,IAAkB,CAA3B;CACAE,EAAAA,CAAC,KAAM,CAACF,KAAR;CACAA,EAAAA,KAAK,IAAIH,IAAT;;CACA,SAAOG,KAAK,GAAG,CAAf,EAAkBL,CAAC,GAAIA,CAAC,GAAG,GAAL,GAAYL,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAtB,EAAoCA,CAAC,IAAIuC,CAAzC,EAA4CD,KAAK,IAAI,CAAvE,EAA0E;;CAE1EJ,EAAAA,CAAC,GAAGD,CAAC,GAAI,CAAC,KAAM,CAACK,KAAR,IAAkB,CAA3B;CACAL,EAAAA,CAAC,KAAM,CAACK,KAAR;CACAA,EAAAA,KAAK,IAAIP,IAAT;;CACA,SAAOO,KAAK,GAAG,CAAf,EAAkBJ,CAAC,GAAIA,CAAC,GAAG,GAAL,GAAYN,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAtB,EAAoCA,CAAC,IAAIuC,CAAzC,EAA4CD,KAAK,IAAI,CAAvE,EAA0E;;CAE1E,MAAIL,CAAC,KAAK,CAAV,EAAa;CACXA,IAAAA,CAAC,GAAG,IAAII,KAAR;CACD,GAFD,MAEO,IAAIJ,CAAC,KAAKG,IAAV,EAAgB;CACrB,WAAOF,CAAC,GAAGO,GAAH,GAAU,CAACD,CAAC,GAAG,CAAC,CAAJ,GAAQ,CAAV,IAAeE,QAAjC;CACD,GAFM,MAEA;CACLR,IAAAA,CAAC,GAAGA,CAAC,GAAGS,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYb,IAAZ,CAAR;CACAE,IAAAA,CAAC,GAAGA,CAAC,GAAGI,KAAR;CACD;;CACD,SAAO,CAACG,CAAC,GAAG,CAAC,CAAJ,GAAQ,CAAV,IAAeN,CAAf,GAAmBS,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYX,CAAC,GAAGF,IAAhB,CAA1B;CACD,CA/BD;;CAiCA,SAAa,GAAG,cAAA,CAAUH,MAAV,EAAkBiB,KAAlB,EAAyBhB,MAAzB,EAAiCC,IAAjC,EAAuCC,IAAvC,EAA6CC,MAA7C,EAAqD;CACnE,MAAIC,CAAJ,EAAOC,CAAP,EAAUY,CAAV;CACA,MAAIX,IAAI,GAAIH,MAAM,GAAG,CAAV,GAAeD,IAAf,GAAsB,CAAjC;CACA,MAAIK,IAAI,GAAG,CAAC,KAAKD,IAAN,IAAc,CAAzB;CACA,MAAIE,KAAK,GAAGD,IAAI,IAAI,CAApB;CACA,MAAIW,EAAE,GAAIhB,IAAI,KAAK,EAAT,GAAcY,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,CAAC,EAAb,IAAmBD,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,CAAC,EAAb,CAAjC,GAAoD,CAA9D;CACA,MAAI5C,CAAC,GAAG8B,IAAI,GAAG,CAAH,GAAQE,MAAM,GAAG,CAA7B;CACA,MAAIO,CAAC,GAAGT,IAAI,GAAG,CAAH,GAAO,CAAC,CAApB;CACA,MAAIU,CAAC,GAAGK,KAAK,GAAG,CAAR,IAAcA,KAAK,KAAK,CAAV,IAAe,IAAIA,KAAJ,GAAY,CAAzC,GAA8C,CAA9C,GAAkD,CAA1D;CAEAA,EAAAA,KAAK,GAAGF,IAAI,CAACK,GAAL,CAASH,KAAT,CAAR;;CAEA,MAAII,KAAK,CAACJ,KAAD,CAAL,IAAgBA,KAAK,KAAKH,QAA9B,EAAwC;CACtCR,IAAAA,CAAC,GAAGe,KAAK,CAACJ,KAAD,CAAL,GAAe,CAAf,GAAmB,CAAvB;CACAZ,IAAAA,CAAC,GAAGG,IAAJ;CACD,GAHD,MAGO;CACLH,IAAAA,CAAC,GAAGU,IAAI,CAACO,KAAL,CAAWP,IAAI,CAACQ,GAAL,CAASN,KAAT,IAAkBF,IAAI,CAACS,GAAlC,CAAJ;;CACA,QAAIP,KAAK,IAAIC,CAAC,GAAGH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,CAACX,CAAb,CAAR,CAAL,GAAgC,CAApC,EAAuC;CACrCA,MAAAA,CAAC;CACDa,MAAAA,CAAC,IAAI,CAAL;CACD;;CACD,QAAIb,CAAC,GAAGI,KAAJ,IAAa,CAAjB,EAAoB;CAClBQ,MAAAA,KAAK,IAAIE,EAAE,GAAGD,CAAd;CACD,KAFD,MAEO;CACLD,MAAAA,KAAK,IAAIE,EAAE,GAAGJ,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIP,KAAhB,CAAd;CACD;;CACD,QAAIQ,KAAK,GAAGC,CAAR,IAAa,CAAjB,EAAoB;CAClBb,MAAAA,CAAC;CACDa,MAAAA,CAAC,IAAI,CAAL;CACD;;CAED,QAAIb,CAAC,GAAGI,KAAJ,IAAaD,IAAjB,EAAuB;CACrBF,MAAAA,CAAC,GAAG,CAAJ;CACAD,MAAAA,CAAC,GAAGG,IAAJ;CACD,KAHD,MAGO,IAAIH,CAAC,GAAGI,KAAJ,IAAa,CAAjB,EAAoB;CACzBH,MAAAA,CAAC,GAAG,CAAEW,KAAK,GAAGC,CAAT,GAAc,CAAf,IAAoBH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYb,IAAZ,CAAxB;CACAE,MAAAA,CAAC,GAAGA,CAAC,GAAGI,KAAR;CACD,KAHM,MAGA;CACLH,MAAAA,CAAC,GAAGW,KAAK,GAAGF,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYP,KAAK,GAAG,CAApB,CAAR,GAAiCM,IAAI,CAACC,GAAL,CAAS,CAAT,EAAYb,IAAZ,CAArC;CACAE,MAAAA,CAAC,GAAG,CAAJ;CACD;CACF;;CAED,SAAOF,IAAI,IAAI,CAAf,EAAkBH,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAN,GAAqBkC,CAAC,GAAG,IAAzB,EAA+BlC,CAAC,IAAIuC,CAApC,EAAuCL,CAAC,IAAI,GAA5C,EAAiDH,IAAI,IAAI,CAA3E,EAA8E;;CAE9EE,EAAAA,CAAC,GAAIA,CAAC,IAAIF,IAAN,GAAcG,CAAlB;CACAC,EAAAA,IAAI,IAAIJ,IAAR;;CACA,SAAOI,IAAI,GAAG,CAAd,EAAiBP,MAAM,CAACC,MAAM,GAAG7B,CAAV,CAAN,GAAqBiC,CAAC,GAAG,IAAzB,EAA+BjC,CAAC,IAAIuC,CAApC,EAAuCN,CAAC,IAAI,GAA5C,EAAiDE,IAAI,IAAI,CAA1E,EAA6E;;CAE7EP,EAAAA,MAAM,CAACC,MAAM,GAAG7B,CAAT,GAAauC,CAAd,CAAN,IAA0BC,CAAC,GAAG,GAA9B;EAjDF;;;;;;;;;CCtBA,MAAIa,mBAAmB,GACpB,OAAOC,MAAP,KAAkB,UAAlB,IAAgC,OAAOA,MAAM,CAAC,KAAD,CAAb,KAAyB,UAA1D;CACIA,EAAAA,MAAM,CAAC,KAAD,CAAN,CAAc,4BAAd,CADJ;CAAA,IAEI,IAHN;CAKAC,EAAAA,cAAA,GAAiBC,MAAjB;CACAD,EAAAA,kBAAA,GAAqBE,UAArB;CACAF,EAAAA,yBAAA,GAA4B,EAA5B;CAEA,MAAIG,YAAY,GAAG,UAAnB;CACAH,EAAAA,kBAAA,GAAqBG,YAArB;CAEA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;CACAF,EAAAA,MAAM,CAACG,mBAAP,GAA6BC,iBAAiB,EAA9C;;CAEA,MAAI,CAACJ,MAAM,CAACG,mBAAR,IAA+B,OAAOE,OAAP,KAAmB,WAAlD,IACA,OAAOA,OAAO,CAACC,KAAf,KAAyB,UAD7B,EACyC;CACvCD,IAAAA,OAAO,CAACC,KAAR,CACE,8EACA,sEAFF;CAID;;CAED,WAASF,iBAAT,GAA8B;;CAE5B,QAAI;CACF,UAAI/C,GAAG,GAAG,IAAIhB,UAAJ,CAAe,CAAf,CAAV;CACA,UAAIkE,KAAK,GAAG;CAAEC,QAAAA,GAAG,EAAE,eAAY;CAAE,iBAAO,EAAP;CAAW;CAAhC,OAAZ;CACAC,MAAAA,MAAM,CAACC,cAAP,CAAsBH,KAAtB,EAA6BlE,UAAU,CAACsE,SAAxC;CACAF,MAAAA,MAAM,CAACC,cAAP,CAAsBrD,GAAtB,EAA2BkD,KAA3B;CACA,aAAOlD,GAAG,CAACmD,GAAJ,OAAc,EAArB;CACD,KAND,CAME,OAAO/B,CAAP,EAAU;CACV,aAAO,KAAP;CACD;CACF;;CAEDgC,EAAAA,MAAM,CAACG,cAAP,CAAsBZ,MAAM,CAACW,SAA7B,EAAwC,QAAxC,EAAkD;CAChDE,IAAAA,UAAU,EAAE,IADoC;CAEhDC,IAAAA,GAAG,EAAE,eAAY;CACf,UAAI,CAACd,MAAM,CAACe,QAAP,CAAgB,IAAhB,CAAL,EAA4B,OAAOC,SAAP;CAC5B,aAAO,KAAK5C,MAAZ;CACD;CAL+C,GAAlD;CAQAqC,EAAAA,MAAM,CAACG,cAAP,CAAsBZ,MAAM,CAACW,SAA7B,EAAwC,QAAxC,EAAkD;CAChDE,IAAAA,UAAU,EAAE,IADoC;CAEhDC,IAAAA,GAAG,EAAE,eAAY;CACf,UAAI,CAACd,MAAM,CAACe,QAAP,CAAgB,IAAhB,CAAL,EAA4B,OAAOC,SAAP;CAC5B,aAAO,KAAKC,UAAZ;CACD;CAL+C,GAAlD;;CAQA,WAASC,YAAT,CAAuBxE,MAAvB,EAA+B;CAC7B,QAAIA,MAAM,GAAGwD,YAAb,EAA2B;CACzB,YAAM,IAAIiB,UAAJ,CAAe,gBAAgBzE,MAAhB,GAAyB,gCAAxC,CAAN;CACD,KAH4B;;;CAK7B,QAAI0E,GAAG,GAAG,IAAI/E,UAAJ,CAAeK,MAAf,CAAV;CACA+D,IAAAA,MAAM,CAACC,cAAP,CAAsBU,GAAtB,EAA2BpB,MAAM,CAACW,SAAlC;CACA,WAAOS,GAAP;CACD;CAED;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;;CAEA,WAASpB,MAAT,CAAiBqB,GAAjB,EAAsBC,gBAAtB,EAAwC5E,MAAxC,EAAgD;;CAE9C,QAAI,OAAO2E,GAAP,KAAe,QAAnB,EAA6B;CAC3B,UAAI,OAAOC,gBAAP,KAA4B,QAAhC,EAA0C;CACxC,cAAM,IAAIC,SAAJ,CACJ,oEADI,CAAN;CAGD;;CACD,aAAOC,WAAW,CAACH,GAAD,CAAlB;CACD;;CACD,WAAOI,IAAI,CAACJ,GAAD,EAAMC,gBAAN,EAAwB5E,MAAxB,CAAX;CACD;;CAEDsD,EAAAA,MAAM,CAAC0B,QAAP,GAAkB,IAAlB;;CAEA,WAASD,IAAT,CAAepC,KAAf,EAAsBiC,gBAAtB,EAAwC5E,MAAxC,EAAgD;CAC9C,QAAI,OAAO2C,KAAP,KAAiB,QAArB,EAA+B;CAC7B,aAAOsC,UAAU,CAACtC,KAAD,EAAQiC,gBAAR,CAAjB;CACD;;CAED,QAAIM,WAAW,CAACC,MAAZ,CAAmBxC,KAAnB,CAAJ,EAA+B;CAC7B,aAAOyC,aAAa,CAACzC,KAAD,CAApB;CACD;;CAED,QAAIA,KAAK,IAAI,IAAb,EAAmB;CACjB,YAAM,IAAIkC,SAAJ,CACJ,gFACA,sCADA,0BACiDlC,KADjD,CADI,CAAN;CAID;;CAED,QAAI0C,UAAU,CAAC1C,KAAD,EAAQuC,WAAR,CAAV,IACCvC,KAAK,IAAI0C,UAAU,CAAC1C,KAAK,CAACjB,MAAP,EAAewD,WAAf,CADxB,EACsD;CACpD,aAAOI,eAAe,CAAC3C,KAAD,EAAQiC,gBAAR,EAA0B5E,MAA1B,CAAtB;CACD;;CAED,QAAI,OAAOuF,iBAAP,KAA6B,WAA7B,KACCF,UAAU,CAAC1C,KAAD,EAAQ4C,iBAAR,CAAV,IACA5C,KAAK,IAAI0C,UAAU,CAAC1C,KAAK,CAACjB,MAAP,EAAe6D,iBAAf,CAFpB,CAAJ,EAE6D;CAC3D,aAAOD,eAAe,CAAC3C,KAAD,EAAQiC,gBAAR,EAA0B5E,MAA1B,CAAtB;CACD;;CAED,QAAI,OAAO2C,KAAP,KAAiB,QAArB,EAA+B;CAC7B,YAAM,IAAIkC,SAAJ,CACJ,uEADI,CAAN;CAGD;;CAED,QAAIW,OAAO,GAAG7C,KAAK,CAAC6C,OAAN,IAAiB7C,KAAK,CAAC6C,OAAN,EAA/B;;CACA,QAAIA,OAAO,IAAI,IAAX,IAAmBA,OAAO,KAAK7C,KAAnC,EAA0C;CACxC,aAAOW,MAAM,CAACyB,IAAP,CAAYS,OAAZ,EAAqBZ,gBAArB,EAAuC5E,MAAvC,CAAP;CACD;;CAED,QAAIyF,CAAC,GAAGC,UAAU,CAAC/C,KAAD,CAAlB;CACA,QAAI8C,CAAJ,EAAO,OAAOA,CAAP;;CAEP,QAAI,OAAOrC,MAAP,KAAkB,WAAlB,IAAiCA,MAAM,CAACuC,WAAP,IAAsB,IAAvD,IACA,OAAOhD,KAAK,CAACS,MAAM,CAACuC,WAAR,CAAZ,KAAqC,UADzC,EACqD;CACnD,aAAOrC,MAAM,CAACyB,IAAP,CACLpC,KAAK,CAACS,MAAM,CAACuC,WAAR,CAAL,CAA0B,QAA1B,CADK,EACgCf,gBADhC,EACkD5E,MADlD,CAAP;CAGD;;CAED,UAAM,IAAI6E,SAAJ,CACJ,gFACA,sCADA,0BACiDlC,KADjD,CADI,CAAN;CAID;CAED;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;;CACAW,EAAAA,MAAM,CAACyB,IAAP,GAAc,UAAUpC,KAAV,EAAiBiC,gBAAjB,EAAmC5E,MAAnC,EAA2C;CACvD,WAAO+E,IAAI,CAACpC,KAAD,EAAQiC,gBAAR,EAA0B5E,MAA1B,CAAX;CACD,GAFD;CAKA;;;CACA+D,EAAAA,MAAM,CAACC,cAAP,CAAsBV,MAAM,CAACW,SAA7B,EAAwCtE,UAAU,CAACsE,SAAnD;CACAF,EAAAA,MAAM,CAACC,cAAP,CAAsBV,MAAtB,EAA8B3D,UAA9B;;CAEA,WAASiG,UAAT,CAAqBC,IAArB,EAA2B;CACzB,QAAI,OAAOA,IAAP,KAAgB,QAApB,EAA8B;CAC5B,YAAM,IAAIhB,SAAJ,CAAc,wCAAd,CAAN;CACD,KAFD,MAEO,IAAIgB,IAAI,GAAG,CAAX,EAAc;CACnB,YAAM,IAAIpB,UAAJ,CAAe,gBAAgBoB,IAAhB,GAAuB,gCAAtC,CAAN;CACD;CACF;;CAED,WAASC,KAAT,CAAgBD,IAAhB,EAAsBE,IAAtB,EAA4BC,QAA5B,EAAsC;CACpCJ,IAAAA,UAAU,CAACC,IAAD,CAAV;;CACA,QAAIA,IAAI,IAAI,CAAZ,EAAe;CACb,aAAOrB,YAAY,CAACqB,IAAD,CAAnB;CACD;;CACD,QAAIE,IAAI,KAAKzB,SAAb,EAAwB;;;;CAItB,aAAO,OAAO0B,QAAP,KAAoB,QAApB,GACHxB,YAAY,CAACqB,IAAD,CAAZ,CAAmBE,IAAnB,CAAwBA,IAAxB,EAA8BC,QAA9B,CADG,GAEHxB,YAAY,CAACqB,IAAD,CAAZ,CAAmBE,IAAnB,CAAwBA,IAAxB,CAFJ;CAGD;;CACD,WAAOvB,YAAY,CAACqB,IAAD,CAAnB;CACD;CAED;CACA;CACA;CACA;;;CACAvC,EAAAA,MAAM,CAACwC,KAAP,GAAe,UAAUD,IAAV,EAAgBE,IAAhB,EAAsBC,QAAtB,EAAgC;CAC7C,WAAOF,KAAK,CAACD,IAAD,EAAOE,IAAP,EAAaC,QAAb,CAAZ;CACD,GAFD;;CAIA,WAASlB,WAAT,CAAsBe,IAAtB,EAA4B;CAC1BD,IAAAA,UAAU,CAACC,IAAD,CAAV;CACA,WAAOrB,YAAY,CAACqB,IAAI,GAAG,CAAP,GAAW,CAAX,GAAeI,OAAO,CAACJ,IAAD,CAAP,GAAgB,CAAhC,CAAnB;CACD;CAED;CACA;CACA;;;CACAvC,EAAAA,MAAM,CAACwB,WAAP,GAAqB,UAAUe,IAAV,EAAgB;CACnC,WAAOf,WAAW,CAACe,IAAD,CAAlB;CACD,GAFD;CAGA;CACA;CACA;;;CACAvC,EAAAA,MAAM,CAAC4C,eAAP,GAAyB,UAAUL,IAAV,EAAgB;CACvC,WAAOf,WAAW,CAACe,IAAD,CAAlB;CACD,GAFD;;CAIA,WAASZ,UAAT,CAAqBkB,MAArB,EAA6BH,QAA7B,EAAuC;CACrC,QAAI,OAAOA,QAAP,KAAoB,QAApB,IAAgCA,QAAQ,KAAK,EAAjD,EAAqD;CACnDA,MAAAA,QAAQ,GAAG,MAAX;CACD;;CAED,QAAI,CAAC1C,MAAM,CAAC8C,UAAP,CAAkBJ,QAAlB,CAAL,EAAkC;CAChC,YAAM,IAAInB,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;CACD;;CAED,QAAIhG,MAAM,GAAGX,UAAU,CAAC8G,MAAD,EAASH,QAAT,CAAV,GAA+B,CAA5C;CACA,QAAItB,GAAG,GAAGF,YAAY,CAACxE,MAAD,CAAtB;CAEA,QAAIqG,MAAM,GAAG3B,GAAG,CAAC4B,KAAJ,CAAUH,MAAV,EAAkBH,QAAlB,CAAb;;CAEA,QAAIK,MAAM,KAAKrG,MAAf,EAAuB;;;;CAIrB0E,MAAAA,GAAG,GAAGA,GAAG,CAAC6B,KAAJ,CAAU,CAAV,EAAaF,MAAb,CAAN;CACD;;CAED,WAAO3B,GAAP;CACD;;CAED,WAAS8B,aAAT,CAAwBC,KAAxB,EAA+B;CAC7B,QAAIzG,MAAM,GAAGyG,KAAK,CAACzG,MAAN,GAAe,CAAf,GAAmB,CAAnB,GAAuBiG,OAAO,CAACQ,KAAK,CAACzG,MAAP,CAAP,GAAwB,CAA5D;CACA,QAAI0E,GAAG,GAAGF,YAAY,CAACxE,MAAD,CAAtB;;CACA,SAAK,IAAIF,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4BF,CAAC,IAAI,CAAjC,EAAoC;CAClC4E,MAAAA,GAAG,CAAC5E,CAAD,CAAH,GAAS2G,KAAK,CAAC3G,CAAD,CAAL,GAAW,GAApB;CACD;;CACD,WAAO4E,GAAP;CACD;;CAED,WAASU,aAAT,CAAwBsB,SAAxB,EAAmC;CACjC,QAAIrB,UAAU,CAACqB,SAAD,EAAY/G,UAAZ,CAAd,EAAuC;CACrC,UAAIgH,IAAI,GAAG,IAAIhH,UAAJ,CAAe+G,SAAf,CAAX;CACA,aAAOpB,eAAe,CAACqB,IAAI,CAACjF,MAAN,EAAciF,IAAI,CAACpC,UAAnB,EAA+BoC,IAAI,CAACtH,UAApC,CAAtB;CACD;;CACD,WAAOmH,aAAa,CAACE,SAAD,CAApB;CACD;;CAED,WAASpB,eAAT,CAA0BmB,KAA1B,EAAiClC,UAAjC,EAA6CvE,MAA7C,EAAqD;CACnD,QAAIuE,UAAU,GAAG,CAAb,IAAkBkC,KAAK,CAACpH,UAAN,GAAmBkF,UAAzC,EAAqD;CACnD,YAAM,IAAIE,UAAJ,CAAe,sCAAf,CAAN;CACD;;CAED,QAAIgC,KAAK,CAACpH,UAAN,GAAmBkF,UAAU,IAAIvE,MAAM,IAAI,CAAd,CAAjC,EAAmD;CACjD,YAAM,IAAIyE,UAAJ,CAAe,sCAAf,CAAN;CACD;;CAED,QAAIC,GAAJ;;CACA,QAAIH,UAAU,KAAKD,SAAf,IAA4BtE,MAAM,KAAKsE,SAA3C,EAAsD;CACpDI,MAAAA,GAAG,GAAG,IAAI/E,UAAJ,CAAe8G,KAAf,CAAN;CACD,KAFD,MAEO,IAAIzG,MAAM,KAAKsE,SAAf,EAA0B;CAC/BI,MAAAA,GAAG,GAAG,IAAI/E,UAAJ,CAAe8G,KAAf,EAAsBlC,UAAtB,CAAN;CACD,KAFM,MAEA;CACLG,MAAAA,GAAG,GAAG,IAAI/E,UAAJ,CAAe8G,KAAf,EAAsBlC,UAAtB,EAAkCvE,MAAlC,CAAN;CACD,KAhBkD;;;CAmBnD+D,IAAAA,MAAM,CAACC,cAAP,CAAsBU,GAAtB,EAA2BpB,MAAM,CAACW,SAAlC;CAEA,WAAOS,GAAP;CACD;;CAED,WAASgB,UAAT,CAAqBkB,GAArB,EAA0B;CACxB,QAAItD,MAAM,CAACe,QAAP,CAAgBuC,GAAhB,CAAJ,EAA0B;CACxB,UAAI7G,GAAG,GAAGkG,OAAO,CAACW,GAAG,CAAC5G,MAAL,CAAP,GAAsB,CAAhC;CACA,UAAI0E,GAAG,GAAGF,YAAY,CAACzE,GAAD,CAAtB;;CAEA,UAAI2E,GAAG,CAAC1E,MAAJ,KAAe,CAAnB,EAAsB;CACpB,eAAO0E,GAAP;CACD;;CAEDkC,MAAAA,GAAG,CAACD,IAAJ,CAASjC,GAAT,EAAc,CAAd,EAAiB,CAAjB,EAAoB3E,GAApB;CACA,aAAO2E,GAAP;CACD;;CAED,QAAIkC,GAAG,CAAC5G,MAAJ,KAAesE,SAAnB,EAA8B;CAC5B,UAAI,OAAOsC,GAAG,CAAC5G,MAAX,KAAsB,QAAtB,IAAkC6G,WAAW,CAACD,GAAG,CAAC5G,MAAL,CAAjD,EAA+D;CAC7D,eAAOwE,YAAY,CAAC,CAAD,CAAnB;CACD;;CACD,aAAOgC,aAAa,CAACI,GAAD,CAApB;CACD;;CAED,QAAIA,GAAG,CAACE,IAAJ,KAAa,QAAb,IAAyBlH,KAAK,CAACmH,OAAN,CAAcH,GAAG,CAACI,IAAlB,CAA7B,EAAsD;CACpD,aAAOR,aAAa,CAACI,GAAG,CAACI,IAAL,CAApB;CACD;CACF;;CAED,WAASf,OAAT,CAAkBjG,MAAlB,EAA0B;;;CAGxB,QAAIA,MAAM,IAAIwD,YAAd,EAA4B;CAC1B,YAAM,IAAIiB,UAAJ,CAAe,oDACA,UADA,GACajB,YAAY,CAACyD,QAAb,CAAsB,EAAtB,CADb,GACyC,QADxD,CAAN;CAED;;CACD,WAAOjH,MAAM,GAAG,CAAhB;CACD;;CAED,WAASuD,UAAT,CAAqBvD,MAArB,EAA6B;CAC3B,QAAI,CAACA,MAAD,IAAWA,MAAf,EAAuB;;CACrBA,MAAAA,MAAM,GAAG,CAAT;CACD;;CACD,WAAOsD,MAAM,CAACwC,KAAP,CAAa,CAAC9F,MAAd,CAAP;CACD;;CAEDsD,EAAAA,MAAM,CAACe,QAAP,GAAkB,SAASA,QAAT,CAAmBoB,CAAnB,EAAsB;CACtC,WAAOA,CAAC,IAAI,IAAL,IAAaA,CAAC,CAACyB,SAAF,KAAgB,IAA7B,IACLzB,CAAC,KAAKnC,MAAM,CAACW,SADf,CADsC;CAGvC,GAHD;;CAKAX,EAAAA,MAAM,CAAC6D,OAAP,GAAiB,SAASA,OAAT,CAAkBC,CAAlB,EAAqB3B,CAArB,EAAwB;CACvC,QAAIJ,UAAU,CAAC+B,CAAD,EAAIzH,UAAJ,CAAd,EAA+ByH,CAAC,GAAG9D,MAAM,CAACyB,IAAP,CAAYqC,CAAZ,EAAeA,CAAC,CAACzF,MAAjB,EAAyByF,CAAC,CAAC/H,UAA3B,CAAJ;CAC/B,QAAIgG,UAAU,CAACI,CAAD,EAAI9F,UAAJ,CAAd,EAA+B8F,CAAC,GAAGnC,MAAM,CAACyB,IAAP,CAAYU,CAAZ,EAAeA,CAAC,CAAC9D,MAAjB,EAAyB8D,CAAC,CAACpG,UAA3B,CAAJ;;CAC/B,QAAI,CAACiE,MAAM,CAACe,QAAP,CAAgB+C,CAAhB,CAAD,IAAuB,CAAC9D,MAAM,CAACe,QAAP,CAAgBoB,CAAhB,CAA5B,EAAgD;CAC9C,YAAM,IAAIZ,SAAJ,CACJ,uEADI,CAAN;CAGD;;CAED,QAAIuC,CAAC,KAAK3B,CAAV,EAAa,OAAO,CAAP;CAEb,QAAI4B,CAAC,GAAGD,CAAC,CAACpH,MAAV;CACA,QAAIsH,CAAC,GAAG7B,CAAC,CAACzF,MAAV;;CAEA,SAAK,IAAIF,CAAC,GAAG,CAAR,EAAWC,GAAG,GAAG0C,IAAI,CAAC8E,GAAL,CAASF,CAAT,EAAYC,CAAZ,CAAtB,EAAsCxH,CAAC,GAAGC,GAA1C,EAA+C,EAAED,CAAjD,EAAoD;CAClD,UAAIsH,CAAC,CAACtH,CAAD,CAAD,KAAS2F,CAAC,CAAC3F,CAAD,CAAd,EAAmB;CACjBuH,QAAAA,CAAC,GAAGD,CAAC,CAACtH,CAAD,CAAL;CACAwH,QAAAA,CAAC,GAAG7B,CAAC,CAAC3F,CAAD,CAAL;CACA;CACD;CACF;;CAED,QAAIuH,CAAC,GAAGC,CAAR,EAAW,OAAO,CAAC,CAAR;CACX,QAAIA,CAAC,GAAGD,CAAR,EAAW,OAAO,CAAP;CACX,WAAO,CAAP;CACD,GAzBD;;CA2BA/D,EAAAA,MAAM,CAAC8C,UAAP,GAAoB,SAASA,UAAT,CAAqBJ,QAArB,EAA+B;CACjD,YAAQwB,MAAM,CAACxB,QAAD,CAAN,CAAiByB,WAAjB,EAAR;CACE,WAAK,KAAL;CACA,WAAK,MAAL;CACA,WAAK,OAAL;CACA,WAAK,OAAL;CACA,WAAK,QAAL;CACA,WAAK,QAAL;CACA,WAAK,QAAL;CACA,WAAK,MAAL;CACA,WAAK,OAAL;CACA,WAAK,SAAL;CACA,WAAK,UAAL;CACE,eAAO,IAAP;;CACF;CACE,eAAO,KAAP;CAdJ;CAgBD,GAjBD;;CAmBAnE,EAAAA,MAAM,CAACoE,MAAP,GAAgB,SAASA,MAAT,CAAiBC,IAAjB,EAAuB3H,MAAvB,EAA+B;CAC7C,QAAI,CAACJ,KAAK,CAACmH,OAAN,CAAcY,IAAd,CAAL,EAA0B;CACxB,YAAM,IAAI9C,SAAJ,CAAc,6CAAd,CAAN;CACD;;CAED,QAAI8C,IAAI,CAAC3H,MAAL,KAAgB,CAApB,EAAuB;CACrB,aAAOsD,MAAM,CAACwC,KAAP,CAAa,CAAb,CAAP;CACD;;CAED,QAAIhG,CAAJ;;CACA,QAAIE,MAAM,KAAKsE,SAAf,EAA0B;CACxBtE,MAAAA,MAAM,GAAG,CAAT;;CACA,WAAKF,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAG6H,IAAI,CAAC3H,MAArB,EAA6B,EAAEF,CAA/B,EAAkC;CAChCE,QAAAA,MAAM,IAAI2H,IAAI,CAAC7H,CAAD,CAAJ,CAAQE,MAAlB;CACD;CACF;;CAED,QAAI0B,MAAM,GAAG4B,MAAM,CAACwB,WAAP,CAAmB9E,MAAnB,CAAb;CACA,QAAI4H,GAAG,GAAG,CAAV;;CACA,SAAK9H,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAG6H,IAAI,CAAC3H,MAArB,EAA6B,EAAEF,CAA/B,EAAkC;CAChC,UAAI4E,GAAG,GAAGiD,IAAI,CAAC7H,CAAD,CAAd;;CACA,UAAIuF,UAAU,CAACX,GAAD,EAAM/E,UAAN,CAAd,EAAiC;CAC/B,YAAIiI,GAAG,GAAGlD,GAAG,CAAC1E,MAAV,GAAmB0B,MAAM,CAAC1B,MAA9B,EAAsC;CACpCsD,UAAAA,MAAM,CAACyB,IAAP,CAAYL,GAAZ,EAAiBiC,IAAjB,CAAsBjF,MAAtB,EAA8BkG,GAA9B;CACD,SAFD,MAEO;CACLjI,UAAAA,UAAU,CAACsE,SAAX,CAAqB4D,GAArB,CAAyBC,IAAzB,CACEpG,MADF,EAEEgD,GAFF,EAGEkD,GAHF;CAKD;CACF,OAVD,MAUO,IAAI,CAACtE,MAAM,CAACe,QAAP,CAAgBK,GAAhB,CAAL,EAA2B;CAChC,cAAM,IAAIG,SAAJ,CAAc,6CAAd,CAAN;CACD,OAFM,MAEA;CACLH,QAAAA,GAAG,CAACiC,IAAJ,CAASjF,MAAT,EAAiBkG,GAAjB;CACD;;CACDA,MAAAA,GAAG,IAAIlD,GAAG,CAAC1E,MAAX;CACD;;CACD,WAAO0B,MAAP;CACD,GAvCD;;CAyCA,WAASrC,UAAT,CAAqB8G,MAArB,EAA6BH,QAA7B,EAAuC;CACrC,QAAI1C,MAAM,CAACe,QAAP,CAAgB8B,MAAhB,CAAJ,EAA6B;CAC3B,aAAOA,MAAM,CAACnG,MAAd;CACD;;CACD,QAAIkF,WAAW,CAACC,MAAZ,CAAmBgB,MAAnB,KAA8Bd,UAAU,CAACc,MAAD,EAASjB,WAAT,CAA5C,EAAmE;CACjE,aAAOiB,MAAM,CAAC9G,UAAd;CACD;;CACD,QAAI,OAAO8G,MAAP,KAAkB,QAAtB,EAAgC;CAC9B,YAAM,IAAItB,SAAJ,CACJ,+EACA,gBADA,0BAC0BsB,MAD1B,CADI,CAAN;CAID;;CAED,QAAIpG,GAAG,GAAGoG,MAAM,CAACnG,MAAjB;CACA,QAAI+H,SAAS,GAAIC,SAAS,CAAChI,MAAV,GAAmB,CAAnB,IAAwBgI,SAAS,CAAC,CAAD,CAAT,KAAiB,IAA1D;CACA,QAAI,CAACD,SAAD,IAAchI,GAAG,KAAK,CAA1B,EAA6B,OAAO,CAAP,CAhBQ;;CAmBrC,QAAIkI,WAAW,GAAG,KAAlB;;CACA,aAAS;CACP,cAAQjC,QAAR;CACE,aAAK,OAAL;CACA,aAAK,QAAL;CACA,aAAK,QAAL;CACE,iBAAOjG,GAAP;;CACF,aAAK,MAAL;CACA,aAAK,OAAL;CACE,iBAAOmI,WAAW,CAAC/B,MAAD,CAAX,CAAoBnG,MAA3B;;CACF,aAAK,MAAL;CACA,aAAK,OAAL;CACA,aAAK,SAAL;CACA,aAAK,UAAL;CACE,iBAAOD,GAAG,GAAG,CAAb;;CACF,aAAK,KAAL;CACE,iBAAOA,GAAG,KAAK,CAAf;;CACF,aAAK,QAAL;CACE,iBAAOoI,aAAa,CAAChC,MAAD,CAAb,CAAsBnG,MAA7B;;CACF;CACE,cAAIiI,WAAJ,EAAiB;CACf,mBAAOF,SAAS,GAAG,CAAC,CAAJ,GAAQG,WAAW,CAAC/B,MAAD,CAAX,CAAoBnG,MAA5C,CADe;CAEhB;;CACDgG,UAAAA,QAAQ,GAAG,CAAC,KAAKA,QAAN,EAAgByB,WAAhB,EAAX;CACAQ,UAAAA,WAAW,GAAG,IAAd;CAtBJ;CAwBD;CACF;;CACD3E,EAAAA,MAAM,CAACjE,UAAP,GAAoBA,UAApB;;CAEA,WAAS+I,YAAT,CAAuBpC,QAAvB,EAAiC/E,KAAjC,EAAwCC,GAAxC,EAA6C;CAC3C,QAAI+G,WAAW,GAAG,KAAlB,CAD2C;;;;;;;CAU3C,QAAIhH,KAAK,KAAKqD,SAAV,IAAuBrD,KAAK,GAAG,CAAnC,EAAsC;CACpCA,MAAAA,KAAK,GAAG,CAAR;CACD,KAZ0C;;;;CAe3C,QAAIA,KAAK,GAAG,KAAKjB,MAAjB,EAAyB;CACvB,aAAO,EAAP;CACD;;CAED,QAAIkB,GAAG,KAAKoD,SAAR,IAAqBpD,GAAG,GAAG,KAAKlB,MAApC,EAA4C;CAC1CkB,MAAAA,GAAG,GAAG,KAAKlB,MAAX;CACD;;CAED,QAAIkB,GAAG,IAAI,CAAX,EAAc;CACZ,aAAO,EAAP;CACD,KAzB0C;;;CA4B3CA,IAAAA,GAAG,MAAM,CAAT;CACAD,IAAAA,KAAK,MAAM,CAAX;;CAEA,QAAIC,GAAG,IAAID,KAAX,EAAkB;CAChB,aAAO,EAAP;CACD;;CAED,QAAI,CAAC+E,QAAL,EAAeA,QAAQ,GAAG,MAAX;;CAEf,WAAO,IAAP,EAAa;CACX,cAAQA,QAAR;CACE,aAAK,KAAL;CACE,iBAAOqC,QAAQ,CAAC,IAAD,EAAOpH,KAAP,EAAcC,GAAd,CAAf;;CAEF,aAAK,MAAL;CACA,aAAK,OAAL;CACE,iBAAOoH,SAAS,CAAC,IAAD,EAAOrH,KAAP,EAAcC,GAAd,CAAhB;;CAEF,aAAK,OAAL;CACE,iBAAOqH,UAAU,CAAC,IAAD,EAAOtH,KAAP,EAAcC,GAAd,CAAjB;;CAEF,aAAK,QAAL;CACA,aAAK,QAAL;CACE,iBAAOsH,WAAW,CAAC,IAAD,EAAOvH,KAAP,EAAcC,GAAd,CAAlB;;CAEF,aAAK,QAAL;CACE,iBAAOuH,WAAW,CAAC,IAAD,EAAOxH,KAAP,EAAcC,GAAd,CAAlB;;CAEF,aAAK,MAAL;CACA,aAAK,OAAL;CACA,aAAK,SAAL;CACA,aAAK,UAAL;CACE,iBAAOwH,YAAY,CAAC,IAAD,EAAOzH,KAAP,EAAcC,GAAd,CAAnB;;CAEF;CACE,cAAI+G,WAAJ,EAAiB,MAAM,IAAIpD,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;CACjBA,UAAAA,QAAQ,GAAG,CAACA,QAAQ,GAAG,EAAZ,EAAgByB,WAAhB,EAAX;CACAQ,UAAAA,WAAW,GAAG,IAAd;CA3BJ;CA6BD;CACF;CAGD;CACA;CACA;CACA;CACA;;;CACA3E,EAAAA,MAAM,CAACW,SAAP,CAAiBiD,SAAjB,GAA6B,IAA7B;;CAEA,WAASyB,IAAT,CAAelD,CAAf,EAAkBmD,CAAlB,EAAqB5G,CAArB,EAAwB;CACtB,QAAIlC,CAAC,GAAG2F,CAAC,CAACmD,CAAD,CAAT;CACAnD,IAAAA,CAAC,CAACmD,CAAD,CAAD,GAAOnD,CAAC,CAACzD,CAAD,CAAR;CACAyD,IAAAA,CAAC,CAACzD,CAAD,CAAD,GAAOlC,CAAP;CACD;;CAEDwD,EAAAA,MAAM,CAACW,SAAP,CAAiB4E,MAAjB,GAA0B,SAASA,MAAT,GAAmB;CAC3C,QAAI9I,GAAG,GAAG,KAAKC,MAAf;;CACA,QAAID,GAAG,GAAG,CAAN,KAAY,CAAhB,EAAmB;CACjB,YAAM,IAAI0E,UAAJ,CAAe,2CAAf,CAAN;CACD;;CACD,SAAK,IAAI3E,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyBD,CAAC,IAAI,CAA9B,EAAiC;CAC/B6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAP,EAAUA,CAAC,GAAG,CAAd,CAAJ;CACD;;CACD,WAAO,IAAP;CACD,GATD;;CAWAwD,EAAAA,MAAM,CAACW,SAAP,CAAiB6E,MAAjB,GAA0B,SAASA,MAAT,GAAmB;CAC3C,QAAI/I,GAAG,GAAG,KAAKC,MAAf;;CACA,QAAID,GAAG,GAAG,CAAN,KAAY,CAAhB,EAAmB;CACjB,YAAM,IAAI0E,UAAJ,CAAe,2CAAf,CAAN;CACD;;CACD,SAAK,IAAI3E,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyBD,CAAC,IAAI,CAA9B,EAAiC;CAC/B6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAP,EAAUA,CAAC,GAAG,CAAd,CAAJ;CACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;CACD;;CACD,WAAO,IAAP;CACD,GAVD;;CAYAwD,EAAAA,MAAM,CAACW,SAAP,CAAiB8E,MAAjB,GAA0B,SAASA,MAAT,GAAmB;CAC3C,QAAIhJ,GAAG,GAAG,KAAKC,MAAf;;CACA,QAAID,GAAG,GAAG,CAAN,KAAY,CAAhB,EAAmB;CACjB,YAAM,IAAI0E,UAAJ,CAAe,2CAAf,CAAN;CACD;;CACD,SAAK,IAAI3E,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyBD,CAAC,IAAI,CAA9B,EAAiC;CAC/B6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAP,EAAUA,CAAC,GAAG,CAAd,CAAJ;CACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;CACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;CACA6I,MAAAA,IAAI,CAAC,IAAD,EAAO7I,CAAC,GAAG,CAAX,EAAcA,CAAC,GAAG,CAAlB,CAAJ;CACD;;CACD,WAAO,IAAP;CACD,GAZD;;CAcAwD,EAAAA,MAAM,CAACW,SAAP,CAAiBgD,QAAjB,GAA4B,SAASA,QAAT,GAAqB;CAC/C,QAAIjH,MAAM,GAAG,KAAKA,MAAlB;CACA,QAAIA,MAAM,KAAK,CAAf,EAAkB,OAAO,EAAP;CAClB,QAAIgI,SAAS,CAAChI,MAAV,KAAqB,CAAzB,EAA4B,OAAOsI,SAAS,CAAC,IAAD,EAAO,CAAP,EAAUtI,MAAV,CAAhB;CAC5B,WAAOoI,YAAY,CAACY,KAAb,CAAmB,IAAnB,EAAyBhB,SAAzB,CAAP;CACD,GALD;;CAOA1E,EAAAA,MAAM,CAACW,SAAP,CAAiBgF,cAAjB,GAAkC3F,MAAM,CAACW,SAAP,CAAiBgD,QAAnD;;CAEA3D,EAAAA,MAAM,CAACW,SAAP,CAAiBiF,MAAjB,GAA0B,SAASA,MAAT,CAAiBzD,CAAjB,EAAoB;CAC5C,QAAI,CAACnC,MAAM,CAACe,QAAP,CAAgBoB,CAAhB,CAAL,EAAyB,MAAM,IAAIZ,SAAJ,CAAc,2BAAd,CAAN;CACzB,QAAI,SAASY,CAAb,EAAgB,OAAO,IAAP;CAChB,WAAOnC,MAAM,CAAC6D,OAAP,CAAe,IAAf,EAAqB1B,CAArB,MAA4B,CAAnC;CACD,GAJD;;CAMAnC,EAAAA,MAAM,CAACW,SAAP,CAAiBkF,OAAjB,GAA2B,SAASA,OAAT,GAAoB;CAC7C,QAAIC,GAAG,GAAG,EAAV;CACA,QAAIC,GAAG,GAAGhG,OAAO,CAACiG,iBAAlB;CACAF,IAAAA,GAAG,GAAG,KAAKnC,QAAL,CAAc,KAAd,EAAqB,CAArB,EAAwBoC,GAAxB,EAA6BE,OAA7B,CAAqC,SAArC,EAAgD,KAAhD,EAAuDC,IAAvD,EAAN;CACA,QAAI,KAAKxJ,MAAL,GAAcqJ,GAAlB,EAAuBD,GAAG,IAAI,OAAP;CACvB,WAAO,aAAaA,GAAb,GAAmB,GAA1B;CACD,GAND;;CAOA,MAAIjG,mBAAJ,EAAyB;CACvBG,IAAAA,MAAM,CAACW,SAAP,CAAiBd,mBAAjB,IAAwCG,MAAM,CAACW,SAAP,CAAiBkF,OAAzD;CACD;;CAED7F,EAAAA,MAAM,CAACW,SAAP,CAAiBkD,OAAjB,GAA2B,SAASA,OAAT,CAAkBsC,MAAlB,EAA0BxI,KAA1B,EAAiCC,GAAjC,EAAsCwI,SAAtC,EAAiDC,OAAjD,EAA0D;CACnF,QAAItE,UAAU,CAACoE,MAAD,EAAS9J,UAAT,CAAd,EAAoC;CAClC8J,MAAAA,MAAM,GAAGnG,MAAM,CAACyB,IAAP,CAAY0E,MAAZ,EAAoBA,MAAM,CAAC9H,MAA3B,EAAmC8H,MAAM,CAACpK,UAA1C,CAAT;CACD;;CACD,QAAI,CAACiE,MAAM,CAACe,QAAP,CAAgBoF,MAAhB,CAAL,EAA8B;CAC5B,YAAM,IAAI5E,SAAJ,CACJ,qEACA,gBADA,0BAC2B4E,MAD3B,CADI,CAAN;CAID;;CAED,QAAIxI,KAAK,KAAKqD,SAAd,EAAyB;CACvBrD,MAAAA,KAAK,GAAG,CAAR;CACD;;CACD,QAAIC,GAAG,KAAKoD,SAAZ,EAAuB;CACrBpD,MAAAA,GAAG,GAAGuI,MAAM,GAAGA,MAAM,CAACzJ,MAAV,GAAmB,CAA/B;CACD;;CACD,QAAI0J,SAAS,KAAKpF,SAAlB,EAA6B;CAC3BoF,MAAAA,SAAS,GAAG,CAAZ;CACD;;CACD,QAAIC,OAAO,KAAKrF,SAAhB,EAA2B;CACzBqF,MAAAA,OAAO,GAAG,KAAK3J,MAAf;CACD;;CAED,QAAIiB,KAAK,GAAG,CAAR,IAAaC,GAAG,GAAGuI,MAAM,CAACzJ,MAA1B,IAAoC0J,SAAS,GAAG,CAAhD,IAAqDC,OAAO,GAAG,KAAK3J,MAAxE,EAAgF;CAC9E,YAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;CACD;;CAED,QAAIiF,SAAS,IAAIC,OAAb,IAAwB1I,KAAK,IAAIC,GAArC,EAA0C;CACxC,aAAO,CAAP;CACD;;CACD,QAAIwI,SAAS,IAAIC,OAAjB,EAA0B;CACxB,aAAO,CAAC,CAAR;CACD;;CACD,QAAI1I,KAAK,IAAIC,GAAb,EAAkB;CAChB,aAAO,CAAP;CACD;;CAEDD,IAAAA,KAAK,MAAM,CAAX;CACAC,IAAAA,GAAG,MAAM,CAAT;CACAwI,IAAAA,SAAS,MAAM,CAAf;CACAC,IAAAA,OAAO,MAAM,CAAb;CAEA,QAAI,SAASF,MAAb,EAAqB,OAAO,CAAP;CAErB,QAAIpC,CAAC,GAAGsC,OAAO,GAAGD,SAAlB;CACA,QAAIpC,CAAC,GAAGpG,GAAG,GAAGD,KAAd;CACA,QAAIlB,GAAG,GAAG0C,IAAI,CAAC8E,GAAL,CAASF,CAAT,EAAYC,CAAZ,CAAV;CAEA,QAAIsC,QAAQ,GAAG,KAAKrD,KAAL,CAAWmD,SAAX,EAAsBC,OAAtB,CAAf;CACA,QAAIE,UAAU,GAAGJ,MAAM,CAAClD,KAAP,CAAatF,KAAb,EAAoBC,GAApB,CAAjB;;CAEA,SAAK,IAAIpB,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,GAApB,EAAyB,EAAED,CAA3B,EAA8B;CAC5B,UAAI8J,QAAQ,CAAC9J,CAAD,CAAR,KAAgB+J,UAAU,CAAC/J,CAAD,CAA9B,EAAmC;CACjCuH,QAAAA,CAAC,GAAGuC,QAAQ,CAAC9J,CAAD,CAAZ;CACAwH,QAAAA,CAAC,GAAGuC,UAAU,CAAC/J,CAAD,CAAd;CACA;CACD;CACF;;CAED,QAAIuH,CAAC,GAAGC,CAAR,EAAW,OAAO,CAAC,CAAR;CACX,QAAIA,CAAC,GAAGD,CAAR,EAAW,OAAO,CAAP;CACX,WAAO,CAAP;CACD,GA/DD;CAkEA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;;CACA,WAASyC,oBAAT,CAA+BpI,MAA/B,EAAuCqI,GAAvC,EAA4CxF,UAA5C,EAAwDyB,QAAxD,EAAkEgE,GAAlE,EAAuE;;CAErE,QAAItI,MAAM,CAAC1B,MAAP,KAAkB,CAAtB,EAAyB,OAAO,CAAC,CAAR,CAF4C;;CAKrE,QAAI,OAAOuE,UAAP,KAAsB,QAA1B,EAAoC;CAClCyB,MAAAA,QAAQ,GAAGzB,UAAX;CACAA,MAAAA,UAAU,GAAG,CAAb;CACD,KAHD,MAGO,IAAIA,UAAU,GAAG,UAAjB,EAA6B;CAClCA,MAAAA,UAAU,GAAG,UAAb;CACD,KAFM,MAEA,IAAIA,UAAU,GAAG,CAAC,UAAlB,EAA8B;CACnCA,MAAAA,UAAU,GAAG,CAAC,UAAd;CACD;;CACDA,IAAAA,UAAU,GAAG,CAACA,UAAd,CAbqE;;CAcrE,QAAIsC,WAAW,CAACtC,UAAD,CAAf,EAA6B;;CAE3BA,MAAAA,UAAU,GAAGyF,GAAG,GAAG,CAAH,GAAQtI,MAAM,CAAC1B,MAAP,GAAgB,CAAxC;CACD,KAjBoE;;;CAoBrE,QAAIuE,UAAU,GAAG,CAAjB,EAAoBA,UAAU,GAAG7C,MAAM,CAAC1B,MAAP,GAAgBuE,UAA7B;;CACpB,QAAIA,UAAU,IAAI7C,MAAM,CAAC1B,MAAzB,EAAiC;CAC/B,UAAIgK,GAAJ,EAAS,OAAO,CAAC,CAAR,CAAT,KACKzF,UAAU,GAAG7C,MAAM,CAAC1B,MAAP,GAAgB,CAA7B;CACN,KAHD,MAGO,IAAIuE,UAAU,GAAG,CAAjB,EAAoB;CACzB,UAAIyF,GAAJ,EAASzF,UAAU,GAAG,CAAb,CAAT,KACK,OAAO,CAAC,CAAR;CACN,KA3BoE;;;CA8BrE,QAAI,OAAOwF,GAAP,KAAe,QAAnB,EAA6B;CAC3BA,MAAAA,GAAG,GAAGzG,MAAM,CAACyB,IAAP,CAAYgF,GAAZ,EAAiB/D,QAAjB,CAAN;CACD,KAhCoE;;;CAmCrE,QAAI1C,MAAM,CAACe,QAAP,CAAgB0F,GAAhB,CAAJ,EAA0B;;CAExB,UAAIA,GAAG,CAAC/J,MAAJ,KAAe,CAAnB,EAAsB;CACpB,eAAO,CAAC,CAAR;CACD;;CACD,aAAOiK,YAAY,CAACvI,MAAD,EAASqI,GAAT,EAAcxF,UAAd,EAA0ByB,QAA1B,EAAoCgE,GAApC,CAAnB;CACD,KAND,MAMO,IAAI,OAAOD,GAAP,KAAe,QAAnB,EAA6B;CAClCA,MAAAA,GAAG,GAAGA,GAAG,GAAG,IAAZ,CADkC;;CAElC,UAAI,OAAOpK,UAAU,CAACsE,SAAX,CAAqB3D,OAA5B,KAAwC,UAA5C,EAAwD;CACtD,YAAI0J,GAAJ,EAAS;CACP,iBAAOrK,UAAU,CAACsE,SAAX,CAAqB3D,OAArB,CAA6BwH,IAA7B,CAAkCpG,MAAlC,EAA0CqI,GAA1C,EAA+CxF,UAA/C,CAAP;CACD,SAFD,MAEO;CACL,iBAAO5E,UAAU,CAACsE,SAAX,CAAqBiG,WAArB,CAAiCpC,IAAjC,CAAsCpG,MAAtC,EAA8CqI,GAA9C,EAAmDxF,UAAnD,CAAP;CACD;CACF;;CACD,aAAO0F,YAAY,CAACvI,MAAD,EAAS,CAACqI,GAAD,CAAT,EAAgBxF,UAAhB,EAA4ByB,QAA5B,EAAsCgE,GAAtC,CAAnB;CACD;;CAED,UAAM,IAAInF,SAAJ,CAAc,sCAAd,CAAN;CACD;;CAED,WAASoF,YAAT,CAAuBtJ,GAAvB,EAA4BoJ,GAA5B,EAAiCxF,UAAjC,EAA6CyB,QAA7C,EAAuDgE,GAAvD,EAA4D;CAC1D,QAAIG,SAAS,GAAG,CAAhB;CACA,QAAIC,SAAS,GAAGzJ,GAAG,CAACX,MAApB;CACA,QAAIqK,SAAS,GAAGN,GAAG,CAAC/J,MAApB;;CAEA,QAAIgG,QAAQ,KAAK1B,SAAjB,EAA4B;CAC1B0B,MAAAA,QAAQ,GAAGwB,MAAM,CAACxB,QAAD,CAAN,CAAiByB,WAAjB,EAAX;;CACA,UAAIzB,QAAQ,KAAK,MAAb,IAAuBA,QAAQ,KAAK,OAApC,IACAA,QAAQ,KAAK,SADb,IAC0BA,QAAQ,KAAK,UAD3C,EACuD;CACrD,YAAIrF,GAAG,CAACX,MAAJ,GAAa,CAAb,IAAkB+J,GAAG,CAAC/J,MAAJ,GAAa,CAAnC,EAAsC;CACpC,iBAAO,CAAC,CAAR;CACD;;CACDmK,QAAAA,SAAS,GAAG,CAAZ;CACAC,QAAAA,SAAS,IAAI,CAAb;CACAC,QAAAA,SAAS,IAAI,CAAb;CACA9F,QAAAA,UAAU,IAAI,CAAd;CACD;CACF;;CAED,aAAS+F,IAAT,CAAe5F,GAAf,EAAoB5E,CAApB,EAAuB;CACrB,UAAIqK,SAAS,KAAK,CAAlB,EAAqB;CACnB,eAAOzF,GAAG,CAAC5E,CAAD,CAAV;CACD,OAFD,MAEO;CACL,eAAO4E,GAAG,CAAC6F,YAAJ,CAAiBzK,CAAC,GAAGqK,SAArB,CAAP;CACD;CACF;;CAED,QAAIrK,CAAJ;;CACA,QAAIkK,GAAJ,EAAS;CACP,UAAIQ,UAAU,GAAG,CAAC,CAAlB;;CACA,WAAK1K,CAAC,GAAGyE,UAAT,EAAqBzE,CAAC,GAAGsK,SAAzB,EAAoCtK,CAAC,EAArC,EAAyC;CACvC,YAAIwK,IAAI,CAAC3J,GAAD,EAAMb,CAAN,CAAJ,KAAiBwK,IAAI,CAACP,GAAD,EAAMS,UAAU,KAAK,CAAC,CAAhB,GAAoB,CAApB,GAAwB1K,CAAC,GAAG0K,UAAlC,CAAzB,EAAwE;CACtE,cAAIA,UAAU,KAAK,CAAC,CAApB,EAAuBA,UAAU,GAAG1K,CAAb;CACvB,cAAIA,CAAC,GAAG0K,UAAJ,GAAiB,CAAjB,KAAuBH,SAA3B,EAAsC,OAAOG,UAAU,GAAGL,SAApB;CACvC,SAHD,MAGO;CACL,cAAIK,UAAU,KAAK,CAAC,CAApB,EAAuB1K,CAAC,IAAIA,CAAC,GAAG0K,UAAT;CACvBA,UAAAA,UAAU,GAAG,CAAC,CAAd;CACD;CACF;CACF,KAXD,MAWO;CACL,UAAIjG,UAAU,GAAG8F,SAAb,GAAyBD,SAA7B,EAAwC7F,UAAU,GAAG6F,SAAS,GAAGC,SAAzB;;CACxC,WAAKvK,CAAC,GAAGyE,UAAT,EAAqBzE,CAAC,IAAI,CAA1B,EAA6BA,CAAC,EAA9B,EAAkC;CAChC,YAAI2K,KAAK,GAAG,IAAZ;;CACA,aAAK,IAAIC,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGL,SAApB,EAA+BK,CAAC,EAAhC,EAAoC;CAClC,cAAIJ,IAAI,CAAC3J,GAAD,EAAMb,CAAC,GAAG4K,CAAV,CAAJ,KAAqBJ,IAAI,CAACP,GAAD,EAAMW,CAAN,CAA7B,EAAuC;CACrCD,YAAAA,KAAK,GAAG,KAAR;CACA;CACD;CACF;;CACD,YAAIA,KAAJ,EAAW,OAAO3K,CAAP;CACZ;CACF;;CAED,WAAO,CAAC,CAAR;CACD;;CAEDwD,EAAAA,MAAM,CAACW,SAAP,CAAiB0G,QAAjB,GAA4B,SAASA,QAAT,CAAmBZ,GAAnB,EAAwBxF,UAAxB,EAAoCyB,QAApC,EAA8C;CACxE,WAAO,KAAK1F,OAAL,CAAayJ,GAAb,EAAkBxF,UAAlB,EAA8ByB,QAA9B,MAA4C,CAAC,CAApD;CACD,GAFD;;CAIA1C,EAAAA,MAAM,CAACW,SAAP,CAAiB3D,OAAjB,GAA2B,SAASA,OAAT,CAAkByJ,GAAlB,EAAuBxF,UAAvB,EAAmCyB,QAAnC,EAA6C;CACtE,WAAO8D,oBAAoB,CAAC,IAAD,EAAOC,GAAP,EAAYxF,UAAZ,EAAwByB,QAAxB,EAAkC,IAAlC,CAA3B;CACD,GAFD;;CAIA1C,EAAAA,MAAM,CAACW,SAAP,CAAiBiG,WAAjB,GAA+B,SAASA,WAAT,CAAsBH,GAAtB,EAA2BxF,UAA3B,EAAuCyB,QAAvC,EAAiD;CAC9E,WAAO8D,oBAAoB,CAAC,IAAD,EAAOC,GAAP,EAAYxF,UAAZ,EAAwByB,QAAxB,EAAkC,KAAlC,CAA3B;CACD,GAFD;;CAIA,WAAS4E,QAAT,CAAmBlG,GAAnB,EAAwByB,MAAxB,EAAgCxE,MAAhC,EAAwC3B,MAAxC,EAAgD;CAC9C2B,IAAAA,MAAM,GAAGkJ,MAAM,CAAClJ,MAAD,CAAN,IAAkB,CAA3B;CACA,QAAImJ,SAAS,GAAGpG,GAAG,CAAC1E,MAAJ,GAAa2B,MAA7B;;CACA,QAAI,CAAC3B,MAAL,EAAa;CACXA,MAAAA,MAAM,GAAG8K,SAAT;CACD,KAFD,MAEO;CACL9K,MAAAA,MAAM,GAAG6K,MAAM,CAAC7K,MAAD,CAAf;;CACA,UAAIA,MAAM,GAAG8K,SAAb,EAAwB;CACtB9K,QAAAA,MAAM,GAAG8K,SAAT;CACD;CACF;;CAED,QAAIC,MAAM,GAAG5E,MAAM,CAACnG,MAApB;;CAEA,QAAIA,MAAM,GAAG+K,MAAM,GAAG,CAAtB,EAAyB;CACvB/K,MAAAA,MAAM,GAAG+K,MAAM,GAAG,CAAlB;CACD;;CACD,SAAK,IAAIjL,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4B,EAAEF,CAA9B,EAAiC;CAC/B,UAAIkL,MAAM,GAAGC,QAAQ,CAAC9E,MAAM,CAAC+E,MAAP,CAAcpL,CAAC,GAAG,CAAlB,EAAqB,CAArB,CAAD,EAA0B,EAA1B,CAArB;CACA,UAAI+G,WAAW,CAACmE,MAAD,CAAf,EAAyB,OAAOlL,CAAP;CACzB4E,MAAAA,GAAG,CAAC/C,MAAM,GAAG7B,CAAV,CAAH,GAAkBkL,MAAlB;CACD;;CACD,WAAOlL,CAAP;CACD;;CAED,WAASqL,SAAT,CAAoBzG,GAApB,EAAyByB,MAAzB,EAAiCxE,MAAjC,EAAyC3B,MAAzC,EAAiD;CAC/C,WAAOoL,UAAU,CAAClD,WAAW,CAAC/B,MAAD,EAASzB,GAAG,CAAC1E,MAAJ,GAAa2B,MAAtB,CAAZ,EAA2C+C,GAA3C,EAAgD/C,MAAhD,EAAwD3B,MAAxD,CAAjB;CACD;;CAED,WAASqL,UAAT,CAAqB3G,GAArB,EAA0ByB,MAA1B,EAAkCxE,MAAlC,EAA0C3B,MAA1C,EAAkD;CAChD,WAAOoL,UAAU,CAACE,YAAY,CAACnF,MAAD,CAAb,EAAuBzB,GAAvB,EAA4B/C,MAA5B,EAAoC3B,MAApC,CAAjB;CACD;;CAED,WAASuL,WAAT,CAAsB7G,GAAtB,EAA2ByB,MAA3B,EAAmCxE,MAAnC,EAA2C3B,MAA3C,EAAmD;CACjD,WAAOoL,UAAU,CAACjD,aAAa,CAAChC,MAAD,CAAd,EAAwBzB,GAAxB,EAA6B/C,MAA7B,EAAqC3B,MAArC,CAAjB;CACD;;CAED,WAASwL,SAAT,CAAoB9G,GAApB,EAAyByB,MAAzB,EAAiCxE,MAAjC,EAAyC3B,MAAzC,EAAiD;CAC/C,WAAOoL,UAAU,CAACK,cAAc,CAACtF,MAAD,EAASzB,GAAG,CAAC1E,MAAJ,GAAa2B,MAAtB,CAAf,EAA8C+C,GAA9C,EAAmD/C,MAAnD,EAA2D3B,MAA3D,CAAjB;CACD;;CAEDsD,EAAAA,MAAM,CAACW,SAAP,CAAiBqC,KAAjB,GAAyB,SAASA,KAAT,CAAgBH,MAAhB,EAAwBxE,MAAxB,EAAgC3B,MAAhC,EAAwCgG,QAAxC,EAAkD;;CAEzE,QAAIrE,MAAM,KAAK2C,SAAf,EAA0B;CACxB0B,MAAAA,QAAQ,GAAG,MAAX;CACAhG,MAAAA,MAAM,GAAG,KAAKA,MAAd;CACA2B,MAAAA,MAAM,GAAG,CAAT,CAHwB;CAKzB,KALD,MAKO,IAAI3B,MAAM,KAAKsE,SAAX,IAAwB,OAAO3C,MAAP,KAAkB,QAA9C,EAAwD;CAC7DqE,MAAAA,QAAQ,GAAGrE,MAAX;CACA3B,MAAAA,MAAM,GAAG,KAAKA,MAAd;CACA2B,MAAAA,MAAM,GAAG,CAAT,CAH6D;CAK9D,KALM,MAKA,IAAI+J,QAAQ,CAAC/J,MAAD,CAAZ,EAAsB;CAC3BA,MAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;CACA,UAAI+J,QAAQ,CAAC1L,MAAD,CAAZ,EAAsB;CACpBA,QAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,YAAIgG,QAAQ,KAAK1B,SAAjB,EAA4B0B,QAAQ,GAAG,MAAX;CAC7B,OAHD,MAGO;CACLA,QAAAA,QAAQ,GAAGhG,MAAX;CACAA,QAAAA,MAAM,GAAGsE,SAAT;CACD;CACF,KATM,MASA;CACL,YAAM,IAAIlE,KAAJ,CACJ,yEADI,CAAN;CAGD;;CAED,QAAI0K,SAAS,GAAG,KAAK9K,MAAL,GAAc2B,MAA9B;CACA,QAAI3B,MAAM,KAAKsE,SAAX,IAAwBtE,MAAM,GAAG8K,SAArC,EAAgD9K,MAAM,GAAG8K,SAAT;;CAEhD,QAAK3E,MAAM,CAACnG,MAAP,GAAgB,CAAhB,KAAsBA,MAAM,GAAG,CAAT,IAAc2B,MAAM,GAAG,CAA7C,CAAD,IAAqDA,MAAM,GAAG,KAAK3B,MAAvE,EAA+E;CAC7E,YAAM,IAAIyE,UAAJ,CAAe,wCAAf,CAAN;CACD;;CAED,QAAI,CAACuB,QAAL,EAAeA,QAAQ,GAAG,MAAX;CAEf,QAAIiC,WAAW,GAAG,KAAlB;;CACA,aAAS;CACP,cAAQjC,QAAR;CACE,aAAK,KAAL;CACE,iBAAO4E,QAAQ,CAAC,IAAD,EAAOzE,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAf;;CAEF,aAAK,MAAL;CACA,aAAK,OAAL;CACE,iBAAOmL,SAAS,CAAC,IAAD,EAAOhF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAhB;;CAEF,aAAK,OAAL;CACA,aAAK,QAAL;CACA,aAAK,QAAL;CACE,iBAAOqL,UAAU,CAAC,IAAD,EAAOlF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAjB;;CAEF,aAAK,QAAL;;CAEE,iBAAOuL,WAAW,CAAC,IAAD,EAAOpF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAlB;;CAEF,aAAK,MAAL;CACA,aAAK,OAAL;CACA,aAAK,SAAL;CACA,aAAK,UAAL;CACE,iBAAOwL,SAAS,CAAC,IAAD,EAAOrF,MAAP,EAAexE,MAAf,EAAuB3B,MAAvB,CAAhB;;CAEF;CACE,cAAIiI,WAAJ,EAAiB,MAAM,IAAIpD,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;CACjBA,UAAAA,QAAQ,GAAG,CAAC,KAAKA,QAAN,EAAgByB,WAAhB,EAAX;CACAQ,UAAAA,WAAW,GAAG,IAAd;CA1BJ;CA4BD;CACF,GAnED;;CAqEA3E,EAAAA,MAAM,CAACW,SAAP,CAAiB0H,MAAjB,GAA0B,SAASA,MAAT,GAAmB;CAC3C,WAAO;CACL7E,MAAAA,IAAI,EAAE,QADD;CAELE,MAAAA,IAAI,EAAEpH,KAAK,CAACqE,SAAN,CAAgBsC,KAAhB,CAAsBuB,IAAtB,CAA2B,KAAK8D,IAAL,IAAa,IAAxC,EAA8C,CAA9C;CAFD,KAAP;CAID,GALD;;CAOA,WAASnD,WAAT,CAAsB/D,GAAtB,EAA2BzD,KAA3B,EAAkCC,GAAlC,EAAuC;CACrC,QAAID,KAAK,KAAK,CAAV,IAAeC,GAAG,KAAKwD,GAAG,CAAC1E,MAA/B,EAAuC;CACrC,aAAO6L,QAAM,CAACtM,aAAP,CAAqBmF,GAArB,CAAP;CACD,KAFD,MAEO;CACL,aAAOmH,QAAM,CAACtM,aAAP,CAAqBmF,GAAG,CAAC6B,KAAJ,CAAUtF,KAAV,EAAiBC,GAAjB,CAArB,CAAP;CACD;CACF;;CAED,WAASoH,SAAT,CAAoB5D,GAApB,EAAyBzD,KAAzB,EAAgCC,GAAhC,EAAqC;CACnCA,IAAAA,GAAG,GAAGuB,IAAI,CAAC8E,GAAL,CAAS7C,GAAG,CAAC1E,MAAb,EAAqBkB,GAArB,CAAN;CACA,QAAI4K,GAAG,GAAG,EAAV;CAEA,QAAIhM,CAAC,GAAGmB,KAAR;;CACA,WAAOnB,CAAC,GAAGoB,GAAX,EAAgB;CACd,UAAI6K,SAAS,GAAGrH,GAAG,CAAC5E,CAAD,CAAnB;CACA,UAAIkM,SAAS,GAAG,IAAhB;CACA,UAAIC,gBAAgB,GAAIF,SAAS,GAAG,IAAb,GACnB,CADmB,GAElBA,SAAS,GAAG,IAAb,GACI,CADJ,GAEKA,SAAS,GAAG,IAAb,GACI,CADJ,GAEI,CANZ;;CAQA,UAAIjM,CAAC,GAAGmM,gBAAJ,IAAwB/K,GAA5B,EAAiC;CAC/B,YAAIgL,UAAJ,EAAgBC,SAAhB,EAA2BC,UAA3B,EAAuCC,aAAvC;;CAEA,gBAAQJ,gBAAR;CACE,eAAK,CAAL;CACE,gBAAIF,SAAS,GAAG,IAAhB,EAAsB;CACpBC,cAAAA,SAAS,GAAGD,SAAZ;CACD;;CACD;;CACF,eAAK,CAAL;CACEG,YAAAA,UAAU,GAAGxH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;;CACA,gBAAI,CAACoM,UAAU,GAAG,IAAd,MAAwB,IAA5B,EAAkC;CAChCG,cAAAA,aAAa,GAAG,CAACN,SAAS,GAAG,IAAb,KAAsB,GAAtB,GAA6BG,UAAU,GAAG,IAA1D;;CACA,kBAAIG,aAAa,GAAG,IAApB,EAA0B;CACxBL,gBAAAA,SAAS,GAAGK,aAAZ;CACD;CACF;;CACD;;CACF,eAAK,CAAL;CACEH,YAAAA,UAAU,GAAGxH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;CACAqM,YAAAA,SAAS,GAAGzH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAf;;CACA,gBAAI,CAACoM,UAAU,GAAG,IAAd,MAAwB,IAAxB,IAAgC,CAACC,SAAS,GAAG,IAAb,MAAuB,IAA3D,EAAiE;CAC/DE,cAAAA,aAAa,GAAG,CAACN,SAAS,GAAG,GAAb,KAAqB,GAArB,GAA2B,CAACG,UAAU,GAAG,IAAd,KAAuB,GAAlD,GAAyDC,SAAS,GAAG,IAArF;;CACA,kBAAIE,aAAa,GAAG,KAAhB,KAA0BA,aAAa,GAAG,MAAhB,IAA0BA,aAAa,GAAG,MAApE,CAAJ,EAAiF;CAC/EL,gBAAAA,SAAS,GAAGK,aAAZ;CACD;CACF;;CACD;;CACF,eAAK,CAAL;CACEH,YAAAA,UAAU,GAAGxH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;CACAqM,YAAAA,SAAS,GAAGzH,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAf;CACAsM,YAAAA,UAAU,GAAG1H,GAAG,CAAC5E,CAAC,GAAG,CAAL,CAAhB;;CACA,gBAAI,CAACoM,UAAU,GAAG,IAAd,MAAwB,IAAxB,IAAgC,CAACC,SAAS,GAAG,IAAb,MAAuB,IAAvD,IAA+D,CAACC,UAAU,GAAG,IAAd,MAAwB,IAA3F,EAAiG;CAC/FC,cAAAA,aAAa,GAAG,CAACN,SAAS,GAAG,GAAb,KAAqB,IAArB,GAA4B,CAACG,UAAU,GAAG,IAAd,KAAuB,GAAnD,GAAyD,CAACC,SAAS,GAAG,IAAb,KAAsB,GAA/E,GAAsFC,UAAU,GAAG,IAAnH;;CACA,kBAAIC,aAAa,GAAG,MAAhB,IAA0BA,aAAa,GAAG,QAA9C,EAAwD;CACtDL,gBAAAA,SAAS,GAAGK,aAAZ;CACD;CACF;;CAlCL;CAoCD;;CAED,UAAIL,SAAS,KAAK,IAAlB,EAAwB;;;CAGtBA,QAAAA,SAAS,GAAG,MAAZ;CACAC,QAAAA,gBAAgB,GAAG,CAAnB;CACD,OALD,MAKO,IAAID,SAAS,GAAG,MAAhB,EAAwB;;CAE7BA,QAAAA,SAAS,IAAI,OAAb;CACAF,QAAAA,GAAG,CAAC1K,IAAJ,CAAS4K,SAAS,KAAK,EAAd,GAAmB,KAAnB,GAA2B,MAApC;CACAA,QAAAA,SAAS,GAAG,SAASA,SAAS,GAAG,KAAjC;CACD;;CAEDF,MAAAA,GAAG,CAAC1K,IAAJ,CAAS4K,SAAT;CACAlM,MAAAA,CAAC,IAAImM,gBAAL;CACD;;CAED,WAAOK,qBAAqB,CAACR,GAAD,CAA5B;CACD;CAGD;CACA;;;CACA,MAAIS,oBAAoB,GAAG,MAA3B;;CAEA,WAASD,qBAAT,CAAgCE,UAAhC,EAA4C;CAC1C,QAAIzM,GAAG,GAAGyM,UAAU,CAACxM,MAArB;;CACA,QAAID,GAAG,IAAIwM,oBAAX,EAAiC;CAC/B,aAAO/E,MAAM,CAACiF,YAAP,CAAoBzD,KAApB,CAA0BxB,MAA1B,EAAkCgF,UAAlC,CAAP,CAD+B;CAEhC,KAJyC;;;CAO1C,QAAIV,GAAG,GAAG,EAAV;CACA,QAAIhM,CAAC,GAAG,CAAR;;CACA,WAAOA,CAAC,GAAGC,GAAX,EAAgB;CACd+L,MAAAA,GAAG,IAAItE,MAAM,CAACiF,YAAP,CAAoBzD,KAApB,CACLxB,MADK,EAELgF,UAAU,CAACjG,KAAX,CAAiBzG,CAAjB,EAAoBA,CAAC,IAAIyM,oBAAzB,CAFK,CAAP;CAID;;CACD,WAAOT,GAAP;CACD;;CAED,WAASvD,UAAT,CAAqB7D,GAArB,EAA0BzD,KAA1B,EAAiCC,GAAjC,EAAsC;CACpC,QAAIwL,GAAG,GAAG,EAAV;CACAxL,IAAAA,GAAG,GAAGuB,IAAI,CAAC8E,GAAL,CAAS7C,GAAG,CAAC1E,MAAb,EAAqBkB,GAArB,CAAN;;CAEA,SAAK,IAAIpB,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6B,EAAEpB,CAA/B,EAAkC;CAChC4M,MAAAA,GAAG,IAAIlF,MAAM,CAACiF,YAAP,CAAoB/H,GAAG,CAAC5E,CAAD,CAAH,GAAS,IAA7B,CAAP;CACD;;CACD,WAAO4M,GAAP;CACD;;CAED,WAASlE,WAAT,CAAsB9D,GAAtB,EAA2BzD,KAA3B,EAAkCC,GAAlC,EAAuC;CACrC,QAAIwL,GAAG,GAAG,EAAV;CACAxL,IAAAA,GAAG,GAAGuB,IAAI,CAAC8E,GAAL,CAAS7C,GAAG,CAAC1E,MAAb,EAAqBkB,GAArB,CAAN;;CAEA,SAAK,IAAIpB,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6B,EAAEpB,CAA/B,EAAkC;CAChC4M,MAAAA,GAAG,IAAIlF,MAAM,CAACiF,YAAP,CAAoB/H,GAAG,CAAC5E,CAAD,CAAvB,CAAP;CACD;;CACD,WAAO4M,GAAP;CACD;;CAED,WAASrE,QAAT,CAAmB3D,GAAnB,EAAwBzD,KAAxB,EAA+BC,GAA/B,EAAoC;CAClC,QAAInB,GAAG,GAAG2E,GAAG,CAAC1E,MAAd;CAEA,QAAI,CAACiB,KAAD,IAAUA,KAAK,GAAG,CAAtB,EAAyBA,KAAK,GAAG,CAAR;CACzB,QAAI,CAACC,GAAD,IAAQA,GAAG,GAAG,CAAd,IAAmBA,GAAG,GAAGnB,GAA7B,EAAkCmB,GAAG,GAAGnB,GAAN;CAElC,QAAI4M,GAAG,GAAG,EAAV;;CACA,SAAK,IAAI7M,CAAC,GAAGmB,KAAb,EAAoBnB,CAAC,GAAGoB,GAAxB,EAA6B,EAAEpB,CAA/B,EAAkC;CAChC6M,MAAAA,GAAG,IAAIC,mBAAmB,CAAClI,GAAG,CAAC5E,CAAD,CAAJ,CAA1B;CACD;;CACD,WAAO6M,GAAP;CACD;;CAED,WAASjE,YAAT,CAAuBhE,GAAvB,EAA4BzD,KAA5B,EAAmCC,GAAnC,EAAwC;CACtC,QAAI2L,KAAK,GAAGnI,GAAG,CAAC6B,KAAJ,CAAUtF,KAAV,EAAiBC,GAAjB,CAAZ;CACA,QAAI4K,GAAG,GAAG,EAAV,CAFsC;;CAItC,SAAK,IAAIhM,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAG+M,KAAK,CAAC7M,MAAN,GAAe,CAAnC,EAAsCF,CAAC,IAAI,CAA3C,EAA8C;CAC5CgM,MAAAA,GAAG,IAAItE,MAAM,CAACiF,YAAP,CAAoBI,KAAK,CAAC/M,CAAD,CAAL,GAAY+M,KAAK,CAAC/M,CAAC,GAAG,CAAL,CAAL,GAAe,GAA/C,CAAP;CACD;;CACD,WAAOgM,GAAP;CACD;;CAEDxI,EAAAA,MAAM,CAACW,SAAP,CAAiBsC,KAAjB,GAAyB,SAASA,KAAT,CAAgBtF,KAAhB,EAAuBC,GAAvB,EAA4B;CACnD,QAAInB,GAAG,GAAG,KAAKC,MAAf;CACAiB,IAAAA,KAAK,GAAG,CAAC,CAACA,KAAV;CACAC,IAAAA,GAAG,GAAGA,GAAG,KAAKoD,SAAR,GAAoBvE,GAApB,GAA0B,CAAC,CAACmB,GAAlC;;CAEA,QAAID,KAAK,GAAG,CAAZ,EAAe;CACbA,MAAAA,KAAK,IAAIlB,GAAT;CACA,UAAIkB,KAAK,GAAG,CAAZ,EAAeA,KAAK,GAAG,CAAR;CAChB,KAHD,MAGO,IAAIA,KAAK,GAAGlB,GAAZ,EAAiB;CACtBkB,MAAAA,KAAK,GAAGlB,GAAR;CACD;;CAED,QAAImB,GAAG,GAAG,CAAV,EAAa;CACXA,MAAAA,GAAG,IAAInB,GAAP;CACA,UAAImB,GAAG,GAAG,CAAV,EAAaA,GAAG,GAAG,CAAN;CACd,KAHD,MAGO,IAAIA,GAAG,GAAGnB,GAAV,EAAe;CACpBmB,MAAAA,GAAG,GAAGnB,GAAN;CACD;;CAED,QAAImB,GAAG,GAAGD,KAAV,EAAiBC,GAAG,GAAGD,KAAN;CAEjB,QAAI6L,MAAM,GAAG,KAAKC,QAAL,CAAc9L,KAAd,EAAqBC,GAArB,CAAb,CArBmD;;CAuBnD6C,IAAAA,MAAM,CAACC,cAAP,CAAsB8I,MAAtB,EAA8BxJ,MAAM,CAACW,SAArC;CAEA,WAAO6I,MAAP;CACD,GA1BD;CA4BA;CACA;CACA;;;CACA,WAASE,WAAT,CAAsBrL,MAAtB,EAA8BsL,GAA9B,EAAmCjN,MAAnC,EAA2C;CACzC,QAAK2B,MAAM,GAAG,CAAV,KAAiB,CAAjB,IAAsBA,MAAM,GAAG,CAAnC,EAAsC,MAAM,IAAI8C,UAAJ,CAAe,oBAAf,CAAN;CACtC,QAAI9C,MAAM,GAAGsL,GAAT,GAAejN,MAAnB,EAA2B,MAAM,IAAIyE,UAAJ,CAAe,uCAAf,CAAN;CAC5B;;CAEDnB,EAAAA,MAAM,CAACW,SAAP,CAAiBiJ,UAAjB,GACA5J,MAAM,CAACW,SAAP,CAAiBkJ,UAAjB,GAA8B,SAASA,UAAT,CAAqBxL,MAArB,EAA6BtC,UAA7B,EAAyC+N,QAAzC,EAAmD;CAC/EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;CACA,QAAI,CAAC+N,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;CAEf,QAAI+J,GAAG,GAAG,KAAKpI,MAAL,CAAV;CACA,QAAI0L,GAAG,GAAG,CAAV;CACA,QAAIvN,CAAC,GAAG,CAAR;;CACA,WAAO,EAAEA,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;CACzCtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG7B,CAAd,IAAmBuN,GAA1B;CACD;;CAED,WAAOtD,GAAP;CACD,GAdD;;CAgBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBqJ,UAAjB,GACAhK,MAAM,CAACW,SAAP,CAAiBsJ,UAAjB,GAA8B,SAASA,UAAT,CAAqB5L,MAArB,EAA6BtC,UAA7B,EAAyC+N,QAAzC,EAAmD;CAC/EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;;CACA,QAAI,CAAC+N,QAAL,EAAe;CACbJ,MAAAA,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;CACD;;CAED,QAAI+J,GAAG,GAAG,KAAKpI,MAAM,GAAG,EAAEtC,UAAhB,CAAV;CACA,QAAIgO,GAAG,GAAG,CAAV;;CACA,WAAOhO,UAAU,GAAG,CAAb,KAAmBgO,GAAG,IAAI,KAA1B,CAAP,EAAyC;CACvCtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG,EAAEtC,UAAhB,IAA8BgO,GAArC;CACD;;CAED,WAAOtD,GAAP;CACD,GAfD;;CAiBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBuJ,SAAjB,GACAlK,MAAM,CAACW,SAAP,CAAiBwJ,SAAjB,GAA6B,SAASA,SAAT,CAAoB9L,MAApB,EAA4ByL,QAA5B,EAAsC;CACjEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAO,KAAK2B,MAAL,CAAP;CACD,GALD;;CAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiByJ,YAAjB,GACApK,MAAM,CAACW,SAAP,CAAiB0J,YAAjB,GAAgC,SAASA,YAAT,CAAuBhM,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAO,KAAK2B,MAAL,IAAgB,KAAKA,MAAM,GAAG,CAAd,KAAoB,CAA3C;CACD,GALD;;CAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB2J,YAAjB,GACAtK,MAAM,CAACW,SAAP,CAAiBsG,YAAjB,GAAgC,SAASA,YAAT,CAAuB5I,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAQ,KAAK2B,MAAL,KAAgB,CAAjB,GAAsB,KAAKA,MAAM,GAAG,CAAd,CAA7B;CACD,GALD;;CAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB4J,YAAjB,GACAvK,MAAM,CAACW,SAAP,CAAiB6J,YAAjB,GAAgC,SAASA,YAAT,CAAuBnM,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CAEf,WAAO,CAAE,KAAK2B,MAAL,CAAD,GACH,KAAKA,MAAM,GAAG,CAAd,KAAoB,CADjB,GAEH,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAFlB,IAGF,KAAKA,MAAM,GAAG,CAAd,IAAmB,SAHxB;CAID,GATD;;CAWA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB8J,YAAjB,GACAzK,MAAM,CAACW,SAAP,CAAiB+J,YAAjB,GAAgC,SAASA,YAAT,CAAuBrM,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CAEf,WAAQ,KAAK2B,MAAL,IAAe,SAAhB,IACH,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAArB,GACA,KAAKA,MAAM,GAAG,CAAd,KAAoB,CADpB,GAED,KAAKA,MAAM,GAAG,CAAd,CAHK,CAAP;CAID,GATD;;CAWA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBgK,SAAjB,GAA6B,SAASA,SAAT,CAAoBtM,MAApB,EAA4BtC,UAA5B,EAAwC+N,QAAxC,EAAkD;CAC7EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;CACA,QAAI,CAAC+N,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;CAEf,QAAI+J,GAAG,GAAG,KAAKpI,MAAL,CAAV;CACA,QAAI0L,GAAG,GAAG,CAAV;CACA,QAAIvN,CAAC,GAAG,CAAR;;CACA,WAAO,EAAEA,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;CACzCtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG7B,CAAd,IAAmBuN,GAA1B;CACD;;CACDA,IAAAA,GAAG,IAAI,IAAP;CAEA,QAAItD,GAAG,IAAIsD,GAAX,EAAgBtD,GAAG,IAAItH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,CAAP;CAEhB,WAAO0K,GAAP;CACD,GAhBD;;CAkBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBiK,SAAjB,GAA6B,SAASA,SAAT,CAAoBvM,MAApB,EAA4BtC,UAA5B,EAAwC+N,QAAxC,EAAkD;CAC7EzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;CACA,QAAI,CAAC+N,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAStC,UAAT,EAAqB,KAAKW,MAA1B,CAAX;CAEf,QAAIF,CAAC,GAAGT,UAAR;CACA,QAAIgO,GAAG,GAAG,CAAV;CACA,QAAItD,GAAG,GAAG,KAAKpI,MAAM,GAAG,EAAE7B,CAAhB,CAAV;;CACA,WAAOA,CAAC,GAAG,CAAJ,KAAUuN,GAAG,IAAI,KAAjB,CAAP,EAAgC;CAC9BtD,MAAAA,GAAG,IAAI,KAAKpI,MAAM,GAAG,EAAE7B,CAAhB,IAAqBuN,GAA5B;CACD;;CACDA,IAAAA,GAAG,IAAI,IAAP;CAEA,QAAItD,GAAG,IAAIsD,GAAX,EAAgBtD,GAAG,IAAItH,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,CAAP;CAEhB,WAAO0K,GAAP;CACD,GAhBD;;CAkBAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBkK,QAAjB,GAA4B,SAASA,QAAT,CAAmBxM,MAAnB,EAA2ByL,QAA3B,EAAqC;CAC/DzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,QAAI,EAAE,KAAK2B,MAAL,IAAe,IAAjB,CAAJ,EAA4B,OAAQ,KAAKA,MAAL,CAAR;CAC5B,WAAQ,CAAC,OAAO,KAAKA,MAAL,CAAP,GAAsB,CAAvB,IAA4B,CAAC,CAArC;CACD,GALD;;CAOA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBmK,WAAjB,GAA+B,SAASA,WAAT,CAAsBzM,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,QAAI+J,GAAG,GAAG,KAAKpI,MAAL,IAAgB,KAAKA,MAAM,GAAG,CAAd,KAAoB,CAA9C;CACA,WAAQoI,GAAG,GAAG,MAAP,GAAiBA,GAAG,GAAG,UAAvB,GAAoCA,GAA3C;CACD,GALD;;CAOAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBoK,WAAjB,GAA+B,SAASA,WAAT,CAAsB1M,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,QAAI+J,GAAG,GAAG,KAAKpI,MAAM,GAAG,CAAd,IAAoB,KAAKA,MAAL,KAAgB,CAA9C;CACA,WAAQoI,GAAG,GAAG,MAAP,GAAiBA,GAAG,GAAG,UAAvB,GAAoCA,GAA3C;CACD,GALD;;CAOAzG,EAAAA,MAAM,CAACW,SAAP,CAAiBqK,WAAjB,GAA+B,SAASA,WAAT,CAAsB3M,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CAEf,WAAQ,KAAK2B,MAAL,CAAD,GACJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,CADhB,GAEJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAFhB,GAGJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,EAHvB;CAID,GARD;;CAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBsK,WAAjB,GAA+B,SAASA,WAAT,CAAsB5M,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CAEf,WAAQ,KAAK2B,MAAL,KAAgB,EAAjB,GACJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,EADhB,GAEJ,KAAKA,MAAM,GAAG,CAAd,KAAoB,CAFhB,GAGJ,KAAKA,MAAM,GAAG,CAAd,CAHH;CAID,GARD;;CAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBuK,WAAjB,GAA+B,SAASA,WAAT,CAAsB7M,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,IAA3B,EAAiC,EAAjC,EAAqC,CAArC,CAAP;CACD,GAJD;;CAMA2B,EAAAA,MAAM,CAACW,SAAP,CAAiByK,WAAjB,GAA+B,SAASA,WAAT,CAAsB/M,MAAtB,EAA8ByL,QAA9B,EAAwC;CACrEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,KAA3B,EAAkC,EAAlC,EAAsC,CAAtC,CAAP;CACD,GAJD;;CAMA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB0K,YAAjB,GAAgC,SAASA,YAAT,CAAuBhN,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,IAA3B,EAAiC,EAAjC,EAAqC,CAArC,CAAP;CACD,GAJD;;CAMA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB2K,YAAjB,GAAgC,SAASA,YAAT,CAAuBjN,MAAvB,EAA+ByL,QAA/B,EAAyC;CACvEzL,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeJ,WAAW,CAACrL,MAAD,EAAS,CAAT,EAAY,KAAK3B,MAAjB,CAAX;CACf,WAAOyO,OAAO,CAACnE,IAAR,CAAa,IAAb,EAAmB3I,MAAnB,EAA2B,KAA3B,EAAkC,EAAlC,EAAsC,CAAtC,CAAP;CACD,GAJD;;CAMA,WAASkN,QAAT,CAAmBnK,GAAnB,EAAwB/B,KAAxB,EAA+BhB,MAA/B,EAAuCsL,GAAvC,EAA4C5D,GAA5C,EAAiD9B,GAAjD,EAAsD;CACpD,QAAI,CAACjE,MAAM,CAACe,QAAP,CAAgBK,GAAhB,CAAL,EAA2B,MAAM,IAAIG,SAAJ,CAAc,6CAAd,CAAN;CAC3B,QAAIlC,KAAK,GAAG0G,GAAR,IAAe1G,KAAK,GAAG4E,GAA3B,EAAgC,MAAM,IAAI9C,UAAJ,CAAe,mCAAf,CAAN;CAChC,QAAI9C,MAAM,GAAGsL,GAAT,GAAevI,GAAG,CAAC1E,MAAvB,EAA+B,MAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;CAChC;;CAEDnB,EAAAA,MAAM,CAACW,SAAP,CAAiB6K,WAAjB,GACAxL,MAAM,CAACW,SAAP,CAAiB8K,WAAjB,GAA+B,SAASA,WAAT,CAAsBpM,KAAtB,EAA6BhB,MAA7B,EAAqCtC,UAArC,EAAiD+N,QAAjD,EAA2D;CACxFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;;CACA,QAAI,CAAC+N,QAAL,EAAe;CACb,UAAI4B,QAAQ,GAAGvM,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,IAA8B,CAA7C;CACAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkC2P,QAAlC,EAA4C,CAA5C,CAAR;CACD;;CAED,QAAI3B,GAAG,GAAG,CAAV;CACA,QAAIvN,CAAC,GAAG,CAAR;CACA,SAAK6B,MAAL,IAAegB,KAAK,GAAG,IAAvB;;CACA,WAAO,EAAE7C,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;CACzC,WAAK1L,MAAM,GAAG7B,CAAd,IAAoB6C,KAAK,GAAG0K,GAAT,GAAgB,IAAnC;CACD;;CAED,WAAO1L,MAAM,GAAGtC,UAAhB;CACD,GAlBD;;CAoBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiBgL,WAAjB,GACA3L,MAAM,CAACW,SAAP,CAAiBiL,WAAjB,GAA+B,SAASA,WAAT,CAAsBvM,KAAtB,EAA6BhB,MAA7B,EAAqCtC,UAArC,EAAiD+N,QAAjD,EAA2D;CACxFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACAtC,IAAAA,UAAU,GAAGA,UAAU,KAAK,CAA5B;;CACA,QAAI,CAAC+N,QAAL,EAAe;CACb,UAAI4B,QAAQ,GAAGvM,IAAI,CAACC,GAAL,CAAS,CAAT,EAAY,IAAIrD,UAAhB,IAA8B,CAA7C;CACAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkC2P,QAAlC,EAA4C,CAA5C,CAAR;CACD;;CAED,QAAIlP,CAAC,GAAGT,UAAU,GAAG,CAArB;CACA,QAAIgO,GAAG,GAAG,CAAV;CACA,SAAK1L,MAAM,GAAG7B,CAAd,IAAmB6C,KAAK,GAAG,IAA3B;;CACA,WAAO,EAAE7C,CAAF,IAAO,CAAP,KAAauN,GAAG,IAAI,KAApB,CAAP,EAAmC;CACjC,WAAK1L,MAAM,GAAG7B,CAAd,IAAoB6C,KAAK,GAAG0K,GAAT,GAAgB,IAAnC;CACD;;CAED,WAAO1L,MAAM,GAAGtC,UAAhB;CACD,GAlBD;;CAoBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiBkL,UAAjB,GACA7L,MAAM,CAACW,SAAP,CAAiBmL,UAAjB,GAA8B,SAASA,UAAT,CAAqBzM,KAArB,EAA4BhB,MAA5B,EAAoCyL,QAApC,EAA8C;CAC1EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,IAAzB,EAA+B,CAA/B,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAPD;;CASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBoL,aAAjB,GACA/L,MAAM,CAACW,SAAP,CAAiBqL,aAAjB,GAAiC,SAASA,aAAT,CAAwB3M,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAjC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GARD;;CAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBsL,aAAjB,GACAjM,MAAM,CAACW,SAAP,CAAiBuL,aAAjB,GAAiC,SAASA,aAAT,CAAwB7M,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAjC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,KAAK,CAA1B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GARD;;CAUA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBwL,aAAjB,GACAnM,MAAM,CAACW,SAAP,CAAiByL,aAAjB,GAAiC,SAASA,aAAT,CAAwB/M,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAArC,CAAR;CACf,SAAKA,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,SAAKhB,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAVD;;CAYA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB0L,aAAjB,GACArM,MAAM,CAACW,SAAP,CAAiB2L,aAAjB,GAAiC,SAASA,aAAT,CAAwBjN,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAArC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,KAAK,EAA1B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAVD;;CAYA2B,EAAAA,MAAM,CAACW,SAAP,CAAiB4L,UAAjB,GAA8B,SAASA,UAAT,CAAqBlN,KAArB,EAA4BhB,MAA5B,EAAoCtC,UAApC,EAAgD+N,QAAhD,EAA0D;CACtFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;CACA,QAAI,CAACyL,QAAL,EAAe;CACb,UAAI0C,KAAK,GAAGrN,IAAI,CAACC,GAAL,CAAS,CAAT,EAAa,IAAIrD,UAAL,GAAmB,CAA/B,CAAZ;CAEAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkCyQ,KAAK,GAAG,CAA1C,EAA6C,CAACA,KAA9C,CAAR;CACD;;CAED,QAAIhQ,CAAC,GAAG,CAAR;CACA,QAAIuN,GAAG,GAAG,CAAV;CACA,QAAI0C,GAAG,GAAG,CAAV;CACA,SAAKpO,MAAL,IAAegB,KAAK,GAAG,IAAvB;;CACA,WAAO,EAAE7C,CAAF,GAAMT,UAAN,KAAqBgO,GAAG,IAAI,KAA5B,CAAP,EAA2C;CACzC,UAAI1K,KAAK,GAAG,CAAR,IAAaoN,GAAG,KAAK,CAArB,IAA0B,KAAKpO,MAAM,GAAG7B,CAAT,GAAa,CAAlB,MAAyB,CAAvD,EAA0D;CACxDiQ,QAAAA,GAAG,GAAG,CAAN;CACD;;CACD,WAAKpO,MAAM,GAAG7B,CAAd,IAAmB,CAAE6C,KAAK,GAAG0K,GAAT,IAAiB,CAAlB,IAAuB0C,GAAvB,GAA6B,IAAhD;CACD;;CAED,WAAOpO,MAAM,GAAGtC,UAAhB;CACD,GArBD;;CAuBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiB+L,UAAjB,GAA8B,SAASA,UAAT,CAAqBrN,KAArB,EAA4BhB,MAA5B,EAAoCtC,UAApC,EAAgD+N,QAAhD,EAA0D;CACtFzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;CACA,QAAI,CAACyL,QAAL,EAAe;CACb,UAAI0C,KAAK,GAAGrN,IAAI,CAACC,GAAL,CAAS,CAAT,EAAa,IAAIrD,UAAL,GAAmB,CAA/B,CAAZ;CAEAwP,MAAAA,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsBtC,UAAtB,EAAkCyQ,KAAK,GAAG,CAA1C,EAA6C,CAACA,KAA9C,CAAR;CACD;;CAED,QAAIhQ,CAAC,GAAGT,UAAU,GAAG,CAArB;CACA,QAAIgO,GAAG,GAAG,CAAV;CACA,QAAI0C,GAAG,GAAG,CAAV;CACA,SAAKpO,MAAM,GAAG7B,CAAd,IAAmB6C,KAAK,GAAG,IAA3B;;CACA,WAAO,EAAE7C,CAAF,IAAO,CAAP,KAAauN,GAAG,IAAI,KAApB,CAAP,EAAmC;CACjC,UAAI1K,KAAK,GAAG,CAAR,IAAaoN,GAAG,KAAK,CAArB,IAA0B,KAAKpO,MAAM,GAAG7B,CAAT,GAAa,CAAlB,MAAyB,CAAvD,EAA0D;CACxDiQ,QAAAA,GAAG,GAAG,CAAN;CACD;;CACD,WAAKpO,MAAM,GAAG7B,CAAd,IAAmB,CAAE6C,KAAK,GAAG0K,GAAT,IAAiB,CAAlB,IAAuB0C,GAAvB,GAA6B,IAAhD;CACD;;CAED,WAAOpO,MAAM,GAAGtC,UAAhB;CACD,GArBD;;CAuBAiE,EAAAA,MAAM,CAACW,SAAP,CAAiBgM,SAAjB,GAA6B,SAASA,SAAT,CAAoBtN,KAApB,EAA2BhB,MAA3B,EAAmCyL,QAAnC,EAA6C;CACxEzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,IAAzB,EAA+B,CAAC,IAAhC,CAAR;CACf,QAAIgB,KAAK,GAAG,CAAZ,EAAeA,KAAK,GAAG,OAAOA,KAAP,GAAe,CAAvB;CACf,SAAKhB,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAPD;;CASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBiM,YAAjB,GAAgC,SAASA,YAAT,CAAuBvN,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAC,MAAlC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAPD;;CASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBkM,YAAjB,GAAgC,SAASA,YAAT,CAAuBxN,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,MAAzB,EAAiC,CAAC,MAAlC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,KAAK,CAA1B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAPD;;CASA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBmM,YAAjB,GAAgC,SAASA,YAAT,CAAuBzN,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAAC,UAAtC,CAAR;CACf,SAAKA,MAAL,IAAgBgB,KAAK,GAAG,IAAxB;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GATD;;CAWA2B,EAAAA,MAAM,CAACW,SAAP,CAAiBoM,YAAjB,GAAgC,SAASA,YAAT,CAAuB1N,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9EzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;CACA,QAAI,CAACyL,QAAL,EAAeyB,QAAQ,CAAC,IAAD,EAAOlM,KAAP,EAAchB,MAAd,EAAsB,CAAtB,EAAyB,UAAzB,EAAqC,CAAC,UAAtC,CAAR;CACf,QAAIgB,KAAK,GAAG,CAAZ,EAAeA,KAAK,GAAG,aAAaA,KAAb,GAAqB,CAA7B;CACf,SAAKhB,MAAL,IAAgBgB,KAAK,KAAK,EAA1B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,EAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,KAAK,CAA9B;CACA,SAAKhB,MAAM,GAAG,CAAd,IAAoBgB,KAAK,GAAG,IAA5B;CACA,WAAOhB,MAAM,GAAG,CAAhB;CACD,GAVD;;CAYA,WAAS2O,YAAT,CAAuB5L,GAAvB,EAA4B/B,KAA5B,EAAmChB,MAAnC,EAA2CsL,GAA3C,EAAgD5D,GAAhD,EAAqD9B,GAArD,EAA0D;CACxD,QAAI5F,MAAM,GAAGsL,GAAT,GAAevI,GAAG,CAAC1E,MAAvB,EAA+B,MAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;CAC/B,QAAI9C,MAAM,GAAG,CAAb,EAAgB,MAAM,IAAI8C,UAAJ,CAAe,oBAAf,CAAN;CACjB;;CAED,WAAS8L,UAAT,CAAqB7L,GAArB,EAA0B/B,KAA1B,EAAiChB,MAAjC,EAAyC6O,YAAzC,EAAuDpD,QAAvD,EAAiE;CAC/DzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;CACA,QAAI,CAACyL,QAAL,EAAe;CACbkD,MAAAA,YAAY,CAAC5L,GAAD,EAAM/B,KAAN,EAAahB,MAAb,EAAqB,CAArB,CAAZ;CACD;;CACD8M,IAAAA,OAAO,CAACnI,KAAR,CAAc5B,GAAd,EAAmB/B,KAAnB,EAA0BhB,MAA1B,EAAkC6O,YAAlC,EAAgD,EAAhD,EAAoD,CAApD;CACA,WAAO7O,MAAM,GAAG,CAAhB;CACD;;CAED2B,EAAAA,MAAM,CAACW,SAAP,CAAiBwM,YAAjB,GAAgC,SAASA,YAAT,CAAuB9N,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9E,WAAOmD,UAAU,CAAC,IAAD,EAAO5N,KAAP,EAAchB,MAAd,EAAsB,IAAtB,EAA4ByL,QAA5B,CAAjB;CACD,GAFD;;CAIA9J,EAAAA,MAAM,CAACW,SAAP,CAAiByM,YAAjB,GAAgC,SAASA,YAAT,CAAuB/N,KAAvB,EAA8BhB,MAA9B,EAAsCyL,QAAtC,EAAgD;CAC9E,WAAOmD,UAAU,CAAC,IAAD,EAAO5N,KAAP,EAAchB,MAAd,EAAsB,KAAtB,EAA6ByL,QAA7B,CAAjB;CACD,GAFD;;CAIA,WAASuD,WAAT,CAAsBjM,GAAtB,EAA2B/B,KAA3B,EAAkChB,MAAlC,EAA0C6O,YAA1C,EAAwDpD,QAAxD,EAAkE;CAChEzK,IAAAA,KAAK,GAAG,CAACA,KAAT;CACAhB,IAAAA,MAAM,GAAGA,MAAM,KAAK,CAApB;;CACA,QAAI,CAACyL,QAAL,EAAe;CACbkD,MAAAA,YAAY,CAAC5L,GAAD,EAAM/B,KAAN,EAAahB,MAAb,EAAqB,CAArB,CAAZ;CACD;;CACD8M,IAAAA,OAAO,CAACnI,KAAR,CAAc5B,GAAd,EAAmB/B,KAAnB,EAA0BhB,MAA1B,EAAkC6O,YAAlC,EAAgD,EAAhD,EAAoD,CAApD;CACA,WAAO7O,MAAM,GAAG,CAAhB;CACD;;CAED2B,EAAAA,MAAM,CAACW,SAAP,CAAiB2M,aAAjB,GAAiC,SAASA,aAAT,CAAwBjO,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChF,WAAOuD,WAAW,CAAC,IAAD,EAAOhO,KAAP,EAAchB,MAAd,EAAsB,IAAtB,EAA4ByL,QAA5B,CAAlB;CACD,GAFD;;CAIA9J,EAAAA,MAAM,CAACW,SAAP,CAAiB4M,aAAjB,GAAiC,SAASA,aAAT,CAAwBlO,KAAxB,EAA+BhB,MAA/B,EAAuCyL,QAAvC,EAAiD;CAChF,WAAOuD,WAAW,CAAC,IAAD,EAAOhO,KAAP,EAAchB,MAAd,EAAsB,KAAtB,EAA6ByL,QAA7B,CAAlB;CACD,GAFD;;;CAKA9J,EAAAA,MAAM,CAACW,SAAP,CAAiB0C,IAAjB,GAAwB,SAASA,IAAT,CAAe8C,MAAf,EAAuBqH,WAAvB,EAAoC7P,KAApC,EAA2CC,GAA3C,EAAgD;CACtE,QAAI,CAACoC,MAAM,CAACe,QAAP,CAAgBoF,MAAhB,CAAL,EAA8B,MAAM,IAAI5E,SAAJ,CAAc,6BAAd,CAAN;CAC9B,QAAI,CAAC5D,KAAL,EAAYA,KAAK,GAAG,CAAR;CACZ,QAAI,CAACC,GAAD,IAAQA,GAAG,KAAK,CAApB,EAAuBA,GAAG,GAAG,KAAKlB,MAAX;CACvB,QAAI8Q,WAAW,IAAIrH,MAAM,CAACzJ,MAA1B,EAAkC8Q,WAAW,GAAGrH,MAAM,CAACzJ,MAArB;CAClC,QAAI,CAAC8Q,WAAL,EAAkBA,WAAW,GAAG,CAAd;CAClB,QAAI5P,GAAG,GAAG,CAAN,IAAWA,GAAG,GAAGD,KAArB,EAA4BC,GAAG,GAAGD,KAAN,CAN0C;;CAStE,QAAIC,GAAG,KAAKD,KAAZ,EAAmB,OAAO,CAAP;CACnB,QAAIwI,MAAM,CAACzJ,MAAP,KAAkB,CAAlB,IAAuB,KAAKA,MAAL,KAAgB,CAA3C,EAA8C,OAAO,CAAP,CAVwB;;CAatE,QAAI8Q,WAAW,GAAG,CAAlB,EAAqB;CACnB,YAAM,IAAIrM,UAAJ,CAAe,2BAAf,CAAN;CACD;;CACD,QAAIxD,KAAK,GAAG,CAAR,IAAaA,KAAK,IAAI,KAAKjB,MAA/B,EAAuC,MAAM,IAAIyE,UAAJ,CAAe,oBAAf,CAAN;CACvC,QAAIvD,GAAG,GAAG,CAAV,EAAa,MAAM,IAAIuD,UAAJ,CAAe,yBAAf,CAAN,CAjByD;;CAoBtE,QAAIvD,GAAG,GAAG,KAAKlB,MAAf,EAAuBkB,GAAG,GAAG,KAAKlB,MAAX;;CACvB,QAAIyJ,MAAM,CAACzJ,MAAP,GAAgB8Q,WAAhB,GAA8B5P,GAAG,GAAGD,KAAxC,EAA+C;CAC7CC,MAAAA,GAAG,GAAGuI,MAAM,CAACzJ,MAAP,GAAgB8Q,WAAhB,GAA8B7P,KAApC;CACD;;CAED,QAAIlB,GAAG,GAAGmB,GAAG,GAAGD,KAAhB;;CAEA,QAAI,SAASwI,MAAT,IAAmB,OAAO9J,UAAU,CAACsE,SAAX,CAAqB8M,UAA5B,KAA2C,UAAlE,EAA8E;;CAE5E,WAAKA,UAAL,CAAgBD,WAAhB,EAA6B7P,KAA7B,EAAoCC,GAApC;CACD,KAHD,MAGO;CACLvB,MAAAA,UAAU,CAACsE,SAAX,CAAqB4D,GAArB,CAAyBC,IAAzB,CACE2B,MADF,EAEE,KAAKsD,QAAL,CAAc9L,KAAd,EAAqBC,GAArB,CAFF,EAGE4P,WAHF;CAKD;;CAED,WAAO/Q,GAAP;CACD,GAvCD;CA0CA;CACA;CACA;;;CACAuD,EAAAA,MAAM,CAACW,SAAP,CAAiB8B,IAAjB,GAAwB,SAASA,IAAT,CAAegE,GAAf,EAAoB9I,KAApB,EAA2BC,GAA3B,EAAgC8E,QAAhC,EAA0C;;CAEhE,QAAI,OAAO+D,GAAP,KAAe,QAAnB,EAA6B;CAC3B,UAAI,OAAO9I,KAAP,KAAiB,QAArB,EAA+B;CAC7B+E,QAAAA,QAAQ,GAAG/E,KAAX;CACAA,QAAAA,KAAK,GAAG,CAAR;CACAC,QAAAA,GAAG,GAAG,KAAKlB,MAAX;CACD,OAJD,MAIO,IAAI,OAAOkB,GAAP,KAAe,QAAnB,EAA6B;CAClC8E,QAAAA,QAAQ,GAAG9E,GAAX;CACAA,QAAAA,GAAG,GAAG,KAAKlB,MAAX;CACD;;CACD,UAAIgG,QAAQ,KAAK1B,SAAb,IAA0B,OAAO0B,QAAP,KAAoB,QAAlD,EAA4D;CAC1D,cAAM,IAAInB,SAAJ,CAAc,2BAAd,CAAN;CACD;;CACD,UAAI,OAAOmB,QAAP,KAAoB,QAApB,IAAgC,CAAC1C,MAAM,CAAC8C,UAAP,CAAkBJ,QAAlB,CAArC,EAAkE;CAChE,cAAM,IAAInB,SAAJ,CAAc,uBAAuBmB,QAArC,CAAN;CACD;;CACD,UAAI+D,GAAG,CAAC/J,MAAJ,KAAe,CAAnB,EAAsB;CACpB,YAAIH,IAAI,GAAGkK,GAAG,CAAC9J,UAAJ,CAAe,CAAf,CAAX;;CACA,YAAK+F,QAAQ,KAAK,MAAb,IAAuBnG,IAAI,GAAG,GAA/B,IACAmG,QAAQ,KAAK,QADjB,EAC2B;;CAEzB+D,UAAAA,GAAG,GAAGlK,IAAN;CACD;CACF;CACF,KAvBD,MAuBO,IAAI,OAAOkK,GAAP,KAAe,QAAnB,EAA6B;CAClCA,MAAAA,GAAG,GAAGA,GAAG,GAAG,GAAZ;CACD,KAFM,MAEA,IAAI,OAAOA,GAAP,KAAe,SAAnB,EAA8B;CACnCA,MAAAA,GAAG,GAAGc,MAAM,CAACd,GAAD,CAAZ;CACD,KA7B+D;;;CAgChE,QAAI9I,KAAK,GAAG,CAAR,IAAa,KAAKjB,MAAL,GAAciB,KAA3B,IAAoC,KAAKjB,MAAL,GAAckB,GAAtD,EAA2D;CACzD,YAAM,IAAIuD,UAAJ,CAAe,oBAAf,CAAN;CACD;;CAED,QAAIvD,GAAG,IAAID,KAAX,EAAkB;CAChB,aAAO,IAAP;CACD;;CAEDA,IAAAA,KAAK,GAAGA,KAAK,KAAK,CAAlB;CACAC,IAAAA,GAAG,GAAGA,GAAG,KAAKoD,SAAR,GAAoB,KAAKtE,MAAzB,GAAkCkB,GAAG,KAAK,CAAhD;CAEA,QAAI,CAAC6I,GAAL,EAAUA,GAAG,GAAG,CAAN;CAEV,QAAIjK,CAAJ;;CACA,QAAI,OAAOiK,GAAP,KAAe,QAAnB,EAA6B;CAC3B,WAAKjK,CAAC,GAAGmB,KAAT,EAAgBnB,CAAC,GAAGoB,GAApB,EAAyB,EAAEpB,CAA3B,EAA8B;CAC5B,aAAKA,CAAL,IAAUiK,GAAV;CACD;CACF,KAJD,MAIO;CACL,UAAI8C,KAAK,GAAGvJ,MAAM,CAACe,QAAP,CAAgB0F,GAAhB,IACRA,GADQ,GAERzG,MAAM,CAACyB,IAAP,CAAYgF,GAAZ,EAAiB/D,QAAjB,CAFJ;CAGA,UAAIjG,GAAG,GAAG8M,KAAK,CAAC7M,MAAhB;;CACA,UAAID,GAAG,KAAK,CAAZ,EAAe;CACb,cAAM,IAAI8E,SAAJ,CAAc,gBAAgBkF,GAAhB,GAClB,mCADI,CAAN;CAED;;CACD,WAAKjK,CAAC,GAAG,CAAT,EAAYA,CAAC,GAAGoB,GAAG,GAAGD,KAAtB,EAA6B,EAAEnB,CAA/B,EAAkC;CAChC,aAAKA,CAAC,GAAGmB,KAAT,IAAkB4L,KAAK,CAAC/M,CAAC,GAAGC,GAAL,CAAvB;CACD;CACF;;CAED,WAAO,IAAP;CACD,GAjED;CAoEA;;;CAEA,MAAIiR,iBAAiB,GAAG,mBAAxB;;CAEA,WAASC,WAAT,CAAsB7H,GAAtB,EAA2B;;CAEzBA,IAAAA,GAAG,GAAGA,GAAG,CAAC8H,KAAJ,CAAU,GAAV,EAAe,CAAf,CAAN,CAFyB;;CAIzB9H,IAAAA,GAAG,GAAGA,GAAG,CAACI,IAAJ,GAAWD,OAAX,CAAmByH,iBAAnB,EAAsC,EAAtC,CAAN,CAJyB;;CAMzB,QAAI5H,GAAG,CAACpJ,MAAJ,GAAa,CAAjB,EAAoB,OAAO,EAAP,CANK;;CAQzB,WAAOoJ,GAAG,CAACpJ,MAAJ,GAAa,CAAb,KAAmB,CAA1B,EAA6B;CAC3BoJ,MAAAA,GAAG,GAAGA,GAAG,GAAG,GAAZ;CACD;;CACD,WAAOA,GAAP;CACD;;CAED,WAASlB,WAAT,CAAsB/B,MAAtB,EAA8BgL,KAA9B,EAAqC;CACnCA,IAAAA,KAAK,GAAGA,KAAK,IAAI3O,QAAjB;CACA,QAAIwJ,SAAJ;CACA,QAAIhM,MAAM,GAAGmG,MAAM,CAACnG,MAApB;CACA,QAAIoR,aAAa,GAAG,IAApB;CACA,QAAIvE,KAAK,GAAG,EAAZ;;CAEA,SAAK,IAAI/M,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4B,EAAEF,CAA9B,EAAiC;CAC/BkM,MAAAA,SAAS,GAAG7F,MAAM,CAAClG,UAAP,CAAkBH,CAAlB,CAAZ,CAD+B;;CAI/B,UAAIkM,SAAS,GAAG,MAAZ,IAAsBA,SAAS,GAAG,MAAtC,EAA8C;;CAE5C,YAAI,CAACoF,aAAL,EAAoB;;CAElB,cAAIpF,SAAS,GAAG,MAAhB,EAAwB;;CAEtB,gBAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;CACvB;CACD,WAJD,MAIO,IAAItB,CAAC,GAAG,CAAJ,KAAUE,MAAd,EAAsB;;CAE3B,gBAAI,CAACmR,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;CACvB;CACD,WAViB;;;CAalBgQ,UAAAA,aAAa,GAAGpF,SAAhB;CAEA;CACD,SAlB2C;;;CAqB5C,YAAIA,SAAS,GAAG,MAAhB,EAAwB;CACtB,cAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;CACvBgQ,UAAAA,aAAa,GAAGpF,SAAhB;CACA;CACD,SAzB2C;;;CA4B5CA,QAAAA,SAAS,GAAG,CAACoF,aAAa,GAAG,MAAhB,IAA0B,EAA1B,GAA+BpF,SAAS,GAAG,MAA5C,IAAsD,OAAlE;CACD,OA7BD,MA6BO,IAAIoF,aAAJ,EAAmB;;CAExB,YAAI,CAACD,KAAK,IAAI,CAAV,IAAe,CAAC,CAApB,EAAuBtE,KAAK,CAACzL,IAAN,CAAW,IAAX,EAAiB,IAAjB,EAAuB,IAAvB;CACxB;;CAEDgQ,MAAAA,aAAa,GAAG,IAAhB,CAtC+B;;CAyC/B,UAAIpF,SAAS,GAAG,IAAhB,EAAsB;CACpB,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;CACtBtE,QAAAA,KAAK,CAACzL,IAAN,CAAW4K,SAAX;CACD,OAHD,MAGO,IAAIA,SAAS,GAAG,KAAhB,EAAuB;CAC5B,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;CACtBtE,QAAAA,KAAK,CAACzL,IAAN,CACE4K,SAAS,IAAI,GAAb,GAAmB,IADrB,EAEEA,SAAS,GAAG,IAAZ,GAAmB,IAFrB;CAID,OANM,MAMA,IAAIA,SAAS,GAAG,OAAhB,EAAyB;CAC9B,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;CACtBtE,QAAAA,KAAK,CAACzL,IAAN,CACE4K,SAAS,IAAI,GAAb,GAAmB,IADrB,EAEEA,SAAS,IAAI,GAAb,GAAmB,IAAnB,GAA0B,IAF5B,EAGEA,SAAS,GAAG,IAAZ,GAAmB,IAHrB;CAKD,OAPM,MAOA,IAAIA,SAAS,GAAG,QAAhB,EAA0B;CAC/B,YAAI,CAACmF,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;CACtBtE,QAAAA,KAAK,CAACzL,IAAN,CACE4K,SAAS,IAAI,IAAb,GAAoB,IADtB,EAEEA,SAAS,IAAI,GAAb,GAAmB,IAAnB,GAA0B,IAF5B,EAGEA,SAAS,IAAI,GAAb,GAAmB,IAAnB,GAA0B,IAH5B,EAIEA,SAAS,GAAG,IAAZ,GAAmB,IAJrB;CAMD,OARM,MAQA;CACL,cAAM,IAAI5L,KAAJ,CAAU,oBAAV,CAAN;CACD;CACF;;CAED,WAAOyM,KAAP;CACD;;CAED,WAASvB,YAAT,CAAuBlC,GAAvB,EAA4B;CAC1B,QAAIiI,SAAS,GAAG,EAAhB;;CACA,SAAK,IAAIvR,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGsJ,GAAG,CAACpJ,MAAxB,EAAgC,EAAEF,CAAlC,EAAqC;;CAEnCuR,MAAAA,SAAS,CAACjQ,IAAV,CAAegI,GAAG,CAACnJ,UAAJ,CAAeH,CAAf,IAAoB,IAAnC;CACD;;CACD,WAAOuR,SAAP;CACD;;CAED,WAAS5F,cAAT,CAAyBrC,GAAzB,EAA8B+H,KAA9B,EAAqC;CACnC,QAAIvO,CAAJ,EAAO0O,EAAP,EAAWC,EAAX;CACA,QAAIF,SAAS,GAAG,EAAhB;;CACA,SAAK,IAAIvR,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGsJ,GAAG,CAACpJ,MAAxB,EAAgC,EAAEF,CAAlC,EAAqC;CACnC,UAAI,CAACqR,KAAK,IAAI,CAAV,IAAe,CAAnB,EAAsB;CAEtBvO,MAAAA,CAAC,GAAGwG,GAAG,CAACnJ,UAAJ,CAAeH,CAAf,CAAJ;CACAwR,MAAAA,EAAE,GAAG1O,CAAC,IAAI,CAAV;CACA2O,MAAAA,EAAE,GAAG3O,CAAC,GAAG,GAAT;CACAyO,MAAAA,SAAS,CAACjQ,IAAV,CAAemQ,EAAf;CACAF,MAAAA,SAAS,CAACjQ,IAAV,CAAekQ,EAAf;CACD;;CAED,WAAOD,SAAP;CACD;;CAED,WAASlJ,aAAT,CAAwBiB,GAAxB,EAA6B;CAC3B,WAAOyC,QAAM,CAACvM,WAAP,CAAmB2R,WAAW,CAAC7H,GAAD,CAA9B,CAAP;CACD;;CAED,WAASgC,UAAT,CAAqBoG,GAArB,EAA0BC,GAA1B,EAA+B9P,MAA/B,EAAuC3B,MAAvC,EAA+C;CAC7C,SAAK,IAAIF,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGE,MAApB,EAA4B,EAAEF,CAA9B,EAAiC;CAC/B,UAAKA,CAAC,GAAG6B,MAAJ,IAAc8P,GAAG,CAACzR,MAAnB,IAA+BF,CAAC,IAAI0R,GAAG,CAACxR,MAA5C,EAAqD;CACrDyR,MAAAA,GAAG,CAAC3R,CAAC,GAAG6B,MAAL,CAAH,GAAkB6P,GAAG,CAAC1R,CAAD,CAArB;CACD;;CACD,WAAOA,CAAP;CACD;CAGD;CACA;;;CACA,WAASuF,UAAT,CAAqBuB,GAArB,EAA0BE,IAA1B,EAAgC;CAC9B,WAAOF,GAAG,YAAYE,IAAf,IACJF,GAAG,IAAI,IAAP,IAAeA,GAAG,CAAC8K,WAAJ,IAAmB,IAAlC,IAA0C9K,GAAG,CAAC8K,WAAJ,CAAgBC,IAAhB,IAAwB,IAAlE,IACC/K,GAAG,CAAC8K,WAAJ,CAAgBC,IAAhB,KAAyB7K,IAAI,CAAC6K,IAFlC;CAGD;;CACD,WAAS9K,WAAT,CAAsBD,GAAtB,EAA2B;;CAEzB,WAAOA,GAAG,KAAKA,GAAf,CAFyB;CAG1B;CAGD;;;CACA,MAAIgG,mBAAmB,GAAI,YAAY;CACrC,QAAIgF,QAAQ,GAAG,kBAAf;CACA,QAAIC,KAAK,GAAG,IAAIjS,KAAJ,CAAU,GAAV,CAAZ;;CACA,SAAK,IAAIE,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAG,EAApB,EAAwB,EAAEA,CAA1B,EAA6B;CAC3B,UAAIgS,GAAG,GAAGhS,CAAC,GAAG,EAAd;;CACA,WAAK,IAAI4K,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAG,EAApB,EAAwB,EAAEA,CAA1B,EAA6B;CAC3BmH,QAAAA,KAAK,CAACC,GAAG,GAAGpH,CAAP,CAAL,GAAiBkH,QAAQ,CAAC9R,CAAD,CAAR,GAAc8R,QAAQ,CAAClH,CAAD,CAAvC;CACD;CACF;;CACD,WAAOmH,KAAP;CACD,GAVyB,EAA1B;;;;;;;AC9wDA,kBAAe,EAAf;;CCAA;;CAoKA,IAAIE,WAAW,GAAGC,MAAM,CAACD,WAAP,IAAsB,EAAxC;;CAEEA,WAAW,CAACE,GAAZ,IACAF,WAAW,CAACG,MADZ,IAEAH,WAAW,CAACI,KAFZ,IAGAJ,WAAW,CAACK,IAHZ,IAIAL,WAAW,CAACM,SAJZ,IAKA,YAAU;CAAE,SAAQ,IAAIC,IAAJ,EAAD,CAAaC,OAAb,EAAP;CAA+B;;CC1K7C,IAAIC,QAAJ;;CACA,IAAI,OAAOzO,MAAM,CAAC0O,MAAd,KAAyB,UAA7B,EAAwC;CACtCD,EAAAA,QAAQ,GAAG,SAASA,QAAT,CAAkBE,IAAlB,EAAwBC,SAAxB,EAAmC;CAC5C;CACAD,IAAAA,IAAI,CAACE,MAAL,GAAcD,SAAd;CACAD,IAAAA,IAAI,CAACzO,SAAL,GAAiBF,MAAM,CAAC0O,MAAP,CAAcE,SAAS,CAAC1O,SAAxB,EAAmC;CAClDyN,MAAAA,WAAW,EAAE;CACX/O,QAAAA,KAAK,EAAE+P,IADI;CAEXvO,QAAAA,UAAU,EAAE,KAFD;CAGX0O,QAAAA,QAAQ,EAAE,IAHC;CAIXC,QAAAA,YAAY,EAAE;CAJH;CADqC,KAAnC,CAAjB;CAQD,GAXD;CAYD,CAbD,MAaO;CACLN,EAAAA,QAAQ,GAAG,SAASA,QAAT,CAAkBE,IAAlB,EAAwBC,SAAxB,EAAmC;CAC5CD,IAAAA,IAAI,CAACE,MAAL,GAAcD,SAAd;;CACA,QAAII,QAAQ,GAAG,SAAXA,QAAW,GAAY,EAA3B;;CACAA,IAAAA,QAAQ,CAAC9O,SAAT,GAAqB0O,SAAS,CAAC1O,SAA/B;CACAyO,IAAAA,IAAI,CAACzO,SAAL,GAAiB,IAAI8O,QAAJ,EAAjB;CACAL,IAAAA,IAAI,CAACzO,SAAL,CAAeyN,WAAf,GAA6BgB,IAA7B;CACD,GAND;CAOD;;AACD,kBAAeF,QAAf;;CCxBA;CAqBA,IAAIQ,YAAY,GAAG,UAAnB;CACO,SAASC,MAAT,CAAgBC,CAAhB,EAAmB;CACxB,MAAI,CAACC,QAAQ,CAACD,CAAD,CAAb,EAAkB;CAChB,QAAIE,OAAO,GAAG,EAAd;;CACA,SAAK,IAAItT,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGkI,SAAS,CAAChI,MAA9B,EAAsCF,CAAC,EAAvC,EAA2C;CACzCsT,MAAAA,OAAO,CAAChS,IAAR,CAAa+H,OAAO,CAACnB,SAAS,CAAClI,CAAD,CAAV,CAApB;CACD;;CACD,WAAOsT,OAAO,CAAC/R,IAAR,CAAa,GAAb,CAAP;CACD;;CAED,MAAIvB,CAAC,GAAG,CAAR;CACA,MAAIuT,IAAI,GAAGrL,SAAX;CACA,MAAIjI,GAAG,GAAGsT,IAAI,CAACrT,MAAf;CACA,MAAIoJ,GAAG,GAAG5B,MAAM,CAAC0L,CAAD,CAAN,CAAU3J,OAAV,CAAkByJ,YAAlB,EAAgC,UAAS3L,CAAT,EAAY;CACpD,QAAIA,CAAC,KAAK,IAAV,EAAgB,OAAO,GAAP;CAChB,QAAIvH,CAAC,IAAIC,GAAT,EAAc,OAAOsH,CAAP;;CACd,YAAQA,CAAR;CACE,WAAK,IAAL;CAAW,eAAOG,MAAM,CAAC6L,IAAI,CAACvT,CAAC,EAAF,CAAL,CAAb;;CACX,WAAK,IAAL;CAAW,eAAO+K,MAAM,CAACwI,IAAI,CAACvT,CAAC,EAAF,CAAL,CAAb;;CACX,WAAK,IAAL;CACE,YAAI;CACF,iBAAOwT,IAAI,CAACC,SAAL,CAAeF,IAAI,CAACvT,CAAC,EAAF,CAAnB,CAAP;CACD,SAFD,CAEE,OAAO0T,CAAP,EAAU;CACV,iBAAO,YAAP;CACD;;CACH;CACE,eAAOnM,CAAP;CAVJ;CAYD,GAfS,CAAV;;CAgBA,OAAK,IAAIA,CAAC,GAAGgM,IAAI,CAACvT,CAAD,CAAjB,EAAsBA,CAAC,GAAGC,GAA1B,EAA+BsH,CAAC,GAAGgM,IAAI,CAAC,EAAEvT,CAAH,CAAvC,EAA8C;CAC5C,QAAI2T,MAAM,CAACpM,CAAD,CAAN,IAAa,CAACqM,QAAQ,CAACrM,CAAD,CAA1B,EAA+B;CAC7B+B,MAAAA,GAAG,IAAI,MAAM/B,CAAb;CACD,KAFD,MAEO;CACL+B,MAAAA,GAAG,IAAI,MAAMD,OAAO,CAAC9B,CAAD,CAApB;CACD;CACF;;CACD,SAAO+B,GAAP;CACD;CAID;CACA;;CACO,SAASuK,SAAT,CAAmBC,EAAnB,EAAuBC,GAAvB,EAA4B;CACjC;CACA,MAAIC,WAAW,CAAC9B,MAAM,CAAC+B,OAAR,CAAf,EAAiC;CAC/B,WAAO,YAAW;CAChB,aAAOJ,SAAS,CAACC,EAAD,EAAKC,GAAL,CAAT,CAAmB7K,KAAnB,CAAyB,IAAzB,EAA+BhB,SAA/B,CAAP;CACD,KAFD;CAGD;;CAMD,MAAIgM,MAAM,GAAG,KAAb;;CACA,WAASC,UAAT,GAAsB;CACpB,QAAI,CAACD,MAAL,EAAa;CACX,MAIO;CACLrQ,QAAAA,OAAO,CAACC,KAAR,CAAciQ,GAAd;CACD;;CACDG,MAAAA,MAAM,GAAG,IAAT;CACD;;CACD,WAAOJ,EAAE,CAAC5K,KAAH,CAAS,IAAT,EAAehB,SAAf,CAAP;CACD;;CAED,SAAOiM,UAAP;CACD;CAGD,IAAIC,MAAM,GAAG,EAAb;CACA,IAAIC,YAAJ;CACO,SAASC,QAAT,CAAkBvM,GAAlB,EAAuB;CAC5B,MAAIiM,WAAW,CAACK,YAAD,CAAf,EACEA,YAAY,GAA6B,EAAzC;CACFtM,EAAAA,GAAG,GAAGA,GAAG,CAACwM,WAAJ,EAAN;;CACA,MAAI,CAACH,MAAM,CAACrM,GAAD,CAAX,EAAkB;CAChB,QAAI,IAAIyM,MAAJ,CAAW,QAAQzM,GAAR,GAAc,KAAzB,EAAgC,GAAhC,EAAqC0M,IAArC,CAA0CJ,YAA1C,CAAJ,EAA6D;CAC3D,UAAIK,GAAG,GAAG,CAAV;;CACAN,MAAAA,MAAM,CAACrM,GAAD,CAAN,GAAc,YAAW;CACvB,YAAIgM,GAAG,GAAGZ,MAAM,CAACjK,KAAP,CAAa,IAAb,EAAmBhB,SAAnB,CAAV;CACArE,QAAAA,OAAO,CAACC,KAAR,CAAc,WAAd,EAA2BiE,GAA3B,EAAgC2M,GAAhC,EAAqCX,GAArC;CACD,OAHD;CAID,KAND,MAMO;CACLK,MAAAA,MAAM,CAACrM,GAAD,CAAN,GAAc,YAAW,EAAzB;CACD;CACF;;CACD,SAAOqM,MAAM,CAACrM,GAAD,CAAb;CACD;CAGD;CACA;CACA;CACA;CACA;CACA;CACA;;CACA;;CACO,SAASsB,OAAT,CAAiBvC,GAAjB,EAAsB6N,IAAtB,EAA4B;CACjC;CACA,MAAIC,GAAG,GAAG;CACRC,IAAAA,IAAI,EAAE,EADE;CAERC,IAAAA,OAAO,EAAEC;CAFD,GAAV,CAFiC;;CAOjC,MAAI7M,SAAS,CAAChI,MAAV,IAAoB,CAAxB,EAA2B0U,GAAG,CAACI,KAAJ,GAAY9M,SAAS,CAAC,CAAD,CAArB;CAC3B,MAAIA,SAAS,CAAChI,MAAV,IAAoB,CAAxB,EAA2B0U,GAAG,CAACK,MAAJ,GAAa/M,SAAS,CAAC,CAAD,CAAtB;;CAC3B,MAAIgN,SAAS,CAACP,IAAD,CAAb,EAAqB;CACnB;CACAC,IAAAA,GAAG,CAACO,UAAJ,GAAiBR,IAAjB;CACD,GAHD,MAGO,IAAIA,IAAJ,EAAU;CACf;CACAS,IAAAA,OAAO,CAACR,GAAD,EAAMD,IAAN,CAAP;CACD,GAfgC;;;CAiBjC,MAAIX,WAAW,CAACY,GAAG,CAACO,UAAL,CAAf,EAAiCP,GAAG,CAACO,UAAJ,GAAiB,KAAjB;CACjC,MAAInB,WAAW,CAACY,GAAG,CAACI,KAAL,CAAf,EAA4BJ,GAAG,CAACI,KAAJ,GAAY,CAAZ;CAC5B,MAAIhB,WAAW,CAACY,GAAG,CAACK,MAAL,CAAf,EAA6BL,GAAG,CAACK,MAAJ,GAAa,KAAb;CAC7B,MAAIjB,WAAW,CAACY,GAAG,CAACS,aAAL,CAAf,EAAoCT,GAAG,CAACS,aAAJ,GAAoB,IAApB;CACpC,MAAIT,GAAG,CAACK,MAAR,EAAgBL,GAAG,CAACE,OAAJ,GAAcQ,gBAAd;CAChB,SAAOC,WAAW,CAACX,GAAD,EAAM9N,GAAN,EAAW8N,GAAG,CAACI,KAAf,CAAlB;CACD;;CAGD3L,OAAO,CAAC4L,MAAR,GAAiB;CACf,UAAS,CAAC,CAAD,EAAI,EAAJ,CADM;CAEf,YAAW,CAAC,CAAD,EAAI,EAAJ,CAFI;CAGf,eAAc,CAAC,CAAD,EAAI,EAAJ,CAHC;CAIf,aAAY,CAAC,CAAD,EAAI,EAAJ,CAJG;CAKf,WAAU,CAAC,EAAD,EAAK,EAAL,CALK;CAMf,UAAS,CAAC,EAAD,EAAK,EAAL,CANM;CAOf,WAAU,CAAC,EAAD,EAAK,EAAL,CAPK;CAQf,UAAS,CAAC,EAAD,EAAK,EAAL,CARM;CASf,UAAS,CAAC,EAAD,EAAK,EAAL,CATM;CAUf,WAAU,CAAC,EAAD,EAAK,EAAL,CAVK;CAWf,aAAY,CAAC,EAAD,EAAK,EAAL,CAXG;CAYf,SAAQ,CAAC,EAAD,EAAK,EAAL,CAZO;CAaf,YAAW,CAAC,EAAD,EAAK,EAAL;CAbI,CAAjB;;CAiBA5L,OAAO,CAACmM,MAAR,GAAiB;CACf,aAAW,MADI;CAEf,YAAU,QAFK;CAGf,aAAW,QAHI;CAIf,eAAa,MAJE;CAKf,UAAQ,MALO;CAMf,YAAU,OANK;CAOf,UAAQ,SAPO;CAQf;CACA,YAAU;CATK,CAAjB;;CAaA,SAASF,gBAAT,CAA0BhM,GAA1B,EAA+BmM,SAA/B,EAA0C;CACxC,MAAIC,KAAK,GAAGrM,OAAO,CAACmM,MAAR,CAAeC,SAAf,CAAZ;;CAEA,MAAIC,KAAJ,EAAW;CACT,WAAO,UAAYrM,OAAO,CAAC4L,MAAR,CAAeS,KAAf,EAAsB,CAAtB,CAAZ,GAAuC,GAAvC,GAA6CpM,GAA7C,GACA,OADA,GACYD,OAAO,CAAC4L,MAAR,CAAeS,KAAf,EAAsB,CAAtB,CADZ,GACuC,GAD9C;CAED,GAHD,MAGO;CACL,WAAOpM,GAAP;CACD;CACF;;CAGD,SAASyL,cAAT,CAAwBzL,GAAxB,EAA6BmM,SAA7B,EAAwC;CACtC,SAAOnM,GAAP;CACD;;CAGD,SAASqM,WAAT,CAAqBhP,KAArB,EAA4B;CAC1B,MAAIiP,IAAI,GAAG,EAAX;CAEAjP,EAAAA,KAAK,CAACkP,OAAN,CAAc,UAAS5L,GAAT,EAAc6L,GAAd,EAAmB;CAC/BF,IAAAA,IAAI,CAAC3L,GAAD,CAAJ,GAAY,IAAZ;CACD,GAFD;CAIA,SAAO2L,IAAP;CACD;;CAGD,SAASL,WAAT,CAAqBX,GAArB,EAA0B/R,KAA1B,EAAiCkT,YAAjC,EAA+C;CAC7C;CACA;CACA,MAAInB,GAAG,CAACS,aAAJ,IACAxS,KADA,IAEAmT,UAAU,CAACnT,KAAK,CAACwG,OAAP,CAFV;CAIAxG,EAAAA,KAAK,CAACwG,OAAN,KAAkBA,OAJlB;CAMA,IAAExG,KAAK,CAAC+O,WAAN,IAAqB/O,KAAK,CAAC+O,WAAN,CAAkBzN,SAAlB,KAAgCtB,KAAvD,CANJ,EAMmE;CACjE,QAAI+J,GAAG,GAAG/J,KAAK,CAACwG,OAAN,CAAc0M,YAAd,EAA4BnB,GAA5B,CAAV;;CACA,QAAI,CAACvB,QAAQ,CAACzG,GAAD,CAAb,EAAoB;CAClBA,MAAAA,GAAG,GAAG2I,WAAW,CAACX,GAAD,EAAMhI,GAAN,EAAWmJ,YAAX,CAAjB;CACD;;CACD,WAAOnJ,GAAP;CACD,GAf4C;;;CAkB7C,MAAIqJ,SAAS,GAAGC,eAAe,CAACtB,GAAD,EAAM/R,KAAN,CAA/B;;CACA,MAAIoT,SAAJ,EAAe;CACb,WAAOA,SAAP;CACD,GArB4C;;;CAwB7C,MAAIE,IAAI,GAAGlS,MAAM,CAACkS,IAAP,CAAYtT,KAAZ,CAAX;CACA,MAAIuT,WAAW,GAAGT,WAAW,CAACQ,IAAD,CAA7B;;CAEA,MAAIvB,GAAG,CAACO,UAAR,EAAoB;CAClBgB,IAAAA,IAAI,GAAGlS,MAAM,CAACoS,mBAAP,CAA2BxT,KAA3B,CAAP;CACD,GA7B4C;CAgC7C;;;CACA,MAAIyT,OAAO,CAACzT,KAAD,CAAP,KACIsT,IAAI,CAAC3V,OAAL,CAAa,SAAb,KAA2B,CAA3B,IAAgC2V,IAAI,CAAC3V,OAAL,CAAa,aAAb,KAA+B,CADnE,CAAJ,EAC2E;CACzE,WAAO+V,WAAW,CAAC1T,KAAD,CAAlB;CACD,GApC4C;;;CAuC7C,MAAIsT,IAAI,CAACjW,MAAL,KAAgB,CAApB,EAAuB;CACrB,QAAI8V,UAAU,CAACnT,KAAD,CAAd,EAAuB;CACrB,UAAIgP,IAAI,GAAGhP,KAAK,CAACgP,IAAN,GAAa,OAAOhP,KAAK,CAACgP,IAA1B,GAAiC,EAA5C;CACA,aAAO+C,GAAG,CAACE,OAAJ,CAAY,cAAcjD,IAAd,GAAqB,GAAjC,EAAsC,SAAtC,CAAP;CACD;;CACD,QAAI2E,QAAQ,CAAC3T,KAAD,CAAZ,EAAqB;CACnB,aAAO+R,GAAG,CAACE,OAAJ,CAAYN,MAAM,CAACrQ,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BnF,KAA/B,CAAZ,EAAmD,QAAnD,CAAP;CACD;;CACD,QAAI4T,MAAM,CAAC5T,KAAD,CAAV,EAAmB;CACjB,aAAO+R,GAAG,CAACE,OAAJ,CAAYtC,IAAI,CAACrO,SAAL,CAAegD,QAAf,CAAwBa,IAAxB,CAA6BnF,KAA7B,CAAZ,EAAiD,MAAjD,CAAP;CACD;;CACD,QAAIyT,OAAO,CAACzT,KAAD,CAAX,EAAoB;CAClB,aAAO0T,WAAW,CAAC1T,KAAD,CAAlB;CACD;CACF;;CAED,MAAI6T,IAAI,GAAG,EAAX;CAAA,MAAe/P,KAAK,GAAG,KAAvB;CAAA,MAA8BgQ,MAAM,GAAG,CAAC,GAAD,EAAM,GAAN,CAAvC,CAvD6C;;CA0D7C,MAAI1P,OAAO,CAACpE,KAAD,CAAX,EAAoB;CAClB8D,IAAAA,KAAK,GAAG,IAAR;CACAgQ,IAAAA,MAAM,GAAG,CAAC,GAAD,EAAM,GAAN,CAAT;CACD,GA7D4C;;;CAgE7C,MAAIX,UAAU,CAACnT,KAAD,CAAd,EAAuB;CACrB,QAAIiG,CAAC,GAAGjG,KAAK,CAACgP,IAAN,GAAa,OAAOhP,KAAK,CAACgP,IAA1B,GAAiC,EAAzC;CACA6E,IAAAA,IAAI,GAAG,eAAe5N,CAAf,GAAmB,GAA1B;CACD,GAnE4C;;;CAsE7C,MAAI0N,QAAQ,CAAC3T,KAAD,CAAZ,EAAqB;CACnB6T,IAAAA,IAAI,GAAG,MAAMlC,MAAM,CAACrQ,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BnF,KAA/B,CAAb;CACD,GAxE4C;;;CA2E7C,MAAI4T,MAAM,CAAC5T,KAAD,CAAV,EAAmB;CACjB6T,IAAAA,IAAI,GAAG,MAAMlE,IAAI,CAACrO,SAAL,CAAeyS,WAAf,CAA2B5O,IAA3B,CAAgCnF,KAAhC,CAAb;CACD,GA7E4C;;;CAgF7C,MAAIyT,OAAO,CAACzT,KAAD,CAAX,EAAoB;CAClB6T,IAAAA,IAAI,GAAG,MAAMH,WAAW,CAAC1T,KAAD,CAAxB;CACD;;CAED,MAAIsT,IAAI,CAACjW,MAAL,KAAgB,CAAhB,KAAsB,CAACyG,KAAD,IAAU9D,KAAK,CAAC3C,MAAN,IAAgB,CAAhD,CAAJ,EAAwD;CACtD,WAAOyW,MAAM,CAAC,CAAD,CAAN,GAAYD,IAAZ,GAAmBC,MAAM,CAAC,CAAD,CAAhC;CACD;;CAED,MAAIZ,YAAY,GAAG,CAAnB,EAAsB;CACpB,QAAIS,QAAQ,CAAC3T,KAAD,CAAZ,EAAqB;CACnB,aAAO+R,GAAG,CAACE,OAAJ,CAAYN,MAAM,CAACrQ,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BnF,KAA/B,CAAZ,EAAmD,QAAnD,CAAP;CACD,KAFD,MAEO;CACL,aAAO+R,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAP;CACD;CACF;;CAEDF,EAAAA,GAAG,CAACC,IAAJ,CAASvT,IAAT,CAAcuB,KAAd;CAEA,MAAIxB,MAAJ;;CACA,MAAIsF,KAAJ,EAAW;CACTtF,IAAAA,MAAM,GAAGwV,WAAW,CAACjC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EAAwCD,IAAxC,CAApB;CACD,GAFD,MAEO;CACL9U,IAAAA,MAAM,GAAG8U,IAAI,CAACW,GAAL,CAAS,UAASC,GAAT,EAAc;CAC9B,aAAOC,cAAc,CAACpC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EAAwCW,GAAxC,EAA6CpQ,KAA7C,CAArB;CACD,KAFQ,CAAT;CAGD;;CAEDiO,EAAAA,GAAG,CAACC,IAAJ,CAASoC,GAAT;CAEA,SAAOC,oBAAoB,CAAC7V,MAAD,EAASqV,IAAT,EAAeC,MAAf,CAA3B;CACD;;CAGD,SAAST,eAAT,CAAyBtB,GAAzB,EAA8B/R,KAA9B,EAAqC;CACnC,MAAImR,WAAW,CAACnR,KAAD,CAAf,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,WAAZ,EAAyB,WAAzB,CAAP;;CACF,MAAIzB,QAAQ,CAACxQ,KAAD,CAAZ,EAAqB;CACnB,QAAIsU,MAAM,GAAG,OAAO3D,IAAI,CAACC,SAAL,CAAe5Q,KAAf,EAAsB4G,OAAtB,CAA8B,QAA9B,EAAwC,EAAxC,EACsBA,OADtB,CAC8B,IAD9B,EACoC,KADpC,EAEsBA,OAFtB,CAE8B,MAF9B,EAEsC,GAFtC,CAAP,GAEoD,IAFjE;CAGA,WAAOmL,GAAG,CAACE,OAAJ,CAAYqC,MAAZ,EAAoB,QAApB,CAAP;CACD;;CACD,MAAIC,QAAQ,CAACvU,KAAD,CAAZ,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,KAAKjS,KAAjB,EAAwB,QAAxB,CAAP;CACF,MAAIqS,SAAS,CAACrS,KAAD,CAAb,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,KAAKjS,KAAjB,EAAwB,SAAxB,CAAP,CAZiC;;CAcnC,MAAI8Q,MAAM,CAAC9Q,KAAD,CAAV,EACE,OAAO+R,GAAG,CAACE,OAAJ,CAAY,MAAZ,EAAoB,MAApB,CAAP;CACH;;CAGD,SAASyB,WAAT,CAAqB1T,KAArB,EAA4B;CAC1B,SAAO,MAAMvC,KAAK,CAAC6D,SAAN,CAAgBgD,QAAhB,CAAyBa,IAAzB,CAA8BnF,KAA9B,CAAN,GAA6C,GAApD;CACD;;CAGD,SAASgU,WAAT,CAAqBjC,GAArB,EAA0B/R,KAA1B,EAAiCkT,YAAjC,EAA+CK,WAA/C,EAA4DD,IAA5D,EAAkE;CAChE,MAAI9U,MAAM,GAAG,EAAb;;CACA,OAAK,IAAIrB,CAAC,GAAG,CAAR,EAAWqX,CAAC,GAAGxU,KAAK,CAAC3C,MAA1B,EAAkCF,CAAC,GAAGqX,CAAtC,EAAyC,EAAErX,CAA3C,EAA8C;CAC5C,QAAIsX,cAAc,CAACzU,KAAD,EAAQ6E,MAAM,CAAC1H,CAAD,CAAd,CAAlB,EAAsC;CACpCqB,MAAAA,MAAM,CAACC,IAAP,CAAY0V,cAAc,CAACpC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EACtB1O,MAAM,CAAC1H,CAAD,CADgB,EACX,IADW,CAA1B;CAED,KAHD,MAGO;CACLqB,MAAAA,MAAM,CAACC,IAAP,CAAY,EAAZ;CACD;CACF;;CACD6U,EAAAA,IAAI,CAACN,OAAL,CAAa,UAASkB,GAAT,EAAc;CACzB,QAAI,CAACA,GAAG,CAACQ,KAAJ,CAAU,OAAV,CAAL,EAAyB;CACvBlW,MAAAA,MAAM,CAACC,IAAP,CAAY0V,cAAc,CAACpC,GAAD,EAAM/R,KAAN,EAAakT,YAAb,EAA2BK,WAA3B,EACtBW,GADsB,EACjB,IADiB,CAA1B;CAED;CACF,GALD;CAMA,SAAO1V,MAAP;CACD;;CAGD,SAAS2V,cAAT,CAAwBpC,GAAxB,EAA6B/R,KAA7B,EAAoCkT,YAApC,EAAkDK,WAAlD,EAA+DW,GAA/D,EAAoEpQ,KAApE,EAA2E;CACzE,MAAIkL,IAAJ,EAAUvI,GAAV,EAAekO,IAAf;CACAA,EAAAA,IAAI,GAAGvT,MAAM,CAACwT,wBAAP,CAAgC5U,KAAhC,EAAuCkU,GAAvC,KAA+C;CAAElU,IAAAA,KAAK,EAAEA,KAAK,CAACkU,GAAD;CAAd,GAAtD;;CACA,MAAIS,IAAI,CAAClT,GAAT,EAAc;CACZ,QAAIkT,IAAI,CAACzP,GAAT,EAAc;CACZuB,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,iBAAZ,EAA+B,SAA/B,CAAN;CACD,KAFD,MAEO;CACLxL,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAN;CACD;CACF,GAND,MAMO;CACL,QAAI0C,IAAI,CAACzP,GAAT,EAAc;CACZuB,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAN;CACD;CACF;;CACD,MAAI,CAACwC,cAAc,CAAClB,WAAD,EAAcW,GAAd,CAAnB,EAAuC;CACrClF,IAAAA,IAAI,GAAG,MAAMkF,GAAN,GAAY,GAAnB;CACD;;CACD,MAAI,CAACzN,GAAL,EAAU;CACR,QAAIsL,GAAG,CAACC,IAAJ,CAASrU,OAAT,CAAiBgX,IAAI,CAAC3U,KAAtB,IAA+B,CAAnC,EAAsC;CACpC,UAAI8Q,MAAM,CAACoC,YAAD,CAAV,EAA0B;CACxBzM,QAAAA,GAAG,GAAGiM,WAAW,CAACX,GAAD,EAAM4C,IAAI,CAAC3U,KAAX,EAAkB,IAAlB,CAAjB;CACD,OAFD,MAEO;CACLyG,QAAAA,GAAG,GAAGiM,WAAW,CAACX,GAAD,EAAM4C,IAAI,CAAC3U,KAAX,EAAkBkT,YAAY,GAAG,CAAjC,CAAjB;CACD;;CACD,UAAIzM,GAAG,CAAC9I,OAAJ,CAAY,IAAZ,IAAoB,CAAC,CAAzB,EAA4B;CAC1B,YAAImG,KAAJ,EAAW;CACT2C,UAAAA,GAAG,GAAGA,GAAG,CAAC8H,KAAJ,CAAU,IAAV,EAAgB0F,GAAhB,CAAoB,UAASY,IAAT,EAAe;CACvC,mBAAO,OAAOA,IAAd;CACD,WAFK,EAEHnW,IAFG,CAEE,IAFF,EAEQ6J,MAFR,CAEe,CAFf,CAAN;CAGD,SAJD,MAIO;CACL9B,UAAAA,GAAG,GAAG,OAAOA,GAAG,CAAC8H,KAAJ,CAAU,IAAV,EAAgB0F,GAAhB,CAAoB,UAASY,IAAT,EAAe;CAC9C,mBAAO,QAAQA,IAAf;CACD,WAFY,EAEVnW,IAFU,CAEL,IAFK,CAAb;CAGD;CACF;CACF,KAjBD,MAiBO;CACL+H,MAAAA,GAAG,GAAGsL,GAAG,CAACE,OAAJ,CAAY,YAAZ,EAA0B,SAA1B,CAAN;CACD;CACF;;CACD,MAAId,WAAW,CAACnC,IAAD,CAAf,EAAuB;CACrB,QAAIlL,KAAK,IAAIoQ,GAAG,CAACQ,KAAJ,CAAU,OAAV,CAAb,EAAiC;CAC/B,aAAOjO,GAAP;CACD;;CACDuI,IAAAA,IAAI,GAAG2B,IAAI,CAACC,SAAL,CAAe,KAAKsD,GAApB,CAAP;;CACA,QAAIlF,IAAI,CAAC0F,KAAL,CAAW,8BAAX,CAAJ,EAAgD;CAC9C1F,MAAAA,IAAI,GAAGA,IAAI,CAACzG,MAAL,CAAY,CAAZ,EAAeyG,IAAI,CAAC3R,MAAL,GAAc,CAA7B,CAAP;CACA2R,MAAAA,IAAI,GAAG+C,GAAG,CAACE,OAAJ,CAAYjD,IAAZ,EAAkB,MAAlB,CAAP;CACD,KAHD,MAGO;CACLA,MAAAA,IAAI,GAAGA,IAAI,CAACpI,OAAL,CAAa,IAAb,EAAmB,KAAnB,EACKA,OADL,CACa,MADb,EACqB,GADrB,EAEKA,OAFL,CAEa,UAFb,EAEyB,GAFzB,CAAP;CAGAoI,MAAAA,IAAI,GAAG+C,GAAG,CAACE,OAAJ,CAAYjD,IAAZ,EAAkB,QAAlB,CAAP;CACD;CACF;;CAED,SAAOA,IAAI,GAAG,IAAP,GAAcvI,GAArB;CACD;;CAGD,SAAS4N,oBAAT,CAA8B7V,MAA9B,EAAsCqV,IAAtC,EAA4CC,MAA5C,EAAoD;CAElD,MAAIzW,MAAM,GAAGmB,MAAM,CAACsW,MAAP,CAAc,UAASC,IAAT,EAAeC,GAAf,EAAoB;CAE7C,QAAIA,GAAG,CAACrX,OAAJ,CAAY,IAAZ,KAAqB,CAAzB,EAA4BsX;CAC5B,WAAOF,IAAI,GAAGC,GAAG,CAACpO,OAAJ,CAAY,iBAAZ,EAA+B,EAA/B,EAAmCvJ,MAA1C,GAAmD,CAA1D;CACD,GAJY,EAIV,CAJU,CAAb;;CAMA,MAAIA,MAAM,GAAG,EAAb,EAAiB;CACf,WAAOyW,MAAM,CAAC,CAAD,CAAN,IACCD,IAAI,KAAK,EAAT,GAAc,EAAd,GAAmBA,IAAI,GAAG,KAD3B,IAEA,GAFA,GAGArV,MAAM,CAACE,IAAP,CAAY,OAAZ,CAHA,GAIA,GAJA,GAKAoV,MAAM,CAAC,CAAD,CALb;CAMD;;CAED,SAAOA,MAAM,CAAC,CAAD,CAAN,GAAYD,IAAZ,GAAmB,GAAnB,GAAyBrV,MAAM,CAACE,IAAP,CAAY,IAAZ,CAAzB,GAA6C,GAA7C,GAAmDoV,MAAM,CAAC,CAAD,CAAhE;CACD;CAID;;;CACO,SAAS1P,OAAT,CAAiB8Q,EAAjB,EAAqB;CAC1B,SAAOjY,KAAK,CAACmH,OAAN,CAAc8Q,EAAd,CAAP;CACD;CAEM,SAAS7C,SAAT,CAAmBrQ,GAAnB,EAAwB;CAC7B,SAAO,OAAOA,GAAP,KAAe,SAAtB;CACD;CAEM,SAAS8O,MAAT,CAAgB9O,GAAhB,EAAqB;CAC1B,SAAOA,GAAG,KAAK,IAAf;CACD;CAEM,SAASmT,iBAAT,CAA2BnT,GAA3B,EAAgC;CACrC,SAAOA,GAAG,IAAI,IAAd;CACD;CAEM,SAASuS,QAAT,CAAkBvS,GAAlB,EAAuB;CAC5B,SAAO,OAAOA,GAAP,KAAe,QAAtB;CACD;CAEM,SAASwO,QAAT,CAAkBxO,GAAlB,EAAuB;CAC5B,SAAO,OAAOA,GAAP,KAAe,QAAtB;CACD;CAEM,SAASoT,QAAT,CAAkBpT,GAAlB,EAAuB;CAC5B,SAAO,uBAAOA,GAAP,MAAe,QAAtB;CACD;CAEM,SAASmP,WAAT,CAAqBnP,GAArB,EAA0B;CAC/B,SAAOA,GAAG,KAAK,KAAK,CAApB;CACD;CAEM,SAAS2R,QAAT,CAAkB0B,EAAlB,EAAsB;CAC3B,SAAOtE,QAAQ,CAACsE,EAAD,CAAR,IAAgBC,cAAc,CAACD,EAAD,CAAd,KAAuB,iBAA9C;CACD;CAEM,SAAStE,QAAT,CAAkB/O,GAAlB,EAAuB;CAC5B,SAAO,uBAAOA,GAAP,MAAe,QAAf,IAA2BA,GAAG,KAAK,IAA1C;CACD;CAEM,SAAS4R,MAAT,CAAgBlU,CAAhB,EAAmB;CACxB,SAAOqR,QAAQ,CAACrR,CAAD,CAAR,IAAe4V,cAAc,CAAC5V,CAAD,CAAd,KAAsB,eAA5C;CACD;CAEM,SAAS+T,OAAT,CAAiBrU,CAAjB,EAAoB;CACzB,SAAO2R,QAAQ,CAAC3R,CAAD,CAAR,KACFkW,cAAc,CAAClW,CAAD,CAAd,KAAsB,gBAAtB,IAA0CA,CAAC,YAAY3B,KADrD,CAAP;CAED;CAEM,SAAS0V,UAAT,CAAoBnR,GAApB,EAAyB;CAC9B,SAAO,OAAOA,GAAP,KAAe,UAAtB;CACD;CAEM,SAASuT,WAAT,CAAqBvT,GAArB,EAA0B;CAC/B,SAAOA,GAAG,KAAK,IAAR,IACA,OAAOA,GAAP,KAAe,SADf,IAEA,OAAOA,GAAP,KAAe,QAFf,IAGA,OAAOA,GAAP,KAAe,QAHf,IAIA,uBAAOA,GAAP,MAAe,QAJf;CAKA,SAAOA,GAAP,KAAe,WALtB;CAMD;CAEM,SAASN,QAAT,CAAkB8T,QAAlB,EAA4B;CACjC,SAAO7U,MAAM,CAACe,QAAP,CAAgB8T,QAAhB,CAAP;CACD;;CAED,SAASF,cAAT,CAAwBG,CAAxB,EAA2B;CACzB,SAAOrU,MAAM,CAACE,SAAP,CAAiBgD,QAAjB,CAA0Ba,IAA1B,CAA+BsQ,CAA/B,CAAP;CACD;;CAGD,SAASC,GAAT,CAAazP,CAAb,EAAgB;CACd,SAAOA,CAAC,GAAG,EAAJ,GAAS,MAAMA,CAAC,CAAC3B,QAAF,CAAW,EAAX,CAAf,GAAgC2B,CAAC,CAAC3B,QAAF,CAAW,EAAX,CAAvC;CACD;;CAGD,IAAIqR,MAAM,GAAG,CAAC,KAAD,EAAQ,KAAR,EAAe,KAAf,EAAsB,KAAtB,EAA6B,KAA7B,EAAoC,KAApC,EAA2C,KAA3C,EAAkD,KAAlD,EAAyD,KAAzD,EACC,KADD,EACQ,KADR,EACe,KADf,CAAb;;CAIA,SAASC,WAAT,GAAqB;CACnB,MAAIlW,CAAC,GAAG,IAAIiQ,IAAJ,EAAR;CACA,MAAIkG,IAAI,GAAG,CAACH,GAAG,CAAChW,CAAC,CAACoW,QAAF,EAAD,CAAJ,EACCJ,GAAG,CAAChW,CAAC,CAACqW,UAAF,EAAD,CADJ,EAECL,GAAG,CAAChW,CAAC,CAACsW,UAAF,EAAD,CAFJ,EAEsBtX,IAFtB,CAE2B,GAF3B,CAAX;CAGA,SAAO,CAACgB,CAAC,CAACuW,OAAF,EAAD,EAAcN,MAAM,CAACjW,CAAC,CAACwW,QAAF,EAAD,CAApB,EAAoCL,IAApC,EAA0CnX,IAA1C,CAA+C,GAA/C,CAAP;CACD;;;CAIM,SAAS4B,GAAT,GAAe;CACpBU,EAAAA,OAAO,CAACV,GAAR,CAAY,SAAZ,EAAuBsV,WAAS,EAAhC,EAAoCtF,MAAM,CAACjK,KAAP,CAAa,IAAb,EAAmBhB,SAAnB,CAApC;CACD;CAmBM,SAASkN,OAAT,CAAiB4D,MAAjB,EAAyBC,GAAzB,EAA8B;CACnC;CACA,MAAI,CAACA,GAAD,IAAQ,CAACrF,QAAQ,CAACqF,GAAD,CAArB,EAA4B,OAAOD,MAAP;CAE5B,MAAI7C,IAAI,GAAGlS,MAAM,CAACkS,IAAP,CAAY8C,GAAZ,CAAX;CACA,MAAIjZ,CAAC,GAAGmW,IAAI,CAACjW,MAAb;;CACA,SAAOF,CAAC,EAAR,EAAY;CACVgZ,IAAAA,MAAM,CAAC7C,IAAI,CAACnW,CAAD,CAAL,CAAN,GAAkBiZ,GAAG,CAAC9C,IAAI,CAACnW,CAAD,CAAL,CAArB;CACD;;CACD,SAAOgZ,MAAP;CACD;;CAED,SAAS1B,cAAT,CAAwBxQ,GAAxB,EAA6BoS,IAA7B,EAAmC;CACjC,SAAOjV,MAAM,CAACE,SAAP,CAAiBmT,cAAjB,CAAgCtP,IAAhC,CAAqClB,GAArC,EAA0CoS,IAA1C,CAAP;CACD;;AAED,kBAAe;CACbxG,EAAAA,QAAQ,EAAEA,UADG;CAEb0C,EAAAA,OAAO,EAAEA,OAFI;CAGbjS,EAAAA,GAAG,EAAEA,GAHQ;CAIboB,EAAAA,QAAQ,EAAEA,QAJG;CAKb6T,EAAAA,WAAW,EAAEA,WALA;CAMbpC,EAAAA,UAAU,EAAEA,UANC;CAObM,EAAAA,OAAO,EAAEA,OAPI;CAQbG,EAAAA,MAAM,EAAEA,MARK;CASb7C,EAAAA,QAAQ,EAAEA,QATG;CAUb4C,EAAAA,QAAQ,EAAEA,QAVG;CAWbxC,EAAAA,WAAW,EAAEA,WAXA;CAYbiE,EAAAA,QAAQ,EAAEA,QAZG;CAab5E,EAAAA,QAAQ,EAAEA,QAbG;CAcb+D,EAAAA,QAAQ,EAAEA,QAdG;CAebY,EAAAA,iBAAiB,EAAEA,iBAfN;CAgBbrE,EAAAA,MAAM,EAAEA,MAhBK;CAiBbuB,EAAAA,SAAS,EAAEA,SAjBE;CAkBbjO,EAAAA,OAAO,EAAEA,OAlBI;CAmBboC,EAAAA,OAAO,EAAEA,OAnBI;CAoBbwK,EAAAA,SAAS,EAAEA,SApBE;CAqBbV,EAAAA,MAAM,EAAEA,MArBK;CAsBbmB,EAAAA,QAAQ,EAAEA;CAtBG,CAAf;;;;;AC9jBgC;CAIhC;;;;CAIA,SAAgB,wBAAwB,CAAC,EAAY;KACnD,OAAO,EAAE,CAAC,QAAQ,EAAE,CAAC,OAAO,CAAC,WAAW,EAAE,YAAY,CAAC,CAAC;CAC1D,CAAC;CAFD,4DAEC;CAED,IAAM,aAAa,GACjB,OAAOpC,cAAM,CAAC,SAAS,KAAK,QAAQ,IAAIA,cAAM,CAAC,SAAS,CAAC,OAAO,KAAK,aAAa,CAAC;CAErF,IAAM,eAAe,GAAG,aAAa;OACjC,0IAA0I;OAC1I,+GAA+G,CAAC;CAEpH,IAAM,mBAAmB,GAAwB,SAAS,mBAAmB,CAAC,IAAY;KACxF,OAAO,CAAC,IAAI,CAAC,eAAe,CAAC,CAAC;KAE9B,IAAM,MAAM,GAAGiH,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;KAClC,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,EAAE,EAAE,CAAC;SAAE,MAAM,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,MAAM,EAAE,GAAG,GAAG,CAAC,CAAC;KAC3E,OAAO,MAAM,CAAC;CAChB,CAAC,CAAC;CAUF,IAAM,iBAAiB,GAAG;KACxB,IAAI,OAAO,MAAM,KAAK,WAAW,EAAE;;SAEjC,IAAM,QAAM,GAAG,MAAM,CAAC,MAAM,IAAI,MAAM,CAAC,QAAQ,CAAC;SAChD,IAAI,QAAM,IAAI,QAAM,CAAC,eAAe,EAAE;aACpC,OAAO,UAAA,IAAI,IAAI,OAAA,QAAM,CAAC,eAAe,CAACA,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,GAAA,CAAC;UAC3D;MACF;KAED,IAAI,OAAOjH,cAAM,KAAK,WAAW,IAAIA,cAAM,CAAC,MAAM,IAAIA,cAAM,CAAC,MAAM,CAAC,eAAe,EAAE;;SAEnF,OAAO,UAAA,IAAI,IAAI,OAAAA,cAAM,CAAC,MAAM,CAAC,eAAe,CAACiH,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,GAAA,CAAC;MAClE;KAED,IAAI,mBAA2D,CAAC;KAChE,IAAI;;SAEF,mBAAmB,GAAG,UAAiB,CAAC,WAAW,CAAC;MACrD;KAAC,OAAO,CAAC,EAAE;;MAEX;;KAID,OAAO,mBAAmB,IAAI,mBAAmB,CAAC;CACpD,CAAC,CAAC;CAEW,mBAAW,GAAG,iBAAiB,EAAE,CAAC;CAE/C,SAAgB,gBAAgB,CAAC,KAAc;KAC7C,OAAO,CAAC,sBAAsB,EAAE,4BAA4B,CAAC,CAAC,QAAQ,CACpE,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CACtC,CAAC;CACJ,CAAC;CAJD,4CAIC;CAED,SAAgB,YAAY,CAAC,KAAc;KACzC,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,qBAAqB,CAAC;CACzE,CAAC;CAFD,oCAEC;CAED,SAAgB,eAAe,CAAC,KAAc;KAC5C,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,wBAAwB,CAAC;CAC5E,CAAC;CAFD,0CAEC;CAED,SAAgB,gBAAgB,CAAC,KAAc;KAC7C,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,yBAAyB,CAAC;CAC7E,CAAC;CAFD,4CAEC;CAED,SAAgB,QAAQ,CAAC,CAAU;KACjC,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,iBAAiB,CAAC;CACjE,CAAC;CAFD,4BAEC;CAED,SAAgB,KAAK,CAAC,CAAU;KAC9B,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,cAAc,CAAC;CAC9D,CAAC;CAFD,sBAEC;CAED;CACA,SAAgB,UAAU;KACxB,OAAO,OAAOjH,cAAM,KAAK,WAAW,IAAI,OAAOA,cAAM,CAAC,MAAM,KAAK,WAAW,CAAC;CAC/E,CAAC;CAFD,gCAEC;CAED;CACA,SAAgB,MAAM,CAAC,CAAU;KAC/B,OAAO,YAAY,CAAC,CAAC,CAAC,IAAI,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,eAAe,CAAC;CAClF,CAAC;CAFD,wBAEC;CAED;;;;;CAKA,SAAgB,YAAY,CAAC,SAAkB;KAC7C,OAAO,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,KAAK,IAAI,CAAC;CAC7D,CAAC;CAFD,oCAEC;CAGD,SAAgB,SAAS,CAAqB,EAAK,EAAE,OAAe;KAClE,IAAI,OAAO,MAAM,KAAK,WAAW,IAAI,OAAO,IAAI,KAAK,WAAW,EAAE;;SAEhE,OAAO,UAAe,CAAC,SAAS,CAAC,EAAE,EAAE,OAAO,CAAC,CAAC;MAC/C;KACD,IAAI,MAAM,GAAG,KAAK,CAAC;KACnB,SAAS,UAAU;SAAgB,cAAkB;cAAlB,UAAkB,EAAlB,qBAAkB,EAAlB,IAAkB;aAAlB,yBAAkB;;SACnD,IAAI,CAAC,MAAM,EAAE;aACX,OAAO,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC;aACtB,MAAM,GAAG,IAAI,CAAC;UACf;SACD,OAAO,EAAE,CAAC,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;MAC7B;KACD,OAAQ,UAA2B,CAAC;CACtC,CAAC;CAdD,8BAcC;;;;;;;;;;;;;;;;;;;;;AC5H+B;AACkB;CAElD;;;;;;;;CAQA,SAAgB,YAAY,CAAC,eAAuD;KAClF,IAAI,WAAW,CAAC,MAAM,CAAC,eAAe,CAAC,EAAE;SACvC,OAAOiH,aAAM,CAAC,IAAI,CAChB,eAAe,CAAC,MAAM,EACtB,eAAe,CAAC,UAAU,EAC1B,eAAe,CAAC,UAAU,CAC3B,CAAC;MACH;KAED,IAAIC,sBAAgB,CAAC,eAAe,CAAC,EAAE;SACrC,OAAOD,aAAM,CAAC,IAAI,CAAC,eAAe,CAAC,CAAC;MACrC;KAED,MAAM,IAAI,SAAS,CAAC,sCAAsC,CAAC,CAAC;CAC9D,CAAC;CAdD,oCAcC;;;;;;;;;;ACzB+B;CAEhC;CACA,IAAM,gBAAgB,GAAG,uHAAuH,CAAC;CAE1I,IAAM,kBAAkB,GAAG,UAAC,GAAW;KAC5C,OAAA,OAAO,GAAG,KAAK,QAAQ,IAAI,gBAAgB,CAAC,IAAI,CAAC,GAAG,CAAC;CAArD,CAAqD,CAAC;CAD3C,0BAAkB,sBACyB;CAEjD,IAAM,qBAAqB,GAAG,UAAC,SAAiB;KACrD,IAAI,CAAC,0BAAkB,CAAC,SAAS,CAAC,EAAE;SAClC,MAAM,IAAI,SAAS,CACjB,uLAAuL,CACxL,CAAC;MACH;KAED,IAAM,kBAAkB,GAAG,SAAS,CAAC,OAAO,CAAC,IAAI,EAAE,EAAE,CAAC,CAAC;KACvD,OAAOA,aAAM,CAAC,IAAI,CAAC,kBAAkB,EAAE,KAAK,CAAC,CAAC;CAChD,CAAC,CAAC;CATW,6BAAqB,yBAShC;CAEK,IAAM,qBAAqB,GAAG,UAAC,MAAc,EAAE,aAAoB;KAApB,8BAAA,EAAA,oBAAoB;KACxE,OAAA,aAAa;WACT,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;aAC5B,GAAG;aACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;aAC5B,GAAG;aACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;aAC5B,GAAG;aACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,EAAE,CAAC;aAC7B,GAAG;aACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,EAAE,EAAE,EAAE,CAAC;WAC9B,MAAM,CAAC,QAAQ,CAAC,KAAK,CAAC;CAV1B,CAU0B,CAAC;CAXhB,6BAAqB,yBAWL;;;;;;;;;;;;AC9BG;AACe;AACb;AAC8D;AACrC;CAO3D,IAAM,WAAW,GAAG,EAAE,CAAC;CAEvB,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;CAEzB;;;;CAIA;;;;;;KAgBE,cAAY,KAA8B;SACxC,IAAI,OAAO,KAAK,KAAK,WAAW,EAAE;;aAEhC,IAAI,CAAC,EAAE,GAAG,IAAI,CAAC,QAAQ,EAAE,CAAC;UAC3B;cAAM,IAAI,KAAK,YAAY,IAAI,EAAE;aAChC,IAAI,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;aAClC,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,CAAC;UACxB;cAAM,IAAI,WAAW,CAAC,MAAM,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,UAAU,KAAK,WAAW,EAAE;aACxE,IAAI,CAAC,EAAE,GAAGE,0BAAY,CAAC,KAAK,CAAC,CAAC;UAC/B;cAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;aACpC,IAAI,CAAC,EAAE,GAAGC,gCAAqB,CAAC,KAAK,CAAC,CAAC;UACxC;cAAM;aACL,MAAM,IAAI,SAAS,CACjB,gLAAgL,CACjL,CAAC;UACH;MACF;KAMD,sBAAI,oBAAE;;;;;cAAN;aACE,OAAO,IAAI,CAAC,GAAG,CAAC,CAAC;UAClB;cAED,UAAO,KAAa;aAClB,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;aAElB,IAAI,IAAI,CAAC,cAAc,EAAE;iBACvB,IAAI,CAAC,IAAI,GAAGA,gCAAqB,CAAC,KAAK,CAAC,CAAC;cAC1C;UACF;;;QARA;;;;;;;;KAkBD,0BAAW,GAAX,UAAY,aAAoB;SAApB,8BAAA,EAAA,oBAAoB;SAC9B,IAAI,IAAI,CAAC,cAAc,IAAI,IAAI,CAAC,IAAI,EAAE;aACpC,OAAO,IAAI,CAAC,IAAI,CAAC;UAClB;SAED,IAAM,aAAa,GAAGA,gCAAqB,CAAC,IAAI,CAAC,EAAE,EAAE,aAAa,CAAC,CAAC;SAEpE,IAAI,IAAI,CAAC,cAAc,EAAE;aACvB,IAAI,CAAC,IAAI,GAAG,aAAa,CAAC;UAC3B;SAED,OAAO,aAAa,CAAC;MACtB;;;;;KAMD,uBAAQ,GAAR,UAAS,QAAiB;SACxB,OAAO,QAAQ,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,QAAQ,CAAC,GAAG,IAAI,CAAC,WAAW,EAAE,CAAC;MACnE;;;;;KAMD,qBAAM,GAAN;SACE,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;MAC3B;;;;;;KAOD,qBAAM,GAAN,UAAO,OAA+B;SACpC,IAAI,CAAC,OAAO,EAAE;aACZ,OAAO,KAAK,CAAC;UACd;SAED,IAAI,OAAO,YAAY,IAAI,EAAE;aAC3B,OAAO,OAAO,CAAC,EAAE,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;UACnC;SAED,IAAI;aACF,OAAO,IAAI,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;UAC7C;SAAC,WAAM;aACN,OAAO,KAAK,CAAC;UACd;MACF;;;;KAKD,uBAAQ,GAAR;SACE,OAAO,IAAIC,aAAM,CAAC,IAAI,CAAC,EAAE,EAAEA,aAAM,CAAC,YAAY,CAAC,CAAC;MACjD;;;;KAKM,aAAQ,GAAf;SACE,IAAM,KAAK,GAAGH,iBAAW,CAAC,WAAW,CAAC,CAAC;;;SAIvC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,IAAI,IAAI,CAAC;SACpC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,IAAI,IAAI,CAAC;SAEpC,OAAOD,aAAM,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;MAC3B;;;;;KAMM,YAAO,GAAd,UAAe,KAA6B;SAC1C,IAAI,CAAC,KAAK,EAAE;aACV,OAAO,KAAK,CAAC;UACd;SAED,IAAI,KAAK,YAAY,IAAI,EAAE;aACzB,OAAO,IAAI,CAAC;UACb;SAED,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;aAC7B,OAAOG,6BAAkB,CAAC,KAAK,CAAC,CAAC;UAClC;SAED,IAAIF,kBAAY,CAAC,KAAK,CAAC,EAAE;;aAEvB,IAAI,KAAK,CAAC,MAAM,KAAK,WAAW,EAAE;iBAChC,OAAO,KAAK,CAAC;cACd;aAED,IAAI;;;iBAGF,OAAO,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,QAAQ,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,KAAKG,aAAM,CAAC,YAAY,CAAC;cACvE;aAAC,WAAM;iBACN,OAAO,KAAK,CAAC;cACd;UACF;SAED,OAAO,KAAK,CAAC;MACd;;;;;KAMM,wBAAmB,GAA1B,UAA2B,SAAiB;SAC1C,IAAM,MAAM,GAAGD,gCAAqB,CAAC,SAAS,CAAC,CAAC;SAChD,OAAO,IAAI,IAAI,CAAC,MAAM,CAAC,CAAC;MACzB;;;;;;;KAQD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,sBAAO,GAAP;SACE,OAAO,gBAAa,IAAI,CAAC,WAAW,EAAE,QAAI,CAAC;MAC5C;KACH,WAAC;CAAD,CAAC,IAAA;CA3LY,oBAAI;CA6LjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;AChNtC;AACe;AACM;AACT;CAoB5C;;;;CAIA;;;;;KAkCE,gBAAY1X,QAAgC,EAAE,OAAgB;SAC5D,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;aAAE,OAAO,IAAI,MAAM,CAACA,QAAM,EAAE,OAAO,CAAC,CAAC;SAElE,IACE,EAAEA,QAAM,IAAI,IAAI,CAAC;aACjB,EAAE,OAAOA,QAAM,KAAK,QAAQ,CAAC;aAC7B,CAAC,WAAW,CAAC,MAAM,CAACA,QAAM,CAAC;aAC3B,EAAEA,QAAM,YAAY,WAAW,CAAC;aAChC,CAAC,KAAK,CAAC,OAAO,CAACA,QAAM,CAAC,EACtB;aACA,MAAM,IAAI,SAAS,CACjB,kFAAkF,CACnF,CAAC;UACH;SAED,IAAI,CAAC,QAAQ,GAAG,OAAO,aAAP,OAAO,cAAP,OAAO,GAAI,MAAM,CAAC,2BAA2B,CAAC;SAE9D,IAAIA,QAAM,IAAI,IAAI,EAAE;;aAElB,IAAI,CAAC,MAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,MAAM,CAAC,WAAW,CAAC,CAAC;aAC/C,IAAI,CAAC,QAAQ,GAAG,CAAC,CAAC;UACnB;cAAM;aACL,IAAI,OAAOvX,QAAM,KAAK,QAAQ,EAAE;;iBAE9B,IAAI,CAAC,MAAM,GAAGuX,aAAM,CAAC,IAAI,CAACvX,QAAM,EAAE,QAAQ,CAAC,CAAC;cAC7C;kBAAM,IAAI,KAAK,CAAC,OAAO,CAACA,QAAM,CAAC,EAAE;;iBAEhC,IAAI,CAAC,MAAM,GAAGuX,aAAM,CAAC,IAAI,CAACvX,QAAM,CAAC,CAAC;cACnC;kBAAM;;iBAEL,IAAI,CAAC,MAAM,GAAGyX,0BAAY,CAACzX,QAAM,CAAC,CAAC;cACpC;aAED,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;UACxC;MACF;;;;;;KAOD,oBAAG,GAAH,UAAI,SAA2D;;SAE7D,IAAI,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,CAAC,MAAM,KAAK,CAAC,EAAE;aAC3D,MAAM,IAAI,SAAS,CAAC,sCAAsC,CAAC,CAAC;UAC7D;cAAM,IAAI,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,CAAC,MAAM,KAAK,CAAC;aAChE,MAAM,IAAI,SAAS,CAAC,mDAAmD,CAAC,CAAC;;SAG3E,IAAI,WAAmB,CAAC;SACxB,IAAI,OAAO,SAAS,KAAK,QAAQ,EAAE;aACjC,WAAW,GAAG,SAAS,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;UACvC;cAAM,IAAI,OAAO,SAAS,KAAK,QAAQ,EAAE;aACxC,WAAW,GAAG,SAAS,CAAC;UACzB;cAAM;aACL,WAAW,GAAG,SAAS,CAAC,CAAC,CAAC,CAAC;UAC5B;SAED,IAAI,WAAW,GAAG,CAAC,IAAI,WAAW,GAAG,GAAG,EAAE;aACxC,MAAM,IAAI,SAAS,CAAC,0DAA0D,CAAC,CAAC;UACjF;SAED,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,EAAE;aACtC,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,GAAG,WAAW,CAAC;UAC5C;cAAM;aACL,IAAMA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,MAAM,CAAC,WAAW,GAAG,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;;aAErE,IAAI,CAAC,MAAM,CAAC,IAAI,CAACvX,QAAM,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;aACnD,IAAI,CAAC,MAAM,GAAGA,QAAM,CAAC;aACrB,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,GAAG,WAAW,CAAC;UAC5C;MACF;;;;;;;KAQD,sBAAK,GAAL,UAAM,QAAiC,EAAE,MAAc;SACrD,MAAM,GAAG,OAAO,MAAM,KAAK,QAAQ,GAAG,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;;SAG7D,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,EAAE;aACjD,IAAMA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,QAAQ,CAAC,MAAM,CAAC,CAAC;aAClE,IAAI,CAAC,MAAM,CAAC,IAAI,CAACvX,QAAM,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;;aAGnD,IAAI,CAAC,MAAM,GAAGA,QAAM,CAAC;UACtB;SAED,IAAI,WAAW,CAAC,MAAM,CAAC,QAAQ,CAAC,EAAE;aAChC,IAAI,CAAC,MAAM,CAAC,GAAG,CAACyX,0BAAY,CAAC,QAAQ,CAAC,EAAE,MAAM,CAAC,CAAC;aAChD,IAAI,CAAC,QAAQ;iBACX,MAAM,GAAG,QAAQ,CAAC,UAAU,GAAG,IAAI,CAAC,QAAQ,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;UAC3F;cAAM,IAAI,OAAO,QAAQ,KAAK,QAAQ,EAAE;aACvC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,QAAQ,EAAE,MAAM,EAAE,QAAQ,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;aAC/D,IAAI,CAAC,QAAQ;iBACX,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;UACvF;MACF;;;;;;;KAQD,qBAAI,GAAJ,UAAK,QAAgB,EAAE,MAAc;SACnC,MAAM,GAAG,MAAM,IAAI,MAAM,GAAG,CAAC,GAAG,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;;SAGvD,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,QAAQ,EAAE,QAAQ,GAAG,MAAM,CAAC,CAAC;MACvD;;;;;;;KAQD,sBAAK,GAAL,UAAM,KAAe;SACnB,KAAK,GAAG,CAAC,CAAC,KAAK,CAAC;;SAGhB,IAAI,KAAK,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,KAAK,IAAI,CAAC,QAAQ,EAAE;aACjD,OAAO,IAAI,CAAC,MAAM,CAAC;UACpB;;SAGD,IAAI,KAAK,EAAE;aACT,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;UAC5C;SACD,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MACzD;;KAGD,uBAAM,GAAN;SACE,OAAO,IAAI,CAAC,QAAQ,CAAC;MACtB;;KAGD,uBAAM,GAAN;SACE,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;MACvC;;KAGD,yBAAQ,GAAR,UAAS,MAAc;SACrB,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC;MACrC;;KAGD,+BAAc,GAAd,UAAe,OAAsB;SACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,IAAM,YAAY,GAAG,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;SAEpD,IAAM,OAAO,GAAG,MAAM,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,QAAQ,CAAC,EAAE,CAAC,CAAC;SACnD,IAAI,OAAO,CAAC,MAAM,EAAE;aAClB,OAAO;iBACL,OAAO,EAAE,YAAY;iBACrB,KAAK,EAAE,OAAO,CAAC,MAAM,KAAK,CAAC,GAAG,GAAG,GAAG,OAAO,GAAG,OAAO;cACtD,CAAC;UACH;SACD,OAAO;aACL,OAAO,EAAE;iBACP,MAAM,EAAE,YAAY;iBACpB,OAAO,EAAE,OAAO,CAAC,MAAM,KAAK,CAAC,GAAG,GAAG,GAAG,OAAO,GAAG,OAAO;cACxD;UACF,CAAC;MACH;;KAGD,uBAAM,GAAN;SACE,IAAI,IAAI,CAAC,QAAQ,KAAK,MAAM,CAAC,YAAY,EAAE;aACzC,OAAO,IAAIG,SAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC;UACtD;SAED,MAAM,IAAI,KAAK,CACb,uBAAoB,IAAI,CAAC,QAAQ,2DAAoD,MAAM,CAAC,YAAY,+BAA2B,CACpI,CAAC;MACH;;KAGM,uBAAgB,GAAvB,UACE,GAAyD,EACzD,OAAsB;SAEtB,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,IAAI,IAAwB,CAAC;SAC7B,IAAI,IAAI,CAAC;SACT,IAAI,SAAS,IAAI,GAAG,EAAE;aACpB,IAAI,OAAO,CAAC,MAAM,IAAI,OAAO,GAAG,CAAC,OAAO,KAAK,QAAQ,IAAI,OAAO,IAAI,GAAG,EAAE;iBACvE,IAAI,GAAG,GAAG,CAAC,KAAK,GAAG,QAAQ,CAAC,GAAG,CAAC,KAAK,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;iBAC/C,IAAI,GAAGL,aAAM,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;cAC3C;kBAAM;iBACL,IAAI,OAAO,GAAG,CAAC,OAAO,KAAK,QAAQ,EAAE;qBACnC,IAAI,GAAG,GAAG,CAAC,OAAO,CAAC,OAAO,GAAG,QAAQ,CAAC,GAAG,CAAC,OAAO,CAAC,OAAO,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;qBACnE,IAAI,GAAGA,aAAM,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;kBAClD;cACF;UACF;cAAM,IAAI,OAAO,IAAI,GAAG,EAAE;aACzB,IAAI,GAAG,CAAC,CAAC;aACT,IAAI,GAAGG,gCAAqB,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC;UACzC;SACD,IAAI,CAAC,IAAI,EAAE;aACT,MAAM,IAAI,SAAS,CAAC,4CAA0C,IAAI,CAAC,SAAS,CAAC,GAAG,CAAG,CAAC,CAAC;UACtF;SACD,OAAO,IAAI,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;MAC/B;;KAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,wBAAO,GAAP;SACE,IAAM,QAAQ,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;SAClC,OAAO,8BAA2B,QAAQ,CAAC,QAAQ,CAAC,KAAK,CAAC,sBAAc,IAAI,CAAC,QAAQ,MAAG,CAAC;MAC1F;;;;;KAtPuB,kCAA2B,GAAG,CAAC,CAAC;;KAGxC,kBAAW,GAAG,GAAG,CAAC;;KAElB,sBAAe,GAAG,CAAC,CAAC;;KAEpB,uBAAgB,GAAG,CAAC,CAAC;;KAErB,yBAAkB,GAAG,CAAC,CAAC;;KAEvB,uBAAgB,GAAG,CAAC,CAAC;;KAErB,mBAAY,GAAG,CAAC,CAAC;;KAEjB,kBAAW,GAAG,CAAC,CAAC;;KAEhB,2BAAoB,GAAG,GAAG,CAAC;KAsO7C,aAAC;EA9PD,IA8PC;CA9PY,wBAAM;CAgQnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;CCnR1E;;;;CAIA;;;;;KASE,cAAY,IAAuB,EAAE,KAAgB;SACnD,IAAI,EAAE,IAAI,YAAY,IAAI,CAAC;aAAE,OAAO,IAAI,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;SAE1D,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC;SACjB,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;MACpB;;KAGD,qBAAM,GAAN;SACE,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,IAAI,EAAE,KAAK,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;MAC/C;;KAGD,6BAAc,GAAd;SACE,IAAI,IAAI,CAAC,KAAK,EAAE;aACd,OAAO,EAAE,KAAK,EAAE,IAAI,CAAC,IAAI,EAAE,MAAM,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;UACjD;SAED,OAAO,EAAE,KAAK,EAAE,IAAI,CAAC,IAAI,EAAE,CAAC;MAC7B;;KAGM,qBAAgB,GAAvB,UAAwB,GAAiB;SACvC,OAAO,IAAI,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,MAAM,CAAC,CAAC;MACxC;;KAGD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,sBAAO,GAAP;SACE,IAAM,QAAQ,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC;SAC/B,OAAO,gBAAa,QAAQ,CAAC,IAAI,WAC/B,QAAQ,CAAC,KAAK,GAAG,OAAK,IAAI,CAAC,SAAS,CAAC,QAAQ,CAAC,KAAK,CAAG,GAAG,EAAE,OAC1D,CAAC;MACL;KACH,WAAC;CAAD,CAAC,IAAA;CA9CY,oBAAI;CAgDjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;ACzDxB;CAS9C;CACA,SAAgB,WAAW,CAAC,KAAc;KACxC,QACEF,kBAAY,CAAC,KAAK,CAAC;SACnB,KAAK,CAAC,GAAG,IAAI,IAAI;SACjB,OAAO,KAAK,CAAC,IAAI,KAAK,QAAQ;UAC7B,KAAK,CAAC,GAAG,IAAI,IAAI,IAAI,OAAO,KAAK,CAAC,GAAG,KAAK,QAAQ,CAAC,EACpD;CACJ,CAAC;CAPD,kCAOC;CAED;;;;CAIA;;;;;;KAaE,eAAY,UAAkB,EAAE,GAAa,EAAE,EAAW,EAAE,MAAiB;SAC3E,IAAI,EAAE,IAAI,YAAY,KAAK,CAAC;aAAE,OAAO,IAAI,KAAK,CAAC,UAAU,EAAE,GAAG,EAAE,EAAE,EAAE,MAAM,CAAC,CAAC;;SAG5E,IAAM,KAAK,GAAG,UAAU,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;SACpC,IAAI,KAAK,CAAC,MAAM,KAAK,CAAC,EAAE;aACtB,EAAE,GAAG,KAAK,CAAC,KAAK,EAAE,CAAC;;aAEnB,UAAU,GAAG,KAAK,CAAC,KAAK,EAAG,CAAC;UAC7B;SAED,IAAI,CAAC,UAAU,GAAG,UAAU,CAAC;SAC7B,IAAI,CAAC,GAAG,GAAG,GAAG,CAAC;SACf,IAAI,CAAC,EAAE,GAAG,EAAE,CAAC;SACb,IAAI,CAAC,MAAM,GAAG,MAAM,IAAI,EAAE,CAAC;MAC5B;KAMD,sBAAI,4BAAS;;;;cAAb;aACE,OAAO,IAAI,CAAC,UAAU,CAAC;UACxB;cAED,UAAc,KAAa;aACzB,IAAI,CAAC,UAAU,GAAG,KAAK,CAAC;UACzB;;;QAJA;;KAOD,sBAAM,GAAN;SACE,IAAM,CAAC,GAAG,MAAM,CAAC,MAAM,CACrB;aACE,IAAI,EAAE,IAAI,CAAC,UAAU;aACrB,GAAG,EAAE,IAAI,CAAC,GAAG;UACd,EACD,IAAI,CAAC,MAAM,CACZ,CAAC;SAEF,IAAI,IAAI,CAAC,EAAE,IAAI,IAAI;aAAE,CAAC,CAAC,GAAG,GAAG,IAAI,CAAC,EAAE,CAAC;SACrC,OAAO,CAAC,CAAC;MACV;;KAGD,8BAAc,GAAd,UAAe,OAAsB;SACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,IAAI,CAAC,GAAc;aACjB,IAAI,EAAE,IAAI,CAAC,UAAU;aACrB,GAAG,EAAE,IAAI,CAAC,GAAG;UACd,CAAC;SAEF,IAAI,OAAO,CAAC,MAAM,EAAE;aAClB,OAAO,CAAC,CAAC;UACV;SAED,IAAI,IAAI,CAAC,EAAE;aAAE,CAAC,CAAC,GAAG,GAAG,IAAI,CAAC,EAAE,CAAC;SAC7B,CAAC,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,CAAC;SAClC,OAAO,CAAC,CAAC;MACV;;KAGM,sBAAgB,GAAvB,UAAwB,GAAc;SACpC,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,GAAG,CAAuB,CAAC;SAC1D,OAAO,IAAI,CAAC,IAAI,CAAC;SACjB,OAAO,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO,IAAI,KAAK,CAAC,GAAG,CAAC,IAAI,EAAE,GAAG,CAAC,GAAG,EAAE,GAAG,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;MACpD;;KAGD,gBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,uBAAO,GAAP;;SAEE,IAAM,GAAG,GACP,IAAI,CAAC,GAAG,KAAK,SAAS,IAAI,IAAI,CAAC,GAAG,CAAC,QAAQ,KAAK,SAAS,GAAG,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,QAAQ,EAAE,CAAC;SAC7F,OAAO,iBAAc,IAAI,CAAC,SAAS,2BAAoB,GAAG,YACxD,IAAI,CAAC,EAAE,GAAG,SAAM,IAAI,CAAC,EAAE,OAAG,GAAG,EAAE,OAC9B,CAAC;MACL;KACH,YAAC;CAAD,CAAC,IAAA;CA/FY,sBAAK;CAiGlB,MAAM,CAAC,cAAc,CAAC,KAAK,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,OAAO,EAAE,CAAC,CAAC;;;;;;;;;;;AC1H1B;CAkB9C;;;CAGA,IAAI,IAAI,GAAgC,SAAS,CAAC;CAMlD,IAAI;KACF,IAAI,GAAI,IAAI,WAAW,CAAC,QAAQ,CAC9B,IAAI,WAAW,CAAC,MAAM;;KAEpB,IAAI,UAAU,CAAC,CAAC,CAAC,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,CAAC,CAAC,CAC/oC,EACD,EAAE,CACH,CAAC,OAAsC,CAAC;EAC1C;CAAC,WAAM;;EAEP;CAED,IAAM,cAAc,GAAG,CAAC,IAAI,EAAE,CAAC;CAC/B,IAAM,cAAc,GAAG,CAAC,IAAI,EAAE,CAAC;CAC/B,IAAM,cAAc,GAAG,cAAc,GAAG,cAAc,CAAC;CACvD,IAAM,cAAc,GAAG,cAAc,GAAG,cAAc,CAAC;CACvD,IAAM,cAAc,GAAG,cAAc,GAAG,CAAC,CAAC;CAE1C;CACA,IAAM,SAAS,GAA4B,EAAE,CAAC;CAE9C;CACA,IAAM,UAAU,GAA4B,EAAE,CAAC;CAO/C;;;;;;;;;;;;;;;;;;CAkBA;;;;;;;;;;;;;;KAkCE,cAAY,GAAiC,EAAE,IAAuB,EAAE,QAAkB;SAA9E,oBAAA,EAAA,OAAiC;SAC3C,IAAI,EAAE,IAAI,YAAY,IAAI,CAAC;aAAE,OAAO,IAAI,IAAI,CAAC,GAAG,EAAE,IAAI,EAAE,QAAQ,CAAC,CAAC;SAElE,IAAI,OAAO,GAAG,KAAK,QAAQ,EAAE;aAC3B,MAAM,CAAC,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;UACnD;cAAM,IAAI,OAAO,GAAG,KAAK,QAAQ,EAAE;aAClC,MAAM,CAAC,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;UACnD;cAAM;aACL,IAAI,CAAC,GAAG,GAAG,GAAG,GAAG,CAAC,CAAC;aACnB,IAAI,CAAC,IAAI,GAAI,IAAe,GAAG,CAAC,CAAC;aACjC,IAAI,CAAC,QAAQ,GAAG,CAAC,CAAC,QAAQ,CAAC;UAC5B;SAED,MAAM,CAAC,cAAc,CAAC,IAAI,EAAE,YAAY,EAAE;aACxC,KAAK,EAAE,IAAI;aACX,YAAY,EAAE,KAAK;aACnB,QAAQ,EAAE,KAAK;aACf,UAAU,EAAE,KAAK;UAClB,CAAC,CAAC;MACJ;;;;;;;;;KA6BM,aAAQ,GAAf,UAAgB,OAAe,EAAE,QAAgB,EAAE,QAAkB;SACnE,OAAO,IAAI,IAAI,CAAC,OAAO,EAAE,QAAQ,EAAE,QAAQ,CAAC,CAAC;MAC9C;;;;;;;KAQM,YAAO,GAAd,UAAe,KAAa,EAAE,QAAkB;SAC9C,IAAI,GAAG,EAAE,SAAS,EAAE,KAAK,CAAC;SAC1B,IAAI,QAAQ,EAAE;aACZ,KAAK,MAAM,CAAC,CAAC;aACb,KAAK,KAAK,GAAG,CAAC,IAAI,KAAK,IAAI,KAAK,GAAG,GAAG,GAAG;iBACvC,SAAS,GAAG,UAAU,CAAC,KAAK,CAAC,CAAC;iBAC9B,IAAI,SAAS;qBAAE,OAAO,SAAS,CAAC;cACjC;aACD,GAAG,GAAG,IAAI,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,KAAK,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,EAAE,IAAI,CAAC,CAAC;aAC3D,IAAI,KAAK;iBAAE,UAAU,CAAC,KAAK,CAAC,GAAG,GAAG,CAAC;aACnC,OAAO,GAAG,CAAC;UACZ;cAAM;aACL,KAAK,IAAI,CAAC,CAAC;aACX,KAAK,KAAK,GAAG,CAAC,GAAG,IAAI,KAAK,IAAI,KAAK,GAAG,GAAG,GAAG;iBAC1C,SAAS,GAAG,SAAS,CAAC,KAAK,CAAC,CAAC;iBAC7B,IAAI,SAAS;qBAAE,OAAO,SAAS,CAAC;cACjC;aACD,GAAG,GAAG,IAAI,CAAC,QAAQ,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;aACtD,IAAI,KAAK;iBAAE,SAAS,CAAC,KAAK,CAAC,GAAG,GAAG,CAAC;aAClC,OAAO,GAAG,CAAC;UACZ;MACF;;;;;;;KAQM,eAAU,GAAjB,UAAkB,KAAa,EAAE,QAAkB;SACjD,IAAI,KAAK,CAAC,KAAK,CAAC;aAAE,OAAO,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;SAC3D,IAAI,QAAQ,EAAE;aACZ,IAAI,KAAK,GAAG,CAAC;iBAAE,OAAO,IAAI,CAAC,KAAK,CAAC;aACjC,IAAI,KAAK,IAAI,cAAc;iBAAE,OAAO,IAAI,CAAC,kBAAkB,CAAC;UAC7D;cAAM;aACL,IAAI,KAAK,IAAI,CAAC,cAAc;iBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;aACpD,IAAI,KAAK,GAAG,CAAC,IAAI,cAAc;iBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;UACxD;SACD,IAAI,KAAK,GAAG,CAAC;aAAE,OAAO,IAAI,CAAC,UAAU,CAAC,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC,GAAG,EAAE,CAAC;SAC9D,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,GAAG,cAAc,GAAG,CAAC,EAAE,CAAC,KAAK,GAAG,cAAc,IAAI,CAAC,EAAE,QAAQ,CAAC,CAAC;MAC1F;;;;;;;KAQM,eAAU,GAAjB,UAAkB,KAAa,EAAE,QAAkB;SACjD,OAAO,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,QAAQ,EAAE,EAAE,QAAQ,CAAC,CAAC;MACpD;;;;;;;;KASM,eAAU,GAAjB,UAAkB,GAAW,EAAE,QAAkB,EAAE,KAAc;SAC/D,IAAI,GAAG,CAAC,MAAM,KAAK,CAAC;aAAE,MAAM,KAAK,CAAC,cAAc,CAAC,CAAC;SAClD,IAAI,GAAG,KAAK,KAAK,IAAI,GAAG,KAAK,UAAU,IAAI,GAAG,KAAK,WAAW,IAAI,GAAG,KAAK,WAAW;aACnF,OAAO,IAAI,CAAC,IAAI,CAAC;SACnB,IAAI,OAAO,QAAQ,KAAK,QAAQ,EAAE;;aAEhC,CAAC,KAAK,GAAG,QAAQ,IAAI,QAAQ,GAAG,KAAK,CAAC,CAAC;UACxC;cAAM;aACL,QAAQ,GAAG,CAAC,CAAC,QAAQ,CAAC;UACvB;SACD,KAAK,GAAG,KAAK,IAAI,EAAE,CAAC;SACpB,IAAI,KAAK,GAAG,CAAC,IAAI,EAAE,GAAG,KAAK;aAAE,MAAM,UAAU,CAAC,OAAO,CAAC,CAAC;SAEvD,IAAI,CAAC,CAAC;SACN,IAAI,CAAC,CAAC,GAAG,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,CAAC;aAAE,MAAM,KAAK,CAAC,iBAAiB,CAAC,CAAC;cAC1D,IAAI,CAAC,KAAK,CAAC,EAAE;aAChB,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,CAAC,EAAE,QAAQ,EAAE,KAAK,CAAC,CAAC,GAAG,EAAE,CAAC;UACjE;;;SAID,IAAM,YAAY,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC,CAAC;SAEzD,IAAI,MAAM,GAAG,IAAI,CAAC,IAAI,CAAC;SACvB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,GAAG,CAAC,MAAM,EAAE,CAAC,IAAI,CAAC,EAAE;aACtC,IAAM,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,GAAG,CAAC,MAAM,GAAG,CAAC,CAAC,EACtC,KAAK,GAAG,QAAQ,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,EAAE,KAAK,CAAC,CAAC;aACtD,IAAI,IAAI,GAAG,CAAC,EAAE;iBACZ,IAAM,KAAK,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;iBACrD,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,CAAC;cACxD;kBAAM;iBACL,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC;iBAClC,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,CAAC;cAC7C;UACF;SACD,MAAM,CAAC,QAAQ,GAAG,QAAQ,CAAC;SAC3B,OAAO,MAAM,CAAC;MACf;;;;;;;;KASM,cAAS,GAAhB,UAAiB,KAAe,EAAE,QAAkB,EAAE,EAAY;SAChE,OAAO,EAAE,GAAG,IAAI,CAAC,WAAW,CAAC,KAAK,EAAE,QAAQ,CAAC,GAAG,IAAI,CAAC,WAAW,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC;MACnF;;;;;;;KAQM,gBAAW,GAAlB,UAAmB,KAAe,EAAE,QAAkB;SACpD,OAAO,IAAI,IAAI,CACb,KAAK,CAAC,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,EAChE,KAAK,CAAC,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,EAChE,QAAQ,CACT,CAAC;MACH;;;;;;;KAQM,gBAAW,GAAlB,UAAmB,KAAe,EAAE,QAAkB;SACpD,OAAO,IAAI,IAAI,CACb,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,KAAK,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,EAChE,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,KAAK,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,EAChE,QAAQ,CACT,CAAC;MACH;;;;;KAMM,WAAM,GAAb,UAAc,KAAU;SACtB,OAAOA,kBAAY,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,YAAY,CAAC,KAAK,IAAI,CAAC;MAC5D;;;;;KAMM,cAAS,GAAhB,UACE,GAAwE,EACxE,QAAkB;SAElB,IAAI,OAAO,GAAG,KAAK,QAAQ;aAAE,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;SACnE,IAAI,OAAO,GAAG,KAAK,QAAQ;aAAE,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;;SAEnE,OAAO,IAAI,CAAC,QAAQ,CAClB,GAAG,CAAC,GAAG,EACP,GAAG,CAAC,IAAI,EACR,OAAO,QAAQ,KAAK,SAAS,GAAG,QAAQ,GAAG,GAAG,CAAC,QAAQ,CACxD,CAAC;MACH;;KAGD,kBAAG,GAAH,UAAI,MAA0C;SAC5C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC;aAAE,MAAM,GAAG,IAAI,CAAC,SAAS,CAAC,MAAM,CAAC,CAAC;;SAI1D,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,EAAE,CAAC;SAC7B,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,GAAG,MAAM,CAAC;SAC/B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC;SAC5B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,GAAG,MAAM,CAAC;SAE9B,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,KAAK,EAAE,CAAC;SAC/B,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,GAAG,MAAM,CAAC;SACjC,IAAM,GAAG,GAAG,MAAM,CAAC,GAAG,KAAK,EAAE,CAAC;SAC9B,IAAM,GAAG,GAAG,MAAM,CAAC,GAAG,GAAG,MAAM,CAAC;SAEhC,IAAI,GAAG,GAAG,CAAC,EACT,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,CAAC;SACV,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,MAAM,CAAC;SACd,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MAC3E;;;;;KAMD,kBAAG,GAAH,UAAI,KAAyC;SAC3C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;aAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;SACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MACnF;;;;;KAMD,sBAAO,GAAP,UAAQ,KAAyC;SAC/C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;aAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;SACvD,IAAI,IAAI,CAAC,EAAE,CAAC,KAAK,CAAC;aAAE,OAAO,CAAC,CAAC;SAC7B,IAAM,OAAO,GAAG,IAAI,CAAC,UAAU,EAAE,EAC/B,QAAQ,GAAG,KAAK,CAAC,UAAU,EAAE,CAAC;SAChC,IAAI,OAAO,IAAI,CAAC,QAAQ;aAAE,OAAO,CAAC,CAAC,CAAC;SACpC,IAAI,CAAC,OAAO,IAAI,QAAQ;aAAE,OAAO,CAAC,CAAC;;SAEnC,IAAI,CAAC,IAAI,CAAC,QAAQ;aAAE,OAAO,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,UAAU,EAAE,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;SAEjE,OAAO,KAAK,CAAC,IAAI,KAAK,CAAC,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC;cACtC,KAAK,CAAC,IAAI,KAAK,IAAI,CAAC,IAAI,IAAI,KAAK,CAAC,GAAG,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;eAC5D,CAAC,CAAC;eACF,CAAC,CAAC;MACP;;KAGD,mBAAI,GAAJ,UAAK,KAAyC;SAC5C,OAAO,IAAI,CAAC,OAAO,CAAC,KAAK,CAAC,CAAC;MAC5B;;;;;KAMD,qBAAM,GAAN,UAAO,OAA2C;SAChD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;aAAE,OAAO,GAAG,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;SAC7D,IAAI,OAAO,CAAC,MAAM,EAAE;aAAE,MAAM,KAAK,CAAC,kBAAkB,CAAC,CAAC;;SAGtD,IAAI,IAAI,EAAE;;;;aAIR,IACE,CAAC,IAAI,CAAC,QAAQ;iBACd,IAAI,CAAC,IAAI,KAAK,CAAC,UAAU;iBACzB,OAAO,CAAC,GAAG,KAAK,CAAC,CAAC;iBAClB,OAAO,CAAC,IAAI,KAAK,CAAC,CAAC,EACnB;;iBAEA,OAAO,IAAI,CAAC;cACb;aACD,IAAM,GAAG,GAAG,CAAC,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,KAAK,EAClD,IAAI,CAAC,GAAG,EACR,IAAI,CAAC,IAAI,EACT,OAAO,CAAC,GAAG,EACX,OAAO,CAAC,IAAI,CACb,CAAC;aACF,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;UAC3D;SAED,IAAI,IAAI,CAAC,MAAM,EAAE;aAAE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;SACjE,IAAI,MAAM,EAAE,GAAG,EAAE,GAAG,CAAC;SACrB,IAAI,CAAC,IAAI,CAAC,QAAQ,EAAE;;;aAGlB,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;iBAC3B,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,GAAG,CAAC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,OAAO,CAAC;qBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;;sBAEvE,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;qBAAE,OAAO,IAAI,CAAC,GAAG,CAAC;sBAChD;;qBAEH,IAAM,QAAQ,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;qBAC7B,MAAM,GAAG,QAAQ,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;qBACtC,IAAI,MAAM,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,EAAE;yBACxB,OAAO,OAAO,CAAC,UAAU,EAAE,GAAG,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC,OAAO,CAAC;sBACvD;0BAAM;yBACL,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,MAAM,CAAC,CAAC,CAAC;yBACpC,GAAG,GAAG,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,CAAC;yBACnC,OAAO,GAAG,CAAC;sBACZ;kBACF;cACF;kBAAM,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;iBAAE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;aACrF,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;iBACrB,IAAI,OAAO,CAAC,UAAU,EAAE;qBAAE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC;iBAC/D,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,EAAE,CAAC;cACtC;kBAAM,IAAI,OAAO,CAAC,UAAU,EAAE;iBAAE,OAAO,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC;aACtE,GAAG,GAAG,IAAI,CAAC,IAAI,CAAC;UACjB;cAAM;;;aAGL,IAAI,CAAC,OAAO,CAAC,QAAQ;iBAAE,OAAO,GAAG,OAAO,CAAC,UAAU,EAAE,CAAC;aACtD,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC;iBAAE,OAAO,IAAI,CAAC,KAAK,CAAC;aACxC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;;iBAE1B,OAAO,IAAI,CAAC,IAAI,CAAC;aACnB,GAAG,GAAG,IAAI,CAAC,KAAK,CAAC;UAClB;;;;;;SAOD,GAAG,GAAG,IAAI,CAAC;SACX,OAAO,GAAG,CAAC,GAAG,CAAC,OAAO,CAAC,EAAE;;;aAGvB,MAAM,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,QAAQ,EAAE,GAAG,OAAO,CAAC,QAAQ,EAAE,CAAC,CAAC,CAAC;;;aAItE,IAAM,IAAI,GAAG,IAAI,CAAC,IAAI,CAAC,IAAI,CAAC,GAAG,CAAC,MAAM,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC;aACpD,IAAM,KAAK,GAAG,IAAI,IAAI,EAAE,GAAG,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,GAAG,EAAE,CAAC,CAAC;;;aAGtD,IAAI,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,MAAM,CAAC,CAAC;aACxC,IAAI,SAAS,GAAG,SAAS,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;aACvC,OAAO,SAAS,CAAC,UAAU,EAAE,IAAI,SAAS,CAAC,EAAE,CAAC,GAAG,CAAC,EAAE;iBAClD,MAAM,IAAI,KAAK,CAAC;iBAChB,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,MAAM,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;iBACnD,SAAS,GAAG,SAAS,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;cACpC;;;aAID,IAAI,SAAS,CAAC,MAAM,EAAE;iBAAE,SAAS,GAAG,IAAI,CAAC,GAAG,CAAC;aAE7C,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;aACzB,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;UAC1B;SACD,OAAO,GAAG,CAAC;MACZ;;KAGD,kBAAG,GAAH,UAAI,OAA2C;SAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;MAC7B;;;;;KAMD,qBAAM,GAAN,UAAO,KAAyC;SAC9C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;aAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;SACvD,IAAI,IAAI,CAAC,QAAQ,KAAK,KAAK,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,KAAK,EAAE,KAAK,CAAC,IAAI,KAAK,CAAC,IAAI,KAAK,EAAE,KAAK,CAAC;aACvF,OAAO,KAAK,CAAC;SACf,OAAO,IAAI,CAAC,IAAI,KAAK,KAAK,CAAC,IAAI,IAAI,IAAI,CAAC,GAAG,KAAK,KAAK,CAAC,GAAG,CAAC;MAC3D;;KAGD,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC;MAC3B;;KAGD,0BAAW,GAAX;SACE,OAAO,IAAI,CAAC,IAAI,CAAC;MAClB;;KAGD,kCAAmB,GAAnB;SACE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC;MACxB;;KAGD,yBAAU,GAAV;SACE,OAAO,IAAI,CAAC,GAAG,CAAC;MACjB;;KAGD,iCAAkB,GAAlB;SACE,OAAO,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;MACvB;;KAGD,4BAAa,GAAb;SACE,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;;aAErB,OAAO,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,GAAG,EAAE,GAAG,IAAI,CAAC,GAAG,EAAE,CAAC,aAAa,EAAE,CAAC;UAClE;SACD,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC,GAAG,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC;SACnD,IAAI,GAAW,CAAC;SAChB,KAAK,GAAG,GAAG,EAAE,EAAE,GAAG,GAAG,CAAC,EAAE,GAAG,EAAE;aAAE,IAAI,CAAC,GAAG,IAAI,CAAC,IAAI,GAAG,CAAC,MAAM,CAAC;iBAAE,MAAM;SACnE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,GAAG,GAAG,GAAG,EAAE,GAAG,GAAG,GAAG,CAAC,CAAC;MAC7C;;KAGD,0BAAW,GAAX,UAAY,KAAyC;SACnD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;MAC7B;;KAGD,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,WAAW,CAAC,KAAK,CAAC,CAAC;MAChC;;KAGD,iCAAkB,GAAlB,UAAmB,KAAyC;SAC1D,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;MAC9B;;KAGD,kBAAG,GAAH,UAAI,KAAyC;SAC3C,OAAO,IAAI,CAAC,kBAAkB,CAAC,KAAK,CAAC,CAAC;MACvC;;KAED,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,kBAAkB,CAAC,KAAK,CAAC,CAAC;MACvC;;KAGD,qBAAM,GAAN;SACE,OAAO,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC,MAAM,CAAC,CAAC;MAC7B;;KAGD,yBAAU,GAAV;SACE,OAAO,CAAC,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,GAAG,CAAC,CAAC;MACxC;;KAGD,oBAAK,GAAL;SACE,OAAO,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC,MAAM,CAAC,CAAC;MAC7B;;KAGD,yBAAU,GAAV;SACE,OAAO,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC,CAAC;MACxC;;KAGD,qBAAM,GAAN;SACE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;MAC1C;;KAGD,uBAAQ,GAAR,UAAS,KAAyC;SAChD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;MAC7B;;KAGD,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;MAC7B;;KAGD,8BAAe,GAAf,UAAgB,KAAyC;SACvD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;MAC9B;;KAGD,kBAAG,GAAH,UAAI,KAAyC;SAC3C,OAAO,IAAI,CAAC,eAAe,CAAC,KAAK,CAAC,CAAC;MACpC;;KAGD,qBAAM,GAAN,UAAO,OAA2C;SAChD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;aAAE,OAAO,GAAG,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;;SAG7D,IAAI,IAAI,EAAE;aACR,IAAM,GAAG,GAAG,CAAC,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,KAAK,EAClD,IAAI,CAAC,GAAG,EACR,IAAI,CAAC,IAAI,EACT,OAAO,CAAC,GAAG,EACX,OAAO,CAAC,IAAI,CACb,CAAC;aACF,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;UAC3D;SAED,OAAO,IAAI,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,CAAC;MACjD;;KAGD,kBAAG,GAAH,UAAI,OAA2C;SAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;MAC7B;;KAED,kBAAG,GAAH,UAAI,OAA2C;SAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;MAC7B;;;;;;KAOD,uBAAQ,GAAR,UAAS,UAA8C;SACrD,IAAI,IAAI,CAAC,MAAM,EAAE;aAAE,OAAO,IAAI,CAAC,IAAI,CAAC;SACpC,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;aAAE,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;;SAGtE,IAAI,IAAI,EAAE;aACR,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,UAAU,CAAC,GAAG,EAAE,UAAU,CAAC,IAAI,CAAC,CAAC;aAC3E,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;UAC3D;SAED,IAAI,UAAU,CAAC,MAAM,EAAE;aAAE,OAAO,IAAI,CAAC,IAAI,CAAC;SAC1C,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;aAAE,OAAO,UAAU,CAAC,KAAK,EAAE,GAAG,IAAI,CAAC,SAAS,GAAG,IAAI,CAAC,IAAI,CAAC;SACpF,IAAI,UAAU,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;aAAE,OAAO,IAAI,CAAC,KAAK,EAAE,GAAG,IAAI,CAAC,SAAS,GAAG,IAAI,CAAC,IAAI,CAAC;SAEpF,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;aACrB,IAAI,UAAU,CAAC,UAAU,EAAE;iBAAE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC;;iBAChE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC,GAAG,EAAE,CAAC;UAC9C;cAAM,IAAI,UAAU,CAAC,UAAU,EAAE;aAAE,OAAO,IAAI,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC;;SAG5E,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,UAAU,CAAC,IAAI,UAAU,CAAC,EAAE,CAAC,IAAI,CAAC,UAAU,CAAC;aAC5D,OAAO,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,QAAQ,EAAE,GAAG,UAAU,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;;SAKjF,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,EAAE,CAAC;SAC7B,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,GAAG,MAAM,CAAC;SAC/B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC;SAC5B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,GAAG,MAAM,CAAC;SAE9B,IAAM,GAAG,GAAG,UAAU,CAAC,IAAI,KAAK,EAAE,CAAC;SACnC,IAAM,GAAG,GAAG,UAAU,CAAC,IAAI,GAAG,MAAM,CAAC;SACrC,IAAM,GAAG,GAAG,UAAU,CAAC,GAAG,KAAK,EAAE,CAAC;SAClC,IAAM,GAAG,GAAG,UAAU,CAAC,GAAG,GAAG,MAAM,CAAC;SAEpC,IAAI,GAAG,GAAG,CAAC,EACT,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,CAAC;SACV,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;SACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;SAClB,GAAG,IAAI,MAAM,CAAC;SACd,GAAG,IAAI,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,CAAC;SACrD,GAAG,IAAI,MAAM,CAAC;SACd,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MAC3E;;KAGD,kBAAG,GAAH,UAAI,UAA8C;SAChD,OAAO,IAAI,CAAC,QAAQ,CAAC,UAAU,CAAC,CAAC;MAClC;;KAGD,qBAAM,GAAN;SACE,IAAI,CAAC,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;aAAE,OAAO,IAAI,CAAC,SAAS,CAAC;SACrE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;MACjC;;KAGD,kBAAG,GAAH;SACE,OAAO,IAAI,CAAC,MAAM,EAAE,CAAC;MACtB;;KAGD,kBAAG,GAAH;SACE,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MAC5D;;KAGD,wBAAS,GAAT,UAAU,KAAyC;SACjD,OAAO,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC;MAC5B;;KAGD,kBAAG,GAAH,UAAI,KAAyC;SAC3C,OAAO,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;MAC9B;;KAED,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;MAC9B;;;;KAKD,iBAAE,GAAF,UAAG,KAA6B;SAC9B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;aAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;SACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MACnF;;;;;;KAOD,wBAAS,GAAT,UAAU,OAAsB;SAC9B,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;aAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;SACpD,IAAI,CAAC,OAAO,IAAI,EAAE,MAAM,CAAC;aAAE,OAAO,IAAI,CAAC;cAClC,IAAI,OAAO,GAAG,EAAE;aACnB,OAAO,IAAI,CAAC,QAAQ,CAClB,IAAI,CAAC,GAAG,IAAI,OAAO,EACnB,CAAC,IAAI,CAAC,IAAI,IAAI,OAAO,KAAK,IAAI,CAAC,GAAG,MAAM,EAAE,GAAG,OAAO,CAAC,CAAC,EACtD,IAAI,CAAC,QAAQ,CACd,CAAC;;aACC,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,EAAE,IAAI,CAAC,GAAG,KAAK,OAAO,GAAG,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MACzE;;KAGD,kBAAG,GAAH,UAAI,OAAsB;SACxB,OAAO,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;MAChC;;;;;;KAOD,yBAAU,GAAV,UAAW,OAAsB;SAC/B,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;aAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;SACpD,IAAI,CAAC,OAAO,IAAI,EAAE,MAAM,CAAC;aAAE,OAAO,IAAI,CAAC;cAClC,IAAI,OAAO,GAAG,EAAE;aACnB,OAAO,IAAI,CAAC,QAAQ,CAClB,CAAC,IAAI,CAAC,GAAG,KAAK,OAAO,KAAK,IAAI,CAAC,IAAI,KAAK,EAAE,GAAG,OAAO,CAAC,CAAC,EACtD,IAAI,CAAC,IAAI,IAAI,OAAO,EACpB,IAAI,CAAC,QAAQ,CACd,CAAC;;aACC,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,IAAI,KAAK,OAAO,GAAG,EAAE,CAAC,EAAE,IAAI,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MAChG;;KAGD,kBAAG,GAAH,UAAI,OAAsB;SACxB,OAAO,IAAI,CAAC,UAAU,CAAC,OAAO,CAAC,CAAC;MACjC;;;;;;KAOD,iCAAkB,GAAlB,UAAmB,OAAsB;SACvC,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;aAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;SACpD,OAAO,IAAI,EAAE,CAAC;SACd,IAAI,OAAO,KAAK,CAAC;aAAE,OAAO,IAAI,CAAC;cAC1B;aACH,IAAM,IAAI,GAAG,IAAI,CAAC,IAAI,CAAC;aACvB,IAAI,OAAO,GAAG,EAAE,EAAE;iBAChB,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC;iBACrB,OAAO,IAAI,CAAC,QAAQ,CAClB,CAAC,GAAG,KAAK,OAAO,KAAK,IAAI,KAAK,EAAE,GAAG,OAAO,CAAC,CAAC,EAC5C,IAAI,KAAK,OAAO,EAChB,IAAI,CAAC,QAAQ,CACd,CAAC;cACH;kBAAM,IAAI,OAAO,KAAK,EAAE;iBAAE,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;iBACnE,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,MAAM,OAAO,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;UACtE;MACF;;KAGD,oBAAK,GAAL,UAAM,OAAsB;SAC1B,OAAO,IAAI,CAAC,kBAAkB,CAAC,OAAO,CAAC,CAAC;MACzC;;KAED,mBAAI,GAAJ,UAAK,OAAsB;SACzB,OAAO,IAAI,CAAC,kBAAkB,CAAC,OAAO,CAAC,CAAC;MACzC;;;;;;KAOD,uBAAQ,GAAR,UAAS,UAA8C;SACrD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;aAAE,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;SACtE,OAAO,IAAI,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC;MACnC;;KAGD,kBAAG,GAAH,UAAI,UAA8C;SAChD,OAAO,IAAI,CAAC,QAAQ,CAAC,UAAU,CAAC,CAAC;MAClC;;KAGD,oBAAK,GAAL;SACE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC;MAClD;;KAGD,uBAAQ,GAAR;SACE,IAAI,IAAI,CAAC,QAAQ;aAAE,OAAO,CAAC,IAAI,CAAC,IAAI,KAAK,CAAC,IAAI,cAAc,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC;SAChF,OAAO,IAAI,CAAC,IAAI,GAAG,cAAc,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC;MACtD;;KAGD,uBAAQ,GAAR;SACE,OAAO,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,CAAC;MAChC;;;;;;KAOD,sBAAO,GAAP,UAAQ,EAAY;SAClB,OAAO,EAAE,GAAG,IAAI,CAAC,SAAS,EAAE,GAAG,IAAI,CAAC,SAAS,EAAE,CAAC;MACjD;;;;;KAMD,wBAAS,GAAT;SACE,IAAM,EAAE,GAAG,IAAI,CAAC,IAAI,EAClB,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO;aACL,EAAE,GAAG,IAAI;aACT,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;aACjB,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;aAClB,EAAE,KAAK,EAAE;aACT,EAAE,GAAG,IAAI;aACT,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;aACjB,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;aAClB,EAAE,KAAK,EAAE;UACV,CAAC;MACH;;;;;KAMD,wBAAS,GAAT;SACE,IAAM,EAAE,GAAG,IAAI,CAAC,IAAI,EAClB,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO;aACL,EAAE,KAAK,EAAE;aACT,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;aAClB,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;aACjB,EAAE,GAAG,IAAI;aACT,EAAE,KAAK,EAAE;aACT,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;aAClB,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;aACjB,EAAE,GAAG,IAAI;UACV,CAAC;MACH;;;;KAKD,uBAAQ,GAAR;SACE,IAAI,CAAC,IAAI,CAAC,QAAQ;aAAE,OAAO,IAAI,CAAC;SAChC,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;MAClD;;;;;;KAOD,uBAAQ,GAAR,UAAS,KAAc;SACrB,KAAK,GAAG,KAAK,IAAI,EAAE,CAAC;SACpB,IAAI,KAAK,GAAG,CAAC,IAAI,EAAE,GAAG,KAAK;aAAE,MAAM,UAAU,CAAC,OAAO,CAAC,CAAC;SACvD,IAAI,IAAI,CAAC,MAAM,EAAE;aAAE,OAAO,GAAG,CAAC;SAC9B,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;;aAErB,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;;;iBAG3B,IAAM,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,EACtC,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,SAAS,CAAC,EACzB,IAAI,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;iBACtC,OAAO,GAAG,CAAC,QAAQ,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC,KAAK,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;cAC3D;;iBAAM,OAAO,GAAG,GAAG,IAAI,CAAC,GAAG,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;UAChD;;;SAID,IAAM,YAAY,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;SAExE,IAAI,GAAG,GAAS,IAAI,CAAC;SACrB,IAAI,MAAM,GAAG,EAAE,CAAC;;SAEhB,OAAO,IAAI,EAAE;aACX,IAAM,MAAM,GAAG,GAAG,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC;aACrC,IAAM,MAAM,GAAG,GAAG,CAAC,GAAG,CAAC,MAAM,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC,CAAC,KAAK,EAAE,KAAK,CAAC,CAAC;aAC/D,IAAI,MAAM,GAAG,MAAM,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aACpC,GAAG,GAAG,MAAM,CAAC;aACb,IAAI,GAAG,CAAC,MAAM,EAAE,EAAE;iBAChB,OAAO,MAAM,GAAG,MAAM,CAAC;cACxB;kBAAM;iBACL,OAAO,MAAM,CAAC,MAAM,GAAG,CAAC;qBAAE,MAAM,GAAG,GAAG,GAAG,MAAM,CAAC;iBAChD,MAAM,GAAG,EAAE,GAAG,MAAM,GAAG,MAAM,CAAC;cAC/B;UACF;MACF;;KAGD,yBAAU,GAAV;SACE,IAAI,IAAI,CAAC,QAAQ;aAAE,OAAO,IAAI,CAAC;SAC/B,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;MACjD;;KAGD,kBAAG,GAAH,UAAI,KAA6B;SAC/B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;aAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;SACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;MACnF;;KAGD,kBAAG,GAAH;SACE,OAAO,IAAI,CAAC,MAAM,EAAE,CAAC;MACtB;;KAGD,iBAAE,GAAF,UAAG,KAAyC;SAC1C,OAAO,IAAI,CAAC,eAAe,CAAC,KAAK,CAAC,CAAC;MACpC;;;;;;KAOD,6BAAc,GAAd,UAAe,OAAsB;SACnC,IAAI,OAAO,IAAI,OAAO,CAAC,OAAO;aAAE,OAAO,IAAI,CAAC,QAAQ,EAAE,CAAC;SACvD,OAAO,EAAE,WAAW,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;MACzC;KACM,qBAAgB,GAAvB,UAAwB,GAA4B,EAAE,OAAsB;SAC1E,IAAM,MAAM,GAAG,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,WAAW,CAAC,CAAC;SAChD,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,MAAM,CAAC,QAAQ,EAAE,GAAG,MAAM,CAAC;MAChE;;KAGD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,sBAAO,GAAP;SACE,OAAO,gBAAa,IAAI,CAAC,QAAQ,EAAE,WAAI,IAAI,CAAC,QAAQ,GAAG,QAAQ,GAAG,EAAE,OAAG,CAAC;MACzE;KA/2BM,eAAU,GAAG,IAAI,CAAC,OAAO,CAAC,cAAc,CAAC,CAAC;;KAG1C,uBAAkB,GAAG,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,IAAI,CAAC,CAAC;;KAEzE,SAAI,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;;KAEvB,UAAK,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;;KAE9B,QAAG,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;;KAEtB,SAAI,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;;KAE7B,YAAO,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC,CAAC;;KAE3B,cAAS,GAAG,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;;KAEjE,cAAS,GAAG,IAAI,CAAC,QAAQ,CAAC,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;KA+1B7D,WAAC;EAv6BD,IAu6BC;CAv6BY,oBAAI;CAy6BjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,YAAY,EAAE,EAAE,KAAK,EAAE,IAAI,EAAE,CAAC,CAAC;CACrE,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;ACr/BtC;AACF;CAE9B,IAAM,mBAAmB,GAAG,+CAA+C,CAAC;CAC5E,IAAM,gBAAgB,GAAG,0BAA0B,CAAC;CACpD,IAAM,gBAAgB,GAAG,eAAe,CAAC;CAEzC,IAAM,YAAY,GAAG,IAAI,CAAC;CAC1B,IAAM,YAAY,GAAG,CAAC,IAAI,CAAC;CAC3B,IAAM,aAAa,GAAG,IAAI,CAAC;CAC3B,IAAM,UAAU,GAAG,EAAE,CAAC;CAEtB;CACA,IAAM,UAAU,GAAG;KACjB,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;EACL,CAAC,OAAO,EAAE,CAAC;CACZ;CACA,IAAM,mBAAmB,GAAG;KAC1B,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;EACL,CAAC,OAAO,EAAE,CAAC;CACZ,IAAM,mBAAmB,GAAG;KAC1B,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;KACJ,IAAI;EACL,CAAC,OAAO,EAAE,CAAC;CAEZ,IAAM,cAAc,GAAG,iBAAiB,CAAC;CAEzC;CACA,IAAM,gBAAgB,GAAG,IAAI,CAAC;CAC9B;CACA,IAAM,aAAa,GAAG,MAAM,CAAC;CAC7B;CACA,IAAM,oBAAoB,GAAG,EAAE,CAAC;CAChC;CACA,IAAM,eAAe,GAAG,EAAE,CAAC;CAE3B;CACA,SAAS,OAAO,CAAC,KAAa;KAC5B,OAAO,CAAC,KAAK,CAAC,QAAQ,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,CAAC;CACrC,CAAC;CAED;CACA,SAAS,UAAU,CAAC,KAAkD;KACpE,IAAM,OAAO,GAAG,WAAI,CAAC,UAAU,CAAC,IAAI,GAAG,IAAI,GAAG,IAAI,CAAC,CAAC;KACpD,IAAI,IAAI,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;KAE9B,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE;SAC5E,OAAO,EAAE,QAAQ,EAAE,KAAK,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;MACvC;KAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;;SAE3B,IAAI,GAAG,IAAI,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC;;SAE1B,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC,IAAI,WAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;SAC7C,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC;SACvC,IAAI,GAAG,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;MAC7B;KAED,OAAO,EAAE,QAAQ,EAAE,KAAK,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;CACxC,CAAC;CAED;CACA,SAAS,YAAY,CAAC,IAAU,EAAE,KAAW;KAC3C,IAAI,CAAC,IAAI,IAAI,CAAC,KAAK,EAAE;SACnB,OAAO,EAAE,IAAI,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,GAAG,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,CAAC;MAC9D;KAED,IAAM,QAAQ,GAAG,IAAI,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC;KAC7C,IAAM,OAAO,GAAG,IAAI,WAAI,CAAC,IAAI,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC;KAC/C,IAAM,SAAS,GAAG,KAAK,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC;KAC/C,IAAM,QAAQ,GAAG,IAAI,WAAI,CAAC,KAAK,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC;KAEjD,IAAI,WAAW,GAAG,QAAQ,CAAC,QAAQ,CAAC,SAAS,CAAC,CAAC;KAC/C,IAAI,UAAU,GAAG,QAAQ,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;KAC7C,IAAM,WAAW,GAAG,OAAO,CAAC,QAAQ,CAAC,SAAS,CAAC,CAAC;KAChD,IAAI,UAAU,GAAG,OAAO,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;KAE5C,WAAW,GAAG,WAAW,CAAC,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;KACjE,UAAU,GAAG,IAAI,WAAI,CAAC,UAAU,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC;UAC9C,GAAG,CAAC,WAAW,CAAC;UAChB,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;KAE1C,WAAW,GAAG,WAAW,CAAC,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;KACjE,UAAU,GAAG,UAAU,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,GAAG,CAAC,IAAI,WAAI,CAAC,UAAU,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC,CAAC;;KAGhF,OAAO,EAAE,IAAI,EAAE,WAAW,EAAE,GAAG,EAAE,UAAU,EAAE,CAAC;CAChD,CAAC;CAED,SAAS,QAAQ,CAAC,IAAU,EAAE,KAAW;;KAEvC,IAAM,MAAM,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC;KAC/B,IAAM,OAAO,GAAG,KAAK,CAAC,IAAI,KAAK,CAAC,CAAC;;KAGjC,IAAI,MAAM,GAAG,OAAO,EAAE;SACpB,OAAO,IAAI,CAAC;MACb;UAAM,IAAI,MAAM,KAAK,OAAO,EAAE;SAC7B,IAAM,MAAM,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;SAC9B,IAAM,OAAO,GAAG,KAAK,CAAC,GAAG,KAAK,CAAC,CAAC;SAChC,IAAI,MAAM,GAAG,OAAO;aAAE,OAAO,IAAI,CAAC;MACnC;KAED,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,UAAU,CAAC,MAAc,EAAE,OAAe;KACjD,MAAM,IAAI,SAAS,CAAC,OAAI,MAAM,8CAAwC,OAAS,CAAC,CAAC;CACnF,CAAC;CAOD;;;;CAIA;;;;;KASE,oBAAY,KAAsB;SAChC,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;aAAE,OAAO,IAAI,UAAU,CAAC,KAAK,CAAC,CAAC;SAEhE,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;aAC7B,IAAI,CAAC,KAAK,GAAG,UAAU,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,KAAK,CAAC;UACjD;cAAM;aACL,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;UACpB;MACF;;;;;;KAOM,qBAAU,GAAjB,UAAkB,cAAsB;;SAEtC,IAAI,UAAU,GAAG,KAAK,CAAC;SACvB,IAAI,QAAQ,GAAG,KAAK,CAAC;SACrB,IAAI,YAAY,GAAG,KAAK,CAAC;;SAGzB,IAAI,iBAAiB,GAAG,CAAC,CAAC;;SAE1B,IAAI,WAAW,GAAG,CAAC,CAAC;;SAEpB,IAAI,OAAO,GAAG,CAAC,CAAC;;SAEhB,IAAI,aAAa,GAAG,CAAC,CAAC;;SAEtB,IAAI,YAAY,GAAG,CAAC,CAAC;;SAGrB,IAAM,MAAM,GAAG,CAAC,CAAC,CAAC,CAAC;;SAEnB,IAAI,aAAa,GAAG,CAAC,CAAC;;SAEtB,IAAI,YAAY,GAAG,CAAC,CAAC;;SAErB,IAAI,UAAU,GAAG,CAAC,CAAC;;SAEnB,IAAI,SAAS,GAAG,CAAC,CAAC;;SAGlB,IAAI,QAAQ,GAAG,CAAC,CAAC;;SAEjB,IAAI,CAAC,GAAG,CAAC,CAAC;;SAEV,IAAI,eAAe,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;;SAErC,IAAI,cAAc,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;;SAEpC,IAAI,cAAc,GAAG,CAAC,CAAC;;SAGvB,IAAI,KAAK,GAAG,CAAC,CAAC;;;;SAKd,IAAI,cAAc,CAAC,MAAM,IAAI,IAAI,EAAE;aACjC,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;UAC7E;;SAGD,IAAM,WAAW,GAAG,cAAc,CAAC,KAAK,CAAC,mBAAmB,CAAC,CAAC;SAC9D,IAAM,QAAQ,GAAG,cAAc,CAAC,KAAK,CAAC,gBAAgB,CAAC,CAAC;SACxD,IAAM,QAAQ,GAAG,cAAc,CAAC,KAAK,CAAC,gBAAgB,CAAC,CAAC;;SAGxD,IAAI,CAAC,CAAC,WAAW,IAAI,CAAC,QAAQ,IAAI,CAAC,QAAQ,KAAK,cAAc,CAAC,MAAM,KAAK,CAAC,EAAE;aAC3E,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;UAC7E;SAED,IAAI,WAAW,EAAE;;;aAIf,IAAM,cAAc,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;;;aAItC,IAAM,CAAC,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;aACzB,IAAM,OAAO,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;aAC/B,IAAM,SAAS,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;;aAGjC,IAAI,CAAC,IAAI,SAAS,KAAK,SAAS;iBAAE,UAAU,CAAC,cAAc,EAAE,wBAAwB,CAAC,CAAC;;aAGvF,IAAI,CAAC,IAAI,cAAc,KAAK,SAAS;iBAAE,UAAU,CAAC,cAAc,EAAE,uBAAuB,CAAC,CAAC;aAE3F,IAAI,CAAC,KAAK,SAAS,KAAK,OAAO,IAAI,SAAS,CAAC,EAAE;iBAC7C,UAAU,CAAC,cAAc,EAAE,2BAA2B,CAAC,CAAC;cACzD;UACF;;SAGD,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;aAClE,UAAU,GAAG,cAAc,CAAC,KAAK,EAAE,CAAC,KAAK,GAAG,CAAC;UAC9C;;SAGD,IAAI,CAAC,OAAO,CAAC,cAAc,CAAC,KAAK,CAAC,CAAC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;aACpE,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;iBAClE,OAAO,IAAI,UAAU,CAACD,aAAM,CAAC,IAAI,CAAC,UAAU,GAAG,mBAAmB,GAAG,mBAAmB,CAAC,CAAC,CAAC;cAC5F;kBAAM,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;iBACxC,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;cAChD;UACF;;SAGD,OAAO,OAAO,CAAC,cAAc,CAAC,KAAK,CAAC,CAAC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;aACtE,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;iBACjC,IAAI,QAAQ;qBAAE,UAAU,CAAC,cAAc,EAAE,2BAA2B,CAAC,CAAC;iBAEtE,QAAQ,GAAG,IAAI,CAAC;iBAChB,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;iBAClB,SAAS;cACV;aAED,IAAI,aAAa,GAAG,EAAE,EAAE;iBACtB,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,YAAY,EAAE;qBACjD,IAAI,CAAC,YAAY,EAAE;yBACjB,YAAY,GAAG,WAAW,CAAC;sBAC5B;qBAED,YAAY,GAAG,IAAI,CAAC;;qBAGpB,MAAM,CAAC,YAAY,EAAE,CAAC,GAAG,QAAQ,CAAC,cAAc,CAAC,KAAK,CAAC,EAAE,EAAE,CAAC,CAAC;qBAC7D,aAAa,GAAG,aAAa,GAAG,CAAC,CAAC;kBACnC;cACF;aAED,IAAI,YAAY;iBAAE,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC;aACxC,IAAI,QAAQ;iBAAE,aAAa,GAAG,aAAa,GAAG,CAAC,CAAC;aAEhD,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;aAC9B,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;UACnB;SAED,IAAI,QAAQ,IAAI,CAAC,WAAW;aAC1B,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;;SAG9E,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;;aAElE,IAAM,KAAK,GAAG,cAAc,CAAC,MAAM,CAAC,EAAE,KAAK,CAAC,CAAC,KAAK,CAAC,cAAc,CAAC,CAAC;;aAGnE,IAAI,CAAC,KAAK,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC;iBAAE,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;;aAGxE,QAAQ,GAAG,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;;aAGlC,KAAK,GAAG,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC;UACjC;;SAGD,IAAI,cAAc,CAAC,KAAK,CAAC;aAAE,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;;;SAI1E,UAAU,GAAG,CAAC,CAAC;SAEf,IAAI,CAAC,aAAa,EAAE;aAClB,UAAU,GAAG,CAAC,CAAC;aACf,SAAS,GAAG,CAAC,CAAC;aACd,MAAM,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;aACd,OAAO,GAAG,CAAC,CAAC;aACZ,aAAa,GAAG,CAAC,CAAC;aAClB,iBAAiB,GAAG,CAAC,CAAC;UACvB;cAAM;aACL,SAAS,GAAG,aAAa,GAAG,CAAC,CAAC;aAC9B,iBAAiB,GAAG,OAAO,CAAC;aAC5B,IAAI,iBAAiB,KAAK,CAAC,EAAE;iBAC3B,OAAO,cAAc,CAAC,YAAY,GAAG,iBAAiB,GAAG,CAAC,CAAC,KAAK,GAAG,EAAE;qBACnE,iBAAiB,GAAG,iBAAiB,GAAG,CAAC,CAAC;kBAC3C;cACF;UACF;;;;;SAOD,IAAI,QAAQ,IAAI,aAAa,IAAI,aAAa,GAAG,QAAQ,GAAG,CAAC,IAAI,EAAE,EAAE;aACnE,QAAQ,GAAG,YAAY,CAAC;UACzB;cAAM;aACL,QAAQ,GAAG,QAAQ,GAAG,aAAa,CAAC;UACrC;;SAGD,OAAO,QAAQ,GAAG,YAAY,EAAE;;aAE9B,SAAS,GAAG,SAAS,GAAG,CAAC,CAAC;aAE1B,IAAI,SAAS,GAAG,UAAU,GAAG,UAAU,EAAE;;iBAEvC,IAAM,YAAY,GAAG,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;iBACrC,IAAI,YAAY,CAAC,KAAK,CAAC,MAAM,CAAC,EAAE;qBAC9B,QAAQ,GAAG,YAAY,CAAC;qBACxB,MAAM;kBACP;iBAED,UAAU,CAAC,cAAc,EAAE,UAAU,CAAC,CAAC;cACxC;aACD,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;UACzB;SAED,OAAO,QAAQ,GAAG,YAAY,IAAI,aAAa,GAAG,OAAO,EAAE;;aAEzD,IAAI,SAAS,KAAK,CAAC,IAAI,iBAAiB,GAAG,aAAa,EAAE;iBACxD,QAAQ,GAAG,YAAY,CAAC;iBACxB,iBAAiB,GAAG,CAAC,CAAC;iBACtB,MAAM;cACP;aAED,IAAI,aAAa,GAAG,OAAO,EAAE;;iBAE3B,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC;cACvB;kBAAM;;iBAEL,SAAS,GAAG,SAAS,GAAG,CAAC,CAAC;cAC3B;aAED,IAAI,QAAQ,GAAG,YAAY,EAAE;iBAC3B,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;cACzB;kBAAM;;iBAEL,IAAM,YAAY,GAAG,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;iBACrC,IAAI,YAAY,CAAC,KAAK,CAAC,MAAM,CAAC,EAAE;qBAC9B,QAAQ,GAAG,YAAY,CAAC;qBACxB,MAAM;kBACP;iBACD,UAAU,CAAC,cAAc,EAAE,UAAU,CAAC,CAAC;cACxC;UACF;;;SAID,IAAI,SAAS,GAAG,UAAU,GAAG,CAAC,GAAG,iBAAiB,EAAE;aAClD,IAAI,WAAW,GAAG,WAAW,CAAC;;;;aAK9B,IAAI,QAAQ,EAAE;iBACZ,YAAY,GAAG,YAAY,GAAG,CAAC,CAAC;iBAChC,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;cAC/B;;aAED,IAAI,UAAU,EAAE;iBACd,YAAY,GAAG,YAAY,GAAG,CAAC,CAAC;iBAChC,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;cAC/B;aAED,IAAM,UAAU,GAAG,QAAQ,CAAC,cAAc,CAAC,YAAY,GAAG,SAAS,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;aAC9E,IAAI,QAAQ,GAAG,CAAC,CAAC;aAEjB,IAAI,UAAU,IAAI,CAAC,EAAE;iBACnB,QAAQ,GAAG,CAAC,CAAC;iBACb,IAAI,UAAU,KAAK,CAAC,EAAE;qBACpB,QAAQ,GAAG,MAAM,CAAC,SAAS,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;qBAC/C,KAAK,CAAC,GAAG,YAAY,GAAG,SAAS,GAAG,CAAC,EAAE,CAAC,GAAG,WAAW,EAAE,CAAC,EAAE,EAAE;yBAC3D,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE;6BACnC,QAAQ,GAAG,CAAC,CAAC;6BACb,MAAM;0BACP;sBACF;kBACF;cACF;aAED,IAAI,QAAQ,EAAE;iBACZ,IAAI,IAAI,GAAG,SAAS,CAAC;iBAErB,OAAO,IAAI,IAAI,CAAC,EAAE,IAAI,EAAE,EAAE;qBACxB,IAAI,EAAE,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,EAAE;yBACtB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;yBAGjB,IAAI,IAAI,KAAK,CAAC,EAAE;6BACd,IAAI,QAAQ,GAAG,YAAY,EAAE;iCAC3B,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;iCACxB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;8BAClB;kCAAM;iCACL,OAAO,IAAI,UAAU,CACnBA,aAAM,CAAC,IAAI,CAAC,UAAU,GAAG,mBAAmB,GAAG,mBAAmB,CAAC,CACpE,CAAC;8BACH;0BACF;sBACF;kBACF;cACF;UACF;;;SAID,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;;SAErC,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;;SAGpC,IAAI,iBAAiB,KAAK,CAAC,EAAE;aAC3B,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;aACrC,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;UACrC;cAAM,IAAI,SAAS,GAAG,UAAU,GAAG,EAAE,EAAE;aACtC,IAAI,IAAI,GAAG,UAAU,CAAC;aACtB,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;aACjD,eAAe,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;aAEjC,OAAO,IAAI,IAAI,SAAS,EAAE,IAAI,EAAE,EAAE;iBAChC,cAAc,GAAG,cAAc,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;iBAC9D,cAAc,GAAG,cAAc,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;cACpE;UACF;cAAM;aACL,IAAI,IAAI,GAAG,UAAU,CAAC;aACtB,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;aAElD,OAAO,IAAI,IAAI,SAAS,GAAG,EAAE,EAAE,IAAI,EAAE,EAAE;iBACrC,eAAe,GAAG,eAAe,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;iBAChE,eAAe,GAAG,eAAe,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;cACtE;aAED,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;aAEjD,OAAO,IAAI,IAAI,SAAS,EAAE,IAAI,EAAE,EAAE;iBAChC,cAAc,GAAG,cAAc,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;iBAC9D,cAAc,GAAG,cAAc,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;cACpE;UACF;SAED,IAAM,WAAW,GAAG,YAAY,CAAC,eAAe,EAAE,WAAI,CAAC,UAAU,CAAC,oBAAoB,CAAC,CAAC,CAAC;SACzF,WAAW,CAAC,GAAG,GAAG,WAAW,CAAC,GAAG,CAAC,GAAG,CAAC,cAAc,CAAC,CAAC;SAEtD,IAAI,QAAQ,CAAC,WAAW,CAAC,GAAG,EAAE,cAAc,CAAC,EAAE;aAC7C,WAAW,CAAC,IAAI,GAAG,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC;UAC7D;;SAGD,cAAc,GAAG,QAAQ,GAAG,aAAa,CAAC;SAC1C,IAAM,GAAG,GAAG,EAAE,GAAG,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,IAAI,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,CAAC;;SAGlE,IACE,WAAW,CAAC,IAAI,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,EAC1F;;aAEA,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,GAAG,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAAC;aAC3D,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CACpB,WAAI,CAAC,UAAU,CAAC,cAAc,CAAC,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAC3E,CAAC;aACF,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,cAAc,CAAC,CAAC,CAAC,CAAC;UAC/E;cAAM;aACL,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,cAAc,GAAG,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAAC;aAC/E,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,eAAe,CAAC,CAAC,CAAC,CAAC;UAChF;SAED,GAAG,CAAC,GAAG,GAAG,WAAW,CAAC,GAAG,CAAC;;SAG1B,IAAI,UAAU,EAAE;aACd,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,qBAAqB,CAAC,CAAC,CAAC;UAChE;;SAGD,IAAMvX,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;SAChC,KAAK,GAAG,CAAC,CAAC;;;SAIVvX,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,GAAG,CAAC,GAAG,GAAG,IAAI,CAAC;SACrCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;SAC5CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;SAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;;SAE7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,GAAG,CAAC,IAAI,GAAG,IAAI,CAAC;SACtCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;SAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;SAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;;SAI9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC;SACtCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;SAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;SAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;;SAE9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC;SACvCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;SAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;SAC/CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;SAG/C,OAAO,IAAI,UAAU,CAACA,QAAM,CAAC,CAAC;MAC/B;;KAGD,6BAAQ,GAAR;;;;SAKE,IAAI,eAAe,CAAC;;SAEpB,IAAI,kBAAkB,GAAG,CAAC,CAAC;;SAE3B,IAAM,WAAW,GAAG,IAAI,KAAK,CAAS,EAAE,CAAC,CAAC;SAC1C,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,WAAW,CAAC,MAAM,EAAE,CAAC,EAAE;aAAE,WAAW,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;;SAEhE,IAAI,KAAK,GAAG,CAAC,CAAC;;SAGd,IAAI,OAAO,GAAG,KAAK,CAAC;;SAGpB,IAAI,eAAe,CAAC;;SAEpB,IAAI,cAAc,GAAgD,EAAE,KAAK,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,EAAE,CAAC;;SAE1F,IAAI,CAAC,EAAE,CAAC,CAAC;;SAGT,IAAM,MAAM,GAAa,EAAE,CAAC;;SAG5B,KAAK,GAAG,CAAC,CAAC;;SAGV,IAAM,MAAM,GAAG,IAAI,CAAC,KAAK,CAAC;;;SAI1B,IAAM,GAAG,GACP,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;SAE/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;;SAI/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;SAE/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;SAG/F,KAAK,GAAG,CAAC,CAAC;;SAGV,IAAM,GAAG,GAAG;aACV,GAAG,EAAE,IAAI,WAAI,CAAC,GAAG,EAAE,IAAI,CAAC;aACxB,IAAI,EAAE,IAAI,WAAI,CAAC,IAAI,EAAE,IAAI,CAAC;UAC3B,CAAC;SAEF,IAAI,GAAG,CAAC,IAAI,CAAC,QAAQ,CAAC,WAAI,CAAC,IAAI,CAAC,EAAE;aAChC,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;UAClB;;;SAID,IAAM,WAAW,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,gBAAgB,CAAC;SAEpD,IAAI,WAAW,IAAI,CAAC,KAAK,CAAC,EAAE;;aAE1B,IAAI,WAAW,KAAK,oBAAoB,EAAE;iBACxC,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,GAAG,UAAU,CAAC;cACrC;kBAAM,IAAI,WAAW,KAAK,eAAe,EAAE;iBAC1C,OAAO,KAAK,CAAC;cACd;kBAAM;iBACL,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,aAAa,CAAC;iBAC/C,eAAe,GAAG,IAAI,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC,CAAC;cAChD;UACF;cAAM;aACL,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;aACtC,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,aAAa,CAAC;UAChD;;SAGD,IAAM,QAAQ,GAAG,eAAe,GAAG,aAAa,CAAC;;;;;SAOjD,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,IAAI,GAAG,MAAM,KAAK,CAAC,eAAe,GAAG,GAAG,KAAK,EAAE,CAAC,CAAC;SAC5E,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC;SAC/B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC;SAC/B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;SAE9B,IACE,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;aAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;aAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;aAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC,EAC7B;aACA,OAAO,GAAG,IAAI,CAAC;UAChB;cAAM;aACL,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;iBACvB,IAAI,YAAY,GAAG,CAAC,CAAC;;iBAErB,IAAM,MAAM,GAAG,UAAU,CAAC,cAAc,CAAC,CAAC;iBAC1C,cAAc,GAAG,MAAM,CAAC,QAAQ,CAAC;iBACjC,YAAY,GAAG,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;;;iBAI9B,IAAI,CAAC,YAAY;qBAAE,SAAS;iBAE5B,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;;qBAEvB,WAAW,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,YAAY,GAAG,EAAE,CAAC;;qBAE3C,YAAY,GAAG,IAAI,CAAC,KAAK,CAAC,YAAY,GAAG,EAAE,CAAC,CAAC;kBAC9C;cACF;UACF;;;;SAMD,IAAI,OAAO,EAAE;aACX,kBAAkB,GAAG,CAAC,CAAC;aACvB,WAAW,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;UACxB;cAAM;aACL,kBAAkB,GAAG,EAAE,CAAC;aACxB,OAAO,CAAC,WAAW,CAAC,KAAK,CAAC,EAAE;iBAC1B,kBAAkB,GAAG,kBAAkB,GAAG,CAAC,CAAC;iBAC5C,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;cACnB;UACF;;SAGD,IAAM,mBAAmB,GAAG,kBAAkB,GAAG,CAAC,GAAG,QAAQ,CAAC;;;;;;;;SAS9D,IAAI,mBAAmB,IAAI,EAAE,IAAI,mBAAmB,IAAI,CAAC,CAAC,IAAI,QAAQ,GAAG,CAAC,EAAE;;;;;aAM1E,IAAI,kBAAkB,GAAG,EAAE,EAAE;iBAC3B,MAAM,CAAC,IAAI,CAAC,KAAG,CAAG,CAAC,CAAC;iBACpB,IAAI,QAAQ,GAAG,CAAC;qBAAE,MAAM,CAAC,IAAI,CAAC,IAAI,GAAG,QAAQ,CAAC,CAAC;sBAC1C,IAAI,QAAQ,GAAG,CAAC;qBAAE,MAAM,CAAC,IAAI,CAAC,GAAG,GAAG,QAAQ,CAAC,CAAC;iBACnD,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;cACxB;aAED,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;aACvC,kBAAkB,GAAG,kBAAkB,GAAG,CAAC,CAAC;aAE5C,IAAI,kBAAkB,EAAE;iBACtB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;cAClB;aAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,EAAE,CAAC,EAAE,EAAE;iBAC3C,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;cACxC;;aAGD,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;aACjB,IAAI,mBAAmB,GAAG,CAAC,EAAE;iBAC3B,MAAM,CAAC,IAAI,CAAC,GAAG,GAAG,mBAAmB,CAAC,CAAC;cACxC;kBAAM;iBACL,MAAM,CAAC,IAAI,CAAC,KAAG,mBAAqB,CAAC,CAAC;cACvC;UACF;cAAM;;aAEL,IAAI,QAAQ,IAAI,CAAC,EAAE;iBACjB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,EAAE,CAAC,EAAE,EAAE;qBAC3C,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;kBACxC;cACF;kBAAM;iBACL,IAAI,cAAc,GAAG,kBAAkB,GAAG,QAAQ,CAAC;;iBAGnD,IAAI,cAAc,GAAG,CAAC,EAAE;qBACtB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,cAAc,EAAE,CAAC,EAAE,EAAE;yBACvC,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;sBACxC;kBACF;sBAAM;qBACL,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;kBAClB;iBAED,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;iBAEjB,OAAO,cAAc,EAAE,GAAG,CAAC,EAAE;qBAC3B,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;kBAClB;iBAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,GAAG,IAAI,CAAC,GAAG,CAAC,cAAc,GAAG,CAAC,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,EAAE;qBAC7E,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;kBACxC;cACF;UACF;SAED,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;MACxB;KAED,2BAAM,GAAN;SACE,OAAO,EAAE,cAAc,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;MAC5C;;KAGD,mCAAc,GAAd;SACE,OAAO,EAAE,cAAc,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;MAC5C;;KAGM,2BAAgB,GAAvB,UAAwB,GAAuB;SAC7C,OAAO,UAAU,CAAC,UAAU,CAAC,GAAG,CAAC,cAAc,CAAC,CAAC;MAClD;;KAGD,qBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,4BAAO,GAAP;SACE,OAAO,sBAAmB,IAAI,CAAC,QAAQ,EAAE,QAAI,CAAC;MAC/C;KACH,iBAAC;CAAD,CAAC,IAAA;CAnoBY,gCAAU;CAqoBvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,YAAY,EAAE,CAAC,CAAC;;;;;;;;;;CClyBlF;;;;CAIA;;;;;;KASE,gBAAY,KAAa;SACvB,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;aAAE,OAAO,IAAI,MAAM,CAAC,KAAK,CAAC,CAAC;SAExD,IAAK,KAAiB,YAAY,MAAM,EAAE;aACxC,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;UACzB;SAED,IAAI,CAAC,KAAK,GAAG,CAAC,KAAK,CAAC;MACrB;;;;;;KAOD,wBAAO,GAAP;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,uBAAM,GAAN;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,+BAAc,GAAd,UAAe,OAAsB;SACnC,IAAI,OAAO,KAAK,OAAO,CAAC,MAAM,KAAK,OAAO,CAAC,OAAO,IAAI,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE;aAC5E,OAAO,IAAI,CAAC,KAAK,CAAC;UACnB;;;SAID,IAAI,MAAM,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,CAAC,EAAE;aACxC,OAAO,EAAE,aAAa,EAAE,MAAI,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAG,EAAE,CAAC;UACvD;SAED,IAAI,aAAqB,CAAC;SAC1B,IAAI,MAAM,CAAC,SAAS,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE;aAChC,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;aACtC,IAAI,aAAa,CAAC,MAAM,IAAI,EAAE,EAAE;iBAC9B,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,aAAa,CAAC,EAAE,CAAC,CAAC,WAAW,EAAE,CAAC;cAC5D;UACF;cAAM;aACL,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,QAAQ,EAAE,CAAC;UACvC;SAED,OAAO,EAAE,aAAa,eAAA,EAAE,CAAC;MAC1B;;KAGM,uBAAgB,GAAvB,UAAwB,GAAmB,EAAE,OAAsB;SACjE,IAAM,WAAW,GAAG,UAAU,CAAC,GAAG,CAAC,aAAa,CAAC,CAAC;SAClD,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,WAAW,GAAG,IAAI,MAAM,CAAC,WAAW,CAAC,CAAC;MAC3E;;KAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,wBAAO,GAAP;SACE,IAAM,KAAK,GAAG,IAAI,CAAC,cAAc,EAAoB,CAAC;SACtD,OAAO,gBAAc,KAAK,CAAC,aAAa,MAAG,CAAC;MAC7C;KACH,aAAC;CAAD,CAAC,IAAA;CAzEY,wBAAM;CA2EnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;CC/E1E;;;;CAIA;;;;;;KASE,eAAY,KAAsB;SAChC,IAAI,EAAE,IAAI,YAAY,KAAK,CAAC;aAAE,OAAO,IAAI,KAAK,CAAC,KAAK,CAAC,CAAC;SAEtD,IAAK,KAAiB,YAAY,MAAM,EAAE;aACxC,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;UACzB;SAED,IAAI,CAAC,KAAK,GAAG,CAAC,KAAK,CAAC;MACrB;;;;;;KAOD,uBAAO,GAAP;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,sBAAM,GAAN;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,8BAAc,GAAd,UAAe,OAAsB;SACnC,IAAI,OAAO,KAAK,OAAO,CAAC,OAAO,IAAI,OAAO,CAAC,MAAM,CAAC;aAAE,OAAO,IAAI,CAAC,KAAK,CAAC;SACtE,OAAO,EAAE,UAAU,EAAE,IAAI,CAAC,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;MAC9C;;KAGM,sBAAgB,GAAvB,UAAwB,GAAkB,EAAE,OAAsB;SAChE,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,QAAQ,CAAC,GAAG,CAAC,UAAU,EAAE,EAAE,CAAC,GAAG,IAAI,KAAK,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC;MAC9F;;KAGD,gBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,uBAAO,GAAP;SACE,OAAO,eAAa,IAAI,CAAC,OAAO,EAAE,MAAG,CAAC;MACvC;KACH,YAAC;CAAD,CAAC,IAAA;CApDY,sBAAK;CAsDlB,MAAM,CAAC,cAAc,CAAC,KAAK,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,OAAO,EAAE,CAAC,CAAC;;;;;;;;;;CC5DxE;;;;CAIA;KAGE;SACE,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;aAAE,OAAO,IAAI,MAAM,EAAE,CAAC;MACpD;;KAGD,+BAAc,GAAd;SACE,OAAO,EAAE,OAAO,EAAE,CAAC,EAAE,CAAC;MACvB;;KAGM,uBAAgB,GAAvB;SACE,OAAO,IAAI,MAAM,EAAE,CAAC;MACrB;;KAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,wBAAO,GAAP;SACE,OAAO,cAAc,CAAC;MACvB;KACH,aAAC;CAAD,CAAC,IAAA;CAzBY,wBAAM;CA2BnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;CC/B1E;;;;CAIA;KAGE;SACE,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;aAAE,OAAO,IAAI,MAAM,EAAE,CAAC;MACpD;;KAGD,+BAAc,GAAd;SACE,OAAO,EAAE,OAAO,EAAE,CAAC,EAAE,CAAC;MACvB;;KAGM,uBAAgB,GAAvB;SACE,OAAO,IAAI,MAAM,EAAE,CAAC;MACrB;;KAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,wBAAO,GAAP;SACE,OAAO,cAAc,CAAC;MACvB;KACH,aAAC;CAAD,CAAC,IAAA;CAzBY,wBAAM;CA2BnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;ACpC1C;AACe;AACuB;CAEtE;CACA,IAAM,iBAAiB,GAAG,IAAI,MAAM,CAAC,mBAAmB,CAAC,CAAC;CAE1D;CACA,IAAI,cAAc,GAAsB,IAAI,CAAC;CAc7C,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;CAEzB;;;;CAIA;;;;;;KAkBE,kBAAY,EAAuD;SACjE,IAAI,EAAE,IAAI,YAAY,QAAQ,CAAC;aAAE,OAAO,IAAI,QAAQ,CAAC,EAAE,CAAC,CAAC;;SAGzD,IAAI,EAAE,YAAY,QAAQ,EAAE;aAC1B,IAAI,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,CAAC;aAClB,IAAI,CAAC,IAAI,GAAG,EAAE,CAAC,IAAI,CAAC;UACrB;SAED,IAAI,OAAO,EAAE,KAAK,QAAQ,IAAI,EAAE,IAAI,IAAI,IAAI,EAAE,EAAE;aAC9C,IAAI,aAAa,IAAI,EAAE,IAAI,OAAO,EAAE,CAAC,WAAW,KAAK,UAAU,EAAE;iBAC/D,IAAI,CAAC,GAAG,CAAC,GAAGuX,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,EAAE,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM;iBACL,IAAI,CAAC,GAAG,CAAC,GAAG,OAAO,EAAE,CAAC,EAAE,KAAK,QAAQ,GAAGA,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,EAAE,CAAC,EAAE,CAAC;cACpE;UACF;;SAGD,IAAI,EAAE,IAAI,IAAI,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;;aAExC,IAAI,CAAC,GAAG,CAAC,GAAG,QAAQ,CAAC,QAAQ,CAAC,OAAO,EAAE,KAAK,QAAQ,GAAG,EAAE,GAAG,SAAS,CAAC,CAAC;;aAEvE,IAAI,QAAQ,CAAC,cAAc,EAAE;iBAC3B,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;cACrC;UACF;SAED,IAAI,WAAW,CAAC,MAAM,CAAC,EAAE,CAAC,IAAI,EAAE,CAAC,UAAU,KAAK,EAAE,EAAE;aAClD,IAAI,CAAC,GAAG,CAAC,GAAGE,0BAAY,CAAC,EAAE,CAAC,CAAC;UAC9B;SAED,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;aAC1B,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,EAAE;iBACpB,IAAM,KAAK,GAAGF,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;iBAC9B,IAAI,KAAK,CAAC,UAAU,KAAK,EAAE,EAAE;qBAC3B,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;kBACnB;cACF;kBAAM,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE;iBACzD,IAAI,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,IAAI,CAAC,EAAE,EAAE,KAAK,CAAC,CAAC;cACpC;kBAAM;iBACL,MAAM,IAAI,SAAS,CACjB,4FAA4F,CAC7F,CAAC;cACH;UACF;SAED,IAAI,QAAQ,CAAC,cAAc,EAAE;aAC3B,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;UACrC;MACF;KAMD,sBAAI,wBAAE;;;;;cAAN;aACE,OAAO,IAAI,CAAC,GAAG,CAAC,CAAC;UAClB;cAED,UAAO,KAAa;aAClB,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;aAClB,IAAI,QAAQ,CAAC,cAAc,EAAE;iBAC3B,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;cACnC;UACF;;;QAPA;KAaD,sBAAI,oCAAc;;;;;cAAlB;aACE,OAAO,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,CAAC,CAAC,CAAC;UAC/B;cAED,UAAmB,KAAa;;aAE9B,IAAI,CAAC,EAAE,CAAC,aAAa,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC;UACjC;;;QALA;;KAQD,8BAAW,GAAX;SACE,IAAI,QAAQ,CAAC,cAAc,IAAI,IAAI,CAAC,IAAI,EAAE;aACxC,OAAO,IAAI,CAAC,IAAI,CAAC;UAClB;SAED,IAAM,SAAS,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;SAE1C,IAAI,QAAQ,CAAC,cAAc,IAAI,CAAC,IAAI,CAAC,IAAI,EAAE;aACzC,IAAI,CAAC,IAAI,GAAG,SAAS,CAAC;UACvB;SAED,OAAO,SAAS,CAAC;MAClB;;;;;;;KAQM,eAAM,GAAb;SACE,QAAQ,QAAQ,CAAC,KAAK,GAAG,CAAC,QAAQ,CAAC,KAAK,GAAG,CAAC,IAAI,QAAQ,EAAE;MAC3D;;;;;;KAOM,iBAAQ,GAAf,UAAgB,IAAa;SAC3B,IAAI,QAAQ,KAAK,OAAO,IAAI,EAAE;aAC5B,IAAI,GAAG,CAAC,EAAE,IAAI,CAAC,GAAG,EAAE,GAAG,IAAI,CAAC,CAAC;UAC9B;SAED,IAAM,GAAG,GAAG,QAAQ,CAAC,MAAM,EAAE,CAAC;SAC9B,IAAMvX,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;;SAGhCvX,QAAM,CAAC,aAAa,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;;SAG9B,IAAI,cAAc,KAAK,IAAI,EAAE;aAC3B,cAAc,GAAGwX,iBAAW,CAAC,CAAC,CAAC,CAAC;UACjC;;SAGDxX,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;SAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;SAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;SAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;SAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;;SAG9BA,QAAM,CAAC,EAAE,CAAC,GAAG,GAAG,GAAG,IAAI,CAAC;SACxBA,QAAM,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;SAC/BA,QAAM,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;SAE/B,OAAOA,QAAM,CAAC;MACf;;;;;;;KAQD,2BAAQ,GAAR,UAAS,MAAe;;SAEtB,IAAI,MAAM;aAAE,OAAO,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC;SAC5C,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;MAC3B;;;;;KAMD,yBAAM,GAAN;SACE,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;MAC3B;;;;;;KAOD,yBAAM,GAAN,UAAO,OAAyC;SAC9C,IAAI,OAAO,KAAK,SAAS,IAAI,OAAO,KAAK,IAAI,EAAE;aAC7C,OAAO,KAAK,CAAC;UACd;SAED,IAAI,OAAO,YAAY,QAAQ,EAAE;aAC/B,OAAO,IAAI,CAAC,QAAQ,EAAE,KAAK,OAAO,CAAC,QAAQ,EAAE,CAAC;UAC/C;SAED,IACE,OAAO,OAAO,KAAK,QAAQ;aAC3B,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC;aACzB,OAAO,CAAC,MAAM,KAAK,EAAE;aACrBwX,kBAAY,CAAC,IAAI,CAAC,EAAE,CAAC,EACrB;aACA,OAAO,OAAO,KAAKD,aAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,IAAI,CAAC,EAAE,EAAE,QAAQ,CAAC,CAAC;UACtE;SAED,IAAI,OAAO,OAAO,KAAK,QAAQ,IAAI,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC,IAAI,OAAO,CAAC,MAAM,KAAK,EAAE,EAAE;aACrF,OAAO,OAAO,CAAC,WAAW,EAAE,KAAK,IAAI,CAAC,WAAW,EAAE,CAAC;UACrD;SAED,IAAI,OAAO,OAAO,KAAK,QAAQ,IAAI,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC,IAAI,OAAO,CAAC,MAAM,KAAK,EAAE,EAAE;aACrF,OAAOA,aAAM,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;UAC7C;SAED,IACE,OAAO,OAAO,KAAK,QAAQ;aAC3B,aAAa,IAAI,OAAO;aACxB,OAAO,OAAO,CAAC,WAAW,KAAK,UAAU,EACzC;aACA,OAAO,OAAO,CAAC,WAAW,EAAE,KAAK,IAAI,CAAC,WAAW,EAAE,CAAC;UACrD;SAED,OAAO,KAAK,CAAC;MACd;;KAGD,+BAAY,GAAZ;SACE,IAAM,SAAS,GAAG,IAAI,IAAI,EAAE,CAAC;SAC7B,IAAM,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,YAAY,CAAC,CAAC,CAAC,CAAC;SACrC,SAAS,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,CAAC;SAC3C,OAAO,SAAS,CAAC;MAClB;;KAGM,iBAAQ,GAAf;SACE,OAAO,IAAI,QAAQ,EAAE,CAAC;MACvB;;;;;;KAOM,uBAAc,GAArB,UAAsB,IAAY;SAChC,IAAMvX,QAAM,GAAGuX,aAAM,CAAC,IAAI,CAAC,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;;SAEjEvX,QAAM,CAAC,aAAa,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;;SAE9B,OAAO,IAAI,QAAQ,CAACA,QAAM,CAAC,CAAC;MAC7B;;;;;;KAOM,4BAAmB,GAA1B,UAA2B,SAAiB;;SAE1C,IAAI,OAAO,SAAS,KAAK,WAAW,KAAK,SAAS,IAAI,IAAI,IAAI,SAAS,CAAC,MAAM,KAAK,EAAE,CAAC,EAAE;aACtF,MAAM,IAAI,SAAS,CACjB,yFAAyF,CAC1F,CAAC;UACH;SAED,OAAO,IAAI,QAAQ,CAACuX,aAAM,CAAC,IAAI,CAAC,SAAS,EAAE,KAAK,CAAC,CAAC,CAAC;MACpD;;;;;;KAOM,gBAAO,GAAd,UAAe,EAA0D;SACvE,IAAI,EAAE,IAAI,IAAI;aAAE,OAAO,KAAK,CAAC;SAE7B,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;aAC1B,OAAO,IAAI,CAAC;UACb;SAED,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;aAC1B,OAAO,EAAE,CAAC,MAAM,KAAK,EAAE,KAAK,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC;UAC7E;SAED,IAAI,EAAE,YAAY,QAAQ,EAAE;aAC1B,OAAO,IAAI,CAAC;UACb;SAED,IAAIC,kBAAY,CAAC,EAAE,CAAC,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,EAAE;aACxC,OAAO,IAAI,CAAC;UACb;;SAGD,IAAI,OAAO,EAAE,KAAK,QAAQ,IAAI,aAAa,IAAI,EAAE,IAAI,OAAO,EAAE,CAAC,WAAW,KAAK,UAAU,EAAE;aACzF,IAAI,OAAO,EAAE,CAAC,EAAE,KAAK,QAAQ,EAAE;iBAC7B,OAAO,EAAE,CAAC,EAAE,CAAC,MAAM,KAAK,EAAE,CAAC;cAC5B;aACD,OAAO,EAAE,CAAC,WAAW,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC;UACxF;SAED,OAAO,KAAK,CAAC;MACd;;KAGD,iCAAc,GAAd;SACE,IAAI,IAAI,CAAC,WAAW;aAAE,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,WAAW,EAAE,EAAE,CAAC;SAC1D,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,EAAE,CAAC;MACvC;;KAGM,yBAAgB,GAAvB,UAAwB,GAAqB;SAC3C,OAAO,IAAI,QAAQ,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;MAC/B;;;;;;;KAQD,mBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,0BAAO,GAAP;SACE,OAAO,oBAAiB,IAAI,CAAC,WAAW,EAAE,QAAI,CAAC;MAChD;;KA5TM,cAAK,GAAG,CAAC,EAAE,IAAI,CAAC,MAAM,EAAE,GAAG,QAAQ,CAAC,CAAC;KA6T9C,eAAC;EAjUD,IAiUC;CAjUY,4BAAQ;CAmUrB;CACA,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,UAAU,EAAE;KACpD,KAAK,EAAEA,eAAS,CACd,UAAC,IAAY,IAAK,OAAA,QAAQ,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAA,EACzC,yDAAyD,CAC1D;EACF,CAAC,CAAC;CAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,QAAQ,EAAE;KAClD,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;EAC/F,CAAC,CAAC;CAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,SAAS,EAAE;KACnD,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;EAC/F,CAAC,CAAC;CAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,EAAE,SAAS,EAAE;KACzC,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;EAC/F,CAAC,CAAC;CAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,UAAU,EAAE,CAAC,CAAC;;;;;;;;;;CCjX9E,SAAS,WAAW,CAAC,GAAW;KAC9B,OAAO,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;CACvC,CAAC;CAgBD;;;;CAIA;;;;;KASE,oBAAY,OAAe,EAAE,OAAgB;SAC3C,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;aAAE,OAAO,IAAI,UAAU,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;SAE3E,IAAI,CAAC,OAAO,GAAG,OAAO,CAAC;SACvB,IAAI,CAAC,OAAO,GAAG,WAAW,CAAC,OAAO,aAAP,OAAO,cAAP,OAAO,GAAI,EAAE,CAAC,CAAC;;SAG1C,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,OAAO,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;aAC5C,IACE,EACE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;iBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;iBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;iBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;iBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;iBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG,CACxB,EACD;iBACA,MAAM,IAAI,KAAK,CAAC,oCAAkC,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,uBAAoB,CAAC,CAAC;cACxF;UACF;MACF;KAEM,uBAAY,GAAnB,UAAoB,OAAgB;SAClC,OAAO,OAAO,GAAG,OAAO,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,GAAG,EAAE,CAAC;MACzD;;KAGD,mCAAc,GAAd,UAAe,OAAsB;SACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,IAAI,OAAO,CAAC,MAAM,EAAE;aAClB,OAAO,EAAE,MAAM,EAAE,IAAI,CAAC,OAAO,EAAE,QAAQ,EAAE,IAAI,CAAC,OAAO,EAAE,CAAC;UACzD;SACD,OAAO,EAAE,kBAAkB,EAAE,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,EAAE,CAAC;MACjF;;KAGM,2BAAgB,GAAvB,UAAwB,GAAkD;SACxE,IAAI,QAAQ,IAAI,GAAG,EAAE;aACnB,IAAI,OAAO,GAAG,CAAC,MAAM,KAAK,QAAQ,EAAE;;iBAElC,IAAI,GAAG,CAAC,MAAM,CAAC,SAAS,KAAK,YAAY,EAAE;qBACzC,OAAQ,GAA6B,CAAC;kBACvC;cACF;kBAAM;iBACL,OAAO,IAAI,UAAU,CAAC,GAAG,CAAC,MAAM,EAAE,UAAU,CAAC,YAAY,CAAC,GAAG,CAAC,QAAQ,CAAC,CAAC,CAAC;cAC1E;UACF;SACD,IAAI,oBAAoB,IAAI,GAAG,EAAE;aAC/B,OAAO,IAAI,UAAU,CACnB,GAAG,CAAC,kBAAkB,CAAC,OAAO,EAC9B,UAAU,CAAC,YAAY,CAAC,GAAG,CAAC,kBAAkB,CAAC,OAAO,CAAC,CACxD,CAAC;UACH;SACD,MAAM,IAAI,SAAS,CAAC,8CAA4C,IAAI,CAAC,SAAS,CAAC,GAAG,CAAG,CAAC,CAAC;MACxF;KACH,iBAAC;CAAD,CAAC,IAAA;CAjEY,gCAAU;CAmEvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,YAAY,EAAE,CAAC,CAAC;;;;;;;;;;CCtFlF;;;;CAIA;;;;KAOE,oBAAY,KAAa;SACvB,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;aAAE,OAAO,IAAI,UAAU,CAAC,KAAK,CAAC,CAAC;SAEhE,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;MACpB;;KAGD,4BAAO,GAAP;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,6BAAQ,GAAR;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,4BAAO,GAAP;SACE,OAAO,sBAAmB,IAAI,CAAC,KAAK,QAAI,CAAC;MAC1C;;KAGD,2BAAM,GAAN;SACE,OAAO,IAAI,CAAC,KAAK,CAAC;MACnB;;KAGD,mCAAc,GAAd;SACE,OAAO,EAAE,OAAO,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;MAChC;;KAGM,2BAAgB,GAAvB,UAAwB,GAAuB;SAC7C,OAAO,IAAI,UAAU,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;MACpC;;KAGD,qBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KACH,iBAAC;CAAD,CAAC,IAAA;CA/CY,gCAAU;CAiDvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;CC1D9E;CACA;AACA;CACA;CACA;AACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;CACA;CAEA,IAAIK,cAAa,GAAG,uBAASlX,CAAT,EAAYoD,CAAZ,EAAe;CAC/B8T,EAAAA,cAAa,GAAGxV,MAAM,CAACC,cAAP,IACX;CAAEwV,IAAAA,SAAS,EAAE;CAAb,eAA6B5Z,KAA7B,IAAsC,UAAUyC,CAAV,EAAaoD,CAAb,EAAgB;CAAEpD,IAAAA,CAAC,CAACmX,SAAF,GAAc/T,CAAd;CAAkB,GAD/D,IAEZ,UAAUpD,CAAV,EAAaoD,CAAb,EAAgB;CAAE,SAAK,IAAIgU,CAAT,IAAchU,CAAd;CAAiB,UAAIA,CAAC,CAAC2R,cAAF,CAAiBqC,CAAjB,CAAJ,EAAyBpX,CAAC,CAACoX,CAAD,CAAD,GAAOhU,CAAC,CAACgU,CAAD,CAAR;CAA1C;CAAwD,GAF9E;;CAGA,SAAOF,cAAa,CAAClX,CAAD,EAAIoD,CAAJ,CAApB;CACH,CALD;;CAOO,SAASiU,SAAT,CAAmBrX,CAAnB,EAAsBoD,CAAtB,EAAyB;CAC5B8T,EAAAA,cAAa,CAAClX,CAAD,EAAIoD,CAAJ,CAAb;;CACA,WAASkU,EAAT,GAAc;CAAE,SAAKjI,WAAL,GAAmBrP,CAAnB;CAAuB;;CACvCA,EAAAA,CAAC,CAAC4B,SAAF,GAAcwB,CAAC,KAAK,IAAN,GAAa1B,MAAM,CAAC0O,MAAP,CAAchN,CAAd,CAAb,IAAiCkU,EAAE,CAAC1V,SAAH,GAAewB,CAAC,CAACxB,SAAjB,EAA4B,IAAI0V,EAAJ,EAA7D,CAAd;CACH;;CAEM,IAAIC,OAAQ,GAAG,oBAAW;CAC7BA,EAAAA,OAAQ,GAAG7V,MAAM,CAAC8V,MAAP,IAAiB,SAASD,QAAT,CAAkBE,CAAlB,EAAqB;CAC7C,SAAK,IAAIxX,CAAJ,EAAOxC,CAAC,GAAG,CAAX,EAAc8I,CAAC,GAAGZ,SAAS,CAAChI,MAAjC,EAAyCF,CAAC,GAAG8I,CAA7C,EAAgD9I,CAAC,EAAjD,EAAqD;CACjDwC,MAAAA,CAAC,GAAG0F,SAAS,CAAClI,CAAD,CAAb;;CACA,WAAK,IAAI2Z,CAAT,IAAcnX,CAAd;CAAiB,YAAIyB,MAAM,CAACE,SAAP,CAAiBmT,cAAjB,CAAgCtP,IAAhC,CAAqCxF,CAArC,EAAwCmX,CAAxC,CAAJ,EAAgDK,CAAC,CAACL,CAAD,CAAD,GAAOnX,CAAC,CAACmX,CAAD,CAAR;CAAjE;CACH;;CACD,WAAOK,CAAP;CACH,GAND;;CAOA,SAAOF,OAAQ,CAAC5Q,KAAT,CAAe,IAAf,EAAqBhB,SAArB,CAAP;CACH,CATM;CAWA,SAAS+R,MAAT,CAAgBzX,CAAhB,EAAmBP,CAAnB,EAAsB;CACzB,MAAI+X,CAAC,GAAG,EAAR;;CACA,OAAK,IAAIL,CAAT,IAAcnX,CAAd;CAAiB,QAAIyB,MAAM,CAACE,SAAP,CAAiBmT,cAAjB,CAAgCtP,IAAhC,CAAqCxF,CAArC,EAAwCmX,CAAxC,KAA8C1X,CAAC,CAACzB,OAAF,CAAUmZ,CAAV,IAAe,CAAjE,EACbK,CAAC,CAACL,CAAD,CAAD,GAAOnX,CAAC,CAACmX,CAAD,CAAR;CADJ;;CAEA,MAAInX,CAAC,IAAI,IAAL,IAAa,OAAOyB,MAAM,CAACiW,qBAAd,KAAwC,UAAzD,EACI,KAAK,IAAIla,CAAC,GAAG,CAAR,EAAW2Z,CAAC,GAAG1V,MAAM,CAACiW,qBAAP,CAA6B1X,CAA7B,CAApB,EAAqDxC,CAAC,GAAG2Z,CAAC,CAACzZ,MAA3D,EAAmEF,CAAC,EAApE,EAAwE;CACpE,QAAIiC,CAAC,CAACzB,OAAF,CAAUmZ,CAAC,CAAC3Z,CAAD,CAAX,IAAkB,CAAlB,IAAuBiE,MAAM,CAACE,SAAP,CAAiBgW,oBAAjB,CAAsCnS,IAAtC,CAA2CxF,CAA3C,EAA8CmX,CAAC,CAAC3Z,CAAD,CAA/C,CAA3B,EACIga,CAAC,CAACL,CAAC,CAAC3Z,CAAD,CAAF,CAAD,GAAUwC,CAAC,CAACmX,CAAC,CAAC3Z,CAAD,CAAF,CAAX;CACP;CACL,SAAOga,CAAP;CACH;CAEM,SAASI,UAAT,CAAoBC,UAApB,EAAgC1Q,MAAhC,EAAwCoN,GAAxC,EAA6CS,IAA7C,EAAmD;CACtD,MAAI1U,CAAC,GAAGoF,SAAS,CAAChI,MAAlB;CAAA,MAA0Boa,CAAC,GAAGxX,CAAC,GAAG,CAAJ,GAAQ6G,MAAR,GAAiB6N,IAAI,KAAK,IAAT,GAAgBA,IAAI,GAAGvT,MAAM,CAACwT,wBAAP,CAAgC9N,MAAhC,EAAwCoN,GAAxC,CAAvB,GAAsES,IAArH;CAAA,MAA2HjV,CAA3H;CACA,MAAI,QAAOgY,OAAP,wDAAOA,OAAP,OAAmB,QAAnB,IAA+B,OAAOA,OAAO,CAACC,QAAf,KAA4B,UAA/D,EAA2EF,CAAC,GAAGC,OAAO,CAACC,QAAR,CAAiBH,UAAjB,EAA6B1Q,MAA7B,EAAqCoN,GAArC,EAA0CS,IAA1C,CAAJ,CAA3E,KACK,KAAK,IAAIxX,CAAC,GAAGqa,UAAU,CAACna,MAAX,GAAoB,CAAjC,EAAoCF,CAAC,IAAI,CAAzC,EAA4CA,CAAC,EAA7C;CAAiD,QAAIuC,CAAC,GAAG8X,UAAU,CAACra,CAAD,CAAlB,EAAuBsa,CAAC,GAAG,CAACxX,CAAC,GAAG,CAAJ,GAAQP,CAAC,CAAC+X,CAAD,CAAT,GAAexX,CAAC,GAAG,CAAJ,GAAQP,CAAC,CAACoH,MAAD,EAASoN,GAAT,EAAcuD,CAAd,CAAT,GAA4B/X,CAAC,CAACoH,MAAD,EAASoN,GAAT,CAA7C,KAA+DuD,CAAnE;CAAxE;CACL,SAAOxX,CAAC,GAAG,CAAJ,IAASwX,CAAT,IAAcrW,MAAM,CAACG,cAAP,CAAsBuF,MAAtB,EAA8BoN,GAA9B,EAAmCuD,CAAnC,CAAd,EAAqDA,CAA5D;CACH;CAEM,SAASG,OAAT,CAAiBC,UAAjB,EAA6BC,SAA7B,EAAwC;CAC3C,SAAO,UAAUhR,MAAV,EAAkBoN,GAAlB,EAAuB;CAAE4D,IAAAA,SAAS,CAAChR,MAAD,EAASoN,GAAT,EAAc2D,UAAd,CAAT;CAAqC,GAArE;CACH;CAEM,SAASE,UAAT,CAAoBC,WAApB,EAAiCC,aAAjC,EAAgD;CACnD,MAAI,QAAOP,OAAP,wDAAOA,OAAP,OAAmB,QAAnB,IAA+B,OAAOA,OAAO,CAACQ,QAAf,KAA4B,UAA/D,EAA2E,OAAOR,OAAO,CAACQ,QAAR,CAAiBF,WAAjB,EAA8BC,aAA9B,CAAP;CAC9E;CAEM,SAASE,SAAT,CAAmBC,OAAnB,EAA4BC,UAA5B,EAAwCC,CAAxC,EAA2CC,SAA3C,EAAsD;CACzD,WAASC,KAAT,CAAexY,KAAf,EAAsB;CAAE,WAAOA,KAAK,YAAYsY,CAAjB,GAAqBtY,KAArB,GAA6B,IAAIsY,CAAJ,CAAM,UAAUG,OAAV,EAAmB;CAAEA,MAAAA,OAAO,CAACzY,KAAD,CAAP;CAAiB,KAA5C,CAApC;CAAoF;;CAC5G,SAAO,KAAKsY,CAAC,KAAKA,CAAC,GAAGI,OAAT,CAAN,EAAyB,UAAUD,OAAV,EAAmBE,MAAnB,EAA2B;CACvD,aAASC,SAAT,CAAmB5Y,KAAnB,EAA0B;CAAE,UAAI;CAAE6Y,QAAAA,IAAI,CAACN,SAAS,CAACO,IAAV,CAAe9Y,KAAf,CAAD,CAAJ;CAA8B,OAApC,CAAqC,OAAOZ,CAAP,EAAU;CAAEuZ,QAAAA,MAAM,CAACvZ,CAAD,CAAN;CAAY;CAAE;;CAC3F,aAAS2Z,QAAT,CAAkB/Y,KAAlB,EAAyB;CAAE,UAAI;CAAE6Y,QAAAA,IAAI,CAACN,SAAS,CAAC,OAAD,CAAT,CAAmBvY,KAAnB,CAAD,CAAJ;CAAkC,OAAxC,CAAyC,OAAOZ,CAAP,EAAU;CAAEuZ,QAAAA,MAAM,CAACvZ,CAAD,CAAN;CAAY;CAAE;;CAC9F,aAASyZ,IAAT,CAAcG,MAAd,EAAsB;CAAEA,MAAAA,MAAM,CAACC,IAAP,GAAcR,OAAO,CAACO,MAAM,CAAChZ,KAAR,CAArB,GAAsCwY,KAAK,CAACQ,MAAM,CAAChZ,KAAR,CAAL,CAAoBkZ,IAApB,CAAyBN,SAAzB,EAAoCG,QAApC,CAAtC;CAAsF;;CAC9GF,IAAAA,IAAI,CAAC,CAACN,SAAS,GAAGA,SAAS,CAAClS,KAAV,CAAgB+R,OAAhB,EAAyBC,UAAU,IAAI,EAAvC,CAAb,EAAyDS,IAAzD,EAAD,CAAJ;CACH,GALM,CAAP;CAMH;CAEM,SAASK,WAAT,CAAqBf,OAArB,EAA8BgB,IAA9B,EAAoC;CACvC,MAAIvI,CAAC,GAAG;CAAEwI,IAAAA,KAAK,EAAE,CAAT;CAAYC,IAAAA,IAAI,EAAE,gBAAW;CAAE,UAAInC,CAAC,CAAC,CAAD,CAAD,GAAO,CAAX,EAAc,MAAMA,CAAC,CAAC,CAAD,CAAP;CAAY,aAAOA,CAAC,CAAC,CAAD,CAAR;CAAc,KAAvE;CAAyEoC,IAAAA,IAAI,EAAE,EAA/E;CAAmFC,IAAAA,GAAG,EAAE;CAAxF,GAAR;CAAA,MAAsGjJ,CAAtG;CAAA,MAAyG5L,CAAzG;CAAA,MAA4GwS,CAA5G;CAAA,MAA+GsC,CAA/G;CACA,SAAOA,CAAC,GAAG;CAAEX,IAAAA,IAAI,EAAEY,IAAI,CAAC,CAAD,CAAZ;CAAiB,aAASA,IAAI,CAAC,CAAD,CAA9B;CAAmC,cAAUA,IAAI,CAAC,CAAD;CAAjD,GAAJ,EAA4D,OAAOjZ,MAAP,KAAkB,UAAlB,KAAiCgZ,CAAC,CAAChZ,MAAM,CAACkZ,QAAR,CAAD,GAAqB,YAAW;CAAE,WAAO,IAAP;CAAc,GAAjF,CAA5D,EAAgJF,CAAvJ;;CACA,WAASC,IAAT,CAAczT,CAAd,EAAiB;CAAE,WAAO,UAAU2T,CAAV,EAAa;CAAE,aAAOf,IAAI,CAAC,CAAC5S,CAAD,EAAI2T,CAAJ,CAAD,CAAX;CAAsB,KAA5C;CAA+C;;CAClE,WAASf,IAAT,CAAcgB,EAAd,EAAkB;CACd,QAAItJ,CAAJ,EAAO,MAAM,IAAIrO,SAAJ,CAAc,iCAAd,CAAN;;CACP,WAAO2O,CAAP;CAAU,UAAI;CACV,YAAIN,CAAC,GAAG,CAAJ,EAAO5L,CAAC,KAAKwS,CAAC,GAAG0C,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAR,GAAYlV,CAAC,CAAC,QAAD,CAAb,GAA0BkV,EAAE,CAAC,CAAD,CAAF,GAAQlV,CAAC,CAAC,OAAD,CAAD,KAAe,CAACwS,CAAC,GAAGxS,CAAC,CAAC,QAAD,CAAN,KAAqBwS,CAAC,CAAChS,IAAF,CAAOR,CAAP,CAArB,EAAgC,CAA/C,CAAR,GAA4DA,CAAC,CAACmU,IAAjG,CAAD,IAA2G,CAAC,CAAC3B,CAAC,GAAGA,CAAC,CAAChS,IAAF,CAAOR,CAAP,EAAUkV,EAAE,CAAC,CAAD,CAAZ,CAAL,EAAuBZ,IAA9I,EAAoJ,OAAO9B,CAAP;CACpJ,YAAIxS,CAAC,GAAG,CAAJ,EAAOwS,CAAX,EAAc0C,EAAE,GAAG,CAACA,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAT,EAAY1C,CAAC,CAACnX,KAAd,CAAL;;CACd,gBAAQ6Z,EAAE,CAAC,CAAD,CAAV;CACI,eAAK,CAAL;CAAQ,eAAK,CAAL;CAAQ1C,YAAAA,CAAC,GAAG0C,EAAJ;CAAQ;;CACxB,eAAK,CAAL;CAAQhJ,YAAAA,CAAC,CAACwI,KAAF;CAAW,mBAAO;CAAErZ,cAAAA,KAAK,EAAE6Z,EAAE,CAAC,CAAD,CAAX;CAAgBZ,cAAAA,IAAI,EAAE;CAAtB,aAAP;;CACnB,eAAK,CAAL;CAAQpI,YAAAA,CAAC,CAACwI,KAAF;CAAW1U,YAAAA,CAAC,GAAGkV,EAAE,CAAC,CAAD,CAAN;CAAWA,YAAAA,EAAE,GAAG,CAAC,CAAD,CAAL;CAAU;;CACxC,eAAK,CAAL;CAAQA,YAAAA,EAAE,GAAGhJ,CAAC,CAAC2I,GAAF,CAAMpF,GAAN,EAAL;;CAAkBvD,YAAAA,CAAC,CAAC0I,IAAF,CAAOnF,GAAP;;CAAc;;CACxC;CACI,gBAAI,EAAE+C,CAAC,GAAGtG,CAAC,CAAC0I,IAAN,EAAYpC,CAAC,GAAGA,CAAC,CAAC9Z,MAAF,GAAW,CAAX,IAAgB8Z,CAAC,CAACA,CAAC,CAAC9Z,MAAF,GAAW,CAAZ,CAAnC,MAAuDwc,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,IAAeA,EAAE,CAAC,CAAD,CAAF,KAAU,CAAhF,CAAJ,EAAwF;CAAEhJ,cAAAA,CAAC,GAAG,CAAJ;CAAO;CAAW;;CAC5G,gBAAIgJ,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,KAAgB,CAAC1C,CAAD,IAAO0C,EAAE,CAAC,CAAD,CAAF,GAAQ1C,CAAC,CAAC,CAAD,CAAT,IAAgB0C,EAAE,CAAC,CAAD,CAAF,GAAQ1C,CAAC,CAAC,CAAD,CAAhD,CAAJ,EAA2D;CAAEtG,cAAAA,CAAC,CAACwI,KAAF,GAAUQ,EAAE,CAAC,CAAD,CAAZ;CAAiB;CAAQ;;CACtF,gBAAIA,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,IAAehJ,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAA9B,EAAmC;CAAEtG,cAAAA,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAAX;CAAgBA,cAAAA,CAAC,GAAG0C,EAAJ;CAAQ;CAAQ;;CACrE,gBAAI1C,CAAC,IAAItG,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAApB,EAAyB;CAAEtG,cAAAA,CAAC,CAACwI,KAAF,GAAUlC,CAAC,CAAC,CAAD,CAAX;;CAAgBtG,cAAAA,CAAC,CAAC2I,GAAF,CAAM/a,IAAN,CAAWob,EAAX;;CAAgB;CAAQ;;CACnE,gBAAI1C,CAAC,CAAC,CAAD,CAAL,EAAUtG,CAAC,CAAC2I,GAAF,CAAMpF,GAAN;;CACVvD,YAAAA,CAAC,CAAC0I,IAAF,CAAOnF,GAAP;;CAAc;CAXtB;;CAaAyF,QAAAA,EAAE,GAAGT,IAAI,CAACjU,IAAL,CAAUiT,OAAV,EAAmBvH,CAAnB,CAAL;CACH,OAjBS,CAiBR,OAAOzR,CAAP,EAAU;CAAEya,QAAAA,EAAE,GAAG,CAAC,CAAD,EAAIza,CAAJ,CAAL;CAAauF,QAAAA,CAAC,GAAG,CAAJ;CAAQ,OAjBzB,SAiBkC;CAAE4L,QAAAA,CAAC,GAAG4G,CAAC,GAAG,CAAR;CAAY;CAjB1D;;CAkBA,QAAI0C,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAZ,EAAe,MAAMA,EAAE,CAAC,CAAD,CAAR;CAAa,WAAO;CAAE7Z,MAAAA,KAAK,EAAE6Z,EAAE,CAAC,CAAD,CAAF,GAAQA,EAAE,CAAC,CAAD,CAAV,GAAgB,KAAK,CAA9B;CAAiCZ,MAAAA,IAAI,EAAE;CAAvC,KAAP;CAC/B;CACJ;CAEM,SAASa,eAAT,CAAyBrE,CAAzB,EAA4BpW,CAA5B,EAA+B0a,CAA/B,EAAkCC,EAAlC,EAAsC;CACzC,MAAIA,EAAE,KAAKrY,SAAX,EAAsBqY,EAAE,GAAGD,CAAL;CACtBtE,EAAAA,CAAC,CAACuE,EAAD,CAAD,GAAQ3a,CAAC,CAAC0a,CAAD,CAAT;CACH;CAEM,SAASE,YAAT,CAAsB5a,CAAtB,EAAyBqB,OAAzB,EAAkC;CACrC,OAAK,IAAIoW,CAAT,IAAczX,CAAd;CAAiB,QAAIyX,CAAC,KAAK,SAAN,IAAmB,CAACpW,OAAO,CAAC+T,cAAR,CAAuBqC,CAAvB,CAAxB,EAAmDpW,OAAO,CAACoW,CAAD,CAAP,GAAazX,CAAC,CAACyX,CAAD,CAAd;CAApE;CACH;CAEM,SAASoD,QAAT,CAAkBzE,CAAlB,EAAqB;CACxB,MAAI9V,CAAC,GAAG,OAAOc,MAAP,KAAkB,UAAlB,IAAgCA,MAAM,CAACkZ,QAA/C;CAAA,MAAyDta,CAAC,GAAGM,CAAC,IAAI8V,CAAC,CAAC9V,CAAD,CAAnE;CAAA,MAAwExC,CAAC,GAAG,CAA5E;CACA,MAAIkC,CAAJ,EAAO,OAAOA,CAAC,CAAC8F,IAAF,CAAOsQ,CAAP,CAAP;CACP,MAAIA,CAAC,IAAI,OAAOA,CAAC,CAACpY,MAAT,KAAoB,QAA7B,EAAuC,OAAO;CAC1Cyb,IAAAA,IAAI,EAAE,gBAAY;CACd,UAAIrD,CAAC,IAAItY,CAAC,IAAIsY,CAAC,CAACpY,MAAhB,EAAwBoY,CAAC,GAAG,KAAK,CAAT;CACxB,aAAO;CAAEzV,QAAAA,KAAK,EAAEyV,CAAC,IAAIA,CAAC,CAACtY,CAAC,EAAF,CAAf;CAAsB8b,QAAAA,IAAI,EAAE,CAACxD;CAA7B,OAAP;CACH;CAJyC,GAAP;CAMvC,QAAM,IAAIvT,SAAJ,CAAcvC,CAAC,GAAG,yBAAH,GAA+B,iCAA9C,CAAN;CACH;CAEM,SAASwa,MAAT,CAAgB1E,CAAhB,EAAmBxP,CAAnB,EAAsB;CACzB,MAAI5G,CAAC,GAAG,OAAOoB,MAAP,KAAkB,UAAlB,IAAgCgV,CAAC,CAAChV,MAAM,CAACkZ,QAAR,CAAzC;CACA,MAAI,CAACta,CAAL,EAAQ,OAAOoW,CAAP;CACR,MAAItY,CAAC,GAAGkC,CAAC,CAAC8F,IAAF,CAAOsQ,CAAP,CAAR;CAAA,MAAmBgC,CAAnB;CAAA,MAAsBvC,EAAE,GAAG,EAA3B;CAAA,MAA+B9V,CAA/B;;CACA,MAAI;CACA,WAAO,CAAC6G,CAAC,KAAK,KAAK,CAAX,IAAgBA,CAAC,KAAK,CAAvB,KAA6B,CAAC,CAACwR,CAAC,GAAGta,CAAC,CAAC2b,IAAF,EAAL,EAAeG,IAApD;CAA0D/D,MAAAA,EAAE,CAACzW,IAAH,CAAQgZ,CAAC,CAACzX,KAAV;CAA1D;CACH,GAFD,CAGA,OAAOiB,KAAP,EAAc;CAAE7B,IAAAA,CAAC,GAAG;CAAE6B,MAAAA,KAAK,EAAEA;CAAT,KAAJ;CAAuB,GAHvC,SAIQ;CACJ,QAAI;CACA,UAAIwW,CAAC,IAAI,CAACA,CAAC,CAACwB,IAAR,KAAiB5Z,CAAC,GAAGlC,CAAC,CAAC,QAAD,CAAtB,CAAJ,EAAuCkC,CAAC,CAAC8F,IAAF,CAAOhI,CAAP;CAC1C,KAFD,SAGQ;CAAE,UAAIiC,CAAJ,EAAO,MAAMA,CAAC,CAAC6B,KAAR;CAAgB;CACpC;;CACD,SAAOiU,EAAP;CACH;CAEM,SAASkF,QAAT,GAAoB;CACvB,OAAK,IAAIlF,EAAE,GAAG,EAAT,EAAa/X,CAAC,GAAG,CAAtB,EAAyBA,CAAC,GAAGkI,SAAS,CAAChI,MAAvC,EAA+CF,CAAC,EAAhD;CACI+X,IAAAA,EAAE,GAAGA,EAAE,CAACnQ,MAAH,CAAUoV,MAAM,CAAC9U,SAAS,CAAClI,CAAD,CAAV,CAAhB,CAAL;CADJ;;CAEA,SAAO+X,EAAP;CACH;CAEM,SAASmF,cAAT,GAA0B;CAC7B,OAAK,IAAI1a,CAAC,GAAG,CAAR,EAAWxC,CAAC,GAAG,CAAf,EAAkBmd,EAAE,GAAGjV,SAAS,CAAChI,MAAtC,EAA8CF,CAAC,GAAGmd,EAAlD,EAAsDnd,CAAC,EAAvD;CAA2DwC,IAAAA,CAAC,IAAI0F,SAAS,CAAClI,CAAD,CAAT,CAAaE,MAAlB;CAA3D;;CACA,OAAK,IAAIoa,CAAC,GAAGxa,KAAK,CAAC0C,CAAD,CAAb,EAAkBoa,CAAC,GAAG,CAAtB,EAAyB5c,CAAC,GAAG,CAAlC,EAAqCA,CAAC,GAAGmd,EAAzC,EAA6Cnd,CAAC,EAA9C;CACI,SAAK,IAAIsH,CAAC,GAAGY,SAAS,CAAClI,CAAD,CAAjB,EAAsB4K,CAAC,GAAG,CAA1B,EAA6BwS,EAAE,GAAG9V,CAAC,CAACpH,MAAzC,EAAiD0K,CAAC,GAAGwS,EAArD,EAAyDxS,CAAC,IAAIgS,CAAC,EAA/D;CACItC,MAAAA,CAAC,CAACsC,CAAD,CAAD,GAAOtV,CAAC,CAACsD,CAAD,CAAR;CADJ;CADJ;;CAGA,SAAO0P,CAAP;CACH;CAEM,SAAS+C,OAAT,CAAiBZ,CAAjB,EAAoB;CACvB,SAAO,gBAAgBY,OAAhB,IAA2B,KAAKZ,CAAL,GAASA,CAAT,EAAY,IAAvC,IAA+C,IAAIY,OAAJ,CAAYZ,CAAZ,CAAtD;CACH;CAEM,SAASa,gBAAT,CAA0BrC,OAA1B,EAAmCC,UAAnC,EAA+CE,SAA/C,EAA0D;CAC7D,MAAI,CAAC9X,MAAM,CAACia,aAAZ,EAA2B,MAAM,IAAIxY,SAAJ,CAAc,sCAAd,CAAN;CAC3B,MAAIuX,CAAC,GAAGlB,SAAS,CAAClS,KAAV,CAAgB+R,OAAhB,EAAyBC,UAAU,IAAI,EAAvC,CAAR;CAAA,MAAoDlb,CAApD;CAAA,MAAuDwd,CAAC,GAAG,EAA3D;CACA,SAAOxd,CAAC,GAAG,EAAJ,EAAQuc,IAAI,CAAC,MAAD,CAAZ,EAAsBA,IAAI,CAAC,OAAD,CAA1B,EAAqCA,IAAI,CAAC,QAAD,CAAzC,EAAqDvc,CAAC,CAACsD,MAAM,CAACia,aAAR,CAAD,GAA0B,YAAY;CAAE,WAAO,IAAP;CAAc,GAA3G,EAA6Gvd,CAApH;;CACA,WAASuc,IAAT,CAAczT,CAAd,EAAiB;CAAE,QAAIwT,CAAC,CAACxT,CAAD,CAAL,EAAU9I,CAAC,CAAC8I,CAAD,CAAD,GAAO,UAAU2T,CAAV,EAAa;CAAE,aAAO,IAAIlB,OAAJ,CAAY,UAAUjU,CAAV,EAAa3B,CAAb,EAAgB;CAAE6X,QAAAA,CAAC,CAAClc,IAAF,CAAO,CAACwH,CAAD,EAAI2T,CAAJ,EAAOnV,CAAP,EAAU3B,CAAV,CAAP,IAAuB,CAAvB,IAA4B8X,MAAM,CAAC3U,CAAD,EAAI2T,CAAJ,CAAlC;CAA2C,OAAzE,CAAP;CAAoF,KAA1G;CAA6G;;CAC1I,WAASgB,MAAT,CAAgB3U,CAAhB,EAAmB2T,CAAnB,EAAsB;CAAE,QAAI;CAAEf,MAAAA,IAAI,CAACY,CAAC,CAACxT,CAAD,CAAD,CAAK2T,CAAL,CAAD,CAAJ;CAAgB,KAAtB,CAAuB,OAAOxa,CAAP,EAAU;CAAEyb,MAAAA,MAAM,CAACF,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUvb,CAAV,CAAN;CAAqB;CAAE;;CAClF,WAASyZ,IAAT,CAAcpB,CAAd,EAAiB;CAAEA,IAAAA,CAAC,CAACzX,KAAF,YAAmBwa,OAAnB,GAA6B9B,OAAO,CAACD,OAAR,CAAgBhB,CAAC,CAACzX,KAAF,CAAQ4Z,CAAxB,EAA2BV,IAA3B,CAAgC4B,OAAhC,EAAyCnC,MAAzC,CAA7B,GAAgFkC,MAAM,CAACF,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUlD,CAAV,CAAtF;CAAqG;;CACxH,WAASqD,OAAT,CAAiB9a,KAAjB,EAAwB;CAAE4a,IAAAA,MAAM,CAAC,MAAD,EAAS5a,KAAT,CAAN;CAAwB;;CAClD,WAAS2Y,MAAT,CAAgB3Y,KAAhB,EAAuB;CAAE4a,IAAAA,MAAM,CAAC,OAAD,EAAU5a,KAAV,CAAN;CAAyB;;CAClD,WAAS6a,MAAT,CAAgBtK,CAAhB,EAAmBqJ,CAAnB,EAAsB;CAAE,QAAIrJ,CAAC,CAACqJ,CAAD,CAAD,EAAMe,CAAC,CAACI,KAAF,EAAN,EAAiBJ,CAAC,CAACtd,MAAvB,EAA+Bud,MAAM,CAACD,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUA,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAV,CAAN;CAA2B;CACrF;CAEM,SAASK,gBAAT,CAA0BvF,CAA1B,EAA6B;CAChC,MAAItY,CAAJ,EAAO2Z,CAAP;CACA,SAAO3Z,CAAC,GAAG,EAAJ,EAAQuc,IAAI,CAAC,MAAD,CAAZ,EAAsBA,IAAI,CAAC,OAAD,EAAU,UAAUta,CAAV,EAAa;CAAE,UAAMA,CAAN;CAAU,GAAnC,CAA1B,EAAgEsa,IAAI,CAAC,QAAD,CAApE,EAAgFvc,CAAC,CAACsD,MAAM,CAACkZ,QAAR,CAAD,GAAqB,YAAY;CAAE,WAAO,IAAP;CAAc,GAAjI,EAAmIxc,CAA1I;;CACA,WAASuc,IAAT,CAAczT,CAAd,EAAiBsK,CAAjB,EAAoB;CAAEpT,IAAAA,CAAC,CAAC8I,CAAD,CAAD,GAAOwP,CAAC,CAACxP,CAAD,CAAD,GAAO,UAAU2T,CAAV,EAAa;CAAE,aAAO,CAAC9C,CAAC,GAAG,CAACA,CAAN,IAAW;CAAE9W,QAAAA,KAAK,EAAEwa,OAAO,CAAC/E,CAAC,CAACxP,CAAD,CAAD,CAAK2T,CAAL,CAAD,CAAhB;CAA2BX,QAAAA,IAAI,EAAEhT,CAAC,KAAK;CAAvC,OAAX,GAA+DsK,CAAC,GAAGA,CAAC,CAACqJ,CAAD,CAAJ,GAAUA,CAAjF;CAAqF,KAA3G,GAA8GrJ,CAArH;CAAyH;CAClJ;CAEM,SAAS0K,aAAT,CAAuBxF,CAAvB,EAA0B;CAC7B,MAAI,CAAChV,MAAM,CAACia,aAAZ,EAA2B,MAAM,IAAIxY,SAAJ,CAAc,sCAAd,CAAN;CAC3B,MAAI7C,CAAC,GAAGoW,CAAC,CAAChV,MAAM,CAACia,aAAR,CAAT;CAAA,MAAiCvd,CAAjC;CACA,SAAOkC,CAAC,GAAGA,CAAC,CAAC8F,IAAF,CAAOsQ,CAAP,CAAH,IAAgBA,CAAC,GAAG,OAAOyE,QAAP,KAAoB,UAApB,GAAiCA,QAAQ,CAACzE,CAAD,CAAzC,GAA+CA,CAAC,CAAChV,MAAM,CAACkZ,QAAR,CAAD,EAAnD,EAAyExc,CAAC,GAAG,EAA7E,EAAiFuc,IAAI,CAAC,MAAD,CAArF,EAA+FA,IAAI,CAAC,OAAD,CAAnG,EAA8GA,IAAI,CAAC,QAAD,CAAlH,EAA8Hvc,CAAC,CAACsD,MAAM,CAACia,aAAR,CAAD,GAA0B,YAAY;CAAE,WAAO,IAAP;CAAc,GAApL,EAAsLvd,CAAtM,CAAR;;CACA,WAASuc,IAAT,CAAczT,CAAd,EAAiB;CAAE9I,IAAAA,CAAC,CAAC8I,CAAD,CAAD,GAAOwP,CAAC,CAACxP,CAAD,CAAD,IAAQ,UAAU2T,CAAV,EAAa;CAAE,aAAO,IAAIlB,OAAJ,CAAY,UAAUD,OAAV,EAAmBE,MAAnB,EAA2B;CAAEiB,QAAAA,CAAC,GAAGnE,CAAC,CAACxP,CAAD,CAAD,CAAK2T,CAAL,CAAJ,EAAaiB,MAAM,CAACpC,OAAD,EAAUE,MAAV,EAAkBiB,CAAC,CAACX,IAApB,EAA0BW,CAAC,CAAC5Z,KAA5B,CAAnB;CAAwD,OAAjG,CAAP;CAA4G,KAA1I;CAA6I;;CAChK,WAAS6a,MAAT,CAAgBpC,OAAhB,EAAyBE,MAAzB,EAAiCjZ,CAAjC,EAAoCka,CAApC,EAAuC;CAAElB,IAAAA,OAAO,CAACD,OAAR,CAAgBmB,CAAhB,EAAmBV,IAAnB,CAAwB,UAASU,CAAT,EAAY;CAAEnB,MAAAA,OAAO,CAAC;CAAEzY,QAAAA,KAAK,EAAE4Z,CAAT;CAAYX,QAAAA,IAAI,EAAEvZ;CAAlB,OAAD,CAAP;CAAiC,KAAvE,EAAyEiZ,MAAzE;CAAmF;CAC/H;CAEM,SAASuC,oBAAT,CAA8BC,MAA9B,EAAsCC,GAAtC,EAA2C;CAC9C,MAAIha,MAAM,CAACG,cAAX,EAA2B;CAAEH,IAAAA,MAAM,CAACG,cAAP,CAAsB4Z,MAAtB,EAA8B,KAA9B,EAAqC;CAAEnb,MAAAA,KAAK,EAAEob;CAAT,KAArC;CAAuD,GAApF,MAA0F;CAAED,IAAAA,MAAM,CAACC,GAAP,GAAaA,GAAb;CAAmB;;CAC/G,SAAOD,MAAP;CACH;CAEM,SAASE,YAAT,CAAsBC,GAAtB,EAA2B;CAC9B,MAAIA,GAAG,IAAIA,GAAG,CAACC,UAAf,EAA2B,OAAOD,GAAP;CAC3B,MAAItC,MAAM,GAAG,EAAb;CACA,MAAIsC,GAAG,IAAI,IAAX,EAAiB,KAAK,IAAIvB,CAAT,IAAcuB,GAAd;CAAmB,QAAIla,MAAM,CAACqT,cAAP,CAAsBtP,IAAtB,CAA2BmW,GAA3B,EAAgCvB,CAAhC,CAAJ,EAAwCf,MAAM,CAACe,CAAD,CAAN,GAAYuB,GAAG,CAACvB,CAAD,CAAf;CAA3D;CACjBf,EAAAA,MAAM,WAAN,GAAiBsC,GAAjB;CACA,SAAOtC,MAAP;CACH;CAEM,SAASwC,eAAT,CAAyBF,GAAzB,EAA8B;CACjC,SAAQA,GAAG,IAAIA,GAAG,CAACC,UAAZ,GAA0BD,GAA1B,GAAgC;CAAE,eAASA;CAAX,GAAvC;CACH;CAEM,SAASG,sBAAT,CAAgCC,QAAhC,EAA0CC,UAA1C,EAAsD;CACzD,MAAI,CAACA,UAAU,CAACC,GAAX,CAAeF,QAAf,CAAL,EAA+B;CAC3B,UAAM,IAAIxZ,SAAJ,CAAc,gDAAd,CAAN;CACH;;CACD,SAAOyZ,UAAU,CAACla,GAAX,CAAeia,QAAf,CAAP;CACH;CAEM,SAASG,sBAAT,CAAgCH,QAAhC,EAA0CC,UAA1C,EAAsD3b,KAAtD,EAA6D;CAChE,MAAI,CAAC2b,UAAU,CAACC,GAAX,CAAeF,QAAf,CAAL,EAA+B;CAC3B,UAAM,IAAIxZ,SAAJ,CAAc,gDAAd,CAAN;CACH;;CACDyZ,EAAAA,UAAU,CAACzW,GAAX,CAAewW,QAAf,EAAyB1b,KAAzB;CACA,SAAOA,KAAP;CACH;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;ACzN6B;CAQ9B;CACa,iCAAyB,GAA0B,WAAwC,CAAC;CAUzG;CACA;KAA+B8b,uCAAyB;KAetD,mBAAY,GAAkB,EAAE,IAAa;SAA7C,iBAgBC;;;SAbC,IAAI,EAAE,KAAI,YAAY,SAAS,CAAC;aAAE,OAAO,IAAI,SAAS,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;SAElE,IAAI,WAAI,CAAC,MAAM,CAAC,GAAG,CAAC,EAAE;aACpB,QAAA,kBAAM,GAAG,CAAC,GAAG,EAAE,GAAG,CAAC,IAAI,EAAE,IAAI,CAAC,SAAC;UAChC;cAAM;aACL,QAAA,kBAAM,GAAG,EAAE,IAAI,EAAE,IAAI,CAAC,SAAC;UACxB;SACD,MAAM,CAAC,cAAc,CAAC,KAAI,EAAE,WAAW,EAAE;aACvC,KAAK,EAAE,WAAW;aAClB,QAAQ,EAAE,KAAK;aACf,YAAY,EAAE,KAAK;aACnB,UAAU,EAAE,KAAK;UAClB,CAAC,CAAC;;MACJ;KAED,0BAAM,GAAN;SACE,OAAO;aACL,UAAU,EAAE,IAAI,CAAC,QAAQ,EAAE;UAC5B,CAAC;MACH;;KAGM,iBAAO,GAAd,UAAe,KAAa;SAC1B,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,OAAO,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;MACjD;;KAGM,oBAAU,GAAjB,UAAkB,KAAa;SAC7B,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,UAAU,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;MACpD;;;;;;;KAQM,kBAAQ,GAAf,UAAgB,OAAe,EAAE,QAAgB;SAC/C,OAAO,IAAI,SAAS,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;MACzC;;;;;;;KAQM,oBAAU,GAAjB,UAAkB,GAAW,EAAE,QAAgB;SAC7C,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,UAAU,CAAC,GAAG,EAAE,IAAI,EAAE,QAAQ,CAAC,CAAC,CAAC;MAC5D;;KAGD,kCAAc,GAAd;SACE,OAAO,EAAE,UAAU,EAAE,EAAE,CAAC,EAAE,IAAI,CAAC,IAAI,KAAK,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,GAAG,KAAK,CAAC,EAAE,EAAE,CAAC;MAClE;;KAGM,0BAAgB,GAAvB,UAAwB,GAAsB;SAC5C,OAAO,IAAI,SAAS,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC,EAAE,GAAG,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;MAC1D;;KAGD,oBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;SACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;MACvB;KAED,2BAAO,GAAP;SACE,OAAO,mBAAiB,IAAI,CAAC,UAAU,EAAE,CAAC,QAAQ,EAAE,UAAK,IAAI,CAAC,WAAW,EAAE,CAAC,QAAQ,EAAE,MAAG,CAAC;MAC3F;KAnFe,mBAAS,GAAG,WAAI,CAAC,kBAAkB,CAAC;KAoFtD,gBAAC;EAAA,CAvF8B,iCAAyB,GAuFvD;CAvFY,8BAAS;;;;;;;;;;;ACpBY;AAEJ;AACgB;AACJ;AACR;AACD;AACH;AACK;AACA;AACG;AAC0B;AAC1B;AACA;AACE;CAqBxC,SAAgB,UAAU,CAAC,KAAc;KACvC,QACEvF,kBAAY,CAAC,KAAK,CAAC,IAAI,OAAO,CAAC,GAAG,CAAC,KAAK,EAAE,WAAW,CAAC,IAAI,OAAO,KAAK,CAAC,SAAS,KAAK,QAAQ,EAC7F;CACJ,CAAC;CAJD,gCAIC;CAED;CACA,IAAM,cAAc,GAAG,UAAU,CAAC;CAClC,IAAM,cAAc,GAAG,CAAC,UAAU,CAAC;CACnC;CACA,IAAM,cAAc,GAAG,kBAAkB,CAAC;CAC1C,IAAM,cAAc,GAAG,CAAC,kBAAkB,CAAC;CAE3C;CACA;CACA,IAAM,YAAY,GAAG;KACnB,IAAI,EAAEwF,iBAAQ;KACd,OAAO,EAAErF,aAAM;KACf,KAAK,EAAEA,aAAM;KACb,OAAO,EAAEsF,iBAAU;KACnB,UAAU,EAAEC,YAAK;KACjB,cAAc,EAAEC,qBAAU;KAC1B,aAAa,EAAE,eAAM;KACrB,WAAW,EAAE,WAAI;KACjB,OAAO,EAAEC,cAAM;KACf,OAAO,EAAEC,cAAM;KACf,MAAM,EAAEC,iBAAU;KAClB,kBAAkB,EAAEA,iBAAU;KAC9B,UAAU,EAAEC,mBAAS;EACb,CAAC;CAEX;CACA,SAAS,gBAAgB,CAAC,KAAU,EAAE,OAA2B;KAA3B,wBAAA,EAAA,YAA2B;KAC/D,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;SAC7B,IAAI,OAAO,CAAC,OAAO,IAAI,OAAO,CAAC,MAAM,EAAE;aACrC,OAAO,KAAK,CAAC;UACd;;;SAID,IAAI,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK,EAAE;aAC/B,IAAI,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc;iBAAE,OAAO,IAAIL,YAAK,CAAC,KAAK,CAAC,CAAC;aAChF,IAAI,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc;iBAAE,OAAO,WAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC;UACvF;;SAGD,OAAO,IAAI,eAAM,CAAC,KAAK,CAAC,CAAC;MAC1B;;KAGD,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ;SAAE,OAAO,KAAK,CAAC;;KAG7D,IAAI,KAAK,CAAC,UAAU;SAAE,OAAO,IAAI,CAAC;KAElC,IAAM,IAAI,GAAG,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,MAAM,CACpC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,IAAI,GAAA,CACV,CAAC;KACnC,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;SACpC,IAAM,CAAC,GAAG,YAAY,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,CAAC;SAChC,IAAI,CAAC;aAAE,OAAO,CAAC,CAAC,gBAAgB,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;MAClD;KAED,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,EAAE;SACvB,IAAM,CAAC,GAAG,KAAK,CAAC,KAAK,CAAC;SACtB,IAAM,IAAI,GAAG,IAAI,IAAI,EAAE,CAAC;SAExB,IAAI,OAAO,CAAC,MAAM,EAAE;aAClB,IAAI,OAAO,CAAC,KAAK,QAAQ;iBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;kBACtC,IAAI,OAAO,CAAC,KAAK,QAAQ;iBAAE,IAAI,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;UAC7D;cAAM;aACL,IAAI,OAAO,CAAC,KAAK,QAAQ;iBAAE,IAAI,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;kBAClD,IAAI,WAAI,CAAC,MAAM,CAAC,CAAC,CAAC;iBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,QAAQ,EAAE,CAAC,CAAC;kBAC/C,IAAI,OAAO,CAAC,KAAK,QAAQ,IAAI,OAAO,CAAC,OAAO;iBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;UACpE;SACD,OAAO,IAAI,CAAC;MACb;KAED,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,EAAE;SACvB,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,KAAK,CAAC,CAAC;SACtC,IAAI,KAAK,CAAC,MAAM,EAAE;aAChB,IAAI,CAAC,MAAM,GAAG,gBAAgB,CAAC,KAAK,CAAC,MAAM,CAAC,CAAC;UAC9C;SAED,OAAOM,SAAI,CAAC,gBAAgB,CAAC,KAAK,CAAC,CAAC;MACrC;KAED,IAAIC,kBAAW,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,UAAU,EAAE;SAC1C,IAAM,CAAC,GAAG,KAAK,CAAC,IAAI,GAAG,KAAK,GAAG,KAAK,CAAC,UAAU,CAAC;;;SAIhD,IAAI,CAAC,YAAYA,YAAK;aAAE,OAAO,CAAC,CAAC;SAEjC,IAAM,UAAU,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,GAAA,CAAC,CAAC;SACjE,IAAI,OAAK,GAAG,IAAI,CAAC;SACjB,UAAU,CAAC,OAAO,CAAC,UAAA,CAAC;aAClB,IAAI,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC;iBAAE,OAAK,GAAG,KAAK,CAAC;UAC7D,CAAC,CAAC;;SAGH,IAAI,OAAK;aAAE,OAAOA,YAAK,CAAC,gBAAgB,CAAC,CAAC,CAAC,CAAC;MAC7C;KAED,OAAO,KAAK,CAAC;CACf,CAAC;CAMD;CACA,SAAS,cAAc,CAAC,KAAY,EAAE,OAA8B;KAClE,OAAO,KAAK,CAAC,GAAG,CAAC,UAAC,CAAU,EAAE,KAAa;SACzC,OAAO,CAAC,WAAW,CAAC,IAAI,CAAC,EAAE,YAAY,EAAE,WAAS,KAAO,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC,CAAC;SACxE,IAAI;aACF,OAAO,cAAc,CAAC,CAAC,EAAE,OAAO,CAAC,CAAC;UACnC;iBAAS;aACR,OAAO,CAAC,WAAW,CAAC,GAAG,EAAE,CAAC;UAC3B;MACF,CAAC,CAAC;CACL,CAAC;CAED,SAAS,YAAY,CAAC,IAAU;KAC9B,IAAM,MAAM,GAAG,IAAI,CAAC,WAAW,EAAE,CAAC;;KAElC,OAAO,IAAI,CAAC,kBAAkB,EAAE,KAAK,CAAC,GAAG,MAAM,GAAG,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;CAC9E,CAAC;CAED;CACA,SAAS,cAAc,CAAC,KAAU,EAAE,OAA8B;KAChE,IAAI,CAAC,OAAO,KAAK,KAAK,QAAQ,IAAI,OAAO,KAAK,KAAK,UAAU,KAAK,KAAK,KAAK,IAAI,EAAE;SAChF,IAAM,KAAK,GAAG,OAAO,CAAC,WAAW,CAAC,SAAS,CAAC,UAAA,KAAK,IAAI,OAAA,KAAK,CAAC,GAAG,KAAK,KAAK,GAAA,CAAC,CAAC;SAC1E,IAAI,KAAK,KAAK,CAAC,CAAC,EAAE;aAChB,IAAM,KAAK,GAAG,OAAO,CAAC,WAAW,CAAC,GAAG,CAAC,UAAA,KAAK,IAAI,OAAA,KAAK,CAAC,YAAY,GAAA,CAAC,CAAC;aACnE,IAAM,WAAW,GAAG,KAAK;kBACtB,KAAK,CAAC,CAAC,EAAE,KAAK,CAAC;kBACf,GAAG,CAAC,UAAA,IAAI,IAAI,OAAG,IAAI,SAAM,GAAA,CAAC;kBAC1B,IAAI,CAAC,EAAE,CAAC,CAAC;aACZ,IAAM,WAAW,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC;aACjC,IAAM,YAAY,GAChB,MAAM;iBACN,KAAK;sBACF,KAAK,CAAC,KAAK,GAAG,CAAC,EAAE,KAAK,CAAC,MAAM,GAAG,CAAC,CAAC;sBAClC,GAAG,CAAC,UAAA,IAAI,IAAI,OAAG,IAAI,SAAM,GAAA,CAAC;sBAC1B,IAAI,CAAC,EAAE,CAAC,CAAC;aACd,IAAM,OAAO,GAAG,KAAK,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;aACxC,IAAM,YAAY,GAAG,GAAG,CAAC,MAAM,CAAC,WAAW,CAAC,MAAM,GAAG,WAAW,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;aAC7E,IAAM,MAAM,GAAG,GAAG,CAAC,MAAM,CACvB,YAAY,CAAC,MAAM,GAAG,CAAC,WAAW,CAAC,MAAM,GAAG,OAAO,CAAC,MAAM,IAAI,CAAC,GAAG,CAAC,CACpE,CAAC;aAEF,MAAM,IAAI,SAAS,CACjB,2CAA2C;kBACzC,SAAO,WAAW,GAAG,WAAW,GAAG,YAAY,GAAG,OAAO,OAAI,CAAA;kBAC7D,SAAO,YAAY,UAAK,MAAM,MAAG,CAAA,CACpC,CAAC;UACH;SACD,OAAO,CAAC,WAAW,CAAC,OAAO,CAAC,WAAW,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC,GAAG,GAAG,KAAK,CAAC;MACjE;KAED,IAAI,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC;SAAE,OAAO,cAAc,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;KAEhE,IAAI,KAAK,KAAK,SAAS;SAAE,OAAO,IAAI,CAAC;KAErC,IAAI,KAAK,YAAY,IAAI,IAAIjG,YAAM,CAAC,KAAK,CAAC,EAAE;SAC1C,IAAM,OAAO,GAAG,KAAK,CAAC,OAAO,EAAE;;SAE7B,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC,IAAI,OAAO,GAAG,eAAe,CAAC;SAEtD,IAAI,OAAO,CAAC,MAAM,EAAE;aAClB,OAAO,OAAO,CAAC,OAAO,IAAI,OAAO;mBAC7B,EAAE,KAAK,EAAE,KAAK,CAAC,OAAO,EAAE,EAAE;mBAC1B,EAAE,KAAK,EAAE,YAAY,CAAC,KAAK,CAAC,EAAE,CAAC;UACpC;SACD,OAAO,OAAO,CAAC,OAAO,IAAI,OAAO;eAC7B,EAAE,KAAK,EAAE,YAAY,CAAC,KAAK,CAAC,EAAE;eAC9B,EAAE,KAAK,EAAE,EAAE,WAAW,EAAE,KAAK,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE,EAAE,EAAE,CAAC;MAC5D;KAED,IAAI,OAAO,KAAK,KAAK,QAAQ,KAAK,CAAC,OAAO,CAAC,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC,EAAE;;SAEvE,IAAI,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK,EAAE;aAC/B,IAAM,UAAU,GAAG,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc,EACnE,UAAU,GAAG,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc,CAAC;;aAGlE,IAAI,UAAU;iBAAE,OAAO,EAAE,UAAU,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;aACxD,IAAI,UAAU;iBAAE,OAAO,EAAE,WAAW,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;UAC1D;SACD,OAAO,EAAE,aAAa,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;MAC5C;KAED,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;SAC9C,IAAI,KAAK,GAAG,KAAK,CAAC,KAAK,CAAC;SACxB,IAAI,KAAK,KAAK,SAAS,EAAE;aACvB,IAAM,KAAK,GAAG,KAAK,CAAC,QAAQ,EAAE,CAAC,KAAK,CAAC,WAAW,CAAC,CAAC;aAClD,IAAI,KAAK,EAAE;iBACT,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;cAClB;UACF;SAED,IAAM,EAAE,GAAG,IAAI8F,iBAAU,CAAC,KAAK,CAAC,MAAM,EAAE,KAAK,CAAC,CAAC;SAC/C,OAAO,EAAE,CAAC,cAAc,CAAC,OAAO,CAAC,CAAC;MACnC;KAED,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ;SAAE,OAAO,iBAAiB,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;KACzF,OAAO,KAAK,CAAC;CACf,CAAC;CAED,IAAM,kBAAkB,GAAG;KACzB,MAAM,EAAE,UAAC,CAAS,IAAK,OAAA,IAAI3F,aAAM,CAAC,CAAC,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,QAAQ,CAAC,GAAA;KACxD,IAAI,EAAE,UAAC,CAAO,IAAK,OAAA,IAAI6F,SAAI,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC,KAAK,CAAC,GAAA;KAC5C,KAAK,EAAE,UAAC,CAAQ,IAAK,OAAA,IAAIC,YAAK,CAAC,CAAC,CAAC,UAAU,IAAI,CAAC,CAAC,SAAS,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,MAAM,CAAC,GAAA;KAClF,UAAU,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIN,qBAAU,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;KACtD,MAAM,EAAE,UAAC,CAAS,IAAK,OAAA,IAAI,eAAM,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;KAC1C,KAAK,EAAE,UAAC,CAAQ,IAAK,OAAA,IAAID,YAAK,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;KACvC,IAAI,EAAE,UACJ,CAIC;SAED,OAAA,WAAI,CAAC,QAAQ;;SAEX,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,GAAG,GAAG,CAAC,CAAC,IAAI,EAC9B,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,CAAC,KAAK,EAChC,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,QAAQ,GAAG,CAAC,CAAC,SAAS,CACzC;MAAA;KACH,MAAM,EAAE,cAAM,OAAA,IAAIG,cAAM,EAAE,GAAA;KAC1B,MAAM,EAAE,cAAM,OAAA,IAAID,cAAM,EAAE,GAAA;KAC1B,QAAQ,EAAE,UAAC,CAAW,IAAK,OAAA,IAAIJ,iBAAQ,CAAC,CAAC,CAAC,GAAA;KAC1C,QAAQ,EAAE,UAAC,CAAW,IAAK,OAAA,IAAIA,iBAAQ,CAAC,CAAC,CAAC,GAAA;KAC1C,UAAU,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIM,iBAAU,CAAC,CAAC,CAAC,OAAO,EAAE,CAAC,CAAC,OAAO,CAAC,GAAA;KACnE,MAAM,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIL,iBAAU,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;KAClD,SAAS,EAAE,UAAC,CAAY,IAAK,OAAAM,mBAAS,CAAC,QAAQ,CAAC,CAAC,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,GAAA;EACtD,CAAC;CAEX;CACA,SAAS,iBAAiB,CAAC,GAAQ,EAAE,OAA8B;KACjE,IAAI,GAAG,IAAI,IAAI,IAAI,OAAO,GAAG,KAAK,QAAQ;SAAE,MAAM,IAAI,KAAK,CAAC,wBAAwB,CAAC,CAAC;KAEtF,IAAM,QAAQ,GAA0B,GAAG,CAAC,SAAS,CAAC;KACtD,IAAI,OAAO,QAAQ,KAAK,WAAW,EAAE;;SAEnC,IAAM,IAAI,GAAa,EAAE,CAAC;SAC1B,KAAK,IAAM,IAAI,IAAI,GAAG,EAAE;aACtB,OAAO,CAAC,WAAW,CAAC,IAAI,CAAC,EAAE,YAAY,EAAE,IAAI,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC,CAAC;aAC5D,IAAI;iBACF,IAAI,CAAC,IAAI,CAAC,GAAG,cAAc,CAAC,GAAG,CAAC,IAAI,CAAC,EAAE,OAAO,CAAC,CAAC;cACjD;qBAAS;iBACR,OAAO,CAAC,WAAW,CAAC,GAAG,EAAE,CAAC;cAC3B;UACF;SACD,OAAO,IAAI,CAAC;MACb;UAAM,IAAI,UAAU,CAAC,GAAG,CAAC,EAAE;;;SAG1B,IAAI,MAAM,GAAQ,GAAG,CAAC;SACtB,IAAI,OAAO,MAAM,CAAC,cAAc,KAAK,UAAU,EAAE;;;;;aAK/C,IAAM,MAAM,GAAG,kBAAkB,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;aACjD,IAAI,CAAC,MAAM,EAAE;iBACX,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,GAAG,CAAC,SAAS,CAAC,CAAC;cAC5E;aACD,MAAM,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC;UACzB;;SAGD,IAAI,QAAQ,KAAK,MAAM,IAAI,MAAM,CAAC,KAAK,EAAE;aACvC,MAAM,GAAG,IAAIC,SAAI,CAAC,MAAM,CAAC,IAAI,EAAE,cAAc,CAAC,MAAM,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC,CAAC;UACvE;cAAM,IAAI,QAAQ,KAAK,OAAO,IAAI,MAAM,CAAC,GAAG,EAAE;aAC7C,MAAM,GAAG,IAAIC,YAAK,CAChB,cAAc,CAAC,MAAM,CAAC,UAAU,EAAE,OAAO,CAAC,EAC1C,cAAc,CAAC,MAAM,CAAC,GAAG,EAAE,OAAO,CAAC,EACnC,cAAc,CAAC,MAAM,CAAC,EAAE,EAAE,OAAO,CAAC,EAClC,cAAc,CAAC,MAAM,CAAC,MAAM,EAAE,OAAO,CAAC,CACvC,CAAC;UACH;SAED,OAAO,MAAM,CAAC,cAAc,CAAC,OAAO,CAAC,CAAC;MACvC;UAAM;SACL,MAAM,IAAI,KAAK,CAAC,uCAAuC,GAAG,OAAO,QAAQ,CAAC,CAAC;MAC5E;CACH,CAAC;CASD,WAAiB,KAAK;;;;;;;;;;;;;;;;;KA6BpB,SAAgB,KAAK,CAAC,IAAY,EAAE,OAAuB;SACzD,IAAM,YAAY,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,EAAE,OAAO,EAAE,IAAI,EAAE,MAAM,EAAE,KAAK,EAAE,EAAE,OAAO,CAAC,CAAC;;SAGlF,IAAI,OAAO,YAAY,CAAC,OAAO,KAAK,SAAS;aAAE,YAAY,CAAC,MAAM,GAAG,CAAC,YAAY,CAAC,OAAO,CAAC;SAC3F,IAAI,OAAO,YAAY,CAAC,MAAM,KAAK,SAAS;aAAE,YAAY,CAAC,OAAO,GAAG,CAAC,YAAY,CAAC,MAAM,CAAC;SAE1F,OAAO,IAAI,CAAC,KAAK,CAAC,IAAI,EAAE,UAAC,IAAI,EAAE,KAAK,IAAK,OAAA,gBAAgB,CAAC,KAAK,EAAE,YAAY,CAAC,GAAA,CAAC,CAAC;MACjF;KARe,WAAK,QAQpB,CAAA;;;;;;;;;;;;;;;;;;;;;;;;KA4BD,SAAgB,SAAS,CACvB,KAAwB;;KAExB,QAA8F,EAC9F,KAAuB,EACvB,OAAuB;SAEvB,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;aAC9C,OAAO,GAAG,KAAK,CAAC;aAChB,KAAK,GAAG,CAAC,CAAC;UACX;SACD,IAAI,QAAQ,IAAI,IAAI,IAAI,OAAO,QAAQ,KAAK,QAAQ,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,QAAQ,CAAC,EAAE;aAChF,OAAO,GAAG,QAAQ,CAAC;aACnB,QAAQ,GAAG,SAAS,CAAC;aACrB,KAAK,GAAG,CAAC,CAAC;UACX;SACD,IAAM,gBAAgB,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,OAAO,EAAE,IAAI,EAAE,MAAM,EAAE,KAAK,EAAE,EAAE,OAAO,EAAE;aAChF,WAAW,EAAE,CAAC,EAAE,YAAY,EAAE,QAAQ,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;UACrD,CAAC,CAAC;SAEH,IAAM,GAAG,GAAG,cAAc,CAAC,KAAK,EAAE,gBAAgB,CAAC,CAAC;SACpD,OAAO,IAAI,CAAC,SAAS,CAAC,GAAG,EAAE,QAA4C,EAAE,KAAK,CAAC,CAAC;MACjF;KAtBe,eAAS,YAsBxB,CAAA;;;;;;;KAQD,SAAgB,SAAS,CAAC,KAAwB,EAAE,OAAuB;SACzE,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,OAAO,IAAI,CAAC,KAAK,CAAC,SAAS,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC,CAAC;MAC9C;KAHe,eAAS,YAGxB,CAAA;;;;;;;KAQD,SAAgB,WAAW,CAAC,KAAe,EAAE,OAAuB;SAClE,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;SACxB,OAAO,KAAK,CAAC,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,EAAE,OAAO,CAAC,CAAC;MAC9C;KAHe,iBAAW,cAG1B,CAAA;CACH,CAAC,EA9GgB,aAAK,KAAL,aAAK,QA8GrB;;;;;;;;;CC1bD;CACA;;;CAOA;CACA,IAAI,OAAuB,CAAC;CAiIR,sBAAG;CA/HvB,IAAM,KAAK,GAAG,UAAU,eAAoB;;KAE1C,OAAO,eAAe,IAAI,eAAe,CAAC,IAAI,IAAI,IAAI,IAAI,eAAe,CAAC;CAC5E,CAAC,CAAC;CAEF;CACA,SAAS,SAAS;;KAEhB,QACE,KAAK,CAAC,OAAO,UAAU,KAAK,QAAQ,IAAI,UAAU,CAAC;SACnD,KAAK,CAAC,OAAO,MAAM,KAAK,QAAQ,IAAI,MAAM,CAAC;SAC3C,KAAK,CAAC,OAAO,IAAI,KAAK,QAAQ,IAAI,IAAI,CAAC;SACvC,KAAK,CAAC,OAAOnN,cAAM,KAAK,QAAQ,IAAIA,cAAM,CAAC;SAC3C,QAAQ,CAAC,aAAa,CAAC,EAAE,EACzB;CACJ,CAAC;CAED,IAAM,UAAU,GAAG,SAAS,EAAE,CAAC;CAC/B,IAAI,MAAM,CAAC,SAAS,CAAC,cAAc,CAAC,IAAI,CAAC,UAAU,EAAE,KAAK,CAAC,EAAE;KAC3D,cAAA,OAAO,GAAG,UAAU,CAAC,GAAG,CAAC;EAC1B;MAAM;;KAEL,cAAA,OAAO;SAGL,aAAY,KAA2B;aAA3B,sBAAA,EAAA,UAA2B;aACrC,IAAI,CAAC,KAAK,GAAG,EAAE,CAAC;aAChB,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC;aAElB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;iBACrC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,IAAI;qBAAE,SAAS;iBAC/B,IAAM,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;iBACvB,IAAM,GAAG,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;iBACrB,IAAM,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;;iBAEvB,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;;iBAGrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,EAAE,CAAC;cAC5D;UACF;SACD,mBAAK,GAAL;aACE,IAAI,CAAC,KAAK,GAAG,EAAE,CAAC;aAChB,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC;UACnB;SACD,oBAAM,GAAN,UAAO,GAAW;aAChB,IAAM,KAAK,GAAG,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC;aAChC,IAAI,KAAK,IAAI,IAAI;iBAAE,OAAO,KAAK,CAAC;;aAEhC,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC;;aAEzB,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;aAC9B,OAAO,IAAI,CAAC;UACb;SACD,qBAAO,GAAP;aAAA,iBAYC;aAXC,IAAI,KAAK,GAAG,CAAC,CAAC;aAEd,OAAO;iBACL,IAAI,EAAE;qBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;qBAChC,OAAO;yBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,CAAC,GAAG,EAAE,KAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,GAAG,SAAS;yBACjE,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;sBACvC,CAAC;kBACH;cACF,CAAC;UACH;SACD,qBAAO,GAAP,UAAQ,QAAmE,EAAE,IAAW;aACtF,IAAI,GAAG,IAAI,IAAI,IAAI,CAAC;aAEpB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;iBAC1C,IAAM,GAAG,GAAG,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;;iBAE1B,QAAQ,CAAC,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,EAAE,GAAG,EAAE,IAAI,CAAC,CAAC;cACrD;UACF;SACD,iBAAG,GAAH,UAAI,GAAW;aACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,SAAS,CAAC;UAC5D;SACD,iBAAG,GAAH,UAAI,GAAW;aACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC;UAClC;SACD,kBAAI,GAAJ;aAAA,iBAYC;aAXC,IAAI,KAAK,GAAG,CAAC,CAAC;aAEd,OAAO;iBACL,IAAI,EAAE;qBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;qBAChC,OAAO;yBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,GAAG,GAAG,SAAS;yBAC1C,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;sBACvC,CAAC;kBACH;cACF,CAAC;UACH;SACD,iBAAG,GAAH,UAAI,GAAW,EAAE,KAAU;aACzB,IAAI,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;iBACrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,KAAK,CAAC;iBAC5B,OAAO,IAAI,CAAC;cACb;;aAGD,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;;aAGrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,EAAE,CAAC;aAC3D,OAAO,IAAI,CAAC;UACb;SACD,oBAAM,GAAN;aAAA,iBAYC;aAXC,IAAI,KAAK,GAAG,CAAC,CAAC;aAEd,OAAO;iBACL,IAAI,EAAE;qBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;qBAChC,OAAO;yBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,KAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,SAAS;yBAC1D,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;sBACvC,CAAC;kBACH;cACF,CAAC;UACH;SACD,sBAAI,qBAAI;kBAAR;iBACE,OAAO,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC;cAC1B;;;YAAA;SACH,UAAC;MAtGU,GAsGoB,CAAC;EACjC;;;;;;;;;;CCxID;CACa,sBAAc,GAAG,UAAU,CAAC;CACzC;CACa,sBAAc,GAAG,CAAC,UAAU,CAAC;CAC1C;CACa,sBAAc,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;CAClD;CACa,sBAAc,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;CAE/C;;;;CAIa,kBAAU,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;CAE1C;;;;CAIa,kBAAU,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;CAE3C;CACa,wBAAgB,GAAG,CAAC,CAAC;CAElC;CACa,wBAAgB,GAAG,CAAC,CAAC;CAElC;CACa,wBAAgB,GAAG,CAAC,CAAC;CAElC;CACa,uBAAe,GAAG,CAAC,CAAC;CAEjC;CACa,wBAAgB,GAAG,CAAC,CAAC;CAElC;CACa,2BAAmB,GAAG,CAAC,CAAC;CAErC;CACa,qBAAa,GAAG,CAAC,CAAC;CAE/B;CACa,yBAAiB,GAAG,CAAC,CAAC;CAEnC;CACa,sBAAc,GAAG,CAAC,CAAC;CAEhC;CACa,sBAAc,GAAG,EAAE,CAAC;CAEjC;CACa,wBAAgB,GAAG,EAAE,CAAC;CAEnC;CACa,2BAAmB,GAAG,EAAE,CAAC;CAEtC;CACa,sBAAc,GAAG,EAAE,CAAC;CAEjC;CACa,wBAAgB,GAAG,EAAE,CAAC;CAEnC;CACa,8BAAsB,GAAG,EAAE,CAAC;CAEzC;CACa,qBAAa,GAAG,EAAE,CAAC;CAEhC;CACa,2BAAmB,GAAG,EAAE,CAAC;CAEtC;CACa,sBAAc,GAAG,EAAE,CAAC;CAEjC;CACa,4BAAoB,GAAG,EAAE,CAAC;CAEvC;CACa,yBAAiB,GAAG,IAAI,CAAC;CAEtC;CACa,yBAAiB,GAAG,IAAI,CAAC;CAEtC;CACa,mCAA2B,GAAG,CAAC,CAAC;CAE7C;CACa,oCAA4B,GAAG,CAAC,CAAC;CAE9C;CACa,sCAA8B,GAAG,CAAC,CAAC;CAEhD;CACa,gCAAwB,GAAG,CAAC,CAAC;CAE1C;CACa,oCAA4B,GAAG,CAAC,CAAC;CAE9C;CACa,+BAAuB,GAAG,CAAC,CAAC;CAEzC;CACa,wCAAgC,GAAG,GAAG,CAAC;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;ACvGpB;AACG;AAEO;AAC6C;CAEvF,SAAgB,mBAAmB,CACjC,MAAgB,EAChB,kBAA4B,EAC5B,eAAyB;KAEzB,IAAI,WAAW,GAAG,CAAC,GAAG,CAAC,CAAC;KAExB,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;SACzB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;aACtC,WAAW,IAAI,gBAAgB,CAC7B,CAAC,CAAC,QAAQ,EAAE,EACZ,MAAM,CAAC,CAAC,CAAC,EACT,kBAAkB,EAClB,IAAI,EACJ,eAAe,CAChB,CAAC;UACH;MACF;UAAM;;SAGL,IAAI,MAAM,CAAC,MAAM,EAAE;aACjB,MAAM,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC;UAC1B;;SAGD,KAAK,IAAM,GAAG,IAAI,MAAM,EAAE;aACxB,WAAW,IAAI,gBAAgB,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,CAAC,EAAE,kBAAkB,EAAE,KAAK,EAAE,eAAe,CAAC,CAAC;UAC/F;MACF;KAED,OAAO,WAAW,CAAC;CACrB,CAAC;CA/BD,kDA+BC;CAED;CACA,SAAS,gBAAgB,CACvB,IAAY;CACZ;CACA,KAAU,EACV,kBAA0B,EAC1B,OAAe,EACf,eAAuB;KAFvB,mCAAA,EAAA,0BAA0B;KAC1B,wBAAA,EAAA,eAAe;KACf,gCAAA,EAAA,uBAAuB;;KAGvB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;SACzB,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;MACxB;KAED,QAAQ,OAAO,KAAK;SAClB,KAAK,QAAQ;aACX,OAAO,CAAC,GAAGiH,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,UAAU,CAAC,KAAK,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;SAC5F,KAAK,QAAQ;aACX,IACE,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK;iBAC3B,KAAK,IAAI,SAAS,CAAC,UAAU;iBAC7B,KAAK,IAAI,SAAS,CAAC,UAAU,EAC7B;iBACA,IAAI,KAAK,IAAI,SAAS,CAAC,cAAc,IAAI,KAAK,IAAI,SAAS,CAAC,cAAc,EAAE;;qBAE1E,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;kBAC3E;sBAAM;qBACL,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;kBAC3E;cACF;kBAAM;;iBAEL,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;cAC3E;SACH,KAAK,WAAW;aACd,IAAI,OAAO,IAAI,CAAC,eAAe;iBAC7B,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;aACtE,OAAO,CAAC,CAAC;SACX,KAAK,SAAS;aACZ,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;SAC5E,KAAK,QAAQ;aACX,IAAI,KAAK,IAAI,IAAI,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBACvF,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;cACrE;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;iBACjF,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,CAAC,CAAC;cAC5E;kBAAM,IAAI,KAAK,YAAY,IAAI,IAAIC,YAAM,CAAC,KAAK,CAAC,EAAE;iBACjD,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;cAC3E;kBAAM,IACL,WAAW,CAAC,MAAM,CAAC,KAAK,CAAC;iBACzB,KAAK,YAAY,WAAW;iBAC5BC,sBAAgB,CAAC,KAAK,CAAC,EACvB;iBACA,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,KAAK,CAAC,UAAU,EACzF;cACH;kBAAM,IACL,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM;iBAC7B,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ;iBAC/B,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAClC;iBACA,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;cAC3E;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBAC9C,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,CAAC,CAAC;cAC5E;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;;iBAExC,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,MAAM,GAAG,CAAC,EAAE;qBAC9D,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;yBACvD,CAAC;yBACD,CAAC;yBACD,CAAC;yBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,EAAE,MAAM,CAAC;yBAChD,CAAC;yBACD,mBAAmB,CAAC,KAAK,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACrE;kBACH;sBAAM;qBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;yBACvD,CAAC;yBACD,CAAC;yBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,EAAE,MAAM,CAAC;yBAChD,CAAC,EACD;kBACH;cACF;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;;iBAE1C,IAAI,KAAK,CAAC,QAAQ,KAAKI,aAAM,CAAC,kBAAkB,EAAE;qBAChD,QACE,CAAC,IAAI,IAAI,IAAI,GAAGJ,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;0BACtD,KAAK,CAAC,QAAQ,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EAChC;kBACH;sBAAM;qBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,KAAK,CAAC,QAAQ,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EACvF;kBACH;cACF;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,KAAK,EAAE,MAAM,CAAC;qBACtC,CAAC;qBACD,CAAC;qBACD,CAAC,EACD;cACH;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;;iBAEzC,IAAM,cAAc,GAAG,MAAM,CAAC,MAAM,CAClC;qBACE,IAAI,EAAE,KAAK,CAAC,UAAU;qBACtB,GAAG,EAAE,KAAK,CAAC,GAAG;kBACf,EACD,KAAK,CAAC,MAAM,CACb,CAAC;;iBAGF,IAAI,KAAK,CAAC,EAAE,IAAI,IAAI,EAAE;qBACpB,cAAc,CAAC,KAAK,CAAC,GAAG,KAAK,CAAC,EAAE,CAAC;kBAClC;iBAED,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvD,CAAC;qBACD,mBAAmB,CAAC,cAAc,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACxE;cACH;kBAAM,IAAI,KAAK,YAAY,MAAM,IAAIC,cAAQ,CAAC,KAAK,CAAC,EAAE;iBACrD,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvD,CAAC;qBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,MAAM,EAAE,MAAM,CAAC;qBACvC,CAAC;sBACA,KAAK,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;sBACrB,KAAK,CAAC,UAAU,GAAG,CAAC,GAAG,CAAC,CAAC;sBACzB,KAAK,CAAC,SAAS,GAAG,CAAC,GAAG,CAAC,CAAC;qBACzB,CAAC,EACD;cACH;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBAC9C,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvD,CAAC;qBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,MAAM,CAAC;qBACxC,CAAC;qBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,MAAM,CAAC;qBACxC,CAAC,EACD;cACH;kBAAM;iBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvD,mBAAmB,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC;qBAC/D,CAAC,EACD;cACH;SACH,KAAK,UAAU;;aAEb,IAAI,KAAK,YAAY,MAAM,IAAIC,cAAQ,CAAC,KAAK,CAAC,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,iBAAiB,EAAE;iBAC1F,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;qBACvD,CAAC;qBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,MAAM,EAAE,MAAM,CAAC;qBACvC,CAAC;sBACA,KAAK,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;sBACrB,KAAK,CAAC,UAAU,GAAG,CAAC,GAAG,CAAC,CAAC;sBACzB,KAAK,CAAC,SAAS,GAAG,CAAC,GAAG,CAAC,CAAC;qBACzB,CAAC,EACD;cACH;kBAAM;iBACL,IAAI,kBAAkB,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,MAAM,GAAG,CAAC,EAAE;qBACpF,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;yBACvD,CAAC;yBACD,CAAC;yBACD,CAAC;yBACDA,aAAM,CAAC,UAAU,CAACC,8BAAwB,CAAC,KAAK,CAAC,EAAE,MAAM,CAAC;yBAC1D,CAAC;yBACD,mBAAmB,CAAC,KAAK,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACrE;kBACH;sBAAM,IAAI,kBAAkB,EAAE;qBAC7B,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;yBACvD,CAAC;yBACD,CAAC;yBACDA,aAAM,CAAC,UAAU,CAACC,8BAAwB,CAAC,KAAK,CAAC,EAAE,MAAM,CAAC;yBAC1D,CAAC,EACD;kBACH;cACF;MACJ;KAED,OAAO,CAAC,CAAC;CACX,CAAC;;;;;;;;;;CClOD,IAAM,SAAS,GAAG,IAAI,CAAC;CACvB,IAAM,cAAc,GAAG,IAAI,CAAC;CAC5B,IAAM,gBAAgB,GAAG,IAAI,CAAC;CAC9B,IAAM,eAAe,GAAG,IAAI,CAAC;CAC7B,IAAM,eAAe,GAAG,IAAI,CAAC;CAE7B,IAAM,YAAY,GAAG,IAAI,CAAC;CAC1B,IAAM,cAAc,GAAG,IAAI,CAAC;CAC5B,IAAM,aAAa,GAAG,IAAI,CAAC;CAC3B,IAAM,eAAe,GAAG,IAAI,CAAC;CAE7B;;;;;;CAMA,SAAgB,YAAY,CAC1B,KAAkC,EAClC,KAAa,EACb,GAAW;KAEX,IAAI,YAAY,GAAG,CAAC,CAAC;KAErB,KAAK,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC,GAAG,GAAG,EAAE,CAAC,IAAI,CAAC,EAAE;SACnC,IAAM,IAAI,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;SAEtB,IAAI,YAAY,EAAE;aAChB,IAAI,CAAC,IAAI,GAAG,cAAc,MAAM,eAAe,EAAE;iBAC/C,OAAO,KAAK,CAAC;cACd;aACD,YAAY,IAAI,CAAC,CAAC;UACnB;cAAM,IAAI,IAAI,GAAG,SAAS,EAAE;aAC3B,IAAI,CAAC,IAAI,GAAG,gBAAgB,MAAM,YAAY,EAAE;iBAC9C,YAAY,GAAG,CAAC,CAAC;cAClB;kBAAM,IAAI,CAAC,IAAI,GAAG,eAAe,MAAM,cAAc,EAAE;iBACtD,YAAY,GAAG,CAAC,CAAC;cAClB;kBAAM,IAAI,CAAC,IAAI,GAAG,eAAe,MAAM,aAAa,EAAE;iBACrD,YAAY,GAAG,CAAC,CAAC;cAClB;kBAAM;iBACL,OAAO,KAAK,CAAC;cACd;UACF;MACF;KAED,OAAO,CAAC,YAAY,CAAC;CACvB,CAAC;CA7BD,oCA6BC;;;;;;;;;;AC9C+B;AACG;AAEJ;AACW;AACgB;AACf;AACR;AACD;AACH;AACK;AACA;AACG;AACA;AACA;AACE;AACO;CAgChD;CACA,IAAM,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;CAC9D,IAAM,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;CAE9D,IAAM,aAAa,GAAiC,EAAE,CAAC;CAEvD,SAAgB,WAAW,CACzB,MAAc,EACd,OAA2B,EAC3B,OAAiB;KAEjB,OAAO,GAAG,OAAO,IAAI,IAAI,GAAG,EAAE,GAAG,OAAO,CAAC;KACzC,IAAM,KAAK,GAAG,OAAO,IAAI,OAAO,CAAC,KAAK,GAAG,OAAO,CAAC,KAAK,GAAG,CAAC,CAAC;;KAE3D,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,CAAC;UACZ,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;UACvB,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;UACxB,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;KAE5B,IAAI,IAAI,GAAG,CAAC,EAAE;SACZ,MAAM,IAAI,KAAK,CAAC,gCAA8B,IAAM,CAAC,CAAC;MACvD;KAED,IAAI,OAAO,CAAC,gCAAgC,IAAI,MAAM,CAAC,MAAM,GAAG,IAAI,EAAE;SACpE,MAAM,IAAI,KAAK,CAAC,mBAAiB,MAAM,CAAC,MAAM,8BAAyB,IAAM,CAAC,CAAC;MAChF;KAED,IAAI,CAAC,OAAO,CAAC,gCAAgC,IAAI,MAAM,CAAC,MAAM,KAAK,IAAI,EAAE;SACvE,MAAM,IAAI,KAAK,CAAC,mBAAiB,MAAM,CAAC,MAAM,4BAAuB,IAAM,CAAC,CAAC;MAC9E;KAED,IAAI,IAAI,GAAG,KAAK,GAAG,MAAM,CAAC,UAAU,EAAE;SACpC,MAAM,IAAI,KAAK,CACb,gBAAc,IAAI,yBAAoB,KAAK,kCAA6B,MAAM,CAAC,UAAU,MAAG,CAC7F,CAAC;MACH;;KAGD,IAAI,MAAM,CAAC,KAAK,GAAG,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC,EAAE;SAClC,MAAM,IAAI,KAAK,CAAC,6EAA6E,CAAC,CAAC;MAChG;;KAGD,OAAO,iBAAiB,CAAC,MAAM,EAAE,KAAK,EAAE,OAAO,EAAE,OAAO,CAAC,CAAC;CAC5D,CAAC;CAvCD,kCAuCC;CAED,SAAS,iBAAiB,CACxBxX,QAAc,EACd,KAAa,EACb,OAA2B,EAC3B,OAAe;KAAf,wBAAA,EAAA,eAAe;KAEf,IAAM,aAAa,GAAG,OAAO,CAAC,eAAe,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,eAAe,CAAC,CAAC;KAC1F,IAAM,cAAc,GAAG,OAAO,CAAC,gBAAgB,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,gBAAgB,CAAC,CAAC;KAE7F,IAAM,WAAW,GAAG,OAAO,CAAC,aAAa,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,aAAa,CAAC,CAAC;;KAGnF,IAAM,GAAG,GAAG,OAAO,CAAC,KAAK,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,KAAK,CAAC,CAAC;;KAG5D,IAAM,UAAU,GAAG,OAAO,OAAO,CAAC,YAAY,CAAC,KAAK,SAAS,GAAG,OAAO,CAAC,YAAY,CAAC,GAAG,KAAK,CAAC;;KAG9F,IAAM,cAAc,GAAG,OAAO,CAAC,gBAAgB,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,gBAAgB,CAAC,CAAC;KAC7F,IAAM,YAAY,GAAG,OAAO,CAAC,cAAc,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,cAAc,CAAC,CAAC;KACtF,IAAM,aAAa,GAAG,OAAO,CAAC,eAAe,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,eAAe,CAAC,CAAC;;KAGzF,IAAM,UAAU,GAAG,KAAK,CAAC;;KAGzB,IAAIA,QAAM,CAAC,MAAM,GAAG,CAAC;SAAE,MAAM,IAAI,KAAK,CAAC,qCAAqC,CAAC,CAAC;;KAG9E,IAAM,IAAI,GACRA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;KAG/F,IAAI,IAAI,GAAG,CAAC,IAAI,IAAI,GAAGA,QAAM,CAAC,MAAM;SAAE,MAAM,IAAI,KAAK,CAAC,sBAAsB,CAAC,CAAC;;KAG9E,IAAM,MAAM,GAAa,OAAO,GAAG,EAAE,GAAG,EAAE,CAAC;;KAE3C,IAAI,UAAU,GAAG,CAAC,CAAC;KACnB,IAAM,IAAI,GAAG,KAAK,CAAC;;KAGnB,OAAO,CAAC,IAAI,EAAE;;SAEZ,IAAM,WAAW,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,CAAC;;SAGpC,IAAI,WAAW,KAAK,CAAC;aAAE,MAAM;;SAG7B,IAAI,CAAC,GAAG,KAAK,CAAC;;SAEd,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;aAC9C,CAAC,EAAE,CAAC;UACL;;SAGD,IAAI,CAAC,IAAIA,QAAM,CAAC,UAAU;aAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;SAClF,IAAM,IAAI,GAAG,OAAO,GAAG,UAAU,EAAE,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;SACxE,IAAI,KAAK,SAAA,CAAC;SAEV,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;SAEd,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;aAC9C,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IACE,UAAU,IAAI,CAAC;iBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;iBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;aAE/C,IAAI,CAAC0d,0BAAY,CAAC1d,QAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,EAAE;iBACxD,MAAM,IAAI,KAAK,CAAC,uCAAuC,CAAC,CAAC;cAC1D;aAED,KAAK,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;aAE/D,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;UAC5B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,EAAE;aAClD,IAAM,GAAG,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;aAC7BvX,QAAM,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;aACvC,KAAK,GAAG,IAAIgd,iBAAQ,CAAC,GAAG,CAAC,CAAC;aAC1B,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;UACpB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,IAAI,aAAa,KAAK,KAAK,EAAE;aAC7E,KAAK,GAAG,IAAIE,YAAK,CACfld,QAAM,CAAC,KAAK,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAC7F,CAAC;UACH;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,EAAE;aAClD,KAAK;iBACHA,QAAM,CAAC,KAAK,EAAE,CAAC;sBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;sBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;sBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;UAC3B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,aAAa,KAAK,KAAK,EAAE;aAChF,KAAK,GAAG,IAAI,eAAM,CAACA,QAAM,CAAC,YAAY,CAAC,KAAK,CAAC,CAAC,CAAC;aAC/C,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;UACnB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;aACrD,KAAK,GAAGA,QAAM,CAAC,YAAY,CAAC,KAAK,CAAC,CAAC;aACnC,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;UACnB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;aACnD,IAAM,OAAO,GACXA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,KAAK,GAAG,IAAI,IAAI,CAAC,IAAI,WAAI,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC,QAAQ,EAAE,CAAC,CAAC;UAC1D;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;aACtD,IAAIA,QAAM,CAAC,KAAK,CAAC,KAAK,CAAC,IAAIA,QAAM,CAAC,KAAK,CAAC,KAAK,CAAC;iBAAE,MAAM,IAAI,KAAK,CAAC,4BAA4B,CAAC,CAAC;aAC9F,KAAK,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,KAAK,CAAC,CAAC;UAC/B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;aACrD,IAAM,MAAM,GAAG,KAAK,CAAC;aACrB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;kBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;kBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;kBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;aAC5B,IAAI,UAAU,IAAI,CAAC,IAAI,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBACvD,MAAM,IAAI,KAAK,CAAC,sCAAsC,CAAC,CAAC;;aAG1D,IAAI,GAAG,EAAE;iBACP,KAAK,GAAGA,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,CAAC;cACjD;kBAAM;iBACL,KAAK,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC;cAC3D;aAED,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;UAC5B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,eAAe,EAAE;aACpD,IAAM,MAAM,GAAG,KAAK,CAAC;aACrB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;kBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;kBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;kBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;aAC5B,IAAI,YAAY,GAAG,OAAO,CAAC;;aAG3B,IAAM,SAAS,GAAG,KAAK,GAAG,UAAU,CAAC;;aAGrC,IAAI,WAAW,IAAI,WAAW,CAAC,IAAI,CAAC,EAAE;iBACpC,YAAY,GAAG,EAAE,CAAC;iBAClB,KAAK,IAAM,CAAC,IAAI,OAAO,EAAE;qBACtB,YAEC,CAAC,CAAC,CAAC,GAAG,OAAO,CAAC,CAA6B,CAAC,CAAC;kBAChD;iBACD,YAAY,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC;cAC5B;aAED,KAAK,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,YAAY,EAAE,IAAI,CAAC,CAAC;aAC9D,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;aAE3B,IAAIA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,KAAK,CAAC;iBAAE,MAAM,IAAI,KAAK,CAAC,+BAA+B,CAAC,CAAC;aAC9E,IAAI,KAAK,KAAK,SAAS;iBAAE,MAAM,IAAI,KAAK,CAAC,sBAAsB,CAAC,CAAC;UAClE;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;aACxD,KAAK,GAAG,SAAS,CAAC;UACnB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;aACnD,KAAK,GAAG,IAAI,CAAC;UACd;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;;aAEnD,IAAM,OAAO,GACXA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IAAM,IAAI,GAAG,IAAI,WAAI,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;;aAEzC,IAAI,YAAY,IAAI,aAAa,KAAK,IAAI,EAAE;iBAC1C,KAAK;qBACH,IAAI,CAAC,eAAe,CAAC,eAAe,CAAC,IAAI,IAAI,CAAC,kBAAkB,CAAC,eAAe,CAAC;2BAC7E,IAAI,CAAC,QAAQ,EAAE;2BACf,IAAI,CAAC;cACZ;kBAAM;iBACL,KAAK,GAAG,IAAI,CAAC;cACd;UACF;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,oBAAoB,EAAE;;aAEzD,IAAM,KAAK,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;;aAE/BvX,QAAM,CAAC,IAAI,CAAC,KAAK,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;;aAEzC,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;;aAEnB,IAAM2d,YAAU,GAAG,IAAIR,qBAAU,CAAC,KAAK,CAAyC,CAAC;;aAEjF,IAAI,UAAU,IAAIQ,YAAU,IAAI,OAAOA,YAAU,CAAC,QAAQ,KAAK,UAAU,EAAE;iBACzE,KAAK,GAAGA,YAAU,CAAC,QAAQ,EAAE,CAAC;cAC/B;kBAAM;iBACL,KAAK,GAAGA,YAAU,CAAC;cACpB;UACF;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;aACrD,IAAI,UAAU,GACZ3d,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IAAM,eAAe,GAAG,UAAU,CAAC;aACnC,IAAM,OAAO,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,CAAC;;aAGhC,IAAI,UAAU,GAAG,CAAC;iBAAE,MAAM,IAAI,KAAK,CAAC,yCAAyC,CAAC,CAAC;;aAG/E,IAAI,UAAU,GAAGA,QAAM,CAAC,UAAU;iBAChC,MAAM,IAAI,KAAK,CAAC,4CAA4C,CAAC,CAAC;;aAGhE,IAAIA,QAAM,CAAC,OAAO,CAAC,IAAI,IAAI,EAAE;;iBAE3B,IAAI,OAAO,KAAK2X,aAAM,CAAC,kBAAkB,EAAE;qBACzC,UAAU;yBACR3X,QAAM,CAAC,KAAK,EAAE,CAAC;8BACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;8BACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;8BACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;qBAC1B,IAAI,UAAU,GAAG,CAAC;yBAChB,MAAM,IAAI,KAAK,CAAC,0DAA0D,CAAC,CAAC;qBAC9E,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;yBAClC,MAAM,IAAI,KAAK,CAAC,6DAA6D,CAAC,CAAC;qBACjF,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;yBAClC,MAAM,IAAI,KAAK,CAAC,8DAA8D,CAAC,CAAC;kBACnF;iBAED,IAAI,cAAc,IAAI,aAAa,EAAE;qBACnC,KAAK,GAAGA,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,CAAC;kBACjD;sBAAM;qBACL,KAAK,GAAG,IAAI2X,aAAM,CAAC3X,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,EAAE,OAAO,CAAC,CAAC;kBACtE;cACF;kBAAM;iBACL,IAAM,OAAO,GAAGuX,aAAM,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC;;iBAEzC,IAAI,OAAO,KAAKI,aAAM,CAAC,kBAAkB,EAAE;qBACzC,UAAU;yBACR3X,QAAM,CAAC,KAAK,EAAE,CAAC;8BACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;8BACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;8BACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;qBAC1B,IAAI,UAAU,GAAG,CAAC;yBAChB,MAAM,IAAI,KAAK,CAAC,0DAA0D,CAAC,CAAC;qBAC9E,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;yBAClC,MAAM,IAAI,KAAK,CAAC,6DAA6D,CAAC,CAAC;qBACjF,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;yBAClC,MAAM,IAAI,KAAK,CAAC,8DAA8D,CAAC,CAAC;kBACnF;;iBAGD,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,UAAU,EAAE,CAAC,EAAE,EAAE;qBAC/B,OAAO,CAAC,CAAC,CAAC,GAAGA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,CAAC;kBAChC;iBAED,IAAI,cAAc,IAAI,aAAa,EAAE;qBACnC,KAAK,GAAG,OAAO,CAAC;kBACjB;sBAAM;qBACL,KAAK,GAAG,IAAI2X,aAAM,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;kBACtC;cACF;;aAGD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;UAC5B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,UAAU,KAAK,KAAK,EAAE;;aAE7E,CAAC,GAAG,KAAK,CAAC;;aAEV,OAAO3X,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;iBAC9C,CAAC,EAAE,CAAC;cACL;;aAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;iBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;aAE9E,IAAM,MAAM,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;;aAEjD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;aAGd,CAAC,GAAG,KAAK,CAAC;;aAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;iBAC9C,CAAC,EAAE,CAAC;cACL;;aAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;iBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;aAE9E,IAAM,aAAa,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;aACxD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;aAGd,IAAM,YAAY,GAAG,IAAI,KAAK,CAAC,aAAa,CAAC,MAAM,CAAC,CAAC;;aAGrD,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,aAAa,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;iBACzC,QAAQ,aAAa,CAAC,CAAC,CAAC;qBACtB,KAAK,GAAG;yBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;yBACtB,MAAM;qBACR,KAAK,GAAG;yBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;yBACtB,MAAM;qBACR,KAAK,GAAG;yBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;yBACtB,MAAM;kBACT;cACF;aAED,KAAK,GAAG,IAAI,MAAM,CAAC,MAAM,EAAE,YAAY,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC;UACnD;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,UAAU,KAAK,IAAI,EAAE;;aAE5E,CAAC,GAAG,KAAK,CAAC;;aAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;iBAC9C,CAAC,EAAE,CAAC;cACL;;aAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;iBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;aAE9E,IAAM,MAAM,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;aACjD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;aAGd,CAAC,GAAG,KAAK,CAAC;;aAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;iBAC9C,CAAC,EAAE,CAAC;cACL;;aAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;iBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;aAE9E,IAAM,aAAa,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;aACxD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;aAGd,KAAK,GAAG,IAAIsd,iBAAU,CAAC,MAAM,EAAE,aAAa,CAAC,CAAC;UAC/C;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;aACrD,IAAM,UAAU,GACdtd,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IACE,UAAU,IAAI,CAAC;iBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;iBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;aAC/C,IAAM4d,QAAM,GAAG5d,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;aACtE,KAAK,GAAG,aAAa,GAAG4d,QAAM,GAAG,IAAIX,iBAAU,CAACW,QAAM,CAAC,CAAC;aACxD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;UAC5B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;aACxD,IAAM,OAAO,GACX5d,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAE1B,KAAK,GAAG,IAAIud,mBAAS,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;UAC1C;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;aACtD,KAAK,GAAG,IAAIH,cAAM,EAAE,CAAC;UACtB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;aACtD,KAAK,GAAG,IAAIC,cAAM,EAAE,CAAC;UACtB;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;aACnD,IAAM,UAAU,GACdrd,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;aAC1B,IACE,UAAU,IAAI,CAAC;iBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;iBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;aAC/C,IAAM,cAAc,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;aAG9E,IAAI,aAAa,EAAE;;iBAEjB,IAAI,cAAc,EAAE;;qBAElB,KAAK,GAAG,WAAW,CAAC,cAAc,EAAE,aAAa,EAAE,MAAM,CAAC,CAAC;kBAC5D;sBAAM;qBACL,KAAK,GAAG,WAAW,CAAC,cAAc,CAAC,CAAC;kBACrC;cACF;kBAAM;iBACL,KAAK,GAAG,IAAIwd,SAAI,CAAC,cAAc,CAAC,CAAC;cAClC;;aAGD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;UAC5B;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,sBAAsB,EAAE;aAC3D,IAAM,SAAS,GACbxd,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;aAG1B,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;iBAC7B,MAAM,IAAI,KAAK,CAAC,yDAAyD,CAAC,CAAC;cAC5E;;aAGD,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;aAE1B,IACE,UAAU,IAAI,CAAC;iBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;iBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;;aAG/C,IAAM,cAAc,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;aAE9E,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;aAE3B,IAAM,MAAM,GAAG,KAAK,CAAC;;aAErB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;kBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;kBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;kBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;;aAE5B,IAAM,WAAW,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC;;aAEtE,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;aAG3B,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,UAAU,GAAG,UAAU,EAAE;iBAC/C,MAAM,IAAI,KAAK,CAAC,wDAAwD,CAAC,CAAC;cAC3E;;aAGD,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,UAAU,GAAG,UAAU,EAAE;iBAC/C,MAAM,IAAI,KAAK,CAAC,2DAA2D,CAAC,CAAC;cAC9E;;aAGD,IAAI,aAAa,EAAE;;iBAEjB,IAAI,cAAc,EAAE;;qBAElB,KAAK,GAAG,WAAW,CAAC,cAAc,EAAE,aAAa,EAAE,MAAM,CAAC,CAAC;kBAC5D;sBAAM;qBACL,KAAK,GAAG,WAAW,CAAC,cAAc,CAAC,CAAC;kBACrC;iBAED,KAAK,CAAC,KAAK,GAAG,WAAW,CAAC;cAC3B;kBAAM;iBACL,KAAK,GAAG,IAAIwd,SAAI,CAAC,cAAc,EAAE,WAAW,CAAC,CAAC;cAC/C;UACF;cAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;;aAExD,IAAM,UAAU,GACdxd,QAAM,CAAC,KAAK,EAAE,CAAC;kBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;kBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;kBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;aAE1B,IACE,UAAU,IAAI,CAAC;iBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;iBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;iBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;;aAE/C,IAAI,CAAC0d,0BAAY,CAAC1d,QAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,EAAE;iBACxD,MAAM,IAAI,KAAK,CAAC,uCAAuC,CAAC,CAAC;cAC1D;aACD,IAAM,SAAS,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;aAEzE,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;aAG3B,IAAM,SAAS,GAAGuX,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;aACnCvX,QAAM,CAAC,IAAI,CAAC,SAAS,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;aAC7C,IAAM,GAAG,GAAG,IAAIgd,iBAAQ,CAAC,SAAS,CAAC,CAAC;;aAGpC,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;;aAGnB,KAAK,GAAG,IAAIS,YAAK,CAAC,SAAS,EAAE,GAAG,CAAC,CAAC;UACnC;cAAM;aACL,MAAM,IAAI,KAAK,CACb,6BAA6B,GAAG,WAAW,CAAC,QAAQ,CAAC,EAAE,CAAC,GAAG,kBAAkB,GAAG,IAAI,GAAG,GAAG,CAC3F,CAAC;UACH;SACD,IAAI,IAAI,KAAK,WAAW,EAAE;aACxB,MAAM,CAAC,cAAc,CAAC,MAAM,EAAE,IAAI,EAAE;iBAClC,KAAK,OAAA;iBACL,QAAQ,EAAE,IAAI;iBACd,UAAU,EAAE,IAAI;iBAChB,YAAY,EAAE,IAAI;cACnB,CAAC,CAAC;UACJ;cAAM;aACL,MAAM,CAAC,IAAI,CAAC,GAAG,KAAK,CAAC;UACtB;MACF;;KAGD,IAAI,IAAI,KAAK,KAAK,GAAG,UAAU,EAAE;SAC/B,IAAI,OAAO;aAAE,MAAM,IAAI,KAAK,CAAC,oBAAoB,CAAC,CAAC;SACnD,MAAM,IAAI,KAAK,CAAC,qBAAqB,CAAC,CAAC;MACxC;;KAGD,IAAM,UAAU,GAAG,MAAM,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,MAAM,CAAC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,GAAA,CAAC,CAAC;KACtE,IAAI,KAAK,GAAG,IAAI,CAAC;KACjB,UAAU,CAAC,OAAO,CAAC,UAAA,CAAC;SAClB,IAAI,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC;aAAE,KAAK,GAAG,KAAK,CAAC;MAC7D,CAAC,CAAC;;KAGH,IAAI,CAAC,KAAK;SAAE,OAAO,MAAM,CAAC;KAE1B,IAAIA,kBAAW,CAAC,MAAM,CAAC,EAAE;SACvB,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,MAAM,CAAuB,CAAC;SAC7D,OAAO,IAAI,CAAC,IAAI,CAAC;SACjB,OAAO,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO,IAAI,CAAC,GAAG,CAAC;SAChB,OAAO,IAAIA,YAAK,CAAC,MAAM,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;MAC7D;KAED,OAAO,MAAM,CAAC;CAChB,CAAC;CAED;;;;;CAKA,SAAS,WAAW,CAClB,cAAsB,EACtB,aAA4C,EAC5C,MAAiB;KAEjB,IAAI,CAAC,aAAa;SAAE,OAAO,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC;;KAExD,IAAI,aAAa,CAAC,cAAc,CAAC,IAAI,IAAI,EAAE;SACzC,aAAa,CAAC,cAAc,CAAC,GAAG,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC;MAC9D;;KAGD,OAAO,aAAa,CAAC,cAAc,CAAC,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;CACpD,CAAC;;;;;;;;CCxpBD;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;CACA;;;CAIA,SAAgB,WAAW,CACzB,MAAyB,EACzB,MAAc,EACd,MAAwB,EACxB,IAAY,EACZ,MAAc;KAEd,IAAI,CAAS,CAAC;KACd,IAAI,CAAS,CAAC;KACd,IAAM,GAAG,GAAG,MAAM,KAAK,KAAK,CAAC;KAC7B,IAAM,IAAI,GAAG,MAAM,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;KACnC,IAAM,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;KAC7B,IAAM,KAAK,GAAG,IAAI,IAAI,CAAC,CAAC;KACxB,IAAI,KAAK,GAAG,CAAC,CAAC,CAAC;KACf,IAAI,CAAC,GAAG,GAAG,GAAG,CAAC,GAAG,MAAM,GAAG,CAAC,CAAC;KAC7B,IAAM,CAAC,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,CAAC,CAAC;KACvB,IAAI,CAAC,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;KAE3B,CAAC,IAAI,CAAC,CAAC;KAEP,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC,IAAI,CAAC,KAAK,IAAI,CAAC,CAAC,CAAC;KAC5B,CAAC,KAAK,CAAC,KAAK,CAAC;KACb,KAAK,IAAI,IAAI,CAAC;KACd,OAAO,KAAK,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,KAAK,IAAI,CAAC;SAAC,CAAC;KAExE,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC,IAAI,CAAC,KAAK,IAAI,CAAC,CAAC,CAAC;KAC5B,CAAC,KAAK,CAAC,KAAK,CAAC;KACb,KAAK,IAAI,IAAI,CAAC;KACd,OAAO,KAAK,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,KAAK,IAAI,CAAC;SAAC,CAAC;KAExE,IAAI,CAAC,KAAK,CAAC,EAAE;SACX,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;MACf;UAAM,IAAI,CAAC,KAAK,IAAI,EAAE;SACrB,OAAO,CAAC,GAAG,GAAG,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,QAAQ,CAAC;MAC1C;UAAM;SACL,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;SAC1B,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;MACf;KACD,OAAO,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,CAAC;CAClD,CAAC;CAvCD,kCAuCC;CAED,SAAgB,YAAY,CAC1B,MAAyB,EACzB,KAAa,EACb,MAAc,EACd,MAAwB,EACxB,IAAY,EACZ,MAAc;KAEd,IAAI,CAAS,CAAC;KACd,IAAI,CAAS,CAAC;KACd,IAAI,CAAS,CAAC;KACd,IAAM,GAAG,GAAG,MAAM,KAAK,KAAK,CAAC;KAC7B,IAAI,IAAI,GAAG,MAAM,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;KACjC,IAAM,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;KAC7B,IAAM,KAAK,GAAG,IAAI,IAAI,CAAC,CAAC;KACxB,IAAM,EAAE,GAAG,IAAI,KAAK,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,CAAC,CAAC;KACjE,IAAI,CAAC,GAAG,GAAG,GAAG,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;KAC7B,IAAM,CAAC,GAAG,GAAG,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;KACvB,IAAM,CAAC,GAAG,KAAK,GAAG,CAAC,KAAK,KAAK,KAAK,CAAC,IAAI,CAAC,GAAG,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;KAE9D,KAAK,GAAG,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC;KAExB,IAAI,KAAK,CAAC,KAAK,CAAC,IAAI,KAAK,KAAK,QAAQ,EAAE;SACtC,CAAC,GAAG,KAAK,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;SACzB,CAAC,GAAG,IAAI,CAAC;MACV;UAAM;SACL,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC;SAC3C,IAAI,KAAK,IAAI,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,EAAE;aACrC,CAAC,EAAE,CAAC;aACJ,CAAC,IAAI,CAAC,CAAC;UACR;SACD,IAAI,CAAC,GAAG,KAAK,IAAI,CAAC,EAAE;aAClB,KAAK,IAAI,EAAE,GAAG,CAAC,CAAC;UACjB;cAAM;aACL,KAAK,IAAI,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,GAAG,KAAK,CAAC,CAAC;UACtC;SACD,IAAI,KAAK,GAAG,CAAC,IAAI,CAAC,EAAE;aAClB,CAAC,EAAE,CAAC;aACJ,CAAC,IAAI,CAAC,CAAC;UACR;SAED,IAAI,CAAC,GAAG,KAAK,IAAI,IAAI,EAAE;aACrB,CAAC,GAAG,CAAC,CAAC;aACN,CAAC,GAAG,IAAI,CAAC;UACV;cAAM,IAAI,CAAC,GAAG,KAAK,IAAI,CAAC,EAAE;aACzB,CAAC,GAAG,CAAC,KAAK,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;aACxC,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;UACf;cAAM;aACL,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,KAAK,GAAG,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;aACvD,CAAC,GAAG,CAAC,CAAC;UACP;MACF;KAED,IAAI,KAAK,CAAC,KAAK,CAAC;SAAE,CAAC,GAAG,CAAC,CAAC;KAExB,OAAO,IAAI,IAAI,CAAC,EAAE;SAChB,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC;SAC9B,CAAC,IAAI,CAAC,CAAC;SACP,CAAC,IAAI,GAAG,CAAC;SACT,IAAI,IAAI,CAAC,CAAC;MACX;KAED,CAAC,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;KAEpB,IAAI,KAAK,CAAC,KAAK,CAAC;SAAE,CAAC,IAAI,CAAC,CAAC;KAEzB,IAAI,IAAI,IAAI,CAAC;KAEb,OAAO,IAAI,GAAG,CAAC,EAAE;SACf,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC;SAC9B,CAAC,IAAI,CAAC,CAAC;SACP,CAAC,IAAI,GAAG,CAAC;SACT,IAAI,IAAI,CAAC,CAAC;MACX;KAED,MAAM,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC;CACpC,CAAC;CA5ED,oCA4EC;;;;;;;;;;;ACtJkC;AAGO;AAIM;AACF;AACC;AAEhB;AACF;AAYZ;CAgBjB,IAAM,MAAM,GAAG,MAAM,CAAC;CACtB,IAAM,UAAU,GAAG,IAAI,GAAG,CAAC,CAAC,KAAK,EAAE,MAAM,EAAE,KAAK,EAAE,cAAc,CAAC,CAAC,CAAC;CAEnE;;;;;CAMA,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,GAAG,CAAC,CAAC;KACzC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;KAEtB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;KAE/D,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,EAAE,IAAI,IAAI,CAAC;KAC9C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,EAAE,IAAI,IAAI,CAAC;KAC9C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,CAAC,IAAI,IAAI,CAAC;KAC7C,MAAM,CAAC,KAAK,CAAC,GAAG,CAAC,IAAI,GAAG,CAAC,IAAI,IAAI,CAAC;;KAElC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,CAAC;;KAEzB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;;KAIjB,IACE,MAAM,CAAC,SAAS,CAAC,KAAK,CAAC;SACvB,KAAK,IAAI,SAAS,CAAC,cAAc;SACjC,KAAK,IAAI,SAAS,CAAC,cAAc,EACjC;;;SAGA,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;SAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;eACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;eAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;SAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;SACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;SAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC;SAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,CAAC,IAAI,IAAI,CAAC;SACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;SACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;MACxC;UAAM;;SAEL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;SAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;eACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;eAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;SAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;SACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;SAEpBI,yBAAY,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,EAAE,QAAQ,EAAE,EAAE,EAAE,CAAC,CAAC,CAAC;;SAEpD,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;MACnB;KAED,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,CAAU,EAAE,KAAa,EAAE,OAAiB;;KAE9F,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;KAG3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,gBAAgB,CACvB,MAAc,EACd,GAAW,EACX,KAAc,EACd,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;;KAE9C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;KAChC,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,KAAW,EAAE,KAAa,EAAE,OAAiB;;KAE/F,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;KAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAGpB,IAAM,WAAW,GAAG,WAAI,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,CAAC,CAAC;KACrD,IAAM,OAAO,GAAG,WAAW,CAAC,UAAU,EAAE,CAAC;KACzC,IAAM,QAAQ,GAAG,WAAW,CAAC,WAAW,EAAE,CAAC;;KAE3C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,OAAO,GAAG,IAAI,CAAC;KACjC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,CAAC,IAAI,IAAI,CAAC;KACxC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;KACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;;KAEzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;KAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;KACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;KAC1C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;KAC1C,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,IAAI,KAAK,CAAC,MAAM,IAAI,KAAK,CAAC,MAAM,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;SACtD,MAAM,KAAK,CAAC,QAAQ,GAAG,KAAK,CAAC,MAAM,GAAG,8BAA8B,CAAC,CAAC;MACvE;;KAED,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,MAAM,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;KAErE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;KAEvB,IAAI,KAAK,CAAC,UAAU;SAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;KAC7C,IAAI,KAAK,CAAC,MAAM;SAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;KACzC,IAAI,KAAK,CAAC,SAAS;SAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;KAG5C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;KACvB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,mBAAmB,CAC1B,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAGpB,IAAI,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;SAGvC,MAAM,KAAK,CAAC,UAAU,GAAG,KAAK,CAAC,OAAO,GAAG,8BAA8B,CAAC,CAAC;MAC1E;;KAGD,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,OAAO,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;KAEtE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;KAEvB,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;KAEhG,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;KACvB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAsB,EACtB,KAAa,EACb,OAAiB;;KAGjB,IAAI,KAAK,KAAK,IAAI,EAAE;SAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;MAC5C;UAAM,IAAI,KAAK,CAAC,SAAS,KAAK,QAAQ,EAAE;SACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;MAC/C;UAAM;SACL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;MAC/C;;KAGD,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,iBAAiB,CACxB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;KAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAGpB,IAAI,OAAO,KAAK,CAAC,EAAE,KAAK,QAAQ,EAAE;SAChC,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,EAAE,EAAE,KAAK,EAAE,SAAS,EAAE,QAAQ,CAAC,CAAC;MACpD;UAAM,IAAIrG,kBAAY,CAAC,KAAK,CAAC,EAAE,CAAC,EAAE;;;SAGjC,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,QAAQ,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE,KAAK,CAAC,CAAC;MAC7C;UAAM;SACL,MAAM,IAAI,SAAS,CAAC,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,GAAG,2BAA2B,CAAC,CAAC;MACvF;;KAGD,OAAO,KAAK,GAAG,EAAE,CAAC;CACpB,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAA0B,EAC1B,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,IAAM,IAAI,GAAG,KAAK,CAAC,MAAM,CAAC;;KAE1B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;KAEtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,2BAA2B,CAAC;;KAExD,MAAM,CAAC,GAAG,CAACC,0BAAY,CAAC,KAAK,CAAC,EAAE,KAAK,CAAC,CAAC;;KAEvC,KAAK,GAAG,KAAK,GAAG,IAAI,CAAC;KACrB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,SAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,OAAe,EACf,IAAqB;KALrB,0BAAA,EAAA,iBAAiB;KACjB,sBAAA,EAAA,SAAS;KACT,mCAAA,EAAA,0BAA0B;KAC1B,gCAAA,EAAA,sBAAsB;KACtB,wBAAA,EAAA,eAAe;KACf,qBAAA,EAAA,SAAqB;KAErB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;SACpC,IAAI,IAAI,CAAC,CAAC,CAAC,KAAK,KAAK;aAAE,MAAM,IAAI,KAAK,CAAC,4BAA4B,CAAC,CAAC;MACtE;;KAGD,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;;KAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,GAAG,SAAS,CAAC,eAAe,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAEhG,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,IAAM,QAAQ,GAAG,aAAa,CAC5B,MAAM,EACN,KAAK,EACL,SAAS,EACT,KAAK,EACL,KAAK,GAAG,CAAC,EACT,kBAAkB,EAClB,eAAe,EACf,IAAI,CACL,CAAC;;KAEF,IAAI,CAAC,GAAG,EAAE,CAAC;KACX,OAAO,QAAQ,CAAC;CAClB,CAAC;CAED,SAAS,mBAAmB,CAC1B,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;KAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,oBAAoB,CAAC;;KAEjD,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;;;KAIpB,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,KAAK,CAAC,QAAQ,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE,KAAK,CAAC,CAAC;KAC/C,OAAO,KAAK,GAAG,EAAE,CAAC;CACpB,CAAC;CAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,KAAW,EAAE,KAAa,EAAE,OAAiB;;KAE/F,MAAM,CAAC,KAAK,EAAE,CAAC;SACb,KAAK,CAAC,SAAS,KAAK,MAAM,GAAG,SAAS,CAAC,cAAc,GAAG,SAAS,CAAC,mBAAmB,CAAC;;KAExF,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,IAAM,OAAO,GAAG,KAAK,CAAC,UAAU,EAAE,CAAC;KACnC,IAAM,QAAQ,GAAG,KAAK,CAAC,WAAW,EAAE,CAAC;;KAErC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,OAAO,GAAG,IAAI,CAAC;KACjC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,CAAC,IAAI,IAAI,CAAC;KACxC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;KACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;;KAEzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;KAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;KACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;KAC1C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;KAC1C,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,cAAc,CACrB,MAAc,EACd,GAAW,EACX,KAAqB,EACrB,KAAa,EACb,OAAiB;KAEjB,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;;KAExB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;KAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC;KAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,CAAC,IAAI,IAAI,CAAC;KACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;KACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;KACvC,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAG7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAGpBoG,yBAAY,CAAC,MAAM,EAAE,KAAK,CAAC,KAAK,EAAE,KAAK,EAAE,QAAQ,EAAE,EAAE,EAAE,CAAC,CAAC,CAAC;;KAG1D,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;KAClB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,iBAAiB,CACxB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,UAAkB,EAClB,MAAU,EACV,OAAiB;KAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;KAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,IAAM,cAAc,GAAGrG,8BAAwB,CAAC,KAAK,CAAC,CAAC;;KAGvD,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;KAE5E,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;KAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;KAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACpB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,aAAa,CACpB,MAAc,EACd,GAAW,EACX,KAAW,EACX,KAAa,EACb,SAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,OAAe;KAJf,0BAAA,EAAA,iBAAiB;KACjB,sBAAA,EAAA,SAAS;KACT,mCAAA,EAAA,0BAA0B;KAC1B,gCAAA,EAAA,sBAAsB;KACtB,wBAAA,EAAA,eAAe;KAEf,IAAI,KAAK,CAAC,KAAK,IAAI,OAAO,KAAK,CAAC,KAAK,KAAK,QAAQ,EAAE;;SAElD,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,sBAAsB,CAAC;;SAEnD,IAAM,oBAAoB,GAAG,CAAC,OAAO;eACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;eAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;SAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;SACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;SAGpB,IAAI,UAAU,GAAG,KAAK,CAAC;;;SAIvB,IAAM,cAAc,GAAG,OAAO,KAAK,CAAC,IAAI,KAAK,QAAQ,GAAG,KAAK,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC;;SAE3F,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;;SAElB,IAAM,QAAQ,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;SAEhF,MAAM,CAAC,KAAK,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;SAChC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;SAC3C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;SAC5C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;;SAE5C,MAAM,CAAC,KAAK,GAAG,CAAC,GAAG,QAAQ,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;SAErC,KAAK,GAAG,KAAK,GAAG,QAAQ,GAAG,CAAC,CAAC;;;SAI7B,IAAM,QAAQ,GAAG,aAAa,CAC5B,MAAM,EACN,KAAK,CAAC,KAAK,EACX,SAAS,EACT,KAAK,EACL,KAAK,GAAG,CAAC,EACT,kBAAkB,EAClB,eAAe,CAChB,CAAC;SACF,KAAK,GAAG,QAAQ,GAAG,CAAC,CAAC;;SAGrB,IAAM,SAAS,GAAG,QAAQ,GAAG,UAAU,CAAC;;SAGxC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,SAAS,GAAG,IAAI,CAAC;SACxC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,CAAC,IAAI,IAAI,CAAC;SAC/C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,EAAE,IAAI,IAAI,CAAC;SAChD,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,EAAE,IAAI,IAAI,CAAC;;SAEhD,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;MACrB;UAAM;SACL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;SAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;eACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;eAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;SAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;SACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;SAEpB,IAAM,cAAc,GAAG,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC;;SAE7C,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;SAE5E,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;SAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;SACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;SACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;SAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;SAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;MACrB;KAED,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,IAAM,IAAI,GAAG,KAAK,CAAC,KAAK,CAAC,IAAI,CAAwB,CAAC;;KAEtD,IAAI,IAAI,GAAG,KAAK,CAAC,QAAQ,CAAC;;KAE1B,IAAI,KAAK,CAAC,QAAQ,KAAKG,aAAM,CAAC,kBAAkB;SAAE,IAAI,GAAG,IAAI,GAAG,CAAC,CAAC;;KAElE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;KAEtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,QAAQ,CAAC;;KAGjC,IAAI,KAAK,CAAC,QAAQ,KAAKA,aAAM,CAAC,kBAAkB,EAAE;SAChD,IAAI,GAAG,IAAI,GAAG,CAAC,CAAC;SAChB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;SAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;SACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;SACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;MACvC;;KAGD,MAAM,CAAC,GAAG,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;;KAExB,KAAK,GAAG,KAAK,GAAG,KAAK,CAAC,QAAQ,CAAC;KAC/B,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;KAEpB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;KAEzE,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;KAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;KAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;KACvB,OAAO,KAAK,CAAC;CACf,CAAC;CAED,SAAS,cAAc,CACrB,MAAc,EACd,GAAW,EACX,KAAY,EACZ,KAAa,EACb,KAAa,EACb,kBAA2B,EAC3B,OAAiB;;KAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;KAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;WACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;WAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;KAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;KACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KAEpB,IAAI,UAAU,GAAG,KAAK,CAAC;KACvB,IAAI,MAAM,GAAc;SACtB,IAAI,EAAE,KAAK,CAAC,UAAU,IAAI,KAAK,CAAC,SAAS;SACzC,GAAG,EAAE,KAAK,CAAC,GAAG;MACf,CAAC;KAEF,IAAI,KAAK,CAAC,EAAE,IAAI,IAAI,EAAE;SACpB,MAAM,CAAC,GAAG,GAAG,KAAK,CAAC,EAAE,CAAC;MACvB;KAED,MAAM,GAAG,MAAM,CAAC,MAAM,CAAC,MAAM,EAAE,KAAK,CAAC,MAAM,CAAC,CAAC;KAC7C,IAAM,QAAQ,GAAG,aAAa,CAAC,MAAM,EAAE,MAAM,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,kBAAkB,CAAC,CAAC;;KAG5F,IAAM,IAAI,GAAG,QAAQ,GAAG,UAAU,CAAC;;KAEnC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KACnC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KAC1C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KAC3C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;KAE3C,OAAO,QAAQ,CAAC;CAClB,CAAC;CAED,SAAgB,aAAa,CAC3B,MAAc,EACd,MAAgB,EAChB,SAAiB,EACjB,aAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,IAAqB;KALrB,0BAAA,EAAA,iBAAiB;KACjB,8BAAA,EAAA,iBAAiB;KACjB,sBAAA,EAAA,SAAS;KACT,mCAAA,EAAA,0BAA0B;KAC1B,gCAAA,EAAA,sBAAsB;KACtB,qBAAA,EAAA,SAAqB;KAErB,aAAa,GAAG,aAAa,IAAI,CAAC,CAAC;KACnC,IAAI,GAAG,IAAI,IAAI,EAAE,CAAC;;KAGlB,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;;KAGlB,IAAI,KAAK,GAAG,aAAa,GAAG,CAAC,CAAC;;KAG9B,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;;SAEzB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;aACtC,IAAM,GAAG,GAAG,EAAE,GAAG,CAAC,CAAC;aACnB,IAAI,KAAK,GAAG,MAAM,CAAC,CAAC,CAAC,CAAC;;aAGtB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;iBACzB,IAAI,OAAO,KAAK,CAAC,MAAM,KAAK,UAAU;qBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;iBACxF,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;cACxB;aAED,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;iBAC7B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;iBACpC,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;iBACpC,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;cACvE;kBAAM,IAAI,OAAO,KAAK,KAAK,SAAS,EAAE;iBACrC,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC3D;kBAAM,IAAI,KAAK,YAAY,IAAI,IAAIH,YAAM,CAAC,KAAK,CAAC,EAAE;iBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,KAAK,SAAS,EAAE;iBAC9B,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,KAAK,IAAI,EAAE;iBACzB,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;iBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC5D;kBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;iBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;iBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;iBAClE,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,IAAI,EACJ,IAAI,CACL,CAAC;cACH;kBAAM,IACL,OAAO,KAAK,KAAK,QAAQ;iBACzBsG,wBAAU,CAAC,KAAK,CAAC;iBACjB,KAAK,CAAC,SAAS,KAAK,YAAY,EAChC;iBACA,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC9D;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;iBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC9E;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;iBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,IAAI,CACL,CAAC;cACH;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,EAAE,IAAI,CAAC,CAAC;cACpF;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC9D;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cACzD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;cAC1D;kBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;cACjF;UACF;MACF;UAAM,IAAI,MAAM,YAAYC,OAAG,IAAIvG,WAAK,CAAC,MAAM,CAAC,EAAE;SACjD,IAAM,QAAQ,GAAG,MAAM,CAAC,OAAO,EAAE,CAAC;SAClC,IAAI,IAAI,GAAG,KAAK,CAAC;SAEjB,OAAO,CAAC,IAAI,EAAE;;aAEZ,IAAM,KAAK,GAAG,QAAQ,CAAC,IAAI,EAAE,CAAC;aAC9B,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC,IAAI,CAAC;;aAEpB,IAAI,IAAI;iBAAE,SAAS;;aAGnB,IAAM,GAAG,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;aAC3B,IAAM,KAAK,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;;aAG7B,IAAM,IAAI,GAAG,OAAO,KAAK,CAAC;;aAG1B,IAAI,OAAO,GAAG,KAAK,QAAQ,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;iBACnD,IAAI,GAAG,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;qBAG7B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,8BAA8B,CAAC,CAAC;kBAC5D;iBAED,IAAI,SAAS,EAAE;qBACb,IAAI,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,EAAE;yBAClB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,0BAA0B,CAAC,CAAC;sBACxD;0BAAM,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;yBAC5B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,uBAAuB,CAAC,CAAC;sBACrD;kBACF;cACF;aAED,IAAI,IAAI,KAAK,QAAQ,EAAE;iBACrB,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;iBAC5B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,IAAIA,qBAAe,CAAC,KAAK,CAAC,IAAIA,sBAAgB,CAAC,KAAK,CAAC,EAAE;iBACjF,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;cACvE;kBAAM,IAAI,IAAI,KAAK,SAAS,EAAE;iBAC7B,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACrD;kBAAM,IAAI,KAAK,YAAY,IAAI,IAAIA,YAAM,CAAC,KAAK,CAAC,EAAE;iBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,KAAK,IAAI,KAAK,KAAK,KAAK,SAAS,IAAI,eAAe,KAAK,KAAK,CAAC,EAAE;iBAC/E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;iBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACtD;kBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;iBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;iBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;iBAC1D,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,KAAK,EACL,IAAI,CACL,CAAC;cACH;kBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBACnE,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;iBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,CAChB,CAAC;cACH;kBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;iBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;cAC5F;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;cAC9E;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACnD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;cACjF;UACF;MACF;UAAM;;SAEL,IAAI,MAAM,CAAC,MAAM,EAAE;aACjB,IAAI,OAAO,MAAM,CAAC,MAAM,KAAK,UAAU;iBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;aACzF,MAAM,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC;aACzB,IAAI,MAAM,IAAI,IAAI,IAAI,OAAO,MAAM,KAAK,QAAQ;iBAC9C,MAAM,IAAI,SAAS,CAAC,0CAA0C,CAAC,CAAC;UACnE;;SAGD,KAAK,IAAM,GAAG,IAAI,MAAM,EAAE;aACxB,IAAI,KAAK,GAAG,MAAM,CAAC,GAAG,CAAC,CAAC;;aAExB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;iBACzB,IAAI,OAAO,KAAK,CAAC,MAAM,KAAK,UAAU;qBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;iBACxF,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;cACxB;;aAGD,IAAM,IAAI,GAAG,OAAO,KAAK,CAAC;;aAG1B,IAAI,OAAO,GAAG,KAAK,QAAQ,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;iBACnD,IAAI,GAAG,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;qBAG7B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,8BAA8B,CAAC,CAAC;kBAC5D;iBAED,IAAI,SAAS,EAAE;qBACb,IAAI,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,EAAE;yBAClB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,0BAA0B,CAAC,CAAC;sBACxD;0BAAM,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;yBAC5B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,uBAAuB,CAAC,CAAC;sBACrD;kBACF;cACF;aAED,IAAI,IAAI,KAAK,QAAQ,EAAE;iBACrB,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;iBAC5B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;iBAC5B,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;cACvE;kBAAM,IAAI,IAAI,KAAK,SAAS,EAAE;iBAC7B,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACrD;kBAAM,IAAI,KAAK,YAAY,IAAI,IAAIA,YAAM,CAAC,KAAK,CAAC,EAAE;iBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,KAAK,SAAS,EAAE;iBAC9B,IAAI,eAAe,KAAK,KAAK;qBAAE,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACjF;kBAAM,IAAI,KAAK,KAAK,IAAI,EAAE;iBACzB,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;iBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACtD;kBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;iBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;iBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;iBAC1D,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,KAAK,EACL,IAAI,CACL,CAAC;cACH;kBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBACnE,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cAClD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;iBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,CAChB,CAAC;cACH;kBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;iBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;cAC5F;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;cAC9E;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;iBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACxD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;iBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACnD;kBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;iBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;cACpD;kBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;iBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;cACjF;UACF;MACF;;KAGD,IAAI,CAAC,GAAG,EAAE,CAAC;;KAGX,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;KAGvB,IAAM,IAAI,GAAG,KAAK,GAAG,aAAa,CAAC;;KAEnC,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;KACtC,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;KAC7C,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KAC9C,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KAC9C,OAAO,KAAK,CAAC;CACf,CAAC;CAxUD,sCAwUC;;;;;;;;;;;AC7iC+B;AACE;CAiFhC,uFAjFOG,aAAM,OAiFP;AAhFsB;CA4E5B,qFA5EO6F,SAAI,OA4EP;AA3E2B;CA8E/B,sFA9EOC,YAAK,OA8EP;AA7EmC;CAwFxC,2FAxFON,qBAAU,OAwFP;AAvFsB;CAkFhC,uFAlFO,eAAM,OAkFP;AAjFuC;AACP;AACP;CAgF/B,sFAhFOD,YAAK,OAgFP;AA/EuB;CA4E5B,qFA5EO,WAAI,OA4EP;AA3EsB;CAqE1B,oFArEOa,OAAG,OAqEP;AApE8B;CA+EjC,uFA/EOV,cAAM,OA+EP;AA9E2B;CA6EjC,uFA7EOD,cAAM,OA6EP;AA5E8B;CAsEpC,yFAtEOJ,iBAAQ,OAsEP;CAaI,yFAnFLA,iBAAQ,OAmFK;AAlFuE;CAC7F;AAC+F;AACJ;AACrD;CAyEpC,2FAzEOM,iBAAU,OAyEP;AAxE0B;CA6DpC,2FA7DOL,iBAAU,OA6DP;AA5D4B;CAkEtC,0FAlEOM,mBAAS,OAkEP;AAjEmB;CA+D5B,qFA/DO3F,SAAI,OA+DP;AA3Be;CAhCnB,yHAAA,8BAA8B,OAAA;CAC9B,sHAAA,2BAA2B,OAAA;CAC3B,uHAAA,4BAA4B,OAAA;CAC5B,kHAAA,uBAAuB,OAAA;CACvB,2HAAA,gCAAgC,OAAA;CAChC,mHAAA,wBAAwB,OAAA;CACxB,uHAAA,4BAA4B,OAAA;CAC5B,0GAAA,eAAe,OAAA;CACf,2GAAA,gBAAgB,OAAA;CAChB,4GAAA,iBAAiB,OAAA;CACjB,yGAAA,cAAc,OAAA;CACd,iHAAA,sBAAsB,OAAA;CACtB,yGAAA,cAAc,OAAA;CACd,8GAAA,mBAAmB,OAAA;CACnB,+GAAA,oBAAoB,OAAA;CACpB,wGAAA,aAAa,OAAA;CACb,yGAAA,cAAc,OAAA;CACd,4GAAA,iBAAiB,OAAA;CACjB,4GAAA,iBAAiB,OAAA;CACjB,yGAAA,cAAc,OAAA;CACd,2GAAA,gBAAgB,OAAA;CAChB,2GAAA,gBAAgB,OAAA;CAChB,wGAAA,aAAa,OAAA;CACb,2GAAA,gBAAgB,OAAA;CAChB,2GAAA,gBAAgB,OAAA;CAChB,2GAAA,gBAAgB,OAAA;CAChB,8GAAA,mBAAmB,OAAA;CACnB,8GAAA,mBAAmB,OAAA;CACnB,yGAAA,cAAc,OAAA;CACd,yGAAA,cAAc,OAAA;CACd,yGAAA,cAAc,OAAA;CACd,yGAAA,cAAc,OAAA;CAKhB,oCAAsD;CAA7C,sGAAA,KAAK,OAAA;CAQd,4BAKqB;CAHnB,sHAAA,yBAAyB,OAAA;CAkC3B;CACA;CACA,IAAM,OAAO,GAAG,IAAI,GAAG,IAAI,GAAG,EAAE,CAAC;CAEjC;CACA,IAAI5X,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC;CAEnC;;;;;;CAMA,SAAgB,qBAAqB,CAAC,IAAY;;KAEhD,IAAIvX,QAAM,CAAC,MAAM,GAAG,IAAI,EAAE;SACxBA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;MAC7B;CACH,CAAC;CALD,sDAKC;CAED;;;;;;;CAOA,SAAgB,SAAS,CAAC,MAAgB,EAAE,OAA8B;KAA9B,wBAAA,EAAA,YAA8B;;KAExE,IAAM,SAAS,GAAG,OAAO,OAAO,CAAC,SAAS,KAAK,SAAS,GAAG,OAAO,CAAC,SAAS,GAAG,KAAK,CAAC;KACrF,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;KACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;KAChF,IAAM,qBAAqB,GACzB,OAAO,OAAO,CAAC,qBAAqB,KAAK,QAAQ,GAAG,OAAO,CAAC,qBAAqB,GAAG,OAAO,CAAC;;KAG9F,IAAIvX,QAAM,CAAC,MAAM,GAAG,qBAAqB,EAAE;SACzCA,QAAM,GAAGuX,aAAM,CAAC,KAAK,CAAC,qBAAqB,CAAC,CAAC;MAC9C;;KAGD,IAAM,kBAAkB,GAAGyG,wBAAiB,CAC1Che,QAAM,EACN,MAAM,EACN,SAAS,EACT,CAAC,EACD,CAAC,EACD,kBAAkB,EAClB,eAAe,EACf,EAAE,CACH,CAAC;;KAGF,IAAM,cAAc,GAAGuX,aAAM,CAAC,KAAK,CAAC,kBAAkB,CAAC,CAAC;;KAGxDvX,QAAM,CAAC,IAAI,CAAC,cAAc,EAAE,CAAC,EAAE,CAAC,EAAE,cAAc,CAAC,MAAM,CAAC,CAAC;;KAGzD,OAAO,cAAc,CAAC;CACxB,CAAC;CAnCD,8BAmCC;CAED;;;;;;;;;CASA,SAAgB,2BAA2B,CACzC,MAAgB,EAChB,WAAmB,EACnB,OAA8B;KAA9B,wBAAA,EAAA,YAA8B;;KAG9B,IAAM,SAAS,GAAG,OAAO,OAAO,CAAC,SAAS,KAAK,SAAS,GAAG,OAAO,CAAC,SAAS,GAAG,KAAK,CAAC;KACrF,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;KACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;KAChF,IAAM,UAAU,GAAG,OAAO,OAAO,CAAC,KAAK,KAAK,QAAQ,GAAG,OAAO,CAAC,KAAK,GAAG,CAAC,CAAC;;KAGzE,IAAM,kBAAkB,GAAGge,wBAAiB,CAC1Che,QAAM,EACN,MAAM,EACN,SAAS,EACT,CAAC,EACD,CAAC,EACD,kBAAkB,EAClB,eAAe,CAChB,CAAC;KACFA,QAAM,CAAC,IAAI,CAAC,WAAW,EAAE,UAAU,EAAE,CAAC,EAAE,kBAAkB,CAAC,CAAC;;KAG5D,OAAO,UAAU,GAAG,kBAAkB,GAAG,CAAC,CAAC;CAC7C,CAAC;CA3BD,kEA2BC;CAED;;;;;;;CAOA,SAAgB,WAAW,CACzB,MAA8C,EAC9C,OAAgC;KAAhC,wBAAA,EAAA,YAAgC;KAEhC,OAAOie,wBAAmB,CAACxG,0BAAY,CAAC,MAAM,CAAC,EAAE,OAAO,CAAC,CAAC;CAC5D,CAAC;CALD,kCAKC;CAQD;;;;;;;CAOA,SAAgB,mBAAmB,CACjC,MAAgB,EAChB,OAAwC;KAAxC,wBAAA,EAAA,YAAwC;KAExC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;KAExB,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;KACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;KAEhF,OAAOyG,kCAA2B,CAAC,MAAM,EAAE,kBAAkB,EAAE,eAAe,CAAC,CAAC;CAClF,CAAC;CAZD,kDAYC;CAED;;;;;;;;;;;;CAYA,SAAgB,iBAAiB,CAC/B,IAA4C,EAC5C,UAAkB,EAClB,iBAAyB,EACzB,SAAqB,EACrB,aAAqB,EACrB,OAA2B;KAE3B,IAAM,eAAe,GAAG,MAAM,CAAC,MAAM,CACnC,EAAE,gCAAgC,EAAE,IAAI,EAAE,KAAK,EAAE,CAAC,EAAE,EACpD,OAAO,CACR,CAAC;KACF,IAAM,UAAU,GAAGzG,0BAAY,CAAC,IAAI,CAAC,CAAC;KAEtC,IAAI,KAAK,GAAG,UAAU,CAAC;;KAEvB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,iBAAiB,EAAE,CAAC,EAAE,EAAE;;SAE1C,IAAM,IAAI,GACR,UAAU,CAAC,KAAK,CAAC;cAChB,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;cAC3B,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;cAC5B,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;;SAEhC,eAAe,CAAC,KAAK,GAAG,KAAK,CAAC;;SAE9B,SAAS,CAAC,aAAa,GAAG,CAAC,CAAC,GAAGwG,wBAAmB,CAAC,UAAU,EAAE,eAAe,CAAC,CAAC;;SAEhF,KAAK,GAAG,KAAK,GAAG,IAAI,CAAC;MACtB;;KAGD,OAAO,KAAK,CAAC;CACf,CAAC;CAjCD,8CAiCC;CAED;;;;;;;;CAQA,IAAM,IAAI,GAAG;KACX,MAAM,eAAA;KACN,IAAI,WAAA;KACJ,KAAK,cAAA;KACL,UAAU,uBAAA;KACV,MAAM,iBAAA;KACN,KAAK,cAAA;KACL,IAAI,aAAA;KACJ,IAAI,WAAA;KACJ,GAAG,SAAA;KACH,MAAM,gBAAA;KACN,MAAM,gBAAA;KACN,QAAQ,mBAAA;KACR,QAAQ,EAAEjB,iBAAQ;KAClB,UAAU,mBAAA;KACV,UAAU,mBAAA;KACV,SAAS,qBAAA;KACT,KAAK,qBAAA;KACL,qBAAqB,uBAAA;KACrB,SAAS,WAAA;KACT,2BAA2B,6BAAA;KAC3B,WAAW,aAAA;KACX,mBAAmB,qBAAA;KACnB,iBAAiB,mBAAA;EAClB,CAAC;CACF,kBAAe,IAAI,CAAC;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;"}
\ No newline at end of file
diff --git a/node_modules/bson/dist/bson.esm.js b/node_modules/bson/dist/bson.esm.js
new file mode 100644
index 0000000..0e5d452
--- /dev/null
+++ b/node_modules/bson/dist/bson.esm.js
@@ -0,0 +1,6925 @@
+import buffer from 'buffer';
+
+var commonjsGlobal = typeof globalThis !== 'undefined' ? globalThis : typeof window !== 'undefined' ? window : typeof global !== 'undefined' ? global : typeof self !== 'undefined' ? self : {};
+
+function unwrapExports (x) {
+ return x && x.__esModule && Object.prototype.hasOwnProperty.call(x, 'default') ? x['default'] : x;
+}
+
+function createCommonjsModule(fn, module) {
+ return module = { exports: {} }, fn(module, module.exports), module.exports;
+}
+
+var require$$0 = {};
+
+// shim for using process in browser
+
+var performance = global.performance || {};
+
+performance.now || performance.mozNow || performance.msNow || performance.oNow || performance.webkitNow || function () {
+ return new Date().getTime();
+}; // generate timestamp or delta
+
+var inherits;
+
+if (typeof Object.create === 'function') {
+ inherits = function inherits(ctor, superCtor) {
+ // implementation from standard node.js 'util' module
+ ctor.super_ = superCtor;
+ ctor.prototype = Object.create(superCtor.prototype, {
+ constructor: {
+ value: ctor,
+ enumerable: false,
+ writable: true,
+ configurable: true
+ }
+ });
+ };
+} else {
+ inherits = function inherits(ctor, superCtor) {
+ ctor.super_ = superCtor;
+
+ var TempCtor = function TempCtor() {};
+
+ TempCtor.prototype = superCtor.prototype;
+ ctor.prototype = new TempCtor();
+ ctor.prototype.constructor = ctor;
+ };
+}
+
+var inherits$1 = inherits;
+
+// Copyright Joyent, Inc. and other Node contributors.
+var formatRegExp = /%[sdj%]/g;
+function format(f) {
+ if (!isString(f)) {
+ var objects = [];
+
+ for (var i = 0; i < arguments.length; i++) {
+ objects.push(inspect(arguments[i]));
+ }
+
+ return objects.join(' ');
+ }
+
+ var i = 1;
+ var args = arguments;
+ var len = args.length;
+ var str = String(f).replace(formatRegExp, function (x) {
+ if (x === '%%') return '%';
+ if (i >= len) return x;
+
+ switch (x) {
+ case '%s':
+ return String(args[i++]);
+
+ case '%d':
+ return Number(args[i++]);
+
+ case '%j':
+ try {
+ return JSON.stringify(args[i++]);
+ } catch (_) {
+ return '[Circular]';
+ }
+
+ default:
+ return x;
+ }
+ });
+
+ for (var x = args[i]; i < len; x = args[++i]) {
+ if (isNull(x) || !isObject(x)) {
+ str += ' ' + x;
+ } else {
+ str += ' ' + inspect(x);
+ }
+ }
+
+ return str;
+}
+// Returns a modified function which warns once by default.
+// If --no-deprecation is set, then it is a no-op.
+
+function deprecate(fn, msg) {
+ // Allow for deprecating things in the process of starting up.
+ if (isUndefined(global.process)) {
+ return function () {
+ return deprecate(fn, msg).apply(this, arguments);
+ };
+ }
+
+ var warned = false;
+
+ function deprecated() {
+ if (!warned) {
+ {
+ console.error(msg);
+ }
+
+ warned = true;
+ }
+
+ return fn.apply(this, arguments);
+ }
+
+ return deprecated;
+}
+var debugs = {};
+var debugEnviron;
+function debuglog(set) {
+ if (isUndefined(debugEnviron)) debugEnviron = '';
+ set = set.toUpperCase();
+
+ if (!debugs[set]) {
+ if (new RegExp('\\b' + set + '\\b', 'i').test(debugEnviron)) {
+ var pid = 0;
+
+ debugs[set] = function () {
+ var msg = format.apply(null, arguments);
+ console.error('%s %d: %s', set, pid, msg);
+ };
+ } else {
+ debugs[set] = function () {};
+ }
+ }
+
+ return debugs[set];
+}
+/**
+ * Echos the value of a value. Trys to print the value out
+ * in the best way possible given the different types.
+ *
+ * @param {Object} obj The object to print out.
+ * @param {Object} opts Optional options object that alters the output.
+ */
+
+/* legacy: obj, showHidden, depth, colors*/
+
+function inspect(obj, opts) {
+ // default options
+ var ctx = {
+ seen: [],
+ stylize: stylizeNoColor
+ }; // legacy...
+
+ if (arguments.length >= 3) ctx.depth = arguments[2];
+ if (arguments.length >= 4) ctx.colors = arguments[3];
+
+ if (isBoolean(opts)) {
+ // legacy...
+ ctx.showHidden = opts;
+ } else if (opts) {
+ // got an "options" object
+ _extend(ctx, opts);
+ } // set default options
+
+
+ if (isUndefined(ctx.showHidden)) ctx.showHidden = false;
+ if (isUndefined(ctx.depth)) ctx.depth = 2;
+ if (isUndefined(ctx.colors)) ctx.colors = false;
+ if (isUndefined(ctx.customInspect)) ctx.customInspect = true;
+ if (ctx.colors) ctx.stylize = stylizeWithColor;
+ return formatValue(ctx, obj, ctx.depth);
+} // http://en.wikipedia.org/wiki/ANSI_escape_code#graphics
+
+inspect.colors = {
+ 'bold': [1, 22],
+ 'italic': [3, 23],
+ 'underline': [4, 24],
+ 'inverse': [7, 27],
+ 'white': [37, 39],
+ 'grey': [90, 39],
+ 'black': [30, 39],
+ 'blue': [34, 39],
+ 'cyan': [36, 39],
+ 'green': [32, 39],
+ 'magenta': [35, 39],
+ 'red': [31, 39],
+ 'yellow': [33, 39]
+}; // Don't use 'blue' not visible on cmd.exe
+
+inspect.styles = {
+ 'special': 'cyan',
+ 'number': 'yellow',
+ 'boolean': 'yellow',
+ 'undefined': 'grey',
+ 'null': 'bold',
+ 'string': 'green',
+ 'date': 'magenta',
+ // "name": intentionally not styling
+ 'regexp': 'red'
+};
+
+function stylizeWithColor(str, styleType) {
+ var style = inspect.styles[styleType];
+
+ if (style) {
+ return "\x1B[" + inspect.colors[style][0] + 'm' + str + "\x1B[" + inspect.colors[style][1] + 'm';
+ } else {
+ return str;
+ }
+}
+
+function stylizeNoColor(str, styleType) {
+ return str;
+}
+
+function arrayToHash(array) {
+ var hash = {};
+ array.forEach(function (val, idx) {
+ hash[val] = true;
+ });
+ return hash;
+}
+
+function formatValue(ctx, value, recurseTimes) {
+ // Provide a hook for user-specified inspect functions.
+ // Check that value is an object with an inspect function on it
+ if (ctx.customInspect && value && isFunction(value.inspect) && // Filter out the util module, it's inspect function is special
+ value.inspect !== inspect && // Also filter out any prototype objects using the circular check.
+ !(value.constructor && value.constructor.prototype === value)) {
+ var ret = value.inspect(recurseTimes, ctx);
+
+ if (!isString(ret)) {
+ ret = formatValue(ctx, ret, recurseTimes);
+ }
+
+ return ret;
+ } // Primitive types cannot have properties
+
+
+ var primitive = formatPrimitive(ctx, value);
+
+ if (primitive) {
+ return primitive;
+ } // Look up the keys of the object.
+
+
+ var keys = Object.keys(value);
+ var visibleKeys = arrayToHash(keys);
+
+ if (ctx.showHidden) {
+ keys = Object.getOwnPropertyNames(value);
+ } // IE doesn't make error fields non-enumerable
+ // http://msdn.microsoft.com/en-us/library/ie/dww52sbt(v=vs.94).aspx
+
+
+ if (isError(value) && (keys.indexOf('message') >= 0 || keys.indexOf('description') >= 0)) {
+ return formatError(value);
+ } // Some type of object without properties can be shortcutted.
+
+
+ if (keys.length === 0) {
+ if (isFunction(value)) {
+ var name = value.name ? ': ' + value.name : '';
+ return ctx.stylize('[Function' + name + ']', 'special');
+ }
+
+ if (isRegExp(value)) {
+ return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
+ }
+
+ if (isDate(value)) {
+ return ctx.stylize(Date.prototype.toString.call(value), 'date');
+ }
+
+ if (isError(value)) {
+ return formatError(value);
+ }
+ }
+
+ var base = '',
+ array = false,
+ braces = ['{', '}']; // Make Array say that they are Array
+
+ if (isArray(value)) {
+ array = true;
+ braces = ['[', ']'];
+ } // Make functions say that they are functions
+
+
+ if (isFunction(value)) {
+ var n = value.name ? ': ' + value.name : '';
+ base = ' [Function' + n + ']';
+ } // Make RegExps say that they are RegExps
+
+
+ if (isRegExp(value)) {
+ base = ' ' + RegExp.prototype.toString.call(value);
+ } // Make dates with properties first say the date
+
+
+ if (isDate(value)) {
+ base = ' ' + Date.prototype.toUTCString.call(value);
+ } // Make error with message first say the error
+
+
+ if (isError(value)) {
+ base = ' ' + formatError(value);
+ }
+
+ if (keys.length === 0 && (!array || value.length == 0)) {
+ return braces[0] + base + braces[1];
+ }
+
+ if (recurseTimes < 0) {
+ if (isRegExp(value)) {
+ return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
+ } else {
+ return ctx.stylize('[Object]', 'special');
+ }
+ }
+
+ ctx.seen.push(value);
+ var output;
+
+ if (array) {
+ output = formatArray(ctx, value, recurseTimes, visibleKeys, keys);
+ } else {
+ output = keys.map(function (key) {
+ return formatProperty(ctx, value, recurseTimes, visibleKeys, key, array);
+ });
+ }
+
+ ctx.seen.pop();
+ return reduceToSingleString(output, base, braces);
+}
+
+function formatPrimitive(ctx, value) {
+ if (isUndefined(value)) return ctx.stylize('undefined', 'undefined');
+
+ if (isString(value)) {
+ var simple = '\'' + JSON.stringify(value).replace(/^"|"$/g, '').replace(/'/g, "\\'").replace(/\\"/g, '"') + '\'';
+ return ctx.stylize(simple, 'string');
+ }
+
+ if (isNumber(value)) return ctx.stylize('' + value, 'number');
+ if (isBoolean(value)) return ctx.stylize('' + value, 'boolean'); // For some reason typeof null is "object", so special case here.
+
+ if (isNull(value)) return ctx.stylize('null', 'null');
+}
+
+function formatError(value) {
+ return '[' + Error.prototype.toString.call(value) + ']';
+}
+
+function formatArray(ctx, value, recurseTimes, visibleKeys, keys) {
+ var output = [];
+
+ for (var i = 0, l = value.length; i < l; ++i) {
+ if (hasOwnProperty(value, String(i))) {
+ output.push(formatProperty(ctx, value, recurseTimes, visibleKeys, String(i), true));
+ } else {
+ output.push('');
+ }
+ }
+
+ keys.forEach(function (key) {
+ if (!key.match(/^\d+$/)) {
+ output.push(formatProperty(ctx, value, recurseTimes, visibleKeys, key, true));
+ }
+ });
+ return output;
+}
+
+function formatProperty(ctx, value, recurseTimes, visibleKeys, key, array) {
+ var name, str, desc;
+ desc = Object.getOwnPropertyDescriptor(value, key) || {
+ value: value[key]
+ };
+
+ if (desc.get) {
+ if (desc.set) {
+ str = ctx.stylize('[Getter/Setter]', 'special');
+ } else {
+ str = ctx.stylize('[Getter]', 'special');
+ }
+ } else {
+ if (desc.set) {
+ str = ctx.stylize('[Setter]', 'special');
+ }
+ }
+
+ if (!hasOwnProperty(visibleKeys, key)) {
+ name = '[' + key + ']';
+ }
+
+ if (!str) {
+ if (ctx.seen.indexOf(desc.value) < 0) {
+ if (isNull(recurseTimes)) {
+ str = formatValue(ctx, desc.value, null);
+ } else {
+ str = formatValue(ctx, desc.value, recurseTimes - 1);
+ }
+
+ if (str.indexOf('\n') > -1) {
+ if (array) {
+ str = str.split('\n').map(function (line) {
+ return ' ' + line;
+ }).join('\n').substr(2);
+ } else {
+ str = '\n' + str.split('\n').map(function (line) {
+ return ' ' + line;
+ }).join('\n');
+ }
+ }
+ } else {
+ str = ctx.stylize('[Circular]', 'special');
+ }
+ }
+
+ if (isUndefined(name)) {
+ if (array && key.match(/^\d+$/)) {
+ return str;
+ }
+
+ name = JSON.stringify('' + key);
+
+ if (name.match(/^"([a-zA-Z_][a-zA-Z_0-9]*)"$/)) {
+ name = name.substr(1, name.length - 2);
+ name = ctx.stylize(name, 'name');
+ } else {
+ name = name.replace(/'/g, "\\'").replace(/\\"/g, '"').replace(/(^"|"$)/g, "'");
+ name = ctx.stylize(name, 'string');
+ }
+ }
+
+ return name + ': ' + str;
+}
+
+function reduceToSingleString(output, base, braces) {
+ var length = output.reduce(function (prev, cur) {
+ if (cur.indexOf('\n') >= 0) ;
+ return prev + cur.replace(/\u001b\[\d\d?m/g, '').length + 1;
+ }, 0);
+
+ if (length > 60) {
+ return braces[0] + (base === '' ? '' : base + '\n ') + ' ' + output.join(',\n ') + ' ' + braces[1];
+ }
+
+ return braces[0] + base + ' ' + output.join(', ') + ' ' + braces[1];
+} // NOTE: These type checking functions intentionally don't use `instanceof`
+// because it is fragile and can be easily faked with `Object.create()`.
+
+
+function isArray(ar) {
+ return Array.isArray(ar);
+}
+function isBoolean(arg) {
+ return typeof arg === 'boolean';
+}
+function isNull(arg) {
+ return arg === null;
+}
+function isNullOrUndefined(arg) {
+ return arg == null;
+}
+function isNumber(arg) {
+ return typeof arg === 'number';
+}
+function isString(arg) {
+ return typeof arg === 'string';
+}
+function isSymbol(arg) {
+ return babelHelpers["typeof"](arg) === 'symbol';
+}
+function isUndefined(arg) {
+ return arg === void 0;
+}
+function isRegExp(re) {
+ return isObject(re) && objectToString(re) === '[object RegExp]';
+}
+function isObject(arg) {
+ return babelHelpers["typeof"](arg) === 'object' && arg !== null;
+}
+function isDate(d) {
+ return isObject(d) && objectToString(d) === '[object Date]';
+}
+function isError(e) {
+ return isObject(e) && (objectToString(e) === '[object Error]' || e instanceof Error);
+}
+function isFunction(arg) {
+ return typeof arg === 'function';
+}
+function isPrimitive(arg) {
+ return arg === null || typeof arg === 'boolean' || typeof arg === 'number' || typeof arg === 'string' || babelHelpers["typeof"](arg) === 'symbol' || // ES6 symbol
+ typeof arg === 'undefined';
+}
+function isBuffer(maybeBuf) {
+ return Buffer.isBuffer(maybeBuf);
+}
+
+function objectToString(o) {
+ return Object.prototype.toString.call(o);
+}
+
+function pad(n) {
+ return n < 10 ? '0' + n.toString(10) : n.toString(10);
+}
+
+var months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']; // 26 Feb 16:19:34
+
+function timestamp$1() {
+ var d = new Date();
+ var time = [pad(d.getHours()), pad(d.getMinutes()), pad(d.getSeconds())].join(':');
+ return [d.getDate(), months[d.getMonth()], time].join(' ');
+} // log is just a thin wrapper to console.log that prepends a timestamp
+
+
+function log() {
+ console.log('%s - %s', timestamp$1(), format.apply(null, arguments));
+}
+function _extend(origin, add) {
+ // Don't do anything if add isn't an object
+ if (!add || !isObject(add)) return origin;
+ var keys = Object.keys(add);
+ var i = keys.length;
+
+ while (i--) {
+ origin[keys[i]] = add[keys[i]];
+ }
+
+ return origin;
+}
+
+function hasOwnProperty(obj, prop) {
+ return Object.prototype.hasOwnProperty.call(obj, prop);
+}
+
+var require$$1 = {
+ inherits: inherits$1,
+ _extend: _extend,
+ log: log,
+ isBuffer: isBuffer,
+ isPrimitive: isPrimitive,
+ isFunction: isFunction,
+ isError: isError,
+ isDate: isDate,
+ isObject: isObject,
+ isRegExp: isRegExp,
+ isUndefined: isUndefined,
+ isSymbol: isSymbol,
+ isString: isString,
+ isNumber: isNumber,
+ isNullOrUndefined: isNullOrUndefined,
+ isNull: isNull,
+ isBoolean: isBoolean,
+ isArray: isArray,
+ inspect: inspect,
+ deprecate: deprecate,
+ format: format,
+ debuglog: debuglog
+};
+
+var utils = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.deprecate = exports.isObjectLike = exports.isDate = exports.haveBuffer = exports.isMap = exports.isRegExp = exports.isBigUInt64Array = exports.isBigInt64Array = exports.isUint8Array = exports.isAnyArrayBuffer = exports.randomBytes = exports.normalizedFunctionString = void 0;
+
+/**
+ * Normalizes our expected stringified form of a function across versions of node
+ * @param fn - The function to stringify
+ */
+function normalizedFunctionString(fn) {
+ return fn.toString().replace('function(', 'function (');
+}
+exports.normalizedFunctionString = normalizedFunctionString;
+var isReactNative = typeof commonjsGlobal.navigator === 'object' && commonjsGlobal.navigator.product === 'ReactNative';
+var insecureWarning = isReactNative
+ ? 'BSON: For React Native please polyfill crypto.getRandomValues, e.g. using: https://www.npmjs.com/package/react-native-get-random-values.'
+ : 'BSON: No cryptographic implementation for random bytes present, falling back to a less secure implementation.';
+var insecureRandomBytes = function insecureRandomBytes(size) {
+ console.warn(insecureWarning);
+ var result = buffer.Buffer.alloc(size);
+ for (var i = 0; i < size; ++i)
+ result[i] = Math.floor(Math.random() * 256);
+ return result;
+};
+var detectRandomBytes = function () {
+ if (typeof window !== 'undefined') {
+ // browser crypto implementation(s)
+ var target_1 = window.crypto || window.msCrypto; // allow for IE11
+ if (target_1 && target_1.getRandomValues) {
+ return function (size) { return target_1.getRandomValues(buffer.Buffer.alloc(size)); };
+ }
+ }
+ if (typeof commonjsGlobal !== 'undefined' && commonjsGlobal.crypto && commonjsGlobal.crypto.getRandomValues) {
+ // allow for RN packages such as https://www.npmjs.com/package/react-native-get-random-values to populate global
+ return function (size) { return commonjsGlobal.crypto.getRandomValues(buffer.Buffer.alloc(size)); };
+ }
+ var requiredRandomBytes;
+ try {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ requiredRandomBytes = require$$0.randomBytes;
+ }
+ catch (e) {
+ // keep the fallback
+ }
+ // NOTE: in transpiled cases the above require might return null/undefined
+ return requiredRandomBytes || insecureRandomBytes;
+};
+exports.randomBytes = detectRandomBytes();
+function isAnyArrayBuffer(value) {
+ return ['[object ArrayBuffer]', '[object SharedArrayBuffer]'].includes(Object.prototype.toString.call(value));
+}
+exports.isAnyArrayBuffer = isAnyArrayBuffer;
+function isUint8Array(value) {
+ return Object.prototype.toString.call(value) === '[object Uint8Array]';
+}
+exports.isUint8Array = isUint8Array;
+function isBigInt64Array(value) {
+ return Object.prototype.toString.call(value) === '[object BigInt64Array]';
+}
+exports.isBigInt64Array = isBigInt64Array;
+function isBigUInt64Array(value) {
+ return Object.prototype.toString.call(value) === '[object BigUint64Array]';
+}
+exports.isBigUInt64Array = isBigUInt64Array;
+function isRegExp(d) {
+ return Object.prototype.toString.call(d) === '[object RegExp]';
+}
+exports.isRegExp = isRegExp;
+function isMap(d) {
+ return Object.prototype.toString.call(d) === '[object Map]';
+}
+exports.isMap = isMap;
+/** Call to check if your environment has `Buffer` */
+function haveBuffer() {
+ return typeof commonjsGlobal !== 'undefined' && typeof commonjsGlobal.Buffer !== 'undefined';
+}
+exports.haveBuffer = haveBuffer;
+// To ensure that 0.4 of node works correctly
+function isDate(d) {
+ return isObjectLike(d) && Object.prototype.toString.call(d) === '[object Date]';
+}
+exports.isDate = isDate;
+/**
+ * @internal
+ * this is to solve the `'someKey' in x` problem where x is unknown.
+ * https://github.com/typescript-eslint/typescript-eslint/issues/1071#issuecomment-541955753
+ */
+function isObjectLike(candidate) {
+ return typeof candidate === 'object' && candidate !== null;
+}
+exports.isObjectLike = isObjectLike;
+function deprecate(fn, message) {
+ if (typeof window === 'undefined' && typeof self === 'undefined') {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ return require$$1.deprecate(fn, message);
+ }
+ var warned = false;
+ function deprecated() {
+ var args = [];
+ for (var _i = 0; _i < arguments.length; _i++) {
+ args[_i] = arguments[_i];
+ }
+ if (!warned) {
+ console.warn(message);
+ warned = true;
+ }
+ return fn.apply(this, args);
+ }
+ return deprecated;
+}
+exports.deprecate = deprecate;
+
+});
+
+unwrapExports(utils);
+utils.deprecate;
+utils.isObjectLike;
+utils.isDate;
+utils.haveBuffer;
+utils.isMap;
+utils.isRegExp;
+utils.isBigUInt64Array;
+utils.isBigInt64Array;
+utils.isUint8Array;
+utils.isAnyArrayBuffer;
+utils.randomBytes;
+utils.normalizedFunctionString;
+
+var ensure_buffer = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.ensureBuffer = void 0;
+
+
+/**
+ * Makes sure that, if a Uint8Array is passed in, it is wrapped in a Buffer.
+ *
+ * @param potentialBuffer - The potential buffer
+ * @returns Buffer the input if potentialBuffer is a buffer, or a buffer that
+ * wraps a passed in Uint8Array
+ * @throws TypeError If anything other than a Buffer or Uint8Array is passed in
+ */
+function ensureBuffer(potentialBuffer) {
+ if (ArrayBuffer.isView(potentialBuffer)) {
+ return buffer.Buffer.from(potentialBuffer.buffer, potentialBuffer.byteOffset, potentialBuffer.byteLength);
+ }
+ if (utils.isAnyArrayBuffer(potentialBuffer)) {
+ return buffer.Buffer.from(potentialBuffer);
+ }
+ throw new TypeError('Must use either Buffer or TypedArray');
+}
+exports.ensureBuffer = ensureBuffer;
+
+});
+
+unwrapExports(ensure_buffer);
+ensure_buffer.ensureBuffer;
+
+var uuid_utils = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.bufferToUuidHexString = exports.uuidHexStringToBuffer = exports.uuidValidateString = void 0;
+
+// Validation regex for v4 uuid (validates with or without dashes)
+var VALIDATION_REGEX = /^(?:[0-9a-f]{8}-[0-9a-f]{4}-4[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}|[0-9a-f]{12}4[0-9a-f]{3}[89ab][0-9a-f]{15})$/i;
+var uuidValidateString = function (str) {
+ return typeof str === 'string' && VALIDATION_REGEX.test(str);
+};
+exports.uuidValidateString = uuidValidateString;
+var uuidHexStringToBuffer = function (hexString) {
+ if (!exports.uuidValidateString(hexString)) {
+ throw new TypeError('UUID string representations must be a 32 or 36 character hex string (dashes excluded/included). Format: "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx" or "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx".');
+ }
+ var sanitizedHexString = hexString.replace(/-/g, '');
+ return buffer.Buffer.from(sanitizedHexString, 'hex');
+};
+exports.uuidHexStringToBuffer = uuidHexStringToBuffer;
+var bufferToUuidHexString = function (buffer, includeDashes) {
+ if (includeDashes === void 0) { includeDashes = true; }
+ return includeDashes
+ ? buffer.toString('hex', 0, 4) +
+ '-' +
+ buffer.toString('hex', 4, 6) +
+ '-' +
+ buffer.toString('hex', 6, 8) +
+ '-' +
+ buffer.toString('hex', 8, 10) +
+ '-' +
+ buffer.toString('hex', 10, 16)
+ : buffer.toString('hex');
+};
+exports.bufferToUuidHexString = bufferToUuidHexString;
+
+});
+
+unwrapExports(uuid_utils);
+uuid_utils.bufferToUuidHexString;
+uuid_utils.uuidHexStringToBuffer;
+uuid_utils.uuidValidateString;
+
+var uuid = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.UUID = void 0;
+
+
+
+
+
+var BYTE_LENGTH = 16;
+var kId = Symbol('id');
+/**
+ * A class representation of the BSON UUID type.
+ * @public
+ */
+var UUID = /** @class */ (function () {
+ /**
+ * Create an UUID type
+ *
+ * @param input - Can be a 32 or 36 character hex string (dashes excluded/included) or a 16 byte binary Buffer.
+ */
+ function UUID(input) {
+ if (typeof input === 'undefined') {
+ // The most common use case (blank id, new UUID() instance)
+ this.id = UUID.generate();
+ }
+ else if (input instanceof UUID) {
+ this[kId] = buffer.Buffer.from(input.id);
+ this.__id = input.__id;
+ }
+ else if (ArrayBuffer.isView(input) && input.byteLength === BYTE_LENGTH) {
+ this.id = ensure_buffer.ensureBuffer(input);
+ }
+ else if (typeof input === 'string') {
+ this.id = uuid_utils.uuidHexStringToBuffer(input);
+ }
+ else {
+ throw new TypeError('Argument passed in UUID constructor must be a UUID, a 16 byte Buffer or a 32/36 character hex string (dashes excluded/included, format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx).');
+ }
+ }
+ Object.defineProperty(UUID.prototype, "id", {
+ /**
+ * The UUID bytes
+ * @readonly
+ */
+ get: function () {
+ return this[kId];
+ },
+ set: function (value) {
+ this[kId] = value;
+ if (UUID.cacheHexString) {
+ this.__id = uuid_utils.bufferToUuidHexString(value);
+ }
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /**
+ * Generate a 16 byte uuid v4 buffer used in UUIDs
+ */
+ /**
+ * Returns the UUID id as a 32 or 36 character hex string representation, excluding/including dashes (defaults to 36 character dash separated)
+ * @param includeDashes - should the string exclude dash-separators.
+ * */
+ UUID.prototype.toHexString = function (includeDashes) {
+ if (includeDashes === void 0) { includeDashes = true; }
+ if (UUID.cacheHexString && this.__id) {
+ return this.__id;
+ }
+ var uuidHexString = uuid_utils.bufferToUuidHexString(this.id, includeDashes);
+ if (UUID.cacheHexString) {
+ this.__id = uuidHexString;
+ }
+ return uuidHexString;
+ };
+ /**
+ * Converts the id into a 36 character (dashes included) hex string, unless a encoding is specified.
+ * @internal
+ */
+ UUID.prototype.toString = function (encoding) {
+ return encoding ? this.id.toString(encoding) : this.toHexString();
+ };
+ /**
+ * Converts the id into its JSON string representation. A 36 character (dashes included) hex string in the format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx
+ * @internal
+ */
+ UUID.prototype.toJSON = function () {
+ return this.toHexString();
+ };
+ /**
+ * Compares the equality of this UUID with `otherID`.
+ *
+ * @param otherId - UUID instance to compare against.
+ */
+ UUID.prototype.equals = function (otherId) {
+ if (!otherId) {
+ return false;
+ }
+ if (otherId instanceof UUID) {
+ return otherId.id.equals(this.id);
+ }
+ try {
+ return new UUID(otherId).id.equals(this.id);
+ }
+ catch (_a) {
+ return false;
+ }
+ };
+ /**
+ * Creates a Binary instance from the current UUID.
+ */
+ UUID.prototype.toBinary = function () {
+ return new binary.Binary(this.id, binary.Binary.SUBTYPE_UUID);
+ };
+ /**
+ * Generates a populated buffer containing a v4 uuid
+ */
+ UUID.generate = function () {
+ var bytes = utils.randomBytes(BYTE_LENGTH);
+ // Per 4.4, set bits for version and `clock_seq_hi_and_reserved`
+ // Kindly borrowed from https://github.com/uuidjs/uuid/blob/master/src/v4.js
+ bytes[6] = (bytes[6] & 0x0f) | 0x40;
+ bytes[8] = (bytes[8] & 0x3f) | 0x80;
+ return buffer.Buffer.from(bytes);
+ };
+ /**
+ * Checks if a value is a valid bson UUID
+ * @param input - UUID, string or Buffer to validate.
+ */
+ UUID.isValid = function (input) {
+ if (!input) {
+ return false;
+ }
+ if (input instanceof UUID) {
+ return true;
+ }
+ if (typeof input === 'string') {
+ return uuid_utils.uuidValidateString(input);
+ }
+ if (utils.isUint8Array(input)) {
+ // check for length & uuid version (https://tools.ietf.org/html/rfc4122#section-4.1.3)
+ if (input.length !== BYTE_LENGTH) {
+ return false;
+ }
+ try {
+ // get this byte as hex: xxxxxxxx-xxxx-XXxx-xxxx-xxxxxxxxxxxx
+ // check first part as uuid version: xxxxxxxx-xxxx-Xxxx-xxxx-xxxxxxxxxxxx
+ return parseInt(input[6].toString(16)[0], 10) === binary.Binary.SUBTYPE_UUID;
+ }
+ catch (_a) {
+ return false;
+ }
+ }
+ return false;
+ };
+ /**
+ * Creates an UUID from a hex string representation of an UUID.
+ * @param hexString - 32 or 36 character hex string (dashes excluded/included).
+ */
+ UUID.createFromHexString = function (hexString) {
+ var buffer = uuid_utils.uuidHexStringToBuffer(hexString);
+ return new UUID(buffer);
+ };
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 36 character hex string representation.
+ * @internal
+ */
+ UUID.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ UUID.prototype.inspect = function () {
+ return "new UUID(\"" + this.toHexString() + "\")";
+ };
+ return UUID;
+}());
+exports.UUID = UUID;
+Object.defineProperty(UUID.prototype, '_bsontype', { value: 'UUID' });
+
+});
+
+unwrapExports(uuid);
+uuid.UUID;
+
+var binary = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Binary = void 0;
+
+
+
+
+/**
+ * A class representation of the BSON Binary type.
+ * @public
+ */
+var Binary = /** @class */ (function () {
+ /**
+ * @param buffer - a buffer object containing the binary data.
+ * @param subType - the option binary type.
+ */
+ function Binary(buffer$1, subType) {
+ if (!(this instanceof Binary))
+ return new Binary(buffer$1, subType);
+ if (!(buffer$1 == null) &&
+ !(typeof buffer$1 === 'string') &&
+ !ArrayBuffer.isView(buffer$1) &&
+ !(buffer$1 instanceof ArrayBuffer) &&
+ !Array.isArray(buffer$1)) {
+ throw new TypeError('Binary can only be constructed from string, Buffer, TypedArray, or Array');
+ }
+ this.sub_type = subType !== null && subType !== void 0 ? subType : Binary.BSON_BINARY_SUBTYPE_DEFAULT;
+ if (buffer$1 == null) {
+ // create an empty binary buffer
+ this.buffer = buffer.Buffer.alloc(Binary.BUFFER_SIZE);
+ this.position = 0;
+ }
+ else {
+ if (typeof buffer$1 === 'string') {
+ // string
+ this.buffer = buffer.Buffer.from(buffer$1, 'binary');
+ }
+ else if (Array.isArray(buffer$1)) {
+ // number[]
+ this.buffer = buffer.Buffer.from(buffer$1);
+ }
+ else {
+ // Buffer | TypedArray | ArrayBuffer
+ this.buffer = ensure_buffer.ensureBuffer(buffer$1);
+ }
+ this.position = this.buffer.byteLength;
+ }
+ }
+ /**
+ * Updates this binary with byte_value.
+ *
+ * @param byteValue - a single byte we wish to write.
+ */
+ Binary.prototype.put = function (byteValue) {
+ // If it's a string and a has more than one character throw an error
+ if (typeof byteValue === 'string' && byteValue.length !== 1) {
+ throw new TypeError('only accepts single character String');
+ }
+ else if (typeof byteValue !== 'number' && byteValue.length !== 1)
+ throw new TypeError('only accepts single character Uint8Array or Array');
+ // Decode the byte value once
+ var decodedByte;
+ if (typeof byteValue === 'string') {
+ decodedByte = byteValue.charCodeAt(0);
+ }
+ else if (typeof byteValue === 'number') {
+ decodedByte = byteValue;
+ }
+ else {
+ decodedByte = byteValue[0];
+ }
+ if (decodedByte < 0 || decodedByte > 255) {
+ throw new TypeError('only accepts number in a valid unsigned byte range 0-255');
+ }
+ if (this.buffer.length > this.position) {
+ this.buffer[this.position++] = decodedByte;
+ }
+ else {
+ var buffer$1 = buffer.Buffer.alloc(Binary.BUFFER_SIZE + this.buffer.length);
+ // Combine the two buffers together
+ this.buffer.copy(buffer$1, 0, 0, this.buffer.length);
+ this.buffer = buffer$1;
+ this.buffer[this.position++] = decodedByte;
+ }
+ };
+ /**
+ * Writes a buffer or string to the binary.
+ *
+ * @param sequence - a string or buffer to be written to the Binary BSON object.
+ * @param offset - specify the binary of where to write the content.
+ */
+ Binary.prototype.write = function (sequence, offset) {
+ offset = typeof offset === 'number' ? offset : this.position;
+ // If the buffer is to small let's extend the buffer
+ if (this.buffer.length < offset + sequence.length) {
+ var buffer$1 = buffer.Buffer.alloc(this.buffer.length + sequence.length);
+ this.buffer.copy(buffer$1, 0, 0, this.buffer.length);
+ // Assign the new buffer
+ this.buffer = buffer$1;
+ }
+ if (ArrayBuffer.isView(sequence)) {
+ this.buffer.set(ensure_buffer.ensureBuffer(sequence), offset);
+ this.position =
+ offset + sequence.byteLength > this.position ? offset + sequence.length : this.position;
+ }
+ else if (typeof sequence === 'string') {
+ this.buffer.write(sequence, offset, sequence.length, 'binary');
+ this.position =
+ offset + sequence.length > this.position ? offset + sequence.length : this.position;
+ }
+ };
+ /**
+ * Reads **length** bytes starting at **position**.
+ *
+ * @param position - read from the given position in the Binary.
+ * @param length - the number of bytes to read.
+ */
+ Binary.prototype.read = function (position, length) {
+ length = length && length > 0 ? length : this.position;
+ // Let's return the data based on the type we have
+ return this.buffer.slice(position, position + length);
+ };
+ /**
+ * Returns the value of this binary as a string.
+ * @param asRaw - Will skip converting to a string
+ * @remarks
+ * This is handy when calling this function conditionally for some key value pairs and not others
+ */
+ Binary.prototype.value = function (asRaw) {
+ asRaw = !!asRaw;
+ // Optimize to serialize for the situation where the data == size of buffer
+ if (asRaw && this.buffer.length === this.position) {
+ return this.buffer;
+ }
+ // If it's a node.js buffer object
+ if (asRaw) {
+ return this.buffer.slice(0, this.position);
+ }
+ return this.buffer.toString('binary', 0, this.position);
+ };
+ /** the length of the binary sequence */
+ Binary.prototype.length = function () {
+ return this.position;
+ };
+ /** @internal */
+ Binary.prototype.toJSON = function () {
+ return this.buffer.toString('base64');
+ };
+ /** @internal */
+ Binary.prototype.toString = function (format) {
+ return this.buffer.toString(format);
+ };
+ /** @internal */
+ Binary.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ var base64String = this.buffer.toString('base64');
+ var subType = Number(this.sub_type).toString(16);
+ if (options.legacy) {
+ return {
+ $binary: base64String,
+ $type: subType.length === 1 ? '0' + subType : subType
+ };
+ }
+ return {
+ $binary: {
+ base64: base64String,
+ subType: subType.length === 1 ? '0' + subType : subType
+ }
+ };
+ };
+ /** @internal */
+ Binary.prototype.toUUID = function () {
+ if (this.sub_type === Binary.SUBTYPE_UUID) {
+ return new uuid.UUID(this.buffer.slice(0, this.position));
+ }
+ throw new Error("Binary sub_type \"" + this.sub_type + "\" is not supported for converting to UUID. Only \"" + Binary.SUBTYPE_UUID + "\" is currently supported.");
+ };
+ /** @internal */
+ Binary.fromExtendedJSON = function (doc, options) {
+ options = options || {};
+ var data;
+ var type;
+ if ('$binary' in doc) {
+ if (options.legacy && typeof doc.$binary === 'string' && '$type' in doc) {
+ type = doc.$type ? parseInt(doc.$type, 16) : 0;
+ data = buffer.Buffer.from(doc.$binary, 'base64');
+ }
+ else {
+ if (typeof doc.$binary !== 'string') {
+ type = doc.$binary.subType ? parseInt(doc.$binary.subType, 16) : 0;
+ data = buffer.Buffer.from(doc.$binary.base64, 'base64');
+ }
+ }
+ }
+ else if ('$uuid' in doc) {
+ type = 4;
+ data = uuid_utils.uuidHexStringToBuffer(doc.$uuid);
+ }
+ if (!data) {
+ throw new TypeError("Unexpected Binary Extended JSON format " + JSON.stringify(doc));
+ }
+ return new Binary(data, type);
+ };
+ /** @internal */
+ Binary.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Binary.prototype.inspect = function () {
+ var asBuffer = this.value(true);
+ return "new Binary(Buffer.from(\"" + asBuffer.toString('hex') + "\", \"hex\"), " + this.sub_type + ")";
+ };
+ /**
+ * Binary default subtype
+ * @internal
+ */
+ Binary.BSON_BINARY_SUBTYPE_DEFAULT = 0;
+ /** Initial buffer default size */
+ Binary.BUFFER_SIZE = 256;
+ /** Default BSON type */
+ Binary.SUBTYPE_DEFAULT = 0;
+ /** Function BSON type */
+ Binary.SUBTYPE_FUNCTION = 1;
+ /** Byte Array BSON type */
+ Binary.SUBTYPE_BYTE_ARRAY = 2;
+ /** Deprecated UUID BSON type @deprecated Please use SUBTYPE_UUID */
+ Binary.SUBTYPE_UUID_OLD = 3;
+ /** UUID BSON type */
+ Binary.SUBTYPE_UUID = 4;
+ /** MD5 BSON type */
+ Binary.SUBTYPE_MD5 = 5;
+ /** User BSON type */
+ Binary.SUBTYPE_USER_DEFINED = 128;
+ return Binary;
+}());
+exports.Binary = Binary;
+Object.defineProperty(Binary.prototype, '_bsontype', { value: 'Binary' });
+
+});
+
+unwrapExports(binary);
+binary.Binary;
+
+var code = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Code = void 0;
+/**
+ * A class representation of the BSON Code type.
+ * @public
+ */
+var Code = /** @class */ (function () {
+ /**
+ * @param code - a string or function.
+ * @param scope - an optional scope for the function.
+ */
+ function Code(code, scope) {
+ if (!(this instanceof Code))
+ return new Code(code, scope);
+ this.code = code;
+ this.scope = scope;
+ }
+ /** @internal */
+ Code.prototype.toJSON = function () {
+ return { code: this.code, scope: this.scope };
+ };
+ /** @internal */
+ Code.prototype.toExtendedJSON = function () {
+ if (this.scope) {
+ return { $code: this.code, $scope: this.scope };
+ }
+ return { $code: this.code };
+ };
+ /** @internal */
+ Code.fromExtendedJSON = function (doc) {
+ return new Code(doc.$code, doc.$scope);
+ };
+ /** @internal */
+ Code.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Code.prototype.inspect = function () {
+ var codeJson = this.toJSON();
+ return "new Code(\"" + codeJson.code + "\"" + (codeJson.scope ? ", " + JSON.stringify(codeJson.scope) : '') + ")";
+ };
+ return Code;
+}());
+exports.Code = Code;
+Object.defineProperty(Code.prototype, '_bsontype', { value: 'Code' });
+
+});
+
+unwrapExports(code);
+code.Code;
+
+var db_ref = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.DBRef = exports.isDBRefLike = void 0;
+
+/** @internal */
+function isDBRefLike(value) {
+ return (utils.isObjectLike(value) &&
+ value.$id != null &&
+ typeof value.$ref === 'string' &&
+ (value.$db == null || typeof value.$db === 'string'));
+}
+exports.isDBRefLike = isDBRefLike;
+/**
+ * A class representation of the BSON DBRef type.
+ * @public
+ */
+var DBRef = /** @class */ (function () {
+ /**
+ * @param collection - the collection name.
+ * @param oid - the reference ObjectId.
+ * @param db - optional db name, if omitted the reference is local to the current db.
+ */
+ function DBRef(collection, oid, db, fields) {
+ if (!(this instanceof DBRef))
+ return new DBRef(collection, oid, db, fields);
+ // check if namespace has been provided
+ var parts = collection.split('.');
+ if (parts.length === 2) {
+ db = parts.shift();
+ // eslint-disable-next-line @typescript-eslint/no-non-null-assertion
+ collection = parts.shift();
+ }
+ this.collection = collection;
+ this.oid = oid;
+ this.db = db;
+ this.fields = fields || {};
+ }
+ Object.defineProperty(DBRef.prototype, "namespace", {
+ // Property provided for compatibility with the 1.x parser
+ // the 1.x parser used a "namespace" property, while 4.x uses "collection"
+ /** @internal */
+ get: function () {
+ return this.collection;
+ },
+ set: function (value) {
+ this.collection = value;
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /** @internal */
+ DBRef.prototype.toJSON = function () {
+ var o = Object.assign({
+ $ref: this.collection,
+ $id: this.oid
+ }, this.fields);
+ if (this.db != null)
+ o.$db = this.db;
+ return o;
+ };
+ /** @internal */
+ DBRef.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ var o = {
+ $ref: this.collection,
+ $id: this.oid
+ };
+ if (options.legacy) {
+ return o;
+ }
+ if (this.db)
+ o.$db = this.db;
+ o = Object.assign(o, this.fields);
+ return o;
+ };
+ /** @internal */
+ DBRef.fromExtendedJSON = function (doc) {
+ var copy = Object.assign({}, doc);
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new DBRef(doc.$ref, doc.$id, doc.$db, copy);
+ };
+ /** @internal */
+ DBRef.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ DBRef.prototype.inspect = function () {
+ // NOTE: if OID is an ObjectId class it will just print the oid string.
+ var oid = this.oid === undefined || this.oid.toString === undefined ? this.oid : this.oid.toString();
+ return "new DBRef(\"" + this.namespace + "\", new ObjectId(\"" + oid + "\")" + (this.db ? ", \"" + this.db + "\"" : '') + ")";
+ };
+ return DBRef;
+}());
+exports.DBRef = DBRef;
+Object.defineProperty(DBRef.prototype, '_bsontype', { value: 'DBRef' });
+
+});
+
+unwrapExports(db_ref);
+db_ref.DBRef;
+db_ref.isDBRefLike;
+
+var long_1 = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Long = void 0;
+
+/**
+ * wasm optimizations, to do native i64 multiplication and divide
+ */
+var wasm = undefined;
+try {
+ wasm = new WebAssembly.Instance(new WebAssembly.Module(
+ // prettier-ignore
+ new Uint8Array([0, 97, 115, 109, 1, 0, 0, 0, 1, 13, 2, 96, 0, 1, 127, 96, 4, 127, 127, 127, 127, 1, 127, 3, 7, 6, 0, 1, 1, 1, 1, 1, 6, 6, 1, 127, 1, 65, 0, 11, 7, 50, 6, 3, 109, 117, 108, 0, 1, 5, 100, 105, 118, 95, 115, 0, 2, 5, 100, 105, 118, 95, 117, 0, 3, 5, 114, 101, 109, 95, 115, 0, 4, 5, 114, 101, 109, 95, 117, 0, 5, 8, 103, 101, 116, 95, 104, 105, 103, 104, 0, 0, 10, 191, 1, 6, 4, 0, 35, 0, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 126, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 127, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 128, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 129, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 130, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11])), {}).exports;
+}
+catch (_a) {
+ // no wasm support
+}
+var TWO_PWR_16_DBL = 1 << 16;
+var TWO_PWR_24_DBL = 1 << 24;
+var TWO_PWR_32_DBL = TWO_PWR_16_DBL * TWO_PWR_16_DBL;
+var TWO_PWR_64_DBL = TWO_PWR_32_DBL * TWO_PWR_32_DBL;
+var TWO_PWR_63_DBL = TWO_PWR_64_DBL / 2;
+/** A cache of the Long representations of small integer values. */
+var INT_CACHE = {};
+/** A cache of the Long representations of small unsigned integer values. */
+var UINT_CACHE = {};
+/**
+ * A class representing a 64-bit integer
+ * @public
+ * @remarks
+ * The internal representation of a long is the two given signed, 32-bit values.
+ * We use 32-bit pieces because these are the size of integers on which
+ * Javascript performs bit-operations. For operations like addition and
+ * multiplication, we split each number into 16 bit pieces, which can easily be
+ * multiplied within Javascript's floating-point representation without overflow
+ * or change in sign.
+ * In the algorithms below, we frequently reduce the negative case to the
+ * positive case by negating the input(s) and then post-processing the result.
+ * Note that we must ALWAYS check specially whether those values are MIN_VALUE
+ * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
+ * a positive number, it overflows back into a negative). Not handling this
+ * case would often result in infinite recursion.
+ * Common constant values ZERO, ONE, NEG_ONE, etc. are found as static properties on this class.
+ */
+var Long = /** @class */ (function () {
+ /**
+ * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers.
+ * See the from* functions below for more convenient ways of constructing Longs.
+ *
+ * Acceptable signatures are:
+ * - Long(low, high, unsigned?)
+ * - Long(bigint, unsigned?)
+ * - Long(string, unsigned?)
+ *
+ * @param low - The low (signed) 32 bits of the long
+ * @param high - The high (signed) 32 bits of the long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ function Long(low, high, unsigned) {
+ if (low === void 0) { low = 0; }
+ if (!(this instanceof Long))
+ return new Long(low, high, unsigned);
+ if (typeof low === 'bigint') {
+ Object.assign(this, Long.fromBigInt(low, !!high));
+ }
+ else if (typeof low === 'string') {
+ Object.assign(this, Long.fromString(low, !!high));
+ }
+ else {
+ this.low = low | 0;
+ this.high = high | 0;
+ this.unsigned = !!unsigned;
+ }
+ Object.defineProperty(this, '__isLong__', {
+ value: true,
+ configurable: false,
+ writable: false,
+ enumerable: false
+ });
+ }
+ /**
+ * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits.
+ * Each is assumed to use 32 bits.
+ * @param lowBits - The low 32 bits
+ * @param highBits - The high 32 bits
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBits = function (lowBits, highBits, unsigned) {
+ return new Long(lowBits, highBits, unsigned);
+ };
+ /**
+ * Returns a Long representing the given 32 bit integer value.
+ * @param value - The 32 bit integer in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromInt = function (value, unsigned) {
+ var obj, cachedObj, cache;
+ if (unsigned) {
+ value >>>= 0;
+ if ((cache = 0 <= value && value < 256)) {
+ cachedObj = UINT_CACHE[value];
+ if (cachedObj)
+ return cachedObj;
+ }
+ obj = Long.fromBits(value, (value | 0) < 0 ? -1 : 0, true);
+ if (cache)
+ UINT_CACHE[value] = obj;
+ return obj;
+ }
+ else {
+ value |= 0;
+ if ((cache = -128 <= value && value < 128)) {
+ cachedObj = INT_CACHE[value];
+ if (cachedObj)
+ return cachedObj;
+ }
+ obj = Long.fromBits(value, value < 0 ? -1 : 0, false);
+ if (cache)
+ INT_CACHE[value] = obj;
+ return obj;
+ }
+ };
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromNumber = function (value, unsigned) {
+ if (isNaN(value))
+ return unsigned ? Long.UZERO : Long.ZERO;
+ if (unsigned) {
+ if (value < 0)
+ return Long.UZERO;
+ if (value >= TWO_PWR_64_DBL)
+ return Long.MAX_UNSIGNED_VALUE;
+ }
+ else {
+ if (value <= -TWO_PWR_63_DBL)
+ return Long.MIN_VALUE;
+ if (value + 1 >= TWO_PWR_63_DBL)
+ return Long.MAX_VALUE;
+ }
+ if (value < 0)
+ return Long.fromNumber(-value, unsigned).neg();
+ return Long.fromBits(value % TWO_PWR_32_DBL | 0, (value / TWO_PWR_32_DBL) | 0, unsigned);
+ };
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBigInt = function (value, unsigned) {
+ return Long.fromString(value.toString(), unsigned);
+ };
+ /**
+ * Returns a Long representation of the given string, written using the specified radix.
+ * @param str - The textual representation of the Long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param radix - The radix in which the text is written (2-36), defaults to 10
+ * @returns The corresponding Long value
+ */
+ Long.fromString = function (str, unsigned, radix) {
+ if (str.length === 0)
+ throw Error('empty string');
+ if (str === 'NaN' || str === 'Infinity' || str === '+Infinity' || str === '-Infinity')
+ return Long.ZERO;
+ if (typeof unsigned === 'number') {
+ // For goog.math.long compatibility
+ (radix = unsigned), (unsigned = false);
+ }
+ else {
+ unsigned = !!unsigned;
+ }
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix)
+ throw RangeError('radix');
+ var p;
+ if ((p = str.indexOf('-')) > 0)
+ throw Error('interior hyphen');
+ else if (p === 0) {
+ return Long.fromString(str.substring(1), unsigned, radix).neg();
+ }
+ // Do several (8) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ var radixToPower = Long.fromNumber(Math.pow(radix, 8));
+ var result = Long.ZERO;
+ for (var i = 0; i < str.length; i += 8) {
+ var size = Math.min(8, str.length - i), value = parseInt(str.substring(i, i + size), radix);
+ if (size < 8) {
+ var power = Long.fromNumber(Math.pow(radix, size));
+ result = result.mul(power).add(Long.fromNumber(value));
+ }
+ else {
+ result = result.mul(radixToPower);
+ result = result.add(Long.fromNumber(value));
+ }
+ }
+ result.unsigned = unsigned;
+ return result;
+ };
+ /**
+ * Creates a Long from its byte representation.
+ * @param bytes - Byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns The corresponding Long value
+ */
+ Long.fromBytes = function (bytes, unsigned, le) {
+ return le ? Long.fromBytesLE(bytes, unsigned) : Long.fromBytesBE(bytes, unsigned);
+ };
+ /**
+ * Creates a Long from its little endian byte representation.
+ * @param bytes - Little endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBytesLE = function (bytes, unsigned) {
+ return new Long(bytes[0] | (bytes[1] << 8) | (bytes[2] << 16) | (bytes[3] << 24), bytes[4] | (bytes[5] << 8) | (bytes[6] << 16) | (bytes[7] << 24), unsigned);
+ };
+ /**
+ * Creates a Long from its big endian byte representation.
+ * @param bytes - Big endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBytesBE = function (bytes, unsigned) {
+ return new Long((bytes[4] << 24) | (bytes[5] << 16) | (bytes[6] << 8) | bytes[7], (bytes[0] << 24) | (bytes[1] << 16) | (bytes[2] << 8) | bytes[3], unsigned);
+ };
+ /**
+ * Tests if the specified object is a Long.
+ */
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any, @typescript-eslint/explicit-module-boundary-types
+ Long.isLong = function (value) {
+ return utils.isObjectLike(value) && value['__isLong__'] === true;
+ };
+ /**
+ * Converts the specified value to a Long.
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ Long.fromValue = function (val, unsigned) {
+ if (typeof val === 'number')
+ return Long.fromNumber(val, unsigned);
+ if (typeof val === 'string')
+ return Long.fromString(val, unsigned);
+ // Throws for non-objects, converts non-instanceof Long:
+ return Long.fromBits(val.low, val.high, typeof unsigned === 'boolean' ? unsigned : val.unsigned);
+ };
+ /** Returns the sum of this and the specified Long. */
+ Long.prototype.add = function (addend) {
+ if (!Long.isLong(addend))
+ addend = Long.fromValue(addend);
+ // Divide each number into 4 chunks of 16 bits, and then sum the chunks.
+ var a48 = this.high >>> 16;
+ var a32 = this.high & 0xffff;
+ var a16 = this.low >>> 16;
+ var a00 = this.low & 0xffff;
+ var b48 = addend.high >>> 16;
+ var b32 = addend.high & 0xffff;
+ var b16 = addend.low >>> 16;
+ var b00 = addend.low & 0xffff;
+ var c48 = 0, c32 = 0, c16 = 0, c00 = 0;
+ c00 += a00 + b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 + b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 + b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 + b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ };
+ /**
+ * Returns the sum of this and the specified Long.
+ * @returns Sum
+ */
+ Long.prototype.and = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low & other.low, this.high & other.high, this.unsigned);
+ };
+ /**
+ * Compares this Long's value with the specified's.
+ * @returns 0 if they are the same, 1 if the this is greater and -1 if the given one is greater
+ */
+ Long.prototype.compare = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ if (this.eq(other))
+ return 0;
+ var thisNeg = this.isNegative(), otherNeg = other.isNegative();
+ if (thisNeg && !otherNeg)
+ return -1;
+ if (!thisNeg && otherNeg)
+ return 1;
+ // At this point the sign bits are the same
+ if (!this.unsigned)
+ return this.sub(other).isNegative() ? -1 : 1;
+ // Both are positive if at least one is unsigned
+ return other.high >>> 0 > this.high >>> 0 ||
+ (other.high === this.high && other.low >>> 0 > this.low >>> 0)
+ ? -1
+ : 1;
+ };
+ /** This is an alias of {@link Long.compare} */
+ Long.prototype.comp = function (other) {
+ return this.compare(other);
+ };
+ /**
+ * Returns this Long divided by the specified. The result is signed if this Long is signed or unsigned if this Long is unsigned.
+ * @returns Quotient
+ */
+ Long.prototype.divide = function (divisor) {
+ if (!Long.isLong(divisor))
+ divisor = Long.fromValue(divisor);
+ if (divisor.isZero())
+ throw Error('division by zero');
+ // use wasm support if present
+ if (wasm) {
+ // guard against signed division overflow: the largest
+ // negative number / -1 would be 1 larger than the largest
+ // positive number, due to two's complement.
+ if (!this.unsigned &&
+ this.high === -0x80000000 &&
+ divisor.low === -1 &&
+ divisor.high === -1) {
+ // be consistent with non-wasm code path
+ return this;
+ }
+ var low = (this.unsigned ? wasm.div_u : wasm.div_s)(this.low, this.high, divisor.low, divisor.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ if (this.isZero())
+ return this.unsigned ? Long.UZERO : Long.ZERO;
+ var approx, rem, res;
+ if (!this.unsigned) {
+ // This section is only relevant for signed longs and is derived from the
+ // closure library as a whole.
+ if (this.eq(Long.MIN_VALUE)) {
+ if (divisor.eq(Long.ONE) || divisor.eq(Long.NEG_ONE))
+ return Long.MIN_VALUE;
+ // recall that -MIN_VALUE == MIN_VALUE
+ else if (divisor.eq(Long.MIN_VALUE))
+ return Long.ONE;
+ else {
+ // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.
+ var halfThis = this.shr(1);
+ approx = halfThis.div(divisor).shl(1);
+ if (approx.eq(Long.ZERO)) {
+ return divisor.isNegative() ? Long.ONE : Long.NEG_ONE;
+ }
+ else {
+ rem = this.sub(divisor.mul(approx));
+ res = approx.add(rem.div(divisor));
+ return res;
+ }
+ }
+ }
+ else if (divisor.eq(Long.MIN_VALUE))
+ return this.unsigned ? Long.UZERO : Long.ZERO;
+ if (this.isNegative()) {
+ if (divisor.isNegative())
+ return this.neg().div(divisor.neg());
+ return this.neg().div(divisor).neg();
+ }
+ else if (divisor.isNegative())
+ return this.div(divisor.neg()).neg();
+ res = Long.ZERO;
+ }
+ else {
+ // The algorithm below has not been made for unsigned longs. It's therefore
+ // required to take special care of the MSB prior to running it.
+ if (!divisor.unsigned)
+ divisor = divisor.toUnsigned();
+ if (divisor.gt(this))
+ return Long.UZERO;
+ if (divisor.gt(this.shru(1)))
+ // 15 >>> 1 = 7 ; with divisor = 8 ; true
+ return Long.UONE;
+ res = Long.UZERO;
+ }
+ // Repeat the following until the remainder is less than other: find a
+ // floating-point that approximates remainder / other *from below*, add this
+ // into the result, and subtract it from the remainder. It is critical that
+ // the approximate value is less than or equal to the real value so that the
+ // remainder never becomes negative.
+ rem = this;
+ while (rem.gte(divisor)) {
+ // Approximate the result of division. This may be a little greater or
+ // smaller than the actual value.
+ approx = Math.max(1, Math.floor(rem.toNumber() / divisor.toNumber()));
+ // We will tweak the approximate result by changing it in the 48-th digit or
+ // the smallest non-fractional digit, whichever is larger.
+ var log2 = Math.ceil(Math.log(approx) / Math.LN2);
+ var delta = log2 <= 48 ? 1 : Math.pow(2, log2 - 48);
+ // Decrease the approximation until it is smaller than the remainder. Note
+ // that if it is too large, the product overflows and is negative.
+ var approxRes = Long.fromNumber(approx);
+ var approxRem = approxRes.mul(divisor);
+ while (approxRem.isNegative() || approxRem.gt(rem)) {
+ approx -= delta;
+ approxRes = Long.fromNumber(approx, this.unsigned);
+ approxRem = approxRes.mul(divisor);
+ }
+ // We know the answer can't be zero... and actually, zero would cause
+ // infinite recursion since we would make no progress.
+ if (approxRes.isZero())
+ approxRes = Long.ONE;
+ res = res.add(approxRes);
+ rem = rem.sub(approxRem);
+ }
+ return res;
+ };
+ /**This is an alias of {@link Long.divide} */
+ Long.prototype.div = function (divisor) {
+ return this.divide(divisor);
+ };
+ /**
+ * Tests if this Long's value equals the specified's.
+ * @param other - Other value
+ */
+ Long.prototype.equals = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ if (this.unsigned !== other.unsigned && this.high >>> 31 === 1 && other.high >>> 31 === 1)
+ return false;
+ return this.high === other.high && this.low === other.low;
+ };
+ /** This is an alias of {@link Long.equals} */
+ Long.prototype.eq = function (other) {
+ return this.equals(other);
+ };
+ /** Gets the high 32 bits as a signed integer. */
+ Long.prototype.getHighBits = function () {
+ return this.high;
+ };
+ /** Gets the high 32 bits as an unsigned integer. */
+ Long.prototype.getHighBitsUnsigned = function () {
+ return this.high >>> 0;
+ };
+ /** Gets the low 32 bits as a signed integer. */
+ Long.prototype.getLowBits = function () {
+ return this.low;
+ };
+ /** Gets the low 32 bits as an unsigned integer. */
+ Long.prototype.getLowBitsUnsigned = function () {
+ return this.low >>> 0;
+ };
+ /** Gets the number of bits needed to represent the absolute value of this Long. */
+ Long.prototype.getNumBitsAbs = function () {
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ return this.eq(Long.MIN_VALUE) ? 64 : this.neg().getNumBitsAbs();
+ }
+ var val = this.high !== 0 ? this.high : this.low;
+ var bit;
+ for (bit = 31; bit > 0; bit--)
+ if ((val & (1 << bit)) !== 0)
+ break;
+ return this.high !== 0 ? bit + 33 : bit + 1;
+ };
+ /** Tests if this Long's value is greater than the specified's. */
+ Long.prototype.greaterThan = function (other) {
+ return this.comp(other) > 0;
+ };
+ /** This is an alias of {@link Long.greaterThan} */
+ Long.prototype.gt = function (other) {
+ return this.greaterThan(other);
+ };
+ /** Tests if this Long's value is greater than or equal the specified's. */
+ Long.prototype.greaterThanOrEqual = function (other) {
+ return this.comp(other) >= 0;
+ };
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ Long.prototype.gte = function (other) {
+ return this.greaterThanOrEqual(other);
+ };
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ Long.prototype.ge = function (other) {
+ return this.greaterThanOrEqual(other);
+ };
+ /** Tests if this Long's value is even. */
+ Long.prototype.isEven = function () {
+ return (this.low & 1) === 0;
+ };
+ /** Tests if this Long's value is negative. */
+ Long.prototype.isNegative = function () {
+ return !this.unsigned && this.high < 0;
+ };
+ /** Tests if this Long's value is odd. */
+ Long.prototype.isOdd = function () {
+ return (this.low & 1) === 1;
+ };
+ /** Tests if this Long's value is positive. */
+ Long.prototype.isPositive = function () {
+ return this.unsigned || this.high >= 0;
+ };
+ /** Tests if this Long's value equals zero. */
+ Long.prototype.isZero = function () {
+ return this.high === 0 && this.low === 0;
+ };
+ /** Tests if this Long's value is less than the specified's. */
+ Long.prototype.lessThan = function (other) {
+ return this.comp(other) < 0;
+ };
+ /** This is an alias of {@link Long#lessThan}. */
+ Long.prototype.lt = function (other) {
+ return this.lessThan(other);
+ };
+ /** Tests if this Long's value is less than or equal the specified's. */
+ Long.prototype.lessThanOrEqual = function (other) {
+ return this.comp(other) <= 0;
+ };
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ Long.prototype.lte = function (other) {
+ return this.lessThanOrEqual(other);
+ };
+ /** Returns this Long modulo the specified. */
+ Long.prototype.modulo = function (divisor) {
+ if (!Long.isLong(divisor))
+ divisor = Long.fromValue(divisor);
+ // use wasm support if present
+ if (wasm) {
+ var low = (this.unsigned ? wasm.rem_u : wasm.rem_s)(this.low, this.high, divisor.low, divisor.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ return this.sub(this.div(divisor).mul(divisor));
+ };
+ /** This is an alias of {@link Long.modulo} */
+ Long.prototype.mod = function (divisor) {
+ return this.modulo(divisor);
+ };
+ /** This is an alias of {@link Long.modulo} */
+ Long.prototype.rem = function (divisor) {
+ return this.modulo(divisor);
+ };
+ /**
+ * Returns the product of this and the specified Long.
+ * @param multiplier - Multiplier
+ * @returns Product
+ */
+ Long.prototype.multiply = function (multiplier) {
+ if (this.isZero())
+ return Long.ZERO;
+ if (!Long.isLong(multiplier))
+ multiplier = Long.fromValue(multiplier);
+ // use wasm support if present
+ if (wasm) {
+ var low = wasm.mul(this.low, this.high, multiplier.low, multiplier.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ if (multiplier.isZero())
+ return Long.ZERO;
+ if (this.eq(Long.MIN_VALUE))
+ return multiplier.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (multiplier.eq(Long.MIN_VALUE))
+ return this.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (this.isNegative()) {
+ if (multiplier.isNegative())
+ return this.neg().mul(multiplier.neg());
+ else
+ return this.neg().mul(multiplier).neg();
+ }
+ else if (multiplier.isNegative())
+ return this.mul(multiplier.neg()).neg();
+ // If both longs are small, use float multiplication
+ if (this.lt(Long.TWO_PWR_24) && multiplier.lt(Long.TWO_PWR_24))
+ return Long.fromNumber(this.toNumber() * multiplier.toNumber(), this.unsigned);
+ // Divide each long into 4 chunks of 16 bits, and then add up 4x4 products.
+ // We can skip products that would overflow.
+ var a48 = this.high >>> 16;
+ var a32 = this.high & 0xffff;
+ var a16 = this.low >>> 16;
+ var a00 = this.low & 0xffff;
+ var b48 = multiplier.high >>> 16;
+ var b32 = multiplier.high & 0xffff;
+ var b16 = multiplier.low >>> 16;
+ var b00 = multiplier.low & 0xffff;
+ var c48 = 0, c32 = 0, c16 = 0, c00 = 0;
+ c00 += a00 * b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 * b00;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c16 += a00 * b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 * b00;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a16 * b16;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a00 * b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ };
+ /** This is an alias of {@link Long.multiply} */
+ Long.prototype.mul = function (multiplier) {
+ return this.multiply(multiplier);
+ };
+ /** Returns the Negation of this Long's value. */
+ Long.prototype.negate = function () {
+ if (!this.unsigned && this.eq(Long.MIN_VALUE))
+ return Long.MIN_VALUE;
+ return this.not().add(Long.ONE);
+ };
+ /** This is an alias of {@link Long.negate} */
+ Long.prototype.neg = function () {
+ return this.negate();
+ };
+ /** Returns the bitwise NOT of this Long. */
+ Long.prototype.not = function () {
+ return Long.fromBits(~this.low, ~this.high, this.unsigned);
+ };
+ /** Tests if this Long's value differs from the specified's. */
+ Long.prototype.notEquals = function (other) {
+ return !this.equals(other);
+ };
+ /** This is an alias of {@link Long.notEquals} */
+ Long.prototype.neq = function (other) {
+ return this.notEquals(other);
+ };
+ /** This is an alias of {@link Long.notEquals} */
+ Long.prototype.ne = function (other) {
+ return this.notEquals(other);
+ };
+ /**
+ * Returns the bitwise OR of this Long and the specified.
+ */
+ Long.prototype.or = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low | other.low, this.high | other.high, this.unsigned);
+ };
+ /**
+ * Returns this Long with bits shifted to the left by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftLeft = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ if ((numBits &= 63) === 0)
+ return this;
+ else if (numBits < 32)
+ return Long.fromBits(this.low << numBits, (this.high << numBits) | (this.low >>> (32 - numBits)), this.unsigned);
+ else
+ return Long.fromBits(0, this.low << (numBits - 32), this.unsigned);
+ };
+ /** This is an alias of {@link Long.shiftLeft} */
+ Long.prototype.shl = function (numBits) {
+ return this.shiftLeft(numBits);
+ };
+ /**
+ * Returns this Long with bits arithmetically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftRight = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ if ((numBits &= 63) === 0)
+ return this;
+ else if (numBits < 32)
+ return Long.fromBits((this.low >>> numBits) | (this.high << (32 - numBits)), this.high >> numBits, this.unsigned);
+ else
+ return Long.fromBits(this.high >> (numBits - 32), this.high >= 0 ? 0 : -1, this.unsigned);
+ };
+ /** This is an alias of {@link Long.shiftRight} */
+ Long.prototype.shr = function (numBits) {
+ return this.shiftRight(numBits);
+ };
+ /**
+ * Returns this Long with bits logically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftRightUnsigned = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ numBits &= 63;
+ if (numBits === 0)
+ return this;
+ else {
+ var high = this.high;
+ if (numBits < 32) {
+ var low = this.low;
+ return Long.fromBits((low >>> numBits) | (high << (32 - numBits)), high >>> numBits, this.unsigned);
+ }
+ else if (numBits === 32)
+ return Long.fromBits(high, 0, this.unsigned);
+ else
+ return Long.fromBits(high >>> (numBits - 32), 0, this.unsigned);
+ }
+ };
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ Long.prototype.shr_u = function (numBits) {
+ return this.shiftRightUnsigned(numBits);
+ };
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ Long.prototype.shru = function (numBits) {
+ return this.shiftRightUnsigned(numBits);
+ };
+ /**
+ * Returns the difference of this and the specified Long.
+ * @param subtrahend - Subtrahend
+ * @returns Difference
+ */
+ Long.prototype.subtract = function (subtrahend) {
+ if (!Long.isLong(subtrahend))
+ subtrahend = Long.fromValue(subtrahend);
+ return this.add(subtrahend.neg());
+ };
+ /** This is an alias of {@link Long.subtract} */
+ Long.prototype.sub = function (subtrahend) {
+ return this.subtract(subtrahend);
+ };
+ /** Converts the Long to a 32 bit integer, assuming it is a 32 bit integer. */
+ Long.prototype.toInt = function () {
+ return this.unsigned ? this.low >>> 0 : this.low;
+ };
+ /** Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa). */
+ Long.prototype.toNumber = function () {
+ if (this.unsigned)
+ return (this.high >>> 0) * TWO_PWR_32_DBL + (this.low >>> 0);
+ return this.high * TWO_PWR_32_DBL + (this.low >>> 0);
+ };
+ /** Converts the Long to a BigInt (arbitrary precision). */
+ Long.prototype.toBigInt = function () {
+ return BigInt(this.toString());
+ };
+ /**
+ * Converts this Long to its byte representation.
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns Byte representation
+ */
+ Long.prototype.toBytes = function (le) {
+ return le ? this.toBytesLE() : this.toBytesBE();
+ };
+ /**
+ * Converts this Long to its little endian byte representation.
+ * @returns Little endian byte representation
+ */
+ Long.prototype.toBytesLE = function () {
+ var hi = this.high, lo = this.low;
+ return [
+ lo & 0xff,
+ (lo >>> 8) & 0xff,
+ (lo >>> 16) & 0xff,
+ lo >>> 24,
+ hi & 0xff,
+ (hi >>> 8) & 0xff,
+ (hi >>> 16) & 0xff,
+ hi >>> 24
+ ];
+ };
+ /**
+ * Converts this Long to its big endian byte representation.
+ * @returns Big endian byte representation
+ */
+ Long.prototype.toBytesBE = function () {
+ var hi = this.high, lo = this.low;
+ return [
+ hi >>> 24,
+ (hi >>> 16) & 0xff,
+ (hi >>> 8) & 0xff,
+ hi & 0xff,
+ lo >>> 24,
+ (lo >>> 16) & 0xff,
+ (lo >>> 8) & 0xff,
+ lo & 0xff
+ ];
+ };
+ /**
+ * Converts this Long to signed.
+ */
+ Long.prototype.toSigned = function () {
+ if (!this.unsigned)
+ return this;
+ return Long.fromBits(this.low, this.high, false);
+ };
+ /**
+ * Converts the Long to a string written in the specified radix.
+ * @param radix - Radix (2-36), defaults to 10
+ * @throws RangeError If `radix` is out of range
+ */
+ Long.prototype.toString = function (radix) {
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix)
+ throw RangeError('radix');
+ if (this.isZero())
+ return '0';
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ if (this.eq(Long.MIN_VALUE)) {
+ // We need to change the Long value before it can be negated, so we remove
+ // the bottom-most digit in this base and then recurse to do the rest.
+ var radixLong = Long.fromNumber(radix), div = this.div(radixLong), rem1 = div.mul(radixLong).sub(this);
+ return div.toString(radix) + rem1.toInt().toString(radix);
+ }
+ else
+ return '-' + this.neg().toString(radix);
+ }
+ // Do several (6) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ var radixToPower = Long.fromNumber(Math.pow(radix, 6), this.unsigned);
+ // eslint-disable-next-line @typescript-eslint/no-this-alias
+ var rem = this;
+ var result = '';
+ // eslint-disable-next-line no-constant-condition
+ while (true) {
+ var remDiv = rem.div(radixToPower);
+ var intval = rem.sub(remDiv.mul(radixToPower)).toInt() >>> 0;
+ var digits = intval.toString(radix);
+ rem = remDiv;
+ if (rem.isZero()) {
+ return digits + result;
+ }
+ else {
+ while (digits.length < 6)
+ digits = '0' + digits;
+ result = '' + digits + result;
+ }
+ }
+ };
+ /** Converts this Long to unsigned. */
+ Long.prototype.toUnsigned = function () {
+ if (this.unsigned)
+ return this;
+ return Long.fromBits(this.low, this.high, true);
+ };
+ /** Returns the bitwise XOR of this Long and the given one. */
+ Long.prototype.xor = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low ^ other.low, this.high ^ other.high, this.unsigned);
+ };
+ /** This is an alias of {@link Long.isZero} */
+ Long.prototype.eqz = function () {
+ return this.isZero();
+ };
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ Long.prototype.le = function (other) {
+ return this.lessThanOrEqual(other);
+ };
+ /*
+ ****************************************************************
+ * BSON SPECIFIC ADDITIONS *
+ ****************************************************************
+ */
+ Long.prototype.toExtendedJSON = function (options) {
+ if (options && options.relaxed)
+ return this.toNumber();
+ return { $numberLong: this.toString() };
+ };
+ Long.fromExtendedJSON = function (doc, options) {
+ var result = Long.fromString(doc.$numberLong);
+ return options && options.relaxed ? result.toNumber() : result;
+ };
+ /** @internal */
+ Long.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Long.prototype.inspect = function () {
+ return "new Long(\"" + this.toString() + "\"" + (this.unsigned ? ', true' : '') + ")";
+ };
+ Long.TWO_PWR_24 = Long.fromInt(TWO_PWR_24_DBL);
+ /** Maximum unsigned value. */
+ Long.MAX_UNSIGNED_VALUE = Long.fromBits(0xffffffff | 0, 0xffffffff | 0, true);
+ /** Signed zero */
+ Long.ZERO = Long.fromInt(0);
+ /** Unsigned zero. */
+ Long.UZERO = Long.fromInt(0, true);
+ /** Signed one. */
+ Long.ONE = Long.fromInt(1);
+ /** Unsigned one. */
+ Long.UONE = Long.fromInt(1, true);
+ /** Signed negative one. */
+ Long.NEG_ONE = Long.fromInt(-1);
+ /** Maximum signed value. */
+ Long.MAX_VALUE = Long.fromBits(0xffffffff | 0, 0x7fffffff | 0, false);
+ /** Minimum signed value. */
+ Long.MIN_VALUE = Long.fromBits(0, 0x80000000 | 0, false);
+ return Long;
+}());
+exports.Long = Long;
+Object.defineProperty(Long.prototype, '__isLong__', { value: true });
+Object.defineProperty(Long.prototype, '_bsontype', { value: 'Long' });
+
+});
+
+unwrapExports(long_1);
+long_1.Long;
+
+var decimal128 = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Decimal128 = void 0;
+
+
+var PARSE_STRING_REGEXP = /^(\+|-)?(\d+|(\d*\.\d*))?(E|e)?([-+])?(\d+)?$/;
+var PARSE_INF_REGEXP = /^(\+|-)?(Infinity|inf)$/i;
+var PARSE_NAN_REGEXP = /^(\+|-)?NaN$/i;
+var EXPONENT_MAX = 6111;
+var EXPONENT_MIN = -6176;
+var EXPONENT_BIAS = 6176;
+var MAX_DIGITS = 34;
+// Nan value bits as 32 bit values (due to lack of longs)
+var NAN_BUFFER = [
+ 0x7c,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+// Infinity value bits 32 bit values (due to lack of longs)
+var INF_NEGATIVE_BUFFER = [
+ 0xf8,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+var INF_POSITIVE_BUFFER = [
+ 0x78,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+var EXPONENT_REGEX = /^([-+])?(\d+)?$/;
+// Extract least significant 5 bits
+var COMBINATION_MASK = 0x1f;
+// Extract least significant 14 bits
+var EXPONENT_MASK = 0x3fff;
+// Value of combination field for Inf
+var COMBINATION_INFINITY = 30;
+// Value of combination field for NaN
+var COMBINATION_NAN = 31;
+// Detect if the value is a digit
+function isDigit(value) {
+ return !isNaN(parseInt(value, 10));
+}
+// Divide two uint128 values
+function divideu128(value) {
+ var DIVISOR = long_1.Long.fromNumber(1000 * 1000 * 1000);
+ var _rem = long_1.Long.fromNumber(0);
+ if (!value.parts[0] && !value.parts[1] && !value.parts[2] && !value.parts[3]) {
+ return { quotient: value, rem: _rem };
+ }
+ for (var i = 0; i <= 3; i++) {
+ // Adjust remainder to match value of next dividend
+ _rem = _rem.shiftLeft(32);
+ // Add the divided to _rem
+ _rem = _rem.add(new long_1.Long(value.parts[i], 0));
+ value.parts[i] = _rem.div(DIVISOR).low;
+ _rem = _rem.modulo(DIVISOR);
+ }
+ return { quotient: value, rem: _rem };
+}
+// Multiply two Long values and return the 128 bit value
+function multiply64x2(left, right) {
+ if (!left && !right) {
+ return { high: long_1.Long.fromNumber(0), low: long_1.Long.fromNumber(0) };
+ }
+ var leftHigh = left.shiftRightUnsigned(32);
+ var leftLow = new long_1.Long(left.getLowBits(), 0);
+ var rightHigh = right.shiftRightUnsigned(32);
+ var rightLow = new long_1.Long(right.getLowBits(), 0);
+ var productHigh = leftHigh.multiply(rightHigh);
+ var productMid = leftHigh.multiply(rightLow);
+ var productMid2 = leftLow.multiply(rightHigh);
+ var productLow = leftLow.multiply(rightLow);
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productMid = new long_1.Long(productMid.getLowBits(), 0)
+ .add(productMid2)
+ .add(productLow.shiftRightUnsigned(32));
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productLow = productMid.shiftLeft(32).add(new long_1.Long(productLow.getLowBits(), 0));
+ // Return the 128 bit result
+ return { high: productHigh, low: productLow };
+}
+function lessThan(left, right) {
+ // Make values unsigned
+ var uhleft = left.high >>> 0;
+ var uhright = right.high >>> 0;
+ // Compare high bits first
+ if (uhleft < uhright) {
+ return true;
+ }
+ else if (uhleft === uhright) {
+ var ulleft = left.low >>> 0;
+ var ulright = right.low >>> 0;
+ if (ulleft < ulright)
+ return true;
+ }
+ return false;
+}
+function invalidErr(string, message) {
+ throw new TypeError("\"" + string + "\" is not a valid Decimal128 string - " + message);
+}
+/**
+ * A class representation of the BSON Decimal128 type.
+ * @public
+ */
+var Decimal128 = /** @class */ (function () {
+ /**
+ * @param bytes - a buffer containing the raw Decimal128 bytes in little endian order,
+ * or a string representation as returned by .toString()
+ */
+ function Decimal128(bytes) {
+ if (!(this instanceof Decimal128))
+ return new Decimal128(bytes);
+ if (typeof bytes === 'string') {
+ this.bytes = Decimal128.fromString(bytes).bytes;
+ }
+ else {
+ this.bytes = bytes;
+ }
+ }
+ /**
+ * Create a Decimal128 instance from a string representation
+ *
+ * @param representation - a numeric string representation.
+ */
+ Decimal128.fromString = function (representation) {
+ // Parse state tracking
+ var isNegative = false;
+ var sawRadix = false;
+ var foundNonZero = false;
+ // Total number of significant digits (no leading or trailing zero)
+ var significantDigits = 0;
+ // Total number of significand digits read
+ var nDigitsRead = 0;
+ // Total number of digits (no leading zeros)
+ var nDigits = 0;
+ // The number of the digits after radix
+ var radixPosition = 0;
+ // The index of the first non-zero in *str*
+ var firstNonZero = 0;
+ // Digits Array
+ var digits = [0];
+ // The number of digits in digits
+ var nDigitsStored = 0;
+ // Insertion pointer for digits
+ var digitsInsert = 0;
+ // The index of the first non-zero digit
+ var firstDigit = 0;
+ // The index of the last digit
+ var lastDigit = 0;
+ // Exponent
+ var exponent = 0;
+ // loop index over array
+ var i = 0;
+ // The high 17 digits of the significand
+ var significandHigh = new long_1.Long(0, 0);
+ // The low 17 digits of the significand
+ var significandLow = new long_1.Long(0, 0);
+ // The biased exponent
+ var biasedExponent = 0;
+ // Read index
+ var index = 0;
+ // Naively prevent against REDOS attacks.
+ // TODO: implementing a custom parsing for this, or refactoring the regex would yield
+ // further gains.
+ if (representation.length >= 7000) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+ // Results
+ var stringMatch = representation.match(PARSE_STRING_REGEXP);
+ var infMatch = representation.match(PARSE_INF_REGEXP);
+ var nanMatch = representation.match(PARSE_NAN_REGEXP);
+ // Validate the string
+ if ((!stringMatch && !infMatch && !nanMatch) || representation.length === 0) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+ if (stringMatch) {
+ // full_match = stringMatch[0]
+ // sign = stringMatch[1]
+ var unsignedNumber = stringMatch[2];
+ // stringMatch[3] is undefined if a whole number (ex "1", 12")
+ // but defined if a number w/ decimal in it (ex "1.0, 12.2")
+ var e = stringMatch[4];
+ var expSign = stringMatch[5];
+ var expNumber = stringMatch[6];
+ // they provided e, but didn't give an exponent number. for ex "1e"
+ if (e && expNumber === undefined)
+ invalidErr(representation, 'missing exponent power');
+ // they provided e, but didn't give a number before it. for ex "e1"
+ if (e && unsignedNumber === undefined)
+ invalidErr(representation, 'missing exponent base');
+ if (e === undefined && (expSign || expNumber)) {
+ invalidErr(representation, 'missing e before exponent');
+ }
+ }
+ // Get the negative or positive sign
+ if (representation[index] === '+' || representation[index] === '-') {
+ isNegative = representation[index++] === '-';
+ }
+ // Check if user passed Infinity or NaN
+ if (!isDigit(representation[index]) && representation[index] !== '.') {
+ if (representation[index] === 'i' || representation[index] === 'I') {
+ return new Decimal128(buffer.Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ }
+ else if (representation[index] === 'N') {
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ }
+ }
+ // Read all the digits
+ while (isDigit(representation[index]) || representation[index] === '.') {
+ if (representation[index] === '.') {
+ if (sawRadix)
+ invalidErr(representation, 'contains multiple periods');
+ sawRadix = true;
+ index = index + 1;
+ continue;
+ }
+ if (nDigitsStored < 34) {
+ if (representation[index] !== '0' || foundNonZero) {
+ if (!foundNonZero) {
+ firstNonZero = nDigitsRead;
+ }
+ foundNonZero = true;
+ // Only store 34 digits
+ digits[digitsInsert++] = parseInt(representation[index], 10);
+ nDigitsStored = nDigitsStored + 1;
+ }
+ }
+ if (foundNonZero)
+ nDigits = nDigits + 1;
+ if (sawRadix)
+ radixPosition = radixPosition + 1;
+ nDigitsRead = nDigitsRead + 1;
+ index = index + 1;
+ }
+ if (sawRadix && !nDigitsRead)
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ // Read exponent if exists
+ if (representation[index] === 'e' || representation[index] === 'E') {
+ // Read exponent digits
+ var match = representation.substr(++index).match(EXPONENT_REGEX);
+ // No digits read
+ if (!match || !match[2])
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ // Get exponent
+ exponent = parseInt(match[0], 10);
+ // Adjust the index
+ index = index + match[0].length;
+ }
+ // Return not a number
+ if (representation[index])
+ return new Decimal128(buffer.Buffer.from(NAN_BUFFER));
+ // Done reading input
+ // Find first non-zero digit in digits
+ firstDigit = 0;
+ if (!nDigitsStored) {
+ firstDigit = 0;
+ lastDigit = 0;
+ digits[0] = 0;
+ nDigits = 1;
+ nDigitsStored = 1;
+ significantDigits = 0;
+ }
+ else {
+ lastDigit = nDigitsStored - 1;
+ significantDigits = nDigits;
+ if (significantDigits !== 1) {
+ while (representation[firstNonZero + significantDigits - 1] === '0') {
+ significantDigits = significantDigits - 1;
+ }
+ }
+ }
+ // Normalization of exponent
+ // Correct exponent based on radix position, and shift significand as needed
+ // to represent user input
+ // Overflow prevention
+ if (exponent <= radixPosition && radixPosition - exponent > 1 << 14) {
+ exponent = EXPONENT_MIN;
+ }
+ else {
+ exponent = exponent - radixPosition;
+ }
+ // Attempt to normalize the exponent
+ while (exponent > EXPONENT_MAX) {
+ // Shift exponent to significand and decrease
+ lastDigit = lastDigit + 1;
+ if (lastDigit - firstDigit > MAX_DIGITS) {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ var digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ exponent = exponent - 1;
+ }
+ while (exponent < EXPONENT_MIN || nDigitsStored < nDigits) {
+ // Shift last digit. can only do this if < significant digits than # stored.
+ if (lastDigit === 0 && significantDigits < nDigitsStored) {
+ exponent = EXPONENT_MIN;
+ significantDigits = 0;
+ break;
+ }
+ if (nDigitsStored < nDigits) {
+ // adjust to match digits not stored
+ nDigits = nDigits - 1;
+ }
+ else {
+ // adjust to round
+ lastDigit = lastDigit - 1;
+ }
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ }
+ else {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ var digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ }
+ // Round
+ // We've normalized the exponent, but might still need to round.
+ if (lastDigit - firstDigit + 1 < significantDigits) {
+ var endOfString = nDigitsRead;
+ // If we have seen a radix point, 'string' is 1 longer than we have
+ // documented with ndigits_read, so inc the position of the first nonzero
+ // digit and the position that digits are read to.
+ if (sawRadix) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ // if negative, we need to increment again to account for - sign at start.
+ if (isNegative) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ var roundDigit = parseInt(representation[firstNonZero + lastDigit + 1], 10);
+ var roundBit = 0;
+ if (roundDigit >= 5) {
+ roundBit = 1;
+ if (roundDigit === 5) {
+ roundBit = digits[lastDigit] % 2 === 1 ? 1 : 0;
+ for (i = firstNonZero + lastDigit + 2; i < endOfString; i++) {
+ if (parseInt(representation[i], 10)) {
+ roundBit = 1;
+ break;
+ }
+ }
+ }
+ }
+ if (roundBit) {
+ var dIdx = lastDigit;
+ for (; dIdx >= 0; dIdx--) {
+ if (++digits[dIdx] > 9) {
+ digits[dIdx] = 0;
+ // overflowed most significant digit
+ if (dIdx === 0) {
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ digits[dIdx] = 1;
+ }
+ else {
+ return new Decimal128(buffer.Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ }
+ }
+ }
+ }
+ }
+ }
+ // Encode significand
+ // The high 17 digits of the significand
+ significandHigh = long_1.Long.fromNumber(0);
+ // The low 17 digits of the significand
+ significandLow = long_1.Long.fromNumber(0);
+ // read a zero
+ if (significantDigits === 0) {
+ significandHigh = long_1.Long.fromNumber(0);
+ significandLow = long_1.Long.fromNumber(0);
+ }
+ else if (lastDigit - firstDigit < 17) {
+ var dIdx = firstDigit;
+ significandLow = long_1.Long.fromNumber(digits[dIdx++]);
+ significandHigh = new long_1.Long(0, 0);
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(long_1.Long.fromNumber(10));
+ significandLow = significandLow.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ }
+ else {
+ var dIdx = firstDigit;
+ significandHigh = long_1.Long.fromNumber(digits[dIdx++]);
+ for (; dIdx <= lastDigit - 17; dIdx++) {
+ significandHigh = significandHigh.multiply(long_1.Long.fromNumber(10));
+ significandHigh = significandHigh.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ significandLow = long_1.Long.fromNumber(digits[dIdx++]);
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(long_1.Long.fromNumber(10));
+ significandLow = significandLow.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ }
+ var significand = multiply64x2(significandHigh, long_1.Long.fromString('100000000000000000'));
+ significand.low = significand.low.add(significandLow);
+ if (lessThan(significand.low, significandLow)) {
+ significand.high = significand.high.add(long_1.Long.fromNumber(1));
+ }
+ // Biased exponent
+ biasedExponent = exponent + EXPONENT_BIAS;
+ var dec = { low: long_1.Long.fromNumber(0), high: long_1.Long.fromNumber(0) };
+ // Encode combination, exponent, and significand.
+ if (significand.high.shiftRightUnsigned(49).and(long_1.Long.fromNumber(1)).equals(long_1.Long.fromNumber(1))) {
+ // Encode '11' into bits 1 to 3
+ dec.high = dec.high.or(long_1.Long.fromNumber(0x3).shiftLeft(61));
+ dec.high = dec.high.or(long_1.Long.fromNumber(biasedExponent).and(long_1.Long.fromNumber(0x3fff).shiftLeft(47)));
+ dec.high = dec.high.or(significand.high.and(long_1.Long.fromNumber(0x7fffffffffff)));
+ }
+ else {
+ dec.high = dec.high.or(long_1.Long.fromNumber(biasedExponent & 0x3fff).shiftLeft(49));
+ dec.high = dec.high.or(significand.high.and(long_1.Long.fromNumber(0x1ffffffffffff)));
+ }
+ dec.low = significand.low;
+ // Encode sign
+ if (isNegative) {
+ dec.high = dec.high.or(long_1.Long.fromString('9223372036854775808'));
+ }
+ // Encode into a buffer
+ var buffer$1 = buffer.Buffer.alloc(16);
+ index = 0;
+ // Encode the low 64 bits of the decimal
+ // Encode low bits
+ buffer$1[index++] = dec.low.low & 0xff;
+ buffer$1[index++] = (dec.low.low >> 8) & 0xff;
+ buffer$1[index++] = (dec.low.low >> 16) & 0xff;
+ buffer$1[index++] = (dec.low.low >> 24) & 0xff;
+ // Encode high bits
+ buffer$1[index++] = dec.low.high & 0xff;
+ buffer$1[index++] = (dec.low.high >> 8) & 0xff;
+ buffer$1[index++] = (dec.low.high >> 16) & 0xff;
+ buffer$1[index++] = (dec.low.high >> 24) & 0xff;
+ // Encode the high 64 bits of the decimal
+ // Encode low bits
+ buffer$1[index++] = dec.high.low & 0xff;
+ buffer$1[index++] = (dec.high.low >> 8) & 0xff;
+ buffer$1[index++] = (dec.high.low >> 16) & 0xff;
+ buffer$1[index++] = (dec.high.low >> 24) & 0xff;
+ // Encode high bits
+ buffer$1[index++] = dec.high.high & 0xff;
+ buffer$1[index++] = (dec.high.high >> 8) & 0xff;
+ buffer$1[index++] = (dec.high.high >> 16) & 0xff;
+ buffer$1[index++] = (dec.high.high >> 24) & 0xff;
+ // Return the new Decimal128
+ return new Decimal128(buffer$1);
+ };
+ /** Create a string representation of the raw Decimal128 value */
+ Decimal128.prototype.toString = function () {
+ // Note: bits in this routine are referred to starting at 0,
+ // from the sign bit, towards the coefficient.
+ // decoded biased exponent (14 bits)
+ var biased_exponent;
+ // the number of significand digits
+ var significand_digits = 0;
+ // the base-10 digits in the significand
+ var significand = new Array(36);
+ for (var i = 0; i < significand.length; i++)
+ significand[i] = 0;
+ // read pointer into significand
+ var index = 0;
+ // true if the number is zero
+ var is_zero = false;
+ // the most significant significand bits (50-46)
+ var significand_msb;
+ // temporary storage for significand decoding
+ var significand128 = { parts: [0, 0, 0, 0] };
+ // indexing variables
+ var j, k;
+ // Output string
+ var string = [];
+ // Unpack index
+ index = 0;
+ // Buffer reference
+ var buffer = this.bytes;
+ // Unpack the low 64bits into a long
+ // bits 96 - 127
+ var low = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 64 - 95
+ var midl = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Unpack the high 64bits into a long
+ // bits 32 - 63
+ var midh = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 0 - 31
+ var high = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Unpack index
+ index = 0;
+ // Create the state of the decimal
+ var dec = {
+ low: new long_1.Long(low, midl),
+ high: new long_1.Long(midh, high)
+ };
+ if (dec.high.lessThan(long_1.Long.ZERO)) {
+ string.push('-');
+ }
+ // Decode combination field and exponent
+ // bits 1 - 5
+ var combination = (high >> 26) & COMBINATION_MASK;
+ if (combination >> 3 === 3) {
+ // Check for 'special' values
+ if (combination === COMBINATION_INFINITY) {
+ return string.join('') + 'Infinity';
+ }
+ else if (combination === COMBINATION_NAN) {
+ return 'NaN';
+ }
+ else {
+ biased_exponent = (high >> 15) & EXPONENT_MASK;
+ significand_msb = 0x08 + ((high >> 14) & 0x01);
+ }
+ }
+ else {
+ significand_msb = (high >> 14) & 0x07;
+ biased_exponent = (high >> 17) & EXPONENT_MASK;
+ }
+ // unbiased exponent
+ var exponent = biased_exponent - EXPONENT_BIAS;
+ // Create string of significand digits
+ // Convert the 114-bit binary number represented by
+ // (significand_high, significand_low) to at most 34 decimal
+ // digits through modulo and division.
+ significand128.parts[0] = (high & 0x3fff) + ((significand_msb & 0xf) << 14);
+ significand128.parts[1] = midh;
+ significand128.parts[2] = midl;
+ significand128.parts[3] = low;
+ if (significand128.parts[0] === 0 &&
+ significand128.parts[1] === 0 &&
+ significand128.parts[2] === 0 &&
+ significand128.parts[3] === 0) {
+ is_zero = true;
+ }
+ else {
+ for (k = 3; k >= 0; k--) {
+ var least_digits = 0;
+ // Perform the divide
+ var result = divideu128(significand128);
+ significand128 = result.quotient;
+ least_digits = result.rem.low;
+ // We now have the 9 least significant digits (in base 2).
+ // Convert and output to string.
+ if (!least_digits)
+ continue;
+ for (j = 8; j >= 0; j--) {
+ // significand[k * 9 + j] = Math.round(least_digits % 10);
+ significand[k * 9 + j] = least_digits % 10;
+ // least_digits = Math.round(least_digits / 10);
+ least_digits = Math.floor(least_digits / 10);
+ }
+ }
+ }
+ // Output format options:
+ // Scientific - [-]d.dddE(+/-)dd or [-]dE(+/-)dd
+ // Regular - ddd.ddd
+ if (is_zero) {
+ significand_digits = 1;
+ significand[index] = 0;
+ }
+ else {
+ significand_digits = 36;
+ while (!significand[index]) {
+ significand_digits = significand_digits - 1;
+ index = index + 1;
+ }
+ }
+ // the exponent if scientific notation is used
+ var scientific_exponent = significand_digits - 1 + exponent;
+ // The scientific exponent checks are dictated by the string conversion
+ // specification and are somewhat arbitrary cutoffs.
+ //
+ // We must check exponent > 0, because if this is the case, the number
+ // has trailing zeros. However, we *cannot* output these trailing zeros,
+ // because doing so would change the precision of the value, and would
+ // change stored data if the string converted number is round tripped.
+ if (scientific_exponent >= 34 || scientific_exponent <= -7 || exponent > 0) {
+ // Scientific format
+ // if there are too many significant digits, we should just be treating numbers
+ // as + or - 0 and using the non-scientific exponent (this is for the "invalid
+ // representation should be treated as 0/-0" spec cases in decimal128-1.json)
+ if (significand_digits > 34) {
+ string.push("" + 0);
+ if (exponent > 0)
+ string.push('E+' + exponent);
+ else if (exponent < 0)
+ string.push('E' + exponent);
+ return string.join('');
+ }
+ string.push("" + significand[index++]);
+ significand_digits = significand_digits - 1;
+ if (significand_digits) {
+ string.push('.');
+ }
+ for (var i = 0; i < significand_digits; i++) {
+ string.push("" + significand[index++]);
+ }
+ // Exponent
+ string.push('E');
+ if (scientific_exponent > 0) {
+ string.push('+' + scientific_exponent);
+ }
+ else {
+ string.push("" + scientific_exponent);
+ }
+ }
+ else {
+ // Regular format with no decimal place
+ if (exponent >= 0) {
+ for (var i = 0; i < significand_digits; i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ else {
+ var radix_position = significand_digits + exponent;
+ // non-zero digits before radix
+ if (radix_position > 0) {
+ for (var i = 0; i < radix_position; i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ else {
+ string.push('0');
+ }
+ string.push('.');
+ // add leading zeros after radix
+ while (radix_position++ < 0) {
+ string.push('0');
+ }
+ for (var i = 0; i < significand_digits - Math.max(radix_position - 1, 0); i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ }
+ return string.join('');
+ };
+ Decimal128.prototype.toJSON = function () {
+ return { $numberDecimal: this.toString() };
+ };
+ /** @internal */
+ Decimal128.prototype.toExtendedJSON = function () {
+ return { $numberDecimal: this.toString() };
+ };
+ /** @internal */
+ Decimal128.fromExtendedJSON = function (doc) {
+ return Decimal128.fromString(doc.$numberDecimal);
+ };
+ /** @internal */
+ Decimal128.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Decimal128.prototype.inspect = function () {
+ return "new Decimal128(\"" + this.toString() + "\")";
+ };
+ return Decimal128;
+}());
+exports.Decimal128 = Decimal128;
+Object.defineProperty(Decimal128.prototype, '_bsontype', { value: 'Decimal128' });
+
+});
+
+unwrapExports(decimal128);
+decimal128.Decimal128;
+
+var double_1 = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Double = void 0;
+/**
+ * A class representation of the BSON Double type.
+ * @public
+ */
+var Double = /** @class */ (function () {
+ /**
+ * Create a Double type
+ *
+ * @param value - the number we want to represent as a double.
+ */
+ function Double(value) {
+ if (!(this instanceof Double))
+ return new Double(value);
+ if (value instanceof Number) {
+ value = value.valueOf();
+ }
+ this.value = +value;
+ }
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped double number.
+ */
+ Double.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ Double.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ Double.prototype.toExtendedJSON = function (options) {
+ if (options && (options.legacy || (options.relaxed && isFinite(this.value)))) {
+ return this.value;
+ }
+ // NOTE: JavaScript has +0 and -0, apparently to model limit calculations. If a user
+ // explicitly provided `-0` then we need to ensure the sign makes it into the output
+ if (Object.is(Math.sign(this.value), -0)) {
+ return { $numberDouble: "-" + this.value.toFixed(1) };
+ }
+ var $numberDouble;
+ if (Number.isInteger(this.value)) {
+ $numberDouble = this.value.toFixed(1);
+ if ($numberDouble.length >= 13) {
+ $numberDouble = this.value.toExponential(13).toUpperCase();
+ }
+ }
+ else {
+ $numberDouble = this.value.toString();
+ }
+ return { $numberDouble: $numberDouble };
+ };
+ /** @internal */
+ Double.fromExtendedJSON = function (doc, options) {
+ var doubleValue = parseFloat(doc.$numberDouble);
+ return options && options.relaxed ? doubleValue : new Double(doubleValue);
+ };
+ /** @internal */
+ Double.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Double.prototype.inspect = function () {
+ var eJSON = this.toExtendedJSON();
+ return "new Double(" + eJSON.$numberDouble + ")";
+ };
+ return Double;
+}());
+exports.Double = Double;
+Object.defineProperty(Double.prototype, '_bsontype', { value: 'Double' });
+
+});
+
+unwrapExports(double_1);
+double_1.Double;
+
+var int_32 = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Int32 = void 0;
+/**
+ * A class representation of a BSON Int32 type.
+ * @public
+ */
+var Int32 = /** @class */ (function () {
+ /**
+ * Create an Int32 type
+ *
+ * @param value - the number we want to represent as an int32.
+ */
+ function Int32(value) {
+ if (!(this instanceof Int32))
+ return new Int32(value);
+ if (value instanceof Number) {
+ value = value.valueOf();
+ }
+ this.value = +value;
+ }
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped int32 number.
+ */
+ Int32.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ Int32.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ Int32.prototype.toExtendedJSON = function (options) {
+ if (options && (options.relaxed || options.legacy))
+ return this.value;
+ return { $numberInt: this.value.toString() };
+ };
+ /** @internal */
+ Int32.fromExtendedJSON = function (doc, options) {
+ return options && options.relaxed ? parseInt(doc.$numberInt, 10) : new Int32(doc.$numberInt);
+ };
+ /** @internal */
+ Int32.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Int32.prototype.inspect = function () {
+ return "new Int32(" + this.valueOf() + ")";
+ };
+ return Int32;
+}());
+exports.Int32 = Int32;
+Object.defineProperty(Int32.prototype, '_bsontype', { value: 'Int32' });
+
+});
+
+unwrapExports(int_32);
+int_32.Int32;
+
+var max_key = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.MaxKey = void 0;
+/**
+ * A class representation of the BSON MaxKey type.
+ * @public
+ */
+var MaxKey = /** @class */ (function () {
+ function MaxKey() {
+ if (!(this instanceof MaxKey))
+ return new MaxKey();
+ }
+ /** @internal */
+ MaxKey.prototype.toExtendedJSON = function () {
+ return { $maxKey: 1 };
+ };
+ /** @internal */
+ MaxKey.fromExtendedJSON = function () {
+ return new MaxKey();
+ };
+ /** @internal */
+ MaxKey.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ MaxKey.prototype.inspect = function () {
+ return 'new MaxKey()';
+ };
+ return MaxKey;
+}());
+exports.MaxKey = MaxKey;
+Object.defineProperty(MaxKey.prototype, '_bsontype', { value: 'MaxKey' });
+
+});
+
+unwrapExports(max_key);
+max_key.MaxKey;
+
+var min_key = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.MinKey = void 0;
+/**
+ * A class representation of the BSON MinKey type.
+ * @public
+ */
+var MinKey = /** @class */ (function () {
+ function MinKey() {
+ if (!(this instanceof MinKey))
+ return new MinKey();
+ }
+ /** @internal */
+ MinKey.prototype.toExtendedJSON = function () {
+ return { $minKey: 1 };
+ };
+ /** @internal */
+ MinKey.fromExtendedJSON = function () {
+ return new MinKey();
+ };
+ /** @internal */
+ MinKey.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ MinKey.prototype.inspect = function () {
+ return 'new MinKey()';
+ };
+ return MinKey;
+}());
+exports.MinKey = MinKey;
+Object.defineProperty(MinKey.prototype, '_bsontype', { value: 'MinKey' });
+
+});
+
+unwrapExports(min_key);
+min_key.MinKey;
+
+var objectid = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.ObjectId = void 0;
+
+
+
+// Regular expression that checks for hex value
+var checkForHexRegExp = new RegExp('^[0-9a-fA-F]{24}$');
+// Unique sequence for the current process (initialized on first use)
+var PROCESS_UNIQUE = null;
+var kId = Symbol('id');
+/**
+ * A class representation of the BSON ObjectId type.
+ * @public
+ */
+var ObjectId = /** @class */ (function () {
+ /**
+ * Create an ObjectId type
+ *
+ * @param id - Can be a 24 character hex string, 12 byte binary Buffer, or a number.
+ */
+ function ObjectId(id) {
+ if (!(this instanceof ObjectId))
+ return new ObjectId(id);
+ // Duck-typing to support ObjectId from different npm packages
+ if (id instanceof ObjectId) {
+ this[kId] = id.id;
+ this.__id = id.__id;
+ }
+ if (typeof id === 'object' && id && 'id' in id) {
+ if ('toHexString' in id && typeof id.toHexString === 'function') {
+ this[kId] = buffer.Buffer.from(id.toHexString(), 'hex');
+ }
+ else {
+ this[kId] = typeof id.id === 'string' ? buffer.Buffer.from(id.id) : id.id;
+ }
+ }
+ // The most common use case (blank id, new objectId instance)
+ if (id == null || typeof id === 'number') {
+ // Generate a new id
+ this[kId] = ObjectId.generate(typeof id === 'number' ? id : undefined);
+ // If we are caching the hex string
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+ if (ArrayBuffer.isView(id) && id.byteLength === 12) {
+ this[kId] = ensure_buffer.ensureBuffer(id);
+ }
+ if (typeof id === 'string') {
+ if (id.length === 12) {
+ var bytes = buffer.Buffer.from(id);
+ if (bytes.byteLength === 12) {
+ this[kId] = bytes;
+ }
+ }
+ else if (id.length === 24 && checkForHexRegExp.test(id)) {
+ this[kId] = buffer.Buffer.from(id, 'hex');
+ }
+ else {
+ throw new TypeError('Argument passed in must be a Buffer or string of 12 bytes or a string of 24 hex characters');
+ }
+ }
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+ Object.defineProperty(ObjectId.prototype, "id", {
+ /**
+ * The ObjectId bytes
+ * @readonly
+ */
+ get: function () {
+ return this[kId];
+ },
+ set: function (value) {
+ this[kId] = value;
+ if (ObjectId.cacheHexString) {
+ this.__id = value.toString('hex');
+ }
+ },
+ enumerable: false,
+ configurable: true
+ });
+ Object.defineProperty(ObjectId.prototype, "generationTime", {
+ /**
+ * The generation time of this ObjectId instance
+ * @deprecated Please use getTimestamp / createFromTime which returns an int32 epoch
+ */
+ get: function () {
+ return this.id.readInt32BE(0);
+ },
+ set: function (value) {
+ // Encode time into first 4 bytes
+ this.id.writeUInt32BE(value, 0);
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /** Returns the ObjectId id as a 24 character hex string representation */
+ ObjectId.prototype.toHexString = function () {
+ if (ObjectId.cacheHexString && this.__id) {
+ return this.__id;
+ }
+ var hexString = this.id.toString('hex');
+ if (ObjectId.cacheHexString && !this.__id) {
+ this.__id = hexString;
+ }
+ return hexString;
+ };
+ /**
+ * Update the ObjectId index
+ * @privateRemarks
+ * Used in generating new ObjectId's on the driver
+ * @internal
+ */
+ ObjectId.getInc = function () {
+ return (ObjectId.index = (ObjectId.index + 1) % 0xffffff);
+ };
+ /**
+ * Generate a 12 byte id buffer used in ObjectId's
+ *
+ * @param time - pass in a second based timestamp.
+ */
+ ObjectId.generate = function (time) {
+ if ('number' !== typeof time) {
+ time = ~~(Date.now() / 1000);
+ }
+ var inc = ObjectId.getInc();
+ var buffer$1 = buffer.Buffer.alloc(12);
+ // 4-byte timestamp
+ buffer$1.writeUInt32BE(time, 0);
+ // set PROCESS_UNIQUE if yet not initialized
+ if (PROCESS_UNIQUE === null) {
+ PROCESS_UNIQUE = utils.randomBytes(5);
+ }
+ // 5-byte process unique
+ buffer$1[4] = PROCESS_UNIQUE[0];
+ buffer$1[5] = PROCESS_UNIQUE[1];
+ buffer$1[6] = PROCESS_UNIQUE[2];
+ buffer$1[7] = PROCESS_UNIQUE[3];
+ buffer$1[8] = PROCESS_UNIQUE[4];
+ // 3-byte counter
+ buffer$1[11] = inc & 0xff;
+ buffer$1[10] = (inc >> 8) & 0xff;
+ buffer$1[9] = (inc >> 16) & 0xff;
+ return buffer$1;
+ };
+ /**
+ * Converts the id into a 24 character hex string for printing
+ *
+ * @param format - The Buffer toString format parameter.
+ * @internal
+ */
+ ObjectId.prototype.toString = function (format) {
+ // Is the id a buffer then use the buffer toString method to return the format
+ if (format)
+ return this.id.toString(format);
+ return this.toHexString();
+ };
+ /**
+ * Converts to its JSON the 24 character hex string representation.
+ * @internal
+ */
+ ObjectId.prototype.toJSON = function () {
+ return this.toHexString();
+ };
+ /**
+ * Compares the equality of this ObjectId with `otherID`.
+ *
+ * @param otherId - ObjectId instance to compare against.
+ */
+ ObjectId.prototype.equals = function (otherId) {
+ if (otherId === undefined || otherId === null) {
+ return false;
+ }
+ if (otherId instanceof ObjectId) {
+ return this.toString() === otherId.toString();
+ }
+ if (typeof otherId === 'string' &&
+ ObjectId.isValid(otherId) &&
+ otherId.length === 12 &&
+ utils.isUint8Array(this.id)) {
+ return otherId === buffer.Buffer.prototype.toString.call(this.id, 'latin1');
+ }
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 24) {
+ return otherId.toLowerCase() === this.toHexString();
+ }
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 12) {
+ return buffer.Buffer.from(otherId).equals(this.id);
+ }
+ if (typeof otherId === 'object' &&
+ 'toHexString' in otherId &&
+ typeof otherId.toHexString === 'function') {
+ return otherId.toHexString() === this.toHexString();
+ }
+ return false;
+ };
+ /** Returns the generation date (accurate up to the second) that this ID was generated. */
+ ObjectId.prototype.getTimestamp = function () {
+ var timestamp = new Date();
+ var time = this.id.readUInt32BE(0);
+ timestamp.setTime(Math.floor(time) * 1000);
+ return timestamp;
+ };
+ /** @internal */
+ ObjectId.createPk = function () {
+ return new ObjectId();
+ };
+ /**
+ * Creates an ObjectId from a second based number, with the rest of the ObjectId zeroed out. Used for comparisons or sorting the ObjectId.
+ *
+ * @param time - an integer number representing a number of seconds.
+ */
+ ObjectId.createFromTime = function (time) {
+ var buffer$1 = buffer.Buffer.from([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]);
+ // Encode time into first 4 bytes
+ buffer$1.writeUInt32BE(time, 0);
+ // Return the new objectId
+ return new ObjectId(buffer$1);
+ };
+ /**
+ * Creates an ObjectId from a hex string representation of an ObjectId.
+ *
+ * @param hexString - create a ObjectId from a passed in 24 character hexstring.
+ */
+ ObjectId.createFromHexString = function (hexString) {
+ // Throw an error if it's not a valid setup
+ if (typeof hexString === 'undefined' || (hexString != null && hexString.length !== 24)) {
+ throw new TypeError('Argument passed in must be a single String of 12 bytes or a string of 24 hex characters');
+ }
+ return new ObjectId(buffer.Buffer.from(hexString, 'hex'));
+ };
+ /**
+ * Checks if a value is a valid bson ObjectId
+ *
+ * @param id - ObjectId instance to validate.
+ */
+ ObjectId.isValid = function (id) {
+ if (id == null)
+ return false;
+ if (typeof id === 'number') {
+ return true;
+ }
+ if (typeof id === 'string') {
+ return id.length === 12 || (id.length === 24 && checkForHexRegExp.test(id));
+ }
+ if (id instanceof ObjectId) {
+ return true;
+ }
+ if (utils.isUint8Array(id) && id.length === 12) {
+ return true;
+ }
+ // Duck-Typing detection of ObjectId like objects
+ if (typeof id === 'object' && 'toHexString' in id && typeof id.toHexString === 'function') {
+ if (typeof id.id === 'string') {
+ return id.id.length === 12;
+ }
+ return id.toHexString().length === 24 && checkForHexRegExp.test(id.id.toString('hex'));
+ }
+ return false;
+ };
+ /** @internal */
+ ObjectId.prototype.toExtendedJSON = function () {
+ if (this.toHexString)
+ return { $oid: this.toHexString() };
+ return { $oid: this.toString('hex') };
+ };
+ /** @internal */
+ ObjectId.fromExtendedJSON = function (doc) {
+ return new ObjectId(doc.$oid);
+ };
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 24 character hex string representation.
+ * @internal
+ */
+ ObjectId.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ ObjectId.prototype.inspect = function () {
+ return "new ObjectId(\"" + this.toHexString() + "\")";
+ };
+ /** @internal */
+ ObjectId.index = ~~(Math.random() * 0xffffff);
+ return ObjectId;
+}());
+exports.ObjectId = ObjectId;
+// Deprecated methods
+Object.defineProperty(ObjectId.prototype, 'generate', {
+ value: utils.deprecate(function (time) { return ObjectId.generate(time); }, 'Please use the static `ObjectId.generate(time)` instead')
+});
+Object.defineProperty(ObjectId.prototype, 'getInc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+});
+Object.defineProperty(ObjectId.prototype, 'get_inc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+});
+Object.defineProperty(ObjectId, 'get_inc', {
+ value: utils.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+});
+Object.defineProperty(ObjectId.prototype, '_bsontype', { value: 'ObjectID' });
+
+});
+
+unwrapExports(objectid);
+objectid.ObjectId;
+
+var regexp = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.BSONRegExp = void 0;
+function alphabetize(str) {
+ return str.split('').sort().join('');
+}
+/**
+ * A class representation of the BSON RegExp type.
+ * @public
+ */
+var BSONRegExp = /** @class */ (function () {
+ /**
+ * @param pattern - The regular expression pattern to match
+ * @param options - The regular expression options
+ */
+ function BSONRegExp(pattern, options) {
+ if (!(this instanceof BSONRegExp))
+ return new BSONRegExp(pattern, options);
+ this.pattern = pattern;
+ this.options = alphabetize(options !== null && options !== void 0 ? options : '');
+ // Validate options
+ for (var i = 0; i < this.options.length; i++) {
+ if (!(this.options[i] === 'i' ||
+ this.options[i] === 'm' ||
+ this.options[i] === 'x' ||
+ this.options[i] === 'l' ||
+ this.options[i] === 's' ||
+ this.options[i] === 'u')) {
+ throw new Error("The regular expression option [" + this.options[i] + "] is not supported");
+ }
+ }
+ }
+ BSONRegExp.parseOptions = function (options) {
+ return options ? options.split('').sort().join('') : '';
+ };
+ /** @internal */
+ BSONRegExp.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ if (options.legacy) {
+ return { $regex: this.pattern, $options: this.options };
+ }
+ return { $regularExpression: { pattern: this.pattern, options: this.options } };
+ };
+ /** @internal */
+ BSONRegExp.fromExtendedJSON = function (doc) {
+ if ('$regex' in doc) {
+ if (typeof doc.$regex !== 'string') {
+ // This is for $regex query operators that have extended json values.
+ if (doc.$regex._bsontype === 'BSONRegExp') {
+ return doc;
+ }
+ }
+ else {
+ return new BSONRegExp(doc.$regex, BSONRegExp.parseOptions(doc.$options));
+ }
+ }
+ if ('$regularExpression' in doc) {
+ return new BSONRegExp(doc.$regularExpression.pattern, BSONRegExp.parseOptions(doc.$regularExpression.options));
+ }
+ throw new TypeError("Unexpected BSONRegExp EJSON object form: " + JSON.stringify(doc));
+ };
+ return BSONRegExp;
+}());
+exports.BSONRegExp = BSONRegExp;
+Object.defineProperty(BSONRegExp.prototype, '_bsontype', { value: 'BSONRegExp' });
+
+});
+
+unwrapExports(regexp);
+regexp.BSONRegExp;
+
+var symbol = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.BSONSymbol = void 0;
+/**
+ * A class representation of the BSON Symbol type.
+ * @public
+ */
+var BSONSymbol = /** @class */ (function () {
+ /**
+ * @param value - the string representing the symbol.
+ */
+ function BSONSymbol(value) {
+ if (!(this instanceof BSONSymbol))
+ return new BSONSymbol(value);
+ this.value = value;
+ }
+ /** Access the wrapped string value. */
+ BSONSymbol.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.toString = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.inspect = function () {
+ return "new BSONSymbol(\"" + this.value + "\")";
+ };
+ /** @internal */
+ BSONSymbol.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.toExtendedJSON = function () {
+ return { $symbol: this.value };
+ };
+ /** @internal */
+ BSONSymbol.fromExtendedJSON = function (doc) {
+ return new BSONSymbol(doc.$symbol);
+ };
+ /** @internal */
+ BSONSymbol.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ return BSONSymbol;
+}());
+exports.BSONSymbol = BSONSymbol;
+Object.defineProperty(BSONSymbol.prototype, '_bsontype', { value: 'Symbol' });
+
+});
+
+unwrapExports(symbol);
+symbol.BSONSymbol;
+
+/*! *****************************************************************************
+Copyright (c) Microsoft Corporation.
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH
+REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY
+AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,
+INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM
+LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR
+OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR
+PERFORMANCE OF THIS SOFTWARE.
+***************************************************************************** */
+
+/* global Reflect, Promise */
+var _extendStatics = function extendStatics(d, b) {
+ _extendStatics = Object.setPrototypeOf || {
+ __proto__: []
+ } instanceof Array && function (d, b) {
+ d.__proto__ = b;
+ } || function (d, b) {
+ for (var p in b) {
+ if (b.hasOwnProperty(p)) d[p] = b[p];
+ }
+ };
+
+ return _extendStatics(d, b);
+};
+
+function __extends(d, b) {
+ _extendStatics(d, b);
+
+ function __() {
+ this.constructor = d;
+ }
+
+ d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
+}
+
+var _assign = function __assign() {
+ _assign = Object.assign || function __assign(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+
+ for (var p in s) {
+ if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p];
+ }
+ }
+
+ return t;
+ };
+
+ return _assign.apply(this, arguments);
+};
+function __rest(s, e) {
+ var t = {};
+
+ for (var p in s) {
+ if (Object.prototype.hasOwnProperty.call(s, p) && e.indexOf(p) < 0) t[p] = s[p];
+ }
+
+ if (s != null && typeof Object.getOwnPropertySymbols === "function") for (var i = 0, p = Object.getOwnPropertySymbols(s); i < p.length; i++) {
+ if (e.indexOf(p[i]) < 0 && Object.prototype.propertyIsEnumerable.call(s, p[i])) t[p[i]] = s[p[i]];
+ }
+ return t;
+}
+function __decorate(decorators, target, key, desc) {
+ var c = arguments.length,
+ r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc,
+ d;
+ if ((typeof Reflect === "undefined" ? "undefined" : babelHelpers["typeof"](Reflect)) === "object" && typeof Reflect.decorate === "function") r = Reflect.decorate(decorators, target, key, desc);else for (var i = decorators.length - 1; i >= 0; i--) {
+ if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;
+ }
+ return c > 3 && r && Object.defineProperty(target, key, r), r;
+}
+function __param(paramIndex, decorator) {
+ return function (target, key) {
+ decorator(target, key, paramIndex);
+ };
+}
+function __metadata(metadataKey, metadataValue) {
+ if ((typeof Reflect === "undefined" ? "undefined" : babelHelpers["typeof"](Reflect)) === "object" && typeof Reflect.metadata === "function") return Reflect.metadata(metadataKey, metadataValue);
+}
+function __awaiter(thisArg, _arguments, P, generator) {
+ function adopt(value) {
+ return value instanceof P ? value : new P(function (resolve) {
+ resolve(value);
+ });
+ }
+
+ return new (P || (P = Promise))(function (resolve, reject) {
+ function fulfilled(value) {
+ try {
+ step(generator.next(value));
+ } catch (e) {
+ reject(e);
+ }
+ }
+
+ function rejected(value) {
+ try {
+ step(generator["throw"](value));
+ } catch (e) {
+ reject(e);
+ }
+ }
+
+ function step(result) {
+ result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected);
+ }
+
+ step((generator = generator.apply(thisArg, _arguments || [])).next());
+ });
+}
+function __generator(thisArg, body) {
+ var _ = {
+ label: 0,
+ sent: function sent() {
+ if (t[0] & 1) throw t[1];
+ return t[1];
+ },
+ trys: [],
+ ops: []
+ },
+ f,
+ y,
+ t,
+ g;
+ return g = {
+ next: verb(0),
+ "throw": verb(1),
+ "return": verb(2)
+ }, typeof Symbol === "function" && (g[Symbol.iterator] = function () {
+ return this;
+ }), g;
+
+ function verb(n) {
+ return function (v) {
+ return step([n, v]);
+ };
+ }
+
+ function step(op) {
+ if (f) throw new TypeError("Generator is already executing.");
+
+ while (_) {
+ try {
+ if (f = 1, y && (t = op[0] & 2 ? y["return"] : op[0] ? y["throw"] || ((t = y["return"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done) return t;
+ if (y = 0, t) op = [op[0] & 2, t.value];
+
+ switch (op[0]) {
+ case 0:
+ case 1:
+ t = op;
+ break;
+
+ case 4:
+ _.label++;
+ return {
+ value: op[1],
+ done: false
+ };
+
+ case 5:
+ _.label++;
+ y = op[1];
+ op = [0];
+ continue;
+
+ case 7:
+ op = _.ops.pop();
+
+ _.trys.pop();
+
+ continue;
+
+ default:
+ if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) {
+ _ = 0;
+ continue;
+ }
+
+ if (op[0] === 3 && (!t || op[1] > t[0] && op[1] < t[3])) {
+ _.label = op[1];
+ break;
+ }
+
+ if (op[0] === 6 && _.label < t[1]) {
+ _.label = t[1];
+ t = op;
+ break;
+ }
+
+ if (t && _.label < t[2]) {
+ _.label = t[2];
+
+ _.ops.push(op);
+
+ break;
+ }
+
+ if (t[2]) _.ops.pop();
+
+ _.trys.pop();
+
+ continue;
+ }
+
+ op = body.call(thisArg, _);
+ } catch (e) {
+ op = [6, e];
+ y = 0;
+ } finally {
+ f = t = 0;
+ }
+ }
+
+ if (op[0] & 5) throw op[1];
+ return {
+ value: op[0] ? op[1] : void 0,
+ done: true
+ };
+ }
+}
+function __createBinding(o, m, k, k2) {
+ if (k2 === undefined) k2 = k;
+ o[k2] = m[k];
+}
+function __exportStar(m, exports) {
+ for (var p in m) {
+ if (p !== "default" && !exports.hasOwnProperty(p)) exports[p] = m[p];
+ }
+}
+function __values(o) {
+ var s = typeof Symbol === "function" && Symbol.iterator,
+ m = s && o[s],
+ i = 0;
+ if (m) return m.call(o);
+ if (o && typeof o.length === "number") return {
+ next: function next() {
+ if (o && i >= o.length) o = void 0;
+ return {
+ value: o && o[i++],
+ done: !o
+ };
+ }
+ };
+ throw new TypeError(s ? "Object is not iterable." : "Symbol.iterator is not defined.");
+}
+function __read(o, n) {
+ var m = typeof Symbol === "function" && o[Symbol.iterator];
+ if (!m) return o;
+ var i = m.call(o),
+ r,
+ ar = [],
+ e;
+
+ try {
+ while ((n === void 0 || n-- > 0) && !(r = i.next()).done) {
+ ar.push(r.value);
+ }
+ } catch (error) {
+ e = {
+ error: error
+ };
+ } finally {
+ try {
+ if (r && !r.done && (m = i["return"])) m.call(i);
+ } finally {
+ if (e) throw e.error;
+ }
+ }
+
+ return ar;
+}
+function __spread() {
+ for (var ar = [], i = 0; i < arguments.length; i++) {
+ ar = ar.concat(__read(arguments[i]));
+ }
+
+ return ar;
+}
+function __spreadArrays() {
+ for (var s = 0, i = 0, il = arguments.length; i < il; i++) {
+ s += arguments[i].length;
+ }
+
+ for (var r = Array(s), k = 0, i = 0; i < il; i++) {
+ for (var a = arguments[i], j = 0, jl = a.length; j < jl; j++, k++) {
+ r[k] = a[j];
+ }
+ }
+
+ return r;
+}
+function __await(v) {
+ return this instanceof __await ? (this.v = v, this) : new __await(v);
+}
+function __asyncGenerator(thisArg, _arguments, generator) {
+ if (!Symbol.asyncIterator) throw new TypeError("Symbol.asyncIterator is not defined.");
+ var g = generator.apply(thisArg, _arguments || []),
+ i,
+ q = [];
+ return i = {}, verb("next"), verb("throw"), verb("return"), i[Symbol.asyncIterator] = function () {
+ return this;
+ }, i;
+
+ function verb(n) {
+ if (g[n]) i[n] = function (v) {
+ return new Promise(function (a, b) {
+ q.push([n, v, a, b]) > 1 || resume(n, v);
+ });
+ };
+ }
+
+ function resume(n, v) {
+ try {
+ step(g[n](v));
+ } catch (e) {
+ settle(q[0][3], e);
+ }
+ }
+
+ function step(r) {
+ r.value instanceof __await ? Promise.resolve(r.value.v).then(fulfill, reject) : settle(q[0][2], r);
+ }
+
+ function fulfill(value) {
+ resume("next", value);
+ }
+
+ function reject(value) {
+ resume("throw", value);
+ }
+
+ function settle(f, v) {
+ if (f(v), q.shift(), q.length) resume(q[0][0], q[0][1]);
+ }
+}
+function __asyncDelegator(o) {
+ var i, p;
+ return i = {}, verb("next"), verb("throw", function (e) {
+ throw e;
+ }), verb("return"), i[Symbol.iterator] = function () {
+ return this;
+ }, i;
+
+ function verb(n, f) {
+ i[n] = o[n] ? function (v) {
+ return (p = !p) ? {
+ value: __await(o[n](v)),
+ done: n === "return"
+ } : f ? f(v) : v;
+ } : f;
+ }
+}
+function __asyncValues(o) {
+ if (!Symbol.asyncIterator) throw new TypeError("Symbol.asyncIterator is not defined.");
+ var m = o[Symbol.asyncIterator],
+ i;
+ return m ? m.call(o) : (o = typeof __values === "function" ? __values(o) : o[Symbol.iterator](), i = {}, verb("next"), verb("throw"), verb("return"), i[Symbol.asyncIterator] = function () {
+ return this;
+ }, i);
+
+ function verb(n) {
+ i[n] = o[n] && function (v) {
+ return new Promise(function (resolve, reject) {
+ v = o[n](v), settle(resolve, reject, v.done, v.value);
+ });
+ };
+ }
+
+ function settle(resolve, reject, d, v) {
+ Promise.resolve(v).then(function (v) {
+ resolve({
+ value: v,
+ done: d
+ });
+ }, reject);
+ }
+}
+function __makeTemplateObject(cooked, raw) {
+ if (Object.defineProperty) {
+ Object.defineProperty(cooked, "raw", {
+ value: raw
+ });
+ } else {
+ cooked.raw = raw;
+ }
+
+ return cooked;
+}
+function __importStar(mod) {
+ if (mod && mod.__esModule) return mod;
+ var result = {};
+ if (mod != null) for (var k in mod) {
+ if (Object.hasOwnProperty.call(mod, k)) result[k] = mod[k];
+ }
+ result["default"] = mod;
+ return result;
+}
+function __importDefault(mod) {
+ return mod && mod.__esModule ? mod : {
+ "default": mod
+ };
+}
+function __classPrivateFieldGet(receiver, privateMap) {
+ if (!privateMap.has(receiver)) {
+ throw new TypeError("attempted to get private field on non-instance");
+ }
+
+ return privateMap.get(receiver);
+}
+function __classPrivateFieldSet(receiver, privateMap, value) {
+ if (!privateMap.has(receiver)) {
+ throw new TypeError("attempted to set private field on non-instance");
+ }
+
+ privateMap.set(receiver, value);
+ return value;
+}
+
+var tslib_es6 = /*#__PURE__*/Object.freeze({
+ __proto__: null,
+ __extends: __extends,
+ get __assign () { return _assign; },
+ __rest: __rest,
+ __decorate: __decorate,
+ __param: __param,
+ __metadata: __metadata,
+ __awaiter: __awaiter,
+ __generator: __generator,
+ __createBinding: __createBinding,
+ __exportStar: __exportStar,
+ __values: __values,
+ __read: __read,
+ __spread: __spread,
+ __spreadArrays: __spreadArrays,
+ __await: __await,
+ __asyncGenerator: __asyncGenerator,
+ __asyncDelegator: __asyncDelegator,
+ __asyncValues: __asyncValues,
+ __makeTemplateObject: __makeTemplateObject,
+ __importStar: __importStar,
+ __importDefault: __importDefault,
+ __classPrivateFieldGet: __classPrivateFieldGet,
+ __classPrivateFieldSet: __classPrivateFieldSet
+});
+
+var timestamp = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Timestamp = exports.LongWithoutOverridesClass = void 0;
+
+
+/** @public */
+exports.LongWithoutOverridesClass = long_1.Long;
+/** @public */
+var Timestamp = /** @class */ (function (_super) {
+ tslib_es6.__extends(Timestamp, _super);
+ function Timestamp(low, high) {
+ var _this = this;
+ // eslint-disable-next-line @typescript-eslint/ban-ts-comment
+ ///@ts-expect-error
+ if (!(_this instanceof Timestamp))
+ return new Timestamp(low, high);
+ if (long_1.Long.isLong(low)) {
+ _this = _super.call(this, low.low, low.high, true) || this;
+ }
+ else {
+ _this = _super.call(this, low, high, true) || this;
+ }
+ Object.defineProperty(_this, '_bsontype', {
+ value: 'Timestamp',
+ writable: false,
+ configurable: false,
+ enumerable: false
+ });
+ return _this;
+ }
+ Timestamp.prototype.toJSON = function () {
+ return {
+ $timestamp: this.toString()
+ };
+ };
+ /** Returns a Timestamp represented by the given (32-bit) integer value. */
+ Timestamp.fromInt = function (value) {
+ return new Timestamp(long_1.Long.fromInt(value, true));
+ };
+ /** Returns a Timestamp representing the given number value, provided that it is a finite number. Otherwise, zero is returned. */
+ Timestamp.fromNumber = function (value) {
+ return new Timestamp(long_1.Long.fromNumber(value, true));
+ };
+ /**
+ * Returns a Timestamp for the given high and low bits. Each is assumed to use 32 bits.
+ *
+ * @param lowBits - the low 32-bits.
+ * @param highBits - the high 32-bits.
+ */
+ Timestamp.fromBits = function (lowBits, highBits) {
+ return new Timestamp(lowBits, highBits);
+ };
+ /**
+ * Returns a Timestamp from the given string, optionally using the given radix.
+ *
+ * @param str - the textual representation of the Timestamp.
+ * @param optRadix - the radix in which the text is written.
+ */
+ Timestamp.fromString = function (str, optRadix) {
+ return new Timestamp(long_1.Long.fromString(str, true, optRadix));
+ };
+ /** @internal */
+ Timestamp.prototype.toExtendedJSON = function () {
+ return { $timestamp: { t: this.high >>> 0, i: this.low >>> 0 } };
+ };
+ /** @internal */
+ Timestamp.fromExtendedJSON = function (doc) {
+ return new Timestamp(doc.$timestamp.i, doc.$timestamp.t);
+ };
+ /** @internal */
+ Timestamp.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Timestamp.prototype.inspect = function () {
+ return "new Timestamp(" + this.getLowBits().toString() + ", " + this.getHighBits().toString() + ")";
+ };
+ Timestamp.MAX_VALUE = long_1.Long.MAX_UNSIGNED_VALUE;
+ return Timestamp;
+}(exports.LongWithoutOverridesClass));
+exports.Timestamp = Timestamp;
+
+});
+
+unwrapExports(timestamp);
+timestamp.Timestamp;
+timestamp.LongWithoutOverridesClass;
+
+var extended_json = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.EJSON = exports.isBSONType = void 0;
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+function isBSONType(value) {
+ return (utils.isObjectLike(value) && Reflect.has(value, '_bsontype') && typeof value._bsontype === 'string');
+}
+exports.isBSONType = isBSONType;
+// INT32 boundaries
+var BSON_INT32_MAX = 0x7fffffff;
+var BSON_INT32_MIN = -0x80000000;
+// INT64 boundaries
+var BSON_INT64_MAX = 0x7fffffffffffffff;
+var BSON_INT64_MIN = -0x8000000000000000;
+// all the types where we don't need to do any special processing and can just pass the EJSON
+//straight to type.fromExtendedJSON
+var keysToCodecs = {
+ $oid: objectid.ObjectId,
+ $binary: binary.Binary,
+ $uuid: binary.Binary,
+ $symbol: symbol.BSONSymbol,
+ $numberInt: int_32.Int32,
+ $numberDecimal: decimal128.Decimal128,
+ $numberDouble: double_1.Double,
+ $numberLong: long_1.Long,
+ $minKey: min_key.MinKey,
+ $maxKey: max_key.MaxKey,
+ $regex: regexp.BSONRegExp,
+ $regularExpression: regexp.BSONRegExp,
+ $timestamp: timestamp.Timestamp
+};
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function deserializeValue(value, options) {
+ if (options === void 0) { options = {}; }
+ if (typeof value === 'number') {
+ if (options.relaxed || options.legacy) {
+ return value;
+ }
+ // if it's an integer, should interpret as smallest BSON integer
+ // that can represent it exactly. (if out of range, interpret as double.)
+ if (Math.floor(value) === value) {
+ if (value >= BSON_INT32_MIN && value <= BSON_INT32_MAX)
+ return new int_32.Int32(value);
+ if (value >= BSON_INT64_MIN && value <= BSON_INT64_MAX)
+ return long_1.Long.fromNumber(value);
+ }
+ // If the number is a non-integer or out of integer range, should interpret as BSON Double.
+ return new double_1.Double(value);
+ }
+ // from here on out we're looking for bson types, so bail if its not an object
+ if (value == null || typeof value !== 'object')
+ return value;
+ // upgrade deprecated undefined to null
+ if (value.$undefined)
+ return null;
+ var keys = Object.keys(value).filter(function (k) { return k.startsWith('$') && value[k] != null; });
+ for (var i = 0; i < keys.length; i++) {
+ var c = keysToCodecs[keys[i]];
+ if (c)
+ return c.fromExtendedJSON(value, options);
+ }
+ if (value.$date != null) {
+ var d = value.$date;
+ var date = new Date();
+ if (options.legacy) {
+ if (typeof d === 'number')
+ date.setTime(d);
+ else if (typeof d === 'string')
+ date.setTime(Date.parse(d));
+ }
+ else {
+ if (typeof d === 'string')
+ date.setTime(Date.parse(d));
+ else if (long_1.Long.isLong(d))
+ date.setTime(d.toNumber());
+ else if (typeof d === 'number' && options.relaxed)
+ date.setTime(d);
+ }
+ return date;
+ }
+ if (value.$code != null) {
+ var copy = Object.assign({}, value);
+ if (value.$scope) {
+ copy.$scope = deserializeValue(value.$scope);
+ }
+ return code.Code.fromExtendedJSON(value);
+ }
+ if (db_ref.isDBRefLike(value) || value.$dbPointer) {
+ var v = value.$ref ? value : value.$dbPointer;
+ // we run into this in a "degenerate EJSON" case (with $id and $ref order flipped)
+ // because of the order JSON.parse goes through the document
+ if (v instanceof db_ref.DBRef)
+ return v;
+ var dollarKeys = Object.keys(v).filter(function (k) { return k.startsWith('$'); });
+ var valid_1 = true;
+ dollarKeys.forEach(function (k) {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1)
+ valid_1 = false;
+ });
+ // only make DBRef if $ keys are all valid
+ if (valid_1)
+ return db_ref.DBRef.fromExtendedJSON(v);
+ }
+ return value;
+}
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeArray(array, options) {
+ return array.map(function (v, index) {
+ options.seenObjects.push({ propertyName: "index " + index, obj: null });
+ try {
+ return serializeValue(v, options);
+ }
+ finally {
+ options.seenObjects.pop();
+ }
+ });
+}
+function getISOString(date) {
+ var isoStr = date.toISOString();
+ // we should only show milliseconds in timestamp if they're non-zero
+ return date.getUTCMilliseconds() !== 0 ? isoStr : isoStr.slice(0, -5) + 'Z';
+}
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeValue(value, options) {
+ if ((typeof value === 'object' || typeof value === 'function') && value !== null) {
+ var index = options.seenObjects.findIndex(function (entry) { return entry.obj === value; });
+ if (index !== -1) {
+ var props = options.seenObjects.map(function (entry) { return entry.propertyName; });
+ var leadingPart = props
+ .slice(0, index)
+ .map(function (prop) { return prop + " -> "; })
+ .join('');
+ var alreadySeen = props[index];
+ var circularPart = ' -> ' +
+ props
+ .slice(index + 1, props.length - 1)
+ .map(function (prop) { return prop + " -> "; })
+ .join('');
+ var current = props[props.length - 1];
+ var leadingSpace = ' '.repeat(leadingPart.length + alreadySeen.length / 2);
+ var dashes = '-'.repeat(circularPart.length + (alreadySeen.length + current.length) / 2 - 1);
+ throw new TypeError('Converting circular structure to EJSON:\n' +
+ (" " + leadingPart + alreadySeen + circularPart + current + "\n") +
+ (" " + leadingSpace + "\\" + dashes + "/"));
+ }
+ options.seenObjects[options.seenObjects.length - 1].obj = value;
+ }
+ if (Array.isArray(value))
+ return serializeArray(value, options);
+ if (value === undefined)
+ return null;
+ if (value instanceof Date || utils.isDate(value)) {
+ var dateNum = value.getTime(),
+ // is it in year range 1970-9999?
+ inRange = dateNum > -1 && dateNum < 253402318800000;
+ if (options.legacy) {
+ return options.relaxed && inRange
+ ? { $date: value.getTime() }
+ : { $date: getISOString(value) };
+ }
+ return options.relaxed && inRange
+ ? { $date: getISOString(value) }
+ : { $date: { $numberLong: value.getTime().toString() } };
+ }
+ if (typeof value === 'number' && (!options.relaxed || !isFinite(value))) {
+ // it's an integer
+ if (Math.floor(value) === value) {
+ var int32Range = value >= BSON_INT32_MIN && value <= BSON_INT32_MAX, int64Range = value >= BSON_INT64_MIN && value <= BSON_INT64_MAX;
+ // interpret as being of the smallest BSON integer type that can represent the number exactly
+ if (int32Range)
+ return { $numberInt: value.toString() };
+ if (int64Range)
+ return { $numberLong: value.toString() };
+ }
+ return { $numberDouble: value.toString() };
+ }
+ if (value instanceof RegExp || utils.isRegExp(value)) {
+ var flags = value.flags;
+ if (flags === undefined) {
+ var match = value.toString().match(/[gimuy]*$/);
+ if (match) {
+ flags = match[0];
+ }
+ }
+ var rx = new regexp.BSONRegExp(value.source, flags);
+ return rx.toExtendedJSON(options);
+ }
+ if (value != null && typeof value === 'object')
+ return serializeDocument(value, options);
+ return value;
+}
+var BSON_TYPE_MAPPINGS = {
+ Binary: function (o) { return new binary.Binary(o.value(), o.sub_type); },
+ Code: function (o) { return new code.Code(o.code, o.scope); },
+ DBRef: function (o) { return new db_ref.DBRef(o.collection || o.namespace, o.oid, o.db, o.fields); },
+ Decimal128: function (o) { return new decimal128.Decimal128(o.bytes); },
+ Double: function (o) { return new double_1.Double(o.value); },
+ Int32: function (o) { return new int_32.Int32(o.value); },
+ Long: function (o) {
+ return long_1.Long.fromBits(
+ // underscore variants for 1.x backwards compatibility
+ o.low != null ? o.low : o.low_, o.low != null ? o.high : o.high_, o.low != null ? o.unsigned : o.unsigned_);
+ },
+ MaxKey: function () { return new max_key.MaxKey(); },
+ MinKey: function () { return new min_key.MinKey(); },
+ ObjectID: function (o) { return new objectid.ObjectId(o); },
+ ObjectId: function (o) { return new objectid.ObjectId(o); },
+ BSONRegExp: function (o) { return new regexp.BSONRegExp(o.pattern, o.options); },
+ Symbol: function (o) { return new symbol.BSONSymbol(o.value); },
+ Timestamp: function (o) { return timestamp.Timestamp.fromBits(o.low, o.high); }
+};
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeDocument(doc, options) {
+ if (doc == null || typeof doc !== 'object')
+ throw new Error('not an object instance');
+ var bsontype = doc._bsontype;
+ if (typeof bsontype === 'undefined') {
+ // It's a regular object. Recursively serialize its property values.
+ var _doc = {};
+ for (var name in doc) {
+ options.seenObjects.push({ propertyName: name, obj: null });
+ try {
+ _doc[name] = serializeValue(doc[name], options);
+ }
+ finally {
+ options.seenObjects.pop();
+ }
+ }
+ return _doc;
+ }
+ else if (isBSONType(doc)) {
+ // the "document" is really just a BSON type object
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ var outDoc = doc;
+ if (typeof outDoc.toExtendedJSON !== 'function') {
+ // There's no EJSON serialization function on the object. It's probably an
+ // object created by a previous version of this library (or another library)
+ // that's duck-typing objects to look like they were generated by this library).
+ // Copy the object into this library's version of that type.
+ var mapper = BSON_TYPE_MAPPINGS[doc._bsontype];
+ if (!mapper) {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + doc._bsontype);
+ }
+ outDoc = mapper(outDoc);
+ }
+ // Two BSON types may have nested objects that may need to be serialized too
+ if (bsontype === 'Code' && outDoc.scope) {
+ outDoc = new code.Code(outDoc.code, serializeValue(outDoc.scope, options));
+ }
+ else if (bsontype === 'DBRef' && outDoc.oid) {
+ outDoc = new db_ref.DBRef(serializeValue(outDoc.collection, options), serializeValue(outDoc.oid, options), serializeValue(outDoc.db, options), serializeValue(outDoc.fields, options));
+ }
+ return outDoc.toExtendedJSON(options);
+ }
+ else {
+ throw new Error('_bsontype must be a string, but was: ' + typeof bsontype);
+ }
+}
+(function (EJSON) {
+ /**
+ * Parse an Extended JSON string, constructing the JavaScript value or object described by that
+ * string.
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const text = '{ "int32": { "$numberInt": "10" } }';
+ *
+ * // prints { int32: { [String: '10'] _bsontype: 'Int32', value: '10' } }
+ * console.log(EJSON.parse(text, { relaxed: false }));
+ *
+ * // prints { int32: 10 }
+ * console.log(EJSON.parse(text));
+ * ```
+ */
+ function parse(text, options) {
+ var finalOptions = Object.assign({}, { relaxed: true, legacy: false }, options);
+ // relaxed implies not strict
+ if (typeof finalOptions.relaxed === 'boolean')
+ finalOptions.strict = !finalOptions.relaxed;
+ if (typeof finalOptions.strict === 'boolean')
+ finalOptions.relaxed = !finalOptions.strict;
+ return JSON.parse(text, function (_key, value) { return deserializeValue(value, finalOptions); });
+ }
+ EJSON.parse = parse;
+ /**
+ * Converts a BSON document to an Extended JSON string, optionally replacing values if a replacer
+ * function is specified or optionally including only the specified properties if a replacer array
+ * is specified.
+ *
+ * @param value - The value to convert to extended JSON
+ * @param replacer - A function that alters the behavior of the stringification process, or an array of String and Number objects that serve as a whitelist for selecting/filtering the properties of the value object to be included in the JSON string. If this value is null or not provided, all properties of the object are included in the resulting JSON string
+ * @param space - A String or Number object that's used to insert white space into the output JSON string for readability purposes.
+ * @param options - Optional settings
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const Int32 = require('mongodb').Int32;
+ * const doc = { int32: new Int32(10) };
+ *
+ * // prints '{"int32":{"$numberInt":"10"}}'
+ * console.log(EJSON.stringify(doc, { relaxed: false }));
+ *
+ * // prints '{"int32":10}'
+ * console.log(EJSON.stringify(doc));
+ * ```
+ */
+ function stringify(value,
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ replacer, space, options) {
+ if (space != null && typeof space === 'object') {
+ options = space;
+ space = 0;
+ }
+ if (replacer != null && typeof replacer === 'object' && !Array.isArray(replacer)) {
+ options = replacer;
+ replacer = undefined;
+ space = 0;
+ }
+ var serializeOptions = Object.assign({ relaxed: true, legacy: false }, options, {
+ seenObjects: [{ propertyName: '(root)', obj: null }]
+ });
+ var doc = serializeValue(value, serializeOptions);
+ return JSON.stringify(doc, replacer, space);
+ }
+ EJSON.stringify = stringify;
+ /**
+ * Serializes an object to an Extended JSON string, and reparse it as a JavaScript object.
+ *
+ * @param value - The object to serialize
+ * @param options - Optional settings passed to the `stringify` function
+ */
+ function serialize(value, options) {
+ options = options || {};
+ return JSON.parse(stringify(value, options));
+ }
+ EJSON.serialize = serialize;
+ /**
+ * Deserializes an Extended JSON object into a plain JavaScript object with native/BSON types
+ *
+ * @param ejson - The Extended JSON object to deserialize
+ * @param options - Optional settings passed to the parse method
+ */
+ function deserialize(ejson, options) {
+ options = options || {};
+ return parse(JSON.stringify(ejson), options);
+ }
+ EJSON.deserialize = deserialize;
+})(exports.EJSON || (exports.EJSON = {}));
+
+});
+
+unwrapExports(extended_json);
+extended_json.EJSON;
+extended_json.isBSONType;
+
+var map = createCommonjsModule(function (module, exports) {
+/* eslint-disable @typescript-eslint/no-explicit-any */
+// We have an ES6 Map available, return the native instance
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Map = void 0;
+/** @public */
+var bsonMap;
+exports.Map = bsonMap;
+var check = function (potentialGlobal) {
+ // eslint-disable-next-line eqeqeq
+ return potentialGlobal && potentialGlobal.Math == Math && potentialGlobal;
+};
+// https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+function getGlobal() {
+ // eslint-disable-next-line no-undef
+ return (check(typeof globalThis === 'object' && globalThis) ||
+ check(typeof window === 'object' && window) ||
+ check(typeof self === 'object' && self) ||
+ check(typeof commonjsGlobal === 'object' && commonjsGlobal) ||
+ Function('return this')());
+}
+var bsonGlobal = getGlobal();
+if (Object.prototype.hasOwnProperty.call(bsonGlobal, 'Map')) {
+ exports.Map = bsonMap = bsonGlobal.Map;
+}
+else {
+ // We will return a polyfill
+ exports.Map = bsonMap = /** @class */ (function () {
+ function Map(array) {
+ if (array === void 0) { array = []; }
+ this._keys = [];
+ this._values = {};
+ for (var i = 0; i < array.length; i++) {
+ if (array[i] == null)
+ continue; // skip null and undefined
+ var entry = array[i];
+ var key = entry[0];
+ var value = entry[1];
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ }
+ }
+ Map.prototype.clear = function () {
+ this._keys = [];
+ this._values = {};
+ };
+ Map.prototype.delete = function (key) {
+ var value = this._values[key];
+ if (value == null)
+ return false;
+ // Delete entry
+ delete this._values[key];
+ // Remove the key from the ordered keys list
+ this._keys.splice(value.i, 1);
+ return true;
+ };
+ Map.prototype.entries = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? [key, _this._values[key].v] : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Map.prototype.forEach = function (callback, self) {
+ self = self || this;
+ for (var i = 0; i < this._keys.length; i++) {
+ var key = this._keys[i];
+ // Call the forEach callback
+ callback.call(self, this._values[key].v, key, self);
+ }
+ };
+ Map.prototype.get = function (key) {
+ return this._values[key] ? this._values[key].v : undefined;
+ };
+ Map.prototype.has = function (key) {
+ return this._values[key] != null;
+ };
+ Map.prototype.keys = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? key : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Map.prototype.set = function (key, value) {
+ if (this._values[key]) {
+ this._values[key].v = value;
+ return this;
+ }
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ return this;
+ };
+ Map.prototype.values = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? _this._values[key].v : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Object.defineProperty(Map.prototype, "size", {
+ get: function () {
+ return this._keys.length;
+ },
+ enumerable: false,
+ configurable: true
+ });
+ return Map;
+ }());
+}
+
+});
+
+unwrapExports(map);
+map.Map;
+
+var constants = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.BSON_BINARY_SUBTYPE_USER_DEFINED = exports.BSON_BINARY_SUBTYPE_MD5 = exports.BSON_BINARY_SUBTYPE_UUID_NEW = exports.BSON_BINARY_SUBTYPE_UUID = exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = exports.BSON_BINARY_SUBTYPE_FUNCTION = exports.BSON_BINARY_SUBTYPE_DEFAULT = exports.BSON_DATA_MAX_KEY = exports.BSON_DATA_MIN_KEY = exports.BSON_DATA_DECIMAL128 = exports.BSON_DATA_LONG = exports.BSON_DATA_TIMESTAMP = exports.BSON_DATA_INT = exports.BSON_DATA_CODE_W_SCOPE = exports.BSON_DATA_SYMBOL = exports.BSON_DATA_CODE = exports.BSON_DATA_DBPOINTER = exports.BSON_DATA_REGEXP = exports.BSON_DATA_NULL = exports.BSON_DATA_DATE = exports.BSON_DATA_BOOLEAN = exports.BSON_DATA_OID = exports.BSON_DATA_UNDEFINED = exports.BSON_DATA_BINARY = exports.BSON_DATA_ARRAY = exports.BSON_DATA_OBJECT = exports.BSON_DATA_STRING = exports.BSON_DATA_NUMBER = exports.JS_INT_MIN = exports.JS_INT_MAX = exports.BSON_INT64_MIN = exports.BSON_INT64_MAX = exports.BSON_INT32_MIN = exports.BSON_INT32_MAX = void 0;
+/** @internal */
+exports.BSON_INT32_MAX = 0x7fffffff;
+/** @internal */
+exports.BSON_INT32_MIN = -0x80000000;
+/** @internal */
+exports.BSON_INT64_MAX = Math.pow(2, 63) - 1;
+/** @internal */
+exports.BSON_INT64_MIN = -Math.pow(2, 63);
+/**
+ * Any integer up to 2^53 can be precisely represented by a double.
+ * @internal
+ */
+exports.JS_INT_MAX = Math.pow(2, 53);
+/**
+ * Any integer down to -2^53 can be precisely represented by a double.
+ * @internal
+ */
+exports.JS_INT_MIN = -Math.pow(2, 53);
+/** Number BSON Type @internal */
+exports.BSON_DATA_NUMBER = 1;
+/** String BSON Type @internal */
+exports.BSON_DATA_STRING = 2;
+/** Object BSON Type @internal */
+exports.BSON_DATA_OBJECT = 3;
+/** Array BSON Type @internal */
+exports.BSON_DATA_ARRAY = 4;
+/** Binary BSON Type @internal */
+exports.BSON_DATA_BINARY = 5;
+/** Binary BSON Type @internal */
+exports.BSON_DATA_UNDEFINED = 6;
+/** ObjectId BSON Type @internal */
+exports.BSON_DATA_OID = 7;
+/** Boolean BSON Type @internal */
+exports.BSON_DATA_BOOLEAN = 8;
+/** Date BSON Type @internal */
+exports.BSON_DATA_DATE = 9;
+/** null BSON Type @internal */
+exports.BSON_DATA_NULL = 10;
+/** RegExp BSON Type @internal */
+exports.BSON_DATA_REGEXP = 11;
+/** Code BSON Type @internal */
+exports.BSON_DATA_DBPOINTER = 12;
+/** Code BSON Type @internal */
+exports.BSON_DATA_CODE = 13;
+/** Symbol BSON Type @internal */
+exports.BSON_DATA_SYMBOL = 14;
+/** Code with Scope BSON Type @internal */
+exports.BSON_DATA_CODE_W_SCOPE = 15;
+/** 32 bit Integer BSON Type @internal */
+exports.BSON_DATA_INT = 16;
+/** Timestamp BSON Type @internal */
+exports.BSON_DATA_TIMESTAMP = 17;
+/** Long BSON Type @internal */
+exports.BSON_DATA_LONG = 18;
+/** Decimal128 BSON Type @internal */
+exports.BSON_DATA_DECIMAL128 = 19;
+/** MinKey BSON Type @internal */
+exports.BSON_DATA_MIN_KEY = 0xff;
+/** MaxKey BSON Type @internal */
+exports.BSON_DATA_MAX_KEY = 0x7f;
+/** Binary Default Type @internal */
+exports.BSON_BINARY_SUBTYPE_DEFAULT = 0;
+/** Binary Function Type @internal */
+exports.BSON_BINARY_SUBTYPE_FUNCTION = 1;
+/** Binary Byte Array Type @internal */
+exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
+/** Binary Deprecated UUID Type @deprecated Please use BSON_BINARY_SUBTYPE_UUID_NEW @internal */
+exports.BSON_BINARY_SUBTYPE_UUID = 3;
+/** Binary UUID Type @internal */
+exports.BSON_BINARY_SUBTYPE_UUID_NEW = 4;
+/** Binary MD5 Type @internal */
+exports.BSON_BINARY_SUBTYPE_MD5 = 5;
+/** Binary User Defined Type @internal */
+exports.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
+
+});
+
+unwrapExports(constants);
+constants.BSON_BINARY_SUBTYPE_USER_DEFINED;
+constants.BSON_BINARY_SUBTYPE_MD5;
+constants.BSON_BINARY_SUBTYPE_UUID_NEW;
+constants.BSON_BINARY_SUBTYPE_UUID;
+constants.BSON_BINARY_SUBTYPE_BYTE_ARRAY;
+constants.BSON_BINARY_SUBTYPE_FUNCTION;
+constants.BSON_BINARY_SUBTYPE_DEFAULT;
+constants.BSON_DATA_MAX_KEY;
+constants.BSON_DATA_MIN_KEY;
+constants.BSON_DATA_DECIMAL128;
+constants.BSON_DATA_LONG;
+constants.BSON_DATA_TIMESTAMP;
+constants.BSON_DATA_INT;
+constants.BSON_DATA_CODE_W_SCOPE;
+constants.BSON_DATA_SYMBOL;
+constants.BSON_DATA_CODE;
+constants.BSON_DATA_DBPOINTER;
+constants.BSON_DATA_REGEXP;
+constants.BSON_DATA_NULL;
+constants.BSON_DATA_DATE;
+constants.BSON_DATA_BOOLEAN;
+constants.BSON_DATA_OID;
+constants.BSON_DATA_UNDEFINED;
+constants.BSON_DATA_BINARY;
+constants.BSON_DATA_ARRAY;
+constants.BSON_DATA_OBJECT;
+constants.BSON_DATA_STRING;
+constants.BSON_DATA_NUMBER;
+constants.JS_INT_MIN;
+constants.JS_INT_MAX;
+constants.BSON_INT64_MIN;
+constants.BSON_INT64_MAX;
+constants.BSON_INT32_MIN;
+constants.BSON_INT32_MAX;
+
+var calculate_size = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.calculateObjectSize = void 0;
+
+
+
+
+function calculateObjectSize(object, serializeFunctions, ignoreUndefined) {
+ var totalLength = 4 + 1;
+ if (Array.isArray(object)) {
+ for (var i = 0; i < object.length; i++) {
+ totalLength += calculateElement(i.toString(), object[i], serializeFunctions, true, ignoreUndefined);
+ }
+ }
+ else {
+ // If we have toBSON defined, override the current object
+ if (object.toBSON) {
+ object = object.toBSON();
+ }
+ // Calculate size
+ for (var key in object) {
+ totalLength += calculateElement(key, object[key], serializeFunctions, false, ignoreUndefined);
+ }
+ }
+ return totalLength;
+}
+exports.calculateObjectSize = calculateObjectSize;
+/** @internal */
+function calculateElement(name,
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+value, serializeFunctions, isArray, ignoreUndefined) {
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (isArray === void 0) { isArray = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = false; }
+ // If we have toBSON defined, override the current object
+ if (value && value.toBSON) {
+ value = value.toBSON();
+ }
+ switch (typeof value) {
+ case 'string':
+ return 1 + buffer.Buffer.byteLength(name, 'utf8') + 1 + 4 + buffer.Buffer.byteLength(value, 'utf8') + 1;
+ case 'number':
+ if (Math.floor(value) === value &&
+ value >= constants.JS_INT_MIN &&
+ value <= constants.JS_INT_MAX) {
+ if (value >= constants.BSON_INT32_MIN && value <= constants.BSON_INT32_MAX) {
+ // 32 bit
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (4 + 1);
+ }
+ else {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ }
+ else {
+ // 64 bit
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ case 'undefined':
+ if (isArray || !ignoreUndefined)
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ return 0;
+ case 'boolean':
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 1);
+ case 'object':
+ if (value == null || value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (12 + 1);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ else if (ArrayBuffer.isView(value) ||
+ value instanceof ArrayBuffer ||
+ utils.isAnyArrayBuffer(value)) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 4 + 1) + value.byteLength);
+ }
+ else if (value['_bsontype'] === 'Long' ||
+ value['_bsontype'] === 'Double' ||
+ value['_bsontype'] === 'Timestamp') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ else if (value['_bsontype'] === 'Decimal128') {
+ return (name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (16 + 1);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ // Calculate size depending on the availability of a scope
+ if (value.scope != null && Object.keys(value.scope).length > 0) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ buffer.Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined));
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ buffer.Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1);
+ }
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ // Check what kind of subtype we have
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ (value.position + 1 + 4 + 1 + 4));
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) + (value.position + 1 + 4 + 1));
+ }
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ buffer.Buffer.byteLength(value.value, 'utf8') +
+ 4 +
+ 1 +
+ 1);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ // Set up correct object for serialization
+ var ordered_values = Object.assign({
+ $ref: value.collection,
+ $id: value.oid
+ }, value.fields);
+ // Add db reference if it exists
+ if (value.db != null) {
+ ordered_values['$db'] = value.db;
+ }
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ calculateObjectSize(ordered_values, serializeFunctions, ignoreUndefined));
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.pattern, 'utf8') +
+ 1 +
+ buffer.Buffer.byteLength(value.options, 'utf8') +
+ 1);
+ }
+ else {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ calculateObjectSize(value, serializeFunctions, ignoreUndefined) +
+ 1);
+ }
+ case 'function':
+ // WTF for 0.4.X where typeof /someregexp/ === 'function'
+ if (value instanceof RegExp || utils.isRegExp(value) || String.call(value) === '[object RegExp]') {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer.Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1);
+ }
+ else {
+ if (serializeFunctions && value.scope != null && Object.keys(value.scope).length > 0) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ buffer.Buffer.byteLength(utils.normalizedFunctionString(value), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined));
+ }
+ else if (serializeFunctions) {
+ return ((name != null ? buffer.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ buffer.Buffer.byteLength(utils.normalizedFunctionString(value), 'utf8') +
+ 1);
+ }
+ }
+ }
+ return 0;
+}
+
+});
+
+unwrapExports(calculate_size);
+calculate_size.calculateObjectSize;
+
+var validate_utf8 = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.validateUtf8 = void 0;
+var FIRST_BIT = 0x80;
+var FIRST_TWO_BITS = 0xc0;
+var FIRST_THREE_BITS = 0xe0;
+var FIRST_FOUR_BITS = 0xf0;
+var FIRST_FIVE_BITS = 0xf8;
+var TWO_BIT_CHAR = 0xc0;
+var THREE_BIT_CHAR = 0xe0;
+var FOUR_BIT_CHAR = 0xf0;
+var CONTINUING_CHAR = 0x80;
+/**
+ * Determines if the passed in bytes are valid utf8
+ * @param bytes - An array of 8-bit bytes. Must be indexable and have length property
+ * @param start - The index to start validating
+ * @param end - The index to end validating
+ */
+function validateUtf8(bytes, start, end) {
+ var continuation = 0;
+ for (var i = start; i < end; i += 1) {
+ var byte = bytes[i];
+ if (continuation) {
+ if ((byte & FIRST_TWO_BITS) !== CONTINUING_CHAR) {
+ return false;
+ }
+ continuation -= 1;
+ }
+ else if (byte & FIRST_BIT) {
+ if ((byte & FIRST_THREE_BITS) === TWO_BIT_CHAR) {
+ continuation = 1;
+ }
+ else if ((byte & FIRST_FOUR_BITS) === THREE_BIT_CHAR) {
+ continuation = 2;
+ }
+ else if ((byte & FIRST_FIVE_BITS) === FOUR_BIT_CHAR) {
+ continuation = 3;
+ }
+ else {
+ return false;
+ }
+ }
+ }
+ return !continuation;
+}
+exports.validateUtf8 = validateUtf8;
+
+});
+
+unwrapExports(validate_utf8);
+validate_utf8.validateUtf8;
+
+var deserializer = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.deserialize = void 0;
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+// Internal long versions
+var JS_INT_MAX_LONG = long_1.Long.fromNumber(constants.JS_INT_MAX);
+var JS_INT_MIN_LONG = long_1.Long.fromNumber(constants.JS_INT_MIN);
+var functionCache = {};
+function deserialize(buffer, options, isArray) {
+ options = options == null ? {} : options;
+ var index = options && options.index ? options.index : 0;
+ // Read the document size
+ var size = buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ if (size < 5) {
+ throw new Error("bson size must be >= 5, is " + size);
+ }
+ if (options.allowObjectSmallerThanBufferSize && buffer.length < size) {
+ throw new Error("buffer length " + buffer.length + " must be >= bson size " + size);
+ }
+ if (!options.allowObjectSmallerThanBufferSize && buffer.length !== size) {
+ throw new Error("buffer length " + buffer.length + " must === bson size " + size);
+ }
+ if (size + index > buffer.byteLength) {
+ throw new Error("(bson size " + size + " + options.index " + index + " must be <= buffer length " + buffer.byteLength + ")");
+ }
+ // Illegal end value
+ if (buffer[index + size - 1] !== 0) {
+ throw new Error("One object, sized correctly, with a spot for an EOO, but the EOO isn't 0x00");
+ }
+ // Start deserializtion
+ return deserializeObject(buffer, index, options, isArray);
+}
+exports.deserialize = deserialize;
+function deserializeObject(buffer$1, index, options, isArray) {
+ if (isArray === void 0) { isArray = false; }
+ var evalFunctions = options['evalFunctions'] == null ? false : options['evalFunctions'];
+ var cacheFunctions = options['cacheFunctions'] == null ? false : options['cacheFunctions'];
+ var fieldsAsRaw = options['fieldsAsRaw'] == null ? null : options['fieldsAsRaw'];
+ // Return raw bson buffer instead of parsing it
+ var raw = options['raw'] == null ? false : options['raw'];
+ // Return BSONRegExp objects instead of native regular expressions
+ var bsonRegExp = typeof options['bsonRegExp'] === 'boolean' ? options['bsonRegExp'] : false;
+ // Controls the promotion of values vs wrapper classes
+ var promoteBuffers = options['promoteBuffers'] == null ? false : options['promoteBuffers'];
+ var promoteLongs = options['promoteLongs'] == null ? true : options['promoteLongs'];
+ var promoteValues = options['promoteValues'] == null ? true : options['promoteValues'];
+ // Set the start index
+ var startIndex = index;
+ // Validate that we have at least 4 bytes of buffer
+ if (buffer$1.length < 5)
+ throw new Error('corrupt bson message < 5 bytes long');
+ // Read the document size
+ var size = buffer$1[index++] | (buffer$1[index++] << 8) | (buffer$1[index++] << 16) | (buffer$1[index++] << 24);
+ // Ensure buffer is valid size
+ if (size < 5 || size > buffer$1.length)
+ throw new Error('corrupt bson message');
+ // Create holding object
+ var object = isArray ? [] : {};
+ // Used for arrays to skip having to perform utf8 decoding
+ var arrayIndex = 0;
+ var done = false;
+ // While we have more left data left keep parsing
+ while (!done) {
+ // Read the type
+ var elementType = buffer$1[index++];
+ // If we get a zero it's the last byte, exit
+ if (elementType === 0)
+ break;
+ // Get the start search index
+ var i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.byteLength)
+ throw new Error('Bad BSON Document: illegal CString');
+ var name = isArray ? arrayIndex++ : buffer$1.toString('utf8', index, i);
+ var value = void 0;
+ index = i + 1;
+ if (elementType === constants.BSON_DATA_STRING) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ if (!validate_utf8.validateUtf8(buffer$1, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ value = buffer$1.toString('utf8', index, index + stringSize - 1);
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_OID) {
+ var oid = buffer.Buffer.alloc(12);
+ buffer$1.copy(oid, 0, index, index + 12);
+ value = new objectid.ObjectId(oid);
+ index = index + 12;
+ }
+ else if (elementType === constants.BSON_DATA_INT && promoteValues === false) {
+ value = new int_32.Int32(buffer$1[index++] | (buffer$1[index++] << 8) | (buffer$1[index++] << 16) | (buffer$1[index++] << 24));
+ }
+ else if (elementType === constants.BSON_DATA_INT) {
+ value =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ }
+ else if (elementType === constants.BSON_DATA_NUMBER && promoteValues === false) {
+ value = new double_1.Double(buffer$1.readDoubleLE(index));
+ index = index + 8;
+ }
+ else if (elementType === constants.BSON_DATA_NUMBER) {
+ value = buffer$1.readDoubleLE(index);
+ index = index + 8;
+ }
+ else if (elementType === constants.BSON_DATA_DATE) {
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ value = new Date(new long_1.Long(lowBits, highBits).toNumber());
+ }
+ else if (elementType === constants.BSON_DATA_BOOLEAN) {
+ if (buffer$1[index] !== 0 && buffer$1[index] !== 1)
+ throw new Error('illegal boolean type value');
+ value = buffer$1[index++] === 1;
+ }
+ else if (elementType === constants.BSON_DATA_OBJECT) {
+ var _index = index;
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ if (objectSize <= 0 || objectSize > buffer$1.length - index)
+ throw new Error('bad embedded document length in bson');
+ // We have a raw value
+ if (raw) {
+ value = buffer$1.slice(index, index + objectSize);
+ }
+ else {
+ value = deserializeObject(buffer$1, _index, options, false);
+ }
+ index = index + objectSize;
+ }
+ else if (elementType === constants.BSON_DATA_ARRAY) {
+ var _index = index;
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ var arrayOptions = options;
+ // Stop index
+ var stopIndex = index + objectSize;
+ // All elements of array to be returned as raw bson
+ if (fieldsAsRaw && fieldsAsRaw[name]) {
+ arrayOptions = {};
+ for (var n in options) {
+ arrayOptions[n] = options[n];
+ }
+ arrayOptions['raw'] = true;
+ }
+ value = deserializeObject(buffer$1, _index, arrayOptions, true);
+ index = index + objectSize;
+ if (buffer$1[index - 1] !== 0)
+ throw new Error('invalid array terminator byte');
+ if (index !== stopIndex)
+ throw new Error('corrupted array bson');
+ }
+ else if (elementType === constants.BSON_DATA_UNDEFINED) {
+ value = undefined;
+ }
+ else if (elementType === constants.BSON_DATA_NULL) {
+ value = null;
+ }
+ else if (elementType === constants.BSON_DATA_LONG) {
+ // Unpack the low and high bits
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var long = new long_1.Long(lowBits, highBits);
+ // Promote the long if possible
+ if (promoteLongs && promoteValues === true) {
+ value =
+ long.lessThanOrEqual(JS_INT_MAX_LONG) && long.greaterThanOrEqual(JS_INT_MIN_LONG)
+ ? long.toNumber()
+ : long;
+ }
+ else {
+ value = long;
+ }
+ }
+ else if (elementType === constants.BSON_DATA_DECIMAL128) {
+ // Buffer to contain the decimal bytes
+ var bytes = buffer.Buffer.alloc(16);
+ // Copy the next 16 bytes into the bytes buffer
+ buffer$1.copy(bytes, 0, index, index + 16);
+ // Update index
+ index = index + 16;
+ // Assign the new Decimal128 value
+ var decimal128$1 = new decimal128.Decimal128(bytes);
+ // If we have an alternative mapper use that
+ if ('toObject' in decimal128$1 && typeof decimal128$1.toObject === 'function') {
+ value = decimal128$1.toObject();
+ }
+ else {
+ value = decimal128$1;
+ }
+ }
+ else if (elementType === constants.BSON_DATA_BINARY) {
+ var binarySize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var totalBinarySize = binarySize;
+ var subType = buffer$1[index++];
+ // Did we have a negative binary size, throw
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found');
+ // Is the length longer than the document
+ if (binarySize > buffer$1.byteLength)
+ throw new Error('Binary type size larger than document size');
+ // Decode as raw Buffer object if options specifies it
+ if (buffer$1['slice'] != null) {
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+ if (promoteBuffers && promoteValues) {
+ value = buffer$1.slice(index, index + binarySize);
+ }
+ else {
+ value = new binary.Binary(buffer$1.slice(index, index + binarySize), subType);
+ }
+ }
+ else {
+ var _buffer = buffer.Buffer.alloc(binarySize);
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+ // Copy the data
+ for (i = 0; i < binarySize; i++) {
+ _buffer[i] = buffer$1[index + i];
+ }
+ if (promoteBuffers && promoteValues) {
+ value = _buffer;
+ }
+ else {
+ value = new binary.Binary(_buffer, subType);
+ }
+ }
+ // Update the index
+ index = index + binarySize;
+ }
+ else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === false) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var source = buffer$1.toString('utf8', index, i);
+ // Create the regexp
+ index = i + 1;
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var regExpOptions = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // For each option add the corresponding one for javascript
+ var optionsArray = new Array(regExpOptions.length);
+ // Parse options
+ for (i = 0; i < regExpOptions.length; i++) {
+ switch (regExpOptions[i]) {
+ case 'm':
+ optionsArray[i] = 'm';
+ break;
+ case 's':
+ optionsArray[i] = 'g';
+ break;
+ case 'i':
+ optionsArray[i] = 'i';
+ break;
+ }
+ }
+ value = new RegExp(source, optionsArray.join(''));
+ }
+ else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === true) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var source = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer$1[i] !== 0x00 && i < buffer$1.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer$1.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var regExpOptions = buffer$1.toString('utf8', index, i);
+ index = i + 1;
+ // Set the object
+ value = new regexp.BSONRegExp(source, regExpOptions);
+ }
+ else if (elementType === constants.BSON_DATA_SYMBOL) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ var symbol$1 = buffer$1.toString('utf8', index, index + stringSize - 1);
+ value = promoteValues ? symbol$1 : new symbol.BSONSymbol(symbol$1);
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_TIMESTAMP) {
+ var lowBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ var highBits = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ value = new timestamp.Timestamp(lowBits, highBits);
+ }
+ else if (elementType === constants.BSON_DATA_MIN_KEY) {
+ value = new min_key.MinKey();
+ }
+ else if (elementType === constants.BSON_DATA_MAX_KEY) {
+ value = new max_key.MaxKey();
+ }
+ else if (elementType === constants.BSON_DATA_CODE) {
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ var functionString = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ }
+ else {
+ value = isolateEval(functionString);
+ }
+ }
+ else {
+ value = new code.Code(functionString);
+ }
+ // Update parse index position
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_CODE_W_SCOPE) {
+ var totalSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Element cannot be shorter than totalSize + stringSize + documentSize + terminator
+ if (totalSize < 4 + 4 + 4 + 1) {
+ throw new Error('code_w_scope total size shorter minimum expected length');
+ }
+ // Get the code string size
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Check if we have a valid string
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ // Javascript function
+ var functionString = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Parse the element
+ var _index = index;
+ // Decode the size of the object document
+ var objectSize = buffer$1[index] |
+ (buffer$1[index + 1] << 8) |
+ (buffer$1[index + 2] << 16) |
+ (buffer$1[index + 3] << 24);
+ // Decode the scope object
+ var scopeObject = deserializeObject(buffer$1, _index, options, false);
+ // Adjust the index
+ index = index + objectSize;
+ // Check if field length is too short
+ if (totalSize < 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too short, truncating scope');
+ }
+ // Check if totalSize field is too long
+ if (totalSize > 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too long, clips outer document');
+ }
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ }
+ else {
+ value = isolateEval(functionString);
+ }
+ value.scope = scopeObject;
+ }
+ else {
+ value = new code.Code(functionString, scopeObject);
+ }
+ }
+ else if (elementType === constants.BSON_DATA_DBPOINTER) {
+ // Get the code string size
+ var stringSize = buffer$1[index++] |
+ (buffer$1[index++] << 8) |
+ (buffer$1[index++] << 16) |
+ (buffer$1[index++] << 24);
+ // Check if we have a valid string
+ if (stringSize <= 0 ||
+ stringSize > buffer$1.length - index ||
+ buffer$1[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ // Namespace
+ if (!validate_utf8.validateUtf8(buffer$1, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ var namespace = buffer$1.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Read the oid
+ var oidBuffer = buffer.Buffer.alloc(12);
+ buffer$1.copy(oidBuffer, 0, index, index + 12);
+ var oid = new objectid.ObjectId(oidBuffer);
+ // Update the index
+ index = index + 12;
+ // Upgrade to DBRef type
+ value = new db_ref.DBRef(namespace, oid);
+ }
+ else {
+ throw new Error('Detected unknown BSON type ' + elementType.toString(16) + ' for fieldname "' + name + '"');
+ }
+ if (name === '__proto__') {
+ Object.defineProperty(object, name, {
+ value: value,
+ writable: true,
+ enumerable: true,
+ configurable: true
+ });
+ }
+ else {
+ object[name] = value;
+ }
+ }
+ // Check if the deserialization was against a valid array/object
+ if (size !== index - startIndex) {
+ if (isArray)
+ throw new Error('corrupt array bson');
+ throw new Error('corrupt object bson');
+ }
+ // check if object's $ keys are those of a DBRef
+ var dollarKeys = Object.keys(object).filter(function (k) { return k.startsWith('$'); });
+ var valid = true;
+ dollarKeys.forEach(function (k) {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1)
+ valid = false;
+ });
+ // if a $key not in "$ref", "$id", "$db", don't make a DBRef
+ if (!valid)
+ return object;
+ if (db_ref.isDBRefLike(object)) {
+ var copy = Object.assign({}, object);
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new db_ref.DBRef(object.$ref, object.$id, object.$db, copy);
+ }
+ return object;
+}
+/**
+ * Ensure eval is isolated, store the result in functionCache.
+ *
+ * @internal
+ */
+function isolateEval(functionString, functionCache, object) {
+ if (!functionCache)
+ return new Function(functionString);
+ // Check for cache hit, eval if missing and return cached function
+ if (functionCache[functionString] == null) {
+ functionCache[functionString] = new Function(functionString);
+ }
+ // Set the object
+ return functionCache[functionString].bind(object);
+}
+
+});
+
+unwrapExports(deserializer);
+deserializer.deserialize;
+
+var float_parser = createCommonjsModule(function (module, exports) {
+// Copyright (c) 2008, Fair Oaks Labs, Inc.
+// All rights reserved.
+//
+// Redistribution and use in source and binary forms, with or without
+// modification, are permitted provided that the following conditions are met:
+//
+// * Redistributions of source code must retain the above copyright notice,
+// this list of conditions and the following disclaimer.
+//
+// * Redistributions in binary form must reproduce the above copyright notice,
+// this list of conditions and the following disclaimer in the documentation
+// and/or other materials provided with the distribution.
+//
+// * Neither the name of Fair Oaks Labs, Inc. nor the names of its contributors
+// may be used to endorse or promote products derived from this software
+// without specific prior written permission.
+//
+// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+// AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
+// ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
+// LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
+// CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
+// SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
+// INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
+// CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
+// ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
+// POSSIBILITY OF SUCH DAMAGE.
+//
+//
+// Modifications to writeIEEE754 to support negative zeroes made by Brian White
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.writeIEEE754 = exports.readIEEE754 = void 0;
+function readIEEE754(buffer, offset, endian, mLen, nBytes) {
+ var e;
+ var m;
+ var bBE = endian === 'big';
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var nBits = -7;
+ var i = bBE ? 0 : nBytes - 1;
+ var d = bBE ? 1 : -1;
+ var s = buffer[offset + i];
+ i += d;
+ e = s & ((1 << -nBits) - 1);
+ s >>= -nBits;
+ nBits += eLen;
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8)
+ ;
+ m = e & ((1 << -nBits) - 1);
+ e >>= -nBits;
+ nBits += mLen;
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8)
+ ;
+ if (e === 0) {
+ e = 1 - eBias;
+ }
+ else if (e === eMax) {
+ return m ? NaN : (s ? -1 : 1) * Infinity;
+ }
+ else {
+ m = m + Math.pow(2, mLen);
+ e = e - eBias;
+ }
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
+}
+exports.readIEEE754 = readIEEE754;
+function writeIEEE754(buffer, value, offset, endian, mLen, nBytes) {
+ var e;
+ var m;
+ var c;
+ var bBE = endian === 'big';
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0;
+ var i = bBE ? nBytes - 1 : 0;
+ var d = bBE ? -1 : 1;
+ var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0;
+ value = Math.abs(value);
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0;
+ e = eMax;
+ }
+ else {
+ e = Math.floor(Math.log(value) / Math.LN2);
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--;
+ c *= 2;
+ }
+ if (e + eBias >= 1) {
+ value += rt / c;
+ }
+ else {
+ value += rt * Math.pow(2, 1 - eBias);
+ }
+ if (value * c >= 2) {
+ e++;
+ c /= 2;
+ }
+ if (e + eBias >= eMax) {
+ m = 0;
+ e = eMax;
+ }
+ else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen);
+ e = e + eBias;
+ }
+ else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
+ e = 0;
+ }
+ }
+ if (isNaN(value))
+ m = 0;
+ while (mLen >= 8) {
+ buffer[offset + i] = m & 0xff;
+ i += d;
+ m /= 256;
+ mLen -= 8;
+ }
+ e = (e << mLen) | m;
+ if (isNaN(value))
+ e += 8;
+ eLen += mLen;
+ while (eLen > 0) {
+ buffer[offset + i] = e & 0xff;
+ i += d;
+ e /= 256;
+ eLen -= 8;
+ }
+ buffer[offset + i - d] |= s * 128;
+}
+exports.writeIEEE754 = writeIEEE754;
+
+});
+
+unwrapExports(float_parser);
+float_parser.writeIEEE754;
+float_parser.readIEEE754;
+
+var serializer = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.serializeInto = void 0;
+
+
+
+
+
+
+
+
+var regexp = /\x00/; // eslint-disable-line no-control-regex
+var ignoreKeys = new Set(['$db', '$ref', '$id', '$clusterTime']);
+/*
+ * isArray indicates if we are writing to a BSON array (type 0x04)
+ * which forces the "key" which really an array index as a string to be written as ascii
+ * This will catch any errors in index as a string generation
+ */
+function serializeString(buffer, key, value, index, isArray) {
+ // Encode String type
+ buffer[index++] = constants.BSON_DATA_STRING;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes + 1;
+ buffer[index - 1] = 0;
+ // Write the string
+ var size = buffer.write(value, index + 4, undefined, 'utf8');
+ // Write the size of the string to buffer
+ buffer[index + 3] = ((size + 1) >> 24) & 0xff;
+ buffer[index + 2] = ((size + 1) >> 16) & 0xff;
+ buffer[index + 1] = ((size + 1) >> 8) & 0xff;
+ buffer[index] = (size + 1) & 0xff;
+ // Update index
+ index = index + 4 + size;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+}
+function serializeNumber(buffer, key, value, index, isArray) {
+ // We have an integer value
+ // TODO(NODE-2529): Add support for big int
+ if (Number.isInteger(value) &&
+ value >= constants.BSON_INT32_MIN &&
+ value <= constants.BSON_INT32_MAX) {
+ // If the value fits in 32 bits encode as int32
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ }
+ else {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ float_parser.writeIEEE754(buffer, value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ }
+ return index;
+}
+function serializeNull(buffer, key, _, index, isArray) {
+ // Set long type
+ buffer[index++] = constants.BSON_DATA_NULL;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+}
+function serializeBoolean(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BOOLEAN;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Encode the boolean value
+ buffer[index++] = value ? 1 : 0;
+ return index;
+}
+function serializeDate(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_DATE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ var dateInMilis = long_1.Long.fromNumber(value.getTime());
+ var lowBits = dateInMilis.getLowBits();
+ var highBits = dateInMilis.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+}
+function serializeRegExp(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ if (value.source && value.source.match(regexp) != null) {
+ throw Error('value ' + value.source + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.source, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the parameters
+ if (value.ignoreCase)
+ buffer[index++] = 0x69; // i
+ if (value.global)
+ buffer[index++] = 0x73; // s
+ if (value.multiline)
+ buffer[index++] = 0x6d; // m
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+}
+function serializeBSONRegExp(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Check the pattern for 0 bytes
+ if (value.pattern.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('pattern ' + value.pattern + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.pattern, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the options
+ index = index + buffer.write(value.options.split('').sort().join(''), index, undefined, 'utf8');
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+}
+function serializeMinMax(buffer, key, value, index, isArray) {
+ // Write the type of either min or max key
+ if (value === null) {
+ buffer[index++] = constants.BSON_DATA_NULL;
+ }
+ else if (value._bsontype === 'MinKey') {
+ buffer[index++] = constants.BSON_DATA_MIN_KEY;
+ }
+ else {
+ buffer[index++] = constants.BSON_DATA_MAX_KEY;
+ }
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+}
+function serializeObjectId(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OID;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the objectId into the shared buffer
+ if (typeof value.id === 'string') {
+ buffer.write(value.id, index, undefined, 'binary');
+ }
+ else if (utils.isUint8Array(value.id)) {
+ // Use the standard JS methods here because buffer.copy() is buggy with the
+ // browser polyfill
+ buffer.set(value.id.subarray(0, 12), index);
+ }
+ else {
+ throw new TypeError('object [' + JSON.stringify(value) + '] is not a valid ObjectId');
+ }
+ // Adjust index
+ return index + 12;
+}
+function serializeBuffer(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Get size of the buffer (current write point)
+ var size = value.length;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the default subtype
+ buffer[index++] = constants.BSON_BINARY_SUBTYPE_DEFAULT;
+ // Copy the content form the binary field to the buffer
+ buffer.set(ensure_buffer.ensureBuffer(value), index);
+ // Adjust the index
+ index = index + size;
+ return index;
+}
+function serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray, path) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (isArray === void 0) { isArray = false; }
+ if (path === void 0) { path = []; }
+ for (var i = 0; i < path.length; i++) {
+ if (path[i] === value)
+ throw new Error('cyclic dependency detected');
+ }
+ // Push value to stack
+ path.push(value);
+ // Write the type
+ buffer[index++] = Array.isArray(value) ? constants.BSON_DATA_ARRAY : constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ var endIndex = serializeInto(buffer, value, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined, path);
+ // Pop stack
+ path.pop();
+ return endIndex;
+}
+function serializeDecimal128(buffer, key, value, index, isArray) {
+ buffer[index++] = constants.BSON_DATA_DECIMAL128;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the data from the value
+ // Prefer the standard JS methods because their typechecking is not buggy,
+ // unlike the `buffer` polyfill's.
+ buffer.set(value.bytes.subarray(0, 16), index);
+ return index + 16;
+}
+function serializeLong(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] =
+ value._bsontype === 'Long' ? constants.BSON_DATA_LONG : constants.BSON_DATA_TIMESTAMP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ var lowBits = value.getLowBits();
+ var highBits = value.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+}
+function serializeInt32(buffer, key, value, index, isArray) {
+ value = value.valueOf();
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ return index;
+}
+function serializeDouble(buffer, key, value, index, isArray) {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ float_parser.writeIEEE754(buffer, value.value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ return index;
+}
+function serializeFunction(buffer, key, value, index, _checkKeys, _depth, isArray) {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ var functionString = utils.normalizedFunctionString(value);
+ // Write the string
+ var size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+}
+function serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (isArray === void 0) { isArray = false; }
+ if (value.scope && typeof value.scope === 'object') {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_CODE_W_SCOPE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Starting index
+ var startIndex = index;
+ // Serialize the function
+ // Get the function string
+ var functionString = typeof value.code === 'string' ? value.code : value.code.toString();
+ // Index adjustment
+ index = index + 4;
+ // Write string into buffer
+ var codeSize = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = codeSize & 0xff;
+ buffer[index + 1] = (codeSize >> 8) & 0xff;
+ buffer[index + 2] = (codeSize >> 16) & 0xff;
+ buffer[index + 3] = (codeSize >> 24) & 0xff;
+ // Write end 0
+ buffer[index + 4 + codeSize - 1] = 0;
+ // Write the
+ index = index + codeSize + 4;
+ //
+ // Serialize the scope value
+ var endIndex = serializeInto(buffer, value.scope, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined);
+ index = endIndex - 1;
+ // Writ the total
+ var totalSize = endIndex - startIndex;
+ // Write the total size of the object
+ buffer[startIndex++] = totalSize & 0xff;
+ buffer[startIndex++] = (totalSize >> 8) & 0xff;
+ buffer[startIndex++] = (totalSize >> 16) & 0xff;
+ buffer[startIndex++] = (totalSize >> 24) & 0xff;
+ // Write trailing zero
+ buffer[index++] = 0;
+ }
+ else {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ var functionString = value.code.toString();
+ // Write the string
+ var size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ }
+ return index;
+}
+function serializeBinary(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Extract the buffer
+ var data = value.value(true);
+ // Calculate size
+ var size = value.position;
+ // Add the deprecated 02 type 4 bytes of size to total
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY)
+ size = size + 4;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the subtype to the buffer
+ buffer[index++] = value.sub_type;
+ // If we have binary type 2 the 4 first bytes are the size
+ if (value.sub_type === binary.Binary.SUBTYPE_BYTE_ARRAY) {
+ size = size - 4;
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ }
+ // Write the data to the object
+ buffer.set(data, index);
+ // Adjust the index
+ index = index + value.position;
+ return index;
+}
+function serializeSymbol(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_SYMBOL;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the string
+ var size = buffer.write(value.value, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0x00;
+ return index;
+}
+function serializeDBRef(buffer, key, value, index, depth, serializeFunctions, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ var startIndex = index;
+ var output = {
+ $ref: value.collection || value.namespace,
+ $id: value.oid
+ };
+ if (value.db != null) {
+ output.$db = value.db;
+ }
+ output = Object.assign(output, value.fields);
+ var endIndex = serializeInto(buffer, output, false, index, depth + 1, serializeFunctions);
+ // Calculate object size
+ var size = endIndex - startIndex;
+ // Write the size
+ buffer[startIndex++] = size & 0xff;
+ buffer[startIndex++] = (size >> 8) & 0xff;
+ buffer[startIndex++] = (size >> 16) & 0xff;
+ buffer[startIndex++] = (size >> 24) & 0xff;
+ // Set index
+ return endIndex;
+}
+function serializeInto(buffer, object, checkKeys, startingIndex, depth, serializeFunctions, ignoreUndefined, path) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (startingIndex === void 0) { startingIndex = 0; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (path === void 0) { path = []; }
+ startingIndex = startingIndex || 0;
+ path = path || [];
+ // Push the object to the path
+ path.push(object);
+ // Start place to serialize into
+ var index = startingIndex + 4;
+ // Special case isArray
+ if (Array.isArray(object)) {
+ // Get object keys
+ for (var i = 0; i < object.length; i++) {
+ var key = '' + i;
+ var value = object[i];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+ if (typeof value === 'string') {
+ index = serializeString(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'number') {
+ index = serializeNumber(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (typeof value === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index, true);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index, true);
+ }
+ else if (value === undefined) {
+ index = serializeNull(buffer, key, value, index, true);
+ }
+ else if (value === null) {
+ index = serializeNull(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index, true);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index, true);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true, path);
+ }
+ else if (typeof value === 'object' &&
+ extended_json.isBSONType(value) &&
+ value._bsontype === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, true);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions, true);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index, true);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ else if (object instanceof map.Map || utils.isMap(object)) {
+ var iterator = object.entries();
+ var done = false;
+ while (!done) {
+ // Unpack the next entry
+ var entry = iterator.next();
+ done = !!entry.done;
+ // Are we done, then skip and terminate
+ if (done)
+ continue;
+ // Get the entry values
+ var key = entry.value[0];
+ var value = entry.value[1];
+ // Check the type of the value
+ var type = typeof value;
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ }
+ else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ }
+ else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ }
+ else if (type === 'bigint' || utils.isBigInt64Array(value) || utils.isBigUInt64Array(value)) {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ }
+ else if (value === null || (value === undefined && ignoreUndefined === false)) {
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ }
+ else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
+ }
+ else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ else {
+ // Did we provide a custom serialization method
+ if (object.toBSON) {
+ if (typeof object.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ object = object.toBSON();
+ if (object != null && typeof object !== 'object')
+ throw new TypeError('toBSON function did not return an object');
+ }
+ // Iterate over all the keys
+ for (var key in object) {
+ var value = object[key];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+ // Check the type of the value
+ var type = typeof value;
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ }
+ else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ }
+ else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ }
+ else if (type === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ }
+ else if (value instanceof Date || utils.isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ }
+ else if (value === undefined) {
+ if (ignoreUndefined === false)
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value === null) {
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ }
+ else if (utils.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ }
+ else if (value instanceof RegExp || utils.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ }
+ else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
+ }
+ else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ // Remove the path
+ path.pop();
+ // Final padding byte for object
+ buffer[index++] = 0x00;
+ // Final size
+ var size = index - startingIndex;
+ // Write the size of the object
+ buffer[startingIndex++] = size & 0xff;
+ buffer[startingIndex++] = (size >> 8) & 0xff;
+ buffer[startingIndex++] = (size >> 16) & 0xff;
+ buffer[startingIndex++] = (size >> 24) & 0xff;
+ return index;
+}
+exports.serializeInto = serializeInto;
+
+});
+
+unwrapExports(serializer);
+serializer.serializeInto;
+
+var bson = createCommonjsModule(function (module, exports) {
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.ObjectID = exports.Decimal128 = exports.BSONRegExp = exports.MaxKey = exports.MinKey = exports.Int32 = exports.Double = exports.Timestamp = exports.Long = exports.UUID = exports.ObjectId = exports.Binary = exports.DBRef = exports.BSONSymbol = exports.Map = exports.Code = exports.LongWithoutOverridesClass = exports.EJSON = exports.BSON_INT64_MIN = exports.BSON_INT64_MAX = exports.BSON_INT32_MIN = exports.BSON_INT32_MAX = exports.BSON_DATA_UNDEFINED = exports.BSON_DATA_TIMESTAMP = exports.BSON_DATA_SYMBOL = exports.BSON_DATA_STRING = exports.BSON_DATA_REGEXP = exports.BSON_DATA_OID = exports.BSON_DATA_OBJECT = exports.BSON_DATA_NUMBER = exports.BSON_DATA_NULL = exports.BSON_DATA_MIN_KEY = exports.BSON_DATA_MAX_KEY = exports.BSON_DATA_LONG = exports.BSON_DATA_INT = exports.BSON_DATA_DECIMAL128 = exports.BSON_DATA_DBPOINTER = exports.BSON_DATA_DATE = exports.BSON_DATA_CODE_W_SCOPE = exports.BSON_DATA_CODE = exports.BSON_DATA_BOOLEAN = exports.BSON_DATA_BINARY = exports.BSON_DATA_ARRAY = exports.BSON_BINARY_SUBTYPE_UUID_NEW = exports.BSON_BINARY_SUBTYPE_UUID = exports.BSON_BINARY_SUBTYPE_USER_DEFINED = exports.BSON_BINARY_SUBTYPE_MD5 = exports.BSON_BINARY_SUBTYPE_FUNCTION = exports.BSON_BINARY_SUBTYPE_DEFAULT = exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = void 0;
+exports.deserializeStream = exports.calculateObjectSize = exports.deserialize = exports.serializeWithBufferAndIndex = exports.serialize = exports.setInternalBufferSize = void 0;
+
+
+Object.defineProperty(exports, "Binary", { enumerable: true, get: function () { return binary.Binary; } });
+
+Object.defineProperty(exports, "Code", { enumerable: true, get: function () { return code.Code; } });
+
+Object.defineProperty(exports, "DBRef", { enumerable: true, get: function () { return db_ref.DBRef; } });
+
+Object.defineProperty(exports, "Decimal128", { enumerable: true, get: function () { return decimal128.Decimal128; } });
+
+Object.defineProperty(exports, "Double", { enumerable: true, get: function () { return double_1.Double; } });
+
+
+
+Object.defineProperty(exports, "Int32", { enumerable: true, get: function () { return int_32.Int32; } });
+
+Object.defineProperty(exports, "Long", { enumerable: true, get: function () { return long_1.Long; } });
+
+Object.defineProperty(exports, "Map", { enumerable: true, get: function () { return map.Map; } });
+
+Object.defineProperty(exports, "MaxKey", { enumerable: true, get: function () { return max_key.MaxKey; } });
+
+Object.defineProperty(exports, "MinKey", { enumerable: true, get: function () { return min_key.MinKey; } });
+
+Object.defineProperty(exports, "ObjectId", { enumerable: true, get: function () { return objectid.ObjectId; } });
+Object.defineProperty(exports, "ObjectID", { enumerable: true, get: function () { return objectid.ObjectId; } });
+
+// Parts of the parser
+
+
+
+Object.defineProperty(exports, "BSONRegExp", { enumerable: true, get: function () { return regexp.BSONRegExp; } });
+
+Object.defineProperty(exports, "BSONSymbol", { enumerable: true, get: function () { return symbol.BSONSymbol; } });
+
+Object.defineProperty(exports, "Timestamp", { enumerable: true, get: function () { return timestamp.Timestamp; } });
+
+Object.defineProperty(exports, "UUID", { enumerable: true, get: function () { return uuid.UUID; } });
+
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_BYTE_ARRAY", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_BYTE_ARRAY; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_DEFAULT", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_DEFAULT; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_FUNCTION", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_FUNCTION; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_MD5", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_MD5; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_USER_DEFINED", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_USER_DEFINED; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_UUID", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_UUID; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_UUID_NEW", { enumerable: true, get: function () { return constants.BSON_BINARY_SUBTYPE_UUID_NEW; } });
+Object.defineProperty(exports, "BSON_DATA_ARRAY", { enumerable: true, get: function () { return constants.BSON_DATA_ARRAY; } });
+Object.defineProperty(exports, "BSON_DATA_BINARY", { enumerable: true, get: function () { return constants.BSON_DATA_BINARY; } });
+Object.defineProperty(exports, "BSON_DATA_BOOLEAN", { enumerable: true, get: function () { return constants.BSON_DATA_BOOLEAN; } });
+Object.defineProperty(exports, "BSON_DATA_CODE", { enumerable: true, get: function () { return constants.BSON_DATA_CODE; } });
+Object.defineProperty(exports, "BSON_DATA_CODE_W_SCOPE", { enumerable: true, get: function () { return constants.BSON_DATA_CODE_W_SCOPE; } });
+Object.defineProperty(exports, "BSON_DATA_DATE", { enumerable: true, get: function () { return constants.BSON_DATA_DATE; } });
+Object.defineProperty(exports, "BSON_DATA_DBPOINTER", { enumerable: true, get: function () { return constants.BSON_DATA_DBPOINTER; } });
+Object.defineProperty(exports, "BSON_DATA_DECIMAL128", { enumerable: true, get: function () { return constants.BSON_DATA_DECIMAL128; } });
+Object.defineProperty(exports, "BSON_DATA_INT", { enumerable: true, get: function () { return constants.BSON_DATA_INT; } });
+Object.defineProperty(exports, "BSON_DATA_LONG", { enumerable: true, get: function () { return constants.BSON_DATA_LONG; } });
+Object.defineProperty(exports, "BSON_DATA_MAX_KEY", { enumerable: true, get: function () { return constants.BSON_DATA_MAX_KEY; } });
+Object.defineProperty(exports, "BSON_DATA_MIN_KEY", { enumerable: true, get: function () { return constants.BSON_DATA_MIN_KEY; } });
+Object.defineProperty(exports, "BSON_DATA_NULL", { enumerable: true, get: function () { return constants.BSON_DATA_NULL; } });
+Object.defineProperty(exports, "BSON_DATA_NUMBER", { enumerable: true, get: function () { return constants.BSON_DATA_NUMBER; } });
+Object.defineProperty(exports, "BSON_DATA_OBJECT", { enumerable: true, get: function () { return constants.BSON_DATA_OBJECT; } });
+Object.defineProperty(exports, "BSON_DATA_OID", { enumerable: true, get: function () { return constants.BSON_DATA_OID; } });
+Object.defineProperty(exports, "BSON_DATA_REGEXP", { enumerable: true, get: function () { return constants.BSON_DATA_REGEXP; } });
+Object.defineProperty(exports, "BSON_DATA_STRING", { enumerable: true, get: function () { return constants.BSON_DATA_STRING; } });
+Object.defineProperty(exports, "BSON_DATA_SYMBOL", { enumerable: true, get: function () { return constants.BSON_DATA_SYMBOL; } });
+Object.defineProperty(exports, "BSON_DATA_TIMESTAMP", { enumerable: true, get: function () { return constants.BSON_DATA_TIMESTAMP; } });
+Object.defineProperty(exports, "BSON_DATA_UNDEFINED", { enumerable: true, get: function () { return constants.BSON_DATA_UNDEFINED; } });
+Object.defineProperty(exports, "BSON_INT32_MAX", { enumerable: true, get: function () { return constants.BSON_INT32_MAX; } });
+Object.defineProperty(exports, "BSON_INT32_MIN", { enumerable: true, get: function () { return constants.BSON_INT32_MIN; } });
+Object.defineProperty(exports, "BSON_INT64_MAX", { enumerable: true, get: function () { return constants.BSON_INT64_MAX; } });
+Object.defineProperty(exports, "BSON_INT64_MIN", { enumerable: true, get: function () { return constants.BSON_INT64_MIN; } });
+var extended_json_2 = extended_json;
+Object.defineProperty(exports, "EJSON", { enumerable: true, get: function () { return extended_json_2.EJSON; } });
+var timestamp_2 = timestamp;
+Object.defineProperty(exports, "LongWithoutOverridesClass", { enumerable: true, get: function () { return timestamp_2.LongWithoutOverridesClass; } });
+/** @internal */
+// Default Max Size
+var MAXSIZE = 1024 * 1024 * 17;
+// Current Internal Temporary Serialization Buffer
+var buffer$1 = buffer.Buffer.alloc(MAXSIZE);
+/**
+ * Sets the size of the internal serialization buffer.
+ *
+ * @param size - The desired size for the internal serialization buffer
+ * @public
+ */
+function setInternalBufferSize(size) {
+ // Resize the internal serialization buffer if needed
+ if (buffer$1.length < size) {
+ buffer$1 = buffer.Buffer.alloc(size);
+ }
+}
+exports.setInternalBufferSize = setInternalBufferSize;
+/**
+ * Serialize a Javascript object.
+ *
+ * @param object - the Javascript object to serialize.
+ * @returns Buffer object containing the serialized object.
+ * @public
+ */
+function serialize(object, options) {
+ if (options === void 0) { options = {}; }
+ // Unpack the options
+ var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ var minInternalBufferSize = typeof options.minInternalBufferSize === 'number' ? options.minInternalBufferSize : MAXSIZE;
+ // Resize the internal serialization buffer if needed
+ if (buffer$1.length < minInternalBufferSize) {
+ buffer$1 = buffer.Buffer.alloc(minInternalBufferSize);
+ }
+ // Attempt to serialize
+ var serializationIndex = serializer.serializeInto(buffer$1, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined, []);
+ // Create the final buffer
+ var finishedBuffer = buffer.Buffer.alloc(serializationIndex);
+ // Copy into the finished buffer
+ buffer$1.copy(finishedBuffer, 0, 0, finishedBuffer.length);
+ // Return the buffer
+ return finishedBuffer;
+}
+exports.serialize = serialize;
+/**
+ * Serialize a Javascript object using a predefined Buffer and index into the buffer,
+ * useful when pre-allocating the space for serialization.
+ *
+ * @param object - the Javascript object to serialize.
+ * @param finalBuffer - the Buffer you pre-allocated to store the serialized BSON object.
+ * @returns the index pointing to the last written byte in the buffer.
+ * @public
+ */
+function serializeWithBufferAndIndex(object, finalBuffer, options) {
+ if (options === void 0) { options = {}; }
+ // Unpack the options
+ var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ var startIndex = typeof options.index === 'number' ? options.index : 0;
+ // Attempt to serialize
+ var serializationIndex = serializer.serializeInto(buffer$1, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined);
+ buffer$1.copy(finalBuffer, startIndex, 0, serializationIndex);
+ // Return the index
+ return startIndex + serializationIndex - 1;
+}
+exports.serializeWithBufferAndIndex = serializeWithBufferAndIndex;
+/**
+ * Deserialize data as BSON.
+ *
+ * @param buffer - the buffer containing the serialized set of BSON documents.
+ * @returns returns the deserialized Javascript Object.
+ * @public
+ */
+function deserialize(buffer, options) {
+ if (options === void 0) { options = {}; }
+ return deserializer.deserialize(ensure_buffer.ensureBuffer(buffer), options);
+}
+exports.deserialize = deserialize;
+/**
+ * Calculate the bson size for a passed in Javascript object.
+ *
+ * @param object - the Javascript object to calculate the BSON byte size for
+ * @returns size of BSON object in bytes
+ * @public
+ */
+function calculateObjectSize(object, options) {
+ if (options === void 0) { options = {}; }
+ options = options || {};
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ return calculate_size.calculateObjectSize(object, serializeFunctions, ignoreUndefined);
+}
+exports.calculateObjectSize = calculateObjectSize;
+/**
+ * Deserialize stream data as BSON documents.
+ *
+ * @param data - the buffer containing the serialized set of BSON documents.
+ * @param startIndex - the start index in the data Buffer where the deserialization is to start.
+ * @param numberOfDocuments - number of documents to deserialize.
+ * @param documents - an array where to store the deserialized documents.
+ * @param docStartIndex - the index in the documents array from where to start inserting documents.
+ * @param options - additional options used for the deserialization.
+ * @returns next index in the buffer after deserialization **x** numbers of documents.
+ * @public
+ */
+function deserializeStream(data, startIndex, numberOfDocuments, documents, docStartIndex, options) {
+ var internalOptions = Object.assign({ allowObjectSmallerThanBufferSize: true, index: 0 }, options);
+ var bufferData = ensure_buffer.ensureBuffer(data);
+ var index = startIndex;
+ // Loop over all documents
+ for (var i = 0; i < numberOfDocuments; i++) {
+ // Find size of the document
+ var size = bufferData[index] |
+ (bufferData[index + 1] << 8) |
+ (bufferData[index + 2] << 16) |
+ (bufferData[index + 3] << 24);
+ // Update options with index
+ internalOptions.index = index;
+ // Parse the document at this point
+ documents[docStartIndex + i] = deserializer.deserialize(bufferData, internalOptions);
+ // Adjust index by the document size
+ index = index + size;
+ }
+ // Return object containing end index of parsing and list of documents
+ return index;
+}
+exports.deserializeStream = deserializeStream;
+/**
+ * BSON default export
+ * @deprecated Please use named exports
+ * @privateRemarks
+ * We want to someday deprecate the default export,
+ * so none of the new TS types are being exported on the default
+ * @public
+ */
+var BSON = {
+ Binary: binary.Binary,
+ Code: code.Code,
+ DBRef: db_ref.DBRef,
+ Decimal128: decimal128.Decimal128,
+ Double: double_1.Double,
+ Int32: int_32.Int32,
+ Long: long_1.Long,
+ UUID: uuid.UUID,
+ Map: map.Map,
+ MaxKey: max_key.MaxKey,
+ MinKey: min_key.MinKey,
+ ObjectId: objectid.ObjectId,
+ ObjectID: objectid.ObjectId,
+ BSONRegExp: regexp.BSONRegExp,
+ BSONSymbol: symbol.BSONSymbol,
+ Timestamp: timestamp.Timestamp,
+ EJSON: extended_json.EJSON,
+ setInternalBufferSize: setInternalBufferSize,
+ serialize: serialize,
+ serializeWithBufferAndIndex: serializeWithBufferAndIndex,
+ deserialize: deserialize,
+ calculateObjectSize: calculateObjectSize,
+ deserializeStream: deserializeStream
+};
+exports.default = BSON;
+
+});
+
+var bson$1 = unwrapExports(bson);
+var bson_1 = bson.ObjectID;
+var bson_2 = bson.Decimal128;
+var bson_3 = bson.BSONRegExp;
+var bson_4 = bson.MaxKey;
+var bson_5 = bson.MinKey;
+var bson_6 = bson.Int32;
+var bson_7 = bson.Double;
+var bson_8 = bson.Timestamp;
+var bson_9 = bson.Long;
+var bson_10 = bson.UUID;
+var bson_11 = bson.ObjectId;
+var bson_12 = bson.Binary;
+var bson_13 = bson.DBRef;
+var bson_14 = bson.BSONSymbol;
+var bson_15 = bson.Map;
+var bson_16 = bson.Code;
+var bson_17 = bson.LongWithoutOverridesClass;
+var bson_18 = bson.EJSON;
+var bson_19 = bson.BSON_INT64_MIN;
+var bson_20 = bson.BSON_INT64_MAX;
+var bson_21 = bson.BSON_INT32_MIN;
+var bson_22 = bson.BSON_INT32_MAX;
+var bson_23 = bson.BSON_DATA_UNDEFINED;
+var bson_24 = bson.BSON_DATA_TIMESTAMP;
+var bson_25 = bson.BSON_DATA_SYMBOL;
+var bson_26 = bson.BSON_DATA_STRING;
+var bson_27 = bson.BSON_DATA_REGEXP;
+var bson_28 = bson.BSON_DATA_OID;
+var bson_29 = bson.BSON_DATA_OBJECT;
+var bson_30 = bson.BSON_DATA_NUMBER;
+var bson_31 = bson.BSON_DATA_NULL;
+var bson_32 = bson.BSON_DATA_MIN_KEY;
+var bson_33 = bson.BSON_DATA_MAX_KEY;
+var bson_34 = bson.BSON_DATA_LONG;
+var bson_35 = bson.BSON_DATA_INT;
+var bson_36 = bson.BSON_DATA_DECIMAL128;
+var bson_37 = bson.BSON_DATA_DBPOINTER;
+var bson_38 = bson.BSON_DATA_DATE;
+var bson_39 = bson.BSON_DATA_CODE_W_SCOPE;
+var bson_40 = bson.BSON_DATA_CODE;
+var bson_41 = bson.BSON_DATA_BOOLEAN;
+var bson_42 = bson.BSON_DATA_BINARY;
+var bson_43 = bson.BSON_DATA_ARRAY;
+var bson_44 = bson.BSON_BINARY_SUBTYPE_UUID_NEW;
+var bson_45 = bson.BSON_BINARY_SUBTYPE_UUID;
+var bson_46 = bson.BSON_BINARY_SUBTYPE_USER_DEFINED;
+var bson_47 = bson.BSON_BINARY_SUBTYPE_MD5;
+var bson_48 = bson.BSON_BINARY_SUBTYPE_FUNCTION;
+var bson_49 = bson.BSON_BINARY_SUBTYPE_DEFAULT;
+var bson_50 = bson.BSON_BINARY_SUBTYPE_BYTE_ARRAY;
+var bson_51 = bson.deserializeStream;
+var bson_52 = bson.calculateObjectSize;
+var bson_53 = bson.deserialize;
+var bson_54 = bson.serializeWithBufferAndIndex;
+var bson_55 = bson.serialize;
+var bson_56 = bson.setInternalBufferSize;
+
+export default bson$1;
+export { bson_3 as BSONRegExp, bson_14 as BSONSymbol, bson_50 as BSON_BINARY_SUBTYPE_BYTE_ARRAY, bson_49 as BSON_BINARY_SUBTYPE_DEFAULT, bson_48 as BSON_BINARY_SUBTYPE_FUNCTION, bson_47 as BSON_BINARY_SUBTYPE_MD5, bson_46 as BSON_BINARY_SUBTYPE_USER_DEFINED, bson_45 as BSON_BINARY_SUBTYPE_UUID, bson_44 as BSON_BINARY_SUBTYPE_UUID_NEW, bson_43 as BSON_DATA_ARRAY, bson_42 as BSON_DATA_BINARY, bson_41 as BSON_DATA_BOOLEAN, bson_40 as BSON_DATA_CODE, bson_39 as BSON_DATA_CODE_W_SCOPE, bson_38 as BSON_DATA_DATE, bson_37 as BSON_DATA_DBPOINTER, bson_36 as BSON_DATA_DECIMAL128, bson_35 as BSON_DATA_INT, bson_34 as BSON_DATA_LONG, bson_33 as BSON_DATA_MAX_KEY, bson_32 as BSON_DATA_MIN_KEY, bson_31 as BSON_DATA_NULL, bson_30 as BSON_DATA_NUMBER, bson_29 as BSON_DATA_OBJECT, bson_28 as BSON_DATA_OID, bson_27 as BSON_DATA_REGEXP, bson_26 as BSON_DATA_STRING, bson_25 as BSON_DATA_SYMBOL, bson_24 as BSON_DATA_TIMESTAMP, bson_23 as BSON_DATA_UNDEFINED, bson_22 as BSON_INT32_MAX, bson_21 as BSON_INT32_MIN, bson_20 as BSON_INT64_MAX, bson_19 as BSON_INT64_MIN, bson_12 as Binary, bson_16 as Code, bson_13 as DBRef, bson_2 as Decimal128, bson_7 as Double, bson_18 as EJSON, bson_6 as Int32, bson_9 as Long, bson_17 as LongWithoutOverridesClass, bson_15 as Map, bson_4 as MaxKey, bson_5 as MinKey, bson_1 as ObjectID, bson_11 as ObjectId, bson_8 as Timestamp, bson_10 as UUID, bson_52 as calculateObjectSize, bson_53 as deserialize, bson_51 as deserializeStream, bson_55 as serialize, bson_54 as serializeWithBufferAndIndex, bson_56 as setInternalBufferSize };
+//# sourceMappingURL=bson.esm.js.map
diff --git a/node_modules/bson/dist/bson.esm.js.map b/node_modules/bson/dist/bson.esm.js.map
new file mode 100644
index 0000000..61a5f64
--- /dev/null
+++ b/node_modules/bson/dist/bson.esm.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"bson.esm.js","sources":["../node_modules/rollup-plugin-node-builtins/src/es6/empty.js","../node_modules/process-es6/browser.js","../node_modules/rollup-plugin-node-builtins/src/es6/inherits.js","../node_modules/rollup-plugin-node-builtins/src/es6/util.js","../src/parser/utils.ts","../src/ensure_buffer.ts","../src/uuid_utils.ts","../src/uuid.ts","../src/binary.ts","../src/code.ts","../src/db_ref.ts","../src/long.ts","../src/decimal128.ts","../src/double.ts","../src/int_32.ts","../src/max_key.ts","../src/min_key.ts","../src/objectid.ts","../src/regexp.ts","../src/symbol.ts","../node_modules/tslib/tslib.es6.js","../src/timestamp.ts","../src/extended_json.ts","../src/map.ts","../src/constants.ts","../src/parser/calculate_size.ts","../src/validate_utf8.ts","../src/parser/deserializer.ts","../src/float_parser.ts","../src/parser/serializer.ts","../src/bson.ts"],"sourcesContent":["export default {};\n","// shim for using process in browser\n// based off https://github.com/defunctzombie/node-process/blob/master/browser.js\n\nfunction defaultSetTimout() {\n throw new Error('setTimeout has not been defined');\n}\nfunction defaultClearTimeout () {\n throw new Error('clearTimeout has not been defined');\n}\nvar cachedSetTimeout = defaultSetTimout;\nvar cachedClearTimeout = defaultClearTimeout;\nif (typeof global.setTimeout === 'function') {\n cachedSetTimeout = setTimeout;\n}\nif (typeof global.clearTimeout === 'function') {\n cachedClearTimeout = clearTimeout;\n}\n\nfunction runTimeout(fun) {\n if (cachedSetTimeout === setTimeout) {\n //normal enviroments in sane situations\n return setTimeout(fun, 0);\n }\n // if setTimeout wasn't available but was latter defined\n if ((cachedSetTimeout === defaultSetTimout || !cachedSetTimeout) && setTimeout) {\n cachedSetTimeout = setTimeout;\n return setTimeout(fun, 0);\n }\n try {\n // when when somebody has screwed with setTimeout but no I.E. maddness\n return cachedSetTimeout(fun, 0);\n } catch(e){\n try {\n // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally\n return cachedSetTimeout.call(null, fun, 0);\n } catch(e){\n // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error\n return cachedSetTimeout.call(this, fun, 0);\n }\n }\n\n\n}\nfunction runClearTimeout(marker) {\n if (cachedClearTimeout === clearTimeout) {\n //normal enviroments in sane situations\n return clearTimeout(marker);\n }\n // if clearTimeout wasn't available but was latter defined\n if ((cachedClearTimeout === defaultClearTimeout || !cachedClearTimeout) && clearTimeout) {\n cachedClearTimeout = clearTimeout;\n return clearTimeout(marker);\n }\n try {\n // when when somebody has screwed with setTimeout but no I.E. maddness\n return cachedClearTimeout(marker);\n } catch (e){\n try {\n // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally\n return cachedClearTimeout.call(null, marker);\n } catch (e){\n // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error.\n // Some versions of I.E. have different rules for clearTimeout vs setTimeout\n return cachedClearTimeout.call(this, marker);\n }\n }\n\n\n\n}\nvar queue = [];\nvar draining = false;\nvar currentQueue;\nvar queueIndex = -1;\n\nfunction cleanUpNextTick() {\n if (!draining || !currentQueue) {\n return;\n }\n draining = false;\n if (currentQueue.length) {\n queue = currentQueue.concat(queue);\n } else {\n queueIndex = -1;\n }\n if (queue.length) {\n drainQueue();\n }\n}\n\nfunction drainQueue() {\n if (draining) {\n return;\n }\n var timeout = runTimeout(cleanUpNextTick);\n draining = true;\n\n var len = queue.length;\n while(len) {\n currentQueue = queue;\n queue = [];\n while (++queueIndex < len) {\n if (currentQueue) {\n currentQueue[queueIndex].run();\n }\n }\n queueIndex = -1;\n len = queue.length;\n }\n currentQueue = null;\n draining = false;\n runClearTimeout(timeout);\n}\nexport function nextTick(fun) {\n var args = new Array(arguments.length - 1);\n if (arguments.length > 1) {\n for (var i = 1; i < arguments.length; i++) {\n args[i - 1] = arguments[i];\n }\n }\n queue.push(new Item(fun, args));\n if (queue.length === 1 && !draining) {\n runTimeout(drainQueue);\n }\n}\n// v8 likes predictible objects\nfunction Item(fun, array) {\n this.fun = fun;\n this.array = array;\n}\nItem.prototype.run = function () {\n this.fun.apply(null, this.array);\n};\nexport var title = 'browser';\nexport var platform = 'browser';\nexport var browser = true;\nexport var env = {};\nexport var argv = [];\nexport var version = ''; // empty string to avoid regexp issues\nexport var versions = {};\nexport var release = {};\nexport var config = {};\n\nfunction noop() {}\n\nexport var on = noop;\nexport var addListener = noop;\nexport var once = noop;\nexport var off = noop;\nexport var removeListener = noop;\nexport var removeAllListeners = noop;\nexport var emit = noop;\n\nexport function binding(name) {\n throw new Error('process.binding is not supported');\n}\n\nexport function cwd () { return '/' }\nexport function chdir (dir) {\n throw new Error('process.chdir is not supported');\n};\nexport function umask() { return 0; }\n\n// from https://github.com/kumavis/browser-process-hrtime/blob/master/index.js\nvar performance = global.performance || {}\nvar performanceNow =\n performance.now ||\n performance.mozNow ||\n performance.msNow ||\n performance.oNow ||\n performance.webkitNow ||\n function(){ return (new Date()).getTime() }\n\n// generate timestamp or delta\n// see http://nodejs.org/api/process.html#process_process_hrtime\nexport function hrtime(previousTimestamp){\n var clocktime = performanceNow.call(performance)*1e-3\n var seconds = Math.floor(clocktime)\n var nanoseconds = Math.floor((clocktime%1)*1e9)\n if (previousTimestamp) {\n seconds = seconds - previousTimestamp[0]\n nanoseconds = nanoseconds - previousTimestamp[1]\n if (nanoseconds<0) {\n seconds--\n nanoseconds += 1e9\n }\n }\n return [seconds,nanoseconds]\n}\n\nvar startTime = new Date();\nexport function uptime() {\n var currentTime = new Date();\n var dif = currentTime - startTime;\n return dif / 1000;\n}\n\nexport default {\n nextTick: nextTick,\n title: title,\n browser: browser,\n env: env,\n argv: argv,\n version: version,\n versions: versions,\n on: on,\n addListener: addListener,\n once: once,\n off: off,\n removeListener: removeListener,\n removeAllListeners: removeAllListeners,\n emit: emit,\n binding: binding,\n cwd: cwd,\n chdir: chdir,\n umask: umask,\n hrtime: hrtime,\n platform: platform,\n release: release,\n config: config,\n uptime: uptime\n};\n","\nvar inherits;\nif (typeof Object.create === 'function'){\n inherits = function inherits(ctor, superCtor) {\n // implementation from standard node.js 'util' module\n ctor.super_ = superCtor\n ctor.prototype = Object.create(superCtor.prototype, {\n constructor: {\n value: ctor,\n enumerable: false,\n writable: true,\n configurable: true\n }\n });\n };\n} else {\n inherits = function inherits(ctor, superCtor) {\n ctor.super_ = superCtor\n var TempCtor = function () {}\n TempCtor.prototype = superCtor.prototype\n ctor.prototype = new TempCtor()\n ctor.prototype.constructor = ctor\n }\n}\nexport default inherits;\n","// Copyright Joyent, Inc. and other Node contributors.\n//\n// Permission is hereby granted, free of charge, to any person obtaining a\n// copy of this software and associated documentation files (the\n// \"Software\"), to deal in the Software without restriction, including\n// without limitation the rights to use, copy, modify, merge, publish,\n// distribute, sublicense, and/or sell copies of the Software, and to permit\n// persons to whom the Software is furnished to do so, subject to the\n// following conditions:\n//\n// The above copyright notice and this permission notice shall be included\n// in all copies or substantial portions of the Software.\n//\n// THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS\n// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF\n// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN\n// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,\n// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR\n// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE\n// USE OR OTHER DEALINGS IN THE SOFTWARE.\nimport process from 'process';\nvar formatRegExp = /%[sdj%]/g;\nexport function format(f) {\n if (!isString(f)) {\n var objects = [];\n for (var i = 0; i < arguments.length; i++) {\n objects.push(inspect(arguments[i]));\n }\n return objects.join(' ');\n }\n\n var i = 1;\n var args = arguments;\n var len = args.length;\n var str = String(f).replace(formatRegExp, function(x) {\n if (x === '%%') return '%';\n if (i >= len) return x;\n switch (x) {\n case '%s': return String(args[i++]);\n case '%d': return Number(args[i++]);\n case '%j':\n try {\n return JSON.stringify(args[i++]);\n } catch (_) {\n return '[Circular]';\n }\n default:\n return x;\n }\n });\n for (var x = args[i]; i < len; x = args[++i]) {\n if (isNull(x) || !isObject(x)) {\n str += ' ' + x;\n } else {\n str += ' ' + inspect(x);\n }\n }\n return str;\n};\n\n\n// Mark that a method should not be used.\n// Returns a modified function which warns once by default.\n// If --no-deprecation is set, then it is a no-op.\nexport function deprecate(fn, msg) {\n // Allow for deprecating things in the process of starting up.\n if (isUndefined(global.process)) {\n return function() {\n return deprecate(fn, msg).apply(this, arguments);\n };\n }\n\n if (process.noDeprecation === true) {\n return fn;\n }\n\n var warned = false;\n function deprecated() {\n if (!warned) {\n if (process.throwDeprecation) {\n throw new Error(msg);\n } else if (process.traceDeprecation) {\n console.trace(msg);\n } else {\n console.error(msg);\n }\n warned = true;\n }\n return fn.apply(this, arguments);\n }\n\n return deprecated;\n};\n\n\nvar debugs = {};\nvar debugEnviron;\nexport function debuglog(set) {\n if (isUndefined(debugEnviron))\n debugEnviron = process.env.NODE_DEBUG || '';\n set = set.toUpperCase();\n if (!debugs[set]) {\n if (new RegExp('\\\\b' + set + '\\\\b', 'i').test(debugEnviron)) {\n var pid = 0;\n debugs[set] = function() {\n var msg = format.apply(null, arguments);\n console.error('%s %d: %s', set, pid, msg);\n };\n } else {\n debugs[set] = function() {};\n }\n }\n return debugs[set];\n};\n\n\n/**\n * Echos the value of a value. Trys to print the value out\n * in the best way possible given the different types.\n *\n * @param {Object} obj The object to print out.\n * @param {Object} opts Optional options object that alters the output.\n */\n/* legacy: obj, showHidden, depth, colors*/\nexport function inspect(obj, opts) {\n // default options\n var ctx = {\n seen: [],\n stylize: stylizeNoColor\n };\n // legacy...\n if (arguments.length >= 3) ctx.depth = arguments[2];\n if (arguments.length >= 4) ctx.colors = arguments[3];\n if (isBoolean(opts)) {\n // legacy...\n ctx.showHidden = opts;\n } else if (opts) {\n // got an \"options\" object\n _extend(ctx, opts);\n }\n // set default options\n if (isUndefined(ctx.showHidden)) ctx.showHidden = false;\n if (isUndefined(ctx.depth)) ctx.depth = 2;\n if (isUndefined(ctx.colors)) ctx.colors = false;\n if (isUndefined(ctx.customInspect)) ctx.customInspect = true;\n if (ctx.colors) ctx.stylize = stylizeWithColor;\n return formatValue(ctx, obj, ctx.depth);\n}\n\n// http://en.wikipedia.org/wiki/ANSI_escape_code#graphics\ninspect.colors = {\n 'bold' : [1, 22],\n 'italic' : [3, 23],\n 'underline' : [4, 24],\n 'inverse' : [7, 27],\n 'white' : [37, 39],\n 'grey' : [90, 39],\n 'black' : [30, 39],\n 'blue' : [34, 39],\n 'cyan' : [36, 39],\n 'green' : [32, 39],\n 'magenta' : [35, 39],\n 'red' : [31, 39],\n 'yellow' : [33, 39]\n};\n\n// Don't use 'blue' not visible on cmd.exe\ninspect.styles = {\n 'special': 'cyan',\n 'number': 'yellow',\n 'boolean': 'yellow',\n 'undefined': 'grey',\n 'null': 'bold',\n 'string': 'green',\n 'date': 'magenta',\n // \"name\": intentionally not styling\n 'regexp': 'red'\n};\n\n\nfunction stylizeWithColor(str, styleType) {\n var style = inspect.styles[styleType];\n\n if (style) {\n return '\\u001b[' + inspect.colors[style][0] + 'm' + str +\n '\\u001b[' + inspect.colors[style][1] + 'm';\n } else {\n return str;\n }\n}\n\n\nfunction stylizeNoColor(str, styleType) {\n return str;\n}\n\n\nfunction arrayToHash(array) {\n var hash = {};\n\n array.forEach(function(val, idx) {\n hash[val] = true;\n });\n\n return hash;\n}\n\n\nfunction formatValue(ctx, value, recurseTimes) {\n // Provide a hook for user-specified inspect functions.\n // Check that value is an object with an inspect function on it\n if (ctx.customInspect &&\n value &&\n isFunction(value.inspect) &&\n // Filter out the util module, it's inspect function is special\n value.inspect !== inspect &&\n // Also filter out any prototype objects using the circular check.\n !(value.constructor && value.constructor.prototype === value)) {\n var ret = value.inspect(recurseTimes, ctx);\n if (!isString(ret)) {\n ret = formatValue(ctx, ret, recurseTimes);\n }\n return ret;\n }\n\n // Primitive types cannot have properties\n var primitive = formatPrimitive(ctx, value);\n if (primitive) {\n return primitive;\n }\n\n // Look up the keys of the object.\n var keys = Object.keys(value);\n var visibleKeys = arrayToHash(keys);\n\n if (ctx.showHidden) {\n keys = Object.getOwnPropertyNames(value);\n }\n\n // IE doesn't make error fields non-enumerable\n // http://msdn.microsoft.com/en-us/library/ie/dww52sbt(v=vs.94).aspx\n if (isError(value)\n && (keys.indexOf('message') >= 0 || keys.indexOf('description') >= 0)) {\n return formatError(value);\n }\n\n // Some type of object without properties can be shortcutted.\n if (keys.length === 0) {\n if (isFunction(value)) {\n var name = value.name ? ': ' + value.name : '';\n return ctx.stylize('[Function' + name + ']', 'special');\n }\n if (isRegExp(value)) {\n return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');\n }\n if (isDate(value)) {\n return ctx.stylize(Date.prototype.toString.call(value), 'date');\n }\n if (isError(value)) {\n return formatError(value);\n }\n }\n\n var base = '', array = false, braces = ['{', '}'];\n\n // Make Array say that they are Array\n if (isArray(value)) {\n array = true;\n braces = ['[', ']'];\n }\n\n // Make functions say that they are functions\n if (isFunction(value)) {\n var n = value.name ? ': ' + value.name : '';\n base = ' [Function' + n + ']';\n }\n\n // Make RegExps say that they are RegExps\n if (isRegExp(value)) {\n base = ' ' + RegExp.prototype.toString.call(value);\n }\n\n // Make dates with properties first say the date\n if (isDate(value)) {\n base = ' ' + Date.prototype.toUTCString.call(value);\n }\n\n // Make error with message first say the error\n if (isError(value)) {\n base = ' ' + formatError(value);\n }\n\n if (keys.length === 0 && (!array || value.length == 0)) {\n return braces[0] + base + braces[1];\n }\n\n if (recurseTimes < 0) {\n if (isRegExp(value)) {\n return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');\n } else {\n return ctx.stylize('[Object]', 'special');\n }\n }\n\n ctx.seen.push(value);\n\n var output;\n if (array) {\n output = formatArray(ctx, value, recurseTimes, visibleKeys, keys);\n } else {\n output = keys.map(function(key) {\n return formatProperty(ctx, value, recurseTimes, visibleKeys, key, array);\n });\n }\n\n ctx.seen.pop();\n\n return reduceToSingleString(output, base, braces);\n}\n\n\nfunction formatPrimitive(ctx, value) {\n if (isUndefined(value))\n return ctx.stylize('undefined', 'undefined');\n if (isString(value)) {\n var simple = '\\'' + JSON.stringify(value).replace(/^\"|\"$/g, '')\n .replace(/'/g, \"\\\\'\")\n .replace(/\\\\\"/g, '\"') + '\\'';\n return ctx.stylize(simple, 'string');\n }\n if (isNumber(value))\n return ctx.stylize('' + value, 'number');\n if (isBoolean(value))\n return ctx.stylize('' + value, 'boolean');\n // For some reason typeof null is \"object\", so special case here.\n if (isNull(value))\n return ctx.stylize('null', 'null');\n}\n\n\nfunction formatError(value) {\n return '[' + Error.prototype.toString.call(value) + ']';\n}\n\n\nfunction formatArray(ctx, value, recurseTimes, visibleKeys, keys) {\n var output = [];\n for (var i = 0, l = value.length; i < l; ++i) {\n if (hasOwnProperty(value, String(i))) {\n output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,\n String(i), true));\n } else {\n output.push('');\n }\n }\n keys.forEach(function(key) {\n if (!key.match(/^\\d+$/)) {\n output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,\n key, true));\n }\n });\n return output;\n}\n\n\nfunction formatProperty(ctx, value, recurseTimes, visibleKeys, key, array) {\n var name, str, desc;\n desc = Object.getOwnPropertyDescriptor(value, key) || { value: value[key] };\n if (desc.get) {\n if (desc.set) {\n str = ctx.stylize('[Getter/Setter]', 'special');\n } else {\n str = ctx.stylize('[Getter]', 'special');\n }\n } else {\n if (desc.set) {\n str = ctx.stylize('[Setter]', 'special');\n }\n }\n if (!hasOwnProperty(visibleKeys, key)) {\n name = '[' + key + ']';\n }\n if (!str) {\n if (ctx.seen.indexOf(desc.value) < 0) {\n if (isNull(recurseTimes)) {\n str = formatValue(ctx, desc.value, null);\n } else {\n str = formatValue(ctx, desc.value, recurseTimes - 1);\n }\n if (str.indexOf('\\n') > -1) {\n if (array) {\n str = str.split('\\n').map(function(line) {\n return ' ' + line;\n }).join('\\n').substr(2);\n } else {\n str = '\\n' + str.split('\\n').map(function(line) {\n return ' ' + line;\n }).join('\\n');\n }\n }\n } else {\n str = ctx.stylize('[Circular]', 'special');\n }\n }\n if (isUndefined(name)) {\n if (array && key.match(/^\\d+$/)) {\n return str;\n }\n name = JSON.stringify('' + key);\n if (name.match(/^\"([a-zA-Z_][a-zA-Z_0-9]*)\"$/)) {\n name = name.substr(1, name.length - 2);\n name = ctx.stylize(name, 'name');\n } else {\n name = name.replace(/'/g, \"\\\\'\")\n .replace(/\\\\\"/g, '\"')\n .replace(/(^\"|\"$)/g, \"'\");\n name = ctx.stylize(name, 'string');\n }\n }\n\n return name + ': ' + str;\n}\n\n\nfunction reduceToSingleString(output, base, braces) {\n var numLinesEst = 0;\n var length = output.reduce(function(prev, cur) {\n numLinesEst++;\n if (cur.indexOf('\\n') >= 0) numLinesEst++;\n return prev + cur.replace(/\\u001b\\[\\d\\d?m/g, '').length + 1;\n }, 0);\n\n if (length > 60) {\n return braces[0] +\n (base === '' ? '' : base + '\\n ') +\n ' ' +\n output.join(',\\n ') +\n ' ' +\n braces[1];\n }\n\n return braces[0] + base + ' ' + output.join(', ') + ' ' + braces[1];\n}\n\n\n// NOTE: These type checking functions intentionally don't use `instanceof`\n// because it is fragile and can be easily faked with `Object.create()`.\nexport function isArray(ar) {\n return Array.isArray(ar);\n}\n\nexport function isBoolean(arg) {\n return typeof arg === 'boolean';\n}\n\nexport function isNull(arg) {\n return arg === null;\n}\n\nexport function isNullOrUndefined(arg) {\n return arg == null;\n}\n\nexport function isNumber(arg) {\n return typeof arg === 'number';\n}\n\nexport function isString(arg) {\n return typeof arg === 'string';\n}\n\nexport function isSymbol(arg) {\n return typeof arg === 'symbol';\n}\n\nexport function isUndefined(arg) {\n return arg === void 0;\n}\n\nexport function isRegExp(re) {\n return isObject(re) && objectToString(re) === '[object RegExp]';\n}\n\nexport function isObject(arg) {\n return typeof arg === 'object' && arg !== null;\n}\n\nexport function isDate(d) {\n return isObject(d) && objectToString(d) === '[object Date]';\n}\n\nexport function isError(e) {\n return isObject(e) &&\n (objectToString(e) === '[object Error]' || e instanceof Error);\n}\n\nexport function isFunction(arg) {\n return typeof arg === 'function';\n}\n\nexport function isPrimitive(arg) {\n return arg === null ||\n typeof arg === 'boolean' ||\n typeof arg === 'number' ||\n typeof arg === 'string' ||\n typeof arg === 'symbol' || // ES6 symbol\n typeof arg === 'undefined';\n}\n\nexport function isBuffer(maybeBuf) {\n return Buffer.isBuffer(maybeBuf);\n}\n\nfunction objectToString(o) {\n return Object.prototype.toString.call(o);\n}\n\n\nfunction pad(n) {\n return n < 10 ? '0' + n.toString(10) : n.toString(10);\n}\n\n\nvar months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep',\n 'Oct', 'Nov', 'Dec'];\n\n// 26 Feb 16:19:34\nfunction timestamp() {\n var d = new Date();\n var time = [pad(d.getHours()),\n pad(d.getMinutes()),\n pad(d.getSeconds())].join(':');\n return [d.getDate(), months[d.getMonth()], time].join(' ');\n}\n\n\n// log is just a thin wrapper to console.log that prepends a timestamp\nexport function log() {\n console.log('%s - %s', timestamp(), format.apply(null, arguments));\n}\n\n\n/**\n * Inherit the prototype methods from one constructor into another.\n *\n * The Function.prototype.inherits from lang.js rewritten as a standalone\n * function (not on Function.prototype). NOTE: If this file is to be loaded\n * during bootstrapping this function needs to be rewritten using some native\n * functions as prototype setup using normal JavaScript does not work as\n * expected during bootstrapping (see mirror.js in r114903).\n *\n * @param {function} ctor Constructor function which needs to inherit the\n * prototype.\n * @param {function} superCtor Constructor function to inherit prototype from.\n */\nimport inherits from './inherits';\nexport {inherits}\n\nexport function _extend(origin, add) {\n // Don't do anything if add isn't an object\n if (!add || !isObject(add)) return origin;\n\n var keys = Object.keys(add);\n var i = keys.length;\n while (i--) {\n origin[keys[i]] = add[keys[i]];\n }\n return origin;\n};\n\nfunction hasOwnProperty(obj, prop) {\n return Object.prototype.hasOwnProperty.call(obj, prop);\n}\n\nexport default {\n inherits: inherits,\n _extend: _extend,\n log: log,\n isBuffer: isBuffer,\n isPrimitive: isPrimitive,\n isFunction: isFunction,\n isError: isError,\n isDate: isDate,\n isObject: isObject,\n isRegExp: isRegExp,\n isUndefined: isUndefined,\n isSymbol: isSymbol,\n isString: isString,\n isNumber: isNumber,\n isNullOrUndefined: isNullOrUndefined,\n isNull: isNull,\n isBoolean: isBoolean,\n isArray: isArray,\n inspect: inspect,\n deprecate: deprecate,\n format: format,\n debuglog: debuglog\n}\n",null,null,null,null,null,null,null,null,null,null,null,null,null,null,null,null,"/*! *****************************************************************************\r\nCopyright (c) Microsoft Corporation.\r\n\r\nPermission to use, copy, modify, and/or distribute this software for any\r\npurpose with or without fee is hereby granted.\r\n\r\nTHE SOFTWARE IS PROVIDED \"AS IS\" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH\r\nREGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY\r\nAND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,\r\nINDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM\r\nLOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR\r\nOTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR\r\nPERFORMANCE OF THIS SOFTWARE.\r\n***************************************************************************** */\r\n/* global Reflect, Promise */\r\n\r\nvar extendStatics = function(d, b) {\r\n extendStatics = Object.setPrototypeOf ||\r\n ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||\r\n function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; };\r\n return extendStatics(d, b);\r\n};\r\n\r\nexport function __extends(d, b) {\r\n extendStatics(d, b);\r\n function __() { this.constructor = d; }\r\n d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());\r\n}\r\n\r\nexport var __assign = function() {\r\n __assign = Object.assign || function __assign(t) {\r\n for (var s, i = 1, n = arguments.length; i < n; i++) {\r\n s = arguments[i];\r\n for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p];\r\n }\r\n return t;\r\n }\r\n return __assign.apply(this, arguments);\r\n}\r\n\r\nexport function __rest(s, e) {\r\n var t = {};\r\n for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p) && e.indexOf(p) < 0)\r\n t[p] = s[p];\r\n if (s != null && typeof Object.getOwnPropertySymbols === \"function\")\r\n for (var i = 0, p = Object.getOwnPropertySymbols(s); i < p.length; i++) {\r\n if (e.indexOf(p[i]) < 0 && Object.prototype.propertyIsEnumerable.call(s, p[i]))\r\n t[p[i]] = s[p[i]];\r\n }\r\n return t;\r\n}\r\n\r\nexport function __decorate(decorators, target, key, desc) {\r\n var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;\r\n if (typeof Reflect === \"object\" && typeof Reflect.decorate === \"function\") r = Reflect.decorate(decorators, target, key, desc);\r\n else for (var i = decorators.length - 1; i >= 0; i--) if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;\r\n return c > 3 && r && Object.defineProperty(target, key, r), r;\r\n}\r\n\r\nexport function __param(paramIndex, decorator) {\r\n return function (target, key) { decorator(target, key, paramIndex); }\r\n}\r\n\r\nexport function __metadata(metadataKey, metadataValue) {\r\n if (typeof Reflect === \"object\" && typeof Reflect.metadata === \"function\") return Reflect.metadata(metadataKey, metadataValue);\r\n}\r\n\r\nexport function __awaiter(thisArg, _arguments, P, generator) {\r\n function adopt(value) { return value instanceof P ? value : new P(function (resolve) { resolve(value); }); }\r\n return new (P || (P = Promise))(function (resolve, reject) {\r\n function fulfilled(value) { try { step(generator.next(value)); } catch (e) { reject(e); } }\r\n function rejected(value) { try { step(generator[\"throw\"](value)); } catch (e) { reject(e); } }\r\n function step(result) { result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected); }\r\n step((generator = generator.apply(thisArg, _arguments || [])).next());\r\n });\r\n}\r\n\r\nexport function __generator(thisArg, body) {\r\n var _ = { label: 0, sent: function() { if (t[0] & 1) throw t[1]; return t[1]; }, trys: [], ops: [] }, f, y, t, g;\r\n return g = { next: verb(0), \"throw\": verb(1), \"return\": verb(2) }, typeof Symbol === \"function\" && (g[Symbol.iterator] = function() { return this; }), g;\r\n function verb(n) { return function (v) { return step([n, v]); }; }\r\n function step(op) {\r\n if (f) throw new TypeError(\"Generator is already executing.\");\r\n while (_) try {\r\n if (f = 1, y && (t = op[0] & 2 ? y[\"return\"] : op[0] ? y[\"throw\"] || ((t = y[\"return\"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done) return t;\r\n if (y = 0, t) op = [op[0] & 2, t.value];\r\n switch (op[0]) {\r\n case 0: case 1: t = op; break;\r\n case 4: _.label++; return { value: op[1], done: false };\r\n case 5: _.label++; y = op[1]; op = [0]; continue;\r\n case 7: op = _.ops.pop(); _.trys.pop(); continue;\r\n default:\r\n if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) { _ = 0; continue; }\r\n if (op[0] === 3 && (!t || (op[1] > t[0] && op[1] < t[3]))) { _.label = op[1]; break; }\r\n if (op[0] === 6 && _.label < t[1]) { _.label = t[1]; t = op; break; }\r\n if (t && _.label < t[2]) { _.label = t[2]; _.ops.push(op); break; }\r\n if (t[2]) _.ops.pop();\r\n _.trys.pop(); continue;\r\n }\r\n op = body.call(thisArg, _);\r\n } catch (e) { op = [6, e]; y = 0; } finally { f = t = 0; }\r\n if (op[0] & 5) throw op[1]; return { value: op[0] ? op[1] : void 0, done: true };\r\n }\r\n}\r\n\r\nexport function __createBinding(o, m, k, k2) {\r\n if (k2 === undefined) k2 = k;\r\n o[k2] = m[k];\r\n}\r\n\r\nexport function __exportStar(m, exports) {\r\n for (var p in m) if (p !== \"default\" && !exports.hasOwnProperty(p)) exports[p] = m[p];\r\n}\r\n\r\nexport function __values(o) {\r\n var s = typeof Symbol === \"function\" && Symbol.iterator, m = s && o[s], i = 0;\r\n if (m) return m.call(o);\r\n if (o && typeof o.length === \"number\") return {\r\n next: function () {\r\n if (o && i >= o.length) o = void 0;\r\n return { value: o && o[i++], done: !o };\r\n }\r\n };\r\n throw new TypeError(s ? \"Object is not iterable.\" : \"Symbol.iterator is not defined.\");\r\n}\r\n\r\nexport function __read(o, n) {\r\n var m = typeof Symbol === \"function\" && o[Symbol.iterator];\r\n if (!m) return o;\r\n var i = m.call(o), r, ar = [], e;\r\n try {\r\n while ((n === void 0 || n-- > 0) && !(r = i.next()).done) ar.push(r.value);\r\n }\r\n catch (error) { e = { error: error }; }\r\n finally {\r\n try {\r\n if (r && !r.done && (m = i[\"return\"])) m.call(i);\r\n }\r\n finally { if (e) throw e.error; }\r\n }\r\n return ar;\r\n}\r\n\r\nexport function __spread() {\r\n for (var ar = [], i = 0; i < arguments.length; i++)\r\n ar = ar.concat(__read(arguments[i]));\r\n return ar;\r\n}\r\n\r\nexport function __spreadArrays() {\r\n for (var s = 0, i = 0, il = arguments.length; i < il; i++) s += arguments[i].length;\r\n for (var r = Array(s), k = 0, i = 0; i < il; i++)\r\n for (var a = arguments[i], j = 0, jl = a.length; j < jl; j++, k++)\r\n r[k] = a[j];\r\n return r;\r\n};\r\n\r\nexport function __await(v) {\r\n return this instanceof __await ? (this.v = v, this) : new __await(v);\r\n}\r\n\r\nexport function __asyncGenerator(thisArg, _arguments, generator) {\r\n if (!Symbol.asyncIterator) throw new TypeError(\"Symbol.asyncIterator is not defined.\");\r\n var g = generator.apply(thisArg, _arguments || []), i, q = [];\r\n return i = {}, verb(\"next\"), verb(\"throw\"), verb(\"return\"), i[Symbol.asyncIterator] = function () { return this; }, i;\r\n function verb(n) { if (g[n]) i[n] = function (v) { return new Promise(function (a, b) { q.push([n, v, a, b]) > 1 || resume(n, v); }); }; }\r\n function resume(n, v) { try { step(g[n](v)); } catch (e) { settle(q[0][3], e); } }\r\n function step(r) { r.value instanceof __await ? Promise.resolve(r.value.v).then(fulfill, reject) : settle(q[0][2], r); }\r\n function fulfill(value) { resume(\"next\", value); }\r\n function reject(value) { resume(\"throw\", value); }\r\n function settle(f, v) { if (f(v), q.shift(), q.length) resume(q[0][0], q[0][1]); }\r\n}\r\n\r\nexport function __asyncDelegator(o) {\r\n var i, p;\r\n return i = {}, verb(\"next\"), verb(\"throw\", function (e) { throw e; }), verb(\"return\"), i[Symbol.iterator] = function () { return this; }, i;\r\n function verb(n, f) { i[n] = o[n] ? function (v) { return (p = !p) ? { value: __await(o[n](v)), done: n === \"return\" } : f ? f(v) : v; } : f; }\r\n}\r\n\r\nexport function __asyncValues(o) {\r\n if (!Symbol.asyncIterator) throw new TypeError(\"Symbol.asyncIterator is not defined.\");\r\n var m = o[Symbol.asyncIterator], i;\r\n return m ? m.call(o) : (o = typeof __values === \"function\" ? __values(o) : o[Symbol.iterator](), i = {}, verb(\"next\"), verb(\"throw\"), verb(\"return\"), i[Symbol.asyncIterator] = function () { return this; }, i);\r\n function verb(n) { i[n] = o[n] && function (v) { return new Promise(function (resolve, reject) { v = o[n](v), settle(resolve, reject, v.done, v.value); }); }; }\r\n function settle(resolve, reject, d, v) { Promise.resolve(v).then(function(v) { resolve({ value: v, done: d }); }, reject); }\r\n}\r\n\r\nexport function __makeTemplateObject(cooked, raw) {\r\n if (Object.defineProperty) { Object.defineProperty(cooked, \"raw\", { value: raw }); } else { cooked.raw = raw; }\r\n return cooked;\r\n};\r\n\r\nexport function __importStar(mod) {\r\n if (mod && mod.__esModule) return mod;\r\n var result = {};\r\n if (mod != null) for (var k in mod) if (Object.hasOwnProperty.call(mod, k)) result[k] = mod[k];\r\n result.default = mod;\r\n return result;\r\n}\r\n\r\nexport function __importDefault(mod) {\r\n return (mod && mod.__esModule) ? mod : { default: mod };\r\n}\r\n\r\nexport function __classPrivateFieldGet(receiver, privateMap) {\r\n if (!privateMap.has(receiver)) {\r\n throw new TypeError(\"attempted to get private field on non-instance\");\r\n }\r\n return privateMap.get(receiver);\r\n}\r\n\r\nexport function __classPrivateFieldSet(receiver, privateMap, value) {\r\n if (!privateMap.has(receiver)) {\r\n throw new TypeError(\"attempted to set private field on non-instance\");\r\n }\r\n privateMap.set(receiver, value);\r\n return value;\r\n}\r\n",null,null,null,null,null,null,null,null,null,null],"names":["performance","global","now","mozNow","msNow","oNow","webkitNow","Date","getTime","inherits","Object","create","ctor","superCtor","super_","prototype","constructor","value","enumerable","writable","configurable","TempCtor","formatRegExp","format","f","isString","objects","i","arguments","length","push","inspect","join","args","len","str","String","replace","x","Number","JSON","stringify","_","isNull","isObject","deprecate","fn","msg","isUndefined","process","apply","warned","deprecated","console","error","debugs","debugEnviron","debuglog","set","toUpperCase","RegExp","test","pid","obj","opts","ctx","seen","stylize","stylizeNoColor","depth","colors","isBoolean","showHidden","_extend","customInspect","stylizeWithColor","formatValue","styles","styleType","style","arrayToHash","array","hash","forEach","val","idx","recurseTimes","isFunction","ret","primitive","formatPrimitive","keys","visibleKeys","getOwnPropertyNames","isError","indexOf","formatError","name","isRegExp","toString","call","isDate","base","braces","isArray","n","toUTCString","output","formatArray","map","key","formatProperty","pop","reduceToSingleString","simple","isNumber","Error","l","hasOwnProperty","match","desc","getOwnPropertyDescriptor","get","split","line","substr","reduce","prev","cur","numLinesEst","ar","Array","arg","isNullOrUndefined","isSymbol","re","objectToString","d","e","isPrimitive","isBuffer","maybeBuf","Buffer","o","pad","months","timestamp","time","getHours","getMinutes","getSeconds","getDate","getMonth","log","origin","add","prop","buffer_1","utils_1","ensure_buffer_1","uuid_utils_1","binary_1","buffer","uuid_1","extendStatics","b","setPrototypeOf","__proto__","p","__extends","__","__assign","assign","t","s","__rest","getOwnPropertySymbols","propertyIsEnumerable","__decorate","decorators","target","c","r","Reflect","decorate","defineProperty","__param","paramIndex","decorator","__metadata","metadataKey","metadataValue","metadata","__awaiter","thisArg","_arguments","P","generator","adopt","resolve","Promise","reject","fulfilled","step","next","rejected","result","done","then","__generator","body","label","sent","trys","ops","y","g","verb","Symbol","iterator","v","op","TypeError","__createBinding","m","k","k2","undefined","__exportStar","exports","__values","__read","__spread","concat","__spreadArrays","il","a","j","jl","__await","__asyncGenerator","asyncIterator","q","resume","settle","fulfill","shift","__asyncDelegator","__asyncValues","__makeTemplateObject","cooked","raw","__importStar","mod","__esModule","__importDefault","__classPrivateFieldGet","receiver","privateMap","has","__classPrivateFieldSet","tslib_1","objectid_1","symbol_1","int_32_1","decimal128_1","min_key_1","max_key_1","regexp_1","timestamp_1","code_1","db_ref_1","validate_utf8_1","decimal128","symbol","float_parser_1","extended_json_1","map_1","serializer_1","deserializer_1","calculate_size_1"],"mappings":";;;;;;;;;;;;AAAA,iBAAe,EAAf;;ACAA;;AAoKA,IAAIA,WAAW,GAAGC,MAAM,CAACD,WAAP,IAAsB,EAAxC;;AAEEA,WAAW,CAACE,GAAZ,IACAF,WAAW,CAACG,MADZ,IAEAH,WAAW,CAACI,KAFZ,IAGAJ,WAAW,CAACK,IAHZ,IAIAL,WAAW,CAACM,SAJZ,IAKA,YAAU;AAAE,SAAQ,IAAIC,IAAJ,EAAD,CAAaC,OAAb,EAAP;AAA+B;;AC1K7C,IAAIC,QAAJ;;AACA,IAAI,OAAOC,MAAM,CAACC,MAAd,KAAyB,UAA7B,EAAwC;AACtCF,EAAAA,QAAQ,GAAG,SAASA,QAAT,CAAkBG,IAAlB,EAAwBC,SAAxB,EAAmC;AAC5C;AACAD,IAAAA,IAAI,CAACE,MAAL,GAAcD,SAAd;AACAD,IAAAA,IAAI,CAACG,SAAL,GAAiBL,MAAM,CAACC,MAAP,CAAcE,SAAS,CAACE,SAAxB,EAAmC;AAClDC,MAAAA,WAAW,EAAE;AACXC,QAAAA,KAAK,EAAEL,IADI;AAEXM,QAAAA,UAAU,EAAE,KAFD;AAGXC,QAAAA,QAAQ,EAAE,IAHC;AAIXC,QAAAA,YAAY,EAAE;AAJH;AADqC,KAAnC,CAAjB;AAQD,GAXD;AAYD,CAbD,MAaO;AACLX,EAAAA,QAAQ,GAAG,SAASA,QAAT,CAAkBG,IAAlB,EAAwBC,SAAxB,EAAmC;AAC5CD,IAAAA,IAAI,CAACE,MAAL,GAAcD,SAAd;;AACA,QAAIQ,QAAQ,GAAG,SAAXA,QAAW,GAAY,EAA3B;;AACAA,IAAAA,QAAQ,CAACN,SAAT,GAAqBF,SAAS,CAACE,SAA/B;AACAH,IAAAA,IAAI,CAACG,SAAL,GAAiB,IAAIM,QAAJ,EAAjB;AACAT,IAAAA,IAAI,CAACG,SAAL,CAAeC,WAAf,GAA6BJ,IAA7B;AACD,GAND;AAOD;;AACD,iBAAeH,QAAf;;ACxBA;AAqBA,IAAIa,YAAY,GAAG,UAAnB;AACO,SAASC,MAAT,CAAgBC,CAAhB,EAAmB;AACxB,MAAI,CAACC,QAAQ,CAACD,CAAD,CAAb,EAAkB;AAChB,QAAIE,OAAO,GAAG,EAAd;;AACA,SAAK,IAAIC,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGC,SAAS,CAACC,MAA9B,EAAsCF,CAAC,EAAvC,EAA2C;AACzCD,MAAAA,OAAO,CAACI,IAAR,CAAaC,OAAO,CAACH,SAAS,CAACD,CAAD,CAAV,CAApB;AACD;;AACD,WAAOD,OAAO,CAACM,IAAR,CAAa,GAAb,CAAP;AACD;;AAED,MAAIL,CAAC,GAAG,CAAR;AACA,MAAIM,IAAI,GAAGL,SAAX;AACA,MAAIM,GAAG,GAAGD,IAAI,CAACJ,MAAf;AACA,MAAIM,GAAG,GAAGC,MAAM,CAACZ,CAAD,CAAN,CAAUa,OAAV,CAAkBf,YAAlB,EAAgC,UAASgB,CAAT,EAAY;AACpD,QAAIA,CAAC,KAAK,IAAV,EAAgB,OAAO,GAAP;AAChB,QAAIX,CAAC,IAAIO,GAAT,EAAc,OAAOI,CAAP;;AACd,YAAQA,CAAR;AACE,WAAK,IAAL;AAAW,eAAOF,MAAM,CAACH,IAAI,CAACN,CAAC,EAAF,CAAL,CAAb;;AACX,WAAK,IAAL;AAAW,eAAOY,MAAM,CAACN,IAAI,CAACN,CAAC,EAAF,CAAL,CAAb;;AACX,WAAK,IAAL;AACE,YAAI;AACF,iBAAOa,IAAI,CAACC,SAAL,CAAeR,IAAI,CAACN,CAAC,EAAF,CAAnB,CAAP;AACD,SAFD,CAEE,OAAOe,CAAP,EAAU;AACV,iBAAO,YAAP;AACD;;AACH;AACE,eAAOJ,CAAP;AAVJ;AAYD,GAfS,CAAV;;AAgBA,OAAK,IAAIA,CAAC,GAAGL,IAAI,CAACN,CAAD,CAAjB,EAAsBA,CAAC,GAAGO,GAA1B,EAA+BI,CAAC,GAAGL,IAAI,CAAC,EAAEN,CAAH,CAAvC,EAA8C;AAC5C,QAAIgB,MAAM,CAACL,CAAD,CAAN,IAAa,CAACM,QAAQ,CAACN,CAAD,CAA1B,EAA+B;AAC7BH,MAAAA,GAAG,IAAI,MAAMG,CAAb;AACD,KAFD,MAEO;AACLH,MAAAA,GAAG,IAAI,MAAMJ,OAAO,CAACO,CAAD,CAApB;AACD;AACF;;AACD,SAAOH,GAAP;AACD;AAID;AACA;;AACO,SAASU,SAAT,CAAmBC,EAAnB,EAAuBC,GAAvB,EAA4B;AACjC;AACA,MAAIC,WAAW,CAAC/C,MAAM,CAACgD,OAAR,CAAf,EAAiC;AAC/B,WAAO,YAAW;AAChB,aAAOJ,SAAS,CAACC,EAAD,EAAKC,GAAL,CAAT,CAAmBG,KAAnB,CAAyB,IAAzB,EAA+BtB,SAA/B,CAAP;AACD,KAFD;AAGD;;AAMD,MAAIuB,MAAM,GAAG,KAAb;;AACA,WAASC,UAAT,GAAsB;AACpB,QAAI,CAACD,MAAL,EAAa;AACX,MAIO;AACLE,QAAAA,OAAO,CAACC,KAAR,CAAcP,GAAd;AACD;;AACDI,MAAAA,MAAM,GAAG,IAAT;AACD;;AACD,WAAOL,EAAE,CAACI,KAAH,CAAS,IAAT,EAAetB,SAAf,CAAP;AACD;;AAED,SAAOwB,UAAP;AACD;AAGD,IAAIG,MAAM,GAAG,EAAb;AACA,IAAIC,YAAJ;AACO,SAASC,QAAT,CAAkBC,GAAlB,EAAuB;AAC5B,MAAIV,WAAW,CAACQ,YAAD,CAAf,EACEA,YAAY,GAA6B,EAAzC;AACFE,EAAAA,GAAG,GAAGA,GAAG,CAACC,WAAJ,EAAN;;AACA,MAAI,CAACJ,MAAM,CAACG,GAAD,CAAX,EAAkB;AAChB,QAAI,IAAIE,MAAJ,CAAW,QAAQF,GAAR,GAAc,KAAzB,EAAgC,GAAhC,EAAqCG,IAArC,CAA0CL,YAA1C,CAAJ,EAA6D;AAC3D,UAAIM,GAAG,GAAG,CAAV;;AACAP,MAAAA,MAAM,CAACG,GAAD,CAAN,GAAc,YAAW;AACvB,YAAIX,GAAG,GAAGxB,MAAM,CAAC2B,KAAP,CAAa,IAAb,EAAmBtB,SAAnB,CAAV;AACAyB,QAAAA,OAAO,CAACC,KAAR,CAAc,WAAd,EAA2BI,GAA3B,EAAgCI,GAAhC,EAAqCf,GAArC;AACD,OAHD;AAID,KAND,MAMO;AACLQ,MAAAA,MAAM,CAACG,GAAD,CAAN,GAAc,YAAW,EAAzB;AACD;AACF;;AACD,SAAOH,MAAM,CAACG,GAAD,CAAb;AACD;AAGD;AACA;AACA;AACA;AACA;AACA;AACA;;AACA;;AACO,SAAS3B,OAAT,CAAiBgC,GAAjB,EAAsBC,IAAtB,EAA4B;AACjC;AACA,MAAIC,GAAG,GAAG;AACRC,IAAAA,IAAI,EAAE,EADE;AAERC,IAAAA,OAAO,EAAEC;AAFD,GAAV,CAFiC;;AAOjC,MAAIxC,SAAS,CAACC,MAAV,IAAoB,CAAxB,EAA2BoC,GAAG,CAACI,KAAJ,GAAYzC,SAAS,CAAC,CAAD,CAArB;AAC3B,MAAIA,SAAS,CAACC,MAAV,IAAoB,CAAxB,EAA2BoC,GAAG,CAACK,MAAJ,GAAa1C,SAAS,CAAC,CAAD,CAAtB;;AAC3B,MAAI2C,SAAS,CAACP,IAAD,CAAb,EAAqB;AACnB;AACAC,IAAAA,GAAG,CAACO,UAAJ,GAAiBR,IAAjB;AACD,GAHD,MAGO,IAAIA,IAAJ,EAAU;AACf;AACAS,IAAAA,OAAO,CAACR,GAAD,EAAMD,IAAN,CAAP;AACD,GAfgC;;;AAiBjC,MAAIhB,WAAW,CAACiB,GAAG,CAACO,UAAL,CAAf,EAAiCP,GAAG,CAACO,UAAJ,GAAiB,KAAjB;AACjC,MAAIxB,WAAW,CAACiB,GAAG,CAACI,KAAL,CAAf,EAA4BJ,GAAG,CAACI,KAAJ,GAAY,CAAZ;AAC5B,MAAIrB,WAAW,CAACiB,GAAG,CAACK,MAAL,CAAf,EAA6BL,GAAG,CAACK,MAAJ,GAAa,KAAb;AAC7B,MAAItB,WAAW,CAACiB,GAAG,CAACS,aAAL,CAAf,EAAoCT,GAAG,CAACS,aAAJ,GAAoB,IAApB;AACpC,MAAIT,GAAG,CAACK,MAAR,EAAgBL,GAAG,CAACE,OAAJ,GAAcQ,gBAAd;AAChB,SAAOC,WAAW,CAACX,GAAD,EAAMF,GAAN,EAAWE,GAAG,CAACI,KAAf,CAAlB;AACD;;AAGDtC,OAAO,CAACuC,MAAR,GAAiB;AACf,UAAS,CAAC,CAAD,EAAI,EAAJ,CADM;AAEf,YAAW,CAAC,CAAD,EAAI,EAAJ,CAFI;AAGf,eAAc,CAAC,CAAD,EAAI,EAAJ,CAHC;AAIf,aAAY,CAAC,CAAD,EAAI,EAAJ,CAJG;AAKf,WAAU,CAAC,EAAD,EAAK,EAAL,CALK;AAMf,UAAS,CAAC,EAAD,EAAK,EAAL,CANM;AAOf,WAAU,CAAC,EAAD,EAAK,EAAL,CAPK;AAQf,UAAS,CAAC,EAAD,EAAK,EAAL,CARM;AASf,UAAS,CAAC,EAAD,EAAK,EAAL,CATM;AAUf,WAAU,CAAC,EAAD,EAAK,EAAL,CAVK;AAWf,aAAY,CAAC,EAAD,EAAK,EAAL,CAXG;AAYf,SAAQ,CAAC,EAAD,EAAK,EAAL,CAZO;AAaf,YAAW,CAAC,EAAD,EAAK,EAAL;AAbI,CAAjB;;AAiBAvC,OAAO,CAAC8C,MAAR,GAAiB;AACf,aAAW,MADI;AAEf,YAAU,QAFK;AAGf,aAAW,QAHI;AAIf,eAAa,MAJE;AAKf,UAAQ,MALO;AAMf,YAAU,OANK;AAOf,UAAQ,SAPO;AAQf;AACA,YAAU;AATK,CAAjB;;AAaA,SAASF,gBAAT,CAA0BxC,GAA1B,EAA+B2C,SAA/B,EAA0C;AACxC,MAAIC,KAAK,GAAGhD,OAAO,CAAC8C,MAAR,CAAeC,SAAf,CAAZ;;AAEA,MAAIC,KAAJ,EAAW;AACT,WAAO,UAAYhD,OAAO,CAACuC,MAAR,CAAeS,KAAf,EAAsB,CAAtB,CAAZ,GAAuC,GAAvC,GAA6C5C,GAA7C,GACA,OADA,GACYJ,OAAO,CAACuC,MAAR,CAAeS,KAAf,EAAsB,CAAtB,CADZ,GACuC,GAD9C;AAED,GAHD,MAGO;AACL,WAAO5C,GAAP;AACD;AACF;;AAGD,SAASiC,cAAT,CAAwBjC,GAAxB,EAA6B2C,SAA7B,EAAwC;AACtC,SAAO3C,GAAP;AACD;;AAGD,SAAS6C,WAAT,CAAqBC,KAArB,EAA4B;AAC1B,MAAIC,IAAI,GAAG,EAAX;AAEAD,EAAAA,KAAK,CAACE,OAAN,CAAc,UAASC,GAAT,EAAcC,GAAd,EAAmB;AAC/BH,IAAAA,IAAI,CAACE,GAAD,CAAJ,GAAY,IAAZ;AACD,GAFD;AAIA,SAAOF,IAAP;AACD;;AAGD,SAASN,WAAT,CAAqBX,GAArB,EAA0BhD,KAA1B,EAAiCqE,YAAjC,EAA+C;AAC7C;AACA;AACA,MAAIrB,GAAG,CAACS,aAAJ,IACAzD,KADA,IAEAsE,UAAU,CAACtE,KAAK,CAACc,OAAP,CAFV;AAIAd,EAAAA,KAAK,CAACc,OAAN,KAAkBA,OAJlB;AAMA,IAAEd,KAAK,CAACD,WAAN,IAAqBC,KAAK,CAACD,WAAN,CAAkBD,SAAlB,KAAgCE,KAAvD,CANJ,EAMmE;AACjE,QAAIuE,GAAG,GAAGvE,KAAK,CAACc,OAAN,CAAcuD,YAAd,EAA4BrB,GAA5B,CAAV;;AACA,QAAI,CAACxC,QAAQ,CAAC+D,GAAD,CAAb,EAAoB;AAClBA,MAAAA,GAAG,GAAGZ,WAAW,CAACX,GAAD,EAAMuB,GAAN,EAAWF,YAAX,CAAjB;AACD;;AACD,WAAOE,GAAP;AACD,GAf4C;;;AAkB7C,MAAIC,SAAS,GAAGC,eAAe,CAACzB,GAAD,EAAMhD,KAAN,CAA/B;;AACA,MAAIwE,SAAJ,EAAe;AACb,WAAOA,SAAP;AACD,GArB4C;;;AAwB7C,MAAIE,IAAI,GAAGjF,MAAM,CAACiF,IAAP,CAAY1E,KAAZ,CAAX;AACA,MAAI2E,WAAW,GAAGZ,WAAW,CAACW,IAAD,CAA7B;;AAEA,MAAI1B,GAAG,CAACO,UAAR,EAAoB;AAClBmB,IAAAA,IAAI,GAAGjF,MAAM,CAACmF,mBAAP,CAA2B5E,KAA3B,CAAP;AACD,GA7B4C;AAgC7C;;;AACA,MAAI6E,OAAO,CAAC7E,KAAD,CAAP,KACI0E,IAAI,CAACI,OAAL,CAAa,SAAb,KAA2B,CAA3B,IAAgCJ,IAAI,CAACI,OAAL,CAAa,aAAb,KAA+B,CADnE,CAAJ,EAC2E;AACzE,WAAOC,WAAW,CAAC/E,KAAD,CAAlB;AACD,GApC4C;;;AAuC7C,MAAI0E,IAAI,CAAC9D,MAAL,KAAgB,CAApB,EAAuB;AACrB,QAAI0D,UAAU,CAACtE,KAAD,CAAd,EAAuB;AACrB,UAAIgF,IAAI,GAAGhF,KAAK,CAACgF,IAAN,GAAa,OAAOhF,KAAK,CAACgF,IAA1B,GAAiC,EAA5C;AACA,aAAOhC,GAAG,CAACE,OAAJ,CAAY,cAAc8B,IAAd,GAAqB,GAAjC,EAAsC,SAAtC,CAAP;AACD;;AACD,QAAIC,QAAQ,CAACjF,KAAD,CAAZ,EAAqB;AACnB,aAAOgD,GAAG,CAACE,OAAJ,CAAYP,MAAM,CAAC7C,SAAP,CAAiBoF,QAAjB,CAA0BC,IAA1B,CAA+BnF,KAA/B,CAAZ,EAAmD,QAAnD,CAAP;AACD;;AACD,QAAIoF,MAAM,CAACpF,KAAD,CAAV,EAAmB;AACjB,aAAOgD,GAAG,CAACE,OAAJ,CAAY5D,IAAI,CAACQ,SAAL,CAAeoF,QAAf,CAAwBC,IAAxB,CAA6BnF,KAA7B,CAAZ,EAAiD,MAAjD,CAAP;AACD;;AACD,QAAI6E,OAAO,CAAC7E,KAAD,CAAX,EAAoB;AAClB,aAAO+E,WAAW,CAAC/E,KAAD,CAAlB;AACD;AACF;;AAED,MAAIqF,IAAI,GAAG,EAAX;AAAA,MAAerB,KAAK,GAAG,KAAvB;AAAA,MAA8BsB,MAAM,GAAG,CAAC,GAAD,EAAM,GAAN,CAAvC,CAvD6C;;AA0D7C,MAAIC,OAAO,CAACvF,KAAD,CAAX,EAAoB;AAClBgE,IAAAA,KAAK,GAAG,IAAR;AACAsB,IAAAA,MAAM,GAAG,CAAC,GAAD,EAAM,GAAN,CAAT;AACD,GA7D4C;;;AAgE7C,MAAIhB,UAAU,CAACtE,KAAD,CAAd,EAAuB;AACrB,QAAIwF,CAAC,GAAGxF,KAAK,CAACgF,IAAN,GAAa,OAAOhF,KAAK,CAACgF,IAA1B,GAAiC,EAAzC;AACAK,IAAAA,IAAI,GAAG,eAAeG,CAAf,GAAmB,GAA1B;AACD,GAnE4C;;;AAsE7C,MAAIP,QAAQ,CAACjF,KAAD,CAAZ,EAAqB;AACnBqF,IAAAA,IAAI,GAAG,MAAM1C,MAAM,CAAC7C,SAAP,CAAiBoF,QAAjB,CAA0BC,IAA1B,CAA+BnF,KAA/B,CAAb;AACD,GAxE4C;;;AA2E7C,MAAIoF,MAAM,CAACpF,KAAD,CAAV,EAAmB;AACjBqF,IAAAA,IAAI,GAAG,MAAM/F,IAAI,CAACQ,SAAL,CAAe2F,WAAf,CAA2BN,IAA3B,CAAgCnF,KAAhC,CAAb;AACD,GA7E4C;;;AAgF7C,MAAI6E,OAAO,CAAC7E,KAAD,CAAX,EAAoB;AAClBqF,IAAAA,IAAI,GAAG,MAAMN,WAAW,CAAC/E,KAAD,CAAxB;AACD;;AAED,MAAI0E,IAAI,CAAC9D,MAAL,KAAgB,CAAhB,KAAsB,CAACoD,KAAD,IAAUhE,KAAK,CAACY,MAAN,IAAgB,CAAhD,CAAJ,EAAwD;AACtD,WAAO0E,MAAM,CAAC,CAAD,CAAN,GAAYD,IAAZ,GAAmBC,MAAM,CAAC,CAAD,CAAhC;AACD;;AAED,MAAIjB,YAAY,GAAG,CAAnB,EAAsB;AACpB,QAAIY,QAAQ,CAACjF,KAAD,CAAZ,EAAqB;AACnB,aAAOgD,GAAG,CAACE,OAAJ,CAAYP,MAAM,CAAC7C,SAAP,CAAiBoF,QAAjB,CAA0BC,IAA1B,CAA+BnF,KAA/B,CAAZ,EAAmD,QAAnD,CAAP;AACD,KAFD,MAEO;AACL,aAAOgD,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAP;AACD;AACF;;AAEDF,EAAAA,GAAG,CAACC,IAAJ,CAASpC,IAAT,CAAcb,KAAd;AAEA,MAAI0F,MAAJ;;AACA,MAAI1B,KAAJ,EAAW;AACT0B,IAAAA,MAAM,GAAGC,WAAW,CAAC3C,GAAD,EAAMhD,KAAN,EAAaqE,YAAb,EAA2BM,WAA3B,EAAwCD,IAAxC,CAApB;AACD,GAFD,MAEO;AACLgB,IAAAA,MAAM,GAAGhB,IAAI,CAACkB,GAAL,CAAS,UAASC,GAAT,EAAc;AAC9B,aAAOC,cAAc,CAAC9C,GAAD,EAAMhD,KAAN,EAAaqE,YAAb,EAA2BM,WAA3B,EAAwCkB,GAAxC,EAA6C7B,KAA7C,CAArB;AACD,KAFQ,CAAT;AAGD;;AAEDhB,EAAAA,GAAG,CAACC,IAAJ,CAAS8C,GAAT;AAEA,SAAOC,oBAAoB,CAACN,MAAD,EAASL,IAAT,EAAeC,MAAf,CAA3B;AACD;;AAGD,SAASb,eAAT,CAAyBzB,GAAzB,EAA8BhD,KAA9B,EAAqC;AACnC,MAAI+B,WAAW,CAAC/B,KAAD,CAAf,EACE,OAAOgD,GAAG,CAACE,OAAJ,CAAY,WAAZ,EAAyB,WAAzB,CAAP;;AACF,MAAI1C,QAAQ,CAACR,KAAD,CAAZ,EAAqB;AACnB,QAAIiG,MAAM,GAAG,OAAO1E,IAAI,CAACC,SAAL,CAAexB,KAAf,EAAsBoB,OAAtB,CAA8B,QAA9B,EAAwC,EAAxC,EACsBA,OADtB,CAC8B,IAD9B,EACoC,KADpC,EAEsBA,OAFtB,CAE8B,MAF9B,EAEsC,GAFtC,CAAP,GAEoD,IAFjE;AAGA,WAAO4B,GAAG,CAACE,OAAJ,CAAY+C,MAAZ,EAAoB,QAApB,CAAP;AACD;;AACD,MAAIC,QAAQ,CAAClG,KAAD,CAAZ,EACE,OAAOgD,GAAG,CAACE,OAAJ,CAAY,KAAKlD,KAAjB,EAAwB,QAAxB,CAAP;AACF,MAAIsD,SAAS,CAACtD,KAAD,CAAb,EACE,OAAOgD,GAAG,CAACE,OAAJ,CAAY,KAAKlD,KAAjB,EAAwB,SAAxB,CAAP,CAZiC;;AAcnC,MAAI0B,MAAM,CAAC1B,KAAD,CAAV,EACE,OAAOgD,GAAG,CAACE,OAAJ,CAAY,MAAZ,EAAoB,MAApB,CAAP;AACH;;AAGD,SAAS6B,WAAT,CAAqB/E,KAArB,EAA4B;AAC1B,SAAO,MAAMmG,KAAK,CAACrG,SAAN,CAAgBoF,QAAhB,CAAyBC,IAAzB,CAA8BnF,KAA9B,CAAN,GAA6C,GAApD;AACD;;AAGD,SAAS2F,WAAT,CAAqB3C,GAArB,EAA0BhD,KAA1B,EAAiCqE,YAAjC,EAA+CM,WAA/C,EAA4DD,IAA5D,EAAkE;AAChE,MAAIgB,MAAM,GAAG,EAAb;;AACA,OAAK,IAAIhF,CAAC,GAAG,CAAR,EAAW0F,CAAC,GAAGpG,KAAK,CAACY,MAA1B,EAAkCF,CAAC,GAAG0F,CAAtC,EAAyC,EAAE1F,CAA3C,EAA8C;AAC5C,QAAI2F,cAAc,CAACrG,KAAD,EAAQmB,MAAM,CAACT,CAAD,CAAd,CAAlB,EAAsC;AACpCgF,MAAAA,MAAM,CAAC7E,IAAP,CAAYiF,cAAc,CAAC9C,GAAD,EAAMhD,KAAN,EAAaqE,YAAb,EAA2BM,WAA3B,EACtBxD,MAAM,CAACT,CAAD,CADgB,EACX,IADW,CAA1B;AAED,KAHD,MAGO;AACLgF,MAAAA,MAAM,CAAC7E,IAAP,CAAY,EAAZ;AACD;AACF;;AACD6D,EAAAA,IAAI,CAACR,OAAL,CAAa,UAAS2B,GAAT,EAAc;AACzB,QAAI,CAACA,GAAG,CAACS,KAAJ,CAAU,OAAV,CAAL,EAAyB;AACvBZ,MAAAA,MAAM,CAAC7E,IAAP,CAAYiF,cAAc,CAAC9C,GAAD,EAAMhD,KAAN,EAAaqE,YAAb,EAA2BM,WAA3B,EACtBkB,GADsB,EACjB,IADiB,CAA1B;AAED;AACF,GALD;AAMA,SAAOH,MAAP;AACD;;AAGD,SAASI,cAAT,CAAwB9C,GAAxB,EAA6BhD,KAA7B,EAAoCqE,YAApC,EAAkDM,WAAlD,EAA+DkB,GAA/D,EAAoE7B,KAApE,EAA2E;AACzE,MAAIgB,IAAJ,EAAU9D,GAAV,EAAeqF,IAAf;AACAA,EAAAA,IAAI,GAAG9G,MAAM,CAAC+G,wBAAP,CAAgCxG,KAAhC,EAAuC6F,GAAvC,KAA+C;AAAE7F,IAAAA,KAAK,EAAEA,KAAK,CAAC6F,GAAD;AAAd,GAAtD;;AACA,MAAIU,IAAI,CAACE,GAAT,EAAc;AACZ,QAAIF,IAAI,CAAC9D,GAAT,EAAc;AACZvB,MAAAA,GAAG,GAAG8B,GAAG,CAACE,OAAJ,CAAY,iBAAZ,EAA+B,SAA/B,CAAN;AACD,KAFD,MAEO;AACLhC,MAAAA,GAAG,GAAG8B,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAN;AACD;AACF,GAND,MAMO;AACL,QAAIqD,IAAI,CAAC9D,GAAT,EAAc;AACZvB,MAAAA,GAAG,GAAG8B,GAAG,CAACE,OAAJ,CAAY,UAAZ,EAAwB,SAAxB,CAAN;AACD;AACF;;AACD,MAAI,CAACmD,cAAc,CAAC1B,WAAD,EAAckB,GAAd,CAAnB,EAAuC;AACrCb,IAAAA,IAAI,GAAG,MAAMa,GAAN,GAAY,GAAnB;AACD;;AACD,MAAI,CAAC3E,GAAL,EAAU;AACR,QAAI8B,GAAG,CAACC,IAAJ,CAAS6B,OAAT,CAAiByB,IAAI,CAACvG,KAAtB,IAA+B,CAAnC,EAAsC;AACpC,UAAI0B,MAAM,CAAC2C,YAAD,CAAV,EAA0B;AACxBnD,QAAAA,GAAG,GAAGyC,WAAW,CAACX,GAAD,EAAMuD,IAAI,CAACvG,KAAX,EAAkB,IAAlB,CAAjB;AACD,OAFD,MAEO;AACLkB,QAAAA,GAAG,GAAGyC,WAAW,CAACX,GAAD,EAAMuD,IAAI,CAACvG,KAAX,EAAkBqE,YAAY,GAAG,CAAjC,CAAjB;AACD;;AACD,UAAInD,GAAG,CAAC4D,OAAJ,CAAY,IAAZ,IAAoB,CAAC,CAAzB,EAA4B;AAC1B,YAAId,KAAJ,EAAW;AACT9C,UAAAA,GAAG,GAAGA,GAAG,CAACwF,KAAJ,CAAU,IAAV,EAAgBd,GAAhB,CAAoB,UAASe,IAAT,EAAe;AACvC,mBAAO,OAAOA,IAAd;AACD,WAFK,EAEH5F,IAFG,CAEE,IAFF,EAEQ6F,MAFR,CAEe,CAFf,CAAN;AAGD,SAJD,MAIO;AACL1F,UAAAA,GAAG,GAAG,OAAOA,GAAG,CAACwF,KAAJ,CAAU,IAAV,EAAgBd,GAAhB,CAAoB,UAASe,IAAT,EAAe;AAC9C,mBAAO,QAAQA,IAAf;AACD,WAFY,EAEV5F,IAFU,CAEL,IAFK,CAAb;AAGD;AACF;AACF,KAjBD,MAiBO;AACLG,MAAAA,GAAG,GAAG8B,GAAG,CAACE,OAAJ,CAAY,YAAZ,EAA0B,SAA1B,CAAN;AACD;AACF;;AACD,MAAInB,WAAW,CAACiD,IAAD,CAAf,EAAuB;AACrB,QAAIhB,KAAK,IAAI6B,GAAG,CAACS,KAAJ,CAAU,OAAV,CAAb,EAAiC;AAC/B,aAAOpF,GAAP;AACD;;AACD8D,IAAAA,IAAI,GAAGzD,IAAI,CAACC,SAAL,CAAe,KAAKqE,GAApB,CAAP;;AACA,QAAIb,IAAI,CAACsB,KAAL,CAAW,8BAAX,CAAJ,EAAgD;AAC9CtB,MAAAA,IAAI,GAAGA,IAAI,CAAC4B,MAAL,CAAY,CAAZ,EAAe5B,IAAI,CAACpE,MAAL,GAAc,CAA7B,CAAP;AACAoE,MAAAA,IAAI,GAAGhC,GAAG,CAACE,OAAJ,CAAY8B,IAAZ,EAAkB,MAAlB,CAAP;AACD,KAHD,MAGO;AACLA,MAAAA,IAAI,GAAGA,IAAI,CAAC5D,OAAL,CAAa,IAAb,EAAmB,KAAnB,EACKA,OADL,CACa,MADb,EACqB,GADrB,EAEKA,OAFL,CAEa,UAFb,EAEyB,GAFzB,CAAP;AAGA4D,MAAAA,IAAI,GAAGhC,GAAG,CAACE,OAAJ,CAAY8B,IAAZ,EAAkB,QAAlB,CAAP;AACD;AACF;;AAED,SAAOA,IAAI,GAAG,IAAP,GAAc9D,GAArB;AACD;;AAGD,SAAS8E,oBAAT,CAA8BN,MAA9B,EAAsCL,IAAtC,EAA4CC,MAA5C,EAAoD;AAElD,MAAI1E,MAAM,GAAG8E,MAAM,CAACmB,MAAP,CAAc,UAASC,IAAT,EAAeC,GAAf,EAAoB;AAE7C,QAAIA,GAAG,CAACjC,OAAJ,CAAY,IAAZ,KAAqB,CAAzB,EAA4BkC;AAC5B,WAAOF,IAAI,GAAGC,GAAG,CAAC3F,OAAJ,CAAY,iBAAZ,EAA+B,EAA/B,EAAmCR,MAA1C,GAAmD,CAA1D;AACD,GAJY,EAIV,CAJU,CAAb;;AAMA,MAAIA,MAAM,GAAG,EAAb,EAAiB;AACf,WAAO0E,MAAM,CAAC,CAAD,CAAN,IACCD,IAAI,KAAK,EAAT,GAAc,EAAd,GAAmBA,IAAI,GAAG,KAD3B,IAEA,GAFA,GAGAK,MAAM,CAAC3E,IAAP,CAAY,OAAZ,CAHA,GAIA,GAJA,GAKAuE,MAAM,CAAC,CAAD,CALb;AAMD;;AAED,SAAOA,MAAM,CAAC,CAAD,CAAN,GAAYD,IAAZ,GAAmB,GAAnB,GAAyBK,MAAM,CAAC3E,IAAP,CAAY,IAAZ,CAAzB,GAA6C,GAA7C,GAAmDuE,MAAM,CAAC,CAAD,CAAhE;AACD;AAID;;;AACO,SAASC,OAAT,CAAiB0B,EAAjB,EAAqB;AAC1B,SAAOC,KAAK,CAAC3B,OAAN,CAAc0B,EAAd,CAAP;AACD;AAEM,SAAS3D,SAAT,CAAmB6D,GAAnB,EAAwB;AAC7B,SAAO,OAAOA,GAAP,KAAe,SAAtB;AACD;AAEM,SAASzF,MAAT,CAAgByF,GAAhB,EAAqB;AAC1B,SAAOA,GAAG,KAAK,IAAf;AACD;AAEM,SAASC,iBAAT,CAA2BD,GAA3B,EAAgC;AACrC,SAAOA,GAAG,IAAI,IAAd;AACD;AAEM,SAASjB,QAAT,CAAkBiB,GAAlB,EAAuB;AAC5B,SAAO,OAAOA,GAAP,KAAe,QAAtB;AACD;AAEM,SAAS3G,QAAT,CAAkB2G,GAAlB,EAAuB;AAC5B,SAAO,OAAOA,GAAP,KAAe,QAAtB;AACD;AAEM,SAASE,QAAT,CAAkBF,GAAlB,EAAuB;AAC5B,SAAO,uBAAOA,GAAP,MAAe,QAAtB;AACD;AAEM,SAASpF,WAAT,CAAqBoF,GAArB,EAA0B;AAC/B,SAAOA,GAAG,KAAK,KAAK,CAApB;AACD;AAEM,SAASlC,QAAT,CAAkBqC,EAAlB,EAAsB;AAC3B,SAAO3F,QAAQ,CAAC2F,EAAD,CAAR,IAAgBC,cAAc,CAACD,EAAD,CAAd,KAAuB,iBAA9C;AACD;AAEM,SAAS3F,QAAT,CAAkBwF,GAAlB,EAAuB;AAC5B,SAAO,uBAAOA,GAAP,MAAe,QAAf,IAA2BA,GAAG,KAAK,IAA1C;AACD;AAEM,SAAS/B,MAAT,CAAgBoC,CAAhB,EAAmB;AACxB,SAAO7F,QAAQ,CAAC6F,CAAD,CAAR,IAAeD,cAAc,CAACC,CAAD,CAAd,KAAsB,eAA5C;AACD;AAEM,SAAS3C,OAAT,CAAiB4C,CAAjB,EAAoB;AACzB,SAAO9F,QAAQ,CAAC8F,CAAD,CAAR,KACFF,cAAc,CAACE,CAAD,CAAd,KAAsB,gBAAtB,IAA0CA,CAAC,YAAYtB,KADrD,CAAP;AAED;AAEM,SAAS7B,UAAT,CAAoB6C,GAApB,EAAyB;AAC9B,SAAO,OAAOA,GAAP,KAAe,UAAtB;AACD;AAEM,SAASO,WAAT,CAAqBP,GAArB,EAA0B;AAC/B,SAAOA,GAAG,KAAK,IAAR,IACA,OAAOA,GAAP,KAAe,SADf,IAEA,OAAOA,GAAP,KAAe,QAFf,IAGA,OAAOA,GAAP,KAAe,QAHf,IAIA,uBAAOA,GAAP,MAAe,QAJf;AAKA,SAAOA,GAAP,KAAe,WALtB;AAMD;AAEM,SAASQ,QAAT,CAAkBC,QAAlB,EAA4B;AACjC,SAAOC,MAAM,CAACF,QAAP,CAAgBC,QAAhB,CAAP;AACD;;AAED,SAASL,cAAT,CAAwBO,CAAxB,EAA2B;AACzB,SAAOrI,MAAM,CAACK,SAAP,CAAiBoF,QAAjB,CAA0BC,IAA1B,CAA+B2C,CAA/B,CAAP;AACD;;AAGD,SAASC,GAAT,CAAavC,CAAb,EAAgB;AACd,SAAOA,CAAC,GAAG,EAAJ,GAAS,MAAMA,CAAC,CAACN,QAAF,CAAW,EAAX,CAAf,GAAgCM,CAAC,CAACN,QAAF,CAAW,EAAX,CAAvC;AACD;;AAGD,IAAI8C,MAAM,GAAG,CAAC,KAAD,EAAQ,KAAR,EAAe,KAAf,EAAsB,KAAtB,EAA6B,KAA7B,EAAoC,KAApC,EAA2C,KAA3C,EAAkD,KAAlD,EAAyD,KAAzD,EACC,KADD,EACQ,KADR,EACe,KADf,CAAb;;AAIA,SAASC,WAAT,GAAqB;AACnB,MAAIT,CAAC,GAAG,IAAIlI,IAAJ,EAAR;AACA,MAAI4I,IAAI,GAAG,CAACH,GAAG,CAACP,CAAC,CAACW,QAAF,EAAD,CAAJ,EACCJ,GAAG,CAACP,CAAC,CAACY,UAAF,EAAD,CADJ,EAECL,GAAG,CAACP,CAAC,CAACa,UAAF,EAAD,CAFJ,EAEsBtH,IAFtB,CAE2B,GAF3B,CAAX;AAGA,SAAO,CAACyG,CAAC,CAACc,OAAF,EAAD,EAAcN,MAAM,CAACR,CAAC,CAACe,QAAF,EAAD,CAApB,EAAoCL,IAApC,EAA0CnH,IAA1C,CAA+C,GAA/C,CAAP;AACD;;;AAIM,SAASyH,GAAT,GAAe;AACpBpG,EAAAA,OAAO,CAACoG,GAAR,CAAY,SAAZ,EAAuBP,WAAS,EAAhC,EAAoC3H,MAAM,CAAC2B,KAAP,CAAa,IAAb,EAAmBtB,SAAnB,CAApC;AACD;AAmBM,SAAS6C,OAAT,CAAiBiF,MAAjB,EAAyBC,GAAzB,EAA8B;AACnC;AACA,MAAI,CAACA,GAAD,IAAQ,CAAC/G,QAAQ,CAAC+G,GAAD,CAArB,EAA4B,OAAOD,MAAP;AAE5B,MAAI/D,IAAI,GAAGjF,MAAM,CAACiF,IAAP,CAAYgE,GAAZ,CAAX;AACA,MAAIhI,CAAC,GAAGgE,IAAI,CAAC9D,MAAb;;AACA,SAAOF,CAAC,EAAR,EAAY;AACV+H,IAAAA,MAAM,CAAC/D,IAAI,CAAChE,CAAD,CAAL,CAAN,GAAkBgI,GAAG,CAAChE,IAAI,CAAChE,CAAD,CAAL,CAArB;AACD;;AACD,SAAO+H,MAAP;AACD;;AAED,SAASpC,cAAT,CAAwBvD,GAAxB,EAA6B6F,IAA7B,EAAmC;AACjC,SAAOlJ,MAAM,CAACK,SAAP,CAAiBuG,cAAjB,CAAgClB,IAAhC,CAAqCrC,GAArC,EAA0C6F,IAA1C,CAAP;AACD;;AAED,iBAAe;AACbnJ,EAAAA,QAAQ,EAAEA,UADG;AAEbgE,EAAAA,OAAO,EAAEA,OAFI;AAGbgF,EAAAA,GAAG,EAAEA,GAHQ;AAIbb,EAAAA,QAAQ,EAAEA,QAJG;AAKbD,EAAAA,WAAW,EAAEA,WALA;AAMbpD,EAAAA,UAAU,EAAEA,UANC;AAObO,EAAAA,OAAO,EAAEA,OAPI;AAQbO,EAAAA,MAAM,EAAEA,MARK;AASbzD,EAAAA,QAAQ,EAAEA,QATG;AAUbsD,EAAAA,QAAQ,EAAEA,QAVG;AAWblD,EAAAA,WAAW,EAAEA,WAXA;AAYbsF,EAAAA,QAAQ,EAAEA,QAZG;AAab7G,EAAAA,QAAQ,EAAEA,QAbG;AAcb0F,EAAAA,QAAQ,EAAEA,QAdG;AAebkB,EAAAA,iBAAiB,EAAEA,iBAfN;AAgBb1F,EAAAA,MAAM,EAAEA,MAhBK;AAiBb4B,EAAAA,SAAS,EAAEA,SAjBE;AAkBbiC,EAAAA,OAAO,EAAEA,OAlBI;AAmBbzE,EAAAA,OAAO,EAAEA,OAnBI;AAoBbc,EAAAA,SAAS,EAAEA,SApBE;AAqBbtB,EAAAA,MAAM,EAAEA,MArBK;AAsBbkC,EAAAA,QAAQ,EAAEA;AAtBG,CAAf;;;;;AC9jBgC;AAIhC;;;;AAIA,SAAgB,wBAAwB,CAAC,EAAY;IACnD,OAAO,EAAE,CAAC,QAAQ,EAAE,CAAC,OAAO,CAAC,WAAW,EAAE,YAAY,CAAC,CAAC;AAC1D,CAAC;AAFD,4DAEC;AAED,IAAM,aAAa,GACjB,OAAOxD,cAAM,CAAC,SAAS,KAAK,QAAQ,IAAIA,cAAM,CAAC,SAAS,CAAC,OAAO,KAAK,aAAa,CAAC;AAErF,IAAM,eAAe,GAAG,aAAa;MACjC,0IAA0I;MAC1I,+GAA+G,CAAC;AAEpH,IAAM,mBAAmB,GAAwB,SAAS,mBAAmB,CAAC,IAAY;IACxF,OAAO,CAAC,IAAI,CAAC,eAAe,CAAC,CAAC;IAE9B,IAAM,MAAM,GAAG4J,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;IAClC,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,EAAE,EAAE,CAAC;QAAE,MAAM,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,MAAM,EAAE,GAAG,GAAG,CAAC,CAAC;IAC3E,OAAO,MAAM,CAAC;AAChB,CAAC,CAAC;AAUF,IAAM,iBAAiB,GAAG;IACxB,IAAI,OAAO,MAAM,KAAK,WAAW,EAAE;;QAEjC,IAAM,QAAM,GAAG,MAAM,CAAC,MAAM,IAAI,MAAM,CAAC,QAAQ,CAAC;QAChD,IAAI,QAAM,IAAI,QAAM,CAAC,eAAe,EAAE;YACpC,OAAO,UAAA,IAAI,IAAI,OAAA,QAAM,CAAC,eAAe,CAACA,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,GAAA,CAAC;SAC3D;KACF;IAED,IAAI,OAAO5J,cAAM,KAAK,WAAW,IAAIA,cAAM,CAAC,MAAM,IAAIA,cAAM,CAAC,MAAM,CAAC,eAAe,EAAE;;QAEnF,OAAO,UAAA,IAAI,IAAI,OAAAA,cAAM,CAAC,MAAM,CAAC,eAAe,CAAC4J,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,GAAA,CAAC;KAClE;IAED,IAAI,mBAA2D,CAAC;IAChE,IAAI;;QAEF,mBAAmB,GAAG,UAAiB,CAAC,WAAW,CAAC;KACrD;IAAC,OAAO,CAAC,EAAE;;KAEX;;IAID,OAAO,mBAAmB,IAAI,mBAAmB,CAAC;AACpD,CAAC,CAAC;AAEW,mBAAW,GAAG,iBAAiB,EAAE,CAAC;AAE/C,SAAgB,gBAAgB,CAAC,KAAc;IAC7C,OAAO,CAAC,sBAAsB,EAAE,4BAA4B,CAAC,CAAC,QAAQ,CACpE,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CACtC,CAAC;AACJ,CAAC;AAJD,4CAIC;AAED,SAAgB,YAAY,CAAC,KAAc;IACzC,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,qBAAqB,CAAC;AACzE,CAAC;AAFD,oCAEC;AAED,SAAgB,eAAe,CAAC,KAAc;IAC5C,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,wBAAwB,CAAC;AAC5E,CAAC;AAFD,0CAEC;AAED,SAAgB,gBAAgB,CAAC,KAAc;IAC7C,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,yBAAyB,CAAC;AAC7E,CAAC;AAFD,4CAEC;AAED,SAAgB,QAAQ,CAAC,CAAU;IACjC,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,iBAAiB,CAAC;AACjE,CAAC;AAFD,4BAEC;AAED,SAAgB,KAAK,CAAC,CAAU;IAC9B,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,cAAc,CAAC;AAC9D,CAAC;AAFD,sBAEC;AAED;AACA,SAAgB,UAAU;IACxB,OAAO,OAAO5J,cAAM,KAAK,WAAW,IAAI,OAAOA,cAAM,CAAC,MAAM,KAAK,WAAW,CAAC;AAC/E,CAAC;AAFD,gCAEC;AAED;AACA,SAAgB,MAAM,CAAC,CAAU;IAC/B,OAAO,YAAY,CAAC,CAAC,CAAC,IAAI,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,eAAe,CAAC;AAClF,CAAC;AAFD,wBAEC;AAED;;;;;AAKA,SAAgB,YAAY,CAAC,SAAkB;IAC7C,OAAO,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,KAAK,IAAI,CAAC;AAC7D,CAAC;AAFD,oCAEC;AAGD,SAAgB,SAAS,CAAqB,EAAK,EAAE,OAAe;IAClE,IAAI,OAAO,MAAM,KAAK,WAAW,IAAI,OAAO,IAAI,KAAK,WAAW,EAAE;;QAEhE,OAAO,UAAe,CAAC,SAAS,CAAC,EAAE,EAAE,OAAO,CAAC,CAAC;KAC/C;IACD,IAAI,MAAM,GAAG,KAAK,CAAC;IACnB,SAAS,UAAU;QAAgB,cAAkB;aAAlB,UAAkB,EAAlB,qBAAkB,EAAlB,IAAkB;YAAlB,yBAAkB;;QACnD,IAAI,CAAC,MAAM,EAAE;YACX,OAAO,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC;YACtB,MAAM,GAAG,IAAI,CAAC;SACf;QACD,OAAO,EAAE,CAAC,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;KAC7B;IACD,OAAQ,UAA2B,CAAC;AACtC,CAAC;AAdD,8BAcC;;;;;;;;;;;;;;;;;;;;;AC5H+B;AACkB;AAElD;;;;;;;;AAQA,SAAgB,YAAY,CAAC,eAAuD;IAClF,IAAI,WAAW,CAAC,MAAM,CAAC,eAAe,CAAC,EAAE;QACvC,OAAO4J,aAAM,CAAC,IAAI,CAChB,eAAe,CAAC,MAAM,EACtB,eAAe,CAAC,UAAU,EAC1B,eAAe,CAAC,UAAU,CAC3B,CAAC;KACH;IAED,IAAIC,sBAAgB,CAAC,eAAe,CAAC,EAAE;QACrC,OAAOD,aAAM,CAAC,IAAI,CAAC,eAAe,CAAC,CAAC;KACrC;IAED,MAAM,IAAI,SAAS,CAAC,sCAAsC,CAAC,CAAC;AAC9D,CAAC;AAdD,oCAcC;;;;;;;;;;ACzB+B;AAEhC;AACA,IAAM,gBAAgB,GAAG,uHAAuH,CAAC;AAE1I,IAAM,kBAAkB,GAAG,UAAC,GAAW;IAC5C,OAAA,OAAO,GAAG,KAAK,QAAQ,IAAI,gBAAgB,CAAC,IAAI,CAAC,GAAG,CAAC;AAArD,CAAqD,CAAC;AAD3C,0BAAkB,sBACyB;AAEjD,IAAM,qBAAqB,GAAG,UAAC,SAAiB;IACrD,IAAI,CAAC,0BAAkB,CAAC,SAAS,CAAC,EAAE;QAClC,MAAM,IAAI,SAAS,CACjB,uLAAuL,CACxL,CAAC;KACH;IAED,IAAM,kBAAkB,GAAG,SAAS,CAAC,OAAO,CAAC,IAAI,EAAE,EAAE,CAAC,CAAC;IACvD,OAAOA,aAAM,CAAC,IAAI,CAAC,kBAAkB,EAAE,KAAK,CAAC,CAAC;AAChD,CAAC,CAAC;AATW,6BAAqB,yBAShC;AAEK,IAAM,qBAAqB,GAAG,UAAC,MAAc,EAAE,aAAoB;IAApB,8BAAA,EAAA,oBAAoB;IACxE,OAAA,aAAa;UACT,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;YAC5B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;YAC5B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;YAC5B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,EAAE,CAAC;YAC7B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,EAAE,EAAE,EAAE,CAAC;UAC9B,MAAM,CAAC,QAAQ,CAAC,KAAK,CAAC;AAV1B,CAU0B,CAAC;AAXhB,6BAAqB,yBAWL;;;;;;;;;;;;AC9BG;AACe;AACb;AAC8D;AACrC;AAO3D,IAAM,WAAW,GAAG,EAAE,CAAC;AAEvB,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;AAEzB;;;;AAIA;;;;;;IAgBE,cAAY,KAA8B;QACxC,IAAI,OAAO,KAAK,KAAK,WAAW,EAAE;;YAEhC,IAAI,CAAC,EAAE,GAAG,IAAI,CAAC,QAAQ,EAAE,CAAC;SAC3B;aAAM,IAAI,KAAK,YAAY,IAAI,EAAE;YAChC,IAAI,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;YAClC,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,CAAC;SACxB;aAAM,IAAI,WAAW,CAAC,MAAM,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,UAAU,KAAK,WAAW,EAAE;YACxE,IAAI,CAAC,EAAE,GAAGE,0BAAY,CAAC,KAAK,CAAC,CAAC;SAC/B;aAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YACpC,IAAI,CAAC,EAAE,GAAGC,gCAAqB,CAAC,KAAK,CAAC,CAAC;SACxC;aAAM;YACL,MAAM,IAAI,SAAS,CACjB,gLAAgL,CACjL,CAAC;SACH;KACF;IAMD,sBAAI,oBAAE;;;;;aAAN;YACE,OAAO,IAAI,CAAC,GAAG,CAAC,CAAC;SAClB;aAED,UAAO,KAAa;YAClB,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;YAElB,IAAI,IAAI,CAAC,cAAc,EAAE;gBACvB,IAAI,CAAC,IAAI,GAAGA,gCAAqB,CAAC,KAAK,CAAC,CAAC;aAC1C;SACF;;;OARA;;;;;;;;IAkBD,0BAAW,GAAX,UAAY,aAAoB;QAApB,8BAAA,EAAA,oBAAoB;QAC9B,IAAI,IAAI,CAAC,cAAc,IAAI,IAAI,CAAC,IAAI,EAAE;YACpC,OAAO,IAAI,CAAC,IAAI,CAAC;SAClB;QAED,IAAM,aAAa,GAAGA,gCAAqB,CAAC,IAAI,CAAC,EAAE,EAAE,aAAa,CAAC,CAAC;QAEpE,IAAI,IAAI,CAAC,cAAc,EAAE;YACvB,IAAI,CAAC,IAAI,GAAG,aAAa,CAAC;SAC3B;QAED,OAAO,aAAa,CAAC;KACtB;;;;;IAMD,uBAAQ,GAAR,UAAS,QAAiB;QACxB,OAAO,QAAQ,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,QAAQ,CAAC,GAAG,IAAI,CAAC,WAAW,EAAE,CAAC;KACnE;;;;;IAMD,qBAAM,GAAN;QACE,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;KAC3B;;;;;;IAOD,qBAAM,GAAN,UAAO,OAA+B;QACpC,IAAI,CAAC,OAAO,EAAE;YACZ,OAAO,KAAK,CAAC;SACd;QAED,IAAI,OAAO,YAAY,IAAI,EAAE;YAC3B,OAAO,OAAO,CAAC,EAAE,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;SACnC;QAED,IAAI;YACF,OAAO,IAAI,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;SAC7C;QAAC,WAAM;YACN,OAAO,KAAK,CAAC;SACd;KACF;;;;IAKD,uBAAQ,GAAR;QACE,OAAO,IAAIC,aAAM,CAAC,IAAI,CAAC,EAAE,EAAEA,aAAM,CAAC,YAAY,CAAC,CAAC;KACjD;;;;IAKM,aAAQ,GAAf;QACE,IAAM,KAAK,GAAGH,iBAAW,CAAC,WAAW,CAAC,CAAC;;;QAIvC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,IAAI,IAAI,CAAC;QACpC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,IAAI,IAAI,CAAC;QAEpC,OAAOD,aAAM,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;KAC3B;;;;;IAMM,YAAO,GAAd,UAAe,KAA6B;QAC1C,IAAI,CAAC,KAAK,EAAE;YACV,OAAO,KAAK,CAAC;SACd;QAED,IAAI,KAAK,YAAY,IAAI,EAAE;YACzB,OAAO,IAAI,CAAC;SACb;QAED,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YAC7B,OAAOG,6BAAkB,CAAC,KAAK,CAAC,CAAC;SAClC;QAED,IAAIF,kBAAY,CAAC,KAAK,CAAC,EAAE;;YAEvB,IAAI,KAAK,CAAC,MAAM,KAAK,WAAW,EAAE;gBAChC,OAAO,KAAK,CAAC;aACd;YAED,IAAI;;;gBAGF,OAAO,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,QAAQ,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,KAAKG,aAAM,CAAC,YAAY,CAAC;aACvE;YAAC,WAAM;gBACN,OAAO,KAAK,CAAC;aACd;SACF;QAED,OAAO,KAAK,CAAC;KACd;;;;;IAMM,wBAAmB,GAA1B,UAA2B,SAAiB;QAC1C,IAAM,MAAM,GAAGD,gCAAqB,CAAC,SAAS,CAAC,CAAC;QAChD,OAAO,IAAI,IAAI,CAAC,MAAM,CAAC,CAAC;KACzB;;;;;;;IAQD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,sBAAO,GAAP;QACE,OAAO,gBAAa,IAAI,CAAC,WAAW,EAAE,QAAI,CAAC;KAC5C;IACH,WAAC;AAAD,CAAC,IAAA;AA3LY,oBAAI;AA6LjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;AChNtC;AACe;AACM;AACT;AAoB5C;;;;AAIA;;;;;IAkCE,gBAAYE,QAAgC,EAAE,OAAgB;QAC5D,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,CAACA,QAAM,EAAE,OAAO,CAAC,CAAC;QAElE,IACE,EAAEA,QAAM,IAAI,IAAI,CAAC;YACjB,EAAE,OAAOA,QAAM,KAAK,QAAQ,CAAC;YAC7B,CAAC,WAAW,CAAC,MAAM,CAACA,QAAM,CAAC;YAC3B,EAAEA,QAAM,YAAY,WAAW,CAAC;YAChC,CAAC,KAAK,CAAC,OAAO,CAACA,QAAM,CAAC,EACtB;YACA,MAAM,IAAI,SAAS,CACjB,kFAAkF,CACnF,CAAC;SACH;QAED,IAAI,CAAC,QAAQ,GAAG,OAAO,aAAP,OAAO,cAAP,OAAO,GAAI,MAAM,CAAC,2BAA2B,CAAC;QAE9D,IAAIA,QAAM,IAAI,IAAI,EAAE;;YAElB,IAAI,CAAC,MAAM,GAAGL,aAAM,CAAC,KAAK,CAAC,MAAM,CAAC,WAAW,CAAC,CAAC;YAC/C,IAAI,CAAC,QAAQ,GAAG,CAAC,CAAC;SACnB;aAAM;YACL,IAAI,OAAOK,QAAM,KAAK,QAAQ,EAAE;;gBAE9B,IAAI,CAAC,MAAM,GAAGL,aAAM,CAAC,IAAI,CAACK,QAAM,EAAE,QAAQ,CAAC,CAAC;aAC7C;iBAAM,IAAI,KAAK,CAAC,OAAO,CAACA,QAAM,CAAC,EAAE;;gBAEhC,IAAI,CAAC,MAAM,GAAGL,aAAM,CAAC,IAAI,CAACK,QAAM,CAAC,CAAC;aACnC;iBAAM;;gBAEL,IAAI,CAAC,MAAM,GAAGH,0BAAY,CAACG,QAAM,CAAC,CAAC;aACpC;YAED,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;SACxC;KACF;;;;;;IAOD,oBAAG,GAAH,UAAI,SAA2D;;QAE7D,IAAI,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,CAAC,MAAM,KAAK,CAAC,EAAE;YAC3D,MAAM,IAAI,SAAS,CAAC,sCAAsC,CAAC,CAAC;SAC7D;aAAM,IAAI,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,CAAC,MAAM,KAAK,CAAC;YAChE,MAAM,IAAI,SAAS,CAAC,mDAAmD,CAAC,CAAC;;QAG3E,IAAI,WAAmB,CAAC;QACxB,IAAI,OAAO,SAAS,KAAK,QAAQ,EAAE;YACjC,WAAW,GAAG,SAAS,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;SACvC;aAAM,IAAI,OAAO,SAAS,KAAK,QAAQ,EAAE;YACxC,WAAW,GAAG,SAAS,CAAC;SACzB;aAAM;YACL,WAAW,GAAG,SAAS,CAAC,CAAC,CAAC,CAAC;SAC5B;QAED,IAAI,WAAW,GAAG,CAAC,IAAI,WAAW,GAAG,GAAG,EAAE;YACxC,MAAM,IAAI,SAAS,CAAC,0DAA0D,CAAC,CAAC;SACjF;QAED,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,EAAE;YACtC,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,GAAG,WAAW,CAAC;SAC5C;aAAM;YACL,IAAMA,QAAM,GAAGL,aAAM,CAAC,KAAK,CAAC,MAAM,CAAC,WAAW,GAAG,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;;YAErE,IAAI,CAAC,MAAM,CAAC,IAAI,CAACK,QAAM,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;YACnD,IAAI,CAAC,MAAM,GAAGA,QAAM,CAAC;YACrB,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,GAAG,WAAW,CAAC;SAC5C;KACF;;;;;;;IAQD,sBAAK,GAAL,UAAM,QAAiC,EAAE,MAAc;QACrD,MAAM,GAAG,OAAO,MAAM,KAAK,QAAQ,GAAG,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;;QAG7D,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,EAAE;YACjD,IAAMA,QAAM,GAAGL,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,QAAQ,CAAC,MAAM,CAAC,CAAC;YAClE,IAAI,CAAC,MAAM,CAAC,IAAI,CAACK,QAAM,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;;YAGnD,IAAI,CAAC,MAAM,GAAGA,QAAM,CAAC;SACtB;QAED,IAAI,WAAW,CAAC,MAAM,CAAC,QAAQ,CAAC,EAAE;YAChC,IAAI,CAAC,MAAM,CAAC,GAAG,CAACH,0BAAY,CAAC,QAAQ,CAAC,EAAE,MAAM,CAAC,CAAC;YAChD,IAAI,CAAC,QAAQ;gBACX,MAAM,GAAG,QAAQ,CAAC,UAAU,GAAG,IAAI,CAAC,QAAQ,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;SAC3F;aAAM,IAAI,OAAO,QAAQ,KAAK,QAAQ,EAAE;YACvC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,QAAQ,EAAE,MAAM,EAAE,QAAQ,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;YAC/D,IAAI,CAAC,QAAQ;gBACX,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;SACvF;KACF;;;;;;;IAQD,qBAAI,GAAJ,UAAK,QAAgB,EAAE,MAAc;QACnC,MAAM,GAAG,MAAM,IAAI,MAAM,GAAG,CAAC,GAAG,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC;;QAGvD,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,QAAQ,EAAE,QAAQ,GAAG,MAAM,CAAC,CAAC;KACvD;;;;;;;IAQD,sBAAK,GAAL,UAAM,KAAe;QACnB,KAAK,GAAG,CAAC,CAAC,KAAK,CAAC;;QAGhB,IAAI,KAAK,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,KAAK,IAAI,CAAC,QAAQ,EAAE;YACjD,OAAO,IAAI,CAAC,MAAM,CAAC;SACpB;;QAGD,IAAI,KAAK,EAAE;YACT,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC5C;QACD,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KACzD;;IAGD,uBAAM,GAAN;QACE,OAAO,IAAI,CAAC,QAAQ,CAAC;KACtB;;IAGD,uBAAM,GAAN;QACE,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;KACvC;;IAGD,yBAAQ,GAAR,UAAS,MAAc;QACrB,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC;KACrC;;IAGD,+BAAc,GAAd,UAAe,OAAsB;QACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAM,YAAY,GAAG,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;QAEpD,IAAM,OAAO,GAAG,MAAM,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,QAAQ,CAAC,EAAE,CAAC,CAAC;QACnD,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO;gBACL,OAAO,EAAE,YAAY;gBACrB,KAAK,EAAE,OAAO,CAAC,MAAM,KAAK,CAAC,GAAG,GAAG,GAAG,OAAO,GAAG,OAAO;aACtD,CAAC;SACH;QACD,OAAO;YACL,OAAO,EAAE;gBACP,MAAM,EAAE,YAAY;gBACpB,OAAO,EAAE,OAAO,CAAC,MAAM,KAAK,CAAC,GAAG,GAAG,GAAG,OAAO,GAAG,OAAO;aACxD;SACF,CAAC;KACH;;IAGD,uBAAM,GAAN;QACE,IAAI,IAAI,CAAC,QAAQ,KAAK,MAAM,CAAC,YAAY,EAAE;YACzC,OAAO,IAAII,SAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC;SACtD;QAED,MAAM,IAAI,KAAK,CACb,uBAAoB,IAAI,CAAC,QAAQ,2DAAoD,MAAM,CAAC,YAAY,+BAA2B,CACpI,CAAC;KACH;;IAGM,uBAAgB,GAAvB,UACE,GAAyD,EACzD,OAAsB;QAEtB,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAI,IAAwB,CAAC;QAC7B,IAAI,IAAI,CAAC;QACT,IAAI,SAAS,IAAI,GAAG,EAAE;YACpB,IAAI,OAAO,CAAC,MAAM,IAAI,OAAO,GAAG,CAAC,OAAO,KAAK,QAAQ,IAAI,OAAO,IAAI,GAAG,EAAE;gBACvE,IAAI,GAAG,GAAG,CAAC,KAAK,GAAG,QAAQ,CAAC,GAAG,CAAC,KAAK,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;gBAC/C,IAAI,GAAGN,aAAM,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;aAC3C;iBAAM;gBACL,IAAI,OAAO,GAAG,CAAC,OAAO,KAAK,QAAQ,EAAE;oBACnC,IAAI,GAAG,GAAG,CAAC,OAAO,CAAC,OAAO,GAAG,QAAQ,CAAC,GAAG,CAAC,OAAO,CAAC,OAAO,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;oBACnE,IAAI,GAAGA,aAAM,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;iBAClD;aACF;SACF;aAAM,IAAI,OAAO,IAAI,GAAG,EAAE;YACzB,IAAI,GAAG,CAAC,CAAC;YACT,IAAI,GAAGG,gCAAqB,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC;SACzC;QACD,IAAI,CAAC,IAAI,EAAE;YACT,MAAM,IAAI,SAAS,CAAC,4CAA0C,IAAI,CAAC,SAAS,CAAC,GAAG,CAAG,CAAC,CAAC;SACtF;QACD,OAAO,IAAI,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;KAC/B;;IAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,wBAAO,GAAP;QACE,IAAM,QAAQ,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;QAClC,OAAO,8BAA2B,QAAQ,CAAC,QAAQ,CAAC,KAAK,CAAC,sBAAc,IAAI,CAAC,QAAQ,MAAG,CAAC;KAC1F;;;;;IAtPuB,kCAA2B,GAAG,CAAC,CAAC;;IAGxC,kBAAW,GAAG,GAAG,CAAC;;IAElB,sBAAe,GAAG,CAAC,CAAC;;IAEpB,uBAAgB,GAAG,CAAC,CAAC;;IAErB,yBAAkB,GAAG,CAAC,CAAC;;IAEvB,uBAAgB,GAAG,CAAC,CAAC;;IAErB,mBAAY,GAAG,CAAC,CAAC;;IAEjB,kBAAW,GAAG,CAAC,CAAC;;IAEhB,2BAAoB,GAAG,GAAG,CAAC;IAsO7C,aAAC;CA9PD,IA8PC;AA9PY,wBAAM;AAgQnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;ACnR1E;;;;AAIA;;;;;IASE,cAAY,IAAuB,EAAE,KAAgB;QACnD,IAAI,EAAE,IAAI,YAAY,IAAI,CAAC;YAAE,OAAO,IAAI,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;QAE1D,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC;QACjB,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;KACpB;;IAGD,qBAAM,GAAN;QACE,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,IAAI,EAAE,KAAK,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;KAC/C;;IAGD,6BAAc,GAAd;QACE,IAAI,IAAI,CAAC,KAAK,EAAE;YACd,OAAO,EAAE,KAAK,EAAE,IAAI,CAAC,IAAI,EAAE,MAAM,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;SACjD;QAED,OAAO,EAAE,KAAK,EAAE,IAAI,CAAC,IAAI,EAAE,CAAC;KAC7B;;IAGM,qBAAgB,GAAvB,UAAwB,GAAiB;QACvC,OAAO,IAAI,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,MAAM,CAAC,CAAC;KACxC;;IAGD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,sBAAO,GAAP;QACE,IAAM,QAAQ,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC;QAC/B,OAAO,gBAAa,QAAQ,CAAC,IAAI,WAC/B,QAAQ,CAAC,KAAK,GAAG,OAAK,IAAI,CAAC,SAAS,CAAC,QAAQ,CAAC,KAAK,CAAG,GAAG,EAAE,OAC1D,CAAC;KACL;IACH,WAAC;AAAD,CAAC,IAAA;AA9CY,oBAAI;AAgDjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;ACzDxB;AAS9C;AACA,SAAgB,WAAW,CAAC,KAAc;IACxC,QACEF,kBAAY,CAAC,KAAK,CAAC;QACnB,KAAK,CAAC,GAAG,IAAI,IAAI;QACjB,OAAO,KAAK,CAAC,IAAI,KAAK,QAAQ;SAC7B,KAAK,CAAC,GAAG,IAAI,IAAI,IAAI,OAAO,KAAK,CAAC,GAAG,KAAK,QAAQ,CAAC,EACpD;AACJ,CAAC;AAPD,kCAOC;AAED;;;;AAIA;;;;;;IAaE,eAAY,UAAkB,EAAE,GAAa,EAAE,EAAW,EAAE,MAAiB;QAC3E,IAAI,EAAE,IAAI,YAAY,KAAK,CAAC;YAAE,OAAO,IAAI,KAAK,CAAC,UAAU,EAAE,GAAG,EAAE,EAAE,EAAE,MAAM,CAAC,CAAC;;QAG5E,IAAM,KAAK,GAAG,UAAU,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;QACpC,IAAI,KAAK,CAAC,MAAM,KAAK,CAAC,EAAE;YACtB,EAAE,GAAG,KAAK,CAAC,KAAK,EAAE,CAAC;;YAEnB,UAAU,GAAG,KAAK,CAAC,KAAK,EAAG,CAAC;SAC7B;QAED,IAAI,CAAC,UAAU,GAAG,UAAU,CAAC;QAC7B,IAAI,CAAC,GAAG,GAAG,GAAG,CAAC;QACf,IAAI,CAAC,EAAE,GAAG,EAAE,CAAC;QACb,IAAI,CAAC,MAAM,GAAG,MAAM,IAAI,EAAE,CAAC;KAC5B;IAMD,sBAAI,4BAAS;;;;aAAb;YACE,OAAO,IAAI,CAAC,UAAU,CAAC;SACxB;aAED,UAAc,KAAa;YACzB,IAAI,CAAC,UAAU,GAAG,KAAK,CAAC;SACzB;;;OAJA;;IAOD,sBAAM,GAAN;QACE,IAAM,CAAC,GAAG,MAAM,CAAC,MAAM,CACrB;YACE,IAAI,EAAE,IAAI,CAAC,UAAU;YACrB,GAAG,EAAE,IAAI,CAAC,GAAG;SACd,EACD,IAAI,CAAC,MAAM,CACZ,CAAC;QAEF,IAAI,IAAI,CAAC,EAAE,IAAI,IAAI;YAAE,CAAC,CAAC,GAAG,GAAG,IAAI,CAAC,EAAE,CAAC;QACrC,OAAO,CAAC,CAAC;KACV;;IAGD,8BAAc,GAAd,UAAe,OAAsB;QACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAI,CAAC,GAAc;YACjB,IAAI,EAAE,IAAI,CAAC,UAAU;YACrB,GAAG,EAAE,IAAI,CAAC,GAAG;SACd,CAAC;QAEF,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO,CAAC,CAAC;SACV;QAED,IAAI,IAAI,CAAC,EAAE;YAAE,CAAC,CAAC,GAAG,GAAG,IAAI,CAAC,EAAE,CAAC;QAC7B,CAAC,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,CAAC;QAClC,OAAO,CAAC,CAAC;KACV;;IAGM,sBAAgB,GAAvB,UAAwB,GAAc;QACpC,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,GAAG,CAAuB,CAAC;QAC1D,OAAO,IAAI,CAAC,IAAI,CAAC;QACjB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAI,KAAK,CAAC,GAAG,CAAC,IAAI,EAAE,GAAG,CAAC,GAAG,EAAE,GAAG,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;KACpD;;IAGD,gBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,uBAAO,GAAP;;QAEE,IAAM,GAAG,GACP,IAAI,CAAC,GAAG,KAAK,SAAS,IAAI,IAAI,CAAC,GAAG,CAAC,QAAQ,KAAK,SAAS,GAAG,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,QAAQ,EAAE,CAAC;QAC7F,OAAO,iBAAc,IAAI,CAAC,SAAS,2BAAoB,GAAG,YACxD,IAAI,CAAC,EAAE,GAAG,SAAM,IAAI,CAAC,EAAE,OAAG,GAAG,EAAE,OAC9B,CAAC;KACL;IACH,YAAC;AAAD,CAAC,IAAA;AA/FY,sBAAK;AAiGlB,MAAM,CAAC,cAAc,CAAC,KAAK,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,OAAO,EAAE,CAAC,CAAC;;;;;;;;;;;AC1H1B;AAkB9C;;;AAGA,IAAI,IAAI,GAAgC,SAAS,CAAC;AAMlD,IAAI;IACF,IAAI,GAAI,IAAI,WAAW,CAAC,QAAQ,CAC9B,IAAI,WAAW,CAAC,MAAM;;IAEpB,IAAI,UAAU,CAAC,CAAC,CAAC,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,CAAC,CAAC,CAC/oC,EACD,EAAE,CACH,CAAC,OAAsC,CAAC;CAC1C;AAAC,WAAM;;CAEP;AAED,IAAM,cAAc,GAAG,CAAC,IAAI,EAAE,CAAC;AAC/B,IAAM,cAAc,GAAG,CAAC,IAAI,EAAE,CAAC;AAC/B,IAAM,cAAc,GAAG,cAAc,GAAG,cAAc,CAAC;AACvD,IAAM,cAAc,GAAG,cAAc,GAAG,cAAc,CAAC;AACvD,IAAM,cAAc,GAAG,cAAc,GAAG,CAAC,CAAC;AAE1C;AACA,IAAM,SAAS,GAA4B,EAAE,CAAC;AAE9C;AACA,IAAM,UAAU,GAA4B,EAAE,CAAC;AAO/C;;;;;;;;;;;;;;;;;;AAkBA;;;;;;;;;;;;;;IAkCE,cAAY,GAAiC,EAAE,IAAuB,EAAE,QAAkB;QAA9E,oBAAA,EAAA,OAAiC;QAC3C,IAAI,EAAE,IAAI,YAAY,IAAI,CAAC;YAAE,OAAO,IAAI,IAAI,CAAC,GAAG,EAAE,IAAI,EAAE,QAAQ,CAAC,CAAC;QAElE,IAAI,OAAO,GAAG,KAAK,QAAQ,EAAE;YAC3B,MAAM,CAAC,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;SACnD;aAAM,IAAI,OAAO,GAAG,KAAK,QAAQ,EAAE;YAClC,MAAM,CAAC,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;SACnD;aAAM;YACL,IAAI,CAAC,GAAG,GAAG,GAAG,GAAG,CAAC,CAAC;YACnB,IAAI,CAAC,IAAI,GAAI,IAAe,GAAG,CAAC,CAAC;YACjC,IAAI,CAAC,QAAQ,GAAG,CAAC,CAAC,QAAQ,CAAC;SAC5B;QAED,MAAM,CAAC,cAAc,CAAC,IAAI,EAAE,YAAY,EAAE;YACxC,KAAK,EAAE,IAAI;YACX,YAAY,EAAE,KAAK;YACnB,QAAQ,EAAE,KAAK;YACf,UAAU,EAAE,KAAK;SAClB,CAAC,CAAC;KACJ;;;;;;;;;IA6BM,aAAQ,GAAf,UAAgB,OAAe,EAAE,QAAgB,EAAE,QAAkB;QACnE,OAAO,IAAI,IAAI,CAAC,OAAO,EAAE,QAAQ,EAAE,QAAQ,CAAC,CAAC;KAC9C;;;;;;;IAQM,YAAO,GAAd,UAAe,KAAa,EAAE,QAAkB;QAC9C,IAAI,GAAG,EAAE,SAAS,EAAE,KAAK,CAAC;QAC1B,IAAI,QAAQ,EAAE;YACZ,KAAK,MAAM,CAAC,CAAC;YACb,KAAK,KAAK,GAAG,CAAC,IAAI,KAAK,IAAI,KAAK,GAAG,GAAG,GAAG;gBACvC,SAAS,GAAG,UAAU,CAAC,KAAK,CAAC,CAAC;gBAC9B,IAAI,SAAS;oBAAE,OAAO,SAAS,CAAC;aACjC;YACD,GAAG,GAAG,IAAI,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,KAAK,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,EAAE,IAAI,CAAC,CAAC;YAC3D,IAAI,KAAK;gBAAE,UAAU,CAAC,KAAK,CAAC,GAAG,GAAG,CAAC;YACnC,OAAO,GAAG,CAAC;SACZ;aAAM;YACL,KAAK,IAAI,CAAC,CAAC;YACX,KAAK,KAAK,GAAG,CAAC,GAAG,IAAI,KAAK,IAAI,KAAK,GAAG,GAAG,GAAG;gBAC1C,SAAS,GAAG,SAAS,CAAC,KAAK,CAAC,CAAC;gBAC7B,IAAI,SAAS;oBAAE,OAAO,SAAS,CAAC;aACjC;YACD,GAAG,GAAG,IAAI,CAAC,QAAQ,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;YACtD,IAAI,KAAK;gBAAE,SAAS,CAAC,KAAK,CAAC,GAAG,GAAG,CAAC;YAClC,OAAO,GAAG,CAAC;SACZ;KACF;;;;;;;IAQM,eAAU,GAAjB,UAAkB,KAAa,EAAE,QAAkB;QACjD,IAAI,KAAK,CAAC,KAAK,CAAC;YAAE,OAAO,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;QAC3D,IAAI,QAAQ,EAAE;YACZ,IAAI,KAAK,GAAG,CAAC;gBAAE,OAAO,IAAI,CAAC,KAAK,CAAC;YACjC,IAAI,KAAK,IAAI,cAAc;gBAAE,OAAO,IAAI,CAAC,kBAAkB,CAAC;SAC7D;aAAM;YACL,IAAI,KAAK,IAAI,CAAC,cAAc;gBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;YACpD,IAAI,KAAK,GAAG,CAAC,IAAI,cAAc;gBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;SACxD;QACD,IAAI,KAAK,GAAG,CAAC;YAAE,OAAO,IAAI,CAAC,UAAU,CAAC,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC,GAAG,EAAE,CAAC;QAC9D,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,GAAG,cAAc,GAAG,CAAC,EAAE,CAAC,KAAK,GAAG,cAAc,IAAI,CAAC,EAAE,QAAQ,CAAC,CAAC;KAC1F;;;;;;;IAQM,eAAU,GAAjB,UAAkB,KAAa,EAAE,QAAkB;QACjD,OAAO,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,QAAQ,EAAE,EAAE,QAAQ,CAAC,CAAC;KACpD;;;;;;;;IASM,eAAU,GAAjB,UAAkB,GAAW,EAAE,QAAkB,EAAE,KAAc;QAC/D,IAAI,GAAG,CAAC,MAAM,KAAK,CAAC;YAAE,MAAM,KAAK,CAAC,cAAc,CAAC,CAAC;QAClD,IAAI,GAAG,KAAK,KAAK,IAAI,GAAG,KAAK,UAAU,IAAI,GAAG,KAAK,WAAW,IAAI,GAAG,KAAK,WAAW;YACnF,OAAO,IAAI,CAAC,IAAI,CAAC;QACnB,IAAI,OAAO,QAAQ,KAAK,QAAQ,EAAE;;YAEhC,CAAC,KAAK,GAAG,QAAQ,IAAI,QAAQ,GAAG,KAAK,CAAC,CAAC;SACxC;aAAM;YACL,QAAQ,GAAG,CAAC,CAAC,QAAQ,CAAC;SACvB;QACD,KAAK,GAAG,KAAK,IAAI,EAAE,CAAC;QACpB,IAAI,KAAK,GAAG,CAAC,IAAI,EAAE,GAAG,KAAK;YAAE,MAAM,UAAU,CAAC,OAAO,CAAC,CAAC;QAEvD,IAAI,CAAC,CAAC;QACN,IAAI,CAAC,CAAC,GAAG,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,CAAC;YAAE,MAAM,KAAK,CAAC,iBAAiB,CAAC,CAAC;aAC1D,IAAI,CAAC,KAAK,CAAC,EAAE;YAChB,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,CAAC,EAAE,QAAQ,EAAE,KAAK,CAAC,CAAC,GAAG,EAAE,CAAC;SACjE;;;QAID,IAAM,YAAY,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC,CAAC;QAEzD,IAAI,MAAM,GAAG,IAAI,CAAC,IAAI,CAAC;QACvB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,GAAG,CAAC,MAAM,EAAE,CAAC,IAAI,CAAC,EAAE;YACtC,IAAM,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,GAAG,CAAC,MAAM,GAAG,CAAC,CAAC,EACtC,KAAK,GAAG,QAAQ,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,EAAE,KAAK,CAAC,CAAC;YACtD,IAAI,IAAI,GAAG,CAAC,EAAE;gBACZ,IAAM,KAAK,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;gBACrD,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,CAAC;aACxD;iBAAM;gBACL,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC;gBAClC,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,CAAC;aAC7C;SACF;QACD,MAAM,CAAC,QAAQ,GAAG,QAAQ,CAAC;QAC3B,OAAO,MAAM,CAAC;KACf;;;;;;;;IASM,cAAS,GAAhB,UAAiB,KAAe,EAAE,QAAkB,EAAE,EAAY;QAChE,OAAO,EAAE,GAAG,IAAI,CAAC,WAAW,CAAC,KAAK,EAAE,QAAQ,CAAC,GAAG,IAAI,CAAC,WAAW,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC;KACnF;;;;;;;IAQM,gBAAW,GAAlB,UAAmB,KAAe,EAAE,QAAkB;QACpD,OAAO,IAAI,IAAI,CACb,KAAK,CAAC,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,EAChE,KAAK,CAAC,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,EAChE,QAAQ,CACT,CAAC;KACH;;;;;;;IAQM,gBAAW,GAAlB,UAAmB,KAAe,EAAE,QAAkB;QACpD,OAAO,IAAI,IAAI,CACb,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,KAAK,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,EAChE,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,KAAK,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,EAChE,QAAQ,CACT,CAAC;KACH;;;;;IAMM,WAAM,GAAb,UAAc,KAAU;QACtB,OAAOA,kBAAY,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,YAAY,CAAC,KAAK,IAAI,CAAC;KAC5D;;;;;IAMM,cAAS,GAAhB,UACE,GAAwE,EACxE,QAAkB;QAElB,IAAI,OAAO,GAAG,KAAK,QAAQ;YAAE,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;QACnE,IAAI,OAAO,GAAG,KAAK,QAAQ;YAAE,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;;QAEnE,OAAO,IAAI,CAAC,QAAQ,CAClB,GAAG,CAAC,GAAG,EACP,GAAG,CAAC,IAAI,EACR,OAAO,QAAQ,KAAK,SAAS,GAAG,QAAQ,GAAG,GAAG,CAAC,QAAQ,CACxD,CAAC;KACH;;IAGD,kBAAG,GAAH,UAAI,MAA0C;QAC5C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC;YAAE,MAAM,GAAG,IAAI,CAAC,SAAS,CAAC,MAAM,CAAC,CAAC;;QAI1D,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,EAAE,CAAC;QAC7B,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,GAAG,MAAM,CAAC;QAC/B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC;QAC5B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,GAAG,MAAM,CAAC;QAE9B,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,KAAK,EAAE,CAAC;QAC/B,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,GAAG,MAAM,CAAC;QACjC,IAAM,GAAG,GAAG,MAAM,CAAC,GAAG,KAAK,EAAE,CAAC;QAC9B,IAAM,GAAG,GAAG,MAAM,CAAC,GAAG,GAAG,MAAM,CAAC;QAEhC,IAAI,GAAG,GAAG,CAAC,EACT,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,CAAC;QACV,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,MAAM,CAAC;QACd,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KAC3E;;;;;IAMD,kBAAG,GAAH,UAAI,KAAyC;QAC3C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KACnF;;;;;IAMD,sBAAO,GAAP,UAAQ,KAAyC;QAC/C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,IAAI,IAAI,CAAC,EAAE,CAAC,KAAK,CAAC;YAAE,OAAO,CAAC,CAAC;QAC7B,IAAM,OAAO,GAAG,IAAI,CAAC,UAAU,EAAE,EAC/B,QAAQ,GAAG,KAAK,CAAC,UAAU,EAAE,CAAC;QAChC,IAAI,OAAO,IAAI,CAAC,QAAQ;YAAE,OAAO,CAAC,CAAC,CAAC;QACpC,IAAI,CAAC,OAAO,IAAI,QAAQ;YAAE,OAAO,CAAC,CAAC;;QAEnC,IAAI,CAAC,IAAI,CAAC,QAAQ;YAAE,OAAO,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,UAAU,EAAE,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;QAEjE,OAAO,KAAK,CAAC,IAAI,KAAK,CAAC,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC;aACtC,KAAK,CAAC,IAAI,KAAK,IAAI,CAAC,IAAI,IAAI,KAAK,CAAC,GAAG,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;cAC5D,CAAC,CAAC;cACF,CAAC,CAAC;KACP;;IAGD,mBAAI,GAAJ,UAAK,KAAyC;QAC5C,OAAO,IAAI,CAAC,OAAO,CAAC,KAAK,CAAC,CAAC;KAC5B;;;;;IAMD,qBAAM,GAAN,UAAO,OAA2C;QAChD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;QAC7D,IAAI,OAAO,CAAC,MAAM,EAAE;YAAE,MAAM,KAAK,CAAC,kBAAkB,CAAC,CAAC;;QAGtD,IAAI,IAAI,EAAE;;;;YAIR,IACE,CAAC,IAAI,CAAC,QAAQ;gBACd,IAAI,CAAC,IAAI,KAAK,CAAC,UAAU;gBACzB,OAAO,CAAC,GAAG,KAAK,CAAC,CAAC;gBAClB,OAAO,CAAC,IAAI,KAAK,CAAC,CAAC,EACnB;;gBAEA,OAAO,IAAI,CAAC;aACb;YACD,IAAM,GAAG,GAAG,CAAC,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,KAAK,EAClD,IAAI,CAAC,GAAG,EACR,IAAI,CAAC,IAAI,EACT,OAAO,CAAC,GAAG,EACX,OAAO,CAAC,IAAI,CACb,CAAC;YACF,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC3D;QAED,IAAI,IAAI,CAAC,MAAM,EAAE;YAAE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;QACjE,IAAI,MAAM,EAAE,GAAG,EAAE,GAAG,CAAC;QACrB,IAAI,CAAC,IAAI,CAAC,QAAQ,EAAE;;;YAGlB,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;gBAC3B,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,GAAG,CAAC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,OAAO,CAAC;oBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;;qBAEvE,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;oBAAE,OAAO,IAAI,CAAC,GAAG,CAAC;qBAChD;;oBAEH,IAAM,QAAQ,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;oBAC7B,MAAM,GAAG,QAAQ,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;oBACtC,IAAI,MAAM,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,EAAE;wBACxB,OAAO,OAAO,CAAC,UAAU,EAAE,GAAG,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC,OAAO,CAAC;qBACvD;yBAAM;wBACL,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,MAAM,CAAC,CAAC,CAAC;wBACpC,GAAG,GAAG,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,CAAC;wBACnC,OAAO,GAAG,CAAC;qBACZ;iBACF;aACF;iBAAM,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;gBAAE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,IAAI,CAAC;YACrF,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;gBACrB,IAAI,OAAO,CAAC,UAAU,EAAE;oBAAE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC;gBAC/D,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,EAAE,CAAC;aACtC;iBAAM,IAAI,OAAO,CAAC,UAAU,EAAE;gBAAE,OAAO,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC;YACtE,GAAG,GAAG,IAAI,CAAC,IAAI,CAAC;SACjB;aAAM;;;YAGL,IAAI,CAAC,OAAO,CAAC,QAAQ;gBAAE,OAAO,GAAG,OAAO,CAAC,UAAU,EAAE,CAAC;YACtD,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC;gBAAE,OAAO,IAAI,CAAC,KAAK,CAAC;YACxC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;;gBAE1B,OAAO,IAAI,CAAC,IAAI,CAAC;YACnB,GAAG,GAAG,IAAI,CAAC,KAAK,CAAC;SAClB;;;;;;QAOD,GAAG,GAAG,IAAI,CAAC;QACX,OAAO,GAAG,CAAC,GAAG,CAAC,OAAO,CAAC,EAAE;;;YAGvB,MAAM,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,QAAQ,EAAE,GAAG,OAAO,CAAC,QAAQ,EAAE,CAAC,CAAC,CAAC;;;YAItE,IAAM,IAAI,GAAG,IAAI,CAAC,IAAI,CAAC,IAAI,CAAC,GAAG,CAAC,MAAM,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC;YACpD,IAAM,KAAK,GAAG,IAAI,IAAI,EAAE,GAAG,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,GAAG,EAAE,CAAC,CAAC;;;YAGtD,IAAI,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,MAAM,CAAC,CAAC;YACxC,IAAI,SAAS,GAAG,SAAS,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;YACvC,OAAO,SAAS,CAAC,UAAU,EAAE,IAAI,SAAS,CAAC,EAAE,CAAC,GAAG,CAAC,EAAE;gBAClD,MAAM,IAAI,KAAK,CAAC;gBAChB,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,MAAM,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;gBACnD,SAAS,GAAG,SAAS,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;aACpC;;;YAID,IAAI,SAAS,CAAC,MAAM,EAAE;gBAAE,SAAS,GAAG,IAAI,CAAC,GAAG,CAAC;YAE7C,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;YACzB,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;SAC1B;QACD,OAAO,GAAG,CAAC;KACZ;;IAGD,kBAAG,GAAH,UAAI,OAA2C;QAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;KAC7B;;;;;IAMD,qBAAM,GAAN,UAAO,KAAyC;QAC9C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,IAAI,IAAI,CAAC,QAAQ,KAAK,KAAK,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,KAAK,EAAE,KAAK,CAAC,IAAI,KAAK,CAAC,IAAI,KAAK,EAAE,KAAK,CAAC;YACvF,OAAO,KAAK,CAAC;QACf,OAAO,IAAI,CAAC,IAAI,KAAK,KAAK,CAAC,IAAI,IAAI,IAAI,CAAC,GAAG,KAAK,KAAK,CAAC,GAAG,CAAC;KAC3D;;IAGD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC;KAC3B;;IAGD,0BAAW,GAAX;QACE,OAAO,IAAI,CAAC,IAAI,CAAC;KAClB;;IAGD,kCAAmB,GAAnB;QACE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC;KACxB;;IAGD,yBAAU,GAAV;QACE,OAAO,IAAI,CAAC,GAAG,CAAC;KACjB;;IAGD,iCAAkB,GAAlB;QACE,OAAO,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;KACvB;;IAGD,4BAAa,GAAb;QACE,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;;YAErB,OAAO,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,GAAG,EAAE,GAAG,IAAI,CAAC,GAAG,EAAE,CAAC,aAAa,EAAE,CAAC;SAClE;QACD,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC,GAAG,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC;QACnD,IAAI,GAAW,CAAC;QAChB,KAAK,GAAG,GAAG,EAAE,EAAE,GAAG,GAAG,CAAC,EAAE,GAAG,EAAE;YAAE,IAAI,CAAC,GAAG,IAAI,CAAC,IAAI,GAAG,CAAC,MAAM,CAAC;gBAAE,MAAM;QACnE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,GAAG,GAAG,GAAG,EAAE,GAAG,GAAG,GAAG,CAAC,CAAC;KAC7C;;IAGD,0BAAW,GAAX,UAAY,KAAyC;QACnD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;KAC7B;;IAGD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,WAAW,CAAC,KAAK,CAAC,CAAC;KAChC;;IAGD,iCAAkB,GAAlB,UAAmB,KAAyC;QAC1D,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;KAC9B;;IAGD,kBAAG,GAAH,UAAI,KAAyC;QAC3C,OAAO,IAAI,CAAC,kBAAkB,CAAC,KAAK,CAAC,CAAC;KACvC;;IAED,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,kBAAkB,CAAC,KAAK,CAAC,CAAC;KACvC;;IAGD,qBAAM,GAAN;QACE,OAAO,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC,MAAM,CAAC,CAAC;KAC7B;;IAGD,yBAAU,GAAV;QACE,OAAO,CAAC,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,GAAG,CAAC,CAAC;KACxC;;IAGD,oBAAK,GAAL;QACE,OAAO,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC,MAAM,CAAC,CAAC;KAC7B;;IAGD,yBAAU,GAAV;QACE,OAAO,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC,CAAC;KACxC;;IAGD,qBAAM,GAAN;QACE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;KAC1C;;IAGD,uBAAQ,GAAR,UAAS,KAAyC;QAChD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;KAC7B;;IAGD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;KAC7B;;IAGD,8BAAe,GAAf,UAAgB,KAAyC;QACvD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;KAC9B;;IAGD,kBAAG,GAAH,UAAI,KAAyC;QAC3C,OAAO,IAAI,CAAC,eAAe,CAAC,KAAK,CAAC,CAAC;KACpC;;IAGD,qBAAM,GAAN,UAAO,OAA2C;QAChD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;;QAG7D,IAAI,IAAI,EAAE;YACR,IAAM,GAAG,GAAG,CAAC,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,KAAK,GAAG,IAAI,CAAC,KAAK,EAClD,IAAI,CAAC,GAAG,EACR,IAAI,CAAC,IAAI,EACT,OAAO,CAAC,GAAG,EACX,OAAO,CAAC,IAAI,CACb,CAAC;YACF,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC3D;QAED,OAAO,IAAI,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,CAAC;KACjD;;IAGD,kBAAG,GAAH,UAAI,OAA2C;QAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;KAC7B;;IAED,kBAAG,GAAH,UAAI,OAA2C;QAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;KAC7B;;;;;;IAOD,uBAAQ,GAAR,UAAS,UAA8C;QACrD,IAAI,IAAI,CAAC,MAAM,EAAE;YAAE,OAAO,IAAI,CAAC,IAAI,CAAC;QACpC,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;YAAE,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;;QAGtE,IAAI,IAAI,EAAE;YACR,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,UAAU,CAAC,GAAG,EAAE,UAAU,CAAC,IAAI,CAAC,CAAC;YAC3E,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC3D;QAED,IAAI,UAAU,CAAC,MAAM,EAAE;YAAE,OAAO,IAAI,CAAC,IAAI,CAAC;QAC1C,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;YAAE,OAAO,UAAU,CAAC,KAAK,EAAE,GAAG,IAAI,CAAC,SAAS,GAAG,IAAI,CAAC,IAAI,CAAC;QACpF,IAAI,UAAU,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;YAAE,OAAO,IAAI,CAAC,KAAK,EAAE,GAAG,IAAI,CAAC,SAAS,GAAG,IAAI,CAAC,IAAI,CAAC;QAEpF,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;YACrB,IAAI,UAAU,CAAC,UAAU,EAAE;gBAAE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC;;gBAChE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC,GAAG,EAAE,CAAC;SAC9C;aAAM,IAAI,UAAU,CAAC,UAAU,EAAE;YAAE,OAAO,IAAI,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC;;QAG5E,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,UAAU,CAAC,IAAI,UAAU,CAAC,EAAE,CAAC,IAAI,CAAC,UAAU,CAAC;YAC5D,OAAO,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,QAAQ,EAAE,GAAG,UAAU,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;;QAKjF,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,EAAE,CAAC;QAC7B,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,GAAG,MAAM,CAAC;QAC/B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC;QAC5B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,GAAG,MAAM,CAAC;QAE9B,IAAM,GAAG,GAAG,UAAU,CAAC,IAAI,KAAK,EAAE,CAAC;QACnC,IAAM,GAAG,GAAG,UAAU,CAAC,IAAI,GAAG,MAAM,CAAC;QACrC,IAAM,GAAG,GAAG,UAAU,CAAC,GAAG,KAAK,EAAE,CAAC;QAClC,IAAM,GAAG,GAAG,UAAU,CAAC,GAAG,GAAG,MAAM,CAAC;QAEpC,IAAI,GAAG,GAAG,CAAC,EACT,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,CAAC;QACV,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,CAAC;QACrD,GAAG,IAAI,MAAM,CAAC;QACd,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,CAAC,GAAG,IAAI,EAAE,IAAI,GAAG,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KAC3E;;IAGD,kBAAG,GAAH,UAAI,UAA8C;QAChD,OAAO,IAAI,CAAC,QAAQ,CAAC,UAAU,CAAC,CAAC;KAClC;;IAGD,qBAAM,GAAN;QACE,IAAI,CAAC,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;YAAE,OAAO,IAAI,CAAC,SAAS,CAAC;QACrE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;KACjC;;IAGD,kBAAG,GAAH;QACE,OAAO,IAAI,CAAC,MAAM,EAAE,CAAC;KACtB;;IAGD,kBAAG,GAAH;QACE,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KAC5D;;IAGD,wBAAS,GAAT,UAAU,KAAyC;QACjD,OAAO,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC;KAC5B;;IAGD,kBAAG,GAAH,UAAI,KAAyC;QAC3C,OAAO,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;KAC9B;;IAED,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;KAC9B;;;;IAKD,iBAAE,GAAF,UAAG,KAA6B;QAC9B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KACnF;;;;;;IAOD,wBAAS,GAAT,UAAU,OAAsB;QAC9B,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;QACpD,IAAI,CAAC,OAAO,IAAI,EAAE,MAAM,CAAC;YAAE,OAAO,IAAI,CAAC;aAClC,IAAI,OAAO,GAAG,EAAE;YACnB,OAAO,IAAI,CAAC,QAAQ,CAClB,IAAI,CAAC,GAAG,IAAI,OAAO,EACnB,CAAC,IAAI,CAAC,IAAI,IAAI,OAAO,KAAK,IAAI,CAAC,GAAG,MAAM,EAAE,GAAG,OAAO,CAAC,CAAC,EACtD,IAAI,CAAC,QAAQ,CACd,CAAC;;YACC,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,EAAE,IAAI,CAAC,GAAG,KAAK,OAAO,GAAG,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KACzE;;IAGD,kBAAG,GAAH,UAAI,OAAsB;QACxB,OAAO,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;KAChC;;;;;;IAOD,yBAAU,GAAV,UAAW,OAAsB;QAC/B,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;QACpD,IAAI,CAAC,OAAO,IAAI,EAAE,MAAM,CAAC;YAAE,OAAO,IAAI,CAAC;aAClC,IAAI,OAAO,GAAG,EAAE;YACnB,OAAO,IAAI,CAAC,QAAQ,CAClB,CAAC,IAAI,CAAC,GAAG,KAAK,OAAO,KAAK,IAAI,CAAC,IAAI,KAAK,EAAE,GAAG,OAAO,CAAC,CAAC,EACtD,IAAI,CAAC,IAAI,IAAI,OAAO,EACpB,IAAI,CAAC,QAAQ,CACd,CAAC;;YACC,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,IAAI,KAAK,OAAO,GAAG,EAAE,CAAC,EAAE,IAAI,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KAChG;;IAGD,kBAAG,GAAH,UAAI,OAAsB;QACxB,OAAO,IAAI,CAAC,UAAU,CAAC,OAAO,CAAC,CAAC;KACjC;;;;;;IAOD,iCAAkB,GAAlB,UAAmB,OAAsB;QACvC,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;QACpD,OAAO,IAAI,EAAE,CAAC;QACd,IAAI,OAAO,KAAK,CAAC;YAAE,OAAO,IAAI,CAAC;aAC1B;YACH,IAAM,IAAI,GAAG,IAAI,CAAC,IAAI,CAAC;YACvB,IAAI,OAAO,GAAG,EAAE,EAAE;gBAChB,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC;gBACrB,OAAO,IAAI,CAAC,QAAQ,CAClB,CAAC,GAAG,KAAK,OAAO,KAAK,IAAI,KAAK,EAAE,GAAG,OAAO,CAAC,CAAC,EAC5C,IAAI,KAAK,OAAO,EAChB,IAAI,CAAC,QAAQ,CACd,CAAC;aACH;iBAAM,IAAI,OAAO,KAAK,EAAE;gBAAE,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;gBACnE,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,MAAM,OAAO,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SACtE;KACF;;IAGD,oBAAK,GAAL,UAAM,OAAsB;QAC1B,OAAO,IAAI,CAAC,kBAAkB,CAAC,OAAO,CAAC,CAAC;KACzC;;IAED,mBAAI,GAAJ,UAAK,OAAsB;QACzB,OAAO,IAAI,CAAC,kBAAkB,CAAC,OAAO,CAAC,CAAC;KACzC;;;;;;IAOD,uBAAQ,GAAR,UAAS,UAA8C;QACrD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;YAAE,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;QACtE,OAAO,IAAI,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC;KACnC;;IAGD,kBAAG,GAAH,UAAI,UAA8C;QAChD,OAAO,IAAI,CAAC,QAAQ,CAAC,UAAU,CAAC,CAAC;KAClC;;IAGD,oBAAK,GAAL;QACE,OAAO,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC;KAClD;;IAGD,uBAAQ,GAAR;QACE,IAAI,IAAI,CAAC,QAAQ;YAAE,OAAO,CAAC,IAAI,CAAC,IAAI,KAAK,CAAC,IAAI,cAAc,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC;QAChF,OAAO,IAAI,CAAC,IAAI,GAAG,cAAc,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC;KACtD;;IAGD,uBAAQ,GAAR;QACE,OAAO,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,CAAC;KAChC;;;;;;IAOD,sBAAO,GAAP,UAAQ,EAAY;QAClB,OAAO,EAAE,GAAG,IAAI,CAAC,SAAS,EAAE,GAAG,IAAI,CAAC,SAAS,EAAE,CAAC;KACjD;;;;;IAMD,wBAAS,GAAT;QACE,IAAM,EAAE,GAAG,IAAI,CAAC,IAAI,EAClB,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO;YACL,EAAE,GAAG,IAAI;YACT,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;YACjB,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;YAClB,EAAE,KAAK,EAAE;YACT,EAAE,GAAG,IAAI;YACT,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;YACjB,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;YAClB,EAAE,KAAK,EAAE;SACV,CAAC;KACH;;;;;IAMD,wBAAS,GAAT;QACE,IAAM,EAAE,GAAG,IAAI,CAAC,IAAI,EAClB,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO;YACL,EAAE,KAAK,EAAE;YACT,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;YAClB,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;YACjB,EAAE,GAAG,IAAI;YACT,EAAE,KAAK,EAAE;YACT,CAAC,EAAE,KAAK,EAAE,IAAI,IAAI;YAClB,CAAC,EAAE,KAAK,CAAC,IAAI,IAAI;YACjB,EAAE,GAAG,IAAI;SACV,CAAC;KACH;;;;IAKD,uBAAQ,GAAR;QACE,IAAI,CAAC,IAAI,CAAC,QAAQ;YAAE,OAAO,IAAI,CAAC;QAChC,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;KAClD;;;;;;IAOD,uBAAQ,GAAR,UAAS,KAAc;QACrB,KAAK,GAAG,KAAK,IAAI,EAAE,CAAC;QACpB,IAAI,KAAK,GAAG,CAAC,IAAI,EAAE,GAAG,KAAK;YAAE,MAAM,UAAU,CAAC,OAAO,CAAC,CAAC;QACvD,IAAI,IAAI,CAAC,MAAM,EAAE;YAAE,OAAO,GAAG,CAAC;QAC9B,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;;YAErB,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;;;gBAG3B,IAAM,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,EACtC,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,SAAS,CAAC,EACzB,IAAI,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;gBACtC,OAAO,GAAG,CAAC,QAAQ,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC,KAAK,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aAC3D;;gBAAM,OAAO,GAAG,GAAG,IAAI,CAAC,GAAG,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;SAChD;;;QAID,IAAM,YAAY,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;QAExE,IAAI,GAAG,GAAS,IAAI,CAAC;QACrB,IAAI,MAAM,GAAG,EAAE,CAAC;;QAEhB,OAAO,IAAI,EAAE;YACX,IAAM,MAAM,GAAG,GAAG,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC;YACrC,IAAM,MAAM,GAAG,GAAG,CAAC,GAAG,CAAC,MAAM,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC,CAAC,KAAK,EAAE,KAAK,CAAC,CAAC;YAC/D,IAAI,MAAM,GAAG,MAAM,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;YACpC,GAAG,GAAG,MAAM,CAAC;YACb,IAAI,GAAG,CAAC,MAAM,EAAE,EAAE;gBAChB,OAAO,MAAM,GAAG,MAAM,CAAC;aACxB;iBAAM;gBACL,OAAO,MAAM,CAAC,MAAM,GAAG,CAAC;oBAAE,MAAM,GAAG,GAAG,GAAG,MAAM,CAAC;gBAChD,MAAM,GAAG,EAAE,GAAG,MAAM,GAAG,MAAM,CAAC;aAC/B;SACF;KACF;;IAGD,yBAAU,GAAV;QACE,IAAI,IAAI,CAAC,QAAQ;YAAE,OAAO,IAAI,CAAC;QAC/B,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;KACjD;;IAGD,kBAAG,GAAH,UAAI,KAA6B;QAC/B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;KACnF;;IAGD,kBAAG,GAAH;QACE,OAAO,IAAI,CAAC,MAAM,EAAE,CAAC;KACtB;;IAGD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,eAAe,CAAC,KAAK,CAAC,CAAC;KACpC;;;;;;IAOD,6BAAc,GAAd,UAAe,OAAsB;QACnC,IAAI,OAAO,IAAI,OAAO,CAAC,OAAO;YAAE,OAAO,IAAI,CAAC,QAAQ,EAAE,CAAC;QACvD,OAAO,EAAE,WAAW,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;KACzC;IACM,qBAAgB,GAAvB,UAAwB,GAA4B,EAAE,OAAsB;QAC1E,IAAM,MAAM,GAAG,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,WAAW,CAAC,CAAC;QAChD,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,MAAM,CAAC,QAAQ,EAAE,GAAG,MAAM,CAAC;KAChE;;IAGD,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,sBAAO,GAAP;QACE,OAAO,gBAAa,IAAI,CAAC,QAAQ,EAAE,WAAI,IAAI,CAAC,QAAQ,GAAG,QAAQ,GAAG,EAAE,OAAG,CAAC;KACzE;IA/2BM,eAAU,GAAG,IAAI,CAAC,OAAO,CAAC,cAAc,CAAC,CAAC;;IAG1C,uBAAkB,GAAG,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,IAAI,CAAC,CAAC;;IAEzE,SAAI,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;;IAEvB,UAAK,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;;IAE9B,QAAG,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;;IAEtB,SAAI,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;;IAE7B,YAAO,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC,CAAC;;IAE3B,cAAS,GAAG,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;;IAEjE,cAAS,GAAG,IAAI,CAAC,QAAQ,CAAC,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;IA+1B7D,WAAC;CAv6BD,IAu6BC;AAv6BY,oBAAI;AAy6BjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,YAAY,EAAE,EAAE,KAAK,EAAE,IAAI,EAAE,CAAC,CAAC;AACrE,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC;;;;;;;;;;ACr/BtC;AACF;AAE9B,IAAM,mBAAmB,GAAG,+CAA+C,CAAC;AAC5E,IAAM,gBAAgB,GAAG,0BAA0B,CAAC;AACpD,IAAM,gBAAgB,GAAG,eAAe,CAAC;AAEzC,IAAM,YAAY,GAAG,IAAI,CAAC;AAC1B,IAAM,YAAY,GAAG,CAAC,IAAI,CAAC;AAC3B,IAAM,aAAa,GAAG,IAAI,CAAC;AAC3B,IAAM,UAAU,GAAG,EAAE,CAAC;AAEtB;AACA,IAAM,UAAU,GAAG;IACjB,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;CACL,CAAC,OAAO,EAAE,CAAC;AACZ;AACA,IAAM,mBAAmB,GAAG;IAC1B,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;CACL,CAAC,OAAO,EAAE,CAAC;AACZ,IAAM,mBAAmB,GAAG;IAC1B,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;CACL,CAAC,OAAO,EAAE,CAAC;AAEZ,IAAM,cAAc,GAAG,iBAAiB,CAAC;AAEzC;AACA,IAAM,gBAAgB,GAAG,IAAI,CAAC;AAC9B;AACA,IAAM,aAAa,GAAG,MAAM,CAAC;AAC7B;AACA,IAAM,oBAAoB,GAAG,EAAE,CAAC;AAChC;AACA,IAAM,eAAe,GAAG,EAAE,CAAC;AAE3B;AACA,SAAS,OAAO,CAAC,KAAa;IAC5B,OAAO,CAAC,KAAK,CAAC,QAAQ,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,CAAC;AACrC,CAAC;AAED;AACA,SAAS,UAAU,CAAC,KAAkD;IACpE,IAAM,OAAO,GAAG,WAAI,CAAC,UAAU,CAAC,IAAI,GAAG,IAAI,GAAG,IAAI,CAAC,CAAC;IACpD,IAAI,IAAI,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;IAE9B,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE;QAC5E,OAAO,EAAE,QAAQ,EAAE,KAAK,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;KACvC;IAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;;QAE3B,IAAI,GAAG,IAAI,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC;;QAE1B,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC,IAAI,WAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;QAC7C,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC;QACvC,IAAI,GAAG,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;KAC7B;IAED,OAAO,EAAE,QAAQ,EAAE,KAAK,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;AACxC,CAAC;AAED;AACA,SAAS,YAAY,CAAC,IAAU,EAAE,KAAW;IAC3C,IAAI,CAAC,IAAI,IAAI,CAAC,KAAK,EAAE;QACnB,OAAO,EAAE,IAAI,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,GAAG,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,CAAC;KAC9D;IAED,IAAM,QAAQ,GAAG,IAAI,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC;IAC7C,IAAM,OAAO,GAAG,IAAI,WAAI,CAAC,IAAI,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC;IAC/C,IAAM,SAAS,GAAG,KAAK,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC;IAC/C,IAAM,QAAQ,GAAG,IAAI,WAAI,CAAC,KAAK,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC;IAEjD,IAAI,WAAW,GAAG,QAAQ,CAAC,QAAQ,CAAC,SAAS,CAAC,CAAC;IAC/C,IAAI,UAAU,GAAG,QAAQ,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;IAC7C,IAAM,WAAW,GAAG,OAAO,CAAC,QAAQ,CAAC,SAAS,CAAC,CAAC;IAChD,IAAI,UAAU,GAAG,OAAO,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;IAE5C,WAAW,GAAG,WAAW,CAAC,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;IACjE,UAAU,GAAG,IAAI,WAAI,CAAC,UAAU,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC;SAC9C,GAAG,CAAC,WAAW,CAAC;SAChB,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;IAE1C,WAAW,GAAG,WAAW,CAAC,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;IACjE,UAAU,GAAG,UAAU,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,GAAG,CAAC,IAAI,WAAI,CAAC,UAAU,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC,CAAC;;IAGhF,OAAO,EAAE,IAAI,EAAE,WAAW,EAAE,GAAG,EAAE,UAAU,EAAE,CAAC;AAChD,CAAC;AAED,SAAS,QAAQ,CAAC,IAAU,EAAE,KAAW;;IAEvC,IAAM,MAAM,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC;IAC/B,IAAM,OAAO,GAAG,KAAK,CAAC,IAAI,KAAK,CAAC,CAAC;;IAGjC,IAAI,MAAM,GAAG,OAAO,EAAE;QACpB,OAAO,IAAI,CAAC;KACb;SAAM,IAAI,MAAM,KAAK,OAAO,EAAE;QAC7B,IAAM,MAAM,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;QAC9B,IAAM,OAAO,GAAG,KAAK,CAAC,GAAG,KAAK,CAAC,CAAC;QAChC,IAAI,MAAM,GAAG,OAAO;YAAE,OAAO,IAAI,CAAC;KACnC;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,UAAU,CAAC,MAAc,EAAE,OAAe;IACjD,MAAM,IAAI,SAAS,CAAC,OAAI,MAAM,8CAAwC,OAAS,CAAC,CAAC;AACnF,CAAC;AAOD;;;;AAIA;;;;;IASE,oBAAY,KAAsB;QAChC,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;YAAE,OAAO,IAAI,UAAU,CAAC,KAAK,CAAC,CAAC;QAEhE,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YAC7B,IAAI,CAAC,KAAK,GAAG,UAAU,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,KAAK,CAAC;SACjD;aAAM;YACL,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;SACpB;KACF;;;;;;IAOM,qBAAU,GAAjB,UAAkB,cAAsB;;QAEtC,IAAI,UAAU,GAAG,KAAK,CAAC;QACvB,IAAI,QAAQ,GAAG,KAAK,CAAC;QACrB,IAAI,YAAY,GAAG,KAAK,CAAC;;QAGzB,IAAI,iBAAiB,GAAG,CAAC,CAAC;;QAE1B,IAAI,WAAW,GAAG,CAAC,CAAC;;QAEpB,IAAI,OAAO,GAAG,CAAC,CAAC;;QAEhB,IAAI,aAAa,GAAG,CAAC,CAAC;;QAEtB,IAAI,YAAY,GAAG,CAAC,CAAC;;QAGrB,IAAM,MAAM,GAAG,CAAC,CAAC,CAAC,CAAC;;QAEnB,IAAI,aAAa,GAAG,CAAC,CAAC;;QAEtB,IAAI,YAAY,GAAG,CAAC,CAAC;;QAErB,IAAI,UAAU,GAAG,CAAC,CAAC;;QAEnB,IAAI,SAAS,GAAG,CAAC,CAAC;;QAGlB,IAAI,QAAQ,GAAG,CAAC,CAAC;;QAEjB,IAAI,CAAC,GAAG,CAAC,CAAC;;QAEV,IAAI,eAAe,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;;QAErC,IAAI,cAAc,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;;QAEpC,IAAI,cAAc,GAAG,CAAC,CAAC;;QAGvB,IAAI,KAAK,GAAG,CAAC,CAAC;;;;QAKd,IAAI,cAAc,CAAC,MAAM,IAAI,IAAI,EAAE;YACjC,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;SAC7E;;QAGD,IAAM,WAAW,GAAG,cAAc,CAAC,KAAK,CAAC,mBAAmB,CAAC,CAAC;QAC9D,IAAM,QAAQ,GAAG,cAAc,CAAC,KAAK,CAAC,gBAAgB,CAAC,CAAC;QACxD,IAAM,QAAQ,GAAG,cAAc,CAAC,KAAK,CAAC,gBAAgB,CAAC,CAAC;;QAGxD,IAAI,CAAC,CAAC,WAAW,IAAI,CAAC,QAAQ,IAAI,CAAC,QAAQ,KAAK,cAAc,CAAC,MAAM,KAAK,CAAC,EAAE;YAC3E,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;SAC7E;QAED,IAAI,WAAW,EAAE;;;YAIf,IAAM,cAAc,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;;;YAItC,IAAM,CAAC,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;YACzB,IAAM,OAAO,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;YAC/B,IAAM,SAAS,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;;YAGjC,IAAI,CAAC,IAAI,SAAS,KAAK,SAAS;gBAAE,UAAU,CAAC,cAAc,EAAE,wBAAwB,CAAC,CAAC;;YAGvF,IAAI,CAAC,IAAI,cAAc,KAAK,SAAS;gBAAE,UAAU,CAAC,cAAc,EAAE,uBAAuB,CAAC,CAAC;YAE3F,IAAI,CAAC,KAAK,SAAS,KAAK,OAAO,IAAI,SAAS,CAAC,EAAE;gBAC7C,UAAU,CAAC,cAAc,EAAE,2BAA2B,CAAC,CAAC;aACzD;SACF;;QAGD,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;YAClE,UAAU,GAAG,cAAc,CAAC,KAAK,EAAE,CAAC,KAAK,GAAG,CAAC;SAC9C;;QAGD,IAAI,CAAC,OAAO,CAAC,cAAc,CAAC,KAAK,CAAC,CAAC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;YACpE,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;gBAClE,OAAO,IAAI,UAAU,CAACD,aAAM,CAAC,IAAI,CAAC,UAAU,GAAG,mBAAmB,GAAG,mBAAmB,CAAC,CAAC,CAAC;aAC5F;iBAAM,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;gBACxC,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;aAChD;SACF;;QAGD,OAAO,OAAO,CAAC,cAAc,CAAC,KAAK,CAAC,CAAC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;YACtE,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;gBACjC,IAAI,QAAQ;oBAAE,UAAU,CAAC,cAAc,EAAE,2BAA2B,CAAC,CAAC;gBAEtE,QAAQ,GAAG,IAAI,CAAC;gBAChB,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;gBAClB,SAAS;aACV;YAED,IAAI,aAAa,GAAG,EAAE,EAAE;gBACtB,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,YAAY,EAAE;oBACjD,IAAI,CAAC,YAAY,EAAE;wBACjB,YAAY,GAAG,WAAW,CAAC;qBAC5B;oBAED,YAAY,GAAG,IAAI,CAAC;;oBAGpB,MAAM,CAAC,YAAY,EAAE,CAAC,GAAG,QAAQ,CAAC,cAAc,CAAC,KAAK,CAAC,EAAE,EAAE,CAAC,CAAC;oBAC7D,aAAa,GAAG,aAAa,GAAG,CAAC,CAAC;iBACnC;aACF;YAED,IAAI,YAAY;gBAAE,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC;YACxC,IAAI,QAAQ;gBAAE,aAAa,GAAG,aAAa,GAAG,CAAC,CAAC;YAEhD,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;YAC9B,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;SACnB;QAED,IAAI,QAAQ,IAAI,CAAC,WAAW;YAC1B,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;;QAG9E,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;;YAElE,IAAM,KAAK,GAAG,cAAc,CAAC,MAAM,CAAC,EAAE,KAAK,CAAC,CAAC,KAAK,CAAC,cAAc,CAAC,CAAC;;YAGnE,IAAI,CAAC,KAAK,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC;gBAAE,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;;YAGxE,QAAQ,GAAG,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;;YAGlC,KAAK,GAAG,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC;SACjC;;QAGD,IAAI,cAAc,CAAC,KAAK,CAAC;YAAE,OAAO,IAAI,UAAU,CAACA,aAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;;;QAI1E,UAAU,GAAG,CAAC,CAAC;QAEf,IAAI,CAAC,aAAa,EAAE;YAClB,UAAU,GAAG,CAAC,CAAC;YACf,SAAS,GAAG,CAAC,CAAC;YACd,MAAM,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;YACd,OAAO,GAAG,CAAC,CAAC;YACZ,aAAa,GAAG,CAAC,CAAC;YAClB,iBAAiB,GAAG,CAAC,CAAC;SACvB;aAAM;YACL,SAAS,GAAG,aAAa,GAAG,CAAC,CAAC;YAC9B,iBAAiB,GAAG,OAAO,CAAC;YAC5B,IAAI,iBAAiB,KAAK,CAAC,EAAE;gBAC3B,OAAO,cAAc,CAAC,YAAY,GAAG,iBAAiB,GAAG,CAAC,CAAC,KAAK,GAAG,EAAE;oBACnE,iBAAiB,GAAG,iBAAiB,GAAG,CAAC,CAAC;iBAC3C;aACF;SACF;;;;;QAOD,IAAI,QAAQ,IAAI,aAAa,IAAI,aAAa,GAAG,QAAQ,GAAG,CAAC,IAAI,EAAE,EAAE;YACnE,QAAQ,GAAG,YAAY,CAAC;SACzB;aAAM;YACL,QAAQ,GAAG,QAAQ,GAAG,aAAa,CAAC;SACrC;;QAGD,OAAO,QAAQ,GAAG,YAAY,EAAE;;YAE9B,SAAS,GAAG,SAAS,GAAG,CAAC,CAAC;YAE1B,IAAI,SAAS,GAAG,UAAU,GAAG,UAAU,EAAE;;gBAEvC,IAAM,YAAY,GAAG,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;gBACrC,IAAI,YAAY,CAAC,KAAK,CAAC,MAAM,CAAC,EAAE;oBAC9B,QAAQ,GAAG,YAAY,CAAC;oBACxB,MAAM;iBACP;gBAED,UAAU,CAAC,cAAc,EAAE,UAAU,CAAC,CAAC;aACxC;YACD,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;SACzB;QAED,OAAO,QAAQ,GAAG,YAAY,IAAI,aAAa,GAAG,OAAO,EAAE;;YAEzD,IAAI,SAAS,KAAK,CAAC,IAAI,iBAAiB,GAAG,aAAa,EAAE;gBACxD,QAAQ,GAAG,YAAY,CAAC;gBACxB,iBAAiB,GAAG,CAAC,CAAC;gBACtB,MAAM;aACP;YAED,IAAI,aAAa,GAAG,OAAO,EAAE;;gBAE3B,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC;aACvB;iBAAM;;gBAEL,SAAS,GAAG,SAAS,GAAG,CAAC,CAAC;aAC3B;YAED,IAAI,QAAQ,GAAG,YAAY,EAAE;gBAC3B,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;aACzB;iBAAM;;gBAEL,IAAM,YAAY,GAAG,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;gBACrC,IAAI,YAAY,CAAC,KAAK,CAAC,MAAM,CAAC,EAAE;oBAC9B,QAAQ,GAAG,YAAY,CAAC;oBACxB,MAAM;iBACP;gBACD,UAAU,CAAC,cAAc,EAAE,UAAU,CAAC,CAAC;aACxC;SACF;;;QAID,IAAI,SAAS,GAAG,UAAU,GAAG,CAAC,GAAG,iBAAiB,EAAE;YAClD,IAAI,WAAW,GAAG,WAAW,CAAC;;;;YAK9B,IAAI,QAAQ,EAAE;gBACZ,YAAY,GAAG,YAAY,GAAG,CAAC,CAAC;gBAChC,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;aAC/B;;YAED,IAAI,UAAU,EAAE;gBACd,YAAY,GAAG,YAAY,GAAG,CAAC,CAAC;gBAChC,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;aAC/B;YAED,IAAM,UAAU,GAAG,QAAQ,CAAC,cAAc,CAAC,YAAY,GAAG,SAAS,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;YAC9E,IAAI,QAAQ,GAAG,CAAC,CAAC;YAEjB,IAAI,UAAU,IAAI,CAAC,EAAE;gBACnB,QAAQ,GAAG,CAAC,CAAC;gBACb,IAAI,UAAU,KAAK,CAAC,EAAE;oBACpB,QAAQ,GAAG,MAAM,CAAC,SAAS,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;oBAC/C,KAAK,CAAC,GAAG,YAAY,GAAG,SAAS,GAAG,CAAC,EAAE,CAAC,GAAG,WAAW,EAAE,CAAC,EAAE,EAAE;wBAC3D,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE;4BACnC,QAAQ,GAAG,CAAC,CAAC;4BACb,MAAM;yBACP;qBACF;iBACF;aACF;YAED,IAAI,QAAQ,EAAE;gBACZ,IAAI,IAAI,GAAG,SAAS,CAAC;gBAErB,OAAO,IAAI,IAAI,CAAC,EAAE,IAAI,EAAE,EAAE;oBACxB,IAAI,EAAE,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,EAAE;wBACtB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;wBAGjB,IAAI,IAAI,KAAK,CAAC,EAAE;4BACd,IAAI,QAAQ,GAAG,YAAY,EAAE;gCAC3B,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;gCACxB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;6BAClB;iCAAM;gCACL,OAAO,IAAI,UAAU,CACnBA,aAAM,CAAC,IAAI,CAAC,UAAU,GAAG,mBAAmB,GAAG,mBAAmB,CAAC,CACpE,CAAC;6BACH;yBACF;qBACF;iBACF;aACF;SACF;;;QAID,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;;QAErC,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;;QAGpC,IAAI,iBAAiB,KAAK,CAAC,EAAE;YAC3B,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;YACrC,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;SACrC;aAAM,IAAI,SAAS,GAAG,UAAU,GAAG,EAAE,EAAE;YACtC,IAAI,IAAI,GAAG,UAAU,CAAC;YACtB,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;YACjD,eAAe,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;YAEjC,OAAO,IAAI,IAAI,SAAS,EAAE,IAAI,EAAE,EAAE;gBAChC,cAAc,GAAG,cAAc,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;gBAC9D,cAAc,GAAG,cAAc,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;aACpE;SACF;aAAM;YACL,IAAI,IAAI,GAAG,UAAU,CAAC;YACtB,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;YAElD,OAAO,IAAI,IAAI,SAAS,GAAG,EAAE,EAAE,IAAI,EAAE,EAAE;gBACrC,eAAe,GAAG,eAAe,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;gBAChE,eAAe,GAAG,eAAe,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;aACtE;YAED,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;YAEjD,OAAO,IAAI,IAAI,SAAS,EAAE,IAAI,EAAE,EAAE;gBAChC,cAAc,GAAG,cAAc,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;gBAC9D,cAAc,GAAG,cAAc,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;aACpE;SACF;QAED,IAAM,WAAW,GAAG,YAAY,CAAC,eAAe,EAAE,WAAI,CAAC,UAAU,CAAC,oBAAoB,CAAC,CAAC,CAAC;QACzF,WAAW,CAAC,GAAG,GAAG,WAAW,CAAC,GAAG,CAAC,GAAG,CAAC,cAAc,CAAC,CAAC;QAEtD,IAAI,QAAQ,CAAC,WAAW,CAAC,GAAG,EAAE,cAAc,CAAC,EAAE;YAC7C,WAAW,CAAC,IAAI,GAAG,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC;SAC7D;;QAGD,cAAc,GAAG,QAAQ,GAAG,aAAa,CAAC;QAC1C,IAAM,GAAG,GAAG,EAAE,GAAG,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,IAAI,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,CAAC;;QAGlE,IACE,WAAW,CAAC,IAAI,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,EAC1F;;YAEA,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,GAAG,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAAC;YAC3D,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CACpB,WAAI,CAAC,UAAU,CAAC,cAAc,CAAC,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAC3E,CAAC;YACF,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,cAAc,CAAC,CAAC,CAAC,CAAC;SAC/E;aAAM;YACL,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,cAAc,GAAG,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAAC;YAC/E,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,eAAe,CAAC,CAAC,CAAC,CAAC;SAChF;QAED,GAAG,CAAC,GAAG,GAAG,WAAW,CAAC,GAAG,CAAC;;QAG1B,IAAI,UAAU,EAAE;YACd,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,qBAAqB,CAAC,CAAC,CAAC;SAChE;;QAGD,IAAMK,QAAM,GAAGL,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;QAChC,KAAK,GAAG,CAAC,CAAC;;;QAIVK,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,GAAG,CAAC,GAAG,GAAG,IAAI,CAAC;QACrCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;QAC5CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;QAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;;QAE7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,GAAG,CAAC,IAAI,GAAG,IAAI,CAAC;QACtCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;QAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;QAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;;QAI9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC;QACtCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;QAC7CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;QAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;;QAE9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC;QACvCA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;QAC9CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;QAC/CA,QAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;QAG/C,OAAO,IAAI,UAAU,CAACA,QAAM,CAAC,CAAC;KAC/B;;IAGD,6BAAQ,GAAR;;;;QAKE,IAAI,eAAe,CAAC;;QAEpB,IAAI,kBAAkB,GAAG,CAAC,CAAC;;QAE3B,IAAM,WAAW,GAAG,IAAI,KAAK,CAAS,EAAE,CAAC,CAAC;QAC1C,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,WAAW,CAAC,MAAM,EAAE,CAAC,EAAE;YAAE,WAAW,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;;QAEhE,IAAI,KAAK,GAAG,CAAC,CAAC;;QAGd,IAAI,OAAO,GAAG,KAAK,CAAC;;QAGpB,IAAI,eAAe,CAAC;;QAEpB,IAAI,cAAc,GAAgD,EAAE,KAAK,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,EAAE,CAAC;;QAE1F,IAAI,CAAC,EAAE,CAAC,CAAC;;QAGT,IAAM,MAAM,GAAa,EAAE,CAAC;;QAG5B,KAAK,GAAG,CAAC,CAAC;;QAGV,IAAM,MAAM,GAAG,IAAI,CAAC,KAAK,CAAC;;;QAI1B,IAAM,GAAG,GACP,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;QAE/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;;QAI/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;QAE/F,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAI,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;QAG/F,KAAK,GAAG,CAAC,CAAC;;QAGV,IAAM,GAAG,GAAG;YACV,GAAG,EAAE,IAAI,WAAI,CAAC,GAAG,EAAE,IAAI,CAAC;YACxB,IAAI,EAAE,IAAI,WAAI,CAAC,IAAI,EAAE,IAAI,CAAC;SAC3B,CAAC;QAEF,IAAI,GAAG,CAAC,IAAI,CAAC,QAAQ,CAAC,WAAI,CAAC,IAAI,CAAC,EAAE;YAChC,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;SAClB;;;QAID,IAAM,WAAW,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,gBAAgB,CAAC;QAEpD,IAAI,WAAW,IAAI,CAAC,KAAK,CAAC,EAAE;;YAE1B,IAAI,WAAW,KAAK,oBAAoB,EAAE;gBACxC,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,GAAG,UAAU,CAAC;aACrC;iBAAM,IAAI,WAAW,KAAK,eAAe,EAAE;gBAC1C,OAAO,KAAK,CAAC;aACd;iBAAM;gBACL,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,aAAa,CAAC;gBAC/C,eAAe,GAAG,IAAI,IAAI,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC,CAAC;aAChD;SACF;aAAM;YACL,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;YACtC,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,aAAa,CAAC;SAChD;;QAGD,IAAM,QAAQ,GAAG,eAAe,GAAG,aAAa,CAAC;;;;;QAOjD,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,IAAI,GAAG,MAAM,KAAK,CAAC,eAAe,GAAG,GAAG,KAAK,EAAE,CAAC,CAAC;QAC5E,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC;QAC/B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC;QAC/B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;QAE9B,IACE,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;YAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;YAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;YAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC,EAC7B;YACA,OAAO,GAAG,IAAI,CAAC;SAChB;aAAM;YACL,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;gBACvB,IAAI,YAAY,GAAG,CAAC,CAAC;;gBAErB,IAAM,MAAM,GAAG,UAAU,CAAC,cAAc,CAAC,CAAC;gBAC1C,cAAc,GAAG,MAAM,CAAC,QAAQ,CAAC;gBACjC,YAAY,GAAG,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;;;gBAI9B,IAAI,CAAC,YAAY;oBAAE,SAAS;gBAE5B,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;;oBAEvB,WAAW,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,YAAY,GAAG,EAAE,CAAC;;oBAE3C,YAAY,GAAG,IAAI,CAAC,KAAK,CAAC,YAAY,GAAG,EAAE,CAAC,CAAC;iBAC9C;aACF;SACF;;;;QAMD,IAAI,OAAO,EAAE;YACX,kBAAkB,GAAG,CAAC,CAAC;YACvB,WAAW,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;SACxB;aAAM;YACL,kBAAkB,GAAG,EAAE,CAAC;YACxB,OAAO,CAAC,WAAW,CAAC,KAAK,CAAC,EAAE;gBAC1B,kBAAkB,GAAG,kBAAkB,GAAG,CAAC,CAAC;gBAC5C,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;aACnB;SACF;;QAGD,IAAM,mBAAmB,GAAG,kBAAkB,GAAG,CAAC,GAAG,QAAQ,CAAC;;;;;;;;QAS9D,IAAI,mBAAmB,IAAI,EAAE,IAAI,mBAAmB,IAAI,CAAC,CAAC,IAAI,QAAQ,GAAG,CAAC,EAAE;;;;;YAM1E,IAAI,kBAAkB,GAAG,EAAE,EAAE;gBAC3B,MAAM,CAAC,IAAI,CAAC,KAAG,CAAG,CAAC,CAAC;gBACpB,IAAI,QAAQ,GAAG,CAAC;oBAAE,MAAM,CAAC,IAAI,CAAC,IAAI,GAAG,QAAQ,CAAC,CAAC;qBAC1C,IAAI,QAAQ,GAAG,CAAC;oBAAE,MAAM,CAAC,IAAI,CAAC,GAAG,GAAG,QAAQ,CAAC,CAAC;gBACnD,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;aACxB;YAED,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;YACvC,kBAAkB,GAAG,kBAAkB,GAAG,CAAC,CAAC;YAE5C,IAAI,kBAAkB,EAAE;gBACtB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;aAClB;YAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,EAAE,CAAC,EAAE,EAAE;gBAC3C,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;aACxC;;YAGD,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;YACjB,IAAI,mBAAmB,GAAG,CAAC,EAAE;gBAC3B,MAAM,CAAC,IAAI,CAAC,GAAG,GAAG,mBAAmB,CAAC,CAAC;aACxC;iBAAM;gBACL,MAAM,CAAC,IAAI,CAAC,KAAG,mBAAqB,CAAC,CAAC;aACvC;SACF;aAAM;;YAEL,IAAI,QAAQ,IAAI,CAAC,EAAE;gBACjB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,EAAE,CAAC,EAAE,EAAE;oBAC3C,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;iBACxC;aACF;iBAAM;gBACL,IAAI,cAAc,GAAG,kBAAkB,GAAG,QAAQ,CAAC;;gBAGnD,IAAI,cAAc,GAAG,CAAC,EAAE;oBACtB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,cAAc,EAAE,CAAC,EAAE,EAAE;wBACvC,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;qBACxC;iBACF;qBAAM;oBACL,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;iBAClB;gBAED,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;gBAEjB,OAAO,cAAc,EAAE,GAAG,CAAC,EAAE;oBAC3B,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;iBAClB;gBAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,GAAG,IAAI,CAAC,GAAG,CAAC,cAAc,GAAG,CAAC,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,EAAE;oBAC7E,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;iBACxC;aACF;SACF;QAED,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;KACxB;IAED,2BAAM,GAAN;QACE,OAAO,EAAE,cAAc,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;KAC5C;;IAGD,mCAAc,GAAd;QACE,OAAO,EAAE,cAAc,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;KAC5C;;IAGM,2BAAgB,GAAvB,UAAwB,GAAuB;QAC7C,OAAO,UAAU,CAAC,UAAU,CAAC,GAAG,CAAC,cAAc,CAAC,CAAC;KAClD;;IAGD,qBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,4BAAO,GAAP;QACE,OAAO,sBAAmB,IAAI,CAAC,QAAQ,EAAE,QAAI,CAAC;KAC/C;IACH,iBAAC;AAAD,CAAC,IAAA;AAnoBY,gCAAU;AAqoBvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,YAAY,EAAE,CAAC,CAAC;;;;;;;;;;AClyBlF;;;;AAIA;;;;;;IASE,gBAAY,KAAa;QACvB,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,CAAC,KAAK,CAAC,CAAC;QAExD,IAAK,KAAiB,YAAY,MAAM,EAAE;YACxC,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;SACzB;QAED,IAAI,CAAC,KAAK,GAAG,CAAC,KAAK,CAAC;KACrB;;;;;;IAOD,wBAAO,GAAP;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,uBAAM,GAAN;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,+BAAc,GAAd,UAAe,OAAsB;QACnC,IAAI,OAAO,KAAK,OAAO,CAAC,MAAM,KAAK,OAAO,CAAC,OAAO,IAAI,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE;YAC5E,OAAO,IAAI,CAAC,KAAK,CAAC;SACnB;;;QAID,IAAI,MAAM,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,CAAC,EAAE;YACxC,OAAO,EAAE,aAAa,EAAE,MAAI,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAG,EAAE,CAAC;SACvD;QAED,IAAI,aAAqB,CAAC;QAC1B,IAAI,MAAM,CAAC,SAAS,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE;YAChC,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;YACtC,IAAI,aAAa,CAAC,MAAM,IAAI,EAAE,EAAE;gBAC9B,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,aAAa,CAAC,EAAE,CAAC,CAAC,WAAW,EAAE,CAAC;aAC5D;SACF;aAAM;YACL,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,QAAQ,EAAE,CAAC;SACvC;QAED,OAAO,EAAE,aAAa,eAAA,EAAE,CAAC;KAC1B;;IAGM,uBAAgB,GAAvB,UAAwB,GAAmB,EAAE,OAAsB;QACjE,IAAM,WAAW,GAAG,UAAU,CAAC,GAAG,CAAC,aAAa,CAAC,CAAC;QAClD,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,WAAW,GAAG,IAAI,MAAM,CAAC,WAAW,CAAC,CAAC;KAC3E;;IAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,wBAAO,GAAP;QACE,IAAM,KAAK,GAAG,IAAI,CAAC,cAAc,EAAoB,CAAC;QACtD,OAAO,gBAAc,KAAK,CAAC,aAAa,MAAG,CAAC;KAC7C;IACH,aAAC;AAAD,CAAC,IAAA;AAzEY,wBAAM;AA2EnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;AC/E1E;;;;AAIA;;;;;;IASE,eAAY,KAAsB;QAChC,IAAI,EAAE,IAAI,YAAY,KAAK,CAAC;YAAE,OAAO,IAAI,KAAK,CAAC,KAAK,CAAC,CAAC;QAEtD,IAAK,KAAiB,YAAY,MAAM,EAAE;YACxC,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;SACzB;QAED,IAAI,CAAC,KAAK,GAAG,CAAC,KAAK,CAAC;KACrB;;;;;;IAOD,uBAAO,GAAP;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,sBAAM,GAAN;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,8BAAc,GAAd,UAAe,OAAsB;QACnC,IAAI,OAAO,KAAK,OAAO,CAAC,OAAO,IAAI,OAAO,CAAC,MAAM,CAAC;YAAE,OAAO,IAAI,CAAC,KAAK,CAAC;QACtE,OAAO,EAAE,UAAU,EAAE,IAAI,CAAC,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;KAC9C;;IAGM,sBAAgB,GAAvB,UAAwB,GAAkB,EAAE,OAAsB;QAChE,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,GAAG,QAAQ,CAAC,GAAG,CAAC,UAAU,EAAE,EAAE,CAAC,GAAG,IAAI,KAAK,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC;KAC9F;;IAGD,gBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,uBAAO,GAAP;QACE,OAAO,eAAa,IAAI,CAAC,OAAO,EAAE,MAAG,CAAC;KACvC;IACH,YAAC;AAAD,CAAC,IAAA;AApDY,sBAAK;AAsDlB,MAAM,CAAC,cAAc,CAAC,KAAK,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,OAAO,EAAE,CAAC,CAAC;;;;;;;;;;AC5DxE;;;;AAIA;IAGE;QACE,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,EAAE,CAAC;KACpD;;IAGD,+BAAc,GAAd;QACE,OAAO,EAAE,OAAO,EAAE,CAAC,EAAE,CAAC;KACvB;;IAGM,uBAAgB,GAAvB;QACE,OAAO,IAAI,MAAM,EAAE,CAAC;KACrB;;IAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,wBAAO,GAAP;QACE,OAAO,cAAc,CAAC;KACvB;IACH,aAAC;AAAD,CAAC,IAAA;AAzBY,wBAAM;AA2BnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;AC/B1E;;;;AAIA;IAGE;QACE,IAAI,EAAE,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,EAAE,CAAC;KACpD;;IAGD,+BAAc,GAAd;QACE,OAAO,EAAE,OAAO,EAAE,CAAC,EAAE,CAAC;KACvB;;IAGM,uBAAgB,GAAvB;QACE,OAAO,IAAI,MAAM,EAAE,CAAC;KACrB;;IAGD,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,wBAAO,GAAP;QACE,OAAO,cAAc,CAAC;KACvB;IACH,aAAC;AAAD,CAAC,IAAA;AAzBY,wBAAM;AA2BnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;;;;ACpC1C;AACe;AACuB;AAEtE;AACA,IAAM,iBAAiB,GAAG,IAAI,MAAM,CAAC,mBAAmB,CAAC,CAAC;AAE1D;AACA,IAAI,cAAc,GAAsB,IAAI,CAAC;AAc7C,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;AAEzB;;;;AAIA;;;;;;IAkBE,kBAAY,EAAuD;QACjE,IAAI,EAAE,IAAI,YAAY,QAAQ,CAAC;YAAE,OAAO,IAAI,QAAQ,CAAC,EAAE,CAAC,CAAC;;QAGzD,IAAI,EAAE,YAAY,QAAQ,EAAE;YAC1B,IAAI,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,CAAC;YAClB,IAAI,CAAC,IAAI,GAAG,EAAE,CAAC,IAAI,CAAC;SACrB;QAED,IAAI,OAAO,EAAE,KAAK,QAAQ,IAAI,EAAE,IAAI,IAAI,IAAI,EAAE,EAAE;YAC9C,IAAI,aAAa,IAAI,EAAE,IAAI,OAAO,EAAE,CAAC,WAAW,KAAK,UAAU,EAAE;gBAC/D,IAAI,CAAC,GAAG,CAAC,GAAGL,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,EAAE,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM;gBACL,IAAI,CAAC,GAAG,CAAC,GAAG,OAAO,EAAE,CAAC,EAAE,KAAK,QAAQ,GAAGA,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,EAAE,CAAC,EAAE,CAAC;aACpE;SACF;;QAGD,IAAI,EAAE,IAAI,IAAI,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;;YAExC,IAAI,CAAC,GAAG,CAAC,GAAG,QAAQ,CAAC,QAAQ,CAAC,OAAO,EAAE,KAAK,QAAQ,GAAG,EAAE,GAAG,SAAS,CAAC,CAAC;;YAEvE,IAAI,QAAQ,CAAC,cAAc,EAAE;gBAC3B,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aACrC;SACF;QAED,IAAI,WAAW,CAAC,MAAM,CAAC,EAAE,CAAC,IAAI,EAAE,CAAC,UAAU,KAAK,EAAE,EAAE;YAClD,IAAI,CAAC,GAAG,CAAC,GAAGE,0BAAY,CAAC,EAAE,CAAC,CAAC;SAC9B;QAED,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;YAC1B,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,EAAE;gBACpB,IAAM,KAAK,GAAGF,aAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;gBAC9B,IAAI,KAAK,CAAC,UAAU,KAAK,EAAE,EAAE;oBAC3B,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;iBACnB;aACF;iBAAM,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE;gBACzD,IAAI,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,IAAI,CAAC,EAAE,EAAE,KAAK,CAAC,CAAC;aACpC;iBAAM;gBACL,MAAM,IAAI,SAAS,CACjB,4FAA4F,CAC7F,CAAC;aACH;SACF;QAED,IAAI,QAAQ,CAAC,cAAc,EAAE;YAC3B,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;SACrC;KACF;IAMD,sBAAI,wBAAE;;;;;aAAN;YACE,OAAO,IAAI,CAAC,GAAG,CAAC,CAAC;SAClB;aAED,UAAO,KAAa;YAClB,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;YAClB,IAAI,QAAQ,CAAC,cAAc,EAAE;gBAC3B,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aACnC;SACF;;;OAPA;IAaD,sBAAI,oCAAc;;;;;aAAlB;YACE,OAAO,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,CAAC,CAAC,CAAC;SAC/B;aAED,UAAmB,KAAa;;YAE9B,IAAI,CAAC,EAAE,CAAC,aAAa,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC;SACjC;;;OALA;;IAQD,8BAAW,GAAX;QACE,IAAI,QAAQ,CAAC,cAAc,IAAI,IAAI,CAAC,IAAI,EAAE;YACxC,OAAO,IAAI,CAAC,IAAI,CAAC;SAClB;QAED,IAAM,SAAS,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;QAE1C,IAAI,QAAQ,CAAC,cAAc,IAAI,CAAC,IAAI,CAAC,IAAI,EAAE;YACzC,IAAI,CAAC,IAAI,GAAG,SAAS,CAAC;SACvB;QAED,OAAO,SAAS,CAAC;KAClB;;;;;;;IAQM,eAAM,GAAb;QACE,QAAQ,QAAQ,CAAC,KAAK,GAAG,CAAC,QAAQ,CAAC,KAAK,GAAG,CAAC,IAAI,QAAQ,EAAE;KAC3D;;;;;;IAOM,iBAAQ,GAAf,UAAgB,IAAa;QAC3B,IAAI,QAAQ,KAAK,OAAO,IAAI,EAAE;YAC5B,IAAI,GAAG,CAAC,EAAE,IAAI,CAAC,GAAG,EAAE,GAAG,IAAI,CAAC,CAAC;SAC9B;QAED,IAAM,GAAG,GAAG,QAAQ,CAAC,MAAM,EAAE,CAAC;QAC9B,IAAMK,QAAM,GAAGL,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;;QAGhCK,QAAM,CAAC,aAAa,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;;QAG9B,IAAI,cAAc,KAAK,IAAI,EAAE;YAC3B,cAAc,GAAGJ,iBAAW,CAAC,CAAC,CAAC,CAAC;SACjC;;QAGDI,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9BA,QAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;;QAG9BA,QAAM,CAAC,EAAE,CAAC,GAAG,GAAG,GAAG,IAAI,CAAC;QACxBA,QAAM,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,IAAI,IAAI,CAAC;QAC/BA,QAAM,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,IAAI,IAAI,CAAC;QAE/B,OAAOA,QAAM,CAAC;KACf;;;;;;;IAQD,2BAAQ,GAAR,UAAS,MAAe;;QAEtB,IAAI,MAAM;YAAE,OAAO,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC;QAC5C,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;KAC3B;;;;;IAMD,yBAAM,GAAN;QACE,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;KAC3B;;;;;;IAOD,yBAAM,GAAN,UAAO,OAAyC;QAC9C,IAAI,OAAO,KAAK,SAAS,IAAI,OAAO,KAAK,IAAI,EAAE;YAC7C,OAAO,KAAK,CAAC;SACd;QAED,IAAI,OAAO,YAAY,QAAQ,EAAE;YAC/B,OAAO,IAAI,CAAC,QAAQ,EAAE,KAAK,OAAO,CAAC,QAAQ,EAAE,CAAC;SAC/C;QAED,IACE,OAAO,OAAO,KAAK,QAAQ;YAC3B,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC;YACzB,OAAO,CAAC,MAAM,KAAK,EAAE;YACrBJ,kBAAY,CAAC,IAAI,CAAC,EAAE,CAAC,EACrB;YACA,OAAO,OAAO,KAAKD,aAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,IAAI,CAAC,EAAE,EAAE,QAAQ,CAAC,CAAC;SACtE;QAED,IAAI,OAAO,OAAO,KAAK,QAAQ,IAAI,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC,IAAI,OAAO,CAAC,MAAM,KAAK,EAAE,EAAE;YACrF,OAAO,OAAO,CAAC,WAAW,EAAE,KAAK,IAAI,CAAC,WAAW,EAAE,CAAC;SACrD;QAED,IAAI,OAAO,OAAO,KAAK,QAAQ,IAAI,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC,IAAI,OAAO,CAAC,MAAM,KAAK,EAAE,EAAE;YACrF,OAAOA,aAAM,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;SAC7C;QAED,IACE,OAAO,OAAO,KAAK,QAAQ;YAC3B,aAAa,IAAI,OAAO;YACxB,OAAO,OAAO,CAAC,WAAW,KAAK,UAAU,EACzC;YACA,OAAO,OAAO,CAAC,WAAW,EAAE,KAAK,IAAI,CAAC,WAAW,EAAE,CAAC;SACrD;QAED,OAAO,KAAK,CAAC;KACd;;IAGD,+BAAY,GAAZ;QACE,IAAM,SAAS,GAAG,IAAI,IAAI,EAAE,CAAC;QAC7B,IAAM,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,YAAY,CAAC,CAAC,CAAC,CAAC;QACrC,SAAS,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,CAAC;QAC3C,OAAO,SAAS,CAAC;KAClB;;IAGM,iBAAQ,GAAf;QACE,OAAO,IAAI,QAAQ,EAAE,CAAC;KACvB;;;;;;IAOM,uBAAc,GAArB,UAAsB,IAAY;QAChC,IAAMK,QAAM,GAAGL,aAAM,CAAC,IAAI,CAAC,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;;QAEjEK,QAAM,CAAC,aAAa,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;;QAE9B,OAAO,IAAI,QAAQ,CAACA,QAAM,CAAC,CAAC;KAC7B;;;;;;IAOM,4BAAmB,GAA1B,UAA2B,SAAiB;;QAE1C,IAAI,OAAO,SAAS,KAAK,WAAW,KAAK,SAAS,IAAI,IAAI,IAAI,SAAS,CAAC,MAAM,KAAK,EAAE,CAAC,EAAE;YACtF,MAAM,IAAI,SAAS,CACjB,yFAAyF,CAC1F,CAAC;SACH;QAED,OAAO,IAAI,QAAQ,CAACL,aAAM,CAAC,IAAI,CAAC,SAAS,EAAE,KAAK,CAAC,CAAC,CAAC;KACpD;;;;;;IAOM,gBAAO,GAAd,UAAe,EAA0D;QACvE,IAAI,EAAE,IAAI,IAAI;YAAE,OAAO,KAAK,CAAC;QAE7B,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;YAC1B,OAAO,IAAI,CAAC;SACb;QAED,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;YAC1B,OAAO,EAAE,CAAC,MAAM,KAAK,EAAE,KAAK,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC;SAC7E;QAED,IAAI,EAAE,YAAY,QAAQ,EAAE;YAC1B,OAAO,IAAI,CAAC;SACb;QAED,IAAIC,kBAAY,CAAC,EAAE,CAAC,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,EAAE;YACxC,OAAO,IAAI,CAAC;SACb;;QAGD,IAAI,OAAO,EAAE,KAAK,QAAQ,IAAI,aAAa,IAAI,EAAE,IAAI,OAAO,EAAE,CAAC,WAAW,KAAK,UAAU,EAAE;YACzF,IAAI,OAAO,EAAE,CAAC,EAAE,KAAK,QAAQ,EAAE;gBAC7B,OAAO,EAAE,CAAC,EAAE,CAAC,MAAM,KAAK,EAAE,CAAC;aAC5B;YACD,OAAO,EAAE,CAAC,WAAW,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC;SACxF;QAED,OAAO,KAAK,CAAC;KACd;;IAGD,iCAAc,GAAd;QACE,IAAI,IAAI,CAAC,WAAW;YAAE,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,WAAW,EAAE,EAAE,CAAC;QAC1D,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,EAAE,CAAC;KACvC;;IAGM,yBAAgB,GAAvB,UAAwB,GAAqB;QAC3C,OAAO,IAAI,QAAQ,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;KAC/B;;;;;;;IAQD,mBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,0BAAO,GAAP;QACE,OAAO,oBAAiB,IAAI,CAAC,WAAW,EAAE,QAAI,CAAC;KAChD;;IA5TM,cAAK,GAAG,CAAC,EAAE,IAAI,CAAC,MAAM,EAAE,GAAG,QAAQ,CAAC,CAAC;IA6T9C,eAAC;CAjUD,IAiUC;AAjUY,4BAAQ;AAmUrB;AACA,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,UAAU,EAAE;IACpD,KAAK,EAAEA,eAAS,CACd,UAAC,IAAY,IAAK,OAAA,QAAQ,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAA,EACzC,yDAAyD,CAC1D;CACF,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,QAAQ,EAAE;IAClD,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;CAC/F,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,SAAS,EAAE;IACnD,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;CAC/F,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,EAAE,SAAS,EAAE;IACzC,KAAK,EAAEA,eAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,GAAA,EAAE,mDAAmD,CAAC;CAC/F,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,UAAU,EAAE,CAAC,CAAC;;;;;;;;;;ACjX9E,SAAS,WAAW,CAAC,GAAW;IAC9B,OAAO,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;AACvC,CAAC;AAgBD;;;;AAIA;;;;;IASE,oBAAY,OAAe,EAAE,OAAgB;QAC3C,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;YAAE,OAAO,IAAI,UAAU,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;QAE3E,IAAI,CAAC,OAAO,GAAG,OAAO,CAAC;QACvB,IAAI,CAAC,OAAO,GAAG,WAAW,CAAC,OAAO,aAAP,OAAO,cAAP,OAAO,GAAI,EAAE,CAAC,CAAC;;QAG1C,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,OAAO,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;YAC5C,IACE,EACE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG,CACxB,EACD;gBACA,MAAM,IAAI,KAAK,CAAC,oCAAkC,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,uBAAoB,CAAC,CAAC;aACxF;SACF;KACF;IAEM,uBAAY,GAAnB,UAAoB,OAAgB;QAClC,OAAO,OAAO,GAAG,OAAO,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,GAAG,EAAE,CAAC;KACzD;;IAGD,mCAAc,GAAd,UAAe,OAAsB;QACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO,EAAE,MAAM,EAAE,IAAI,CAAC,OAAO,EAAE,QAAQ,EAAE,IAAI,CAAC,OAAO,EAAE,CAAC;SACzD;QACD,OAAO,EAAE,kBAAkB,EAAE,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,EAAE,CAAC;KACjF;;IAGM,2BAAgB,GAAvB,UAAwB,GAAkD;QACxE,IAAI,QAAQ,IAAI,GAAG,EAAE;YACnB,IAAI,OAAO,GAAG,CAAC,MAAM,KAAK,QAAQ,EAAE;;gBAElC,IAAI,GAAG,CAAC,MAAM,CAAC,SAAS,KAAK,YAAY,EAAE;oBACzC,OAAQ,GAA6B,CAAC;iBACvC;aACF;iBAAM;gBACL,OAAO,IAAI,UAAU,CAAC,GAAG,CAAC,MAAM,EAAE,UAAU,CAAC,YAAY,CAAC,GAAG,CAAC,QAAQ,CAAC,CAAC,CAAC;aAC1E;SACF;QACD,IAAI,oBAAoB,IAAI,GAAG,EAAE;YAC/B,OAAO,IAAI,UAAU,CACnB,GAAG,CAAC,kBAAkB,CAAC,OAAO,EAC9B,UAAU,CAAC,YAAY,CAAC,GAAG,CAAC,kBAAkB,CAAC,OAAO,CAAC,CACxD,CAAC;SACH;QACD,MAAM,IAAI,SAAS,CAAC,8CAA4C,IAAI,CAAC,SAAS,CAAC,GAAG,CAAG,CAAC,CAAC;KACxF;IACH,iBAAC;AAAD,CAAC,IAAA;AAjEY,gCAAU;AAmEvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,YAAY,EAAE,CAAC,CAAC;;;;;;;;;;ACtFlF;;;;AAIA;;;;IAOE,oBAAY,KAAa;QACvB,IAAI,EAAE,IAAI,YAAY,UAAU,CAAC;YAAE,OAAO,IAAI,UAAU,CAAC,KAAK,CAAC,CAAC;QAEhE,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;KACpB;;IAGD,4BAAO,GAAP;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,6BAAQ,GAAR;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,4BAAO,GAAP;QACE,OAAO,sBAAmB,IAAI,CAAC,KAAK,QAAI,CAAC;KAC1C;;IAGD,2BAAM,GAAN;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;KACnB;;IAGD,mCAAc,GAAd;QACE,OAAO,EAAE,OAAO,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;KAChC;;IAGM,2BAAgB,GAAvB,UAAwB,GAAuB;QAC7C,OAAO,IAAI,UAAU,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;KACpC;;IAGD,qBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IACH,iBAAC;AAAD,CAAC,IAAA;AA/CY,gCAAU;AAiDvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC;;;;;;;AC1D9E;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;AACA;AAEA,IAAIM,cAAa,GAAG,uBAAS3B,CAAT,EAAY4B,CAAZ,EAAe;AAC/BD,EAAAA,cAAa,GAAG1J,MAAM,CAAC4J,cAAP,IACX;AAAEC,IAAAA,SAAS,EAAE;AAAb,eAA6BpC,KAA7B,IAAsC,UAAUM,CAAV,EAAa4B,CAAb,EAAgB;AAAE5B,IAAAA,CAAC,CAAC8B,SAAF,GAAcF,CAAd;AAAkB,GAD/D,IAEZ,UAAU5B,CAAV,EAAa4B,CAAb,EAAgB;AAAE,SAAK,IAAIG,CAAT,IAAcH,CAAd;AAAiB,UAAIA,CAAC,CAAC/C,cAAF,CAAiBkD,CAAjB,CAAJ,EAAyB/B,CAAC,CAAC+B,CAAD,CAAD,GAAOH,CAAC,CAACG,CAAD,CAAR;AAA1C;AAAwD,GAF9E;;AAGA,SAAOJ,cAAa,CAAC3B,CAAD,EAAI4B,CAAJ,CAApB;AACH,CALD;;AAOO,SAASI,SAAT,CAAmBhC,CAAnB,EAAsB4B,CAAtB,EAAyB;AAC5BD,EAAAA,cAAa,CAAC3B,CAAD,EAAI4B,CAAJ,CAAb;;AACA,WAASK,EAAT,GAAc;AAAE,SAAK1J,WAAL,GAAmByH,CAAnB;AAAuB;;AACvCA,EAAAA,CAAC,CAAC1H,SAAF,GAAcsJ,CAAC,KAAK,IAAN,GAAa3J,MAAM,CAACC,MAAP,CAAc0J,CAAd,CAAb,IAAiCK,EAAE,CAAC3J,SAAH,GAAesJ,CAAC,CAACtJ,SAAjB,EAA4B,IAAI2J,EAAJ,EAA7D,CAAd;AACH;;AAEM,IAAIC,OAAQ,GAAG,oBAAW;AAC7BA,EAAAA,OAAQ,GAAGjK,MAAM,CAACkK,MAAP,IAAiB,SAASD,QAAT,CAAkBE,CAAlB,EAAqB;AAC7C,SAAK,IAAIC,CAAJ,EAAOnJ,CAAC,GAAG,CAAX,EAAc8E,CAAC,GAAG7E,SAAS,CAACC,MAAjC,EAAyCF,CAAC,GAAG8E,CAA7C,EAAgD9E,CAAC,EAAjD,EAAqD;AACjDmJ,MAAAA,CAAC,GAAGlJ,SAAS,CAACD,CAAD,CAAb;;AACA,WAAK,IAAI6I,CAAT,IAAcM,CAAd;AAAiB,YAAIpK,MAAM,CAACK,SAAP,CAAiBuG,cAAjB,CAAgClB,IAAhC,CAAqC0E,CAArC,EAAwCN,CAAxC,CAAJ,EAAgDK,CAAC,CAACL,CAAD,CAAD,GAAOM,CAAC,CAACN,CAAD,CAAR;AAAjE;AACH;;AACD,WAAOK,CAAP;AACH,GAND;;AAOA,SAAOF,OAAQ,CAACzH,KAAT,CAAe,IAAf,EAAqBtB,SAArB,CAAP;AACH,CATM;AAWA,SAASmJ,MAAT,CAAgBD,CAAhB,EAAmBpC,CAAnB,EAAsB;AACzB,MAAImC,CAAC,GAAG,EAAR;;AACA,OAAK,IAAIL,CAAT,IAAcM,CAAd;AAAiB,QAAIpK,MAAM,CAACK,SAAP,CAAiBuG,cAAjB,CAAgClB,IAAhC,CAAqC0E,CAArC,EAAwCN,CAAxC,KAA8C9B,CAAC,CAAC3C,OAAF,CAAUyE,CAAV,IAAe,CAAjE,EACbK,CAAC,CAACL,CAAD,CAAD,GAAOM,CAAC,CAACN,CAAD,CAAR;AADJ;;AAEA,MAAIM,CAAC,IAAI,IAAL,IAAa,OAAOpK,MAAM,CAACsK,qBAAd,KAAwC,UAAzD,EACI,KAAK,IAAIrJ,CAAC,GAAG,CAAR,EAAW6I,CAAC,GAAG9J,MAAM,CAACsK,qBAAP,CAA6BF,CAA7B,CAApB,EAAqDnJ,CAAC,GAAG6I,CAAC,CAAC3I,MAA3D,EAAmEF,CAAC,EAApE,EAAwE;AACpE,QAAI+G,CAAC,CAAC3C,OAAF,CAAUyE,CAAC,CAAC7I,CAAD,CAAX,IAAkB,CAAlB,IAAuBjB,MAAM,CAACK,SAAP,CAAiBkK,oBAAjB,CAAsC7E,IAAtC,CAA2C0E,CAA3C,EAA8CN,CAAC,CAAC7I,CAAD,CAA/C,CAA3B,EACIkJ,CAAC,CAACL,CAAC,CAAC7I,CAAD,CAAF,CAAD,GAAUmJ,CAAC,CAACN,CAAC,CAAC7I,CAAD,CAAF,CAAX;AACP;AACL,SAAOkJ,CAAP;AACH;AAEM,SAASK,UAAT,CAAoBC,UAApB,EAAgCC,MAAhC,EAAwCtE,GAAxC,EAA6CU,IAA7C,EAAmD;AACtD,MAAI6D,CAAC,GAAGzJ,SAAS,CAACC,MAAlB;AAAA,MAA0ByJ,CAAC,GAAGD,CAAC,GAAG,CAAJ,GAAQD,MAAR,GAAiB5D,IAAI,KAAK,IAAT,GAAgBA,IAAI,GAAG9G,MAAM,CAAC+G,wBAAP,CAAgC2D,MAAhC,EAAwCtE,GAAxC,CAAvB,GAAsEU,IAArH;AAAA,MAA2HiB,CAA3H;AACA,MAAI,QAAO8C,OAAP,wDAAOA,OAAP,OAAmB,QAAnB,IAA+B,OAAOA,OAAO,CAACC,QAAf,KAA4B,UAA/D,EAA2EF,CAAC,GAAGC,OAAO,CAACC,QAAR,CAAiBL,UAAjB,EAA6BC,MAA7B,EAAqCtE,GAArC,EAA0CU,IAA1C,CAAJ,CAA3E,KACK,KAAK,IAAI7F,CAAC,GAAGwJ,UAAU,CAACtJ,MAAX,GAAoB,CAAjC,EAAoCF,CAAC,IAAI,CAAzC,EAA4CA,CAAC,EAA7C;AAAiD,QAAI8G,CAAC,GAAG0C,UAAU,CAACxJ,CAAD,CAAlB,EAAuB2J,CAAC,GAAG,CAACD,CAAC,GAAG,CAAJ,GAAQ5C,CAAC,CAAC6C,CAAD,CAAT,GAAeD,CAAC,GAAG,CAAJ,GAAQ5C,CAAC,CAAC2C,MAAD,EAAStE,GAAT,EAAcwE,CAAd,CAAT,GAA4B7C,CAAC,CAAC2C,MAAD,EAAStE,GAAT,CAA7C,KAA+DwE,CAAnE;AAAxE;AACL,SAAOD,CAAC,GAAG,CAAJ,IAASC,CAAT,IAAc5K,MAAM,CAAC+K,cAAP,CAAsBL,MAAtB,EAA8BtE,GAA9B,EAAmCwE,CAAnC,CAAd,EAAqDA,CAA5D;AACH;AAEM,SAASI,OAAT,CAAiBC,UAAjB,EAA6BC,SAA7B,EAAwC;AAC3C,SAAO,UAAUR,MAAV,EAAkBtE,GAAlB,EAAuB;AAAE8E,IAAAA,SAAS,CAACR,MAAD,EAAStE,GAAT,EAAc6E,UAAd,CAAT;AAAqC,GAArE;AACH;AAEM,SAASE,UAAT,CAAoBC,WAApB,EAAiCC,aAAjC,EAAgD;AACnD,MAAI,QAAOR,OAAP,wDAAOA,OAAP,OAAmB,QAAnB,IAA+B,OAAOA,OAAO,CAACS,QAAf,KAA4B,UAA/D,EAA2E,OAAOT,OAAO,CAACS,QAAR,CAAiBF,WAAjB,EAA8BC,aAA9B,CAAP;AAC9E;AAEM,SAASE,SAAT,CAAmBC,OAAnB,EAA4BC,UAA5B,EAAwCC,CAAxC,EAA2CC,SAA3C,EAAsD;AACzD,WAASC,KAAT,CAAerL,KAAf,EAAsB;AAAE,WAAOA,KAAK,YAAYmL,CAAjB,GAAqBnL,KAArB,GAA6B,IAAImL,CAAJ,CAAM,UAAUG,OAAV,EAAmB;AAAEA,MAAAA,OAAO,CAACtL,KAAD,CAAP;AAAiB,KAA5C,CAApC;AAAoF;;AAC5G,SAAO,KAAKmL,CAAC,KAAKA,CAAC,GAAGI,OAAT,CAAN,EAAyB,UAAUD,OAAV,EAAmBE,MAAnB,EAA2B;AACvD,aAASC,SAAT,CAAmBzL,KAAnB,EAA0B;AAAE,UAAI;AAAE0L,QAAAA,IAAI,CAACN,SAAS,CAACO,IAAV,CAAe3L,KAAf,CAAD,CAAJ;AAA8B,OAApC,CAAqC,OAAOyH,CAAP,EAAU;AAAE+D,QAAAA,MAAM,CAAC/D,CAAD,CAAN;AAAY;AAAE;;AAC3F,aAASmE,QAAT,CAAkB5L,KAAlB,EAAyB;AAAE,UAAI;AAAE0L,QAAAA,IAAI,CAACN,SAAS,CAAC,OAAD,CAAT,CAAmBpL,KAAnB,CAAD,CAAJ;AAAkC,OAAxC,CAAyC,OAAOyH,CAAP,EAAU;AAAE+D,QAAAA,MAAM,CAAC/D,CAAD,CAAN;AAAY;AAAE;;AAC9F,aAASiE,IAAT,CAAcG,MAAd,EAAsB;AAAEA,MAAAA,MAAM,CAACC,IAAP,GAAcR,OAAO,CAACO,MAAM,CAAC7L,KAAR,CAArB,GAAsCqL,KAAK,CAACQ,MAAM,CAAC7L,KAAR,CAAL,CAAoB+L,IAApB,CAAyBN,SAAzB,EAAoCG,QAApC,CAAtC;AAAsF;;AAC9GF,IAAAA,IAAI,CAAC,CAACN,SAAS,GAAGA,SAAS,CAACnJ,KAAV,CAAgBgJ,OAAhB,EAAyBC,UAAU,IAAI,EAAvC,CAAb,EAAyDS,IAAzD,EAAD,CAAJ;AACH,GALM,CAAP;AAMH;AAEM,SAASK,WAAT,CAAqBf,OAArB,EAA8BgB,IAA9B,EAAoC;AACvC,MAAIxK,CAAC,GAAG;AAAEyK,IAAAA,KAAK,EAAE,CAAT;AAAYC,IAAAA,IAAI,EAAE,gBAAW;AAAE,UAAIvC,CAAC,CAAC,CAAD,CAAD,GAAO,CAAX,EAAc,MAAMA,CAAC,CAAC,CAAD,CAAP;AAAY,aAAOA,CAAC,CAAC,CAAD,CAAR;AAAc,KAAvE;AAAyEwC,IAAAA,IAAI,EAAE,EAA/E;AAAmFC,IAAAA,GAAG,EAAE;AAAxF,GAAR;AAAA,MAAsG9L,CAAtG;AAAA,MAAyG+L,CAAzG;AAAA,MAA4G1C,CAA5G;AAAA,MAA+G2C,CAA/G;AACA,SAAOA,CAAC,GAAG;AAAEZ,IAAAA,IAAI,EAAEa,IAAI,CAAC,CAAD,CAAZ;AAAiB,aAASA,IAAI,CAAC,CAAD,CAA9B;AAAmC,cAAUA,IAAI,CAAC,CAAD;AAAjD,GAAJ,EAA4D,OAAOC,MAAP,KAAkB,UAAlB,KAAiCF,CAAC,CAACE,MAAM,CAACC,QAAR,CAAD,GAAqB,YAAW;AAAE,WAAO,IAAP;AAAc,GAAjF,CAA5D,EAAgJH,CAAvJ;;AACA,WAASC,IAAT,CAAchH,CAAd,EAAiB;AAAE,WAAO,UAAUmH,CAAV,EAAa;AAAE,aAAOjB,IAAI,CAAC,CAAClG,CAAD,EAAImH,CAAJ,CAAD,CAAX;AAAsB,KAA5C;AAA+C;;AAClE,WAASjB,IAAT,CAAckB,EAAd,EAAkB;AACd,QAAIrM,CAAJ,EAAO,MAAM,IAAIsM,SAAJ,CAAc,iCAAd,CAAN;;AACP,WAAOpL,CAAP;AAAU,UAAI;AACV,YAAIlB,CAAC,GAAG,CAAJ,EAAO+L,CAAC,KAAK1C,CAAC,GAAGgD,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAR,GAAYN,CAAC,CAAC,QAAD,CAAb,GAA0BM,EAAE,CAAC,CAAD,CAAF,GAAQN,CAAC,CAAC,OAAD,CAAD,KAAe,CAAC1C,CAAC,GAAG0C,CAAC,CAAC,QAAD,CAAN,KAAqB1C,CAAC,CAACzE,IAAF,CAAOmH,CAAP,CAArB,EAAgC,CAA/C,CAAR,GAA4DA,CAAC,CAACX,IAAjG,CAAD,IAA2G,CAAC,CAAC/B,CAAC,GAAGA,CAAC,CAACzE,IAAF,CAAOmH,CAAP,EAAUM,EAAE,CAAC,CAAD,CAAZ,CAAL,EAAuBd,IAA9I,EAAoJ,OAAOlC,CAAP;AACpJ,YAAI0C,CAAC,GAAG,CAAJ,EAAO1C,CAAX,EAAcgD,EAAE,GAAG,CAACA,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAT,EAAYhD,CAAC,CAAC5J,KAAd,CAAL;;AACd,gBAAQ4M,EAAE,CAAC,CAAD,CAAV;AACI,eAAK,CAAL;AAAQ,eAAK,CAAL;AAAQhD,YAAAA,CAAC,GAAGgD,EAAJ;AAAQ;;AACxB,eAAK,CAAL;AAAQnL,YAAAA,CAAC,CAACyK,KAAF;AAAW,mBAAO;AAAElM,cAAAA,KAAK,EAAE4M,EAAE,CAAC,CAAD,CAAX;AAAgBd,cAAAA,IAAI,EAAE;AAAtB,aAAP;;AACnB,eAAK,CAAL;AAAQrK,YAAAA,CAAC,CAACyK,KAAF;AAAWI,YAAAA,CAAC,GAAGM,EAAE,CAAC,CAAD,CAAN;AAAWA,YAAAA,EAAE,GAAG,CAAC,CAAD,CAAL;AAAU;;AACxC,eAAK,CAAL;AAAQA,YAAAA,EAAE,GAAGnL,CAAC,CAAC4K,GAAF,CAAMtG,GAAN,EAAL;;AAAkBtE,YAAAA,CAAC,CAAC2K,IAAF,CAAOrG,GAAP;;AAAc;;AACxC;AACI,gBAAI,EAAE6D,CAAC,GAAGnI,CAAC,CAAC2K,IAAN,EAAYxC,CAAC,GAAGA,CAAC,CAAChJ,MAAF,GAAW,CAAX,IAAgBgJ,CAAC,CAACA,CAAC,CAAChJ,MAAF,GAAW,CAAZ,CAAnC,MAAuDgM,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,IAAeA,EAAE,CAAC,CAAD,CAAF,KAAU,CAAhF,CAAJ,EAAwF;AAAEnL,cAAAA,CAAC,GAAG,CAAJ;AAAO;AAAW;;AAC5G,gBAAImL,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,KAAgB,CAAChD,CAAD,IAAOgD,EAAE,CAAC,CAAD,CAAF,GAAQhD,CAAC,CAAC,CAAD,CAAT,IAAgBgD,EAAE,CAAC,CAAD,CAAF,GAAQhD,CAAC,CAAC,CAAD,CAAhD,CAAJ,EAA2D;AAAEnI,cAAAA,CAAC,CAACyK,KAAF,GAAUU,EAAE,CAAC,CAAD,CAAZ;AAAiB;AAAQ;;AACtF,gBAAIA,EAAE,CAAC,CAAD,CAAF,KAAU,CAAV,IAAenL,CAAC,CAACyK,KAAF,GAAUtC,CAAC,CAAC,CAAD,CAA9B,EAAmC;AAAEnI,cAAAA,CAAC,CAACyK,KAAF,GAAUtC,CAAC,CAAC,CAAD,CAAX;AAAgBA,cAAAA,CAAC,GAAGgD,EAAJ;AAAQ;AAAQ;;AACrE,gBAAIhD,CAAC,IAAInI,CAAC,CAACyK,KAAF,GAAUtC,CAAC,CAAC,CAAD,CAApB,EAAyB;AAAEnI,cAAAA,CAAC,CAACyK,KAAF,GAAUtC,CAAC,CAAC,CAAD,CAAX;;AAAgBnI,cAAAA,CAAC,CAAC4K,GAAF,CAAMxL,IAAN,CAAW+L,EAAX;;AAAgB;AAAQ;;AACnE,gBAAIhD,CAAC,CAAC,CAAD,CAAL,EAAUnI,CAAC,CAAC4K,GAAF,CAAMtG,GAAN;;AACVtE,YAAAA,CAAC,CAAC2K,IAAF,CAAOrG,GAAP;;AAAc;AAXtB;;AAaA6G,QAAAA,EAAE,GAAGX,IAAI,CAAC9G,IAAL,CAAU8F,OAAV,EAAmBxJ,CAAnB,CAAL;AACH,OAjBS,CAiBR,OAAOgG,CAAP,EAAU;AAAEmF,QAAAA,EAAE,GAAG,CAAC,CAAD,EAAInF,CAAJ,CAAL;AAAa6E,QAAAA,CAAC,GAAG,CAAJ;AAAQ,OAjBzB,SAiBkC;AAAE/L,QAAAA,CAAC,GAAGqJ,CAAC,GAAG,CAAR;AAAY;AAjB1D;;AAkBA,QAAIgD,EAAE,CAAC,CAAD,CAAF,GAAQ,CAAZ,EAAe,MAAMA,EAAE,CAAC,CAAD,CAAR;AAAa,WAAO;AAAE5M,MAAAA,KAAK,EAAE4M,EAAE,CAAC,CAAD,CAAF,GAAQA,EAAE,CAAC,CAAD,CAAV,GAAgB,KAAK,CAA9B;AAAiCd,MAAAA,IAAI,EAAE;AAAvC,KAAP;AAC/B;AACJ;AAEM,SAASgB,eAAT,CAAyBhF,CAAzB,EAA4BiF,CAA5B,EAA+BC,CAA/B,EAAkCC,EAAlC,EAAsC;AACzC,MAAIA,EAAE,KAAKC,SAAX,EAAsBD,EAAE,GAAGD,CAAL;AACtBlF,EAAAA,CAAC,CAACmF,EAAD,CAAD,GAAQF,CAAC,CAACC,CAAD,CAAT;AACH;AAEM,SAASG,YAAT,CAAsBJ,CAAtB,EAAyBK,OAAzB,EAAkC;AACrC,OAAK,IAAI7D,CAAT,IAAcwD,CAAd;AAAiB,QAAIxD,CAAC,KAAK,SAAN,IAAmB,CAAC6D,OAAO,CAAC/G,cAAR,CAAuBkD,CAAvB,CAAxB,EAAmD6D,OAAO,CAAC7D,CAAD,CAAP,GAAawD,CAAC,CAACxD,CAAD,CAAd;AAApE;AACH;AAEM,SAAS8D,QAAT,CAAkBvF,CAAlB,EAAqB;AACxB,MAAI+B,CAAC,GAAG,OAAO4C,MAAP,KAAkB,UAAlB,IAAgCA,MAAM,CAACC,QAA/C;AAAA,MAAyDK,CAAC,GAAGlD,CAAC,IAAI/B,CAAC,CAAC+B,CAAD,CAAnE;AAAA,MAAwEnJ,CAAC,GAAG,CAA5E;AACA,MAAIqM,CAAJ,EAAO,OAAOA,CAAC,CAAC5H,IAAF,CAAO2C,CAAP,CAAP;AACP,MAAIA,CAAC,IAAI,OAAOA,CAAC,CAAClH,MAAT,KAAoB,QAA7B,EAAuC,OAAO;AAC1C+K,IAAAA,IAAI,EAAE,gBAAY;AACd,UAAI7D,CAAC,IAAIpH,CAAC,IAAIoH,CAAC,CAAClH,MAAhB,EAAwBkH,CAAC,GAAG,KAAK,CAAT;AACxB,aAAO;AAAE9H,QAAAA,KAAK,EAAE8H,CAAC,IAAIA,CAAC,CAACpH,CAAC,EAAF,CAAf;AAAsBoL,QAAAA,IAAI,EAAE,CAAChE;AAA7B,OAAP;AACH;AAJyC,GAAP;AAMvC,QAAM,IAAI+E,SAAJ,CAAchD,CAAC,GAAG,yBAAH,GAA+B,iCAA9C,CAAN;AACH;AAEM,SAASyD,MAAT,CAAgBxF,CAAhB,EAAmBtC,CAAnB,EAAsB;AACzB,MAAIuH,CAAC,GAAG,OAAON,MAAP,KAAkB,UAAlB,IAAgC3E,CAAC,CAAC2E,MAAM,CAACC,QAAR,CAAzC;AACA,MAAI,CAACK,CAAL,EAAQ,OAAOjF,CAAP;AACR,MAAIpH,CAAC,GAAGqM,CAAC,CAAC5H,IAAF,CAAO2C,CAAP,CAAR;AAAA,MAAmBuC,CAAnB;AAAA,MAAsBpD,EAAE,GAAG,EAA3B;AAAA,MAA+BQ,CAA/B;;AACA,MAAI;AACA,WAAO,CAACjC,CAAC,KAAK,KAAK,CAAX,IAAgBA,CAAC,KAAK,CAAvB,KAA6B,CAAC,CAAC6E,CAAC,GAAG3J,CAAC,CAACiL,IAAF,EAAL,EAAeG,IAApD;AAA0D7E,MAAAA,EAAE,CAACpG,IAAH,CAAQwJ,CAAC,CAACrK,KAAV;AAA1D;AACH,GAFD,CAGA,OAAOqC,KAAP,EAAc;AAAEoF,IAAAA,CAAC,GAAG;AAAEpF,MAAAA,KAAK,EAAEA;AAAT,KAAJ;AAAuB,GAHvC,SAIQ;AACJ,QAAI;AACA,UAAIgI,CAAC,IAAI,CAACA,CAAC,CAACyB,IAAR,KAAiBiB,CAAC,GAAGrM,CAAC,CAAC,QAAD,CAAtB,CAAJ,EAAuCqM,CAAC,CAAC5H,IAAF,CAAOzE,CAAP;AAC1C,KAFD,SAGQ;AAAE,UAAI+G,CAAJ,EAAO,MAAMA,CAAC,CAACpF,KAAR;AAAgB;AACpC;;AACD,SAAO4E,EAAP;AACH;AAEM,SAASsG,QAAT,GAAoB;AACvB,OAAK,IAAItG,EAAE,GAAG,EAAT,EAAavG,CAAC,GAAG,CAAtB,EAAyBA,CAAC,GAAGC,SAAS,CAACC,MAAvC,EAA+CF,CAAC,EAAhD;AACIuG,IAAAA,EAAE,GAAGA,EAAE,CAACuG,MAAH,CAAUF,MAAM,CAAC3M,SAAS,CAACD,CAAD,CAAV,CAAhB,CAAL;AADJ;;AAEA,SAAOuG,EAAP;AACH;AAEM,SAASwG,cAAT,GAA0B;AAC7B,OAAK,IAAI5D,CAAC,GAAG,CAAR,EAAWnJ,CAAC,GAAG,CAAf,EAAkBgN,EAAE,GAAG/M,SAAS,CAACC,MAAtC,EAA8CF,CAAC,GAAGgN,EAAlD,EAAsDhN,CAAC,EAAvD;AAA2DmJ,IAAAA,CAAC,IAAIlJ,SAAS,CAACD,CAAD,CAAT,CAAaE,MAAlB;AAA3D;;AACA,OAAK,IAAIyJ,CAAC,GAAGnD,KAAK,CAAC2C,CAAD,CAAb,EAAkBmD,CAAC,GAAG,CAAtB,EAAyBtM,CAAC,GAAG,CAAlC,EAAqCA,CAAC,GAAGgN,EAAzC,EAA6ChN,CAAC,EAA9C;AACI,SAAK,IAAIiN,CAAC,GAAGhN,SAAS,CAACD,CAAD,CAAjB,EAAsBkN,CAAC,GAAG,CAA1B,EAA6BC,EAAE,GAAGF,CAAC,CAAC/M,MAAzC,EAAiDgN,CAAC,GAAGC,EAArD,EAAyDD,CAAC,IAAIZ,CAAC,EAA/D;AACI3C,MAAAA,CAAC,CAAC2C,CAAD,CAAD,GAAOW,CAAC,CAACC,CAAD,CAAR;AADJ;AADJ;;AAGA,SAAOvD,CAAP;AACH;AAEM,SAASyD,OAAT,CAAiBnB,CAAjB,EAAoB;AACvB,SAAO,gBAAgBmB,OAAhB,IAA2B,KAAKnB,CAAL,GAASA,CAAT,EAAY,IAAvC,IAA+C,IAAImB,OAAJ,CAAYnB,CAAZ,CAAtD;AACH;AAEM,SAASoB,gBAAT,CAA0B9C,OAA1B,EAAmCC,UAAnC,EAA+CE,SAA/C,EAA0D;AAC7D,MAAI,CAACqB,MAAM,CAACuB,aAAZ,EAA2B,MAAM,IAAInB,SAAJ,CAAc,sCAAd,CAAN;AAC3B,MAAIN,CAAC,GAAGnB,SAAS,CAACnJ,KAAV,CAAgBgJ,OAAhB,EAAyBC,UAAU,IAAI,EAAvC,CAAR;AAAA,MAAoDxK,CAApD;AAAA,MAAuDuN,CAAC,GAAG,EAA3D;AACA,SAAOvN,CAAC,GAAG,EAAJ,EAAQ8L,IAAI,CAAC,MAAD,CAAZ,EAAsBA,IAAI,CAAC,OAAD,CAA1B,EAAqCA,IAAI,CAAC,QAAD,CAAzC,EAAqD9L,CAAC,CAAC+L,MAAM,CAACuB,aAAR,CAAD,GAA0B,YAAY;AAAE,WAAO,IAAP;AAAc,GAA3G,EAA6GtN,CAApH;;AACA,WAAS8L,IAAT,CAAchH,CAAd,EAAiB;AAAE,QAAI+G,CAAC,CAAC/G,CAAD,CAAL,EAAU9E,CAAC,CAAC8E,CAAD,CAAD,GAAO,UAAUmH,CAAV,EAAa;AAAE,aAAO,IAAIpB,OAAJ,CAAY,UAAUoC,CAAV,EAAavE,CAAb,EAAgB;AAAE6E,QAAAA,CAAC,CAACpN,IAAF,CAAO,CAAC2E,CAAD,EAAImH,CAAJ,EAAOgB,CAAP,EAAUvE,CAAV,CAAP,IAAuB,CAAvB,IAA4B8E,MAAM,CAAC1I,CAAD,EAAImH,CAAJ,CAAlC;AAA2C,OAAzE,CAAP;AAAoF,KAA1G;AAA6G;;AAC1I,WAASuB,MAAT,CAAgB1I,CAAhB,EAAmBmH,CAAnB,EAAsB;AAAE,QAAI;AAAEjB,MAAAA,IAAI,CAACa,CAAC,CAAC/G,CAAD,CAAD,CAAKmH,CAAL,CAAD,CAAJ;AAAgB,KAAtB,CAAuB,OAAOlF,CAAP,EAAU;AAAE0G,MAAAA,MAAM,CAACF,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUxG,CAAV,CAAN;AAAqB;AAAE;;AAClF,WAASiE,IAAT,CAAcrB,CAAd,EAAiB;AAAEA,IAAAA,CAAC,CAACrK,KAAF,YAAmB8N,OAAnB,GAA6BvC,OAAO,CAACD,OAAR,CAAgBjB,CAAC,CAACrK,KAAF,CAAQ2M,CAAxB,EAA2BZ,IAA3B,CAAgCqC,OAAhC,EAAyC5C,MAAzC,CAA7B,GAAgF2C,MAAM,CAACF,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAU5D,CAAV,CAAtF;AAAqG;;AACxH,WAAS+D,OAAT,CAAiBpO,KAAjB,EAAwB;AAAEkO,IAAAA,MAAM,CAAC,MAAD,EAASlO,KAAT,CAAN;AAAwB;;AAClD,WAASwL,MAAT,CAAgBxL,KAAhB,EAAuB;AAAEkO,IAAAA,MAAM,CAAC,OAAD,EAAUlO,KAAV,CAAN;AAAyB;;AAClD,WAASmO,MAAT,CAAgB5N,CAAhB,EAAmBoM,CAAnB,EAAsB;AAAE,QAAIpM,CAAC,CAACoM,CAAD,CAAD,EAAMsB,CAAC,CAACI,KAAF,EAAN,EAAiBJ,CAAC,CAACrN,MAAvB,EAA+BsN,MAAM,CAACD,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAD,EAAUA,CAAC,CAAC,CAAD,CAAD,CAAK,CAAL,CAAV,CAAN;AAA2B;AACrF;AAEM,SAASK,gBAAT,CAA0BxG,CAA1B,EAA6B;AAChC,MAAIpH,CAAJ,EAAO6I,CAAP;AACA,SAAO7I,CAAC,GAAG,EAAJ,EAAQ8L,IAAI,CAAC,MAAD,CAAZ,EAAsBA,IAAI,CAAC,OAAD,EAAU,UAAU/E,CAAV,EAAa;AAAE,UAAMA,CAAN;AAAU,GAAnC,CAA1B,EAAgE+E,IAAI,CAAC,QAAD,CAApE,EAAgF9L,CAAC,CAAC+L,MAAM,CAACC,QAAR,CAAD,GAAqB,YAAY;AAAE,WAAO,IAAP;AAAc,GAAjI,EAAmIhM,CAA1I;;AACA,WAAS8L,IAAT,CAAchH,CAAd,EAAiBjF,CAAjB,EAAoB;AAAEG,IAAAA,CAAC,CAAC8E,CAAD,CAAD,GAAOsC,CAAC,CAACtC,CAAD,CAAD,GAAO,UAAUmH,CAAV,EAAa;AAAE,aAAO,CAACpD,CAAC,GAAG,CAACA,CAAN,IAAW;AAAEvJ,QAAAA,KAAK,EAAE8N,OAAO,CAAChG,CAAC,CAACtC,CAAD,CAAD,CAAKmH,CAAL,CAAD,CAAhB;AAA2Bb,QAAAA,IAAI,EAAEtG,CAAC,KAAK;AAAvC,OAAX,GAA+DjF,CAAC,GAAGA,CAAC,CAACoM,CAAD,CAAJ,GAAUA,CAAjF;AAAqF,KAA3G,GAA8GpM,CAArH;AAAyH;AAClJ;AAEM,SAASgO,aAAT,CAAuBzG,CAAvB,EAA0B;AAC7B,MAAI,CAAC2E,MAAM,CAACuB,aAAZ,EAA2B,MAAM,IAAInB,SAAJ,CAAc,sCAAd,CAAN;AAC3B,MAAIE,CAAC,GAAGjF,CAAC,CAAC2E,MAAM,CAACuB,aAAR,CAAT;AAAA,MAAiCtN,CAAjC;AACA,SAAOqM,CAAC,GAAGA,CAAC,CAAC5H,IAAF,CAAO2C,CAAP,CAAH,IAAgBA,CAAC,GAAG,OAAOuF,QAAP,KAAoB,UAApB,GAAiCA,QAAQ,CAACvF,CAAD,CAAzC,GAA+CA,CAAC,CAAC2E,MAAM,CAACC,QAAR,CAAD,EAAnD,EAAyEhM,CAAC,GAAG,EAA7E,EAAiF8L,IAAI,CAAC,MAAD,CAArF,EAA+FA,IAAI,CAAC,OAAD,CAAnG,EAA8GA,IAAI,CAAC,QAAD,CAAlH,EAA8H9L,CAAC,CAAC+L,MAAM,CAACuB,aAAR,CAAD,GAA0B,YAAY;AAAE,WAAO,IAAP;AAAc,GAApL,EAAsLtN,CAAtM,CAAR;;AACA,WAAS8L,IAAT,CAAchH,CAAd,EAAiB;AAAE9E,IAAAA,CAAC,CAAC8E,CAAD,CAAD,GAAOsC,CAAC,CAACtC,CAAD,CAAD,IAAQ,UAAUmH,CAAV,EAAa;AAAE,aAAO,IAAIpB,OAAJ,CAAY,UAAUD,OAAV,EAAmBE,MAAnB,EAA2B;AAAEmB,QAAAA,CAAC,GAAG7E,CAAC,CAACtC,CAAD,CAAD,CAAKmH,CAAL,CAAJ,EAAawB,MAAM,CAAC7C,OAAD,EAAUE,MAAV,EAAkBmB,CAAC,CAACb,IAApB,EAA0Ba,CAAC,CAAC3M,KAA5B,CAAnB;AAAwD,OAAjG,CAAP;AAA4G,KAA1I;AAA6I;;AAChK,WAASmO,MAAT,CAAgB7C,OAAhB,EAAyBE,MAAzB,EAAiChE,CAAjC,EAAoCmF,CAApC,EAAuC;AAAEpB,IAAAA,OAAO,CAACD,OAAR,CAAgBqB,CAAhB,EAAmBZ,IAAnB,CAAwB,UAASY,CAAT,EAAY;AAAErB,MAAAA,OAAO,CAAC;AAAEtL,QAAAA,KAAK,EAAE2M,CAAT;AAAYb,QAAAA,IAAI,EAAEtE;AAAlB,OAAD,CAAP;AAAiC,KAAvE,EAAyEgE,MAAzE;AAAmF;AAC/H;AAEM,SAASgD,oBAAT,CAA8BC,MAA9B,EAAsCC,GAAtC,EAA2C;AAC9C,MAAIjP,MAAM,CAAC+K,cAAX,EAA2B;AAAE/K,IAAAA,MAAM,CAAC+K,cAAP,CAAsBiE,MAAtB,EAA8B,KAA9B,EAAqC;AAAEzO,MAAAA,KAAK,EAAE0O;AAAT,KAArC;AAAuD,GAApF,MAA0F;AAAED,IAAAA,MAAM,CAACC,GAAP,GAAaA,GAAb;AAAmB;;AAC/G,SAAOD,MAAP;AACH;AAEM,SAASE,YAAT,CAAsBC,GAAtB,EAA2B;AAC9B,MAAIA,GAAG,IAAIA,GAAG,CAACC,UAAf,EAA2B,OAAOD,GAAP;AAC3B,MAAI/C,MAAM,GAAG,EAAb;AACA,MAAI+C,GAAG,IAAI,IAAX,EAAiB,KAAK,IAAI5B,CAAT,IAAc4B,GAAd;AAAmB,QAAInP,MAAM,CAAC4G,cAAP,CAAsBlB,IAAtB,CAA2ByJ,GAA3B,EAAgC5B,CAAhC,CAAJ,EAAwCnB,MAAM,CAACmB,CAAD,CAAN,GAAY4B,GAAG,CAAC5B,CAAD,CAAf;AAA3D;AACjBnB,EAAAA,MAAM,WAAN,GAAiB+C,GAAjB;AACA,SAAO/C,MAAP;AACH;AAEM,SAASiD,eAAT,CAAyBF,GAAzB,EAA8B;AACjC,SAAQA,GAAG,IAAIA,GAAG,CAACC,UAAZ,GAA0BD,GAA1B,GAAgC;AAAE,eAASA;AAAX,GAAvC;AACH;AAEM,SAASG,sBAAT,CAAgCC,QAAhC,EAA0CC,UAA1C,EAAsD;AACzD,MAAI,CAACA,UAAU,CAACC,GAAX,CAAeF,QAAf,CAAL,EAA+B;AAC3B,UAAM,IAAInC,SAAJ,CAAc,gDAAd,CAAN;AACH;;AACD,SAAOoC,UAAU,CAACxI,GAAX,CAAeuI,QAAf,CAAP;AACH;AAEM,SAASG,sBAAT,CAAgCH,QAAhC,EAA0CC,UAA1C,EAAsDjP,KAAtD,EAA6D;AAChE,MAAI,CAACiP,UAAU,CAACC,GAAX,CAAeF,QAAf,CAAL,EAA+B;AAC3B,UAAM,IAAInC,SAAJ,CAAc,gDAAd,CAAN;AACH;;AACDoC,EAAAA,UAAU,CAACxM,GAAX,CAAeuM,QAAf,EAAyBhP,KAAzB;AACA,SAAOA,KAAP;AACH;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;ACzN6B;AAQ9B;AACa,iCAAyB,GAA0B,WAAwC,CAAC;AAUzG;AACA;IAA+BoP,uCAAyB;IAetD,mBAAY,GAAkB,EAAE,IAAa;QAA7C,iBAgBC;;;QAbC,IAAI,EAAE,KAAI,YAAY,SAAS,CAAC;YAAE,OAAO,IAAI,SAAS,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;QAElE,IAAI,WAAI,CAAC,MAAM,CAAC,GAAG,CAAC,EAAE;YACpB,QAAA,kBAAM,GAAG,CAAC,GAAG,EAAE,GAAG,CAAC,IAAI,EAAE,IAAI,CAAC,SAAC;SAChC;aAAM;YACL,QAAA,kBAAM,GAAG,EAAE,IAAI,EAAE,IAAI,CAAC,SAAC;SACxB;QACD,MAAM,CAAC,cAAc,CAAC,KAAI,EAAE,WAAW,EAAE;YACvC,KAAK,EAAE,WAAW;YAClB,QAAQ,EAAE,KAAK;YACf,YAAY,EAAE,KAAK;YACnB,UAAU,EAAE,KAAK;SAClB,CAAC,CAAC;;KACJ;IAED,0BAAM,GAAN;QACE,OAAO;YACL,UAAU,EAAE,IAAI,CAAC,QAAQ,EAAE;SAC5B,CAAC;KACH;;IAGM,iBAAO,GAAd,UAAe,KAAa;QAC1B,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,OAAO,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;KACjD;;IAGM,oBAAU,GAAjB,UAAkB,KAAa;QAC7B,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,UAAU,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;KACpD;;;;;;;IAQM,kBAAQ,GAAf,UAAgB,OAAe,EAAE,QAAgB;QAC/C,OAAO,IAAI,SAAS,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;KACzC;;;;;;;IAQM,oBAAU,GAAjB,UAAkB,GAAW,EAAE,QAAgB;QAC7C,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,UAAU,CAAC,GAAG,EAAE,IAAI,EAAE,QAAQ,CAAC,CAAC,CAAC;KAC5D;;IAGD,kCAAc,GAAd;QACE,OAAO,EAAE,UAAU,EAAE,EAAE,CAAC,EAAE,IAAI,CAAC,IAAI,KAAK,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,GAAG,KAAK,CAAC,EAAE,EAAE,CAAC;KAClE;;IAGM,0BAAgB,GAAvB,UAAwB,GAAsB;QAC5C,OAAO,IAAI,SAAS,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC,EAAE,GAAG,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;KAC1D;;IAGD,oBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;KACvB;IAED,2BAAO,GAAP;QACE,OAAO,mBAAiB,IAAI,CAAC,UAAU,EAAE,CAAC,QAAQ,EAAE,UAAK,IAAI,CAAC,WAAW,EAAE,CAAC,QAAQ,EAAE,MAAG,CAAC;KAC3F;IAnFe,mBAAS,GAAG,WAAI,CAAC,kBAAkB,CAAC;IAoFtD,gBAAC;CAAA,CAvF8B,iCAAyB,GAuFvD;AAvFY,8BAAS;;;;;;;;;;;ACpBY;AAEJ;AACgB;AACJ;AACR;AACD;AACH;AACK;AACA;AACG;AAC0B;AAC1B;AACA;AACE;AAqBxC,SAAgB,UAAU,CAAC,KAAc;IACvC,QACEvG,kBAAY,CAAC,KAAK,CAAC,IAAI,OAAO,CAAC,GAAG,CAAC,KAAK,EAAE,WAAW,CAAC,IAAI,OAAO,KAAK,CAAC,SAAS,KAAK,QAAQ,EAC7F;AACJ,CAAC;AAJD,gCAIC;AAED;AACA,IAAM,cAAc,GAAG,UAAU,CAAC;AAClC,IAAM,cAAc,GAAG,CAAC,UAAU,CAAC;AACnC;AACA,IAAM,cAAc,GAAG,kBAAkB,CAAC;AAC1C,IAAM,cAAc,GAAG,CAAC,kBAAkB,CAAC;AAE3C;AACA;AACA,IAAM,YAAY,GAAG;IACnB,IAAI,EAAEwG,iBAAQ;IACd,OAAO,EAAErG,aAAM;IACf,KAAK,EAAEA,aAAM;IACb,OAAO,EAAEsG,iBAAU;IACnB,UAAU,EAAEC,YAAK;IACjB,cAAc,EAAEC,qBAAU;IAC1B,aAAa,EAAE,eAAM;IACrB,WAAW,EAAE,WAAI;IACjB,OAAO,EAAEC,cAAM;IACf,OAAO,EAAEC,cAAM;IACf,MAAM,EAAEC,iBAAU;IAClB,kBAAkB,EAAEA,iBAAU;IAC9B,UAAU,EAAEC,mBAAS;CACb,CAAC;AAEX;AACA,SAAS,gBAAgB,CAAC,KAAU,EAAE,OAA2B;IAA3B,wBAAA,EAAA,YAA2B;IAC/D,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;QAC7B,IAAI,OAAO,CAAC,OAAO,IAAI,OAAO,CAAC,MAAM,EAAE;YACrC,OAAO,KAAK,CAAC;SACd;;;QAID,IAAI,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK,EAAE;YAC/B,IAAI,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc;gBAAE,OAAO,IAAIL,YAAK,CAAC,KAAK,CAAC,CAAC;YAChF,IAAI,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc;gBAAE,OAAO,WAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC;SACvF;;QAGD,OAAO,IAAI,eAAM,CAAC,KAAK,CAAC,CAAC;KAC1B;;IAGD,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ;QAAE,OAAO,KAAK,CAAC;;IAG7D,IAAI,KAAK,CAAC,UAAU;QAAE,OAAO,IAAI,CAAC;IAElC,IAAM,IAAI,GAAG,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,MAAM,CACpC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,IAAI,GAAA,CACV,CAAC;IACnC,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;QACpC,IAAM,CAAC,GAAG,YAAY,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,CAAC;QAChC,IAAI,CAAC;YAAE,OAAO,CAAC,CAAC,gBAAgB,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;KAClD;IAED,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,EAAE;QACvB,IAAM,CAAC,GAAG,KAAK,CAAC,KAAK,CAAC;QACtB,IAAM,IAAI,GAAG,IAAI,IAAI,EAAE,CAAC;QAExB,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,IAAI,OAAO,CAAC,KAAK,QAAQ;gBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;iBACtC,IAAI,OAAO,CAAC,KAAK,QAAQ;gBAAE,IAAI,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;SAC7D;aAAM;YACL,IAAI,OAAO,CAAC,KAAK,QAAQ;gBAAE,IAAI,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;iBAClD,IAAI,WAAI,CAAC,MAAM,CAAC,CAAC,CAAC;gBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,QAAQ,EAAE,CAAC,CAAC;iBAC/C,IAAI,OAAO,CAAC,KAAK,QAAQ,IAAI,OAAO,CAAC,OAAO;gBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;SACpE;QACD,OAAO,IAAI,CAAC;KACb;IAED,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,EAAE;QACvB,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,KAAK,CAAC,CAAC;QACtC,IAAI,KAAK,CAAC,MAAM,EAAE;YAChB,IAAI,CAAC,MAAM,GAAG,gBAAgB,CAAC,KAAK,CAAC,MAAM,CAAC,CAAC;SAC9C;QAED,OAAOM,SAAI,CAAC,gBAAgB,CAAC,KAAK,CAAC,CAAC;KACrC;IAED,IAAIC,kBAAW,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,UAAU,EAAE;QAC1C,IAAM,CAAC,GAAG,KAAK,CAAC,IAAI,GAAG,KAAK,GAAG,KAAK,CAAC,UAAU,CAAC;;;QAIhD,IAAI,CAAC,YAAYA,YAAK;YAAE,OAAO,CAAC,CAAC;QAEjC,IAAM,UAAU,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,GAAA,CAAC,CAAC;QACjE,IAAI,OAAK,GAAG,IAAI,CAAC;QACjB,UAAU,CAAC,OAAO,CAAC,UAAA,CAAC;YAClB,IAAI,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC;gBAAE,OAAK,GAAG,KAAK,CAAC;SAC7D,CAAC,CAAC;;QAGH,IAAI,OAAK;YAAE,OAAOA,YAAK,CAAC,gBAAgB,CAAC,CAAC,CAAC,CAAC;KAC7C;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAMD;AACA,SAAS,cAAc,CAAC,KAAY,EAAE,OAA8B;IAClE,OAAO,KAAK,CAAC,GAAG,CAAC,UAAC,CAAU,EAAE,KAAa;QACzC,OAAO,CAAC,WAAW,CAAC,IAAI,CAAC,EAAE,YAAY,EAAE,WAAS,KAAO,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC,CAAC;QACxE,IAAI;YACF,OAAO,cAAc,CAAC,CAAC,EAAE,OAAO,CAAC,CAAC;SACnC;gBAAS;YACR,OAAO,CAAC,WAAW,CAAC,GAAG,EAAE,CAAC;SAC3B;KACF,CAAC,CAAC;AACL,CAAC;AAED,SAAS,YAAY,CAAC,IAAU;IAC9B,IAAM,MAAM,GAAG,IAAI,CAAC,WAAW,EAAE,CAAC;;IAElC,OAAO,IAAI,CAAC,kBAAkB,EAAE,KAAK,CAAC,GAAG,MAAM,GAAG,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;AAC9E,CAAC;AAED;AACA,SAAS,cAAc,CAAC,KAAU,EAAE,OAA8B;IAChE,IAAI,CAAC,OAAO,KAAK,KAAK,QAAQ,IAAI,OAAO,KAAK,KAAK,UAAU,KAAK,KAAK,KAAK,IAAI,EAAE;QAChF,IAAM,KAAK,GAAG,OAAO,CAAC,WAAW,CAAC,SAAS,CAAC,UAAA,KAAK,IAAI,OAAA,KAAK,CAAC,GAAG,KAAK,KAAK,GAAA,CAAC,CAAC;QAC1E,IAAI,KAAK,KAAK,CAAC,CAAC,EAAE;YAChB,IAAM,KAAK,GAAG,OAAO,CAAC,WAAW,CAAC,GAAG,CAAC,UAAA,KAAK,IAAI,OAAA,KAAK,CAAC,YAAY,GAAA,CAAC,CAAC;YACnE,IAAM,WAAW,GAAG,KAAK;iBACtB,KAAK,CAAC,CAAC,EAAE,KAAK,CAAC;iBACf,GAAG,CAAC,UAAA,IAAI,IAAI,OAAG,IAAI,SAAM,GAAA,CAAC;iBAC1B,IAAI,CAAC,EAAE,CAAC,CAAC;YACZ,IAAM,WAAW,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC;YACjC,IAAM,YAAY,GAChB,MAAM;gBACN,KAAK;qBACF,KAAK,CAAC,KAAK,GAAG,CAAC,EAAE,KAAK,CAAC,MAAM,GAAG,CAAC,CAAC;qBAClC,GAAG,CAAC,UAAA,IAAI,IAAI,OAAG,IAAI,SAAM,GAAA,CAAC;qBAC1B,IAAI,CAAC,EAAE,CAAC,CAAC;YACd,IAAM,OAAO,GAAG,KAAK,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;YACxC,IAAM,YAAY,GAAG,GAAG,CAAC,MAAM,CAAC,WAAW,CAAC,MAAM,GAAG,WAAW,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;YAC7E,IAAM,MAAM,GAAG,GAAG,CAAC,MAAM,CACvB,YAAY,CAAC,MAAM,GAAG,CAAC,WAAW,CAAC,MAAM,GAAG,OAAO,CAAC,MAAM,IAAI,CAAC,GAAG,CAAC,CACpE,CAAC;YAEF,MAAM,IAAI,SAAS,CACjB,2CAA2C;iBACzC,SAAO,WAAW,GAAG,WAAW,GAAG,YAAY,GAAG,OAAO,OAAI,CAAA;iBAC7D,SAAO,YAAY,UAAK,MAAM,MAAG,CAAA,CACpC,CAAC;SACH;QACD,OAAO,CAAC,WAAW,CAAC,OAAO,CAAC,WAAW,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC,GAAG,GAAG,KAAK,CAAC;KACjE;IAED,IAAI,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC;QAAE,OAAO,cAAc,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;IAEhE,IAAI,KAAK,KAAK,SAAS;QAAE,OAAO,IAAI,CAAC;IAErC,IAAI,KAAK,YAAY,IAAI,IAAIjH,YAAM,CAAC,KAAK,CAAC,EAAE;QAC1C,IAAM,OAAO,GAAG,KAAK,CAAC,OAAO,EAAE;;QAE7B,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC,IAAI,OAAO,GAAG,eAAe,CAAC;QAEtD,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO,OAAO,CAAC,OAAO,IAAI,OAAO;kBAC7B,EAAE,KAAK,EAAE,KAAK,CAAC,OAAO,EAAE,EAAE;kBAC1B,EAAE,KAAK,EAAE,YAAY,CAAC,KAAK,CAAC,EAAE,CAAC;SACpC;QACD,OAAO,OAAO,CAAC,OAAO,IAAI,OAAO;cAC7B,EAAE,KAAK,EAAE,YAAY,CAAC,KAAK,CAAC,EAAE;cAC9B,EAAE,KAAK,EAAE,EAAE,WAAW,EAAE,KAAK,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE,EAAE,EAAE,CAAC;KAC5D;IAED,IAAI,OAAO,KAAK,KAAK,QAAQ,KAAK,CAAC,OAAO,CAAC,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC,EAAE;;QAEvE,IAAI,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK,EAAE;YAC/B,IAAM,UAAU,GAAG,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc,EACnE,UAAU,GAAG,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc,CAAC;;YAGlE,IAAI,UAAU;gBAAE,OAAO,EAAE,UAAU,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;YACxD,IAAI,UAAU;gBAAE,OAAO,EAAE,WAAW,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;SAC1D;QACD,OAAO,EAAE,aAAa,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;KAC5C;IAED,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;QAC9C,IAAI,KAAK,GAAG,KAAK,CAAC,KAAK,CAAC;QACxB,IAAI,KAAK,KAAK,SAAS,EAAE;YACvB,IAAM,KAAK,GAAG,KAAK,CAAC,QAAQ,EAAE,CAAC,KAAK,CAAC,WAAW,CAAC,CAAC;YAClD,IAAI,KAAK,EAAE;gBACT,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;aAClB;SACF;QAED,IAAM,EAAE,GAAG,IAAI8G,iBAAU,CAAC,KAAK,CAAC,MAAM,EAAE,KAAK,CAAC,CAAC;QAC/C,OAAO,EAAE,CAAC,cAAc,CAAC,OAAO,CAAC,CAAC;KACnC;IAED,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ;QAAE,OAAO,iBAAiB,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;IACzF,OAAO,KAAK,CAAC;AACf,CAAC;AAED,IAAM,kBAAkB,GAAG;IACzB,MAAM,EAAE,UAAC,CAAS,IAAK,OAAA,IAAI3G,aAAM,CAAC,CAAC,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,QAAQ,CAAC,GAAA;IACxD,IAAI,EAAE,UAAC,CAAO,IAAK,OAAA,IAAI6G,SAAI,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC,KAAK,CAAC,GAAA;IAC5C,KAAK,EAAE,UAAC,CAAQ,IAAK,OAAA,IAAIC,YAAK,CAAC,CAAC,CAAC,UAAU,IAAI,CAAC,CAAC,SAAS,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,MAAM,CAAC,GAAA;IAClF,UAAU,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIN,qBAAU,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;IACtD,MAAM,EAAE,UAAC,CAAS,IAAK,OAAA,IAAI,eAAM,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;IAC1C,KAAK,EAAE,UAAC,CAAQ,IAAK,OAAA,IAAID,YAAK,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;IACvC,IAAI,EAAE,UACJ,CAIC;QAED,OAAA,WAAI,CAAC,QAAQ;;QAEX,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,GAAG,GAAG,CAAC,CAAC,IAAI,EAC9B,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,CAAC,KAAK,EAChC,CAAC,CAAC,GAAG,IAAI,IAAI,GAAG,CAAC,CAAC,QAAQ,GAAG,CAAC,CAAC,SAAS,CACzC;KAAA;IACH,MAAM,EAAE,cAAM,OAAA,IAAIG,cAAM,EAAE,GAAA;IAC1B,MAAM,EAAE,cAAM,OAAA,IAAID,cAAM,EAAE,GAAA;IAC1B,QAAQ,EAAE,UAAC,CAAW,IAAK,OAAA,IAAIJ,iBAAQ,CAAC,CAAC,CAAC,GAAA;IAC1C,QAAQ,EAAE,UAAC,CAAW,IAAK,OAAA,IAAIA,iBAAQ,CAAC,CAAC,CAAC,GAAA;IAC1C,UAAU,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIM,iBAAU,CAAC,CAAC,CAAC,OAAO,EAAE,CAAC,CAAC,OAAO,CAAC,GAAA;IACnE,MAAM,EAAE,UAAC,CAAa,IAAK,OAAA,IAAIL,iBAAU,CAAC,CAAC,CAAC,KAAK,CAAC,GAAA;IAClD,SAAS,EAAE,UAAC,CAAY,IAAK,OAAAM,mBAAS,CAAC,QAAQ,CAAC,CAAC,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,GAAA;CACtD,CAAC;AAEX;AACA,SAAS,iBAAiB,CAAC,GAAQ,EAAE,OAA8B;IACjE,IAAI,GAAG,IAAI,IAAI,IAAI,OAAO,GAAG,KAAK,QAAQ;QAAE,MAAM,IAAI,KAAK,CAAC,wBAAwB,CAAC,CAAC;IAEtF,IAAM,QAAQ,GAA0B,GAAG,CAAC,SAAS,CAAC;IACtD,IAAI,OAAO,QAAQ,KAAK,WAAW,EAAE;;QAEnC,IAAM,IAAI,GAAa,EAAE,CAAC;QAC1B,KAAK,IAAM,IAAI,IAAI,GAAG,EAAE;YACtB,OAAO,CAAC,WAAW,CAAC,IAAI,CAAC,EAAE,YAAY,EAAE,IAAI,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC,CAAC;YAC5D,IAAI;gBACF,IAAI,CAAC,IAAI,CAAC,GAAG,cAAc,CAAC,GAAG,CAAC,IAAI,CAAC,EAAE,OAAO,CAAC,CAAC;aACjD;oBAAS;gBACR,OAAO,CAAC,WAAW,CAAC,GAAG,EAAE,CAAC;aAC3B;SACF;QACD,OAAO,IAAI,CAAC;KACb;SAAM,IAAI,UAAU,CAAC,GAAG,CAAC,EAAE;;;QAG1B,IAAI,MAAM,GAAQ,GAAG,CAAC;QACtB,IAAI,OAAO,MAAM,CAAC,cAAc,KAAK,UAAU,EAAE;;;;;YAK/C,IAAM,MAAM,GAAG,kBAAkB,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;YACjD,IAAI,CAAC,MAAM,EAAE;gBACX,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,GAAG,CAAC,SAAS,CAAC,CAAC;aAC5E;YACD,MAAM,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC;SACzB;;QAGD,IAAI,QAAQ,KAAK,MAAM,IAAI,MAAM,CAAC,KAAK,EAAE;YACvC,MAAM,GAAG,IAAIC,SAAI,CAAC,MAAM,CAAC,IAAI,EAAE,cAAc,CAAC,MAAM,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC,CAAC;SACvE;aAAM,IAAI,QAAQ,KAAK,OAAO,IAAI,MAAM,CAAC,GAAG,EAAE;YAC7C,MAAM,GAAG,IAAIC,YAAK,CAChB,cAAc,CAAC,MAAM,CAAC,UAAU,EAAE,OAAO,CAAC,EAC1C,cAAc,CAAC,MAAM,CAAC,GAAG,EAAE,OAAO,CAAC,EACnC,cAAc,CAAC,MAAM,CAAC,EAAE,EAAE,OAAO,CAAC,EAClC,cAAc,CAAC,MAAM,CAAC,MAAM,EAAE,OAAO,CAAC,CACvC,CAAC;SACH;QAED,OAAO,MAAM,CAAC,cAAc,CAAC,OAAO,CAAC,CAAC;KACvC;SAAM;QACL,MAAM,IAAI,KAAK,CAAC,uCAAuC,GAAG,OAAO,QAAQ,CAAC,CAAC;KAC5E;AACH,CAAC;AASD,WAAiB,KAAK;;;;;;;;;;;;;;;;;IA6BpB,SAAgB,KAAK,CAAC,IAAY,EAAE,OAAuB;QACzD,IAAM,YAAY,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,EAAE,OAAO,EAAE,IAAI,EAAE,MAAM,EAAE,KAAK,EAAE,EAAE,OAAO,CAAC,CAAC;;QAGlF,IAAI,OAAO,YAAY,CAAC,OAAO,KAAK,SAAS;YAAE,YAAY,CAAC,MAAM,GAAG,CAAC,YAAY,CAAC,OAAO,CAAC;QAC3F,IAAI,OAAO,YAAY,CAAC,MAAM,KAAK,SAAS;YAAE,YAAY,CAAC,OAAO,GAAG,CAAC,YAAY,CAAC,MAAM,CAAC;QAE1F,OAAO,IAAI,CAAC,KAAK,CAAC,IAAI,EAAE,UAAC,IAAI,EAAE,KAAK,IAAK,OAAA,gBAAgB,CAAC,KAAK,EAAE,YAAY,CAAC,GAAA,CAAC,CAAC;KACjF;IARe,WAAK,QAQpB,CAAA;;;;;;;;;;;;;;;;;;;;;;;;IA4BD,SAAgB,SAAS,CACvB,KAAwB;;IAExB,QAA8F,EAC9F,KAAuB,EACvB,OAAuB;QAEvB,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YAC9C,OAAO,GAAG,KAAK,CAAC;YAChB,KAAK,GAAG,CAAC,CAAC;SACX;QACD,IAAI,QAAQ,IAAI,IAAI,IAAI,OAAO,QAAQ,KAAK,QAAQ,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,QAAQ,CAAC,EAAE;YAChF,OAAO,GAAG,QAAQ,CAAC;YACnB,QAAQ,GAAG,SAAS,CAAC;YACrB,KAAK,GAAG,CAAC,CAAC;SACX;QACD,IAAM,gBAAgB,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,OAAO,EAAE,IAAI,EAAE,MAAM,EAAE,KAAK,EAAE,EAAE,OAAO,EAAE;YAChF,WAAW,EAAE,CAAC,EAAE,YAAY,EAAE,QAAQ,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;SACrD,CAAC,CAAC;QAEH,IAAM,GAAG,GAAG,cAAc,CAAC,KAAK,EAAE,gBAAgB,CAAC,CAAC;QACpD,OAAO,IAAI,CAAC,SAAS,CAAC,GAAG,EAAE,QAA4C,EAAE,KAAK,CAAC,CAAC;KACjF;IAtBe,eAAS,YAsBxB,CAAA;;;;;;;IAQD,SAAgB,SAAS,CAAC,KAAwB,EAAE,OAAuB;QACzE,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,OAAO,IAAI,CAAC,KAAK,CAAC,SAAS,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC,CAAC;KAC9C;IAHe,eAAS,YAGxB,CAAA;;;;;;;IAQD,SAAgB,WAAW,CAAC,KAAe,EAAE,OAAuB;QAClE,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,OAAO,KAAK,CAAC,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,EAAE,OAAO,CAAC,CAAC;KAC9C;IAHe,iBAAW,cAG1B,CAAA;AACH,CAAC,EA9GgB,aAAK,KAAL,aAAK,QA8GrB;;;;;;;;;AC1bD;AACA;;;AAOA;AACA,IAAI,OAAuB,CAAC;AAiIR,sBAAG;AA/HvB,IAAM,KAAK,GAAG,UAAU,eAAoB;;IAE1C,OAAO,eAAe,IAAI,eAAe,CAAC,IAAI,IAAI,IAAI,IAAI,eAAe,CAAC;AAC5E,CAAC,CAAC;AAEF;AACA,SAAS,SAAS;;IAEhB,QACE,KAAK,CAAC,OAAO,UAAU,KAAK,QAAQ,IAAI,UAAU,CAAC;QACnD,KAAK,CAAC,OAAO,MAAM,KAAK,QAAQ,IAAI,MAAM,CAAC;QAC3C,KAAK,CAAC,OAAO,IAAI,KAAK,QAAQ,IAAI,IAAI,CAAC;QACvC,KAAK,CAAC,OAAO9Q,cAAM,KAAK,QAAQ,IAAIA,cAAM,CAAC;QAC3C,QAAQ,CAAC,aAAa,CAAC,EAAE,EACzB;AACJ,CAAC;AAED,IAAM,UAAU,GAAG,SAAS,EAAE,CAAC;AAC/B,IAAI,MAAM,CAAC,SAAS,CAAC,cAAc,CAAC,IAAI,CAAC,UAAU,EAAE,KAAK,CAAC,EAAE;IAC3D,cAAA,OAAO,GAAG,UAAU,CAAC,GAAG,CAAC;CAC1B;KAAM;;IAEL,cAAA,OAAO;QAGL,aAAY,KAA2B;YAA3B,sBAAA,EAAA,UAA2B;YACrC,IAAI,CAAC,KAAK,GAAG,EAAE,CAAC;YAChB,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC;YAElB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;gBACrC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,IAAI;oBAAE,SAAS;gBAC/B,IAAM,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;gBACvB,IAAM,GAAG,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;gBACrB,IAAM,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;;gBAEvB,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;;gBAGrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,EAAE,CAAC;aAC5D;SACF;QACD,mBAAK,GAAL;YACE,IAAI,CAAC,KAAK,GAAG,EAAE,CAAC;YAChB,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC;SACnB;QACD,oBAAM,GAAN,UAAO,GAAW;YAChB,IAAM,KAAK,GAAG,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC;YAChC,IAAI,KAAK,IAAI,IAAI;gBAAE,OAAO,KAAK,CAAC;;YAEhC,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC;;YAEzB,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;YAC9B,OAAO,IAAI,CAAC;SACb;QACD,qBAAO,GAAP;YAAA,iBAYC;YAXC,IAAI,KAAK,GAAG,CAAC,CAAC;YAEd,OAAO;gBACL,IAAI,EAAE;oBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;oBAChC,OAAO;wBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,CAAC,GAAG,EAAE,KAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,GAAG,SAAS;wBACjE,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;qBACvC,CAAC;iBACH;aACF,CAAC;SACH;QACD,qBAAO,GAAP,UAAQ,QAAmE,EAAE,IAAW;YACtF,IAAI,GAAG,IAAI,IAAI,IAAI,CAAC;YAEpB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;gBAC1C,IAAM,GAAG,GAAG,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;;gBAE1B,QAAQ,CAAC,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,EAAE,GAAG,EAAE,IAAI,CAAC,CAAC;aACrD;SACF;QACD,iBAAG,GAAH,UAAI,GAAW;YACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,SAAS,CAAC;SAC5D;QACD,iBAAG,GAAH,UAAI,GAAW;YACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC;SAClC;QACD,kBAAI,GAAJ;YAAA,iBAYC;YAXC,IAAI,KAAK,GAAG,CAAC,CAAC;YAEd,OAAO;gBACL,IAAI,EAAE;oBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;oBAChC,OAAO;wBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,GAAG,GAAG,SAAS;wBAC1C,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;qBACvC,CAAC;iBACH;aACF,CAAC;SACH;QACD,iBAAG,GAAH,UAAI,GAAW,EAAE,KAAU;YACzB,IAAI,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;gBACrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,KAAK,CAAC;gBAC5B,OAAO,IAAI,CAAC;aACb;;YAGD,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;;;YAGrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,EAAE,CAAC;YAC3D,OAAO,IAAI,CAAC;SACb;QACD,oBAAM,GAAN;YAAA,iBAYC;YAXC,IAAI,KAAK,GAAG,CAAC,CAAC;YAEd,OAAO;gBACL,IAAI,EAAE;oBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;oBAChC,OAAO;wBACL,KAAK,EAAE,GAAG,KAAK,SAAS,GAAG,KAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,SAAS;wBAC1D,IAAI,EAAE,GAAG,KAAK,SAAS,GAAG,KAAK,GAAG,IAAI;qBACvC,CAAC;iBACH;aACF,CAAC;SACH;QACD,sBAAI,qBAAI;iBAAR;gBACE,OAAO,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC;aAC1B;;;WAAA;QACH,UAAC;KAtGU,GAsGoB,CAAC;CACjC;;;;;;;;;;ACxID;AACa,sBAAc,GAAG,UAAU,CAAC;AACzC;AACa,sBAAc,GAAG,CAAC,UAAU,CAAC;AAC1C;AACa,sBAAc,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;AAClD;AACa,sBAAc,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;AAE/C;;;;AAIa,kBAAU,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;AAE1C;;;;AAIa,kBAAU,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;AAE3C;AACa,wBAAgB,GAAG,CAAC,CAAC;AAElC;AACa,wBAAgB,GAAG,CAAC,CAAC;AAElC;AACa,wBAAgB,GAAG,CAAC,CAAC;AAElC;AACa,uBAAe,GAAG,CAAC,CAAC;AAEjC;AACa,wBAAgB,GAAG,CAAC,CAAC;AAElC;AACa,2BAAmB,GAAG,CAAC,CAAC;AAErC;AACa,qBAAa,GAAG,CAAC,CAAC;AAE/B;AACa,yBAAiB,GAAG,CAAC,CAAC;AAEnC;AACa,sBAAc,GAAG,CAAC,CAAC;AAEhC;AACa,sBAAc,GAAG,EAAE,CAAC;AAEjC;AACa,wBAAgB,GAAG,EAAE,CAAC;AAEnC;AACa,2BAAmB,GAAG,EAAE,CAAC;AAEtC;AACa,sBAAc,GAAG,EAAE,CAAC;AAEjC;AACa,wBAAgB,GAAG,EAAE,CAAC;AAEnC;AACa,8BAAsB,GAAG,EAAE,CAAC;AAEzC;AACa,qBAAa,GAAG,EAAE,CAAC;AAEhC;AACa,2BAAmB,GAAG,EAAE,CAAC;AAEtC;AACa,sBAAc,GAAG,EAAE,CAAC;AAEjC;AACa,4BAAoB,GAAG,EAAE,CAAC;AAEvC;AACa,yBAAiB,GAAG,IAAI,CAAC;AAEtC;AACa,yBAAiB,GAAG,IAAI,CAAC;AAEtC;AACa,mCAA2B,GAAG,CAAC,CAAC;AAE7C;AACa,oCAA4B,GAAG,CAAC,CAAC;AAE9C;AACa,sCAA8B,GAAG,CAAC,CAAC;AAEhD;AACa,gCAAwB,GAAG,CAAC,CAAC;AAE1C;AACa,oCAA4B,GAAG,CAAC,CAAC;AAE9C;AACa,+BAAuB,GAAG,CAAC,CAAC;AAEzC;AACa,wCAAgC,GAAG,GAAG,CAAC;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;ACvGpB;AACG;AAEO;AAC6C;AAEvF,SAAgB,mBAAmB,CACjC,MAAgB,EAChB,kBAA4B,EAC5B,eAAyB;IAEzB,IAAI,WAAW,GAAG,CAAC,GAAG,CAAC,CAAC;IAExB,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;QACzB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;YACtC,WAAW,IAAI,gBAAgB,CAC7B,CAAC,CAAC,QAAQ,EAAE,EACZ,MAAM,CAAC,CAAC,CAAC,EACT,kBAAkB,EAClB,IAAI,EACJ,eAAe,CAChB,CAAC;SACH;KACF;SAAM;;QAGL,IAAI,MAAM,CAAC,MAAM,EAAE;YACjB,MAAM,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC;SAC1B;;QAGD,KAAK,IAAM,GAAG,IAAI,MAAM,EAAE;YACxB,WAAW,IAAI,gBAAgB,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,CAAC,EAAE,kBAAkB,EAAE,KAAK,EAAE,eAAe,CAAC,CAAC;SAC/F;KACF;IAED,OAAO,WAAW,CAAC;AACrB,CAAC;AA/BD,kDA+BC;AAED;AACA,SAAS,gBAAgB,CACvB,IAAY;AACZ;AACA,KAAU,EACV,kBAA0B,EAC1B,OAAe,EACf,eAAuB;IAFvB,mCAAA,EAAA,0BAA0B;IAC1B,wBAAA,EAAA,eAAe;IACf,gCAAA,EAAA,uBAAuB;;IAGvB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;QACzB,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;KACxB;IAED,QAAQ,OAAO,KAAK;QAClB,KAAK,QAAQ;YACX,OAAO,CAAC,GAAG4J,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,GAAGA,aAAM,CAAC,UAAU,CAAC,KAAK,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;QAC5F,KAAK,QAAQ;YACX,IACE,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK;gBAC3B,KAAK,IAAI,SAAS,CAAC,UAAU;gBAC7B,KAAK,IAAI,SAAS,CAAC,UAAU,EAC7B;gBACA,IAAI,KAAK,IAAI,SAAS,CAAC,cAAc,IAAI,KAAK,IAAI,SAAS,CAAC,cAAc,EAAE;;oBAE1E,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;iBAC3E;qBAAM;oBACL,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;iBAC3E;aACF;iBAAM;;gBAEL,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;aAC3E;QACH,KAAK,WAAW;YACd,IAAI,OAAO,IAAI,CAAC,eAAe;gBAC7B,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;YACtE,OAAO,CAAC,CAAC;QACX,KAAK,SAAS;YACZ,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;QAC5E,KAAK,QAAQ;YACX,IAAI,KAAK,IAAI,IAAI,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBACvF,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;aACrE;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,CAAC,CAAC;aAC5E;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAIC,YAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;aAC3E;iBAAM,IACL,WAAW,CAAC,MAAM,CAAC,KAAK,CAAC;gBACzB,KAAK,YAAY,WAAW;gBAC5BC,sBAAgB,CAAC,KAAK,CAAC,EACvB;gBACA,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,KAAK,CAAC,UAAU,EACzF;aACH;iBAAM,IACL,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM;gBAC7B,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ;gBAC/B,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAClC;gBACA,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;aAC3E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,OAAO,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,CAAC,CAAC;aAC5E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;;gBAExC,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,MAAM,GAAG,CAAC,EAAE;oBAC9D,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;wBACvD,CAAC;wBACD,CAAC;wBACD,CAAC;wBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,EAAE,MAAM,CAAC;wBAChD,CAAC;wBACD,mBAAmB,CAAC,KAAK,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACrE;iBACH;qBAAM;oBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;wBACvD,CAAC;wBACD,CAAC;wBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,EAAE,MAAM,CAAC;wBAChD,CAAC,EACD;iBACH;aACF;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;;gBAE1C,IAAI,KAAK,CAAC,QAAQ,KAAKI,aAAM,CAAC,kBAAkB,EAAE;oBAChD,QACE,CAAC,IAAI,IAAI,IAAI,GAAGJ,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;yBACtD,KAAK,CAAC,QAAQ,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EAChC;iBACH;qBAAM;oBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,KAAK,KAAK,CAAC,QAAQ,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,EACvF;iBACH;aACF;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,KAAK,EAAE,MAAM,CAAC;oBACtC,CAAC;oBACD,CAAC;oBACD,CAAC,EACD;aACH;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;;gBAEzC,IAAM,cAAc,GAAG,MAAM,CAAC,MAAM,CAClC;oBACE,IAAI,EAAE,KAAK,CAAC,UAAU;oBACtB,GAAG,EAAE,KAAK,CAAC,GAAG;iBACf,EACD,KAAK,CAAC,MAAM,CACb,CAAC;;gBAGF,IAAI,KAAK,CAAC,EAAE,IAAI,IAAI,EAAE;oBACpB,cAAc,CAAC,KAAK,CAAC,GAAG,KAAK,CAAC,EAAE,CAAC;iBAClC;gBAED,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvD,CAAC;oBACD,mBAAmB,CAAC,cAAc,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACxE;aACH;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAIC,cAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvD,CAAC;oBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,MAAM,EAAE,MAAM,CAAC;oBACvC,CAAC;qBACA,KAAK,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;qBACrB,KAAK,CAAC,UAAU,GAAG,CAAC,GAAG,CAAC,CAAC;qBACzB,KAAK,CAAC,SAAS,GAAG,CAAC,GAAG,CAAC,CAAC;oBACzB,CAAC,EACD;aACH;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvD,CAAC;oBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,MAAM,CAAC;oBACxC,CAAC;oBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,MAAM,CAAC;oBACxC,CAAC,EACD;aACH;iBAAM;gBACL,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvD,mBAAmB,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC;oBAC/D,CAAC,EACD;aACH;QACH,KAAK,UAAU;;YAEb,IAAI,KAAK,YAAY,MAAM,IAAIC,cAAQ,CAAC,KAAK,CAAC,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,iBAAiB,EAAE;gBAC1F,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;oBACvD,CAAC;oBACDA,aAAM,CAAC,UAAU,CAAC,KAAK,CAAC,MAAM,EAAE,MAAM,CAAC;oBACvC,CAAC;qBACA,KAAK,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;qBACrB,KAAK,CAAC,UAAU,GAAG,CAAC,GAAG,CAAC,CAAC;qBACzB,KAAK,CAAC,SAAS,GAAG,CAAC,GAAG,CAAC,CAAC;oBACzB,CAAC,EACD;aACH;iBAAM;gBACL,IAAI,kBAAkB,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,MAAM,GAAG,CAAC,EAAE;oBACpF,QACE,CAAC,IAAI,IAAI,IAAI,GAAGA,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;wBACvD,CAAC;wBACD,CAAC;wBACD,CAAC;wBACDA,aAAM,CAAC,UAAU,CAACC,8BAAwB,CAAC,KAAK,CAAC,EAAE,MAAM,CAAC;wBAC1D,CAAC;wBACD,mBAAmB,CAAC,KAAK,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC,EACrE;iBACH;qBAAM,IAAI,kBAAkB,EAAE;oBAC7B,QACE,CAAC,IAAI,IAAI,IAAI,GAAGD,aAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;wBACvD,CAAC;wBACD,CAAC;wBACDA,aAAM,CAAC,UAAU,CAACC,8BAAwB,CAAC,KAAK,CAAC,EAAE,MAAM,CAAC;wBAC1D,CAAC,EACD;iBACH;aACF;KACJ;IAED,OAAO,CAAC,CAAC;AACX,CAAC;;;;;;;;;;AClOD,IAAM,SAAS,GAAG,IAAI,CAAC;AACvB,IAAM,cAAc,GAAG,IAAI,CAAC;AAC5B,IAAM,gBAAgB,GAAG,IAAI,CAAC;AAC9B,IAAM,eAAe,GAAG,IAAI,CAAC;AAC7B,IAAM,eAAe,GAAG,IAAI,CAAC;AAE7B,IAAM,YAAY,GAAG,IAAI,CAAC;AAC1B,IAAM,cAAc,GAAG,IAAI,CAAC;AAC5B,IAAM,aAAa,GAAG,IAAI,CAAC;AAC3B,IAAM,eAAe,GAAG,IAAI,CAAC;AAE7B;;;;;;AAMA,SAAgB,YAAY,CAC1B,KAAkC,EAClC,KAAa,EACb,GAAW;IAEX,IAAI,YAAY,GAAG,CAAC,CAAC;IAErB,KAAK,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC,GAAG,GAAG,EAAE,CAAC,IAAI,CAAC,EAAE;QACnC,IAAM,IAAI,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;QAEtB,IAAI,YAAY,EAAE;YAChB,IAAI,CAAC,IAAI,GAAG,cAAc,MAAM,eAAe,EAAE;gBAC/C,OAAO,KAAK,CAAC;aACd;YACD,YAAY,IAAI,CAAC,CAAC;SACnB;aAAM,IAAI,IAAI,GAAG,SAAS,EAAE;YAC3B,IAAI,CAAC,IAAI,GAAG,gBAAgB,MAAM,YAAY,EAAE;gBAC9C,YAAY,GAAG,CAAC,CAAC;aAClB;iBAAM,IAAI,CAAC,IAAI,GAAG,eAAe,MAAM,cAAc,EAAE;gBACtD,YAAY,GAAG,CAAC,CAAC;aAClB;iBAAM,IAAI,CAAC,IAAI,GAAG,eAAe,MAAM,aAAa,EAAE;gBACrD,YAAY,GAAG,CAAC,CAAC;aAClB;iBAAM;gBACL,OAAO,KAAK,CAAC;aACd;SACF;KACF;IAED,OAAO,CAAC,YAAY,CAAC;AACvB,CAAC;AA7BD,oCA6BC;;;;;;;;;;AC9C+B;AACG;AAEJ;AACW;AACgB;AACf;AACR;AACD;AACH;AACK;AACA;AACG;AACA;AACA;AACE;AACO;AAgChD;AACA,IAAM,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;AAC9D,IAAM,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;AAE9D,IAAM,aAAa,GAAiC,EAAE,CAAC;AAEvD,SAAgB,WAAW,CACzB,MAAc,EACd,OAA2B,EAC3B,OAAiB;IAEjB,OAAO,GAAG,OAAO,IAAI,IAAI,GAAG,EAAE,GAAG,OAAO,CAAC;IACzC,IAAM,KAAK,GAAG,OAAO,IAAI,OAAO,CAAC,KAAK,GAAG,OAAO,CAAC,KAAK,GAAG,CAAC,CAAC;;IAE3D,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,CAAC;SACZ,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;SACvB,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;SACxB,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;IAE5B,IAAI,IAAI,GAAG,CAAC,EAAE;QACZ,MAAM,IAAI,KAAK,CAAC,gCAA8B,IAAM,CAAC,CAAC;KACvD;IAED,IAAI,OAAO,CAAC,gCAAgC,IAAI,MAAM,CAAC,MAAM,GAAG,IAAI,EAAE;QACpE,MAAM,IAAI,KAAK,CAAC,mBAAiB,MAAM,CAAC,MAAM,8BAAyB,IAAM,CAAC,CAAC;KAChF;IAED,IAAI,CAAC,OAAO,CAAC,gCAAgC,IAAI,MAAM,CAAC,MAAM,KAAK,IAAI,EAAE;QACvE,MAAM,IAAI,KAAK,CAAC,mBAAiB,MAAM,CAAC,MAAM,4BAAuB,IAAM,CAAC,CAAC;KAC9E;IAED,IAAI,IAAI,GAAG,KAAK,GAAG,MAAM,CAAC,UAAU,EAAE;QACpC,MAAM,IAAI,KAAK,CACb,gBAAc,IAAI,yBAAoB,KAAK,kCAA6B,MAAM,CAAC,UAAU,MAAG,CAC7F,CAAC;KACH;;IAGD,IAAI,MAAM,CAAC,KAAK,GAAG,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC,EAAE;QAClC,MAAM,IAAI,KAAK,CAAC,6EAA6E,CAAC,CAAC;KAChG;;IAGD,OAAO,iBAAiB,CAAC,MAAM,EAAE,KAAK,EAAE,OAAO,EAAE,OAAO,CAAC,CAAC;AAC5D,CAAC;AAvCD,kCAuCC;AAED,SAAS,iBAAiB,CACxBI,QAAc,EACd,KAAa,EACb,OAA2B,EAC3B,OAAe;IAAf,wBAAA,EAAA,eAAe;IAEf,IAAM,aAAa,GAAG,OAAO,CAAC,eAAe,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,eAAe,CAAC,CAAC;IAC1F,IAAM,cAAc,GAAG,OAAO,CAAC,gBAAgB,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,gBAAgB,CAAC,CAAC;IAE7F,IAAM,WAAW,GAAG,OAAO,CAAC,aAAa,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,aAAa,CAAC,CAAC;;IAGnF,IAAM,GAAG,GAAG,OAAO,CAAC,KAAK,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,KAAK,CAAC,CAAC;;IAG5D,IAAM,UAAU,GAAG,OAAO,OAAO,CAAC,YAAY,CAAC,KAAK,SAAS,GAAG,OAAO,CAAC,YAAY,CAAC,GAAG,KAAK,CAAC;;IAG9F,IAAM,cAAc,GAAG,OAAO,CAAC,gBAAgB,CAAC,IAAI,IAAI,GAAG,KAAK,GAAG,OAAO,CAAC,gBAAgB,CAAC,CAAC;IAC7F,IAAM,YAAY,GAAG,OAAO,CAAC,cAAc,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,cAAc,CAAC,CAAC;IACtF,IAAM,aAAa,GAAG,OAAO,CAAC,eAAe,CAAC,IAAI,IAAI,GAAG,IAAI,GAAG,OAAO,CAAC,eAAe,CAAC,CAAC;;IAGzF,IAAM,UAAU,GAAG,KAAK,CAAC;;IAGzB,IAAIA,QAAM,CAAC,MAAM,GAAG,CAAC;QAAE,MAAM,IAAI,KAAK,CAAC,qCAAqC,CAAC,CAAC;;IAG9E,IAAM,IAAI,GACRA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;IAG/F,IAAI,IAAI,GAAG,CAAC,IAAI,IAAI,GAAGA,QAAM,CAAC,MAAM;QAAE,MAAM,IAAI,KAAK,CAAC,sBAAsB,CAAC,CAAC;;IAG9E,IAAM,MAAM,GAAa,OAAO,GAAG,EAAE,GAAG,EAAE,CAAC;;IAE3C,IAAI,UAAU,GAAG,CAAC,CAAC;IACnB,IAAM,IAAI,GAAG,KAAK,CAAC;;IAGnB,OAAO,CAAC,IAAI,EAAE;;QAEZ,IAAM,WAAW,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,CAAC;;QAGpC,IAAI,WAAW,KAAK,CAAC;YAAE,MAAM;;QAG7B,IAAI,CAAC,GAAG,KAAK,CAAC;;QAEd,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;YAC9C,CAAC,EAAE,CAAC;SACL;;QAGD,IAAI,CAAC,IAAIA,QAAM,CAAC,UAAU;YAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;QAClF,IAAM,IAAI,GAAG,OAAO,GAAG,UAAU,EAAE,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;QACxE,IAAI,KAAK,SAAA,CAAC;QAEV,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;QAEd,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YAC9C,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAE/C,IAAI,CAAC8G,0BAAY,CAAC9G,QAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,EAAE;gBACxD,MAAM,IAAI,KAAK,CAAC,uCAAuC,CAAC,CAAC;aAC1D;YAED,KAAK,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;YAE/D,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,EAAE;YAClD,IAAM,GAAG,GAAGL,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;YAC7BK,QAAM,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;YACvC,KAAK,GAAG,IAAIoG,iBAAQ,CAAC,GAAG,CAAC,CAAC;YAC1B,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;SACpB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,IAAI,aAAa,KAAK,KAAK,EAAE;YAC7E,KAAK,GAAG,IAAIE,YAAK,CACftG,QAAM,CAAC,KAAK,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,IAAIA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAC7F,CAAC;SACH;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,EAAE;YAClD,KAAK;gBACHA,QAAM,CAAC,KAAK,EAAE,CAAC;qBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;qBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;qBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;SAC3B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,aAAa,KAAK,KAAK,EAAE;YAChF,KAAK,GAAG,IAAI,eAAM,CAACA,QAAM,CAAC,YAAY,CAAC,KAAK,CAAC,CAAC,CAAC;YAC/C,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;SACnB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,KAAK,GAAGA,QAAM,CAAC,YAAY,CAAC,KAAK,CAAC,CAAC;YACnC,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;SACnB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;YACnD,IAAM,OAAO,GACXA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,KAAK,GAAG,IAAI,IAAI,CAAC,IAAI,WAAI,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC,QAAQ,EAAE,CAAC,CAAC;SAC1D;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;YACtD,IAAIA,QAAM,CAAC,KAAK,CAAC,KAAK,CAAC,IAAIA,QAAM,CAAC,KAAK,CAAC,KAAK,CAAC;gBAAE,MAAM,IAAI,KAAK,CAAC,4BAA4B,CAAC,CAAC;YAC9F,KAAK,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,KAAK,CAAC,CAAC;SAC/B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,IAAM,MAAM,GAAG,KAAK,CAAC;YACrB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;iBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;iBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;iBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;YAC5B,IAAI,UAAU,IAAI,CAAC,IAAI,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBACvD,MAAM,IAAI,KAAK,CAAC,sCAAsC,CAAC,CAAC;;YAG1D,IAAI,GAAG,EAAE;gBACP,KAAK,GAAGA,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,CAAC;aACjD;iBAAM;gBACL,KAAK,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC;aAC3D;YAED,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,eAAe,EAAE;YACpD,IAAM,MAAM,GAAG,KAAK,CAAC;YACrB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;iBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;iBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;iBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;YAC5B,IAAI,YAAY,GAAG,OAAO,CAAC;;YAG3B,IAAM,SAAS,GAAG,KAAK,GAAG,UAAU,CAAC;;YAGrC,IAAI,WAAW,IAAI,WAAW,CAAC,IAAI,CAAC,EAAE;gBACpC,YAAY,GAAG,EAAE,CAAC;gBAClB,KAAK,IAAM,CAAC,IAAI,OAAO,EAAE;oBACtB,YAEC,CAAC,CAAC,CAAC,GAAG,OAAO,CAAC,CAA6B,CAAC,CAAC;iBAChD;gBACD,YAAY,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC;aAC5B;YAED,KAAK,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,YAAY,EAAE,IAAI,CAAC,CAAC;YAC9D,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;YAE3B,IAAIA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,KAAK,CAAC;gBAAE,MAAM,IAAI,KAAK,CAAC,+BAA+B,CAAC,CAAC;YAC9E,IAAI,KAAK,KAAK,SAAS;gBAAE,MAAM,IAAI,KAAK,CAAC,sBAAsB,CAAC,CAAC;SAClE;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;YACxD,KAAK,GAAG,SAAS,CAAC;SACnB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;YACnD,KAAK,GAAG,IAAI,CAAC;SACd;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;;YAEnD,IAAM,OAAO,GACXA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,IAAI,GAAG,IAAI,WAAI,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;;YAEzC,IAAI,YAAY,IAAI,aAAa,KAAK,IAAI,EAAE;gBAC1C,KAAK;oBACH,IAAI,CAAC,eAAe,CAAC,eAAe,CAAC,IAAI,IAAI,CAAC,kBAAkB,CAAC,eAAe,CAAC;0BAC7E,IAAI,CAAC,QAAQ,EAAE;0BACf,IAAI,CAAC;aACZ;iBAAM;gBACL,KAAK,GAAG,IAAI,CAAC;aACd;SACF;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,oBAAoB,EAAE;;YAEzD,IAAM,KAAK,GAAGL,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;;YAE/BK,QAAM,CAAC,IAAI,CAAC,KAAK,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;;YAEzC,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;;YAEnB,IAAM+G,YAAU,GAAG,IAAIR,qBAAU,CAAC,KAAK,CAAyC,CAAC;;YAEjF,IAAI,UAAU,IAAIQ,YAAU,IAAI,OAAOA,YAAU,CAAC,QAAQ,KAAK,UAAU,EAAE;gBACzE,KAAK,GAAGA,YAAU,CAAC,QAAQ,EAAE,CAAC;aAC/B;iBAAM;gBACL,KAAK,GAAGA,YAAU,CAAC;aACpB;SACF;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,IAAI,UAAU,GACZ/G,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,eAAe,GAAG,UAAU,CAAC;YACnC,IAAM,OAAO,GAAGA,QAAM,CAAC,KAAK,EAAE,CAAC,CAAC;;YAGhC,IAAI,UAAU,GAAG,CAAC;gBAAE,MAAM,IAAI,KAAK,CAAC,yCAAyC,CAAC,CAAC;;YAG/E,IAAI,UAAU,GAAGA,QAAM,CAAC,UAAU;gBAChC,MAAM,IAAI,KAAK,CAAC,4CAA4C,CAAC,CAAC;;YAGhE,IAAIA,QAAM,CAAC,OAAO,CAAC,IAAI,IAAI,EAAE;;gBAE3B,IAAI,OAAO,KAAKD,aAAM,CAAC,kBAAkB,EAAE;oBACzC,UAAU;wBACRC,QAAM,CAAC,KAAK,EAAE,CAAC;6BACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;6BACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;6BACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;oBAC1B,IAAI,UAAU,GAAG,CAAC;wBAChB,MAAM,IAAI,KAAK,CAAC,0DAA0D,CAAC,CAAC;oBAC9E,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,6DAA6D,CAAC,CAAC;oBACjF,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,8DAA8D,CAAC,CAAC;iBACnF;gBAED,IAAI,cAAc,IAAI,aAAa,EAAE;oBACnC,KAAK,GAAGA,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,CAAC;iBACjD;qBAAM;oBACL,KAAK,GAAG,IAAID,aAAM,CAACC,QAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,EAAE,OAAO,CAAC,CAAC;iBACtE;aACF;iBAAM;gBACL,IAAM,OAAO,GAAGL,aAAM,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC;;gBAEzC,IAAI,OAAO,KAAKI,aAAM,CAAC,kBAAkB,EAAE;oBACzC,UAAU;wBACRC,QAAM,CAAC,KAAK,EAAE,CAAC;6BACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;6BACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;6BACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;oBAC1B,IAAI,UAAU,GAAG,CAAC;wBAChB,MAAM,IAAI,KAAK,CAAC,0DAA0D,CAAC,CAAC;oBAC9E,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,6DAA6D,CAAC,CAAC;oBACjF,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,8DAA8D,CAAC,CAAC;iBACnF;;gBAGD,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,UAAU,EAAE,CAAC,EAAE,EAAE;oBAC/B,OAAO,CAAC,CAAC,CAAC,GAAGA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,CAAC;iBAChC;gBAED,IAAI,cAAc,IAAI,aAAa,EAAE;oBACnC,KAAK,GAAG,OAAO,CAAC;iBACjB;qBAAM;oBACL,KAAK,GAAG,IAAID,aAAM,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;iBACtC;aACF;;YAGD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,UAAU,KAAK,KAAK,EAAE;;YAE7E,CAAC,GAAG,KAAK,CAAC;;YAEV,OAAOC,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;;YAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;YAE9E,IAAM,MAAM,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;;YAEjD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;YAGd,CAAC,GAAG,KAAK,CAAC;;YAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;;YAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;YAE9E,IAAM,aAAa,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;YACxD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;YAGd,IAAM,YAAY,GAAG,IAAI,KAAK,CAAC,aAAa,CAAC,MAAM,CAAC,CAAC;;YAGrD,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,aAAa,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;gBACzC,QAAQ,aAAa,CAAC,CAAC,CAAC;oBACtB,KAAK,GAAG;wBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;wBACtB,MAAM;oBACR,KAAK,GAAG;wBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;wBACtB,MAAM;oBACR,KAAK,GAAG;wBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;wBACtB,MAAM;iBACT;aACF;YAED,KAAK,GAAG,IAAI,MAAM,CAAC,MAAM,EAAE,YAAY,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC;SACnD;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,UAAU,KAAK,IAAI,EAAE;;YAE5E,CAAC,GAAG,KAAK,CAAC;;YAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;;YAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;YAE9E,IAAM,MAAM,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;YACjD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;YAGd,CAAC,GAAG,KAAK,CAAC;;YAEV,OAAOA,QAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAGA,QAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;;YAED,IAAI,CAAC,IAAIA,QAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;;YAE9E,IAAM,aAAa,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;YACxD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;;YAGd,KAAK,GAAG,IAAI0G,iBAAU,CAAC,MAAM,EAAE,aAAa,CAAC,CAAC;SAC/C;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,IAAM,UAAU,GACd1G,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAC/C,IAAMgH,QAAM,GAAGhH,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;YACtE,KAAK,GAAG,aAAa,GAAGgH,QAAM,GAAG,IAAIX,iBAAU,CAACW,QAAM,CAAC,CAAC;YACxD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;YACxD,IAAM,OAAO,GACXhH,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,QAAQ,GACZA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAE1B,KAAK,GAAG,IAAI2G,mBAAS,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;SAC1C;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;YACtD,KAAK,GAAG,IAAIH,cAAM,EAAE,CAAC;SACtB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;YACtD,KAAK,GAAG,IAAIC,cAAM,EAAE,CAAC;SACtB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;YACnD,IAAM,UAAU,GACdzG,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAC/C,IAAM,cAAc,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;YAG9E,IAAI,aAAa,EAAE;;gBAEjB,IAAI,cAAc,EAAE;;oBAElB,KAAK,GAAG,WAAW,CAAC,cAAc,EAAE,aAAa,EAAE,MAAM,CAAC,CAAC;iBAC5D;qBAAM;oBACL,KAAK,GAAG,WAAW,CAAC,cAAc,CAAC,CAAC;iBACrC;aACF;iBAAM;gBACL,KAAK,GAAG,IAAI4G,SAAI,CAAC,cAAc,CAAC,CAAC;aAClC;;YAGD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,sBAAsB,EAAE;YAC3D,IAAM,SAAS,GACb5G,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;YAG1B,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;gBAC7B,MAAM,IAAI,KAAK,CAAC,yDAAyD,CAAC,CAAC;aAC5E;;YAGD,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;YAE1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;;YAG/C,IAAM,cAAc,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;YAE9E,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;YAE3B,IAAM,MAAM,GAAG,KAAK,CAAC;;YAErB,IAAM,UAAU,GACdA,QAAM,CAAC,KAAK,CAAC;iBACZA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;iBACvBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;iBACxBA,QAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;;YAE5B,IAAM,WAAW,GAAG,iBAAiB,CAACA,QAAM,EAAE,MAAM,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC;;YAEtE,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;YAG3B,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,UAAU,GAAG,UAAU,EAAE;gBAC/C,MAAM,IAAI,KAAK,CAAC,wDAAwD,CAAC,CAAC;aAC3E;;YAGD,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,UAAU,GAAG,UAAU,EAAE;gBAC/C,MAAM,IAAI,KAAK,CAAC,2DAA2D,CAAC,CAAC;aAC9E;;YAGD,IAAI,aAAa,EAAE;;gBAEjB,IAAI,cAAc,EAAE;;oBAElB,KAAK,GAAG,WAAW,CAAC,cAAc,EAAE,aAAa,EAAE,MAAM,CAAC,CAAC;iBAC5D;qBAAM;oBACL,KAAK,GAAG,WAAW,CAAC,cAAc,CAAC,CAAC;iBACrC;gBAED,KAAK,CAAC,KAAK,GAAG,WAAW,CAAC;aAC3B;iBAAM;gBACL,KAAK,GAAG,IAAI4G,SAAI,CAAC,cAAc,EAAE,WAAW,CAAC,CAAC;aAC/C;SACF;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;;YAExD,IAAM,UAAU,GACd5G,QAAM,CAAC,KAAK,EAAE,CAAC;iBACdA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;iBACrBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;iBACtBA,QAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;;YAE1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAGA,QAAM,CAAC,MAAM,GAAG,KAAK;gBAClCA,QAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;;YAE/C,IAAI,CAAC8G,0BAAY,CAAC9G,QAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,EAAE;gBACxD,MAAM,IAAI,KAAK,CAAC,uCAAuC,CAAC,CAAC;aAC1D;YACD,IAAM,SAAS,GAAGA,QAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;;YAEzE,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;;YAG3B,IAAM,SAAS,GAAGL,aAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;YACnCK,QAAM,CAAC,IAAI,CAAC,SAAS,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;YAC7C,IAAM,GAAG,GAAG,IAAIoG,iBAAQ,CAAC,SAAS,CAAC,CAAC;;YAGpC,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;;YAGnB,KAAK,GAAG,IAAIS,YAAK,CAAC,SAAS,EAAE,GAAG,CAAC,CAAC;SACnC;aAAM;YACL,MAAM,IAAI,KAAK,CACb,6BAA6B,GAAG,WAAW,CAAC,QAAQ,CAAC,EAAE,CAAC,GAAG,kBAAkB,GAAG,IAAI,GAAG,GAAG,CAC3F,CAAC;SACH;QACD,IAAI,IAAI,KAAK,WAAW,EAAE;YACxB,MAAM,CAAC,cAAc,CAAC,MAAM,EAAE,IAAI,EAAE;gBAClC,KAAK,OAAA;gBACL,QAAQ,EAAE,IAAI;gBACd,UAAU,EAAE,IAAI;gBAChB,YAAY,EAAE,IAAI;aACnB,CAAC,CAAC;SACJ;aAAM;YACL,MAAM,CAAC,IAAI,CAAC,GAAG,KAAK,CAAC;SACtB;KACF;;IAGD,IAAI,IAAI,KAAK,KAAK,GAAG,UAAU,EAAE;QAC/B,IAAI,OAAO;YAAE,MAAM,IAAI,KAAK,CAAC,oBAAoB,CAAC,CAAC;QACnD,MAAM,IAAI,KAAK,CAAC,qBAAqB,CAAC,CAAC;KACxC;;IAGD,IAAM,UAAU,GAAG,MAAM,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,MAAM,CAAC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,GAAA,CAAC,CAAC;IACtE,IAAI,KAAK,GAAG,IAAI,CAAC;IACjB,UAAU,CAAC,OAAO,CAAC,UAAA,CAAC;QAClB,IAAI,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC;YAAE,KAAK,GAAG,KAAK,CAAC;KAC7D,CAAC,CAAC;;IAGH,IAAI,CAAC,KAAK;QAAE,OAAO,MAAM,CAAC;IAE1B,IAAIA,kBAAW,CAAC,MAAM,CAAC,EAAE;QACvB,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,MAAM,CAAuB,CAAC;QAC7D,OAAO,IAAI,CAAC,IAAI,CAAC;QACjB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAIA,YAAK,CAAC,MAAM,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;KAC7D;IAED,OAAO,MAAM,CAAC;AAChB,CAAC;AAED;;;;;AAKA,SAAS,WAAW,CAClB,cAAsB,EACtB,aAA4C,EAC5C,MAAiB;IAEjB,IAAI,CAAC,aAAa;QAAE,OAAO,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC;;IAExD,IAAI,aAAa,CAAC,cAAc,CAAC,IAAI,IAAI,EAAE;QACzC,aAAa,CAAC,cAAc,CAAC,GAAG,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC;KAC9D;;IAGD,OAAO,aAAa,CAAC,cAAc,CAAC,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;AACpD,CAAC;;;;;;;;ACxpBD;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;;AAIA,SAAgB,WAAW,CACzB,MAAyB,EACzB,MAAc,EACd,MAAwB,EACxB,IAAY,EACZ,MAAc;IAEd,IAAI,CAAS,CAAC;IACd,IAAI,CAAS,CAAC;IACd,IAAM,GAAG,GAAG,MAAM,KAAK,KAAK,CAAC;IAC7B,IAAM,IAAI,GAAG,MAAM,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;IACnC,IAAM,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;IAC7B,IAAM,KAAK,GAAG,IAAI,IAAI,CAAC,CAAC;IACxB,IAAI,KAAK,GAAG,CAAC,CAAC,CAAC;IACf,IAAI,CAAC,GAAG,GAAG,GAAG,CAAC,GAAG,MAAM,GAAG,CAAC,CAAC;IAC7B,IAAM,CAAC,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,CAAC,CAAC;IACvB,IAAI,CAAC,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;IAE3B,CAAC,IAAI,CAAC,CAAC;IAEP,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC,IAAI,CAAC,KAAK,IAAI,CAAC,CAAC,CAAC;IAC5B,CAAC,KAAK,CAAC,KAAK,CAAC;IACb,KAAK,IAAI,IAAI,CAAC;IACd,OAAO,KAAK,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,KAAK,IAAI,CAAC;QAAC,CAAC;IAExE,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC,IAAI,CAAC,KAAK,IAAI,CAAC,CAAC,CAAC;IAC5B,CAAC,KAAK,CAAC,KAAK,CAAC;IACb,KAAK,IAAI,IAAI,CAAC;IACd,OAAO,KAAK,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,KAAK,IAAI,CAAC;QAAC,CAAC;IAExE,IAAI,CAAC,KAAK,CAAC,EAAE;QACX,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;KACf;SAAM,IAAI,CAAC,KAAK,IAAI,EAAE;QACrB,OAAO,CAAC,GAAG,GAAG,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,QAAQ,CAAC;KAC1C;SAAM;QACL,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;QAC1B,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;KACf;IACD,OAAO,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,CAAC;AAClD,CAAC;AAvCD,kCAuCC;AAED,SAAgB,YAAY,CAC1B,MAAyB,EACzB,KAAa,EACb,MAAc,EACd,MAAwB,EACxB,IAAY,EACZ,MAAc;IAEd,IAAI,CAAS,CAAC;IACd,IAAI,CAAS,CAAC;IACd,IAAI,CAAS,CAAC;IACd,IAAM,GAAG,GAAG,MAAM,KAAK,KAAK,CAAC;IAC7B,IAAI,IAAI,GAAG,MAAM,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;IACjC,IAAM,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;IAC7B,IAAM,KAAK,GAAG,IAAI,IAAI,CAAC,CAAC;IACxB,IAAM,EAAE,GAAG,IAAI,KAAK,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,CAAC,CAAC;IACjE,IAAI,CAAC,GAAG,GAAG,GAAG,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC;IAC7B,IAAM,CAAC,GAAG,GAAG,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;IACvB,IAAM,CAAC,GAAG,KAAK,GAAG,CAAC,KAAK,KAAK,KAAK,CAAC,IAAI,CAAC,GAAG,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;IAE9D,KAAK,GAAG,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC;IAExB,IAAI,KAAK,CAAC,KAAK,CAAC,IAAI,KAAK,KAAK,QAAQ,EAAE;QACtC,CAAC,GAAG,KAAK,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;QACzB,CAAC,GAAG,IAAI,CAAC;KACV;SAAM;QACL,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC;QAC3C,IAAI,KAAK,IAAI,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,EAAE;YACrC,CAAC,EAAE,CAAC;YACJ,CAAC,IAAI,CAAC,CAAC;SACR;QACD,IAAI,CAAC,GAAG,KAAK,IAAI,CAAC,EAAE;YAClB,KAAK,IAAI,EAAE,GAAG,CAAC,CAAC;SACjB;aAAM;YACL,KAAK,IAAI,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,GAAG,KAAK,CAAC,CAAC;SACtC;QACD,IAAI,KAAK,GAAG,CAAC,IAAI,CAAC,EAAE;YAClB,CAAC,EAAE,CAAC;YACJ,CAAC,IAAI,CAAC,CAAC;SACR;QAED,IAAI,CAAC,GAAG,KAAK,IAAI,IAAI,EAAE;YACrB,CAAC,GAAG,CAAC,CAAC;YACN,CAAC,GAAG,IAAI,CAAC;SACV;aAAM,IAAI,CAAC,GAAG,KAAK,IAAI,CAAC,EAAE;YACzB,CAAC,GAAG,CAAC,KAAK,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;YACxC,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;SACf;aAAM;YACL,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,KAAK,GAAG,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;YACvD,CAAC,GAAG,CAAC,CAAC;SACP;KACF;IAED,IAAI,KAAK,CAAC,KAAK,CAAC;QAAE,CAAC,GAAG,CAAC,CAAC;IAExB,OAAO,IAAI,IAAI,CAAC,EAAE;QAChB,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC;QAC9B,CAAC,IAAI,CAAC,CAAC;QACP,CAAC,IAAI,GAAG,CAAC;QACT,IAAI,IAAI,CAAC,CAAC;KACX;IAED,CAAC,GAAG,CAAC,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC;IAEpB,IAAI,KAAK,CAAC,KAAK,CAAC;QAAE,CAAC,IAAI,CAAC,CAAC;IAEzB,IAAI,IAAI,IAAI,CAAC;IAEb,OAAO,IAAI,GAAG,CAAC,EAAE;QACf,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC;QAC9B,CAAC,IAAI,CAAC,CAAC;QACP,CAAC,IAAI,GAAG,CAAC;QACT,IAAI,IAAI,CAAC,CAAC;KACX;IAED,MAAM,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC;AACpC,CAAC;AA5ED,oCA4EC;;;;;;;;;;;ACtJkC;AAGO;AAIM;AACF;AACC;AAEhB;AACF;AAYZ;AAgBjB,IAAM,MAAM,GAAG,MAAM,CAAC;AACtB,IAAM,UAAU,GAAG,IAAI,GAAG,CAAC,CAAC,KAAK,EAAE,MAAM,EAAE,KAAK,EAAE,cAAc,CAAC,CAAC,CAAC;AAEnE;;;;;AAMA,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,GAAG,CAAC,CAAC;IACzC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;IAEtB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;IAE/D,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,EAAE,IAAI,IAAI,CAAC;IAC9C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,EAAE,IAAI,IAAI,CAAC;IAC9C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,KAAK,CAAC,IAAI,IAAI,CAAC;IAC7C,MAAM,CAAC,KAAK,CAAC,GAAG,CAAC,IAAI,GAAG,CAAC,IAAI,IAAI,CAAC;;IAElC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,CAAC;;IAEzB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;;IAIjB,IACE,MAAM,CAAC,SAAS,CAAC,KAAK,CAAC;QACvB,KAAK,IAAI,SAAS,CAAC,cAAc;QACjC,KAAK,IAAI,SAAS,CAAC,cAAc,EACjC;;;QAGA,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;QAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;cACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;cAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;QAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;QAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC;QAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,CAAC,IAAI,IAAI,CAAC;QACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;QACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;KACxC;SAAM;;QAEL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;QAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;cACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;cAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;QAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;QAEpBI,yBAAY,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,EAAE,QAAQ,EAAE,EAAE,EAAE,CAAC,CAAC,CAAC;;QAEpD,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;KACnB;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,CAAU,EAAE,KAAa,EAAE,OAAiB;;IAE9F,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;IAG3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,gBAAgB,CACvB,MAAc,EACd,GAAW,EACX,KAAc,EACd,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;;IAE9C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;IAChC,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,KAAW,EAAE,KAAa,EAAE,OAAiB;;IAE/F,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;IAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAGpB,IAAM,WAAW,GAAG,WAAI,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,CAAC,CAAC;IACrD,IAAM,OAAO,GAAG,WAAW,CAAC,UAAU,EAAE,CAAC;IACzC,IAAM,QAAQ,GAAG,WAAW,CAAC,WAAW,EAAE,CAAC;;IAE3C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,OAAO,GAAG,IAAI,CAAC;IACjC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,CAAC,IAAI,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;;IAEzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;IAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;IAC1C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;IAC1C,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,IAAI,KAAK,CAAC,MAAM,IAAI,KAAK,CAAC,MAAM,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;QACtD,MAAM,KAAK,CAAC,QAAQ,GAAG,KAAK,CAAC,MAAM,GAAG,8BAA8B,CAAC,CAAC;KACvE;;IAED,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,MAAM,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;IAErE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;IAEvB,IAAI,KAAK,CAAC,UAAU;QAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IAC7C,IAAI,KAAK,CAAC,MAAM;QAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACzC,IAAI,KAAK,CAAC,SAAS;QAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;IAG5C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,mBAAmB,CAC1B,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAGpB,IAAI,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;QAGvC,MAAM,KAAK,CAAC,UAAU,GAAG,KAAK,CAAC,OAAO,GAAG,8BAA8B,CAAC,CAAC;KAC1E;;IAGD,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,OAAO,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;IAEtE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;IAEvB,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;;IAEhG,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAsB,EACtB,KAAa,EACb,OAAiB;;IAGjB,IAAI,KAAK,KAAK,IAAI,EAAE;QAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;KAC5C;SAAM,IAAI,KAAK,CAAC,SAAS,KAAK,QAAQ,EAAE;QACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;KAC/C;SAAM;QACL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;KAC/C;;IAGD,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,iBAAiB,CACxB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;IAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAGpB,IAAI,OAAO,KAAK,CAAC,EAAE,KAAK,QAAQ,EAAE;QAChC,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,EAAE,EAAE,KAAK,EAAE,SAAS,EAAE,QAAQ,CAAC,CAAC;KACpD;SAAM,IAAIrH,kBAAY,CAAC,KAAK,CAAC,EAAE,CAAC,EAAE;;;QAGjC,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,QAAQ,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE,KAAK,CAAC,CAAC;KAC7C;SAAM;QACL,MAAM,IAAI,SAAS,CAAC,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,GAAG,2BAA2B,CAAC,CAAC;KACvF;;IAGD,OAAO,KAAK,GAAG,EAAE,CAAC;AACpB,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAA0B,EAC1B,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,IAAM,IAAI,GAAG,KAAK,CAAC,MAAM,CAAC;;IAE1B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;IAEtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,2BAA2B,CAAC;;IAExD,MAAM,CAAC,GAAG,CAACC,0BAAY,CAAC,KAAK,CAAC,EAAE,KAAK,CAAC,CAAC;;IAEvC,KAAK,GAAG,KAAK,GAAG,IAAI,CAAC;IACrB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,SAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,OAAe,EACf,IAAqB;IALrB,0BAAA,EAAA,iBAAiB;IACjB,sBAAA,EAAA,SAAS;IACT,mCAAA,EAAA,0BAA0B;IAC1B,gCAAA,EAAA,sBAAsB;IACtB,wBAAA,EAAA,eAAe;IACf,qBAAA,EAAA,SAAqB;IAErB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;QACpC,IAAI,IAAI,CAAC,CAAC,CAAC,KAAK,KAAK;YAAE,MAAM,IAAI,KAAK,CAAC,4BAA4B,CAAC,CAAC;KACtE;;IAGD,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;;IAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,GAAG,SAAS,CAAC,eAAe,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAEhG,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,IAAM,QAAQ,GAAG,aAAa,CAC5B,MAAM,EACN,KAAK,EACL,SAAS,EACT,KAAK,EACL,KAAK,GAAG,CAAC,EACT,kBAAkB,EAClB,eAAe,EACf,IAAI,CACL,CAAC;;IAEF,IAAI,CAAC,GAAG,EAAE,CAAC;IACX,OAAO,QAAQ,CAAC;AAClB,CAAC;AAED,SAAS,mBAAmB,CAC1B,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;IAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,oBAAoB,CAAC;;IAEjD,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;;;IAIpB,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,KAAK,CAAC,QAAQ,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE,KAAK,CAAC,CAAC;IAC/C,OAAO,KAAK,GAAG,EAAE,CAAC;AACpB,CAAC;AAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,KAAW,EAAE,KAAa,EAAE,OAAiB;;IAE/F,MAAM,CAAC,KAAK,EAAE,CAAC;QACb,KAAK,CAAC,SAAS,KAAK,MAAM,GAAG,SAAS,CAAC,cAAc,GAAG,SAAS,CAAC,mBAAmB,CAAC;;IAExF,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,IAAM,OAAO,GAAG,KAAK,CAAC,UAAU,EAAE,CAAC;IACnC,IAAM,QAAQ,GAAG,KAAK,CAAC,WAAW,EAAE,CAAC;;IAErC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,OAAO,GAAG,IAAI,CAAC;IACjC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,CAAC,IAAI,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,IAAI,IAAI,CAAC;;IAEzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;IAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;IAC1C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;IAC1C,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,cAAc,CACrB,MAAc,EACd,GAAW,EACX,KAAqB,EACrB,KAAa,EACb,OAAiB;IAEjB,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;;IAExB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;;IAE1C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC;IAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,CAAC,IAAI,IAAI,CAAC;IACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;IACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,IAAI,IAAI,CAAC;IACvC,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAG7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAGpBoH,yBAAY,CAAC,MAAM,EAAE,KAAK,CAAC,KAAK,EAAE,KAAK,EAAE,QAAQ,EAAE,EAAE,EAAE,CAAC,CAAC,CAAC;;IAG1D,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;IAClB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,iBAAiB,CACxB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,UAAkB,EAClB,MAAU,EACV,OAAiB;IAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;IAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,IAAM,cAAc,GAAGrH,8BAAwB,CAAC,KAAK,CAAC,CAAC;;IAGvD,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;IAE5E,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;IAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;IAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,aAAa,CACpB,MAAc,EACd,GAAW,EACX,KAAW,EACX,KAAa,EACb,SAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,OAAe;IAJf,0BAAA,EAAA,iBAAiB;IACjB,sBAAA,EAAA,SAAS;IACT,mCAAA,EAAA,0BAA0B;IAC1B,gCAAA,EAAA,sBAAsB;IACtB,wBAAA,EAAA,eAAe;IAEf,IAAI,KAAK,CAAC,KAAK,IAAI,OAAO,KAAK,CAAC,KAAK,KAAK,QAAQ,EAAE;;QAElD,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,sBAAsB,CAAC;;QAEnD,IAAM,oBAAoB,GAAG,CAAC,OAAO;cACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;cAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;QAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;QAGpB,IAAI,UAAU,GAAG,KAAK,CAAC;;;QAIvB,IAAM,cAAc,GAAG,OAAO,KAAK,CAAC,IAAI,KAAK,QAAQ,GAAG,KAAK,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC;;QAE3F,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;;QAElB,IAAM,QAAQ,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;QAEhF,MAAM,CAAC,KAAK,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;QAChC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,IAAI,IAAI,CAAC;QAC3C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;QAC5C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,IAAI,IAAI,CAAC;;QAE5C,MAAM,CAAC,KAAK,GAAG,CAAC,GAAG,QAAQ,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;;QAErC,KAAK,GAAG,KAAK,GAAG,QAAQ,GAAG,CAAC,CAAC;;;QAI7B,IAAM,QAAQ,GAAG,aAAa,CAC5B,MAAM,EACN,KAAK,CAAC,KAAK,EACX,SAAS,EACT,KAAK,EACL,KAAK,GAAG,CAAC,EACT,kBAAkB,EAClB,eAAe,CAChB,CAAC;QACF,KAAK,GAAG,QAAQ,GAAG,CAAC,CAAC;;QAGrB,IAAM,SAAS,GAAG,QAAQ,GAAG,UAAU,CAAC;;QAGxC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,SAAS,GAAG,IAAI,CAAC;QACxC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,CAAC,IAAI,IAAI,CAAC;QAC/C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,EAAE,IAAI,IAAI,CAAC;QAChD,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,EAAE,IAAI,IAAI,CAAC;;QAEhD,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACrB;SAAM;QACL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;;QAE3C,IAAM,oBAAoB,GAAG,CAAC,OAAO;cACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;cAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;QAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;QAEpB,IAAM,cAAc,GAAG,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC;;QAE7C,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;QAE5E,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;QAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;QACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;QACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;QAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;QAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACrB;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,IAAM,IAAI,GAAG,KAAK,CAAC,KAAK,CAAC,IAAI,CAAwB,CAAC;;IAEtD,IAAI,IAAI,GAAG,KAAK,CAAC,QAAQ,CAAC;;IAE1B,IAAI,KAAK,CAAC,QAAQ,KAAKG,aAAM,CAAC,kBAAkB;QAAE,IAAI,GAAG,IAAI,GAAG,CAAC,CAAC;;IAElE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;IAEtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,QAAQ,CAAC;;IAGjC,IAAI,KAAK,CAAC,QAAQ,KAAKA,aAAM,CAAC,kBAAkB,EAAE;QAChD,IAAI,GAAG,IAAI,GAAG,CAAC,CAAC;QAChB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;QAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;QACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;KACvC;;IAGD,MAAM,CAAC,GAAG,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;;IAExB,KAAK,GAAG,KAAK,GAAG,KAAK,CAAC,QAAQ,CAAC;IAC/B,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAEjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;;IAEpB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;;IAEzE,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;IAExC,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;;IAE7B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,cAAc,CACrB,MAAc,EACd,GAAW,EACX,KAAY,EACZ,KAAa,EACb,KAAa,EACb,kBAA2B,EAC3B,OAAiB;;IAGjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;;IAE7C,IAAM,oBAAoB,GAAG,CAAC,OAAO;UACjC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;UAC3C,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;;IAGjD,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IAEpB,IAAI,UAAU,GAAG,KAAK,CAAC;IACvB,IAAI,MAAM,GAAc;QACtB,IAAI,EAAE,KAAK,CAAC,UAAU,IAAI,KAAK,CAAC,SAAS;QACzC,GAAG,EAAE,KAAK,CAAC,GAAG;KACf,CAAC;IAEF,IAAI,KAAK,CAAC,EAAE,IAAI,IAAI,EAAE;QACpB,MAAM,CAAC,GAAG,GAAG,KAAK,CAAC,EAAE,CAAC;KACvB;IAED,MAAM,GAAG,MAAM,CAAC,MAAM,CAAC,MAAM,EAAE,KAAK,CAAC,MAAM,CAAC,CAAC;IAC7C,IAAM,QAAQ,GAAG,aAAa,CAAC,MAAM,EAAE,MAAM,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,kBAAkB,CAAC,CAAC;;IAG5F,IAAM,IAAI,GAAG,QAAQ,GAAG,UAAU,CAAC;;IAEnC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IACnC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IAC1C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IAC3C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;;IAE3C,OAAO,QAAQ,CAAC;AAClB,CAAC;AAED,SAAgB,aAAa,CAC3B,MAAc,EACd,MAAgB,EAChB,SAAiB,EACjB,aAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,IAAqB;IALrB,0BAAA,EAAA,iBAAiB;IACjB,8BAAA,EAAA,iBAAiB;IACjB,sBAAA,EAAA,SAAS;IACT,mCAAA,EAAA,0BAA0B;IAC1B,gCAAA,EAAA,sBAAsB;IACtB,qBAAA,EAAA,SAAqB;IAErB,aAAa,GAAG,aAAa,IAAI,CAAC,CAAC;IACnC,IAAI,GAAG,IAAI,IAAI,EAAE,CAAC;;IAGlB,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;;IAGlB,IAAI,KAAK,GAAG,aAAa,GAAG,CAAC,CAAC;;IAG9B,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;;QAEzB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;YACtC,IAAM,GAAG,GAAG,EAAE,GAAG,CAAC,CAAC;YACnB,IAAI,KAAK,GAAG,MAAM,CAAC,CAAC,CAAC,CAAC;;YAGtB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;gBACzB,IAAI,OAAO,KAAK,CAAC,MAAM,KAAK,UAAU;oBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;gBACxF,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;aACxB;YAED,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;gBAC7B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;gBACpC,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;gBACpC,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;aACvE;iBAAM,IAAI,OAAO,KAAK,KAAK,SAAS,EAAE;gBACrC,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC3D;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAIH,YAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,KAAK,SAAS,EAAE;gBAC9B,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,KAAK,IAAI,EAAE;gBACzB,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC5D;iBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;gBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;gBAClE,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,IAAI,EACJ,IAAI,CACL,CAAC;aACH;iBAAM,IACL,OAAO,KAAK,KAAK,QAAQ;gBACzBsH,wBAAU,CAAC,KAAK,CAAC;gBACjB,KAAK,CAAC,SAAS,KAAK,YAAY,EAChC;gBACA,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC9D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;gBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC9E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;gBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,IAAI,CACL,CAAC;aACH;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,EAAE,IAAI,CAAC,CAAC;aACpF;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC9D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACzD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;aACjF;SACF;KACF;SAAM,IAAI,MAAM,YAAYC,OAAG,IAAIvH,WAAK,CAAC,MAAM,CAAC,EAAE;QACjD,IAAM,QAAQ,GAAG,MAAM,CAAC,OAAO,EAAE,CAAC;QAClC,IAAI,IAAI,GAAG,KAAK,CAAC;QAEjB,OAAO,CAAC,IAAI,EAAE;;YAEZ,IAAM,KAAK,GAAG,QAAQ,CAAC,IAAI,EAAE,CAAC;YAC9B,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC,IAAI,CAAC;;YAEpB,IAAI,IAAI;gBAAE,SAAS;;YAGnB,IAAM,GAAG,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;YAC3B,IAAM,KAAK,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;;YAG7B,IAAM,IAAI,GAAG,OAAO,KAAK,CAAC;;YAG1B,IAAI,OAAO,GAAG,KAAK,QAAQ,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;gBACnD,IAAI,GAAG,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;oBAG7B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,8BAA8B,CAAC,CAAC;iBAC5D;gBAED,IAAI,SAAS,EAAE;oBACb,IAAI,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,EAAE;wBAClB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,0BAA0B,CAAC,CAAC;qBACxD;yBAAM,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;wBAC5B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,uBAAuB,CAAC,CAAC;qBACrD;iBACF;aACF;YAED,IAAI,IAAI,KAAK,QAAQ,EAAE;gBACrB,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;gBAC5B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAIA,qBAAe,CAAC,KAAK,CAAC,IAAIA,sBAAgB,CAAC,KAAK,CAAC,EAAE;gBACjF,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;aACvE;iBAAM,IAAI,IAAI,KAAK,SAAS,EAAE;gBAC7B,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACrD;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAIA,YAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,KAAK,IAAI,KAAK,KAAK,KAAK,SAAS,IAAI,eAAe,KAAK,KAAK,CAAC,EAAE;gBAC/E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACtD;iBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;gBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;gBAC1D,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,KAAK,EACL,IAAI,CACL,CAAC;aACH;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBACnE,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;gBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,CAChB,CAAC;aACH;iBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;gBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC5F;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC9E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACnD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;aACjF;SACF;KACF;SAAM;;QAEL,IAAI,MAAM,CAAC,MAAM,EAAE;YACjB,IAAI,OAAO,MAAM,CAAC,MAAM,KAAK,UAAU;gBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;YACzF,MAAM,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC;YACzB,IAAI,MAAM,IAAI,IAAI,IAAI,OAAO,MAAM,KAAK,QAAQ;gBAC9C,MAAM,IAAI,SAAS,CAAC,0CAA0C,CAAC,CAAC;SACnE;;QAGD,KAAK,IAAM,GAAG,IAAI,MAAM,EAAE;YACxB,IAAI,KAAK,GAAG,MAAM,CAAC,GAAG,CAAC,CAAC;;YAExB,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;gBACzB,IAAI,OAAO,KAAK,CAAC,MAAM,KAAK,UAAU;oBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;gBACxF,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;aACxB;;YAGD,IAAM,IAAI,GAAG,OAAO,KAAK,CAAC;;YAG1B,IAAI,OAAO,GAAG,KAAK,QAAQ,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;gBACnD,IAAI,GAAG,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;;;oBAG7B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,8BAA8B,CAAC,CAAC;iBAC5D;gBAED,IAAI,SAAS,EAAE;oBACb,IAAI,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,EAAE;wBAClB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,0BAA0B,CAAC,CAAC;qBACxD;yBAAM,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;wBAC5B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,uBAAuB,CAAC,CAAC;qBACrD;iBACF;aACF;YAED,IAAI,IAAI,KAAK,QAAQ,EAAE;gBACrB,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;gBAC5B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;gBAC5B,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;aACvE;iBAAM,IAAI,IAAI,KAAK,SAAS,EAAE;gBAC7B,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACrD;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAIA,YAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,KAAK,SAAS,EAAE;gBAC9B,IAAI,eAAe,KAAK,KAAK;oBAAE,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACjF;iBAAM,IAAI,KAAK,KAAK,IAAI,EAAE;gBACzB,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACtD;iBAAM,IAAIA,kBAAY,CAAC,KAAK,CAAC,EAAE;gBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAIA,cAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;gBAC1D,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,KAAK,EACL,IAAI,CACL,CAAC;aACH;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBACnE,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;gBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,CAChB,CAAC;aACH;iBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;gBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC5F;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC9E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACnD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;aACjF;SACF;KACF;;IAGD,IAAI,CAAC,GAAG,EAAE,CAAC;;IAGX,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;;IAGvB,IAAM,IAAI,GAAG,KAAK,GAAG,aAAa,CAAC;;IAEnC,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IACtC,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC;IAC7C,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IAC9C,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,IAAI,IAAI,CAAC;IAC9C,OAAO,KAAK,CAAC;AACf,CAAC;AAxUD,sCAwUC;;;;;;;;;;;AC7iC+B;AACE;AAiFhC,uFAjFOG,aAAM,OAiFP;AAhFsB;AA4E5B,qFA5EO6G,SAAI,OA4EP;AA3E2B;AA8E/B,sFA9EOC,YAAK,OA8EP;AA7EmC;AAwFxC,2FAxFON,qBAAU,OAwFP;AAvFsB;AAkFhC,uFAlFO,eAAM,OAkFP;AAjFuC;AACP;AACP;AAgF/B,sFAhFOD,YAAK,OAgFP;AA/EuB;AA4E5B,qFA5EO,WAAI,OA4EP;AA3EsB;AAqE1B,oFArEOa,OAAG,OAqEP;AApE8B;AA+EjC,uFA/EOV,cAAM,OA+EP;AA9E2B;AA6EjC,uFA7EOD,cAAM,OA6EP;AA5E8B;AAsEpC,yFAtEOJ,iBAAQ,OAsEP;AAaI,yFAnFLA,iBAAQ,OAmFK;AAlFuE;AAC7F;AAC+F;AACJ;AACrD;AAyEpC,2FAzEOM,iBAAU,OAyEP;AAxE0B;AA6DpC,2FA7DOL,iBAAU,OA6DP;AA5D4B;AAkEtC,0FAlEOM,mBAAS,OAkEP;AAjEmB;AA+D5B,qFA/DO1G,SAAI,OA+DP;AA3Be;AAhCnB,yHAAA,8BAA8B,OAAA;AAC9B,sHAAA,2BAA2B,OAAA;AAC3B,uHAAA,4BAA4B,OAAA;AAC5B,kHAAA,uBAAuB,OAAA;AACvB,2HAAA,gCAAgC,OAAA;AAChC,mHAAA,wBAAwB,OAAA;AACxB,uHAAA,4BAA4B,OAAA;AAC5B,0GAAA,eAAe,OAAA;AACf,2GAAA,gBAAgB,OAAA;AAChB,4GAAA,iBAAiB,OAAA;AACjB,yGAAA,cAAc,OAAA;AACd,iHAAA,sBAAsB,OAAA;AACtB,yGAAA,cAAc,OAAA;AACd,8GAAA,mBAAmB,OAAA;AACnB,+GAAA,oBAAoB,OAAA;AACpB,wGAAA,aAAa,OAAA;AACb,yGAAA,cAAc,OAAA;AACd,4GAAA,iBAAiB,OAAA;AACjB,4GAAA,iBAAiB,OAAA;AACjB,yGAAA,cAAc,OAAA;AACd,2GAAA,gBAAgB,OAAA;AAChB,2GAAA,gBAAgB,OAAA;AAChB,wGAAA,aAAa,OAAA;AACb,2GAAA,gBAAgB,OAAA;AAChB,2GAAA,gBAAgB,OAAA;AAChB,2GAAA,gBAAgB,OAAA;AAChB,8GAAA,mBAAmB,OAAA;AACnB,8GAAA,mBAAmB,OAAA;AACnB,yGAAA,cAAc,OAAA;AACd,yGAAA,cAAc,OAAA;AACd,yGAAA,cAAc,OAAA;AACd,yGAAA,cAAc,OAAA;AAKhB,oCAAsD;AAA7C,sGAAA,KAAK,OAAA;AAQd,4BAKqB;AAHnB,sHAAA,yBAAyB,OAAA;AAkC3B;AACA;AACA,IAAM,OAAO,GAAG,IAAI,GAAG,IAAI,GAAG,EAAE,CAAC;AAEjC;AACA,IAAID,QAAM,GAAGL,aAAM,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC;AAEnC;;;;;;AAMA,SAAgB,qBAAqB,CAAC,IAAY;;IAEhD,IAAIK,QAAM,CAAC,MAAM,GAAG,IAAI,EAAE;QACxBA,QAAM,GAAGL,aAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;KAC7B;AACH,CAAC;AALD,sDAKC;AAED;;;;;;;AAOA,SAAgB,SAAS,CAAC,MAAgB,EAAE,OAA8B;IAA9B,wBAAA,EAAA,YAA8B;;IAExE,IAAM,SAAS,GAAG,OAAO,OAAO,CAAC,SAAS,KAAK,SAAS,GAAG,OAAO,CAAC,SAAS,GAAG,KAAK,CAAC;IACrF,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;IACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;IAChF,IAAM,qBAAqB,GACzB,OAAO,OAAO,CAAC,qBAAqB,KAAK,QAAQ,GAAG,OAAO,CAAC,qBAAqB,GAAG,OAAO,CAAC;;IAG9F,IAAIK,QAAM,CAAC,MAAM,GAAG,qBAAqB,EAAE;QACzCA,QAAM,GAAGL,aAAM,CAAC,KAAK,CAAC,qBAAqB,CAAC,CAAC;KAC9C;;IAGD,IAAM,kBAAkB,GAAGyH,wBAAiB,CAC1CpH,QAAM,EACN,MAAM,EACN,SAAS,EACT,CAAC,EACD,CAAC,EACD,kBAAkB,EAClB,eAAe,EACf,EAAE,CACH,CAAC;;IAGF,IAAM,cAAc,GAAGL,aAAM,CAAC,KAAK,CAAC,kBAAkB,CAAC,CAAC;;IAGxDK,QAAM,CAAC,IAAI,CAAC,cAAc,EAAE,CAAC,EAAE,CAAC,EAAE,cAAc,CAAC,MAAM,CAAC,CAAC;;IAGzD,OAAO,cAAc,CAAC;AACxB,CAAC;AAnCD,8BAmCC;AAED;;;;;;;;;AASA,SAAgB,2BAA2B,CACzC,MAAgB,EAChB,WAAmB,EACnB,OAA8B;IAA9B,wBAAA,EAAA,YAA8B;;IAG9B,IAAM,SAAS,GAAG,OAAO,OAAO,CAAC,SAAS,KAAK,SAAS,GAAG,OAAO,CAAC,SAAS,GAAG,KAAK,CAAC;IACrF,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;IACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;IAChF,IAAM,UAAU,GAAG,OAAO,OAAO,CAAC,KAAK,KAAK,QAAQ,GAAG,OAAO,CAAC,KAAK,GAAG,CAAC,CAAC;;IAGzE,IAAM,kBAAkB,GAAGoH,wBAAiB,CAC1CpH,QAAM,EACN,MAAM,EACN,SAAS,EACT,CAAC,EACD,CAAC,EACD,kBAAkB,EAClB,eAAe,CAChB,CAAC;IACFA,QAAM,CAAC,IAAI,CAAC,WAAW,EAAE,UAAU,EAAE,CAAC,EAAE,kBAAkB,CAAC,CAAC;;IAG5D,OAAO,UAAU,GAAG,kBAAkB,GAAG,CAAC,CAAC;AAC7C,CAAC;AA3BD,kEA2BC;AAED;;;;;;;AAOA,SAAgB,WAAW,CACzB,MAA8C,EAC9C,OAAgC;IAAhC,wBAAA,EAAA,YAAgC;IAEhC,OAAOqH,wBAAmB,CAACxH,0BAAY,CAAC,MAAM,CAAC,EAAE,OAAO,CAAC,CAAC;AAC5D,CAAC;AALD,kCAKC;AAQD;;;;;;;AAOA,SAAgB,mBAAmB,CACjC,MAAgB,EAChB,OAAwC;IAAxC,wBAAA,EAAA,YAAwC;IAExC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;IAExB,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,GAAG,OAAO,CAAC,kBAAkB,GAAG,KAAK,CAAC;IACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,GAAG,OAAO,CAAC,eAAe,GAAG,IAAI,CAAC;IAEhF,OAAOyH,kCAA2B,CAAC,MAAM,EAAE,kBAAkB,EAAE,eAAe,CAAC,CAAC;AAClF,CAAC;AAZD,kDAYC;AAED;;;;;;;;;;;;AAYA,SAAgB,iBAAiB,CAC/B,IAA4C,EAC5C,UAAkB,EAClB,iBAAyB,EACzB,SAAqB,EACrB,aAAqB,EACrB,OAA2B;IAE3B,IAAM,eAAe,GAAG,MAAM,CAAC,MAAM,CACnC,EAAE,gCAAgC,EAAE,IAAI,EAAE,KAAK,EAAE,CAAC,EAAE,EACpD,OAAO,CACR,CAAC;IACF,IAAM,UAAU,GAAGzH,0BAAY,CAAC,IAAI,CAAC,CAAC;IAEtC,IAAI,KAAK,GAAG,UAAU,CAAC;;IAEvB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,iBAAiB,EAAE,CAAC,EAAE,EAAE;;QAE1C,IAAM,IAAI,GACR,UAAU,CAAC,KAAK,CAAC;aAChB,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;aAC3B,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;aAC5B,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;;QAEhC,eAAe,CAAC,KAAK,GAAG,KAAK,CAAC;;QAE9B,SAAS,CAAC,aAAa,GAAG,CAAC,CAAC,GAAGwH,wBAAmB,CAAC,UAAU,EAAE,eAAe,CAAC,CAAC;;QAEhF,KAAK,GAAG,KAAK,GAAG,IAAI,CAAC;KACtB;;IAGD,OAAO,KAAK,CAAC;AACf,CAAC;AAjCD,8CAiCC;AAED;;;;;;;;AAQA,IAAM,IAAI,GAAG;IACX,MAAM,eAAA;IACN,IAAI,WAAA;IACJ,KAAK,cAAA;IACL,UAAU,uBAAA;IACV,MAAM,iBAAA;IACN,KAAK,cAAA;IACL,IAAI,aAAA;IACJ,IAAI,WAAA;IACJ,GAAG,SAAA;IACH,MAAM,gBAAA;IACN,MAAM,gBAAA;IACN,QAAQ,mBAAA;IACR,QAAQ,EAAEjB,iBAAQ;IAClB,UAAU,mBAAA;IACV,UAAU,mBAAA;IACV,SAAS,qBAAA;IACT,KAAK,qBAAA;IACL,qBAAqB,uBAAA;IACrB,SAAS,WAAA;IACT,2BAA2B,6BAAA;IAC3B,WAAW,aAAA;IACX,mBAAmB,qBAAA;IACnB,iBAAiB,mBAAA;CAClB,CAAC;AACF,kBAAe,IAAI,CAAC;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;"}
\ No newline at end of file
diff --git a/node_modules/bson/etc/prepare.js b/node_modules/bson/etc/prepare.js
new file mode 100755
index 0000000..91e6f3a
--- /dev/null
+++ b/node_modules/bson/etc/prepare.js
@@ -0,0 +1,19 @@
+#! /usr/bin/env node
+var cp = require('child_process');
+var fs = require('fs');
+
+var nodeMajorVersion = +process.version.match(/^v(\d+)\.\d+/)[1];
+
+if (fs.existsSync('src') && nodeMajorVersion >= 10) {
+ cp.spawnSync('npm', ['run', 'build'], { stdio: 'inherit', shell: true });
+} else {
+ if (!fs.existsSync('lib')) {
+ console.warn('BSON: No compiled javascript present, the library is not installed correctly.');
+ if (nodeMajorVersion < 10) {
+ console.warn(
+ 'This library can only be compiled in nodejs version 10 or later, currently running: ' +
+ nodeMajorVersion
+ );
+ }
+ }
+}
diff --git a/node_modules/bson/index.js b/node_modules/bson/index.js
deleted file mode 100644
index 6502552..0000000
--- a/node_modules/bson/index.js
+++ /dev/null
@@ -1,46 +0,0 @@
-var BSON = require('./lib/bson/bson'),
- Binary = require('./lib/bson/binary'),
- Code = require('./lib/bson/code'),
- DBRef = require('./lib/bson/db_ref'),
- Decimal128 = require('./lib/bson/decimal128'),
- Double = require('./lib/bson/double'),
- Int32 = require('./lib/bson/int_32'),
- Long = require('./lib/bson/long'),
- Map = require('./lib/bson/map'),
- MaxKey = require('./lib/bson/max_key'),
- MinKey = require('./lib/bson/min_key'),
- ObjectId = require('./lib/bson/objectid'),
- BSONRegExp = require('./lib/bson/regexp'),
- Symbol = require('./lib/bson/symbol'),
- Timestamp = require('./lib/bson/timestamp');
-
-// BSON MAX VALUES
-BSON.BSON_INT32_MAX = 0x7fffffff;
-BSON.BSON_INT32_MIN = -0x80000000;
-
-BSON.BSON_INT64_MAX = Math.pow(2, 63) - 1;
-BSON.BSON_INT64_MIN = -Math.pow(2, 63);
-
-// JS MAX PRECISE VALUES
-BSON.JS_INT_MAX = 0x20000000000000; // Any integer up to 2^53 can be precisely represented by a double.
-BSON.JS_INT_MIN = -0x20000000000000; // Any integer down to -2^53 can be precisely represented by a double.
-
-// Add BSON types to function creation
-BSON.Binary = Binary;
-BSON.Code = Code;
-BSON.DBRef = DBRef;
-BSON.Decimal128 = Decimal128;
-BSON.Double = Double;
-BSON.Int32 = Int32;
-BSON.Long = Long;
-BSON.Map = Map;
-BSON.MaxKey = MaxKey;
-BSON.MinKey = MinKey;
-BSON.ObjectId = ObjectId;
-BSON.ObjectID = ObjectId;
-BSON.BSONRegExp = BSONRegExp;
-BSON.Symbol = Symbol;
-BSON.Timestamp = Timestamp;
-
-// Return the BSON
-module.exports = BSON;
diff --git a/node_modules/bson/lib/binary.js b/node_modules/bson/lib/binary.js
new file mode 100644
index 0000000..513af96
--- /dev/null
+++ b/node_modules/bson/lib/binary.js
@@ -0,0 +1,237 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Binary = void 0;
+var buffer_1 = require("buffer");
+var ensure_buffer_1 = require("./ensure_buffer");
+var uuid_utils_1 = require("./uuid_utils");
+var uuid_1 = require("./uuid");
+/**
+ * A class representation of the BSON Binary type.
+ * @public
+ */
+var Binary = /** @class */ (function () {
+ /**
+ * @param buffer - a buffer object containing the binary data.
+ * @param subType - the option binary type.
+ */
+ function Binary(buffer, subType) {
+ if (!(this instanceof Binary))
+ return new Binary(buffer, subType);
+ if (!(buffer == null) &&
+ !(typeof buffer === 'string') &&
+ !ArrayBuffer.isView(buffer) &&
+ !(buffer instanceof ArrayBuffer) &&
+ !Array.isArray(buffer)) {
+ throw new TypeError('Binary can only be constructed from string, Buffer, TypedArray, or Array');
+ }
+ this.sub_type = subType !== null && subType !== void 0 ? subType : Binary.BSON_BINARY_SUBTYPE_DEFAULT;
+ if (buffer == null) {
+ // create an empty binary buffer
+ this.buffer = buffer_1.Buffer.alloc(Binary.BUFFER_SIZE);
+ this.position = 0;
+ }
+ else {
+ if (typeof buffer === 'string') {
+ // string
+ this.buffer = buffer_1.Buffer.from(buffer, 'binary');
+ }
+ else if (Array.isArray(buffer)) {
+ // number[]
+ this.buffer = buffer_1.Buffer.from(buffer);
+ }
+ else {
+ // Buffer | TypedArray | ArrayBuffer
+ this.buffer = ensure_buffer_1.ensureBuffer(buffer);
+ }
+ this.position = this.buffer.byteLength;
+ }
+ }
+ /**
+ * Updates this binary with byte_value.
+ *
+ * @param byteValue - a single byte we wish to write.
+ */
+ Binary.prototype.put = function (byteValue) {
+ // If it's a string and a has more than one character throw an error
+ if (typeof byteValue === 'string' && byteValue.length !== 1) {
+ throw new TypeError('only accepts single character String');
+ }
+ else if (typeof byteValue !== 'number' && byteValue.length !== 1)
+ throw new TypeError('only accepts single character Uint8Array or Array');
+ // Decode the byte value once
+ var decodedByte;
+ if (typeof byteValue === 'string') {
+ decodedByte = byteValue.charCodeAt(0);
+ }
+ else if (typeof byteValue === 'number') {
+ decodedByte = byteValue;
+ }
+ else {
+ decodedByte = byteValue[0];
+ }
+ if (decodedByte < 0 || decodedByte > 255) {
+ throw new TypeError('only accepts number in a valid unsigned byte range 0-255');
+ }
+ if (this.buffer.length > this.position) {
+ this.buffer[this.position++] = decodedByte;
+ }
+ else {
+ var buffer = buffer_1.Buffer.alloc(Binary.BUFFER_SIZE + this.buffer.length);
+ // Combine the two buffers together
+ this.buffer.copy(buffer, 0, 0, this.buffer.length);
+ this.buffer = buffer;
+ this.buffer[this.position++] = decodedByte;
+ }
+ };
+ /**
+ * Writes a buffer or string to the binary.
+ *
+ * @param sequence - a string or buffer to be written to the Binary BSON object.
+ * @param offset - specify the binary of where to write the content.
+ */
+ Binary.prototype.write = function (sequence, offset) {
+ offset = typeof offset === 'number' ? offset : this.position;
+ // If the buffer is to small let's extend the buffer
+ if (this.buffer.length < offset + sequence.length) {
+ var buffer = buffer_1.Buffer.alloc(this.buffer.length + sequence.length);
+ this.buffer.copy(buffer, 0, 0, this.buffer.length);
+ // Assign the new buffer
+ this.buffer = buffer;
+ }
+ if (ArrayBuffer.isView(sequence)) {
+ this.buffer.set(ensure_buffer_1.ensureBuffer(sequence), offset);
+ this.position =
+ offset + sequence.byteLength > this.position ? offset + sequence.length : this.position;
+ }
+ else if (typeof sequence === 'string') {
+ this.buffer.write(sequence, offset, sequence.length, 'binary');
+ this.position =
+ offset + sequence.length > this.position ? offset + sequence.length : this.position;
+ }
+ };
+ /**
+ * Reads **length** bytes starting at **position**.
+ *
+ * @param position - read from the given position in the Binary.
+ * @param length - the number of bytes to read.
+ */
+ Binary.prototype.read = function (position, length) {
+ length = length && length > 0 ? length : this.position;
+ // Let's return the data based on the type we have
+ return this.buffer.slice(position, position + length);
+ };
+ /**
+ * Returns the value of this binary as a string.
+ * @param asRaw - Will skip converting to a string
+ * @remarks
+ * This is handy when calling this function conditionally for some key value pairs and not others
+ */
+ Binary.prototype.value = function (asRaw) {
+ asRaw = !!asRaw;
+ // Optimize to serialize for the situation where the data == size of buffer
+ if (asRaw && this.buffer.length === this.position) {
+ return this.buffer;
+ }
+ // If it's a node.js buffer object
+ if (asRaw) {
+ return this.buffer.slice(0, this.position);
+ }
+ return this.buffer.toString('binary', 0, this.position);
+ };
+ /** the length of the binary sequence */
+ Binary.prototype.length = function () {
+ return this.position;
+ };
+ /** @internal */
+ Binary.prototype.toJSON = function () {
+ return this.buffer.toString('base64');
+ };
+ /** @internal */
+ Binary.prototype.toString = function (format) {
+ return this.buffer.toString(format);
+ };
+ /** @internal */
+ Binary.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ var base64String = this.buffer.toString('base64');
+ var subType = Number(this.sub_type).toString(16);
+ if (options.legacy) {
+ return {
+ $binary: base64String,
+ $type: subType.length === 1 ? '0' + subType : subType
+ };
+ }
+ return {
+ $binary: {
+ base64: base64String,
+ subType: subType.length === 1 ? '0' + subType : subType
+ }
+ };
+ };
+ /** @internal */
+ Binary.prototype.toUUID = function () {
+ if (this.sub_type === Binary.SUBTYPE_UUID) {
+ return new uuid_1.UUID(this.buffer.slice(0, this.position));
+ }
+ throw new Error("Binary sub_type \"" + this.sub_type + "\" is not supported for converting to UUID. Only \"" + Binary.SUBTYPE_UUID + "\" is currently supported.");
+ };
+ /** @internal */
+ Binary.fromExtendedJSON = function (doc, options) {
+ options = options || {};
+ var data;
+ var type;
+ if ('$binary' in doc) {
+ if (options.legacy && typeof doc.$binary === 'string' && '$type' in doc) {
+ type = doc.$type ? parseInt(doc.$type, 16) : 0;
+ data = buffer_1.Buffer.from(doc.$binary, 'base64');
+ }
+ else {
+ if (typeof doc.$binary !== 'string') {
+ type = doc.$binary.subType ? parseInt(doc.$binary.subType, 16) : 0;
+ data = buffer_1.Buffer.from(doc.$binary.base64, 'base64');
+ }
+ }
+ }
+ else if ('$uuid' in doc) {
+ type = 4;
+ data = uuid_utils_1.uuidHexStringToBuffer(doc.$uuid);
+ }
+ if (!data) {
+ throw new TypeError("Unexpected Binary Extended JSON format " + JSON.stringify(doc));
+ }
+ return new Binary(data, type);
+ };
+ /** @internal */
+ Binary.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Binary.prototype.inspect = function () {
+ var asBuffer = this.value(true);
+ return "new Binary(Buffer.from(\"" + asBuffer.toString('hex') + "\", \"hex\"), " + this.sub_type + ")";
+ };
+ /**
+ * Binary default subtype
+ * @internal
+ */
+ Binary.BSON_BINARY_SUBTYPE_DEFAULT = 0;
+ /** Initial buffer default size */
+ Binary.BUFFER_SIZE = 256;
+ /** Default BSON type */
+ Binary.SUBTYPE_DEFAULT = 0;
+ /** Function BSON type */
+ Binary.SUBTYPE_FUNCTION = 1;
+ /** Byte Array BSON type */
+ Binary.SUBTYPE_BYTE_ARRAY = 2;
+ /** Deprecated UUID BSON type @deprecated Please use SUBTYPE_UUID */
+ Binary.SUBTYPE_UUID_OLD = 3;
+ /** UUID BSON type */
+ Binary.SUBTYPE_UUID = 4;
+ /** MD5 BSON type */
+ Binary.SUBTYPE_MD5 = 5;
+ /** User BSON type */
+ Binary.SUBTYPE_USER_DEFINED = 128;
+ return Binary;
+}());
+exports.Binary = Binary;
+Object.defineProperty(Binary.prototype, '_bsontype', { value: 'Binary' });
+//# sourceMappingURL=binary.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/binary.js.map b/node_modules/bson/lib/binary.js.map
new file mode 100644
index 0000000..ee2e92d
--- /dev/null
+++ b/node_modules/bson/lib/binary.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"binary.js","sourceRoot":"","sources":["../src/binary.ts"],"names":[],"mappings":";;;AAAA,iCAAgC;AAChC,iDAA+C;AAC/C,2CAAqD;AACrD,+BAA4C;AAoB5C;;;GAGG;AACH;IA8BE;;;OAGG;IACH,gBAAY,MAAgC,EAAE,OAAgB;QAC5D,IAAI,CAAC,CAAC,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,CAAC,MAAM,EAAE,OAAO,CAAC,CAAC;QAElE,IACE,CAAC,CAAC,MAAM,IAAI,IAAI,CAAC;YACjB,CAAC,CAAC,OAAO,MAAM,KAAK,QAAQ,CAAC;YAC7B,CAAC,WAAW,CAAC,MAAM,CAAC,MAAM,CAAC;YAC3B,CAAC,CAAC,MAAM,YAAY,WAAW,CAAC;YAChC,CAAC,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EACtB;YACA,MAAM,IAAI,SAAS,CACjB,kFAAkF,CACnF,CAAC;SACH;QAED,IAAI,CAAC,QAAQ,GAAG,OAAO,aAAP,OAAO,cAAP,OAAO,GAAI,MAAM,CAAC,2BAA2B,CAAC;QAE9D,IAAI,MAAM,IAAI,IAAI,EAAE;YAClB,gCAAgC;YAChC,IAAI,CAAC,MAAM,GAAG,eAAM,CAAC,KAAK,CAAC,MAAM,CAAC,WAAW,CAAC,CAAC;YAC/C,IAAI,CAAC,QAAQ,GAAG,CAAC,CAAC;SACnB;aAAM;YACL,IAAI,OAAO,MAAM,KAAK,QAAQ,EAAE;gBAC9B,SAAS;gBACT,IAAI,CAAC,MAAM,GAAG,eAAM,CAAC,IAAI,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;aAC7C;iBAAM,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;gBAChC,WAAW;gBACX,IAAI,CAAC,MAAM,GAAG,eAAM,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;aACnC;iBAAM;gBACL,oCAAoC;gBACpC,IAAI,CAAC,MAAM,GAAG,4BAAY,CAAC,MAAM,CAAC,CAAC;aACpC;YAED,IAAI,CAAC,QAAQ,GAAG,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;SACxC;IACH,CAAC;IAED;;;;OAIG;IACH,oBAAG,GAAH,UAAI,SAA2D;QAC7D,oEAAoE;QACpE,IAAI,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,CAAC,MAAM,KAAK,CAAC,EAAE;YAC3D,MAAM,IAAI,SAAS,CAAC,sCAAsC,CAAC,CAAC;SAC7D;aAAM,IAAI,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,CAAC,MAAM,KAAK,CAAC;YAChE,MAAM,IAAI,SAAS,CAAC,mDAAmD,CAAC,CAAC;QAE3E,6BAA6B;QAC7B,IAAI,WAAmB,CAAC;QACxB,IAAI,OAAO,SAAS,KAAK,QAAQ,EAAE;YACjC,WAAW,GAAG,SAAS,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;SACvC;aAAM,IAAI,OAAO,SAAS,KAAK,QAAQ,EAAE;YACxC,WAAW,GAAG,SAAS,CAAC;SACzB;aAAM;YACL,WAAW,GAAG,SAAS,CAAC,CAAC,CAAC,CAAC;SAC5B;QAED,IAAI,WAAW,GAAG,CAAC,IAAI,WAAW,GAAG,GAAG,EAAE;YACxC,MAAM,IAAI,SAAS,CAAC,0DAA0D,CAAC,CAAC;SACjF;QAED,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,EAAE;YACtC,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,GAAG,WAAW,CAAC;SAC5C;aAAM;YACL,IAAM,MAAM,GAAG,eAAM,CAAC,KAAK,CAAC,MAAM,CAAC,WAAW,GAAG,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;YACrE,mCAAmC;YACnC,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;YACnD,IAAI,CAAC,MAAM,GAAG,MAAM,CAAC;YACrB,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,GAAG,WAAW,CAAC;SAC5C;IACH,CAAC;IAED;;;;;OAKG;IACH,sBAAK,GAAL,UAAM,QAAiC,EAAE,MAAc;QACrD,MAAM,GAAG,OAAO,MAAM,KAAK,QAAQ,CAAC,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,IAAI,CAAC,QAAQ,CAAC;QAE7D,oDAAoD;QACpD,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,MAAM,GAAG,QAAQ,CAAC,MAAM,EAAE;YACjD,IAAM,MAAM,GAAG,eAAM,CAAC,KAAK,CAAC,IAAI,CAAC,MAAM,CAAC,MAAM,GAAG,QAAQ,CAAC,MAAM,CAAC,CAAC;YAClE,IAAI,CAAC,MAAM,CAAC,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;YAEnD,wBAAwB;YACxB,IAAI,CAAC,MAAM,GAAG,MAAM,CAAC;SACtB;QAED,IAAI,WAAW,CAAC,MAAM,CAAC,QAAQ,CAAC,EAAE;YAChC,IAAI,CAAC,MAAM,CAAC,GAAG,CAAC,4BAAY,CAAC,QAAQ,CAAC,EAAE,MAAM,CAAC,CAAC;YAChD,IAAI,CAAC,QAAQ;gBACX,MAAM,GAAG,QAAQ,CAAC,UAAU,GAAG,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC,MAAM,GAAG,QAAQ,CAAC,MAAM,CAAC,CAAC,CAAC,IAAI,CAAC,QAAQ,CAAC;SAC3F;aAAM,IAAI,OAAO,QAAQ,KAAK,QAAQ,EAAE;YACvC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,QAAQ,EAAE,MAAM,EAAE,QAAQ,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;YAC/D,IAAI,CAAC,QAAQ;gBACX,MAAM,GAAG,QAAQ,CAAC,MAAM,GAAG,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC,MAAM,GAAG,QAAQ,CAAC,MAAM,CAAC,CAAC,CAAC,IAAI,CAAC,QAAQ,CAAC;SACvF;IACH,CAAC;IAED;;;;;OAKG;IACH,qBAAI,GAAJ,UAAK,QAAgB,EAAE,MAAc;QACnC,MAAM,GAAG,MAAM,IAAI,MAAM,GAAG,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,IAAI,CAAC,QAAQ,CAAC;QAEvD,kDAAkD;QAClD,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,QAAQ,EAAE,QAAQ,GAAG,MAAM,CAAC,CAAC;IACxD,CAAC;IAED;;;;;OAKG;IACH,sBAAK,GAAL,UAAM,KAAe;QACnB,KAAK,GAAG,CAAC,CAAC,KAAK,CAAC;QAEhB,2EAA2E;QAC3E,IAAI,KAAK,IAAI,IAAI,CAAC,MAAM,CAAC,MAAM,KAAK,IAAI,CAAC,QAAQ,EAAE;YACjD,OAAO,IAAI,CAAC,MAAM,CAAC;SACpB;QAED,kCAAkC;QAClC,IAAI,KAAK,EAAE;YACT,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC5C;QACD,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;IAC1D,CAAC;IAED,wCAAwC;IACxC,uBAAM,GAAN;QACE,OAAO,IAAI,CAAC,QAAQ,CAAC;IACvB,CAAC;IAED,gBAAgB;IAChB,uBAAM,GAAN;QACE,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;IACxC,CAAC;IAED,gBAAgB;IAChB,yBAAQ,GAAR,UAAS,MAAc;QACrB,OAAO,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC;IACtC,CAAC;IAED,gBAAgB;IAChB,+BAAc,GAAd,UAAe,OAAsB;QACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAM,YAAY,GAAG,IAAI,CAAC,MAAM,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;QAEpD,IAAM,OAAO,GAAG,MAAM,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,QAAQ,CAAC,EAAE,CAAC,CAAC;QACnD,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO;gBACL,OAAO,EAAE,YAAY;gBACrB,KAAK,EAAE,OAAO,CAAC,MAAM,KAAK,CAAC,CAAC,CAAC,CAAC,GAAG,GAAG,OAAO,CAAC,CAAC,CAAC,OAAO;aACtD,CAAC;SACH;QACD,OAAO;YACL,OAAO,EAAE;gBACP,MAAM,EAAE,YAAY;gBACpB,OAAO,EAAE,OAAO,CAAC,MAAM,KAAK,CAAC,CAAC,CAAC,CAAC,GAAG,GAAG,OAAO,CAAC,CAAC,CAAC,OAAO;aACxD;SACF,CAAC;IACJ,CAAC;IAED,gBAAgB;IAChB,uBAAM,GAAN;QACE,IAAI,IAAI,CAAC,QAAQ,KAAK,MAAM,CAAC,YAAY,EAAE;YACzC,OAAO,IAAI,WAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC;SACtD;QAED,MAAM,IAAI,KAAK,CACb,uBAAoB,IAAI,CAAC,QAAQ,2DAAoD,MAAM,CAAC,YAAY,+BAA2B,CACpI,CAAC;IACJ,CAAC;IAED,gBAAgB;IACT,uBAAgB,GAAvB,UACE,GAAyD,EACzD,OAAsB;QAEtB,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAI,IAAwB,CAAC;QAC7B,IAAI,IAAI,CAAC;QACT,IAAI,SAAS,IAAI,GAAG,EAAE;YACpB,IAAI,OAAO,CAAC,MAAM,IAAI,OAAO,GAAG,CAAC,OAAO,KAAK,QAAQ,IAAI,OAAO,IAAI,GAAG,EAAE;gBACvE,IAAI,GAAG,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,QAAQ,CAAC,GAAG,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;gBAC/C,IAAI,GAAG,eAAM,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;aAC3C;iBAAM;gBACL,IAAI,OAAO,GAAG,CAAC,OAAO,KAAK,QAAQ,EAAE;oBACnC,IAAI,GAAG,GAAG,CAAC,OAAO,CAAC,OAAO,CAAC,CAAC,CAAC,QAAQ,CAAC,GAAG,CAAC,OAAO,CAAC,OAAO,EAAE,EAAE,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACnE,IAAI,GAAG,eAAM,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,MAAM,EAAE,QAAQ,CAAC,CAAC;iBAClD;aACF;SACF;aAAM,IAAI,OAAO,IAAI,GAAG,EAAE;YACzB,IAAI,GAAG,CAAC,CAAC;YACT,IAAI,GAAG,kCAAqB,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC;SACzC;QACD,IAAI,CAAC,IAAI,EAAE;YACT,MAAM,IAAI,SAAS,CAAC,4CAA0C,IAAI,CAAC,SAAS,CAAC,GAAG,CAAG,CAAC,CAAC;SACtF;QACD,OAAO,IAAI,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;IAChC,CAAC;IAED,gBAAgB;IAChB,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,wBAAO,GAAP;QACE,IAAM,QAAQ,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;QAClC,OAAO,8BAA2B,QAAQ,CAAC,QAAQ,CAAC,KAAK,CAAC,sBAAc,IAAI,CAAC,QAAQ,MAAG,CAAC;IAC3F,CAAC;IA1PD;;;OAGG;IACqB,kCAA2B,GAAG,CAAC,CAAC;IAExD,kCAAkC;IAClB,kBAAW,GAAG,GAAG,CAAC;IAClC,wBAAwB;IACR,sBAAe,GAAG,CAAC,CAAC;IACpC,yBAAyB;IACT,uBAAgB,GAAG,CAAC,CAAC;IACrC,2BAA2B;IACX,yBAAkB,GAAG,CAAC,CAAC;IACvC,oEAAoE;IACpD,uBAAgB,GAAG,CAAC,CAAC;IACrC,qBAAqB;IACL,mBAAY,GAAG,CAAC,CAAC;IACjC,oBAAoB;IACJ,kBAAW,GAAG,CAAC,CAAC;IAChC,qBAAqB;IACL,2BAAoB,GAAG,GAAG,CAAC;IAsO7C,aAAC;CAAA,AA9PD,IA8PC;AA9PY,wBAAM;AAgQnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/bson.js b/node_modules/bson/lib/bson.js
new file mode 100644
index 0000000..710e597
--- /dev/null
+++ b/node_modules/bson/lib/bson.js
@@ -0,0 +1,244 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.ObjectID = exports.Decimal128 = exports.BSONRegExp = exports.MaxKey = exports.MinKey = exports.Int32 = exports.Double = exports.Timestamp = exports.Long = exports.UUID = exports.ObjectId = exports.Binary = exports.DBRef = exports.BSONSymbol = exports.Map = exports.Code = exports.LongWithoutOverridesClass = exports.EJSON = exports.BSON_INT64_MIN = exports.BSON_INT64_MAX = exports.BSON_INT32_MIN = exports.BSON_INT32_MAX = exports.BSON_DATA_UNDEFINED = exports.BSON_DATA_TIMESTAMP = exports.BSON_DATA_SYMBOL = exports.BSON_DATA_STRING = exports.BSON_DATA_REGEXP = exports.BSON_DATA_OID = exports.BSON_DATA_OBJECT = exports.BSON_DATA_NUMBER = exports.BSON_DATA_NULL = exports.BSON_DATA_MIN_KEY = exports.BSON_DATA_MAX_KEY = exports.BSON_DATA_LONG = exports.BSON_DATA_INT = exports.BSON_DATA_DECIMAL128 = exports.BSON_DATA_DBPOINTER = exports.BSON_DATA_DATE = exports.BSON_DATA_CODE_W_SCOPE = exports.BSON_DATA_CODE = exports.BSON_DATA_BOOLEAN = exports.BSON_DATA_BINARY = exports.BSON_DATA_ARRAY = exports.BSON_BINARY_SUBTYPE_UUID_NEW = exports.BSON_BINARY_SUBTYPE_UUID = exports.BSON_BINARY_SUBTYPE_USER_DEFINED = exports.BSON_BINARY_SUBTYPE_MD5 = exports.BSON_BINARY_SUBTYPE_FUNCTION = exports.BSON_BINARY_SUBTYPE_DEFAULT = exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = void 0;
+exports.deserializeStream = exports.calculateObjectSize = exports.deserialize = exports.serializeWithBufferAndIndex = exports.serialize = exports.setInternalBufferSize = void 0;
+var buffer_1 = require("buffer");
+var binary_1 = require("./binary");
+Object.defineProperty(exports, "Binary", { enumerable: true, get: function () { return binary_1.Binary; } });
+var code_1 = require("./code");
+Object.defineProperty(exports, "Code", { enumerable: true, get: function () { return code_1.Code; } });
+var db_ref_1 = require("./db_ref");
+Object.defineProperty(exports, "DBRef", { enumerable: true, get: function () { return db_ref_1.DBRef; } });
+var decimal128_1 = require("./decimal128");
+Object.defineProperty(exports, "Decimal128", { enumerable: true, get: function () { return decimal128_1.Decimal128; } });
+var double_1 = require("./double");
+Object.defineProperty(exports, "Double", { enumerable: true, get: function () { return double_1.Double; } });
+var ensure_buffer_1 = require("./ensure_buffer");
+var extended_json_1 = require("./extended_json");
+var int_32_1 = require("./int_32");
+Object.defineProperty(exports, "Int32", { enumerable: true, get: function () { return int_32_1.Int32; } });
+var long_1 = require("./long");
+Object.defineProperty(exports, "Long", { enumerable: true, get: function () { return long_1.Long; } });
+var map_1 = require("./map");
+Object.defineProperty(exports, "Map", { enumerable: true, get: function () { return map_1.Map; } });
+var max_key_1 = require("./max_key");
+Object.defineProperty(exports, "MaxKey", { enumerable: true, get: function () { return max_key_1.MaxKey; } });
+var min_key_1 = require("./min_key");
+Object.defineProperty(exports, "MinKey", { enumerable: true, get: function () { return min_key_1.MinKey; } });
+var objectid_1 = require("./objectid");
+Object.defineProperty(exports, "ObjectId", { enumerable: true, get: function () { return objectid_1.ObjectId; } });
+Object.defineProperty(exports, "ObjectID", { enumerable: true, get: function () { return objectid_1.ObjectId; } });
+var calculate_size_1 = require("./parser/calculate_size");
+// Parts of the parser
+var deserializer_1 = require("./parser/deserializer");
+var serializer_1 = require("./parser/serializer");
+var regexp_1 = require("./regexp");
+Object.defineProperty(exports, "BSONRegExp", { enumerable: true, get: function () { return regexp_1.BSONRegExp; } });
+var symbol_1 = require("./symbol");
+Object.defineProperty(exports, "BSONSymbol", { enumerable: true, get: function () { return symbol_1.BSONSymbol; } });
+var timestamp_1 = require("./timestamp");
+Object.defineProperty(exports, "Timestamp", { enumerable: true, get: function () { return timestamp_1.Timestamp; } });
+var uuid_1 = require("./uuid");
+Object.defineProperty(exports, "UUID", { enumerable: true, get: function () { return uuid_1.UUID; } });
+var constants_1 = require("./constants");
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_BYTE_ARRAY", { enumerable: true, get: function () { return constants_1.BSON_BINARY_SUBTYPE_BYTE_ARRAY; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_DEFAULT", { enumerable: true, get: function () { return constants_1.BSON_BINARY_SUBTYPE_DEFAULT; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_FUNCTION", { enumerable: true, get: function () { return constants_1.BSON_BINARY_SUBTYPE_FUNCTION; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_MD5", { enumerable: true, get: function () { return constants_1.BSON_BINARY_SUBTYPE_MD5; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_USER_DEFINED", { enumerable: true, get: function () { return constants_1.BSON_BINARY_SUBTYPE_USER_DEFINED; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_UUID", { enumerable: true, get: function () { return constants_1.BSON_BINARY_SUBTYPE_UUID; } });
+Object.defineProperty(exports, "BSON_BINARY_SUBTYPE_UUID_NEW", { enumerable: true, get: function () { return constants_1.BSON_BINARY_SUBTYPE_UUID_NEW; } });
+Object.defineProperty(exports, "BSON_DATA_ARRAY", { enumerable: true, get: function () { return constants_1.BSON_DATA_ARRAY; } });
+Object.defineProperty(exports, "BSON_DATA_BINARY", { enumerable: true, get: function () { return constants_1.BSON_DATA_BINARY; } });
+Object.defineProperty(exports, "BSON_DATA_BOOLEAN", { enumerable: true, get: function () { return constants_1.BSON_DATA_BOOLEAN; } });
+Object.defineProperty(exports, "BSON_DATA_CODE", { enumerable: true, get: function () { return constants_1.BSON_DATA_CODE; } });
+Object.defineProperty(exports, "BSON_DATA_CODE_W_SCOPE", { enumerable: true, get: function () { return constants_1.BSON_DATA_CODE_W_SCOPE; } });
+Object.defineProperty(exports, "BSON_DATA_DATE", { enumerable: true, get: function () { return constants_1.BSON_DATA_DATE; } });
+Object.defineProperty(exports, "BSON_DATA_DBPOINTER", { enumerable: true, get: function () { return constants_1.BSON_DATA_DBPOINTER; } });
+Object.defineProperty(exports, "BSON_DATA_DECIMAL128", { enumerable: true, get: function () { return constants_1.BSON_DATA_DECIMAL128; } });
+Object.defineProperty(exports, "BSON_DATA_INT", { enumerable: true, get: function () { return constants_1.BSON_DATA_INT; } });
+Object.defineProperty(exports, "BSON_DATA_LONG", { enumerable: true, get: function () { return constants_1.BSON_DATA_LONG; } });
+Object.defineProperty(exports, "BSON_DATA_MAX_KEY", { enumerable: true, get: function () { return constants_1.BSON_DATA_MAX_KEY; } });
+Object.defineProperty(exports, "BSON_DATA_MIN_KEY", { enumerable: true, get: function () { return constants_1.BSON_DATA_MIN_KEY; } });
+Object.defineProperty(exports, "BSON_DATA_NULL", { enumerable: true, get: function () { return constants_1.BSON_DATA_NULL; } });
+Object.defineProperty(exports, "BSON_DATA_NUMBER", { enumerable: true, get: function () { return constants_1.BSON_DATA_NUMBER; } });
+Object.defineProperty(exports, "BSON_DATA_OBJECT", { enumerable: true, get: function () { return constants_1.BSON_DATA_OBJECT; } });
+Object.defineProperty(exports, "BSON_DATA_OID", { enumerable: true, get: function () { return constants_1.BSON_DATA_OID; } });
+Object.defineProperty(exports, "BSON_DATA_REGEXP", { enumerable: true, get: function () { return constants_1.BSON_DATA_REGEXP; } });
+Object.defineProperty(exports, "BSON_DATA_STRING", { enumerable: true, get: function () { return constants_1.BSON_DATA_STRING; } });
+Object.defineProperty(exports, "BSON_DATA_SYMBOL", { enumerable: true, get: function () { return constants_1.BSON_DATA_SYMBOL; } });
+Object.defineProperty(exports, "BSON_DATA_TIMESTAMP", { enumerable: true, get: function () { return constants_1.BSON_DATA_TIMESTAMP; } });
+Object.defineProperty(exports, "BSON_DATA_UNDEFINED", { enumerable: true, get: function () { return constants_1.BSON_DATA_UNDEFINED; } });
+Object.defineProperty(exports, "BSON_INT32_MAX", { enumerable: true, get: function () { return constants_1.BSON_INT32_MAX; } });
+Object.defineProperty(exports, "BSON_INT32_MIN", { enumerable: true, get: function () { return constants_1.BSON_INT32_MIN; } });
+Object.defineProperty(exports, "BSON_INT64_MAX", { enumerable: true, get: function () { return constants_1.BSON_INT64_MAX; } });
+Object.defineProperty(exports, "BSON_INT64_MIN", { enumerable: true, get: function () { return constants_1.BSON_INT64_MIN; } });
+var extended_json_2 = require("./extended_json");
+Object.defineProperty(exports, "EJSON", { enumerable: true, get: function () { return extended_json_2.EJSON; } });
+var timestamp_2 = require("./timestamp");
+Object.defineProperty(exports, "LongWithoutOverridesClass", { enumerable: true, get: function () { return timestamp_2.LongWithoutOverridesClass; } });
+/** @internal */
+// Default Max Size
+var MAXSIZE = 1024 * 1024 * 17;
+// Current Internal Temporary Serialization Buffer
+var buffer = buffer_1.Buffer.alloc(MAXSIZE);
+/**
+ * Sets the size of the internal serialization buffer.
+ *
+ * @param size - The desired size for the internal serialization buffer
+ * @public
+ */
+function setInternalBufferSize(size) {
+ // Resize the internal serialization buffer if needed
+ if (buffer.length < size) {
+ buffer = buffer_1.Buffer.alloc(size);
+ }
+}
+exports.setInternalBufferSize = setInternalBufferSize;
+/**
+ * Serialize a Javascript object.
+ *
+ * @param object - the Javascript object to serialize.
+ * @returns Buffer object containing the serialized object.
+ * @public
+ */
+function serialize(object, options) {
+ if (options === void 0) { options = {}; }
+ // Unpack the options
+ var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ var minInternalBufferSize = typeof options.minInternalBufferSize === 'number' ? options.minInternalBufferSize : MAXSIZE;
+ // Resize the internal serialization buffer if needed
+ if (buffer.length < minInternalBufferSize) {
+ buffer = buffer_1.Buffer.alloc(minInternalBufferSize);
+ }
+ // Attempt to serialize
+ var serializationIndex = serializer_1.serializeInto(buffer, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined, []);
+ // Create the final buffer
+ var finishedBuffer = buffer_1.Buffer.alloc(serializationIndex);
+ // Copy into the finished buffer
+ buffer.copy(finishedBuffer, 0, 0, finishedBuffer.length);
+ // Return the buffer
+ return finishedBuffer;
+}
+exports.serialize = serialize;
+/**
+ * Serialize a Javascript object using a predefined Buffer and index into the buffer,
+ * useful when pre-allocating the space for serialization.
+ *
+ * @param object - the Javascript object to serialize.
+ * @param finalBuffer - the Buffer you pre-allocated to store the serialized BSON object.
+ * @returns the index pointing to the last written byte in the buffer.
+ * @public
+ */
+function serializeWithBufferAndIndex(object, finalBuffer, options) {
+ if (options === void 0) { options = {}; }
+ // Unpack the options
+ var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ var startIndex = typeof options.index === 'number' ? options.index : 0;
+ // Attempt to serialize
+ var serializationIndex = serializer_1.serializeInto(buffer, object, checkKeys, 0, 0, serializeFunctions, ignoreUndefined);
+ buffer.copy(finalBuffer, startIndex, 0, serializationIndex);
+ // Return the index
+ return startIndex + serializationIndex - 1;
+}
+exports.serializeWithBufferAndIndex = serializeWithBufferAndIndex;
+/**
+ * Deserialize data as BSON.
+ *
+ * @param buffer - the buffer containing the serialized set of BSON documents.
+ * @returns returns the deserialized Javascript Object.
+ * @public
+ */
+function deserialize(buffer, options) {
+ if (options === void 0) { options = {}; }
+ return deserializer_1.deserialize(ensure_buffer_1.ensureBuffer(buffer), options);
+}
+exports.deserialize = deserialize;
+/**
+ * Calculate the bson size for a passed in Javascript object.
+ *
+ * @param object - the Javascript object to calculate the BSON byte size for
+ * @returns size of BSON object in bytes
+ * @public
+ */
+function calculateObjectSize(object, options) {
+ if (options === void 0) { options = {}; }
+ options = options || {};
+ var serializeFunctions = typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ var ignoreUndefined = typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ return calculate_size_1.calculateObjectSize(object, serializeFunctions, ignoreUndefined);
+}
+exports.calculateObjectSize = calculateObjectSize;
+/**
+ * Deserialize stream data as BSON documents.
+ *
+ * @param data - the buffer containing the serialized set of BSON documents.
+ * @param startIndex - the start index in the data Buffer where the deserialization is to start.
+ * @param numberOfDocuments - number of documents to deserialize.
+ * @param documents - an array where to store the deserialized documents.
+ * @param docStartIndex - the index in the documents array from where to start inserting documents.
+ * @param options - additional options used for the deserialization.
+ * @returns next index in the buffer after deserialization **x** numbers of documents.
+ * @public
+ */
+function deserializeStream(data, startIndex, numberOfDocuments, documents, docStartIndex, options) {
+ var internalOptions = Object.assign({ allowObjectSmallerThanBufferSize: true, index: 0 }, options);
+ var bufferData = ensure_buffer_1.ensureBuffer(data);
+ var index = startIndex;
+ // Loop over all documents
+ for (var i = 0; i < numberOfDocuments; i++) {
+ // Find size of the document
+ var size = bufferData[index] |
+ (bufferData[index + 1] << 8) |
+ (bufferData[index + 2] << 16) |
+ (bufferData[index + 3] << 24);
+ // Update options with index
+ internalOptions.index = index;
+ // Parse the document at this point
+ documents[docStartIndex + i] = deserializer_1.deserialize(bufferData, internalOptions);
+ // Adjust index by the document size
+ index = index + size;
+ }
+ // Return object containing end index of parsing and list of documents
+ return index;
+}
+exports.deserializeStream = deserializeStream;
+/**
+ * BSON default export
+ * @deprecated Please use named exports
+ * @privateRemarks
+ * We want to someday deprecate the default export,
+ * so none of the new TS types are being exported on the default
+ * @public
+ */
+var BSON = {
+ Binary: binary_1.Binary,
+ Code: code_1.Code,
+ DBRef: db_ref_1.DBRef,
+ Decimal128: decimal128_1.Decimal128,
+ Double: double_1.Double,
+ Int32: int_32_1.Int32,
+ Long: long_1.Long,
+ UUID: uuid_1.UUID,
+ Map: map_1.Map,
+ MaxKey: max_key_1.MaxKey,
+ MinKey: min_key_1.MinKey,
+ ObjectId: objectid_1.ObjectId,
+ ObjectID: objectid_1.ObjectId,
+ BSONRegExp: regexp_1.BSONRegExp,
+ BSONSymbol: symbol_1.BSONSymbol,
+ Timestamp: timestamp_1.Timestamp,
+ EJSON: extended_json_1.EJSON,
+ setInternalBufferSize: setInternalBufferSize,
+ serialize: serialize,
+ serializeWithBufferAndIndex: serializeWithBufferAndIndex,
+ deserialize: deserialize,
+ calculateObjectSize: calculateObjectSize,
+ deserializeStream: deserializeStream
+};
+exports.default = BSON;
+//# sourceMappingURL=bson.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/bson.js.map b/node_modules/bson/lib/bson.js.map
new file mode 100644
index 0000000..e899606
--- /dev/null
+++ b/node_modules/bson/lib/bson.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"bson.js","sourceRoot":"","sources":["../src/bson.ts"],"names":[],"mappings":";;;;AAAA,iCAAgC;AAChC,mCAAkC;AAiFhC,uFAjFO,eAAM,OAiFP;AAhFR,+BAA8B;AA4E5B,qFA5EO,WAAI,OA4EP;AA3EN,mCAAiC;AA8E/B,sFA9EO,cAAK,OA8EP;AA7EP,2CAA0C;AAwFxC,2FAxFO,uBAAU,OAwFP;AAvFZ,mCAAkC;AAkFhC,uFAlFO,eAAM,OAkFP;AAjFR,iDAA+C;AAC/C,iDAAwC;AACxC,mCAAiC;AAgF/B,sFAhFO,cAAK,OAgFP;AA/EP,+BAA8B;AA4E5B,qFA5EO,WAAI,OA4EP;AA3EN,6BAA4B;AAqE1B,oFArEO,SAAG,OAqEP;AApEL,qCAAmC;AA+EjC,uFA/EO,gBAAM,OA+EP;AA9ER,qCAAmC;AA6EjC,uFA7EO,gBAAM,OA6EP;AA5ER,uCAAsC;AAsEpC,yFAtEO,mBAAQ,OAsEP;AAaI,yFAnFL,mBAAQ,OAmFK;AAlFtB,0DAA6F;AAC7F,sBAAsB;AACtB,sDAA+F;AAC/F,kDAA2F;AAC3F,mCAAsC;AAyEpC,2FAzEO,mBAAU,OAyEP;AAxEZ,mCAAsC;AA6DpC,2FA7DO,mBAAU,OA6DP;AA5DZ,yCAAwC;AAkEtC,0FAlEO,qBAAS,OAkEP;AAjEX,+BAA8B;AA+D5B,qFA/DO,WAAI,OA+DP;AA5DN,yCAiCqB;AAhCnB,2HAAA,8BAA8B,OAAA;AAC9B,wHAAA,2BAA2B,OAAA;AAC3B,yHAAA,4BAA4B,OAAA;AAC5B,oHAAA,uBAAuB,OAAA;AACvB,6HAAA,gCAAgC,OAAA;AAChC,qHAAA,wBAAwB,OAAA;AACxB,yHAAA,4BAA4B,OAAA;AAC5B,4GAAA,eAAe,OAAA;AACf,6GAAA,gBAAgB,OAAA;AAChB,8GAAA,iBAAiB,OAAA;AACjB,2GAAA,cAAc,OAAA;AACd,mHAAA,sBAAsB,OAAA;AACtB,2GAAA,cAAc,OAAA;AACd,gHAAA,mBAAmB,OAAA;AACnB,iHAAA,oBAAoB,OAAA;AACpB,0GAAA,aAAa,OAAA;AACb,2GAAA,cAAc,OAAA;AACd,8GAAA,iBAAiB,OAAA;AACjB,8GAAA,iBAAiB,OAAA;AACjB,2GAAA,cAAc,OAAA;AACd,6GAAA,gBAAgB,OAAA;AAChB,6GAAA,gBAAgB,OAAA;AAChB,0GAAA,aAAa,OAAA;AACb,6GAAA,gBAAgB,OAAA;AAChB,6GAAA,gBAAgB,OAAA;AAChB,6GAAA,gBAAgB,OAAA;AAChB,gHAAA,mBAAmB,OAAA;AACnB,gHAAA,mBAAmB,OAAA;AACnB,2GAAA,cAAc,OAAA;AACd,2GAAA,cAAc,OAAA;AACd,2GAAA,cAAc,OAAA;AACd,2GAAA,cAAc,OAAA;AAKhB,iDAAsD;AAA7C,sGAAA,KAAK,OAAA;AAQd,yCAKqB;AAHnB,sHAAA,yBAAyB,OAAA;AAkC3B,gBAAgB;AAChB,mBAAmB;AACnB,IAAM,OAAO,GAAG,IAAI,GAAG,IAAI,GAAG,EAAE,CAAC;AAEjC,kDAAkD;AAClD,IAAI,MAAM,GAAG,eAAM,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC;AAEnC;;;;;GAKG;AACH,SAAgB,qBAAqB,CAAC,IAAY;IAChD,qDAAqD;IACrD,IAAI,MAAM,CAAC,MAAM,GAAG,IAAI,EAAE;QACxB,MAAM,GAAG,eAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;KAC7B;AACH,CAAC;AALD,sDAKC;AAED;;;;;;GAMG;AACH,SAAgB,SAAS,CAAC,MAAgB,EAAE,OAA8B;IAA9B,wBAAA,EAAA,YAA8B;IACxE,qBAAqB;IACrB,IAAM,SAAS,GAAG,OAAO,OAAO,CAAC,SAAS,KAAK,SAAS,CAAC,CAAC,CAAC,OAAO,CAAC,SAAS,CAAC,CAAC,CAAC,KAAK,CAAC;IACrF,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,CAAC,CAAC,CAAC,OAAO,CAAC,kBAAkB,CAAC,CAAC,CAAC,KAAK,CAAC;IACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,CAAC,CAAC,CAAC,OAAO,CAAC,eAAe,CAAC,CAAC,CAAC,IAAI,CAAC;IAChF,IAAM,qBAAqB,GACzB,OAAO,OAAO,CAAC,qBAAqB,KAAK,QAAQ,CAAC,CAAC,CAAC,OAAO,CAAC,qBAAqB,CAAC,CAAC,CAAC,OAAO,CAAC;IAE9F,qDAAqD;IACrD,IAAI,MAAM,CAAC,MAAM,GAAG,qBAAqB,EAAE;QACzC,MAAM,GAAG,eAAM,CAAC,KAAK,CAAC,qBAAqB,CAAC,CAAC;KAC9C;IAED,uBAAuB;IACvB,IAAM,kBAAkB,GAAG,0BAAiB,CAC1C,MAAM,EACN,MAAM,EACN,SAAS,EACT,CAAC,EACD,CAAC,EACD,kBAAkB,EAClB,eAAe,EACf,EAAE,CACH,CAAC;IAEF,0BAA0B;IAC1B,IAAM,cAAc,GAAG,eAAM,CAAC,KAAK,CAAC,kBAAkB,CAAC,CAAC;IAExD,gCAAgC;IAChC,MAAM,CAAC,IAAI,CAAC,cAAc,EAAE,CAAC,EAAE,CAAC,EAAE,cAAc,CAAC,MAAM,CAAC,CAAC;IAEzD,oBAAoB;IACpB,OAAO,cAAc,CAAC;AACxB,CAAC;AAnCD,8BAmCC;AAED;;;;;;;;GAQG;AACH,SAAgB,2BAA2B,CACzC,MAAgB,EAChB,WAAmB,EACnB,OAA8B;IAA9B,wBAAA,EAAA,YAA8B;IAE9B,qBAAqB;IACrB,IAAM,SAAS,GAAG,OAAO,OAAO,CAAC,SAAS,KAAK,SAAS,CAAC,CAAC,CAAC,OAAO,CAAC,SAAS,CAAC,CAAC,CAAC,KAAK,CAAC;IACrF,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,CAAC,CAAC,CAAC,OAAO,CAAC,kBAAkB,CAAC,CAAC,CAAC,KAAK,CAAC;IACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,CAAC,CAAC,CAAC,OAAO,CAAC,eAAe,CAAC,CAAC,CAAC,IAAI,CAAC;IAChF,IAAM,UAAU,GAAG,OAAO,OAAO,CAAC,KAAK,KAAK,QAAQ,CAAC,CAAC,CAAC,OAAO,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;IAEzE,uBAAuB;IACvB,IAAM,kBAAkB,GAAG,0BAAiB,CAC1C,MAAM,EACN,MAAM,EACN,SAAS,EACT,CAAC,EACD,CAAC,EACD,kBAAkB,EAClB,eAAe,CAChB,CAAC;IACF,MAAM,CAAC,IAAI,CAAC,WAAW,EAAE,UAAU,EAAE,CAAC,EAAE,kBAAkB,CAAC,CAAC;IAE5D,mBAAmB;IACnB,OAAO,UAAU,GAAG,kBAAkB,GAAG,CAAC,CAAC;AAC7C,CAAC;AA3BD,kEA2BC;AAED;;;;;;GAMG;AACH,SAAgB,WAAW,CACzB,MAA8C,EAC9C,OAAgC;IAAhC,wBAAA,EAAA,YAAgC;IAEhC,OAAO,0BAAmB,CAAC,4BAAY,CAAC,MAAM,CAAC,EAAE,OAAO,CAAC,CAAC;AAC5D,CAAC;AALD,kCAKC;AAQD;;;;;;GAMG;AACH,SAAgB,mBAAmB,CACjC,MAAgB,EAChB,OAAwC;IAAxC,wBAAA,EAAA,YAAwC;IAExC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;IAExB,IAAM,kBAAkB,GACtB,OAAO,OAAO,CAAC,kBAAkB,KAAK,SAAS,CAAC,CAAC,CAAC,OAAO,CAAC,kBAAkB,CAAC,CAAC,CAAC,KAAK,CAAC;IACvF,IAAM,eAAe,GACnB,OAAO,OAAO,CAAC,eAAe,KAAK,SAAS,CAAC,CAAC,CAAC,OAAO,CAAC,eAAe,CAAC,CAAC,CAAC,IAAI,CAAC;IAEhF,OAAO,oCAA2B,CAAC,MAAM,EAAE,kBAAkB,EAAE,eAAe,CAAC,CAAC;AAClF,CAAC;AAZD,kDAYC;AAED;;;;;;;;;;;GAWG;AACH,SAAgB,iBAAiB,CAC/B,IAA4C,EAC5C,UAAkB,EAClB,iBAAyB,EACzB,SAAqB,EACrB,aAAqB,EACrB,OAA2B;IAE3B,IAAM,eAAe,GAAG,MAAM,CAAC,MAAM,CACnC,EAAE,gCAAgC,EAAE,IAAI,EAAE,KAAK,EAAE,CAAC,EAAE,EACpD,OAAO,CACR,CAAC;IACF,IAAM,UAAU,GAAG,4BAAY,CAAC,IAAI,CAAC,CAAC;IAEtC,IAAI,KAAK,GAAG,UAAU,CAAC;IACvB,0BAA0B;IAC1B,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,iBAAiB,EAAE,CAAC,EAAE,EAAE;QAC1C,4BAA4B;QAC5B,IAAM,IAAI,GACR,UAAU,CAAC,KAAK,CAAC;YACjB,CAAC,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;YAC5B,CAAC,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;YAC7B,CAAC,UAAU,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;QAChC,4BAA4B;QAC5B,eAAe,CAAC,KAAK,GAAG,KAAK,CAAC;QAC9B,mCAAmC;QACnC,SAAS,CAAC,aAAa,GAAG,CAAC,CAAC,GAAG,0BAAmB,CAAC,UAAU,EAAE,eAAe,CAAC,CAAC;QAChF,oCAAoC;QACpC,KAAK,GAAG,KAAK,GAAG,IAAI,CAAC;KACtB;IAED,sEAAsE;IACtE,OAAO,KAAK,CAAC;AACf,CAAC;AAjCD,8CAiCC;AAED;;;;;;;GAOG;AACH,IAAM,IAAI,GAAG;IACX,MAAM,iBAAA;IACN,IAAI,aAAA;IACJ,KAAK,gBAAA;IACL,UAAU,yBAAA;IACV,MAAM,iBAAA;IACN,KAAK,gBAAA;IACL,IAAI,aAAA;IACJ,IAAI,aAAA;IACJ,GAAG,WAAA;IACH,MAAM,kBAAA;IACN,MAAM,kBAAA;IACN,QAAQ,qBAAA;IACR,QAAQ,EAAE,mBAAQ;IAClB,UAAU,qBAAA;IACV,UAAU,qBAAA;IACV,SAAS,uBAAA;IACT,KAAK,uBAAA;IACL,qBAAqB,uBAAA;IACrB,SAAS,WAAA;IACT,2BAA2B,6BAAA;IAC3B,WAAW,aAAA;IACX,mBAAmB,qBAAA;IACnB,iBAAiB,mBAAA;CAClB,CAAC;AACF,kBAAe,IAAI,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/bson/binary.js b/node_modules/bson/lib/bson/binary.js
deleted file mode 100644
index 6d190bc..0000000
--- a/node_modules/bson/lib/bson/binary.js
+++ /dev/null
@@ -1,384 +0,0 @@
-/**
- * Module dependencies.
- * @ignore
- */
-
-// Test if we're in Node via presence of "global" not absence of "window"
-// to support hybrid environments like Electron
-if (typeof global !== 'undefined') {
- var Buffer = require('buffer').Buffer; // TODO just use global Buffer
-}
-
-var utils = require('./parser/utils');
-
-/**
- * A class representation of the BSON Binary type.
- *
- * Sub types
- * - **BSON.BSON_BINARY_SUBTYPE_DEFAULT**, default BSON type.
- * - **BSON.BSON_BINARY_SUBTYPE_FUNCTION**, BSON function type.
- * - **BSON.BSON_BINARY_SUBTYPE_BYTE_ARRAY**, BSON byte array type.
- * - **BSON.BSON_BINARY_SUBTYPE_UUID**, BSON uuid type.
- * - **BSON.BSON_BINARY_SUBTYPE_MD5**, BSON md5 type.
- * - **BSON.BSON_BINARY_SUBTYPE_USER_DEFINED**, BSON user defined type.
- *
- * @class
- * @param {Buffer} buffer a buffer object containing the binary data.
- * @param {Number} [subType] the option binary type.
- * @return {Binary}
- */
-function Binary(buffer, subType) {
- if (!(this instanceof Binary)) return new Binary(buffer, subType);
-
- if (
- buffer != null &&
- !(typeof buffer === 'string') &&
- !Buffer.isBuffer(buffer) &&
- !(buffer instanceof Uint8Array) &&
- !Array.isArray(buffer)
- ) {
- throw new Error('only String, Buffer, Uint8Array or Array accepted');
- }
-
- this._bsontype = 'Binary';
-
- if (buffer instanceof Number) {
- this.sub_type = buffer;
- this.position = 0;
- } else {
- this.sub_type = subType == null ? BSON_BINARY_SUBTYPE_DEFAULT : subType;
- this.position = 0;
- }
-
- if (buffer != null && !(buffer instanceof Number)) {
- // Only accept Buffer, Uint8Array or Arrays
- if (typeof buffer === 'string') {
- // Different ways of writing the length of the string for the different types
- if (typeof Buffer !== 'undefined') {
- this.buffer = utils.toBuffer(buffer);
- } else if (
- typeof Uint8Array !== 'undefined' ||
- Object.prototype.toString.call(buffer) === '[object Array]'
- ) {
- this.buffer = writeStringToArray(buffer);
- } else {
- throw new Error('only String, Buffer, Uint8Array or Array accepted');
- }
- } else {
- this.buffer = buffer;
- }
- this.position = buffer.length;
- } else {
- if (typeof Buffer !== 'undefined') {
- this.buffer = utils.allocBuffer(Binary.BUFFER_SIZE);
- } else if (typeof Uint8Array !== 'undefined') {
- this.buffer = new Uint8Array(new ArrayBuffer(Binary.BUFFER_SIZE));
- } else {
- this.buffer = new Array(Binary.BUFFER_SIZE);
- }
- // Set position to start of buffer
- this.position = 0;
- }
-}
-
-/**
- * Updates this binary with byte_value.
- *
- * @method
- * @param {string} byte_value a single byte we wish to write.
- */
-Binary.prototype.put = function put(byte_value) {
- // If it's a string and a has more than one character throw an error
- if (byte_value['length'] != null && typeof byte_value !== 'number' && byte_value.length !== 1)
- throw new Error('only accepts single character String, Uint8Array or Array');
- if ((typeof byte_value !== 'number' && byte_value < 0) || byte_value > 255)
- throw new Error('only accepts number in a valid unsigned byte range 0-255');
-
- // Decode the byte value once
- var decoded_byte = null;
- if (typeof byte_value === 'string') {
- decoded_byte = byte_value.charCodeAt(0);
- } else if (byte_value['length'] != null) {
- decoded_byte = byte_value[0];
- } else {
- decoded_byte = byte_value;
- }
-
- if (this.buffer.length > this.position) {
- this.buffer[this.position++] = decoded_byte;
- } else {
- if (typeof Buffer !== 'undefined' && Buffer.isBuffer(this.buffer)) {
- // Create additional overflow buffer
- var buffer = utils.allocBuffer(Binary.BUFFER_SIZE + this.buffer.length);
- // Combine the two buffers together
- this.buffer.copy(buffer, 0, 0, this.buffer.length);
- this.buffer = buffer;
- this.buffer[this.position++] = decoded_byte;
- } else {
- buffer = null;
- // Create a new buffer (typed or normal array)
- if (Object.prototype.toString.call(this.buffer) === '[object Uint8Array]') {
- buffer = new Uint8Array(new ArrayBuffer(Binary.BUFFER_SIZE + this.buffer.length));
- } else {
- buffer = new Array(Binary.BUFFER_SIZE + this.buffer.length);
- }
-
- // We need to copy all the content to the new array
- for (var i = 0; i < this.buffer.length; i++) {
- buffer[i] = this.buffer[i];
- }
-
- // Reassign the buffer
- this.buffer = buffer;
- // Write the byte
- this.buffer[this.position++] = decoded_byte;
- }
- }
-};
-
-/**
- * Writes a buffer or string to the binary.
- *
- * @method
- * @param {(Buffer|string)} string a string or buffer to be written to the Binary BSON object.
- * @param {number} offset specify the binary of where to write the content.
- * @return {null}
- */
-Binary.prototype.write = function write(string, offset) {
- offset = typeof offset === 'number' ? offset : this.position;
-
- // If the buffer is to small let's extend the buffer
- if (this.buffer.length < offset + string.length) {
- var buffer = null;
- // If we are in node.js
- if (typeof Buffer !== 'undefined' && Buffer.isBuffer(this.buffer)) {
- buffer = utils.allocBuffer(this.buffer.length + string.length);
- this.buffer.copy(buffer, 0, 0, this.buffer.length);
- } else if (Object.prototype.toString.call(this.buffer) === '[object Uint8Array]') {
- // Create a new buffer
- buffer = new Uint8Array(new ArrayBuffer(this.buffer.length + string.length));
- // Copy the content
- for (var i = 0; i < this.position; i++) {
- buffer[i] = this.buffer[i];
- }
- }
-
- // Assign the new buffer
- this.buffer = buffer;
- }
-
- if (typeof Buffer !== 'undefined' && Buffer.isBuffer(string) && Buffer.isBuffer(this.buffer)) {
- string.copy(this.buffer, offset, 0, string.length);
- this.position = offset + string.length > this.position ? offset + string.length : this.position;
- // offset = string.length
- } else if (
- typeof Buffer !== 'undefined' &&
- typeof string === 'string' &&
- Buffer.isBuffer(this.buffer)
- ) {
- this.buffer.write(string, offset, 'binary');
- this.position = offset + string.length > this.position ? offset + string.length : this.position;
- // offset = string.length;
- } else if (
- Object.prototype.toString.call(string) === '[object Uint8Array]' ||
- (Object.prototype.toString.call(string) === '[object Array]' && typeof string !== 'string')
- ) {
- for (i = 0; i < string.length; i++) {
- this.buffer[offset++] = string[i];
- }
-
- this.position = offset > this.position ? offset : this.position;
- } else if (typeof string === 'string') {
- for (i = 0; i < string.length; i++) {
- this.buffer[offset++] = string.charCodeAt(i);
- }
-
- this.position = offset > this.position ? offset : this.position;
- }
-};
-
-/**
- * Reads **length** bytes starting at **position**.
- *
- * @method
- * @param {number} position read from the given position in the Binary.
- * @param {number} length the number of bytes to read.
- * @return {Buffer}
- */
-Binary.prototype.read = function read(position, length) {
- length = length && length > 0 ? length : this.position;
-
- // Let's return the data based on the type we have
- if (this.buffer['slice']) {
- return this.buffer.slice(position, position + length);
- } else {
- // Create a buffer to keep the result
- var buffer =
- typeof Uint8Array !== 'undefined'
- ? new Uint8Array(new ArrayBuffer(length))
- : new Array(length);
- for (var i = 0; i < length; i++) {
- buffer[i] = this.buffer[position++];
- }
- }
- // Return the buffer
- return buffer;
-};
-
-/**
- * Returns the value of this binary as a string.
- *
- * @method
- * @return {string}
- */
-Binary.prototype.value = function value(asRaw) {
- asRaw = asRaw == null ? false : asRaw;
-
- // Optimize to serialize for the situation where the data == size of buffer
- if (
- asRaw &&
- typeof Buffer !== 'undefined' &&
- Buffer.isBuffer(this.buffer) &&
- this.buffer.length === this.position
- )
- return this.buffer;
-
- // If it's a node.js buffer object
- if (typeof Buffer !== 'undefined' && Buffer.isBuffer(this.buffer)) {
- return asRaw
- ? this.buffer.slice(0, this.position)
- : this.buffer.toString('binary', 0, this.position);
- } else {
- if (asRaw) {
- // we support the slice command use it
- if (this.buffer['slice'] != null) {
- return this.buffer.slice(0, this.position);
- } else {
- // Create a new buffer to copy content to
- var newBuffer =
- Object.prototype.toString.call(this.buffer) === '[object Uint8Array]'
- ? new Uint8Array(new ArrayBuffer(this.position))
- : new Array(this.position);
- // Copy content
- for (var i = 0; i < this.position; i++) {
- newBuffer[i] = this.buffer[i];
- }
- // Return the buffer
- return newBuffer;
- }
- } else {
- return convertArraytoUtf8BinaryString(this.buffer, 0, this.position);
- }
- }
-};
-
-/**
- * Length.
- *
- * @method
- * @return {number} the length of the binary.
- */
-Binary.prototype.length = function length() {
- return this.position;
-};
-
-/**
- * @ignore
- */
-Binary.prototype.toJSON = function() {
- return this.buffer != null ? this.buffer.toString('base64') : '';
-};
-
-/**
- * @ignore
- */
-Binary.prototype.toString = function(format) {
- return this.buffer != null ? this.buffer.slice(0, this.position).toString(format) : '';
-};
-
-/**
- * Binary default subtype
- * @ignore
- */
-var BSON_BINARY_SUBTYPE_DEFAULT = 0;
-
-/**
- * @ignore
- */
-var writeStringToArray = function(data) {
- // Create a buffer
- var buffer =
- typeof Uint8Array !== 'undefined'
- ? new Uint8Array(new ArrayBuffer(data.length))
- : new Array(data.length);
- // Write the content to the buffer
- for (var i = 0; i < data.length; i++) {
- buffer[i] = data.charCodeAt(i);
- }
- // Write the string to the buffer
- return buffer;
-};
-
-/**
- * Convert Array ot Uint8Array to Binary String
- *
- * @ignore
- */
-var convertArraytoUtf8BinaryString = function(byteArray, startIndex, endIndex) {
- var result = '';
- for (var i = startIndex; i < endIndex; i++) {
- result = result + String.fromCharCode(byteArray[i]);
- }
- return result;
-};
-
-Binary.BUFFER_SIZE = 256;
-
-/**
- * Default BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
-Binary.SUBTYPE_DEFAULT = 0;
-/**
- * Function BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
-Binary.SUBTYPE_FUNCTION = 1;
-/**
- * Byte Array BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
-Binary.SUBTYPE_BYTE_ARRAY = 2;
-/**
- * OLD UUID BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
-Binary.SUBTYPE_UUID_OLD = 3;
-/**
- * UUID BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
-Binary.SUBTYPE_UUID = 4;
-/**
- * MD5 BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
-Binary.SUBTYPE_MD5 = 5;
-/**
- * User BSON type
- *
- * @classconstant SUBTYPE_DEFAULT
- **/
-Binary.SUBTYPE_USER_DEFINED = 128;
-
-/**
- * Expose.
- */
-module.exports = Binary;
-module.exports.Binary = Binary;
diff --git a/node_modules/bson/lib/bson/bson.js b/node_modules/bson/lib/bson/bson.js
deleted file mode 100644
index 912c5b9..0000000
--- a/node_modules/bson/lib/bson/bson.js
+++ /dev/null
@@ -1,386 +0,0 @@
-'use strict';
-
-var Map = require('./map'),
- Long = require('./long'),
- Double = require('./double'),
- Timestamp = require('./timestamp'),
- ObjectID = require('./objectid'),
- BSONRegExp = require('./regexp'),
- Symbol = require('./symbol'),
- Int32 = require('./int_32'),
- Code = require('./code'),
- Decimal128 = require('./decimal128'),
- MinKey = require('./min_key'),
- MaxKey = require('./max_key'),
- DBRef = require('./db_ref'),
- Binary = require('./binary');
-
-// Parts of the parser
-var deserialize = require('./parser/deserializer'),
- serializer = require('./parser/serializer'),
- calculateObjectSize = require('./parser/calculate_size'),
- utils = require('./parser/utils');
-
-/**
- * @ignore
- * @api private
- */
-// Default Max Size
-var MAXSIZE = 1024 * 1024 * 17;
-
-// Current Internal Temporary Serialization Buffer
-var buffer = utils.allocBuffer(MAXSIZE);
-
-var BSON = function() {};
-
-/**
- * Serialize a Javascript object.
- *
- * @param {Object} object the Javascript object to serialize.
- * @param {Boolean} [options.checkKeys] the serializer will check if keys are valid.
- * @param {Boolean} [options.serializeFunctions=false] serialize the javascript functions **(default:false)**.
- * @param {Boolean} [options.ignoreUndefined=true] ignore undefined fields **(default:true)**.
- * @param {Number} [options.minInternalBufferSize=1024*1024*17] minimum size of the internal temporary serialization buffer **(default:1024*1024*17)**.
- * @return {Buffer} returns the Buffer object containing the serialized object.
- * @api public
- */
-BSON.prototype.serialize = function serialize(object, options) {
- options = options || {};
- // Unpack the options
- var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
- var serializeFunctions =
- typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
- var ignoreUndefined =
- typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
- var minInternalBufferSize =
- typeof options.minInternalBufferSize === 'number' ? options.minInternalBufferSize : MAXSIZE;
-
- // Resize the internal serialization buffer if needed
- if (buffer.length < minInternalBufferSize) {
- buffer = utils.allocBuffer(minInternalBufferSize);
- }
-
- // Attempt to serialize
- var serializationIndex = serializer(
- buffer,
- object,
- checkKeys,
- 0,
- 0,
- serializeFunctions,
- ignoreUndefined,
- []
- );
- // Create the final buffer
- var finishedBuffer = utils.allocBuffer(serializationIndex);
- // Copy into the finished buffer
- buffer.copy(finishedBuffer, 0, 0, finishedBuffer.length);
- // Return the buffer
- return finishedBuffer;
-};
-
-/**
- * Serialize a Javascript object using a predefined Buffer and index into the buffer, useful when pre-allocating the space for serialization.
- *
- * @param {Object} object the Javascript object to serialize.
- * @param {Buffer} buffer the Buffer you pre-allocated to store the serialized BSON object.
- * @param {Boolean} [options.checkKeys] the serializer will check if keys are valid.
- * @param {Boolean} [options.serializeFunctions=false] serialize the javascript functions **(default:false)**.
- * @param {Boolean} [options.ignoreUndefined=true] ignore undefined fields **(default:true)**.
- * @param {Number} [options.index] the index in the buffer where we wish to start serializing into.
- * @return {Number} returns the index pointing to the last written byte in the buffer.
- * @api public
- */
-BSON.prototype.serializeWithBufferAndIndex = function(object, finalBuffer, options) {
- options = options || {};
- // Unpack the options
- var checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
- var serializeFunctions =
- typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
- var ignoreUndefined =
- typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
- var startIndex = typeof options.index === 'number' ? options.index : 0;
-
- // Attempt to serialize
- var serializationIndex = serializer(
- finalBuffer,
- object,
- checkKeys,
- startIndex || 0,
- 0,
- serializeFunctions,
- ignoreUndefined
- );
-
- // Return the index
- return serializationIndex - 1;
-};
-
-/**
- * Deserialize data as BSON.
- *
- * @param {Buffer} buffer the buffer containing the serialized set of BSON documents.
- * @param {Object} [options.evalFunctions=false] evaluate functions in the BSON document scoped to the object deserialized.
- * @param {Object} [options.cacheFunctions=false] cache evaluated functions for reuse.
- * @param {Object} [options.cacheFunctionsCrc32=false] use a crc32 code for caching, otherwise use the string of the function.
- * @param {Object} [options.promoteLongs=true] when deserializing a Long will fit it into a Number if it's smaller than 53 bits
- * @param {Object} [options.promoteBuffers=false] when deserializing a Binary will return it as a node.js Buffer instance.
- * @param {Object} [options.promoteValues=false] when deserializing will promote BSON values to their Node.js closest equivalent types.
- * @param {Object} [options.fieldsAsRaw=null] allow to specify if there what fields we wish to return as unserialized raw buffer.
- * @param {Object} [options.bsonRegExp=false] return BSON regular expressions as BSONRegExp instances.
- * @return {Object} returns the deserialized Javascript Object.
- * @api public
- */
-BSON.prototype.deserialize = function(buffer, options) {
- return deserialize(buffer, options);
-};
-
-/**
- * Calculate the bson size for a passed in Javascript object.
- *
- * @param {Object} object the Javascript object to calculate the BSON byte size for.
- * @param {Boolean} [options.serializeFunctions=false] serialize the javascript functions **(default:false)**.
- * @param {Boolean} [options.ignoreUndefined=true] ignore undefined fields **(default:true)**.
- * @return {Number} returns the number of bytes the BSON object will take up.
- * @api public
- */
-BSON.prototype.calculateObjectSize = function(object, options) {
- options = options || {};
-
- var serializeFunctions =
- typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
- var ignoreUndefined =
- typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
-
- return calculateObjectSize(object, serializeFunctions, ignoreUndefined);
-};
-
-/**
- * Deserialize stream data as BSON documents.
- *
- * @param {Buffer} data the buffer containing the serialized set of BSON documents.
- * @param {Number} startIndex the start index in the data Buffer where the deserialization is to start.
- * @param {Number} numberOfDocuments number of documents to deserialize.
- * @param {Array} documents an array where to store the deserialized documents.
- * @param {Number} docStartIndex the index in the documents array from where to start inserting documents.
- * @param {Object} [options] additional options used for the deserialization.
- * @param {Object} [options.evalFunctions=false] evaluate functions in the BSON document scoped to the object deserialized.
- * @param {Object} [options.cacheFunctions=false] cache evaluated functions for reuse.
- * @param {Object} [options.cacheFunctionsCrc32=false] use a crc32 code for caching, otherwise use the string of the function.
- * @param {Object} [options.promoteLongs=true] when deserializing a Long will fit it into a Number if it's smaller than 53 bits
- * @param {Object} [options.promoteBuffers=false] when deserializing a Binary will return it as a node.js Buffer instance.
- * @param {Object} [options.promoteValues=false] when deserializing will promote BSON values to their Node.js closest equivalent types.
- * @param {Object} [options.fieldsAsRaw=null] allow to specify if there what fields we wish to return as unserialized raw buffer.
- * @param {Object} [options.bsonRegExp=false] return BSON regular expressions as BSONRegExp instances.
- * @return {Number} returns the next index in the buffer after deserialization **x** numbers of documents.
- * @api public
- */
-BSON.prototype.deserializeStream = function(
- data,
- startIndex,
- numberOfDocuments,
- documents,
- docStartIndex,
- options
-) {
- options = options != null ? options : {};
- var index = startIndex;
- // Loop over all documents
- for (var i = 0; i < numberOfDocuments; i++) {
- // Find size of the document
- var size =
- data[index] | (data[index + 1] << 8) | (data[index + 2] << 16) | (data[index + 3] << 24);
- // Update options with index
- options['index'] = index;
- // Parse the document at this point
- documents[docStartIndex + i] = this.deserialize(data, options);
- // Adjust index by the document size
- index = index + size;
- }
-
- // Return object containing end index of parsing and list of documents
- return index;
-};
-
-/**
- * @ignore
- * @api private
- */
-// BSON MAX VALUES
-BSON.BSON_INT32_MAX = 0x7fffffff;
-BSON.BSON_INT32_MIN = -0x80000000;
-
-BSON.BSON_INT64_MAX = Math.pow(2, 63) - 1;
-BSON.BSON_INT64_MIN = -Math.pow(2, 63);
-
-// JS MAX PRECISE VALUES
-BSON.JS_INT_MAX = 0x20000000000000; // Any integer up to 2^53 can be precisely represented by a double.
-BSON.JS_INT_MIN = -0x20000000000000; // Any integer down to -2^53 can be precisely represented by a double.
-
-// Internal long versions
-// var JS_INT_MAX_LONG = Long.fromNumber(0x20000000000000); // Any integer up to 2^53 can be precisely represented by a double.
-// var JS_INT_MIN_LONG = Long.fromNumber(-0x20000000000000); // Any integer down to -2^53 can be precisely represented by a double.
-
-/**
- * Number BSON Type
- *
- * @classconstant BSON_DATA_NUMBER
- **/
-BSON.BSON_DATA_NUMBER = 1;
-/**
- * String BSON Type
- *
- * @classconstant BSON_DATA_STRING
- **/
-BSON.BSON_DATA_STRING = 2;
-/**
- * Object BSON Type
- *
- * @classconstant BSON_DATA_OBJECT
- **/
-BSON.BSON_DATA_OBJECT = 3;
-/**
- * Array BSON Type
- *
- * @classconstant BSON_DATA_ARRAY
- **/
-BSON.BSON_DATA_ARRAY = 4;
-/**
- * Binary BSON Type
- *
- * @classconstant BSON_DATA_BINARY
- **/
-BSON.BSON_DATA_BINARY = 5;
-/**
- * ObjectID BSON Type
- *
- * @classconstant BSON_DATA_OID
- **/
-BSON.BSON_DATA_OID = 7;
-/**
- * Boolean BSON Type
- *
- * @classconstant BSON_DATA_BOOLEAN
- **/
-BSON.BSON_DATA_BOOLEAN = 8;
-/**
- * Date BSON Type
- *
- * @classconstant BSON_DATA_DATE
- **/
-BSON.BSON_DATA_DATE = 9;
-/**
- * null BSON Type
- *
- * @classconstant BSON_DATA_NULL
- **/
-BSON.BSON_DATA_NULL = 10;
-/**
- * RegExp BSON Type
- *
- * @classconstant BSON_DATA_REGEXP
- **/
-BSON.BSON_DATA_REGEXP = 11;
-/**
- * Code BSON Type
- *
- * @classconstant BSON_DATA_CODE
- **/
-BSON.BSON_DATA_CODE = 13;
-/**
- * Symbol BSON Type
- *
- * @classconstant BSON_DATA_SYMBOL
- **/
-BSON.BSON_DATA_SYMBOL = 14;
-/**
- * Code with Scope BSON Type
- *
- * @classconstant BSON_DATA_CODE_W_SCOPE
- **/
-BSON.BSON_DATA_CODE_W_SCOPE = 15;
-/**
- * 32 bit Integer BSON Type
- *
- * @classconstant BSON_DATA_INT
- **/
-BSON.BSON_DATA_INT = 16;
-/**
- * Timestamp BSON Type
- *
- * @classconstant BSON_DATA_TIMESTAMP
- **/
-BSON.BSON_DATA_TIMESTAMP = 17;
-/**
- * Long BSON Type
- *
- * @classconstant BSON_DATA_LONG
- **/
-BSON.BSON_DATA_LONG = 18;
-/**
- * MinKey BSON Type
- *
- * @classconstant BSON_DATA_MIN_KEY
- **/
-BSON.BSON_DATA_MIN_KEY = 0xff;
-/**
- * MaxKey BSON Type
- *
- * @classconstant BSON_DATA_MAX_KEY
- **/
-BSON.BSON_DATA_MAX_KEY = 0x7f;
-
-/**
- * Binary Default Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_DEFAULT
- **/
-BSON.BSON_BINARY_SUBTYPE_DEFAULT = 0;
-/**
- * Binary Function Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_FUNCTION
- **/
-BSON.BSON_BINARY_SUBTYPE_FUNCTION = 1;
-/**
- * Binary Byte Array Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_BYTE_ARRAY
- **/
-BSON.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
-/**
- * Binary UUID Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_UUID
- **/
-BSON.BSON_BINARY_SUBTYPE_UUID = 3;
-/**
- * Binary MD5 Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_MD5
- **/
-BSON.BSON_BINARY_SUBTYPE_MD5 = 4;
-/**
- * Binary User Defined Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_USER_DEFINED
- **/
-BSON.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
-
-// Return BSON
-module.exports = BSON;
-module.exports.Code = Code;
-module.exports.Map = Map;
-module.exports.Symbol = Symbol;
-module.exports.BSON = BSON;
-module.exports.DBRef = DBRef;
-module.exports.Binary = Binary;
-module.exports.ObjectID = ObjectID;
-module.exports.Long = Long;
-module.exports.Timestamp = Timestamp;
-module.exports.Double = Double;
-module.exports.Int32 = Int32;
-module.exports.MinKey = MinKey;
-module.exports.MaxKey = MaxKey;
-module.exports.BSONRegExp = BSONRegExp;
-module.exports.Decimal128 = Decimal128;
diff --git a/node_modules/bson/lib/bson/code.js b/node_modules/bson/lib/bson/code.js
deleted file mode 100644
index c2984cd..0000000
--- a/node_modules/bson/lib/bson/code.js
+++ /dev/null
@@ -1,24 +0,0 @@
-/**
- * A class representation of the BSON Code type.
- *
- * @class
- * @param {(string|function)} code a string or function.
- * @param {Object} [scope] an optional scope for the function.
- * @return {Code}
- */
-var Code = function Code(code, scope) {
- if (!(this instanceof Code)) return new Code(code, scope);
- this._bsontype = 'Code';
- this.code = code;
- this.scope = scope;
-};
-
-/**
- * @ignore
- */
-Code.prototype.toJSON = function() {
- return { scope: this.scope, code: this.code };
-};
-
-module.exports = Code;
-module.exports.Code = Code;
diff --git a/node_modules/bson/lib/bson/db_ref.js b/node_modules/bson/lib/bson/db_ref.js
deleted file mode 100644
index f95795b..0000000
--- a/node_modules/bson/lib/bson/db_ref.js
+++ /dev/null
@@ -1,32 +0,0 @@
-/**
- * A class representation of the BSON DBRef type.
- *
- * @class
- * @param {string} namespace the collection name.
- * @param {ObjectID} oid the reference ObjectID.
- * @param {string} [db] optional db name, if omitted the reference is local to the current db.
- * @return {DBRef}
- */
-function DBRef(namespace, oid, db) {
- if (!(this instanceof DBRef)) return new DBRef(namespace, oid, db);
-
- this._bsontype = 'DBRef';
- this.namespace = namespace;
- this.oid = oid;
- this.db = db;
-}
-
-/**
- * @ignore
- * @api private
- */
-DBRef.prototype.toJSON = function() {
- return {
- $ref: this.namespace,
- $id: this.oid,
- $db: this.db == null ? '' : this.db
- };
-};
-
-module.exports = DBRef;
-module.exports.DBRef = DBRef;
diff --git a/node_modules/bson/lib/bson/decimal128.js b/node_modules/bson/lib/bson/decimal128.js
deleted file mode 100644
index 924513f..0000000
--- a/node_modules/bson/lib/bson/decimal128.js
+++ /dev/null
@@ -1,820 +0,0 @@
-'use strict';
-
-var Long = require('./long');
-
-var PARSE_STRING_REGEXP = /^(\+|-)?(\d+|(\d*\.\d*))?(E|e)?([-+])?(\d+)?$/;
-var PARSE_INF_REGEXP = /^(\+|-)?(Infinity|inf)$/i;
-var PARSE_NAN_REGEXP = /^(\+|-)?NaN$/i;
-
-var EXPONENT_MAX = 6111;
-var EXPONENT_MIN = -6176;
-var EXPONENT_BIAS = 6176;
-var MAX_DIGITS = 34;
-
-// Nan value bits as 32 bit values (due to lack of longs)
-var NAN_BUFFER = [
- 0x7c,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00
-].reverse();
-// Infinity value bits 32 bit values (due to lack of longs)
-var INF_NEGATIVE_BUFFER = [
- 0xf8,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00
-].reverse();
-var INF_POSITIVE_BUFFER = [
- 0x78,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00,
- 0x00
-].reverse();
-
-var EXPONENT_REGEX = /^([-+])?(\d+)?$/;
-
-var utils = require('./parser/utils');
-
-// Detect if the value is a digit
-var isDigit = function(value) {
- return !isNaN(parseInt(value, 10));
-};
-
-// Divide two uint128 values
-var divideu128 = function(value) {
- var DIVISOR = Long.fromNumber(1000 * 1000 * 1000);
- var _rem = Long.fromNumber(0);
- var i = 0;
-
- if (!value.parts[0] && !value.parts[1] && !value.parts[2] && !value.parts[3]) {
- return { quotient: value, rem: _rem };
- }
-
- for (i = 0; i <= 3; i++) {
- // Adjust remainder to match value of next dividend
- _rem = _rem.shiftLeft(32);
- // Add the divided to _rem
- _rem = _rem.add(new Long(value.parts[i], 0));
- value.parts[i] = _rem.div(DIVISOR).low_;
- _rem = _rem.modulo(DIVISOR);
- }
-
- return { quotient: value, rem: _rem };
-};
-
-// Multiply two Long values and return the 128 bit value
-var multiply64x2 = function(left, right) {
- if (!left && !right) {
- return { high: Long.fromNumber(0), low: Long.fromNumber(0) };
- }
-
- var leftHigh = left.shiftRightUnsigned(32);
- var leftLow = new Long(left.getLowBits(), 0);
- var rightHigh = right.shiftRightUnsigned(32);
- var rightLow = new Long(right.getLowBits(), 0);
-
- var productHigh = leftHigh.multiply(rightHigh);
- var productMid = leftHigh.multiply(rightLow);
- var productMid2 = leftLow.multiply(rightHigh);
- var productLow = leftLow.multiply(rightLow);
-
- productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
- productMid = new Long(productMid.getLowBits(), 0)
- .add(productMid2)
- .add(productLow.shiftRightUnsigned(32));
-
- productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
- productLow = productMid.shiftLeft(32).add(new Long(productLow.getLowBits(), 0));
-
- // Return the 128 bit result
- return { high: productHigh, low: productLow };
-};
-
-var lessThan = function(left, right) {
- // Make values unsigned
- var uhleft = left.high_ >>> 0;
- var uhright = right.high_ >>> 0;
-
- // Compare high bits first
- if (uhleft < uhright) {
- return true;
- } else if (uhleft === uhright) {
- var ulleft = left.low_ >>> 0;
- var ulright = right.low_ >>> 0;
- if (ulleft < ulright) return true;
- }
-
- return false;
-};
-
-// var longtoHex = function(value) {
-// var buffer = utils.allocBuffer(8);
-// var index = 0;
-// // Encode the low 64 bits of the decimal
-// // Encode low bits
-// buffer[index++] = value.low_ & 0xff;
-// buffer[index++] = (value.low_ >> 8) & 0xff;
-// buffer[index++] = (value.low_ >> 16) & 0xff;
-// buffer[index++] = (value.low_ >> 24) & 0xff;
-// // Encode high bits
-// buffer[index++] = value.high_ & 0xff;
-// buffer[index++] = (value.high_ >> 8) & 0xff;
-// buffer[index++] = (value.high_ >> 16) & 0xff;
-// buffer[index++] = (value.high_ >> 24) & 0xff;
-// return buffer.reverse().toString('hex');
-// };
-
-// var int32toHex = function(value) {
-// var buffer = utils.allocBuffer(4);
-// var index = 0;
-// // Encode the low 64 bits of the decimal
-// // Encode low bits
-// buffer[index++] = value & 0xff;
-// buffer[index++] = (value >> 8) & 0xff;
-// buffer[index++] = (value >> 16) & 0xff;
-// buffer[index++] = (value >> 24) & 0xff;
-// return buffer.reverse().toString('hex');
-// };
-
-/**
- * A class representation of the BSON Decimal128 type.
- *
- * @class
- * @param {Buffer} bytes a buffer containing the raw Decimal128 bytes.
- * @return {Double}
- */
-var Decimal128 = function(bytes) {
- this._bsontype = 'Decimal128';
- this.bytes = bytes;
-};
-
-/**
- * Create a Decimal128 instance from a string representation
- *
- * @method
- * @param {string} string a numeric string representation.
- * @return {Decimal128} returns a Decimal128 instance.
- */
-Decimal128.fromString = function(string) {
- // Parse state tracking
- var isNegative = false;
- var sawRadix = false;
- var foundNonZero = false;
-
- // Total number of significant digits (no leading or trailing zero)
- var significantDigits = 0;
- // Total number of significand digits read
- var nDigitsRead = 0;
- // Total number of digits (no leading zeros)
- var nDigits = 0;
- // The number of the digits after radix
- var radixPosition = 0;
- // The index of the first non-zero in *str*
- var firstNonZero = 0;
-
- // Digits Array
- var digits = [0];
- // The number of digits in digits
- var nDigitsStored = 0;
- // Insertion pointer for digits
- var digitsInsert = 0;
- // The index of the first non-zero digit
- var firstDigit = 0;
- // The index of the last digit
- var lastDigit = 0;
-
- // Exponent
- var exponent = 0;
- // loop index over array
- var i = 0;
- // The high 17 digits of the significand
- var significandHigh = [0, 0];
- // The low 17 digits of the significand
- var significandLow = [0, 0];
- // The biased exponent
- var biasedExponent = 0;
-
- // Read index
- var index = 0;
-
- // Trim the string
- string = string.trim();
-
- // Naively prevent against REDOS attacks.
- // TODO: implementing a custom parsing for this, or refactoring the regex would yield
- // further gains.
- if (string.length >= 7000) {
- throw new Error('' + string + ' not a valid Decimal128 string');
- }
-
- // Results
- var stringMatch = string.match(PARSE_STRING_REGEXP);
- var infMatch = string.match(PARSE_INF_REGEXP);
- var nanMatch = string.match(PARSE_NAN_REGEXP);
-
- // Validate the string
- if ((!stringMatch && !infMatch && !nanMatch) || string.length === 0) {
- throw new Error('' + string + ' not a valid Decimal128 string');
- }
-
- // Check if we have an illegal exponent format
- if (stringMatch && stringMatch[4] && stringMatch[2] === undefined) {
- throw new Error('' + string + ' not a valid Decimal128 string');
- }
-
- // Get the negative or positive sign
- if (string[index] === '+' || string[index] === '-') {
- isNegative = string[index++] === '-';
- }
-
- // Check if user passed Infinity or NaN
- if (!isDigit(string[index]) && string[index] !== '.') {
- if (string[index] === 'i' || string[index] === 'I') {
- return new Decimal128(utils.toBuffer(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
- } else if (string[index] === 'N') {
- return new Decimal128(utils.toBuffer(NAN_BUFFER));
- }
- }
-
- // Read all the digits
- while (isDigit(string[index]) || string[index] === '.') {
- if (string[index] === '.') {
- if (sawRadix) {
- return new Decimal128(utils.toBuffer(NAN_BUFFER));
- }
-
- sawRadix = true;
- index = index + 1;
- continue;
- }
-
- if (nDigitsStored < 34) {
- if (string[index] !== '0' || foundNonZero) {
- if (!foundNonZero) {
- firstNonZero = nDigitsRead;
- }
-
- foundNonZero = true;
-
- // Only store 34 digits
- digits[digitsInsert++] = parseInt(string[index], 10);
- nDigitsStored = nDigitsStored + 1;
- }
- }
-
- if (foundNonZero) {
- nDigits = nDigits + 1;
- }
-
- if (sawRadix) {
- radixPosition = radixPosition + 1;
- }
-
- nDigitsRead = nDigitsRead + 1;
- index = index + 1;
- }
-
- if (sawRadix && !nDigitsRead) {
- throw new Error('' + string + ' not a valid Decimal128 string');
- }
-
- // Read exponent if exists
- if (string[index] === 'e' || string[index] === 'E') {
- // Read exponent digits
- var match = string.substr(++index).match(EXPONENT_REGEX);
-
- // No digits read
- if (!match || !match[2]) {
- return new Decimal128(utils.toBuffer(NAN_BUFFER));
- }
-
- // Get exponent
- exponent = parseInt(match[0], 10);
-
- // Adjust the index
- index = index + match[0].length;
- }
-
- // Return not a number
- if (string[index]) {
- return new Decimal128(utils.toBuffer(NAN_BUFFER));
- }
-
- // Done reading input
- // Find first non-zero digit in digits
- firstDigit = 0;
-
- if (!nDigitsStored) {
- firstDigit = 0;
- lastDigit = 0;
- digits[0] = 0;
- nDigits = 1;
- nDigitsStored = 1;
- significantDigits = 0;
- } else {
- lastDigit = nDigitsStored - 1;
- significantDigits = nDigits;
-
- if (exponent !== 0 && significantDigits !== 1) {
- while (string[firstNonZero + significantDigits - 1] === '0') {
- significantDigits = significantDigits - 1;
- }
- }
- }
-
- // Normalization of exponent
- // Correct exponent based on radix position, and shift significand as needed
- // to represent user input
-
- // Overflow prevention
- if (exponent <= radixPosition && radixPosition - exponent > 1 << 14) {
- exponent = EXPONENT_MIN;
- } else {
- exponent = exponent - radixPosition;
- }
-
- // Attempt to normalize the exponent
- while (exponent > EXPONENT_MAX) {
- // Shift exponent to significand and decrease
- lastDigit = lastDigit + 1;
-
- if (lastDigit - firstDigit > MAX_DIGITS) {
- // Check if we have a zero then just hard clamp, otherwise fail
- var digitsString = digits.join('');
- if (digitsString.match(/^0+$/)) {
- exponent = EXPONENT_MAX;
- break;
- } else {
- return new Decimal128(utils.toBuffer(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
- }
- }
-
- exponent = exponent - 1;
- }
-
- while (exponent < EXPONENT_MIN || nDigitsStored < nDigits) {
- // Shift last digit
- if (lastDigit === 0) {
- exponent = EXPONENT_MIN;
- significantDigits = 0;
- break;
- }
-
- if (nDigitsStored < nDigits) {
- // adjust to match digits not stored
- nDigits = nDigits - 1;
- } else {
- // adjust to round
- lastDigit = lastDigit - 1;
- }
-
- if (exponent < EXPONENT_MAX) {
- exponent = exponent + 1;
- } else {
- // Check if we have a zero then just hard clamp, otherwise fail
- digitsString = digits.join('');
- if (digitsString.match(/^0+$/)) {
- exponent = EXPONENT_MAX;
- break;
- } else {
- return new Decimal128(utils.toBuffer(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
- }
- }
- }
-
- // Round
- // We've normalized the exponent, but might still need to round.
- if (lastDigit - firstDigit + 1 < significantDigits && string[significantDigits] !== '0') {
- var endOfString = nDigitsRead;
-
- // If we have seen a radix point, 'string' is 1 longer than we have
- // documented with ndigits_read, so inc the position of the first nonzero
- // digit and the position that digits are read to.
- if (sawRadix && exponent === EXPONENT_MIN) {
- firstNonZero = firstNonZero + 1;
- endOfString = endOfString + 1;
- }
-
- var roundDigit = parseInt(string[firstNonZero + lastDigit + 1], 10);
- var roundBit = 0;
-
- if (roundDigit >= 5) {
- roundBit = 1;
-
- if (roundDigit === 5) {
- roundBit = digits[lastDigit] % 2 === 1;
-
- for (i = firstNonZero + lastDigit + 2; i < endOfString; i++) {
- if (parseInt(string[i], 10)) {
- roundBit = 1;
- break;
- }
- }
- }
- }
-
- if (roundBit) {
- var dIdx = lastDigit;
-
- for (; dIdx >= 0; dIdx--) {
- if (++digits[dIdx] > 9) {
- digits[dIdx] = 0;
-
- // overflowed most significant digit
- if (dIdx === 0) {
- if (exponent < EXPONENT_MAX) {
- exponent = exponent + 1;
- digits[dIdx] = 1;
- } else {
- return new Decimal128(
- utils.toBuffer(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER)
- );
- }
- }
- } else {
- break;
- }
- }
- }
- }
-
- // Encode significand
- // The high 17 digits of the significand
- significandHigh = Long.fromNumber(0);
- // The low 17 digits of the significand
- significandLow = Long.fromNumber(0);
-
- // read a zero
- if (significantDigits === 0) {
- significandHigh = Long.fromNumber(0);
- significandLow = Long.fromNumber(0);
- } else if (lastDigit - firstDigit < 17) {
- dIdx = firstDigit;
- significandLow = Long.fromNumber(digits[dIdx++]);
- significandHigh = new Long(0, 0);
-
- for (; dIdx <= lastDigit; dIdx++) {
- significandLow = significandLow.multiply(Long.fromNumber(10));
- significandLow = significandLow.add(Long.fromNumber(digits[dIdx]));
- }
- } else {
- dIdx = firstDigit;
- significandHigh = Long.fromNumber(digits[dIdx++]);
-
- for (; dIdx <= lastDigit - 17; dIdx++) {
- significandHigh = significandHigh.multiply(Long.fromNumber(10));
- significandHigh = significandHigh.add(Long.fromNumber(digits[dIdx]));
- }
-
- significandLow = Long.fromNumber(digits[dIdx++]);
-
- for (; dIdx <= lastDigit; dIdx++) {
- significandLow = significandLow.multiply(Long.fromNumber(10));
- significandLow = significandLow.add(Long.fromNumber(digits[dIdx]));
- }
- }
-
- var significand = multiply64x2(significandHigh, Long.fromString('100000000000000000'));
-
- significand.low = significand.low.add(significandLow);
-
- if (lessThan(significand.low, significandLow)) {
- significand.high = significand.high.add(Long.fromNumber(1));
- }
-
- // Biased exponent
- biasedExponent = exponent + EXPONENT_BIAS;
- var dec = { low: Long.fromNumber(0), high: Long.fromNumber(0) };
-
- // Encode combination, exponent, and significand.
- if (
- significand.high
- .shiftRightUnsigned(49)
- .and(Long.fromNumber(1))
- .equals(Long.fromNumber)
- ) {
- // Encode '11' into bits 1 to 3
- dec.high = dec.high.or(Long.fromNumber(0x3).shiftLeft(61));
- dec.high = dec.high.or(
- Long.fromNumber(biasedExponent).and(Long.fromNumber(0x3fff).shiftLeft(47))
- );
- dec.high = dec.high.or(significand.high.and(Long.fromNumber(0x7fffffffffff)));
- } else {
- dec.high = dec.high.or(Long.fromNumber(biasedExponent & 0x3fff).shiftLeft(49));
- dec.high = dec.high.or(significand.high.and(Long.fromNumber(0x1ffffffffffff)));
- }
-
- dec.low = significand.low;
-
- // Encode sign
- if (isNegative) {
- dec.high = dec.high.or(Long.fromString('9223372036854775808'));
- }
-
- // Encode into a buffer
- var buffer = utils.allocBuffer(16);
- index = 0;
-
- // Encode the low 64 bits of the decimal
- // Encode low bits
- buffer[index++] = dec.low.low_ & 0xff;
- buffer[index++] = (dec.low.low_ >> 8) & 0xff;
- buffer[index++] = (dec.low.low_ >> 16) & 0xff;
- buffer[index++] = (dec.low.low_ >> 24) & 0xff;
- // Encode high bits
- buffer[index++] = dec.low.high_ & 0xff;
- buffer[index++] = (dec.low.high_ >> 8) & 0xff;
- buffer[index++] = (dec.low.high_ >> 16) & 0xff;
- buffer[index++] = (dec.low.high_ >> 24) & 0xff;
-
- // Encode the high 64 bits of the decimal
- // Encode low bits
- buffer[index++] = dec.high.low_ & 0xff;
- buffer[index++] = (dec.high.low_ >> 8) & 0xff;
- buffer[index++] = (dec.high.low_ >> 16) & 0xff;
- buffer[index++] = (dec.high.low_ >> 24) & 0xff;
- // Encode high bits
- buffer[index++] = dec.high.high_ & 0xff;
- buffer[index++] = (dec.high.high_ >> 8) & 0xff;
- buffer[index++] = (dec.high.high_ >> 16) & 0xff;
- buffer[index++] = (dec.high.high_ >> 24) & 0xff;
-
- // Return the new Decimal128
- return new Decimal128(buffer);
-};
-
-// Extract least significant 5 bits
-var COMBINATION_MASK = 0x1f;
-// Extract least significant 14 bits
-var EXPONENT_MASK = 0x3fff;
-// Value of combination field for Inf
-var COMBINATION_INFINITY = 30;
-// Value of combination field for NaN
-var COMBINATION_NAN = 31;
-// Value of combination field for NaN
-// var COMBINATION_SNAN = 32;
-// decimal128 exponent bias
-EXPONENT_BIAS = 6176;
-
-/**
- * Create a string representation of the raw Decimal128 value
- *
- * @method
- * @return {string} returns a Decimal128 string representation.
- */
-Decimal128.prototype.toString = function() {
- // Note: bits in this routine are referred to starting at 0,
- // from the sign bit, towards the coefficient.
-
- // bits 0 - 31
- var high;
- // bits 32 - 63
- var midh;
- // bits 64 - 95
- var midl;
- // bits 96 - 127
- var low;
- // bits 1 - 5
- var combination;
- // decoded biased exponent (14 bits)
- var biased_exponent;
- // the number of significand digits
- var significand_digits = 0;
- // the base-10 digits in the significand
- var significand = new Array(36);
- for (var i = 0; i < significand.length; i++) significand[i] = 0;
- // read pointer into significand
- var index = 0;
-
- // unbiased exponent
- var exponent;
- // the exponent if scientific notation is used
- var scientific_exponent;
-
- // true if the number is zero
- var is_zero = false;
-
- // the most signifcant significand bits (50-46)
- var significand_msb;
- // temporary storage for significand decoding
- var significand128 = { parts: new Array(4) };
- // indexing variables
- i;
- var j, k;
-
- // Output string
- var string = [];
-
- // Unpack index
- index = 0;
-
- // Buffer reference
- var buffer = this.bytes;
-
- // Unpack the low 64bits into a long
- low =
- buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
- midl =
- buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
-
- // Unpack the high 64bits into a long
- midh =
- buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
- high =
- buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
-
- // Unpack index
- index = 0;
-
- // Create the state of the decimal
- var dec = {
- low: new Long(low, midl),
- high: new Long(midh, high)
- };
-
- if (dec.high.lessThan(Long.ZERO)) {
- string.push('-');
- }
-
- // Decode combination field and exponent
- combination = (high >> 26) & COMBINATION_MASK;
-
- if (combination >> 3 === 3) {
- // Check for 'special' values
- if (combination === COMBINATION_INFINITY) {
- return string.join('') + 'Infinity';
- } else if (combination === COMBINATION_NAN) {
- return 'NaN';
- } else {
- biased_exponent = (high >> 15) & EXPONENT_MASK;
- significand_msb = 0x08 + ((high >> 14) & 0x01);
- }
- } else {
- significand_msb = (high >> 14) & 0x07;
- biased_exponent = (high >> 17) & EXPONENT_MASK;
- }
-
- exponent = biased_exponent - EXPONENT_BIAS;
-
- // Create string of significand digits
-
- // Convert the 114-bit binary number represented by
- // (significand_high, significand_low) to at most 34 decimal
- // digits through modulo and division.
- significand128.parts[0] = (high & 0x3fff) + ((significand_msb & 0xf) << 14);
- significand128.parts[1] = midh;
- significand128.parts[2] = midl;
- significand128.parts[3] = low;
-
- if (
- significand128.parts[0] === 0 &&
- significand128.parts[1] === 0 &&
- significand128.parts[2] === 0 &&
- significand128.parts[3] === 0
- ) {
- is_zero = true;
- } else {
- for (k = 3; k >= 0; k--) {
- var least_digits = 0;
- // Peform the divide
- var result = divideu128(significand128);
- significand128 = result.quotient;
- least_digits = result.rem.low_;
-
- // We now have the 9 least significant digits (in base 2).
- // Convert and output to string.
- if (!least_digits) continue;
-
- for (j = 8; j >= 0; j--) {
- // significand[k * 9 + j] = Math.round(least_digits % 10);
- significand[k * 9 + j] = least_digits % 10;
- // least_digits = Math.round(least_digits / 10);
- least_digits = Math.floor(least_digits / 10);
- }
- }
- }
-
- // Output format options:
- // Scientific - [-]d.dddE(+/-)dd or [-]dE(+/-)dd
- // Regular - ddd.ddd
-
- if (is_zero) {
- significand_digits = 1;
- significand[index] = 0;
- } else {
- significand_digits = 36;
- i = 0;
-
- while (!significand[index]) {
- i++;
- significand_digits = significand_digits - 1;
- index = index + 1;
- }
- }
-
- scientific_exponent = significand_digits - 1 + exponent;
-
- // The scientific exponent checks are dictated by the string conversion
- // specification and are somewhat arbitrary cutoffs.
- //
- // We must check exponent > 0, because if this is the case, the number
- // has trailing zeros. However, we *cannot* output these trailing zeros,
- // because doing so would change the precision of the value, and would
- // change stored data if the string converted number is round tripped.
-
- if (scientific_exponent >= 34 || scientific_exponent <= -7 || exponent > 0) {
- // Scientific format
- string.push(significand[index++]);
- significand_digits = significand_digits - 1;
-
- if (significand_digits) {
- string.push('.');
- }
-
- for (i = 0; i < significand_digits; i++) {
- string.push(significand[index++]);
- }
-
- // Exponent
- string.push('E');
- if (scientific_exponent > 0) {
- string.push('+' + scientific_exponent);
- } else {
- string.push(scientific_exponent);
- }
- } else {
- // Regular format with no decimal place
- if (exponent >= 0) {
- for (i = 0; i < significand_digits; i++) {
- string.push(significand[index++]);
- }
- } else {
- var radix_position = significand_digits + exponent;
-
- // non-zero digits before radix
- if (radix_position > 0) {
- for (i = 0; i < radix_position; i++) {
- string.push(significand[index++]);
- }
- } else {
- string.push('0');
- }
-
- string.push('.');
- // add leading zeros after radix
- while (radix_position++ < 0) {
- string.push('0');
- }
-
- for (i = 0; i < significand_digits - Math.max(radix_position - 1, 0); i++) {
- string.push(significand[index++]);
- }
- }
- }
-
- return string.join('');
-};
-
-Decimal128.prototype.toJSON = function() {
- return { $numberDecimal: this.toString() };
-};
-
-module.exports = Decimal128;
-module.exports.Decimal128 = Decimal128;
diff --git a/node_modules/bson/lib/bson/double.js b/node_modules/bson/lib/bson/double.js
deleted file mode 100644
index 523c21f..0000000
--- a/node_modules/bson/lib/bson/double.js
+++ /dev/null
@@ -1,33 +0,0 @@
-/**
- * A class representation of the BSON Double type.
- *
- * @class
- * @param {number} value the number we want to represent as a double.
- * @return {Double}
- */
-function Double(value) {
- if (!(this instanceof Double)) return new Double(value);
-
- this._bsontype = 'Double';
- this.value = value;
-}
-
-/**
- * Access the number value.
- *
- * @method
- * @return {number} returns the wrapped double number.
- */
-Double.prototype.valueOf = function() {
- return this.value;
-};
-
-/**
- * @ignore
- */
-Double.prototype.toJSON = function() {
- return this.value;
-};
-
-module.exports = Double;
-module.exports.Double = Double;
diff --git a/node_modules/bson/lib/bson/float_parser.js b/node_modules/bson/lib/bson/float_parser.js
deleted file mode 100644
index 0054a2f..0000000
--- a/node_modules/bson/lib/bson/float_parser.js
+++ /dev/null
@@ -1,124 +0,0 @@
-// Copyright (c) 2008, Fair Oaks Labs, Inc.
-// All rights reserved.
-//
-// Redistribution and use in source and binary forms, with or without
-// modification, are permitted provided that the following conditions are met:
-//
-// * Redistributions of source code must retain the above copyright notice,
-// this list of conditions and the following disclaimer.
-//
-// * Redistributions in binary form must reproduce the above copyright notice,
-// this list of conditions and the following disclaimer in the documentation
-// and/or other materials provided with the distribution.
-//
-// * Neither the name of Fair Oaks Labs, Inc. nor the names of its contributors
-// may be used to endorse or promote products derived from this software
-// without specific prior written permission.
-//
-// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
-// AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
-// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
-// ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
-// LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
-// CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
-// SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
-// INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
-// CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
-// ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
-// POSSIBILITY OF SUCH DAMAGE.
-//
-//
-// Modifications to writeIEEE754 to support negative zeroes made by Brian White
-
-var readIEEE754 = function(buffer, offset, endian, mLen, nBytes) {
- var e,
- m,
- bBE = endian === 'big',
- eLen = nBytes * 8 - mLen - 1,
- eMax = (1 << eLen) - 1,
- eBias = eMax >> 1,
- nBits = -7,
- i = bBE ? 0 : nBytes - 1,
- d = bBE ? 1 : -1,
- s = buffer[offset + i];
-
- i += d;
-
- e = s & ((1 << -nBits) - 1);
- s >>= -nBits;
- nBits += eLen;
- for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8);
-
- m = e & ((1 << -nBits) - 1);
- e >>= -nBits;
- nBits += mLen;
- for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8);
-
- if (e === 0) {
- e = 1 - eBias;
- } else if (e === eMax) {
- return m ? NaN : (s ? -1 : 1) * Infinity;
- } else {
- m = m + Math.pow(2, mLen);
- e = e - eBias;
- }
- return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
-};
-
-var writeIEEE754 = function(buffer, value, offset, endian, mLen, nBytes) {
- var e,
- m,
- c,
- bBE = endian === 'big',
- eLen = nBytes * 8 - mLen - 1,
- eMax = (1 << eLen) - 1,
- eBias = eMax >> 1,
- rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0,
- i = bBE ? nBytes - 1 : 0,
- d = bBE ? -1 : 1,
- s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0;
-
- value = Math.abs(value);
-
- if (isNaN(value) || value === Infinity) {
- m = isNaN(value) ? 1 : 0;
- e = eMax;
- } else {
- e = Math.floor(Math.log(value) / Math.LN2);
- if (value * (c = Math.pow(2, -e)) < 1) {
- e--;
- c *= 2;
- }
- if (e + eBias >= 1) {
- value += rt / c;
- } else {
- value += rt * Math.pow(2, 1 - eBias);
- }
- if (value * c >= 2) {
- e++;
- c /= 2;
- }
-
- if (e + eBias >= eMax) {
- m = 0;
- e = eMax;
- } else if (e + eBias >= 1) {
- m = (value * c - 1) * Math.pow(2, mLen);
- e = e + eBias;
- } else {
- m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
- e = 0;
- }
- }
-
- for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8);
-
- e = (e << mLen) | m;
- eLen += mLen;
- for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8);
-
- buffer[offset + i - d] |= s * 128;
-};
-
-exports.readIEEE754 = readIEEE754;
-exports.writeIEEE754 = writeIEEE754;
diff --git a/node_modules/bson/lib/bson/int_32.js b/node_modules/bson/lib/bson/int_32.js
deleted file mode 100644
index 85dbdec..0000000
--- a/node_modules/bson/lib/bson/int_32.js
+++ /dev/null
@@ -1,33 +0,0 @@
-/**
- * A class representation of a BSON Int32 type.
- *
- * @class
- * @param {number} value the number we want to represent as an int32.
- * @return {Int32}
- */
-var Int32 = function(value) {
- if (!(this instanceof Int32)) return new Int32(value);
-
- this._bsontype = 'Int32';
- this.value = value;
-};
-
-/**
- * Access the number value.
- *
- * @method
- * @return {number} returns the wrapped int32 number.
- */
-Int32.prototype.valueOf = function() {
- return this.value;
-};
-
-/**
- * @ignore
- */
-Int32.prototype.toJSON = function() {
- return this.value;
-};
-
-module.exports = Int32;
-module.exports.Int32 = Int32;
diff --git a/node_modules/bson/lib/bson/long.js b/node_modules/bson/lib/bson/long.js
deleted file mode 100644
index 78215aa..0000000
--- a/node_modules/bson/lib/bson/long.js
+++ /dev/null
@@ -1,851 +0,0 @@
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-//
-// Copyright 2009 Google Inc. All Rights Reserved
-
-/**
- * Defines a Long class for representing a 64-bit two's-complement
- * integer value, which faithfully simulates the behavior of a Java "Long". This
- * implementation is derived from LongLib in GWT.
- *
- * Constructs a 64-bit two's-complement integer, given its low and high 32-bit
- * values as *signed* integers. See the from* functions below for more
- * convenient ways of constructing Longs.
- *
- * The internal representation of a Long is the two given signed, 32-bit values.
- * We use 32-bit pieces because these are the size of integers on which
- * Javascript performs bit-operations. For operations like addition and
- * multiplication, we split each number into 16-bit pieces, which can easily be
- * multiplied within Javascript's floating-point representation without overflow
- * or change in sign.
- *
- * In the algorithms below, we frequently reduce the negative case to the
- * positive case by negating the input(s) and then post-processing the result.
- * Note that we must ALWAYS check specially whether those values are MIN_VALUE
- * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
- * a positive number, it overflows back into a negative). Not handling this
- * case would often result in infinite recursion.
- *
- * @class
- * @param {number} low the low (signed) 32 bits of the Long.
- * @param {number} high the high (signed) 32 bits of the Long.
- * @return {Long}
- */
-function Long(low, high) {
- if (!(this instanceof Long)) return new Long(low, high);
-
- this._bsontype = 'Long';
- /**
- * @type {number}
- * @ignore
- */
- this.low_ = low | 0; // force into 32 signed bits.
-
- /**
- * @type {number}
- * @ignore
- */
- this.high_ = high | 0; // force into 32 signed bits.
-}
-
-/**
- * Return the int value.
- *
- * @method
- * @return {number} the value, assuming it is a 32-bit integer.
- */
-Long.prototype.toInt = function() {
- return this.low_;
-};
-
-/**
- * Return the Number value.
- *
- * @method
- * @return {number} the closest floating-point representation to this value.
- */
-Long.prototype.toNumber = function() {
- return this.high_ * Long.TWO_PWR_32_DBL_ + this.getLowBitsUnsigned();
-};
-
-/**
- * Return the JSON value.
- *
- * @method
- * @return {string} the JSON representation.
- */
-Long.prototype.toJSON = function() {
- return this.toString();
-};
-
-/**
- * Return the String value.
- *
- * @method
- * @param {number} [opt_radix] the radix in which the text should be written.
- * @return {string} the textual representation of this value.
- */
-Long.prototype.toString = function(opt_radix) {
- var radix = opt_radix || 10;
- if (radix < 2 || 36 < radix) {
- throw Error('radix out of range: ' + radix);
- }
-
- if (this.isZero()) {
- return '0';
- }
-
- if (this.isNegative()) {
- if (this.equals(Long.MIN_VALUE)) {
- // We need to change the Long value before it can be negated, so we remove
- // the bottom-most digit in this base and then recurse to do the rest.
- var radixLong = Long.fromNumber(radix);
- var div = this.div(radixLong);
- var rem = div.multiply(radixLong).subtract(this);
- return div.toString(radix) + rem.toInt().toString(radix);
- } else {
- return '-' + this.negate().toString(radix);
- }
- }
-
- // Do several (6) digits each time through the loop, so as to
- // minimize the calls to the very expensive emulated div.
- var radixToPower = Long.fromNumber(Math.pow(radix, 6));
-
- rem = this;
- var result = '';
-
- while (!rem.isZero()) {
- var remDiv = rem.div(radixToPower);
- var intval = rem.subtract(remDiv.multiply(radixToPower)).toInt();
- var digits = intval.toString(radix);
-
- rem = remDiv;
- if (rem.isZero()) {
- return digits + result;
- } else {
- while (digits.length < 6) {
- digits = '0' + digits;
- }
- result = '' + digits + result;
- }
- }
-};
-
-/**
- * Return the high 32-bits value.
- *
- * @method
- * @return {number} the high 32-bits as a signed value.
- */
-Long.prototype.getHighBits = function() {
- return this.high_;
-};
-
-/**
- * Return the low 32-bits value.
- *
- * @method
- * @return {number} the low 32-bits as a signed value.
- */
-Long.prototype.getLowBits = function() {
- return this.low_;
-};
-
-/**
- * Return the low unsigned 32-bits value.
- *
- * @method
- * @return {number} the low 32-bits as an unsigned value.
- */
-Long.prototype.getLowBitsUnsigned = function() {
- return this.low_ >= 0 ? this.low_ : Long.TWO_PWR_32_DBL_ + this.low_;
-};
-
-/**
- * Returns the number of bits needed to represent the absolute value of this Long.
- *
- * @method
- * @return {number} Returns the number of bits needed to represent the absolute value of this Long.
- */
-Long.prototype.getNumBitsAbs = function() {
- if (this.isNegative()) {
- if (this.equals(Long.MIN_VALUE)) {
- return 64;
- } else {
- return this.negate().getNumBitsAbs();
- }
- } else {
- var val = this.high_ !== 0 ? this.high_ : this.low_;
- for (var bit = 31; bit > 0; bit--) {
- if ((val & (1 << bit)) !== 0) {
- break;
- }
- }
- return this.high_ !== 0 ? bit + 33 : bit + 1;
- }
-};
-
-/**
- * Return whether this value is zero.
- *
- * @method
- * @return {boolean} whether this value is zero.
- */
-Long.prototype.isZero = function() {
- return this.high_ === 0 && this.low_ === 0;
-};
-
-/**
- * Return whether this value is negative.
- *
- * @method
- * @return {boolean} whether this value is negative.
- */
-Long.prototype.isNegative = function() {
- return this.high_ < 0;
-};
-
-/**
- * Return whether this value is odd.
- *
- * @method
- * @return {boolean} whether this value is odd.
- */
-Long.prototype.isOdd = function() {
- return (this.low_ & 1) === 1;
-};
-
-/**
- * Return whether this Long equals the other
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long equals the other
- */
-Long.prototype.equals = function(other) {
- return this.high_ === other.high_ && this.low_ === other.low_;
-};
-
-/**
- * Return whether this Long does not equal the other.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long does not equal the other.
- */
-Long.prototype.notEquals = function(other) {
- return this.high_ !== other.high_ || this.low_ !== other.low_;
-};
-
-/**
- * Return whether this Long is less than the other.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long is less than the other.
- */
-Long.prototype.lessThan = function(other) {
- return this.compare(other) < 0;
-};
-
-/**
- * Return whether this Long is less than or equal to the other.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long is less than or equal to the other.
- */
-Long.prototype.lessThanOrEqual = function(other) {
- return this.compare(other) <= 0;
-};
-
-/**
- * Return whether this Long is greater than the other.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long is greater than the other.
- */
-Long.prototype.greaterThan = function(other) {
- return this.compare(other) > 0;
-};
-
-/**
- * Return whether this Long is greater than or equal to the other.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} whether this Long is greater than or equal to the other.
- */
-Long.prototype.greaterThanOrEqual = function(other) {
- return this.compare(other) >= 0;
-};
-
-/**
- * Compares this Long with the given one.
- *
- * @method
- * @param {Long} other Long to compare against.
- * @return {boolean} 0 if they are the same, 1 if the this is greater, and -1 if the given one is greater.
- */
-Long.prototype.compare = function(other) {
- if (this.equals(other)) {
- return 0;
- }
-
- var thisNeg = this.isNegative();
- var otherNeg = other.isNegative();
- if (thisNeg && !otherNeg) {
- return -1;
- }
- if (!thisNeg && otherNeg) {
- return 1;
- }
-
- // at this point, the signs are the same, so subtraction will not overflow
- if (this.subtract(other).isNegative()) {
- return -1;
- } else {
- return 1;
- }
-};
-
-/**
- * The negation of this value.
- *
- * @method
- * @return {Long} the negation of this value.
- */
-Long.prototype.negate = function() {
- if (this.equals(Long.MIN_VALUE)) {
- return Long.MIN_VALUE;
- } else {
- return this.not().add(Long.ONE);
- }
-};
-
-/**
- * Returns the sum of this and the given Long.
- *
- * @method
- * @param {Long} other Long to add to this one.
- * @return {Long} the sum of this and the given Long.
- */
-Long.prototype.add = function(other) {
- // Divide each number into 4 chunks of 16 bits, and then sum the chunks.
-
- var a48 = this.high_ >>> 16;
- var a32 = this.high_ & 0xffff;
- var a16 = this.low_ >>> 16;
- var a00 = this.low_ & 0xffff;
-
- var b48 = other.high_ >>> 16;
- var b32 = other.high_ & 0xffff;
- var b16 = other.low_ >>> 16;
- var b00 = other.low_ & 0xffff;
-
- var c48 = 0,
- c32 = 0,
- c16 = 0,
- c00 = 0;
- c00 += a00 + b00;
- c16 += c00 >>> 16;
- c00 &= 0xffff;
- c16 += a16 + b16;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c32 += a32 + b32;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c48 += a48 + b48;
- c48 &= 0xffff;
- return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32);
-};
-
-/**
- * Returns the difference of this and the given Long.
- *
- * @method
- * @param {Long} other Long to subtract from this.
- * @return {Long} the difference of this and the given Long.
- */
-Long.prototype.subtract = function(other) {
- return this.add(other.negate());
-};
-
-/**
- * Returns the product of this and the given Long.
- *
- * @method
- * @param {Long} other Long to multiply with this.
- * @return {Long} the product of this and the other.
- */
-Long.prototype.multiply = function(other) {
- if (this.isZero()) {
- return Long.ZERO;
- } else if (other.isZero()) {
- return Long.ZERO;
- }
-
- if (this.equals(Long.MIN_VALUE)) {
- return other.isOdd() ? Long.MIN_VALUE : Long.ZERO;
- } else if (other.equals(Long.MIN_VALUE)) {
- return this.isOdd() ? Long.MIN_VALUE : Long.ZERO;
- }
-
- if (this.isNegative()) {
- if (other.isNegative()) {
- return this.negate().multiply(other.negate());
- } else {
- return this.negate()
- .multiply(other)
- .negate();
- }
- } else if (other.isNegative()) {
- return this.multiply(other.negate()).negate();
- }
-
- // If both Longs are small, use float multiplication
- if (this.lessThan(Long.TWO_PWR_24_) && other.lessThan(Long.TWO_PWR_24_)) {
- return Long.fromNumber(this.toNumber() * other.toNumber());
- }
-
- // Divide each Long into 4 chunks of 16 bits, and then add up 4x4 products.
- // We can skip products that would overflow.
-
- var a48 = this.high_ >>> 16;
- var a32 = this.high_ & 0xffff;
- var a16 = this.low_ >>> 16;
- var a00 = this.low_ & 0xffff;
-
- var b48 = other.high_ >>> 16;
- var b32 = other.high_ & 0xffff;
- var b16 = other.low_ >>> 16;
- var b00 = other.low_ & 0xffff;
-
- var c48 = 0,
- c32 = 0,
- c16 = 0,
- c00 = 0;
- c00 += a00 * b00;
- c16 += c00 >>> 16;
- c00 &= 0xffff;
- c16 += a16 * b00;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c16 += a00 * b16;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c32 += a32 * b00;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c32 += a16 * b16;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c32 += a00 * b32;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;
- c48 &= 0xffff;
- return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32);
-};
-
-/**
- * Returns this Long divided by the given one.
- *
- * @method
- * @param {Long} other Long by which to divide.
- * @return {Long} this Long divided by the given one.
- */
-Long.prototype.div = function(other) {
- if (other.isZero()) {
- throw Error('division by zero');
- } else if (this.isZero()) {
- return Long.ZERO;
- }
-
- if (this.equals(Long.MIN_VALUE)) {
- if (other.equals(Long.ONE) || other.equals(Long.NEG_ONE)) {
- return Long.MIN_VALUE; // recall that -MIN_VALUE == MIN_VALUE
- } else if (other.equals(Long.MIN_VALUE)) {
- return Long.ONE;
- } else {
- // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.
- var halfThis = this.shiftRight(1);
- var approx = halfThis.div(other).shiftLeft(1);
- if (approx.equals(Long.ZERO)) {
- return other.isNegative() ? Long.ONE : Long.NEG_ONE;
- } else {
- var rem = this.subtract(other.multiply(approx));
- var result = approx.add(rem.div(other));
- return result;
- }
- }
- } else if (other.equals(Long.MIN_VALUE)) {
- return Long.ZERO;
- }
-
- if (this.isNegative()) {
- if (other.isNegative()) {
- return this.negate().div(other.negate());
- } else {
- return this.negate()
- .div(other)
- .negate();
- }
- } else if (other.isNegative()) {
- return this.div(other.negate()).negate();
- }
-
- // Repeat the following until the remainder is less than other: find a
- // floating-point that approximates remainder / other *from below*, add this
- // into the result, and subtract it from the remainder. It is critical that
- // the approximate value is less than or equal to the real value so that the
- // remainder never becomes negative.
- var res = Long.ZERO;
- rem = this;
- while (rem.greaterThanOrEqual(other)) {
- // Approximate the result of division. This may be a little greater or
- // smaller than the actual value.
- approx = Math.max(1, Math.floor(rem.toNumber() / other.toNumber()));
-
- // We will tweak the approximate result by changing it in the 48-th digit or
- // the smallest non-fractional digit, whichever is larger.
- var log2 = Math.ceil(Math.log(approx) / Math.LN2);
- var delta = log2 <= 48 ? 1 : Math.pow(2, log2 - 48);
-
- // Decrease the approximation until it is smaller than the remainder. Note
- // that if it is too large, the product overflows and is negative.
- var approxRes = Long.fromNumber(approx);
- var approxRem = approxRes.multiply(other);
- while (approxRem.isNegative() || approxRem.greaterThan(rem)) {
- approx -= delta;
- approxRes = Long.fromNumber(approx);
- approxRem = approxRes.multiply(other);
- }
-
- // We know the answer can't be zero... and actually, zero would cause
- // infinite recursion since we would make no progress.
- if (approxRes.isZero()) {
- approxRes = Long.ONE;
- }
-
- res = res.add(approxRes);
- rem = rem.subtract(approxRem);
- }
- return res;
-};
-
-/**
- * Returns this Long modulo the given one.
- *
- * @method
- * @param {Long} other Long by which to mod.
- * @return {Long} this Long modulo the given one.
- */
-Long.prototype.modulo = function(other) {
- return this.subtract(this.div(other).multiply(other));
-};
-
-/**
- * The bitwise-NOT of this value.
- *
- * @method
- * @return {Long} the bitwise-NOT of this value.
- */
-Long.prototype.not = function() {
- return Long.fromBits(~this.low_, ~this.high_);
-};
-
-/**
- * Returns the bitwise-AND of this Long and the given one.
- *
- * @method
- * @param {Long} other the Long with which to AND.
- * @return {Long} the bitwise-AND of this and the other.
- */
-Long.prototype.and = function(other) {
- return Long.fromBits(this.low_ & other.low_, this.high_ & other.high_);
-};
-
-/**
- * Returns the bitwise-OR of this Long and the given one.
- *
- * @method
- * @param {Long} other the Long with which to OR.
- * @return {Long} the bitwise-OR of this and the other.
- */
-Long.prototype.or = function(other) {
- return Long.fromBits(this.low_ | other.low_, this.high_ | other.high_);
-};
-
-/**
- * Returns the bitwise-XOR of this Long and the given one.
- *
- * @method
- * @param {Long} other the Long with which to XOR.
- * @return {Long} the bitwise-XOR of this and the other.
- */
-Long.prototype.xor = function(other) {
- return Long.fromBits(this.low_ ^ other.low_, this.high_ ^ other.high_);
-};
-
-/**
- * Returns this Long with bits shifted to the left by the given amount.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Long} this shifted to the left by the given amount.
- */
-Long.prototype.shiftLeft = function(numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var low = this.low_;
- if (numBits < 32) {
- var high = this.high_;
- return Long.fromBits(low << numBits, (high << numBits) | (low >>> (32 - numBits)));
- } else {
- return Long.fromBits(0, low << (numBits - 32));
- }
- }
-};
-
-/**
- * Returns this Long with bits shifted to the right by the given amount.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Long} this shifted to the right by the given amount.
- */
-Long.prototype.shiftRight = function(numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var high = this.high_;
- if (numBits < 32) {
- var low = this.low_;
- return Long.fromBits((low >>> numBits) | (high << (32 - numBits)), high >> numBits);
- } else {
- return Long.fromBits(high >> (numBits - 32), high >= 0 ? 0 : -1);
- }
- }
-};
-
-/**
- * Returns this Long with bits shifted to the right by the given amount, with the new top bits matching the current sign bit.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Long} this shifted to the right by the given amount, with zeros placed into the new leading bits.
- */
-Long.prototype.shiftRightUnsigned = function(numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var high = this.high_;
- if (numBits < 32) {
- var low = this.low_;
- return Long.fromBits((low >>> numBits) | (high << (32 - numBits)), high >>> numBits);
- } else if (numBits === 32) {
- return Long.fromBits(high, 0);
- } else {
- return Long.fromBits(high >>> (numBits - 32), 0);
- }
- }
-};
-
-/**
- * Returns a Long representing the given (32-bit) integer value.
- *
- * @method
- * @param {number} value the 32-bit integer in question.
- * @return {Long} the corresponding Long value.
- */
-Long.fromInt = function(value) {
- if (-128 <= value && value < 128) {
- var cachedObj = Long.INT_CACHE_[value];
- if (cachedObj) {
- return cachedObj;
- }
- }
-
- var obj = new Long(value | 0, value < 0 ? -1 : 0);
- if (-128 <= value && value < 128) {
- Long.INT_CACHE_[value] = obj;
- }
- return obj;
-};
-
-/**
- * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
- *
- * @method
- * @param {number} value the number in question.
- * @return {Long} the corresponding Long value.
- */
-Long.fromNumber = function(value) {
- if (isNaN(value) || !isFinite(value)) {
- return Long.ZERO;
- } else if (value <= -Long.TWO_PWR_63_DBL_) {
- return Long.MIN_VALUE;
- } else if (value + 1 >= Long.TWO_PWR_63_DBL_) {
- return Long.MAX_VALUE;
- } else if (value < 0) {
- return Long.fromNumber(-value).negate();
- } else {
- return new Long((value % Long.TWO_PWR_32_DBL_) | 0, (value / Long.TWO_PWR_32_DBL_) | 0);
- }
-};
-
-/**
- * Returns a Long representing the 64-bit integer that comes by concatenating the given high and low bits. Each is assumed to use 32 bits.
- *
- * @method
- * @param {number} lowBits the low 32-bits.
- * @param {number} highBits the high 32-bits.
- * @return {Long} the corresponding Long value.
- */
-Long.fromBits = function(lowBits, highBits) {
- return new Long(lowBits, highBits);
-};
-
-/**
- * Returns a Long representation of the given string, written using the given radix.
- *
- * @method
- * @param {string} str the textual representation of the Long.
- * @param {number} opt_radix the radix in which the text is written.
- * @return {Long} the corresponding Long value.
- */
-Long.fromString = function(str, opt_radix) {
- if (str.length === 0) {
- throw Error('number format error: empty string');
- }
-
- var radix = opt_radix || 10;
- if (radix < 2 || 36 < radix) {
- throw Error('radix out of range: ' + radix);
- }
-
- if (str.charAt(0) === '-') {
- return Long.fromString(str.substring(1), radix).negate();
- } else if (str.indexOf('-') >= 0) {
- throw Error('number format error: interior "-" character: ' + str);
- }
-
- // Do several (8) digits each time through the loop, so as to
- // minimize the calls to the very expensive emulated div.
- var radixToPower = Long.fromNumber(Math.pow(radix, 8));
-
- var result = Long.ZERO;
- for (var i = 0; i < str.length; i += 8) {
- var size = Math.min(8, str.length - i);
- var value = parseInt(str.substring(i, i + size), radix);
- if (size < 8) {
- var power = Long.fromNumber(Math.pow(radix, size));
- result = result.multiply(power).add(Long.fromNumber(value));
- } else {
- result = result.multiply(radixToPower);
- result = result.add(Long.fromNumber(value));
- }
- }
- return result;
-};
-
-// NOTE: Common constant values ZERO, ONE, NEG_ONE, etc. are defined below the
-// from* methods on which they depend.
-
-/**
- * A cache of the Long representations of small integer values.
- * @type {Object}
- * @ignore
- */
-Long.INT_CACHE_ = {};
-
-// NOTE: the compiler should inline these constant values below and then remove
-// these variables, so there should be no runtime penalty for these.
-
-/**
- * Number used repeated below in calculations. This must appear before the
- * first call to any from* function below.
- * @type {number}
- * @ignore
- */
-Long.TWO_PWR_16_DBL_ = 1 << 16;
-
-/**
- * @type {number}
- * @ignore
- */
-Long.TWO_PWR_24_DBL_ = 1 << 24;
-
-/**
- * @type {number}
- * @ignore
- */
-Long.TWO_PWR_32_DBL_ = Long.TWO_PWR_16_DBL_ * Long.TWO_PWR_16_DBL_;
-
-/**
- * @type {number}
- * @ignore
- */
-Long.TWO_PWR_31_DBL_ = Long.TWO_PWR_32_DBL_ / 2;
-
-/**
- * @type {number}
- * @ignore
- */
-Long.TWO_PWR_48_DBL_ = Long.TWO_PWR_32_DBL_ * Long.TWO_PWR_16_DBL_;
-
-/**
- * @type {number}
- * @ignore
- */
-Long.TWO_PWR_64_DBL_ = Long.TWO_PWR_32_DBL_ * Long.TWO_PWR_32_DBL_;
-
-/**
- * @type {number}
- * @ignore
- */
-Long.TWO_PWR_63_DBL_ = Long.TWO_PWR_64_DBL_ / 2;
-
-/** @type {Long} */
-Long.ZERO = Long.fromInt(0);
-
-/** @type {Long} */
-Long.ONE = Long.fromInt(1);
-
-/** @type {Long} */
-Long.NEG_ONE = Long.fromInt(-1);
-
-/** @type {Long} */
-Long.MAX_VALUE = Long.fromBits(0xffffffff | 0, 0x7fffffff | 0);
-
-/** @type {Long} */
-Long.MIN_VALUE = Long.fromBits(0, 0x80000000 | 0);
-
-/**
- * @type {Long}
- * @ignore
- */
-Long.TWO_PWR_24_ = Long.fromInt(1 << 24);
-
-/**
- * Expose.
- */
-module.exports = Long;
-module.exports.Long = Long;
diff --git a/node_modules/bson/lib/bson/map.js b/node_modules/bson/lib/bson/map.js
deleted file mode 100644
index 7edb4f2..0000000
--- a/node_modules/bson/lib/bson/map.js
+++ /dev/null
@@ -1,128 +0,0 @@
-'use strict';
-
-// We have an ES6 Map available, return the native instance
-if (typeof global.Map !== 'undefined') {
- module.exports = global.Map;
- module.exports.Map = global.Map;
-} else {
- // We will return a polyfill
- var Map = function(array) {
- this._keys = [];
- this._values = {};
-
- for (var i = 0; i < array.length; i++) {
- if (array[i] == null) continue; // skip null and undefined
- var entry = array[i];
- var key = entry[0];
- var value = entry[1];
- // Add the key to the list of keys in order
- this._keys.push(key);
- // Add the key and value to the values dictionary with a point
- // to the location in the ordered keys list
- this._values[key] = { v: value, i: this._keys.length - 1 };
- }
- };
-
- Map.prototype.clear = function() {
- this._keys = [];
- this._values = {};
- };
-
- Map.prototype.delete = function(key) {
- var value = this._values[key];
- if (value == null) return false;
- // Delete entry
- delete this._values[key];
- // Remove the key from the ordered keys list
- this._keys.splice(value.i, 1);
- return true;
- };
-
- Map.prototype.entries = function() {
- var self = this;
- var index = 0;
-
- return {
- next: function() {
- var key = self._keys[index++];
- return {
- value: key !== undefined ? [key, self._values[key].v] : undefined,
- done: key !== undefined ? false : true
- };
- }
- };
- };
-
- Map.prototype.forEach = function(callback, self) {
- self = self || this;
-
- for (var i = 0; i < this._keys.length; i++) {
- var key = this._keys[i];
- // Call the forEach callback
- callback.call(self, this._values[key].v, key, self);
- }
- };
-
- Map.prototype.get = function(key) {
- return this._values[key] ? this._values[key].v : undefined;
- };
-
- Map.prototype.has = function(key) {
- return this._values[key] != null;
- };
-
- Map.prototype.keys = function() {
- var self = this;
- var index = 0;
-
- return {
- next: function() {
- var key = self._keys[index++];
- return {
- value: key !== undefined ? key : undefined,
- done: key !== undefined ? false : true
- };
- }
- };
- };
-
- Map.prototype.set = function(key, value) {
- if (this._values[key]) {
- this._values[key].v = value;
- return this;
- }
-
- // Add the key to the list of keys in order
- this._keys.push(key);
- // Add the key and value to the values dictionary with a point
- // to the location in the ordered keys list
- this._values[key] = { v: value, i: this._keys.length - 1 };
- return this;
- };
-
- Map.prototype.values = function() {
- var self = this;
- var index = 0;
-
- return {
- next: function() {
- var key = self._keys[index++];
- return {
- value: key !== undefined ? self._values[key].v : undefined,
- done: key !== undefined ? false : true
- };
- }
- };
- };
-
- // Last ismaster
- Object.defineProperty(Map.prototype, 'size', {
- enumerable: true,
- get: function() {
- return this._keys.length;
- }
- });
-
- module.exports = Map;
- module.exports.Map = Map;
-}
diff --git a/node_modules/bson/lib/bson/max_key.js b/node_modules/bson/lib/bson/max_key.js
deleted file mode 100644
index eebca7b..0000000
--- a/node_modules/bson/lib/bson/max_key.js
+++ /dev/null
@@ -1,14 +0,0 @@
-/**
- * A class representation of the BSON MaxKey type.
- *
- * @class
- * @return {MaxKey} A MaxKey instance
- */
-function MaxKey() {
- if (!(this instanceof MaxKey)) return new MaxKey();
-
- this._bsontype = 'MaxKey';
-}
-
-module.exports = MaxKey;
-module.exports.MaxKey = MaxKey;
diff --git a/node_modules/bson/lib/bson/min_key.js b/node_modules/bson/lib/bson/min_key.js
deleted file mode 100644
index 15f4522..0000000
--- a/node_modules/bson/lib/bson/min_key.js
+++ /dev/null
@@ -1,14 +0,0 @@
-/**
- * A class representation of the BSON MinKey type.
- *
- * @class
- * @return {MinKey} A MinKey instance
- */
-function MinKey() {
- if (!(this instanceof MinKey)) return new MinKey();
-
- this._bsontype = 'MinKey';
-}
-
-module.exports = MinKey;
-module.exports.MinKey = MinKey;
diff --git a/node_modules/bson/lib/bson/objectid.js b/node_modules/bson/lib/bson/objectid.js
deleted file mode 100644
index 0ebcc03..0000000
--- a/node_modules/bson/lib/bson/objectid.js
+++ /dev/null
@@ -1,392 +0,0 @@
-// Custom inspect property name / symbol.
-var inspect = 'inspect';
-
-var utils = require('./parser/utils');
-
-/**
- * Machine id.
- *
- * Create a random 3-byte value (i.e. unique for this
- * process). Other drivers use a md5 of the machine id here, but
- * that would mean an asyc call to gethostname, so we don't bother.
- * @ignore
- */
-var MACHINE_ID = parseInt(Math.random() * 0xffffff, 10);
-
-// Regular expression that checks for hex value
-var checkForHexRegExp = new RegExp('^[0-9a-fA-F]{24}$');
-
-// Check if buffer exists
-try {
- if (Buffer && Buffer.from) {
- var hasBufferType = true;
- inspect = require('util').inspect.custom || 'inspect';
- }
-} catch (err) {
- hasBufferType = false;
-}
-
-/**
-* Create a new ObjectID instance
-*
-* @class
-* @param {(string|number)} id Can be a 24 byte hex string, 12 byte binary string or a Number.
-* @property {number} generationTime The generation time of this ObjectId instance
-* @return {ObjectID} instance of ObjectID.
-*/
-var ObjectID = function ObjectID(id) {
- // Duck-typing to support ObjectId from different npm packages
- if (id instanceof ObjectID) return id;
- if (!(this instanceof ObjectID)) return new ObjectID(id);
-
- this._bsontype = 'ObjectID';
-
- // The most common usecase (blank id, new objectId instance)
- if (id == null || typeof id === 'number') {
- // Generate a new id
- this.id = this.generate(id);
- // If we are caching the hex string
- if (ObjectID.cacheHexString) this.__id = this.toString('hex');
- // Return the object
- return;
- }
-
- // Check if the passed in id is valid
- var valid = ObjectID.isValid(id);
-
- // Throw an error if it's not a valid setup
- if (!valid && id != null) {
- throw new Error(
- 'Argument passed in must be a single String of 12 bytes or a string of 24 hex characters'
- );
- } else if (valid && typeof id === 'string' && id.length === 24 && hasBufferType) {
- return new ObjectID(utils.toBuffer(id, 'hex'));
- } else if (valid && typeof id === 'string' && id.length === 24) {
- return ObjectID.createFromHexString(id);
- } else if (id != null && id.length === 12) {
- // assume 12 byte string
- this.id = id;
- } else if (id != null && typeof id.toHexString === 'function') {
- // Duck-typing to support ObjectId from different npm packages
- return id;
- } else {
- throw new Error(
- 'Argument passed in must be a single String of 12 bytes or a string of 24 hex characters'
- );
- }
-
- if (ObjectID.cacheHexString) this.__id = this.toString('hex');
-};
-
-// Allow usage of ObjectId as well as ObjectID
-// var ObjectId = ObjectID;
-
-// Precomputed hex table enables speedy hex string conversion
-var hexTable = [];
-for (var i = 0; i < 256; i++) {
- hexTable[i] = (i <= 15 ? '0' : '') + i.toString(16);
-}
-
-/**
-* Return the ObjectID id as a 24 byte hex string representation
-*
-* @method
-* @return {string} return the 24 byte hex string representation.
-*/
-ObjectID.prototype.toHexString = function() {
- if (ObjectID.cacheHexString && this.__id) return this.__id;
-
- var hexString = '';
- if (!this.id || !this.id.length) {
- throw new Error(
- 'invalid ObjectId, ObjectId.id must be either a string or a Buffer, but is [' +
- JSON.stringify(this.id) +
- ']'
- );
- }
-
- if (this.id instanceof _Buffer) {
- hexString = convertToHex(this.id);
- if (ObjectID.cacheHexString) this.__id = hexString;
- return hexString;
- }
-
- for (var i = 0; i < this.id.length; i++) {
- hexString += hexTable[this.id.charCodeAt(i)];
- }
-
- if (ObjectID.cacheHexString) this.__id = hexString;
- return hexString;
-};
-
-/**
-* Update the ObjectID index used in generating new ObjectID's on the driver
-*
-* @method
-* @return {number} returns next index value.
-* @ignore
-*/
-ObjectID.prototype.get_inc = function() {
- return (ObjectID.index = (ObjectID.index + 1) % 0xffffff);
-};
-
-/**
-* Update the ObjectID index used in generating new ObjectID's on the driver
-*
-* @method
-* @return {number} returns next index value.
-* @ignore
-*/
-ObjectID.prototype.getInc = function() {
- return this.get_inc();
-};
-
-/**
-* Generate a 12 byte id buffer used in ObjectID's
-*
-* @method
-* @param {number} [time] optional parameter allowing to pass in a second based timestamp.
-* @return {Buffer} return the 12 byte id buffer string.
-*/
-ObjectID.prototype.generate = function(time) {
- if ('number' !== typeof time) {
- time = ~~(Date.now() / 1000);
- }
-
- // Use pid
- var pid =
- (typeof process === 'undefined' || process.pid === 1
- ? Math.floor(Math.random() * 100000)
- : process.pid) % 0xffff;
- var inc = this.get_inc();
- // Buffer used
- var buffer = utils.allocBuffer(12);
- // Encode time
- buffer[3] = time & 0xff;
- buffer[2] = (time >> 8) & 0xff;
- buffer[1] = (time >> 16) & 0xff;
- buffer[0] = (time >> 24) & 0xff;
- // Encode machine
- buffer[6] = MACHINE_ID & 0xff;
- buffer[5] = (MACHINE_ID >> 8) & 0xff;
- buffer[4] = (MACHINE_ID >> 16) & 0xff;
- // Encode pid
- buffer[8] = pid & 0xff;
- buffer[7] = (pid >> 8) & 0xff;
- // Encode index
- buffer[11] = inc & 0xff;
- buffer[10] = (inc >> 8) & 0xff;
- buffer[9] = (inc >> 16) & 0xff;
- // Return the buffer
- return buffer;
-};
-
-/**
-* Converts the id into a 24 byte hex string for printing
-*
-* @param {String} format The Buffer toString format parameter.
-* @return {String} return the 24 byte hex string representation.
-* @ignore
-*/
-ObjectID.prototype.toString = function(format) {
- // Is the id a buffer then use the buffer toString method to return the format
- if (this.id && this.id.copy) {
- return this.id.toString(typeof format === 'string' ? format : 'hex');
- }
-
- // if(this.buffer )
- return this.toHexString();
-};
-
-/**
-* Converts to a string representation of this Id.
-*
-* @return {String} return the 24 byte hex string representation.
-* @ignore
-*/
-ObjectID.prototype[inspect] = ObjectID.prototype.toString;
-
-/**
-* Converts to its JSON representation.
-*
-* @return {String} return the 24 byte hex string representation.
-* @ignore
-*/
-ObjectID.prototype.toJSON = function() {
- return this.toHexString();
-};
-
-/**
-* Compares the equality of this ObjectID with `otherID`.
-*
-* @method
-* @param {object} otherID ObjectID instance to compare against.
-* @return {boolean} the result of comparing two ObjectID's
-*/
-ObjectID.prototype.equals = function equals(otherId) {
- // var id;
-
- if (otherId instanceof ObjectID) {
- return this.toString() === otherId.toString();
- } else if (
- typeof otherId === 'string' &&
- ObjectID.isValid(otherId) &&
- otherId.length === 12 &&
- this.id instanceof _Buffer
- ) {
- return otherId === this.id.toString('binary');
- } else if (typeof otherId === 'string' && ObjectID.isValid(otherId) && otherId.length === 24) {
- return otherId.toLowerCase() === this.toHexString();
- } else if (typeof otherId === 'string' && ObjectID.isValid(otherId) && otherId.length === 12) {
- return otherId === this.id;
- } else if (otherId != null && (otherId instanceof ObjectID || otherId.toHexString)) {
- return otherId.toHexString() === this.toHexString();
- } else {
- return false;
- }
-};
-
-/**
-* Returns the generation date (accurate up to the second) that this ID was generated.
-*
-* @method
-* @return {date} the generation date
-*/
-ObjectID.prototype.getTimestamp = function() {
- var timestamp = new Date();
- var time = this.id[3] | (this.id[2] << 8) | (this.id[1] << 16) | (this.id[0] << 24);
- timestamp.setTime(Math.floor(time) * 1000);
- return timestamp;
-};
-
-/**
-* @ignore
-*/
-ObjectID.index = ~~(Math.random() * 0xffffff);
-
-/**
-* @ignore
-*/
-ObjectID.createPk = function createPk() {
- return new ObjectID();
-};
-
-/**
-* Creates an ObjectID from a second based number, with the rest of the ObjectID zeroed out. Used for comparisons or sorting the ObjectID.
-*
-* @method
-* @param {number} time an integer number representing a number of seconds.
-* @return {ObjectID} return the created ObjectID
-*/
-ObjectID.createFromTime = function createFromTime(time) {
- var buffer = utils.toBuffer([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]);
- // Encode time into first 4 bytes
- buffer[3] = time & 0xff;
- buffer[2] = (time >> 8) & 0xff;
- buffer[1] = (time >> 16) & 0xff;
- buffer[0] = (time >> 24) & 0xff;
- // Return the new objectId
- return new ObjectID(buffer);
-};
-
-// Lookup tables
-//var encodeLookup = '0123456789abcdef'.split('');
-var decodeLookup = [];
-i = 0;
-while (i < 10) decodeLookup[0x30 + i] = i++;
-while (i < 16) decodeLookup[0x41 - 10 + i] = decodeLookup[0x61 - 10 + i] = i++;
-
-var _Buffer = Buffer;
-var convertToHex = function(bytes) {
- return bytes.toString('hex');
-};
-
-/**
-* Creates an ObjectID from a hex string representation of an ObjectID.
-*
-* @method
-* @param {string} hexString create a ObjectID from a passed in 24 byte hexstring.
-* @return {ObjectID} return the created ObjectID
-*/
-ObjectID.createFromHexString = function createFromHexString(string) {
- // Throw an error if it's not a valid setup
- if (typeof string === 'undefined' || (string != null && string.length !== 24)) {
- throw new Error(
- 'Argument passed in must be a single String of 12 bytes or a string of 24 hex characters'
- );
- }
-
- // Use Buffer.from method if available
- if (hasBufferType) return new ObjectID(utils.toBuffer(string, 'hex'));
-
- // Calculate lengths
- var array = new _Buffer(12);
- var n = 0;
- var i = 0;
-
- while (i < 24) {
- array[n++] = (decodeLookup[string.charCodeAt(i++)] << 4) | decodeLookup[string.charCodeAt(i++)];
- }
-
- return new ObjectID(array);
-};
-
-/**
-* Checks if a value is a valid bson ObjectId
-*
-* @method
-* @return {boolean} return true if the value is a valid bson ObjectId, return false otherwise.
-*/
-ObjectID.isValid = function isValid(id) {
- if (id == null) return false;
-
- if (typeof id === 'number') {
- return true;
- }
-
- if (typeof id === 'string') {
- return id.length === 12 || (id.length === 24 && checkForHexRegExp.test(id));
- }
-
- if (id instanceof ObjectID) {
- return true;
- }
-
- if (id instanceof _Buffer) {
- return true;
- }
-
- // Duck-Typing detection of ObjectId like objects
- if (
- typeof id.toHexString === 'function' &&
- (id.id instanceof _Buffer || typeof id.id === 'string')
- ) {
- return id.id.length === 12 || (id.id.length === 24 && checkForHexRegExp.test(id.id));
- }
-
- return false;
-};
-
-/**
-* @ignore
-*/
-Object.defineProperty(ObjectID.prototype, 'generationTime', {
- enumerable: true,
- get: function() {
- return this.id[3] | (this.id[2] << 8) | (this.id[1] << 16) | (this.id[0] << 24);
- },
- set: function(value) {
- // Encode time into first 4 bytes
- this.id[3] = value & 0xff;
- this.id[2] = (value >> 8) & 0xff;
- this.id[1] = (value >> 16) & 0xff;
- this.id[0] = (value >> 24) & 0xff;
- }
-});
-
-/**
- * Expose.
- */
-module.exports = ObjectID;
-module.exports.ObjectID = ObjectID;
-module.exports.ObjectId = ObjectID;
diff --git a/node_modules/bson/lib/bson/parser/calculate_size.js b/node_modules/bson/lib/bson/parser/calculate_size.js
deleted file mode 100644
index 7e0026c..0000000
--- a/node_modules/bson/lib/bson/parser/calculate_size.js
+++ /dev/null
@@ -1,255 +0,0 @@
-'use strict';
-
-var Long = require('../long').Long,
- Double = require('../double').Double,
- Timestamp = require('../timestamp').Timestamp,
- ObjectID = require('../objectid').ObjectID,
- Symbol = require('../symbol').Symbol,
- BSONRegExp = require('../regexp').BSONRegExp,
- Code = require('../code').Code,
- Decimal128 = require('../decimal128'),
- MinKey = require('../min_key').MinKey,
- MaxKey = require('../max_key').MaxKey,
- DBRef = require('../db_ref').DBRef,
- Binary = require('../binary').Binary;
-
-var normalizedFunctionString = require('./utils').normalizedFunctionString;
-
-// To ensure that 0.4 of node works correctly
-var isDate = function isDate(d) {
- return typeof d === 'object' && Object.prototype.toString.call(d) === '[object Date]';
-};
-
-var calculateObjectSize = function calculateObjectSize(
- object,
- serializeFunctions,
- ignoreUndefined
-) {
- var totalLength = 4 + 1;
-
- if (Array.isArray(object)) {
- for (var i = 0; i < object.length; i++) {
- totalLength += calculateElement(
- i.toString(),
- object[i],
- serializeFunctions,
- true,
- ignoreUndefined
- );
- }
- } else {
- // If we have toBSON defined, override the current object
- if (object.toBSON) {
- object = object.toBSON();
- }
-
- // Calculate size
- for (var key in object) {
- totalLength += calculateElement(key, object[key], serializeFunctions, false, ignoreUndefined);
- }
- }
-
- return totalLength;
-};
-
-/**
- * @ignore
- * @api private
- */
-function calculateElement(name, value, serializeFunctions, isArray, ignoreUndefined) {
- // If we have toBSON defined, override the current object
- if (value && value.toBSON) {
- value = value.toBSON();
- }
-
- switch (typeof value) {
- case 'string':
- return 1 + Buffer.byteLength(name, 'utf8') + 1 + 4 + Buffer.byteLength(value, 'utf8') + 1;
- case 'number':
- if (Math.floor(value) === value && value >= BSON.JS_INT_MIN && value <= BSON.JS_INT_MAX) {
- if (value >= BSON.BSON_INT32_MIN && value <= BSON.BSON_INT32_MAX) {
- // 32 bit
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (4 + 1);
- } else {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
- }
- } else {
- // 64 bit
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
- }
- case 'undefined':
- if (isArray || !ignoreUndefined)
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
- return 0;
- case 'boolean':
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 1);
- case 'object':
- if (
- value == null ||
- value instanceof MinKey ||
- value instanceof MaxKey ||
- value['_bsontype'] === 'MinKey' ||
- value['_bsontype'] === 'MaxKey'
- ) {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
- } else if (value instanceof ObjectID || value['_bsontype'] === 'ObjectID' || value['_bsontype'] === 'ObjectId') {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (12 + 1);
- } else if (value instanceof Date || isDate(value)) {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
- } else if (typeof Buffer !== 'undefined' && Buffer.isBuffer(value)) {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 4 + 1) + value.length
- );
- } else if (
- value instanceof Long ||
- value instanceof Double ||
- value instanceof Timestamp ||
- value['_bsontype'] === 'Long' ||
- value['_bsontype'] === 'Double' ||
- value['_bsontype'] === 'Timestamp'
- ) {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
- } else if (value instanceof Decimal128 || value['_bsontype'] === 'Decimal128') {
- return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (16 + 1);
- } else if (value instanceof Code || value['_bsontype'] === 'Code') {
- // Calculate size depending on the availability of a scope
- if (value.scope != null && Object.keys(value.scope).length > 0) {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- 1 +
- 4 +
- 4 +
- Buffer.byteLength(value.code.toString(), 'utf8') +
- 1 +
- calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined)
- );
- } else {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- 1 +
- 4 +
- Buffer.byteLength(value.code.toString(), 'utf8') +
- 1
- );
- }
- } else if (value instanceof Binary || value['_bsontype'] === 'Binary') {
- // Check what kind of subtype we have
- if (value.sub_type === Binary.SUBTYPE_BYTE_ARRAY) {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- (value.position + 1 + 4 + 1 + 4)
- );
- } else {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (value.position + 1 + 4 + 1)
- );
- }
- } else if (value instanceof Symbol || value['_bsontype'] === 'Symbol') {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- Buffer.byteLength(value.value, 'utf8') +
- 4 +
- 1 +
- 1
- );
- } else if (value instanceof DBRef || value['_bsontype'] === 'DBRef') {
- // Set up correct object for serialization
- var ordered_values = {
- $ref: value.namespace,
- $id: value.oid
- };
-
- // Add db reference if it exists
- if (null != value.db) {
- ordered_values['$db'] = value.db;
- }
-
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- 1 +
- calculateObjectSize(ordered_values, serializeFunctions, ignoreUndefined)
- );
- } else if (
- value instanceof RegExp ||
- Object.prototype.toString.call(value) === '[object RegExp]'
- ) {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- 1 +
- Buffer.byteLength(value.source, 'utf8') +
- 1 +
- (value.global ? 1 : 0) +
- (value.ignoreCase ? 1 : 0) +
- (value.multiline ? 1 : 0) +
- 1
- );
- } else if (value instanceof BSONRegExp || value['_bsontype'] === 'BSONRegExp') {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- 1 +
- Buffer.byteLength(value.pattern, 'utf8') +
- 1 +
- Buffer.byteLength(value.options, 'utf8') +
- 1
- );
- } else {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- calculateObjectSize(value, serializeFunctions, ignoreUndefined) +
- 1
- );
- }
- case 'function':
- // WTF for 0.4.X where typeof /someregexp/ === 'function'
- if (
- value instanceof RegExp ||
- Object.prototype.toString.call(value) === '[object RegExp]' ||
- String.call(value) === '[object RegExp]'
- ) {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- 1 +
- Buffer.byteLength(value.source, 'utf8') +
- 1 +
- (value.global ? 1 : 0) +
- (value.ignoreCase ? 1 : 0) +
- (value.multiline ? 1 : 0) +
- 1
- );
- } else {
- if (serializeFunctions && value.scope != null && Object.keys(value.scope).length > 0) {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- 1 +
- 4 +
- 4 +
- Buffer.byteLength(normalizedFunctionString(value), 'utf8') +
- 1 +
- calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined)
- );
- } else if (serializeFunctions) {
- return (
- (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
- 1 +
- 4 +
- Buffer.byteLength(normalizedFunctionString(value), 'utf8') +
- 1
- );
- }
- }
- }
-
- return 0;
-}
-
-var BSON = {};
-
-// BSON MAX VALUES
-BSON.BSON_INT32_MAX = 0x7fffffff;
-BSON.BSON_INT32_MIN = -0x80000000;
-
-// JS MAX PRECISE VALUES
-BSON.JS_INT_MAX = 0x20000000000000; // Any integer up to 2^53 can be precisely represented by a double.
-BSON.JS_INT_MIN = -0x20000000000000; // Any integer down to -2^53 can be precisely represented by a double.
-
-module.exports = calculateObjectSize;
diff --git a/node_modules/bson/lib/bson/parser/deserializer.js b/node_modules/bson/lib/bson/parser/deserializer.js
deleted file mode 100644
index be3c865..0000000
--- a/node_modules/bson/lib/bson/parser/deserializer.js
+++ /dev/null
@@ -1,782 +0,0 @@
-'use strict';
-
-var Long = require('../long').Long,
- Double = require('../double').Double,
- Timestamp = require('../timestamp').Timestamp,
- ObjectID = require('../objectid').ObjectID,
- Symbol = require('../symbol').Symbol,
- Code = require('../code').Code,
- MinKey = require('../min_key').MinKey,
- MaxKey = require('../max_key').MaxKey,
- Decimal128 = require('../decimal128'),
- Int32 = require('../int_32'),
- DBRef = require('../db_ref').DBRef,
- BSONRegExp = require('../regexp').BSONRegExp,
- Binary = require('../binary').Binary;
-
-var utils = require('./utils');
-
-var deserialize = function(buffer, options, isArray) {
- options = options == null ? {} : options;
- var index = options && options.index ? options.index : 0;
- // Read the document size
- var size =
- buffer[index] |
- (buffer[index + 1] << 8) |
- (buffer[index + 2] << 16) |
- (buffer[index + 3] << 24);
-
- // Ensure buffer is valid size
- if (size < 5 || buffer.length < size || size + index > buffer.length) {
- throw new Error('corrupt bson message');
- }
-
- // Illegal end value
- if (buffer[index + size - 1] !== 0) {
- throw new Error("One object, sized correctly, with a spot for an EOO, but the EOO isn't 0x00");
- }
-
- // Start deserializtion
- return deserializeObject(buffer, index, options, isArray);
-};
-
-var deserializeObject = function(buffer, index, options, isArray) {
- var evalFunctions = options['evalFunctions'] == null ? false : options['evalFunctions'];
- var cacheFunctions = options['cacheFunctions'] == null ? false : options['cacheFunctions'];
- var cacheFunctionsCrc32 =
- options['cacheFunctionsCrc32'] == null ? false : options['cacheFunctionsCrc32'];
-
- if (!cacheFunctionsCrc32) var crc32 = null;
-
- var fieldsAsRaw = options['fieldsAsRaw'] == null ? null : options['fieldsAsRaw'];
-
- // Return raw bson buffer instead of parsing it
- var raw = options['raw'] == null ? false : options['raw'];
-
- // Return BSONRegExp objects instead of native regular expressions
- var bsonRegExp = typeof options['bsonRegExp'] === 'boolean' ? options['bsonRegExp'] : false;
-
- // Controls the promotion of values vs wrapper classes
- var promoteBuffers = options['promoteBuffers'] == null ? false : options['promoteBuffers'];
- var promoteLongs = options['promoteLongs'] == null ? true : options['promoteLongs'];
- var promoteValues = options['promoteValues'] == null ? true : options['promoteValues'];
-
- // Set the start index
- var startIndex = index;
-
- // Validate that we have at least 4 bytes of buffer
- if (buffer.length < 5) throw new Error('corrupt bson message < 5 bytes long');
-
- // Read the document size
- var size =
- buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
-
- // Ensure buffer is valid size
- if (size < 5 || size > buffer.length) throw new Error('corrupt bson message');
-
- // Create holding object
- var object = isArray ? [] : {};
- // Used for arrays to skip having to perform utf8 decoding
- var arrayIndex = 0;
-
- var done = false;
-
- // While we have more left data left keep parsing
- // while (buffer[index + 1] !== 0) {
- while (!done) {
- // Read the type
- var elementType = buffer[index++];
- // If we get a zero it's the last byte, exit
- if (elementType === 0) break;
-
- // Get the start search index
- var i = index;
- // Locate the end of the c string
- while (buffer[i] !== 0x00 && i < buffer.length) {
- i++;
- }
-
- // If are at the end of the buffer there is a problem with the document
- if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
- var name = isArray ? arrayIndex++ : buffer.toString('utf8', index, i);
-
- index = i + 1;
-
- if (elementType === BSON.BSON_DATA_STRING) {
- var stringSize =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- if (
- stringSize <= 0 ||
- stringSize > buffer.length - index ||
- buffer[index + stringSize - 1] !== 0
- )
- throw new Error('bad string length in bson');
- object[name] = buffer.toString('utf8', index, index + stringSize - 1);
- index = index + stringSize;
- } else if (elementType === BSON.BSON_DATA_OID) {
- var oid = utils.allocBuffer(12);
- buffer.copy(oid, 0, index, index + 12);
- object[name] = new ObjectID(oid);
- index = index + 12;
- } else if (elementType === BSON.BSON_DATA_INT && promoteValues === false) {
- object[name] = new Int32(
- buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24)
- );
- } else if (elementType === BSON.BSON_DATA_INT) {
- object[name] =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- } else if (elementType === BSON.BSON_DATA_NUMBER && promoteValues === false) {
- object[name] = new Double(buffer.readDoubleLE(index));
- index = index + 8;
- } else if (elementType === BSON.BSON_DATA_NUMBER) {
- object[name] = buffer.readDoubleLE(index);
- index = index + 8;
- } else if (elementType === BSON.BSON_DATA_DATE) {
- var lowBits =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- var highBits =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- object[name] = new Date(new Long(lowBits, highBits).toNumber());
- } else if (elementType === BSON.BSON_DATA_BOOLEAN) {
- if (buffer[index] !== 0 && buffer[index] !== 1) throw new Error('illegal boolean type value');
- object[name] = buffer[index++] === 1;
- } else if (elementType === BSON.BSON_DATA_OBJECT) {
- var _index = index;
- var objectSize =
- buffer[index] |
- (buffer[index + 1] << 8) |
- (buffer[index + 2] << 16) |
- (buffer[index + 3] << 24);
- if (objectSize <= 0 || objectSize > buffer.length - index)
- throw new Error('bad embedded document length in bson');
-
- // We have a raw value
- if (raw) {
- object[name] = buffer.slice(index, index + objectSize);
- } else {
- object[name] = deserializeObject(buffer, _index, options, false);
- }
-
- index = index + objectSize;
- } else if (elementType === BSON.BSON_DATA_ARRAY) {
- _index = index;
- objectSize =
- buffer[index] |
- (buffer[index + 1] << 8) |
- (buffer[index + 2] << 16) |
- (buffer[index + 3] << 24);
- var arrayOptions = options;
-
- // Stop index
- var stopIndex = index + objectSize;
-
- // All elements of array to be returned as raw bson
- if (fieldsAsRaw && fieldsAsRaw[name]) {
- arrayOptions = {};
- for (var n in options) arrayOptions[n] = options[n];
- arrayOptions['raw'] = true;
- }
-
- object[name] = deserializeObject(buffer, _index, arrayOptions, true);
- index = index + objectSize;
-
- if (buffer[index - 1] !== 0) throw new Error('invalid array terminator byte');
- if (index !== stopIndex) throw new Error('corrupted array bson');
- } else if (elementType === BSON.BSON_DATA_UNDEFINED) {
- object[name] = undefined;
- } else if (elementType === BSON.BSON_DATA_NULL) {
- object[name] = null;
- } else if (elementType === BSON.BSON_DATA_LONG) {
- // Unpack the low and high bits
- lowBits =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- highBits =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- var long = new Long(lowBits, highBits);
- // Promote the long if possible
- if (promoteLongs && promoteValues === true) {
- object[name] =
- long.lessThanOrEqual(JS_INT_MAX_LONG) && long.greaterThanOrEqual(JS_INT_MIN_LONG)
- ? long.toNumber()
- : long;
- } else {
- object[name] = long;
- }
- } else if (elementType === BSON.BSON_DATA_DECIMAL128) {
- // Buffer to contain the decimal bytes
- var bytes = utils.allocBuffer(16);
- // Copy the next 16 bytes into the bytes buffer
- buffer.copy(bytes, 0, index, index + 16);
- // Update index
- index = index + 16;
- // Assign the new Decimal128 value
- var decimal128 = new Decimal128(bytes);
- // If we have an alternative mapper use that
- object[name] = decimal128.toObject ? decimal128.toObject() : decimal128;
- } else if (elementType === BSON.BSON_DATA_BINARY) {
- var binarySize =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- var totalBinarySize = binarySize;
- var subType = buffer[index++];
-
- // Did we have a negative binary size, throw
- if (binarySize < 0) throw new Error('Negative binary type element size found');
-
- // Is the length longer than the document
- if (binarySize > buffer.length) throw new Error('Binary type size larger than document size');
-
- // Decode as raw Buffer object if options specifies it
- if (buffer['slice'] != null) {
- // If we have subtype 2 skip the 4 bytes for the size
- if (subType === Binary.SUBTYPE_BYTE_ARRAY) {
- binarySize =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- if (binarySize < 0)
- throw new Error('Negative binary type element size found for subtype 0x02');
- if (binarySize > totalBinarySize - 4)
- throw new Error('Binary type with subtype 0x02 contains to long binary size');
- if (binarySize < totalBinarySize - 4)
- throw new Error('Binary type with subtype 0x02 contains to short binary size');
- }
-
- if (promoteBuffers && promoteValues) {
- object[name] = buffer.slice(index, index + binarySize);
- } else {
- object[name] = new Binary(buffer.slice(index, index + binarySize), subType);
- }
- } else {
- var _buffer =
- typeof Uint8Array !== 'undefined'
- ? new Uint8Array(new ArrayBuffer(binarySize))
- : new Array(binarySize);
- // If we have subtype 2 skip the 4 bytes for the size
- if (subType === Binary.SUBTYPE_BYTE_ARRAY) {
- binarySize =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- if (binarySize < 0)
- throw new Error('Negative binary type element size found for subtype 0x02');
- if (binarySize > totalBinarySize - 4)
- throw new Error('Binary type with subtype 0x02 contains to long binary size');
- if (binarySize < totalBinarySize - 4)
- throw new Error('Binary type with subtype 0x02 contains to short binary size');
- }
-
- // Copy the data
- for (i = 0; i < binarySize; i++) {
- _buffer[i] = buffer[index + i];
- }
-
- if (promoteBuffers && promoteValues) {
- object[name] = _buffer;
- } else {
- object[name] = new Binary(_buffer, subType);
- }
- }
-
- // Update the index
- index = index + binarySize;
- } else if (elementType === BSON.BSON_DATA_REGEXP && bsonRegExp === false) {
- // Get the start search index
- i = index;
- // Locate the end of the c string
- while (buffer[i] !== 0x00 && i < buffer.length) {
- i++;
- }
- // If are at the end of the buffer there is a problem with the document
- if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
- // Return the C string
- var source = buffer.toString('utf8', index, i);
- // Create the regexp
- index = i + 1;
-
- // Get the start search index
- i = index;
- // Locate the end of the c string
- while (buffer[i] !== 0x00 && i < buffer.length) {
- i++;
- }
- // If are at the end of the buffer there is a problem with the document
- if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
- // Return the C string
- var regExpOptions = buffer.toString('utf8', index, i);
- index = i + 1;
-
- // For each option add the corresponding one for javascript
- var optionsArray = new Array(regExpOptions.length);
-
- // Parse options
- for (i = 0; i < regExpOptions.length; i++) {
- switch (regExpOptions[i]) {
- case 'm':
- optionsArray[i] = 'm';
- break;
- case 's':
- optionsArray[i] = 'g';
- break;
- case 'i':
- optionsArray[i] = 'i';
- break;
- }
- }
-
- object[name] = new RegExp(source, optionsArray.join(''));
- } else if (elementType === BSON.BSON_DATA_REGEXP && bsonRegExp === true) {
- // Get the start search index
- i = index;
- // Locate the end of the c string
- while (buffer[i] !== 0x00 && i < buffer.length) {
- i++;
- }
- // If are at the end of the buffer there is a problem with the document
- if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
- // Return the C string
- source = buffer.toString('utf8', index, i);
- index = i + 1;
-
- // Get the start search index
- i = index;
- // Locate the end of the c string
- while (buffer[i] !== 0x00 && i < buffer.length) {
- i++;
- }
- // If are at the end of the buffer there is a problem with the document
- if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
- // Return the C string
- regExpOptions = buffer.toString('utf8', index, i);
- index = i + 1;
-
- // Set the object
- object[name] = new BSONRegExp(source, regExpOptions);
- } else if (elementType === BSON.BSON_DATA_SYMBOL) {
- stringSize =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- if (
- stringSize <= 0 ||
- stringSize > buffer.length - index ||
- buffer[index + stringSize - 1] !== 0
- )
- throw new Error('bad string length in bson');
- object[name] = new Symbol(buffer.toString('utf8', index, index + stringSize - 1));
- index = index + stringSize;
- } else if (elementType === BSON.BSON_DATA_TIMESTAMP) {
- lowBits =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- highBits =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- object[name] = new Timestamp(lowBits, highBits);
- } else if (elementType === BSON.BSON_DATA_MIN_KEY) {
- object[name] = new MinKey();
- } else if (elementType === BSON.BSON_DATA_MAX_KEY) {
- object[name] = new MaxKey();
- } else if (elementType === BSON.BSON_DATA_CODE) {
- stringSize =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- if (
- stringSize <= 0 ||
- stringSize > buffer.length - index ||
- buffer[index + stringSize - 1] !== 0
- )
- throw new Error('bad string length in bson');
- var functionString = buffer.toString('utf8', index, index + stringSize - 1);
-
- // If we are evaluating the functions
- if (evalFunctions) {
- // If we have cache enabled let's look for the md5 of the function in the cache
- if (cacheFunctions) {
- var hash = cacheFunctionsCrc32 ? crc32(functionString) : functionString;
- // Got to do this to avoid V8 deoptimizing the call due to finding eval
- object[name] = isolateEvalWithHash(functionCache, hash, functionString, object);
- } else {
- object[name] = isolateEval(functionString);
- }
- } else {
- object[name] = new Code(functionString);
- }
-
- // Update parse index position
- index = index + stringSize;
- } else if (elementType === BSON.BSON_DATA_CODE_W_SCOPE) {
- var totalSize =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
-
- // Element cannot be shorter than totalSize + stringSize + documentSize + terminator
- if (totalSize < 4 + 4 + 4 + 1) {
- throw new Error('code_w_scope total size shorter minimum expected length');
- }
-
- // Get the code string size
- stringSize =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- // Check if we have a valid string
- if (
- stringSize <= 0 ||
- stringSize > buffer.length - index ||
- buffer[index + stringSize - 1] !== 0
- )
- throw new Error('bad string length in bson');
-
- // Javascript function
- functionString = buffer.toString('utf8', index, index + stringSize - 1);
- // Update parse index position
- index = index + stringSize;
- // Parse the element
- _index = index;
- // Decode the size of the object document
- objectSize =
- buffer[index] |
- (buffer[index + 1] << 8) |
- (buffer[index + 2] << 16) |
- (buffer[index + 3] << 24);
- // Decode the scope object
- var scopeObject = deserializeObject(buffer, _index, options, false);
- // Adjust the index
- index = index + objectSize;
-
- // Check if field length is to short
- if (totalSize < 4 + 4 + objectSize + stringSize) {
- throw new Error('code_w_scope total size is to short, truncating scope');
- }
-
- // Check if totalSize field is to long
- if (totalSize > 4 + 4 + objectSize + stringSize) {
- throw new Error('code_w_scope total size is to long, clips outer document');
- }
-
- // If we are evaluating the functions
- if (evalFunctions) {
- // If we have cache enabled let's look for the md5 of the function in the cache
- if (cacheFunctions) {
- hash = cacheFunctionsCrc32 ? crc32(functionString) : functionString;
- // Got to do this to avoid V8 deoptimizing the call due to finding eval
- object[name] = isolateEvalWithHash(functionCache, hash, functionString, object);
- } else {
- object[name] = isolateEval(functionString);
- }
-
- object[name].scope = scopeObject;
- } else {
- object[name] = new Code(functionString, scopeObject);
- }
- } else if (elementType === BSON.BSON_DATA_DBPOINTER) {
- // Get the code string size
- stringSize =
- buffer[index++] |
- (buffer[index++] << 8) |
- (buffer[index++] << 16) |
- (buffer[index++] << 24);
- // Check if we have a valid string
- if (
- stringSize <= 0 ||
- stringSize > buffer.length - index ||
- buffer[index + stringSize - 1] !== 0
- )
- throw new Error('bad string length in bson');
- // Namespace
- var namespace = buffer.toString('utf8', index, index + stringSize - 1);
- // Update parse index position
- index = index + stringSize;
-
- // Read the oid
- var oidBuffer = utils.allocBuffer(12);
- buffer.copy(oidBuffer, 0, index, index + 12);
- oid = new ObjectID(oidBuffer);
-
- // Update the index
- index = index + 12;
-
- // Split the namespace
- var parts = namespace.split('.');
- var db = parts.shift();
- var collection = parts.join('.');
- // Upgrade to DBRef type
- object[name] = new DBRef(collection, oid, db);
- } else {
- throw new Error(
- 'Detected unknown BSON type ' +
- elementType.toString(16) +
- ' for fieldname "' +
- name +
- '", are you using the latest BSON parser'
- );
- }
- }
-
- // Check if the deserialization was against a valid array/object
- if (size !== index - startIndex) {
- if (isArray) throw new Error('corrupt array bson');
- throw new Error('corrupt object bson');
- }
-
- // Check if we have a db ref object
- if (object['$id'] != null) object = new DBRef(object['$ref'], object['$id'], object['$db']);
- return object;
-};
-
-/**
- * Ensure eval is isolated.
- *
- * @ignore
- * @api private
- */
-var isolateEvalWithHash = function(functionCache, hash, functionString, object) {
- // Contains the value we are going to set
- var value = null;
-
- // Check for cache hit, eval if missing and return cached function
- if (functionCache[hash] == null) {
- eval('value = ' + functionString);
- functionCache[hash] = value;
- }
- // Set the object
- return functionCache[hash].bind(object);
-};
-
-/**
- * Ensure eval is isolated.
- *
- * @ignore
- * @api private
- */
-var isolateEval = function(functionString) {
- // Contains the value we are going to set
- var value = null;
- // Eval the function
- eval('value = ' + functionString);
- return value;
-};
-
-var BSON = {};
-
-/**
- * Contains the function cache if we have that enable to allow for avoiding the eval step on each deserialization, comparison is by md5
- *
- * @ignore
- * @api private
- */
-var functionCache = (BSON.functionCache = {});
-
-/**
- * Number BSON Type
- *
- * @classconstant BSON_DATA_NUMBER
- **/
-BSON.BSON_DATA_NUMBER = 1;
-/**
- * String BSON Type
- *
- * @classconstant BSON_DATA_STRING
- **/
-BSON.BSON_DATA_STRING = 2;
-/**
- * Object BSON Type
- *
- * @classconstant BSON_DATA_OBJECT
- **/
-BSON.BSON_DATA_OBJECT = 3;
-/**
- * Array BSON Type
- *
- * @classconstant BSON_DATA_ARRAY
- **/
-BSON.BSON_DATA_ARRAY = 4;
-/**
- * Binary BSON Type
- *
- * @classconstant BSON_DATA_BINARY
- **/
-BSON.BSON_DATA_BINARY = 5;
-/**
- * Binary BSON Type
- *
- * @classconstant BSON_DATA_UNDEFINED
- **/
-BSON.BSON_DATA_UNDEFINED = 6;
-/**
- * ObjectID BSON Type
- *
- * @classconstant BSON_DATA_OID
- **/
-BSON.BSON_DATA_OID = 7;
-/**
- * Boolean BSON Type
- *
- * @classconstant BSON_DATA_BOOLEAN
- **/
-BSON.BSON_DATA_BOOLEAN = 8;
-/**
- * Date BSON Type
- *
- * @classconstant BSON_DATA_DATE
- **/
-BSON.BSON_DATA_DATE = 9;
-/**
- * null BSON Type
- *
- * @classconstant BSON_DATA_NULL
- **/
-BSON.BSON_DATA_NULL = 10;
-/**
- * RegExp BSON Type
- *
- * @classconstant BSON_DATA_REGEXP
- **/
-BSON.BSON_DATA_REGEXP = 11;
-/**
- * Code BSON Type
- *
- * @classconstant BSON_DATA_DBPOINTER
- **/
-BSON.BSON_DATA_DBPOINTER = 12;
-/**
- * Code BSON Type
- *
- * @classconstant BSON_DATA_CODE
- **/
-BSON.BSON_DATA_CODE = 13;
-/**
- * Symbol BSON Type
- *
- * @classconstant BSON_DATA_SYMBOL
- **/
-BSON.BSON_DATA_SYMBOL = 14;
-/**
- * Code with Scope BSON Type
- *
- * @classconstant BSON_DATA_CODE_W_SCOPE
- **/
-BSON.BSON_DATA_CODE_W_SCOPE = 15;
-/**
- * 32 bit Integer BSON Type
- *
- * @classconstant BSON_DATA_INT
- **/
-BSON.BSON_DATA_INT = 16;
-/**
- * Timestamp BSON Type
- *
- * @classconstant BSON_DATA_TIMESTAMP
- **/
-BSON.BSON_DATA_TIMESTAMP = 17;
-/**
- * Long BSON Type
- *
- * @classconstant BSON_DATA_LONG
- **/
-BSON.BSON_DATA_LONG = 18;
-/**
- * Long BSON Type
- *
- * @classconstant BSON_DATA_DECIMAL128
- **/
-BSON.BSON_DATA_DECIMAL128 = 19;
-/**
- * MinKey BSON Type
- *
- * @classconstant BSON_DATA_MIN_KEY
- **/
-BSON.BSON_DATA_MIN_KEY = 0xff;
-/**
- * MaxKey BSON Type
- *
- * @classconstant BSON_DATA_MAX_KEY
- **/
-BSON.BSON_DATA_MAX_KEY = 0x7f;
-
-/**
- * Binary Default Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_DEFAULT
- **/
-BSON.BSON_BINARY_SUBTYPE_DEFAULT = 0;
-/**
- * Binary Function Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_FUNCTION
- **/
-BSON.BSON_BINARY_SUBTYPE_FUNCTION = 1;
-/**
- * Binary Byte Array Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_BYTE_ARRAY
- **/
-BSON.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
-/**
- * Binary UUID Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_UUID
- **/
-BSON.BSON_BINARY_SUBTYPE_UUID = 3;
-/**
- * Binary MD5 Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_MD5
- **/
-BSON.BSON_BINARY_SUBTYPE_MD5 = 4;
-/**
- * Binary User Defined Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_USER_DEFINED
- **/
-BSON.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
-
-// BSON MAX VALUES
-BSON.BSON_INT32_MAX = 0x7fffffff;
-BSON.BSON_INT32_MIN = -0x80000000;
-
-BSON.BSON_INT64_MAX = Math.pow(2, 63) - 1;
-BSON.BSON_INT64_MIN = -Math.pow(2, 63);
-
-// JS MAX PRECISE VALUES
-BSON.JS_INT_MAX = 0x20000000000000; // Any integer up to 2^53 can be precisely represented by a double.
-BSON.JS_INT_MIN = -0x20000000000000; // Any integer down to -2^53 can be precisely represented by a double.
-
-// Internal long versions
-var JS_INT_MAX_LONG = Long.fromNumber(0x20000000000000); // Any integer up to 2^53 can be precisely represented by a double.
-var JS_INT_MIN_LONG = Long.fromNumber(-0x20000000000000); // Any integer down to -2^53 can be precisely represented by a double.
-
-module.exports = deserialize;
diff --git a/node_modules/bson/lib/bson/parser/serializer.js b/node_modules/bson/lib/bson/parser/serializer.js
deleted file mode 100644
index 6bb212e..0000000
--- a/node_modules/bson/lib/bson/parser/serializer.js
+++ /dev/null
@@ -1,1188 +0,0 @@
-'use strict';
-
-var writeIEEE754 = require('../float_parser').writeIEEE754,
- Long = require('../long').Long,
- Map = require('../map'),
- Binary = require('../binary').Binary;
-
-var normalizedFunctionString = require('./utils').normalizedFunctionString;
-
-// try {
-// var _Buffer = Uint8Array;
-// } catch (e) {
-// _Buffer = Buffer;
-// }
-
-var regexp = /\x00/; // eslint-disable-line no-control-regex
-var ignoreKeys = ['$db', '$ref', '$id', '$clusterTime'];
-
-// To ensure that 0.4 of node works correctly
-var isDate = function isDate(d) {
- return typeof d === 'object' && Object.prototype.toString.call(d) === '[object Date]';
-};
-
-var isRegExp = function isRegExp(d) {
- return Object.prototype.toString.call(d) === '[object RegExp]';
-};
-
-var serializeString = function(buffer, key, value, index, isArray) {
- // Encode String type
- buffer[index++] = BSON.BSON_DATA_STRING;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes + 1;
- buffer[index - 1] = 0;
- // Write the string
- var size = buffer.write(value, index + 4, 'utf8');
- // Write the size of the string to buffer
- buffer[index + 3] = ((size + 1) >> 24) & 0xff;
- buffer[index + 2] = ((size + 1) >> 16) & 0xff;
- buffer[index + 1] = ((size + 1) >> 8) & 0xff;
- buffer[index] = (size + 1) & 0xff;
- // Update index
- index = index + 4 + size;
- // Write zero
- buffer[index++] = 0;
- return index;
-};
-
-var serializeNumber = function(buffer, key, value, index, isArray) {
- // We have an integer value
- if (Math.floor(value) === value && value >= BSON.JS_INT_MIN && value <= BSON.JS_INT_MAX) {
- // If the value fits in 32 bits encode as int, if it fits in a double
- // encode it as a double, otherwise long
- if (value >= BSON.BSON_INT32_MIN && value <= BSON.BSON_INT32_MAX) {
- // Set int type 32 bits or less
- buffer[index++] = BSON.BSON_DATA_INT;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write the int value
- buffer[index++] = value & 0xff;
- buffer[index++] = (value >> 8) & 0xff;
- buffer[index++] = (value >> 16) & 0xff;
- buffer[index++] = (value >> 24) & 0xff;
- } else if (value >= BSON.JS_INT_MIN && value <= BSON.JS_INT_MAX) {
- // Encode as double
- buffer[index++] = BSON.BSON_DATA_NUMBER;
- // Number of written bytes
- numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write float
- writeIEEE754(buffer, value, index, 'little', 52, 8);
- // Ajust index
- index = index + 8;
- } else {
- // Set long type
- buffer[index++] = BSON.BSON_DATA_LONG;
- // Number of written bytes
- numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- var longVal = Long.fromNumber(value);
- var lowBits = longVal.getLowBits();
- var highBits = longVal.getHighBits();
- // Encode low bits
- buffer[index++] = lowBits & 0xff;
- buffer[index++] = (lowBits >> 8) & 0xff;
- buffer[index++] = (lowBits >> 16) & 0xff;
- buffer[index++] = (lowBits >> 24) & 0xff;
- // Encode high bits
- buffer[index++] = highBits & 0xff;
- buffer[index++] = (highBits >> 8) & 0xff;
- buffer[index++] = (highBits >> 16) & 0xff;
- buffer[index++] = (highBits >> 24) & 0xff;
- }
- } else {
- // Encode as double
- buffer[index++] = BSON.BSON_DATA_NUMBER;
- // Number of written bytes
- numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write float
- writeIEEE754(buffer, value, index, 'little', 52, 8);
- // Ajust index
- index = index + 8;
- }
-
- return index;
-};
-
-var serializeNull = function(buffer, key, value, index, isArray) {
- // Set long type
- buffer[index++] = BSON.BSON_DATA_NULL;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- return index;
-};
-
-var serializeBoolean = function(buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_BOOLEAN;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Encode the boolean value
- buffer[index++] = value ? 1 : 0;
- return index;
-};
-
-var serializeDate = function(buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_DATE;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
-
- // Write the date
- var dateInMilis = Long.fromNumber(value.getTime());
- var lowBits = dateInMilis.getLowBits();
- var highBits = dateInMilis.getHighBits();
- // Encode low bits
- buffer[index++] = lowBits & 0xff;
- buffer[index++] = (lowBits >> 8) & 0xff;
- buffer[index++] = (lowBits >> 16) & 0xff;
- buffer[index++] = (lowBits >> 24) & 0xff;
- // Encode high bits
- buffer[index++] = highBits & 0xff;
- buffer[index++] = (highBits >> 8) & 0xff;
- buffer[index++] = (highBits >> 16) & 0xff;
- buffer[index++] = (highBits >> 24) & 0xff;
- return index;
-};
-
-var serializeRegExp = function(buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_REGEXP;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- if (value.source && value.source.match(regexp) != null) {
- throw Error('value ' + value.source + ' must not contain null bytes');
- }
- // Adjust the index
- index = index + buffer.write(value.source, index, 'utf8');
- // Write zero
- buffer[index++] = 0x00;
- // Write the parameters
- if (value.global) buffer[index++] = 0x73; // s
- if (value.ignoreCase) buffer[index++] = 0x69; // i
- if (value.multiline) buffer[index++] = 0x6d; // m
- // Add ending zero
- buffer[index++] = 0x00;
- return index;
-};
-
-var serializeBSONRegExp = function(buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_REGEXP;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
-
- // Check the pattern for 0 bytes
- if (value.pattern.match(regexp) != null) {
- // The BSON spec doesn't allow keys with null bytes because keys are
- // null-terminated.
- throw Error('pattern ' + value.pattern + ' must not contain null bytes');
- }
-
- // Adjust the index
- index = index + buffer.write(value.pattern, index, 'utf8');
- // Write zero
- buffer[index++] = 0x00;
- // Write the options
- index =
- index +
- buffer.write(
- value.options
- .split('')
- .sort()
- .join(''),
- index,
- 'utf8'
- );
- // Add ending zero
- buffer[index++] = 0x00;
- return index;
-};
-
-var serializeMinMax = function(buffer, key, value, index, isArray) {
- // Write the type of either min or max key
- if (value === null) {
- buffer[index++] = BSON.BSON_DATA_NULL;
- } else if (value._bsontype === 'MinKey') {
- buffer[index++] = BSON.BSON_DATA_MIN_KEY;
- } else {
- buffer[index++] = BSON.BSON_DATA_MAX_KEY;
- }
-
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- return index;
-};
-
-var serializeObjectId = function(buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_OID;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
-
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
-
- // Write the objectId into the shared buffer
- if (typeof value.id === 'string') {
- buffer.write(value.id, index, 'binary');
- } else if (value.id && value.id.copy) {
- value.id.copy(buffer, index, 0, 12);
- } else {
- throw new Error('object [' + JSON.stringify(value) + '] is not a valid ObjectId');
- }
-
- // Ajust index
- return index + 12;
-};
-
-var serializeBuffer = function(buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_BINARY;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Get size of the buffer (current write point)
- var size = value.length;
- // Write the size of the string to buffer
- buffer[index++] = size & 0xff;
- buffer[index++] = (size >> 8) & 0xff;
- buffer[index++] = (size >> 16) & 0xff;
- buffer[index++] = (size >> 24) & 0xff;
- // Write the default subtype
- buffer[index++] = BSON.BSON_BINARY_SUBTYPE_DEFAULT;
- // Copy the content form the binary field to the buffer
- value.copy(buffer, index, 0, size);
- // Adjust the index
- index = index + size;
- return index;
-};
-
-var serializeObject = function(
- buffer,
- key,
- value,
- index,
- checkKeys,
- depth,
- serializeFunctions,
- ignoreUndefined,
- isArray,
- path
-) {
- for (var i = 0; i < path.length; i++) {
- if (path[i] === value) throw new Error('cyclic dependency detected');
- }
-
- // Push value to stack
- path.push(value);
- // Write the type
- buffer[index++] = Array.isArray(value) ? BSON.BSON_DATA_ARRAY : BSON.BSON_DATA_OBJECT;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- var endIndex = serializeInto(
- buffer,
- value,
- checkKeys,
- index,
- depth + 1,
- serializeFunctions,
- ignoreUndefined,
- path
- );
- // Pop stack
- path.pop();
- // Write size
- return endIndex;
-};
-
-var serializeDecimal128 = function(buffer, key, value, index, isArray) {
- buffer[index++] = BSON.BSON_DATA_DECIMAL128;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write the data from the value
- value.bytes.copy(buffer, index, 0, 16);
- return index + 16;
-};
-
-var serializeLong = function(buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = value._bsontype === 'Long' ? BSON.BSON_DATA_LONG : BSON.BSON_DATA_TIMESTAMP;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write the date
- var lowBits = value.getLowBits();
- var highBits = value.getHighBits();
- // Encode low bits
- buffer[index++] = lowBits & 0xff;
- buffer[index++] = (lowBits >> 8) & 0xff;
- buffer[index++] = (lowBits >> 16) & 0xff;
- buffer[index++] = (lowBits >> 24) & 0xff;
- // Encode high bits
- buffer[index++] = highBits & 0xff;
- buffer[index++] = (highBits >> 8) & 0xff;
- buffer[index++] = (highBits >> 16) & 0xff;
- buffer[index++] = (highBits >> 24) & 0xff;
- return index;
-};
-
-var serializeInt32 = function(buffer, key, value, index, isArray) {
- // Set int type 32 bits or less
- buffer[index++] = BSON.BSON_DATA_INT;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write the int value
- buffer[index++] = value & 0xff;
- buffer[index++] = (value >> 8) & 0xff;
- buffer[index++] = (value >> 16) & 0xff;
- buffer[index++] = (value >> 24) & 0xff;
- return index;
-};
-
-var serializeDouble = function(buffer, key, value, index, isArray) {
- // Encode as double
- buffer[index++] = BSON.BSON_DATA_NUMBER;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write float
- writeIEEE754(buffer, value, index, 'little', 52, 8);
- // Ajust index
- index = index + 8;
- return index;
-};
-
-var serializeFunction = function(buffer, key, value, index, checkKeys, depth, isArray) {
- buffer[index++] = BSON.BSON_DATA_CODE;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Function string
- var functionString = normalizedFunctionString(value);
-
- // Write the string
- var size = buffer.write(functionString, index + 4, 'utf8') + 1;
- // Write the size of the string to buffer
- buffer[index] = size & 0xff;
- buffer[index + 1] = (size >> 8) & 0xff;
- buffer[index + 2] = (size >> 16) & 0xff;
- buffer[index + 3] = (size >> 24) & 0xff;
- // Update index
- index = index + 4 + size - 1;
- // Write zero
- buffer[index++] = 0;
- return index;
-};
-
-var serializeCode = function(
- buffer,
- key,
- value,
- index,
- checkKeys,
- depth,
- serializeFunctions,
- ignoreUndefined,
- isArray
-) {
- if (value.scope && typeof value.scope === 'object') {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_CODE_W_SCOPE;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
-
- // Starting index
- var startIndex = index;
-
- // Serialize the function
- // Get the function string
- var functionString = typeof value.code === 'string' ? value.code : value.code.toString();
- // Index adjustment
- index = index + 4;
- // Write string into buffer
- var codeSize = buffer.write(functionString, index + 4, 'utf8') + 1;
- // Write the size of the string to buffer
- buffer[index] = codeSize & 0xff;
- buffer[index + 1] = (codeSize >> 8) & 0xff;
- buffer[index + 2] = (codeSize >> 16) & 0xff;
- buffer[index + 3] = (codeSize >> 24) & 0xff;
- // Write end 0
- buffer[index + 4 + codeSize - 1] = 0;
- // Write the
- index = index + codeSize + 4;
-
- //
- // Serialize the scope value
- var endIndex = serializeInto(
- buffer,
- value.scope,
- checkKeys,
- index,
- depth + 1,
- serializeFunctions,
- ignoreUndefined
- );
- index = endIndex - 1;
-
- // Writ the total
- var totalSize = endIndex - startIndex;
-
- // Write the total size of the object
- buffer[startIndex++] = totalSize & 0xff;
- buffer[startIndex++] = (totalSize >> 8) & 0xff;
- buffer[startIndex++] = (totalSize >> 16) & 0xff;
- buffer[startIndex++] = (totalSize >> 24) & 0xff;
- // Write trailing zero
- buffer[index++] = 0;
- } else {
- buffer[index++] = BSON.BSON_DATA_CODE;
- // Number of written bytes
- numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Function string
- functionString = value.code.toString();
- // Write the string
- var size = buffer.write(functionString, index + 4, 'utf8') + 1;
- // Write the size of the string to buffer
- buffer[index] = size & 0xff;
- buffer[index + 1] = (size >> 8) & 0xff;
- buffer[index + 2] = (size >> 16) & 0xff;
- buffer[index + 3] = (size >> 24) & 0xff;
- // Update index
- index = index + 4 + size - 1;
- // Write zero
- buffer[index++] = 0;
- }
-
- return index;
-};
-
-var serializeBinary = function(buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_BINARY;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Extract the buffer
- var data = value.value(true);
- // Calculate size
- var size = value.position;
- // Add the deprecated 02 type 4 bytes of size to total
- if (value.sub_type === Binary.SUBTYPE_BYTE_ARRAY) size = size + 4;
- // Write the size of the string to buffer
- buffer[index++] = size & 0xff;
- buffer[index++] = (size >> 8) & 0xff;
- buffer[index++] = (size >> 16) & 0xff;
- buffer[index++] = (size >> 24) & 0xff;
- // Write the subtype to the buffer
- buffer[index++] = value.sub_type;
-
- // If we have binary type 2 the 4 first bytes are the size
- if (value.sub_type === Binary.SUBTYPE_BYTE_ARRAY) {
- size = size - 4;
- buffer[index++] = size & 0xff;
- buffer[index++] = (size >> 8) & 0xff;
- buffer[index++] = (size >> 16) & 0xff;
- buffer[index++] = (size >> 24) & 0xff;
- }
-
- // Write the data to the object
- data.copy(buffer, index, 0, value.position);
- // Adjust the index
- index = index + value.position;
- return index;
-};
-
-var serializeSymbol = function(buffer, key, value, index, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_SYMBOL;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
- // Write the string
- var size = buffer.write(value.value, index + 4, 'utf8') + 1;
- // Write the size of the string to buffer
- buffer[index] = size & 0xff;
- buffer[index + 1] = (size >> 8) & 0xff;
- buffer[index + 2] = (size >> 16) & 0xff;
- buffer[index + 3] = (size >> 24) & 0xff;
- // Update index
- index = index + 4 + size - 1;
- // Write zero
- buffer[index++] = 0x00;
- return index;
-};
-
-var serializeDBRef = function(buffer, key, value, index, depth, serializeFunctions, isArray) {
- // Write the type
- buffer[index++] = BSON.BSON_DATA_OBJECT;
- // Number of written bytes
- var numberOfWrittenBytes = !isArray
- ? buffer.write(key, index, 'utf8')
- : buffer.write(key, index, 'ascii');
-
- // Encode the name
- index = index + numberOfWrittenBytes;
- buffer[index++] = 0;
-
- var startIndex = index;
- var endIndex;
-
- // Serialize object
- if (null != value.db) {
- endIndex = serializeInto(
- buffer,
- {
- $ref: value.namespace,
- $id: value.oid,
- $db: value.db
- },
- false,
- index,
- depth + 1,
- serializeFunctions
- );
- } else {
- endIndex = serializeInto(
- buffer,
- {
- $ref: value.namespace,
- $id: value.oid
- },
- false,
- index,
- depth + 1,
- serializeFunctions
- );
- }
-
- // Calculate object size
- var size = endIndex - startIndex;
- // Write the size
- buffer[startIndex++] = size & 0xff;
- buffer[startIndex++] = (size >> 8) & 0xff;
- buffer[startIndex++] = (size >> 16) & 0xff;
- buffer[startIndex++] = (size >> 24) & 0xff;
- // Set index
- return endIndex;
-};
-
-var serializeInto = function serializeInto(
- buffer,
- object,
- checkKeys,
- startingIndex,
- depth,
- serializeFunctions,
- ignoreUndefined,
- path
-) {
- startingIndex = startingIndex || 0;
- path = path || [];
-
- // Push the object to the path
- path.push(object);
-
- // Start place to serialize into
- var index = startingIndex + 4;
- // var self = this;
-
- // Special case isArray
- if (Array.isArray(object)) {
- // Get object keys
- for (var i = 0; i < object.length; i++) {
- var key = '' + i;
- var value = object[i];
-
- // Is there an override value
- if (value && value.toBSON) {
- if (typeof value.toBSON !== 'function') throw new Error('toBSON is not a function');
- value = value.toBSON();
- }
-
- var type = typeof value;
- if (type === 'string') {
- index = serializeString(buffer, key, value, index, true);
- } else if (type === 'number') {
- index = serializeNumber(buffer, key, value, index, true);
- } else if (type === 'boolean') {
- index = serializeBoolean(buffer, key, value, index, true);
- } else if (value instanceof Date || isDate(value)) {
- index = serializeDate(buffer, key, value, index, true);
- } else if (value === undefined) {
- index = serializeNull(buffer, key, value, index, true);
- } else if (value === null) {
- index = serializeNull(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'ObjectID' || value['_bsontype'] === 'ObjectId') {
- index = serializeObjectId(buffer, key, value, index, true);
- } else if (Buffer.isBuffer(value)) {
- index = serializeBuffer(buffer, key, value, index, true);
- } else if (value instanceof RegExp || isRegExp(value)) {
- index = serializeRegExp(buffer, key, value, index, true);
- } else if (type === 'object' && value['_bsontype'] == null) {
- index = serializeObject(
- buffer,
- key,
- value,
- index,
- checkKeys,
- depth,
- serializeFunctions,
- ignoreUndefined,
- true,
- path
- );
- } else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
- index = serializeDecimal128(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
- index = serializeLong(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'Double') {
- index = serializeDouble(buffer, key, value, index, true);
- } else if (typeof value === 'function' && serializeFunctions) {
- index = serializeFunction(
- buffer,
- key,
- value,
- index,
- checkKeys,
- depth,
- serializeFunctions,
- true
- );
- } else if (value['_bsontype'] === 'Code') {
- index = serializeCode(
- buffer,
- key,
- value,
- index,
- checkKeys,
- depth,
- serializeFunctions,
- ignoreUndefined,
- true
- );
- } else if (value['_bsontype'] === 'Binary') {
- index = serializeBinary(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'Symbol') {
- index = serializeSymbol(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'DBRef') {
- index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions, true);
- } else if (value['_bsontype'] === 'BSONRegExp') {
- index = serializeBSONRegExp(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'Int32') {
- index = serializeInt32(buffer, key, value, index, true);
- } else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
- index = serializeMinMax(buffer, key, value, index, true);
- } else if (typeof value['_bsontype'] !== 'undefined') {
- throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
- }
- }
- } else if (object instanceof Map) {
- var iterator = object.entries();
- var done = false;
-
- while (!done) {
- // Unpack the next entry
- var entry = iterator.next();
- done = entry.done;
- // Are we done, then skip and terminate
- if (done) continue;
-
- // Get the entry values
- key = entry.value[0];
- value = entry.value[1];
-
- // Check the type of the value
- type = typeof value;
-
- // Check the key and throw error if it's illegal
- if (typeof key === 'string' && ignoreKeys.indexOf(key) === -1) {
- if (key.match(regexp) != null) {
- // The BSON spec doesn't allow keys with null bytes because keys are
- // null-terminated.
- throw Error('key ' + key + ' must not contain null bytes');
- }
-
- if (checkKeys) {
- if ('$' === key[0]) {
- throw Error('key ' + key + " must not start with '$'");
- } else if (~key.indexOf('.')) {
- throw Error('key ' + key + " must not contain '.'");
- }
- }
- }
-
- if (type === 'string') {
- index = serializeString(buffer, key, value, index);
- } else if (type === 'number') {
- index = serializeNumber(buffer, key, value, index);
- } else if (type === 'boolean') {
- index = serializeBoolean(buffer, key, value, index);
- } else if (value instanceof Date || isDate(value)) {
- index = serializeDate(buffer, key, value, index);
- // } else if (value === undefined && ignoreUndefined === true) {
- } else if (value === null || (value === undefined && ignoreUndefined === false)) {
- index = serializeNull(buffer, key, value, index);
- } else if (value['_bsontype'] === 'ObjectID' || value['_bsontype'] === 'ObjectId') {
- index = serializeObjectId(buffer, key, value, index);
- } else if (Buffer.isBuffer(value)) {
- index = serializeBuffer(buffer, key, value, index);
- } else if (value instanceof RegExp || isRegExp(value)) {
- index = serializeRegExp(buffer, key, value, index);
- } else if (type === 'object' && value['_bsontype'] == null) {
- index = serializeObject(
- buffer,
- key,
- value,
- index,
- checkKeys,
- depth,
- serializeFunctions,
- ignoreUndefined,
- false,
- path
- );
- } else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
- index = serializeDecimal128(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
- index = serializeLong(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Double') {
- index = serializeDouble(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Code') {
- index = serializeCode(
- buffer,
- key,
- value,
- index,
- checkKeys,
- depth,
- serializeFunctions,
- ignoreUndefined
- );
- } else if (typeof value === 'function' && serializeFunctions) {
- index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
- } else if (value['_bsontype'] === 'Binary') {
- index = serializeBinary(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Symbol') {
- index = serializeSymbol(buffer, key, value, index);
- } else if (value['_bsontype'] === 'DBRef') {
- index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
- } else if (value['_bsontype'] === 'BSONRegExp') {
- index = serializeBSONRegExp(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Int32') {
- index = serializeInt32(buffer, key, value, index);
- } else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
- index = serializeMinMax(buffer, key, value, index);
- } else if (typeof value['_bsontype'] !== 'undefined') {
- throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
- }
- }
- } else {
- // Did we provide a custom serialization method
- if (object.toBSON) {
- if (typeof object.toBSON !== 'function') throw new Error('toBSON is not a function');
- object = object.toBSON();
- if (object != null && typeof object !== 'object')
- throw new Error('toBSON function did not return an object');
- }
-
- // Iterate over all the keys
- for (key in object) {
- value = object[key];
- // Is there an override value
- if (value && value.toBSON) {
- if (typeof value.toBSON !== 'function') throw new Error('toBSON is not a function');
- value = value.toBSON();
- }
-
- // Check the type of the value
- type = typeof value;
-
- // Check the key and throw error if it's illegal
- if (typeof key === 'string' && ignoreKeys.indexOf(key) === -1) {
- if (key.match(regexp) != null) {
- // The BSON spec doesn't allow keys with null bytes because keys are
- // null-terminated.
- throw Error('key ' + key + ' must not contain null bytes');
- }
-
- if (checkKeys) {
- if ('$' === key[0]) {
- throw Error('key ' + key + " must not start with '$'");
- } else if (~key.indexOf('.')) {
- throw Error('key ' + key + " must not contain '.'");
- }
- }
- }
-
- if (type === 'string') {
- index = serializeString(buffer, key, value, index);
- } else if (type === 'number') {
- index = serializeNumber(buffer, key, value, index);
- } else if (type === 'boolean') {
- index = serializeBoolean(buffer, key, value, index);
- } else if (value instanceof Date || isDate(value)) {
- index = serializeDate(buffer, key, value, index);
- } else if (value === undefined) {
- if (ignoreUndefined === false) index = serializeNull(buffer, key, value, index);
- } else if (value === null) {
- index = serializeNull(buffer, key, value, index);
- } else if (value['_bsontype'] === 'ObjectID' || value['_bsontype'] === 'ObjectId') {
- index = serializeObjectId(buffer, key, value, index);
- } else if (Buffer.isBuffer(value)) {
- index = serializeBuffer(buffer, key, value, index);
- } else if (value instanceof RegExp || isRegExp(value)) {
- index = serializeRegExp(buffer, key, value, index);
- } else if (type === 'object' && value['_bsontype'] == null) {
- index = serializeObject(
- buffer,
- key,
- value,
- index,
- checkKeys,
- depth,
- serializeFunctions,
- ignoreUndefined,
- false,
- path
- );
- } else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
- index = serializeDecimal128(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
- index = serializeLong(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Double') {
- index = serializeDouble(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Code') {
- index = serializeCode(
- buffer,
- key,
- value,
- index,
- checkKeys,
- depth,
- serializeFunctions,
- ignoreUndefined
- );
- } else if (typeof value === 'function' && serializeFunctions) {
- index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
- } else if (value['_bsontype'] === 'Binary') {
- index = serializeBinary(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Symbol') {
- index = serializeSymbol(buffer, key, value, index);
- } else if (value['_bsontype'] === 'DBRef') {
- index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
- } else if (value['_bsontype'] === 'BSONRegExp') {
- index = serializeBSONRegExp(buffer, key, value, index);
- } else if (value['_bsontype'] === 'Int32') {
- index = serializeInt32(buffer, key, value, index);
- } else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
- index = serializeMinMax(buffer, key, value, index);
- } else if (typeof value['_bsontype'] !== 'undefined') {
- throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
- }
- }
- }
-
- // Remove the path
- path.pop();
-
- // Final padding byte for object
- buffer[index++] = 0x00;
-
- // Final size
- var size = index - startingIndex;
- // Write the size of the object
- buffer[startingIndex++] = size & 0xff;
- buffer[startingIndex++] = (size >> 8) & 0xff;
- buffer[startingIndex++] = (size >> 16) & 0xff;
- buffer[startingIndex++] = (size >> 24) & 0xff;
- return index;
-};
-
-var BSON = {};
-
-/**
- * Contains the function cache if we have that enable to allow for avoiding the eval step on each deserialization, comparison is by md5
- *
- * @ignore
- * @api private
- */
-// var functionCache = (BSON.functionCache = {});
-
-/**
- * Number BSON Type
- *
- * @classconstant BSON_DATA_NUMBER
- **/
-BSON.BSON_DATA_NUMBER = 1;
-/**
- * String BSON Type
- *
- * @classconstant BSON_DATA_STRING
- **/
-BSON.BSON_DATA_STRING = 2;
-/**
- * Object BSON Type
- *
- * @classconstant BSON_DATA_OBJECT
- **/
-BSON.BSON_DATA_OBJECT = 3;
-/**
- * Array BSON Type
- *
- * @classconstant BSON_DATA_ARRAY
- **/
-BSON.BSON_DATA_ARRAY = 4;
-/**
- * Binary BSON Type
- *
- * @classconstant BSON_DATA_BINARY
- **/
-BSON.BSON_DATA_BINARY = 5;
-/**
- * ObjectID BSON Type, deprecated
- *
- * @classconstant BSON_DATA_UNDEFINED
- **/
-BSON.BSON_DATA_UNDEFINED = 6;
-/**
- * ObjectID BSON Type
- *
- * @classconstant BSON_DATA_OID
- **/
-BSON.BSON_DATA_OID = 7;
-/**
- * Boolean BSON Type
- *
- * @classconstant BSON_DATA_BOOLEAN
- **/
-BSON.BSON_DATA_BOOLEAN = 8;
-/**
- * Date BSON Type
- *
- * @classconstant BSON_DATA_DATE
- **/
-BSON.BSON_DATA_DATE = 9;
-/**
- * null BSON Type
- *
- * @classconstant BSON_DATA_NULL
- **/
-BSON.BSON_DATA_NULL = 10;
-/**
- * RegExp BSON Type
- *
- * @classconstant BSON_DATA_REGEXP
- **/
-BSON.BSON_DATA_REGEXP = 11;
-/**
- * Code BSON Type
- *
- * @classconstant BSON_DATA_CODE
- **/
-BSON.BSON_DATA_CODE = 13;
-/**
- * Symbol BSON Type
- *
- * @classconstant BSON_DATA_SYMBOL
- **/
-BSON.BSON_DATA_SYMBOL = 14;
-/**
- * Code with Scope BSON Type
- *
- * @classconstant BSON_DATA_CODE_W_SCOPE
- **/
-BSON.BSON_DATA_CODE_W_SCOPE = 15;
-/**
- * 32 bit Integer BSON Type
- *
- * @classconstant BSON_DATA_INT
- **/
-BSON.BSON_DATA_INT = 16;
-/**
- * Timestamp BSON Type
- *
- * @classconstant BSON_DATA_TIMESTAMP
- **/
-BSON.BSON_DATA_TIMESTAMP = 17;
-/**
- * Long BSON Type
- *
- * @classconstant BSON_DATA_LONG
- **/
-BSON.BSON_DATA_LONG = 18;
-/**
- * Long BSON Type
- *
- * @classconstant BSON_DATA_DECIMAL128
- **/
-BSON.BSON_DATA_DECIMAL128 = 19;
-/**
- * MinKey BSON Type
- *
- * @classconstant BSON_DATA_MIN_KEY
- **/
-BSON.BSON_DATA_MIN_KEY = 0xff;
-/**
- * MaxKey BSON Type
- *
- * @classconstant BSON_DATA_MAX_KEY
- **/
-BSON.BSON_DATA_MAX_KEY = 0x7f;
-/**
- * Binary Default Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_DEFAULT
- **/
-BSON.BSON_BINARY_SUBTYPE_DEFAULT = 0;
-/**
- * Binary Function Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_FUNCTION
- **/
-BSON.BSON_BINARY_SUBTYPE_FUNCTION = 1;
-/**
- * Binary Byte Array Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_BYTE_ARRAY
- **/
-BSON.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
-/**
- * Binary UUID Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_UUID
- **/
-BSON.BSON_BINARY_SUBTYPE_UUID = 3;
-/**
- * Binary MD5 Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_MD5
- **/
-BSON.BSON_BINARY_SUBTYPE_MD5 = 4;
-/**
- * Binary User Defined Type
- *
- * @classconstant BSON_BINARY_SUBTYPE_USER_DEFINED
- **/
-BSON.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
-
-// BSON MAX VALUES
-BSON.BSON_INT32_MAX = 0x7fffffff;
-BSON.BSON_INT32_MIN = -0x80000000;
-
-BSON.BSON_INT64_MAX = Math.pow(2, 63) - 1;
-BSON.BSON_INT64_MIN = -Math.pow(2, 63);
-
-// JS MAX PRECISE VALUES
-BSON.JS_INT_MAX = 0x20000000000000; // Any integer up to 2^53 can be precisely represented by a double.
-BSON.JS_INT_MIN = -0x20000000000000; // Any integer down to -2^53 can be precisely represented by a double.
-
-// Internal long versions
-// var JS_INT_MAX_LONG = Long.fromNumber(0x20000000000000); // Any integer up to 2^53 can be precisely represented by a double.
-// var JS_INT_MIN_LONG = Long.fromNumber(-0x20000000000000); // Any integer down to -2^53 can be precisely represented by a double.
-
-module.exports = serializeInto;
diff --git a/node_modules/bson/lib/bson/parser/utils.js b/node_modules/bson/lib/bson/parser/utils.js
deleted file mode 100644
index 6faa439..0000000
--- a/node_modules/bson/lib/bson/parser/utils.js
+++ /dev/null
@@ -1,28 +0,0 @@
-'use strict';
-
-/**
- * Normalizes our expected stringified form of a function across versions of node
- * @param {Function} fn The function to stringify
- */
-function normalizedFunctionString(fn) {
- return fn.toString().replace(/function *\(/, 'function (');
-}
-
-function newBuffer(item, encoding) {
- return new Buffer(item, encoding);
-}
-
-function allocBuffer() {
- return Buffer.alloc.apply(Buffer, arguments);
-}
-
-function toBuffer() {
- return Buffer.from.apply(Buffer, arguments);
-}
-
-module.exports = {
- normalizedFunctionString: normalizedFunctionString,
- allocBuffer: typeof Buffer.alloc === 'function' ? allocBuffer : newBuffer,
- toBuffer: typeof Buffer.from === 'function' ? toBuffer : newBuffer
-};
-
diff --git a/node_modules/bson/lib/bson/regexp.js b/node_modules/bson/lib/bson/regexp.js
deleted file mode 100644
index 108f016..0000000
--- a/node_modules/bson/lib/bson/regexp.js
+++ /dev/null
@@ -1,33 +0,0 @@
-/**
- * A class representation of the BSON RegExp type.
- *
- * @class
- * @return {BSONRegExp} A MinKey instance
- */
-function BSONRegExp(pattern, options) {
- if (!(this instanceof BSONRegExp)) return new BSONRegExp();
-
- // Execute
- this._bsontype = 'BSONRegExp';
- this.pattern = pattern || '';
- this.options = options || '';
-
- // Validate options
- for (var i = 0; i < this.options.length; i++) {
- if (
- !(
- this.options[i] === 'i' ||
- this.options[i] === 'm' ||
- this.options[i] === 'x' ||
- this.options[i] === 'l' ||
- this.options[i] === 's' ||
- this.options[i] === 'u'
- )
- ) {
- throw new Error('the regular expression options [' + this.options[i] + '] is not supported');
- }
- }
-}
-
-module.exports = BSONRegExp;
-module.exports.BSONRegExp = BSONRegExp;
diff --git a/node_modules/bson/lib/bson/symbol.js b/node_modules/bson/lib/bson/symbol.js
deleted file mode 100644
index ba20cab..0000000
--- a/node_modules/bson/lib/bson/symbol.js
+++ /dev/null
@@ -1,50 +0,0 @@
-// Custom inspect property name / symbol.
-var inspect = Buffer ? require('util').inspect.custom || 'inspect' : 'inspect';
-
-/**
- * A class representation of the BSON Symbol type.
- *
- * @class
- * @deprecated
- * @param {string} value the string representing the symbol.
- * @return {Symbol}
- */
-function Symbol(value) {
- if (!(this instanceof Symbol)) return new Symbol(value);
- this._bsontype = 'Symbol';
- this.value = value;
-}
-
-/**
- * Access the wrapped string value.
- *
- * @method
- * @return {String} returns the wrapped string.
- */
-Symbol.prototype.valueOf = function() {
- return this.value;
-};
-
-/**
- * @ignore
- */
-Symbol.prototype.toString = function() {
- return this.value;
-};
-
-/**
- * @ignore
- */
-Symbol.prototype[inspect] = function() {
- return this.value;
-};
-
-/**
- * @ignore
- */
-Symbol.prototype.toJSON = function() {
- return this.value;
-};
-
-module.exports = Symbol;
-module.exports.Symbol = Symbol;
diff --git a/node_modules/bson/lib/bson/timestamp.js b/node_modules/bson/lib/bson/timestamp.js
deleted file mode 100644
index dc61a6c..0000000
--- a/node_modules/bson/lib/bson/timestamp.js
+++ /dev/null
@@ -1,854 +0,0 @@
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-//
-// Copyright 2009 Google Inc. All Rights Reserved
-
-/**
- * This type is for INTERNAL use in MongoDB only and should not be used in applications.
- * The appropriate corresponding type is the JavaScript Date type.
- *
- * Defines a Timestamp class for representing a 64-bit two's-complement
- * integer value, which faithfully simulates the behavior of a Java "Timestamp". This
- * implementation is derived from TimestampLib in GWT.
- *
- * Constructs a 64-bit two's-complement integer, given its low and high 32-bit
- * values as *signed* integers. See the from* functions below for more
- * convenient ways of constructing Timestamps.
- *
- * The internal representation of a Timestamp is the two given signed, 32-bit values.
- * We use 32-bit pieces because these are the size of integers on which
- * Javascript performs bit-operations. For operations like addition and
- * multiplication, we split each number into 16-bit pieces, which can easily be
- * multiplied within Javascript's floating-point representation without overflow
- * or change in sign.
- *
- * In the algorithms below, we frequently reduce the negative case to the
- * positive case by negating the input(s) and then post-processing the result.
- * Note that we must ALWAYS check specially whether those values are MIN_VALUE
- * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
- * a positive number, it overflows back into a negative). Not handling this
- * case would often result in infinite recursion.
- *
- * @class
- * @param {number} low the low (signed) 32 bits of the Timestamp.
- * @param {number} high the high (signed) 32 bits of the Timestamp.
- */
-function Timestamp(low, high) {
- if (!(this instanceof Timestamp)) return new Timestamp(low, high);
- this._bsontype = 'Timestamp';
- /**
- * @type {number}
- * @ignore
- */
- this.low_ = low | 0; // force into 32 signed bits.
-
- /**
- * @type {number}
- * @ignore
- */
- this.high_ = high | 0; // force into 32 signed bits.
-}
-
-/**
- * Return the int value.
- *
- * @return {number} the value, assuming it is a 32-bit integer.
- */
-Timestamp.prototype.toInt = function() {
- return this.low_;
-};
-
-/**
- * Return the Number value.
- *
- * @method
- * @return {number} the closest floating-point representation to this value.
- */
-Timestamp.prototype.toNumber = function() {
- return this.high_ * Timestamp.TWO_PWR_32_DBL_ + this.getLowBitsUnsigned();
-};
-
-/**
- * Return the JSON value.
- *
- * @method
- * @return {string} the JSON representation.
- */
-Timestamp.prototype.toJSON = function() {
- return this.toString();
-};
-
-/**
- * Return the String value.
- *
- * @method
- * @param {number} [opt_radix] the radix in which the text should be written.
- * @return {string} the textual representation of this value.
- */
-Timestamp.prototype.toString = function(opt_radix) {
- var radix = opt_radix || 10;
- if (radix < 2 || 36 < radix) {
- throw Error('radix out of range: ' + radix);
- }
-
- if (this.isZero()) {
- return '0';
- }
-
- if (this.isNegative()) {
- if (this.equals(Timestamp.MIN_VALUE)) {
- // We need to change the Timestamp value before it can be negated, so we remove
- // the bottom-most digit in this base and then recurse to do the rest.
- var radixTimestamp = Timestamp.fromNumber(radix);
- var div = this.div(radixTimestamp);
- var rem = div.multiply(radixTimestamp).subtract(this);
- return div.toString(radix) + rem.toInt().toString(radix);
- } else {
- return '-' + this.negate().toString(radix);
- }
- }
-
- // Do several (6) digits each time through the loop, so as to
- // minimize the calls to the very expensive emulated div.
- var radixToPower = Timestamp.fromNumber(Math.pow(radix, 6));
-
- rem = this;
- var result = '';
-
- while (!rem.isZero()) {
- var remDiv = rem.div(radixToPower);
- var intval = rem.subtract(remDiv.multiply(radixToPower)).toInt();
- var digits = intval.toString(radix);
-
- rem = remDiv;
- if (rem.isZero()) {
- return digits + result;
- } else {
- while (digits.length < 6) {
- digits = '0' + digits;
- }
- result = '' + digits + result;
- }
- }
-};
-
-/**
- * Return the high 32-bits value.
- *
- * @method
- * @return {number} the high 32-bits as a signed value.
- */
-Timestamp.prototype.getHighBits = function() {
- return this.high_;
-};
-
-/**
- * Return the low 32-bits value.
- *
- * @method
- * @return {number} the low 32-bits as a signed value.
- */
-Timestamp.prototype.getLowBits = function() {
- return this.low_;
-};
-
-/**
- * Return the low unsigned 32-bits value.
- *
- * @method
- * @return {number} the low 32-bits as an unsigned value.
- */
-Timestamp.prototype.getLowBitsUnsigned = function() {
- return this.low_ >= 0 ? this.low_ : Timestamp.TWO_PWR_32_DBL_ + this.low_;
-};
-
-/**
- * Returns the number of bits needed to represent the absolute value of this Timestamp.
- *
- * @method
- * @return {number} Returns the number of bits needed to represent the absolute value of this Timestamp.
- */
-Timestamp.prototype.getNumBitsAbs = function() {
- if (this.isNegative()) {
- if (this.equals(Timestamp.MIN_VALUE)) {
- return 64;
- } else {
- return this.negate().getNumBitsAbs();
- }
- } else {
- var val = this.high_ !== 0 ? this.high_ : this.low_;
- for (var bit = 31; bit > 0; bit--) {
- if ((val & (1 << bit)) !== 0) {
- break;
- }
- }
- return this.high_ !== 0 ? bit + 33 : bit + 1;
- }
-};
-
-/**
- * Return whether this value is zero.
- *
- * @method
- * @return {boolean} whether this value is zero.
- */
-Timestamp.prototype.isZero = function() {
- return this.high_ === 0 && this.low_ === 0;
-};
-
-/**
- * Return whether this value is negative.
- *
- * @method
- * @return {boolean} whether this value is negative.
- */
-Timestamp.prototype.isNegative = function() {
- return this.high_ < 0;
-};
-
-/**
- * Return whether this value is odd.
- *
- * @method
- * @return {boolean} whether this value is odd.
- */
-Timestamp.prototype.isOdd = function() {
- return (this.low_ & 1) === 1;
-};
-
-/**
- * Return whether this Timestamp equals the other
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp equals the other
- */
-Timestamp.prototype.equals = function(other) {
- return this.high_ === other.high_ && this.low_ === other.low_;
-};
-
-/**
- * Return whether this Timestamp does not equal the other.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp does not equal the other.
- */
-Timestamp.prototype.notEquals = function(other) {
- return this.high_ !== other.high_ || this.low_ !== other.low_;
-};
-
-/**
- * Return whether this Timestamp is less than the other.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp is less than the other.
- */
-Timestamp.prototype.lessThan = function(other) {
- return this.compare(other) < 0;
-};
-
-/**
- * Return whether this Timestamp is less than or equal to the other.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp is less than or equal to the other.
- */
-Timestamp.prototype.lessThanOrEqual = function(other) {
- return this.compare(other) <= 0;
-};
-
-/**
- * Return whether this Timestamp is greater than the other.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp is greater than the other.
- */
-Timestamp.prototype.greaterThan = function(other) {
- return this.compare(other) > 0;
-};
-
-/**
- * Return whether this Timestamp is greater than or equal to the other.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} whether this Timestamp is greater than or equal to the other.
- */
-Timestamp.prototype.greaterThanOrEqual = function(other) {
- return this.compare(other) >= 0;
-};
-
-/**
- * Compares this Timestamp with the given one.
- *
- * @method
- * @param {Timestamp} other Timestamp to compare against.
- * @return {boolean} 0 if they are the same, 1 if the this is greater, and -1 if the given one is greater.
- */
-Timestamp.prototype.compare = function(other) {
- if (this.equals(other)) {
- return 0;
- }
-
- var thisNeg = this.isNegative();
- var otherNeg = other.isNegative();
- if (thisNeg && !otherNeg) {
- return -1;
- }
- if (!thisNeg && otherNeg) {
- return 1;
- }
-
- // at this point, the signs are the same, so subtraction will not overflow
- if (this.subtract(other).isNegative()) {
- return -1;
- } else {
- return 1;
- }
-};
-
-/**
- * The negation of this value.
- *
- * @method
- * @return {Timestamp} the negation of this value.
- */
-Timestamp.prototype.negate = function() {
- if (this.equals(Timestamp.MIN_VALUE)) {
- return Timestamp.MIN_VALUE;
- } else {
- return this.not().add(Timestamp.ONE);
- }
-};
-
-/**
- * Returns the sum of this and the given Timestamp.
- *
- * @method
- * @param {Timestamp} other Timestamp to add to this one.
- * @return {Timestamp} the sum of this and the given Timestamp.
- */
-Timestamp.prototype.add = function(other) {
- // Divide each number into 4 chunks of 16 bits, and then sum the chunks.
-
- var a48 = this.high_ >>> 16;
- var a32 = this.high_ & 0xffff;
- var a16 = this.low_ >>> 16;
- var a00 = this.low_ & 0xffff;
-
- var b48 = other.high_ >>> 16;
- var b32 = other.high_ & 0xffff;
- var b16 = other.low_ >>> 16;
- var b00 = other.low_ & 0xffff;
-
- var c48 = 0,
- c32 = 0,
- c16 = 0,
- c00 = 0;
- c00 += a00 + b00;
- c16 += c00 >>> 16;
- c00 &= 0xffff;
- c16 += a16 + b16;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c32 += a32 + b32;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c48 += a48 + b48;
- c48 &= 0xffff;
- return Timestamp.fromBits((c16 << 16) | c00, (c48 << 16) | c32);
-};
-
-/**
- * Returns the difference of this and the given Timestamp.
- *
- * @method
- * @param {Timestamp} other Timestamp to subtract from this.
- * @return {Timestamp} the difference of this and the given Timestamp.
- */
-Timestamp.prototype.subtract = function(other) {
- return this.add(other.negate());
-};
-
-/**
- * Returns the product of this and the given Timestamp.
- *
- * @method
- * @param {Timestamp} other Timestamp to multiply with this.
- * @return {Timestamp} the product of this and the other.
- */
-Timestamp.prototype.multiply = function(other) {
- if (this.isZero()) {
- return Timestamp.ZERO;
- } else if (other.isZero()) {
- return Timestamp.ZERO;
- }
-
- if (this.equals(Timestamp.MIN_VALUE)) {
- return other.isOdd() ? Timestamp.MIN_VALUE : Timestamp.ZERO;
- } else if (other.equals(Timestamp.MIN_VALUE)) {
- return this.isOdd() ? Timestamp.MIN_VALUE : Timestamp.ZERO;
- }
-
- if (this.isNegative()) {
- if (other.isNegative()) {
- return this.negate().multiply(other.negate());
- } else {
- return this.negate()
- .multiply(other)
- .negate();
- }
- } else if (other.isNegative()) {
- return this.multiply(other.negate()).negate();
- }
-
- // If both Timestamps are small, use float multiplication
- if (this.lessThan(Timestamp.TWO_PWR_24_) && other.lessThan(Timestamp.TWO_PWR_24_)) {
- return Timestamp.fromNumber(this.toNumber() * other.toNumber());
- }
-
- // Divide each Timestamp into 4 chunks of 16 bits, and then add up 4x4 products.
- // We can skip products that would overflow.
-
- var a48 = this.high_ >>> 16;
- var a32 = this.high_ & 0xffff;
- var a16 = this.low_ >>> 16;
- var a00 = this.low_ & 0xffff;
-
- var b48 = other.high_ >>> 16;
- var b32 = other.high_ & 0xffff;
- var b16 = other.low_ >>> 16;
- var b00 = other.low_ & 0xffff;
-
- var c48 = 0,
- c32 = 0,
- c16 = 0,
- c00 = 0;
- c00 += a00 * b00;
- c16 += c00 >>> 16;
- c00 &= 0xffff;
- c16 += a16 * b00;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c16 += a00 * b16;
- c32 += c16 >>> 16;
- c16 &= 0xffff;
- c32 += a32 * b00;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c32 += a16 * b16;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c32 += a00 * b32;
- c48 += c32 >>> 16;
- c32 &= 0xffff;
- c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;
- c48 &= 0xffff;
- return Timestamp.fromBits((c16 << 16) | c00, (c48 << 16) | c32);
-};
-
-/**
- * Returns this Timestamp divided by the given one.
- *
- * @method
- * @param {Timestamp} other Timestamp by which to divide.
- * @return {Timestamp} this Timestamp divided by the given one.
- */
-Timestamp.prototype.div = function(other) {
- if (other.isZero()) {
- throw Error('division by zero');
- } else if (this.isZero()) {
- return Timestamp.ZERO;
- }
-
- if (this.equals(Timestamp.MIN_VALUE)) {
- if (other.equals(Timestamp.ONE) || other.equals(Timestamp.NEG_ONE)) {
- return Timestamp.MIN_VALUE; // recall that -MIN_VALUE == MIN_VALUE
- } else if (other.equals(Timestamp.MIN_VALUE)) {
- return Timestamp.ONE;
- } else {
- // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.
- var halfThis = this.shiftRight(1);
- var approx = halfThis.div(other).shiftLeft(1);
- if (approx.equals(Timestamp.ZERO)) {
- return other.isNegative() ? Timestamp.ONE : Timestamp.NEG_ONE;
- } else {
- var rem = this.subtract(other.multiply(approx));
- var result = approx.add(rem.div(other));
- return result;
- }
- }
- } else if (other.equals(Timestamp.MIN_VALUE)) {
- return Timestamp.ZERO;
- }
-
- if (this.isNegative()) {
- if (other.isNegative()) {
- return this.negate().div(other.negate());
- } else {
- return this.negate()
- .div(other)
- .negate();
- }
- } else if (other.isNegative()) {
- return this.div(other.negate()).negate();
- }
-
- // Repeat the following until the remainder is less than other: find a
- // floating-point that approximates remainder / other *from below*, add this
- // into the result, and subtract it from the remainder. It is critical that
- // the approximate value is less than or equal to the real value so that the
- // remainder never becomes negative.
- var res = Timestamp.ZERO;
- rem = this;
- while (rem.greaterThanOrEqual(other)) {
- // Approximate the result of division. This may be a little greater or
- // smaller than the actual value.
- approx = Math.max(1, Math.floor(rem.toNumber() / other.toNumber()));
-
- // We will tweak the approximate result by changing it in the 48-th digit or
- // the smallest non-fractional digit, whichever is larger.
- var log2 = Math.ceil(Math.log(approx) / Math.LN2);
- var delta = log2 <= 48 ? 1 : Math.pow(2, log2 - 48);
-
- // Decrease the approximation until it is smaller than the remainder. Note
- // that if it is too large, the product overflows and is negative.
- var approxRes = Timestamp.fromNumber(approx);
- var approxRem = approxRes.multiply(other);
- while (approxRem.isNegative() || approxRem.greaterThan(rem)) {
- approx -= delta;
- approxRes = Timestamp.fromNumber(approx);
- approxRem = approxRes.multiply(other);
- }
-
- // We know the answer can't be zero... and actually, zero would cause
- // infinite recursion since we would make no progress.
- if (approxRes.isZero()) {
- approxRes = Timestamp.ONE;
- }
-
- res = res.add(approxRes);
- rem = rem.subtract(approxRem);
- }
- return res;
-};
-
-/**
- * Returns this Timestamp modulo the given one.
- *
- * @method
- * @param {Timestamp} other Timestamp by which to mod.
- * @return {Timestamp} this Timestamp modulo the given one.
- */
-Timestamp.prototype.modulo = function(other) {
- return this.subtract(this.div(other).multiply(other));
-};
-
-/**
- * The bitwise-NOT of this value.
- *
- * @method
- * @return {Timestamp} the bitwise-NOT of this value.
- */
-Timestamp.prototype.not = function() {
- return Timestamp.fromBits(~this.low_, ~this.high_);
-};
-
-/**
- * Returns the bitwise-AND of this Timestamp and the given one.
- *
- * @method
- * @param {Timestamp} other the Timestamp with which to AND.
- * @return {Timestamp} the bitwise-AND of this and the other.
- */
-Timestamp.prototype.and = function(other) {
- return Timestamp.fromBits(this.low_ & other.low_, this.high_ & other.high_);
-};
-
-/**
- * Returns the bitwise-OR of this Timestamp and the given one.
- *
- * @method
- * @param {Timestamp} other the Timestamp with which to OR.
- * @return {Timestamp} the bitwise-OR of this and the other.
- */
-Timestamp.prototype.or = function(other) {
- return Timestamp.fromBits(this.low_ | other.low_, this.high_ | other.high_);
-};
-
-/**
- * Returns the bitwise-XOR of this Timestamp and the given one.
- *
- * @method
- * @param {Timestamp} other the Timestamp with which to XOR.
- * @return {Timestamp} the bitwise-XOR of this and the other.
- */
-Timestamp.prototype.xor = function(other) {
- return Timestamp.fromBits(this.low_ ^ other.low_, this.high_ ^ other.high_);
-};
-
-/**
- * Returns this Timestamp with bits shifted to the left by the given amount.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Timestamp} this shifted to the left by the given amount.
- */
-Timestamp.prototype.shiftLeft = function(numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var low = this.low_;
- if (numBits < 32) {
- var high = this.high_;
- return Timestamp.fromBits(low << numBits, (high << numBits) | (low >>> (32 - numBits)));
- } else {
- return Timestamp.fromBits(0, low << (numBits - 32));
- }
- }
-};
-
-/**
- * Returns this Timestamp with bits shifted to the right by the given amount.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Timestamp} this shifted to the right by the given amount.
- */
-Timestamp.prototype.shiftRight = function(numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var high = this.high_;
- if (numBits < 32) {
- var low = this.low_;
- return Timestamp.fromBits((low >>> numBits) | (high << (32 - numBits)), high >> numBits);
- } else {
- return Timestamp.fromBits(high >> (numBits - 32), high >= 0 ? 0 : -1);
- }
- }
-};
-
-/**
- * Returns this Timestamp with bits shifted to the right by the given amount, with the new top bits matching the current sign bit.
- *
- * @method
- * @param {number} numBits the number of bits by which to shift.
- * @return {Timestamp} this shifted to the right by the given amount, with zeros placed into the new leading bits.
- */
-Timestamp.prototype.shiftRightUnsigned = function(numBits) {
- numBits &= 63;
- if (numBits === 0) {
- return this;
- } else {
- var high = this.high_;
- if (numBits < 32) {
- var low = this.low_;
- return Timestamp.fromBits((low >>> numBits) | (high << (32 - numBits)), high >>> numBits);
- } else if (numBits === 32) {
- return Timestamp.fromBits(high, 0);
- } else {
- return Timestamp.fromBits(high >>> (numBits - 32), 0);
- }
- }
-};
-
-/**
- * Returns a Timestamp representing the given (32-bit) integer value.
- *
- * @method
- * @param {number} value the 32-bit integer in question.
- * @return {Timestamp} the corresponding Timestamp value.
- */
-Timestamp.fromInt = function(value) {
- if (-128 <= value && value < 128) {
- var cachedObj = Timestamp.INT_CACHE_[value];
- if (cachedObj) {
- return cachedObj;
- }
- }
-
- var obj = new Timestamp(value | 0, value < 0 ? -1 : 0);
- if (-128 <= value && value < 128) {
- Timestamp.INT_CACHE_[value] = obj;
- }
- return obj;
-};
-
-/**
- * Returns a Timestamp representing the given value, provided that it is a finite number. Otherwise, zero is returned.
- *
- * @method
- * @param {number} value the number in question.
- * @return {Timestamp} the corresponding Timestamp value.
- */
-Timestamp.fromNumber = function(value) {
- if (isNaN(value) || !isFinite(value)) {
- return Timestamp.ZERO;
- } else if (value <= -Timestamp.TWO_PWR_63_DBL_) {
- return Timestamp.MIN_VALUE;
- } else if (value + 1 >= Timestamp.TWO_PWR_63_DBL_) {
- return Timestamp.MAX_VALUE;
- } else if (value < 0) {
- return Timestamp.fromNumber(-value).negate();
- } else {
- return new Timestamp(
- (value % Timestamp.TWO_PWR_32_DBL_) | 0,
- (value / Timestamp.TWO_PWR_32_DBL_) | 0
- );
- }
-};
-
-/**
- * Returns a Timestamp representing the 64-bit integer that comes by concatenating the given high and low bits. Each is assumed to use 32 bits.
- *
- * @method
- * @param {number} lowBits the low 32-bits.
- * @param {number} highBits the high 32-bits.
- * @return {Timestamp} the corresponding Timestamp value.
- */
-Timestamp.fromBits = function(lowBits, highBits) {
- return new Timestamp(lowBits, highBits);
-};
-
-/**
- * Returns a Timestamp representation of the given string, written using the given radix.
- *
- * @method
- * @param {string} str the textual representation of the Timestamp.
- * @param {number} opt_radix the radix in which the text is written.
- * @return {Timestamp} the corresponding Timestamp value.
- */
-Timestamp.fromString = function(str, opt_radix) {
- if (str.length === 0) {
- throw Error('number format error: empty string');
- }
-
- var radix = opt_radix || 10;
- if (radix < 2 || 36 < radix) {
- throw Error('radix out of range: ' + radix);
- }
-
- if (str.charAt(0) === '-') {
- return Timestamp.fromString(str.substring(1), radix).negate();
- } else if (str.indexOf('-') >= 0) {
- throw Error('number format error: interior "-" character: ' + str);
- }
-
- // Do several (8) digits each time through the loop, so as to
- // minimize the calls to the very expensive emulated div.
- var radixToPower = Timestamp.fromNumber(Math.pow(radix, 8));
-
- var result = Timestamp.ZERO;
- for (var i = 0; i < str.length; i += 8) {
- var size = Math.min(8, str.length - i);
- var value = parseInt(str.substring(i, i + size), radix);
- if (size < 8) {
- var power = Timestamp.fromNumber(Math.pow(radix, size));
- result = result.multiply(power).add(Timestamp.fromNumber(value));
- } else {
- result = result.multiply(radixToPower);
- result = result.add(Timestamp.fromNumber(value));
- }
- }
- return result;
-};
-
-// NOTE: Common constant values ZERO, ONE, NEG_ONE, etc. are defined below the
-// from* methods on which they depend.
-
-/**
- * A cache of the Timestamp representations of small integer values.
- * @type {Object}
- * @ignore
- */
-Timestamp.INT_CACHE_ = {};
-
-// NOTE: the compiler should inline these constant values below and then remove
-// these variables, so there should be no runtime penalty for these.
-
-/**
- * Number used repeated below in calculations. This must appear before the
- * first call to any from* function below.
- * @type {number}
- * @ignore
- */
-Timestamp.TWO_PWR_16_DBL_ = 1 << 16;
-
-/**
- * @type {number}
- * @ignore
- */
-Timestamp.TWO_PWR_24_DBL_ = 1 << 24;
-
-/**
- * @type {number}
- * @ignore
- */
-Timestamp.TWO_PWR_32_DBL_ = Timestamp.TWO_PWR_16_DBL_ * Timestamp.TWO_PWR_16_DBL_;
-
-/**
- * @type {number}
- * @ignore
- */
-Timestamp.TWO_PWR_31_DBL_ = Timestamp.TWO_PWR_32_DBL_ / 2;
-
-/**
- * @type {number}
- * @ignore
- */
-Timestamp.TWO_PWR_48_DBL_ = Timestamp.TWO_PWR_32_DBL_ * Timestamp.TWO_PWR_16_DBL_;
-
-/**
- * @type {number}
- * @ignore
- */
-Timestamp.TWO_PWR_64_DBL_ = Timestamp.TWO_PWR_32_DBL_ * Timestamp.TWO_PWR_32_DBL_;
-
-/**
- * @type {number}
- * @ignore
- */
-Timestamp.TWO_PWR_63_DBL_ = Timestamp.TWO_PWR_64_DBL_ / 2;
-
-/** @type {Timestamp} */
-Timestamp.ZERO = Timestamp.fromInt(0);
-
-/** @type {Timestamp} */
-Timestamp.ONE = Timestamp.fromInt(1);
-
-/** @type {Timestamp} */
-Timestamp.NEG_ONE = Timestamp.fromInt(-1);
-
-/** @type {Timestamp} */
-Timestamp.MAX_VALUE = Timestamp.fromBits(0xffffffff | 0, 0x7fffffff | 0);
-
-/** @type {Timestamp} */
-Timestamp.MIN_VALUE = Timestamp.fromBits(0, 0x80000000 | 0);
-
-/**
- * @type {Timestamp}
- * @ignore
- */
-Timestamp.TWO_PWR_24_ = Timestamp.fromInt(1 << 24);
-
-/**
- * Expose.
- */
-module.exports = Timestamp;
-module.exports.Timestamp = Timestamp;
diff --git a/node_modules/bson/lib/code.js b/node_modules/bson/lib/code.js
new file mode 100644
index 0000000..05bc1c9
--- /dev/null
+++ b/node_modules/bson/lib/code.js
@@ -0,0 +1,46 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Code = void 0;
+/**
+ * A class representation of the BSON Code type.
+ * @public
+ */
+var Code = /** @class */ (function () {
+ /**
+ * @param code - a string or function.
+ * @param scope - an optional scope for the function.
+ */
+ function Code(code, scope) {
+ if (!(this instanceof Code))
+ return new Code(code, scope);
+ this.code = code;
+ this.scope = scope;
+ }
+ /** @internal */
+ Code.prototype.toJSON = function () {
+ return { code: this.code, scope: this.scope };
+ };
+ /** @internal */
+ Code.prototype.toExtendedJSON = function () {
+ if (this.scope) {
+ return { $code: this.code, $scope: this.scope };
+ }
+ return { $code: this.code };
+ };
+ /** @internal */
+ Code.fromExtendedJSON = function (doc) {
+ return new Code(doc.$code, doc.$scope);
+ };
+ /** @internal */
+ Code.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Code.prototype.inspect = function () {
+ var codeJson = this.toJSON();
+ return "new Code(\"" + codeJson.code + "\"" + (codeJson.scope ? ", " + JSON.stringify(codeJson.scope) : '') + ")";
+ };
+ return Code;
+}());
+exports.Code = Code;
+Object.defineProperty(Code.prototype, '_bsontype', { value: 'Code' });
+//# sourceMappingURL=code.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/code.js.map b/node_modules/bson/lib/code.js.map
new file mode 100644
index 0000000..5966aeb
--- /dev/null
+++ b/node_modules/bson/lib/code.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"code.js","sourceRoot":"","sources":["../src/code.ts"],"names":[],"mappings":";;;AAQA;;;GAGG;AACH;IAKE;;;OAGG;IACH,cAAY,IAAuB,EAAE,KAAgB;QACnD,IAAI,CAAC,CAAC,IAAI,YAAY,IAAI,CAAC;YAAE,OAAO,IAAI,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;QAE1D,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC;QACjB,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;IACrB,CAAC;IAED,gBAAgB;IAChB,qBAAM,GAAN;QACE,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,IAAI,EAAE,KAAK,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;IAChD,CAAC;IAED,gBAAgB;IAChB,6BAAc,GAAd;QACE,IAAI,IAAI,CAAC,KAAK,EAAE;YACd,OAAO,EAAE,KAAK,EAAE,IAAI,CAAC,IAAI,EAAE,MAAM,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;SACjD;QAED,OAAO,EAAE,KAAK,EAAE,IAAI,CAAC,IAAI,EAAE,CAAC;IAC9B,CAAC;IAED,gBAAgB;IACT,qBAAgB,GAAvB,UAAwB,GAAiB;QACvC,OAAO,IAAI,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,GAAG,CAAC,MAAM,CAAC,CAAC;IACzC,CAAC;IAED,gBAAgB;IAChB,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,sBAAO,GAAP;QACE,IAAM,QAAQ,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC;QAC/B,OAAO,gBAAa,QAAQ,CAAC,IAAI,WAC/B,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,OAAK,IAAI,CAAC,SAAS,CAAC,QAAQ,CAAC,KAAK,CAAG,CAAC,CAAC,CAAC,EAAE,OAC1D,CAAC;IACN,CAAC;IACH,WAAC;AAAD,CAAC,AA9CD,IA8CC;AA9CY,oBAAI;AAgDjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/constants.js b/node_modules/bson/lib/constants.js
new file mode 100644
index 0000000..4018397
--- /dev/null
+++ b/node_modules/bson/lib/constants.js
@@ -0,0 +1,78 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.BSON_BINARY_SUBTYPE_USER_DEFINED = exports.BSON_BINARY_SUBTYPE_MD5 = exports.BSON_BINARY_SUBTYPE_UUID_NEW = exports.BSON_BINARY_SUBTYPE_UUID = exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = exports.BSON_BINARY_SUBTYPE_FUNCTION = exports.BSON_BINARY_SUBTYPE_DEFAULT = exports.BSON_DATA_MAX_KEY = exports.BSON_DATA_MIN_KEY = exports.BSON_DATA_DECIMAL128 = exports.BSON_DATA_LONG = exports.BSON_DATA_TIMESTAMP = exports.BSON_DATA_INT = exports.BSON_DATA_CODE_W_SCOPE = exports.BSON_DATA_SYMBOL = exports.BSON_DATA_CODE = exports.BSON_DATA_DBPOINTER = exports.BSON_DATA_REGEXP = exports.BSON_DATA_NULL = exports.BSON_DATA_DATE = exports.BSON_DATA_BOOLEAN = exports.BSON_DATA_OID = exports.BSON_DATA_UNDEFINED = exports.BSON_DATA_BINARY = exports.BSON_DATA_ARRAY = exports.BSON_DATA_OBJECT = exports.BSON_DATA_STRING = exports.BSON_DATA_NUMBER = exports.JS_INT_MIN = exports.JS_INT_MAX = exports.BSON_INT64_MIN = exports.BSON_INT64_MAX = exports.BSON_INT32_MIN = exports.BSON_INT32_MAX = void 0;
+/** @internal */
+exports.BSON_INT32_MAX = 0x7fffffff;
+/** @internal */
+exports.BSON_INT32_MIN = -0x80000000;
+/** @internal */
+exports.BSON_INT64_MAX = Math.pow(2, 63) - 1;
+/** @internal */
+exports.BSON_INT64_MIN = -Math.pow(2, 63);
+/**
+ * Any integer up to 2^53 can be precisely represented by a double.
+ * @internal
+ */
+exports.JS_INT_MAX = Math.pow(2, 53);
+/**
+ * Any integer down to -2^53 can be precisely represented by a double.
+ * @internal
+ */
+exports.JS_INT_MIN = -Math.pow(2, 53);
+/** Number BSON Type @internal */
+exports.BSON_DATA_NUMBER = 1;
+/** String BSON Type @internal */
+exports.BSON_DATA_STRING = 2;
+/** Object BSON Type @internal */
+exports.BSON_DATA_OBJECT = 3;
+/** Array BSON Type @internal */
+exports.BSON_DATA_ARRAY = 4;
+/** Binary BSON Type @internal */
+exports.BSON_DATA_BINARY = 5;
+/** Binary BSON Type @internal */
+exports.BSON_DATA_UNDEFINED = 6;
+/** ObjectId BSON Type @internal */
+exports.BSON_DATA_OID = 7;
+/** Boolean BSON Type @internal */
+exports.BSON_DATA_BOOLEAN = 8;
+/** Date BSON Type @internal */
+exports.BSON_DATA_DATE = 9;
+/** null BSON Type @internal */
+exports.BSON_DATA_NULL = 10;
+/** RegExp BSON Type @internal */
+exports.BSON_DATA_REGEXP = 11;
+/** Code BSON Type @internal */
+exports.BSON_DATA_DBPOINTER = 12;
+/** Code BSON Type @internal */
+exports.BSON_DATA_CODE = 13;
+/** Symbol BSON Type @internal */
+exports.BSON_DATA_SYMBOL = 14;
+/** Code with Scope BSON Type @internal */
+exports.BSON_DATA_CODE_W_SCOPE = 15;
+/** 32 bit Integer BSON Type @internal */
+exports.BSON_DATA_INT = 16;
+/** Timestamp BSON Type @internal */
+exports.BSON_DATA_TIMESTAMP = 17;
+/** Long BSON Type @internal */
+exports.BSON_DATA_LONG = 18;
+/** Decimal128 BSON Type @internal */
+exports.BSON_DATA_DECIMAL128 = 19;
+/** MinKey BSON Type @internal */
+exports.BSON_DATA_MIN_KEY = 0xff;
+/** MaxKey BSON Type @internal */
+exports.BSON_DATA_MAX_KEY = 0x7f;
+/** Binary Default Type @internal */
+exports.BSON_BINARY_SUBTYPE_DEFAULT = 0;
+/** Binary Function Type @internal */
+exports.BSON_BINARY_SUBTYPE_FUNCTION = 1;
+/** Binary Byte Array Type @internal */
+exports.BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
+/** Binary Deprecated UUID Type @deprecated Please use BSON_BINARY_SUBTYPE_UUID_NEW @internal */
+exports.BSON_BINARY_SUBTYPE_UUID = 3;
+/** Binary UUID Type @internal */
+exports.BSON_BINARY_SUBTYPE_UUID_NEW = 4;
+/** Binary MD5 Type @internal */
+exports.BSON_BINARY_SUBTYPE_MD5 = 5;
+/** Binary User Defined Type @internal */
+exports.BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
+//# sourceMappingURL=constants.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/constants.js.map b/node_modules/bson/lib/constants.js.map
new file mode 100644
index 0000000..8ded6ab
--- /dev/null
+++ b/node_modules/bson/lib/constants.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"constants.js","sourceRoot":"","sources":["../src/constants.ts"],"names":[],"mappings":";;;AAAA,gBAAgB;AACH,QAAA,cAAc,GAAG,UAAU,CAAC;AACzC,gBAAgB;AACH,QAAA,cAAc,GAAG,CAAC,UAAU,CAAC;AAC1C,gBAAgB;AACH,QAAA,cAAc,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,GAAG,CAAC,CAAC;AAClD,gBAAgB;AACH,QAAA,cAAc,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;AAE/C;;;GAGG;AACU,QAAA,UAAU,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;AAE1C;;;GAGG;AACU,QAAA,UAAU,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;AAE3C,iCAAiC;AACpB,QAAA,gBAAgB,GAAG,CAAC,CAAC;AAElC,iCAAiC;AACpB,QAAA,gBAAgB,GAAG,CAAC,CAAC;AAElC,iCAAiC;AACpB,QAAA,gBAAgB,GAAG,CAAC,CAAC;AAElC,gCAAgC;AACnB,QAAA,eAAe,GAAG,CAAC,CAAC;AAEjC,iCAAiC;AACpB,QAAA,gBAAgB,GAAG,CAAC,CAAC;AAElC,iCAAiC;AACpB,QAAA,mBAAmB,GAAG,CAAC,CAAC;AAErC,mCAAmC;AACtB,QAAA,aAAa,GAAG,CAAC,CAAC;AAE/B,kCAAkC;AACrB,QAAA,iBAAiB,GAAG,CAAC,CAAC;AAEnC,+BAA+B;AAClB,QAAA,cAAc,GAAG,CAAC,CAAC;AAEhC,+BAA+B;AAClB,QAAA,cAAc,GAAG,EAAE,CAAC;AAEjC,iCAAiC;AACpB,QAAA,gBAAgB,GAAG,EAAE,CAAC;AAEnC,+BAA+B;AAClB,QAAA,mBAAmB,GAAG,EAAE,CAAC;AAEtC,+BAA+B;AAClB,QAAA,cAAc,GAAG,EAAE,CAAC;AAEjC,iCAAiC;AACpB,QAAA,gBAAgB,GAAG,EAAE,CAAC;AAEnC,0CAA0C;AAC7B,QAAA,sBAAsB,GAAG,EAAE,CAAC;AAEzC,yCAAyC;AAC5B,QAAA,aAAa,GAAG,EAAE,CAAC;AAEhC,oCAAoC;AACvB,QAAA,mBAAmB,GAAG,EAAE,CAAC;AAEtC,+BAA+B;AAClB,QAAA,cAAc,GAAG,EAAE,CAAC;AAEjC,qCAAqC;AACxB,QAAA,oBAAoB,GAAG,EAAE,CAAC;AAEvC,iCAAiC;AACpB,QAAA,iBAAiB,GAAG,IAAI,CAAC;AAEtC,iCAAiC;AACpB,QAAA,iBAAiB,GAAG,IAAI,CAAC;AAEtC,oCAAoC;AACvB,QAAA,2BAA2B,GAAG,CAAC,CAAC;AAE7C,qCAAqC;AACxB,QAAA,4BAA4B,GAAG,CAAC,CAAC;AAE9C,uCAAuC;AAC1B,QAAA,8BAA8B,GAAG,CAAC,CAAC;AAEhD,gGAAgG;AACnF,QAAA,wBAAwB,GAAG,CAAC,CAAC;AAE1C,iCAAiC;AACpB,QAAA,4BAA4B,GAAG,CAAC,CAAC;AAE9C,gCAAgC;AACnB,QAAA,uBAAuB,GAAG,CAAC,CAAC;AAEzC,yCAAyC;AAC5B,QAAA,gCAAgC,GAAG,GAAG,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/db_ref.js b/node_modules/bson/lib/db_ref.js
new file mode 100644
index 0000000..e378ee5
--- /dev/null
+++ b/node_modules/bson/lib/db_ref.js
@@ -0,0 +1,97 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.DBRef = exports.isDBRefLike = void 0;
+var utils_1 = require("./parser/utils");
+/** @internal */
+function isDBRefLike(value) {
+ return (utils_1.isObjectLike(value) &&
+ value.$id != null &&
+ typeof value.$ref === 'string' &&
+ (value.$db == null || typeof value.$db === 'string'));
+}
+exports.isDBRefLike = isDBRefLike;
+/**
+ * A class representation of the BSON DBRef type.
+ * @public
+ */
+var DBRef = /** @class */ (function () {
+ /**
+ * @param collection - the collection name.
+ * @param oid - the reference ObjectId.
+ * @param db - optional db name, if omitted the reference is local to the current db.
+ */
+ function DBRef(collection, oid, db, fields) {
+ if (!(this instanceof DBRef))
+ return new DBRef(collection, oid, db, fields);
+ // check if namespace has been provided
+ var parts = collection.split('.');
+ if (parts.length === 2) {
+ db = parts.shift();
+ // eslint-disable-next-line @typescript-eslint/no-non-null-assertion
+ collection = parts.shift();
+ }
+ this.collection = collection;
+ this.oid = oid;
+ this.db = db;
+ this.fields = fields || {};
+ }
+ Object.defineProperty(DBRef.prototype, "namespace", {
+ // Property provided for compatibility with the 1.x parser
+ // the 1.x parser used a "namespace" property, while 4.x uses "collection"
+ /** @internal */
+ get: function () {
+ return this.collection;
+ },
+ set: function (value) {
+ this.collection = value;
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /** @internal */
+ DBRef.prototype.toJSON = function () {
+ var o = Object.assign({
+ $ref: this.collection,
+ $id: this.oid
+ }, this.fields);
+ if (this.db != null)
+ o.$db = this.db;
+ return o;
+ };
+ /** @internal */
+ DBRef.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ var o = {
+ $ref: this.collection,
+ $id: this.oid
+ };
+ if (options.legacy) {
+ return o;
+ }
+ if (this.db)
+ o.$db = this.db;
+ o = Object.assign(o, this.fields);
+ return o;
+ };
+ /** @internal */
+ DBRef.fromExtendedJSON = function (doc) {
+ var copy = Object.assign({}, doc);
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new DBRef(doc.$ref, doc.$id, doc.$db, copy);
+ };
+ /** @internal */
+ DBRef.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ DBRef.prototype.inspect = function () {
+ // NOTE: if OID is an ObjectId class it will just print the oid string.
+ var oid = this.oid === undefined || this.oid.toString === undefined ? this.oid : this.oid.toString();
+ return "new DBRef(\"" + this.namespace + "\", new ObjectId(\"" + oid + "\")" + (this.db ? ", \"" + this.db + "\"" : '') + ")";
+ };
+ return DBRef;
+}());
+exports.DBRef = DBRef;
+Object.defineProperty(DBRef.prototype, '_bsontype', { value: 'DBRef' });
+//# sourceMappingURL=db_ref.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/db_ref.js.map b/node_modules/bson/lib/db_ref.js.map
new file mode 100644
index 0000000..da60d52
--- /dev/null
+++ b/node_modules/bson/lib/db_ref.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"db_ref.js","sourceRoot":"","sources":["../src/db_ref.ts"],"names":[],"mappings":";;;AAGA,wCAA8C;AAS9C,gBAAgB;AAChB,SAAgB,WAAW,CAAC,KAAc;IACxC,OAAO,CACL,oBAAY,CAAC,KAAK,CAAC;QACnB,KAAK,CAAC,GAAG,IAAI,IAAI;QACjB,OAAO,KAAK,CAAC,IAAI,KAAK,QAAQ;QAC9B,CAAC,KAAK,CAAC,GAAG,IAAI,IAAI,IAAI,OAAO,KAAK,CAAC,GAAG,KAAK,QAAQ,CAAC,CACrD,CAAC;AACJ,CAAC;AAPD,kCAOC;AAED;;;GAGG;AACH;IAQE;;;;OAIG;IACH,eAAY,UAAkB,EAAE,GAAa,EAAE,EAAW,EAAE,MAAiB;QAC3E,IAAI,CAAC,CAAC,IAAI,YAAY,KAAK,CAAC;YAAE,OAAO,IAAI,KAAK,CAAC,UAAU,EAAE,GAAG,EAAE,EAAE,EAAE,MAAM,CAAC,CAAC;QAE5E,uCAAuC;QACvC,IAAM,KAAK,GAAG,UAAU,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;QACpC,IAAI,KAAK,CAAC,MAAM,KAAK,CAAC,EAAE;YACtB,EAAE,GAAG,KAAK,CAAC,KAAK,EAAE,CAAC;YACnB,oEAAoE;YACpE,UAAU,GAAG,KAAK,CAAC,KAAK,EAAG,CAAC;SAC7B;QAED,IAAI,CAAC,UAAU,GAAG,UAAU,CAAC;QAC7B,IAAI,CAAC,GAAG,GAAG,GAAG,CAAC;QACf,IAAI,CAAC,EAAE,GAAG,EAAE,CAAC;QACb,IAAI,CAAC,MAAM,GAAG,MAAM,IAAI,EAAE,CAAC;IAC7B,CAAC;IAMD,sBAAI,4BAAS;QAJb,0DAA0D;QAC1D,0EAA0E;QAE1E,gBAAgB;aAChB;YACE,OAAO,IAAI,CAAC,UAAU,CAAC;QACzB,CAAC;aAED,UAAc,KAAa;YACzB,IAAI,CAAC,UAAU,GAAG,KAAK,CAAC;QAC1B,CAAC;;;OAJA;IAMD,gBAAgB;IAChB,sBAAM,GAAN;QACE,IAAM,CAAC,GAAG,MAAM,CAAC,MAAM,CACrB;YACE,IAAI,EAAE,IAAI,CAAC,UAAU;YACrB,GAAG,EAAE,IAAI,CAAC,GAAG;SACd,EACD,IAAI,CAAC,MAAM,CACZ,CAAC;QAEF,IAAI,IAAI,CAAC,EAAE,IAAI,IAAI;YAAE,CAAC,CAAC,GAAG,GAAG,IAAI,CAAC,EAAE,CAAC;QACrC,OAAO,CAAC,CAAC;IACX,CAAC;IAED,gBAAgB;IAChB,8BAAc,GAAd,UAAe,OAAsB;QACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAI,CAAC,GAAc;YACjB,IAAI,EAAE,IAAI,CAAC,UAAU;YACrB,GAAG,EAAE,IAAI,CAAC,GAAG;SACd,CAAC;QAEF,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO,CAAC,CAAC;SACV;QAED,IAAI,IAAI,CAAC,EAAE;YAAE,CAAC,CAAC,GAAG,GAAG,IAAI,CAAC,EAAE,CAAC;QAC7B,CAAC,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC,EAAE,IAAI,CAAC,MAAM,CAAC,CAAC;QAClC,OAAO,CAAC,CAAC;IACX,CAAC;IAED,gBAAgB;IACT,sBAAgB,GAAvB,UAAwB,GAAc;QACpC,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,GAAG,CAAuB,CAAC;QAC1D,OAAO,IAAI,CAAC,IAAI,CAAC;QACjB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAI,KAAK,CAAC,GAAG,CAAC,IAAI,EAAE,GAAG,CAAC,GAAG,EAAE,GAAG,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;IACrD,CAAC;IAED,gBAAgB;IAChB,gBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,uBAAO,GAAP;QACE,uEAAuE;QACvE,IAAM,GAAG,GACP,IAAI,CAAC,GAAG,KAAK,SAAS,IAAI,IAAI,CAAC,GAAG,CAAC,QAAQ,KAAK,SAAS,CAAC,CAAC,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,CAAC,IAAI,CAAC,GAAG,CAAC,QAAQ,EAAE,CAAC;QAC7F,OAAO,iBAAc,IAAI,CAAC,SAAS,2BAAoB,GAAG,YACxD,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC,SAAM,IAAI,CAAC,EAAE,OAAG,CAAC,CAAC,CAAC,EAAE,OAC9B,CAAC;IACN,CAAC;IACH,YAAC;AAAD,CAAC,AA/FD,IA+FC;AA/FY,sBAAK;AAiGlB,MAAM,CAAC,cAAc,CAAC,KAAK,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,OAAO,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/decimal128.js b/node_modules/bson/lib/decimal128.js
new file mode 100644
index 0000000..b0890e0
--- /dev/null
+++ b/node_modules/bson/lib/decimal128.js
@@ -0,0 +1,705 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Decimal128 = void 0;
+var buffer_1 = require("buffer");
+var long_1 = require("./long");
+var PARSE_STRING_REGEXP = /^(\+|-)?(\d+|(\d*\.\d*))?(E|e)?([-+])?(\d+)?$/;
+var PARSE_INF_REGEXP = /^(\+|-)?(Infinity|inf)$/i;
+var PARSE_NAN_REGEXP = /^(\+|-)?NaN$/i;
+var EXPONENT_MAX = 6111;
+var EXPONENT_MIN = -6176;
+var EXPONENT_BIAS = 6176;
+var MAX_DIGITS = 34;
+// Nan value bits as 32 bit values (due to lack of longs)
+var NAN_BUFFER = [
+ 0x7c,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+// Infinity value bits 32 bit values (due to lack of longs)
+var INF_NEGATIVE_BUFFER = [
+ 0xf8,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+var INF_POSITIVE_BUFFER = [
+ 0x78,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+var EXPONENT_REGEX = /^([-+])?(\d+)?$/;
+// Extract least significant 5 bits
+var COMBINATION_MASK = 0x1f;
+// Extract least significant 14 bits
+var EXPONENT_MASK = 0x3fff;
+// Value of combination field for Inf
+var COMBINATION_INFINITY = 30;
+// Value of combination field for NaN
+var COMBINATION_NAN = 31;
+// Detect if the value is a digit
+function isDigit(value) {
+ return !isNaN(parseInt(value, 10));
+}
+// Divide two uint128 values
+function divideu128(value) {
+ var DIVISOR = long_1.Long.fromNumber(1000 * 1000 * 1000);
+ var _rem = long_1.Long.fromNumber(0);
+ if (!value.parts[0] && !value.parts[1] && !value.parts[2] && !value.parts[3]) {
+ return { quotient: value, rem: _rem };
+ }
+ for (var i = 0; i <= 3; i++) {
+ // Adjust remainder to match value of next dividend
+ _rem = _rem.shiftLeft(32);
+ // Add the divided to _rem
+ _rem = _rem.add(new long_1.Long(value.parts[i], 0));
+ value.parts[i] = _rem.div(DIVISOR).low;
+ _rem = _rem.modulo(DIVISOR);
+ }
+ return { quotient: value, rem: _rem };
+}
+// Multiply two Long values and return the 128 bit value
+function multiply64x2(left, right) {
+ if (!left && !right) {
+ return { high: long_1.Long.fromNumber(0), low: long_1.Long.fromNumber(0) };
+ }
+ var leftHigh = left.shiftRightUnsigned(32);
+ var leftLow = new long_1.Long(left.getLowBits(), 0);
+ var rightHigh = right.shiftRightUnsigned(32);
+ var rightLow = new long_1.Long(right.getLowBits(), 0);
+ var productHigh = leftHigh.multiply(rightHigh);
+ var productMid = leftHigh.multiply(rightLow);
+ var productMid2 = leftLow.multiply(rightHigh);
+ var productLow = leftLow.multiply(rightLow);
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productMid = new long_1.Long(productMid.getLowBits(), 0)
+ .add(productMid2)
+ .add(productLow.shiftRightUnsigned(32));
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productLow = productMid.shiftLeft(32).add(new long_1.Long(productLow.getLowBits(), 0));
+ // Return the 128 bit result
+ return { high: productHigh, low: productLow };
+}
+function lessThan(left, right) {
+ // Make values unsigned
+ var uhleft = left.high >>> 0;
+ var uhright = right.high >>> 0;
+ // Compare high bits first
+ if (uhleft < uhright) {
+ return true;
+ }
+ else if (uhleft === uhright) {
+ var ulleft = left.low >>> 0;
+ var ulright = right.low >>> 0;
+ if (ulleft < ulright)
+ return true;
+ }
+ return false;
+}
+function invalidErr(string, message) {
+ throw new TypeError("\"" + string + "\" is not a valid Decimal128 string - " + message);
+}
+/**
+ * A class representation of the BSON Decimal128 type.
+ * @public
+ */
+var Decimal128 = /** @class */ (function () {
+ /**
+ * @param bytes - a buffer containing the raw Decimal128 bytes in little endian order,
+ * or a string representation as returned by .toString()
+ */
+ function Decimal128(bytes) {
+ if (!(this instanceof Decimal128))
+ return new Decimal128(bytes);
+ if (typeof bytes === 'string') {
+ this.bytes = Decimal128.fromString(bytes).bytes;
+ }
+ else {
+ this.bytes = bytes;
+ }
+ }
+ /**
+ * Create a Decimal128 instance from a string representation
+ *
+ * @param representation - a numeric string representation.
+ */
+ Decimal128.fromString = function (representation) {
+ // Parse state tracking
+ var isNegative = false;
+ var sawRadix = false;
+ var foundNonZero = false;
+ // Total number of significant digits (no leading or trailing zero)
+ var significantDigits = 0;
+ // Total number of significand digits read
+ var nDigitsRead = 0;
+ // Total number of digits (no leading zeros)
+ var nDigits = 0;
+ // The number of the digits after radix
+ var radixPosition = 0;
+ // The index of the first non-zero in *str*
+ var firstNonZero = 0;
+ // Digits Array
+ var digits = [0];
+ // The number of digits in digits
+ var nDigitsStored = 0;
+ // Insertion pointer for digits
+ var digitsInsert = 0;
+ // The index of the first non-zero digit
+ var firstDigit = 0;
+ // The index of the last digit
+ var lastDigit = 0;
+ // Exponent
+ var exponent = 0;
+ // loop index over array
+ var i = 0;
+ // The high 17 digits of the significand
+ var significandHigh = new long_1.Long(0, 0);
+ // The low 17 digits of the significand
+ var significandLow = new long_1.Long(0, 0);
+ // The biased exponent
+ var biasedExponent = 0;
+ // Read index
+ var index = 0;
+ // Naively prevent against REDOS attacks.
+ // TODO: implementing a custom parsing for this, or refactoring the regex would yield
+ // further gains.
+ if (representation.length >= 7000) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+ // Results
+ var stringMatch = representation.match(PARSE_STRING_REGEXP);
+ var infMatch = representation.match(PARSE_INF_REGEXP);
+ var nanMatch = representation.match(PARSE_NAN_REGEXP);
+ // Validate the string
+ if ((!stringMatch && !infMatch && !nanMatch) || representation.length === 0) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+ if (stringMatch) {
+ // full_match = stringMatch[0]
+ // sign = stringMatch[1]
+ var unsignedNumber = stringMatch[2];
+ // stringMatch[3] is undefined if a whole number (ex "1", 12")
+ // but defined if a number w/ decimal in it (ex "1.0, 12.2")
+ var e = stringMatch[4];
+ var expSign = stringMatch[5];
+ var expNumber = stringMatch[6];
+ // they provided e, but didn't give an exponent number. for ex "1e"
+ if (e && expNumber === undefined)
+ invalidErr(representation, 'missing exponent power');
+ // they provided e, but didn't give a number before it. for ex "e1"
+ if (e && unsignedNumber === undefined)
+ invalidErr(representation, 'missing exponent base');
+ if (e === undefined && (expSign || expNumber)) {
+ invalidErr(representation, 'missing e before exponent');
+ }
+ }
+ // Get the negative or positive sign
+ if (representation[index] === '+' || representation[index] === '-') {
+ isNegative = representation[index++] === '-';
+ }
+ // Check if user passed Infinity or NaN
+ if (!isDigit(representation[index]) && representation[index] !== '.') {
+ if (representation[index] === 'i' || representation[index] === 'I') {
+ return new Decimal128(buffer_1.Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ }
+ else if (representation[index] === 'N') {
+ return new Decimal128(buffer_1.Buffer.from(NAN_BUFFER));
+ }
+ }
+ // Read all the digits
+ while (isDigit(representation[index]) || representation[index] === '.') {
+ if (representation[index] === '.') {
+ if (sawRadix)
+ invalidErr(representation, 'contains multiple periods');
+ sawRadix = true;
+ index = index + 1;
+ continue;
+ }
+ if (nDigitsStored < 34) {
+ if (representation[index] !== '0' || foundNonZero) {
+ if (!foundNonZero) {
+ firstNonZero = nDigitsRead;
+ }
+ foundNonZero = true;
+ // Only store 34 digits
+ digits[digitsInsert++] = parseInt(representation[index], 10);
+ nDigitsStored = nDigitsStored + 1;
+ }
+ }
+ if (foundNonZero)
+ nDigits = nDigits + 1;
+ if (sawRadix)
+ radixPosition = radixPosition + 1;
+ nDigitsRead = nDigitsRead + 1;
+ index = index + 1;
+ }
+ if (sawRadix && !nDigitsRead)
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ // Read exponent if exists
+ if (representation[index] === 'e' || representation[index] === 'E') {
+ // Read exponent digits
+ var match = representation.substr(++index).match(EXPONENT_REGEX);
+ // No digits read
+ if (!match || !match[2])
+ return new Decimal128(buffer_1.Buffer.from(NAN_BUFFER));
+ // Get exponent
+ exponent = parseInt(match[0], 10);
+ // Adjust the index
+ index = index + match[0].length;
+ }
+ // Return not a number
+ if (representation[index])
+ return new Decimal128(buffer_1.Buffer.from(NAN_BUFFER));
+ // Done reading input
+ // Find first non-zero digit in digits
+ firstDigit = 0;
+ if (!nDigitsStored) {
+ firstDigit = 0;
+ lastDigit = 0;
+ digits[0] = 0;
+ nDigits = 1;
+ nDigitsStored = 1;
+ significantDigits = 0;
+ }
+ else {
+ lastDigit = nDigitsStored - 1;
+ significantDigits = nDigits;
+ if (significantDigits !== 1) {
+ while (representation[firstNonZero + significantDigits - 1] === '0') {
+ significantDigits = significantDigits - 1;
+ }
+ }
+ }
+ // Normalization of exponent
+ // Correct exponent based on radix position, and shift significand as needed
+ // to represent user input
+ // Overflow prevention
+ if (exponent <= radixPosition && radixPosition - exponent > 1 << 14) {
+ exponent = EXPONENT_MIN;
+ }
+ else {
+ exponent = exponent - radixPosition;
+ }
+ // Attempt to normalize the exponent
+ while (exponent > EXPONENT_MAX) {
+ // Shift exponent to significand and decrease
+ lastDigit = lastDigit + 1;
+ if (lastDigit - firstDigit > MAX_DIGITS) {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ var digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ exponent = exponent - 1;
+ }
+ while (exponent < EXPONENT_MIN || nDigitsStored < nDigits) {
+ // Shift last digit. can only do this if < significant digits than # stored.
+ if (lastDigit === 0 && significantDigits < nDigitsStored) {
+ exponent = EXPONENT_MIN;
+ significantDigits = 0;
+ break;
+ }
+ if (nDigitsStored < nDigits) {
+ // adjust to match digits not stored
+ nDigits = nDigits - 1;
+ }
+ else {
+ // adjust to round
+ lastDigit = lastDigit - 1;
+ }
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ }
+ else {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ var digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ }
+ // Round
+ // We've normalized the exponent, but might still need to round.
+ if (lastDigit - firstDigit + 1 < significantDigits) {
+ var endOfString = nDigitsRead;
+ // If we have seen a radix point, 'string' is 1 longer than we have
+ // documented with ndigits_read, so inc the position of the first nonzero
+ // digit and the position that digits are read to.
+ if (sawRadix) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ // if negative, we need to increment again to account for - sign at start.
+ if (isNegative) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ var roundDigit = parseInt(representation[firstNonZero + lastDigit + 1], 10);
+ var roundBit = 0;
+ if (roundDigit >= 5) {
+ roundBit = 1;
+ if (roundDigit === 5) {
+ roundBit = digits[lastDigit] % 2 === 1 ? 1 : 0;
+ for (i = firstNonZero + lastDigit + 2; i < endOfString; i++) {
+ if (parseInt(representation[i], 10)) {
+ roundBit = 1;
+ break;
+ }
+ }
+ }
+ }
+ if (roundBit) {
+ var dIdx = lastDigit;
+ for (; dIdx >= 0; dIdx--) {
+ if (++digits[dIdx] > 9) {
+ digits[dIdx] = 0;
+ // overflowed most significant digit
+ if (dIdx === 0) {
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ digits[dIdx] = 1;
+ }
+ else {
+ return new Decimal128(buffer_1.Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ }
+ }
+ }
+ }
+ }
+ }
+ // Encode significand
+ // The high 17 digits of the significand
+ significandHigh = long_1.Long.fromNumber(0);
+ // The low 17 digits of the significand
+ significandLow = long_1.Long.fromNumber(0);
+ // read a zero
+ if (significantDigits === 0) {
+ significandHigh = long_1.Long.fromNumber(0);
+ significandLow = long_1.Long.fromNumber(0);
+ }
+ else if (lastDigit - firstDigit < 17) {
+ var dIdx = firstDigit;
+ significandLow = long_1.Long.fromNumber(digits[dIdx++]);
+ significandHigh = new long_1.Long(0, 0);
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(long_1.Long.fromNumber(10));
+ significandLow = significandLow.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ }
+ else {
+ var dIdx = firstDigit;
+ significandHigh = long_1.Long.fromNumber(digits[dIdx++]);
+ for (; dIdx <= lastDigit - 17; dIdx++) {
+ significandHigh = significandHigh.multiply(long_1.Long.fromNumber(10));
+ significandHigh = significandHigh.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ significandLow = long_1.Long.fromNumber(digits[dIdx++]);
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(long_1.Long.fromNumber(10));
+ significandLow = significandLow.add(long_1.Long.fromNumber(digits[dIdx]));
+ }
+ }
+ var significand = multiply64x2(significandHigh, long_1.Long.fromString('100000000000000000'));
+ significand.low = significand.low.add(significandLow);
+ if (lessThan(significand.low, significandLow)) {
+ significand.high = significand.high.add(long_1.Long.fromNumber(1));
+ }
+ // Biased exponent
+ biasedExponent = exponent + EXPONENT_BIAS;
+ var dec = { low: long_1.Long.fromNumber(0), high: long_1.Long.fromNumber(0) };
+ // Encode combination, exponent, and significand.
+ if (significand.high.shiftRightUnsigned(49).and(long_1.Long.fromNumber(1)).equals(long_1.Long.fromNumber(1))) {
+ // Encode '11' into bits 1 to 3
+ dec.high = dec.high.or(long_1.Long.fromNumber(0x3).shiftLeft(61));
+ dec.high = dec.high.or(long_1.Long.fromNumber(biasedExponent).and(long_1.Long.fromNumber(0x3fff).shiftLeft(47)));
+ dec.high = dec.high.or(significand.high.and(long_1.Long.fromNumber(0x7fffffffffff)));
+ }
+ else {
+ dec.high = dec.high.or(long_1.Long.fromNumber(biasedExponent & 0x3fff).shiftLeft(49));
+ dec.high = dec.high.or(significand.high.and(long_1.Long.fromNumber(0x1ffffffffffff)));
+ }
+ dec.low = significand.low;
+ // Encode sign
+ if (isNegative) {
+ dec.high = dec.high.or(long_1.Long.fromString('9223372036854775808'));
+ }
+ // Encode into a buffer
+ var buffer = buffer_1.Buffer.alloc(16);
+ index = 0;
+ // Encode the low 64 bits of the decimal
+ // Encode low bits
+ buffer[index++] = dec.low.low & 0xff;
+ buffer[index++] = (dec.low.low >> 8) & 0xff;
+ buffer[index++] = (dec.low.low >> 16) & 0xff;
+ buffer[index++] = (dec.low.low >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = dec.low.high & 0xff;
+ buffer[index++] = (dec.low.high >> 8) & 0xff;
+ buffer[index++] = (dec.low.high >> 16) & 0xff;
+ buffer[index++] = (dec.low.high >> 24) & 0xff;
+ // Encode the high 64 bits of the decimal
+ // Encode low bits
+ buffer[index++] = dec.high.low & 0xff;
+ buffer[index++] = (dec.high.low >> 8) & 0xff;
+ buffer[index++] = (dec.high.low >> 16) & 0xff;
+ buffer[index++] = (dec.high.low >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = dec.high.high & 0xff;
+ buffer[index++] = (dec.high.high >> 8) & 0xff;
+ buffer[index++] = (dec.high.high >> 16) & 0xff;
+ buffer[index++] = (dec.high.high >> 24) & 0xff;
+ // Return the new Decimal128
+ return new Decimal128(buffer);
+ };
+ /** Create a string representation of the raw Decimal128 value */
+ Decimal128.prototype.toString = function () {
+ // Note: bits in this routine are referred to starting at 0,
+ // from the sign bit, towards the coefficient.
+ // decoded biased exponent (14 bits)
+ var biased_exponent;
+ // the number of significand digits
+ var significand_digits = 0;
+ // the base-10 digits in the significand
+ var significand = new Array(36);
+ for (var i = 0; i < significand.length; i++)
+ significand[i] = 0;
+ // read pointer into significand
+ var index = 0;
+ // true if the number is zero
+ var is_zero = false;
+ // the most significant significand bits (50-46)
+ var significand_msb;
+ // temporary storage for significand decoding
+ var significand128 = { parts: [0, 0, 0, 0] };
+ // indexing variables
+ var j, k;
+ // Output string
+ var string = [];
+ // Unpack index
+ index = 0;
+ // Buffer reference
+ var buffer = this.bytes;
+ // Unpack the low 64bits into a long
+ // bits 96 - 127
+ var low = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 64 - 95
+ var midl = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Unpack the high 64bits into a long
+ // bits 32 - 63
+ var midh = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 0 - 31
+ var high = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Unpack index
+ index = 0;
+ // Create the state of the decimal
+ var dec = {
+ low: new long_1.Long(low, midl),
+ high: new long_1.Long(midh, high)
+ };
+ if (dec.high.lessThan(long_1.Long.ZERO)) {
+ string.push('-');
+ }
+ // Decode combination field and exponent
+ // bits 1 - 5
+ var combination = (high >> 26) & COMBINATION_MASK;
+ if (combination >> 3 === 3) {
+ // Check for 'special' values
+ if (combination === COMBINATION_INFINITY) {
+ return string.join('') + 'Infinity';
+ }
+ else if (combination === COMBINATION_NAN) {
+ return 'NaN';
+ }
+ else {
+ biased_exponent = (high >> 15) & EXPONENT_MASK;
+ significand_msb = 0x08 + ((high >> 14) & 0x01);
+ }
+ }
+ else {
+ significand_msb = (high >> 14) & 0x07;
+ biased_exponent = (high >> 17) & EXPONENT_MASK;
+ }
+ // unbiased exponent
+ var exponent = biased_exponent - EXPONENT_BIAS;
+ // Create string of significand digits
+ // Convert the 114-bit binary number represented by
+ // (significand_high, significand_low) to at most 34 decimal
+ // digits through modulo and division.
+ significand128.parts[0] = (high & 0x3fff) + ((significand_msb & 0xf) << 14);
+ significand128.parts[1] = midh;
+ significand128.parts[2] = midl;
+ significand128.parts[3] = low;
+ if (significand128.parts[0] === 0 &&
+ significand128.parts[1] === 0 &&
+ significand128.parts[2] === 0 &&
+ significand128.parts[3] === 0) {
+ is_zero = true;
+ }
+ else {
+ for (k = 3; k >= 0; k--) {
+ var least_digits = 0;
+ // Perform the divide
+ var result = divideu128(significand128);
+ significand128 = result.quotient;
+ least_digits = result.rem.low;
+ // We now have the 9 least significant digits (in base 2).
+ // Convert and output to string.
+ if (!least_digits)
+ continue;
+ for (j = 8; j >= 0; j--) {
+ // significand[k * 9 + j] = Math.round(least_digits % 10);
+ significand[k * 9 + j] = least_digits % 10;
+ // least_digits = Math.round(least_digits / 10);
+ least_digits = Math.floor(least_digits / 10);
+ }
+ }
+ }
+ // Output format options:
+ // Scientific - [-]d.dddE(+/-)dd or [-]dE(+/-)dd
+ // Regular - ddd.ddd
+ if (is_zero) {
+ significand_digits = 1;
+ significand[index] = 0;
+ }
+ else {
+ significand_digits = 36;
+ while (!significand[index]) {
+ significand_digits = significand_digits - 1;
+ index = index + 1;
+ }
+ }
+ // the exponent if scientific notation is used
+ var scientific_exponent = significand_digits - 1 + exponent;
+ // The scientific exponent checks are dictated by the string conversion
+ // specification and are somewhat arbitrary cutoffs.
+ //
+ // We must check exponent > 0, because if this is the case, the number
+ // has trailing zeros. However, we *cannot* output these trailing zeros,
+ // because doing so would change the precision of the value, and would
+ // change stored data if the string converted number is round tripped.
+ if (scientific_exponent >= 34 || scientific_exponent <= -7 || exponent > 0) {
+ // Scientific format
+ // if there are too many significant digits, we should just be treating numbers
+ // as + or - 0 and using the non-scientific exponent (this is for the "invalid
+ // representation should be treated as 0/-0" spec cases in decimal128-1.json)
+ if (significand_digits > 34) {
+ string.push("" + 0);
+ if (exponent > 0)
+ string.push('E+' + exponent);
+ else if (exponent < 0)
+ string.push('E' + exponent);
+ return string.join('');
+ }
+ string.push("" + significand[index++]);
+ significand_digits = significand_digits - 1;
+ if (significand_digits) {
+ string.push('.');
+ }
+ for (var i = 0; i < significand_digits; i++) {
+ string.push("" + significand[index++]);
+ }
+ // Exponent
+ string.push('E');
+ if (scientific_exponent > 0) {
+ string.push('+' + scientific_exponent);
+ }
+ else {
+ string.push("" + scientific_exponent);
+ }
+ }
+ else {
+ // Regular format with no decimal place
+ if (exponent >= 0) {
+ for (var i = 0; i < significand_digits; i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ else {
+ var radix_position = significand_digits + exponent;
+ // non-zero digits before radix
+ if (radix_position > 0) {
+ for (var i = 0; i < radix_position; i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ else {
+ string.push('0');
+ }
+ string.push('.');
+ // add leading zeros after radix
+ while (radix_position++ < 0) {
+ string.push('0');
+ }
+ for (var i = 0; i < significand_digits - Math.max(radix_position - 1, 0); i++) {
+ string.push("" + significand[index++]);
+ }
+ }
+ }
+ return string.join('');
+ };
+ Decimal128.prototype.toJSON = function () {
+ return { $numberDecimal: this.toString() };
+ };
+ /** @internal */
+ Decimal128.prototype.toExtendedJSON = function () {
+ return { $numberDecimal: this.toString() };
+ };
+ /** @internal */
+ Decimal128.fromExtendedJSON = function (doc) {
+ return Decimal128.fromString(doc.$numberDecimal);
+ };
+ /** @internal */
+ Decimal128.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Decimal128.prototype.inspect = function () {
+ return "new Decimal128(\"" + this.toString() + "\")";
+ };
+ return Decimal128;
+}());
+exports.Decimal128 = Decimal128;
+Object.defineProperty(Decimal128.prototype, '_bsontype', { value: 'Decimal128' });
+//# sourceMappingURL=decimal128.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/decimal128.js.map b/node_modules/bson/lib/decimal128.js.map
new file mode 100644
index 0000000..8d2358b
--- /dev/null
+++ b/node_modules/bson/lib/decimal128.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"decimal128.js","sourceRoot":"","sources":["../src/decimal128.ts"],"names":[],"mappings":";;;AAAA,iCAAgC;AAChC,+BAA8B;AAE9B,IAAM,mBAAmB,GAAG,+CAA+C,CAAC;AAC5E,IAAM,gBAAgB,GAAG,0BAA0B,CAAC;AACpD,IAAM,gBAAgB,GAAG,eAAe,CAAC;AAEzC,IAAM,YAAY,GAAG,IAAI,CAAC;AAC1B,IAAM,YAAY,GAAG,CAAC,IAAI,CAAC;AAC3B,IAAM,aAAa,GAAG,IAAI,CAAC;AAC3B,IAAM,UAAU,GAAG,EAAE,CAAC;AAEtB,yDAAyD;AACzD,IAAM,UAAU,GAAG;IACjB,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;CACL,CAAC,OAAO,EAAE,CAAC;AACZ,2DAA2D;AAC3D,IAAM,mBAAmB,GAAG;IAC1B,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;CACL,CAAC,OAAO,EAAE,CAAC;AACZ,IAAM,mBAAmB,GAAG;IAC1B,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;IACJ,IAAI;CACL,CAAC,OAAO,EAAE,CAAC;AAEZ,IAAM,cAAc,GAAG,iBAAiB,CAAC;AAEzC,mCAAmC;AACnC,IAAM,gBAAgB,GAAG,IAAI,CAAC;AAC9B,oCAAoC;AACpC,IAAM,aAAa,GAAG,MAAM,CAAC;AAC7B,qCAAqC;AACrC,IAAM,oBAAoB,GAAG,EAAE,CAAC;AAChC,qCAAqC;AACrC,IAAM,eAAe,GAAG,EAAE,CAAC;AAE3B,iCAAiC;AACjC,SAAS,OAAO,CAAC,KAAa;IAC5B,OAAO,CAAC,KAAK,CAAC,QAAQ,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,CAAC;AACrC,CAAC;AAED,4BAA4B;AAC5B,SAAS,UAAU,CAAC,KAAkD;IACpE,IAAM,OAAO,GAAG,WAAI,CAAC,UAAU,CAAC,IAAI,GAAG,IAAI,GAAG,IAAI,CAAC,CAAC;IACpD,IAAI,IAAI,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;IAE9B,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE;QAC5E,OAAO,EAAE,QAAQ,EAAE,KAAK,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;KACvC;IAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;QAC3B,mDAAmD;QACnD,IAAI,GAAG,IAAI,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC;QAC1B,0BAA0B;QAC1B,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC,IAAI,WAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;QAC7C,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC;QACvC,IAAI,GAAG,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;KAC7B;IAED,OAAO,EAAE,QAAQ,EAAE,KAAK,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;AACxC,CAAC;AAED,wDAAwD;AACxD,SAAS,YAAY,CAAC,IAAU,EAAE,KAAW;IAC3C,IAAI,CAAC,IAAI,IAAI,CAAC,KAAK,EAAE;QACnB,OAAO,EAAE,IAAI,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,GAAG,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,CAAC;KAC9D;IAED,IAAM,QAAQ,GAAG,IAAI,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC;IAC7C,IAAM,OAAO,GAAG,IAAI,WAAI,CAAC,IAAI,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC;IAC/C,IAAM,SAAS,GAAG,KAAK,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC;IAC/C,IAAM,QAAQ,GAAG,IAAI,WAAI,CAAC,KAAK,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC;IAEjD,IAAI,WAAW,GAAG,QAAQ,CAAC,QAAQ,CAAC,SAAS,CAAC,CAAC;IAC/C,IAAI,UAAU,GAAG,QAAQ,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;IAC7C,IAAM,WAAW,GAAG,OAAO,CAAC,QAAQ,CAAC,SAAS,CAAC,CAAC;IAChD,IAAI,UAAU,GAAG,OAAO,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC;IAE5C,WAAW,GAAG,WAAW,CAAC,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;IACjE,UAAU,GAAG,IAAI,WAAI,CAAC,UAAU,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC;SAC9C,GAAG,CAAC,WAAW,CAAC;SAChB,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;IAE1C,WAAW,GAAG,WAAW,CAAC,GAAG,CAAC,UAAU,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,CAAC;IACjE,UAAU,GAAG,UAAU,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,GAAG,CAAC,IAAI,WAAI,CAAC,UAAU,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC,CAAC;IAEhF,4BAA4B;IAC5B,OAAO,EAAE,IAAI,EAAE,WAAW,EAAE,GAAG,EAAE,UAAU,EAAE,CAAC;AAChD,CAAC;AAED,SAAS,QAAQ,CAAC,IAAU,EAAE,KAAW;IACvC,uBAAuB;IACvB,IAAM,MAAM,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC;IAC/B,IAAM,OAAO,GAAG,KAAK,CAAC,IAAI,KAAK,CAAC,CAAC;IAEjC,0BAA0B;IAC1B,IAAI,MAAM,GAAG,OAAO,EAAE;QACpB,OAAO,IAAI,CAAC;KACb;SAAM,IAAI,MAAM,KAAK,OAAO,EAAE;QAC7B,IAAM,MAAM,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;QAC9B,IAAM,OAAO,GAAG,KAAK,CAAC,GAAG,KAAK,CAAC,CAAC;QAChC,IAAI,MAAM,GAAG,OAAO;YAAE,OAAO,IAAI,CAAC;KACnC;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,UAAU,CAAC,MAAc,EAAE,OAAe;IACjD,MAAM,IAAI,SAAS,CAAC,OAAI,MAAM,8CAAwC,OAAS,CAAC,CAAC;AACnF,CAAC;AAOD;;;GAGG;AACH;IAKE;;;OAGG;IACH,oBAAY,KAAsB;QAChC,IAAI,CAAC,CAAC,IAAI,YAAY,UAAU,CAAC;YAAE,OAAO,IAAI,UAAU,CAAC,KAAK,CAAC,CAAC;QAEhE,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YAC7B,IAAI,CAAC,KAAK,GAAG,UAAU,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,KAAK,CAAC;SACjD;aAAM;YACL,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;SACpB;IACH,CAAC;IAED;;;;OAIG;IACI,qBAAU,GAAjB,UAAkB,cAAsB;QACtC,uBAAuB;QACvB,IAAI,UAAU,GAAG,KAAK,CAAC;QACvB,IAAI,QAAQ,GAAG,KAAK,CAAC;QACrB,IAAI,YAAY,GAAG,KAAK,CAAC;QAEzB,mEAAmE;QACnE,IAAI,iBAAiB,GAAG,CAAC,CAAC;QAC1B,0CAA0C;QAC1C,IAAI,WAAW,GAAG,CAAC,CAAC;QACpB,4CAA4C;QAC5C,IAAI,OAAO,GAAG,CAAC,CAAC;QAChB,uCAAuC;QACvC,IAAI,aAAa,GAAG,CAAC,CAAC;QACtB,2CAA2C;QAC3C,IAAI,YAAY,GAAG,CAAC,CAAC;QAErB,eAAe;QACf,IAAM,MAAM,GAAG,CAAC,CAAC,CAAC,CAAC;QACnB,iCAAiC;QACjC,IAAI,aAAa,GAAG,CAAC,CAAC;QACtB,+BAA+B;QAC/B,IAAI,YAAY,GAAG,CAAC,CAAC;QACrB,wCAAwC;QACxC,IAAI,UAAU,GAAG,CAAC,CAAC;QACnB,8BAA8B;QAC9B,IAAI,SAAS,GAAG,CAAC,CAAC;QAElB,WAAW;QACX,IAAI,QAAQ,GAAG,CAAC,CAAC;QACjB,wBAAwB;QACxB,IAAI,CAAC,GAAG,CAAC,CAAC;QACV,wCAAwC;QACxC,IAAI,eAAe,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;QACrC,uCAAuC;QACvC,IAAI,cAAc,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;QACpC,sBAAsB;QACtB,IAAI,cAAc,GAAG,CAAC,CAAC;QAEvB,aAAa;QACb,IAAI,KAAK,GAAG,CAAC,CAAC;QAEd,yCAAyC;QACzC,qFAAqF;QACrF,uBAAuB;QACvB,IAAI,cAAc,CAAC,MAAM,IAAI,IAAI,EAAE;YACjC,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;SAC7E;QAED,UAAU;QACV,IAAM,WAAW,GAAG,cAAc,CAAC,KAAK,CAAC,mBAAmB,CAAC,CAAC;QAC9D,IAAM,QAAQ,GAAG,cAAc,CAAC,KAAK,CAAC,gBAAgB,CAAC,CAAC;QACxD,IAAM,QAAQ,GAAG,cAAc,CAAC,KAAK,CAAC,gBAAgB,CAAC,CAAC;QAExD,sBAAsB;QACtB,IAAI,CAAC,CAAC,WAAW,IAAI,CAAC,QAAQ,IAAI,CAAC,QAAQ,CAAC,IAAI,cAAc,CAAC,MAAM,KAAK,CAAC,EAAE;YAC3E,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;SAC7E;QAED,IAAI,WAAW,EAAE;YACf,8BAA8B;YAC9B,wBAAwB;YAExB,IAAM,cAAc,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;YACtC,8DAA8D;YAC9D,4DAA4D;YAE5D,IAAM,CAAC,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;YACzB,IAAM,OAAO,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;YAC/B,IAAM,SAAS,GAAG,WAAW,CAAC,CAAC,CAAC,CAAC;YAEjC,mEAAmE;YACnE,IAAI,CAAC,IAAI,SAAS,KAAK,SAAS;gBAAE,UAAU,CAAC,cAAc,EAAE,wBAAwB,CAAC,CAAC;YAEvF,mEAAmE;YACnE,IAAI,CAAC,IAAI,cAAc,KAAK,SAAS;gBAAE,UAAU,CAAC,cAAc,EAAE,uBAAuB,CAAC,CAAC;YAE3F,IAAI,CAAC,KAAK,SAAS,IAAI,CAAC,OAAO,IAAI,SAAS,CAAC,EAAE;gBAC7C,UAAU,CAAC,cAAc,EAAE,2BAA2B,CAAC,CAAC;aACzD;SACF;QAED,oCAAoC;QACpC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;YAClE,UAAU,GAAG,cAAc,CAAC,KAAK,EAAE,CAAC,KAAK,GAAG,CAAC;SAC9C;QAED,uCAAuC;QACvC,IAAI,CAAC,OAAO,CAAC,cAAc,CAAC,KAAK,CAAC,CAAC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;YACpE,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;gBAClE,OAAO,IAAI,UAAU,CAAC,eAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC,mBAAmB,CAAC,CAAC,CAAC,mBAAmB,CAAC,CAAC,CAAC;aAC5F;iBAAM,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;gBACxC,OAAO,IAAI,UAAU,CAAC,eAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;aAChD;SACF;QAED,sBAAsB;QACtB,OAAO,OAAO,CAAC,cAAc,CAAC,KAAK,CAAC,CAAC,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;YACtE,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;gBACjC,IAAI,QAAQ;oBAAE,UAAU,CAAC,cAAc,EAAE,2BAA2B,CAAC,CAAC;gBAEtE,QAAQ,GAAG,IAAI,CAAC;gBAChB,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;gBAClB,SAAS;aACV;YAED,IAAI,aAAa,GAAG,EAAE,EAAE;gBACtB,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,YAAY,EAAE;oBACjD,IAAI,CAAC,YAAY,EAAE;wBACjB,YAAY,GAAG,WAAW,CAAC;qBAC5B;oBAED,YAAY,GAAG,IAAI,CAAC;oBAEpB,uBAAuB;oBACvB,MAAM,CAAC,YAAY,EAAE,CAAC,GAAG,QAAQ,CAAC,cAAc,CAAC,KAAK,CAAC,EAAE,EAAE,CAAC,CAAC;oBAC7D,aAAa,GAAG,aAAa,GAAG,CAAC,CAAC;iBACnC;aACF;YAED,IAAI,YAAY;gBAAE,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC;YACxC,IAAI,QAAQ;gBAAE,aAAa,GAAG,aAAa,GAAG,CAAC,CAAC;YAEhD,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;YAC9B,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;SACnB;QAED,IAAI,QAAQ,IAAI,CAAC,WAAW;YAC1B,MAAM,IAAI,SAAS,CAAC,EAAE,GAAG,cAAc,GAAG,gCAAgC,CAAC,CAAC;QAE9E,0BAA0B;QAC1B,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,IAAI,cAAc,CAAC,KAAK,CAAC,KAAK,GAAG,EAAE;YAClE,uBAAuB;YACvB,IAAM,KAAK,GAAG,cAAc,CAAC,MAAM,CAAC,EAAE,KAAK,CAAC,CAAC,KAAK,CAAC,cAAc,CAAC,CAAC;YAEnE,iBAAiB;YACjB,IAAI,CAAC,KAAK,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC;gBAAE,OAAO,IAAI,UAAU,CAAC,eAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;YAExE,eAAe;YACf,QAAQ,GAAG,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;YAElC,mBAAmB;YACnB,KAAK,GAAG,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC;SACjC;QAED,sBAAsB;QACtB,IAAI,cAAc,CAAC,KAAK,CAAC;YAAE,OAAO,IAAI,UAAU,CAAC,eAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC;QAE1E,qBAAqB;QACrB,sCAAsC;QACtC,UAAU,GAAG,CAAC,CAAC;QAEf,IAAI,CAAC,aAAa,EAAE;YAClB,UAAU,GAAG,CAAC,CAAC;YACf,SAAS,GAAG,CAAC,CAAC;YACd,MAAM,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;YACd,OAAO,GAAG,CAAC,CAAC;YACZ,aAAa,GAAG,CAAC,CAAC;YAClB,iBAAiB,GAAG,CAAC,CAAC;SACvB;aAAM;YACL,SAAS,GAAG,aAAa,GAAG,CAAC,CAAC;YAC9B,iBAAiB,GAAG,OAAO,CAAC;YAC5B,IAAI,iBAAiB,KAAK,CAAC,EAAE;gBAC3B,OAAO,cAAc,CAAC,YAAY,GAAG,iBAAiB,GAAG,CAAC,CAAC,KAAK,GAAG,EAAE;oBACnE,iBAAiB,GAAG,iBAAiB,GAAG,CAAC,CAAC;iBAC3C;aACF;SACF;QAED,4BAA4B;QAC5B,4EAA4E;QAC5E,0BAA0B;QAE1B,sBAAsB;QACtB,IAAI,QAAQ,IAAI,aAAa,IAAI,aAAa,GAAG,QAAQ,GAAG,CAAC,IAAI,EAAE,EAAE;YACnE,QAAQ,GAAG,YAAY,CAAC;SACzB;aAAM;YACL,QAAQ,GAAG,QAAQ,GAAG,aAAa,CAAC;SACrC;QAED,oCAAoC;QACpC,OAAO,QAAQ,GAAG,YAAY,EAAE;YAC9B,6CAA6C;YAC7C,SAAS,GAAG,SAAS,GAAG,CAAC,CAAC;YAE1B,IAAI,SAAS,GAAG,UAAU,GAAG,UAAU,EAAE;gBACvC,+DAA+D;gBAC/D,IAAM,YAAY,GAAG,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;gBACrC,IAAI,YAAY,CAAC,KAAK,CAAC,MAAM,CAAC,EAAE;oBAC9B,QAAQ,GAAG,YAAY,CAAC;oBACxB,MAAM;iBACP;gBAED,UAAU,CAAC,cAAc,EAAE,UAAU,CAAC,CAAC;aACxC;YACD,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;SACzB;QAED,OAAO,QAAQ,GAAG,YAAY,IAAI,aAAa,GAAG,OAAO,EAAE;YACzD,4EAA4E;YAC5E,IAAI,SAAS,KAAK,CAAC,IAAI,iBAAiB,GAAG,aAAa,EAAE;gBACxD,QAAQ,GAAG,YAAY,CAAC;gBACxB,iBAAiB,GAAG,CAAC,CAAC;gBACtB,MAAM;aACP;YAED,IAAI,aAAa,GAAG,OAAO,EAAE;gBAC3B,oCAAoC;gBACpC,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC;aACvB;iBAAM;gBACL,kBAAkB;gBAClB,SAAS,GAAG,SAAS,GAAG,CAAC,CAAC;aAC3B;YAED,IAAI,QAAQ,GAAG,YAAY,EAAE;gBAC3B,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;aACzB;iBAAM;gBACL,+DAA+D;gBAC/D,IAAM,YAAY,GAAG,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;gBACrC,IAAI,YAAY,CAAC,KAAK,CAAC,MAAM,CAAC,EAAE;oBAC9B,QAAQ,GAAG,YAAY,CAAC;oBACxB,MAAM;iBACP;gBACD,UAAU,CAAC,cAAc,EAAE,UAAU,CAAC,CAAC;aACxC;SACF;QAED,QAAQ;QACR,gEAAgE;QAChE,IAAI,SAAS,GAAG,UAAU,GAAG,CAAC,GAAG,iBAAiB,EAAE;YAClD,IAAI,WAAW,GAAG,WAAW,CAAC;YAE9B,mEAAmE;YACnE,yEAAyE;YACzE,kDAAkD;YAClD,IAAI,QAAQ,EAAE;gBACZ,YAAY,GAAG,YAAY,GAAG,CAAC,CAAC;gBAChC,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;aAC/B;YACD,0EAA0E;YAC1E,IAAI,UAAU,EAAE;gBACd,YAAY,GAAG,YAAY,GAAG,CAAC,CAAC;gBAChC,WAAW,GAAG,WAAW,GAAG,CAAC,CAAC;aAC/B;YAED,IAAM,UAAU,GAAG,QAAQ,CAAC,cAAc,CAAC,YAAY,GAAG,SAAS,GAAG,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC;YAC9E,IAAI,QAAQ,GAAG,CAAC,CAAC;YAEjB,IAAI,UAAU,IAAI,CAAC,EAAE;gBACnB,QAAQ,GAAG,CAAC,CAAC;gBACb,IAAI,UAAU,KAAK,CAAC,EAAE;oBACpB,QAAQ,GAAG,MAAM,CAAC,SAAS,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBAC/C,KAAK,CAAC,GAAG,YAAY,GAAG,SAAS,GAAG,CAAC,EAAE,CAAC,GAAG,WAAW,EAAE,CAAC,EAAE,EAAE;wBAC3D,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE;4BACnC,QAAQ,GAAG,CAAC,CAAC;4BACb,MAAM;yBACP;qBACF;iBACF;aACF;YAED,IAAI,QAAQ,EAAE;gBACZ,IAAI,IAAI,GAAG,SAAS,CAAC;gBAErB,OAAO,IAAI,IAAI,CAAC,EAAE,IAAI,EAAE,EAAE;oBACxB,IAAI,EAAE,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,EAAE;wBACtB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;wBAEjB,oCAAoC;wBACpC,IAAI,IAAI,KAAK,CAAC,EAAE;4BACd,IAAI,QAAQ,GAAG,YAAY,EAAE;gCAC3B,QAAQ,GAAG,QAAQ,GAAG,CAAC,CAAC;gCACxB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;6BAClB;iCAAM;gCACL,OAAO,IAAI,UAAU,CACnB,eAAM,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC,CAAC,mBAAmB,CAAC,CAAC,CAAC,mBAAmB,CAAC,CACpE,CAAC;6BACH;yBACF;qBACF;iBACF;aACF;SACF;QAED,qBAAqB;QACrB,wCAAwC;QACxC,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;QACrC,uCAAuC;QACvC,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;QAEpC,cAAc;QACd,IAAI,iBAAiB,KAAK,CAAC,EAAE;YAC3B,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;YACrC,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;SACrC;aAAM,IAAI,SAAS,GAAG,UAAU,GAAG,EAAE,EAAE;YACtC,IAAI,IAAI,GAAG,UAAU,CAAC;YACtB,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;YACjD,eAAe,GAAG,IAAI,WAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;YAEjC,OAAO,IAAI,IAAI,SAAS,EAAE,IAAI,EAAE,EAAE;gBAChC,cAAc,GAAG,cAAc,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;gBAC9D,cAAc,GAAG,cAAc,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;aACpE;SACF;aAAM;YACL,IAAI,IAAI,GAAG,UAAU,CAAC;YACtB,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;YAElD,OAAO,IAAI,IAAI,SAAS,GAAG,EAAE,EAAE,IAAI,EAAE,EAAE;gBACrC,eAAe,GAAG,eAAe,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;gBAChE,eAAe,GAAG,eAAe,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;aACtE;YAED,cAAc,GAAG,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;YAEjD,OAAO,IAAI,IAAI,SAAS,EAAE,IAAI,EAAE,EAAE;gBAChC,cAAc,GAAG,cAAc,CAAC,QAAQ,CAAC,WAAI,CAAC,UAAU,CAAC,EAAE,CAAC,CAAC,CAAC;gBAC9D,cAAc,GAAG,cAAc,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;aACpE;SACF;QAED,IAAM,WAAW,GAAG,YAAY,CAAC,eAAe,EAAE,WAAI,CAAC,UAAU,CAAC,oBAAoB,CAAC,CAAC,CAAC;QACzF,WAAW,CAAC,GAAG,GAAG,WAAW,CAAC,GAAG,CAAC,GAAG,CAAC,cAAc,CAAC,CAAC;QAEtD,IAAI,QAAQ,CAAC,WAAW,CAAC,GAAG,EAAE,cAAc,CAAC,EAAE;YAC7C,WAAW,CAAC,IAAI,GAAG,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC;SAC7D;QAED,kBAAkB;QAClB,cAAc,GAAG,QAAQ,GAAG,aAAa,CAAC;QAC1C,IAAM,GAAG,GAAG,EAAE,GAAG,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,IAAI,EAAE,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,EAAE,CAAC;QAElE,iDAAiD;QACjD,IACE,WAAW,CAAC,IAAI,CAAC,kBAAkB,CAAC,EAAE,CAAC,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,WAAI,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,EAC1F;YACA,+BAA+B;YAC/B,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,GAAG,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAAC;YAC3D,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CACpB,WAAI,CAAC,UAAU,CAAC,cAAc,CAAC,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAC3E,CAAC;YACF,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,cAAc,CAAC,CAAC,CAAC,CAAC;SAC/E;aAAM;YACL,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,cAAc,GAAG,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,CAAC,CAAC,CAAC;YAC/E,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,IAAI,CAAC,GAAG,CAAC,WAAI,CAAC,UAAU,CAAC,eAAe,CAAC,CAAC,CAAC,CAAC;SAChF;QAED,GAAG,CAAC,GAAG,GAAG,WAAW,CAAC,GAAG,CAAC;QAE1B,cAAc;QACd,IAAI,UAAU,EAAE;YACd,GAAG,CAAC,IAAI,GAAG,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,WAAI,CAAC,UAAU,CAAC,qBAAqB,CAAC,CAAC,CAAC;SAChE;QAED,uBAAuB;QACvB,IAAM,MAAM,GAAG,eAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;QAChC,KAAK,GAAG,CAAC,CAAC;QAEV,wCAAwC;QACxC,kBAAkB;QAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,GAAG,CAAC,GAAG,GAAG,IAAI,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;QAC5C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAC7C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAC7C,mBAAmB;QACnB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,GAAG,CAAC,IAAI,GAAG,IAAI,CAAC;QACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;QAC7C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAC9C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAE9C,yCAAyC;QACzC,kBAAkB;QAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,IAAI,CAAC,GAAG,GAAG,IAAI,CAAC;QACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;QAC7C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAC9C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAC9C,mBAAmB;QACnB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,GAAG,CAAC,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC;QACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;QAC9C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAC/C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAE/C,4BAA4B;QAC5B,OAAO,IAAI,UAAU,CAAC,MAAM,CAAC,CAAC;IAChC,CAAC;IAED,iEAAiE;IACjE,6BAAQ,GAAR;QACE,4DAA4D;QAC5D,8CAA8C;QAE9C,oCAAoC;QACpC,IAAI,eAAe,CAAC;QACpB,mCAAmC;QACnC,IAAI,kBAAkB,GAAG,CAAC,CAAC;QAC3B,wCAAwC;QACxC,IAAM,WAAW,GAAG,IAAI,KAAK,CAAS,EAAE,CAAC,CAAC;QAC1C,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,WAAW,CAAC,MAAM,EAAE,CAAC,EAAE;YAAE,WAAW,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;QAChE,gCAAgC;QAChC,IAAI,KAAK,GAAG,CAAC,CAAC;QAEd,6BAA6B;QAC7B,IAAI,OAAO,GAAG,KAAK,CAAC;QAEpB,gDAAgD;QAChD,IAAI,eAAe,CAAC;QACpB,6CAA6C;QAC7C,IAAI,cAAc,GAAgD,EAAE,KAAK,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,EAAE,CAAC;QAC1F,qBAAqB;QACrB,IAAI,CAAC,EAAE,CAAC,CAAC;QAET,gBAAgB;QAChB,IAAM,MAAM,GAAa,EAAE,CAAC;QAE5B,eAAe;QACf,KAAK,GAAG,CAAC,CAAC;QAEV,mBAAmB;QACnB,IAAM,MAAM,GAAG,IAAI,CAAC,KAAK,CAAC;QAE1B,oCAAoC;QACpC,gBAAgB;QAChB,IAAM,GAAG,GACP,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;QAC/F,eAAe;QACf,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;QAE/F,qCAAqC;QACrC,eAAe;QACf,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;QAC/F,cAAc;QACd,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;QAE/F,eAAe;QACf,KAAK,GAAG,CAAC,CAAC;QAEV,kCAAkC;QAClC,IAAM,GAAG,GAAG;YACV,GAAG,EAAE,IAAI,WAAI,CAAC,GAAG,EAAE,IAAI,CAAC;YACxB,IAAI,EAAE,IAAI,WAAI,CAAC,IAAI,EAAE,IAAI,CAAC;SAC3B,CAAC;QAEF,IAAI,GAAG,CAAC,IAAI,CAAC,QAAQ,CAAC,WAAI,CAAC,IAAI,CAAC,EAAE;YAChC,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;SAClB;QAED,wCAAwC;QACxC,aAAa;QACb,IAAM,WAAW,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,gBAAgB,CAAC;QAEpD,IAAI,WAAW,IAAI,CAAC,KAAK,CAAC,EAAE;YAC1B,6BAA6B;YAC7B,IAAI,WAAW,KAAK,oBAAoB,EAAE;gBACxC,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,GAAG,UAAU,CAAC;aACrC;iBAAM,IAAI,WAAW,KAAK,eAAe,EAAE;gBAC1C,OAAO,KAAK,CAAC;aACd;iBAAM;gBACL,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,aAAa,CAAC;gBAC/C,eAAe,GAAG,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC,CAAC;aAChD;SACF;aAAM;YACL,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;YACtC,eAAe,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,aAAa,CAAC;SAChD;QAED,oBAAoB;QACpB,IAAM,QAAQ,GAAG,eAAe,GAAG,aAAa,CAAC;QAEjD,sCAAsC;QAEtC,mDAAmD;QACnD,4DAA4D;QAC5D,sCAAsC;QACtC,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,IAAI,GAAG,MAAM,CAAC,GAAG,CAAC,CAAC,eAAe,GAAG,GAAG,CAAC,IAAI,EAAE,CAAC,CAAC;QAC5E,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC;QAC/B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC;QAC/B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;QAE9B,IACE,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;YAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;YAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC;YAC7B,cAAc,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC,EAC7B;YACA,OAAO,GAAG,IAAI,CAAC;SAChB;aAAM;YACL,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;gBACvB,IAAI,YAAY,GAAG,CAAC,CAAC;gBACrB,qBAAqB;gBACrB,IAAM,MAAM,GAAG,UAAU,CAAC,cAAc,CAAC,CAAC;gBAC1C,cAAc,GAAG,MAAM,CAAC,QAAQ,CAAC;gBACjC,YAAY,GAAG,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC;gBAE9B,0DAA0D;gBAC1D,gCAAgC;gBAChC,IAAI,CAAC,YAAY;oBAAE,SAAS;gBAE5B,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,EAAE;oBACvB,0DAA0D;oBAC1D,WAAW,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,YAAY,GAAG,EAAE,CAAC;oBAC3C,gDAAgD;oBAChD,YAAY,GAAG,IAAI,CAAC,KAAK,CAAC,YAAY,GAAG,EAAE,CAAC,CAAC;iBAC9C;aACF;SACF;QAED,yBAAyB;QACzB,gDAAgD;QAChD,uBAAuB;QAEvB,IAAI,OAAO,EAAE;YACX,kBAAkB,GAAG,CAAC,CAAC;YACvB,WAAW,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;SACxB;aAAM;YACL,kBAAkB,GAAG,EAAE,CAAC;YACxB,OAAO,CAAC,WAAW,CAAC,KAAK,CAAC,EAAE;gBAC1B,kBAAkB,GAAG,kBAAkB,GAAG,CAAC,CAAC;gBAC5C,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;aACnB;SACF;QAED,8CAA8C;QAC9C,IAAM,mBAAmB,GAAG,kBAAkB,GAAG,CAAC,GAAG,QAAQ,CAAC;QAE9D,uEAAuE;QACvE,oDAAoD;QACpD,EAAE;QACF,sEAAsE;QACtE,yEAAyE;QACzE,sEAAsE;QACtE,sEAAsE;QACtE,IAAI,mBAAmB,IAAI,EAAE,IAAI,mBAAmB,IAAI,CAAC,CAAC,IAAI,QAAQ,GAAG,CAAC,EAAE;YAC1E,oBAAoB;YAEpB,+EAA+E;YAC/E,8EAA8E;YAC9E,6EAA6E;YAC7E,IAAI,kBAAkB,GAAG,EAAE,EAAE;gBAC3B,MAAM,CAAC,IAAI,CAAC,KAAG,CAAG,CAAC,CAAC;gBACpB,IAAI,QAAQ,GAAG,CAAC;oBAAE,MAAM,CAAC,IAAI,CAAC,IAAI,GAAG,QAAQ,CAAC,CAAC;qBAC1C,IAAI,QAAQ,GAAG,CAAC;oBAAE,MAAM,CAAC,IAAI,CAAC,GAAG,GAAG,QAAQ,CAAC,CAAC;gBACnD,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;aACxB;YAED,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;YACvC,kBAAkB,GAAG,kBAAkB,GAAG,CAAC,CAAC;YAE5C,IAAI,kBAAkB,EAAE;gBACtB,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;aAClB;YAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,EAAE,CAAC,EAAE,EAAE;gBAC3C,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;aACxC;YAED,WAAW;YACX,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;YACjB,IAAI,mBAAmB,GAAG,CAAC,EAAE;gBAC3B,MAAM,CAAC,IAAI,CAAC,GAAG,GAAG,mBAAmB,CAAC,CAAC;aACxC;iBAAM;gBACL,MAAM,CAAC,IAAI,CAAC,KAAG,mBAAqB,CAAC,CAAC;aACvC;SACF;aAAM;YACL,uCAAuC;YACvC,IAAI,QAAQ,IAAI,CAAC,EAAE;gBACjB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,EAAE,CAAC,EAAE,EAAE;oBAC3C,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;iBACxC;aACF;iBAAM;gBACL,IAAI,cAAc,GAAG,kBAAkB,GAAG,QAAQ,CAAC;gBAEnD,+BAA+B;gBAC/B,IAAI,cAAc,GAAG,CAAC,EAAE;oBACtB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,cAAc,EAAE,CAAC,EAAE,EAAE;wBACvC,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;qBACxC;iBACF;qBAAM;oBACL,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;iBAClB;gBAED,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;gBACjB,gCAAgC;gBAChC,OAAO,cAAc,EAAE,GAAG,CAAC,EAAE;oBAC3B,MAAM,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;iBAClB;gBAED,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,kBAAkB,GAAG,IAAI,CAAC,GAAG,CAAC,cAAc,GAAG,CAAC,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,EAAE;oBAC7E,MAAM,CAAC,IAAI,CAAC,KAAG,WAAW,CAAC,KAAK,EAAE,CAAG,CAAC,CAAC;iBACxC;aACF;SACF;QAED,OAAO,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;IACzB,CAAC;IAED,2BAAM,GAAN;QACE,OAAO,EAAE,cAAc,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;IAC7C,CAAC;IAED,gBAAgB;IAChB,mCAAc,GAAd;QACE,OAAO,EAAE,cAAc,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;IAC7C,CAAC;IAED,gBAAgB;IACT,2BAAgB,GAAvB,UAAwB,GAAuB;QAC7C,OAAO,UAAU,CAAC,UAAU,CAAC,GAAG,CAAC,cAAc,CAAC,CAAC;IACnD,CAAC;IAED,gBAAgB;IAChB,qBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,4BAAO,GAAP;QACE,OAAO,sBAAmB,IAAI,CAAC,QAAQ,EAAE,QAAI,CAAC;IAChD,CAAC;IACH,iBAAC;AAAD,CAAC,AAnoBD,IAmoBC;AAnoBY,gCAAU;AAqoBvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,YAAY,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/double.js b/node_modules/bson/lib/double.js
new file mode 100644
index 0000000..26da4c0
--- /dev/null
+++ b/node_modules/bson/lib/double.js
@@ -0,0 +1,73 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Double = void 0;
+/**
+ * A class representation of the BSON Double type.
+ * @public
+ */
+var Double = /** @class */ (function () {
+ /**
+ * Create a Double type
+ *
+ * @param value - the number we want to represent as a double.
+ */
+ function Double(value) {
+ if (!(this instanceof Double))
+ return new Double(value);
+ if (value instanceof Number) {
+ value = value.valueOf();
+ }
+ this.value = +value;
+ }
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped double number.
+ */
+ Double.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ Double.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ Double.prototype.toExtendedJSON = function (options) {
+ if (options && (options.legacy || (options.relaxed && isFinite(this.value)))) {
+ return this.value;
+ }
+ // NOTE: JavaScript has +0 and -0, apparently to model limit calculations. If a user
+ // explicitly provided `-0` then we need to ensure the sign makes it into the output
+ if (Object.is(Math.sign(this.value), -0)) {
+ return { $numberDouble: "-" + this.value.toFixed(1) };
+ }
+ var $numberDouble;
+ if (Number.isInteger(this.value)) {
+ $numberDouble = this.value.toFixed(1);
+ if ($numberDouble.length >= 13) {
+ $numberDouble = this.value.toExponential(13).toUpperCase();
+ }
+ }
+ else {
+ $numberDouble = this.value.toString();
+ }
+ return { $numberDouble: $numberDouble };
+ };
+ /** @internal */
+ Double.fromExtendedJSON = function (doc, options) {
+ var doubleValue = parseFloat(doc.$numberDouble);
+ return options && options.relaxed ? doubleValue : new Double(doubleValue);
+ };
+ /** @internal */
+ Double.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Double.prototype.inspect = function () {
+ var eJSON = this.toExtendedJSON();
+ return "new Double(" + eJSON.$numberDouble + ")";
+ };
+ return Double;
+}());
+exports.Double = Double;
+Object.defineProperty(Double.prototype, '_bsontype', { value: 'Double' });
+//# sourceMappingURL=double.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/double.js.map b/node_modules/bson/lib/double.js.map
new file mode 100644
index 0000000..75c4736
--- /dev/null
+++ b/node_modules/bson/lib/double.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"double.js","sourceRoot":"","sources":["../src/double.ts"],"names":[],"mappings":";;;AAOA;;;GAGG;AACH;IAIE;;;;OAIG;IACH,gBAAY,KAAa;QACvB,IAAI,CAAC,CAAC,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,CAAC,KAAK,CAAC,CAAC;QAExD,IAAK,KAAiB,YAAY,MAAM,EAAE;YACxC,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;SACzB;QAED,IAAI,CAAC,KAAK,GAAG,CAAC,KAAK,CAAC;IACtB,CAAC;IAED;;;;OAIG;IACH,wBAAO,GAAP;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;IACpB,CAAC;IAED,gBAAgB;IAChB,uBAAM,GAAN;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;IACpB,CAAC;IAED,gBAAgB;IAChB,+BAAc,GAAd,UAAe,OAAsB;QACnC,IAAI,OAAO,IAAI,CAAC,OAAO,CAAC,MAAM,IAAI,CAAC,OAAO,CAAC,OAAO,IAAI,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE;YAC5E,OAAO,IAAI,CAAC,KAAK,CAAC;SACnB;QAED,oFAAoF;QACpF,oFAAoF;QACpF,IAAI,MAAM,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,CAAC,EAAE;YACxC,OAAO,EAAE,aAAa,EAAE,MAAI,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAG,EAAE,CAAC;SACvD;QAED,IAAI,aAAqB,CAAC;QAC1B,IAAI,MAAM,CAAC,SAAS,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE;YAChC,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;YACtC,IAAI,aAAa,CAAC,MAAM,IAAI,EAAE,EAAE;gBAC9B,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,aAAa,CAAC,EAAE,CAAC,CAAC,WAAW,EAAE,CAAC;aAC5D;SACF;aAAM;YACL,aAAa,GAAG,IAAI,CAAC,KAAK,CAAC,QAAQ,EAAE,CAAC;SACvC;QAED,OAAO,EAAE,aAAa,eAAA,EAAE,CAAC;IAC3B,CAAC;IAED,gBAAgB;IACT,uBAAgB,GAAvB,UAAwB,GAAmB,EAAE,OAAsB;QACjE,IAAM,WAAW,GAAG,UAAU,CAAC,GAAG,CAAC,aAAa,CAAC,CAAC;QAClD,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,CAAC,CAAC,CAAC,WAAW,CAAC,CAAC,CAAC,IAAI,MAAM,CAAC,WAAW,CAAC,CAAC;IAC5E,CAAC;IAED,gBAAgB;IAChB,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,wBAAO,GAAP;QACE,IAAM,KAAK,GAAG,IAAI,CAAC,cAAc,EAAoB,CAAC;QACtD,OAAO,gBAAc,KAAK,CAAC,aAAa,MAAG,CAAC;IAC9C,CAAC;IACH,aAAC;AAAD,CAAC,AAzED,IAyEC;AAzEY,wBAAM;AA2EnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/ensure_buffer.js b/node_modules/bson/lib/ensure_buffer.js
new file mode 100644
index 0000000..ea8e961
--- /dev/null
+++ b/node_modules/bson/lib/ensure_buffer.js
@@ -0,0 +1,24 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.ensureBuffer = void 0;
+var buffer_1 = require("buffer");
+var utils_1 = require("./parser/utils");
+/**
+ * Makes sure that, if a Uint8Array is passed in, it is wrapped in a Buffer.
+ *
+ * @param potentialBuffer - The potential buffer
+ * @returns Buffer the input if potentialBuffer is a buffer, or a buffer that
+ * wraps a passed in Uint8Array
+ * @throws TypeError If anything other than a Buffer or Uint8Array is passed in
+ */
+function ensureBuffer(potentialBuffer) {
+ if (ArrayBuffer.isView(potentialBuffer)) {
+ return buffer_1.Buffer.from(potentialBuffer.buffer, potentialBuffer.byteOffset, potentialBuffer.byteLength);
+ }
+ if (utils_1.isAnyArrayBuffer(potentialBuffer)) {
+ return buffer_1.Buffer.from(potentialBuffer);
+ }
+ throw new TypeError('Must use either Buffer or TypedArray');
+}
+exports.ensureBuffer = ensureBuffer;
+//# sourceMappingURL=ensure_buffer.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/ensure_buffer.js.map b/node_modules/bson/lib/ensure_buffer.js.map
new file mode 100644
index 0000000..3af4529
--- /dev/null
+++ b/node_modules/bson/lib/ensure_buffer.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"ensure_buffer.js","sourceRoot":"","sources":["../src/ensure_buffer.ts"],"names":[],"mappings":";;;AAAA,iCAAgC;AAChC,wCAAkD;AAElD;;;;;;;GAOG;AACH,SAAgB,YAAY,CAAC,eAAuD;IAClF,IAAI,WAAW,CAAC,MAAM,CAAC,eAAe,CAAC,EAAE;QACvC,OAAO,eAAM,CAAC,IAAI,CAChB,eAAe,CAAC,MAAM,EACtB,eAAe,CAAC,UAAU,EAC1B,eAAe,CAAC,UAAU,CAC3B,CAAC;KACH;IAED,IAAI,wBAAgB,CAAC,eAAe,CAAC,EAAE;QACrC,OAAO,eAAM,CAAC,IAAI,CAAC,eAAe,CAAC,CAAC;KACrC;IAED,MAAM,IAAI,SAAS,CAAC,sCAAsC,CAAC,CAAC;AAC9D,CAAC;AAdD,oCAcC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/extended_json.js b/node_modules/bson/lib/extended_json.js
new file mode 100644
index 0000000..f8d16d2
--- /dev/null
+++ b/node_modules/bson/lib/extended_json.js
@@ -0,0 +1,372 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.EJSON = exports.isBSONType = void 0;
+var binary_1 = require("./binary");
+var code_1 = require("./code");
+var db_ref_1 = require("./db_ref");
+var decimal128_1 = require("./decimal128");
+var double_1 = require("./double");
+var int_32_1 = require("./int_32");
+var long_1 = require("./long");
+var max_key_1 = require("./max_key");
+var min_key_1 = require("./min_key");
+var objectid_1 = require("./objectid");
+var utils_1 = require("./parser/utils");
+var regexp_1 = require("./regexp");
+var symbol_1 = require("./symbol");
+var timestamp_1 = require("./timestamp");
+function isBSONType(value) {
+ return (utils_1.isObjectLike(value) && Reflect.has(value, '_bsontype') && typeof value._bsontype === 'string');
+}
+exports.isBSONType = isBSONType;
+// INT32 boundaries
+var BSON_INT32_MAX = 0x7fffffff;
+var BSON_INT32_MIN = -0x80000000;
+// INT64 boundaries
+var BSON_INT64_MAX = 0x7fffffffffffffff;
+var BSON_INT64_MIN = -0x8000000000000000;
+// all the types where we don't need to do any special processing and can just pass the EJSON
+//straight to type.fromExtendedJSON
+var keysToCodecs = {
+ $oid: objectid_1.ObjectId,
+ $binary: binary_1.Binary,
+ $uuid: binary_1.Binary,
+ $symbol: symbol_1.BSONSymbol,
+ $numberInt: int_32_1.Int32,
+ $numberDecimal: decimal128_1.Decimal128,
+ $numberDouble: double_1.Double,
+ $numberLong: long_1.Long,
+ $minKey: min_key_1.MinKey,
+ $maxKey: max_key_1.MaxKey,
+ $regex: regexp_1.BSONRegExp,
+ $regularExpression: regexp_1.BSONRegExp,
+ $timestamp: timestamp_1.Timestamp
+};
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function deserializeValue(value, options) {
+ if (options === void 0) { options = {}; }
+ if (typeof value === 'number') {
+ if (options.relaxed || options.legacy) {
+ return value;
+ }
+ // if it's an integer, should interpret as smallest BSON integer
+ // that can represent it exactly. (if out of range, interpret as double.)
+ if (Math.floor(value) === value) {
+ if (value >= BSON_INT32_MIN && value <= BSON_INT32_MAX)
+ return new int_32_1.Int32(value);
+ if (value >= BSON_INT64_MIN && value <= BSON_INT64_MAX)
+ return long_1.Long.fromNumber(value);
+ }
+ // If the number is a non-integer or out of integer range, should interpret as BSON Double.
+ return new double_1.Double(value);
+ }
+ // from here on out we're looking for bson types, so bail if its not an object
+ if (value == null || typeof value !== 'object')
+ return value;
+ // upgrade deprecated undefined to null
+ if (value.$undefined)
+ return null;
+ var keys = Object.keys(value).filter(function (k) { return k.startsWith('$') && value[k] != null; });
+ for (var i = 0; i < keys.length; i++) {
+ var c = keysToCodecs[keys[i]];
+ if (c)
+ return c.fromExtendedJSON(value, options);
+ }
+ if (value.$date != null) {
+ var d = value.$date;
+ var date = new Date();
+ if (options.legacy) {
+ if (typeof d === 'number')
+ date.setTime(d);
+ else if (typeof d === 'string')
+ date.setTime(Date.parse(d));
+ }
+ else {
+ if (typeof d === 'string')
+ date.setTime(Date.parse(d));
+ else if (long_1.Long.isLong(d))
+ date.setTime(d.toNumber());
+ else if (typeof d === 'number' && options.relaxed)
+ date.setTime(d);
+ }
+ return date;
+ }
+ if (value.$code != null) {
+ var copy = Object.assign({}, value);
+ if (value.$scope) {
+ copy.$scope = deserializeValue(value.$scope);
+ }
+ return code_1.Code.fromExtendedJSON(value);
+ }
+ if (db_ref_1.isDBRefLike(value) || value.$dbPointer) {
+ var v = value.$ref ? value : value.$dbPointer;
+ // we run into this in a "degenerate EJSON" case (with $id and $ref order flipped)
+ // because of the order JSON.parse goes through the document
+ if (v instanceof db_ref_1.DBRef)
+ return v;
+ var dollarKeys = Object.keys(v).filter(function (k) { return k.startsWith('$'); });
+ var valid_1 = true;
+ dollarKeys.forEach(function (k) {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1)
+ valid_1 = false;
+ });
+ // only make DBRef if $ keys are all valid
+ if (valid_1)
+ return db_ref_1.DBRef.fromExtendedJSON(v);
+ }
+ return value;
+}
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeArray(array, options) {
+ return array.map(function (v, index) {
+ options.seenObjects.push({ propertyName: "index " + index, obj: null });
+ try {
+ return serializeValue(v, options);
+ }
+ finally {
+ options.seenObjects.pop();
+ }
+ });
+}
+function getISOString(date) {
+ var isoStr = date.toISOString();
+ // we should only show milliseconds in timestamp if they're non-zero
+ return date.getUTCMilliseconds() !== 0 ? isoStr : isoStr.slice(0, -5) + 'Z';
+}
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeValue(value, options) {
+ if ((typeof value === 'object' || typeof value === 'function') && value !== null) {
+ var index = options.seenObjects.findIndex(function (entry) { return entry.obj === value; });
+ if (index !== -1) {
+ var props = options.seenObjects.map(function (entry) { return entry.propertyName; });
+ var leadingPart = props
+ .slice(0, index)
+ .map(function (prop) { return prop + " -> "; })
+ .join('');
+ var alreadySeen = props[index];
+ var circularPart = ' -> ' +
+ props
+ .slice(index + 1, props.length - 1)
+ .map(function (prop) { return prop + " -> "; })
+ .join('');
+ var current = props[props.length - 1];
+ var leadingSpace = ' '.repeat(leadingPart.length + alreadySeen.length / 2);
+ var dashes = '-'.repeat(circularPart.length + (alreadySeen.length + current.length) / 2 - 1);
+ throw new TypeError('Converting circular structure to EJSON:\n' +
+ (" " + leadingPart + alreadySeen + circularPart + current + "\n") +
+ (" " + leadingSpace + "\\" + dashes + "/"));
+ }
+ options.seenObjects[options.seenObjects.length - 1].obj = value;
+ }
+ if (Array.isArray(value))
+ return serializeArray(value, options);
+ if (value === undefined)
+ return null;
+ if (value instanceof Date || utils_1.isDate(value)) {
+ var dateNum = value.getTime(),
+ // is it in year range 1970-9999?
+ inRange = dateNum > -1 && dateNum < 253402318800000;
+ if (options.legacy) {
+ return options.relaxed && inRange
+ ? { $date: value.getTime() }
+ : { $date: getISOString(value) };
+ }
+ return options.relaxed && inRange
+ ? { $date: getISOString(value) }
+ : { $date: { $numberLong: value.getTime().toString() } };
+ }
+ if (typeof value === 'number' && (!options.relaxed || !isFinite(value))) {
+ // it's an integer
+ if (Math.floor(value) === value) {
+ var int32Range = value >= BSON_INT32_MIN && value <= BSON_INT32_MAX, int64Range = value >= BSON_INT64_MIN && value <= BSON_INT64_MAX;
+ // interpret as being of the smallest BSON integer type that can represent the number exactly
+ if (int32Range)
+ return { $numberInt: value.toString() };
+ if (int64Range)
+ return { $numberLong: value.toString() };
+ }
+ return { $numberDouble: value.toString() };
+ }
+ if (value instanceof RegExp || utils_1.isRegExp(value)) {
+ var flags = value.flags;
+ if (flags === undefined) {
+ var match = value.toString().match(/[gimuy]*$/);
+ if (match) {
+ flags = match[0];
+ }
+ }
+ var rx = new regexp_1.BSONRegExp(value.source, flags);
+ return rx.toExtendedJSON(options);
+ }
+ if (value != null && typeof value === 'object')
+ return serializeDocument(value, options);
+ return value;
+}
+var BSON_TYPE_MAPPINGS = {
+ Binary: function (o) { return new binary_1.Binary(o.value(), o.sub_type); },
+ Code: function (o) { return new code_1.Code(o.code, o.scope); },
+ DBRef: function (o) { return new db_ref_1.DBRef(o.collection || o.namespace, o.oid, o.db, o.fields); },
+ Decimal128: function (o) { return new decimal128_1.Decimal128(o.bytes); },
+ Double: function (o) { return new double_1.Double(o.value); },
+ Int32: function (o) { return new int_32_1.Int32(o.value); },
+ Long: function (o) {
+ return long_1.Long.fromBits(
+ // underscore variants for 1.x backwards compatibility
+ o.low != null ? o.low : o.low_, o.low != null ? o.high : o.high_, o.low != null ? o.unsigned : o.unsigned_);
+ },
+ MaxKey: function () { return new max_key_1.MaxKey(); },
+ MinKey: function () { return new min_key_1.MinKey(); },
+ ObjectID: function (o) { return new objectid_1.ObjectId(o); },
+ ObjectId: function (o) { return new objectid_1.ObjectId(o); },
+ BSONRegExp: function (o) { return new regexp_1.BSONRegExp(o.pattern, o.options); },
+ Symbol: function (o) { return new symbol_1.BSONSymbol(o.value); },
+ Timestamp: function (o) { return timestamp_1.Timestamp.fromBits(o.low, o.high); }
+};
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeDocument(doc, options) {
+ if (doc == null || typeof doc !== 'object')
+ throw new Error('not an object instance');
+ var bsontype = doc._bsontype;
+ if (typeof bsontype === 'undefined') {
+ // It's a regular object. Recursively serialize its property values.
+ var _doc = {};
+ for (var name in doc) {
+ options.seenObjects.push({ propertyName: name, obj: null });
+ try {
+ _doc[name] = serializeValue(doc[name], options);
+ }
+ finally {
+ options.seenObjects.pop();
+ }
+ }
+ return _doc;
+ }
+ else if (isBSONType(doc)) {
+ // the "document" is really just a BSON type object
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ var outDoc = doc;
+ if (typeof outDoc.toExtendedJSON !== 'function') {
+ // There's no EJSON serialization function on the object. It's probably an
+ // object created by a previous version of this library (or another library)
+ // that's duck-typing objects to look like they were generated by this library).
+ // Copy the object into this library's version of that type.
+ var mapper = BSON_TYPE_MAPPINGS[doc._bsontype];
+ if (!mapper) {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + doc._bsontype);
+ }
+ outDoc = mapper(outDoc);
+ }
+ // Two BSON types may have nested objects that may need to be serialized too
+ if (bsontype === 'Code' && outDoc.scope) {
+ outDoc = new code_1.Code(outDoc.code, serializeValue(outDoc.scope, options));
+ }
+ else if (bsontype === 'DBRef' && outDoc.oid) {
+ outDoc = new db_ref_1.DBRef(serializeValue(outDoc.collection, options), serializeValue(outDoc.oid, options), serializeValue(outDoc.db, options), serializeValue(outDoc.fields, options));
+ }
+ return outDoc.toExtendedJSON(options);
+ }
+ else {
+ throw new Error('_bsontype must be a string, but was: ' + typeof bsontype);
+ }
+}
+/**
+ * EJSON parse / stringify API
+ * @public
+ */
+// the namespace here is used to emulate `export * as EJSON from '...'`
+// which as of now (sept 2020) api-extractor does not support
+// eslint-disable-next-line @typescript-eslint/no-namespace
+var EJSON;
+(function (EJSON) {
+ /**
+ * Parse an Extended JSON string, constructing the JavaScript value or object described by that
+ * string.
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const text = '{ "int32": { "$numberInt": "10" } }';
+ *
+ * // prints { int32: { [String: '10'] _bsontype: 'Int32', value: '10' } }
+ * console.log(EJSON.parse(text, { relaxed: false }));
+ *
+ * // prints { int32: 10 }
+ * console.log(EJSON.parse(text));
+ * ```
+ */
+ function parse(text, options) {
+ var finalOptions = Object.assign({}, { relaxed: true, legacy: false }, options);
+ // relaxed implies not strict
+ if (typeof finalOptions.relaxed === 'boolean')
+ finalOptions.strict = !finalOptions.relaxed;
+ if (typeof finalOptions.strict === 'boolean')
+ finalOptions.relaxed = !finalOptions.strict;
+ return JSON.parse(text, function (_key, value) { return deserializeValue(value, finalOptions); });
+ }
+ EJSON.parse = parse;
+ /**
+ * Converts a BSON document to an Extended JSON string, optionally replacing values if a replacer
+ * function is specified or optionally including only the specified properties if a replacer array
+ * is specified.
+ *
+ * @param value - The value to convert to extended JSON
+ * @param replacer - A function that alters the behavior of the stringification process, or an array of String and Number objects that serve as a whitelist for selecting/filtering the properties of the value object to be included in the JSON string. If this value is null or not provided, all properties of the object are included in the resulting JSON string
+ * @param space - A String or Number object that's used to insert white space into the output JSON string for readability purposes.
+ * @param options - Optional settings
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const Int32 = require('mongodb').Int32;
+ * const doc = { int32: new Int32(10) };
+ *
+ * // prints '{"int32":{"$numberInt":"10"}}'
+ * console.log(EJSON.stringify(doc, { relaxed: false }));
+ *
+ * // prints '{"int32":10}'
+ * console.log(EJSON.stringify(doc));
+ * ```
+ */
+ function stringify(value,
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ replacer, space, options) {
+ if (space != null && typeof space === 'object') {
+ options = space;
+ space = 0;
+ }
+ if (replacer != null && typeof replacer === 'object' && !Array.isArray(replacer)) {
+ options = replacer;
+ replacer = undefined;
+ space = 0;
+ }
+ var serializeOptions = Object.assign({ relaxed: true, legacy: false }, options, {
+ seenObjects: [{ propertyName: '(root)', obj: null }]
+ });
+ var doc = serializeValue(value, serializeOptions);
+ return JSON.stringify(doc, replacer, space);
+ }
+ EJSON.stringify = stringify;
+ /**
+ * Serializes an object to an Extended JSON string, and reparse it as a JavaScript object.
+ *
+ * @param value - The object to serialize
+ * @param options - Optional settings passed to the `stringify` function
+ */
+ function serialize(value, options) {
+ options = options || {};
+ return JSON.parse(stringify(value, options));
+ }
+ EJSON.serialize = serialize;
+ /**
+ * Deserializes an Extended JSON object into a plain JavaScript object with native/BSON types
+ *
+ * @param ejson - The Extended JSON object to deserialize
+ * @param options - Optional settings passed to the parse method
+ */
+ function deserialize(ejson, options) {
+ options = options || {};
+ return parse(JSON.stringify(ejson), options);
+ }
+ EJSON.deserialize = deserialize;
+})(EJSON = exports.EJSON || (exports.EJSON = {}));
+//# sourceMappingURL=extended_json.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/extended_json.js.map b/node_modules/bson/lib/extended_json.js.map
new file mode 100644
index 0000000..5c9ff17
--- /dev/null
+++ b/node_modules/bson/lib/extended_json.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"extended_json.js","sourceRoot":"","sources":["../src/extended_json.ts"],"names":[],"mappings":";;;AAAA,mCAAkC;AAElC,+BAA8B;AAC9B,mCAA8C;AAC9C,2CAA0C;AAC1C,mCAAkC;AAClC,mCAAiC;AACjC,+BAA8B;AAC9B,qCAAmC;AACnC,qCAAmC;AACnC,uCAAsC;AACtC,wCAAgE;AAChE,mCAAsC;AACtC,mCAAsC;AACtC,yCAAwC;AAqBxC,SAAgB,UAAU,CAAC,KAAc;IACvC,OAAO,CACL,oBAAY,CAAC,KAAK,CAAC,IAAI,OAAO,CAAC,GAAG,CAAC,KAAK,EAAE,WAAW,CAAC,IAAI,OAAO,KAAK,CAAC,SAAS,KAAK,QAAQ,CAC9F,CAAC;AACJ,CAAC;AAJD,gCAIC;AAED,mBAAmB;AACnB,IAAM,cAAc,GAAG,UAAU,CAAC;AAClC,IAAM,cAAc,GAAG,CAAC,UAAU,CAAC;AACnC,mBAAmB;AACnB,IAAM,cAAc,GAAG,kBAAkB,CAAC;AAC1C,IAAM,cAAc,GAAG,CAAC,kBAAkB,CAAC;AAE3C,6FAA6F;AAC7F,mCAAmC;AACnC,IAAM,YAAY,GAAG;IACnB,IAAI,EAAE,mBAAQ;IACd,OAAO,EAAE,eAAM;IACf,KAAK,EAAE,eAAM;IACb,OAAO,EAAE,mBAAU;IACnB,UAAU,EAAE,cAAK;IACjB,cAAc,EAAE,uBAAU;IAC1B,aAAa,EAAE,eAAM;IACrB,WAAW,EAAE,WAAI;IACjB,OAAO,EAAE,gBAAM;IACf,OAAO,EAAE,gBAAM;IACf,MAAM,EAAE,mBAAU;IAClB,kBAAkB,EAAE,mBAAU;IAC9B,UAAU,EAAE,qBAAS;CACb,CAAC;AAEX,8DAA8D;AAC9D,SAAS,gBAAgB,CAAC,KAAU,EAAE,OAA2B;IAA3B,wBAAA,EAAA,YAA2B;IAC/D,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;QAC7B,IAAI,OAAO,CAAC,OAAO,IAAI,OAAO,CAAC,MAAM,EAAE;YACrC,OAAO,KAAK,CAAC;SACd;QAED,gEAAgE;QAChE,yEAAyE;QACzE,IAAI,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK,EAAE;YAC/B,IAAI,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc;gBAAE,OAAO,IAAI,cAAK,CAAC,KAAK,CAAC,CAAC;YAChF,IAAI,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc;gBAAE,OAAO,WAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC;SACvF;QAED,2FAA2F;QAC3F,OAAO,IAAI,eAAM,CAAC,KAAK,CAAC,CAAC;KAC1B;IAED,8EAA8E;IAC9E,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ;QAAE,OAAO,KAAK,CAAC;IAE7D,uCAAuC;IACvC,IAAI,KAAK,CAAC,UAAU;QAAE,OAAO,IAAI,CAAC;IAElC,IAAM,IAAI,GAAG,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,MAAM,CACpC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,IAAI,EAArC,CAAqC,CACV,CAAC;IACnC,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;QACpC,IAAM,CAAC,GAAG,YAAY,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,CAAC;QAChC,IAAI,CAAC;YAAE,OAAO,CAAC,CAAC,gBAAgB,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;KAClD;IAED,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,EAAE;QACvB,IAAM,CAAC,GAAG,KAAK,CAAC,KAAK,CAAC;QACtB,IAAM,IAAI,GAAG,IAAI,IAAI,EAAE,CAAC;QAExB,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,IAAI,OAAO,CAAC,KAAK,QAAQ;gBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;iBACtC,IAAI,OAAO,CAAC,KAAK,QAAQ;gBAAE,IAAI,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;SAC7D;aAAM;YACL,IAAI,OAAO,CAAC,KAAK,QAAQ;gBAAE,IAAI,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;iBAClD,IAAI,WAAI,CAAC,MAAM,CAAC,CAAC,CAAC;gBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,QAAQ,EAAE,CAAC,CAAC;iBAC/C,IAAI,OAAO,CAAC,KAAK,QAAQ,IAAI,OAAO,CAAC,OAAO;gBAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;SACpE;QACD,OAAO,IAAI,CAAC;KACb;IAED,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,EAAE;QACvB,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,KAAK,CAAC,CAAC;QACtC,IAAI,KAAK,CAAC,MAAM,EAAE;YAChB,IAAI,CAAC,MAAM,GAAG,gBAAgB,CAAC,KAAK,CAAC,MAAM,CAAC,CAAC;SAC9C;QAED,OAAO,WAAI,CAAC,gBAAgB,CAAC,KAAK,CAAC,CAAC;KACrC;IAED,IAAI,oBAAW,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,UAAU,EAAE;QAC1C,IAAM,CAAC,GAAG,KAAK,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,KAAK,CAAC,UAAU,CAAC;QAEhD,kFAAkF;QAClF,4DAA4D;QAC5D,IAAI,CAAC,YAAY,cAAK;YAAE,OAAO,CAAC,CAAC;QAEjC,IAAM,UAAU,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,EAAjB,CAAiB,CAAC,CAAC;QACjE,IAAI,OAAK,GAAG,IAAI,CAAC;QACjB,UAAU,CAAC,OAAO,CAAC,UAAA,CAAC;YAClB,IAAI,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC;gBAAE,OAAK,GAAG,KAAK,CAAC;QAC9D,CAAC,CAAC,CAAC;QAEH,0CAA0C;QAC1C,IAAI,OAAK;YAAE,OAAO,cAAK,CAAC,gBAAgB,CAAC,CAAC,CAAC,CAAC;KAC7C;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAMD,8DAA8D;AAC9D,SAAS,cAAc,CAAC,KAAY,EAAE,OAA8B;IAClE,OAAO,KAAK,CAAC,GAAG,CAAC,UAAC,CAAU,EAAE,KAAa;QACzC,OAAO,CAAC,WAAW,CAAC,IAAI,CAAC,EAAE,YAAY,EAAE,WAAS,KAAO,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC,CAAC;QACxE,IAAI;YACF,OAAO,cAAc,CAAC,CAAC,EAAE,OAAO,CAAC,CAAC;SACnC;gBAAS;YACR,OAAO,CAAC,WAAW,CAAC,GAAG,EAAE,CAAC;SAC3B;IACH,CAAC,CAAC,CAAC;AACL,CAAC;AAED,SAAS,YAAY,CAAC,IAAU;IAC9B,IAAM,MAAM,GAAG,IAAI,CAAC,WAAW,EAAE,CAAC;IAClC,oEAAoE;IACpE,OAAO,IAAI,CAAC,kBAAkB,EAAE,KAAK,CAAC,CAAC,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;AAC9E,CAAC;AAED,8DAA8D;AAC9D,SAAS,cAAc,CAAC,KAAU,EAAE,OAA8B;IAChE,IAAI,CAAC,OAAO,KAAK,KAAK,QAAQ,IAAI,OAAO,KAAK,KAAK,UAAU,CAAC,IAAI,KAAK,KAAK,IAAI,EAAE;QAChF,IAAM,KAAK,GAAG,OAAO,CAAC,WAAW,CAAC,SAAS,CAAC,UAAA,KAAK,IAAI,OAAA,KAAK,CAAC,GAAG,KAAK,KAAK,EAAnB,CAAmB,CAAC,CAAC;QAC1E,IAAI,KAAK,KAAK,CAAC,CAAC,EAAE;YAChB,IAAM,KAAK,GAAG,OAAO,CAAC,WAAW,CAAC,GAAG,CAAC,UAAA,KAAK,IAAI,OAAA,KAAK,CAAC,YAAY,EAAlB,CAAkB,CAAC,CAAC;YACnE,IAAM,WAAW,GAAG,KAAK;iBACtB,KAAK,CAAC,CAAC,EAAE,KAAK,CAAC;iBACf,GAAG,CAAC,UAAA,IAAI,IAAI,OAAG,IAAI,SAAM,EAAb,CAAa,CAAC;iBAC1B,IAAI,CAAC,EAAE,CAAC,CAAC;YACZ,IAAM,WAAW,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC;YACjC,IAAM,YAAY,GAChB,MAAM;gBACN,KAAK;qBACF,KAAK,CAAC,KAAK,GAAG,CAAC,EAAE,KAAK,CAAC,MAAM,GAAG,CAAC,CAAC;qBAClC,GAAG,CAAC,UAAA,IAAI,IAAI,OAAG,IAAI,SAAM,EAAb,CAAa,CAAC;qBAC1B,IAAI,CAAC,EAAE,CAAC,CAAC;YACd,IAAM,OAAO,GAAG,KAAK,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;YACxC,IAAM,YAAY,GAAG,GAAG,CAAC,MAAM,CAAC,WAAW,CAAC,MAAM,GAAG,WAAW,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;YAC7E,IAAM,MAAM,GAAG,GAAG,CAAC,MAAM,CACvB,YAAY,CAAC,MAAM,GAAG,CAAC,WAAW,CAAC,MAAM,GAAG,OAAO,CAAC,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,CACpE,CAAC;YAEF,MAAM,IAAI,SAAS,CACjB,2CAA2C;iBACzC,SAAO,WAAW,GAAG,WAAW,GAAG,YAAY,GAAG,OAAO,OAAI,CAAA;iBAC7D,SAAO,YAAY,UAAK,MAAM,MAAG,CAAA,CACpC,CAAC;SACH;QACD,OAAO,CAAC,WAAW,CAAC,OAAO,CAAC,WAAW,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC,GAAG,GAAG,KAAK,CAAC;KACjE;IAED,IAAI,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC;QAAE,OAAO,cAAc,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;IAEhE,IAAI,KAAK,KAAK,SAAS;QAAE,OAAO,IAAI,CAAC;IAErC,IAAI,KAAK,YAAY,IAAI,IAAI,cAAM,CAAC,KAAK,CAAC,EAAE;QAC1C,IAAM,OAAO,GAAG,KAAK,CAAC,OAAO,EAAE;QAC7B,iCAAiC;QACjC,OAAO,GAAG,OAAO,GAAG,CAAC,CAAC,IAAI,OAAO,GAAG,eAAe,CAAC;QAEtD,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO,OAAO,CAAC,OAAO,IAAI,OAAO;gBAC/B,CAAC,CAAC,EAAE,KAAK,EAAE,KAAK,CAAC,OAAO,EAAE,EAAE;gBAC5B,CAAC,CAAC,EAAE,KAAK,EAAE,YAAY,CAAC,KAAK,CAAC,EAAE,CAAC;SACpC;QACD,OAAO,OAAO,CAAC,OAAO,IAAI,OAAO;YAC/B,CAAC,CAAC,EAAE,KAAK,EAAE,YAAY,CAAC,KAAK,CAAC,EAAE;YAChC,CAAC,CAAC,EAAE,KAAK,EAAE,EAAE,WAAW,EAAE,KAAK,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE,EAAE,EAAE,CAAC;KAC5D;IAED,IAAI,OAAO,KAAK,KAAK,QAAQ,IAAI,CAAC,CAAC,OAAO,CAAC,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC,EAAE;QACvE,kBAAkB;QAClB,IAAI,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK,EAAE;YAC/B,IAAM,UAAU,GAAG,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc,EACnE,UAAU,GAAG,KAAK,IAAI,cAAc,IAAI,KAAK,IAAI,cAAc,CAAC;YAElE,6FAA6F;YAC7F,IAAI,UAAU;gBAAE,OAAO,EAAE,UAAU,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;YACxD,IAAI,UAAU;gBAAE,OAAO,EAAE,WAAW,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;SAC1D;QACD,OAAO,EAAE,aAAa,EAAE,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;KAC5C;IAED,IAAI,KAAK,YAAY,MAAM,IAAI,gBAAQ,CAAC,KAAK,CAAC,EAAE;QAC9C,IAAI,KAAK,GAAG,KAAK,CAAC,KAAK,CAAC;QACxB,IAAI,KAAK,KAAK,SAAS,EAAE;YACvB,IAAM,KAAK,GAAG,KAAK,CAAC,QAAQ,EAAE,CAAC,KAAK,CAAC,WAAW,CAAC,CAAC;YAClD,IAAI,KAAK,EAAE;gBACT,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;aAClB;SACF;QAED,IAAM,EAAE,GAAG,IAAI,mBAAU,CAAC,KAAK,CAAC,MAAM,EAAE,KAAK,CAAC,CAAC;QAC/C,OAAO,EAAE,CAAC,cAAc,CAAC,OAAO,CAAC,CAAC;KACnC;IAED,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ;QAAE,OAAO,iBAAiB,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC;IACzF,OAAO,KAAK,CAAC;AACf,CAAC;AAED,IAAM,kBAAkB,GAAG;IACzB,MAAM,EAAE,UAAC,CAAS,IAAK,OAAA,IAAI,eAAM,CAAC,CAAC,CAAC,KAAK,EAAE,EAAE,CAAC,CAAC,QAAQ,CAAC,EAAjC,CAAiC;IACxD,IAAI,EAAE,UAAC,CAAO,IAAK,OAAA,IAAI,WAAI,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC,KAAK,CAAC,EAAzB,CAAyB;IAC5C,KAAK,EAAE,UAAC,CAAQ,IAAK,OAAA,IAAI,cAAK,CAAC,CAAC,CAAC,UAAU,IAAI,CAAC,CAAC,SAAS,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,MAAM,CAAC,EAA7D,CAA6D;IAClF,UAAU,EAAE,UAAC,CAAa,IAAK,OAAA,IAAI,uBAAU,CAAC,CAAC,CAAC,KAAK,CAAC,EAAvB,CAAuB;IACtD,MAAM,EAAE,UAAC,CAAS,IAAK,OAAA,IAAI,eAAM,CAAC,CAAC,CAAC,KAAK,CAAC,EAAnB,CAAmB;IAC1C,KAAK,EAAE,UAAC,CAAQ,IAAK,OAAA,IAAI,cAAK,CAAC,CAAC,CAAC,KAAK,CAAC,EAAlB,CAAkB;IACvC,IAAI,EAAE,UACJ,CAIC;QAED,OAAA,WAAI,CAAC,QAAQ;QACX,sDAAsD;QACtD,CAAC,CAAC,GAAG,IAAI,IAAI,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,IAAI,EAC9B,CAAC,CAAC,GAAG,IAAI,IAAI,CAAC,CAAC,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,CAAC,KAAK,EAChC,CAAC,CAAC,GAAG,IAAI,IAAI,CAAC,CAAC,CAAC,CAAC,CAAC,QAAQ,CAAC,CAAC,CAAC,CAAC,CAAC,SAAS,CACzC;IALD,CAKC;IACH,MAAM,EAAE,cAAM,OAAA,IAAI,gBAAM,EAAE,EAAZ,CAAY;IAC1B,MAAM,EAAE,cAAM,OAAA,IAAI,gBAAM,EAAE,EAAZ,CAAY;IAC1B,QAAQ,EAAE,UAAC,CAAW,IAAK,OAAA,IAAI,mBAAQ,CAAC,CAAC,CAAC,EAAf,CAAe;IAC1C,QAAQ,EAAE,UAAC,CAAW,IAAK,OAAA,IAAI,mBAAQ,CAAC,CAAC,CAAC,EAAf,CAAe;IAC1C,UAAU,EAAE,UAAC,CAAa,IAAK,OAAA,IAAI,mBAAU,CAAC,CAAC,CAAC,OAAO,EAAE,CAAC,CAAC,OAAO,CAAC,EAApC,CAAoC;IACnE,MAAM,EAAE,UAAC,CAAa,IAAK,OAAA,IAAI,mBAAU,CAAC,CAAC,CAAC,KAAK,CAAC,EAAvB,CAAuB;IAClD,SAAS,EAAE,UAAC,CAAY,IAAK,OAAA,qBAAS,CAAC,QAAQ,CAAC,CAAC,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,EAAjC,CAAiC;CACtD,CAAC;AAEX,8DAA8D;AAC9D,SAAS,iBAAiB,CAAC,GAAQ,EAAE,OAA8B;IACjE,IAAI,GAAG,IAAI,IAAI,IAAI,OAAO,GAAG,KAAK,QAAQ;QAAE,MAAM,IAAI,KAAK,CAAC,wBAAwB,CAAC,CAAC;IAEtF,IAAM,QAAQ,GAA0B,GAAG,CAAC,SAAS,CAAC;IACtD,IAAI,OAAO,QAAQ,KAAK,WAAW,EAAE;QACnC,oEAAoE;QACpE,IAAM,IAAI,GAAa,EAAE,CAAC;QAC1B,KAAK,IAAM,IAAI,IAAI,GAAG,EAAE;YACtB,OAAO,CAAC,WAAW,CAAC,IAAI,CAAC,EAAE,YAAY,EAAE,IAAI,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC,CAAC;YAC5D,IAAI;gBACF,IAAI,CAAC,IAAI,CAAC,GAAG,cAAc,CAAC,GAAG,CAAC,IAAI,CAAC,EAAE,OAAO,CAAC,CAAC;aACjD;oBAAS;gBACR,OAAO,CAAC,WAAW,CAAC,GAAG,EAAE,CAAC;aAC3B;SACF;QACD,OAAO,IAAI,CAAC;KACb;SAAM,IAAI,UAAU,CAAC,GAAG,CAAC,EAAE;QAC1B,mDAAmD;QACnD,8DAA8D;QAC9D,IAAI,MAAM,GAAQ,GAAG,CAAC;QACtB,IAAI,OAAO,MAAM,CAAC,cAAc,KAAK,UAAU,EAAE;YAC/C,0EAA0E;YAC1E,4EAA4E;YAC5E,gFAAgF;YAChF,4DAA4D;YAC5D,IAAM,MAAM,GAAG,kBAAkB,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;YACjD,IAAI,CAAC,MAAM,EAAE;gBACX,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,GAAG,CAAC,SAAS,CAAC,CAAC;aAC5E;YACD,MAAM,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC;SACzB;QAED,4EAA4E;QAC5E,IAAI,QAAQ,KAAK,MAAM,IAAI,MAAM,CAAC,KAAK,EAAE;YACvC,MAAM,GAAG,IAAI,WAAI,CAAC,MAAM,CAAC,IAAI,EAAE,cAAc,CAAC,MAAM,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC,CAAC;SACvE;aAAM,IAAI,QAAQ,KAAK,OAAO,IAAI,MAAM,CAAC,GAAG,EAAE;YAC7C,MAAM,GAAG,IAAI,cAAK,CAChB,cAAc,CAAC,MAAM,CAAC,UAAU,EAAE,OAAO,CAAC,EAC1C,cAAc,CAAC,MAAM,CAAC,GAAG,EAAE,OAAO,CAAC,EACnC,cAAc,CAAC,MAAM,CAAC,EAAE,EAAE,OAAO,CAAC,EAClC,cAAc,CAAC,MAAM,CAAC,MAAM,EAAE,OAAO,CAAC,CACvC,CAAC;SACH;QAED,OAAO,MAAM,CAAC,cAAc,CAAC,OAAO,CAAC,CAAC;KACvC;SAAM;QACL,MAAM,IAAI,KAAK,CAAC,uCAAuC,GAAG,OAAO,QAAQ,CAAC,CAAC;KAC5E;AACH,CAAC;AAED;;;GAGG;AACH,uEAAuE;AACvE,6DAA6D;AAC7D,2DAA2D;AAC3D,IAAiB,KAAK,CA8GrB;AA9GD,WAAiB,KAAK;IAapB;;;;;;;;;;;;;;;OAeG;IACH,SAAgB,KAAK,CAAC,IAAY,EAAE,OAAuB;QACzD,IAAM,YAAY,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,EAAE,OAAO,EAAE,IAAI,EAAE,MAAM,EAAE,KAAK,EAAE,EAAE,OAAO,CAAC,CAAC;QAElF,6BAA6B;QAC7B,IAAI,OAAO,YAAY,CAAC,OAAO,KAAK,SAAS;YAAE,YAAY,CAAC,MAAM,GAAG,CAAC,YAAY,CAAC,OAAO,CAAC;QAC3F,IAAI,OAAO,YAAY,CAAC,MAAM,KAAK,SAAS;YAAE,YAAY,CAAC,OAAO,GAAG,CAAC,YAAY,CAAC,MAAM,CAAC;QAE1F,OAAO,IAAI,CAAC,KAAK,CAAC,IAAI,EAAE,UAAC,IAAI,EAAE,KAAK,IAAK,OAAA,gBAAgB,CAAC,KAAK,EAAE,YAAY,CAAC,EAArC,CAAqC,CAAC,CAAC;IAClF,CAAC;IARe,WAAK,QAQpB,CAAA;IAKD;;;;;;;;;;;;;;;;;;;;;;OAsBG;IACH,SAAgB,SAAS,CACvB,KAAwB;IACxB,8DAA8D;IAC9D,QAA8F,EAC9F,KAAuB,EACvB,OAAuB;QAEvB,IAAI,KAAK,IAAI,IAAI,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YAC9C,OAAO,GAAG,KAAK,CAAC;YAChB,KAAK,GAAG,CAAC,CAAC;SACX;QACD,IAAI,QAAQ,IAAI,IAAI,IAAI,OAAO,QAAQ,KAAK,QAAQ,IAAI,CAAC,KAAK,CAAC,OAAO,CAAC,QAAQ,CAAC,EAAE;YAChF,OAAO,GAAG,QAAQ,CAAC;YACnB,QAAQ,GAAG,SAAS,CAAC;YACrB,KAAK,GAAG,CAAC,CAAC;SACX;QACD,IAAM,gBAAgB,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,OAAO,EAAE,IAAI,EAAE,MAAM,EAAE,KAAK,EAAE,EAAE,OAAO,EAAE;YAChF,WAAW,EAAE,CAAC,EAAE,YAAY,EAAE,QAAQ,EAAE,GAAG,EAAE,IAAI,EAAE,CAAC;SACrD,CAAC,CAAC;QAEH,IAAM,GAAG,GAAG,cAAc,CAAC,KAAK,EAAE,gBAAgB,CAAC,CAAC;QACpD,OAAO,IAAI,CAAC,SAAS,CAAC,GAAG,EAAE,QAA4C,EAAE,KAAK,CAAC,CAAC;IAClF,CAAC;IAtBe,eAAS,YAsBxB,CAAA;IAED;;;;;OAKG;IACH,SAAgB,SAAS,CAAC,KAAwB,EAAE,OAAuB;QACzE,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,OAAO,IAAI,CAAC,KAAK,CAAC,SAAS,CAAC,KAAK,EAAE,OAAO,CAAC,CAAC,CAAC;IAC/C,CAAC;IAHe,eAAS,YAGxB,CAAA;IAED;;;;;OAKG;IACH,SAAgB,WAAW,CAAC,KAAe,EAAE,OAAuB;QAClE,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,OAAO,KAAK,CAAC,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,EAAE,OAAO,CAAC,CAAC;IAC/C,CAAC;IAHe,iBAAW,cAG1B,CAAA;AACH,CAAC,EA9GgB,KAAK,GAAL,aAAK,KAAL,aAAK,QA8GrB"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/float_parser.js b/node_modules/bson/lib/float_parser.js
new file mode 100644
index 0000000..f78a3a0
--- /dev/null
+++ b/node_modules/bson/lib/float_parser.js
@@ -0,0 +1,137 @@
+"use strict";
+// Copyright (c) 2008, Fair Oaks Labs, Inc.
+// All rights reserved.
+//
+// Redistribution and use in source and binary forms, with or without
+// modification, are permitted provided that the following conditions are met:
+//
+// * Redistributions of source code must retain the above copyright notice,
+// this list of conditions and the following disclaimer.
+//
+// * Redistributions in binary form must reproduce the above copyright notice,
+// this list of conditions and the following disclaimer in the documentation
+// and/or other materials provided with the distribution.
+//
+// * Neither the name of Fair Oaks Labs, Inc. nor the names of its contributors
+// may be used to endorse or promote products derived from this software
+// without specific prior written permission.
+//
+// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+// AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
+// ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
+// LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
+// CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
+// SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
+// INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
+// CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
+// ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
+// POSSIBILITY OF SUCH DAMAGE.
+//
+//
+// Modifications to writeIEEE754 to support negative zeroes made by Brian White
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.writeIEEE754 = exports.readIEEE754 = void 0;
+function readIEEE754(buffer, offset, endian, mLen, nBytes) {
+ var e;
+ var m;
+ var bBE = endian === 'big';
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var nBits = -7;
+ var i = bBE ? 0 : nBytes - 1;
+ var d = bBE ? 1 : -1;
+ var s = buffer[offset + i];
+ i += d;
+ e = s & ((1 << -nBits) - 1);
+ s >>= -nBits;
+ nBits += eLen;
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8)
+ ;
+ m = e & ((1 << -nBits) - 1);
+ e >>= -nBits;
+ nBits += mLen;
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8)
+ ;
+ if (e === 0) {
+ e = 1 - eBias;
+ }
+ else if (e === eMax) {
+ return m ? NaN : (s ? -1 : 1) * Infinity;
+ }
+ else {
+ m = m + Math.pow(2, mLen);
+ e = e - eBias;
+ }
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
+}
+exports.readIEEE754 = readIEEE754;
+function writeIEEE754(buffer, value, offset, endian, mLen, nBytes) {
+ var e;
+ var m;
+ var c;
+ var bBE = endian === 'big';
+ var eLen = nBytes * 8 - mLen - 1;
+ var eMax = (1 << eLen) - 1;
+ var eBias = eMax >> 1;
+ var rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0;
+ var i = bBE ? nBytes - 1 : 0;
+ var d = bBE ? -1 : 1;
+ var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0;
+ value = Math.abs(value);
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0;
+ e = eMax;
+ }
+ else {
+ e = Math.floor(Math.log(value) / Math.LN2);
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--;
+ c *= 2;
+ }
+ if (e + eBias >= 1) {
+ value += rt / c;
+ }
+ else {
+ value += rt * Math.pow(2, 1 - eBias);
+ }
+ if (value * c >= 2) {
+ e++;
+ c /= 2;
+ }
+ if (e + eBias >= eMax) {
+ m = 0;
+ e = eMax;
+ }
+ else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen);
+ e = e + eBias;
+ }
+ else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
+ e = 0;
+ }
+ }
+ if (isNaN(value))
+ m = 0;
+ while (mLen >= 8) {
+ buffer[offset + i] = m & 0xff;
+ i += d;
+ m /= 256;
+ mLen -= 8;
+ }
+ e = (e << mLen) | m;
+ if (isNaN(value))
+ e += 8;
+ eLen += mLen;
+ while (eLen > 0) {
+ buffer[offset + i] = e & 0xff;
+ i += d;
+ e /= 256;
+ eLen -= 8;
+ }
+ buffer[offset + i - d] |= s * 128;
+}
+exports.writeIEEE754 = writeIEEE754;
+//# sourceMappingURL=float_parser.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/float_parser.js.map b/node_modules/bson/lib/float_parser.js.map
new file mode 100644
index 0000000..5b68843
--- /dev/null
+++ b/node_modules/bson/lib/float_parser.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"float_parser.js","sourceRoot":"","sources":["../src/float_parser.ts"],"names":[],"mappings":";AAAA,2CAA2C;AAC3C,uBAAuB;AACvB,EAAE;AACF,qEAAqE;AACrE,8EAA8E;AAC9E,EAAE;AACF,4EAA4E;AAC5E,2DAA2D;AAC3D,EAAE;AACF,+EAA+E;AAC/E,+EAA+E;AAC/E,4DAA4D;AAC5D,EAAE;AACF,gFAAgF;AAChF,2EAA2E;AAC3E,gDAAgD;AAChD,EAAE;AACF,8EAA8E;AAC9E,4EAA4E;AAC5E,6EAA6E;AAC7E,4EAA4E;AAC5E,sEAAsE;AACtE,uEAAuE;AACvE,2EAA2E;AAC3E,0EAA0E;AAC1E,0EAA0E;AAC1E,6EAA6E;AAC7E,8BAA8B;AAC9B,EAAE;AACF,EAAE;AACF,+EAA+E;;;AAI/E,SAAgB,WAAW,CACzB,MAAyB,EACzB,MAAc,EACd,MAAwB,EACxB,IAAY,EACZ,MAAc;IAEd,IAAI,CAAS,CAAC;IACd,IAAI,CAAS,CAAC;IACd,IAAM,GAAG,GAAG,MAAM,KAAK,KAAK,CAAC;IAC7B,IAAM,IAAI,GAAG,MAAM,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;IACnC,IAAM,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,CAAC;IAC7B,IAAM,KAAK,GAAG,IAAI,IAAI,CAAC,CAAC;IACxB,IAAI,KAAK,GAAG,CAAC,CAAC,CAAC;IACf,IAAI,CAAC,GAAG,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,MAAM,GAAG,CAAC,CAAC;IAC7B,IAAM,CAAC,GAAG,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;IACvB,IAAI,CAAC,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;IAE3B,CAAC,IAAI,CAAC,CAAC;IAEP,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;IAC5B,CAAC,KAAK,CAAC,KAAK,CAAC;IACb,KAAK,IAAI,IAAI,CAAC;IACd,OAAO,KAAK,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,KAAK,IAAI,CAAC;QAAC,CAAC;IAExE,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC,CAAC;IAC5B,CAAC,KAAK,CAAC,KAAK,CAAC;IACb,KAAK,IAAI,IAAI,CAAC;IACd,OAAO,KAAK,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,EAAE,CAAC,IAAI,CAAC,EAAE,KAAK,IAAI,CAAC;QAAC,CAAC;IAExE,IAAI,CAAC,KAAK,CAAC,EAAE;QACX,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;KACf;SAAM,IAAI,CAAC,KAAK,IAAI,EAAE;QACrB,OAAO,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,QAAQ,CAAC;KAC1C;SAAM;QACL,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;QAC1B,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;KACf;IACD,OAAO,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,CAAC;AAClD,CAAC;AAvCD,kCAuCC;AAED,SAAgB,YAAY,CAC1B,MAAyB,EACzB,KAAa,EACb,MAAc,EACd,MAAwB,EACxB,IAAY,EACZ,MAAc;IAEd,IAAI,CAAS,CAAC;IACd,IAAI,CAAS,CAAC;IACd,IAAI,CAAS,CAAC;IACd,IAAM,GAAG,GAAG,MAAM,KAAK,KAAK,CAAC;IAC7B,IAAI,IAAI,GAAG,MAAM,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;IACjC,IAAM,IAAI,GAAG,CAAC,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,CAAC;IAC7B,IAAM,KAAK,GAAG,IAAI,IAAI,CAAC,CAAC;IACxB,IAAM,EAAE,GAAG,IAAI,KAAK,EAAE,CAAC,CAAC,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;IACjE,IAAI,CAAC,GAAG,GAAG,CAAC,CAAC,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;IAC7B,IAAM,CAAC,GAAG,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;IACvB,IAAM,CAAC,GAAG,KAAK,GAAG,CAAC,IAAI,CAAC,KAAK,KAAK,CAAC,IAAI,CAAC,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;IAE9D,KAAK,GAAG,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC;IAExB,IAAI,KAAK,CAAC,KAAK,CAAC,IAAI,KAAK,KAAK,QAAQ,EAAE;QACtC,CAAC,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;QACzB,CAAC,GAAG,IAAI,CAAC;KACV;SAAM;QACL,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC;QAC3C,IAAI,KAAK,GAAG,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,EAAE;YACrC,CAAC,EAAE,CAAC;YACJ,CAAC,IAAI,CAAC,CAAC;SACR;QACD,IAAI,CAAC,GAAG,KAAK,IAAI,CAAC,EAAE;YAClB,KAAK,IAAI,EAAE,GAAG,CAAC,CAAC;SACjB;aAAM;YACL,KAAK,IAAI,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,CAAC,GAAG,KAAK,CAAC,CAAC;SACtC;QACD,IAAI,KAAK,GAAG,CAAC,IAAI,CAAC,EAAE;YAClB,CAAC,EAAE,CAAC;YACJ,CAAC,IAAI,CAAC,CAAC;SACR;QAED,IAAI,CAAC,GAAG,KAAK,IAAI,IAAI,EAAE;YACrB,CAAC,GAAG,CAAC,CAAC;YACN,CAAC,GAAG,IAAI,CAAC;SACV;aAAM,IAAI,CAAC,GAAG,KAAK,IAAI,CAAC,EAAE;YACzB,CAAC,GAAG,CAAC,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;YACxC,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;SACf;aAAM;YACL,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,KAAK,GAAG,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;YACvD,CAAC,GAAG,CAAC,CAAC;SACP;KACF;IAED,IAAI,KAAK,CAAC,KAAK,CAAC;QAAE,CAAC,GAAG,CAAC,CAAC;IAExB,OAAO,IAAI,IAAI,CAAC,EAAE;QAChB,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC;QAC9B,CAAC,IAAI,CAAC,CAAC;QACP,CAAC,IAAI,GAAG,CAAC;QACT,IAAI,IAAI,CAAC,CAAC;KACX;IAED,CAAC,GAAG,CAAC,CAAC,IAAI,IAAI,CAAC,GAAG,CAAC,CAAC;IAEpB,IAAI,KAAK,CAAC,KAAK,CAAC;QAAE,CAAC,IAAI,CAAC,CAAC;IAEzB,IAAI,IAAI,IAAI,CAAC;IAEb,OAAO,IAAI,GAAG,CAAC,EAAE;QACf,MAAM,CAAC,MAAM,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC;QAC9B,CAAC,IAAI,CAAC,CAAC;QACP,CAAC,IAAI,GAAG,CAAC;QACT,IAAI,IAAI,CAAC,CAAC;KACX;IAED,MAAM,CAAC,MAAM,GAAG,CAAC,GAAG,CAAC,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC;AACpC,CAAC;AA5ED,oCA4EC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/int_32.js b/node_modules/bson/lib/int_32.js
new file mode 100644
index 0000000..a442427
--- /dev/null
+++ b/node_modules/bson/lib/int_32.js
@@ -0,0 +1,55 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Int32 = void 0;
+/**
+ * A class representation of a BSON Int32 type.
+ * @public
+ */
+var Int32 = /** @class */ (function () {
+ /**
+ * Create an Int32 type
+ *
+ * @param value - the number we want to represent as an int32.
+ */
+ function Int32(value) {
+ if (!(this instanceof Int32))
+ return new Int32(value);
+ if (value instanceof Number) {
+ value = value.valueOf();
+ }
+ this.value = +value;
+ }
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped int32 number.
+ */
+ Int32.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ Int32.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ Int32.prototype.toExtendedJSON = function (options) {
+ if (options && (options.relaxed || options.legacy))
+ return this.value;
+ return { $numberInt: this.value.toString() };
+ };
+ /** @internal */
+ Int32.fromExtendedJSON = function (doc, options) {
+ return options && options.relaxed ? parseInt(doc.$numberInt, 10) : new Int32(doc.$numberInt);
+ };
+ /** @internal */
+ Int32.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Int32.prototype.inspect = function () {
+ return "new Int32(" + this.valueOf() + ")";
+ };
+ return Int32;
+}());
+exports.Int32 = Int32;
+Object.defineProperty(Int32.prototype, '_bsontype', { value: 'Int32' });
+//# sourceMappingURL=int_32.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/int_32.js.map b/node_modules/bson/lib/int_32.js.map
new file mode 100644
index 0000000..8dcf5eb
--- /dev/null
+++ b/node_modules/bson/lib/int_32.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"int_32.js","sourceRoot":"","sources":["../src/int_32.ts"],"names":[],"mappings":";;;AAOA;;;GAGG;AACH;IAIE;;;;OAIG;IACH,eAAY,KAAsB;QAChC,IAAI,CAAC,CAAC,IAAI,YAAY,KAAK,CAAC;YAAE,OAAO,IAAI,KAAK,CAAC,KAAK,CAAC,CAAC;QAEtD,IAAK,KAAiB,YAAY,MAAM,EAAE;YACxC,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;SACzB;QAED,IAAI,CAAC,KAAK,GAAG,CAAC,KAAK,CAAC;IACtB,CAAC;IAED;;;;OAIG;IACH,uBAAO,GAAP;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;IACpB,CAAC;IAED,gBAAgB;IAChB,sBAAM,GAAN;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;IACpB,CAAC;IAED,gBAAgB;IAChB,8BAAc,GAAd,UAAe,OAAsB;QACnC,IAAI,OAAO,IAAI,CAAC,OAAO,CAAC,OAAO,IAAI,OAAO,CAAC,MAAM,CAAC;YAAE,OAAO,IAAI,CAAC,KAAK,CAAC;QACtE,OAAO,EAAE,UAAU,EAAE,IAAI,CAAC,KAAK,CAAC,QAAQ,EAAE,EAAE,CAAC;IAC/C,CAAC;IAED,gBAAgB;IACT,sBAAgB,GAAvB,UAAwB,GAAkB,EAAE,OAAsB;QAChE,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,CAAC,CAAC,CAAC,QAAQ,CAAC,GAAG,CAAC,UAAU,EAAE,EAAE,CAAC,CAAC,CAAC,CAAC,IAAI,KAAK,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC;IAC/F,CAAC;IAED,gBAAgB;IAChB,gBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,uBAAO,GAAP;QACE,OAAO,eAAa,IAAI,CAAC,OAAO,EAAE,MAAG,CAAC;IACxC,CAAC;IACH,YAAC;AAAD,CAAC,AApDD,IAoDC;AApDY,sBAAK;AAsDlB,MAAM,CAAC,cAAc,CAAC,KAAK,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,OAAO,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/long.js b/node_modules/bson/lib/long.js
new file mode 100644
index 0000000..3de7b1a
--- /dev/null
+++ b/node_modules/bson/lib/long.js
@@ -0,0 +1,899 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Long = void 0;
+var utils_1 = require("./parser/utils");
+/**
+ * wasm optimizations, to do native i64 multiplication and divide
+ */
+var wasm = undefined;
+try {
+ wasm = new WebAssembly.Instance(new WebAssembly.Module(
+ // prettier-ignore
+ new Uint8Array([0, 97, 115, 109, 1, 0, 0, 0, 1, 13, 2, 96, 0, 1, 127, 96, 4, 127, 127, 127, 127, 1, 127, 3, 7, 6, 0, 1, 1, 1, 1, 1, 6, 6, 1, 127, 1, 65, 0, 11, 7, 50, 6, 3, 109, 117, 108, 0, 1, 5, 100, 105, 118, 95, 115, 0, 2, 5, 100, 105, 118, 95, 117, 0, 3, 5, 114, 101, 109, 95, 115, 0, 4, 5, 114, 101, 109, 95, 117, 0, 5, 8, 103, 101, 116, 95, 104, 105, 103, 104, 0, 0, 10, 191, 1, 6, 4, 0, 35, 0, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 126, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 127, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 128, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 129, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 130, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11])), {}).exports;
+}
+catch (_a) {
+ // no wasm support
+}
+var TWO_PWR_16_DBL = 1 << 16;
+var TWO_PWR_24_DBL = 1 << 24;
+var TWO_PWR_32_DBL = TWO_PWR_16_DBL * TWO_PWR_16_DBL;
+var TWO_PWR_64_DBL = TWO_PWR_32_DBL * TWO_PWR_32_DBL;
+var TWO_PWR_63_DBL = TWO_PWR_64_DBL / 2;
+/** A cache of the Long representations of small integer values. */
+var INT_CACHE = {};
+/** A cache of the Long representations of small unsigned integer values. */
+var UINT_CACHE = {};
+/**
+ * A class representing a 64-bit integer
+ * @public
+ * @remarks
+ * The internal representation of a long is the two given signed, 32-bit values.
+ * We use 32-bit pieces because these are the size of integers on which
+ * Javascript performs bit-operations. For operations like addition and
+ * multiplication, we split each number into 16 bit pieces, which can easily be
+ * multiplied within Javascript's floating-point representation without overflow
+ * or change in sign.
+ * In the algorithms below, we frequently reduce the negative case to the
+ * positive case by negating the input(s) and then post-processing the result.
+ * Note that we must ALWAYS check specially whether those values are MIN_VALUE
+ * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
+ * a positive number, it overflows back into a negative). Not handling this
+ * case would often result in infinite recursion.
+ * Common constant values ZERO, ONE, NEG_ONE, etc. are found as static properties on this class.
+ */
+var Long = /** @class */ (function () {
+ /**
+ * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers.
+ * See the from* functions below for more convenient ways of constructing Longs.
+ *
+ * Acceptable signatures are:
+ * - Long(low, high, unsigned?)
+ * - Long(bigint, unsigned?)
+ * - Long(string, unsigned?)
+ *
+ * @param low - The low (signed) 32 bits of the long
+ * @param high - The high (signed) 32 bits of the long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ function Long(low, high, unsigned) {
+ if (low === void 0) { low = 0; }
+ if (!(this instanceof Long))
+ return new Long(low, high, unsigned);
+ if (typeof low === 'bigint') {
+ Object.assign(this, Long.fromBigInt(low, !!high));
+ }
+ else if (typeof low === 'string') {
+ Object.assign(this, Long.fromString(low, !!high));
+ }
+ else {
+ this.low = low | 0;
+ this.high = high | 0;
+ this.unsigned = !!unsigned;
+ }
+ Object.defineProperty(this, '__isLong__', {
+ value: true,
+ configurable: false,
+ writable: false,
+ enumerable: false
+ });
+ }
+ /**
+ * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits.
+ * Each is assumed to use 32 bits.
+ * @param lowBits - The low 32 bits
+ * @param highBits - The high 32 bits
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBits = function (lowBits, highBits, unsigned) {
+ return new Long(lowBits, highBits, unsigned);
+ };
+ /**
+ * Returns a Long representing the given 32 bit integer value.
+ * @param value - The 32 bit integer in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromInt = function (value, unsigned) {
+ var obj, cachedObj, cache;
+ if (unsigned) {
+ value >>>= 0;
+ if ((cache = 0 <= value && value < 256)) {
+ cachedObj = UINT_CACHE[value];
+ if (cachedObj)
+ return cachedObj;
+ }
+ obj = Long.fromBits(value, (value | 0) < 0 ? -1 : 0, true);
+ if (cache)
+ UINT_CACHE[value] = obj;
+ return obj;
+ }
+ else {
+ value |= 0;
+ if ((cache = -128 <= value && value < 128)) {
+ cachedObj = INT_CACHE[value];
+ if (cachedObj)
+ return cachedObj;
+ }
+ obj = Long.fromBits(value, value < 0 ? -1 : 0, false);
+ if (cache)
+ INT_CACHE[value] = obj;
+ return obj;
+ }
+ };
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromNumber = function (value, unsigned) {
+ if (isNaN(value))
+ return unsigned ? Long.UZERO : Long.ZERO;
+ if (unsigned) {
+ if (value < 0)
+ return Long.UZERO;
+ if (value >= TWO_PWR_64_DBL)
+ return Long.MAX_UNSIGNED_VALUE;
+ }
+ else {
+ if (value <= -TWO_PWR_63_DBL)
+ return Long.MIN_VALUE;
+ if (value + 1 >= TWO_PWR_63_DBL)
+ return Long.MAX_VALUE;
+ }
+ if (value < 0)
+ return Long.fromNumber(-value, unsigned).neg();
+ return Long.fromBits(value % TWO_PWR_32_DBL | 0, (value / TWO_PWR_32_DBL) | 0, unsigned);
+ };
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBigInt = function (value, unsigned) {
+ return Long.fromString(value.toString(), unsigned);
+ };
+ /**
+ * Returns a Long representation of the given string, written using the specified radix.
+ * @param str - The textual representation of the Long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param radix - The radix in which the text is written (2-36), defaults to 10
+ * @returns The corresponding Long value
+ */
+ Long.fromString = function (str, unsigned, radix) {
+ if (str.length === 0)
+ throw Error('empty string');
+ if (str === 'NaN' || str === 'Infinity' || str === '+Infinity' || str === '-Infinity')
+ return Long.ZERO;
+ if (typeof unsigned === 'number') {
+ // For goog.math.long compatibility
+ (radix = unsigned), (unsigned = false);
+ }
+ else {
+ unsigned = !!unsigned;
+ }
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix)
+ throw RangeError('radix');
+ var p;
+ if ((p = str.indexOf('-')) > 0)
+ throw Error('interior hyphen');
+ else if (p === 0) {
+ return Long.fromString(str.substring(1), unsigned, radix).neg();
+ }
+ // Do several (8) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ var radixToPower = Long.fromNumber(Math.pow(radix, 8));
+ var result = Long.ZERO;
+ for (var i = 0; i < str.length; i += 8) {
+ var size = Math.min(8, str.length - i), value = parseInt(str.substring(i, i + size), radix);
+ if (size < 8) {
+ var power = Long.fromNumber(Math.pow(radix, size));
+ result = result.mul(power).add(Long.fromNumber(value));
+ }
+ else {
+ result = result.mul(radixToPower);
+ result = result.add(Long.fromNumber(value));
+ }
+ }
+ result.unsigned = unsigned;
+ return result;
+ };
+ /**
+ * Creates a Long from its byte representation.
+ * @param bytes - Byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns The corresponding Long value
+ */
+ Long.fromBytes = function (bytes, unsigned, le) {
+ return le ? Long.fromBytesLE(bytes, unsigned) : Long.fromBytesBE(bytes, unsigned);
+ };
+ /**
+ * Creates a Long from its little endian byte representation.
+ * @param bytes - Little endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBytesLE = function (bytes, unsigned) {
+ return new Long(bytes[0] | (bytes[1] << 8) | (bytes[2] << 16) | (bytes[3] << 24), bytes[4] | (bytes[5] << 8) | (bytes[6] << 16) | (bytes[7] << 24), unsigned);
+ };
+ /**
+ * Creates a Long from its big endian byte representation.
+ * @param bytes - Big endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ Long.fromBytesBE = function (bytes, unsigned) {
+ return new Long((bytes[4] << 24) | (bytes[5] << 16) | (bytes[6] << 8) | bytes[7], (bytes[0] << 24) | (bytes[1] << 16) | (bytes[2] << 8) | bytes[3], unsigned);
+ };
+ /**
+ * Tests if the specified object is a Long.
+ */
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any, @typescript-eslint/explicit-module-boundary-types
+ Long.isLong = function (value) {
+ return utils_1.isObjectLike(value) && value['__isLong__'] === true;
+ };
+ /**
+ * Converts the specified value to a Long.
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ Long.fromValue = function (val, unsigned) {
+ if (typeof val === 'number')
+ return Long.fromNumber(val, unsigned);
+ if (typeof val === 'string')
+ return Long.fromString(val, unsigned);
+ // Throws for non-objects, converts non-instanceof Long:
+ return Long.fromBits(val.low, val.high, typeof unsigned === 'boolean' ? unsigned : val.unsigned);
+ };
+ /** Returns the sum of this and the specified Long. */
+ Long.prototype.add = function (addend) {
+ if (!Long.isLong(addend))
+ addend = Long.fromValue(addend);
+ // Divide each number into 4 chunks of 16 bits, and then sum the chunks.
+ var a48 = this.high >>> 16;
+ var a32 = this.high & 0xffff;
+ var a16 = this.low >>> 16;
+ var a00 = this.low & 0xffff;
+ var b48 = addend.high >>> 16;
+ var b32 = addend.high & 0xffff;
+ var b16 = addend.low >>> 16;
+ var b00 = addend.low & 0xffff;
+ var c48 = 0, c32 = 0, c16 = 0, c00 = 0;
+ c00 += a00 + b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 + b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 + b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 + b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ };
+ /**
+ * Returns the sum of this and the specified Long.
+ * @returns Sum
+ */
+ Long.prototype.and = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low & other.low, this.high & other.high, this.unsigned);
+ };
+ /**
+ * Compares this Long's value with the specified's.
+ * @returns 0 if they are the same, 1 if the this is greater and -1 if the given one is greater
+ */
+ Long.prototype.compare = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ if (this.eq(other))
+ return 0;
+ var thisNeg = this.isNegative(), otherNeg = other.isNegative();
+ if (thisNeg && !otherNeg)
+ return -1;
+ if (!thisNeg && otherNeg)
+ return 1;
+ // At this point the sign bits are the same
+ if (!this.unsigned)
+ return this.sub(other).isNegative() ? -1 : 1;
+ // Both are positive if at least one is unsigned
+ return other.high >>> 0 > this.high >>> 0 ||
+ (other.high === this.high && other.low >>> 0 > this.low >>> 0)
+ ? -1
+ : 1;
+ };
+ /** This is an alias of {@link Long.compare} */
+ Long.prototype.comp = function (other) {
+ return this.compare(other);
+ };
+ /**
+ * Returns this Long divided by the specified. The result is signed if this Long is signed or unsigned if this Long is unsigned.
+ * @returns Quotient
+ */
+ Long.prototype.divide = function (divisor) {
+ if (!Long.isLong(divisor))
+ divisor = Long.fromValue(divisor);
+ if (divisor.isZero())
+ throw Error('division by zero');
+ // use wasm support if present
+ if (wasm) {
+ // guard against signed division overflow: the largest
+ // negative number / -1 would be 1 larger than the largest
+ // positive number, due to two's complement.
+ if (!this.unsigned &&
+ this.high === -0x80000000 &&
+ divisor.low === -1 &&
+ divisor.high === -1) {
+ // be consistent with non-wasm code path
+ return this;
+ }
+ var low = (this.unsigned ? wasm.div_u : wasm.div_s)(this.low, this.high, divisor.low, divisor.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ if (this.isZero())
+ return this.unsigned ? Long.UZERO : Long.ZERO;
+ var approx, rem, res;
+ if (!this.unsigned) {
+ // This section is only relevant for signed longs and is derived from the
+ // closure library as a whole.
+ if (this.eq(Long.MIN_VALUE)) {
+ if (divisor.eq(Long.ONE) || divisor.eq(Long.NEG_ONE))
+ return Long.MIN_VALUE;
+ // recall that -MIN_VALUE == MIN_VALUE
+ else if (divisor.eq(Long.MIN_VALUE))
+ return Long.ONE;
+ else {
+ // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.
+ var halfThis = this.shr(1);
+ approx = halfThis.div(divisor).shl(1);
+ if (approx.eq(Long.ZERO)) {
+ return divisor.isNegative() ? Long.ONE : Long.NEG_ONE;
+ }
+ else {
+ rem = this.sub(divisor.mul(approx));
+ res = approx.add(rem.div(divisor));
+ return res;
+ }
+ }
+ }
+ else if (divisor.eq(Long.MIN_VALUE))
+ return this.unsigned ? Long.UZERO : Long.ZERO;
+ if (this.isNegative()) {
+ if (divisor.isNegative())
+ return this.neg().div(divisor.neg());
+ return this.neg().div(divisor).neg();
+ }
+ else if (divisor.isNegative())
+ return this.div(divisor.neg()).neg();
+ res = Long.ZERO;
+ }
+ else {
+ // The algorithm below has not been made for unsigned longs. It's therefore
+ // required to take special care of the MSB prior to running it.
+ if (!divisor.unsigned)
+ divisor = divisor.toUnsigned();
+ if (divisor.gt(this))
+ return Long.UZERO;
+ if (divisor.gt(this.shru(1)))
+ // 15 >>> 1 = 7 ; with divisor = 8 ; true
+ return Long.UONE;
+ res = Long.UZERO;
+ }
+ // Repeat the following until the remainder is less than other: find a
+ // floating-point that approximates remainder / other *from below*, add this
+ // into the result, and subtract it from the remainder. It is critical that
+ // the approximate value is less than or equal to the real value so that the
+ // remainder never becomes negative.
+ rem = this;
+ while (rem.gte(divisor)) {
+ // Approximate the result of division. This may be a little greater or
+ // smaller than the actual value.
+ approx = Math.max(1, Math.floor(rem.toNumber() / divisor.toNumber()));
+ // We will tweak the approximate result by changing it in the 48-th digit or
+ // the smallest non-fractional digit, whichever is larger.
+ var log2 = Math.ceil(Math.log(approx) / Math.LN2);
+ var delta = log2 <= 48 ? 1 : Math.pow(2, log2 - 48);
+ // Decrease the approximation until it is smaller than the remainder. Note
+ // that if it is too large, the product overflows and is negative.
+ var approxRes = Long.fromNumber(approx);
+ var approxRem = approxRes.mul(divisor);
+ while (approxRem.isNegative() || approxRem.gt(rem)) {
+ approx -= delta;
+ approxRes = Long.fromNumber(approx, this.unsigned);
+ approxRem = approxRes.mul(divisor);
+ }
+ // We know the answer can't be zero... and actually, zero would cause
+ // infinite recursion since we would make no progress.
+ if (approxRes.isZero())
+ approxRes = Long.ONE;
+ res = res.add(approxRes);
+ rem = rem.sub(approxRem);
+ }
+ return res;
+ };
+ /**This is an alias of {@link Long.divide} */
+ Long.prototype.div = function (divisor) {
+ return this.divide(divisor);
+ };
+ /**
+ * Tests if this Long's value equals the specified's.
+ * @param other - Other value
+ */
+ Long.prototype.equals = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ if (this.unsigned !== other.unsigned && this.high >>> 31 === 1 && other.high >>> 31 === 1)
+ return false;
+ return this.high === other.high && this.low === other.low;
+ };
+ /** This is an alias of {@link Long.equals} */
+ Long.prototype.eq = function (other) {
+ return this.equals(other);
+ };
+ /** Gets the high 32 bits as a signed integer. */
+ Long.prototype.getHighBits = function () {
+ return this.high;
+ };
+ /** Gets the high 32 bits as an unsigned integer. */
+ Long.prototype.getHighBitsUnsigned = function () {
+ return this.high >>> 0;
+ };
+ /** Gets the low 32 bits as a signed integer. */
+ Long.prototype.getLowBits = function () {
+ return this.low;
+ };
+ /** Gets the low 32 bits as an unsigned integer. */
+ Long.prototype.getLowBitsUnsigned = function () {
+ return this.low >>> 0;
+ };
+ /** Gets the number of bits needed to represent the absolute value of this Long. */
+ Long.prototype.getNumBitsAbs = function () {
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ return this.eq(Long.MIN_VALUE) ? 64 : this.neg().getNumBitsAbs();
+ }
+ var val = this.high !== 0 ? this.high : this.low;
+ var bit;
+ for (bit = 31; bit > 0; bit--)
+ if ((val & (1 << bit)) !== 0)
+ break;
+ return this.high !== 0 ? bit + 33 : bit + 1;
+ };
+ /** Tests if this Long's value is greater than the specified's. */
+ Long.prototype.greaterThan = function (other) {
+ return this.comp(other) > 0;
+ };
+ /** This is an alias of {@link Long.greaterThan} */
+ Long.prototype.gt = function (other) {
+ return this.greaterThan(other);
+ };
+ /** Tests if this Long's value is greater than or equal the specified's. */
+ Long.prototype.greaterThanOrEqual = function (other) {
+ return this.comp(other) >= 0;
+ };
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ Long.prototype.gte = function (other) {
+ return this.greaterThanOrEqual(other);
+ };
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ Long.prototype.ge = function (other) {
+ return this.greaterThanOrEqual(other);
+ };
+ /** Tests if this Long's value is even. */
+ Long.prototype.isEven = function () {
+ return (this.low & 1) === 0;
+ };
+ /** Tests if this Long's value is negative. */
+ Long.prototype.isNegative = function () {
+ return !this.unsigned && this.high < 0;
+ };
+ /** Tests if this Long's value is odd. */
+ Long.prototype.isOdd = function () {
+ return (this.low & 1) === 1;
+ };
+ /** Tests if this Long's value is positive. */
+ Long.prototype.isPositive = function () {
+ return this.unsigned || this.high >= 0;
+ };
+ /** Tests if this Long's value equals zero. */
+ Long.prototype.isZero = function () {
+ return this.high === 0 && this.low === 0;
+ };
+ /** Tests if this Long's value is less than the specified's. */
+ Long.prototype.lessThan = function (other) {
+ return this.comp(other) < 0;
+ };
+ /** This is an alias of {@link Long#lessThan}. */
+ Long.prototype.lt = function (other) {
+ return this.lessThan(other);
+ };
+ /** Tests if this Long's value is less than or equal the specified's. */
+ Long.prototype.lessThanOrEqual = function (other) {
+ return this.comp(other) <= 0;
+ };
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ Long.prototype.lte = function (other) {
+ return this.lessThanOrEqual(other);
+ };
+ /** Returns this Long modulo the specified. */
+ Long.prototype.modulo = function (divisor) {
+ if (!Long.isLong(divisor))
+ divisor = Long.fromValue(divisor);
+ // use wasm support if present
+ if (wasm) {
+ var low = (this.unsigned ? wasm.rem_u : wasm.rem_s)(this.low, this.high, divisor.low, divisor.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ return this.sub(this.div(divisor).mul(divisor));
+ };
+ /** This is an alias of {@link Long.modulo} */
+ Long.prototype.mod = function (divisor) {
+ return this.modulo(divisor);
+ };
+ /** This is an alias of {@link Long.modulo} */
+ Long.prototype.rem = function (divisor) {
+ return this.modulo(divisor);
+ };
+ /**
+ * Returns the product of this and the specified Long.
+ * @param multiplier - Multiplier
+ * @returns Product
+ */
+ Long.prototype.multiply = function (multiplier) {
+ if (this.isZero())
+ return Long.ZERO;
+ if (!Long.isLong(multiplier))
+ multiplier = Long.fromValue(multiplier);
+ // use wasm support if present
+ if (wasm) {
+ var low = wasm.mul(this.low, this.high, multiplier.low, multiplier.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+ if (multiplier.isZero())
+ return Long.ZERO;
+ if (this.eq(Long.MIN_VALUE))
+ return multiplier.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (multiplier.eq(Long.MIN_VALUE))
+ return this.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (this.isNegative()) {
+ if (multiplier.isNegative())
+ return this.neg().mul(multiplier.neg());
+ else
+ return this.neg().mul(multiplier).neg();
+ }
+ else if (multiplier.isNegative())
+ return this.mul(multiplier.neg()).neg();
+ // If both longs are small, use float multiplication
+ if (this.lt(Long.TWO_PWR_24) && multiplier.lt(Long.TWO_PWR_24))
+ return Long.fromNumber(this.toNumber() * multiplier.toNumber(), this.unsigned);
+ // Divide each long into 4 chunks of 16 bits, and then add up 4x4 products.
+ // We can skip products that would overflow.
+ var a48 = this.high >>> 16;
+ var a32 = this.high & 0xffff;
+ var a16 = this.low >>> 16;
+ var a00 = this.low & 0xffff;
+ var b48 = multiplier.high >>> 16;
+ var b32 = multiplier.high & 0xffff;
+ var b16 = multiplier.low >>> 16;
+ var b00 = multiplier.low & 0xffff;
+ var c48 = 0, c32 = 0, c16 = 0, c00 = 0;
+ c00 += a00 * b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 * b00;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c16 += a00 * b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 * b00;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a16 * b16;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a00 * b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ };
+ /** This is an alias of {@link Long.multiply} */
+ Long.prototype.mul = function (multiplier) {
+ return this.multiply(multiplier);
+ };
+ /** Returns the Negation of this Long's value. */
+ Long.prototype.negate = function () {
+ if (!this.unsigned && this.eq(Long.MIN_VALUE))
+ return Long.MIN_VALUE;
+ return this.not().add(Long.ONE);
+ };
+ /** This is an alias of {@link Long.negate} */
+ Long.prototype.neg = function () {
+ return this.negate();
+ };
+ /** Returns the bitwise NOT of this Long. */
+ Long.prototype.not = function () {
+ return Long.fromBits(~this.low, ~this.high, this.unsigned);
+ };
+ /** Tests if this Long's value differs from the specified's. */
+ Long.prototype.notEquals = function (other) {
+ return !this.equals(other);
+ };
+ /** This is an alias of {@link Long.notEquals} */
+ Long.prototype.neq = function (other) {
+ return this.notEquals(other);
+ };
+ /** This is an alias of {@link Long.notEquals} */
+ Long.prototype.ne = function (other) {
+ return this.notEquals(other);
+ };
+ /**
+ * Returns the bitwise OR of this Long and the specified.
+ */
+ Long.prototype.or = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low | other.low, this.high | other.high, this.unsigned);
+ };
+ /**
+ * Returns this Long with bits shifted to the left by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftLeft = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ if ((numBits &= 63) === 0)
+ return this;
+ else if (numBits < 32)
+ return Long.fromBits(this.low << numBits, (this.high << numBits) | (this.low >>> (32 - numBits)), this.unsigned);
+ else
+ return Long.fromBits(0, this.low << (numBits - 32), this.unsigned);
+ };
+ /** This is an alias of {@link Long.shiftLeft} */
+ Long.prototype.shl = function (numBits) {
+ return this.shiftLeft(numBits);
+ };
+ /**
+ * Returns this Long with bits arithmetically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftRight = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ if ((numBits &= 63) === 0)
+ return this;
+ else if (numBits < 32)
+ return Long.fromBits((this.low >>> numBits) | (this.high << (32 - numBits)), this.high >> numBits, this.unsigned);
+ else
+ return Long.fromBits(this.high >> (numBits - 32), this.high >= 0 ? 0 : -1, this.unsigned);
+ };
+ /** This is an alias of {@link Long.shiftRight} */
+ Long.prototype.shr = function (numBits) {
+ return this.shiftRight(numBits);
+ };
+ /**
+ * Returns this Long with bits logically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ Long.prototype.shiftRightUnsigned = function (numBits) {
+ if (Long.isLong(numBits))
+ numBits = numBits.toInt();
+ numBits &= 63;
+ if (numBits === 0)
+ return this;
+ else {
+ var high = this.high;
+ if (numBits < 32) {
+ var low = this.low;
+ return Long.fromBits((low >>> numBits) | (high << (32 - numBits)), high >>> numBits, this.unsigned);
+ }
+ else if (numBits === 32)
+ return Long.fromBits(high, 0, this.unsigned);
+ else
+ return Long.fromBits(high >>> (numBits - 32), 0, this.unsigned);
+ }
+ };
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ Long.prototype.shr_u = function (numBits) {
+ return this.shiftRightUnsigned(numBits);
+ };
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ Long.prototype.shru = function (numBits) {
+ return this.shiftRightUnsigned(numBits);
+ };
+ /**
+ * Returns the difference of this and the specified Long.
+ * @param subtrahend - Subtrahend
+ * @returns Difference
+ */
+ Long.prototype.subtract = function (subtrahend) {
+ if (!Long.isLong(subtrahend))
+ subtrahend = Long.fromValue(subtrahend);
+ return this.add(subtrahend.neg());
+ };
+ /** This is an alias of {@link Long.subtract} */
+ Long.prototype.sub = function (subtrahend) {
+ return this.subtract(subtrahend);
+ };
+ /** Converts the Long to a 32 bit integer, assuming it is a 32 bit integer. */
+ Long.prototype.toInt = function () {
+ return this.unsigned ? this.low >>> 0 : this.low;
+ };
+ /** Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa). */
+ Long.prototype.toNumber = function () {
+ if (this.unsigned)
+ return (this.high >>> 0) * TWO_PWR_32_DBL + (this.low >>> 0);
+ return this.high * TWO_PWR_32_DBL + (this.low >>> 0);
+ };
+ /** Converts the Long to a BigInt (arbitrary precision). */
+ Long.prototype.toBigInt = function () {
+ return BigInt(this.toString());
+ };
+ /**
+ * Converts this Long to its byte representation.
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns Byte representation
+ */
+ Long.prototype.toBytes = function (le) {
+ return le ? this.toBytesLE() : this.toBytesBE();
+ };
+ /**
+ * Converts this Long to its little endian byte representation.
+ * @returns Little endian byte representation
+ */
+ Long.prototype.toBytesLE = function () {
+ var hi = this.high, lo = this.low;
+ return [
+ lo & 0xff,
+ (lo >>> 8) & 0xff,
+ (lo >>> 16) & 0xff,
+ lo >>> 24,
+ hi & 0xff,
+ (hi >>> 8) & 0xff,
+ (hi >>> 16) & 0xff,
+ hi >>> 24
+ ];
+ };
+ /**
+ * Converts this Long to its big endian byte representation.
+ * @returns Big endian byte representation
+ */
+ Long.prototype.toBytesBE = function () {
+ var hi = this.high, lo = this.low;
+ return [
+ hi >>> 24,
+ (hi >>> 16) & 0xff,
+ (hi >>> 8) & 0xff,
+ hi & 0xff,
+ lo >>> 24,
+ (lo >>> 16) & 0xff,
+ (lo >>> 8) & 0xff,
+ lo & 0xff
+ ];
+ };
+ /**
+ * Converts this Long to signed.
+ */
+ Long.prototype.toSigned = function () {
+ if (!this.unsigned)
+ return this;
+ return Long.fromBits(this.low, this.high, false);
+ };
+ /**
+ * Converts the Long to a string written in the specified radix.
+ * @param radix - Radix (2-36), defaults to 10
+ * @throws RangeError If `radix` is out of range
+ */
+ Long.prototype.toString = function (radix) {
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix)
+ throw RangeError('radix');
+ if (this.isZero())
+ return '0';
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ if (this.eq(Long.MIN_VALUE)) {
+ // We need to change the Long value before it can be negated, so we remove
+ // the bottom-most digit in this base and then recurse to do the rest.
+ var radixLong = Long.fromNumber(radix), div = this.div(radixLong), rem1 = div.mul(radixLong).sub(this);
+ return div.toString(radix) + rem1.toInt().toString(radix);
+ }
+ else
+ return '-' + this.neg().toString(radix);
+ }
+ // Do several (6) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ var radixToPower = Long.fromNumber(Math.pow(radix, 6), this.unsigned);
+ // eslint-disable-next-line @typescript-eslint/no-this-alias
+ var rem = this;
+ var result = '';
+ // eslint-disable-next-line no-constant-condition
+ while (true) {
+ var remDiv = rem.div(radixToPower);
+ var intval = rem.sub(remDiv.mul(radixToPower)).toInt() >>> 0;
+ var digits = intval.toString(radix);
+ rem = remDiv;
+ if (rem.isZero()) {
+ return digits + result;
+ }
+ else {
+ while (digits.length < 6)
+ digits = '0' + digits;
+ result = '' + digits + result;
+ }
+ }
+ };
+ /** Converts this Long to unsigned. */
+ Long.prototype.toUnsigned = function () {
+ if (this.unsigned)
+ return this;
+ return Long.fromBits(this.low, this.high, true);
+ };
+ /** Returns the bitwise XOR of this Long and the given one. */
+ Long.prototype.xor = function (other) {
+ if (!Long.isLong(other))
+ other = Long.fromValue(other);
+ return Long.fromBits(this.low ^ other.low, this.high ^ other.high, this.unsigned);
+ };
+ /** This is an alias of {@link Long.isZero} */
+ Long.prototype.eqz = function () {
+ return this.isZero();
+ };
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ Long.prototype.le = function (other) {
+ return this.lessThanOrEqual(other);
+ };
+ /*
+ ****************************************************************
+ * BSON SPECIFIC ADDITIONS *
+ ****************************************************************
+ */
+ Long.prototype.toExtendedJSON = function (options) {
+ if (options && options.relaxed)
+ return this.toNumber();
+ return { $numberLong: this.toString() };
+ };
+ Long.fromExtendedJSON = function (doc, options) {
+ var result = Long.fromString(doc.$numberLong);
+ return options && options.relaxed ? result.toNumber() : result;
+ };
+ /** @internal */
+ Long.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Long.prototype.inspect = function () {
+ return "new Long(\"" + this.toString() + "\"" + (this.unsigned ? ', true' : '') + ")";
+ };
+ Long.TWO_PWR_24 = Long.fromInt(TWO_PWR_24_DBL);
+ /** Maximum unsigned value. */
+ Long.MAX_UNSIGNED_VALUE = Long.fromBits(0xffffffff | 0, 0xffffffff | 0, true);
+ /** Signed zero */
+ Long.ZERO = Long.fromInt(0);
+ /** Unsigned zero. */
+ Long.UZERO = Long.fromInt(0, true);
+ /** Signed one. */
+ Long.ONE = Long.fromInt(1);
+ /** Unsigned one. */
+ Long.UONE = Long.fromInt(1, true);
+ /** Signed negative one. */
+ Long.NEG_ONE = Long.fromInt(-1);
+ /** Maximum signed value. */
+ Long.MAX_VALUE = Long.fromBits(0xffffffff | 0, 0x7fffffff | 0, false);
+ /** Minimum signed value. */
+ Long.MIN_VALUE = Long.fromBits(0, 0x80000000 | 0, false);
+ return Long;
+}());
+exports.Long = Long;
+Object.defineProperty(Long.prototype, '__isLong__', { value: true });
+Object.defineProperty(Long.prototype, '_bsontype', { value: 'Long' });
+//# sourceMappingURL=long.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/long.js.map b/node_modules/bson/lib/long.js.map
new file mode 100644
index 0000000..89fb7de
--- /dev/null
+++ b/node_modules/bson/lib/long.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"long.js","sourceRoot":"","sources":["../src/long.ts"],"names":[],"mappings":";;;AACA,wCAA8C;AAkB9C;;GAEG;AACH,IAAI,IAAI,GAAgC,SAAS,CAAC;AAMlD,IAAI;IACF,IAAI,GAAI,IAAI,WAAW,CAAC,QAAQ,CAC9B,IAAI,WAAW,CAAC,MAAM;IACpB,kBAAkB;IAClB,IAAI,UAAU,CAAC,CAAC,CAAC,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,EAAE,EAAE,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC,EAAE,EAAE,EAAE,CAAC,EAAE,GAAG,EAAE,EAAE,CAAC,CAAC,CAC/oC,EACD,EAAE,CACH,CAAC,OAAsC,CAAC;CAC1C;AAAC,WAAM;IACN,kBAAkB;CACnB;AAED,IAAM,cAAc,GAAG,CAAC,IAAI,EAAE,CAAC;AAC/B,IAAM,cAAc,GAAG,CAAC,IAAI,EAAE,CAAC;AAC/B,IAAM,cAAc,GAAG,cAAc,GAAG,cAAc,CAAC;AACvD,IAAM,cAAc,GAAG,cAAc,GAAG,cAAc,CAAC;AACvD,IAAM,cAAc,GAAG,cAAc,GAAG,CAAC,CAAC;AAE1C,mEAAmE;AACnE,IAAM,SAAS,GAA4B,EAAE,CAAC;AAE9C,4EAA4E;AAC5E,IAAM,UAAU,GAA4B,EAAE,CAAC;AAO/C;;;;;;;;;;;;;;;;;GAiBG;AACH;IAqBE;;;;;;;;;;;;OAYG;IACH,cAAY,GAAiC,EAAE,IAAuB,EAAE,QAAkB;QAA9E,oBAAA,EAAA,OAAiC;QAC3C,IAAI,CAAC,CAAC,IAAI,YAAY,IAAI,CAAC;YAAE,OAAO,IAAI,IAAI,CAAC,GAAG,EAAE,IAAI,EAAE,QAAQ,CAAC,CAAC;QAElE,IAAI,OAAO,GAAG,KAAK,QAAQ,EAAE;YAC3B,MAAM,CAAC,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;SACnD;aAAM,IAAI,OAAO,GAAG,KAAK,QAAQ,EAAE;YAClC,MAAM,CAAC,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;SACnD;aAAM;YACL,IAAI,CAAC,GAAG,GAAG,GAAG,GAAG,CAAC,CAAC;YACnB,IAAI,CAAC,IAAI,GAAI,IAAe,GAAG,CAAC,CAAC;YACjC,IAAI,CAAC,QAAQ,GAAG,CAAC,CAAC,QAAQ,CAAC;SAC5B;QAED,MAAM,CAAC,cAAc,CAAC,IAAI,EAAE,YAAY,EAAE;YACxC,KAAK,EAAE,IAAI;YACX,YAAY,EAAE,KAAK;YACnB,QAAQ,EAAE,KAAK;YACf,UAAU,EAAE,KAAK;SAClB,CAAC,CAAC;IACL,CAAC;IAqBD;;;;;;;OAOG;IACI,aAAQ,GAAf,UAAgB,OAAe,EAAE,QAAgB,EAAE,QAAkB;QACnE,OAAO,IAAI,IAAI,CAAC,OAAO,EAAE,QAAQ,EAAE,QAAQ,CAAC,CAAC;IAC/C,CAAC;IAED;;;;;OAKG;IACI,YAAO,GAAd,UAAe,KAAa,EAAE,QAAkB;QAC9C,IAAI,GAAG,EAAE,SAAS,EAAE,KAAK,CAAC;QAC1B,IAAI,QAAQ,EAAE;YACZ,KAAK,MAAM,CAAC,CAAC;YACb,IAAI,CAAC,KAAK,GAAG,CAAC,IAAI,KAAK,IAAI,KAAK,GAAG,GAAG,CAAC,EAAE;gBACvC,SAAS,GAAG,UAAU,CAAC,KAAK,CAAC,CAAC;gBAC9B,IAAI,SAAS;oBAAE,OAAO,SAAS,CAAC;aACjC;YACD,GAAG,GAAG,IAAI,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;YAC3D,IAAI,KAAK;gBAAE,UAAU,CAAC,KAAK,CAAC,GAAG,GAAG,CAAC;YACnC,OAAO,GAAG,CAAC;SACZ;aAAM;YACL,KAAK,IAAI,CAAC,CAAC;YACX,IAAI,CAAC,KAAK,GAAG,CAAC,GAAG,IAAI,KAAK,IAAI,KAAK,GAAG,GAAG,CAAC,EAAE;gBAC1C,SAAS,GAAG,SAAS,CAAC,KAAK,CAAC,CAAC;gBAC7B,IAAI,SAAS;oBAAE,OAAO,SAAS,CAAC;aACjC;YACD,GAAG,GAAG,IAAI,CAAC,QAAQ,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,EAAE,KAAK,CAAC,CAAC;YACtD,IAAI,KAAK;gBAAE,SAAS,CAAC,KAAK,CAAC,GAAG,GAAG,CAAC;YAClC,OAAO,GAAG,CAAC;SACZ;IACH,CAAC;IAED;;;;;OAKG;IACI,eAAU,GAAjB,UAAkB,KAAa,EAAE,QAAkB;QACjD,IAAI,KAAK,CAAC,KAAK,CAAC;YAAE,OAAO,QAAQ,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC;QAC3D,IAAI,QAAQ,EAAE;YACZ,IAAI,KAAK,GAAG,CAAC;gBAAE,OAAO,IAAI,CAAC,KAAK,CAAC;YACjC,IAAI,KAAK,IAAI,cAAc;gBAAE,OAAO,IAAI,CAAC,kBAAkB,CAAC;SAC7D;aAAM;YACL,IAAI,KAAK,IAAI,CAAC,cAAc;gBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;YACpD,IAAI,KAAK,GAAG,CAAC,IAAI,cAAc;gBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;SACxD;QACD,IAAI,KAAK,GAAG,CAAC;YAAE,OAAO,IAAI,CAAC,UAAU,CAAC,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC,GAAG,EAAE,CAAC;QAC9D,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,GAAG,cAAc,GAAG,CAAC,EAAE,CAAC,KAAK,GAAG,cAAc,CAAC,GAAG,CAAC,EAAE,QAAQ,CAAC,CAAC;IAC3F,CAAC;IAED;;;;;OAKG;IACI,eAAU,GAAjB,UAAkB,KAAa,EAAE,QAAkB;QACjD,OAAO,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,QAAQ,EAAE,EAAE,QAAQ,CAAC,CAAC;IACrD,CAAC;IAED;;;;;;OAMG;IACI,eAAU,GAAjB,UAAkB,GAAW,EAAE,QAAkB,EAAE,KAAc;QAC/D,IAAI,GAAG,CAAC,MAAM,KAAK,CAAC;YAAE,MAAM,KAAK,CAAC,cAAc,CAAC,CAAC;QAClD,IAAI,GAAG,KAAK,KAAK,IAAI,GAAG,KAAK,UAAU,IAAI,GAAG,KAAK,WAAW,IAAI,GAAG,KAAK,WAAW;YACnF,OAAO,IAAI,CAAC,IAAI,CAAC;QACnB,IAAI,OAAO,QAAQ,KAAK,QAAQ,EAAE;YAChC,mCAAmC;YACnC,CAAC,KAAK,GAAG,QAAQ,CAAC,EAAE,CAAC,QAAQ,GAAG,KAAK,CAAC,CAAC;SACxC;aAAM;YACL,QAAQ,GAAG,CAAC,CAAC,QAAQ,CAAC;SACvB;QACD,KAAK,GAAG,KAAK,IAAI,EAAE,CAAC;QACpB,IAAI,KAAK,GAAG,CAAC,IAAI,EAAE,GAAG,KAAK;YAAE,MAAM,UAAU,CAAC,OAAO,CAAC,CAAC;QAEvD,IAAI,CAAC,CAAC;QACN,IAAI,CAAC,CAAC,GAAG,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC;YAAE,MAAM,KAAK,CAAC,iBAAiB,CAAC,CAAC;aAC1D,IAAI,CAAC,KAAK,CAAC,EAAE;YAChB,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,CAAC,EAAE,QAAQ,EAAE,KAAK,CAAC,CAAC,GAAG,EAAE,CAAC;SACjE;QAED,6DAA6D;QAC7D,yDAAyD;QACzD,IAAM,YAAY,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC,CAAC;QAEzD,IAAI,MAAM,GAAG,IAAI,CAAC,IAAI,CAAC;QACvB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,GAAG,CAAC,MAAM,EAAE,CAAC,IAAI,CAAC,EAAE;YACtC,IAAM,IAAI,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,GAAG,CAAC,MAAM,GAAG,CAAC,CAAC,EACtC,KAAK,GAAG,QAAQ,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,EAAE,KAAK,CAAC,CAAC;YACtD,IAAI,IAAI,GAAG,CAAC,EAAE;gBACZ,IAAM,KAAK,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;gBACrD,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,CAAC;aACxD;iBAAM;gBACL,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC;gBAClC,MAAM,GAAG,MAAM,CAAC,GAAG,CAAC,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,CAAC,CAAC;aAC7C;SACF;QACD,MAAM,CAAC,QAAQ,GAAG,QAAQ,CAAC;QAC3B,OAAO,MAAM,CAAC;IAChB,CAAC;IAED;;;;;;OAMG;IACI,cAAS,GAAhB,UAAiB,KAAe,EAAE,QAAkB,EAAE,EAAY;QAChE,OAAO,EAAE,CAAC,CAAC,CAAC,IAAI,CAAC,WAAW,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC,CAAC,CAAC,IAAI,CAAC,WAAW,CAAC,KAAK,EAAE,QAAQ,CAAC,CAAC;IACpF,CAAC;IAED;;;;;OAKG;IACI,gBAAW,GAAlB,UAAmB,KAAe,EAAE,QAAkB;QACpD,OAAO,IAAI,IAAI,CACb,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,EAChE,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,EAChE,QAAQ,CACT,CAAC;IACJ,CAAC;IAED;;;;;OAKG;IACI,gBAAW,GAAlB,UAAmB,KAAe,EAAE,QAAkB;QACpD,OAAO,IAAI,IAAI,CACb,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,EAChE,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,EAChE,QAAQ,CACT,CAAC;IACJ,CAAC;IAED;;OAEG;IACH,iHAAiH;IAC1G,WAAM,GAAb,UAAc,KAAU;QACtB,OAAO,oBAAY,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,YAAY,CAAC,KAAK,IAAI,CAAC;IAC7D,CAAC;IAED;;;OAGG;IACI,cAAS,GAAhB,UACE,GAAwE,EACxE,QAAkB;QAElB,IAAI,OAAO,GAAG,KAAK,QAAQ;YAAE,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;QACnE,IAAI,OAAO,GAAG,KAAK,QAAQ;YAAE,OAAO,IAAI,CAAC,UAAU,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;QACnE,wDAAwD;QACxD,OAAO,IAAI,CAAC,QAAQ,CAClB,GAAG,CAAC,GAAG,EACP,GAAG,CAAC,IAAI,EACR,OAAO,QAAQ,KAAK,SAAS,CAAC,CAAC,CAAC,QAAQ,CAAC,CAAC,CAAC,GAAG,CAAC,QAAQ,CACxD,CAAC;IACJ,CAAC;IAED,sDAAsD;IACtD,kBAAG,GAAH,UAAI,MAA0C;QAC5C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC;YAAE,MAAM,GAAG,IAAI,CAAC,SAAS,CAAC,MAAM,CAAC,CAAC;QAE1D,wEAAwE;QAExE,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,EAAE,CAAC;QAC7B,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,GAAG,MAAM,CAAC;QAC/B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC;QAC5B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,GAAG,MAAM,CAAC;QAE9B,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,KAAK,EAAE,CAAC;QAC/B,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,GAAG,MAAM,CAAC;QACjC,IAAM,GAAG,GAAG,MAAM,CAAC,GAAG,KAAK,EAAE,CAAC;QAC9B,IAAM,GAAG,GAAG,MAAM,CAAC,GAAG,GAAG,MAAM,CAAC;QAEhC,IAAI,GAAG,GAAG,CAAC,EACT,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,CAAC;QACV,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,MAAM,CAAC;QACd,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,GAAG,IAAI,EAAE,CAAC,GAAG,GAAG,EAAE,CAAC,GAAG,IAAI,EAAE,CAAC,GAAG,GAAG,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;IAC5E,CAAC;IAED;;;OAGG;IACH,kBAAG,GAAH,UAAI,KAAyC;QAC3C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;IACpF,CAAC;IAED;;;OAGG;IACH,sBAAO,GAAP,UAAQ,KAAyC;QAC/C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,IAAI,IAAI,CAAC,EAAE,CAAC,KAAK,CAAC;YAAE,OAAO,CAAC,CAAC;QAC7B,IAAM,OAAO,GAAG,IAAI,CAAC,UAAU,EAAE,EAC/B,QAAQ,GAAG,KAAK,CAAC,UAAU,EAAE,CAAC;QAChC,IAAI,OAAO,IAAI,CAAC,QAAQ;YAAE,OAAO,CAAC,CAAC,CAAC;QACpC,IAAI,CAAC,OAAO,IAAI,QAAQ;YAAE,OAAO,CAAC,CAAC;QACnC,2CAA2C;QAC3C,IAAI,CAAC,IAAI,CAAC,QAAQ;YAAE,OAAO,IAAI,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,UAAU,EAAE,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;QACjE,gDAAgD;QAChD,OAAO,KAAK,CAAC,IAAI,KAAK,CAAC,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC;YACvC,CAAC,KAAK,CAAC,IAAI,KAAK,IAAI,CAAC,IAAI,IAAI,KAAK,CAAC,GAAG,KAAK,CAAC,GAAG,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;YAC9D,CAAC,CAAC,CAAC,CAAC;YACJ,CAAC,CAAC,CAAC,CAAC;IACR,CAAC;IAED,+CAA+C;IAC/C,mBAAI,GAAJ,UAAK,KAAyC;QAC5C,OAAO,IAAI,CAAC,OAAO,CAAC,KAAK,CAAC,CAAC;IAC7B,CAAC;IAED;;;OAGG;IACH,qBAAM,GAAN,UAAO,OAA2C;QAChD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;QAC7D,IAAI,OAAO,CAAC,MAAM,EAAE;YAAE,MAAM,KAAK,CAAC,kBAAkB,CAAC,CAAC;QAEtD,8BAA8B;QAC9B,IAAI,IAAI,EAAE;YACR,sDAAsD;YACtD,0DAA0D;YAC1D,4CAA4C;YAC5C,IACE,CAAC,IAAI,CAAC,QAAQ;gBACd,IAAI,CAAC,IAAI,KAAK,CAAC,UAAU;gBACzB,OAAO,CAAC,GAAG,KAAK,CAAC,CAAC;gBAClB,OAAO,CAAC,IAAI,KAAK,CAAC,CAAC,EACnB;gBACA,wCAAwC;gBACxC,OAAO,IAAI,CAAC;aACb;YACD,IAAM,GAAG,GAAG,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,CACnD,IAAI,CAAC,GAAG,EACR,IAAI,CAAC,IAAI,EACT,OAAO,CAAC,GAAG,EACX,OAAO,CAAC,IAAI,CACb,CAAC;YACF,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC3D;QAED,IAAI,IAAI,CAAC,MAAM,EAAE;YAAE,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC;QACjE,IAAI,MAAM,EAAE,GAAG,EAAE,GAAG,CAAC;QACrB,IAAI,CAAC,IAAI,CAAC,QAAQ,EAAE;YAClB,yEAAyE;YACzE,8BAA8B;YAC9B,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;gBAC3B,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,GAAG,CAAC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,OAAO,CAAC;oBAAE,OAAO,IAAI,CAAC,SAAS,CAAC;gBAC5E,sCAAsC;qBACjC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;oBAAE,OAAO,IAAI,CAAC,GAAG,CAAC;qBAChD;oBACH,sEAAsE;oBACtE,IAAM,QAAQ,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;oBAC7B,MAAM,GAAG,QAAQ,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;oBACtC,IAAI,MAAM,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,EAAE;wBACxB,OAAO,OAAO,CAAC,UAAU,EAAE,CAAC,CAAC,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,CAAC,IAAI,CAAC,OAAO,CAAC;qBACvD;yBAAM;wBACL,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,MAAM,CAAC,CAAC,CAAC;wBACpC,GAAG,GAAG,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,CAAC;wBACnC,OAAO,GAAG,CAAC;qBACZ;iBACF;aACF;iBAAM,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;gBAAE,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC;YACrF,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;gBACrB,IAAI,OAAO,CAAC,UAAU,EAAE;oBAAE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC;gBAC/D,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,EAAE,CAAC;aACtC;iBAAM,IAAI,OAAO,CAAC,UAAU,EAAE;gBAAE,OAAO,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC;YACtE,GAAG,GAAG,IAAI,CAAC,IAAI,CAAC;SACjB;aAAM;YACL,2EAA2E;YAC3E,gEAAgE;YAChE,IAAI,CAAC,OAAO,CAAC,QAAQ;gBAAE,OAAO,GAAG,OAAO,CAAC,UAAU,EAAE,CAAC;YACtD,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC;gBAAE,OAAO,IAAI,CAAC,KAAK,CAAC;YACxC,IAAI,OAAO,CAAC,EAAE,CAAC,IAAI,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC;gBAC1B,yCAAyC;gBACzC,OAAO,IAAI,CAAC,IAAI,CAAC;YACnB,GAAG,GAAG,IAAI,CAAC,KAAK,CAAC;SAClB;QAED,uEAAuE;QACvE,4EAA4E;QAC5E,4EAA4E;QAC5E,4EAA4E;QAC5E,oCAAoC;QACpC,GAAG,GAAG,IAAI,CAAC;QACX,OAAO,GAAG,CAAC,GAAG,CAAC,OAAO,CAAC,EAAE;YACvB,sEAAsE;YACtE,iCAAiC;YACjC,MAAM,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,QAAQ,EAAE,GAAG,OAAO,CAAC,QAAQ,EAAE,CAAC,CAAC,CAAC;YAEtE,4EAA4E;YAC5E,0DAA0D;YAC1D,IAAM,IAAI,GAAG,IAAI,CAAC,IAAI,CAAC,IAAI,CAAC,GAAG,CAAC,MAAM,CAAC,GAAG,IAAI,CAAC,GAAG,CAAC,CAAC;YACpD,IAAM,KAAK,GAAG,IAAI,IAAI,EAAE,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC,EAAE,IAAI,GAAG,EAAE,CAAC,CAAC;YACtD,2EAA2E;YAC3E,kEAAkE;YAClE,IAAI,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,MAAM,CAAC,CAAC;YACxC,IAAI,SAAS,GAAG,SAAS,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;YACvC,OAAO,SAAS,CAAC,UAAU,EAAE,IAAI,SAAS,CAAC,EAAE,CAAC,GAAG,CAAC,EAAE;gBAClD,MAAM,IAAI,KAAK,CAAC;gBAChB,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,MAAM,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;gBACnD,SAAS,GAAG,SAAS,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;aACpC;YAED,qEAAqE;YACrE,sDAAsD;YACtD,IAAI,SAAS,CAAC,MAAM,EAAE;gBAAE,SAAS,GAAG,IAAI,CAAC,GAAG,CAAC;YAE7C,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;YACzB,GAAG,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC;SAC1B;QACD,OAAO,GAAG,CAAC;IACb,CAAC;IAED,6CAA6C;IAC7C,kBAAG,GAAH,UAAI,OAA2C;QAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;IAC9B,CAAC;IAED;;;OAGG;IACH,qBAAM,GAAN,UAAO,KAAyC;QAC9C,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,IAAI,IAAI,CAAC,QAAQ,KAAK,KAAK,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,KAAK,EAAE,KAAK,CAAC,IAAI,KAAK,CAAC,IAAI,KAAK,EAAE,KAAK,CAAC;YACvF,OAAO,KAAK,CAAC;QACf,OAAO,IAAI,CAAC,IAAI,KAAK,KAAK,CAAC,IAAI,IAAI,IAAI,CAAC,GAAG,KAAK,KAAK,CAAC,GAAG,CAAC;IAC5D,CAAC;IAED,8CAA8C;IAC9C,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC;IAC5B,CAAC;IAED,iDAAiD;IACjD,0BAAW,GAAX;QACE,OAAO,IAAI,CAAC,IAAI,CAAC;IACnB,CAAC;IAED,oDAAoD;IACpD,kCAAmB,GAAnB;QACE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC;IACzB,CAAC;IAED,gDAAgD;IAChD,yBAAU,GAAV;QACE,OAAO,IAAI,CAAC,GAAG,CAAC;IAClB,CAAC;IAED,mDAAmD;IACnD,iCAAkB,GAAlB;QACE,OAAO,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;IACxB,CAAC;IAED,mFAAmF;IACnF,4BAAa,GAAb;QACE,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;YACrB,oCAAoC;YACpC,OAAO,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,aAAa,EAAE,CAAC;SAClE;QACD,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,CAAC,CAAC,IAAI,CAAC,GAAG,CAAC;QACnD,IAAI,GAAW,CAAC;QAChB,KAAK,GAAG,GAAG,EAAE,EAAE,GAAG,GAAG,CAAC,EAAE,GAAG,EAAE;YAAE,IAAI,CAAC,GAAG,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC;gBAAE,MAAM;QACnE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC,CAAC,CAAC,GAAG,GAAG,EAAE,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC,CAAC;IAC9C,CAAC;IAED,kEAAkE;IAClE,0BAAW,GAAX,UAAY,KAAyC;QACnD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;IAC9B,CAAC;IAED,mDAAmD;IACnD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,WAAW,CAAC,KAAK,CAAC,CAAC;IACjC,CAAC;IAED,2EAA2E;IAC3E,iCAAkB,GAAlB,UAAmB,KAAyC;QAC1D,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;IAC/B,CAAC;IAED,0DAA0D;IAC1D,kBAAG,GAAH,UAAI,KAAyC;QAC3C,OAAO,IAAI,CAAC,kBAAkB,CAAC,KAAK,CAAC,CAAC;IACxC,CAAC;IACD,0DAA0D;IAC1D,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,kBAAkB,CAAC,KAAK,CAAC,CAAC;IACxC,CAAC;IAED,0CAA0C;IAC1C,qBAAM,GAAN;QACE,OAAO,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC,CAAC,KAAK,CAAC,CAAC;IAC9B,CAAC;IAED,8CAA8C;IAC9C,yBAAU,GAAV;QACE,OAAO,CAAC,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,GAAG,CAAC,CAAC;IACzC,CAAC;IAED,yCAAyC;IACzC,oBAAK,GAAL;QACE,OAAO,CAAC,IAAI,CAAC,GAAG,GAAG,CAAC,CAAC,KAAK,CAAC,CAAC;IAC9B,CAAC;IAED,8CAA8C;IAC9C,yBAAU,GAAV;QACE,OAAO,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,IAAI,IAAI,CAAC,CAAC;IACzC,CAAC;IAED,8CAA8C;IAC9C,qBAAM,GAAN;QACE,OAAO,IAAI,CAAC,IAAI,KAAK,CAAC,IAAI,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC;IAC3C,CAAC;IAED,+DAA+D;IAC/D,uBAAQ,GAAR,UAAS,KAAyC;QAChD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,CAAC;IAC9B,CAAC;IAED,iDAAiD;IACjD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;IAC9B,CAAC;IAED,wEAAwE;IACxE,8BAAe,GAAf,UAAgB,KAAyC;QACvD,OAAO,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;IAC/B,CAAC;IAED,uDAAuD;IACvD,kBAAG,GAAH,UAAI,KAAyC;QAC3C,OAAO,IAAI,CAAC,eAAe,CAAC,KAAK,CAAC,CAAC;IACrC,CAAC;IAED,8CAA8C;IAC9C,qBAAM,GAAN,UAAO,OAA2C;QAChD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;QAE7D,8BAA8B;QAC9B,IAAI,IAAI,EAAE;YACR,IAAM,GAAG,GAAG,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,CACnD,IAAI,CAAC,GAAG,EACR,IAAI,CAAC,IAAI,EACT,OAAO,CAAC,GAAG,EACX,OAAO,CAAC,IAAI,CACb,CAAC;YACF,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC3D;QAED,OAAO,IAAI,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC,CAAC;IAClD,CAAC;IAED,8CAA8C;IAC9C,kBAAG,GAAH,UAAI,OAA2C;QAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;IAC9B,CAAC;IACD,8CAA8C;IAC9C,kBAAG,GAAH,UAAI,OAA2C;QAC7C,OAAO,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,CAAC;IAC9B,CAAC;IAED;;;;OAIG;IACH,uBAAQ,GAAR,UAAS,UAA8C;QACrD,IAAI,IAAI,CAAC,MAAM,EAAE;YAAE,OAAO,IAAI,CAAC,IAAI,CAAC;QACpC,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;YAAE,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;QAEtE,8BAA8B;QAC9B,IAAI,IAAI,EAAE;YACR,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,UAAU,CAAC,GAAG,EAAE,UAAU,CAAC,IAAI,CAAC,CAAC;YAC3E,OAAO,IAAI,CAAC,QAAQ,CAAC,GAAG,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SAC3D;QAED,IAAI,UAAU,CAAC,MAAM,EAAE;YAAE,OAAO,IAAI,CAAC,IAAI,CAAC;QAC1C,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;YAAE,OAAO,UAAU,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC,IAAI,CAAC,SAAS,CAAC,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC;QACpF,IAAI,UAAU,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;YAAE,OAAO,IAAI,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC,IAAI,CAAC,SAAS,CAAC,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC;QAEpF,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;YACrB,IAAI,UAAU,CAAC,UAAU,EAAE;gBAAE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC;;gBAChE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC,GAAG,EAAE,CAAC;SAC9C;aAAM,IAAI,UAAU,CAAC,UAAU,EAAE;YAAE,OAAO,IAAI,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,CAAC;QAE5E,oDAAoD;QACpD,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,UAAU,CAAC,IAAI,UAAU,CAAC,EAAE,CAAC,IAAI,CAAC,UAAU,CAAC;YAC5D,OAAO,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,QAAQ,EAAE,GAAG,UAAU,CAAC,QAAQ,EAAE,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;QAEjF,2EAA2E;QAC3E,4CAA4C;QAE5C,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,KAAK,EAAE,CAAC;QAC7B,IAAM,GAAG,GAAG,IAAI,CAAC,IAAI,GAAG,MAAM,CAAC;QAC/B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC;QAC5B,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,GAAG,MAAM,CAAC;QAE9B,IAAM,GAAG,GAAG,UAAU,CAAC,IAAI,KAAK,EAAE,CAAC;QACnC,IAAM,GAAG,GAAG,UAAU,CAAC,IAAI,GAAG,MAAM,CAAC;QACrC,IAAM,GAAG,GAAG,UAAU,CAAC,GAAG,KAAK,EAAE,CAAC;QAClC,IAAM,GAAG,GAAG,UAAU,CAAC,GAAG,GAAG,MAAM,CAAC;QAEpC,IAAI,GAAG,GAAG,CAAC,EACT,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,EACP,GAAG,GAAG,CAAC,CAAC;QACV,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,CAAC;QACjB,GAAG,IAAI,GAAG,KAAK,EAAE,CAAC;QAClB,GAAG,IAAI,MAAM,CAAC;QACd,GAAG,IAAI,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,GAAG,CAAC;QACrD,GAAG,IAAI,MAAM,CAAC;QACd,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,GAAG,IAAI,EAAE,CAAC,GAAG,GAAG,EAAE,CAAC,GAAG,IAAI,EAAE,CAAC,GAAG,GAAG,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;IAC5E,CAAC;IAED,gDAAgD;IAChD,kBAAG,GAAH,UAAI,UAA8C;QAChD,OAAO,IAAI,CAAC,QAAQ,CAAC,UAAU,CAAC,CAAC;IACnC,CAAC;IAED,iDAAiD;IACjD,qBAAM,GAAN;QACE,IAAI,CAAC,IAAI,CAAC,QAAQ,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC;YAAE,OAAO,IAAI,CAAC,SAAS,CAAC;QACrE,OAAO,IAAI,CAAC,GAAG,EAAE,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;IAClC,CAAC;IAED,8CAA8C;IAC9C,kBAAG,GAAH;QACE,OAAO,IAAI,CAAC,MAAM,EAAE,CAAC;IACvB,CAAC;IAED,4CAA4C;IAC5C,kBAAG,GAAH;QACE,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;IAC7D,CAAC;IAED,+DAA+D;IAC/D,wBAAS,GAAT,UAAU,KAAyC;QACjD,OAAO,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC;IAC7B,CAAC;IAED,iDAAiD;IACjD,kBAAG,GAAH,UAAI,KAAyC;QAC3C,OAAO,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;IAC/B,CAAC;IACD,iDAAiD;IACjD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;IAC/B,CAAC;IAED;;OAEG;IACH,iBAAE,GAAF,UAAG,KAA6B;QAC9B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;IACpF,CAAC;IAED;;;;OAIG;IACH,wBAAS,GAAT,UAAU,OAAsB;QAC9B,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;QACpD,IAAI,CAAC,OAAO,IAAI,EAAE,CAAC,KAAK,CAAC;YAAE,OAAO,IAAI,CAAC;aAClC,IAAI,OAAO,GAAG,EAAE;YACnB,OAAO,IAAI,CAAC,QAAQ,CAClB,IAAI,CAAC,GAAG,IAAI,OAAO,EACnB,CAAC,IAAI,CAAC,IAAI,IAAI,OAAO,CAAC,GAAG,CAAC,IAAI,CAAC,GAAG,KAAK,CAAC,EAAE,GAAG,OAAO,CAAC,CAAC,EACtD,IAAI,CAAC,QAAQ,CACd,CAAC;;YACC,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,EAAE,IAAI,CAAC,GAAG,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;IAC1E,CAAC;IAED,iDAAiD;IACjD,kBAAG,GAAH,UAAI,OAAsB;QACxB,OAAO,IAAI,CAAC,SAAS,CAAC,OAAO,CAAC,CAAC;IACjC,CAAC;IAED;;;;OAIG;IACH,yBAAU,GAAV,UAAW,OAAsB;QAC/B,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;QACpD,IAAI,CAAC,OAAO,IAAI,EAAE,CAAC,KAAK,CAAC;YAAE,OAAO,IAAI,CAAC;aAClC,IAAI,OAAO,GAAG,EAAE;YACnB,OAAO,IAAI,CAAC,QAAQ,CAClB,CAAC,IAAI,CAAC,GAAG,KAAK,OAAO,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,IAAI,CAAC,EAAE,GAAG,OAAO,CAAC,CAAC,EACtD,IAAI,CAAC,IAAI,IAAI,OAAO,EACpB,IAAI,CAAC,QAAQ,CACd,CAAC;;YACC,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,IAAI,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC,EAAE,IAAI,CAAC,IAAI,IAAI,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;IACjG,CAAC;IAED,kDAAkD;IAClD,kBAAG,GAAH,UAAI,OAAsB;QACxB,OAAO,IAAI,CAAC,UAAU,CAAC,OAAO,CAAC,CAAC;IAClC,CAAC;IAED;;;;OAIG;IACH,iCAAkB,GAAlB,UAAmB,OAAsB;QACvC,IAAI,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC;YAAE,OAAO,GAAG,OAAO,CAAC,KAAK,EAAE,CAAC;QACpD,OAAO,IAAI,EAAE,CAAC;QACd,IAAI,OAAO,KAAK,CAAC;YAAE,OAAO,IAAI,CAAC;aAC1B;YACH,IAAM,IAAI,GAAG,IAAI,CAAC,IAAI,CAAC;YACvB,IAAI,OAAO,GAAG,EAAE,EAAE;gBAChB,IAAM,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC;gBACrB,OAAO,IAAI,CAAC,QAAQ,CAClB,CAAC,GAAG,KAAK,OAAO,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,EAAE,GAAG,OAAO,CAAC,CAAC,EAC5C,IAAI,KAAK,OAAO,EAChB,IAAI,CAAC,QAAQ,CACd,CAAC;aACH;iBAAM,IAAI,OAAO,KAAK,EAAE;gBAAE,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;;gBACnE,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,KAAK,CAAC,OAAO,GAAG,EAAE,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;SACtE;IACH,CAAC;IAED,0DAA0D;IAC1D,oBAAK,GAAL,UAAM,OAAsB;QAC1B,OAAO,IAAI,CAAC,kBAAkB,CAAC,OAAO,CAAC,CAAC;IAC1C,CAAC;IACD,0DAA0D;IAC1D,mBAAI,GAAJ,UAAK,OAAsB;QACzB,OAAO,IAAI,CAAC,kBAAkB,CAAC,OAAO,CAAC,CAAC;IAC1C,CAAC;IAED;;;;OAIG;IACH,uBAAQ,GAAR,UAAS,UAA8C;QACrD,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,UAAU,CAAC;YAAE,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;QACtE,OAAO,IAAI,CAAC,GAAG,CAAC,UAAU,CAAC,GAAG,EAAE,CAAC,CAAC;IACpC,CAAC;IAED,gDAAgD;IAChD,kBAAG,GAAH,UAAI,UAA8C;QAChD,OAAO,IAAI,CAAC,QAAQ,CAAC,UAAU,CAAC,CAAC;IACnC,CAAC;IAED,8EAA8E;IAC9E,oBAAK,GAAL;QACE,OAAO,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC,IAAI,CAAC,GAAG,CAAC;IACnD,CAAC;IAED,gHAAgH;IAChH,uBAAQ,GAAR;QACE,IAAI,IAAI,CAAC,QAAQ;YAAE,OAAO,CAAC,IAAI,CAAC,IAAI,KAAK,CAAC,CAAC,GAAG,cAAc,GAAG,CAAC,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC;QAChF,OAAO,IAAI,CAAC,IAAI,GAAG,cAAc,GAAG,CAAC,IAAI,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC;IACvD,CAAC;IAED,2DAA2D;IAC3D,uBAAQ,GAAR;QACE,OAAO,MAAM,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC,CAAC;IACjC,CAAC;IAED;;;;OAIG;IACH,sBAAO,GAAP,UAAQ,EAAY;QAClB,OAAO,EAAE,CAAC,CAAC,CAAC,IAAI,CAAC,SAAS,EAAE,CAAC,CAAC,CAAC,IAAI,CAAC,SAAS,EAAE,CAAC;IAClD,CAAC;IAED;;;OAGG;IACH,wBAAS,GAAT;QACE,IAAM,EAAE,GAAG,IAAI,CAAC,IAAI,EAClB,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO;YACL,EAAE,GAAG,IAAI;YACT,CAAC,EAAE,KAAK,CAAC,CAAC,GAAG,IAAI;YACjB,CAAC,EAAE,KAAK,EAAE,CAAC,GAAG,IAAI;YAClB,EAAE,KAAK,EAAE;YACT,EAAE,GAAG,IAAI;YACT,CAAC,EAAE,KAAK,CAAC,CAAC,GAAG,IAAI;YACjB,CAAC,EAAE,KAAK,EAAE,CAAC,GAAG,IAAI;YAClB,EAAE,KAAK,EAAE;SACV,CAAC;IACJ,CAAC;IAED;;;OAGG;IACH,wBAAS,GAAT;QACE,IAAM,EAAE,GAAG,IAAI,CAAC,IAAI,EAClB,EAAE,GAAG,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO;YACL,EAAE,KAAK,EAAE;YACT,CAAC,EAAE,KAAK,EAAE,CAAC,GAAG,IAAI;YAClB,CAAC,EAAE,KAAK,CAAC,CAAC,GAAG,IAAI;YACjB,EAAE,GAAG,IAAI;YACT,EAAE,KAAK,EAAE;YACT,CAAC,EAAE,KAAK,EAAE,CAAC,GAAG,IAAI;YAClB,CAAC,EAAE,KAAK,CAAC,CAAC,GAAG,IAAI;YACjB,EAAE,GAAG,IAAI;SACV,CAAC;IACJ,CAAC;IAED;;OAEG;IACH,uBAAQ,GAAR;QACE,IAAI,CAAC,IAAI,CAAC,QAAQ;YAAE,OAAO,IAAI,CAAC;QAChC,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;IACnD,CAAC;IAED;;;;OAIG;IACH,uBAAQ,GAAR,UAAS,KAAc;QACrB,KAAK,GAAG,KAAK,IAAI,EAAE,CAAC;QACpB,IAAI,KAAK,GAAG,CAAC,IAAI,EAAE,GAAG,KAAK;YAAE,MAAM,UAAU,CAAC,OAAO,CAAC,CAAC;QACvD,IAAI,IAAI,CAAC,MAAM,EAAE;YAAE,OAAO,GAAG,CAAC;QAC9B,IAAI,IAAI,CAAC,UAAU,EAAE,EAAE;YACrB,oCAAoC;YACpC,IAAI,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;gBAC3B,0EAA0E;gBAC1E,sEAAsE;gBACtE,IAAM,SAAS,GAAG,IAAI,CAAC,UAAU,CAAC,KAAK,CAAC,EACtC,GAAG,GAAG,IAAI,CAAC,GAAG,CAAC,SAAS,CAAC,EACzB,IAAI,GAAG,GAAG,CAAC,GAAG,CAAC,SAAS,CAAC,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;gBACtC,OAAO,GAAG,CAAC,QAAQ,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC,KAAK,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aAC3D;;gBAAM,OAAO,GAAG,GAAG,IAAI,CAAC,GAAG,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;SAChD;QAED,6DAA6D;QAC7D,yDAAyD;QACzD,IAAM,YAAY,GAAG,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC,GAAG,CAAC,KAAK,EAAE,CAAC,CAAC,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;QACxE,4DAA4D;QAC5D,IAAI,GAAG,GAAS,IAAI,CAAC;QACrB,IAAI,MAAM,GAAG,EAAE,CAAC;QAChB,iDAAiD;QACjD,OAAO,IAAI,EAAE;YACX,IAAM,MAAM,GAAG,GAAG,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC;YACrC,IAAM,MAAM,GAAG,GAAG,CAAC,GAAG,CAAC,MAAM,CAAC,GAAG,CAAC,YAAY,CAAC,CAAC,CAAC,KAAK,EAAE,KAAK,CAAC,CAAC;YAC/D,IAAI,MAAM,GAAG,MAAM,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;YACpC,GAAG,GAAG,MAAM,CAAC;YACb,IAAI,GAAG,CAAC,MAAM,EAAE,EAAE;gBAChB,OAAO,MAAM,GAAG,MAAM,CAAC;aACxB;iBAAM;gBACL,OAAO,MAAM,CAAC,MAAM,GAAG,CAAC;oBAAE,MAAM,GAAG,GAAG,GAAG,MAAM,CAAC;gBAChD,MAAM,GAAG,EAAE,GAAG,MAAM,GAAG,MAAM,CAAC;aAC/B;SACF;IACH,CAAC;IAED,sCAAsC;IACtC,yBAAU,GAAV;QACE,IAAI,IAAI,CAAC,QAAQ;YAAE,OAAO,IAAI,CAAC;QAC/B,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;IAClD,CAAC;IAED,8DAA8D;IAC9D,kBAAG,GAAH,UAAI,KAA6B;QAC/B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC;YAAE,KAAK,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,CAAC;QACvD,OAAO,IAAI,CAAC,QAAQ,CAAC,IAAI,CAAC,GAAG,GAAG,KAAK,CAAC,GAAG,EAAE,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,CAAC;IACpF,CAAC;IAED,8CAA8C;IAC9C,kBAAG,GAAH;QACE,OAAO,IAAI,CAAC,MAAM,EAAE,CAAC;IACvB,CAAC;IAED,uDAAuD;IACvD,iBAAE,GAAF,UAAG,KAAyC;QAC1C,OAAO,IAAI,CAAC,eAAe,CAAC,KAAK,CAAC,CAAC;IACrC,CAAC;IAED;;;;OAIG;IACH,6BAAc,GAAd,UAAe,OAAsB;QACnC,IAAI,OAAO,IAAI,OAAO,CAAC,OAAO;YAAE,OAAO,IAAI,CAAC,QAAQ,EAAE,CAAC;QACvD,OAAO,EAAE,WAAW,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC;IAC1C,CAAC;IACM,qBAAgB,GAAvB,UAAwB,GAA4B,EAAE,OAAsB;QAC1E,IAAM,MAAM,GAAG,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,WAAW,CAAC,CAAC;QAChD,OAAO,OAAO,IAAI,OAAO,CAAC,OAAO,CAAC,CAAC,CAAC,MAAM,CAAC,QAAQ,EAAE,CAAC,CAAC,CAAC,MAAM,CAAC;IACjE,CAAC;IAED,gBAAgB;IAChB,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,sBAAO,GAAP;QACE,OAAO,gBAAa,IAAI,CAAC,QAAQ,EAAE,WAAI,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC,QAAQ,CAAC,CAAC,CAAC,EAAE,OAAG,CAAC;IAC1E,CAAC;IA/2BM,eAAU,GAAG,IAAI,CAAC,OAAO,CAAC,cAAc,CAAC,CAAC;IAEjD,8BAA8B;IACvB,uBAAkB,GAAG,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,IAAI,CAAC,CAAC;IAChF,kBAAkB;IACX,SAAI,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;IAC9B,qBAAqB;IACd,UAAK,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;IACrC,kBAAkB;IACX,QAAG,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC;IAC7B,oBAAoB;IACb,SAAI,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,IAAI,CAAC,CAAC;IACpC,2BAA2B;IACpB,YAAO,GAAG,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC,CAAC;IAClC,4BAA4B;IACrB,cAAS,GAAG,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;IACxE,4BAA4B;IACrB,cAAS,GAAG,IAAI,CAAC,QAAQ,CAAC,CAAC,EAAE,UAAU,GAAG,CAAC,EAAE,KAAK,CAAC,CAAC;IA+1B7D,WAAC;CAAA,AAv6BD,IAu6BC;AAv6BY,oBAAI;AAy6BjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,YAAY,EAAE,EAAE,KAAK,EAAE,IAAI,EAAE,CAAC,CAAC;AACrE,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/map.js b/node_modules/bson/lib/map.js
new file mode 100644
index 0000000..bfcc220
--- /dev/null
+++ b/node_modules/bson/lib/map.js
@@ -0,0 +1,135 @@
+"use strict";
+/* eslint-disable @typescript-eslint/no-explicit-any */
+// We have an ES6 Map available, return the native instance
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Map = void 0;
+/** @public */
+var bsonMap;
+exports.Map = bsonMap;
+var check = function (potentialGlobal) {
+ // eslint-disable-next-line eqeqeq
+ return potentialGlobal && potentialGlobal.Math == Math && potentialGlobal;
+};
+// https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+function getGlobal() {
+ // eslint-disable-next-line no-undef
+ return (check(typeof globalThis === 'object' && globalThis) ||
+ check(typeof window === 'object' && window) ||
+ check(typeof self === 'object' && self) ||
+ check(typeof global === 'object' && global) ||
+ Function('return this')());
+}
+var bsonGlobal = getGlobal();
+if (Object.prototype.hasOwnProperty.call(bsonGlobal, 'Map')) {
+ exports.Map = bsonMap = bsonGlobal.Map;
+}
+else {
+ // We will return a polyfill
+ exports.Map = bsonMap = /** @class */ (function () {
+ function Map(array) {
+ if (array === void 0) { array = []; }
+ this._keys = [];
+ this._values = {};
+ for (var i = 0; i < array.length; i++) {
+ if (array[i] == null)
+ continue; // skip null and undefined
+ var entry = array[i];
+ var key = entry[0];
+ var value = entry[1];
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ }
+ }
+ Map.prototype.clear = function () {
+ this._keys = [];
+ this._values = {};
+ };
+ Map.prototype.delete = function (key) {
+ var value = this._values[key];
+ if (value == null)
+ return false;
+ // Delete entry
+ delete this._values[key];
+ // Remove the key from the ordered keys list
+ this._keys.splice(value.i, 1);
+ return true;
+ };
+ Map.prototype.entries = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? [key, _this._values[key].v] : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Map.prototype.forEach = function (callback, self) {
+ self = self || this;
+ for (var i = 0; i < this._keys.length; i++) {
+ var key = this._keys[i];
+ // Call the forEach callback
+ callback.call(self, this._values[key].v, key, self);
+ }
+ };
+ Map.prototype.get = function (key) {
+ return this._values[key] ? this._values[key].v : undefined;
+ };
+ Map.prototype.has = function (key) {
+ return this._values[key] != null;
+ };
+ Map.prototype.keys = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? key : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Map.prototype.set = function (key, value) {
+ if (this._values[key]) {
+ this._values[key].v = value;
+ return this;
+ }
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ return this;
+ };
+ Map.prototype.values = function () {
+ var _this = this;
+ var index = 0;
+ return {
+ next: function () {
+ var key = _this._keys[index++];
+ return {
+ value: key !== undefined ? _this._values[key].v : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ };
+ Object.defineProperty(Map.prototype, "size", {
+ get: function () {
+ return this._keys.length;
+ },
+ enumerable: false,
+ configurable: true
+ });
+ return Map;
+ }());
+}
+//# sourceMappingURL=map.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/map.js.map b/node_modules/bson/lib/map.js.map
new file mode 100644
index 0000000..a2fe982
--- /dev/null
+++ b/node_modules/bson/lib/map.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"map.js","sourceRoot":"","sources":["../src/map.ts"],"names":[],"mappings":";AAAA,uDAAuD;AACvD,2DAA2D;;;AAO3D,cAAc;AACd,IAAI,OAAuB,CAAC;AAiIR,sBAAG;AA/HvB,IAAM,KAAK,GAAG,UAAU,eAAoB;IAC1C,kCAAkC;IAClC,OAAO,eAAe,IAAI,eAAe,CAAC,IAAI,IAAI,IAAI,IAAI,eAAe,CAAC;AAC5E,CAAC,CAAC;AAEF,uEAAuE;AACvE,SAAS,SAAS;IAChB,oCAAoC;IACpC,OAAO,CACL,KAAK,CAAC,OAAO,UAAU,KAAK,QAAQ,IAAI,UAAU,CAAC;QACnD,KAAK,CAAC,OAAO,MAAM,KAAK,QAAQ,IAAI,MAAM,CAAC;QAC3C,KAAK,CAAC,OAAO,IAAI,KAAK,QAAQ,IAAI,IAAI,CAAC;QACvC,KAAK,CAAC,OAAO,MAAM,KAAK,QAAQ,IAAI,MAAM,CAAC;QAC3C,QAAQ,CAAC,aAAa,CAAC,EAAE,CAC1B,CAAC;AACJ,CAAC;AAED,IAAM,UAAU,GAAG,SAAS,EAAE,CAAC;AAC/B,IAAI,MAAM,CAAC,SAAS,CAAC,cAAc,CAAC,IAAI,CAAC,UAAU,EAAE,KAAK,CAAC,EAAE;IAC3D,cAAA,OAAO,GAAG,UAAU,CAAC,GAAG,CAAC;CAC1B;KAAM;IACL,4BAA4B;IAC5B,cAAA,OAAO,GAAI;QAGT,aAAY,KAA2B;YAA3B,sBAAA,EAAA,UAA2B;YACrC,IAAI,CAAC,KAAK,GAAG,EAAE,CAAC;YAChB,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC;YAElB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;gBACrC,IAAI,KAAK,CAAC,CAAC,CAAC,IAAI,IAAI;oBAAE,SAAS,CAAC,0BAA0B;gBAC1D,IAAM,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;gBACvB,IAAM,GAAG,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;gBACrB,IAAM,KAAK,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;gBACvB,2CAA2C;gBAC3C,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;gBACrB,8DAA8D;gBAC9D,2CAA2C;gBAC3C,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,EAAE,CAAC;aAC5D;QACH,CAAC;QACD,mBAAK,GAAL;YACE,IAAI,CAAC,KAAK,GAAG,EAAE,CAAC;YAChB,IAAI,CAAC,OAAO,GAAG,EAAE,CAAC;QACpB,CAAC;QACD,oBAAM,GAAN,UAAO,GAAW;YAChB,IAAM,KAAK,GAAG,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC;YAChC,IAAI,KAAK,IAAI,IAAI;gBAAE,OAAO,KAAK,CAAC;YAChC,eAAe;YACf,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC;YACzB,4CAA4C;YAC5C,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC,KAAK,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;YAC9B,OAAO,IAAI,CAAC;QACd,CAAC;QACD,qBAAO,GAAP;YAAA,iBAYC;YAXC,IAAI,KAAK,GAAG,CAAC,CAAC;YAEd,OAAO;gBACL,IAAI,EAAE;oBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;oBAChC,OAAO;wBACL,KAAK,EAAE,GAAG,KAAK,SAAS,CAAC,CAAC,CAAC,CAAC,GAAG,EAAE,KAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,SAAS;wBACjE,IAAI,EAAE,GAAG,KAAK,SAAS,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI;qBACvC,CAAC;gBACJ,CAAC;aACF,CAAC;QACJ,CAAC;QACD,qBAAO,GAAP,UAAQ,QAAmE,EAAE,IAAW;YACtF,IAAI,GAAG,IAAI,IAAI,IAAI,CAAC;YAEpB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;gBAC1C,IAAM,GAAG,GAAG,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;gBAC1B,4BAA4B;gBAC5B,QAAQ,CAAC,IAAI,CAAC,IAAI,EAAE,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,EAAE,GAAG,EAAE,IAAI,CAAC,CAAC;aACrD;QACH,CAAC;QACD,iBAAG,GAAH,UAAI,GAAW;YACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,SAAS,CAAC;QAC7D,CAAC;QACD,iBAAG,GAAH,UAAI,GAAW;YACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC;QACnC,CAAC;QACD,kBAAI,GAAJ;YAAA,iBAYC;YAXC,IAAI,KAAK,GAAG,CAAC,CAAC;YAEd,OAAO;gBACL,IAAI,EAAE;oBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;oBAChC,OAAO;wBACL,KAAK,EAAE,GAAG,KAAK,SAAS,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,SAAS;wBAC1C,IAAI,EAAE,GAAG,KAAK,SAAS,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI;qBACvC,CAAC;gBACJ,CAAC;aACF,CAAC;QACJ,CAAC;QACD,iBAAG,GAAH,UAAI,GAAW,EAAE,KAAU;YACzB,IAAI,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;gBACrB,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,GAAG,KAAK,CAAC;gBAC5B,OAAO,IAAI,CAAC;aACb;YAED,2CAA2C;YAC3C,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,CAAC,CAAC;YACrB,8DAA8D;YAC9D,2CAA2C;YAC3C,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,CAAC,EAAE,IAAI,CAAC,KAAK,CAAC,MAAM,GAAG,CAAC,EAAE,CAAC;YAC3D,OAAO,IAAI,CAAC;QACd,CAAC;QACD,oBAAM,GAAN;YAAA,iBAYC;YAXC,IAAI,KAAK,GAAG,CAAC,CAAC;YAEd,OAAO;gBACL,IAAI,EAAE;oBACJ,IAAM,GAAG,GAAG,KAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC,CAAC;oBAChC,OAAO;wBACL,KAAK,EAAE,GAAG,KAAK,SAAS,CAAC,CAAC,CAAC,KAAI,CAAC,OAAO,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,SAAS;wBAC1D,IAAI,EAAE,GAAG,KAAK,SAAS,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,IAAI;qBACvC,CAAC;gBACJ,CAAC;aACF,CAAC;QACJ,CAAC;QACD,sBAAI,qBAAI;iBAAR;gBACE,OAAO,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC;YAC3B,CAAC;;;WAAA;QACH,UAAC;IAAD,CAAC,AAtGU,GAsGoB,CAAC;CACjC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/max_key.js b/node_modules/bson/lib/max_key.js
new file mode 100644
index 0000000..c889eb8
--- /dev/null
+++ b/node_modules/bson/lib/max_key.js
@@ -0,0 +1,32 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.MaxKey = void 0;
+/**
+ * A class representation of the BSON MaxKey type.
+ * @public
+ */
+var MaxKey = /** @class */ (function () {
+ function MaxKey() {
+ if (!(this instanceof MaxKey))
+ return new MaxKey();
+ }
+ /** @internal */
+ MaxKey.prototype.toExtendedJSON = function () {
+ return { $maxKey: 1 };
+ };
+ /** @internal */
+ MaxKey.fromExtendedJSON = function () {
+ return new MaxKey();
+ };
+ /** @internal */
+ MaxKey.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ MaxKey.prototype.inspect = function () {
+ return 'new MaxKey()';
+ };
+ return MaxKey;
+}());
+exports.MaxKey = MaxKey;
+Object.defineProperty(MaxKey.prototype, '_bsontype', { value: 'MaxKey' });
+//# sourceMappingURL=max_key.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/max_key.js.map b/node_modules/bson/lib/max_key.js.map
new file mode 100644
index 0000000..5bf01f9
--- /dev/null
+++ b/node_modules/bson/lib/max_key.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"max_key.js","sourceRoot":"","sources":["../src/max_key.ts"],"names":[],"mappings":";;;AAKA;;;GAGG;AACH;IAGE;QACE,IAAI,CAAC,CAAC,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,EAAE,CAAC;IACrD,CAAC;IAED,gBAAgB;IAChB,+BAAc,GAAd;QACE,OAAO,EAAE,OAAO,EAAE,CAAC,EAAE,CAAC;IACxB,CAAC;IAED,gBAAgB;IACT,uBAAgB,GAAvB;QACE,OAAO,IAAI,MAAM,EAAE,CAAC;IACtB,CAAC;IAED,gBAAgB;IAChB,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,wBAAO,GAAP;QACE,OAAO,cAAc,CAAC;IACxB,CAAC;IACH,aAAC;AAAD,CAAC,AAzBD,IAyBC;AAzBY,wBAAM;AA2BnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/min_key.js b/node_modules/bson/lib/min_key.js
new file mode 100644
index 0000000..0912043
--- /dev/null
+++ b/node_modules/bson/lib/min_key.js
@@ -0,0 +1,32 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.MinKey = void 0;
+/**
+ * A class representation of the BSON MinKey type.
+ * @public
+ */
+var MinKey = /** @class */ (function () {
+ function MinKey() {
+ if (!(this instanceof MinKey))
+ return new MinKey();
+ }
+ /** @internal */
+ MinKey.prototype.toExtendedJSON = function () {
+ return { $minKey: 1 };
+ };
+ /** @internal */
+ MinKey.fromExtendedJSON = function () {
+ return new MinKey();
+ };
+ /** @internal */
+ MinKey.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ MinKey.prototype.inspect = function () {
+ return 'new MinKey()';
+ };
+ return MinKey;
+}());
+exports.MinKey = MinKey;
+Object.defineProperty(MinKey.prototype, '_bsontype', { value: 'MinKey' });
+//# sourceMappingURL=min_key.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/min_key.js.map b/node_modules/bson/lib/min_key.js.map
new file mode 100644
index 0000000..753012e
--- /dev/null
+++ b/node_modules/bson/lib/min_key.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"min_key.js","sourceRoot":"","sources":["../src/min_key.ts"],"names":[],"mappings":";;;AAKA;;;GAGG;AACH;IAGE;QACE,IAAI,CAAC,CAAC,IAAI,YAAY,MAAM,CAAC;YAAE,OAAO,IAAI,MAAM,EAAE,CAAC;IACrD,CAAC;IAED,gBAAgB;IAChB,+BAAc,GAAd;QACE,OAAO,EAAE,OAAO,EAAE,CAAC,EAAE,CAAC;IACxB,CAAC;IAED,gBAAgB;IACT,uBAAgB,GAAvB;QACE,OAAO,IAAI,MAAM,EAAE,CAAC;IACtB,CAAC;IAED,gBAAgB;IAChB,iBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,wBAAO,GAAP;QACE,OAAO,cAAc,CAAC;IACxB,CAAC;IACH,aAAC;AAAD,CAAC,AAzBD,IAyBC;AAzBY,wBAAM;AA2BnB,MAAM,CAAC,cAAc,CAAC,MAAM,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/objectid.js b/node_modules/bson/lib/objectid.js
new file mode 100644
index 0000000..4f4fb6b
--- /dev/null
+++ b/node_modules/bson/lib/objectid.js
@@ -0,0 +1,304 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.ObjectId = void 0;
+var buffer_1 = require("buffer");
+var ensure_buffer_1 = require("./ensure_buffer");
+var utils_1 = require("./parser/utils");
+// Regular expression that checks for hex value
+var checkForHexRegExp = new RegExp('^[0-9a-fA-F]{24}$');
+// Unique sequence for the current process (initialized on first use)
+var PROCESS_UNIQUE = null;
+var kId = Symbol('id');
+/**
+ * A class representation of the BSON ObjectId type.
+ * @public
+ */
+var ObjectId = /** @class */ (function () {
+ /**
+ * Create an ObjectId type
+ *
+ * @param id - Can be a 24 character hex string, 12 byte binary Buffer, or a number.
+ */
+ function ObjectId(id) {
+ if (!(this instanceof ObjectId))
+ return new ObjectId(id);
+ // Duck-typing to support ObjectId from different npm packages
+ if (id instanceof ObjectId) {
+ this[kId] = id.id;
+ this.__id = id.__id;
+ }
+ if (typeof id === 'object' && id && 'id' in id) {
+ if ('toHexString' in id && typeof id.toHexString === 'function') {
+ this[kId] = buffer_1.Buffer.from(id.toHexString(), 'hex');
+ }
+ else {
+ this[kId] = typeof id.id === 'string' ? buffer_1.Buffer.from(id.id) : id.id;
+ }
+ }
+ // The most common use case (blank id, new objectId instance)
+ if (id == null || typeof id === 'number') {
+ // Generate a new id
+ this[kId] = ObjectId.generate(typeof id === 'number' ? id : undefined);
+ // If we are caching the hex string
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+ if (ArrayBuffer.isView(id) && id.byteLength === 12) {
+ this[kId] = ensure_buffer_1.ensureBuffer(id);
+ }
+ if (typeof id === 'string') {
+ if (id.length === 12) {
+ var bytes = buffer_1.Buffer.from(id);
+ if (bytes.byteLength === 12) {
+ this[kId] = bytes;
+ }
+ }
+ else if (id.length === 24 && checkForHexRegExp.test(id)) {
+ this[kId] = buffer_1.Buffer.from(id, 'hex');
+ }
+ else {
+ throw new TypeError('Argument passed in must be a Buffer or string of 12 bytes or a string of 24 hex characters');
+ }
+ }
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+ Object.defineProperty(ObjectId.prototype, "id", {
+ /**
+ * The ObjectId bytes
+ * @readonly
+ */
+ get: function () {
+ return this[kId];
+ },
+ set: function (value) {
+ this[kId] = value;
+ if (ObjectId.cacheHexString) {
+ this.__id = value.toString('hex');
+ }
+ },
+ enumerable: false,
+ configurable: true
+ });
+ Object.defineProperty(ObjectId.prototype, "generationTime", {
+ /**
+ * The generation time of this ObjectId instance
+ * @deprecated Please use getTimestamp / createFromTime which returns an int32 epoch
+ */
+ get: function () {
+ return this.id.readInt32BE(0);
+ },
+ set: function (value) {
+ // Encode time into first 4 bytes
+ this.id.writeUInt32BE(value, 0);
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /** Returns the ObjectId id as a 24 character hex string representation */
+ ObjectId.prototype.toHexString = function () {
+ if (ObjectId.cacheHexString && this.__id) {
+ return this.__id;
+ }
+ var hexString = this.id.toString('hex');
+ if (ObjectId.cacheHexString && !this.__id) {
+ this.__id = hexString;
+ }
+ return hexString;
+ };
+ /**
+ * Update the ObjectId index
+ * @privateRemarks
+ * Used in generating new ObjectId's on the driver
+ * @internal
+ */
+ ObjectId.getInc = function () {
+ return (ObjectId.index = (ObjectId.index + 1) % 0xffffff);
+ };
+ /**
+ * Generate a 12 byte id buffer used in ObjectId's
+ *
+ * @param time - pass in a second based timestamp.
+ */
+ ObjectId.generate = function (time) {
+ if ('number' !== typeof time) {
+ time = ~~(Date.now() / 1000);
+ }
+ var inc = ObjectId.getInc();
+ var buffer = buffer_1.Buffer.alloc(12);
+ // 4-byte timestamp
+ buffer.writeUInt32BE(time, 0);
+ // set PROCESS_UNIQUE if yet not initialized
+ if (PROCESS_UNIQUE === null) {
+ PROCESS_UNIQUE = utils_1.randomBytes(5);
+ }
+ // 5-byte process unique
+ buffer[4] = PROCESS_UNIQUE[0];
+ buffer[5] = PROCESS_UNIQUE[1];
+ buffer[6] = PROCESS_UNIQUE[2];
+ buffer[7] = PROCESS_UNIQUE[3];
+ buffer[8] = PROCESS_UNIQUE[4];
+ // 3-byte counter
+ buffer[11] = inc & 0xff;
+ buffer[10] = (inc >> 8) & 0xff;
+ buffer[9] = (inc >> 16) & 0xff;
+ return buffer;
+ };
+ /**
+ * Converts the id into a 24 character hex string for printing
+ *
+ * @param format - The Buffer toString format parameter.
+ * @internal
+ */
+ ObjectId.prototype.toString = function (format) {
+ // Is the id a buffer then use the buffer toString method to return the format
+ if (format)
+ return this.id.toString(format);
+ return this.toHexString();
+ };
+ /**
+ * Converts to its JSON the 24 character hex string representation.
+ * @internal
+ */
+ ObjectId.prototype.toJSON = function () {
+ return this.toHexString();
+ };
+ /**
+ * Compares the equality of this ObjectId with `otherID`.
+ *
+ * @param otherId - ObjectId instance to compare against.
+ */
+ ObjectId.prototype.equals = function (otherId) {
+ if (otherId === undefined || otherId === null) {
+ return false;
+ }
+ if (otherId instanceof ObjectId) {
+ return this.toString() === otherId.toString();
+ }
+ if (typeof otherId === 'string' &&
+ ObjectId.isValid(otherId) &&
+ otherId.length === 12 &&
+ utils_1.isUint8Array(this.id)) {
+ return otherId === buffer_1.Buffer.prototype.toString.call(this.id, 'latin1');
+ }
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 24) {
+ return otherId.toLowerCase() === this.toHexString();
+ }
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 12) {
+ return buffer_1.Buffer.from(otherId).equals(this.id);
+ }
+ if (typeof otherId === 'object' &&
+ 'toHexString' in otherId &&
+ typeof otherId.toHexString === 'function') {
+ return otherId.toHexString() === this.toHexString();
+ }
+ return false;
+ };
+ /** Returns the generation date (accurate up to the second) that this ID was generated. */
+ ObjectId.prototype.getTimestamp = function () {
+ var timestamp = new Date();
+ var time = this.id.readUInt32BE(0);
+ timestamp.setTime(Math.floor(time) * 1000);
+ return timestamp;
+ };
+ /** @internal */
+ ObjectId.createPk = function () {
+ return new ObjectId();
+ };
+ /**
+ * Creates an ObjectId from a second based number, with the rest of the ObjectId zeroed out. Used for comparisons or sorting the ObjectId.
+ *
+ * @param time - an integer number representing a number of seconds.
+ */
+ ObjectId.createFromTime = function (time) {
+ var buffer = buffer_1.Buffer.from([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]);
+ // Encode time into first 4 bytes
+ buffer.writeUInt32BE(time, 0);
+ // Return the new objectId
+ return new ObjectId(buffer);
+ };
+ /**
+ * Creates an ObjectId from a hex string representation of an ObjectId.
+ *
+ * @param hexString - create a ObjectId from a passed in 24 character hexstring.
+ */
+ ObjectId.createFromHexString = function (hexString) {
+ // Throw an error if it's not a valid setup
+ if (typeof hexString === 'undefined' || (hexString != null && hexString.length !== 24)) {
+ throw new TypeError('Argument passed in must be a single String of 12 bytes or a string of 24 hex characters');
+ }
+ return new ObjectId(buffer_1.Buffer.from(hexString, 'hex'));
+ };
+ /**
+ * Checks if a value is a valid bson ObjectId
+ *
+ * @param id - ObjectId instance to validate.
+ */
+ ObjectId.isValid = function (id) {
+ if (id == null)
+ return false;
+ if (typeof id === 'number') {
+ return true;
+ }
+ if (typeof id === 'string') {
+ return id.length === 12 || (id.length === 24 && checkForHexRegExp.test(id));
+ }
+ if (id instanceof ObjectId) {
+ return true;
+ }
+ if (utils_1.isUint8Array(id) && id.length === 12) {
+ return true;
+ }
+ // Duck-Typing detection of ObjectId like objects
+ if (typeof id === 'object' && 'toHexString' in id && typeof id.toHexString === 'function') {
+ if (typeof id.id === 'string') {
+ return id.id.length === 12;
+ }
+ return id.toHexString().length === 24 && checkForHexRegExp.test(id.id.toString('hex'));
+ }
+ return false;
+ };
+ /** @internal */
+ ObjectId.prototype.toExtendedJSON = function () {
+ if (this.toHexString)
+ return { $oid: this.toHexString() };
+ return { $oid: this.toString('hex') };
+ };
+ /** @internal */
+ ObjectId.fromExtendedJSON = function (doc) {
+ return new ObjectId(doc.$oid);
+ };
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 24 character hex string representation.
+ * @internal
+ */
+ ObjectId.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ ObjectId.prototype.inspect = function () {
+ return "new ObjectId(\"" + this.toHexString() + "\")";
+ };
+ /** @internal */
+ ObjectId.index = ~~(Math.random() * 0xffffff);
+ return ObjectId;
+}());
+exports.ObjectId = ObjectId;
+// Deprecated methods
+Object.defineProperty(ObjectId.prototype, 'generate', {
+ value: utils_1.deprecate(function (time) { return ObjectId.generate(time); }, 'Please use the static `ObjectId.generate(time)` instead')
+});
+Object.defineProperty(ObjectId.prototype, 'getInc', {
+ value: utils_1.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+});
+Object.defineProperty(ObjectId.prototype, 'get_inc', {
+ value: utils_1.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+});
+Object.defineProperty(ObjectId, 'get_inc', {
+ value: utils_1.deprecate(function () { return ObjectId.getInc(); }, 'Please use the static `ObjectId.getInc()` instead')
+});
+Object.defineProperty(ObjectId.prototype, '_bsontype', { value: 'ObjectID' });
+//# sourceMappingURL=objectid.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/objectid.js.map b/node_modules/bson/lib/objectid.js.map
new file mode 100644
index 0000000..60a1bc0
--- /dev/null
+++ b/node_modules/bson/lib/objectid.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"objectid.js","sourceRoot":"","sources":["../src/objectid.ts"],"names":[],"mappings":";;;AAAA,iCAAgC;AAChC,iDAA+C;AAC/C,wCAAsE;AAEtE,+CAA+C;AAC/C,IAAM,iBAAiB,GAAG,IAAI,MAAM,CAAC,mBAAmB,CAAC,CAAC;AAE1D,qEAAqE;AACrE,IAAI,cAAc,GAAsB,IAAI,CAAC;AAc7C,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;AAEzB;;;GAGG;AACH;IAaE;;;;OAIG;IACH,kBAAY,EAAuD;QACjE,IAAI,CAAC,CAAC,IAAI,YAAY,QAAQ,CAAC;YAAE,OAAO,IAAI,QAAQ,CAAC,EAAE,CAAC,CAAC;QAEzD,8DAA8D;QAC9D,IAAI,EAAE,YAAY,QAAQ,EAAE;YAC1B,IAAI,CAAC,GAAG,CAAC,GAAG,EAAE,CAAC,EAAE,CAAC;YAClB,IAAI,CAAC,IAAI,GAAG,EAAE,CAAC,IAAI,CAAC;SACrB;QAED,IAAI,OAAO,EAAE,KAAK,QAAQ,IAAI,EAAE,IAAI,IAAI,IAAI,EAAE,EAAE;YAC9C,IAAI,aAAa,IAAI,EAAE,IAAI,OAAO,EAAE,CAAC,WAAW,KAAK,UAAU,EAAE;gBAC/D,IAAI,CAAC,GAAG,CAAC,GAAG,eAAM,CAAC,IAAI,CAAC,EAAE,CAAC,WAAW,EAAE,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM;gBACL,IAAI,CAAC,GAAG,CAAC,GAAG,OAAO,EAAE,CAAC,EAAE,KAAK,QAAQ,CAAC,CAAC,CAAC,eAAM,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC;aACpE;SACF;QAED,6DAA6D;QAC7D,IAAI,EAAE,IAAI,IAAI,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;YACxC,oBAAoB;YACpB,IAAI,CAAC,GAAG,CAAC,GAAG,QAAQ,CAAC,QAAQ,CAAC,OAAO,EAAE,KAAK,QAAQ,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,SAAS,CAAC,CAAC;YACvE,mCAAmC;YACnC,IAAI,QAAQ,CAAC,cAAc,EAAE;gBAC3B,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aACrC;SACF;QAED,IAAI,WAAW,CAAC,MAAM,CAAC,EAAE,CAAC,IAAI,EAAE,CAAC,UAAU,KAAK,EAAE,EAAE;YAClD,IAAI,CAAC,GAAG,CAAC,GAAG,4BAAY,CAAC,EAAE,CAAC,CAAC;SAC9B;QAED,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;YAC1B,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,EAAE;gBACpB,IAAM,KAAK,GAAG,eAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;gBAC9B,IAAI,KAAK,CAAC,UAAU,KAAK,EAAE,EAAE;oBAC3B,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;iBACnB;aACF;iBAAM,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE;gBACzD,IAAI,CAAC,GAAG,CAAC,GAAG,eAAM,CAAC,IAAI,CAAC,EAAE,EAAE,KAAK,CAAC,CAAC;aACpC;iBAAM;gBACL,MAAM,IAAI,SAAS,CACjB,4FAA4F,CAC7F,CAAC;aACH;SACF;QAED,IAAI,QAAQ,CAAC,cAAc,EAAE;YAC3B,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;SACrC;IACH,CAAC;IAMD,sBAAI,wBAAE;QAJN;;;WAGG;aACH;YACE,OAAO,IAAI,CAAC,GAAG,CAAC,CAAC;QACnB,CAAC;aAED,UAAO,KAAa;YAClB,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;YAClB,IAAI,QAAQ,CAAC,cAAc,EAAE;gBAC3B,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;aACnC;QACH,CAAC;;;OAPA;IAaD,sBAAI,oCAAc;QAJlB;;;WAGG;aACH;YACE,OAAO,IAAI,CAAC,EAAE,CAAC,WAAW,CAAC,CAAC,CAAC,CAAC;QAChC,CAAC;aAED,UAAmB,KAAa;YAC9B,iCAAiC;YACjC,IAAI,CAAC,EAAE,CAAC,aAAa,CAAC,KAAK,EAAE,CAAC,CAAC,CAAC;QAClC,CAAC;;;OALA;IAOD,0EAA0E;IAC1E,8BAAW,GAAX;QACE,IAAI,QAAQ,CAAC,cAAc,IAAI,IAAI,CAAC,IAAI,EAAE;YACxC,OAAO,IAAI,CAAC,IAAI,CAAC;SAClB;QAED,IAAM,SAAS,GAAG,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC;QAE1C,IAAI,QAAQ,CAAC,cAAc,IAAI,CAAC,IAAI,CAAC,IAAI,EAAE;YACzC,IAAI,CAAC,IAAI,GAAG,SAAS,CAAC;SACvB;QAED,OAAO,SAAS,CAAC;IACnB,CAAC;IAED;;;;;OAKG;IACI,eAAM,GAAb;QACE,OAAO,CAAC,QAAQ,CAAC,KAAK,GAAG,CAAC,QAAQ,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,QAAQ,CAAC,CAAC;IAC5D,CAAC;IAED;;;;OAIG;IACI,iBAAQ,GAAf,UAAgB,IAAa;QAC3B,IAAI,QAAQ,KAAK,OAAO,IAAI,EAAE;YAC5B,IAAI,GAAG,CAAC,CAAC,CAAC,IAAI,CAAC,GAAG,EAAE,GAAG,IAAI,CAAC,CAAC;SAC9B;QAED,IAAM,GAAG,GAAG,QAAQ,CAAC,MAAM,EAAE,CAAC;QAC9B,IAAM,MAAM,GAAG,eAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;QAEhC,mBAAmB;QACnB,MAAM,CAAC,aAAa,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;QAE9B,4CAA4C;QAC5C,IAAI,cAAc,KAAK,IAAI,EAAE;YAC3B,cAAc,GAAG,mBAAW,CAAC,CAAC,CAAC,CAAC;SACjC;QAED,wBAAwB;QACxB,MAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9B,MAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9B,MAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9B,MAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAC9B,MAAM,CAAC,CAAC,CAAC,GAAG,cAAc,CAAC,CAAC,CAAC,CAAC;QAE9B,iBAAiB;QACjB,MAAM,CAAC,EAAE,CAAC,GAAG,GAAG,GAAG,IAAI,CAAC;QACxB,MAAM,CAAC,EAAE,CAAC,GAAG,CAAC,GAAG,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;QAC/B,MAAM,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAE/B,OAAO,MAAM,CAAC;IAChB,CAAC;IAED;;;;;OAKG;IACH,2BAAQ,GAAR,UAAS,MAAe;QACtB,8EAA8E;QAC9E,IAAI,MAAM;YAAE,OAAO,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC;QAC5C,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;IAC5B,CAAC;IAED;;;OAGG;IACH,yBAAM,GAAN;QACE,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;IAC5B,CAAC;IAED;;;;OAIG;IACH,yBAAM,GAAN,UAAO,OAAyC;QAC9C,IAAI,OAAO,KAAK,SAAS,IAAI,OAAO,KAAK,IAAI,EAAE;YAC7C,OAAO,KAAK,CAAC;SACd;QAED,IAAI,OAAO,YAAY,QAAQ,EAAE;YAC/B,OAAO,IAAI,CAAC,QAAQ,EAAE,KAAK,OAAO,CAAC,QAAQ,EAAE,CAAC;SAC/C;QAED,IACE,OAAO,OAAO,KAAK,QAAQ;YAC3B,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC;YACzB,OAAO,CAAC,MAAM,KAAK,EAAE;YACrB,oBAAY,CAAC,IAAI,CAAC,EAAE,CAAC,EACrB;YACA,OAAO,OAAO,KAAK,eAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,IAAI,CAAC,EAAE,EAAE,QAAQ,CAAC,CAAC;SACtE;QAED,IAAI,OAAO,OAAO,KAAK,QAAQ,IAAI,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC,IAAI,OAAO,CAAC,MAAM,KAAK,EAAE,EAAE;YACrF,OAAO,OAAO,CAAC,WAAW,EAAE,KAAK,IAAI,CAAC,WAAW,EAAE,CAAC;SACrD;QAED,IAAI,OAAO,OAAO,KAAK,QAAQ,IAAI,QAAQ,CAAC,OAAO,CAAC,OAAO,CAAC,IAAI,OAAO,CAAC,MAAM,KAAK,EAAE,EAAE;YACrF,OAAO,eAAM,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;SAC7C;QAED,IACE,OAAO,OAAO,KAAK,QAAQ;YAC3B,aAAa,IAAI,OAAO;YACxB,OAAO,OAAO,CAAC,WAAW,KAAK,UAAU,EACzC;YACA,OAAO,OAAO,CAAC,WAAW,EAAE,KAAK,IAAI,CAAC,WAAW,EAAE,CAAC;SACrD;QAED,OAAO,KAAK,CAAC;IACf,CAAC;IAED,0FAA0F;IAC1F,+BAAY,GAAZ;QACE,IAAM,SAAS,GAAG,IAAI,IAAI,EAAE,CAAC;QAC7B,IAAM,IAAI,GAAG,IAAI,CAAC,EAAE,CAAC,YAAY,CAAC,CAAC,CAAC,CAAC;QACrC,SAAS,CAAC,OAAO,CAAC,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG,IAAI,CAAC,CAAC;QAC3C,OAAO,SAAS,CAAC;IACnB,CAAC;IAED,gBAAgB;IACT,iBAAQ,GAAf;QACE,OAAO,IAAI,QAAQ,EAAE,CAAC;IACxB,CAAC;IAED;;;;OAIG;IACI,uBAAc,GAArB,UAAsB,IAAY;QAChC,IAAM,MAAM,GAAG,eAAM,CAAC,IAAI,CAAC,CAAC,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;QACjE,iCAAiC;QACjC,MAAM,CAAC,aAAa,CAAC,IAAI,EAAE,CAAC,CAAC,CAAC;QAC9B,0BAA0B;QAC1B,OAAO,IAAI,QAAQ,CAAC,MAAM,CAAC,CAAC;IAC9B,CAAC;IAED;;;;OAIG;IACI,4BAAmB,GAA1B,UAA2B,SAAiB;QAC1C,2CAA2C;QAC3C,IAAI,OAAO,SAAS,KAAK,WAAW,IAAI,CAAC,SAAS,IAAI,IAAI,IAAI,SAAS,CAAC,MAAM,KAAK,EAAE,CAAC,EAAE;YACtF,MAAM,IAAI,SAAS,CACjB,yFAAyF,CAC1F,CAAC;SACH;QAED,OAAO,IAAI,QAAQ,CAAC,eAAM,CAAC,IAAI,CAAC,SAAS,EAAE,KAAK,CAAC,CAAC,CAAC;IACrD,CAAC;IAED;;;;OAIG;IACI,gBAAO,GAAd,UAAe,EAA0D;QACvE,IAAI,EAAE,IAAI,IAAI;YAAE,OAAO,KAAK,CAAC;QAE7B,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;YAC1B,OAAO,IAAI,CAAC;SACb;QAED,IAAI,OAAO,EAAE,KAAK,QAAQ,EAAE;YAC1B,OAAO,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,CAAC,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC;SAC7E;QAED,IAAI,EAAE,YAAY,QAAQ,EAAE;YAC1B,OAAO,IAAI,CAAC;SACb;QAED,IAAI,oBAAY,CAAC,EAAE,CAAC,IAAI,EAAE,CAAC,MAAM,KAAK,EAAE,EAAE;YACxC,OAAO,IAAI,CAAC;SACb;QAED,iDAAiD;QACjD,IAAI,OAAO,EAAE,KAAK,QAAQ,IAAI,aAAa,IAAI,EAAE,IAAI,OAAO,EAAE,CAAC,WAAW,KAAK,UAAU,EAAE;YACzF,IAAI,OAAO,EAAE,CAAC,EAAE,KAAK,QAAQ,EAAE;gBAC7B,OAAO,EAAE,CAAC,EAAE,CAAC,MAAM,KAAK,EAAE,CAAC;aAC5B;YACD,OAAO,EAAE,CAAC,WAAW,EAAE,CAAC,MAAM,KAAK,EAAE,IAAI,iBAAiB,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,CAAC,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC;SACxF;QAED,OAAO,KAAK,CAAC;IACf,CAAC;IAED,gBAAgB;IAChB,iCAAc,GAAd;QACE,IAAI,IAAI,CAAC,WAAW;YAAE,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,WAAW,EAAE,EAAE,CAAC;QAC1D,OAAO,EAAE,IAAI,EAAE,IAAI,CAAC,QAAQ,CAAC,KAAK,CAAC,EAAE,CAAC;IACxC,CAAC;IAED,gBAAgB;IACT,yBAAgB,GAAvB,UAAwB,GAAqB;QAC3C,OAAO,IAAI,QAAQ,CAAC,GAAG,CAAC,IAAI,CAAC,CAAC;IAChC,CAAC;IAED;;;;;OAKG;IACH,mBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,0BAAO,GAAP;QACE,OAAO,oBAAiB,IAAI,CAAC,WAAW,EAAE,QAAI,CAAC;IACjD,CAAC;IA7TD,gBAAgB;IACT,cAAK,GAAG,CAAC,CAAC,CAAC,IAAI,CAAC,MAAM,EAAE,GAAG,QAAQ,CAAC,CAAC;IA6T9C,eAAC;CAAA,AAjUD,IAiUC;AAjUY,4BAAQ;AAmUrB,qBAAqB;AACrB,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,UAAU,EAAE;IACpD,KAAK,EAAE,iBAAS,CACd,UAAC,IAAY,IAAK,OAAA,QAAQ,CAAC,QAAQ,CAAC,IAAI,CAAC,EAAvB,CAAuB,EACzC,yDAAyD,CAC1D;CACF,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,QAAQ,EAAE;IAClD,KAAK,EAAE,iBAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,EAAjB,CAAiB,EAAE,mDAAmD,CAAC;CAC/F,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,SAAS,EAAE;IACnD,KAAK,EAAE,iBAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,EAAjB,CAAiB,EAAE,mDAAmD,CAAC;CAC/F,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,EAAE,SAAS,EAAE;IACzC,KAAK,EAAE,iBAAS,CAAC,cAAM,OAAA,QAAQ,CAAC,MAAM,EAAE,EAAjB,CAAiB,EAAE,mDAAmD,CAAC;CAC/F,CAAC,CAAC;AAEH,MAAM,CAAC,cAAc,CAAC,QAAQ,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,UAAU,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/parser/calculate_size.js b/node_modules/bson/lib/parser/calculate_size.js
new file mode 100644
index 0000000..47930ae
--- /dev/null
+++ b/node_modules/bson/lib/parser/calculate_size.js
@@ -0,0 +1,193 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.calculateObjectSize = void 0;
+var buffer_1 = require("buffer");
+var binary_1 = require("../binary");
+var constants = require("../constants");
+var utils_1 = require("./utils");
+function calculateObjectSize(object, serializeFunctions, ignoreUndefined) {
+ var totalLength = 4 + 1;
+ if (Array.isArray(object)) {
+ for (var i = 0; i < object.length; i++) {
+ totalLength += calculateElement(i.toString(), object[i], serializeFunctions, true, ignoreUndefined);
+ }
+ }
+ else {
+ // If we have toBSON defined, override the current object
+ if (object.toBSON) {
+ object = object.toBSON();
+ }
+ // Calculate size
+ for (var key in object) {
+ totalLength += calculateElement(key, object[key], serializeFunctions, false, ignoreUndefined);
+ }
+ }
+ return totalLength;
+}
+exports.calculateObjectSize = calculateObjectSize;
+/** @internal */
+function calculateElement(name,
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+value, serializeFunctions, isArray, ignoreUndefined) {
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (isArray === void 0) { isArray = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = false; }
+ // If we have toBSON defined, override the current object
+ if (value && value.toBSON) {
+ value = value.toBSON();
+ }
+ switch (typeof value) {
+ case 'string':
+ return 1 + buffer_1.Buffer.byteLength(name, 'utf8') + 1 + 4 + buffer_1.Buffer.byteLength(value, 'utf8') + 1;
+ case 'number':
+ if (Math.floor(value) === value &&
+ value >= constants.JS_INT_MIN &&
+ value <= constants.JS_INT_MAX) {
+ if (value >= constants.BSON_INT32_MIN && value <= constants.BSON_INT32_MAX) {
+ // 32 bit
+ return (name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + (4 + 1);
+ }
+ else {
+ return (name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ }
+ else {
+ // 64 bit
+ return (name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ case 'undefined':
+ if (isArray || !ignoreUndefined)
+ return (name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ return 0;
+ case 'boolean':
+ return (name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 1);
+ case 'object':
+ if (value == null || value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ return (name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ return (name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + (12 + 1);
+ }
+ else if (value instanceof Date || utils_1.isDate(value)) {
+ return (name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ else if (ArrayBuffer.isView(value) ||
+ value instanceof ArrayBuffer ||
+ utils_1.isAnyArrayBuffer(value)) {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 4 + 1) + value.byteLength);
+ }
+ else if (value['_bsontype'] === 'Long' ||
+ value['_bsontype'] === 'Double' ||
+ value['_bsontype'] === 'Timestamp') {
+ return (name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ else if (value['_bsontype'] === 'Decimal128') {
+ return (name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + (16 + 1);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ // Calculate size depending on the availability of a scope
+ if (value.scope != null && Object.keys(value.scope).length > 0) {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ buffer_1.Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined));
+ }
+ else {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ buffer_1.Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1);
+ }
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ // Check what kind of subtype we have
+ if (value.sub_type === binary_1.Binary.SUBTYPE_BYTE_ARRAY) {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ (value.position + 1 + 4 + 1 + 4));
+ }
+ else {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) + (value.position + 1 + 4 + 1));
+ }
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ buffer_1.Buffer.byteLength(value.value, 'utf8') +
+ 4 +
+ 1 +
+ 1);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ // Set up correct object for serialization
+ var ordered_values = Object.assign({
+ $ref: value.collection,
+ $id: value.oid
+ }, value.fields);
+ // Add db reference if it exists
+ if (value.db != null) {
+ ordered_values['$db'] = value.db;
+ }
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ calculateObjectSize(ordered_values, serializeFunctions, ignoreUndefined));
+ }
+ else if (value instanceof RegExp || utils_1.isRegExp(value)) {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer_1.Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer_1.Buffer.byteLength(value.pattern, 'utf8') +
+ 1 +
+ buffer_1.Buffer.byteLength(value.options, 'utf8') +
+ 1);
+ }
+ else {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ calculateObjectSize(value, serializeFunctions, ignoreUndefined) +
+ 1);
+ }
+ case 'function':
+ // WTF for 0.4.X where typeof /someregexp/ === 'function'
+ if (value instanceof RegExp || utils_1.isRegExp(value) || String.call(value) === '[object RegExp]') {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ buffer_1.Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1);
+ }
+ else {
+ if (serializeFunctions && value.scope != null && Object.keys(value.scope).length > 0) {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ buffer_1.Buffer.byteLength(utils_1.normalizedFunctionString(value), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined));
+ }
+ else if (serializeFunctions) {
+ return ((name != null ? buffer_1.Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ buffer_1.Buffer.byteLength(utils_1.normalizedFunctionString(value), 'utf8') +
+ 1);
+ }
+ }
+ }
+ return 0;
+}
+//# sourceMappingURL=calculate_size.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/parser/calculate_size.js.map b/node_modules/bson/lib/parser/calculate_size.js.map
new file mode 100644
index 0000000..f257092
--- /dev/null
+++ b/node_modules/bson/lib/parser/calculate_size.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"calculate_size.js","sourceRoot":"","sources":["../../src/parser/calculate_size.ts"],"names":[],"mappings":";;;AAAA,iCAAgC;AAChC,oCAAmC;AAEnC,wCAA0C;AAC1C,iCAAuF;AAEvF,SAAgB,mBAAmB,CACjC,MAAgB,EAChB,kBAA4B,EAC5B,eAAyB;IAEzB,IAAI,WAAW,GAAG,CAAC,GAAG,CAAC,CAAC;IAExB,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;QACzB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;YACtC,WAAW,IAAI,gBAAgB,CAC7B,CAAC,CAAC,QAAQ,EAAE,EACZ,MAAM,CAAC,CAAC,CAAC,EACT,kBAAkB,EAClB,IAAI,EACJ,eAAe,CAChB,CAAC;SACH;KACF;SAAM;QACL,yDAAyD;QAEzD,IAAI,MAAM,CAAC,MAAM,EAAE;YACjB,MAAM,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC;SAC1B;QAED,iBAAiB;QACjB,KAAK,IAAM,GAAG,IAAI,MAAM,EAAE;YACxB,WAAW,IAAI,gBAAgB,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,CAAC,EAAE,kBAAkB,EAAE,KAAK,EAAE,eAAe,CAAC,CAAC;SAC/F;KACF;IAED,OAAO,WAAW,CAAC;AACrB,CAAC;AA/BD,kDA+BC;AAED,gBAAgB;AAChB,SAAS,gBAAgB,CACvB,IAAY;AACZ,8DAA8D;AAC9D,KAAU,EACV,kBAA0B,EAC1B,OAAe,EACf,eAAuB;IAFvB,mCAAA,EAAA,0BAA0B;IAC1B,wBAAA,EAAA,eAAe;IACf,gCAAA,EAAA,uBAAuB;IAEvB,yDAAyD;IACzD,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;QACzB,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;KACxB;IAED,QAAQ,OAAO,KAAK,EAAE;QACpB,KAAK,QAAQ;YACX,OAAO,CAAC,GAAG,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,eAAM,CAAC,UAAU,CAAC,KAAK,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;QAC5F,KAAK,QAAQ;YACX,IACE,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,KAAK;gBAC3B,KAAK,IAAI,SAAS,CAAC,UAAU;gBAC7B,KAAK,IAAI,SAAS,CAAC,UAAU,EAC7B;gBACA,IAAI,KAAK,IAAI,SAAS,CAAC,cAAc,IAAI,KAAK,IAAI,SAAS,CAAC,cAAc,EAAE;oBAC1E,SAAS;oBACT,OAAO,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC;iBAC3E;qBAAM;oBACL,OAAO,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC;iBAC3E;aACF;iBAAM;gBACL,SAAS;gBACT,OAAO,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC;aAC3E;QACH,KAAK,WAAW;YACd,IAAI,OAAO,IAAI,CAAC,eAAe;gBAC7B,OAAO,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;YACtE,OAAO,CAAC,CAAC;QACX,KAAK,SAAS;YACZ,OAAO,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC;QAC5E,KAAK,QAAQ;YACX,IAAI,KAAK,IAAI,IAAI,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBACvF,OAAO,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC;aACrE;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,OAAO,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,EAAE,GAAG,CAAC,CAAC,CAAC;aAC5E;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAI,cAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,OAAO,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC;aAC3E;iBAAM,IACL,WAAW,CAAC,MAAM,CAAC,KAAK,CAAC;gBACzB,KAAK,YAAY,WAAW;gBAC5B,wBAAgB,CAAC,KAAK,CAAC,EACvB;gBACA,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,GAAG,KAAK,CAAC,UAAU,CAC1F,CAAC;aACH;iBAAM,IACL,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM;gBAC7B,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ;gBAC/B,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAClC;gBACA,OAAO,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC;aAC3E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,OAAO,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,EAAE,GAAG,CAAC,CAAC,CAAC;aAC5E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;gBACxC,0DAA0D;gBAC1D,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,MAAM,GAAG,CAAC,EAAE;oBAC9D,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;wBACxD,CAAC;wBACD,CAAC;wBACD,CAAC;wBACD,eAAM,CAAC,UAAU,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,EAAE,MAAM,CAAC;wBAChD,CAAC;wBACD,mBAAmB,CAAC,KAAK,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC,CACtE,CAAC;iBACH;qBAAM;oBACL,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;wBACxD,CAAC;wBACD,CAAC;wBACD,eAAM,CAAC,UAAU,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,EAAE,MAAM,CAAC;wBAChD,CAAC,CACF,CAAC;iBACH;aACF;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,qCAAqC;gBACrC,IAAI,KAAK,CAAC,QAAQ,KAAK,eAAM,CAAC,kBAAkB,EAAE;oBAChD,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;wBACxD,CAAC,KAAK,CAAC,QAAQ,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,CACjC,CAAC;iBACH;qBAAM;oBACL,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,QAAQ,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,CACxF,CAAC;iBACH;aACF;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACxD,eAAM,CAAC,UAAU,CAAC,KAAK,CAAC,KAAK,EAAE,MAAM,CAAC;oBACtC,CAAC;oBACD,CAAC;oBACD,CAAC,CACF,CAAC;aACH;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,0CAA0C;gBAC1C,IAAM,cAAc,GAAG,MAAM,CAAC,MAAM,CAClC;oBACE,IAAI,EAAE,KAAK,CAAC,UAAU;oBACtB,GAAG,EAAE,KAAK,CAAC,GAAG;iBACf,EACD,KAAK,CAAC,MAAM,CACb,CAAC;gBAEF,gCAAgC;gBAChC,IAAI,KAAK,CAAC,EAAE,IAAI,IAAI,EAAE;oBACpB,cAAc,CAAC,KAAK,CAAC,GAAG,KAAK,CAAC,EAAE,CAAC;iBAClC;gBAED,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACxD,CAAC;oBACD,mBAAmB,CAAC,cAAc,EAAE,kBAAkB,EAAE,eAAe,CAAC,CACzE,CAAC;aACH;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAI,gBAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACxD,CAAC;oBACD,eAAM,CAAC,UAAU,CAAC,KAAK,CAAC,MAAM,EAAE,MAAM,CAAC;oBACvC,CAAC;oBACD,CAAC,KAAK,CAAC,MAAM,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACtB,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBAC1B,CAAC,KAAK,CAAC,SAAS,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACzB,CAAC,CACF,CAAC;aACH;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACxD,CAAC;oBACD,eAAM,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,MAAM,CAAC;oBACxC,CAAC;oBACD,eAAM,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,MAAM,CAAC;oBACxC,CAAC,CACF,CAAC;aACH;iBAAM;gBACL,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACxD,mBAAmB,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC;oBAC/D,CAAC,CACF,CAAC;aACH;QACH,KAAK,UAAU;YACb,yDAAyD;YACzD,IAAI,KAAK,YAAY,MAAM,IAAI,gBAAQ,CAAC,KAAK,CAAC,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,iBAAiB,EAAE;gBAC1F,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACxD,CAAC;oBACD,eAAM,CAAC,UAAU,CAAC,KAAK,CAAC,MAAM,EAAE,MAAM,CAAC;oBACvC,CAAC;oBACD,CAAC,KAAK,CAAC,MAAM,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACtB,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBAC1B,CAAC,KAAK,CAAC,SAAS,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;oBACzB,CAAC,CACF,CAAC;aACH;iBAAM;gBACL,IAAI,kBAAkB,IAAI,KAAK,CAAC,KAAK,IAAI,IAAI,IAAI,MAAM,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC,MAAM,GAAG,CAAC,EAAE;oBACpF,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;wBACxD,CAAC;wBACD,CAAC;wBACD,CAAC;wBACD,eAAM,CAAC,UAAU,CAAC,gCAAwB,CAAC,KAAK,CAAC,EAAE,MAAM,CAAC;wBAC1D,CAAC;wBACD,mBAAmB,CAAC,KAAK,CAAC,KAAK,EAAE,kBAAkB,EAAE,eAAe,CAAC,CACtE,CAAC;iBACH;qBAAM,IAAI,kBAAkB,EAAE;oBAC7B,OAAO,CACL,CAAC,IAAI,IAAI,IAAI,CAAC,CAAC,CAAC,eAAM,CAAC,UAAU,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;wBACxD,CAAC;wBACD,CAAC;wBACD,eAAM,CAAC,UAAU,CAAC,gCAAwB,CAAC,KAAK,CAAC,EAAE,MAAM,CAAC;wBAC1D,CAAC,CACF,CAAC;iBACH;aACF;KACJ;IAED,OAAO,CAAC,CAAC;AACX,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/parser/deserializer.js b/node_modules/bson/lib/parser/deserializer.js
new file mode 100644
index 0000000..54270cc
--- /dev/null
+++ b/node_modules/bson/lib/parser/deserializer.js
@@ -0,0 +1,575 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.deserialize = void 0;
+var buffer_1 = require("buffer");
+var binary_1 = require("../binary");
+var code_1 = require("../code");
+var constants = require("../constants");
+var db_ref_1 = require("../db_ref");
+var decimal128_1 = require("../decimal128");
+var double_1 = require("../double");
+var int_32_1 = require("../int_32");
+var long_1 = require("../long");
+var max_key_1 = require("../max_key");
+var min_key_1 = require("../min_key");
+var objectid_1 = require("../objectid");
+var regexp_1 = require("../regexp");
+var symbol_1 = require("../symbol");
+var timestamp_1 = require("../timestamp");
+var validate_utf8_1 = require("../validate_utf8");
+// Internal long versions
+var JS_INT_MAX_LONG = long_1.Long.fromNumber(constants.JS_INT_MAX);
+var JS_INT_MIN_LONG = long_1.Long.fromNumber(constants.JS_INT_MIN);
+var functionCache = {};
+function deserialize(buffer, options, isArray) {
+ options = options == null ? {} : options;
+ var index = options && options.index ? options.index : 0;
+ // Read the document size
+ var size = buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ if (size < 5) {
+ throw new Error("bson size must be >= 5, is " + size);
+ }
+ if (options.allowObjectSmallerThanBufferSize && buffer.length < size) {
+ throw new Error("buffer length " + buffer.length + " must be >= bson size " + size);
+ }
+ if (!options.allowObjectSmallerThanBufferSize && buffer.length !== size) {
+ throw new Error("buffer length " + buffer.length + " must === bson size " + size);
+ }
+ if (size + index > buffer.byteLength) {
+ throw new Error("(bson size " + size + " + options.index " + index + " must be <= buffer length " + buffer.byteLength + ")");
+ }
+ // Illegal end value
+ if (buffer[index + size - 1] !== 0) {
+ throw new Error("One object, sized correctly, with a spot for an EOO, but the EOO isn't 0x00");
+ }
+ // Start deserializtion
+ return deserializeObject(buffer, index, options, isArray);
+}
+exports.deserialize = deserialize;
+function deserializeObject(buffer, index, options, isArray) {
+ if (isArray === void 0) { isArray = false; }
+ var evalFunctions = options['evalFunctions'] == null ? false : options['evalFunctions'];
+ var cacheFunctions = options['cacheFunctions'] == null ? false : options['cacheFunctions'];
+ var fieldsAsRaw = options['fieldsAsRaw'] == null ? null : options['fieldsAsRaw'];
+ // Return raw bson buffer instead of parsing it
+ var raw = options['raw'] == null ? false : options['raw'];
+ // Return BSONRegExp objects instead of native regular expressions
+ var bsonRegExp = typeof options['bsonRegExp'] === 'boolean' ? options['bsonRegExp'] : false;
+ // Controls the promotion of values vs wrapper classes
+ var promoteBuffers = options['promoteBuffers'] == null ? false : options['promoteBuffers'];
+ var promoteLongs = options['promoteLongs'] == null ? true : options['promoteLongs'];
+ var promoteValues = options['promoteValues'] == null ? true : options['promoteValues'];
+ // Set the start index
+ var startIndex = index;
+ // Validate that we have at least 4 bytes of buffer
+ if (buffer.length < 5)
+ throw new Error('corrupt bson message < 5 bytes long');
+ // Read the document size
+ var size = buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // Ensure buffer is valid size
+ if (size < 5 || size > buffer.length)
+ throw new Error('corrupt bson message');
+ // Create holding object
+ var object = isArray ? [] : {};
+ // Used for arrays to skip having to perform utf8 decoding
+ var arrayIndex = 0;
+ var done = false;
+ // While we have more left data left keep parsing
+ while (!done) {
+ // Read the type
+ var elementType = buffer[index++];
+ // If we get a zero it's the last byte, exit
+ if (elementType === 0)
+ break;
+ // Get the start search index
+ var i = index;
+ // Locate the end of the c string
+ while (buffer[i] !== 0x00 && i < buffer.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer.byteLength)
+ throw new Error('Bad BSON Document: illegal CString');
+ var name = isArray ? arrayIndex++ : buffer.toString('utf8', index, i);
+ var value = void 0;
+ index = i + 1;
+ if (elementType === constants.BSON_DATA_STRING) {
+ var stringSize = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer.length - index ||
+ buffer[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ if (!validate_utf8_1.validateUtf8(buffer, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ value = buffer.toString('utf8', index, index + stringSize - 1);
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_OID) {
+ var oid = buffer_1.Buffer.alloc(12);
+ buffer.copy(oid, 0, index, index + 12);
+ value = new objectid_1.ObjectId(oid);
+ index = index + 12;
+ }
+ else if (elementType === constants.BSON_DATA_INT && promoteValues === false) {
+ value = new int_32_1.Int32(buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24));
+ }
+ else if (elementType === constants.BSON_DATA_INT) {
+ value =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ }
+ else if (elementType === constants.BSON_DATA_NUMBER && promoteValues === false) {
+ value = new double_1.Double(buffer.readDoubleLE(index));
+ index = index + 8;
+ }
+ else if (elementType === constants.BSON_DATA_NUMBER) {
+ value = buffer.readDoubleLE(index);
+ index = index + 8;
+ }
+ else if (elementType === constants.BSON_DATA_DATE) {
+ var lowBits = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ var highBits = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ value = new Date(new long_1.Long(lowBits, highBits).toNumber());
+ }
+ else if (elementType === constants.BSON_DATA_BOOLEAN) {
+ if (buffer[index] !== 0 && buffer[index] !== 1)
+ throw new Error('illegal boolean type value');
+ value = buffer[index++] === 1;
+ }
+ else if (elementType === constants.BSON_DATA_OBJECT) {
+ var _index = index;
+ var objectSize = buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ if (objectSize <= 0 || objectSize > buffer.length - index)
+ throw new Error('bad embedded document length in bson');
+ // We have a raw value
+ if (raw) {
+ value = buffer.slice(index, index + objectSize);
+ }
+ else {
+ value = deserializeObject(buffer, _index, options, false);
+ }
+ index = index + objectSize;
+ }
+ else if (elementType === constants.BSON_DATA_ARRAY) {
+ var _index = index;
+ var objectSize = buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ var arrayOptions = options;
+ // Stop index
+ var stopIndex = index + objectSize;
+ // All elements of array to be returned as raw bson
+ if (fieldsAsRaw && fieldsAsRaw[name]) {
+ arrayOptions = {};
+ for (var n in options) {
+ arrayOptions[n] = options[n];
+ }
+ arrayOptions['raw'] = true;
+ }
+ value = deserializeObject(buffer, _index, arrayOptions, true);
+ index = index + objectSize;
+ if (buffer[index - 1] !== 0)
+ throw new Error('invalid array terminator byte');
+ if (index !== stopIndex)
+ throw new Error('corrupted array bson');
+ }
+ else if (elementType === constants.BSON_DATA_UNDEFINED) {
+ value = undefined;
+ }
+ else if (elementType === constants.BSON_DATA_NULL) {
+ value = null;
+ }
+ else if (elementType === constants.BSON_DATA_LONG) {
+ // Unpack the low and high bits
+ var lowBits = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ var highBits = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ var long = new long_1.Long(lowBits, highBits);
+ // Promote the long if possible
+ if (promoteLongs && promoteValues === true) {
+ value =
+ long.lessThanOrEqual(JS_INT_MAX_LONG) && long.greaterThanOrEqual(JS_INT_MIN_LONG)
+ ? long.toNumber()
+ : long;
+ }
+ else {
+ value = long;
+ }
+ }
+ else if (elementType === constants.BSON_DATA_DECIMAL128) {
+ // Buffer to contain the decimal bytes
+ var bytes = buffer_1.Buffer.alloc(16);
+ // Copy the next 16 bytes into the bytes buffer
+ buffer.copy(bytes, 0, index, index + 16);
+ // Update index
+ index = index + 16;
+ // Assign the new Decimal128 value
+ var decimal128 = new decimal128_1.Decimal128(bytes);
+ // If we have an alternative mapper use that
+ if ('toObject' in decimal128 && typeof decimal128.toObject === 'function') {
+ value = decimal128.toObject();
+ }
+ else {
+ value = decimal128;
+ }
+ }
+ else if (elementType === constants.BSON_DATA_BINARY) {
+ var binarySize = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ var totalBinarySize = binarySize;
+ var subType = buffer[index++];
+ // Did we have a negative binary size, throw
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found');
+ // Is the length longer than the document
+ if (binarySize > buffer.byteLength)
+ throw new Error('Binary type size larger than document size');
+ // Decode as raw Buffer object if options specifies it
+ if (buffer['slice'] != null) {
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === binary_1.Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+ if (promoteBuffers && promoteValues) {
+ value = buffer.slice(index, index + binarySize);
+ }
+ else {
+ value = new binary_1.Binary(buffer.slice(index, index + binarySize), subType);
+ }
+ }
+ else {
+ var _buffer = buffer_1.Buffer.alloc(binarySize);
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === binary_1.Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+ // Copy the data
+ for (i = 0; i < binarySize; i++) {
+ _buffer[i] = buffer[index + i];
+ }
+ if (promoteBuffers && promoteValues) {
+ value = _buffer;
+ }
+ else {
+ value = new binary_1.Binary(_buffer, subType);
+ }
+ }
+ // Update the index
+ index = index + binarySize;
+ }
+ else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === false) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer[i] !== 0x00 && i < buffer.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var source = buffer.toString('utf8', index, i);
+ // Create the regexp
+ index = i + 1;
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer[i] !== 0x00 && i < buffer.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var regExpOptions = buffer.toString('utf8', index, i);
+ index = i + 1;
+ // For each option add the corresponding one for javascript
+ var optionsArray = new Array(regExpOptions.length);
+ // Parse options
+ for (i = 0; i < regExpOptions.length; i++) {
+ switch (regExpOptions[i]) {
+ case 'm':
+ optionsArray[i] = 'm';
+ break;
+ case 's':
+ optionsArray[i] = 'g';
+ break;
+ case 'i':
+ optionsArray[i] = 'i';
+ break;
+ }
+ }
+ value = new RegExp(source, optionsArray.join(''));
+ }
+ else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === true) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer[i] !== 0x00 && i < buffer.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var source = buffer.toString('utf8', index, i);
+ index = i + 1;
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer[i] !== 0x00 && i < buffer.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer.length)
+ throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ var regExpOptions = buffer.toString('utf8', index, i);
+ index = i + 1;
+ // Set the object
+ value = new regexp_1.BSONRegExp(source, regExpOptions);
+ }
+ else if (elementType === constants.BSON_DATA_SYMBOL) {
+ var stringSize = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer.length - index ||
+ buffer[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ var symbol = buffer.toString('utf8', index, index + stringSize - 1);
+ value = promoteValues ? symbol : new symbol_1.BSONSymbol(symbol);
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_TIMESTAMP) {
+ var lowBits = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ var highBits = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ value = new timestamp_1.Timestamp(lowBits, highBits);
+ }
+ else if (elementType === constants.BSON_DATA_MIN_KEY) {
+ value = new min_key_1.MinKey();
+ }
+ else if (elementType === constants.BSON_DATA_MAX_KEY) {
+ value = new max_key_1.MaxKey();
+ }
+ else if (elementType === constants.BSON_DATA_CODE) {
+ var stringSize = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ if (stringSize <= 0 ||
+ stringSize > buffer.length - index ||
+ buffer[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ var functionString = buffer.toString('utf8', index, index + stringSize - 1);
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ }
+ else {
+ value = isolateEval(functionString);
+ }
+ }
+ else {
+ value = new code_1.Code(functionString);
+ }
+ // Update parse index position
+ index = index + stringSize;
+ }
+ else if (elementType === constants.BSON_DATA_CODE_W_SCOPE) {
+ var totalSize = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ // Element cannot be shorter than totalSize + stringSize + documentSize + terminator
+ if (totalSize < 4 + 4 + 4 + 1) {
+ throw new Error('code_w_scope total size shorter minimum expected length');
+ }
+ // Get the code string size
+ var stringSize = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ // Check if we have a valid string
+ if (stringSize <= 0 ||
+ stringSize > buffer.length - index ||
+ buffer[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ // Javascript function
+ var functionString = buffer.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Parse the element
+ var _index = index;
+ // Decode the size of the object document
+ var objectSize = buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ // Decode the scope object
+ var scopeObject = deserializeObject(buffer, _index, options, false);
+ // Adjust the index
+ index = index + objectSize;
+ // Check if field length is too short
+ if (totalSize < 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too short, truncating scope');
+ }
+ // Check if totalSize field is too long
+ if (totalSize > 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too long, clips outer document');
+ }
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ }
+ else {
+ value = isolateEval(functionString);
+ }
+ value.scope = scopeObject;
+ }
+ else {
+ value = new code_1.Code(functionString, scopeObject);
+ }
+ }
+ else if (elementType === constants.BSON_DATA_DBPOINTER) {
+ // Get the code string size
+ var stringSize = buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ // Check if we have a valid string
+ if (stringSize <= 0 ||
+ stringSize > buffer.length - index ||
+ buffer[index + stringSize - 1] !== 0)
+ throw new Error('bad string length in bson');
+ // Namespace
+ if (!validate_utf8_1.validateUtf8(buffer, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ var namespace = buffer.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Read the oid
+ var oidBuffer = buffer_1.Buffer.alloc(12);
+ buffer.copy(oidBuffer, 0, index, index + 12);
+ var oid = new objectid_1.ObjectId(oidBuffer);
+ // Update the index
+ index = index + 12;
+ // Upgrade to DBRef type
+ value = new db_ref_1.DBRef(namespace, oid);
+ }
+ else {
+ throw new Error('Detected unknown BSON type ' + elementType.toString(16) + ' for fieldname "' + name + '"');
+ }
+ if (name === '__proto__') {
+ Object.defineProperty(object, name, {
+ value: value,
+ writable: true,
+ enumerable: true,
+ configurable: true
+ });
+ }
+ else {
+ object[name] = value;
+ }
+ }
+ // Check if the deserialization was against a valid array/object
+ if (size !== index - startIndex) {
+ if (isArray)
+ throw new Error('corrupt array bson');
+ throw new Error('corrupt object bson');
+ }
+ // check if object's $ keys are those of a DBRef
+ var dollarKeys = Object.keys(object).filter(function (k) { return k.startsWith('$'); });
+ var valid = true;
+ dollarKeys.forEach(function (k) {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1)
+ valid = false;
+ });
+ // if a $key not in "$ref", "$id", "$db", don't make a DBRef
+ if (!valid)
+ return object;
+ if (db_ref_1.isDBRefLike(object)) {
+ var copy = Object.assign({}, object);
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new db_ref_1.DBRef(object.$ref, object.$id, object.$db, copy);
+ }
+ return object;
+}
+/**
+ * Ensure eval is isolated, store the result in functionCache.
+ *
+ * @internal
+ */
+function isolateEval(functionString, functionCache, object) {
+ if (!functionCache)
+ return new Function(functionString);
+ // Check for cache hit, eval if missing and return cached function
+ if (functionCache[functionString] == null) {
+ functionCache[functionString] = new Function(functionString);
+ }
+ // Set the object
+ return functionCache[functionString].bind(object);
+}
+//# sourceMappingURL=deserializer.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/parser/deserializer.js.map b/node_modules/bson/lib/parser/deserializer.js.map
new file mode 100644
index 0000000..c399401
--- /dev/null
+++ b/node_modules/bson/lib/parser/deserializer.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"deserializer.js","sourceRoot":"","sources":["../../src/parser/deserializer.ts"],"names":[],"mappings":";;;AAAA,iCAAgC;AAChC,oCAAmC;AAEnC,gCAA+B;AAC/B,wCAA0C;AAC1C,oCAA0D;AAC1D,4CAA2C;AAC3C,oCAAmC;AACnC,oCAAkC;AAClC,gCAA+B;AAC/B,sCAAoC;AACpC,sCAAoC;AACpC,wCAAuC;AACvC,oCAAuC;AACvC,oCAAuC;AACvC,0CAAyC;AACzC,kDAAgD;AAgChD,yBAAyB;AACzB,IAAM,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;AAC9D,IAAM,eAAe,GAAG,WAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,CAAC,CAAC;AAE9D,IAAM,aAAa,GAAiC,EAAE,CAAC;AAEvD,SAAgB,WAAW,CACzB,MAAc,EACd,OAA2B,EAC3B,OAAiB;IAEjB,OAAO,GAAG,OAAO,IAAI,IAAI,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,OAAO,CAAC;IACzC,IAAM,KAAK,GAAG,OAAO,IAAI,OAAO,CAAC,KAAK,CAAC,CAAC,CAAC,OAAO,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC;IAC3D,yBAAyB;IACzB,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,CAAC;QACb,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;QACxB,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;QACzB,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;IAE5B,IAAI,IAAI,GAAG,CAAC,EAAE;QACZ,MAAM,IAAI,KAAK,CAAC,gCAA8B,IAAM,CAAC,CAAC;KACvD;IAED,IAAI,OAAO,CAAC,gCAAgC,IAAI,MAAM,CAAC,MAAM,GAAG,IAAI,EAAE;QACpE,MAAM,IAAI,KAAK,CAAC,mBAAiB,MAAM,CAAC,MAAM,8BAAyB,IAAM,CAAC,CAAC;KAChF;IAED,IAAI,CAAC,OAAO,CAAC,gCAAgC,IAAI,MAAM,CAAC,MAAM,KAAK,IAAI,EAAE;QACvE,MAAM,IAAI,KAAK,CAAC,mBAAiB,MAAM,CAAC,MAAM,4BAAuB,IAAM,CAAC,CAAC;KAC9E;IAED,IAAI,IAAI,GAAG,KAAK,GAAG,MAAM,CAAC,UAAU,EAAE;QACpC,MAAM,IAAI,KAAK,CACb,gBAAc,IAAI,yBAAoB,KAAK,kCAA6B,MAAM,CAAC,UAAU,MAAG,CAC7F,CAAC;KACH;IAED,oBAAoB;IACpB,IAAI,MAAM,CAAC,KAAK,GAAG,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC,EAAE;QAClC,MAAM,IAAI,KAAK,CAAC,6EAA6E,CAAC,CAAC;KAChG;IAED,uBAAuB;IACvB,OAAO,iBAAiB,CAAC,MAAM,EAAE,KAAK,EAAE,OAAO,EAAE,OAAO,CAAC,CAAC;AAC5D,CAAC;AAvCD,kCAuCC;AAED,SAAS,iBAAiB,CACxB,MAAc,EACd,KAAa,EACb,OAA2B,EAC3B,OAAe;IAAf,wBAAA,EAAA,eAAe;IAEf,IAAM,aAAa,GAAG,OAAO,CAAC,eAAe,CAAC,IAAI,IAAI,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,OAAO,CAAC,eAAe,CAAC,CAAC;IAC1F,IAAM,cAAc,GAAG,OAAO,CAAC,gBAAgB,CAAC,IAAI,IAAI,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,OAAO,CAAC,gBAAgB,CAAC,CAAC;IAE7F,IAAM,WAAW,GAAG,OAAO,CAAC,aAAa,CAAC,IAAI,IAAI,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC,OAAO,CAAC,aAAa,CAAC,CAAC;IAEnF,+CAA+C;IAC/C,IAAM,GAAG,GAAG,OAAO,CAAC,KAAK,CAAC,IAAI,IAAI,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,OAAO,CAAC,KAAK,CAAC,CAAC;IAE5D,kEAAkE;IAClE,IAAM,UAAU,GAAG,OAAO,OAAO,CAAC,YAAY,CAAC,KAAK,SAAS,CAAC,CAAC,CAAC,OAAO,CAAC,YAAY,CAAC,CAAC,CAAC,CAAC,KAAK,CAAC;IAE9F,sDAAsD;IACtD,IAAM,cAAc,GAAG,OAAO,CAAC,gBAAgB,CAAC,IAAI,IAAI,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,OAAO,CAAC,gBAAgB,CAAC,CAAC;IAC7F,IAAM,YAAY,GAAG,OAAO,CAAC,cAAc,CAAC,IAAI,IAAI,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC,OAAO,CAAC,cAAc,CAAC,CAAC;IACtF,IAAM,aAAa,GAAG,OAAO,CAAC,eAAe,CAAC,IAAI,IAAI,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC,OAAO,CAAC,eAAe,CAAC,CAAC;IAEzF,sBAAsB;IACtB,IAAM,UAAU,GAAG,KAAK,CAAC;IAEzB,mDAAmD;IACnD,IAAI,MAAM,CAAC,MAAM,GAAG,CAAC;QAAE,MAAM,IAAI,KAAK,CAAC,qCAAqC,CAAC,CAAC;IAE9E,yBAAyB;IACzB,IAAM,IAAI,GACR,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;IAE/F,8BAA8B;IAC9B,IAAI,IAAI,GAAG,CAAC,IAAI,IAAI,GAAG,MAAM,CAAC,MAAM;QAAE,MAAM,IAAI,KAAK,CAAC,sBAAsB,CAAC,CAAC;IAE9E,wBAAwB;IACxB,IAAM,MAAM,GAAa,OAAO,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,EAAE,CAAC;IAC3C,0DAA0D;IAC1D,IAAI,UAAU,GAAG,CAAC,CAAC;IACnB,IAAM,IAAI,GAAG,KAAK,CAAC;IAEnB,iDAAiD;IACjD,OAAO,CAAC,IAAI,EAAE;QACZ,gBAAgB;QAChB,IAAM,WAAW,GAAG,MAAM,CAAC,KAAK,EAAE,CAAC,CAAC;QAEpC,4CAA4C;QAC5C,IAAI,WAAW,KAAK,CAAC;YAAE,MAAM;QAE7B,6BAA6B;QAC7B,IAAI,CAAC,GAAG,KAAK,CAAC;QACd,iCAAiC;QACjC,OAAO,MAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE;YAC9C,CAAC,EAAE,CAAC;SACL;QAED,uEAAuE;QACvE,IAAI,CAAC,IAAI,MAAM,CAAC,UAAU;YAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;QAClF,IAAM,IAAI,GAAG,OAAO,CAAC,CAAC,CAAC,UAAU,EAAE,CAAC,CAAC,CAAC,MAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;QACxE,IAAI,KAAK,SAAA,CAAC;QAEV,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;QAEd,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YAC9C,IAAM,UAAU,GACd,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAG,MAAM,CAAC,MAAM,GAAG,KAAK;gBAClC,MAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAE/C,IAAI,CAAC,4BAAY,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,EAAE;gBACxD,MAAM,IAAI,KAAK,CAAC,uCAAuC,CAAC,CAAC;aAC1D;YAED,KAAK,GAAG,MAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;YAE/D,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,EAAE;YAClD,IAAM,GAAG,GAAG,eAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;YAC7B,MAAM,CAAC,IAAI,CAAC,GAAG,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;YACvC,KAAK,GAAG,IAAI,mBAAQ,CAAC,GAAG,CAAC,CAAC;YAC1B,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;SACpB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,IAAI,aAAa,KAAK,KAAK,EAAE;YAC7E,KAAK,GAAG,IAAI,cAAK,CACf,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,GAAG,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAC7F,CAAC;SACH;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,aAAa,EAAE;YAClD,KAAK;gBACH,MAAM,CAAC,KAAK,EAAE,CAAC;oBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;oBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;oBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;SAC3B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,aAAa,KAAK,KAAK,EAAE;YAChF,KAAK,GAAG,IAAI,eAAM,CAAC,MAAM,CAAC,YAAY,CAAC,KAAK,CAAC,CAAC,CAAC;YAC/C,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;SACnB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,KAAK,GAAG,MAAM,CAAC,YAAY,CAAC,KAAK,CAAC,CAAC;YACnC,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;SACnB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;YACnD,IAAM,OAAO,GACX,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,QAAQ,GACZ,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,KAAK,GAAG,IAAI,IAAI,CAAC,IAAI,WAAI,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC,QAAQ,EAAE,CAAC,CAAC;SAC1D;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;YACtD,IAAI,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,IAAI,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC;gBAAE,MAAM,IAAI,KAAK,CAAC,4BAA4B,CAAC,CAAC;YAC9F,KAAK,GAAG,MAAM,CAAC,KAAK,EAAE,CAAC,KAAK,CAAC,CAAC;SAC/B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,IAAM,MAAM,GAAG,KAAK,CAAC;YACrB,IAAM,UAAU,GACd,MAAM,CAAC,KAAK,CAAC;gBACb,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;gBACxB,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;gBACzB,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;YAC5B,IAAI,UAAU,IAAI,CAAC,IAAI,UAAU,GAAG,MAAM,CAAC,MAAM,GAAG,KAAK;gBACvD,MAAM,IAAI,KAAK,CAAC,sCAAsC,CAAC,CAAC;YAE1D,sBAAsB;YACtB,IAAI,GAAG,EAAE;gBACP,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,CAAC;aACjD;iBAAM;gBACL,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,MAAM,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC;aAC3D;YAED,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,eAAe,EAAE;YACpD,IAAM,MAAM,GAAG,KAAK,CAAC;YACrB,IAAM,UAAU,GACd,MAAM,CAAC,KAAK,CAAC;gBACb,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;gBACxB,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;gBACzB,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;YAC5B,IAAI,YAAY,GAAG,OAAO,CAAC;YAE3B,aAAa;YACb,IAAM,SAAS,GAAG,KAAK,GAAG,UAAU,CAAC;YAErC,mDAAmD;YACnD,IAAI,WAAW,IAAI,WAAW,CAAC,IAAI,CAAC,EAAE;gBACpC,YAAY,GAAG,EAAE,CAAC;gBAClB,KAAK,IAAM,CAAC,IAAI,OAAO,EAAE;oBACtB,YAEC,CAAC,CAAC,CAAC,GAAG,OAAO,CAAC,CAA6B,CAAC,CAAC;iBAChD;gBACD,YAAY,CAAC,KAAK,CAAC,GAAG,IAAI,CAAC;aAC5B;YAED,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,MAAM,EAAE,YAAY,EAAE,IAAI,CAAC,CAAC;YAC9D,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;YAE3B,IAAI,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,KAAK,CAAC;gBAAE,MAAM,IAAI,KAAK,CAAC,+BAA+B,CAAC,CAAC;YAC9E,IAAI,KAAK,KAAK,SAAS;gBAAE,MAAM,IAAI,KAAK,CAAC,sBAAsB,CAAC,CAAC;SAClE;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;YACxD,KAAK,GAAG,SAAS,CAAC;SACnB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;YACnD,KAAK,GAAG,IAAI,CAAC;SACd;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;YACnD,+BAA+B;YAC/B,IAAM,OAAO,GACX,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,QAAQ,GACZ,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,IAAI,GAAG,IAAI,WAAI,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;YACzC,+BAA+B;YAC/B,IAAI,YAAY,IAAI,aAAa,KAAK,IAAI,EAAE;gBAC1C,KAAK;oBACH,IAAI,CAAC,eAAe,CAAC,eAAe,CAAC,IAAI,IAAI,CAAC,kBAAkB,CAAC,eAAe,CAAC;wBAC/E,CAAC,CAAC,IAAI,CAAC,QAAQ,EAAE;wBACjB,CAAC,CAAC,IAAI,CAAC;aACZ;iBAAM;gBACL,KAAK,GAAG,IAAI,CAAC;aACd;SACF;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,oBAAoB,EAAE;YACzD,sCAAsC;YACtC,IAAM,KAAK,GAAG,eAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;YAC/B,+CAA+C;YAC/C,MAAM,CAAC,IAAI,CAAC,KAAK,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;YACzC,eAAe;YACf,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;YACnB,kCAAkC;YAClC,IAAM,UAAU,GAAG,IAAI,uBAAU,CAAC,KAAK,CAAyC,CAAC;YACjF,4CAA4C;YAC5C,IAAI,UAAU,IAAI,UAAU,IAAI,OAAO,UAAU,CAAC,QAAQ,KAAK,UAAU,EAAE;gBACzE,KAAK,GAAG,UAAU,CAAC,QAAQ,EAAE,CAAC;aAC/B;iBAAM;gBACL,KAAK,GAAG,UAAU,CAAC;aACpB;SACF;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,IAAI,UAAU,GACZ,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,eAAe,GAAG,UAAU,CAAC;YACnC,IAAM,OAAO,GAAG,MAAM,CAAC,KAAK,EAAE,CAAC,CAAC;YAEhC,4CAA4C;YAC5C,IAAI,UAAU,GAAG,CAAC;gBAAE,MAAM,IAAI,KAAK,CAAC,yCAAyC,CAAC,CAAC;YAE/E,yCAAyC;YACzC,IAAI,UAAU,GAAG,MAAM,CAAC,UAAU;gBAChC,MAAM,IAAI,KAAK,CAAC,4CAA4C,CAAC,CAAC;YAEhE,sDAAsD;YACtD,IAAI,MAAM,CAAC,OAAO,CAAC,IAAI,IAAI,EAAE;gBAC3B,qDAAqD;gBACrD,IAAI,OAAO,KAAK,eAAM,CAAC,kBAAkB,EAAE;oBACzC,UAAU;wBACR,MAAM,CAAC,KAAK,EAAE,CAAC;4BACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;4BACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;4BACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;oBAC1B,IAAI,UAAU,GAAG,CAAC;wBAChB,MAAM,IAAI,KAAK,CAAC,0DAA0D,CAAC,CAAC;oBAC9E,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,6DAA6D,CAAC,CAAC;oBACjF,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,8DAA8D,CAAC,CAAC;iBACnF;gBAED,IAAI,cAAc,IAAI,aAAa,EAAE;oBACnC,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,CAAC;iBACjD;qBAAM;oBACL,KAAK,GAAG,IAAI,eAAM,CAAC,MAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,UAAU,CAAC,EAAE,OAAO,CAAC,CAAC;iBACtE;aACF;iBAAM;gBACL,IAAM,OAAO,GAAG,eAAM,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC;gBACzC,qDAAqD;gBACrD,IAAI,OAAO,KAAK,eAAM,CAAC,kBAAkB,EAAE;oBACzC,UAAU;wBACR,MAAM,CAAC,KAAK,EAAE,CAAC;4BACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;4BACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;4BACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;oBAC1B,IAAI,UAAU,GAAG,CAAC;wBAChB,MAAM,IAAI,KAAK,CAAC,0DAA0D,CAAC,CAAC;oBAC9E,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,6DAA6D,CAAC,CAAC;oBACjF,IAAI,UAAU,GAAG,eAAe,GAAG,CAAC;wBAClC,MAAM,IAAI,KAAK,CAAC,8DAA8D,CAAC,CAAC;iBACnF;gBAED,gBAAgB;gBAChB,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,UAAU,EAAE,CAAC,EAAE,EAAE;oBAC/B,OAAO,CAAC,CAAC,CAAC,GAAG,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,CAAC;iBAChC;gBAED,IAAI,cAAc,IAAI,aAAa,EAAE;oBACnC,KAAK,GAAG,OAAO,CAAC;iBACjB;qBAAM;oBACL,KAAK,GAAG,IAAI,eAAM,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;iBACtC;aACF;YAED,mBAAmB;YACnB,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,UAAU,KAAK,KAAK,EAAE;YAC7E,6BAA6B;YAC7B,CAAC,GAAG,KAAK,CAAC;YACV,iCAAiC;YACjC,OAAO,MAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;YACD,uEAAuE;YACvE,IAAI,CAAC,IAAI,MAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;YAC9E,sBAAsB;YACtB,IAAM,MAAM,GAAG,MAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;YACjD,oBAAoB;YACpB,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;YAEd,6BAA6B;YAC7B,CAAC,GAAG,KAAK,CAAC;YACV,iCAAiC;YACjC,OAAO,MAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;YACD,uEAAuE;YACvE,IAAI,CAAC,IAAI,MAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;YAC9E,sBAAsB;YACtB,IAAM,aAAa,GAAG,MAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;YACxD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;YAEd,2DAA2D;YAC3D,IAAM,YAAY,GAAG,IAAI,KAAK,CAAC,aAAa,CAAC,MAAM,CAAC,CAAC;YAErD,gBAAgB;YAChB,KAAK,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,aAAa,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;gBACzC,QAAQ,aAAa,CAAC,CAAC,CAAC,EAAE;oBACxB,KAAK,GAAG;wBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;wBACtB,MAAM;oBACR,KAAK,GAAG;wBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;wBACtB,MAAM;oBACR,KAAK,GAAG;wBACN,YAAY,CAAC,CAAC,CAAC,GAAG,GAAG,CAAC;wBACtB,MAAM;iBACT;aACF;YAED,KAAK,GAAG,IAAI,MAAM,CAAC,MAAM,EAAE,YAAY,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC;SACnD;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,IAAI,UAAU,KAAK,IAAI,EAAE;YAC5E,6BAA6B;YAC7B,CAAC,GAAG,KAAK,CAAC;YACV,iCAAiC;YACjC,OAAO,MAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;YACD,uEAAuE;YACvE,IAAI,CAAC,IAAI,MAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;YAC9E,sBAAsB;YACtB,IAAM,MAAM,GAAG,MAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;YACjD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;YAEd,6BAA6B;YAC7B,CAAC,GAAG,KAAK,CAAC;YACV,iCAAiC;YACjC,OAAO,MAAM,CAAC,CAAC,CAAC,KAAK,IAAI,IAAI,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE;gBAC9C,CAAC,EAAE,CAAC;aACL;YACD,uEAAuE;YACvE,IAAI,CAAC,IAAI,MAAM,CAAC,MAAM;gBAAE,MAAM,IAAI,KAAK,CAAC,oCAAoC,CAAC,CAAC;YAC9E,sBAAsB;YACtB,IAAM,aAAa,GAAG,MAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,CAAC,CAAC,CAAC;YACxD,KAAK,GAAG,CAAC,GAAG,CAAC,CAAC;YAEd,iBAAiB;YACjB,KAAK,GAAG,IAAI,mBAAU,CAAC,MAAM,EAAE,aAAa,CAAC,CAAC;SAC/C;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,gBAAgB,EAAE;YACrD,IAAM,UAAU,GACd,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAG,MAAM,CAAC,MAAM,GAAG,KAAK;gBAClC,MAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAC/C,IAAM,MAAM,GAAG,MAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;YACtE,KAAK,GAAG,aAAa,CAAC,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,IAAI,mBAAU,CAAC,MAAM,CAAC,CAAC;YACxD,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;YACxD,IAAM,OAAO,GACX,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IAAM,QAAQ,GACZ,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAE1B,KAAK,GAAG,IAAI,qBAAS,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;SAC1C;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;YACtD,KAAK,GAAG,IAAI,gBAAM,EAAE,CAAC;SACtB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,iBAAiB,EAAE;YACtD,KAAK,GAAG,IAAI,gBAAM,EAAE,CAAC;SACtB;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,cAAc,EAAE;YACnD,IAAM,UAAU,GACd,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAG,MAAM,CAAC,MAAM,GAAG,KAAK;gBAClC,MAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAC/C,IAAM,cAAc,GAAG,MAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;YAE9E,qCAAqC;YACrC,IAAI,aAAa,EAAE;gBACjB,+EAA+E;gBAC/E,IAAI,cAAc,EAAE;oBAClB,uEAAuE;oBACvE,KAAK,GAAG,WAAW,CAAC,cAAc,EAAE,aAAa,EAAE,MAAM,CAAC,CAAC;iBAC5D;qBAAM;oBACL,KAAK,GAAG,WAAW,CAAC,cAAc,CAAC,CAAC;iBACrC;aACF;iBAAM;gBACL,KAAK,GAAG,IAAI,WAAI,CAAC,cAAc,CAAC,CAAC;aAClC;YAED,8BAA8B;YAC9B,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;SAC5B;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,sBAAsB,EAAE;YAC3D,IAAM,SAAS,GACb,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAE1B,oFAAoF;YACpF,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;gBAC7B,MAAM,IAAI,KAAK,CAAC,yDAAyD,CAAC,CAAC;aAC5E;YAED,2BAA2B;YAC3B,IAAM,UAAU,GACd,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,kCAAkC;YAClC,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAG,MAAM,CAAC,MAAM,GAAG,KAAK;gBAClC,MAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAE/C,sBAAsB;YACtB,IAAM,cAAc,GAAG,MAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;YAC9E,8BAA8B;YAC9B,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;YAC3B,oBAAoB;YACpB,IAAM,MAAM,GAAG,KAAK,CAAC;YACrB,yCAAyC;YACzC,IAAM,UAAU,GACd,MAAM,CAAC,KAAK,CAAC;gBACb,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC;gBACxB,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC;gBACzB,CAAC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,CAAC;YAC5B,0BAA0B;YAC1B,IAAM,WAAW,GAAG,iBAAiB,CAAC,MAAM,EAAE,MAAM,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC;YACtE,mBAAmB;YACnB,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;YAE3B,qCAAqC;YACrC,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,UAAU,GAAG,UAAU,EAAE;gBAC/C,MAAM,IAAI,KAAK,CAAC,wDAAwD,CAAC,CAAC;aAC3E;YAED,uCAAuC;YACvC,IAAI,SAAS,GAAG,CAAC,GAAG,CAAC,GAAG,UAAU,GAAG,UAAU,EAAE;gBAC/C,MAAM,IAAI,KAAK,CAAC,2DAA2D,CAAC,CAAC;aAC9E;YAED,qCAAqC;YACrC,IAAI,aAAa,EAAE;gBACjB,+EAA+E;gBAC/E,IAAI,cAAc,EAAE;oBAClB,uEAAuE;oBACvE,KAAK,GAAG,WAAW,CAAC,cAAc,EAAE,aAAa,EAAE,MAAM,CAAC,CAAC;iBAC5D;qBAAM;oBACL,KAAK,GAAG,WAAW,CAAC,cAAc,CAAC,CAAC;iBACrC;gBAED,KAAK,CAAC,KAAK,GAAG,WAAW,CAAC;aAC3B;iBAAM;gBACL,KAAK,GAAG,IAAI,WAAI,CAAC,cAAc,EAAE,WAAW,CAAC,CAAC;aAC/C;SACF;aAAM,IAAI,WAAW,KAAK,SAAS,CAAC,mBAAmB,EAAE;YACxD,2BAA2B;YAC3B,IAAM,UAAU,GACd,MAAM,CAAC,KAAK,EAAE,CAAC;gBACf,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,CAAC,CAAC;gBACtB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC;gBACvB,CAAC,MAAM,CAAC,KAAK,EAAE,CAAC,IAAI,EAAE,CAAC,CAAC;YAC1B,kCAAkC;YAClC,IACE,UAAU,IAAI,CAAC;gBACf,UAAU,GAAG,MAAM,CAAC,MAAM,GAAG,KAAK;gBAClC,MAAM,CAAC,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,KAAK,CAAC;gBAEpC,MAAM,IAAI,KAAK,CAAC,2BAA2B,CAAC,CAAC;YAC/C,YAAY;YACZ,IAAI,CAAC,4BAAY,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,EAAE;gBACxD,MAAM,IAAI,KAAK,CAAC,uCAAuC,CAAC,CAAC;aAC1D;YACD,IAAM,SAAS,GAAG,MAAM,CAAC,QAAQ,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,GAAG,UAAU,GAAG,CAAC,CAAC,CAAC;YACzE,8BAA8B;YAC9B,KAAK,GAAG,KAAK,GAAG,UAAU,CAAC;YAE3B,eAAe;YACf,IAAM,SAAS,GAAG,eAAM,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;YACnC,MAAM,CAAC,IAAI,CAAC,SAAS,EAAE,CAAC,EAAE,KAAK,EAAE,KAAK,GAAG,EAAE,CAAC,CAAC;YAC7C,IAAM,GAAG,GAAG,IAAI,mBAAQ,CAAC,SAAS,CAAC,CAAC;YAEpC,mBAAmB;YACnB,KAAK,GAAG,KAAK,GAAG,EAAE,CAAC;YAEnB,wBAAwB;YACxB,KAAK,GAAG,IAAI,cAAK,CAAC,SAAS,EAAE,GAAG,CAAC,CAAC;SACnC;aAAM;YACL,MAAM,IAAI,KAAK,CACb,6BAA6B,GAAG,WAAW,CAAC,QAAQ,CAAC,EAAE,CAAC,GAAG,kBAAkB,GAAG,IAAI,GAAG,GAAG,CAC3F,CAAC;SACH;QACD,IAAI,IAAI,KAAK,WAAW,EAAE;YACxB,MAAM,CAAC,cAAc,CAAC,MAAM,EAAE,IAAI,EAAE;gBAClC,KAAK,OAAA;gBACL,QAAQ,EAAE,IAAI;gBACd,UAAU,EAAE,IAAI;gBAChB,YAAY,EAAE,IAAI;aACnB,CAAC,CAAC;SACJ;aAAM;YACL,MAAM,CAAC,IAAI,CAAC,GAAG,KAAK,CAAC;SACtB;KACF;IAED,gEAAgE;IAChE,IAAI,IAAI,KAAK,KAAK,GAAG,UAAU,EAAE;QAC/B,IAAI,OAAO;YAAE,MAAM,IAAI,KAAK,CAAC,oBAAoB,CAAC,CAAC;QACnD,MAAM,IAAI,KAAK,CAAC,qBAAqB,CAAC,CAAC;KACxC;IAED,gDAAgD;IAChD,IAAM,UAAU,GAAG,MAAM,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,MAAM,CAAC,UAAA,CAAC,IAAI,OAAA,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,EAAjB,CAAiB,CAAC,CAAC;IACtE,IAAI,KAAK,GAAG,IAAI,CAAC;IACjB,UAAU,CAAC,OAAO,CAAC,UAAA,CAAC;QAClB,IAAI,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,CAAC,CAAC;YAAE,KAAK,GAAG,KAAK,CAAC;IAC9D,CAAC,CAAC,CAAC;IAEH,4DAA4D;IAC5D,IAAI,CAAC,KAAK;QAAE,OAAO,MAAM,CAAC;IAE1B,IAAI,oBAAW,CAAC,MAAM,CAAC,EAAE;QACvB,IAAM,IAAI,GAAG,MAAM,CAAC,MAAM,CAAC,EAAE,EAAE,MAAM,CAAuB,CAAC;QAC7D,OAAO,IAAI,CAAC,IAAI,CAAC;QACjB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAI,CAAC,GAAG,CAAC;QAChB,OAAO,IAAI,cAAK,CAAC,MAAM,CAAC,IAAI,EAAE,MAAM,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;KAC7D;IAED,OAAO,MAAM,CAAC;AAChB,CAAC;AAED;;;;GAIG;AACH,SAAS,WAAW,CAClB,cAAsB,EACtB,aAA4C,EAC5C,MAAiB;IAEjB,IAAI,CAAC,aAAa;QAAE,OAAO,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC;IACxD,kEAAkE;IAClE,IAAI,aAAa,CAAC,cAAc,CAAC,IAAI,IAAI,EAAE;QACzC,aAAa,CAAC,cAAc,CAAC,GAAG,IAAI,QAAQ,CAAC,cAAc,CAAC,CAAC;KAC9D;IAED,iBAAiB;IACjB,OAAO,aAAa,CAAC,cAAc,CAAC,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;AACpD,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/parser/serializer.js b/node_modules/bson/lib/parser/serializer.js
new file mode 100644
index 0000000..4a48022
--- /dev/null
+++ b/node_modules/bson/lib/parser/serializer.js
@@ -0,0 +1,868 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.serializeInto = void 0;
+var binary_1 = require("../binary");
+var constants = require("../constants");
+var ensure_buffer_1 = require("../ensure_buffer");
+var extended_json_1 = require("../extended_json");
+var float_parser_1 = require("../float_parser");
+var long_1 = require("../long");
+var map_1 = require("../map");
+var utils_1 = require("./utils");
+var regexp = /\x00/; // eslint-disable-line no-control-regex
+var ignoreKeys = new Set(['$db', '$ref', '$id', '$clusterTime']);
+/*
+ * isArray indicates if we are writing to a BSON array (type 0x04)
+ * which forces the "key" which really an array index as a string to be written as ascii
+ * This will catch any errors in index as a string generation
+ */
+function serializeString(buffer, key, value, index, isArray) {
+ // Encode String type
+ buffer[index++] = constants.BSON_DATA_STRING;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes + 1;
+ buffer[index - 1] = 0;
+ // Write the string
+ var size = buffer.write(value, index + 4, undefined, 'utf8');
+ // Write the size of the string to buffer
+ buffer[index + 3] = ((size + 1) >> 24) & 0xff;
+ buffer[index + 2] = ((size + 1) >> 16) & 0xff;
+ buffer[index + 1] = ((size + 1) >> 8) & 0xff;
+ buffer[index] = (size + 1) & 0xff;
+ // Update index
+ index = index + 4 + size;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+}
+function serializeNumber(buffer, key, value, index, isArray) {
+ // We have an integer value
+ // TODO(NODE-2529): Add support for big int
+ if (Number.isInteger(value) &&
+ value >= constants.BSON_INT32_MIN &&
+ value <= constants.BSON_INT32_MAX) {
+ // If the value fits in 32 bits encode as int32
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ }
+ else {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ float_parser_1.writeIEEE754(buffer, value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ }
+ return index;
+}
+function serializeNull(buffer, key, _, index, isArray) {
+ // Set long type
+ buffer[index++] = constants.BSON_DATA_NULL;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+}
+function serializeBoolean(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BOOLEAN;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Encode the boolean value
+ buffer[index++] = value ? 1 : 0;
+ return index;
+}
+function serializeDate(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_DATE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ var dateInMilis = long_1.Long.fromNumber(value.getTime());
+ var lowBits = dateInMilis.getLowBits();
+ var highBits = dateInMilis.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+}
+function serializeRegExp(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ if (value.source && value.source.match(regexp) != null) {
+ throw Error('value ' + value.source + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.source, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the parameters
+ if (value.ignoreCase)
+ buffer[index++] = 0x69; // i
+ if (value.global)
+ buffer[index++] = 0x73; // s
+ if (value.multiline)
+ buffer[index++] = 0x6d; // m
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+}
+function serializeBSONRegExp(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Check the pattern for 0 bytes
+ if (value.pattern.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('pattern ' + value.pattern + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.pattern, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the options
+ index = index + buffer.write(value.options.split('').sort().join(''), index, undefined, 'utf8');
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+}
+function serializeMinMax(buffer, key, value, index, isArray) {
+ // Write the type of either min or max key
+ if (value === null) {
+ buffer[index++] = constants.BSON_DATA_NULL;
+ }
+ else if (value._bsontype === 'MinKey') {
+ buffer[index++] = constants.BSON_DATA_MIN_KEY;
+ }
+ else {
+ buffer[index++] = constants.BSON_DATA_MAX_KEY;
+ }
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+}
+function serializeObjectId(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OID;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the objectId into the shared buffer
+ if (typeof value.id === 'string') {
+ buffer.write(value.id, index, undefined, 'binary');
+ }
+ else if (utils_1.isUint8Array(value.id)) {
+ // Use the standard JS methods here because buffer.copy() is buggy with the
+ // browser polyfill
+ buffer.set(value.id.subarray(0, 12), index);
+ }
+ else {
+ throw new TypeError('object [' + JSON.stringify(value) + '] is not a valid ObjectId');
+ }
+ // Adjust index
+ return index + 12;
+}
+function serializeBuffer(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Get size of the buffer (current write point)
+ var size = value.length;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the default subtype
+ buffer[index++] = constants.BSON_BINARY_SUBTYPE_DEFAULT;
+ // Copy the content form the binary field to the buffer
+ buffer.set(ensure_buffer_1.ensureBuffer(value), index);
+ // Adjust the index
+ index = index + size;
+ return index;
+}
+function serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray, path) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (isArray === void 0) { isArray = false; }
+ if (path === void 0) { path = []; }
+ for (var i = 0; i < path.length; i++) {
+ if (path[i] === value)
+ throw new Error('cyclic dependency detected');
+ }
+ // Push value to stack
+ path.push(value);
+ // Write the type
+ buffer[index++] = Array.isArray(value) ? constants.BSON_DATA_ARRAY : constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ var endIndex = serializeInto(buffer, value, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined, path);
+ // Pop stack
+ path.pop();
+ return endIndex;
+}
+function serializeDecimal128(buffer, key, value, index, isArray) {
+ buffer[index++] = constants.BSON_DATA_DECIMAL128;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the data from the value
+ // Prefer the standard JS methods because their typechecking is not buggy,
+ // unlike the `buffer` polyfill's.
+ buffer.set(value.bytes.subarray(0, 16), index);
+ return index + 16;
+}
+function serializeLong(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] =
+ value._bsontype === 'Long' ? constants.BSON_DATA_LONG : constants.BSON_DATA_TIMESTAMP;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ var lowBits = value.getLowBits();
+ var highBits = value.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+}
+function serializeInt32(buffer, key, value, index, isArray) {
+ value = value.valueOf();
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ return index;
+}
+function serializeDouble(buffer, key, value, index, isArray) {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ float_parser_1.writeIEEE754(buffer, value.value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ return index;
+}
+function serializeFunction(buffer, key, value, index, _checkKeys, _depth, isArray) {
+ if (_checkKeys === void 0) { _checkKeys = false; }
+ if (_depth === void 0) { _depth = 0; }
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ var functionString = utils_1.normalizedFunctionString(value);
+ // Write the string
+ var size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+}
+function serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, isArray) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (isArray === void 0) { isArray = false; }
+ if (value.scope && typeof value.scope === 'object') {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_CODE_W_SCOPE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Starting index
+ var startIndex = index;
+ // Serialize the function
+ // Get the function string
+ var functionString = typeof value.code === 'string' ? value.code : value.code.toString();
+ // Index adjustment
+ index = index + 4;
+ // Write string into buffer
+ var codeSize = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = codeSize & 0xff;
+ buffer[index + 1] = (codeSize >> 8) & 0xff;
+ buffer[index + 2] = (codeSize >> 16) & 0xff;
+ buffer[index + 3] = (codeSize >> 24) & 0xff;
+ // Write end 0
+ buffer[index + 4 + codeSize - 1] = 0;
+ // Write the
+ index = index + codeSize + 4;
+ //
+ // Serialize the scope value
+ var endIndex = serializeInto(buffer, value.scope, checkKeys, index, depth + 1, serializeFunctions, ignoreUndefined);
+ index = endIndex - 1;
+ // Writ the total
+ var totalSize = endIndex - startIndex;
+ // Write the total size of the object
+ buffer[startIndex++] = totalSize & 0xff;
+ buffer[startIndex++] = (totalSize >> 8) & 0xff;
+ buffer[startIndex++] = (totalSize >> 16) & 0xff;
+ buffer[startIndex++] = (totalSize >> 24) & 0xff;
+ // Write trailing zero
+ buffer[index++] = 0;
+ }
+ else {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ var functionString = value.code.toString();
+ // Write the string
+ var size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ }
+ return index;
+}
+function serializeBinary(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Extract the buffer
+ var data = value.value(true);
+ // Calculate size
+ var size = value.position;
+ // Add the deprecated 02 type 4 bytes of size to total
+ if (value.sub_type === binary_1.Binary.SUBTYPE_BYTE_ARRAY)
+ size = size + 4;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the subtype to the buffer
+ buffer[index++] = value.sub_type;
+ // If we have binary type 2 the 4 first bytes are the size
+ if (value.sub_type === binary_1.Binary.SUBTYPE_BYTE_ARRAY) {
+ size = size - 4;
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ }
+ // Write the data to the object
+ buffer.set(data, index);
+ // Adjust the index
+ index = index + value.position;
+ return index;
+}
+function serializeSymbol(buffer, key, value, index, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_SYMBOL;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the string
+ var size = buffer.write(value.value, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0x00;
+ return index;
+}
+function serializeDBRef(buffer, key, value, index, depth, serializeFunctions, isArray) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ var numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ var startIndex = index;
+ var output = {
+ $ref: value.collection || value.namespace,
+ $id: value.oid
+ };
+ if (value.db != null) {
+ output.$db = value.db;
+ }
+ output = Object.assign(output, value.fields);
+ var endIndex = serializeInto(buffer, output, false, index, depth + 1, serializeFunctions);
+ // Calculate object size
+ var size = endIndex - startIndex;
+ // Write the size
+ buffer[startIndex++] = size & 0xff;
+ buffer[startIndex++] = (size >> 8) & 0xff;
+ buffer[startIndex++] = (size >> 16) & 0xff;
+ buffer[startIndex++] = (size >> 24) & 0xff;
+ // Set index
+ return endIndex;
+}
+function serializeInto(buffer, object, checkKeys, startingIndex, depth, serializeFunctions, ignoreUndefined, path) {
+ if (checkKeys === void 0) { checkKeys = false; }
+ if (startingIndex === void 0) { startingIndex = 0; }
+ if (depth === void 0) { depth = 0; }
+ if (serializeFunctions === void 0) { serializeFunctions = false; }
+ if (ignoreUndefined === void 0) { ignoreUndefined = true; }
+ if (path === void 0) { path = []; }
+ startingIndex = startingIndex || 0;
+ path = path || [];
+ // Push the object to the path
+ path.push(object);
+ // Start place to serialize into
+ var index = startingIndex + 4;
+ // Special case isArray
+ if (Array.isArray(object)) {
+ // Get object keys
+ for (var i = 0; i < object.length; i++) {
+ var key = '' + i;
+ var value = object[i];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+ if (typeof value === 'string') {
+ index = serializeString(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'number') {
+ index = serializeNumber(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (typeof value === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index, true);
+ }
+ else if (value instanceof Date || utils_1.isDate(value)) {
+ index = serializeDate(buffer, key, value, index, true);
+ }
+ else if (value === undefined) {
+ index = serializeNull(buffer, key, value, index, true);
+ }
+ else if (value === null) {
+ index = serializeNull(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index, true);
+ }
+ else if (utils_1.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index, true);
+ }
+ else if (value instanceof RegExp || utils_1.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true, path);
+ }
+ else if (typeof value === 'object' &&
+ extended_json_1.isBSONType(value) &&
+ value._bsontype === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index, true);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, true);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, true);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions, true);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index, true);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index, true);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ else if (object instanceof map_1.Map || utils_1.isMap(object)) {
+ var iterator = object.entries();
+ var done = false;
+ while (!done) {
+ // Unpack the next entry
+ var entry = iterator.next();
+ done = !!entry.done;
+ // Are we done, then skip and terminate
+ if (done)
+ continue;
+ // Get the entry values
+ var key = entry.value[0];
+ var value = entry.value[1];
+ // Check the type of the value
+ var type = typeof value;
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ }
+ else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ }
+ else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ }
+ else if (type === 'bigint' || utils_1.isBigInt64Array(value) || utils_1.isBigUInt64Array(value)) {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ }
+ else if (value instanceof Date || utils_1.isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ }
+ else if (value === null || (value === undefined && ignoreUndefined === false)) {
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ }
+ else if (utils_1.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ }
+ else if (value instanceof RegExp || utils_1.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ }
+ else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
+ }
+ else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ else {
+ // Did we provide a custom serialization method
+ if (object.toBSON) {
+ if (typeof object.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ object = object.toBSON();
+ if (object != null && typeof object !== 'object')
+ throw new TypeError('toBSON function did not return an object');
+ }
+ // Iterate over all the keys
+ for (var key in object) {
+ var value = object[key];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function')
+ throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+ // Check the type of the value
+ var type = typeof value;
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ }
+ else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ }
+ else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ }
+ else if (type === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ }
+ else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ }
+ else if (value instanceof Date || utils_1.isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ }
+ else if (value === undefined) {
+ if (ignoreUndefined === false)
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value === null) {
+ index = serializeNull(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ }
+ else if (utils_1.isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ }
+ else if (value instanceof RegExp || utils_1.isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ }
+ else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined, false, path);
+ }
+ else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(buffer, key, value, index, checkKeys, depth, serializeFunctions, ignoreUndefined);
+ }
+ else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ }
+ else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ }
+ else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ }
+ else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+ // Remove the path
+ path.pop();
+ // Final padding byte for object
+ buffer[index++] = 0x00;
+ // Final size
+ var size = index - startingIndex;
+ // Write the size of the object
+ buffer[startingIndex++] = size & 0xff;
+ buffer[startingIndex++] = (size >> 8) & 0xff;
+ buffer[startingIndex++] = (size >> 16) & 0xff;
+ buffer[startingIndex++] = (size >> 24) & 0xff;
+ return index;
+}
+exports.serializeInto = serializeInto;
+//# sourceMappingURL=serializer.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/parser/serializer.js.map b/node_modules/bson/lib/parser/serializer.js.map
new file mode 100644
index 0000000..bac9b2e
--- /dev/null
+++ b/node_modules/bson/lib/parser/serializer.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"serializer.js","sourceRoot":"","sources":["../../src/parser/serializer.ts"],"names":[],"mappings":";;;AACA,oCAAmC;AAGnC,wCAA0C;AAI1C,kDAAgD;AAChD,kDAA8C;AAC9C,gDAA+C;AAE/C,gCAA+B;AAC/B,8BAA6B;AAI7B,iCAQiB;AAgBjB,IAAM,MAAM,GAAG,MAAM,CAAC,CAAC,uCAAuC;AAC9D,IAAM,UAAU,GAAG,IAAI,GAAG,CAAC,CAAC,KAAK,EAAE,MAAM,EAAE,KAAK,EAAE,cAAc,CAAC,CAAC,CAAC;AAEnE;;;;GAIG;AAEH,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;IAEjB,qBAAqB;IACrB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;IAC7C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,GAAG,CAAC,CAAC;IACzC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;IACtB,mBAAmB;IACnB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;IAC/D,yCAAyC;IACzC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IAC9C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,CAAC,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IAC9C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC,IAAI,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IAC7C,MAAM,CAAC,KAAK,CAAC,GAAG,CAAC,IAAI,GAAG,CAAC,CAAC,GAAG,IAAI,CAAC;IAClC,eAAe;IACf,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,CAAC;IACzB,aAAa;IACb,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;IAEjB,2BAA2B;IAC3B,2CAA2C;IAC3C,IACE,MAAM,CAAC,SAAS,CAAC,KAAK,CAAC;QACvB,KAAK,IAAI,SAAS,CAAC,cAAc;QACjC,KAAK,IAAI,SAAS,CAAC,cAAc,EACjC;QACA,+CAA+C;QAC/C,+BAA+B;QAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;QAC1C,0BAA0B;QAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;YACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;YAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;QACjD,kBAAkB;QAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;QACpB,sBAAsB;QACtB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC;QAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;QACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;KACxC;SAAM;QACL,mBAAmB;QACnB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;QAC7C,0BAA0B;QAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;YACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;YAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;QACjD,kBAAkB;QAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;QACpB,cAAc;QACd,2BAAY,CAAC,MAAM,EAAE,KAAK,EAAE,KAAK,EAAE,QAAQ,EAAE,EAAE,EAAE,CAAC,CAAC,CAAC;QACpD,eAAe;QACf,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;KACnB;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,CAAU,EAAE,KAAa,EAAE,OAAiB;IAC9F,gBAAgB;IAChB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;IAE3C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IAEjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,gBAAgB,CACvB,MAAc,EACd,GAAW,EACX,KAAc,EACd,KAAa,EACb,OAAiB;IAEjB,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;IAC9C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,2BAA2B;IAC3B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;IAChC,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,KAAW,EAAE,KAAa,EAAE,OAAiB;IAC/F,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;IAC3C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IAEpB,iBAAiB;IACjB,IAAM,WAAW,GAAG,WAAI,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,EAAE,CAAC,CAAC;IACrD,IAAM,OAAO,GAAG,WAAW,CAAC,UAAU,EAAE,CAAC;IACzC,IAAM,QAAQ,GAAG,WAAW,CAAC,WAAW,EAAE,CAAC;IAC3C,kBAAkB;IAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,OAAO,GAAG,IAAI,CAAC;IACjC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACzC,mBAAmB;IACnB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;IAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IAC1C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IAC1C,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;IAEjB,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;IAC7C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IAEjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,IAAI,KAAK,CAAC,MAAM,IAAI,KAAK,CAAC,MAAM,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;QACtD,MAAM,KAAK,CAAC,QAAQ,GAAG,KAAK,CAAC,MAAM,GAAG,8BAA8B,CAAC,CAAC;KACvE;IACD,mBAAmB;IACnB,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,MAAM,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;IACrE,aAAa;IACb,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,uBAAuB;IACvB,IAAI,KAAK,CAAC,UAAU;QAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC,CAAC,IAAI;IAClD,IAAI,KAAK,CAAC,MAAM;QAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC,CAAC,IAAI;IAC9C,IAAI,KAAK,CAAC,SAAS;QAAE,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC,CAAC,IAAI;IAEjD,kBAAkB;IAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,mBAAmB,CAC1B,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;IAEjB,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;IAC7C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IAEpB,gCAAgC;IAChC,IAAI,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;QACvC,oEAAoE;QACpE,mBAAmB;QACnB,MAAM,KAAK,CAAC,UAAU,GAAG,KAAK,CAAC,OAAO,GAAG,8BAA8B,CAAC,CAAC;KAC1E;IAED,mBAAmB;IACnB,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,OAAO,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;IACtE,aAAa;IACb,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,oBAAoB;IACpB,KAAK,GAAG,KAAK,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC,CAAC;IAChG,kBAAkB;IAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAsB,EACtB,KAAa,EACb,OAAiB;IAEjB,0CAA0C;IAC1C,IAAI,KAAK,KAAK,IAAI,EAAE;QAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;KAC5C;SAAM,IAAI,KAAK,CAAC,SAAS,KAAK,QAAQ,EAAE;QACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;KAC/C;SAAM;QACL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,iBAAiB,CAAC;KAC/C;IAED,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,iBAAiB,CACxB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,OAAiB;IAEjB,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;IAC1C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IAEjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IAEpB,4CAA4C;IAC5C,IAAI,OAAO,KAAK,CAAC,EAAE,KAAK,QAAQ,EAAE;QAChC,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,EAAE,EAAE,KAAK,EAAE,SAAS,EAAE,QAAQ,CAAC,CAAC;KACpD;SAAM,IAAI,oBAAY,CAAC,KAAK,CAAC,EAAE,CAAC,EAAE;QACjC,2EAA2E;QAC3E,mBAAmB;QACnB,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,QAAQ,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE,KAAK,CAAC,CAAC;KAC7C;SAAM;QACL,MAAM,IAAI,SAAS,CAAC,UAAU,GAAG,IAAI,CAAC,SAAS,CAAC,KAAK,CAAC,GAAG,2BAA2B,CAAC,CAAC;KACvF;IAED,eAAe;IACf,OAAO,KAAK,GAAG,EAAE,CAAC;AACpB,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAA0B,EAC1B,KAAa,EACb,OAAiB;IAEjB,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;IAC7C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,+CAA+C;IAC/C,IAAM,IAAI,GAAG,KAAK,CAAC,MAAM,CAAC;IAC1B,yCAAyC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACtC,4BAA4B;IAC5B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,2BAA2B,CAAC;IACxD,uDAAuD;IACvD,MAAM,CAAC,GAAG,CAAC,4BAAY,CAAC,KAAK,CAAC,EAAE,KAAK,CAAC,CAAC;IACvC,mBAAmB;IACnB,KAAK,GAAG,KAAK,GAAG,IAAI,CAAC;IACrB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,SAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,OAAe,EACf,IAAqB;IALrB,0BAAA,EAAA,iBAAiB;IACjB,sBAAA,EAAA,SAAS;IACT,mCAAA,EAAA,0BAA0B;IAC1B,gCAAA,EAAA,sBAAsB;IACtB,wBAAA,EAAA,eAAe;IACf,qBAAA,EAAA,SAAqB;IAErB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;QACpC,IAAI,IAAI,CAAC,CAAC,CAAC,KAAK,KAAK;YAAE,MAAM,IAAI,KAAK,CAAC,4BAA4B,CAAC,CAAC;KACtE;IAED,sBAAsB;IACtB,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;IACjB,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,OAAO,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,SAAS,CAAC,eAAe,CAAC,CAAC,CAAC,SAAS,CAAC,gBAAgB,CAAC;IAChG,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,IAAM,QAAQ,GAAG,aAAa,CAC5B,MAAM,EACN,KAAK,EACL,SAAS,EACT,KAAK,EACL,KAAK,GAAG,CAAC,EACT,kBAAkB,EAClB,eAAe,EACf,IAAI,CACL,CAAC;IACF,YAAY;IACZ,IAAI,CAAC,GAAG,EAAE,CAAC;IACX,OAAO,QAAQ,CAAC;AAClB,CAAC;AAED,SAAS,mBAAmB,CAC1B,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;IAEjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,oBAAoB,CAAC;IACjD,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,gCAAgC;IAChC,0EAA0E;IAC1E,kCAAkC;IAClC,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,KAAK,CAAC,QAAQ,CAAC,CAAC,EAAE,EAAE,CAAC,EAAE,KAAK,CAAC,CAAC;IAC/C,OAAO,KAAK,GAAG,EAAE,CAAC;AACpB,CAAC;AAED,SAAS,aAAa,CAAC,MAAc,EAAE,GAAW,EAAE,KAAW,EAAE,KAAa,EAAE,OAAiB;IAC/F,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC;QACb,KAAK,CAAC,SAAS,KAAK,MAAM,CAAC,CAAC,CAAC,SAAS,CAAC,cAAc,CAAC,CAAC,CAAC,SAAS,CAAC,mBAAmB,CAAC;IACxF,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,iBAAiB;IACjB,IAAM,OAAO,GAAG,KAAK,CAAC,UAAU,EAAE,CAAC;IACnC,IAAM,QAAQ,GAAG,KAAK,CAAC,WAAW,EAAE,CAAC;IACrC,kBAAkB;IAClB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,OAAO,GAAG,IAAI,CAAC;IACjC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,OAAO,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACzC,mBAAmB;IACnB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;IAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IAC1C,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IAC1C,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,cAAc,CACrB,MAAc,EACd,GAAW,EACX,KAAqB,EACrB,KAAa,EACb,OAAiB;IAEjB,KAAK,GAAG,KAAK,CAAC,OAAO,EAAE,CAAC;IACxB,+BAA+B;IAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,aAAa,CAAC;IAC1C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,sBAAsB;IACtB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,GAAG,IAAI,CAAC;IAC/B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACvC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,KAAK,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACvC,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;IAEjB,mBAAmB;IACnB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;IAE7C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IAEjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IAEpB,cAAc;IACd,2BAAY,CAAC,MAAM,EAAE,KAAK,CAAC,KAAK,EAAE,KAAK,EAAE,QAAQ,EAAE,EAAE,EAAE,CAAC,CAAC,CAAC;IAE1D,eAAe;IACf,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;IAClB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,iBAAiB,CACxB,MAAc,EACd,GAAW,EACX,KAAe,EACf,KAAa,EACb,UAAkB,EAClB,MAAU,EACV,OAAiB;IAFjB,2BAAA,EAAA,kBAAkB;IAClB,uBAAA,EAAA,UAAU;IAGV,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;IAC3C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,kBAAkB;IAClB,IAAM,cAAc,GAAG,gCAAwB,CAAC,KAAK,CAAC,CAAC;IAEvD,mBAAmB;IACnB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;IAC5E,yCAAyC;IACzC,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACxC,eAAe;IACf,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;IAC7B,aAAa;IACb,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,aAAa,CACpB,MAAc,EACd,GAAW,EACX,KAAW,EACX,KAAa,EACb,SAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,OAAe;IAJf,0BAAA,EAAA,iBAAiB;IACjB,sBAAA,EAAA,SAAS;IACT,mCAAA,EAAA,0BAA0B;IAC1B,gCAAA,EAAA,sBAAsB;IACtB,wBAAA,EAAA,eAAe;IAEf,IAAI,KAAK,CAAC,KAAK,IAAI,OAAO,KAAK,CAAC,KAAK,KAAK,QAAQ,EAAE;QAClD,iBAAiB;QACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,sBAAsB,CAAC;QACnD,0BAA0B;QAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;YACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;YAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;QACjD,kBAAkB;QAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;QAEpB,iBAAiB;QACjB,IAAI,UAAU,GAAG,KAAK,CAAC;QAEvB,yBAAyB;QACzB,0BAA0B;QAC1B,IAAM,cAAc,GAAG,OAAO,KAAK,CAAC,IAAI,KAAK,QAAQ,CAAC,CAAC,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC;QAC3F,mBAAmB;QACnB,KAAK,GAAG,KAAK,GAAG,CAAC,CAAC;QAClB,2BAA2B;QAC3B,IAAM,QAAQ,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;QAChF,yCAAyC;QACzC,MAAM,CAAC,KAAK,CAAC,GAAG,QAAQ,GAAG,IAAI,CAAC;QAChC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;QAC3C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAC5C,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,QAAQ,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAC5C,cAAc;QACd,MAAM,CAAC,KAAK,GAAG,CAAC,GAAG,QAAQ,GAAG,CAAC,CAAC,GAAG,CAAC,CAAC;QACrC,YAAY;QACZ,KAAK,GAAG,KAAK,GAAG,QAAQ,GAAG,CAAC,CAAC;QAE7B,EAAE;QACF,4BAA4B;QAC5B,IAAM,QAAQ,GAAG,aAAa,CAC5B,MAAM,EACN,KAAK,CAAC,KAAK,EACX,SAAS,EACT,KAAK,EACL,KAAK,GAAG,CAAC,EACT,kBAAkB,EAClB,eAAe,CAChB,CAAC;QACF,KAAK,GAAG,QAAQ,GAAG,CAAC,CAAC;QAErB,iBAAiB;QACjB,IAAM,SAAS,GAAG,QAAQ,GAAG,UAAU,CAAC;QAExC,qCAAqC;QACrC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,SAAS,GAAG,IAAI,CAAC;QACxC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;QAC/C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAChD,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,SAAS,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QAChD,sBAAsB;QACtB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACrB;SAAM;QACL,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,cAAc,CAAC;QAC3C,0BAA0B;QAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;YACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;YAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;QACjD,kBAAkB;QAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;QACpB,kBAAkB;QAClB,IAAM,cAAc,GAAG,KAAK,CAAC,IAAI,CAAC,QAAQ,EAAE,CAAC;QAC7C,mBAAmB;QACnB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,cAAc,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;QAC5E,yCAAyC;QACzC,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;QAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;QACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QACxC,eAAe;QACf,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;QAC7B,aAAa;QACb,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;KACrB;IAED,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAa,EACb,KAAa,EACb,OAAiB;IAEjB,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;IAC7C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,qBAAqB;IACrB,IAAM,IAAI,GAAG,KAAK,CAAC,KAAK,CAAC,IAAI,CAAwB,CAAC;IACtD,iBAAiB;IACjB,IAAI,IAAI,GAAG,KAAK,CAAC,QAAQ,CAAC;IAC1B,sDAAsD;IACtD,IAAI,KAAK,CAAC,QAAQ,KAAK,eAAM,CAAC,kBAAkB;QAAE,IAAI,GAAG,IAAI,GAAG,CAAC,CAAC;IAClE,yCAAyC;IACzC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACtC,kCAAkC;IAClC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,KAAK,CAAC,QAAQ,CAAC;IAEjC,0DAA0D;IAC1D,IAAI,KAAK,CAAC,QAAQ,KAAK,eAAM,CAAC,kBAAkB,EAAE;QAChD,IAAI,GAAG,IAAI,GAAG,CAAC,CAAC;QAChB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;QAC9B,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;QACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;QACtC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;KACvC;IAED,+BAA+B;IAC/B,MAAM,CAAC,GAAG,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;IACxB,mBAAmB;IACnB,KAAK,GAAG,KAAK,GAAG,KAAK,CAAC,QAAQ,CAAC;IAC/B,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,eAAe,CACtB,MAAc,EACd,GAAW,EACX,KAAiB,EACjB,KAAa,EACb,OAAiB;IAEjB,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;IAC7C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IACjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IACpB,mBAAmB;IACnB,IAAM,IAAI,GAAG,MAAM,CAAC,KAAK,CAAC,KAAK,CAAC,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,SAAS,EAAE,MAAM,CAAC,GAAG,CAAC,CAAC;IACzE,yCAAyC;IACzC,MAAM,CAAC,KAAK,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IAC5B,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IACvC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACxC,MAAM,CAAC,KAAK,GAAG,CAAC,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IACxC,eAAe;IACf,KAAK,GAAG,KAAK,GAAG,CAAC,GAAG,IAAI,GAAG,CAAC,CAAC;IAC7B,aAAa;IACb,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IACvB,OAAO,KAAK,CAAC;AACf,CAAC;AAED,SAAS,cAAc,CACrB,MAAc,EACd,GAAW,EACX,KAAY,EACZ,KAAa,EACb,KAAa,EACb,kBAA2B,EAC3B,OAAiB;IAEjB,iBAAiB;IACjB,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,SAAS,CAAC,gBAAgB,CAAC;IAC7C,0BAA0B;IAC1B,IAAM,oBAAoB,GAAG,CAAC,OAAO;QACnC,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,MAAM,CAAC;QAC7C,CAAC,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,EAAE,KAAK,EAAE,SAAS,EAAE,OAAO,CAAC,CAAC;IAEjD,kBAAkB;IAClB,KAAK,GAAG,KAAK,GAAG,oBAAoB,CAAC;IACrC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,CAAC,CAAC;IAEpB,IAAI,UAAU,GAAG,KAAK,CAAC;IACvB,IAAI,MAAM,GAAc;QACtB,IAAI,EAAE,KAAK,CAAC,UAAU,IAAI,KAAK,CAAC,SAAS;QACzC,GAAG,EAAE,KAAK,CAAC,GAAG;KACf,CAAC;IAEF,IAAI,KAAK,CAAC,EAAE,IAAI,IAAI,EAAE;QACpB,MAAM,CAAC,GAAG,GAAG,KAAK,CAAC,EAAE,CAAC;KACvB;IAED,MAAM,GAAG,MAAM,CAAC,MAAM,CAAC,MAAM,EAAE,KAAK,CAAC,MAAM,CAAC,CAAC;IAC7C,IAAM,QAAQ,GAAG,aAAa,CAAC,MAAM,EAAE,MAAM,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,GAAG,CAAC,EAAE,kBAAkB,CAAC,CAAC;IAE5F,wBAAwB;IACxB,IAAM,IAAI,GAAG,QAAQ,GAAG,UAAU,CAAC;IACnC,iBAAiB;IACjB,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IACnC,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IAC1C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IAC3C,MAAM,CAAC,UAAU,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IAC3C,YAAY;IACZ,OAAO,QAAQ,CAAC;AAClB,CAAC;AAED,SAAgB,aAAa,CAC3B,MAAc,EACd,MAAgB,EAChB,SAAiB,EACjB,aAAiB,EACjB,KAAS,EACT,kBAA0B,EAC1B,eAAsB,EACtB,IAAqB;IALrB,0BAAA,EAAA,iBAAiB;IACjB,8BAAA,EAAA,iBAAiB;IACjB,sBAAA,EAAA,SAAS;IACT,mCAAA,EAAA,0BAA0B;IAC1B,gCAAA,EAAA,sBAAsB;IACtB,qBAAA,EAAA,SAAqB;IAErB,aAAa,GAAG,aAAa,IAAI,CAAC,CAAC;IACnC,IAAI,GAAG,IAAI,IAAI,EAAE,CAAC;IAElB,8BAA8B;IAC9B,IAAI,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;IAElB,gCAAgC;IAChC,IAAI,KAAK,GAAG,aAAa,GAAG,CAAC,CAAC;IAE9B,uBAAuB;IACvB,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;QACzB,kBAAkB;QAClB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;YACtC,IAAM,GAAG,GAAG,EAAE,GAAG,CAAC,CAAC;YACnB,IAAI,KAAK,GAAG,MAAM,CAAC,CAAC,CAAC,CAAC;YAEtB,6BAA6B;YAC7B,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;gBACzB,IAAI,OAAO,KAAK,CAAC,MAAM,KAAK,UAAU;oBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;gBACxF,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;aACxB;YAED,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;gBAC7B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;gBACpC,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;gBACpC,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;aACvE;iBAAM,IAAI,OAAO,KAAK,KAAK,SAAS,EAAE;gBACrC,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC3D;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAI,cAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,KAAK,SAAS,EAAE;gBAC9B,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,KAAK,IAAI,EAAE;gBACzB,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC5D;iBAAM,IAAI,oBAAY,CAAC,KAAK,CAAC,EAAE;gBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAI,gBAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;gBAClE,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,IAAI,EACJ,IAAI,CACL,CAAC;aACH;iBAAM,IACL,OAAO,KAAK,KAAK,QAAQ;gBACzB,0BAAU,CAAC,KAAK,CAAC;gBACjB,KAAK,CAAC,SAAS,KAAK,YAAY,EAChC;gBACA,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC9D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;gBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC9E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;gBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,IAAI,CACL,CAAC;aACH;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,EAAE,IAAI,CAAC,CAAC;aACpF;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC9D;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aACzD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,IAAI,CAAC,CAAC;aAC1D;iBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;aACjF;SACF;KACF;SAAM,IAAI,MAAM,YAAY,SAAG,IAAI,aAAK,CAAC,MAAM,CAAC,EAAE;QACjD,IAAM,QAAQ,GAAG,MAAM,CAAC,OAAO,EAAE,CAAC;QAClC,IAAI,IAAI,GAAG,KAAK,CAAC;QAEjB,OAAO,CAAC,IAAI,EAAE;YACZ,wBAAwB;YACxB,IAAM,KAAK,GAAG,QAAQ,CAAC,IAAI,EAAE,CAAC;YAC9B,IAAI,GAAG,CAAC,CAAC,KAAK,CAAC,IAAI,CAAC;YACpB,uCAAuC;YACvC,IAAI,IAAI;gBAAE,SAAS;YAEnB,uBAAuB;YACvB,IAAM,GAAG,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;YAC3B,IAAM,KAAK,GAAG,KAAK,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC;YAE7B,8BAA8B;YAC9B,IAAM,IAAI,GAAG,OAAO,KAAK,CAAC;YAE1B,gDAAgD;YAChD,IAAI,OAAO,GAAG,KAAK,QAAQ,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;gBACnD,IAAI,GAAG,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;oBAC7B,oEAAoE;oBACpE,mBAAmB;oBACnB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,8BAA8B,CAAC,CAAC;iBAC5D;gBAED,IAAI,SAAS,EAAE;oBACb,IAAI,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,EAAE;wBAClB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,0BAA0B,CAAC,CAAC;qBACxD;yBAAM,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;wBAC5B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,uBAAuB,CAAC,CAAC;qBACrD;iBACF;aACF;YAED,IAAI,IAAI,KAAK,QAAQ,EAAE;gBACrB,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;gBAC5B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,uBAAe,CAAC,KAAK,CAAC,IAAI,wBAAgB,CAAC,KAAK,CAAC,EAAE;gBACjF,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;aACvE;iBAAM,IAAI,IAAI,KAAK,SAAS,EAAE;gBAC7B,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACrD;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAI,cAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,KAAK,IAAI,IAAI,CAAC,KAAK,KAAK,SAAS,IAAI,eAAe,KAAK,KAAK,CAAC,EAAE;gBAC/E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACtD;iBAAM,IAAI,oBAAY,CAAC,KAAK,CAAC,EAAE;gBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAI,gBAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;gBAC1D,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,KAAK,EACL,IAAI,CACL,CAAC;aACH;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBACnE,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;gBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,CAChB,CAAC;aACH;iBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;gBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC5F;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC9E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACnD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;aACjF;SACF;KACF;SAAM;QACL,+CAA+C;QAC/C,IAAI,MAAM,CAAC,MAAM,EAAE;YACjB,IAAI,OAAO,MAAM,CAAC,MAAM,KAAK,UAAU;gBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;YACzF,MAAM,GAAG,MAAM,CAAC,MAAM,EAAE,CAAC;YACzB,IAAI,MAAM,IAAI,IAAI,IAAI,OAAO,MAAM,KAAK,QAAQ;gBAC9C,MAAM,IAAI,SAAS,CAAC,0CAA0C,CAAC,CAAC;SACnE;QAED,4BAA4B;QAC5B,KAAK,IAAM,GAAG,IAAI,MAAM,EAAE;YACxB,IAAI,KAAK,GAAG,MAAM,CAAC,GAAG,CAAC,CAAC;YACxB,6BAA6B;YAC7B,IAAI,KAAK,IAAI,KAAK,CAAC,MAAM,EAAE;gBACzB,IAAI,OAAO,KAAK,CAAC,MAAM,KAAK,UAAU;oBAAE,MAAM,IAAI,SAAS,CAAC,0BAA0B,CAAC,CAAC;gBACxF,KAAK,GAAG,KAAK,CAAC,MAAM,EAAE,CAAC;aACxB;YAED,8BAA8B;YAC9B,IAAM,IAAI,GAAG,OAAO,KAAK,CAAC;YAE1B,gDAAgD;YAChD,IAAI,OAAO,GAAG,KAAK,QAAQ,IAAI,CAAC,UAAU,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;gBACnD,IAAI,GAAG,CAAC,KAAK,CAAC,MAAM,CAAC,IAAI,IAAI,EAAE;oBAC7B,oEAAoE;oBACpE,mBAAmB;oBACnB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,8BAA8B,CAAC,CAAC;iBAC5D;gBAED,IAAI,SAAS,EAAE;oBACb,IAAI,GAAG,KAAK,GAAG,CAAC,CAAC,CAAC,EAAE;wBAClB,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,0BAA0B,CAAC,CAAC;qBACxD;yBAAM,IAAI,CAAC,GAAG,CAAC,OAAO,CAAC,GAAG,CAAC,EAAE;wBAC5B,MAAM,KAAK,CAAC,MAAM,GAAG,GAAG,GAAG,uBAAuB,CAAC,CAAC;qBACrD;iBACF;aACF;YAED,IAAI,IAAI,KAAK,QAAQ,EAAE;gBACrB,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;gBAC5B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,EAAE;gBAC5B,MAAM,IAAI,SAAS,CAAC,gDAAgD,CAAC,CAAC;aACvE;iBAAM,IAAI,IAAI,KAAK,SAAS,EAAE;gBAC7B,KAAK,GAAG,gBAAgB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACrD;iBAAM,IAAI,KAAK,YAAY,IAAI,IAAI,cAAM,CAAC,KAAK,CAAC,EAAE;gBACjD,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,KAAK,SAAS,EAAE;gBAC9B,IAAI,eAAe,KAAK,KAAK;oBAAE,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACjF;iBAAM,IAAI,KAAK,KAAK,IAAI,EAAE;gBACzB,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,UAAU,EAAE;gBACjF,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACtD;iBAAM,IAAI,oBAAY,CAAC,KAAK,CAAC,EAAE;gBAC9B,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,YAAY,MAAM,IAAI,gBAAQ,CAAC,KAAK,CAAC,EAAE;gBACrD,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,IAAI,IAAI,EAAE;gBAC1D,KAAK,GAAG,eAAe,CACrB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,EACf,KAAK,EACL,IAAI,CACL,CAAC;aACH;iBAAM,IAAI,IAAI,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBACnE,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBAC9E,KAAK,GAAG,aAAa,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aAClD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,MAAM,EAAE;gBACxC,KAAK,GAAG,aAAa,CACnB,MAAM,EACN,GAAG,EACH,KAAK,EACL,KAAK,EACL,SAAS,EACT,KAAK,EACL,kBAAkB,EAClB,eAAe,CAChB,CAAC;aACH;iBAAM,IAAI,OAAO,KAAK,KAAK,UAAU,IAAI,kBAAkB,EAAE;gBAC5D,KAAK,GAAG,iBAAiB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,SAAS,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC5F;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC1C,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,EAAE,KAAK,EAAE,kBAAkB,CAAC,CAAC;aAC9E;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,YAAY,EAAE;gBAC9C,KAAK,GAAG,mBAAmB,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACxD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,OAAO,EAAE;gBACzC,KAAK,GAAG,cAAc,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACnD;iBAAM,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,IAAI,KAAK,CAAC,WAAW,CAAC,KAAK,QAAQ,EAAE;gBAC7E,KAAK,GAAG,eAAe,CAAC,MAAM,EAAE,GAAG,EAAE,KAAK,EAAE,KAAK,CAAC,CAAC;aACpD;iBAAM,IAAI,OAAO,KAAK,CAAC,WAAW,CAAC,KAAK,WAAW,EAAE;gBACpD,MAAM,IAAI,SAAS,CAAC,qCAAqC,GAAG,KAAK,CAAC,WAAW,CAAC,CAAC,CAAC;aACjF;SACF;KACF;IAED,kBAAkB;IAClB,IAAI,CAAC,GAAG,EAAE,CAAC;IAEX,gCAAgC;IAChC,MAAM,CAAC,KAAK,EAAE,CAAC,GAAG,IAAI,CAAC;IAEvB,aAAa;IACb,IAAM,IAAI,GAAG,KAAK,GAAG,aAAa,CAAC;IACnC,+BAA+B;IAC/B,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,IAAI,GAAG,IAAI,CAAC;IACtC,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,CAAC,CAAC,GAAG,IAAI,CAAC;IAC7C,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IAC9C,MAAM,CAAC,aAAa,EAAE,CAAC,GAAG,CAAC,IAAI,IAAI,EAAE,CAAC,GAAG,IAAI,CAAC;IAC9C,OAAO,KAAK,CAAC;AACf,CAAC;AAxUD,sCAwUC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/parser/utils.js b/node_modules/bson/lib/parser/utils.js
new file mode 100644
index 0000000..ea9c855
--- /dev/null
+++ b/node_modules/bson/lib/parser/utils.js
@@ -0,0 +1,111 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.deprecate = exports.isObjectLike = exports.isDate = exports.haveBuffer = exports.isMap = exports.isRegExp = exports.isBigUInt64Array = exports.isBigInt64Array = exports.isUint8Array = exports.isAnyArrayBuffer = exports.randomBytes = exports.normalizedFunctionString = void 0;
+var buffer_1 = require("buffer");
+/**
+ * Normalizes our expected stringified form of a function across versions of node
+ * @param fn - The function to stringify
+ */
+function normalizedFunctionString(fn) {
+ return fn.toString().replace('function(', 'function (');
+}
+exports.normalizedFunctionString = normalizedFunctionString;
+var isReactNative = typeof global.navigator === 'object' && global.navigator.product === 'ReactNative';
+var insecureWarning = isReactNative
+ ? 'BSON: For React Native please polyfill crypto.getRandomValues, e.g. using: https://www.npmjs.com/package/react-native-get-random-values.'
+ : 'BSON: No cryptographic implementation for random bytes present, falling back to a less secure implementation.';
+var insecureRandomBytes = function insecureRandomBytes(size) {
+ console.warn(insecureWarning);
+ var result = buffer_1.Buffer.alloc(size);
+ for (var i = 0; i < size; ++i)
+ result[i] = Math.floor(Math.random() * 256);
+ return result;
+};
+var detectRandomBytes = function () {
+ if (typeof window !== 'undefined') {
+ // browser crypto implementation(s)
+ var target_1 = window.crypto || window.msCrypto; // allow for IE11
+ if (target_1 && target_1.getRandomValues) {
+ return function (size) { return target_1.getRandomValues(buffer_1.Buffer.alloc(size)); };
+ }
+ }
+ if (typeof global !== 'undefined' && global.crypto && global.crypto.getRandomValues) {
+ // allow for RN packages such as https://www.npmjs.com/package/react-native-get-random-values to populate global
+ return function (size) { return global.crypto.getRandomValues(buffer_1.Buffer.alloc(size)); };
+ }
+ var requiredRandomBytes;
+ try {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ requiredRandomBytes = require('crypto').randomBytes;
+ }
+ catch (e) {
+ // keep the fallback
+ }
+ // NOTE: in transpiled cases the above require might return null/undefined
+ return requiredRandomBytes || insecureRandomBytes;
+};
+exports.randomBytes = detectRandomBytes();
+function isAnyArrayBuffer(value) {
+ return ['[object ArrayBuffer]', '[object SharedArrayBuffer]'].includes(Object.prototype.toString.call(value));
+}
+exports.isAnyArrayBuffer = isAnyArrayBuffer;
+function isUint8Array(value) {
+ return Object.prototype.toString.call(value) === '[object Uint8Array]';
+}
+exports.isUint8Array = isUint8Array;
+function isBigInt64Array(value) {
+ return Object.prototype.toString.call(value) === '[object BigInt64Array]';
+}
+exports.isBigInt64Array = isBigInt64Array;
+function isBigUInt64Array(value) {
+ return Object.prototype.toString.call(value) === '[object BigUint64Array]';
+}
+exports.isBigUInt64Array = isBigUInt64Array;
+function isRegExp(d) {
+ return Object.prototype.toString.call(d) === '[object RegExp]';
+}
+exports.isRegExp = isRegExp;
+function isMap(d) {
+ return Object.prototype.toString.call(d) === '[object Map]';
+}
+exports.isMap = isMap;
+/** Call to check if your environment has `Buffer` */
+function haveBuffer() {
+ return typeof global !== 'undefined' && typeof global.Buffer !== 'undefined';
+}
+exports.haveBuffer = haveBuffer;
+// To ensure that 0.4 of node works correctly
+function isDate(d) {
+ return isObjectLike(d) && Object.prototype.toString.call(d) === '[object Date]';
+}
+exports.isDate = isDate;
+/**
+ * @internal
+ * this is to solve the `'someKey' in x` problem where x is unknown.
+ * https://github.com/typescript-eslint/typescript-eslint/issues/1071#issuecomment-541955753
+ */
+function isObjectLike(candidate) {
+ return typeof candidate === 'object' && candidate !== null;
+}
+exports.isObjectLike = isObjectLike;
+function deprecate(fn, message) {
+ if (typeof window === 'undefined' && typeof self === 'undefined') {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ return require('util').deprecate(fn, message);
+ }
+ var warned = false;
+ function deprecated() {
+ var args = [];
+ for (var _i = 0; _i < arguments.length; _i++) {
+ args[_i] = arguments[_i];
+ }
+ if (!warned) {
+ console.warn(message);
+ warned = true;
+ }
+ return fn.apply(this, args);
+ }
+ return deprecated;
+}
+exports.deprecate = deprecate;
+//# sourceMappingURL=utils.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/parser/utils.js.map b/node_modules/bson/lib/parser/utils.js.map
new file mode 100644
index 0000000..b0baef2
--- /dev/null
+++ b/node_modules/bson/lib/parser/utils.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"utils.js","sourceRoot":"","sources":["../../src/parser/utils.ts"],"names":[],"mappings":";;;AAAA,iCAAgC;AAIhC;;;GAGG;AACH,SAAgB,wBAAwB,CAAC,EAAY;IACnD,OAAO,EAAE,CAAC,QAAQ,EAAE,CAAC,OAAO,CAAC,WAAW,EAAE,YAAY,CAAC,CAAC;AAC1D,CAAC;AAFD,4DAEC;AAED,IAAM,aAAa,GACjB,OAAO,MAAM,CAAC,SAAS,KAAK,QAAQ,IAAI,MAAM,CAAC,SAAS,CAAC,OAAO,KAAK,aAAa,CAAC;AAErF,IAAM,eAAe,GAAG,aAAa;IACnC,CAAC,CAAC,0IAA0I;IAC5I,CAAC,CAAC,+GAA+G,CAAC;AAEpH,IAAM,mBAAmB,GAAwB,SAAS,mBAAmB,CAAC,IAAY;IACxF,OAAO,CAAC,IAAI,CAAC,eAAe,CAAC,CAAC;IAE9B,IAAM,MAAM,GAAG,eAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;IAClC,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,EAAE,EAAE,CAAC;QAAE,MAAM,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,KAAK,CAAC,IAAI,CAAC,MAAM,EAAE,GAAG,GAAG,CAAC,CAAC;IAC3E,OAAO,MAAM,CAAC;AAChB,CAAC,CAAC;AAUF,IAAM,iBAAiB,GAAG;IACxB,IAAI,OAAO,MAAM,KAAK,WAAW,EAAE;QACjC,mCAAmC;QACnC,IAAM,QAAM,GAAG,MAAM,CAAC,MAAM,IAAI,MAAM,CAAC,QAAQ,CAAC,CAAC,iBAAiB;QAClE,IAAI,QAAM,IAAI,QAAM,CAAC,eAAe,EAAE;YACpC,OAAO,UAAA,IAAI,IAAI,OAAA,QAAM,CAAC,eAAe,CAAC,eAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,EAA1C,CAA0C,CAAC;SAC3D;KACF;IAED,IAAI,OAAO,MAAM,KAAK,WAAW,IAAI,MAAM,CAAC,MAAM,IAAI,MAAM,CAAC,MAAM,CAAC,eAAe,EAAE;QACnF,gHAAgH;QAChH,OAAO,UAAA,IAAI,IAAI,OAAA,MAAM,CAAC,MAAM,CAAC,eAAe,CAAC,eAAM,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,EAAjD,CAAiD,CAAC;KAClE;IAED,IAAI,mBAA2D,CAAC;IAChE,IAAI;QACF,8DAA8D;QAC9D,mBAAmB,GAAG,OAAO,CAAC,QAAQ,CAAC,CAAC,WAAW,CAAC;KACrD;IAAC,OAAO,CAAC,EAAE;QACV,oBAAoB;KACrB;IAED,0EAA0E;IAE1E,OAAO,mBAAmB,IAAI,mBAAmB,CAAC;AACpD,CAAC,CAAC;AAEW,QAAA,WAAW,GAAG,iBAAiB,EAAE,CAAC;AAE/C,SAAgB,gBAAgB,CAAC,KAAc;IAC7C,OAAO,CAAC,sBAAsB,EAAE,4BAA4B,CAAC,CAAC,QAAQ,CACpE,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CACtC,CAAC;AACJ,CAAC;AAJD,4CAIC;AAED,SAAgB,YAAY,CAAC,KAAc;IACzC,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,qBAAqB,CAAC;AACzE,CAAC;AAFD,oCAEC;AAED,SAAgB,eAAe,CAAC,KAAc;IAC5C,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,wBAAwB,CAAC;AAC5E,CAAC;AAFD,0CAEC;AAED,SAAgB,gBAAgB,CAAC,KAAc;IAC7C,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,KAAK,yBAAyB,CAAC;AAC7E,CAAC;AAFD,4CAEC;AAED,SAAgB,QAAQ,CAAC,CAAU;IACjC,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,iBAAiB,CAAC;AACjE,CAAC;AAFD,4BAEC;AAED,SAAgB,KAAK,CAAC,CAAU;IAC9B,OAAO,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,cAAc,CAAC;AAC9D,CAAC;AAFD,sBAEC;AAED,qDAAqD;AACrD,SAAgB,UAAU;IACxB,OAAO,OAAO,MAAM,KAAK,WAAW,IAAI,OAAO,MAAM,CAAC,MAAM,KAAK,WAAW,CAAC;AAC/E,CAAC;AAFD,gCAEC;AAED,6CAA6C;AAC7C,SAAgB,MAAM,CAAC,CAAU;IAC/B,OAAO,YAAY,CAAC,CAAC,CAAC,IAAI,MAAM,CAAC,SAAS,CAAC,QAAQ,CAAC,IAAI,CAAC,CAAC,CAAC,KAAK,eAAe,CAAC;AAClF,CAAC;AAFD,wBAEC;AAED;;;;GAIG;AACH,SAAgB,YAAY,CAAC,SAAkB;IAC7C,OAAO,OAAO,SAAS,KAAK,QAAQ,IAAI,SAAS,KAAK,IAAI,CAAC;AAC7D,CAAC;AAFD,oCAEC;AAGD,SAAgB,SAAS,CAAqB,EAAK,EAAE,OAAe;IAClE,IAAI,OAAO,MAAM,KAAK,WAAW,IAAI,OAAO,IAAI,KAAK,WAAW,EAAE;QAChE,8DAA8D;QAC9D,OAAO,OAAO,CAAC,MAAM,CAAC,CAAC,SAAS,CAAC,EAAE,EAAE,OAAO,CAAC,CAAC;KAC/C;IACD,IAAI,MAAM,GAAG,KAAK,CAAC;IACnB,SAAS,UAAU;QAAgB,cAAkB;aAAlB,UAAkB,EAAlB,qBAAkB,EAAlB,IAAkB;YAAlB,yBAAkB;;QACnD,IAAI,CAAC,MAAM,EAAE;YACX,OAAO,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC;YACtB,MAAM,GAAG,IAAI,CAAC;SACf;QACD,OAAO,EAAE,CAAC,KAAK,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;IAC9B,CAAC;IACD,OAAQ,UAA2B,CAAC;AACtC,CAAC;AAdD,8BAcC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/regexp.js b/node_modules/bson/lib/regexp.js
new file mode 100644
index 0000000..1a83c89
--- /dev/null
+++ b/node_modules/bson/lib/regexp.js
@@ -0,0 +1,66 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.BSONRegExp = void 0;
+function alphabetize(str) {
+ return str.split('').sort().join('');
+}
+/**
+ * A class representation of the BSON RegExp type.
+ * @public
+ */
+var BSONRegExp = /** @class */ (function () {
+ /**
+ * @param pattern - The regular expression pattern to match
+ * @param options - The regular expression options
+ */
+ function BSONRegExp(pattern, options) {
+ if (!(this instanceof BSONRegExp))
+ return new BSONRegExp(pattern, options);
+ this.pattern = pattern;
+ this.options = alphabetize(options !== null && options !== void 0 ? options : '');
+ // Validate options
+ for (var i = 0; i < this.options.length; i++) {
+ if (!(this.options[i] === 'i' ||
+ this.options[i] === 'm' ||
+ this.options[i] === 'x' ||
+ this.options[i] === 'l' ||
+ this.options[i] === 's' ||
+ this.options[i] === 'u')) {
+ throw new Error("The regular expression option [" + this.options[i] + "] is not supported");
+ }
+ }
+ }
+ BSONRegExp.parseOptions = function (options) {
+ return options ? options.split('').sort().join('') : '';
+ };
+ /** @internal */
+ BSONRegExp.prototype.toExtendedJSON = function (options) {
+ options = options || {};
+ if (options.legacy) {
+ return { $regex: this.pattern, $options: this.options };
+ }
+ return { $regularExpression: { pattern: this.pattern, options: this.options } };
+ };
+ /** @internal */
+ BSONRegExp.fromExtendedJSON = function (doc) {
+ if ('$regex' in doc) {
+ if (typeof doc.$regex !== 'string') {
+ // This is for $regex query operators that have extended json values.
+ if (doc.$regex._bsontype === 'BSONRegExp') {
+ return doc;
+ }
+ }
+ else {
+ return new BSONRegExp(doc.$regex, BSONRegExp.parseOptions(doc.$options));
+ }
+ }
+ if ('$regularExpression' in doc) {
+ return new BSONRegExp(doc.$regularExpression.pattern, BSONRegExp.parseOptions(doc.$regularExpression.options));
+ }
+ throw new TypeError("Unexpected BSONRegExp EJSON object form: " + JSON.stringify(doc));
+ };
+ return BSONRegExp;
+}());
+exports.BSONRegExp = BSONRegExp;
+Object.defineProperty(BSONRegExp.prototype, '_bsontype', { value: 'BSONRegExp' });
+//# sourceMappingURL=regexp.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/regexp.js.map b/node_modules/bson/lib/regexp.js.map
new file mode 100644
index 0000000..1cb2e6c
--- /dev/null
+++ b/node_modules/bson/lib/regexp.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"regexp.js","sourceRoot":"","sources":["../src/regexp.ts"],"names":[],"mappings":";;;AAEA,SAAS,WAAW,CAAC,GAAW;IAC9B,OAAO,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;AACvC,CAAC;AAgBD;;;GAGG;AACH;IAKE;;;OAGG;IACH,oBAAY,OAAe,EAAE,OAAgB;QAC3C,IAAI,CAAC,CAAC,IAAI,YAAY,UAAU,CAAC;YAAE,OAAO,IAAI,UAAU,CAAC,OAAO,EAAE,OAAO,CAAC,CAAC;QAE3E,IAAI,CAAC,OAAO,GAAG,OAAO,CAAC;QACvB,IAAI,CAAC,OAAO,GAAG,WAAW,CAAC,OAAO,aAAP,OAAO,cAAP,OAAO,GAAI,EAAE,CAAC,CAAC;QAE1C,mBAAmB;QACnB,KAAK,IAAI,CAAC,GAAG,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC,OAAO,CAAC,MAAM,EAAE,CAAC,EAAE,EAAE;YAC5C,IACE,CAAC,CACC,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG;gBACvB,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,KAAK,GAAG,CACxB,EACD;gBACA,MAAM,IAAI,KAAK,CAAC,oCAAkC,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC,uBAAoB,CAAC,CAAC;aACxF;SACF;IACH,CAAC;IAEM,uBAAY,GAAnB,UAAoB,OAAgB;QAClC,OAAO,OAAO,CAAC,CAAC,CAAC,OAAO,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,EAAE,CAAC;IAC1D,CAAC;IAED,gBAAgB;IAChB,mCAAc,GAAd,UAAe,OAAsB;QACnC,OAAO,GAAG,OAAO,IAAI,EAAE,CAAC;QACxB,IAAI,OAAO,CAAC,MAAM,EAAE;YAClB,OAAO,EAAE,MAAM,EAAE,IAAI,CAAC,OAAO,EAAE,QAAQ,EAAE,IAAI,CAAC,OAAO,EAAE,CAAC;SACzD;QACD,OAAO,EAAE,kBAAkB,EAAE,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,EAAE,CAAC;IAClF,CAAC;IAED,gBAAgB;IACT,2BAAgB,GAAvB,UAAwB,GAAkD;QACxE,IAAI,QAAQ,IAAI,GAAG,EAAE;YACnB,IAAI,OAAO,GAAG,CAAC,MAAM,KAAK,QAAQ,EAAE;gBAClC,qEAAqE;gBACrE,IAAI,GAAG,CAAC,MAAM,CAAC,SAAS,KAAK,YAAY,EAAE;oBACzC,OAAQ,GAA6B,CAAC;iBACvC;aACF;iBAAM;gBACL,OAAO,IAAI,UAAU,CAAC,GAAG,CAAC,MAAM,EAAE,UAAU,CAAC,YAAY,CAAC,GAAG,CAAC,QAAQ,CAAC,CAAC,CAAC;aAC1E;SACF;QACD,IAAI,oBAAoB,IAAI,GAAG,EAAE;YAC/B,OAAO,IAAI,UAAU,CACnB,GAAG,CAAC,kBAAkB,CAAC,OAAO,EAC9B,UAAU,CAAC,YAAY,CAAC,GAAG,CAAC,kBAAkB,CAAC,OAAO,CAAC,CACxD,CAAC;SACH;QACD,MAAM,IAAI,SAAS,CAAC,8CAA4C,IAAI,CAAC,SAAS,CAAC,GAAG,CAAG,CAAC,CAAC;IACzF,CAAC;IACH,iBAAC;AAAD,CAAC,AAjED,IAiEC;AAjEY,gCAAU;AAmEvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,YAAY,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/symbol.js b/node_modules/bson/lib/symbol.js
new file mode 100644
index 0000000..02554df
--- /dev/null
+++ b/node_modules/bson/lib/symbol.js
@@ -0,0 +1,49 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.BSONSymbol = void 0;
+/**
+ * A class representation of the BSON Symbol type.
+ * @public
+ */
+var BSONSymbol = /** @class */ (function () {
+ /**
+ * @param value - the string representing the symbol.
+ */
+ function BSONSymbol(value) {
+ if (!(this instanceof BSONSymbol))
+ return new BSONSymbol(value);
+ this.value = value;
+ }
+ /** Access the wrapped string value. */
+ BSONSymbol.prototype.valueOf = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.toString = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.inspect = function () {
+ return "new BSONSymbol(\"" + this.value + "\")";
+ };
+ /** @internal */
+ BSONSymbol.prototype.toJSON = function () {
+ return this.value;
+ };
+ /** @internal */
+ BSONSymbol.prototype.toExtendedJSON = function () {
+ return { $symbol: this.value };
+ };
+ /** @internal */
+ BSONSymbol.fromExtendedJSON = function (doc) {
+ return new BSONSymbol(doc.$symbol);
+ };
+ /** @internal */
+ BSONSymbol.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ return BSONSymbol;
+}());
+exports.BSONSymbol = BSONSymbol;
+Object.defineProperty(BSONSymbol.prototype, '_bsontype', { value: 'Symbol' });
+//# sourceMappingURL=symbol.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/symbol.js.map b/node_modules/bson/lib/symbol.js.map
new file mode 100644
index 0000000..6525ede
--- /dev/null
+++ b/node_modules/bson/lib/symbol.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"symbol.js","sourceRoot":"","sources":["../src/symbol.ts"],"names":[],"mappings":";;;AAKA;;;GAGG;AACH;IAIE;;OAEG;IACH,oBAAY,KAAa;QACvB,IAAI,CAAC,CAAC,IAAI,YAAY,UAAU,CAAC;YAAE,OAAO,IAAI,UAAU,CAAC,KAAK,CAAC,CAAC;QAEhE,IAAI,CAAC,KAAK,GAAG,KAAK,CAAC;IACrB,CAAC;IAED,uCAAuC;IACvC,4BAAO,GAAP;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;IACpB,CAAC;IAED,gBAAgB;IAChB,6BAAQ,GAAR;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;IACpB,CAAC;IAED,gBAAgB;IAChB,4BAAO,GAAP;QACE,OAAO,sBAAmB,IAAI,CAAC,KAAK,QAAI,CAAC;IAC3C,CAAC;IAED,gBAAgB;IAChB,2BAAM,GAAN;QACE,OAAO,IAAI,CAAC,KAAK,CAAC;IACpB,CAAC;IAED,gBAAgB;IAChB,mCAAc,GAAd;QACE,OAAO,EAAE,OAAO,EAAE,IAAI,CAAC,KAAK,EAAE,CAAC;IACjC,CAAC;IAED,gBAAgB;IACT,2BAAgB,GAAvB,UAAwB,GAAuB;QAC7C,OAAO,IAAI,UAAU,CAAC,GAAG,CAAC,OAAO,CAAC,CAAC;IACrC,CAAC;IAED,gBAAgB;IAChB,qBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IACH,iBAAC;AAAD,CAAC,AA/CD,IA+CC;AA/CY,gCAAU;AAiDvB,MAAM,CAAC,cAAc,CAAC,UAAU,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,QAAQ,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/timestamp.js b/node_modules/bson/lib/timestamp.js
new file mode 100644
index 0000000..148ff4a
--- /dev/null
+++ b/node_modules/bson/lib/timestamp.js
@@ -0,0 +1,95 @@
+"use strict";
+var __extends = (this && this.__extends) || (function () {
+ var extendStatics = function (d, b) {
+ extendStatics = Object.setPrototypeOf ||
+ ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||
+ function (d, b) { for (var p in b) if (Object.prototype.hasOwnProperty.call(b, p)) d[p] = b[p]; };
+ return extendStatics(d, b);
+ };
+ return function (d, b) {
+ if (typeof b !== "function" && b !== null)
+ throw new TypeError("Class extends value " + String(b) + " is not a constructor or null");
+ extendStatics(d, b);
+ function __() { this.constructor = d; }
+ d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
+ };
+})();
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.Timestamp = exports.LongWithoutOverridesClass = void 0;
+var long_1 = require("./long");
+/** @public */
+exports.LongWithoutOverridesClass = long_1.Long;
+/** @public */
+var Timestamp = /** @class */ (function (_super) {
+ __extends(Timestamp, _super);
+ function Timestamp(low, high) {
+ var _this = this;
+ // eslint-disable-next-line @typescript-eslint/ban-ts-comment
+ ///@ts-expect-error
+ if (!(_this instanceof Timestamp))
+ return new Timestamp(low, high);
+ if (long_1.Long.isLong(low)) {
+ _this = _super.call(this, low.low, low.high, true) || this;
+ }
+ else {
+ _this = _super.call(this, low, high, true) || this;
+ }
+ Object.defineProperty(_this, '_bsontype', {
+ value: 'Timestamp',
+ writable: false,
+ configurable: false,
+ enumerable: false
+ });
+ return _this;
+ }
+ Timestamp.prototype.toJSON = function () {
+ return {
+ $timestamp: this.toString()
+ };
+ };
+ /** Returns a Timestamp represented by the given (32-bit) integer value. */
+ Timestamp.fromInt = function (value) {
+ return new Timestamp(long_1.Long.fromInt(value, true));
+ };
+ /** Returns a Timestamp representing the given number value, provided that it is a finite number. Otherwise, zero is returned. */
+ Timestamp.fromNumber = function (value) {
+ return new Timestamp(long_1.Long.fromNumber(value, true));
+ };
+ /**
+ * Returns a Timestamp for the given high and low bits. Each is assumed to use 32 bits.
+ *
+ * @param lowBits - the low 32-bits.
+ * @param highBits - the high 32-bits.
+ */
+ Timestamp.fromBits = function (lowBits, highBits) {
+ return new Timestamp(lowBits, highBits);
+ };
+ /**
+ * Returns a Timestamp from the given string, optionally using the given radix.
+ *
+ * @param str - the textual representation of the Timestamp.
+ * @param optRadix - the radix in which the text is written.
+ */
+ Timestamp.fromString = function (str, optRadix) {
+ return new Timestamp(long_1.Long.fromString(str, true, optRadix));
+ };
+ /** @internal */
+ Timestamp.prototype.toExtendedJSON = function () {
+ return { $timestamp: { t: this.high >>> 0, i: this.low >>> 0 } };
+ };
+ /** @internal */
+ Timestamp.fromExtendedJSON = function (doc) {
+ return new Timestamp(doc.$timestamp.i, doc.$timestamp.t);
+ };
+ /** @internal */
+ Timestamp.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ Timestamp.prototype.inspect = function () {
+ return "new Timestamp(" + this.getLowBits().toString() + ", " + this.getHighBits().toString() + ")";
+ };
+ Timestamp.MAX_VALUE = long_1.Long.MAX_UNSIGNED_VALUE;
+ return Timestamp;
+}(exports.LongWithoutOverridesClass));
+exports.Timestamp = Timestamp;
+//# sourceMappingURL=timestamp.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/timestamp.js.map b/node_modules/bson/lib/timestamp.js.map
new file mode 100644
index 0000000..a7fa078
--- /dev/null
+++ b/node_modules/bson/lib/timestamp.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"timestamp.js","sourceRoot":"","sources":["../src/timestamp.ts"],"names":[],"mappings":";;;;;;;;;;;;;;;;;;AAAA,+BAA8B;AAQ9B,cAAc;AACD,QAAA,yBAAyB,GAA0B,WAAwC,CAAC;AAUzG,cAAc;AACd;IAA+B,6BAAyB;IAetD,mBAAY,GAAkB,EAAE,IAAa;QAA7C,iBAgBC;QAfC,6DAA6D;QAC7D,mBAAmB;QACnB,IAAI,CAAC,CAAC,KAAI,YAAY,SAAS,CAAC;YAAE,OAAO,IAAI,SAAS,CAAC,GAAG,EAAE,IAAI,CAAC,CAAC;QAElE,IAAI,WAAI,CAAC,MAAM,CAAC,GAAG,CAAC,EAAE;YACpB,QAAA,kBAAM,GAAG,CAAC,GAAG,EAAE,GAAG,CAAC,IAAI,EAAE,IAAI,CAAC,SAAC;SAChC;aAAM;YACL,QAAA,kBAAM,GAAG,EAAE,IAAI,EAAE,IAAI,CAAC,SAAC;SACxB;QACD,MAAM,CAAC,cAAc,CAAC,KAAI,EAAE,WAAW,EAAE;YACvC,KAAK,EAAE,WAAW;YAClB,QAAQ,EAAE,KAAK;YACf,YAAY,EAAE,KAAK;YACnB,UAAU,EAAE,KAAK;SAClB,CAAC,CAAC;;IACL,CAAC;IAED,0BAAM,GAAN;QACE,OAAO;YACL,UAAU,EAAE,IAAI,CAAC,QAAQ,EAAE;SAC5B,CAAC;IACJ,CAAC;IAED,2EAA2E;IACpE,iBAAO,GAAd,UAAe,KAAa;QAC1B,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,OAAO,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;IAClD,CAAC;IAED,iIAAiI;IAC1H,oBAAU,GAAjB,UAAkB,KAAa;QAC7B,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,UAAU,CAAC,KAAK,EAAE,IAAI,CAAC,CAAC,CAAC;IACrD,CAAC;IAED;;;;;OAKG;IACI,kBAAQ,GAAf,UAAgB,OAAe,EAAE,QAAgB;QAC/C,OAAO,IAAI,SAAS,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;IAC1C,CAAC;IAED;;;;;OAKG;IACI,oBAAU,GAAjB,UAAkB,GAAW,EAAE,QAAgB;QAC7C,OAAO,IAAI,SAAS,CAAC,WAAI,CAAC,UAAU,CAAC,GAAG,EAAE,IAAI,EAAE,QAAQ,CAAC,CAAC,CAAC;IAC7D,CAAC;IAED,gBAAgB;IAChB,kCAAc,GAAd;QACE,OAAO,EAAE,UAAU,EAAE,EAAE,CAAC,EAAE,IAAI,CAAC,IAAI,KAAK,CAAC,EAAE,CAAC,EAAE,IAAI,CAAC,GAAG,KAAK,CAAC,EAAE,EAAE,CAAC;IACnE,CAAC;IAED,gBAAgB;IACT,0BAAgB,GAAvB,UAAwB,GAAsB;QAC5C,OAAO,IAAI,SAAS,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC,EAAE,GAAG,CAAC,UAAU,CAAC,CAAC,CAAC,CAAC;IAC3D,CAAC;IAED,gBAAgB;IAChB,oBAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,2BAAO,GAAP;QACE,OAAO,mBAAiB,IAAI,CAAC,UAAU,EAAE,CAAC,QAAQ,EAAE,UAAK,IAAI,CAAC,WAAW,EAAE,CAAC,QAAQ,EAAE,MAAG,CAAC;IAC5F,CAAC;IAnFe,mBAAS,GAAG,WAAI,CAAC,kBAAkB,CAAC;IAoFtD,gBAAC;CAAA,AAvFD,CAA+B,iCAAyB,GAuFvD;AAvFY,8BAAS"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/uuid.js b/node_modules/bson/lib/uuid.js
new file mode 100644
index 0000000..7b56226
--- /dev/null
+++ b/node_modules/bson/lib/uuid.js
@@ -0,0 +1,179 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.UUID = void 0;
+var buffer_1 = require("buffer");
+var ensure_buffer_1 = require("./ensure_buffer");
+var binary_1 = require("./binary");
+var uuid_utils_1 = require("./uuid_utils");
+var utils_1 = require("./parser/utils");
+var BYTE_LENGTH = 16;
+var kId = Symbol('id');
+/**
+ * A class representation of the BSON UUID type.
+ * @public
+ */
+var UUID = /** @class */ (function () {
+ /**
+ * Create an UUID type
+ *
+ * @param input - Can be a 32 or 36 character hex string (dashes excluded/included) or a 16 byte binary Buffer.
+ */
+ function UUID(input) {
+ if (typeof input === 'undefined') {
+ // The most common use case (blank id, new UUID() instance)
+ this.id = UUID.generate();
+ }
+ else if (input instanceof UUID) {
+ this[kId] = buffer_1.Buffer.from(input.id);
+ this.__id = input.__id;
+ }
+ else if (ArrayBuffer.isView(input) && input.byteLength === BYTE_LENGTH) {
+ this.id = ensure_buffer_1.ensureBuffer(input);
+ }
+ else if (typeof input === 'string') {
+ this.id = uuid_utils_1.uuidHexStringToBuffer(input);
+ }
+ else {
+ throw new TypeError('Argument passed in UUID constructor must be a UUID, a 16 byte Buffer or a 32/36 character hex string (dashes excluded/included, format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx).');
+ }
+ }
+ Object.defineProperty(UUID.prototype, "id", {
+ /**
+ * The UUID bytes
+ * @readonly
+ */
+ get: function () {
+ return this[kId];
+ },
+ set: function (value) {
+ this[kId] = value;
+ if (UUID.cacheHexString) {
+ this.__id = uuid_utils_1.bufferToUuidHexString(value);
+ }
+ },
+ enumerable: false,
+ configurable: true
+ });
+ /**
+ * Generate a 16 byte uuid v4 buffer used in UUIDs
+ */
+ /**
+ * Returns the UUID id as a 32 or 36 character hex string representation, excluding/including dashes (defaults to 36 character dash separated)
+ * @param includeDashes - should the string exclude dash-separators.
+ * */
+ UUID.prototype.toHexString = function (includeDashes) {
+ if (includeDashes === void 0) { includeDashes = true; }
+ if (UUID.cacheHexString && this.__id) {
+ return this.__id;
+ }
+ var uuidHexString = uuid_utils_1.bufferToUuidHexString(this.id, includeDashes);
+ if (UUID.cacheHexString) {
+ this.__id = uuidHexString;
+ }
+ return uuidHexString;
+ };
+ /**
+ * Converts the id into a 36 character (dashes included) hex string, unless a encoding is specified.
+ * @internal
+ */
+ UUID.prototype.toString = function (encoding) {
+ return encoding ? this.id.toString(encoding) : this.toHexString();
+ };
+ /**
+ * Converts the id into its JSON string representation. A 36 character (dashes included) hex string in the format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx
+ * @internal
+ */
+ UUID.prototype.toJSON = function () {
+ return this.toHexString();
+ };
+ /**
+ * Compares the equality of this UUID with `otherID`.
+ *
+ * @param otherId - UUID instance to compare against.
+ */
+ UUID.prototype.equals = function (otherId) {
+ if (!otherId) {
+ return false;
+ }
+ if (otherId instanceof UUID) {
+ return otherId.id.equals(this.id);
+ }
+ try {
+ return new UUID(otherId).id.equals(this.id);
+ }
+ catch (_a) {
+ return false;
+ }
+ };
+ /**
+ * Creates a Binary instance from the current UUID.
+ */
+ UUID.prototype.toBinary = function () {
+ return new binary_1.Binary(this.id, binary_1.Binary.SUBTYPE_UUID);
+ };
+ /**
+ * Generates a populated buffer containing a v4 uuid
+ */
+ UUID.generate = function () {
+ var bytes = utils_1.randomBytes(BYTE_LENGTH);
+ // Per 4.4, set bits for version and `clock_seq_hi_and_reserved`
+ // Kindly borrowed from https://github.com/uuidjs/uuid/blob/master/src/v4.js
+ bytes[6] = (bytes[6] & 0x0f) | 0x40;
+ bytes[8] = (bytes[8] & 0x3f) | 0x80;
+ return buffer_1.Buffer.from(bytes);
+ };
+ /**
+ * Checks if a value is a valid bson UUID
+ * @param input - UUID, string or Buffer to validate.
+ */
+ UUID.isValid = function (input) {
+ if (!input) {
+ return false;
+ }
+ if (input instanceof UUID) {
+ return true;
+ }
+ if (typeof input === 'string') {
+ return uuid_utils_1.uuidValidateString(input);
+ }
+ if (utils_1.isUint8Array(input)) {
+ // check for length & uuid version (https://tools.ietf.org/html/rfc4122#section-4.1.3)
+ if (input.length !== BYTE_LENGTH) {
+ return false;
+ }
+ try {
+ // get this byte as hex: xxxxxxxx-xxxx-XXxx-xxxx-xxxxxxxxxxxx
+ // check first part as uuid version: xxxxxxxx-xxxx-Xxxx-xxxx-xxxxxxxxxxxx
+ return parseInt(input[6].toString(16)[0], 10) === binary_1.Binary.SUBTYPE_UUID;
+ }
+ catch (_a) {
+ return false;
+ }
+ }
+ return false;
+ };
+ /**
+ * Creates an UUID from a hex string representation of an UUID.
+ * @param hexString - 32 or 36 character hex string (dashes excluded/included).
+ */
+ UUID.createFromHexString = function (hexString) {
+ var buffer = uuid_utils_1.uuidHexStringToBuffer(hexString);
+ return new UUID(buffer);
+ };
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 36 character hex string representation.
+ * @internal
+ */
+ UUID.prototype[Symbol.for('nodejs.util.inspect.custom')] = function () {
+ return this.inspect();
+ };
+ UUID.prototype.inspect = function () {
+ return "new UUID(\"" + this.toHexString() + "\")";
+ };
+ return UUID;
+}());
+exports.UUID = UUID;
+Object.defineProperty(UUID.prototype, '_bsontype', { value: 'UUID' });
+//# sourceMappingURL=uuid.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/uuid.js.map b/node_modules/bson/lib/uuid.js.map
new file mode 100644
index 0000000..87b1a24
--- /dev/null
+++ b/node_modules/bson/lib/uuid.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"uuid.js","sourceRoot":"","sources":["../src/uuid.ts"],"names":[],"mappings":";;;AAAA,iCAAgC;AAChC,iDAA+C;AAC/C,mCAAkC;AAClC,2CAAgG;AAChG,wCAA2D;AAO3D,IAAM,WAAW,GAAG,EAAE,CAAC;AAEvB,IAAM,GAAG,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;AAEzB;;;GAGG;AACH;IAWE;;;;OAIG;IACH,cAAY,KAA8B;QACxC,IAAI,OAAO,KAAK,KAAK,WAAW,EAAE;YAChC,2DAA2D;YAC3D,IAAI,CAAC,EAAE,GAAG,IAAI,CAAC,QAAQ,EAAE,CAAC;SAC3B;aAAM,IAAI,KAAK,YAAY,IAAI,EAAE;YAChC,IAAI,CAAC,GAAG,CAAC,GAAG,eAAM,CAAC,IAAI,CAAC,KAAK,CAAC,EAAE,CAAC,CAAC;YAClC,IAAI,CAAC,IAAI,GAAG,KAAK,CAAC,IAAI,CAAC;SACxB;aAAM,IAAI,WAAW,CAAC,MAAM,CAAC,KAAK,CAAC,IAAI,KAAK,CAAC,UAAU,KAAK,WAAW,EAAE;YACxE,IAAI,CAAC,EAAE,GAAG,4BAAY,CAAC,KAAK,CAAC,CAAC;SAC/B;aAAM,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YACpC,IAAI,CAAC,EAAE,GAAG,kCAAqB,CAAC,KAAK,CAAC,CAAC;SACxC;aAAM;YACL,MAAM,IAAI,SAAS,CACjB,gLAAgL,CACjL,CAAC;SACH;IACH,CAAC;IAMD,sBAAI,oBAAE;QAJN;;;WAGG;aACH;YACE,OAAO,IAAI,CAAC,GAAG,CAAC,CAAC;QACnB,CAAC;aAED,UAAO,KAAa;YAClB,IAAI,CAAC,GAAG,CAAC,GAAG,KAAK,CAAC;YAElB,IAAI,IAAI,CAAC,cAAc,EAAE;gBACvB,IAAI,CAAC,IAAI,GAAG,kCAAqB,CAAC,KAAK,CAAC,CAAC;aAC1C;QACH,CAAC;;;OARA;IAUD;;OAEG;IAEH;;;SAGK;IACL,0BAAW,GAAX,UAAY,aAAoB;QAApB,8BAAA,EAAA,oBAAoB;QAC9B,IAAI,IAAI,CAAC,cAAc,IAAI,IAAI,CAAC,IAAI,EAAE;YACpC,OAAO,IAAI,CAAC,IAAI,CAAC;SAClB;QAED,IAAM,aAAa,GAAG,kCAAqB,CAAC,IAAI,CAAC,EAAE,EAAE,aAAa,CAAC,CAAC;QAEpE,IAAI,IAAI,CAAC,cAAc,EAAE;YACvB,IAAI,CAAC,IAAI,GAAG,aAAa,CAAC;SAC3B;QAED,OAAO,aAAa,CAAC;IACvB,CAAC;IAED;;;OAGG;IACH,uBAAQ,GAAR,UAAS,QAAiB;QACxB,OAAO,QAAQ,CAAC,CAAC,CAAC,IAAI,CAAC,EAAE,CAAC,QAAQ,CAAC,QAAQ,CAAC,CAAC,CAAC,CAAC,IAAI,CAAC,WAAW,EAAE,CAAC;IACpE,CAAC;IAED;;;OAGG;IACH,qBAAM,GAAN;QACE,OAAO,IAAI,CAAC,WAAW,EAAE,CAAC;IAC5B,CAAC;IAED;;;;OAIG;IACH,qBAAM,GAAN,UAAO,OAA+B;QACpC,IAAI,CAAC,OAAO,EAAE;YACZ,OAAO,KAAK,CAAC;SACd;QAED,IAAI,OAAO,YAAY,IAAI,EAAE;YAC3B,OAAO,OAAO,CAAC,EAAE,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;SACnC;QAED,IAAI;YACF,OAAO,IAAI,IAAI,CAAC,OAAO,CAAC,CAAC,EAAE,CAAC,MAAM,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC;SAC7C;QAAC,WAAM;YACN,OAAO,KAAK,CAAC;SACd;IACH,CAAC;IAED;;OAEG;IACH,uBAAQ,GAAR;QACE,OAAO,IAAI,eAAM,CAAC,IAAI,CAAC,EAAE,EAAE,eAAM,CAAC,YAAY,CAAC,CAAC;IAClD,CAAC;IAED;;OAEG;IACI,aAAQ,GAAf;QACE,IAAM,KAAK,GAAG,mBAAW,CAAC,WAAW,CAAC,CAAC;QAEvC,gEAAgE;QAChE,4EAA4E;QAC5E,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,IAAI,CAAC;QACpC,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,KAAK,CAAC,CAAC,CAAC,GAAG,IAAI,CAAC,GAAG,IAAI,CAAC;QAEpC,OAAO,eAAM,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;IAC5B,CAAC;IAED;;;OAGG;IACI,YAAO,GAAd,UAAe,KAA6B;QAC1C,IAAI,CAAC,KAAK,EAAE;YACV,OAAO,KAAK,CAAC;SACd;QAED,IAAI,KAAK,YAAY,IAAI,EAAE;YACzB,OAAO,IAAI,CAAC;SACb;QAED,IAAI,OAAO,KAAK,KAAK,QAAQ,EAAE;YAC7B,OAAO,+BAAkB,CAAC,KAAK,CAAC,CAAC;SAClC;QAED,IAAI,oBAAY,CAAC,KAAK,CAAC,EAAE;YACvB,sFAAsF;YACtF,IAAI,KAAK,CAAC,MAAM,KAAK,WAAW,EAAE;gBAChC,OAAO,KAAK,CAAC;aACd;YAED,IAAI;gBACF,yEAAyE;gBACzE,yEAAyE;gBACzE,OAAO,QAAQ,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,QAAQ,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,KAAK,eAAM,CAAC,YAAY,CAAC;aACvE;YAAC,WAAM;gBACN,OAAO,KAAK,CAAC;aACd;SACF;QAED,OAAO,KAAK,CAAC;IACf,CAAC;IAED;;;OAGG;IACI,wBAAmB,GAA1B,UAA2B,SAAiB;QAC1C,IAAM,MAAM,GAAG,kCAAqB,CAAC,SAAS,CAAC,CAAC;QAChD,OAAO,IAAI,IAAI,CAAC,MAAM,CAAC,CAAC;IAC1B,CAAC;IAED;;;;;OAKG;IACH,eAAC,MAAM,CAAC,GAAG,CAAC,4BAA4B,CAAC,CAAC,GAA1C;QACE,OAAO,IAAI,CAAC,OAAO,EAAE,CAAC;IACxB,CAAC;IAED,sBAAO,GAAP;QACE,OAAO,gBAAa,IAAI,CAAC,WAAW,EAAE,QAAI,CAAC;IAC7C,CAAC;IACH,WAAC;AAAD,CAAC,AA3LD,IA2LC;AA3LY,oBAAI;AA6LjB,MAAM,CAAC,cAAc,CAAC,IAAI,CAAC,SAAS,EAAE,WAAW,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE,CAAC,CAAC"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/uuid_utils.js b/node_modules/bson/lib/uuid_utils.js
new file mode 100644
index 0000000..55dbb6f
--- /dev/null
+++ b/node_modules/bson/lib/uuid_utils.js
@@ -0,0 +1,34 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.bufferToUuidHexString = exports.uuidHexStringToBuffer = exports.uuidValidateString = void 0;
+var buffer_1 = require("buffer");
+// Validation regex for v4 uuid (validates with or without dashes)
+var VALIDATION_REGEX = /^(?:[0-9a-f]{8}-[0-9a-f]{4}-4[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}|[0-9a-f]{12}4[0-9a-f]{3}[89ab][0-9a-f]{15})$/i;
+var uuidValidateString = function (str) {
+ return typeof str === 'string' && VALIDATION_REGEX.test(str);
+};
+exports.uuidValidateString = uuidValidateString;
+var uuidHexStringToBuffer = function (hexString) {
+ if (!exports.uuidValidateString(hexString)) {
+ throw new TypeError('UUID string representations must be a 32 or 36 character hex string (dashes excluded/included). Format: "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx" or "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx".');
+ }
+ var sanitizedHexString = hexString.replace(/-/g, '');
+ return buffer_1.Buffer.from(sanitizedHexString, 'hex');
+};
+exports.uuidHexStringToBuffer = uuidHexStringToBuffer;
+var bufferToUuidHexString = function (buffer, includeDashes) {
+ if (includeDashes === void 0) { includeDashes = true; }
+ return includeDashes
+ ? buffer.toString('hex', 0, 4) +
+ '-' +
+ buffer.toString('hex', 4, 6) +
+ '-' +
+ buffer.toString('hex', 6, 8) +
+ '-' +
+ buffer.toString('hex', 8, 10) +
+ '-' +
+ buffer.toString('hex', 10, 16)
+ : buffer.toString('hex');
+};
+exports.bufferToUuidHexString = bufferToUuidHexString;
+//# sourceMappingURL=uuid_utils.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/uuid_utils.js.map b/node_modules/bson/lib/uuid_utils.js.map
new file mode 100644
index 0000000..5f6b829
--- /dev/null
+++ b/node_modules/bson/lib/uuid_utils.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"uuid_utils.js","sourceRoot":"","sources":["../src/uuid_utils.ts"],"names":[],"mappings":";;;AAAA,iCAAgC;AAEhC,kEAAkE;AAClE,IAAM,gBAAgB,GAAG,uHAAuH,CAAC;AAE1I,IAAM,kBAAkB,GAAG,UAAC,GAAW;IAC5C,OAAA,OAAO,GAAG,KAAK,QAAQ,IAAI,gBAAgB,CAAC,IAAI,CAAC,GAAG,CAAC;AAArD,CAAqD,CAAC;AAD3C,QAAA,kBAAkB,sBACyB;AAEjD,IAAM,qBAAqB,GAAG,UAAC,SAAiB;IACrD,IAAI,CAAC,0BAAkB,CAAC,SAAS,CAAC,EAAE;QAClC,MAAM,IAAI,SAAS,CACjB,uLAAuL,CACxL,CAAC;KACH;IAED,IAAM,kBAAkB,GAAG,SAAS,CAAC,OAAO,CAAC,IAAI,EAAE,EAAE,CAAC,CAAC;IACvD,OAAO,eAAM,CAAC,IAAI,CAAC,kBAAkB,EAAE,KAAK,CAAC,CAAC;AAChD,CAAC,CAAC;AATW,QAAA,qBAAqB,yBAShC;AAEK,IAAM,qBAAqB,GAAG,UAAC,MAAc,EAAE,aAAoB;IAApB,8BAAA,EAAA,oBAAoB;IACxE,OAAA,aAAa;QACX,CAAC,CAAC,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;YAC5B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;YAC5B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,CAAC,CAAC;YAC5B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,CAAC,EAAE,EAAE,CAAC;YAC7B,GAAG;YACH,MAAM,CAAC,QAAQ,CAAC,KAAK,EAAE,EAAE,EAAE,EAAE,CAAC;QAChC,CAAC,CAAC,MAAM,CAAC,QAAQ,CAAC,KAAK,CAAC;AAV1B,CAU0B,CAAC;AAXhB,QAAA,qBAAqB,yBAWL"}
\ No newline at end of file
diff --git a/node_modules/bson/lib/validate_utf8.js b/node_modules/bson/lib/validate_utf8.js
new file mode 100644
index 0000000..ec78016
--- /dev/null
+++ b/node_modules/bson/lib/validate_utf8.js
@@ -0,0 +1,47 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.validateUtf8 = void 0;
+var FIRST_BIT = 0x80;
+var FIRST_TWO_BITS = 0xc0;
+var FIRST_THREE_BITS = 0xe0;
+var FIRST_FOUR_BITS = 0xf0;
+var FIRST_FIVE_BITS = 0xf8;
+var TWO_BIT_CHAR = 0xc0;
+var THREE_BIT_CHAR = 0xe0;
+var FOUR_BIT_CHAR = 0xf0;
+var CONTINUING_CHAR = 0x80;
+/**
+ * Determines if the passed in bytes are valid utf8
+ * @param bytes - An array of 8-bit bytes. Must be indexable and have length property
+ * @param start - The index to start validating
+ * @param end - The index to end validating
+ */
+function validateUtf8(bytes, start, end) {
+ var continuation = 0;
+ for (var i = start; i < end; i += 1) {
+ var byte = bytes[i];
+ if (continuation) {
+ if ((byte & FIRST_TWO_BITS) !== CONTINUING_CHAR) {
+ return false;
+ }
+ continuation -= 1;
+ }
+ else if (byte & FIRST_BIT) {
+ if ((byte & FIRST_THREE_BITS) === TWO_BIT_CHAR) {
+ continuation = 1;
+ }
+ else if ((byte & FIRST_FOUR_BITS) === THREE_BIT_CHAR) {
+ continuation = 2;
+ }
+ else if ((byte & FIRST_FIVE_BITS) === FOUR_BIT_CHAR) {
+ continuation = 3;
+ }
+ else {
+ return false;
+ }
+ }
+ }
+ return !continuation;
+}
+exports.validateUtf8 = validateUtf8;
+//# sourceMappingURL=validate_utf8.js.map
\ No newline at end of file
diff --git a/node_modules/bson/lib/validate_utf8.js.map b/node_modules/bson/lib/validate_utf8.js.map
new file mode 100644
index 0000000..f1e975b
--- /dev/null
+++ b/node_modules/bson/lib/validate_utf8.js.map
@@ -0,0 +1 @@
+{"version":3,"file":"validate_utf8.js","sourceRoot":"","sources":["../src/validate_utf8.ts"],"names":[],"mappings":";;;AAAA,IAAM,SAAS,GAAG,IAAI,CAAC;AACvB,IAAM,cAAc,GAAG,IAAI,CAAC;AAC5B,IAAM,gBAAgB,GAAG,IAAI,CAAC;AAC9B,IAAM,eAAe,GAAG,IAAI,CAAC;AAC7B,IAAM,eAAe,GAAG,IAAI,CAAC;AAE7B,IAAM,YAAY,GAAG,IAAI,CAAC;AAC1B,IAAM,cAAc,GAAG,IAAI,CAAC;AAC5B,IAAM,aAAa,GAAG,IAAI,CAAC;AAC3B,IAAM,eAAe,GAAG,IAAI,CAAC;AAE7B;;;;;GAKG;AACH,SAAgB,YAAY,CAC1B,KAAkC,EAClC,KAAa,EACb,GAAW;IAEX,IAAI,YAAY,GAAG,CAAC,CAAC;IAErB,KAAK,IAAI,CAAC,GAAG,KAAK,EAAE,CAAC,GAAG,GAAG,EAAE,CAAC,IAAI,CAAC,EAAE;QACnC,IAAM,IAAI,GAAG,KAAK,CAAC,CAAC,CAAC,CAAC;QAEtB,IAAI,YAAY,EAAE;YAChB,IAAI,CAAC,IAAI,GAAG,cAAc,CAAC,KAAK,eAAe,EAAE;gBAC/C,OAAO,KAAK,CAAC;aACd;YACD,YAAY,IAAI,CAAC,CAAC;SACnB;aAAM,IAAI,IAAI,GAAG,SAAS,EAAE;YAC3B,IAAI,CAAC,IAAI,GAAG,gBAAgB,CAAC,KAAK,YAAY,EAAE;gBAC9C,YAAY,GAAG,CAAC,CAAC;aAClB;iBAAM,IAAI,CAAC,IAAI,GAAG,eAAe,CAAC,KAAK,cAAc,EAAE;gBACtD,YAAY,GAAG,CAAC,CAAC;aAClB;iBAAM,IAAI,CAAC,IAAI,GAAG,eAAe,CAAC,KAAK,aAAa,EAAE;gBACrD,YAAY,GAAG,CAAC,CAAC;aAClB;iBAAM;gBACL,OAAO,KAAK,CAAC;aACd;SACF;KACF;IAED,OAAO,CAAC,YAAY,CAAC;AACvB,CAAC;AA7BD,oCA6BC"}
\ No newline at end of file
diff --git a/node_modules/bson/package.json b/node_modules/bson/package.json
index 7399622..c6470da 100644
--- a/node_modules/bson/package.json
+++ b/node_modules/bson/package.json
@@ -1,32 +1,35 @@
{
- "_from": "bson@^1.1.4",
- "_id": "bson@1.1.5",
+ "_from": "bson@^4.4.0",
+ "_id": "bson@4.4.1",
"_inBundle": false,
- "_integrity": "sha512-kDuEzldR21lHciPQAIulLs1LZlCXdLziXI6Mb/TDkwXhb//UORJNPXgcRs2CuO4H0DcMkpfT3/ySsP3unoZjBg==",
+ "_integrity": "sha512-Uu4OCZa0jouQJCKOk1EmmyqtdWAP5HVLru4lQxTwzJzxT+sJ13lVpEZU/MATDxtHiekWMAL84oQY3Xn1LpJVSg==",
"_location": "/bson",
"_phantomChildren": {},
"_requested": {
"type": "range",
"registry": true,
- "raw": "bson@^1.1.4",
+ "raw": "bson@^4.4.0",
"name": "bson",
"escapedName": "bson",
- "rawSpec": "^1.1.4",
+ "rawSpec": "^4.4.0",
"saveSpec": null,
- "fetchSpec": "^1.1.4"
+ "fetchSpec": "^4.4.0"
},
"_requiredBy": [
"/mongodb"
],
- "_resolved": "https://registry.npmjs.org/bson/-/bson-1.1.5.tgz",
- "_shasum": "2aaae98fcdf6750c0848b0cba1ddec3c73060a34",
- "_spec": "bson@^1.1.4",
- "_where": "/Users/jasonagus/Desktop/Skyblock Guide/node_modules/mongodb",
+ "_resolved": "https://registry.npmjs.org/bson/-/bson-4.4.1.tgz",
+ "_shasum": "682c3cb8b90b222414ce14ef8398154ba2cc21bc",
+ "_spec": "bson@^4.4.0",
+ "_where": "/Users/jasonagus/Desktop/Guide Project/node_modules/mongodb",
"author": {
"name": "Christian Amor Kvalheim",
"email": "christkv@gmail.com"
},
- "browser": "lib/bson/bson.js",
+ "browser": {
+ "./lib/bson.js": "./dist/bson.browser.umd.js",
+ "./dist/bson.esm.js": "./dist/bson.browser.esm.js"
+ },
"bugs": {
"url": "https://github.com/mongodb/js-bson/issues"
},
@@ -35,33 +38,62 @@
"native": false
},
"contributors": [],
+ "dependencies": {
+ "buffer": "^5.6.0"
+ },
"deprecated": false,
"description": "A bson parser for node.js and the browser",
"devDependencies": {
- "babel-core": "^6.14.0",
- "babel-loader": "^6.2.5",
- "babel-polyfill": "^6.13.0",
- "babel-preset-es2015": "^6.14.0",
- "babel-preset-stage-0": "^6.5.0",
- "babel-register": "^6.14.0",
- "benchmark": "1.0.0",
- "colors": "1.1.0",
- "conventional-changelog-cli": "^1.3.5",
- "nodeunit": "0.9.0",
- "standard-version": "^7.1.0",
- "webpack": "^1.13.2",
- "webpack-polyfills-plugin": "0.0.9"
- },
- "directories": {
- "lib": "./lib/bson"
+ "@babel/plugin-external-helpers": "^7.10.4",
+ "@babel/preset-env": "^7.11.0",
+ "@istanbuljs/nyc-config-typescript": "^1.0.1",
+ "@microsoft/api-extractor": "^7.11.2",
+ "@rollup/plugin-babel": "^5.2.0",
+ "@rollup/plugin-commonjs": "^15.0.0",
+ "@rollup/plugin-json": "^4.1.0",
+ "@rollup/plugin-node-resolve": "^9.0.0",
+ "@rollup/plugin-typescript": "^6.0.0",
+ "@typescript-eslint/eslint-plugin": "^3.10.1",
+ "@typescript-eslint/parser": "^3.10.1",
+ "benchmark": "^2.1.4",
+ "chai": "^4.2.0",
+ "downlevel-dts": "^0.7.0",
+ "eslint": "^7.7.0",
+ "eslint-config-prettier": "^6.11.0",
+ "eslint-plugin-prettier": "^3.1.4",
+ "eslint-plugin-tsdoc": "^0.2.6",
+ "karma": "^5.1.1",
+ "karma-chai": "^0.1.0",
+ "karma-chrome-launcher": "^3.1.0",
+ "karma-mocha": "^2.0.1",
+ "karma-mocha-reporter": "^2.2.5",
+ "karma-rollup-preprocessor": "^7.0.5",
+ "mocha": "5.2.0",
+ "node-fetch": "^2.6.1",
+ "nyc": "^15.1.0",
+ "prettier": "^2.1.1",
+ "rimraf": "^3.0.2",
+ "rollup": "^2.26.5",
+ "rollup-plugin-commonjs": "^10.1.0",
+ "rollup-plugin-node-builtins": "^2.1.2",
+ "rollup-plugin-node-globals": "^1.4.0",
+ "rollup-plugin-node-polyfills": "^0.2.1",
+ "standard-version": "^9.3.0",
+ "ts-node": "^9.0.0",
+ "typedoc": "^0.21.2",
+ "typescript": "^4.0.2",
+ "typescript-cached-transpile": "0.0.6",
+ "uuid": "^8.3.2"
},
"engines": {
- "node": ">=0.6.19"
+ "node": ">=6.9.0"
},
"files": [
"lib",
- "index.js",
- "browser_build",
+ "src",
+ "dist",
+ "bson.d.ts",
+ "etc/prepare.js",
"bower.json"
],
"homepage": "https://github.com/mongodb/js-bson#readme",
@@ -71,19 +103,29 @@
"parser"
],
"license": "Apache-2.0",
- "main": "./index",
+ "main": "lib/bson.js",
+ "module": "dist/bson.esm.js",
"name": "bson",
"repository": {
"type": "git",
"url": "git+https://github.com/mongodb/js-bson.git"
},
"scripts": {
- "build": "webpack --config ./webpack.dist.config.js",
- "changelog": "conventional-changelog -p angular -i HISTORY.md -s",
- "format": "prettier --print-width 100 --tab-width 2 --single-quote --write 'test/**/*.js' 'lib/**/*.js'",
- "lint": "eslint lib test",
+ "build": "npm run build:dts && npm run build:bundle",
+ "build:bundle": "rollup -c rollup.config.js",
+ "build:dts": "npm run build:ts && api-extractor run --typescript-compiler-folder node_modules/typescript --local && rimraf 'lib/**/*.d.ts*' && downlevel-dts bson.d.ts bson.d.ts",
+ "build:ts": "tsc",
+ "coverage": "nyc npm run test-node",
+ "coverage:html": "npm run coverage && open ./coverage/index.html",
+ "docs": "typedoc",
+ "format": "eslint --ext '.js,.ts' src test --fix",
+ "lint": "eslint -v && eslint --ext '.js,.ts' --max-warnings=0 src test && tsc -v && tsc --noEmit",
+ "prepare": "node etc/prepare.js",
"release": "standard-version -i HISTORY.md",
- "test": "nodeunit ./test/node"
+ "test": "npm run build && npm run test-node && npm run test-browser",
+ "test-browser": "karma start karma.conf.js",
+ "test-node": "mocha test/node test/*_tests.js"
},
- "version": "1.1.5"
+ "types": "bson.d.ts",
+ "version": "4.4.1"
}
diff --git a/node_modules/bson/src/binary.ts b/node_modules/bson/src/binary.ts
new file mode 100644
index 0000000..1d31164
--- /dev/null
+++ b/node_modules/bson/src/binary.ts
@@ -0,0 +1,284 @@
+import { Buffer } from 'buffer';
+import { ensureBuffer } from './ensure_buffer';
+import { uuidHexStringToBuffer } from './uuid_utils';
+import { UUID, UUIDExtended } from './uuid';
+import type { EJSONOptions } from './extended_json';
+
+/** @public */
+export type BinarySequence = Uint8Array | Buffer | number[];
+
+/** @public */
+export interface BinaryExtendedLegacy {
+ $type: string;
+ $binary: string;
+}
+
+/** @public */
+export interface BinaryExtended {
+ $binary: {
+ subType: string;
+ base64: string;
+ };
+}
+
+/**
+ * A class representation of the BSON Binary type.
+ * @public
+ */
+export class Binary {
+ _bsontype!: 'Binary';
+
+ /**
+ * Binary default subtype
+ * @internal
+ */
+ private static readonly BSON_BINARY_SUBTYPE_DEFAULT = 0;
+
+ /** Initial buffer default size */
+ static readonly BUFFER_SIZE = 256;
+ /** Default BSON type */
+ static readonly SUBTYPE_DEFAULT = 0;
+ /** Function BSON type */
+ static readonly SUBTYPE_FUNCTION = 1;
+ /** Byte Array BSON type */
+ static readonly SUBTYPE_BYTE_ARRAY = 2;
+ /** Deprecated UUID BSON type @deprecated Please use SUBTYPE_UUID */
+ static readonly SUBTYPE_UUID_OLD = 3;
+ /** UUID BSON type */
+ static readonly SUBTYPE_UUID = 4;
+ /** MD5 BSON type */
+ static readonly SUBTYPE_MD5 = 5;
+ /** User BSON type */
+ static readonly SUBTYPE_USER_DEFINED = 128;
+
+ buffer!: Buffer;
+ sub_type!: number;
+ position!: number;
+
+ /**
+ * @param buffer - a buffer object containing the binary data.
+ * @param subType - the option binary type.
+ */
+ constructor(buffer?: string | BinarySequence, subType?: number) {
+ if (!(this instanceof Binary)) return new Binary(buffer, subType);
+
+ if (
+ !(buffer == null) &&
+ !(typeof buffer === 'string') &&
+ !ArrayBuffer.isView(buffer) &&
+ !(buffer instanceof ArrayBuffer) &&
+ !Array.isArray(buffer)
+ ) {
+ throw new TypeError(
+ 'Binary can only be constructed from string, Buffer, TypedArray, or Array'
+ );
+ }
+
+ this.sub_type = subType ?? Binary.BSON_BINARY_SUBTYPE_DEFAULT;
+
+ if (buffer == null) {
+ // create an empty binary buffer
+ this.buffer = Buffer.alloc(Binary.BUFFER_SIZE);
+ this.position = 0;
+ } else {
+ if (typeof buffer === 'string') {
+ // string
+ this.buffer = Buffer.from(buffer, 'binary');
+ } else if (Array.isArray(buffer)) {
+ // number[]
+ this.buffer = Buffer.from(buffer);
+ } else {
+ // Buffer | TypedArray | ArrayBuffer
+ this.buffer = ensureBuffer(buffer);
+ }
+
+ this.position = this.buffer.byteLength;
+ }
+ }
+
+ /**
+ * Updates this binary with byte_value.
+ *
+ * @param byteValue - a single byte we wish to write.
+ */
+ put(byteValue: string | number | Uint8Array | Buffer | number[]): void {
+ // If it's a string and a has more than one character throw an error
+ if (typeof byteValue === 'string' && byteValue.length !== 1) {
+ throw new TypeError('only accepts single character String');
+ } else if (typeof byteValue !== 'number' && byteValue.length !== 1)
+ throw new TypeError('only accepts single character Uint8Array or Array');
+
+ // Decode the byte value once
+ let decodedByte: number;
+ if (typeof byteValue === 'string') {
+ decodedByte = byteValue.charCodeAt(0);
+ } else if (typeof byteValue === 'number') {
+ decodedByte = byteValue;
+ } else {
+ decodedByte = byteValue[0];
+ }
+
+ if (decodedByte < 0 || decodedByte > 255) {
+ throw new TypeError('only accepts number in a valid unsigned byte range 0-255');
+ }
+
+ if (this.buffer.length > this.position) {
+ this.buffer[this.position++] = decodedByte;
+ } else {
+ const buffer = Buffer.alloc(Binary.BUFFER_SIZE + this.buffer.length);
+ // Combine the two buffers together
+ this.buffer.copy(buffer, 0, 0, this.buffer.length);
+ this.buffer = buffer;
+ this.buffer[this.position++] = decodedByte;
+ }
+ }
+
+ /**
+ * Writes a buffer or string to the binary.
+ *
+ * @param sequence - a string or buffer to be written to the Binary BSON object.
+ * @param offset - specify the binary of where to write the content.
+ */
+ write(sequence: string | BinarySequence, offset: number): void {
+ offset = typeof offset === 'number' ? offset : this.position;
+
+ // If the buffer is to small let's extend the buffer
+ if (this.buffer.length < offset + sequence.length) {
+ const buffer = Buffer.alloc(this.buffer.length + sequence.length);
+ this.buffer.copy(buffer, 0, 0, this.buffer.length);
+
+ // Assign the new buffer
+ this.buffer = buffer;
+ }
+
+ if (ArrayBuffer.isView(sequence)) {
+ this.buffer.set(ensureBuffer(sequence), offset);
+ this.position =
+ offset + sequence.byteLength > this.position ? offset + sequence.length : this.position;
+ } else if (typeof sequence === 'string') {
+ this.buffer.write(sequence, offset, sequence.length, 'binary');
+ this.position =
+ offset + sequence.length > this.position ? offset + sequence.length : this.position;
+ }
+ }
+
+ /**
+ * Reads **length** bytes starting at **position**.
+ *
+ * @param position - read from the given position in the Binary.
+ * @param length - the number of bytes to read.
+ */
+ read(position: number, length: number): BinarySequence {
+ length = length && length > 0 ? length : this.position;
+
+ // Let's return the data based on the type we have
+ return this.buffer.slice(position, position + length);
+ }
+
+ /**
+ * Returns the value of this binary as a string.
+ * @param asRaw - Will skip converting to a string
+ * @remarks
+ * This is handy when calling this function conditionally for some key value pairs and not others
+ */
+ value(asRaw?: boolean): string | BinarySequence {
+ asRaw = !!asRaw;
+
+ // Optimize to serialize for the situation where the data == size of buffer
+ if (asRaw && this.buffer.length === this.position) {
+ return this.buffer;
+ }
+
+ // If it's a node.js buffer object
+ if (asRaw) {
+ return this.buffer.slice(0, this.position);
+ }
+ return this.buffer.toString('binary', 0, this.position);
+ }
+
+ /** the length of the binary sequence */
+ length(): number {
+ return this.position;
+ }
+
+ /** @internal */
+ toJSON(): string {
+ return this.buffer.toString('base64');
+ }
+
+ /** @internal */
+ toString(format: string): string {
+ return this.buffer.toString(format);
+ }
+
+ /** @internal */
+ toExtendedJSON(options?: EJSONOptions): BinaryExtendedLegacy | BinaryExtended {
+ options = options || {};
+ const base64String = this.buffer.toString('base64');
+
+ const subType = Number(this.sub_type).toString(16);
+ if (options.legacy) {
+ return {
+ $binary: base64String,
+ $type: subType.length === 1 ? '0' + subType : subType
+ };
+ }
+ return {
+ $binary: {
+ base64: base64String,
+ subType: subType.length === 1 ? '0' + subType : subType
+ }
+ };
+ }
+
+ /** @internal */
+ toUUID(): UUID {
+ if (this.sub_type === Binary.SUBTYPE_UUID) {
+ return new UUID(this.buffer.slice(0, this.position));
+ }
+
+ throw new Error(
+ `Binary sub_type "${this.sub_type}" is not supported for converting to UUID. Only "${Binary.SUBTYPE_UUID}" is currently supported.`
+ );
+ }
+
+ /** @internal */
+ static fromExtendedJSON(
+ doc: BinaryExtendedLegacy | BinaryExtended | UUIDExtended,
+ options?: EJSONOptions
+ ): Binary {
+ options = options || {};
+ let data: Buffer | undefined;
+ let type;
+ if ('$binary' in doc) {
+ if (options.legacy && typeof doc.$binary === 'string' && '$type' in doc) {
+ type = doc.$type ? parseInt(doc.$type, 16) : 0;
+ data = Buffer.from(doc.$binary, 'base64');
+ } else {
+ if (typeof doc.$binary !== 'string') {
+ type = doc.$binary.subType ? parseInt(doc.$binary.subType, 16) : 0;
+ data = Buffer.from(doc.$binary.base64, 'base64');
+ }
+ }
+ } else if ('$uuid' in doc) {
+ type = 4;
+ data = uuidHexStringToBuffer(doc.$uuid);
+ }
+ if (!data) {
+ throw new TypeError(`Unexpected Binary Extended JSON format ${JSON.stringify(doc)}`);
+ }
+ return new Binary(data, type);
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ const asBuffer = this.value(true);
+ return `new Binary(Buffer.from("${asBuffer.toString('hex')}", "hex"), ${this.sub_type})`;
+ }
+}
+
+Object.defineProperty(Binary.prototype, '_bsontype', { value: 'Binary' });
diff --git a/node_modules/bson/src/bson.ts b/node_modules/bson/src/bson.ts
new file mode 100644
index 0000000..40c74f9
--- /dev/null
+++ b/node_modules/bson/src/bson.ts
@@ -0,0 +1,329 @@
+import { Buffer } from 'buffer';
+import { Binary } from './binary';
+import { Code } from './code';
+import { DBRef } from './db_ref';
+import { Decimal128 } from './decimal128';
+import { Double } from './double';
+import { ensureBuffer } from './ensure_buffer';
+import { EJSON } from './extended_json';
+import { Int32 } from './int_32';
+import { Long } from './long';
+import { Map } from './map';
+import { MaxKey } from './max_key';
+import { MinKey } from './min_key';
+import { ObjectId } from './objectid';
+import { calculateObjectSize as internalCalculateObjectSize } from './parser/calculate_size';
+// Parts of the parser
+import { deserialize as internalDeserialize, DeserializeOptions } from './parser/deserializer';
+import { serializeInto as internalSerialize, SerializeOptions } from './parser/serializer';
+import { BSONRegExp } from './regexp';
+import { BSONSymbol } from './symbol';
+import { Timestamp } from './timestamp';
+import { UUID } from './uuid';
+export { BinaryExtended, BinaryExtendedLegacy, BinarySequence } from './binary';
+export { CodeExtended } from './code';
+export {
+ BSON_BINARY_SUBTYPE_BYTE_ARRAY,
+ BSON_BINARY_SUBTYPE_DEFAULT,
+ BSON_BINARY_SUBTYPE_FUNCTION,
+ BSON_BINARY_SUBTYPE_MD5,
+ BSON_BINARY_SUBTYPE_USER_DEFINED,
+ BSON_BINARY_SUBTYPE_UUID,
+ BSON_BINARY_SUBTYPE_UUID_NEW,
+ BSON_DATA_ARRAY,
+ BSON_DATA_BINARY,
+ BSON_DATA_BOOLEAN,
+ BSON_DATA_CODE,
+ BSON_DATA_CODE_W_SCOPE,
+ BSON_DATA_DATE,
+ BSON_DATA_DBPOINTER,
+ BSON_DATA_DECIMAL128,
+ BSON_DATA_INT,
+ BSON_DATA_LONG,
+ BSON_DATA_MAX_KEY,
+ BSON_DATA_MIN_KEY,
+ BSON_DATA_NULL,
+ BSON_DATA_NUMBER,
+ BSON_DATA_OBJECT,
+ BSON_DATA_OID,
+ BSON_DATA_REGEXP,
+ BSON_DATA_STRING,
+ BSON_DATA_SYMBOL,
+ BSON_DATA_TIMESTAMP,
+ BSON_DATA_UNDEFINED,
+ BSON_INT32_MAX,
+ BSON_INT32_MIN,
+ BSON_INT64_MAX,
+ BSON_INT64_MIN
+} from './constants';
+export { DBRefLike } from './db_ref';
+export { Decimal128Extended } from './decimal128';
+export { DoubleExtended } from './double';
+export { EJSON, EJSONOptions } from './extended_json';
+export { Int32Extended } from './int_32';
+export { LongExtended } from './long';
+export { MaxKeyExtended } from './max_key';
+export { MinKeyExtended } from './min_key';
+export { ObjectIdExtended, ObjectIdLike } from './objectid';
+export { BSONRegExpExtended, BSONRegExpExtendedLegacy } from './regexp';
+export { BSONSymbolExtended } from './symbol';
+export {
+ LongWithoutOverrides,
+ LongWithoutOverridesClass,
+ TimestampExtended,
+ TimestampOverrides
+} from './timestamp';
+export { UUIDExtended } from './uuid';
+export { SerializeOptions, DeserializeOptions };
+export {
+ Code,
+ Map,
+ BSONSymbol,
+ DBRef,
+ Binary,
+ ObjectId,
+ UUID,
+ Long,
+ Timestamp,
+ Double,
+ Int32,
+ MinKey,
+ MaxKey,
+ BSONRegExp,
+ Decimal128,
+ // In 4.0.0 and 4.0.1, this property name was changed to ObjectId to match the class name.
+ // This caused interoperability problems with previous versions of the library, so in
+ // later builds we changed it back to ObjectID (capital D) to match legacy implementations.
+ ObjectId as ObjectID
+};
+
+/** @public */
+export interface Document {
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ [key: string]: any;
+}
+
+/** @internal */
+// Default Max Size
+const MAXSIZE = 1024 * 1024 * 17;
+
+// Current Internal Temporary Serialization Buffer
+let buffer = Buffer.alloc(MAXSIZE);
+
+/**
+ * Sets the size of the internal serialization buffer.
+ *
+ * @param size - The desired size for the internal serialization buffer
+ * @public
+ */
+export function setInternalBufferSize(size: number): void {
+ // Resize the internal serialization buffer if needed
+ if (buffer.length < size) {
+ buffer = Buffer.alloc(size);
+ }
+}
+
+/**
+ * Serialize a Javascript object.
+ *
+ * @param object - the Javascript object to serialize.
+ * @returns Buffer object containing the serialized object.
+ * @public
+ */
+export function serialize(object: Document, options: SerializeOptions = {}): Buffer {
+ // Unpack the options
+ const checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ const serializeFunctions =
+ typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ const ignoreUndefined =
+ typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ const minInternalBufferSize =
+ typeof options.minInternalBufferSize === 'number' ? options.minInternalBufferSize : MAXSIZE;
+
+ // Resize the internal serialization buffer if needed
+ if (buffer.length < minInternalBufferSize) {
+ buffer = Buffer.alloc(minInternalBufferSize);
+ }
+
+ // Attempt to serialize
+ const serializationIndex = internalSerialize(
+ buffer,
+ object,
+ checkKeys,
+ 0,
+ 0,
+ serializeFunctions,
+ ignoreUndefined,
+ []
+ );
+
+ // Create the final buffer
+ const finishedBuffer = Buffer.alloc(serializationIndex);
+
+ // Copy into the finished buffer
+ buffer.copy(finishedBuffer, 0, 0, finishedBuffer.length);
+
+ // Return the buffer
+ return finishedBuffer;
+}
+
+/**
+ * Serialize a Javascript object using a predefined Buffer and index into the buffer,
+ * useful when pre-allocating the space for serialization.
+ *
+ * @param object - the Javascript object to serialize.
+ * @param finalBuffer - the Buffer you pre-allocated to store the serialized BSON object.
+ * @returns the index pointing to the last written byte in the buffer.
+ * @public
+ */
+export function serializeWithBufferAndIndex(
+ object: Document,
+ finalBuffer: Buffer,
+ options: SerializeOptions = {}
+): number {
+ // Unpack the options
+ const checkKeys = typeof options.checkKeys === 'boolean' ? options.checkKeys : false;
+ const serializeFunctions =
+ typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ const ignoreUndefined =
+ typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+ const startIndex = typeof options.index === 'number' ? options.index : 0;
+
+ // Attempt to serialize
+ const serializationIndex = internalSerialize(
+ buffer,
+ object,
+ checkKeys,
+ 0,
+ 0,
+ serializeFunctions,
+ ignoreUndefined
+ );
+ buffer.copy(finalBuffer, startIndex, 0, serializationIndex);
+
+ // Return the index
+ return startIndex + serializationIndex - 1;
+}
+
+/**
+ * Deserialize data as BSON.
+ *
+ * @param buffer - the buffer containing the serialized set of BSON documents.
+ * @returns returns the deserialized Javascript Object.
+ * @public
+ */
+export function deserialize(
+ buffer: Buffer | ArrayBufferView | ArrayBuffer,
+ options: DeserializeOptions = {}
+): Document {
+ return internalDeserialize(ensureBuffer(buffer), options);
+}
+
+/** @public */
+export type CalculateObjectSizeOptions = Pick<
+ SerializeOptions,
+ 'serializeFunctions' | 'ignoreUndefined'
+>;
+
+/**
+ * Calculate the bson size for a passed in Javascript object.
+ *
+ * @param object - the Javascript object to calculate the BSON byte size for
+ * @returns size of BSON object in bytes
+ * @public
+ */
+export function calculateObjectSize(
+ object: Document,
+ options: CalculateObjectSizeOptions = {}
+): number {
+ options = options || {};
+
+ const serializeFunctions =
+ typeof options.serializeFunctions === 'boolean' ? options.serializeFunctions : false;
+ const ignoreUndefined =
+ typeof options.ignoreUndefined === 'boolean' ? options.ignoreUndefined : true;
+
+ return internalCalculateObjectSize(object, serializeFunctions, ignoreUndefined);
+}
+
+/**
+ * Deserialize stream data as BSON documents.
+ *
+ * @param data - the buffer containing the serialized set of BSON documents.
+ * @param startIndex - the start index in the data Buffer where the deserialization is to start.
+ * @param numberOfDocuments - number of documents to deserialize.
+ * @param documents - an array where to store the deserialized documents.
+ * @param docStartIndex - the index in the documents array from where to start inserting documents.
+ * @param options - additional options used for the deserialization.
+ * @returns next index in the buffer after deserialization **x** numbers of documents.
+ * @public
+ */
+export function deserializeStream(
+ data: Buffer | ArrayBufferView | ArrayBuffer,
+ startIndex: number,
+ numberOfDocuments: number,
+ documents: Document[],
+ docStartIndex: number,
+ options: DeserializeOptions
+): number {
+ const internalOptions = Object.assign(
+ { allowObjectSmallerThanBufferSize: true, index: 0 },
+ options
+ );
+ const bufferData = ensureBuffer(data);
+
+ let index = startIndex;
+ // Loop over all documents
+ for (let i = 0; i < numberOfDocuments; i++) {
+ // Find size of the document
+ const size =
+ bufferData[index] |
+ (bufferData[index + 1] << 8) |
+ (bufferData[index + 2] << 16) |
+ (bufferData[index + 3] << 24);
+ // Update options with index
+ internalOptions.index = index;
+ // Parse the document at this point
+ documents[docStartIndex + i] = internalDeserialize(bufferData, internalOptions);
+ // Adjust index by the document size
+ index = index + size;
+ }
+
+ // Return object containing end index of parsing and list of documents
+ return index;
+}
+
+/**
+ * BSON default export
+ * @deprecated Please use named exports
+ * @privateRemarks
+ * We want to someday deprecate the default export,
+ * so none of the new TS types are being exported on the default
+ * @public
+ */
+const BSON = {
+ Binary,
+ Code,
+ DBRef,
+ Decimal128,
+ Double,
+ Int32,
+ Long,
+ UUID,
+ Map,
+ MaxKey,
+ MinKey,
+ ObjectId,
+ ObjectID: ObjectId,
+ BSONRegExp,
+ BSONSymbol,
+ Timestamp,
+ EJSON,
+ setInternalBufferSize,
+ serialize,
+ serializeWithBufferAndIndex,
+ deserialize,
+ calculateObjectSize,
+ deserializeStream
+};
+export default BSON;
diff --git a/node_modules/bson/src/code.ts b/node_modules/bson/src/code.ts
new file mode 100644
index 0000000..47d1c85
--- /dev/null
+++ b/node_modules/bson/src/code.ts
@@ -0,0 +1,61 @@
+import type { Document } from './bson';
+
+/** @public */
+export interface CodeExtended {
+ $code: string | Function;
+ $scope?: Document;
+}
+
+/**
+ * A class representation of the BSON Code type.
+ * @public
+ */
+export class Code {
+ _bsontype!: 'Code';
+
+ code!: string | Function;
+ scope?: Document;
+ /**
+ * @param code - a string or function.
+ * @param scope - an optional scope for the function.
+ */
+ constructor(code: string | Function, scope?: Document) {
+ if (!(this instanceof Code)) return new Code(code, scope);
+
+ this.code = code;
+ this.scope = scope;
+ }
+
+ /** @internal */
+ toJSON(): { code: string | Function; scope?: Document } {
+ return { code: this.code, scope: this.scope };
+ }
+
+ /** @internal */
+ toExtendedJSON(): CodeExtended {
+ if (this.scope) {
+ return { $code: this.code, $scope: this.scope };
+ }
+
+ return { $code: this.code };
+ }
+
+ /** @internal */
+ static fromExtendedJSON(doc: CodeExtended): Code {
+ return new Code(doc.$code, doc.$scope);
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ const codeJson = this.toJSON();
+ return `new Code("${codeJson.code}"${
+ codeJson.scope ? `, ${JSON.stringify(codeJson.scope)}` : ''
+ })`;
+ }
+}
+
+Object.defineProperty(Code.prototype, '_bsontype', { value: 'Code' });
diff --git a/node_modules/bson/src/constants.ts b/node_modules/bson/src/constants.ts
new file mode 100644
index 0000000..22b411e
--- /dev/null
+++ b/node_modules/bson/src/constants.ts
@@ -0,0 +1,104 @@
+/** @internal */
+export const BSON_INT32_MAX = 0x7fffffff;
+/** @internal */
+export const BSON_INT32_MIN = -0x80000000;
+/** @internal */
+export const BSON_INT64_MAX = Math.pow(2, 63) - 1;
+/** @internal */
+export const BSON_INT64_MIN = -Math.pow(2, 63);
+
+/**
+ * Any integer up to 2^53 can be precisely represented by a double.
+ * @internal
+ */
+export const JS_INT_MAX = Math.pow(2, 53);
+
+/**
+ * Any integer down to -2^53 can be precisely represented by a double.
+ * @internal
+ */
+export const JS_INT_MIN = -Math.pow(2, 53);
+
+/** Number BSON Type @internal */
+export const BSON_DATA_NUMBER = 1;
+
+/** String BSON Type @internal */
+export const BSON_DATA_STRING = 2;
+
+/** Object BSON Type @internal */
+export const BSON_DATA_OBJECT = 3;
+
+/** Array BSON Type @internal */
+export const BSON_DATA_ARRAY = 4;
+
+/** Binary BSON Type @internal */
+export const BSON_DATA_BINARY = 5;
+
+/** Binary BSON Type @internal */
+export const BSON_DATA_UNDEFINED = 6;
+
+/** ObjectId BSON Type @internal */
+export const BSON_DATA_OID = 7;
+
+/** Boolean BSON Type @internal */
+export const BSON_DATA_BOOLEAN = 8;
+
+/** Date BSON Type @internal */
+export const BSON_DATA_DATE = 9;
+
+/** null BSON Type @internal */
+export const BSON_DATA_NULL = 10;
+
+/** RegExp BSON Type @internal */
+export const BSON_DATA_REGEXP = 11;
+
+/** Code BSON Type @internal */
+export const BSON_DATA_DBPOINTER = 12;
+
+/** Code BSON Type @internal */
+export const BSON_DATA_CODE = 13;
+
+/** Symbol BSON Type @internal */
+export const BSON_DATA_SYMBOL = 14;
+
+/** Code with Scope BSON Type @internal */
+export const BSON_DATA_CODE_W_SCOPE = 15;
+
+/** 32 bit Integer BSON Type @internal */
+export const BSON_DATA_INT = 16;
+
+/** Timestamp BSON Type @internal */
+export const BSON_DATA_TIMESTAMP = 17;
+
+/** Long BSON Type @internal */
+export const BSON_DATA_LONG = 18;
+
+/** Decimal128 BSON Type @internal */
+export const BSON_DATA_DECIMAL128 = 19;
+
+/** MinKey BSON Type @internal */
+export const BSON_DATA_MIN_KEY = 0xff;
+
+/** MaxKey BSON Type @internal */
+export const BSON_DATA_MAX_KEY = 0x7f;
+
+/** Binary Default Type @internal */
+export const BSON_BINARY_SUBTYPE_DEFAULT = 0;
+
+/** Binary Function Type @internal */
+export const BSON_BINARY_SUBTYPE_FUNCTION = 1;
+
+/** Binary Byte Array Type @internal */
+export const BSON_BINARY_SUBTYPE_BYTE_ARRAY = 2;
+
+/** Binary Deprecated UUID Type @deprecated Please use BSON_BINARY_SUBTYPE_UUID_NEW @internal */
+export const BSON_BINARY_SUBTYPE_UUID = 3;
+
+/** Binary UUID Type @internal */
+export const BSON_BINARY_SUBTYPE_UUID_NEW = 4;
+
+/** Binary MD5 Type @internal */
+export const BSON_BINARY_SUBTYPE_MD5 = 5;
+
+/** Binary User Defined Type @internal */
+export const BSON_BINARY_SUBTYPE_USER_DEFINED = 128;
diff --git a/node_modules/bson/src/db_ref.ts b/node_modules/bson/src/db_ref.ts
new file mode 100644
index 0000000..53b649f
--- /dev/null
+++ b/node_modules/bson/src/db_ref.ts
@@ -0,0 +1,124 @@
+import type { Document } from './bson';
+import type { EJSONOptions } from './extended_json';
+import type { ObjectId } from './objectid';
+import { isObjectLike } from './parser/utils';
+
+/** @public */
+export interface DBRefLike {
+ $ref: string;
+ $id: ObjectId;
+ $db?: string;
+}
+
+/** @internal */
+export function isDBRefLike(value: unknown): value is DBRefLike {
+ return (
+ isObjectLike(value) &&
+ value.$id != null &&
+ typeof value.$ref === 'string' &&
+ (value.$db == null || typeof value.$db === 'string')
+ );
+}
+
+/**
+ * A class representation of the BSON DBRef type.
+ * @public
+ */
+export class DBRef {
+ _bsontype!: 'DBRef';
+
+ collection!: string;
+ oid!: ObjectId;
+ db?: string;
+ fields!: Document;
+
+ /**
+ * @param collection - the collection name.
+ * @param oid - the reference ObjectId.
+ * @param db - optional db name, if omitted the reference is local to the current db.
+ */
+ constructor(collection: string, oid: ObjectId, db?: string, fields?: Document) {
+ if (!(this instanceof DBRef)) return new DBRef(collection, oid, db, fields);
+
+ // check if namespace has been provided
+ const parts = collection.split('.');
+ if (parts.length === 2) {
+ db = parts.shift();
+ // eslint-disable-next-line @typescript-eslint/no-non-null-assertion
+ collection = parts.shift()!;
+ }
+
+ this.collection = collection;
+ this.oid = oid;
+ this.db = db;
+ this.fields = fields || {};
+ }
+
+ // Property provided for compatibility with the 1.x parser
+ // the 1.x parser used a "namespace" property, while 4.x uses "collection"
+
+ /** @internal */
+ get namespace(): string {
+ return this.collection;
+ }
+
+ set namespace(value: string) {
+ this.collection = value;
+ }
+
+ /** @internal */
+ toJSON(): DBRefLike & Document {
+ const o = Object.assign(
+ {
+ $ref: this.collection,
+ $id: this.oid
+ },
+ this.fields
+ );
+
+ if (this.db != null) o.$db = this.db;
+ return o;
+ }
+
+ /** @internal */
+ toExtendedJSON(options?: EJSONOptions): DBRefLike {
+ options = options || {};
+ let o: DBRefLike = {
+ $ref: this.collection,
+ $id: this.oid
+ };
+
+ if (options.legacy) {
+ return o;
+ }
+
+ if (this.db) o.$db = this.db;
+ o = Object.assign(o, this.fields);
+ return o;
+ }
+
+ /** @internal */
+ static fromExtendedJSON(doc: DBRefLike): DBRef {
+ const copy = Object.assign({}, doc) as Partial;
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new DBRef(doc.$ref, doc.$id, doc.$db, copy);
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ // NOTE: if OID is an ObjectId class it will just print the oid string.
+ const oid =
+ this.oid === undefined || this.oid.toString === undefined ? this.oid : this.oid.toString();
+ return `new DBRef("${this.namespace}", new ObjectId("${oid}")${
+ this.db ? `, "${this.db}"` : ''
+ })`;
+ }
+}
+
+Object.defineProperty(DBRef.prototype, '_bsontype', { value: 'DBRef' });
diff --git a/node_modules/bson/src/decimal128.ts b/node_modules/bson/src/decimal128.ts
new file mode 100644
index 0000000..d3d197d
--- /dev/null
+++ b/node_modules/bson/src/decimal128.ts
@@ -0,0 +1,810 @@
+import { Buffer } from 'buffer';
+import { Long } from './long';
+
+const PARSE_STRING_REGEXP = /^(\+|-)?(\d+|(\d*\.\d*))?(E|e)?([-+])?(\d+)?$/;
+const PARSE_INF_REGEXP = /^(\+|-)?(Infinity|inf)$/i;
+const PARSE_NAN_REGEXP = /^(\+|-)?NaN$/i;
+
+const EXPONENT_MAX = 6111;
+const EXPONENT_MIN = -6176;
+const EXPONENT_BIAS = 6176;
+const MAX_DIGITS = 34;
+
+// Nan value bits as 32 bit values (due to lack of longs)
+const NAN_BUFFER = [
+ 0x7c,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+// Infinity value bits 32 bit values (due to lack of longs)
+const INF_NEGATIVE_BUFFER = [
+ 0xf8,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+const INF_POSITIVE_BUFFER = [
+ 0x78,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00,
+ 0x00
+].reverse();
+
+const EXPONENT_REGEX = /^([-+])?(\d+)?$/;
+
+// Extract least significant 5 bits
+const COMBINATION_MASK = 0x1f;
+// Extract least significant 14 bits
+const EXPONENT_MASK = 0x3fff;
+// Value of combination field for Inf
+const COMBINATION_INFINITY = 30;
+// Value of combination field for NaN
+const COMBINATION_NAN = 31;
+
+// Detect if the value is a digit
+function isDigit(value: string): boolean {
+ return !isNaN(parseInt(value, 10));
+}
+
+// Divide two uint128 values
+function divideu128(value: { parts: [number, number, number, number] }) {
+ const DIVISOR = Long.fromNumber(1000 * 1000 * 1000);
+ let _rem = Long.fromNumber(0);
+
+ if (!value.parts[0] && !value.parts[1] && !value.parts[2] && !value.parts[3]) {
+ return { quotient: value, rem: _rem };
+ }
+
+ for (let i = 0; i <= 3; i++) {
+ // Adjust remainder to match value of next dividend
+ _rem = _rem.shiftLeft(32);
+ // Add the divided to _rem
+ _rem = _rem.add(new Long(value.parts[i], 0));
+ value.parts[i] = _rem.div(DIVISOR).low;
+ _rem = _rem.modulo(DIVISOR);
+ }
+
+ return { quotient: value, rem: _rem };
+}
+
+// Multiply two Long values and return the 128 bit value
+function multiply64x2(left: Long, right: Long): { high: Long; low: Long } {
+ if (!left && !right) {
+ return { high: Long.fromNumber(0), low: Long.fromNumber(0) };
+ }
+
+ const leftHigh = left.shiftRightUnsigned(32);
+ const leftLow = new Long(left.getLowBits(), 0);
+ const rightHigh = right.shiftRightUnsigned(32);
+ const rightLow = new Long(right.getLowBits(), 0);
+
+ let productHigh = leftHigh.multiply(rightHigh);
+ let productMid = leftHigh.multiply(rightLow);
+ const productMid2 = leftLow.multiply(rightHigh);
+ let productLow = leftLow.multiply(rightLow);
+
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productMid = new Long(productMid.getLowBits(), 0)
+ .add(productMid2)
+ .add(productLow.shiftRightUnsigned(32));
+
+ productHigh = productHigh.add(productMid.shiftRightUnsigned(32));
+ productLow = productMid.shiftLeft(32).add(new Long(productLow.getLowBits(), 0));
+
+ // Return the 128 bit result
+ return { high: productHigh, low: productLow };
+}
+
+function lessThan(left: Long, right: Long): boolean {
+ // Make values unsigned
+ const uhleft = left.high >>> 0;
+ const uhright = right.high >>> 0;
+
+ // Compare high bits first
+ if (uhleft < uhright) {
+ return true;
+ } else if (uhleft === uhright) {
+ const ulleft = left.low >>> 0;
+ const ulright = right.low >>> 0;
+ if (ulleft < ulright) return true;
+ }
+
+ return false;
+}
+
+function invalidErr(string: string, message: string) {
+ throw new TypeError(`"${string}" is not a valid Decimal128 string - ${message}`);
+}
+
+/** @public */
+export interface Decimal128Extended {
+ $numberDecimal: string;
+}
+
+/**
+ * A class representation of the BSON Decimal128 type.
+ * @public
+ */
+export class Decimal128 {
+ _bsontype!: 'Decimal128';
+
+ readonly bytes!: Buffer;
+
+ /**
+ * @param bytes - a buffer containing the raw Decimal128 bytes in little endian order,
+ * or a string representation as returned by .toString()
+ */
+ constructor(bytes: Buffer | string) {
+ if (!(this instanceof Decimal128)) return new Decimal128(bytes);
+
+ if (typeof bytes === 'string') {
+ this.bytes = Decimal128.fromString(bytes).bytes;
+ } else {
+ this.bytes = bytes;
+ }
+ }
+
+ /**
+ * Create a Decimal128 instance from a string representation
+ *
+ * @param representation - a numeric string representation.
+ */
+ static fromString(representation: string): Decimal128 {
+ // Parse state tracking
+ let isNegative = false;
+ let sawRadix = false;
+ let foundNonZero = false;
+
+ // Total number of significant digits (no leading or trailing zero)
+ let significantDigits = 0;
+ // Total number of significand digits read
+ let nDigitsRead = 0;
+ // Total number of digits (no leading zeros)
+ let nDigits = 0;
+ // The number of the digits after radix
+ let radixPosition = 0;
+ // The index of the first non-zero in *str*
+ let firstNonZero = 0;
+
+ // Digits Array
+ const digits = [0];
+ // The number of digits in digits
+ let nDigitsStored = 0;
+ // Insertion pointer for digits
+ let digitsInsert = 0;
+ // The index of the first non-zero digit
+ let firstDigit = 0;
+ // The index of the last digit
+ let lastDigit = 0;
+
+ // Exponent
+ let exponent = 0;
+ // loop index over array
+ let i = 0;
+ // The high 17 digits of the significand
+ let significandHigh = new Long(0, 0);
+ // The low 17 digits of the significand
+ let significandLow = new Long(0, 0);
+ // The biased exponent
+ let biasedExponent = 0;
+
+ // Read index
+ let index = 0;
+
+ // Naively prevent against REDOS attacks.
+ // TODO: implementing a custom parsing for this, or refactoring the regex would yield
+ // further gains.
+ if (representation.length >= 7000) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+
+ // Results
+ const stringMatch = representation.match(PARSE_STRING_REGEXP);
+ const infMatch = representation.match(PARSE_INF_REGEXP);
+ const nanMatch = representation.match(PARSE_NAN_REGEXP);
+
+ // Validate the string
+ if ((!stringMatch && !infMatch && !nanMatch) || representation.length === 0) {
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+ }
+
+ if (stringMatch) {
+ // full_match = stringMatch[0]
+ // sign = stringMatch[1]
+
+ const unsignedNumber = stringMatch[2];
+ // stringMatch[3] is undefined if a whole number (ex "1", 12")
+ // but defined if a number w/ decimal in it (ex "1.0, 12.2")
+
+ const e = stringMatch[4];
+ const expSign = stringMatch[5];
+ const expNumber = stringMatch[6];
+
+ // they provided e, but didn't give an exponent number. for ex "1e"
+ if (e && expNumber === undefined) invalidErr(representation, 'missing exponent power');
+
+ // they provided e, but didn't give a number before it. for ex "e1"
+ if (e && unsignedNumber === undefined) invalidErr(representation, 'missing exponent base');
+
+ if (e === undefined && (expSign || expNumber)) {
+ invalidErr(representation, 'missing e before exponent');
+ }
+ }
+
+ // Get the negative or positive sign
+ if (representation[index] === '+' || representation[index] === '-') {
+ isNegative = representation[index++] === '-';
+ }
+
+ // Check if user passed Infinity or NaN
+ if (!isDigit(representation[index]) && representation[index] !== '.') {
+ if (representation[index] === 'i' || representation[index] === 'I') {
+ return new Decimal128(Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER));
+ } else if (representation[index] === 'N') {
+ return new Decimal128(Buffer.from(NAN_BUFFER));
+ }
+ }
+
+ // Read all the digits
+ while (isDigit(representation[index]) || representation[index] === '.') {
+ if (representation[index] === '.') {
+ if (sawRadix) invalidErr(representation, 'contains multiple periods');
+
+ sawRadix = true;
+ index = index + 1;
+ continue;
+ }
+
+ if (nDigitsStored < 34) {
+ if (representation[index] !== '0' || foundNonZero) {
+ if (!foundNonZero) {
+ firstNonZero = nDigitsRead;
+ }
+
+ foundNonZero = true;
+
+ // Only store 34 digits
+ digits[digitsInsert++] = parseInt(representation[index], 10);
+ nDigitsStored = nDigitsStored + 1;
+ }
+ }
+
+ if (foundNonZero) nDigits = nDigits + 1;
+ if (sawRadix) radixPosition = radixPosition + 1;
+
+ nDigitsRead = nDigitsRead + 1;
+ index = index + 1;
+ }
+
+ if (sawRadix && !nDigitsRead)
+ throw new TypeError('' + representation + ' not a valid Decimal128 string');
+
+ // Read exponent if exists
+ if (representation[index] === 'e' || representation[index] === 'E') {
+ // Read exponent digits
+ const match = representation.substr(++index).match(EXPONENT_REGEX);
+
+ // No digits read
+ if (!match || !match[2]) return new Decimal128(Buffer.from(NAN_BUFFER));
+
+ // Get exponent
+ exponent = parseInt(match[0], 10);
+
+ // Adjust the index
+ index = index + match[0].length;
+ }
+
+ // Return not a number
+ if (representation[index]) return new Decimal128(Buffer.from(NAN_BUFFER));
+
+ // Done reading input
+ // Find first non-zero digit in digits
+ firstDigit = 0;
+
+ if (!nDigitsStored) {
+ firstDigit = 0;
+ lastDigit = 0;
+ digits[0] = 0;
+ nDigits = 1;
+ nDigitsStored = 1;
+ significantDigits = 0;
+ } else {
+ lastDigit = nDigitsStored - 1;
+ significantDigits = nDigits;
+ if (significantDigits !== 1) {
+ while (representation[firstNonZero + significantDigits - 1] === '0') {
+ significantDigits = significantDigits - 1;
+ }
+ }
+ }
+
+ // Normalization of exponent
+ // Correct exponent based on radix position, and shift significand as needed
+ // to represent user input
+
+ // Overflow prevention
+ if (exponent <= radixPosition && radixPosition - exponent > 1 << 14) {
+ exponent = EXPONENT_MIN;
+ } else {
+ exponent = exponent - radixPosition;
+ }
+
+ // Attempt to normalize the exponent
+ while (exponent > EXPONENT_MAX) {
+ // Shift exponent to significand and decrease
+ lastDigit = lastDigit + 1;
+
+ if (lastDigit - firstDigit > MAX_DIGITS) {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ const digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+
+ invalidErr(representation, 'overflow');
+ }
+ exponent = exponent - 1;
+ }
+
+ while (exponent < EXPONENT_MIN || nDigitsStored < nDigits) {
+ // Shift last digit. can only do this if < significant digits than # stored.
+ if (lastDigit === 0 && significantDigits < nDigitsStored) {
+ exponent = EXPONENT_MIN;
+ significantDigits = 0;
+ break;
+ }
+
+ if (nDigitsStored < nDigits) {
+ // adjust to match digits not stored
+ nDigits = nDigits - 1;
+ } else {
+ // adjust to round
+ lastDigit = lastDigit - 1;
+ }
+
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ } else {
+ // Check if we have a zero then just hard clamp, otherwise fail
+ const digitsString = digits.join('');
+ if (digitsString.match(/^0+$/)) {
+ exponent = EXPONENT_MAX;
+ break;
+ }
+ invalidErr(representation, 'overflow');
+ }
+ }
+
+ // Round
+ // We've normalized the exponent, but might still need to round.
+ if (lastDigit - firstDigit + 1 < significantDigits) {
+ let endOfString = nDigitsRead;
+
+ // If we have seen a radix point, 'string' is 1 longer than we have
+ // documented with ndigits_read, so inc the position of the first nonzero
+ // digit and the position that digits are read to.
+ if (sawRadix) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+ // if negative, we need to increment again to account for - sign at start.
+ if (isNegative) {
+ firstNonZero = firstNonZero + 1;
+ endOfString = endOfString + 1;
+ }
+
+ const roundDigit = parseInt(representation[firstNonZero + lastDigit + 1], 10);
+ let roundBit = 0;
+
+ if (roundDigit >= 5) {
+ roundBit = 1;
+ if (roundDigit === 5) {
+ roundBit = digits[lastDigit] % 2 === 1 ? 1 : 0;
+ for (i = firstNonZero + lastDigit + 2; i < endOfString; i++) {
+ if (parseInt(representation[i], 10)) {
+ roundBit = 1;
+ break;
+ }
+ }
+ }
+ }
+
+ if (roundBit) {
+ let dIdx = lastDigit;
+
+ for (; dIdx >= 0; dIdx--) {
+ if (++digits[dIdx] > 9) {
+ digits[dIdx] = 0;
+
+ // overflowed most significant digit
+ if (dIdx === 0) {
+ if (exponent < EXPONENT_MAX) {
+ exponent = exponent + 1;
+ digits[dIdx] = 1;
+ } else {
+ return new Decimal128(
+ Buffer.from(isNegative ? INF_NEGATIVE_BUFFER : INF_POSITIVE_BUFFER)
+ );
+ }
+ }
+ }
+ }
+ }
+ }
+
+ // Encode significand
+ // The high 17 digits of the significand
+ significandHigh = Long.fromNumber(0);
+ // The low 17 digits of the significand
+ significandLow = Long.fromNumber(0);
+
+ // read a zero
+ if (significantDigits === 0) {
+ significandHigh = Long.fromNumber(0);
+ significandLow = Long.fromNumber(0);
+ } else if (lastDigit - firstDigit < 17) {
+ let dIdx = firstDigit;
+ significandLow = Long.fromNumber(digits[dIdx++]);
+ significandHigh = new Long(0, 0);
+
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(Long.fromNumber(10));
+ significandLow = significandLow.add(Long.fromNumber(digits[dIdx]));
+ }
+ } else {
+ let dIdx = firstDigit;
+ significandHigh = Long.fromNumber(digits[dIdx++]);
+
+ for (; dIdx <= lastDigit - 17; dIdx++) {
+ significandHigh = significandHigh.multiply(Long.fromNumber(10));
+ significandHigh = significandHigh.add(Long.fromNumber(digits[dIdx]));
+ }
+
+ significandLow = Long.fromNumber(digits[dIdx++]);
+
+ for (; dIdx <= lastDigit; dIdx++) {
+ significandLow = significandLow.multiply(Long.fromNumber(10));
+ significandLow = significandLow.add(Long.fromNumber(digits[dIdx]));
+ }
+ }
+
+ const significand = multiply64x2(significandHigh, Long.fromString('100000000000000000'));
+ significand.low = significand.low.add(significandLow);
+
+ if (lessThan(significand.low, significandLow)) {
+ significand.high = significand.high.add(Long.fromNumber(1));
+ }
+
+ // Biased exponent
+ biasedExponent = exponent + EXPONENT_BIAS;
+ const dec = { low: Long.fromNumber(0), high: Long.fromNumber(0) };
+
+ // Encode combination, exponent, and significand.
+ if (
+ significand.high.shiftRightUnsigned(49).and(Long.fromNumber(1)).equals(Long.fromNumber(1))
+ ) {
+ // Encode '11' into bits 1 to 3
+ dec.high = dec.high.or(Long.fromNumber(0x3).shiftLeft(61));
+ dec.high = dec.high.or(
+ Long.fromNumber(biasedExponent).and(Long.fromNumber(0x3fff).shiftLeft(47))
+ );
+ dec.high = dec.high.or(significand.high.and(Long.fromNumber(0x7fffffffffff)));
+ } else {
+ dec.high = dec.high.or(Long.fromNumber(biasedExponent & 0x3fff).shiftLeft(49));
+ dec.high = dec.high.or(significand.high.and(Long.fromNumber(0x1ffffffffffff)));
+ }
+
+ dec.low = significand.low;
+
+ // Encode sign
+ if (isNegative) {
+ dec.high = dec.high.or(Long.fromString('9223372036854775808'));
+ }
+
+ // Encode into a buffer
+ const buffer = Buffer.alloc(16);
+ index = 0;
+
+ // Encode the low 64 bits of the decimal
+ // Encode low bits
+ buffer[index++] = dec.low.low & 0xff;
+ buffer[index++] = (dec.low.low >> 8) & 0xff;
+ buffer[index++] = (dec.low.low >> 16) & 0xff;
+ buffer[index++] = (dec.low.low >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = dec.low.high & 0xff;
+ buffer[index++] = (dec.low.high >> 8) & 0xff;
+ buffer[index++] = (dec.low.high >> 16) & 0xff;
+ buffer[index++] = (dec.low.high >> 24) & 0xff;
+
+ // Encode the high 64 bits of the decimal
+ // Encode low bits
+ buffer[index++] = dec.high.low & 0xff;
+ buffer[index++] = (dec.high.low >> 8) & 0xff;
+ buffer[index++] = (dec.high.low >> 16) & 0xff;
+ buffer[index++] = (dec.high.low >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = dec.high.high & 0xff;
+ buffer[index++] = (dec.high.high >> 8) & 0xff;
+ buffer[index++] = (dec.high.high >> 16) & 0xff;
+ buffer[index++] = (dec.high.high >> 24) & 0xff;
+
+ // Return the new Decimal128
+ return new Decimal128(buffer);
+ }
+
+ /** Create a string representation of the raw Decimal128 value */
+ toString(): string {
+ // Note: bits in this routine are referred to starting at 0,
+ // from the sign bit, towards the coefficient.
+
+ // decoded biased exponent (14 bits)
+ let biased_exponent;
+ // the number of significand digits
+ let significand_digits = 0;
+ // the base-10 digits in the significand
+ const significand = new Array(36);
+ for (let i = 0; i < significand.length; i++) significand[i] = 0;
+ // read pointer into significand
+ let index = 0;
+
+ // true if the number is zero
+ let is_zero = false;
+
+ // the most significant significand bits (50-46)
+ let significand_msb;
+ // temporary storage for significand decoding
+ let significand128: { parts: [number, number, number, number] } = { parts: [0, 0, 0, 0] };
+ // indexing variables
+ let j, k;
+
+ // Output string
+ const string: string[] = [];
+
+ // Unpack index
+ index = 0;
+
+ // Buffer reference
+ const buffer = this.bytes;
+
+ // Unpack the low 64bits into a long
+ // bits 96 - 127
+ const low =
+ buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 64 - 95
+ const midl =
+ buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+
+ // Unpack the high 64bits into a long
+ // bits 32 - 63
+ const midh =
+ buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+ // bits 0 - 31
+ const high =
+ buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+
+ // Unpack index
+ index = 0;
+
+ // Create the state of the decimal
+ const dec = {
+ low: new Long(low, midl),
+ high: new Long(midh, high)
+ };
+
+ if (dec.high.lessThan(Long.ZERO)) {
+ string.push('-');
+ }
+
+ // Decode combination field and exponent
+ // bits 1 - 5
+ const combination = (high >> 26) & COMBINATION_MASK;
+
+ if (combination >> 3 === 3) {
+ // Check for 'special' values
+ if (combination === COMBINATION_INFINITY) {
+ return string.join('') + 'Infinity';
+ } else if (combination === COMBINATION_NAN) {
+ return 'NaN';
+ } else {
+ biased_exponent = (high >> 15) & EXPONENT_MASK;
+ significand_msb = 0x08 + ((high >> 14) & 0x01);
+ }
+ } else {
+ significand_msb = (high >> 14) & 0x07;
+ biased_exponent = (high >> 17) & EXPONENT_MASK;
+ }
+
+ // unbiased exponent
+ const exponent = biased_exponent - EXPONENT_BIAS;
+
+ // Create string of significand digits
+
+ // Convert the 114-bit binary number represented by
+ // (significand_high, significand_low) to at most 34 decimal
+ // digits through modulo and division.
+ significand128.parts[0] = (high & 0x3fff) + ((significand_msb & 0xf) << 14);
+ significand128.parts[1] = midh;
+ significand128.parts[2] = midl;
+ significand128.parts[3] = low;
+
+ if (
+ significand128.parts[0] === 0 &&
+ significand128.parts[1] === 0 &&
+ significand128.parts[2] === 0 &&
+ significand128.parts[3] === 0
+ ) {
+ is_zero = true;
+ } else {
+ for (k = 3; k >= 0; k--) {
+ let least_digits = 0;
+ // Perform the divide
+ const result = divideu128(significand128);
+ significand128 = result.quotient;
+ least_digits = result.rem.low;
+
+ // We now have the 9 least significant digits (in base 2).
+ // Convert and output to string.
+ if (!least_digits) continue;
+
+ for (j = 8; j >= 0; j--) {
+ // significand[k * 9 + j] = Math.round(least_digits % 10);
+ significand[k * 9 + j] = least_digits % 10;
+ // least_digits = Math.round(least_digits / 10);
+ least_digits = Math.floor(least_digits / 10);
+ }
+ }
+ }
+
+ // Output format options:
+ // Scientific - [-]d.dddE(+/-)dd or [-]dE(+/-)dd
+ // Regular - ddd.ddd
+
+ if (is_zero) {
+ significand_digits = 1;
+ significand[index] = 0;
+ } else {
+ significand_digits = 36;
+ while (!significand[index]) {
+ significand_digits = significand_digits - 1;
+ index = index + 1;
+ }
+ }
+
+ // the exponent if scientific notation is used
+ const scientific_exponent = significand_digits - 1 + exponent;
+
+ // The scientific exponent checks are dictated by the string conversion
+ // specification and are somewhat arbitrary cutoffs.
+ //
+ // We must check exponent > 0, because if this is the case, the number
+ // has trailing zeros. However, we *cannot* output these trailing zeros,
+ // because doing so would change the precision of the value, and would
+ // change stored data if the string converted number is round tripped.
+ if (scientific_exponent >= 34 || scientific_exponent <= -7 || exponent > 0) {
+ // Scientific format
+
+ // if there are too many significant digits, we should just be treating numbers
+ // as + or - 0 and using the non-scientific exponent (this is for the "invalid
+ // representation should be treated as 0/-0" spec cases in decimal128-1.json)
+ if (significand_digits > 34) {
+ string.push(`${0}`);
+ if (exponent > 0) string.push('E+' + exponent);
+ else if (exponent < 0) string.push('E' + exponent);
+ return string.join('');
+ }
+
+ string.push(`${significand[index++]}`);
+ significand_digits = significand_digits - 1;
+
+ if (significand_digits) {
+ string.push('.');
+ }
+
+ for (let i = 0; i < significand_digits; i++) {
+ string.push(`${significand[index++]}`);
+ }
+
+ // Exponent
+ string.push('E');
+ if (scientific_exponent > 0) {
+ string.push('+' + scientific_exponent);
+ } else {
+ string.push(`${scientific_exponent}`);
+ }
+ } else {
+ // Regular format with no decimal place
+ if (exponent >= 0) {
+ for (let i = 0; i < significand_digits; i++) {
+ string.push(`${significand[index++]}`);
+ }
+ } else {
+ let radix_position = significand_digits + exponent;
+
+ // non-zero digits before radix
+ if (radix_position > 0) {
+ for (let i = 0; i < radix_position; i++) {
+ string.push(`${significand[index++]}`);
+ }
+ } else {
+ string.push('0');
+ }
+
+ string.push('.');
+ // add leading zeros after radix
+ while (radix_position++ < 0) {
+ string.push('0');
+ }
+
+ for (let i = 0; i < significand_digits - Math.max(radix_position - 1, 0); i++) {
+ string.push(`${significand[index++]}`);
+ }
+ }
+ }
+
+ return string.join('');
+ }
+
+ toJSON(): Decimal128Extended {
+ return { $numberDecimal: this.toString() };
+ }
+
+ /** @internal */
+ toExtendedJSON(): Decimal128Extended {
+ return { $numberDecimal: this.toString() };
+ }
+
+ /** @internal */
+ static fromExtendedJSON(doc: Decimal128Extended): Decimal128 {
+ return Decimal128.fromString(doc.$numberDecimal);
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ return `new Decimal128("${this.toString()}")`;
+ }
+}
+
+Object.defineProperty(Decimal128.prototype, '_bsontype', { value: 'Decimal128' });
diff --git a/node_modules/bson/src/double.ts b/node_modules/bson/src/double.ts
new file mode 100644
index 0000000..f55543c
--- /dev/null
+++ b/node_modules/bson/src/double.ts
@@ -0,0 +1,87 @@
+import type { EJSONOptions } from './extended_json';
+
+/** @public */
+export interface DoubleExtended {
+ $numberDouble: string;
+}
+
+/**
+ * A class representation of the BSON Double type.
+ * @public
+ */
+export class Double {
+ _bsontype!: 'Double';
+
+ value!: number;
+ /**
+ * Create a Double type
+ *
+ * @param value - the number we want to represent as a double.
+ */
+ constructor(value: number) {
+ if (!(this instanceof Double)) return new Double(value);
+
+ if ((value as unknown) instanceof Number) {
+ value = value.valueOf();
+ }
+
+ this.value = +value;
+ }
+
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped double number.
+ */
+ valueOf(): number {
+ return this.value;
+ }
+
+ /** @internal */
+ toJSON(): number {
+ return this.value;
+ }
+
+ /** @internal */
+ toExtendedJSON(options?: EJSONOptions): number | DoubleExtended {
+ if (options && (options.legacy || (options.relaxed && isFinite(this.value)))) {
+ return this.value;
+ }
+
+ // NOTE: JavaScript has +0 and -0, apparently to model limit calculations. If a user
+ // explicitly provided `-0` then we need to ensure the sign makes it into the output
+ if (Object.is(Math.sign(this.value), -0)) {
+ return { $numberDouble: `-${this.value.toFixed(1)}` };
+ }
+
+ let $numberDouble: string;
+ if (Number.isInteger(this.value)) {
+ $numberDouble = this.value.toFixed(1);
+ if ($numberDouble.length >= 13) {
+ $numberDouble = this.value.toExponential(13).toUpperCase();
+ }
+ } else {
+ $numberDouble = this.value.toString();
+ }
+
+ return { $numberDouble };
+ }
+
+ /** @internal */
+ static fromExtendedJSON(doc: DoubleExtended, options?: EJSONOptions): number | Double {
+ const doubleValue = parseFloat(doc.$numberDouble);
+ return options && options.relaxed ? doubleValue : new Double(doubleValue);
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ const eJSON = this.toExtendedJSON() as DoubleExtended;
+ return `new Double(${eJSON.$numberDouble})`;
+ }
+}
+
+Object.defineProperty(Double.prototype, '_bsontype', { value: 'Double' });
diff --git a/node_modules/bson/src/ensure_buffer.ts b/node_modules/bson/src/ensure_buffer.ts
new file mode 100644
index 0000000..62e0dde
--- /dev/null
+++ b/node_modules/bson/src/ensure_buffer.ts
@@ -0,0 +1,26 @@
+import { Buffer } from 'buffer';
+import { isAnyArrayBuffer } from './parser/utils';
+
+/**
+ * Makes sure that, if a Uint8Array is passed in, it is wrapped in a Buffer.
+ *
+ * @param potentialBuffer - The potential buffer
+ * @returns Buffer the input if potentialBuffer is a buffer, or a buffer that
+ * wraps a passed in Uint8Array
+ * @throws TypeError If anything other than a Buffer or Uint8Array is passed in
+ */
+export function ensureBuffer(potentialBuffer: Buffer | ArrayBufferView | ArrayBuffer): Buffer {
+ if (ArrayBuffer.isView(potentialBuffer)) {
+ return Buffer.from(
+ potentialBuffer.buffer,
+ potentialBuffer.byteOffset,
+ potentialBuffer.byteLength
+ );
+ }
+
+ if (isAnyArrayBuffer(potentialBuffer)) {
+ return Buffer.from(potentialBuffer);
+ }
+
+ throw new TypeError('Must use either Buffer or TypedArray');
+}
diff --git a/node_modules/bson/src/extended_json.ts b/node_modules/bson/src/extended_json.ts
new file mode 100644
index 0000000..a8fe192
--- /dev/null
+++ b/node_modules/bson/src/extended_json.ts
@@ -0,0 +1,443 @@
+import { Binary } from './binary';
+import type { Document } from './bson';
+import { Code } from './code';
+import { DBRef, isDBRefLike } from './db_ref';
+import { Decimal128 } from './decimal128';
+import { Double } from './double';
+import { Int32 } from './int_32';
+import { Long } from './long';
+import { MaxKey } from './max_key';
+import { MinKey } from './min_key';
+import { ObjectId } from './objectid';
+import { isDate, isObjectLike, isRegExp } from './parser/utils';
+import { BSONRegExp } from './regexp';
+import { BSONSymbol } from './symbol';
+import { Timestamp } from './timestamp';
+
+/** @public */
+export type EJSONOptions = EJSON.Options;
+
+/** @internal */
+type BSONType =
+ | Binary
+ | Code
+ | DBRef
+ | Decimal128
+ | Double
+ | Int32
+ | Long
+ | MaxKey
+ | MinKey
+ | ObjectId
+ | BSONRegExp
+ | BSONSymbol
+ | Timestamp;
+
+export function isBSONType(value: unknown): value is BSONType {
+ return (
+ isObjectLike(value) && Reflect.has(value, '_bsontype') && typeof value._bsontype === 'string'
+ );
+}
+
+// INT32 boundaries
+const BSON_INT32_MAX = 0x7fffffff;
+const BSON_INT32_MIN = -0x80000000;
+// INT64 boundaries
+const BSON_INT64_MAX = 0x7fffffffffffffff;
+const BSON_INT64_MIN = -0x8000000000000000;
+
+// all the types where we don't need to do any special processing and can just pass the EJSON
+//straight to type.fromExtendedJSON
+const keysToCodecs = {
+ $oid: ObjectId,
+ $binary: Binary,
+ $uuid: Binary,
+ $symbol: BSONSymbol,
+ $numberInt: Int32,
+ $numberDecimal: Decimal128,
+ $numberDouble: Double,
+ $numberLong: Long,
+ $minKey: MinKey,
+ $maxKey: MaxKey,
+ $regex: BSONRegExp,
+ $regularExpression: BSONRegExp,
+ $timestamp: Timestamp
+} as const;
+
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function deserializeValue(value: any, options: EJSON.Options = {}) {
+ if (typeof value === 'number') {
+ if (options.relaxed || options.legacy) {
+ return value;
+ }
+
+ // if it's an integer, should interpret as smallest BSON integer
+ // that can represent it exactly. (if out of range, interpret as double.)
+ if (Math.floor(value) === value) {
+ if (value >= BSON_INT32_MIN && value <= BSON_INT32_MAX) return new Int32(value);
+ if (value >= BSON_INT64_MIN && value <= BSON_INT64_MAX) return Long.fromNumber(value);
+ }
+
+ // If the number is a non-integer or out of integer range, should interpret as BSON Double.
+ return new Double(value);
+ }
+
+ // from here on out we're looking for bson types, so bail if its not an object
+ if (value == null || typeof value !== 'object') return value;
+
+ // upgrade deprecated undefined to null
+ if (value.$undefined) return null;
+
+ const keys = Object.keys(value).filter(
+ k => k.startsWith('$') && value[k] != null
+ ) as (keyof typeof keysToCodecs)[];
+ for (let i = 0; i < keys.length; i++) {
+ const c = keysToCodecs[keys[i]];
+ if (c) return c.fromExtendedJSON(value, options);
+ }
+
+ if (value.$date != null) {
+ const d = value.$date;
+ const date = new Date();
+
+ if (options.legacy) {
+ if (typeof d === 'number') date.setTime(d);
+ else if (typeof d === 'string') date.setTime(Date.parse(d));
+ } else {
+ if (typeof d === 'string') date.setTime(Date.parse(d));
+ else if (Long.isLong(d)) date.setTime(d.toNumber());
+ else if (typeof d === 'number' && options.relaxed) date.setTime(d);
+ }
+ return date;
+ }
+
+ if (value.$code != null) {
+ const copy = Object.assign({}, value);
+ if (value.$scope) {
+ copy.$scope = deserializeValue(value.$scope);
+ }
+
+ return Code.fromExtendedJSON(value);
+ }
+
+ if (isDBRefLike(value) || value.$dbPointer) {
+ const v = value.$ref ? value : value.$dbPointer;
+
+ // we run into this in a "degenerate EJSON" case (with $id and $ref order flipped)
+ // because of the order JSON.parse goes through the document
+ if (v instanceof DBRef) return v;
+
+ const dollarKeys = Object.keys(v).filter(k => k.startsWith('$'));
+ let valid = true;
+ dollarKeys.forEach(k => {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1) valid = false;
+ });
+
+ // only make DBRef if $ keys are all valid
+ if (valid) return DBRef.fromExtendedJSON(v);
+ }
+
+ return value;
+}
+
+type EJSONSerializeOptions = EJSON.Options & {
+ seenObjects: { obj: unknown; propertyName: string }[];
+};
+
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeArray(array: any[], options: EJSONSerializeOptions): any[] {
+ return array.map((v: unknown, index: number) => {
+ options.seenObjects.push({ propertyName: `index ${index}`, obj: null });
+ try {
+ return serializeValue(v, options);
+ } finally {
+ options.seenObjects.pop();
+ }
+ });
+}
+
+function getISOString(date: Date) {
+ const isoStr = date.toISOString();
+ // we should only show milliseconds in timestamp if they're non-zero
+ return date.getUTCMilliseconds() !== 0 ? isoStr : isoStr.slice(0, -5) + 'Z';
+}
+
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeValue(value: any, options: EJSONSerializeOptions): any {
+ if ((typeof value === 'object' || typeof value === 'function') && value !== null) {
+ const index = options.seenObjects.findIndex(entry => entry.obj === value);
+ if (index !== -1) {
+ const props = options.seenObjects.map(entry => entry.propertyName);
+ const leadingPart = props
+ .slice(0, index)
+ .map(prop => `${prop} -> `)
+ .join('');
+ const alreadySeen = props[index];
+ const circularPart =
+ ' -> ' +
+ props
+ .slice(index + 1, props.length - 1)
+ .map(prop => `${prop} -> `)
+ .join('');
+ const current = props[props.length - 1];
+ const leadingSpace = ' '.repeat(leadingPart.length + alreadySeen.length / 2);
+ const dashes = '-'.repeat(
+ circularPart.length + (alreadySeen.length + current.length) / 2 - 1
+ );
+
+ throw new TypeError(
+ 'Converting circular structure to EJSON:\n' +
+ ` ${leadingPart}${alreadySeen}${circularPart}${current}\n` +
+ ` ${leadingSpace}\\${dashes}/`
+ );
+ }
+ options.seenObjects[options.seenObjects.length - 1].obj = value;
+ }
+
+ if (Array.isArray(value)) return serializeArray(value, options);
+
+ if (value === undefined) return null;
+
+ if (value instanceof Date || isDate(value)) {
+ const dateNum = value.getTime(),
+ // is it in year range 1970-9999?
+ inRange = dateNum > -1 && dateNum < 253402318800000;
+
+ if (options.legacy) {
+ return options.relaxed && inRange
+ ? { $date: value.getTime() }
+ : { $date: getISOString(value) };
+ }
+ return options.relaxed && inRange
+ ? { $date: getISOString(value) }
+ : { $date: { $numberLong: value.getTime().toString() } };
+ }
+
+ if (typeof value === 'number' && (!options.relaxed || !isFinite(value))) {
+ // it's an integer
+ if (Math.floor(value) === value) {
+ const int32Range = value >= BSON_INT32_MIN && value <= BSON_INT32_MAX,
+ int64Range = value >= BSON_INT64_MIN && value <= BSON_INT64_MAX;
+
+ // interpret as being of the smallest BSON integer type that can represent the number exactly
+ if (int32Range) return { $numberInt: value.toString() };
+ if (int64Range) return { $numberLong: value.toString() };
+ }
+ return { $numberDouble: value.toString() };
+ }
+
+ if (value instanceof RegExp || isRegExp(value)) {
+ let flags = value.flags;
+ if (flags === undefined) {
+ const match = value.toString().match(/[gimuy]*$/);
+ if (match) {
+ flags = match[0];
+ }
+ }
+
+ const rx = new BSONRegExp(value.source, flags);
+ return rx.toExtendedJSON(options);
+ }
+
+ if (value != null && typeof value === 'object') return serializeDocument(value, options);
+ return value;
+}
+
+const BSON_TYPE_MAPPINGS = {
+ Binary: (o: Binary) => new Binary(o.value(), o.sub_type),
+ Code: (o: Code) => new Code(o.code, o.scope),
+ DBRef: (o: DBRef) => new DBRef(o.collection || o.namespace, o.oid, o.db, o.fields), // "namespace" for 1.x library backwards compat
+ Decimal128: (o: Decimal128) => new Decimal128(o.bytes),
+ Double: (o: Double) => new Double(o.value),
+ Int32: (o: Int32) => new Int32(o.value),
+ Long: (
+ o: Long & {
+ low_: number;
+ high_: number;
+ unsigned_: boolean | undefined;
+ }
+ ) =>
+ Long.fromBits(
+ // underscore variants for 1.x backwards compatibility
+ o.low != null ? o.low : o.low_,
+ o.low != null ? o.high : o.high_,
+ o.low != null ? o.unsigned : o.unsigned_
+ ),
+ MaxKey: () => new MaxKey(),
+ MinKey: () => new MinKey(),
+ ObjectID: (o: ObjectId) => new ObjectId(o),
+ ObjectId: (o: ObjectId) => new ObjectId(o), // support 4.0.0/4.0.1 before _bsontype was reverted back to ObjectID
+ BSONRegExp: (o: BSONRegExp) => new BSONRegExp(o.pattern, o.options),
+ Symbol: (o: BSONSymbol) => new BSONSymbol(o.value),
+ Timestamp: (o: Timestamp) => Timestamp.fromBits(o.low, o.high)
+} as const;
+
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+function serializeDocument(doc: any, options: EJSONSerializeOptions) {
+ if (doc == null || typeof doc !== 'object') throw new Error('not an object instance');
+
+ const bsontype: BSONType['_bsontype'] = doc._bsontype;
+ if (typeof bsontype === 'undefined') {
+ // It's a regular object. Recursively serialize its property values.
+ const _doc: Document = {};
+ for (const name in doc) {
+ options.seenObjects.push({ propertyName: name, obj: null });
+ try {
+ _doc[name] = serializeValue(doc[name], options);
+ } finally {
+ options.seenObjects.pop();
+ }
+ }
+ return _doc;
+ } else if (isBSONType(doc)) {
+ // the "document" is really just a BSON type object
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ let outDoc: any = doc;
+ if (typeof outDoc.toExtendedJSON !== 'function') {
+ // There's no EJSON serialization function on the object. It's probably an
+ // object created by a previous version of this library (or another library)
+ // that's duck-typing objects to look like they were generated by this library).
+ // Copy the object into this library's version of that type.
+ const mapper = BSON_TYPE_MAPPINGS[doc._bsontype];
+ if (!mapper) {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + doc._bsontype);
+ }
+ outDoc = mapper(outDoc);
+ }
+
+ // Two BSON types may have nested objects that may need to be serialized too
+ if (bsontype === 'Code' && outDoc.scope) {
+ outDoc = new Code(outDoc.code, serializeValue(outDoc.scope, options));
+ } else if (bsontype === 'DBRef' && outDoc.oid) {
+ outDoc = new DBRef(
+ serializeValue(outDoc.collection, options),
+ serializeValue(outDoc.oid, options),
+ serializeValue(outDoc.db, options),
+ serializeValue(outDoc.fields, options)
+ );
+ }
+
+ return outDoc.toExtendedJSON(options);
+ } else {
+ throw new Error('_bsontype must be a string, but was: ' + typeof bsontype);
+ }
+}
+
+/**
+ * EJSON parse / stringify API
+ * @public
+ */
+// the namespace here is used to emulate `export * as EJSON from '...'`
+// which as of now (sept 2020) api-extractor does not support
+// eslint-disable-next-line @typescript-eslint/no-namespace
+export namespace EJSON {
+ export interface Options {
+ /** Output using the Extended JSON v1 spec */
+ legacy?: boolean;
+ /** Enable Extended JSON's `relaxed` mode, which attempts to return native JS types where possible, rather than BSON types */
+ relaxed?: boolean;
+ /**
+ * Disable Extended JSON's `relaxed` mode, which attempts to return BSON types where possible, rather than native JS types
+ * @deprecated Please use the relaxed property instead
+ */
+ strict?: boolean;
+ }
+
+ /**
+ * Parse an Extended JSON string, constructing the JavaScript value or object described by that
+ * string.
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const text = '{ "int32": { "$numberInt": "10" } }';
+ *
+ * // prints { int32: { [String: '10'] _bsontype: 'Int32', value: '10' } }
+ * console.log(EJSON.parse(text, { relaxed: false }));
+ *
+ * // prints { int32: 10 }
+ * console.log(EJSON.parse(text));
+ * ```
+ */
+ export function parse(text: string, options?: EJSON.Options): SerializableTypes {
+ const finalOptions = Object.assign({}, { relaxed: true, legacy: false }, options);
+
+ // relaxed implies not strict
+ if (typeof finalOptions.relaxed === 'boolean') finalOptions.strict = !finalOptions.relaxed;
+ if (typeof finalOptions.strict === 'boolean') finalOptions.relaxed = !finalOptions.strict;
+
+ return JSON.parse(text, (_key, value) => deserializeValue(value, finalOptions));
+ }
+
+ export type JSONPrimitive = string | number | boolean | null;
+ export type SerializableTypes = Document | Array | JSONPrimitive;
+
+ /**
+ * Converts a BSON document to an Extended JSON string, optionally replacing values if a replacer
+ * function is specified or optionally including only the specified properties if a replacer array
+ * is specified.
+ *
+ * @param value - The value to convert to extended JSON
+ * @param replacer - A function that alters the behavior of the stringification process, or an array of String and Number objects that serve as a whitelist for selecting/filtering the properties of the value object to be included in the JSON string. If this value is null or not provided, all properties of the object are included in the resulting JSON string
+ * @param space - A String or Number object that's used to insert white space into the output JSON string for readability purposes.
+ * @param options - Optional settings
+ *
+ * @example
+ * ```js
+ * const { EJSON } = require('bson');
+ * const Int32 = require('mongodb').Int32;
+ * const doc = { int32: new Int32(10) };
+ *
+ * // prints '{"int32":{"$numberInt":"10"}}'
+ * console.log(EJSON.stringify(doc, { relaxed: false }));
+ *
+ * // prints '{"int32":10}'
+ * console.log(EJSON.stringify(doc));
+ * ```
+ */
+ export function stringify(
+ value: SerializableTypes,
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ replacer?: (number | string)[] | ((this: any, key: string, value: any) => any) | EJSON.Options,
+ space?: string | number,
+ options?: EJSON.Options
+ ): string {
+ if (space != null && typeof space === 'object') {
+ options = space;
+ space = 0;
+ }
+ if (replacer != null && typeof replacer === 'object' && !Array.isArray(replacer)) {
+ options = replacer;
+ replacer = undefined;
+ space = 0;
+ }
+ const serializeOptions = Object.assign({ relaxed: true, legacy: false }, options, {
+ seenObjects: [{ propertyName: '(root)', obj: null }]
+ });
+
+ const doc = serializeValue(value, serializeOptions);
+ return JSON.stringify(doc, replacer as Parameters[1], space);
+ }
+
+ /**
+ * Serializes an object to an Extended JSON string, and reparse it as a JavaScript object.
+ *
+ * @param value - The object to serialize
+ * @param options - Optional settings passed to the `stringify` function
+ */
+ export function serialize(value: SerializableTypes, options?: EJSON.Options): Document {
+ options = options || {};
+ return JSON.parse(stringify(value, options));
+ }
+
+ /**
+ * Deserializes an Extended JSON object into a plain JavaScript object with native/BSON types
+ *
+ * @param ejson - The Extended JSON object to deserialize
+ * @param options - Optional settings passed to the parse method
+ */
+ export function deserialize(ejson: Document, options?: EJSON.Options): SerializableTypes {
+ options = options || {};
+ return parse(JSON.stringify(ejson), options);
+ }
+}
diff --git a/node_modules/bson/src/float_parser.ts b/node_modules/bson/src/float_parser.ts
new file mode 100644
index 0000000..c881f4c
--- /dev/null
+++ b/node_modules/bson/src/float_parser.ts
@@ -0,0 +1,152 @@
+// Copyright (c) 2008, Fair Oaks Labs, Inc.
+// All rights reserved.
+//
+// Redistribution and use in source and binary forms, with or without
+// modification, are permitted provided that the following conditions are met:
+//
+// * Redistributions of source code must retain the above copyright notice,
+// this list of conditions and the following disclaimer.
+//
+// * Redistributions in binary form must reproduce the above copyright notice,
+// this list of conditions and the following disclaimer in the documentation
+// and/or other materials provided with the distribution.
+//
+// * Neither the name of Fair Oaks Labs, Inc. nor the names of its contributors
+// may be used to endorse or promote products derived from this software
+// without specific prior written permission.
+//
+// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+// AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
+// ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
+// LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
+// CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
+// SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
+// INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
+// CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
+// ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
+// POSSIBILITY OF SUCH DAMAGE.
+//
+//
+// Modifications to writeIEEE754 to support negative zeroes made by Brian White
+
+type NumericalSequence = { [index: number]: number };
+
+export function readIEEE754(
+ buffer: NumericalSequence,
+ offset: number,
+ endian: 'big' | 'little',
+ mLen: number,
+ nBytes: number
+): number {
+ let e: number;
+ let m: number;
+ const bBE = endian === 'big';
+ const eLen = nBytes * 8 - mLen - 1;
+ const eMax = (1 << eLen) - 1;
+ const eBias = eMax >> 1;
+ let nBits = -7;
+ let i = bBE ? 0 : nBytes - 1;
+ const d = bBE ? 1 : -1;
+ let s = buffer[offset + i];
+
+ i += d;
+
+ e = s & ((1 << -nBits) - 1);
+ s >>= -nBits;
+ nBits += eLen;
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8);
+
+ m = e & ((1 << -nBits) - 1);
+ e >>= -nBits;
+ nBits += mLen;
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8);
+
+ if (e === 0) {
+ e = 1 - eBias;
+ } else if (e === eMax) {
+ return m ? NaN : (s ? -1 : 1) * Infinity;
+ } else {
+ m = m + Math.pow(2, mLen);
+ e = e - eBias;
+ }
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen);
+}
+
+export function writeIEEE754(
+ buffer: NumericalSequence,
+ value: number,
+ offset: number,
+ endian: 'big' | 'little',
+ mLen: number,
+ nBytes: number
+): void {
+ let e: number;
+ let m: number;
+ let c: number;
+ const bBE = endian === 'big';
+ let eLen = nBytes * 8 - mLen - 1;
+ const eMax = (1 << eLen) - 1;
+ const eBias = eMax >> 1;
+ const rt = mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0;
+ let i = bBE ? nBytes - 1 : 0;
+ const d = bBE ? -1 : 1;
+ const s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0;
+
+ value = Math.abs(value);
+
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0;
+ e = eMax;
+ } else {
+ e = Math.floor(Math.log(value) / Math.LN2);
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--;
+ c *= 2;
+ }
+ if (e + eBias >= 1) {
+ value += rt / c;
+ } else {
+ value += rt * Math.pow(2, 1 - eBias);
+ }
+ if (value * c >= 2) {
+ e++;
+ c /= 2;
+ }
+
+ if (e + eBias >= eMax) {
+ m = 0;
+ e = eMax;
+ } else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen);
+ e = e + eBias;
+ } else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen);
+ e = 0;
+ }
+ }
+
+ if (isNaN(value)) m = 0;
+
+ while (mLen >= 8) {
+ buffer[offset + i] = m & 0xff;
+ i += d;
+ m /= 256;
+ mLen -= 8;
+ }
+
+ e = (e << mLen) | m;
+
+ if (isNaN(value)) e += 8;
+
+ eLen += mLen;
+
+ while (eLen > 0) {
+ buffer[offset + i] = e & 0xff;
+ i += d;
+ e /= 256;
+ eLen -= 8;
+ }
+
+ buffer[offset + i - d] |= s * 128;
+}
diff --git a/node_modules/bson/src/int_32.ts b/node_modules/bson/src/int_32.ts
new file mode 100644
index 0000000..887def2
--- /dev/null
+++ b/node_modules/bson/src/int_32.ts
@@ -0,0 +1,66 @@
+import type { EJSONOptions } from './extended_json';
+
+/** @public */
+export interface Int32Extended {
+ $numberInt: string;
+}
+
+/**
+ * A class representation of a BSON Int32 type.
+ * @public
+ */
+export class Int32 {
+ _bsontype!: 'Int32';
+
+ value!: number;
+ /**
+ * Create an Int32 type
+ *
+ * @param value - the number we want to represent as an int32.
+ */
+ constructor(value: number | string) {
+ if (!(this instanceof Int32)) return new Int32(value);
+
+ if ((value as unknown) instanceof Number) {
+ value = value.valueOf();
+ }
+
+ this.value = +value;
+ }
+
+ /**
+ * Access the number value.
+ *
+ * @returns returns the wrapped int32 number.
+ */
+ valueOf(): number {
+ return this.value;
+ }
+
+ /** @internal */
+ toJSON(): number {
+ return this.value;
+ }
+
+ /** @internal */
+ toExtendedJSON(options?: EJSONOptions): number | Int32Extended {
+ if (options && (options.relaxed || options.legacy)) return this.value;
+ return { $numberInt: this.value.toString() };
+ }
+
+ /** @internal */
+ static fromExtendedJSON(doc: Int32Extended, options?: EJSONOptions): number | Int32 {
+ return options && options.relaxed ? parseInt(doc.$numberInt, 10) : new Int32(doc.$numberInt);
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ return `new Int32(${this.valueOf()})`;
+ }
+}
+
+Object.defineProperty(Int32.prototype, '_bsontype', { value: 'Int32' });
diff --git a/node_modules/bson/src/long.ts b/node_modules/bson/src/long.ts
new file mode 100644
index 0000000..977a9d3
--- /dev/null
+++ b/node_modules/bson/src/long.ts
@@ -0,0 +1,1014 @@
+import type { EJSONOptions } from './extended_json';
+import { isObjectLike } from './parser/utils';
+import type { Timestamp } from './timestamp';
+
+interface LongWASMHelpers {
+ /** Gets the high bits of the last operation performed */
+ get_high(): number;
+ div_u(lowBits: number, highBits: number, lowBitsDivisor: number, highBitsDivisor: number): number;
+ div_s(lowBits: number, highBits: number, lowBitsDivisor: number, highBitsDivisor: number): number;
+ rem_u(lowBits: number, highBits: number, lowBitsDivisor: number, highBitsDivisor: number): number;
+ rem_s(lowBits: number, highBits: number, lowBitsDivisor: number, highBitsDivisor: number): number;
+ mul(
+ lowBits: number,
+ highBits: number,
+ lowBitsMultiplier: number,
+ highBitsMultiplier: number
+ ): number;
+}
+
+/**
+ * wasm optimizations, to do native i64 multiplication and divide
+ */
+let wasm: LongWASMHelpers | undefined = undefined;
+
+/* We do not want to have to include DOM types just for this check */
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+declare const WebAssembly: any;
+
+try {
+ wasm = (new WebAssembly.Instance(
+ new WebAssembly.Module(
+ // prettier-ignore
+ new Uint8Array([0, 97, 115, 109, 1, 0, 0, 0, 1, 13, 2, 96, 0, 1, 127, 96, 4, 127, 127, 127, 127, 1, 127, 3, 7, 6, 0, 1, 1, 1, 1, 1, 6, 6, 1, 127, 1, 65, 0, 11, 7, 50, 6, 3, 109, 117, 108, 0, 1, 5, 100, 105, 118, 95, 115, 0, 2, 5, 100, 105, 118, 95, 117, 0, 3, 5, 114, 101, 109, 95, 115, 0, 4, 5, 114, 101, 109, 95, 117, 0, 5, 8, 103, 101, 116, 95, 104, 105, 103, 104, 0, 0, 10, 191, 1, 6, 4, 0, 35, 0, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 126, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 127, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 128, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 129, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 130, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11])
+ ),
+ {}
+ ).exports as unknown) as LongWASMHelpers;
+} catch {
+ // no wasm support
+}
+
+const TWO_PWR_16_DBL = 1 << 16;
+const TWO_PWR_24_DBL = 1 << 24;
+const TWO_PWR_32_DBL = TWO_PWR_16_DBL * TWO_PWR_16_DBL;
+const TWO_PWR_64_DBL = TWO_PWR_32_DBL * TWO_PWR_32_DBL;
+const TWO_PWR_63_DBL = TWO_PWR_64_DBL / 2;
+
+/** A cache of the Long representations of small integer values. */
+const INT_CACHE: { [key: number]: Long } = {};
+
+/** A cache of the Long representations of small unsigned integer values. */
+const UINT_CACHE: { [key: number]: Long } = {};
+
+/** @public */
+export interface LongExtended {
+ $numberLong: string;
+}
+
+/**
+ * A class representing a 64-bit integer
+ * @public
+ * @remarks
+ * The internal representation of a long is the two given signed, 32-bit values.
+ * We use 32-bit pieces because these are the size of integers on which
+ * Javascript performs bit-operations. For operations like addition and
+ * multiplication, we split each number into 16 bit pieces, which can easily be
+ * multiplied within Javascript's floating-point representation without overflow
+ * or change in sign.
+ * In the algorithms below, we frequently reduce the negative case to the
+ * positive case by negating the input(s) and then post-processing the result.
+ * Note that we must ALWAYS check specially whether those values are MIN_VALUE
+ * (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as
+ * a positive number, it overflows back into a negative). Not handling this
+ * case would often result in infinite recursion.
+ * Common constant values ZERO, ONE, NEG_ONE, etc. are found as static properties on this class.
+ */
+export class Long {
+ _bsontype!: 'Long';
+
+ /** An indicator used to reliably determine if an object is a Long or not. */
+ __isLong__!: true;
+
+ /**
+ * The high 32 bits as a signed value.
+ */
+ high!: number;
+
+ /**
+ * The low 32 bits as a signed value.
+ */
+ low!: number;
+
+ /**
+ * Whether unsigned or not.
+ */
+ unsigned!: boolean;
+
+ /**
+ * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers.
+ * See the from* functions below for more convenient ways of constructing Longs.
+ *
+ * Acceptable signatures are:
+ * - Long(low, high, unsigned?)
+ * - Long(bigint, unsigned?)
+ * - Long(string, unsigned?)
+ *
+ * @param low - The low (signed) 32 bits of the long
+ * @param high - The high (signed) 32 bits of the long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ constructor(low: number | bigint | string = 0, high?: number | boolean, unsigned?: boolean) {
+ if (!(this instanceof Long)) return new Long(low, high, unsigned);
+
+ if (typeof low === 'bigint') {
+ Object.assign(this, Long.fromBigInt(low, !!high));
+ } else if (typeof low === 'string') {
+ Object.assign(this, Long.fromString(low, !!high));
+ } else {
+ this.low = low | 0;
+ this.high = (high as number) | 0;
+ this.unsigned = !!unsigned;
+ }
+
+ Object.defineProperty(this, '__isLong__', {
+ value: true,
+ configurable: false,
+ writable: false,
+ enumerable: false
+ });
+ }
+
+ static TWO_PWR_24 = Long.fromInt(TWO_PWR_24_DBL);
+
+ /** Maximum unsigned value. */
+ static MAX_UNSIGNED_VALUE = Long.fromBits(0xffffffff | 0, 0xffffffff | 0, true);
+ /** Signed zero */
+ static ZERO = Long.fromInt(0);
+ /** Unsigned zero. */
+ static UZERO = Long.fromInt(0, true);
+ /** Signed one. */
+ static ONE = Long.fromInt(1);
+ /** Unsigned one. */
+ static UONE = Long.fromInt(1, true);
+ /** Signed negative one. */
+ static NEG_ONE = Long.fromInt(-1);
+ /** Maximum signed value. */
+ static MAX_VALUE = Long.fromBits(0xffffffff | 0, 0x7fffffff | 0, false);
+ /** Minimum signed value. */
+ static MIN_VALUE = Long.fromBits(0, 0x80000000 | 0, false);
+
+ /**
+ * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits.
+ * Each is assumed to use 32 bits.
+ * @param lowBits - The low 32 bits
+ * @param highBits - The high 32 bits
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromBits(lowBits: number, highBits: number, unsigned?: boolean): Long {
+ return new Long(lowBits, highBits, unsigned);
+ }
+
+ /**
+ * Returns a Long representing the given 32 bit integer value.
+ * @param value - The 32 bit integer in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromInt(value: number, unsigned?: boolean): Long {
+ let obj, cachedObj, cache;
+ if (unsigned) {
+ value >>>= 0;
+ if ((cache = 0 <= value && value < 256)) {
+ cachedObj = UINT_CACHE[value];
+ if (cachedObj) return cachedObj;
+ }
+ obj = Long.fromBits(value, (value | 0) < 0 ? -1 : 0, true);
+ if (cache) UINT_CACHE[value] = obj;
+ return obj;
+ } else {
+ value |= 0;
+ if ((cache = -128 <= value && value < 128)) {
+ cachedObj = INT_CACHE[value];
+ if (cachedObj) return cachedObj;
+ }
+ obj = Long.fromBits(value, value < 0 ? -1 : 0, false);
+ if (cache) INT_CACHE[value] = obj;
+ return obj;
+ }
+ }
+
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromNumber(value: number, unsigned?: boolean): Long {
+ if (isNaN(value)) return unsigned ? Long.UZERO : Long.ZERO;
+ if (unsigned) {
+ if (value < 0) return Long.UZERO;
+ if (value >= TWO_PWR_64_DBL) return Long.MAX_UNSIGNED_VALUE;
+ } else {
+ if (value <= -TWO_PWR_63_DBL) return Long.MIN_VALUE;
+ if (value + 1 >= TWO_PWR_63_DBL) return Long.MAX_VALUE;
+ }
+ if (value < 0) return Long.fromNumber(-value, unsigned).neg();
+ return Long.fromBits(value % TWO_PWR_32_DBL | 0, (value / TWO_PWR_32_DBL) | 0, unsigned);
+ }
+
+ /**
+ * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.
+ * @param value - The number in question
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromBigInt(value: bigint, unsigned?: boolean): Long {
+ return Long.fromString(value.toString(), unsigned);
+ }
+
+ /**
+ * Returns a Long representation of the given string, written using the specified radix.
+ * @param str - The textual representation of the Long
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param radix - The radix in which the text is written (2-36), defaults to 10
+ * @returns The corresponding Long value
+ */
+ static fromString(str: string, unsigned?: boolean, radix?: number): Long {
+ if (str.length === 0) throw Error('empty string');
+ if (str === 'NaN' || str === 'Infinity' || str === '+Infinity' || str === '-Infinity')
+ return Long.ZERO;
+ if (typeof unsigned === 'number') {
+ // For goog.math.long compatibility
+ (radix = unsigned), (unsigned = false);
+ } else {
+ unsigned = !!unsigned;
+ }
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix) throw RangeError('radix');
+
+ let p;
+ if ((p = str.indexOf('-')) > 0) throw Error('interior hyphen');
+ else if (p === 0) {
+ return Long.fromString(str.substring(1), unsigned, radix).neg();
+ }
+
+ // Do several (8) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ const radixToPower = Long.fromNumber(Math.pow(radix, 8));
+
+ let result = Long.ZERO;
+ for (let i = 0; i < str.length; i += 8) {
+ const size = Math.min(8, str.length - i),
+ value = parseInt(str.substring(i, i + size), radix);
+ if (size < 8) {
+ const power = Long.fromNumber(Math.pow(radix, size));
+ result = result.mul(power).add(Long.fromNumber(value));
+ } else {
+ result = result.mul(radixToPower);
+ result = result.add(Long.fromNumber(value));
+ }
+ }
+ result.unsigned = unsigned;
+ return result;
+ }
+
+ /**
+ * Creates a Long from its byte representation.
+ * @param bytes - Byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns The corresponding Long value
+ */
+ static fromBytes(bytes: number[], unsigned?: boolean, le?: boolean): Long {
+ return le ? Long.fromBytesLE(bytes, unsigned) : Long.fromBytesBE(bytes, unsigned);
+ }
+
+ /**
+ * Creates a Long from its little endian byte representation.
+ * @param bytes - Little endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromBytesLE(bytes: number[], unsigned?: boolean): Long {
+ return new Long(
+ bytes[0] | (bytes[1] << 8) | (bytes[2] << 16) | (bytes[3] << 24),
+ bytes[4] | (bytes[5] << 8) | (bytes[6] << 16) | (bytes[7] << 24),
+ unsigned
+ );
+ }
+
+ /**
+ * Creates a Long from its big endian byte representation.
+ * @param bytes - Big endian byte representation
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ * @returns The corresponding Long value
+ */
+ static fromBytesBE(bytes: number[], unsigned?: boolean): Long {
+ return new Long(
+ (bytes[4] << 24) | (bytes[5] << 16) | (bytes[6] << 8) | bytes[7],
+ (bytes[0] << 24) | (bytes[1] << 16) | (bytes[2] << 8) | bytes[3],
+ unsigned
+ );
+ }
+
+ /**
+ * Tests if the specified object is a Long.
+ */
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any, @typescript-eslint/explicit-module-boundary-types
+ static isLong(value: any): value is Long {
+ return isObjectLike(value) && value['__isLong__'] === true;
+ }
+
+ /**
+ * Converts the specified value to a Long.
+ * @param unsigned - Whether unsigned or not, defaults to signed
+ */
+ static fromValue(
+ val: number | string | { low: number; high: number; unsigned?: boolean },
+ unsigned?: boolean
+ ): Long {
+ if (typeof val === 'number') return Long.fromNumber(val, unsigned);
+ if (typeof val === 'string') return Long.fromString(val, unsigned);
+ // Throws for non-objects, converts non-instanceof Long:
+ return Long.fromBits(
+ val.low,
+ val.high,
+ typeof unsigned === 'boolean' ? unsigned : val.unsigned
+ );
+ }
+
+ /** Returns the sum of this and the specified Long. */
+ add(addend: string | number | Long | Timestamp): Long {
+ if (!Long.isLong(addend)) addend = Long.fromValue(addend);
+
+ // Divide each number into 4 chunks of 16 bits, and then sum the chunks.
+
+ const a48 = this.high >>> 16;
+ const a32 = this.high & 0xffff;
+ const a16 = this.low >>> 16;
+ const a00 = this.low & 0xffff;
+
+ const b48 = addend.high >>> 16;
+ const b32 = addend.high & 0xffff;
+ const b16 = addend.low >>> 16;
+ const b00 = addend.low & 0xffff;
+
+ let c48 = 0,
+ c32 = 0,
+ c16 = 0,
+ c00 = 0;
+ c00 += a00 + b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 + b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 + b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 + b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ }
+
+ /**
+ * Returns the sum of this and the specified Long.
+ * @returns Sum
+ */
+ and(other: string | number | Long | Timestamp): Long {
+ if (!Long.isLong(other)) other = Long.fromValue(other);
+ return Long.fromBits(this.low & other.low, this.high & other.high, this.unsigned);
+ }
+
+ /**
+ * Compares this Long's value with the specified's.
+ * @returns 0 if they are the same, 1 if the this is greater and -1 if the given one is greater
+ */
+ compare(other: string | number | Long | Timestamp): 0 | 1 | -1 {
+ if (!Long.isLong(other)) other = Long.fromValue(other);
+ if (this.eq(other)) return 0;
+ const thisNeg = this.isNegative(),
+ otherNeg = other.isNegative();
+ if (thisNeg && !otherNeg) return -1;
+ if (!thisNeg && otherNeg) return 1;
+ // At this point the sign bits are the same
+ if (!this.unsigned) return this.sub(other).isNegative() ? -1 : 1;
+ // Both are positive if at least one is unsigned
+ return other.high >>> 0 > this.high >>> 0 ||
+ (other.high === this.high && other.low >>> 0 > this.low >>> 0)
+ ? -1
+ : 1;
+ }
+
+ /** This is an alias of {@link Long.compare} */
+ comp(other: string | number | Long | Timestamp): 0 | 1 | -1 {
+ return this.compare(other);
+ }
+
+ /**
+ * Returns this Long divided by the specified. The result is signed if this Long is signed or unsigned if this Long is unsigned.
+ * @returns Quotient
+ */
+ divide(divisor: string | number | Long | Timestamp): Long {
+ if (!Long.isLong(divisor)) divisor = Long.fromValue(divisor);
+ if (divisor.isZero()) throw Error('division by zero');
+
+ // use wasm support if present
+ if (wasm) {
+ // guard against signed division overflow: the largest
+ // negative number / -1 would be 1 larger than the largest
+ // positive number, due to two's complement.
+ if (
+ !this.unsigned &&
+ this.high === -0x80000000 &&
+ divisor.low === -1 &&
+ divisor.high === -1
+ ) {
+ // be consistent with non-wasm code path
+ return this;
+ }
+ const low = (this.unsigned ? wasm.div_u : wasm.div_s)(
+ this.low,
+ this.high,
+ divisor.low,
+ divisor.high
+ );
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+
+ if (this.isZero()) return this.unsigned ? Long.UZERO : Long.ZERO;
+ let approx, rem, res;
+ if (!this.unsigned) {
+ // This section is only relevant for signed longs and is derived from the
+ // closure library as a whole.
+ if (this.eq(Long.MIN_VALUE)) {
+ if (divisor.eq(Long.ONE) || divisor.eq(Long.NEG_ONE)) return Long.MIN_VALUE;
+ // recall that -MIN_VALUE == MIN_VALUE
+ else if (divisor.eq(Long.MIN_VALUE)) return Long.ONE;
+ else {
+ // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.
+ const halfThis = this.shr(1);
+ approx = halfThis.div(divisor).shl(1);
+ if (approx.eq(Long.ZERO)) {
+ return divisor.isNegative() ? Long.ONE : Long.NEG_ONE;
+ } else {
+ rem = this.sub(divisor.mul(approx));
+ res = approx.add(rem.div(divisor));
+ return res;
+ }
+ }
+ } else if (divisor.eq(Long.MIN_VALUE)) return this.unsigned ? Long.UZERO : Long.ZERO;
+ if (this.isNegative()) {
+ if (divisor.isNegative()) return this.neg().div(divisor.neg());
+ return this.neg().div(divisor).neg();
+ } else if (divisor.isNegative()) return this.div(divisor.neg()).neg();
+ res = Long.ZERO;
+ } else {
+ // The algorithm below has not been made for unsigned longs. It's therefore
+ // required to take special care of the MSB prior to running it.
+ if (!divisor.unsigned) divisor = divisor.toUnsigned();
+ if (divisor.gt(this)) return Long.UZERO;
+ if (divisor.gt(this.shru(1)))
+ // 15 >>> 1 = 7 ; with divisor = 8 ; true
+ return Long.UONE;
+ res = Long.UZERO;
+ }
+
+ // Repeat the following until the remainder is less than other: find a
+ // floating-point that approximates remainder / other *from below*, add this
+ // into the result, and subtract it from the remainder. It is critical that
+ // the approximate value is less than or equal to the real value so that the
+ // remainder never becomes negative.
+ rem = this;
+ while (rem.gte(divisor)) {
+ // Approximate the result of division. This may be a little greater or
+ // smaller than the actual value.
+ approx = Math.max(1, Math.floor(rem.toNumber() / divisor.toNumber()));
+
+ // We will tweak the approximate result by changing it in the 48-th digit or
+ // the smallest non-fractional digit, whichever is larger.
+ const log2 = Math.ceil(Math.log(approx) / Math.LN2);
+ const delta = log2 <= 48 ? 1 : Math.pow(2, log2 - 48);
+ // Decrease the approximation until it is smaller than the remainder. Note
+ // that if it is too large, the product overflows and is negative.
+ let approxRes = Long.fromNumber(approx);
+ let approxRem = approxRes.mul(divisor);
+ while (approxRem.isNegative() || approxRem.gt(rem)) {
+ approx -= delta;
+ approxRes = Long.fromNumber(approx, this.unsigned);
+ approxRem = approxRes.mul(divisor);
+ }
+
+ // We know the answer can't be zero... and actually, zero would cause
+ // infinite recursion since we would make no progress.
+ if (approxRes.isZero()) approxRes = Long.ONE;
+
+ res = res.add(approxRes);
+ rem = rem.sub(approxRem);
+ }
+ return res;
+ }
+
+ /**This is an alias of {@link Long.divide} */
+ div(divisor: string | number | Long | Timestamp): Long {
+ return this.divide(divisor);
+ }
+
+ /**
+ * Tests if this Long's value equals the specified's.
+ * @param other - Other value
+ */
+ equals(other: string | number | Long | Timestamp): boolean {
+ if (!Long.isLong(other)) other = Long.fromValue(other);
+ if (this.unsigned !== other.unsigned && this.high >>> 31 === 1 && other.high >>> 31 === 1)
+ return false;
+ return this.high === other.high && this.low === other.low;
+ }
+
+ /** This is an alias of {@link Long.equals} */
+ eq(other: string | number | Long | Timestamp): boolean {
+ return this.equals(other);
+ }
+
+ /** Gets the high 32 bits as a signed integer. */
+ getHighBits(): number {
+ return this.high;
+ }
+
+ /** Gets the high 32 bits as an unsigned integer. */
+ getHighBitsUnsigned(): number {
+ return this.high >>> 0;
+ }
+
+ /** Gets the low 32 bits as a signed integer. */
+ getLowBits(): number {
+ return this.low;
+ }
+
+ /** Gets the low 32 bits as an unsigned integer. */
+ getLowBitsUnsigned(): number {
+ return this.low >>> 0;
+ }
+
+ /** Gets the number of bits needed to represent the absolute value of this Long. */
+ getNumBitsAbs(): number {
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ return this.eq(Long.MIN_VALUE) ? 64 : this.neg().getNumBitsAbs();
+ }
+ const val = this.high !== 0 ? this.high : this.low;
+ let bit: number;
+ for (bit = 31; bit > 0; bit--) if ((val & (1 << bit)) !== 0) break;
+ return this.high !== 0 ? bit + 33 : bit + 1;
+ }
+
+ /** Tests if this Long's value is greater than the specified's. */
+ greaterThan(other: string | number | Long | Timestamp): boolean {
+ return this.comp(other) > 0;
+ }
+
+ /** This is an alias of {@link Long.greaterThan} */
+ gt(other: string | number | Long | Timestamp): boolean {
+ return this.greaterThan(other);
+ }
+
+ /** Tests if this Long's value is greater than or equal the specified's. */
+ greaterThanOrEqual(other: string | number | Long | Timestamp): boolean {
+ return this.comp(other) >= 0;
+ }
+
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ gte(other: string | number | Long | Timestamp): boolean {
+ return this.greaterThanOrEqual(other);
+ }
+ /** This is an alias of {@link Long.greaterThanOrEqual} */
+ ge(other: string | number | Long | Timestamp): boolean {
+ return this.greaterThanOrEqual(other);
+ }
+
+ /** Tests if this Long's value is even. */
+ isEven(): boolean {
+ return (this.low & 1) === 0;
+ }
+
+ /** Tests if this Long's value is negative. */
+ isNegative(): boolean {
+ return !this.unsigned && this.high < 0;
+ }
+
+ /** Tests if this Long's value is odd. */
+ isOdd(): boolean {
+ return (this.low & 1) === 1;
+ }
+
+ /** Tests if this Long's value is positive. */
+ isPositive(): boolean {
+ return this.unsigned || this.high >= 0;
+ }
+
+ /** Tests if this Long's value equals zero. */
+ isZero(): boolean {
+ return this.high === 0 && this.low === 0;
+ }
+
+ /** Tests if this Long's value is less than the specified's. */
+ lessThan(other: string | number | Long | Timestamp): boolean {
+ return this.comp(other) < 0;
+ }
+
+ /** This is an alias of {@link Long#lessThan}. */
+ lt(other: string | number | Long | Timestamp): boolean {
+ return this.lessThan(other);
+ }
+
+ /** Tests if this Long's value is less than or equal the specified's. */
+ lessThanOrEqual(other: string | number | Long | Timestamp): boolean {
+ return this.comp(other) <= 0;
+ }
+
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ lte(other: string | number | Long | Timestamp): boolean {
+ return this.lessThanOrEqual(other);
+ }
+
+ /** Returns this Long modulo the specified. */
+ modulo(divisor: string | number | Long | Timestamp): Long {
+ if (!Long.isLong(divisor)) divisor = Long.fromValue(divisor);
+
+ // use wasm support if present
+ if (wasm) {
+ const low = (this.unsigned ? wasm.rem_u : wasm.rem_s)(
+ this.low,
+ this.high,
+ divisor.low,
+ divisor.high
+ );
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+
+ return this.sub(this.div(divisor).mul(divisor));
+ }
+
+ /** This is an alias of {@link Long.modulo} */
+ mod(divisor: string | number | Long | Timestamp): Long {
+ return this.modulo(divisor);
+ }
+ /** This is an alias of {@link Long.modulo} */
+ rem(divisor: string | number | Long | Timestamp): Long {
+ return this.modulo(divisor);
+ }
+
+ /**
+ * Returns the product of this and the specified Long.
+ * @param multiplier - Multiplier
+ * @returns Product
+ */
+ multiply(multiplier: string | number | Long | Timestamp): Long {
+ if (this.isZero()) return Long.ZERO;
+ if (!Long.isLong(multiplier)) multiplier = Long.fromValue(multiplier);
+
+ // use wasm support if present
+ if (wasm) {
+ const low = wasm.mul(this.low, this.high, multiplier.low, multiplier.high);
+ return Long.fromBits(low, wasm.get_high(), this.unsigned);
+ }
+
+ if (multiplier.isZero()) return Long.ZERO;
+ if (this.eq(Long.MIN_VALUE)) return multiplier.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+ if (multiplier.eq(Long.MIN_VALUE)) return this.isOdd() ? Long.MIN_VALUE : Long.ZERO;
+
+ if (this.isNegative()) {
+ if (multiplier.isNegative()) return this.neg().mul(multiplier.neg());
+ else return this.neg().mul(multiplier).neg();
+ } else if (multiplier.isNegative()) return this.mul(multiplier.neg()).neg();
+
+ // If both longs are small, use float multiplication
+ if (this.lt(Long.TWO_PWR_24) && multiplier.lt(Long.TWO_PWR_24))
+ return Long.fromNumber(this.toNumber() * multiplier.toNumber(), this.unsigned);
+
+ // Divide each long into 4 chunks of 16 bits, and then add up 4x4 products.
+ // We can skip products that would overflow.
+
+ const a48 = this.high >>> 16;
+ const a32 = this.high & 0xffff;
+ const a16 = this.low >>> 16;
+ const a00 = this.low & 0xffff;
+
+ const b48 = multiplier.high >>> 16;
+ const b32 = multiplier.high & 0xffff;
+ const b16 = multiplier.low >>> 16;
+ const b00 = multiplier.low & 0xffff;
+
+ let c48 = 0,
+ c32 = 0,
+ c16 = 0,
+ c00 = 0;
+ c00 += a00 * b00;
+ c16 += c00 >>> 16;
+ c00 &= 0xffff;
+ c16 += a16 * b00;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c16 += a00 * b16;
+ c32 += c16 >>> 16;
+ c16 &= 0xffff;
+ c32 += a32 * b00;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a16 * b16;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c32 += a00 * b32;
+ c48 += c32 >>> 16;
+ c32 &= 0xffff;
+ c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;
+ c48 &= 0xffff;
+ return Long.fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);
+ }
+
+ /** This is an alias of {@link Long.multiply} */
+ mul(multiplier: string | number | Long | Timestamp): Long {
+ return this.multiply(multiplier);
+ }
+
+ /** Returns the Negation of this Long's value. */
+ negate(): Long {
+ if (!this.unsigned && this.eq(Long.MIN_VALUE)) return Long.MIN_VALUE;
+ return this.not().add(Long.ONE);
+ }
+
+ /** This is an alias of {@link Long.negate} */
+ neg(): Long {
+ return this.negate();
+ }
+
+ /** Returns the bitwise NOT of this Long. */
+ not(): Long {
+ return Long.fromBits(~this.low, ~this.high, this.unsigned);
+ }
+
+ /** Tests if this Long's value differs from the specified's. */
+ notEquals(other: string | number | Long | Timestamp): boolean {
+ return !this.equals(other);
+ }
+
+ /** This is an alias of {@link Long.notEquals} */
+ neq(other: string | number | Long | Timestamp): boolean {
+ return this.notEquals(other);
+ }
+ /** This is an alias of {@link Long.notEquals} */
+ ne(other: string | number | Long | Timestamp): boolean {
+ return this.notEquals(other);
+ }
+
+ /**
+ * Returns the bitwise OR of this Long and the specified.
+ */
+ or(other: number | string | Long): Long {
+ if (!Long.isLong(other)) other = Long.fromValue(other);
+ return Long.fromBits(this.low | other.low, this.high | other.high, this.unsigned);
+ }
+
+ /**
+ * Returns this Long with bits shifted to the left by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ shiftLeft(numBits: number | Long): Long {
+ if (Long.isLong(numBits)) numBits = numBits.toInt();
+ if ((numBits &= 63) === 0) return this;
+ else if (numBits < 32)
+ return Long.fromBits(
+ this.low << numBits,
+ (this.high << numBits) | (this.low >>> (32 - numBits)),
+ this.unsigned
+ );
+ else return Long.fromBits(0, this.low << (numBits - 32), this.unsigned);
+ }
+
+ /** This is an alias of {@link Long.shiftLeft} */
+ shl(numBits: number | Long): Long {
+ return this.shiftLeft(numBits);
+ }
+
+ /**
+ * Returns this Long with bits arithmetically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ shiftRight(numBits: number | Long): Long {
+ if (Long.isLong(numBits)) numBits = numBits.toInt();
+ if ((numBits &= 63) === 0) return this;
+ else if (numBits < 32)
+ return Long.fromBits(
+ (this.low >>> numBits) | (this.high << (32 - numBits)),
+ this.high >> numBits,
+ this.unsigned
+ );
+ else return Long.fromBits(this.high >> (numBits - 32), this.high >= 0 ? 0 : -1, this.unsigned);
+ }
+
+ /** This is an alias of {@link Long.shiftRight} */
+ shr(numBits: number | Long): Long {
+ return this.shiftRight(numBits);
+ }
+
+ /**
+ * Returns this Long with bits logically shifted to the right by the given amount.
+ * @param numBits - Number of bits
+ * @returns Shifted Long
+ */
+ shiftRightUnsigned(numBits: Long | number): Long {
+ if (Long.isLong(numBits)) numBits = numBits.toInt();
+ numBits &= 63;
+ if (numBits === 0) return this;
+ else {
+ const high = this.high;
+ if (numBits < 32) {
+ const low = this.low;
+ return Long.fromBits(
+ (low >>> numBits) | (high << (32 - numBits)),
+ high >>> numBits,
+ this.unsigned
+ );
+ } else if (numBits === 32) return Long.fromBits(high, 0, this.unsigned);
+ else return Long.fromBits(high >>> (numBits - 32), 0, this.unsigned);
+ }
+ }
+
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ shr_u(numBits: number | Long): Long {
+ return this.shiftRightUnsigned(numBits);
+ }
+ /** This is an alias of {@link Long.shiftRightUnsigned} */
+ shru(numBits: number | Long): Long {
+ return this.shiftRightUnsigned(numBits);
+ }
+
+ /**
+ * Returns the difference of this and the specified Long.
+ * @param subtrahend - Subtrahend
+ * @returns Difference
+ */
+ subtract(subtrahend: string | number | Long | Timestamp): Long {
+ if (!Long.isLong(subtrahend)) subtrahend = Long.fromValue(subtrahend);
+ return this.add(subtrahend.neg());
+ }
+
+ /** This is an alias of {@link Long.subtract} */
+ sub(subtrahend: string | number | Long | Timestamp): Long {
+ return this.subtract(subtrahend);
+ }
+
+ /** Converts the Long to a 32 bit integer, assuming it is a 32 bit integer. */
+ toInt(): number {
+ return this.unsigned ? this.low >>> 0 : this.low;
+ }
+
+ /** Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa). */
+ toNumber(): number {
+ if (this.unsigned) return (this.high >>> 0) * TWO_PWR_32_DBL + (this.low >>> 0);
+ return this.high * TWO_PWR_32_DBL + (this.low >>> 0);
+ }
+
+ /** Converts the Long to a BigInt (arbitrary precision). */
+ toBigInt(): bigint {
+ return BigInt(this.toString());
+ }
+
+ /**
+ * Converts this Long to its byte representation.
+ * @param le - Whether little or big endian, defaults to big endian
+ * @returns Byte representation
+ */
+ toBytes(le?: boolean): number[] {
+ return le ? this.toBytesLE() : this.toBytesBE();
+ }
+
+ /**
+ * Converts this Long to its little endian byte representation.
+ * @returns Little endian byte representation
+ */
+ toBytesLE(): number[] {
+ const hi = this.high,
+ lo = this.low;
+ return [
+ lo & 0xff,
+ (lo >>> 8) & 0xff,
+ (lo >>> 16) & 0xff,
+ lo >>> 24,
+ hi & 0xff,
+ (hi >>> 8) & 0xff,
+ (hi >>> 16) & 0xff,
+ hi >>> 24
+ ];
+ }
+
+ /**
+ * Converts this Long to its big endian byte representation.
+ * @returns Big endian byte representation
+ */
+ toBytesBE(): number[] {
+ const hi = this.high,
+ lo = this.low;
+ return [
+ hi >>> 24,
+ (hi >>> 16) & 0xff,
+ (hi >>> 8) & 0xff,
+ hi & 0xff,
+ lo >>> 24,
+ (lo >>> 16) & 0xff,
+ (lo >>> 8) & 0xff,
+ lo & 0xff
+ ];
+ }
+
+ /**
+ * Converts this Long to signed.
+ */
+ toSigned(): Long {
+ if (!this.unsigned) return this;
+ return Long.fromBits(this.low, this.high, false);
+ }
+
+ /**
+ * Converts the Long to a string written in the specified radix.
+ * @param radix - Radix (2-36), defaults to 10
+ * @throws RangeError If `radix` is out of range
+ */
+ toString(radix?: number): string {
+ radix = radix || 10;
+ if (radix < 2 || 36 < radix) throw RangeError('radix');
+ if (this.isZero()) return '0';
+ if (this.isNegative()) {
+ // Unsigned Longs are never negative
+ if (this.eq(Long.MIN_VALUE)) {
+ // We need to change the Long value before it can be negated, so we remove
+ // the bottom-most digit in this base and then recurse to do the rest.
+ const radixLong = Long.fromNumber(radix),
+ div = this.div(radixLong),
+ rem1 = div.mul(radixLong).sub(this);
+ return div.toString(radix) + rem1.toInt().toString(radix);
+ } else return '-' + this.neg().toString(radix);
+ }
+
+ // Do several (6) digits each time through the loop, so as to
+ // minimize the calls to the very expensive emulated div.
+ const radixToPower = Long.fromNumber(Math.pow(radix, 6), this.unsigned);
+ // eslint-disable-next-line @typescript-eslint/no-this-alias
+ let rem: Long = this;
+ let result = '';
+ // eslint-disable-next-line no-constant-condition
+ while (true) {
+ const remDiv = rem.div(radixToPower);
+ const intval = rem.sub(remDiv.mul(radixToPower)).toInt() >>> 0;
+ let digits = intval.toString(radix);
+ rem = remDiv;
+ if (rem.isZero()) {
+ return digits + result;
+ } else {
+ while (digits.length < 6) digits = '0' + digits;
+ result = '' + digits + result;
+ }
+ }
+ }
+
+ /** Converts this Long to unsigned. */
+ toUnsigned(): Long {
+ if (this.unsigned) return this;
+ return Long.fromBits(this.low, this.high, true);
+ }
+
+ /** Returns the bitwise XOR of this Long and the given one. */
+ xor(other: Long | number | string): Long {
+ if (!Long.isLong(other)) other = Long.fromValue(other);
+ return Long.fromBits(this.low ^ other.low, this.high ^ other.high, this.unsigned);
+ }
+
+ /** This is an alias of {@link Long.isZero} */
+ eqz(): boolean {
+ return this.isZero();
+ }
+
+ /** This is an alias of {@link Long.lessThanOrEqual} */
+ le(other: string | number | Long | Timestamp): boolean {
+ return this.lessThanOrEqual(other);
+ }
+
+ /*
+ ****************************************************************
+ * BSON SPECIFIC ADDITIONS *
+ ****************************************************************
+ */
+ toExtendedJSON(options?: EJSONOptions): number | LongExtended {
+ if (options && options.relaxed) return this.toNumber();
+ return { $numberLong: this.toString() };
+ }
+ static fromExtendedJSON(doc: { $numberLong: string }, options?: EJSONOptions): number | Long {
+ const result = Long.fromString(doc.$numberLong);
+ return options && options.relaxed ? result.toNumber() : result;
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ return `new Long("${this.toString()}"${this.unsigned ? ', true' : ''})`;
+ }
+}
+
+Object.defineProperty(Long.prototype, '__isLong__', { value: true });
+Object.defineProperty(Long.prototype, '_bsontype', { value: 'Long' });
diff --git a/node_modules/bson/src/map.ts b/node_modules/bson/src/map.ts
new file mode 100644
index 0000000..e5eb32a
--- /dev/null
+++ b/node_modules/bson/src/map.ts
@@ -0,0 +1,139 @@
+/* eslint-disable @typescript-eslint/no-explicit-any */
+// We have an ES6 Map available, return the native instance
+
+/* We do not want to have to include DOM types just for this check */
+declare const window: unknown;
+declare const self: unknown;
+declare const global: unknown;
+
+/** @public */
+let bsonMap: MapConstructor;
+
+const check = function (potentialGlobal: any) {
+ // eslint-disable-next-line eqeqeq
+ return potentialGlobal && potentialGlobal.Math == Math && potentialGlobal;
+};
+
+// https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+function getGlobal() {
+ // eslint-disable-next-line no-undef
+ return (
+ check(typeof globalThis === 'object' && globalThis) ||
+ check(typeof window === 'object' && window) ||
+ check(typeof self === 'object' && self) ||
+ check(typeof global === 'object' && global) ||
+ Function('return this')()
+ );
+}
+
+const bsonGlobal = getGlobal();
+if (Object.prototype.hasOwnProperty.call(bsonGlobal, 'Map')) {
+ bsonMap = bsonGlobal.Map;
+} else {
+ // We will return a polyfill
+ bsonMap = (class Map {
+ private _keys: string[];
+ private _values: Record;
+ constructor(array: [string, any][] = []) {
+ this._keys = [];
+ this._values = {};
+
+ for (let i = 0; i < array.length; i++) {
+ if (array[i] == null) continue; // skip null and undefined
+ const entry = array[i];
+ const key = entry[0];
+ const value = entry[1];
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ }
+ }
+ clear() {
+ this._keys = [];
+ this._values = {};
+ }
+ delete(key: string) {
+ const value = this._values[key];
+ if (value == null) return false;
+ // Delete entry
+ delete this._values[key];
+ // Remove the key from the ordered keys list
+ this._keys.splice(value.i, 1);
+ return true;
+ }
+ entries() {
+ let index = 0;
+
+ return {
+ next: () => {
+ const key = this._keys[index++];
+ return {
+ value: key !== undefined ? [key, this._values[key].v] : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ }
+ forEach(callback: (this: this, value: any, key: string, self: this) => void, self?: this) {
+ self = self || this;
+
+ for (let i = 0; i < this._keys.length; i++) {
+ const key = this._keys[i];
+ // Call the forEach callback
+ callback.call(self, this._values[key].v, key, self);
+ }
+ }
+ get(key: string) {
+ return this._values[key] ? this._values[key].v : undefined;
+ }
+ has(key: string) {
+ return this._values[key] != null;
+ }
+ keys() {
+ let index = 0;
+
+ return {
+ next: () => {
+ const key = this._keys[index++];
+ return {
+ value: key !== undefined ? key : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ }
+ set(key: string, value: any) {
+ if (this._values[key]) {
+ this._values[key].v = value;
+ return this;
+ }
+
+ // Add the key to the list of keys in order
+ this._keys.push(key);
+ // Add the key and value to the values dictionary with a point
+ // to the location in the ordered keys list
+ this._values[key] = { v: value, i: this._keys.length - 1 };
+ return this;
+ }
+ values() {
+ let index = 0;
+
+ return {
+ next: () => {
+ const key = this._keys[index++];
+ return {
+ value: key !== undefined ? this._values[key].v : undefined,
+ done: key !== undefined ? false : true
+ };
+ }
+ };
+ }
+ get size() {
+ return this._keys.length;
+ }
+ } as unknown) as MapConstructor;
+}
+
+export { bsonMap as Map };
diff --git a/node_modules/bson/src/max_key.ts b/node_modules/bson/src/max_key.ts
new file mode 100644
index 0000000..fcfc5be
--- /dev/null
+++ b/node_modules/bson/src/max_key.ts
@@ -0,0 +1,37 @@
+/** @public */
+export interface MaxKeyExtended {
+ $maxKey: 1;
+}
+
+/**
+ * A class representation of the BSON MaxKey type.
+ * @public
+ */
+export class MaxKey {
+ _bsontype!: 'MaxKey';
+
+ constructor() {
+ if (!(this instanceof MaxKey)) return new MaxKey();
+ }
+
+ /** @internal */
+ toExtendedJSON(): MaxKeyExtended {
+ return { $maxKey: 1 };
+ }
+
+ /** @internal */
+ static fromExtendedJSON(): MaxKey {
+ return new MaxKey();
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ return 'new MaxKey()';
+ }
+}
+
+Object.defineProperty(MaxKey.prototype, '_bsontype', { value: 'MaxKey' });
diff --git a/node_modules/bson/src/min_key.ts b/node_modules/bson/src/min_key.ts
new file mode 100644
index 0000000..f1647fc
--- /dev/null
+++ b/node_modules/bson/src/min_key.ts
@@ -0,0 +1,37 @@
+/** @public */
+export interface MinKeyExtended {
+ $minKey: 1;
+}
+
+/**
+ * A class representation of the BSON MinKey type.
+ * @public
+ */
+export class MinKey {
+ _bsontype!: 'MinKey';
+
+ constructor() {
+ if (!(this instanceof MinKey)) return new MinKey();
+ }
+
+ /** @internal */
+ toExtendedJSON(): MinKeyExtended {
+ return { $minKey: 1 };
+ }
+
+ /** @internal */
+ static fromExtendedJSON(): MinKey {
+ return new MinKey();
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ return 'new MinKey()';
+ }
+}
+
+Object.defineProperty(MinKey.prototype, '_bsontype', { value: 'MinKey' });
diff --git a/node_modules/bson/src/objectid.ts b/node_modules/bson/src/objectid.ts
new file mode 100644
index 0000000..c02c561
--- /dev/null
+++ b/node_modules/bson/src/objectid.ts
@@ -0,0 +1,372 @@
+import { Buffer } from 'buffer';
+import { ensureBuffer } from './ensure_buffer';
+import { deprecate, isUint8Array, randomBytes } from './parser/utils';
+
+// Regular expression that checks for hex value
+const checkForHexRegExp = new RegExp('^[0-9a-fA-F]{24}$');
+
+// Unique sequence for the current process (initialized on first use)
+let PROCESS_UNIQUE: Uint8Array | null = null;
+
+/** @public */
+export interface ObjectIdLike {
+ id: string | Buffer;
+ __id?: string;
+ toHexString(): string;
+}
+
+/** @public */
+export interface ObjectIdExtended {
+ $oid: string;
+}
+
+const kId = Symbol('id');
+
+/**
+ * A class representation of the BSON ObjectId type.
+ * @public
+ */
+export class ObjectId {
+ _bsontype!: 'ObjectId';
+
+ /** @internal */
+ static index = ~~(Math.random() * 0xffffff);
+
+ static cacheHexString: boolean;
+
+ /** ObjectId Bytes @internal */
+ private [kId]: Buffer;
+ /** ObjectId hexString cache @internal */
+ private __id?: string;
+
+ /**
+ * Create an ObjectId type
+ *
+ * @param id - Can be a 24 character hex string, 12 byte binary Buffer, or a number.
+ */
+ constructor(id?: string | Buffer | number | ObjectIdLike | ObjectId) {
+ if (!(this instanceof ObjectId)) return new ObjectId(id);
+
+ // Duck-typing to support ObjectId from different npm packages
+ if (id instanceof ObjectId) {
+ this[kId] = id.id;
+ this.__id = id.__id;
+ }
+
+ if (typeof id === 'object' && id && 'id' in id) {
+ if ('toHexString' in id && typeof id.toHexString === 'function') {
+ this[kId] = Buffer.from(id.toHexString(), 'hex');
+ } else {
+ this[kId] = typeof id.id === 'string' ? Buffer.from(id.id) : id.id;
+ }
+ }
+
+ // The most common use case (blank id, new objectId instance)
+ if (id == null || typeof id === 'number') {
+ // Generate a new id
+ this[kId] = ObjectId.generate(typeof id === 'number' ? id : undefined);
+ // If we are caching the hex string
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+
+ if (ArrayBuffer.isView(id) && id.byteLength === 12) {
+ this[kId] = ensureBuffer(id);
+ }
+
+ if (typeof id === 'string') {
+ if (id.length === 12) {
+ const bytes = Buffer.from(id);
+ if (bytes.byteLength === 12) {
+ this[kId] = bytes;
+ }
+ } else if (id.length === 24 && checkForHexRegExp.test(id)) {
+ this[kId] = Buffer.from(id, 'hex');
+ } else {
+ throw new TypeError(
+ 'Argument passed in must be a Buffer or string of 12 bytes or a string of 24 hex characters'
+ );
+ }
+ }
+
+ if (ObjectId.cacheHexString) {
+ this.__id = this.id.toString('hex');
+ }
+ }
+
+ /**
+ * The ObjectId bytes
+ * @readonly
+ */
+ get id(): Buffer {
+ return this[kId];
+ }
+
+ set id(value: Buffer) {
+ this[kId] = value;
+ if (ObjectId.cacheHexString) {
+ this.__id = value.toString('hex');
+ }
+ }
+
+ /**
+ * The generation time of this ObjectId instance
+ * @deprecated Please use getTimestamp / createFromTime which returns an int32 epoch
+ */
+ get generationTime(): number {
+ return this.id.readInt32BE(0);
+ }
+
+ set generationTime(value: number) {
+ // Encode time into first 4 bytes
+ this.id.writeUInt32BE(value, 0);
+ }
+
+ /** Returns the ObjectId id as a 24 character hex string representation */
+ toHexString(): string {
+ if (ObjectId.cacheHexString && this.__id) {
+ return this.__id;
+ }
+
+ const hexString = this.id.toString('hex');
+
+ if (ObjectId.cacheHexString && !this.__id) {
+ this.__id = hexString;
+ }
+
+ return hexString;
+ }
+
+ /**
+ * Update the ObjectId index
+ * @privateRemarks
+ * Used in generating new ObjectId's on the driver
+ * @internal
+ */
+ static getInc(): number {
+ return (ObjectId.index = (ObjectId.index + 1) % 0xffffff);
+ }
+
+ /**
+ * Generate a 12 byte id buffer used in ObjectId's
+ *
+ * @param time - pass in a second based timestamp.
+ */
+ static generate(time?: number): Buffer {
+ if ('number' !== typeof time) {
+ time = ~~(Date.now() / 1000);
+ }
+
+ const inc = ObjectId.getInc();
+ const buffer = Buffer.alloc(12);
+
+ // 4-byte timestamp
+ buffer.writeUInt32BE(time, 0);
+
+ // set PROCESS_UNIQUE if yet not initialized
+ if (PROCESS_UNIQUE === null) {
+ PROCESS_UNIQUE = randomBytes(5);
+ }
+
+ // 5-byte process unique
+ buffer[4] = PROCESS_UNIQUE[0];
+ buffer[5] = PROCESS_UNIQUE[1];
+ buffer[6] = PROCESS_UNIQUE[2];
+ buffer[7] = PROCESS_UNIQUE[3];
+ buffer[8] = PROCESS_UNIQUE[4];
+
+ // 3-byte counter
+ buffer[11] = inc & 0xff;
+ buffer[10] = (inc >> 8) & 0xff;
+ buffer[9] = (inc >> 16) & 0xff;
+
+ return buffer;
+ }
+
+ /**
+ * Converts the id into a 24 character hex string for printing
+ *
+ * @param format - The Buffer toString format parameter.
+ * @internal
+ */
+ toString(format?: string): string {
+ // Is the id a buffer then use the buffer toString method to return the format
+ if (format) return this.id.toString(format);
+ return this.toHexString();
+ }
+
+ /**
+ * Converts to its JSON the 24 character hex string representation.
+ * @internal
+ */
+ toJSON(): string {
+ return this.toHexString();
+ }
+
+ /**
+ * Compares the equality of this ObjectId with `otherID`.
+ *
+ * @param otherId - ObjectId instance to compare against.
+ */
+ equals(otherId: string | ObjectId | ObjectIdLike): boolean {
+ if (otherId === undefined || otherId === null) {
+ return false;
+ }
+
+ if (otherId instanceof ObjectId) {
+ return this.toString() === otherId.toString();
+ }
+
+ if (
+ typeof otherId === 'string' &&
+ ObjectId.isValid(otherId) &&
+ otherId.length === 12 &&
+ isUint8Array(this.id)
+ ) {
+ return otherId === Buffer.prototype.toString.call(this.id, 'latin1');
+ }
+
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 24) {
+ return otherId.toLowerCase() === this.toHexString();
+ }
+
+ if (typeof otherId === 'string' && ObjectId.isValid(otherId) && otherId.length === 12) {
+ return Buffer.from(otherId).equals(this.id);
+ }
+
+ if (
+ typeof otherId === 'object' &&
+ 'toHexString' in otherId &&
+ typeof otherId.toHexString === 'function'
+ ) {
+ return otherId.toHexString() === this.toHexString();
+ }
+
+ return false;
+ }
+
+ /** Returns the generation date (accurate up to the second) that this ID was generated. */
+ getTimestamp(): Date {
+ const timestamp = new Date();
+ const time = this.id.readUInt32BE(0);
+ timestamp.setTime(Math.floor(time) * 1000);
+ return timestamp;
+ }
+
+ /** @internal */
+ static createPk(): ObjectId {
+ return new ObjectId();
+ }
+
+ /**
+ * Creates an ObjectId from a second based number, with the rest of the ObjectId zeroed out. Used for comparisons or sorting the ObjectId.
+ *
+ * @param time - an integer number representing a number of seconds.
+ */
+ static createFromTime(time: number): ObjectId {
+ const buffer = Buffer.from([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]);
+ // Encode time into first 4 bytes
+ buffer.writeUInt32BE(time, 0);
+ // Return the new objectId
+ return new ObjectId(buffer);
+ }
+
+ /**
+ * Creates an ObjectId from a hex string representation of an ObjectId.
+ *
+ * @param hexString - create a ObjectId from a passed in 24 character hexstring.
+ */
+ static createFromHexString(hexString: string): ObjectId {
+ // Throw an error if it's not a valid setup
+ if (typeof hexString === 'undefined' || (hexString != null && hexString.length !== 24)) {
+ throw new TypeError(
+ 'Argument passed in must be a single String of 12 bytes or a string of 24 hex characters'
+ );
+ }
+
+ return new ObjectId(Buffer.from(hexString, 'hex'));
+ }
+
+ /**
+ * Checks if a value is a valid bson ObjectId
+ *
+ * @param id - ObjectId instance to validate.
+ */
+ static isValid(id: number | string | ObjectId | Uint8Array | ObjectIdLike): boolean {
+ if (id == null) return false;
+
+ if (typeof id === 'number') {
+ return true;
+ }
+
+ if (typeof id === 'string') {
+ return id.length === 12 || (id.length === 24 && checkForHexRegExp.test(id));
+ }
+
+ if (id instanceof ObjectId) {
+ return true;
+ }
+
+ if (isUint8Array(id) && id.length === 12) {
+ return true;
+ }
+
+ // Duck-Typing detection of ObjectId like objects
+ if (typeof id === 'object' && 'toHexString' in id && typeof id.toHexString === 'function') {
+ if (typeof id.id === 'string') {
+ return id.id.length === 12;
+ }
+ return id.toHexString().length === 24 && checkForHexRegExp.test(id.id.toString('hex'));
+ }
+
+ return false;
+ }
+
+ /** @internal */
+ toExtendedJSON(): ObjectIdExtended {
+ if (this.toHexString) return { $oid: this.toHexString() };
+ return { $oid: this.toString('hex') };
+ }
+
+ /** @internal */
+ static fromExtendedJSON(doc: ObjectIdExtended): ObjectId {
+ return new ObjectId(doc.$oid);
+ }
+
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 24 character hex string representation.
+ * @internal
+ */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ return `new ObjectId("${this.toHexString()}")`;
+ }
+}
+
+// Deprecated methods
+Object.defineProperty(ObjectId.prototype, 'generate', {
+ value: deprecate(
+ (time: number) => ObjectId.generate(time),
+ 'Please use the static `ObjectId.generate(time)` instead'
+ )
+});
+
+Object.defineProperty(ObjectId.prototype, 'getInc', {
+ value: deprecate(() => ObjectId.getInc(), 'Please use the static `ObjectId.getInc()` instead')
+});
+
+Object.defineProperty(ObjectId.prototype, 'get_inc', {
+ value: deprecate(() => ObjectId.getInc(), 'Please use the static `ObjectId.getInc()` instead')
+});
+
+Object.defineProperty(ObjectId, 'get_inc', {
+ value: deprecate(() => ObjectId.getInc(), 'Please use the static `ObjectId.getInc()` instead')
+});
+
+Object.defineProperty(ObjectId.prototype, '_bsontype', { value: 'ObjectID' });
diff --git a/node_modules/bson/src/parser/calculate_size.ts b/node_modules/bson/src/parser/calculate_size.ts
new file mode 100644
index 0000000..6a67d44
--- /dev/null
+++ b/node_modules/bson/src/parser/calculate_size.ts
@@ -0,0 +1,227 @@
+import { Buffer } from 'buffer';
+import { Binary } from '../binary';
+import type { Document } from '../bson';
+import * as constants from '../constants';
+import { isAnyArrayBuffer, isDate, isRegExp, normalizedFunctionString } from './utils';
+
+export function calculateObjectSize(
+ object: Document,
+ serializeFunctions?: boolean,
+ ignoreUndefined?: boolean
+): number {
+ let totalLength = 4 + 1;
+
+ if (Array.isArray(object)) {
+ for (let i = 0; i < object.length; i++) {
+ totalLength += calculateElement(
+ i.toString(),
+ object[i],
+ serializeFunctions,
+ true,
+ ignoreUndefined
+ );
+ }
+ } else {
+ // If we have toBSON defined, override the current object
+
+ if (object.toBSON) {
+ object = object.toBSON();
+ }
+
+ // Calculate size
+ for (const key in object) {
+ totalLength += calculateElement(key, object[key], serializeFunctions, false, ignoreUndefined);
+ }
+ }
+
+ return totalLength;
+}
+
+/** @internal */
+function calculateElement(
+ name: string,
+ // eslint-disable-next-line @typescript-eslint/no-explicit-any
+ value: any,
+ serializeFunctions = false,
+ isArray = false,
+ ignoreUndefined = false
+) {
+ // If we have toBSON defined, override the current object
+ if (value && value.toBSON) {
+ value = value.toBSON();
+ }
+
+ switch (typeof value) {
+ case 'string':
+ return 1 + Buffer.byteLength(name, 'utf8') + 1 + 4 + Buffer.byteLength(value, 'utf8') + 1;
+ case 'number':
+ if (
+ Math.floor(value) === value &&
+ value >= constants.JS_INT_MIN &&
+ value <= constants.JS_INT_MAX
+ ) {
+ if (value >= constants.BSON_INT32_MIN && value <= constants.BSON_INT32_MAX) {
+ // 32 bit
+ return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (4 + 1);
+ } else {
+ return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ } else {
+ // 64 bit
+ return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ }
+ case 'undefined':
+ if (isArray || !ignoreUndefined)
+ return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ return 0;
+ case 'boolean':
+ return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 1);
+ case 'object':
+ if (value == null || value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + 1;
+ } else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (12 + 1);
+ } else if (value instanceof Date || isDate(value)) {
+ return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ } else if (
+ ArrayBuffer.isView(value) ||
+ value instanceof ArrayBuffer ||
+ isAnyArrayBuffer(value)
+ ) {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (1 + 4 + 1) + value.byteLength
+ );
+ } else if (
+ value['_bsontype'] === 'Long' ||
+ value['_bsontype'] === 'Double' ||
+ value['_bsontype'] === 'Timestamp'
+ ) {
+ return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (8 + 1);
+ } else if (value['_bsontype'] === 'Decimal128') {
+ return (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (16 + 1);
+ } else if (value['_bsontype'] === 'Code') {
+ // Calculate size depending on the availability of a scope
+ if (value.scope != null && Object.keys(value.scope).length > 0) {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined)
+ );
+ } else {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ Buffer.byteLength(value.code.toString(), 'utf8') +
+ 1
+ );
+ }
+ } else if (value['_bsontype'] === 'Binary') {
+ // Check what kind of subtype we have
+ if (value.sub_type === Binary.SUBTYPE_BYTE_ARRAY) {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ (value.position + 1 + 4 + 1 + 4)
+ );
+ } else {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) + (value.position + 1 + 4 + 1)
+ );
+ }
+ } else if (value['_bsontype'] === 'Symbol') {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ Buffer.byteLength(value.value, 'utf8') +
+ 4 +
+ 1 +
+ 1
+ );
+ } else if (value['_bsontype'] === 'DBRef') {
+ // Set up correct object for serialization
+ const ordered_values = Object.assign(
+ {
+ $ref: value.collection,
+ $id: value.oid
+ },
+ value.fields
+ );
+
+ // Add db reference if it exists
+ if (value.db != null) {
+ ordered_values['$db'] = value.db;
+ }
+
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ calculateObjectSize(ordered_values, serializeFunctions, ignoreUndefined)
+ );
+ } else if (value instanceof RegExp || isRegExp(value)) {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1
+ );
+ } else if (value['_bsontype'] === 'BSONRegExp') {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ Buffer.byteLength(value.pattern, 'utf8') +
+ 1 +
+ Buffer.byteLength(value.options, 'utf8') +
+ 1
+ );
+ } else {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ calculateObjectSize(value, serializeFunctions, ignoreUndefined) +
+ 1
+ );
+ }
+ case 'function':
+ // WTF for 0.4.X where typeof /someregexp/ === 'function'
+ if (value instanceof RegExp || isRegExp(value) || String.call(value) === '[object RegExp]') {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ Buffer.byteLength(value.source, 'utf8') +
+ 1 +
+ (value.global ? 1 : 0) +
+ (value.ignoreCase ? 1 : 0) +
+ (value.multiline ? 1 : 0) +
+ 1
+ );
+ } else {
+ if (serializeFunctions && value.scope != null && Object.keys(value.scope).length > 0) {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ 4 +
+ Buffer.byteLength(normalizedFunctionString(value), 'utf8') +
+ 1 +
+ calculateObjectSize(value.scope, serializeFunctions, ignoreUndefined)
+ );
+ } else if (serializeFunctions) {
+ return (
+ (name != null ? Buffer.byteLength(name, 'utf8') + 1 : 0) +
+ 1 +
+ 4 +
+ Buffer.byteLength(normalizedFunctionString(value), 'utf8') +
+ 1
+ );
+ }
+ }
+ }
+
+ return 0;
+}
diff --git a/node_modules/bson/src/parser/deserializer.ts b/node_modules/bson/src/parser/deserializer.ts
new file mode 100644
index 0000000..90b3afb
--- /dev/null
+++ b/node_modules/bson/src/parser/deserializer.ts
@@ -0,0 +1,665 @@
+import { Buffer } from 'buffer';
+import { Binary } from '../binary';
+import type { Document } from '../bson';
+import { Code } from '../code';
+import * as constants from '../constants';
+import { DBRef, DBRefLike, isDBRefLike } from '../db_ref';
+import { Decimal128 } from '../decimal128';
+import { Double } from '../double';
+import { Int32 } from '../int_32';
+import { Long } from '../long';
+import { MaxKey } from '../max_key';
+import { MinKey } from '../min_key';
+import { ObjectId } from '../objectid';
+import { BSONRegExp } from '../regexp';
+import { BSONSymbol } from '../symbol';
+import { Timestamp } from '../timestamp';
+import { validateUtf8 } from '../validate_utf8';
+
+/** @public */
+export interface DeserializeOptions {
+ /** evaluate functions in the BSON document scoped to the object deserialized. */
+ evalFunctions?: boolean;
+ /** cache evaluated functions for reuse. */
+ cacheFunctions?: boolean;
+ /**
+ * use a crc32 code for caching, otherwise use the string of the function.
+ * @deprecated this option to use the crc32 function never worked as intended
+ * due to the fact that the crc32 function itself was never implemented.
+ * */
+ cacheFunctionsCrc32?: boolean;
+ /** when deserializing a Long will fit it into a Number if it's smaller than 53 bits */
+ promoteLongs?: boolean;
+ /** when deserializing a Binary will return it as a node.js Buffer instance. */
+ promoteBuffers?: boolean;
+ /** when deserializing will promote BSON values to their Node.js closest equivalent types. */
+ promoteValues?: boolean;
+ /** allow to specify if there what fields we wish to return as unserialized raw buffer. */
+ fieldsAsRaw?: Document;
+ /** return BSON regular expressions as BSONRegExp instances. */
+ bsonRegExp?: boolean;
+ /** allows the buffer to be larger than the parsed BSON object */
+ allowObjectSmallerThanBufferSize?: boolean;
+ /** Offset into buffer to begin reading document from */
+ index?: number;
+
+ raw?: boolean;
+}
+
+// Internal long versions
+const JS_INT_MAX_LONG = Long.fromNumber(constants.JS_INT_MAX);
+const JS_INT_MIN_LONG = Long.fromNumber(constants.JS_INT_MIN);
+
+const functionCache: { [hash: string]: Function } = {};
+
+export function deserialize(
+ buffer: Buffer,
+ options: DeserializeOptions,
+ isArray?: boolean
+): Document {
+ options = options == null ? {} : options;
+ const index = options && options.index ? options.index : 0;
+ // Read the document size
+ const size =
+ buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+
+ if (size < 5) {
+ throw new Error(`bson size must be >= 5, is ${size}`);
+ }
+
+ if (options.allowObjectSmallerThanBufferSize && buffer.length < size) {
+ throw new Error(`buffer length ${buffer.length} must be >= bson size ${size}`);
+ }
+
+ if (!options.allowObjectSmallerThanBufferSize && buffer.length !== size) {
+ throw new Error(`buffer length ${buffer.length} must === bson size ${size}`);
+ }
+
+ if (size + index > buffer.byteLength) {
+ throw new Error(
+ `(bson size ${size} + options.index ${index} must be <= buffer length ${buffer.byteLength})`
+ );
+ }
+
+ // Illegal end value
+ if (buffer[index + size - 1] !== 0) {
+ throw new Error("One object, sized correctly, with a spot for an EOO, but the EOO isn't 0x00");
+ }
+
+ // Start deserializtion
+ return deserializeObject(buffer, index, options, isArray);
+}
+
+function deserializeObject(
+ buffer: Buffer,
+ index: number,
+ options: DeserializeOptions,
+ isArray = false
+) {
+ const evalFunctions = options['evalFunctions'] == null ? false : options['evalFunctions'];
+ const cacheFunctions = options['cacheFunctions'] == null ? false : options['cacheFunctions'];
+
+ const fieldsAsRaw = options['fieldsAsRaw'] == null ? null : options['fieldsAsRaw'];
+
+ // Return raw bson buffer instead of parsing it
+ const raw = options['raw'] == null ? false : options['raw'];
+
+ // Return BSONRegExp objects instead of native regular expressions
+ const bsonRegExp = typeof options['bsonRegExp'] === 'boolean' ? options['bsonRegExp'] : false;
+
+ // Controls the promotion of values vs wrapper classes
+ const promoteBuffers = options['promoteBuffers'] == null ? false : options['promoteBuffers'];
+ const promoteLongs = options['promoteLongs'] == null ? true : options['promoteLongs'];
+ const promoteValues = options['promoteValues'] == null ? true : options['promoteValues'];
+
+ // Set the start index
+ const startIndex = index;
+
+ // Validate that we have at least 4 bytes of buffer
+ if (buffer.length < 5) throw new Error('corrupt bson message < 5 bytes long');
+
+ // Read the document size
+ const size =
+ buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24);
+
+ // Ensure buffer is valid size
+ if (size < 5 || size > buffer.length) throw new Error('corrupt bson message');
+
+ // Create holding object
+ const object: Document = isArray ? [] : {};
+ // Used for arrays to skip having to perform utf8 decoding
+ let arrayIndex = 0;
+ const done = false;
+
+ // While we have more left data left keep parsing
+ while (!done) {
+ // Read the type
+ const elementType = buffer[index++];
+
+ // If we get a zero it's the last byte, exit
+ if (elementType === 0) break;
+
+ // Get the start search index
+ let i = index;
+ // Locate the end of the c string
+ while (buffer[i] !== 0x00 && i < buffer.length) {
+ i++;
+ }
+
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer.byteLength) throw new Error('Bad BSON Document: illegal CString');
+ const name = isArray ? arrayIndex++ : buffer.toString('utf8', index, i);
+ let value;
+
+ index = i + 1;
+
+ if (elementType === constants.BSON_DATA_STRING) {
+ const stringSize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ if (
+ stringSize <= 0 ||
+ stringSize > buffer.length - index ||
+ buffer[index + stringSize - 1] !== 0
+ )
+ throw new Error('bad string length in bson');
+
+ if (!validateUtf8(buffer, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+
+ value = buffer.toString('utf8', index, index + stringSize - 1);
+
+ index = index + stringSize;
+ } else if (elementType === constants.BSON_DATA_OID) {
+ const oid = Buffer.alloc(12);
+ buffer.copy(oid, 0, index, index + 12);
+ value = new ObjectId(oid);
+ index = index + 12;
+ } else if (elementType === constants.BSON_DATA_INT && promoteValues === false) {
+ value = new Int32(
+ buffer[index++] | (buffer[index++] << 8) | (buffer[index++] << 16) | (buffer[index++] << 24)
+ );
+ } else if (elementType === constants.BSON_DATA_INT) {
+ value =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ } else if (elementType === constants.BSON_DATA_NUMBER && promoteValues === false) {
+ value = new Double(buffer.readDoubleLE(index));
+ index = index + 8;
+ } else if (elementType === constants.BSON_DATA_NUMBER) {
+ value = buffer.readDoubleLE(index);
+ index = index + 8;
+ } else if (elementType === constants.BSON_DATA_DATE) {
+ const lowBits =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ const highBits =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ value = new Date(new Long(lowBits, highBits).toNumber());
+ } else if (elementType === constants.BSON_DATA_BOOLEAN) {
+ if (buffer[index] !== 0 && buffer[index] !== 1) throw new Error('illegal boolean type value');
+ value = buffer[index++] === 1;
+ } else if (elementType === constants.BSON_DATA_OBJECT) {
+ const _index = index;
+ const objectSize =
+ buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ if (objectSize <= 0 || objectSize > buffer.length - index)
+ throw new Error('bad embedded document length in bson');
+
+ // We have a raw value
+ if (raw) {
+ value = buffer.slice(index, index + objectSize);
+ } else {
+ value = deserializeObject(buffer, _index, options, false);
+ }
+
+ index = index + objectSize;
+ } else if (elementType === constants.BSON_DATA_ARRAY) {
+ const _index = index;
+ const objectSize =
+ buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ let arrayOptions = options;
+
+ // Stop index
+ const stopIndex = index + objectSize;
+
+ // All elements of array to be returned as raw bson
+ if (fieldsAsRaw && fieldsAsRaw[name]) {
+ arrayOptions = {};
+ for (const n in options) {
+ (arrayOptions as {
+ [key: string]: DeserializeOptions[keyof DeserializeOptions];
+ })[n] = options[n as keyof DeserializeOptions];
+ }
+ arrayOptions['raw'] = true;
+ }
+
+ value = deserializeObject(buffer, _index, arrayOptions, true);
+ index = index + objectSize;
+
+ if (buffer[index - 1] !== 0) throw new Error('invalid array terminator byte');
+ if (index !== stopIndex) throw new Error('corrupted array bson');
+ } else if (elementType === constants.BSON_DATA_UNDEFINED) {
+ value = undefined;
+ } else if (elementType === constants.BSON_DATA_NULL) {
+ value = null;
+ } else if (elementType === constants.BSON_DATA_LONG) {
+ // Unpack the low and high bits
+ const lowBits =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ const highBits =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ const long = new Long(lowBits, highBits);
+ // Promote the long if possible
+ if (promoteLongs && promoteValues === true) {
+ value =
+ long.lessThanOrEqual(JS_INT_MAX_LONG) && long.greaterThanOrEqual(JS_INT_MIN_LONG)
+ ? long.toNumber()
+ : long;
+ } else {
+ value = long;
+ }
+ } else if (elementType === constants.BSON_DATA_DECIMAL128) {
+ // Buffer to contain the decimal bytes
+ const bytes = Buffer.alloc(16);
+ // Copy the next 16 bytes into the bytes buffer
+ buffer.copy(bytes, 0, index, index + 16);
+ // Update index
+ index = index + 16;
+ // Assign the new Decimal128 value
+ const decimal128 = new Decimal128(bytes) as Decimal128 | { toObject(): unknown };
+ // If we have an alternative mapper use that
+ if ('toObject' in decimal128 && typeof decimal128.toObject === 'function') {
+ value = decimal128.toObject();
+ } else {
+ value = decimal128;
+ }
+ } else if (elementType === constants.BSON_DATA_BINARY) {
+ let binarySize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ const totalBinarySize = binarySize;
+ const subType = buffer[index++];
+
+ // Did we have a negative binary size, throw
+ if (binarySize < 0) throw new Error('Negative binary type element size found');
+
+ // Is the length longer than the document
+ if (binarySize > buffer.byteLength)
+ throw new Error('Binary type size larger than document size');
+
+ // Decode as raw Buffer object if options specifies it
+ if (buffer['slice'] != null) {
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+
+ if (promoteBuffers && promoteValues) {
+ value = buffer.slice(index, index + binarySize);
+ } else {
+ value = new Binary(buffer.slice(index, index + binarySize), subType);
+ }
+ } else {
+ const _buffer = Buffer.alloc(binarySize);
+ // If we have subtype 2 skip the 4 bytes for the size
+ if (subType === Binary.SUBTYPE_BYTE_ARRAY) {
+ binarySize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ if (binarySize < 0)
+ throw new Error('Negative binary type element size found for subtype 0x02');
+ if (binarySize > totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too long binary size');
+ if (binarySize < totalBinarySize - 4)
+ throw new Error('Binary type with subtype 0x02 contains too short binary size');
+ }
+
+ // Copy the data
+ for (i = 0; i < binarySize; i++) {
+ _buffer[i] = buffer[index + i];
+ }
+
+ if (promoteBuffers && promoteValues) {
+ value = _buffer;
+ } else {
+ value = new Binary(_buffer, subType);
+ }
+ }
+
+ // Update the index
+ index = index + binarySize;
+ } else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === false) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer[i] !== 0x00 && i < buffer.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ const source = buffer.toString('utf8', index, i);
+ // Create the regexp
+ index = i + 1;
+
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer[i] !== 0x00 && i < buffer.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ const regExpOptions = buffer.toString('utf8', index, i);
+ index = i + 1;
+
+ // For each option add the corresponding one for javascript
+ const optionsArray = new Array(regExpOptions.length);
+
+ // Parse options
+ for (i = 0; i < regExpOptions.length; i++) {
+ switch (regExpOptions[i]) {
+ case 'm':
+ optionsArray[i] = 'm';
+ break;
+ case 's':
+ optionsArray[i] = 'g';
+ break;
+ case 'i':
+ optionsArray[i] = 'i';
+ break;
+ }
+ }
+
+ value = new RegExp(source, optionsArray.join(''));
+ } else if (elementType === constants.BSON_DATA_REGEXP && bsonRegExp === true) {
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer[i] !== 0x00 && i < buffer.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ const source = buffer.toString('utf8', index, i);
+ index = i + 1;
+
+ // Get the start search index
+ i = index;
+ // Locate the end of the c string
+ while (buffer[i] !== 0x00 && i < buffer.length) {
+ i++;
+ }
+ // If are at the end of the buffer there is a problem with the document
+ if (i >= buffer.length) throw new Error('Bad BSON Document: illegal CString');
+ // Return the C string
+ const regExpOptions = buffer.toString('utf8', index, i);
+ index = i + 1;
+
+ // Set the object
+ value = new BSONRegExp(source, regExpOptions);
+ } else if (elementType === constants.BSON_DATA_SYMBOL) {
+ const stringSize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ if (
+ stringSize <= 0 ||
+ stringSize > buffer.length - index ||
+ buffer[index + stringSize - 1] !== 0
+ )
+ throw new Error('bad string length in bson');
+ const symbol = buffer.toString('utf8', index, index + stringSize - 1);
+ value = promoteValues ? symbol : new BSONSymbol(symbol);
+ index = index + stringSize;
+ } else if (elementType === constants.BSON_DATA_TIMESTAMP) {
+ const lowBits =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ const highBits =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+
+ value = new Timestamp(lowBits, highBits);
+ } else if (elementType === constants.BSON_DATA_MIN_KEY) {
+ value = new MinKey();
+ } else if (elementType === constants.BSON_DATA_MAX_KEY) {
+ value = new MaxKey();
+ } else if (elementType === constants.BSON_DATA_CODE) {
+ const stringSize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ if (
+ stringSize <= 0 ||
+ stringSize > buffer.length - index ||
+ buffer[index + stringSize - 1] !== 0
+ )
+ throw new Error('bad string length in bson');
+ const functionString = buffer.toString('utf8', index, index + stringSize - 1);
+
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ } else {
+ value = isolateEval(functionString);
+ }
+ } else {
+ value = new Code(functionString);
+ }
+
+ // Update parse index position
+ index = index + stringSize;
+ } else if (elementType === constants.BSON_DATA_CODE_W_SCOPE) {
+ const totalSize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+
+ // Element cannot be shorter than totalSize + stringSize + documentSize + terminator
+ if (totalSize < 4 + 4 + 4 + 1) {
+ throw new Error('code_w_scope total size shorter minimum expected length');
+ }
+
+ // Get the code string size
+ const stringSize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ // Check if we have a valid string
+ if (
+ stringSize <= 0 ||
+ stringSize > buffer.length - index ||
+ buffer[index + stringSize - 1] !== 0
+ )
+ throw new Error('bad string length in bson');
+
+ // Javascript function
+ const functionString = buffer.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+ // Parse the element
+ const _index = index;
+ // Decode the size of the object document
+ const objectSize =
+ buffer[index] |
+ (buffer[index + 1] << 8) |
+ (buffer[index + 2] << 16) |
+ (buffer[index + 3] << 24);
+ // Decode the scope object
+ const scopeObject = deserializeObject(buffer, _index, options, false);
+ // Adjust the index
+ index = index + objectSize;
+
+ // Check if field length is too short
+ if (totalSize < 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too short, truncating scope');
+ }
+
+ // Check if totalSize field is too long
+ if (totalSize > 4 + 4 + objectSize + stringSize) {
+ throw new Error('code_w_scope total size is too long, clips outer document');
+ }
+
+ // If we are evaluating the functions
+ if (evalFunctions) {
+ // If we have cache enabled let's look for the md5 of the function in the cache
+ if (cacheFunctions) {
+ // Got to do this to avoid V8 deoptimizing the call due to finding eval
+ value = isolateEval(functionString, functionCache, object);
+ } else {
+ value = isolateEval(functionString);
+ }
+
+ value.scope = scopeObject;
+ } else {
+ value = new Code(functionString, scopeObject);
+ }
+ } else if (elementType === constants.BSON_DATA_DBPOINTER) {
+ // Get the code string size
+ const stringSize =
+ buffer[index++] |
+ (buffer[index++] << 8) |
+ (buffer[index++] << 16) |
+ (buffer[index++] << 24);
+ // Check if we have a valid string
+ if (
+ stringSize <= 0 ||
+ stringSize > buffer.length - index ||
+ buffer[index + stringSize - 1] !== 0
+ )
+ throw new Error('bad string length in bson');
+ // Namespace
+ if (!validateUtf8(buffer, index, index + stringSize - 1)) {
+ throw new Error('Invalid UTF-8 string in BSON document');
+ }
+ const namespace = buffer.toString('utf8', index, index + stringSize - 1);
+ // Update parse index position
+ index = index + stringSize;
+
+ // Read the oid
+ const oidBuffer = Buffer.alloc(12);
+ buffer.copy(oidBuffer, 0, index, index + 12);
+ const oid = new ObjectId(oidBuffer);
+
+ // Update the index
+ index = index + 12;
+
+ // Upgrade to DBRef type
+ value = new DBRef(namespace, oid);
+ } else {
+ throw new Error(
+ 'Detected unknown BSON type ' + elementType.toString(16) + ' for fieldname "' + name + '"'
+ );
+ }
+ if (name === '__proto__') {
+ Object.defineProperty(object, name, {
+ value,
+ writable: true,
+ enumerable: true,
+ configurable: true
+ });
+ } else {
+ object[name] = value;
+ }
+ }
+
+ // Check if the deserialization was against a valid array/object
+ if (size !== index - startIndex) {
+ if (isArray) throw new Error('corrupt array bson');
+ throw new Error('corrupt object bson');
+ }
+
+ // check if object's $ keys are those of a DBRef
+ const dollarKeys = Object.keys(object).filter(k => k.startsWith('$'));
+ let valid = true;
+ dollarKeys.forEach(k => {
+ if (['$ref', '$id', '$db'].indexOf(k) === -1) valid = false;
+ });
+
+ // if a $key not in "$ref", "$id", "$db", don't make a DBRef
+ if (!valid) return object;
+
+ if (isDBRefLike(object)) {
+ const copy = Object.assign({}, object) as Partial;
+ delete copy.$ref;
+ delete copy.$id;
+ delete copy.$db;
+ return new DBRef(object.$ref, object.$id, object.$db, copy);
+ }
+
+ return object;
+}
+
+/**
+ * Ensure eval is isolated, store the result in functionCache.
+ *
+ * @internal
+ */
+function isolateEval(
+ functionString: string,
+ functionCache?: { [hash: string]: Function },
+ object?: Document
+) {
+ if (!functionCache) return new Function(functionString);
+ // Check for cache hit, eval if missing and return cached function
+ if (functionCache[functionString] == null) {
+ functionCache[functionString] = new Function(functionString);
+ }
+
+ // Set the object
+ return functionCache[functionString].bind(object);
+}
diff --git a/node_modules/bson/src/parser/serializer.ts b/node_modules/bson/src/parser/serializer.ts
new file mode 100644
index 0000000..5dd5874
--- /dev/null
+++ b/node_modules/bson/src/parser/serializer.ts
@@ -0,0 +1,1070 @@
+import type { Buffer } from 'buffer';
+import { Binary } from '../binary';
+import type { BSONSymbol, DBRef, Document, MaxKey } from '../bson';
+import type { Code } from '../code';
+import * as constants from '../constants';
+import type { DBRefLike } from '../db_ref';
+import type { Decimal128 } from '../decimal128';
+import type { Double } from '../double';
+import { ensureBuffer } from '../ensure_buffer';
+import { isBSONType } from '../extended_json';
+import { writeIEEE754 } from '../float_parser';
+import type { Int32 } from '../int_32';
+import { Long } from '../long';
+import { Map } from '../map';
+import type { MinKey } from '../min_key';
+import type { ObjectId } from '../objectid';
+import type { BSONRegExp } from '../regexp';
+import {
+ isBigInt64Array,
+ isBigUInt64Array,
+ isDate,
+ isMap,
+ isRegExp,
+ isUint8Array,
+ normalizedFunctionString
+} from './utils';
+
+/** @public */
+export interface SerializeOptions {
+ /** the serializer will check if keys are valid. */
+ checkKeys?: boolean;
+ /** serialize the javascript functions **(default:false)**. */
+ serializeFunctions?: boolean;
+ /** serialize will not emit undefined fields **(default:true)** */
+ ignoreUndefined?: boolean;
+ /** @internal Resize internal buffer */
+ minInternalBufferSize?: number;
+ /** the index in the buffer where we wish to start serializing into */
+ index?: number;
+}
+
+const regexp = /\x00/; // eslint-disable-line no-control-regex
+const ignoreKeys = new Set(['$db', '$ref', '$id', '$clusterTime']);
+
+/*
+ * isArray indicates if we are writing to a BSON array (type 0x04)
+ * which forces the "key" which really an array index as a string to be written as ascii
+ * This will catch any errors in index as a string generation
+ */
+
+function serializeString(
+ buffer: Buffer,
+ key: string,
+ value: string,
+ index: number,
+ isArray?: boolean
+) {
+ // Encode String type
+ buffer[index++] = constants.BSON_DATA_STRING;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes + 1;
+ buffer[index - 1] = 0;
+ // Write the string
+ const size = buffer.write(value, index + 4, undefined, 'utf8');
+ // Write the size of the string to buffer
+ buffer[index + 3] = ((size + 1) >> 24) & 0xff;
+ buffer[index + 2] = ((size + 1) >> 16) & 0xff;
+ buffer[index + 1] = ((size + 1) >> 8) & 0xff;
+ buffer[index] = (size + 1) & 0xff;
+ // Update index
+ index = index + 4 + size;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+}
+
+function serializeNumber(
+ buffer: Buffer,
+ key: string,
+ value: number,
+ index: number,
+ isArray?: boolean
+) {
+ // We have an integer value
+ // TODO(NODE-2529): Add support for big int
+ if (
+ Number.isInteger(value) &&
+ value >= constants.BSON_INT32_MIN &&
+ value <= constants.BSON_INT32_MAX
+ ) {
+ // If the value fits in 32 bits encode as int32
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ } else {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write float
+ writeIEEE754(buffer, value, index, 'little', 52, 8);
+ // Adjust index
+ index = index + 8;
+ }
+
+ return index;
+}
+
+function serializeNull(buffer: Buffer, key: string, _: unknown, index: number, isArray?: boolean) {
+ // Set long type
+ buffer[index++] = constants.BSON_DATA_NULL;
+
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+}
+
+function serializeBoolean(
+ buffer: Buffer,
+ key: string,
+ value: boolean,
+ index: number,
+ isArray?: boolean
+) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BOOLEAN;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Encode the boolean value
+ buffer[index++] = value ? 1 : 0;
+ return index;
+}
+
+function serializeDate(buffer: Buffer, key: string, value: Date, index: number, isArray?: boolean) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_DATE;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+
+ // Write the date
+ const dateInMilis = Long.fromNumber(value.getTime());
+ const lowBits = dateInMilis.getLowBits();
+ const highBits = dateInMilis.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+}
+
+function serializeRegExp(
+ buffer: Buffer,
+ key: string,
+ value: RegExp,
+ index: number,
+ isArray?: boolean
+) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ if (value.source && value.source.match(regexp) != null) {
+ throw Error('value ' + value.source + ' must not contain null bytes');
+ }
+ // Adjust the index
+ index = index + buffer.write(value.source, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the parameters
+ if (value.ignoreCase) buffer[index++] = 0x69; // i
+ if (value.global) buffer[index++] = 0x73; // s
+ if (value.multiline) buffer[index++] = 0x6d; // m
+
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+}
+
+function serializeBSONRegExp(
+ buffer: Buffer,
+ key: string,
+ value: BSONRegExp,
+ index: number,
+ isArray?: boolean
+) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_REGEXP;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+
+ // Check the pattern for 0 bytes
+ if (value.pattern.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('pattern ' + value.pattern + ' must not contain null bytes');
+ }
+
+ // Adjust the index
+ index = index + buffer.write(value.pattern, index, undefined, 'utf8');
+ // Write zero
+ buffer[index++] = 0x00;
+ // Write the options
+ index = index + buffer.write(value.options.split('').sort().join(''), index, undefined, 'utf8');
+ // Add ending zero
+ buffer[index++] = 0x00;
+ return index;
+}
+
+function serializeMinMax(
+ buffer: Buffer,
+ key: string,
+ value: MinKey | MaxKey,
+ index: number,
+ isArray?: boolean
+) {
+ // Write the type of either min or max key
+ if (value === null) {
+ buffer[index++] = constants.BSON_DATA_NULL;
+ } else if (value._bsontype === 'MinKey') {
+ buffer[index++] = constants.BSON_DATA_MIN_KEY;
+ } else {
+ buffer[index++] = constants.BSON_DATA_MAX_KEY;
+ }
+
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ return index;
+}
+
+function serializeObjectId(
+ buffer: Buffer,
+ key: string,
+ value: ObjectId,
+ index: number,
+ isArray?: boolean
+) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OID;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+
+ // Write the objectId into the shared buffer
+ if (typeof value.id === 'string') {
+ buffer.write(value.id, index, undefined, 'binary');
+ } else if (isUint8Array(value.id)) {
+ // Use the standard JS methods here because buffer.copy() is buggy with the
+ // browser polyfill
+ buffer.set(value.id.subarray(0, 12), index);
+ } else {
+ throw new TypeError('object [' + JSON.stringify(value) + '] is not a valid ObjectId');
+ }
+
+ // Adjust index
+ return index + 12;
+}
+
+function serializeBuffer(
+ buffer: Buffer,
+ key: string,
+ value: Buffer | Uint8Array,
+ index: number,
+ isArray?: boolean
+) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Get size of the buffer (current write point)
+ const size = value.length;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the default subtype
+ buffer[index++] = constants.BSON_BINARY_SUBTYPE_DEFAULT;
+ // Copy the content form the binary field to the buffer
+ buffer.set(ensureBuffer(value), index);
+ // Adjust the index
+ index = index + size;
+ return index;
+}
+
+function serializeObject(
+ buffer: Buffer,
+ key: string,
+ value: Document,
+ index: number,
+ checkKeys = false,
+ depth = 0,
+ serializeFunctions = false,
+ ignoreUndefined = true,
+ isArray = false,
+ path: Document[] = []
+) {
+ for (let i = 0; i < path.length; i++) {
+ if (path[i] === value) throw new Error('cyclic dependency detected');
+ }
+
+ // Push value to stack
+ path.push(value);
+ // Write the type
+ buffer[index++] = Array.isArray(value) ? constants.BSON_DATA_ARRAY : constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ const endIndex = serializeInto(
+ buffer,
+ value,
+ checkKeys,
+ index,
+ depth + 1,
+ serializeFunctions,
+ ignoreUndefined,
+ path
+ );
+ // Pop stack
+ path.pop();
+ return endIndex;
+}
+
+function serializeDecimal128(
+ buffer: Buffer,
+ key: string,
+ value: Decimal128,
+ index: number,
+ isArray?: boolean
+) {
+ buffer[index++] = constants.BSON_DATA_DECIMAL128;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the data from the value
+ // Prefer the standard JS methods because their typechecking is not buggy,
+ // unlike the `buffer` polyfill's.
+ buffer.set(value.bytes.subarray(0, 16), index);
+ return index + 16;
+}
+
+function serializeLong(buffer: Buffer, key: string, value: Long, index: number, isArray?: boolean) {
+ // Write the type
+ buffer[index++] =
+ value._bsontype === 'Long' ? constants.BSON_DATA_LONG : constants.BSON_DATA_TIMESTAMP;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the date
+ const lowBits = value.getLowBits();
+ const highBits = value.getHighBits();
+ // Encode low bits
+ buffer[index++] = lowBits & 0xff;
+ buffer[index++] = (lowBits >> 8) & 0xff;
+ buffer[index++] = (lowBits >> 16) & 0xff;
+ buffer[index++] = (lowBits >> 24) & 0xff;
+ // Encode high bits
+ buffer[index++] = highBits & 0xff;
+ buffer[index++] = (highBits >> 8) & 0xff;
+ buffer[index++] = (highBits >> 16) & 0xff;
+ buffer[index++] = (highBits >> 24) & 0xff;
+ return index;
+}
+
+function serializeInt32(
+ buffer: Buffer,
+ key: string,
+ value: Int32 | number,
+ index: number,
+ isArray?: boolean
+) {
+ value = value.valueOf();
+ // Set int type 32 bits or less
+ buffer[index++] = constants.BSON_DATA_INT;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the int value
+ buffer[index++] = value & 0xff;
+ buffer[index++] = (value >> 8) & 0xff;
+ buffer[index++] = (value >> 16) & 0xff;
+ buffer[index++] = (value >> 24) & 0xff;
+ return index;
+}
+
+function serializeDouble(
+ buffer: Buffer,
+ key: string,
+ value: Double,
+ index: number,
+ isArray?: boolean
+) {
+ // Encode as double
+ buffer[index++] = constants.BSON_DATA_NUMBER;
+
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+
+ // Write float
+ writeIEEE754(buffer, value.value, index, 'little', 52, 8);
+
+ // Adjust index
+ index = index + 8;
+ return index;
+}
+
+function serializeFunction(
+ buffer: Buffer,
+ key: string,
+ value: Function,
+ index: number,
+ _checkKeys = false,
+ _depth = 0,
+ isArray?: boolean
+) {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ const functionString = normalizedFunctionString(value);
+
+ // Write the string
+ const size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ return index;
+}
+
+function serializeCode(
+ buffer: Buffer,
+ key: string,
+ value: Code,
+ index: number,
+ checkKeys = false,
+ depth = 0,
+ serializeFunctions = false,
+ ignoreUndefined = true,
+ isArray = false
+) {
+ if (value.scope && typeof value.scope === 'object') {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_CODE_W_SCOPE;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+
+ // Starting index
+ let startIndex = index;
+
+ // Serialize the function
+ // Get the function string
+ const functionString = typeof value.code === 'string' ? value.code : value.code.toString();
+ // Index adjustment
+ index = index + 4;
+ // Write string into buffer
+ const codeSize = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = codeSize & 0xff;
+ buffer[index + 1] = (codeSize >> 8) & 0xff;
+ buffer[index + 2] = (codeSize >> 16) & 0xff;
+ buffer[index + 3] = (codeSize >> 24) & 0xff;
+ // Write end 0
+ buffer[index + 4 + codeSize - 1] = 0;
+ // Write the
+ index = index + codeSize + 4;
+
+ //
+ // Serialize the scope value
+ const endIndex = serializeInto(
+ buffer,
+ value.scope,
+ checkKeys,
+ index,
+ depth + 1,
+ serializeFunctions,
+ ignoreUndefined
+ );
+ index = endIndex - 1;
+
+ // Writ the total
+ const totalSize = endIndex - startIndex;
+
+ // Write the total size of the object
+ buffer[startIndex++] = totalSize & 0xff;
+ buffer[startIndex++] = (totalSize >> 8) & 0xff;
+ buffer[startIndex++] = (totalSize >> 16) & 0xff;
+ buffer[startIndex++] = (totalSize >> 24) & 0xff;
+ // Write trailing zero
+ buffer[index++] = 0;
+ } else {
+ buffer[index++] = constants.BSON_DATA_CODE;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Function string
+ const functionString = value.code.toString();
+ // Write the string
+ const size = buffer.write(functionString, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0;
+ }
+
+ return index;
+}
+
+function serializeBinary(
+ buffer: Buffer,
+ key: string,
+ value: Binary,
+ index: number,
+ isArray?: boolean
+) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_BINARY;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Extract the buffer
+ const data = value.value(true) as Buffer | Uint8Array;
+ // Calculate size
+ let size = value.position;
+ // Add the deprecated 02 type 4 bytes of size to total
+ if (value.sub_type === Binary.SUBTYPE_BYTE_ARRAY) size = size + 4;
+ // Write the size of the string to buffer
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ // Write the subtype to the buffer
+ buffer[index++] = value.sub_type;
+
+ // If we have binary type 2 the 4 first bytes are the size
+ if (value.sub_type === Binary.SUBTYPE_BYTE_ARRAY) {
+ size = size - 4;
+ buffer[index++] = size & 0xff;
+ buffer[index++] = (size >> 8) & 0xff;
+ buffer[index++] = (size >> 16) & 0xff;
+ buffer[index++] = (size >> 24) & 0xff;
+ }
+
+ // Write the data to the object
+ buffer.set(data, index);
+ // Adjust the index
+ index = index + value.position;
+ return index;
+}
+
+function serializeSymbol(
+ buffer: Buffer,
+ key: string,
+ value: BSONSymbol,
+ index: number,
+ isArray?: boolean
+) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_SYMBOL;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+ // Write the string
+ const size = buffer.write(value.value, index + 4, undefined, 'utf8') + 1;
+ // Write the size of the string to buffer
+ buffer[index] = size & 0xff;
+ buffer[index + 1] = (size >> 8) & 0xff;
+ buffer[index + 2] = (size >> 16) & 0xff;
+ buffer[index + 3] = (size >> 24) & 0xff;
+ // Update index
+ index = index + 4 + size - 1;
+ // Write zero
+ buffer[index++] = 0x00;
+ return index;
+}
+
+function serializeDBRef(
+ buffer: Buffer,
+ key: string,
+ value: DBRef,
+ index: number,
+ depth: number,
+ serializeFunctions: boolean,
+ isArray?: boolean
+) {
+ // Write the type
+ buffer[index++] = constants.BSON_DATA_OBJECT;
+ // Number of written bytes
+ const numberOfWrittenBytes = !isArray
+ ? buffer.write(key, index, undefined, 'utf8')
+ : buffer.write(key, index, undefined, 'ascii');
+
+ // Encode the name
+ index = index + numberOfWrittenBytes;
+ buffer[index++] = 0;
+
+ let startIndex = index;
+ let output: DBRefLike = {
+ $ref: value.collection || value.namespace, // "namespace" was what library 1.x called "collection"
+ $id: value.oid
+ };
+
+ if (value.db != null) {
+ output.$db = value.db;
+ }
+
+ output = Object.assign(output, value.fields);
+ const endIndex = serializeInto(buffer, output, false, index, depth + 1, serializeFunctions);
+
+ // Calculate object size
+ const size = endIndex - startIndex;
+ // Write the size
+ buffer[startIndex++] = size & 0xff;
+ buffer[startIndex++] = (size >> 8) & 0xff;
+ buffer[startIndex++] = (size >> 16) & 0xff;
+ buffer[startIndex++] = (size >> 24) & 0xff;
+ // Set index
+ return endIndex;
+}
+
+export function serializeInto(
+ buffer: Buffer,
+ object: Document,
+ checkKeys = false,
+ startingIndex = 0,
+ depth = 0,
+ serializeFunctions = false,
+ ignoreUndefined = true,
+ path: Document[] = []
+): number {
+ startingIndex = startingIndex || 0;
+ path = path || [];
+
+ // Push the object to the path
+ path.push(object);
+
+ // Start place to serialize into
+ let index = startingIndex + 4;
+
+ // Special case isArray
+ if (Array.isArray(object)) {
+ // Get object keys
+ for (let i = 0; i < object.length; i++) {
+ const key = '' + i;
+ let value = object[i];
+
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function') throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+
+ if (typeof value === 'string') {
+ index = serializeString(buffer, key, value, index, true);
+ } else if (typeof value === 'number') {
+ index = serializeNumber(buffer, key, value, index, true);
+ } else if (typeof value === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ } else if (typeof value === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index, true);
+ } else if (value instanceof Date || isDate(value)) {
+ index = serializeDate(buffer, key, value, index, true);
+ } else if (value === undefined) {
+ index = serializeNull(buffer, key, value, index, true);
+ } else if (value === null) {
+ index = serializeNull(buffer, key, value, index, true);
+ } else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index, true);
+ } else if (isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index, true);
+ } else if (value instanceof RegExp || isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index, true);
+ } else if (typeof value === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(
+ buffer,
+ key,
+ value,
+ index,
+ checkKeys,
+ depth,
+ serializeFunctions,
+ ignoreUndefined,
+ true,
+ path
+ );
+ } else if (
+ typeof value === 'object' &&
+ isBSONType(value) &&
+ value._bsontype === 'Decimal128'
+ ) {
+ index = serializeDecimal128(buffer, key, value, index, true);
+ } else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index, true);
+ } else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index, true);
+ } else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, true);
+ } else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(
+ buffer,
+ key,
+ value,
+ index,
+ checkKeys,
+ depth,
+ serializeFunctions,
+ ignoreUndefined,
+ true
+ );
+ } else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index, true);
+ } else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index, true);
+ } else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions, true);
+ } else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index, true);
+ } else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index, true);
+ } else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index, true);
+ } else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ } else if (object instanceof Map || isMap(object)) {
+ const iterator = object.entries();
+ let done = false;
+
+ while (!done) {
+ // Unpack the next entry
+ const entry = iterator.next();
+ done = !!entry.done;
+ // Are we done, then skip and terminate
+ if (done) continue;
+
+ // Get the entry values
+ const key = entry.value[0];
+ const value = entry.value[1];
+
+ // Check the type of the value
+ const type = typeof value;
+
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ } else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ } else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ } else if (type === 'bigint' || isBigInt64Array(value) || isBigUInt64Array(value)) {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ } else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ } else if (value instanceof Date || isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ } else if (value === null || (value === undefined && ignoreUndefined === false)) {
+ index = serializeNull(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ } else if (isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ } else if (value instanceof RegExp || isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ } else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(
+ buffer,
+ key,
+ value,
+ index,
+ checkKeys,
+ depth,
+ serializeFunctions,
+ ignoreUndefined,
+ false,
+ path
+ );
+ } else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(
+ buffer,
+ key,
+ value,
+ index,
+ checkKeys,
+ depth,
+ serializeFunctions,
+ ignoreUndefined
+ );
+ } else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ } else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ } else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ } else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ } else {
+ // Did we provide a custom serialization method
+ if (object.toBSON) {
+ if (typeof object.toBSON !== 'function') throw new TypeError('toBSON is not a function');
+ object = object.toBSON();
+ if (object != null && typeof object !== 'object')
+ throw new TypeError('toBSON function did not return an object');
+ }
+
+ // Iterate over all the keys
+ for (const key in object) {
+ let value = object[key];
+ // Is there an override value
+ if (value && value.toBSON) {
+ if (typeof value.toBSON !== 'function') throw new TypeError('toBSON is not a function');
+ value = value.toBSON();
+ }
+
+ // Check the type of the value
+ const type = typeof value;
+
+ // Check the key and throw error if it's illegal
+ if (typeof key === 'string' && !ignoreKeys.has(key)) {
+ if (key.match(regexp) != null) {
+ // The BSON spec doesn't allow keys with null bytes because keys are
+ // null-terminated.
+ throw Error('key ' + key + ' must not contain null bytes');
+ }
+
+ if (checkKeys) {
+ if ('$' === key[0]) {
+ throw Error('key ' + key + " must not start with '$'");
+ } else if (~key.indexOf('.')) {
+ throw Error('key ' + key + " must not contain '.'");
+ }
+ }
+ }
+
+ if (type === 'string') {
+ index = serializeString(buffer, key, value, index);
+ } else if (type === 'number') {
+ index = serializeNumber(buffer, key, value, index);
+ } else if (type === 'bigint') {
+ throw new TypeError('Unsupported type BigInt, please use Decimal128');
+ } else if (type === 'boolean') {
+ index = serializeBoolean(buffer, key, value, index);
+ } else if (value instanceof Date || isDate(value)) {
+ index = serializeDate(buffer, key, value, index);
+ } else if (value === undefined) {
+ if (ignoreUndefined === false) index = serializeNull(buffer, key, value, index);
+ } else if (value === null) {
+ index = serializeNull(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'ObjectId' || value['_bsontype'] === 'ObjectID') {
+ index = serializeObjectId(buffer, key, value, index);
+ } else if (isUint8Array(value)) {
+ index = serializeBuffer(buffer, key, value, index);
+ } else if (value instanceof RegExp || isRegExp(value)) {
+ index = serializeRegExp(buffer, key, value, index);
+ } else if (type === 'object' && value['_bsontype'] == null) {
+ index = serializeObject(
+ buffer,
+ key,
+ value,
+ index,
+ checkKeys,
+ depth,
+ serializeFunctions,
+ ignoreUndefined,
+ false,
+ path
+ );
+ } else if (type === 'object' && value['_bsontype'] === 'Decimal128') {
+ index = serializeDecimal128(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'Long' || value['_bsontype'] === 'Timestamp') {
+ index = serializeLong(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'Double') {
+ index = serializeDouble(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'Code') {
+ index = serializeCode(
+ buffer,
+ key,
+ value,
+ index,
+ checkKeys,
+ depth,
+ serializeFunctions,
+ ignoreUndefined
+ );
+ } else if (typeof value === 'function' && serializeFunctions) {
+ index = serializeFunction(buffer, key, value, index, checkKeys, depth, serializeFunctions);
+ } else if (value['_bsontype'] === 'Binary') {
+ index = serializeBinary(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'Symbol') {
+ index = serializeSymbol(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'DBRef') {
+ index = serializeDBRef(buffer, key, value, index, depth, serializeFunctions);
+ } else if (value['_bsontype'] === 'BSONRegExp') {
+ index = serializeBSONRegExp(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'Int32') {
+ index = serializeInt32(buffer, key, value, index);
+ } else if (value['_bsontype'] === 'MinKey' || value['_bsontype'] === 'MaxKey') {
+ index = serializeMinMax(buffer, key, value, index);
+ } else if (typeof value['_bsontype'] !== 'undefined') {
+ throw new TypeError('Unrecognized or invalid _bsontype: ' + value['_bsontype']);
+ }
+ }
+ }
+
+ // Remove the path
+ path.pop();
+
+ // Final padding byte for object
+ buffer[index++] = 0x00;
+
+ // Final size
+ const size = index - startingIndex;
+ // Write the size of the object
+ buffer[startingIndex++] = size & 0xff;
+ buffer[startingIndex++] = (size >> 8) & 0xff;
+ buffer[startingIndex++] = (size >> 16) & 0xff;
+ buffer[startingIndex++] = (size >> 24) & 0xff;
+ return index;
+}
diff --git a/node_modules/bson/src/parser/utils.ts b/node_modules/bson/src/parser/utils.ts
new file mode 100644
index 0000000..3f0dcc7
--- /dev/null
+++ b/node_modules/bson/src/parser/utils.ts
@@ -0,0 +1,125 @@
+import { Buffer } from 'buffer';
+
+type RandomBytesFunction = (size: number) => Uint8Array;
+
+/**
+ * Normalizes our expected stringified form of a function across versions of node
+ * @param fn - The function to stringify
+ */
+export function normalizedFunctionString(fn: Function): string {
+ return fn.toString().replace('function(', 'function (');
+}
+
+const isReactNative =
+ typeof global.navigator === 'object' && global.navigator.product === 'ReactNative';
+
+const insecureWarning = isReactNative
+ ? 'BSON: For React Native please polyfill crypto.getRandomValues, e.g. using: https://www.npmjs.com/package/react-native-get-random-values.'
+ : 'BSON: No cryptographic implementation for random bytes present, falling back to a less secure implementation.';
+
+const insecureRandomBytes: RandomBytesFunction = function insecureRandomBytes(size: number) {
+ console.warn(insecureWarning);
+
+ const result = Buffer.alloc(size);
+ for (let i = 0; i < size; ++i) result[i] = Math.floor(Math.random() * 256);
+ return result;
+};
+
+/* We do not want to have to include DOM types just for this check */
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+declare let window: any;
+declare let require: Function;
+// eslint-disable-next-line @typescript-eslint/no-explicit-any
+declare let global: any;
+declare const self: unknown;
+
+const detectRandomBytes = (): RandomBytesFunction => {
+ if (typeof window !== 'undefined') {
+ // browser crypto implementation(s)
+ const target = window.crypto || window.msCrypto; // allow for IE11
+ if (target && target.getRandomValues) {
+ return size => target.getRandomValues(Buffer.alloc(size));
+ }
+ }
+
+ if (typeof global !== 'undefined' && global.crypto && global.crypto.getRandomValues) {
+ // allow for RN packages such as https://www.npmjs.com/package/react-native-get-random-values to populate global
+ return size => global.crypto.getRandomValues(Buffer.alloc(size));
+ }
+
+ let requiredRandomBytes: RandomBytesFunction | null | undefined;
+ try {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ requiredRandomBytes = require('crypto').randomBytes;
+ } catch (e) {
+ // keep the fallback
+ }
+
+ // NOTE: in transpiled cases the above require might return null/undefined
+
+ return requiredRandomBytes || insecureRandomBytes;
+};
+
+export const randomBytes = detectRandomBytes();
+
+export function isAnyArrayBuffer(value: unknown): value is ArrayBuffer {
+ return ['[object ArrayBuffer]', '[object SharedArrayBuffer]'].includes(
+ Object.prototype.toString.call(value)
+ );
+}
+
+export function isUint8Array(value: unknown): value is Uint8Array {
+ return Object.prototype.toString.call(value) === '[object Uint8Array]';
+}
+
+export function isBigInt64Array(value: unknown): value is BigInt64Array {
+ return Object.prototype.toString.call(value) === '[object BigInt64Array]';
+}
+
+export function isBigUInt64Array(value: unknown): value is BigUint64Array {
+ return Object.prototype.toString.call(value) === '[object BigUint64Array]';
+}
+
+export function isRegExp(d: unknown): d is RegExp {
+ return Object.prototype.toString.call(d) === '[object RegExp]';
+}
+
+export function isMap(d: unknown): d is Map {
+ return Object.prototype.toString.call(d) === '[object Map]';
+}
+
+/** Call to check if your environment has `Buffer` */
+export function haveBuffer(): boolean {
+ return typeof global !== 'undefined' && typeof global.Buffer !== 'undefined';
+}
+
+// To ensure that 0.4 of node works correctly
+export function isDate(d: unknown): d is Date {
+ return isObjectLike(d) && Object.prototype.toString.call(d) === '[object Date]';
+}
+
+/**
+ * @internal
+ * this is to solve the `'someKey' in x` problem where x is unknown.
+ * https://github.com/typescript-eslint/typescript-eslint/issues/1071#issuecomment-541955753
+ */
+export function isObjectLike(candidate: unknown): candidate is Record {
+ return typeof candidate === 'object' && candidate !== null;
+}
+
+declare let console: { warn(...message: unknown[]): void };
+export function deprecate(fn: T, message: string): T {
+ if (typeof window === 'undefined' && typeof self === 'undefined') {
+ // eslint-disable-next-line @typescript-eslint/no-var-requires
+ return require('util').deprecate(fn, message);
+ }
+ let warned = false;
+ function deprecated(this: unknown, ...args: unknown[]) {
+ if (!warned) {
+ console.warn(message);
+ warned = true;
+ }
+ return fn.apply(this, args);
+ }
+ return (deprecated as unknown) as T;
+}
diff --git a/node_modules/bson/src/regexp.ts b/node_modules/bson/src/regexp.ts
new file mode 100644
index 0000000..76d1f5e
--- /dev/null
+++ b/node_modules/bson/src/regexp.ts
@@ -0,0 +1,92 @@
+import type { EJSONOptions } from './extended_json';
+
+function alphabetize(str: string): string {
+ return str.split('').sort().join('');
+}
+
+/** @public */
+export interface BSONRegExpExtendedLegacy {
+ $regex: string | BSONRegExp;
+ $options: string;
+}
+
+/** @public */
+export interface BSONRegExpExtended {
+ $regularExpression: {
+ pattern: string;
+ options: string;
+ };
+}
+
+/**
+ * A class representation of the BSON RegExp type.
+ * @public
+ */
+export class BSONRegExp {
+ _bsontype!: 'BSONRegExp';
+
+ pattern!: string;
+ options!: string;
+ /**
+ * @param pattern - The regular expression pattern to match
+ * @param options - The regular expression options
+ */
+ constructor(pattern: string, options?: string) {
+ if (!(this instanceof BSONRegExp)) return new BSONRegExp(pattern, options);
+
+ this.pattern = pattern;
+ this.options = alphabetize(options ?? '');
+
+ // Validate options
+ for (let i = 0; i < this.options.length; i++) {
+ if (
+ !(
+ this.options[i] === 'i' ||
+ this.options[i] === 'm' ||
+ this.options[i] === 'x' ||
+ this.options[i] === 'l' ||
+ this.options[i] === 's' ||
+ this.options[i] === 'u'
+ )
+ ) {
+ throw new Error(`The regular expression option [${this.options[i]}] is not supported`);
+ }
+ }
+ }
+
+ static parseOptions(options?: string): string {
+ return options ? options.split('').sort().join('') : '';
+ }
+
+ /** @internal */
+ toExtendedJSON(options?: EJSONOptions): BSONRegExpExtendedLegacy | BSONRegExpExtended {
+ options = options || {};
+ if (options.legacy) {
+ return { $regex: this.pattern, $options: this.options };
+ }
+ return { $regularExpression: { pattern: this.pattern, options: this.options } };
+ }
+
+ /** @internal */
+ static fromExtendedJSON(doc: BSONRegExpExtendedLegacy | BSONRegExpExtended): BSONRegExp {
+ if ('$regex' in doc) {
+ if (typeof doc.$regex !== 'string') {
+ // This is for $regex query operators that have extended json values.
+ if (doc.$regex._bsontype === 'BSONRegExp') {
+ return (doc as unknown) as BSONRegExp;
+ }
+ } else {
+ return new BSONRegExp(doc.$regex, BSONRegExp.parseOptions(doc.$options));
+ }
+ }
+ if ('$regularExpression' in doc) {
+ return new BSONRegExp(
+ doc.$regularExpression.pattern,
+ BSONRegExp.parseOptions(doc.$regularExpression.options)
+ );
+ }
+ throw new TypeError(`Unexpected BSONRegExp EJSON object form: ${JSON.stringify(doc)}`);
+ }
+}
+
+Object.defineProperty(BSONRegExp.prototype, '_bsontype', { value: 'BSONRegExp' });
diff --git a/node_modules/bson/src/symbol.ts b/node_modules/bson/src/symbol.ts
new file mode 100644
index 0000000..8dcb241
--- /dev/null
+++ b/node_modules/bson/src/symbol.ts
@@ -0,0 +1,59 @@
+/** @public */
+export interface BSONSymbolExtended {
+ $symbol: string;
+}
+
+/**
+ * A class representation of the BSON Symbol type.
+ * @public
+ */
+export class BSONSymbol {
+ _bsontype!: 'Symbol';
+
+ value!: string;
+ /**
+ * @param value - the string representing the symbol.
+ */
+ constructor(value: string) {
+ if (!(this instanceof BSONSymbol)) return new BSONSymbol(value);
+
+ this.value = value;
+ }
+
+ /** Access the wrapped string value. */
+ valueOf(): string {
+ return this.value;
+ }
+
+ /** @internal */
+ toString(): string {
+ return this.value;
+ }
+
+ /** @internal */
+ inspect(): string {
+ return `new BSONSymbol("${this.value}")`;
+ }
+
+ /** @internal */
+ toJSON(): string {
+ return this.value;
+ }
+
+ /** @internal */
+ toExtendedJSON(): BSONSymbolExtended {
+ return { $symbol: this.value };
+ }
+
+ /** @internal */
+ static fromExtendedJSON(doc: BSONSymbolExtended): BSONSymbol {
+ return new BSONSymbol(doc.$symbol);
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+}
+
+Object.defineProperty(BSONSymbol.prototype, '_bsontype', { value: 'Symbol' });
diff --git a/node_modules/bson/src/timestamp.ts b/node_modules/bson/src/timestamp.ts
new file mode 100644
index 0000000..d20b6b5
--- /dev/null
+++ b/node_modules/bson/src/timestamp.ts
@@ -0,0 +1,108 @@
+import { Long } from './long';
+
+/** @public */
+export type TimestampOverrides = '_bsontype' | 'toExtendedJSON' | 'fromExtendedJSON' | 'inspect';
+/** @public */
+export type LongWithoutOverrides = new (low: number | Long, high?: number, unsigned?: boolean) => {
+ [P in Exclude]: Long[P];
+};
+/** @public */
+export const LongWithoutOverridesClass: LongWithoutOverrides = (Long as unknown) as LongWithoutOverrides;
+
+/** @public */
+export interface TimestampExtended {
+ $timestamp: {
+ t: number;
+ i: number;
+ };
+}
+
+/** @public */
+export class Timestamp extends LongWithoutOverridesClass {
+ _bsontype!: 'Timestamp';
+
+ static readonly MAX_VALUE = Long.MAX_UNSIGNED_VALUE;
+
+ /**
+ * @param low - A 64-bit Long representing the Timestamp.
+ */
+ constructor(low: Long);
+ /**
+ * @param low - the low (signed) 32 bits of the Timestamp.
+ * @param high - the high (signed) 32 bits of the Timestamp.
+ */
+ constructor(low: Long);
+ constructor(low: number, high: number);
+ constructor(low: number | Long, high?: number) {
+ // eslint-disable-next-line @typescript-eslint/ban-ts-comment
+ ///@ts-expect-error
+ if (!(this instanceof Timestamp)) return new Timestamp(low, high);
+
+ if (Long.isLong(low)) {
+ super(low.low, low.high, true);
+ } else {
+ super(low, high, true);
+ }
+ Object.defineProperty(this, '_bsontype', {
+ value: 'Timestamp',
+ writable: false,
+ configurable: false,
+ enumerable: false
+ });
+ }
+
+ toJSON(): { $timestamp: string } {
+ return {
+ $timestamp: this.toString()
+ };
+ }
+
+ /** Returns a Timestamp represented by the given (32-bit) integer value. */
+ static fromInt(value: number): Timestamp {
+ return new Timestamp(Long.fromInt(value, true));
+ }
+
+ /** Returns a Timestamp representing the given number value, provided that it is a finite number. Otherwise, zero is returned. */
+ static fromNumber(value: number): Timestamp {
+ return new Timestamp(Long.fromNumber(value, true));
+ }
+
+ /**
+ * Returns a Timestamp for the given high and low bits. Each is assumed to use 32 bits.
+ *
+ * @param lowBits - the low 32-bits.
+ * @param highBits - the high 32-bits.
+ */
+ static fromBits(lowBits: number, highBits: number): Timestamp {
+ return new Timestamp(lowBits, highBits);
+ }
+
+ /**
+ * Returns a Timestamp from the given string, optionally using the given radix.
+ *
+ * @param str - the textual representation of the Timestamp.
+ * @param optRadix - the radix in which the text is written.
+ */
+ static fromString(str: string, optRadix: number): Timestamp {
+ return new Timestamp(Long.fromString(str, true, optRadix));
+ }
+
+ /** @internal */
+ toExtendedJSON(): TimestampExtended {
+ return { $timestamp: { t: this.high >>> 0, i: this.low >>> 0 } };
+ }
+
+ /** @internal */
+ static fromExtendedJSON(doc: TimestampExtended): Timestamp {
+ return new Timestamp(doc.$timestamp.i, doc.$timestamp.t);
+ }
+
+ /** @internal */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ return `new Timestamp(${this.getLowBits().toString()}, ${this.getHighBits().toString()})`;
+ }
+}
diff --git a/node_modules/bson/src/uuid.ts b/node_modules/bson/src/uuid.ts
new file mode 100644
index 0000000..1ac61c5
--- /dev/null
+++ b/node_modules/bson/src/uuid.ts
@@ -0,0 +1,209 @@
+import { Buffer } from 'buffer';
+import { ensureBuffer } from './ensure_buffer';
+import { Binary } from './binary';
+import { bufferToUuidHexString, uuidHexStringToBuffer, uuidValidateString } from './uuid_utils';
+import { isUint8Array, randomBytes } from './parser/utils';
+
+/** @public */
+export type UUIDExtended = {
+ $uuid: string;
+};
+
+const BYTE_LENGTH = 16;
+
+const kId = Symbol('id');
+
+/**
+ * A class representation of the BSON UUID type.
+ * @public
+ */
+export class UUID {
+ // This property is not meant for direct serialization, but simply an indication that this type originates from this package.
+ _bsontype!: 'UUID';
+
+ static cacheHexString: boolean;
+
+ /** UUID Bytes @internal */
+ private [kId]: Buffer;
+ /** UUID hexString cache @internal */
+ private __id?: string;
+
+ /**
+ * Create an UUID type
+ *
+ * @param input - Can be a 32 or 36 character hex string (dashes excluded/included) or a 16 byte binary Buffer.
+ */
+ constructor(input?: string | Buffer | UUID) {
+ if (typeof input === 'undefined') {
+ // The most common use case (blank id, new UUID() instance)
+ this.id = UUID.generate();
+ } else if (input instanceof UUID) {
+ this[kId] = Buffer.from(input.id);
+ this.__id = input.__id;
+ } else if (ArrayBuffer.isView(input) && input.byteLength === BYTE_LENGTH) {
+ this.id = ensureBuffer(input);
+ } else if (typeof input === 'string') {
+ this.id = uuidHexStringToBuffer(input);
+ } else {
+ throw new TypeError(
+ 'Argument passed in UUID constructor must be a UUID, a 16 byte Buffer or a 32/36 character hex string (dashes excluded/included, format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx).'
+ );
+ }
+ }
+
+ /**
+ * The UUID bytes
+ * @readonly
+ */
+ get id(): Buffer {
+ return this[kId];
+ }
+
+ set id(value: Buffer) {
+ this[kId] = value;
+
+ if (UUID.cacheHexString) {
+ this.__id = bufferToUuidHexString(value);
+ }
+ }
+
+ /**
+ * Generate a 16 byte uuid v4 buffer used in UUIDs
+ */
+
+ /**
+ * Returns the UUID id as a 32 or 36 character hex string representation, excluding/including dashes (defaults to 36 character dash separated)
+ * @param includeDashes - should the string exclude dash-separators.
+ * */
+ toHexString(includeDashes = true): string {
+ if (UUID.cacheHexString && this.__id) {
+ return this.__id;
+ }
+
+ const uuidHexString = bufferToUuidHexString(this.id, includeDashes);
+
+ if (UUID.cacheHexString) {
+ this.__id = uuidHexString;
+ }
+
+ return uuidHexString;
+ }
+
+ /**
+ * Converts the id into a 36 character (dashes included) hex string, unless a encoding is specified.
+ * @internal
+ */
+ toString(encoding?: string): string {
+ return encoding ? this.id.toString(encoding) : this.toHexString();
+ }
+
+ /**
+ * Converts the id into its JSON string representation. A 36 character (dashes included) hex string in the format: xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx
+ * @internal
+ */
+ toJSON(): string {
+ return this.toHexString();
+ }
+
+ /**
+ * Compares the equality of this UUID with `otherID`.
+ *
+ * @param otherId - UUID instance to compare against.
+ */
+ equals(otherId: string | Buffer | UUID): boolean {
+ if (!otherId) {
+ return false;
+ }
+
+ if (otherId instanceof UUID) {
+ return otherId.id.equals(this.id);
+ }
+
+ try {
+ return new UUID(otherId).id.equals(this.id);
+ } catch {
+ return false;
+ }
+ }
+
+ /**
+ * Creates a Binary instance from the current UUID.
+ */
+ toBinary(): Binary {
+ return new Binary(this.id, Binary.SUBTYPE_UUID);
+ }
+
+ /**
+ * Generates a populated buffer containing a v4 uuid
+ */
+ static generate(): Buffer {
+ const bytes = randomBytes(BYTE_LENGTH);
+
+ // Per 4.4, set bits for version and `clock_seq_hi_and_reserved`
+ // Kindly borrowed from https://github.com/uuidjs/uuid/blob/master/src/v4.js
+ bytes[6] = (bytes[6] & 0x0f) | 0x40;
+ bytes[8] = (bytes[8] & 0x3f) | 0x80;
+
+ return Buffer.from(bytes);
+ }
+
+ /**
+ * Checks if a value is a valid bson UUID
+ * @param input - UUID, string or Buffer to validate.
+ */
+ static isValid(input: string | Buffer | UUID): boolean {
+ if (!input) {
+ return false;
+ }
+
+ if (input instanceof UUID) {
+ return true;
+ }
+
+ if (typeof input === 'string') {
+ return uuidValidateString(input);
+ }
+
+ if (isUint8Array(input)) {
+ // check for length & uuid version (https://tools.ietf.org/html/rfc4122#section-4.1.3)
+ if (input.length !== BYTE_LENGTH) {
+ return false;
+ }
+
+ try {
+ // get this byte as hex: xxxxxxxx-xxxx-XXxx-xxxx-xxxxxxxxxxxx
+ // check first part as uuid version: xxxxxxxx-xxxx-Xxxx-xxxx-xxxxxxxxxxxx
+ return parseInt(input[6].toString(16)[0], 10) === Binary.SUBTYPE_UUID;
+ } catch {
+ return false;
+ }
+ }
+
+ return false;
+ }
+
+ /**
+ * Creates an UUID from a hex string representation of an UUID.
+ * @param hexString - 32 or 36 character hex string (dashes excluded/included).
+ */
+ static createFromHexString(hexString: string): UUID {
+ const buffer = uuidHexStringToBuffer(hexString);
+ return new UUID(buffer);
+ }
+
+ /**
+ * Converts to a string representation of this Id.
+ *
+ * @returns return the 36 character hex string representation.
+ * @internal
+ */
+ [Symbol.for('nodejs.util.inspect.custom')](): string {
+ return this.inspect();
+ }
+
+ inspect(): string {
+ return `new UUID("${this.toHexString()}")`;
+ }
+}
+
+Object.defineProperty(UUID.prototype, '_bsontype', { value: 'UUID' });
diff --git a/node_modules/bson/src/uuid_utils.ts b/node_modules/bson/src/uuid_utils.ts
new file mode 100644
index 0000000..5c5166c
--- /dev/null
+++ b/node_modules/bson/src/uuid_utils.ts
@@ -0,0 +1,31 @@
+import { Buffer } from 'buffer';
+
+// Validation regex for v4 uuid (validates with or without dashes)
+const VALIDATION_REGEX = /^(?:[0-9a-f]{8}-[0-9a-f]{4}-4[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}|[0-9a-f]{12}4[0-9a-f]{3}[89ab][0-9a-f]{15})$/i;
+
+export const uuidValidateString = (str: string): boolean =>
+ typeof str === 'string' && VALIDATION_REGEX.test(str);
+
+export const uuidHexStringToBuffer = (hexString: string): Buffer => {
+ if (!uuidValidateString(hexString)) {
+ throw new TypeError(
+ 'UUID string representations must be a 32 or 36 character hex string (dashes excluded/included). Format: "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx" or "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx".'
+ );
+ }
+
+ const sanitizedHexString = hexString.replace(/-/g, '');
+ return Buffer.from(sanitizedHexString, 'hex');
+};
+
+export const bufferToUuidHexString = (buffer: Buffer, includeDashes = true): string =>
+ includeDashes
+ ? buffer.toString('hex', 0, 4) +
+ '-' +
+ buffer.toString('hex', 4, 6) +
+ '-' +
+ buffer.toString('hex', 6, 8) +
+ '-' +
+ buffer.toString('hex', 8, 10) +
+ '-' +
+ buffer.toString('hex', 10, 16)
+ : buffer.toString('hex');
diff --git a/node_modules/bson/src/validate_utf8.ts b/node_modules/bson/src/validate_utf8.ts
new file mode 100644
index 0000000..e1da934
--- /dev/null
+++ b/node_modules/bson/src/validate_utf8.ts
@@ -0,0 +1,47 @@
+const FIRST_BIT = 0x80;
+const FIRST_TWO_BITS = 0xc0;
+const FIRST_THREE_BITS = 0xe0;
+const FIRST_FOUR_BITS = 0xf0;
+const FIRST_FIVE_BITS = 0xf8;
+
+const TWO_BIT_CHAR = 0xc0;
+const THREE_BIT_CHAR = 0xe0;
+const FOUR_BIT_CHAR = 0xf0;
+const CONTINUING_CHAR = 0x80;
+
+/**
+ * Determines if the passed in bytes are valid utf8
+ * @param bytes - An array of 8-bit bytes. Must be indexable and have length property
+ * @param start - The index to start validating
+ * @param end - The index to end validating
+ */
+export function validateUtf8(
+ bytes: { [index: number]: number },
+ start: number,
+ end: number
+): boolean {
+ let continuation = 0;
+
+ for (let i = start; i < end; i += 1) {
+ const byte = bytes[i];
+
+ if (continuation) {
+ if ((byte & FIRST_TWO_BITS) !== CONTINUING_CHAR) {
+ return false;
+ }
+ continuation -= 1;
+ } else if (byte & FIRST_BIT) {
+ if ((byte & FIRST_THREE_BITS) === TWO_BIT_CHAR) {
+ continuation = 1;
+ } else if ((byte & FIRST_FOUR_BITS) === THREE_BIT_CHAR) {
+ continuation = 2;
+ } else if ((byte & FIRST_FIVE_BITS) === FOUR_BIT_CHAR) {
+ continuation = 3;
+ } else {
+ return false;
+ }
+ }
+ }
+
+ return !continuation;
+}
diff --git a/node_modules/buffer/AUTHORS.md b/node_modules/buffer/AUTHORS.md
new file mode 100644
index 0000000..22eb171
--- /dev/null
+++ b/node_modules/buffer/AUTHORS.md
@@ -0,0 +1,70 @@
+# Authors
+
+#### Ordered by first contribution.
+
+- Romain Beauxis (toots@rastageeks.org)
+- Tobias Koppers (tobias.koppers@googlemail.com)
+- Janus (ysangkok@gmail.com)
+- Rainer Dreyer (rdrey1@gmail.com)
+- Tõnis Tiigi (tonistiigi@gmail.com)
+- James Halliday (mail@substack.net)
+- Michael Williamson (mike@zwobble.org)
+- elliottcable (github@elliottcable.name)
+- rafael (rvalle@livelens.net)
+- Andrew Kelley (superjoe30@gmail.com)
+- Andreas Madsen (amwebdk@gmail.com)
+- Mike Brevoort (mike.brevoort@pearson.com)
+- Brian White (mscdex@mscdex.net)
+- Feross Aboukhadijeh (feross@feross.org)
+- Ruben Verborgh (ruben@verborgh.org)
+- eliang (eliang.cs@gmail.com)
+- Jesse Tane (jesse.tane@gmail.com)
+- Alfonso Boza (alfonso@cloud.com)
+- Mathias Buus (mathiasbuus@gmail.com)
+- Devon Govett (devongovett@gmail.com)
+- Daniel Cousens (github@dcousens.com)
+- Joseph Dykstra (josephdykstra@gmail.com)
+- Parsha Pourkhomami (parshap+git@gmail.com)
+- Damjan Košir (damjan.kosir@gmail.com)
+- daverayment (dave.rayment@gmail.com)
+- kawanet (u-suke@kawa.net)
+- Linus Unnebäck (linus@folkdatorn.se)
+- Nolan Lawson (nolan.lawson@gmail.com)
+- Calvin Metcalf (calvin.metcalf@gmail.com)
+- Koki Takahashi (hakatasiloving@gmail.com)
+- Guy Bedford (guybedford@gmail.com)
+- Jan Schär (jscissr@gmail.com)
+- RaulTsc (tomescu.raul@gmail.com)
+- Matthieu Monsch (monsch@alum.mit.edu)
+- Dan Ehrenberg (littledan@chromium.org)
+- Kirill Fomichev (fanatid@ya.ru)
+- Yusuke Kawasaki (u-suke@kawa.net)
+- DC (dcposch@dcpos.ch)
+- John-David Dalton (john.david.dalton@gmail.com)
+- adventure-yunfei (adventure030@gmail.com)
+- Emil Bay (github@tixz.dk)
+- Sam Sudar (sudar.sam@gmail.com)
+- Volker Mische (volker.mische@gmail.com)
+- David Walton (support@geekstocks.com)
+- Сковорода Никита Андреевич (chalkerx@gmail.com)
+- greenkeeper[bot] (greenkeeper[bot]@users.noreply.github.com)
+- ukstv (sergey.ukustov@machinomy.com)
+- Renée Kooi (renee@kooi.me)
+- ranbochen (ranbochen@qq.com)
+- Vladimir Borovik (bobahbdb@gmail.com)
+- greenkeeper[bot] (23040076+greenkeeper[bot]@users.noreply.github.com)
+- kumavis (aaron@kumavis.me)
+- Sergey Ukustov (sergey.ukustov@machinomy.com)
+- Fei Liu (liu.feiwood@gmail.com)
+- Blaine Bublitz (blaine.bublitz@gmail.com)
+- clement (clement@seald.io)
+- Koushik Dutta (koushd@gmail.com)
+- Jordan Harband (ljharb@gmail.com)
+- Niklas Mischkulnig (mischnic@users.noreply.github.com)
+- Nikolai Vavilov (vvnicholas@gmail.com)
+- Fedor Nezhivoi (gyzerok@users.noreply.github.com)
+- Peter Newman (peternewman@users.noreply.github.com)
+- mathmakgakpak (44949126+mathmakgakpak@users.noreply.github.com)
+- jkkang (jkkang@smartauth.kr)
+
+#### Generated by bin/update-authors.sh.
diff --git a/node_modules/buffer/LICENSE b/node_modules/buffer/LICENSE
new file mode 100644
index 0000000..d6bf75d
--- /dev/null
+++ b/node_modules/buffer/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Feross Aboukhadijeh, and other contributors.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/buffer/README.md b/node_modules/buffer/README.md
new file mode 100644
index 0000000..9a23d7c
--- /dev/null
+++ b/node_modules/buffer/README.md
@@ -0,0 +1,410 @@
+# buffer [![travis][travis-image]][travis-url] [![npm][npm-image]][npm-url] [![downloads][downloads-image]][downloads-url] [![javascript style guide][standard-image]][standard-url]
+
+[travis-image]: https://img.shields.io/travis/feross/buffer/master.svg
+[travis-url]: https://travis-ci.org/feross/buffer
+[npm-image]: https://img.shields.io/npm/v/buffer.svg
+[npm-url]: https://npmjs.org/package/buffer
+[downloads-image]: https://img.shields.io/npm/dm/buffer.svg
+[downloads-url]: https://npmjs.org/package/buffer
+[standard-image]: https://img.shields.io/badge/code_style-standard-brightgreen.svg
+[standard-url]: https://standardjs.com
+
+#### The buffer module from [node.js](https://nodejs.org/), for the browser.
+
+[![saucelabs][saucelabs-image]][saucelabs-url]
+
+[saucelabs-image]: https://saucelabs.com/browser-matrix/buffer.svg
+[saucelabs-url]: https://saucelabs.com/u/buffer
+
+With [browserify](http://browserify.org), simply `require('buffer')` or use the `Buffer` global and you will get this module.
+
+The goal is to provide an API that is 100% identical to
+[node's Buffer API](https://nodejs.org/api/buffer.html). Read the
+[official docs](https://nodejs.org/api/buffer.html) for the full list of properties,
+instance methods, and class methods that are supported.
+
+## features
+
+- Manipulate binary data like a boss, in all browsers!
+- Super fast. Backed by Typed Arrays (`Uint8Array`/`ArrayBuffer`, not `Object`)
+- Extremely small bundle size (**6.75KB minified + gzipped**, 51.9KB with comments)
+- Excellent browser support (Chrome, Firefox, Edge, Safari 9+, IE 11, iOS 9+, Android, etc.)
+- Preserves Node API exactly, with one minor difference (see below)
+- Square-bracket `buf[4]` notation works!
+- Does not modify any browser prototypes or put anything on `window`
+- Comprehensive test suite (including all buffer tests from node.js core)
+
+## install
+
+To use this module directly (without browserify), install it:
+
+```bash
+npm install buffer
+```
+
+This module was previously called **native-buffer-browserify**, but please use **buffer**
+from now on.
+
+If you do not use a bundler, you can use the [standalone script](https://bundle.run/buffer).
+
+## usage
+
+The module's API is identical to node's `Buffer` API. Read the
+[official docs](https://nodejs.org/api/buffer.html) for the full list of properties,
+instance methods, and class methods that are supported.
+
+As mentioned above, `require('buffer')` or use the `Buffer` global with
+[browserify](http://browserify.org) and this module will automatically be included
+in your bundle. Almost any npm module will work in the browser, even if it assumes that
+the node `Buffer` API will be available.
+
+To depend on this module explicitly (without browserify), require it like this:
+
+```js
+var Buffer = require('buffer/').Buffer // note: the trailing slash is important!
+```
+
+To require this module explicitly, use `require('buffer/')` which tells the node.js module
+lookup algorithm (also used by browserify) to use the **npm module** named `buffer`
+instead of the **node.js core** module named `buffer`!
+
+
+## how does it work?
+
+The Buffer constructor returns instances of `Uint8Array` that have their prototype
+changed to `Buffer.prototype`. Furthermore, `Buffer` is a subclass of `Uint8Array`,
+so the returned instances will have all the node `Buffer` methods and the
+`Uint8Array` methods. Square bracket notation works as expected -- it returns a
+single octet.
+
+The `Uint8Array` prototype remains unmodified.
+
+
+## tracking the latest node api
+
+This module tracks the Buffer API in the latest (unstable) version of node.js. The Buffer
+API is considered **stable** in the
+[node stability index](https://nodejs.org/docs/latest/api/documentation.html#documentation_stability_index),
+so it is unlikely that there will ever be breaking changes.
+Nonetheless, when/if the Buffer API changes in node, this module's API will change
+accordingly.
+
+## related packages
+
+- [`buffer-reverse`](https://www.npmjs.com/package/buffer-reverse) - Reverse a buffer
+- [`buffer-xor`](https://www.npmjs.com/package/buffer-xor) - Bitwise xor a buffer
+- [`is-buffer`](https://www.npmjs.com/package/is-buffer) - Determine if an object is a Buffer without including the whole `Buffer` package
+
+## conversion packages
+
+### convert typed array to buffer
+
+Use [`typedarray-to-buffer`](https://www.npmjs.com/package/typedarray-to-buffer) to convert any kind of typed array to a `Buffer`. Does not perform a copy, so it's super fast.
+
+### convert buffer to typed array
+
+`Buffer` is a subclass of `Uint8Array` (which is a typed array). So there is no need to explicitly convert to typed array. Just use the buffer as a `Uint8Array`.
+
+### convert blob to buffer
+
+Use [`blob-to-buffer`](https://www.npmjs.com/package/blob-to-buffer) to convert a `Blob` to a `Buffer`.
+
+### convert buffer to blob
+
+To convert a `Buffer` to a `Blob`, use the `Blob` constructor:
+
+```js
+var blob = new Blob([ buffer ])
+```
+
+Optionally, specify a mimetype:
+
+```js
+var blob = new Blob([ buffer ], { type: 'text/html' })
+```
+
+### convert arraybuffer to buffer
+
+To convert an `ArrayBuffer` to a `Buffer`, use the `Buffer.from` function. Does not perform a copy, so it's super fast.
+
+```js
+var buffer = Buffer.from(arrayBuffer)
+```
+
+### convert buffer to arraybuffer
+
+To convert a `Buffer` to an `ArrayBuffer`, use the `.buffer` property (which is present on all `Uint8Array` objects):
+
+```js
+var arrayBuffer = buffer.buffer.slice(
+ buffer.byteOffset, buffer.byteOffset + buffer.byteLength
+)
+```
+
+Alternatively, use the [`to-arraybuffer`](https://www.npmjs.com/package/to-arraybuffer) module.
+
+## performance
+
+See perf tests in `/perf`.
+
+`BrowserBuffer` is the browser `buffer` module (this repo). `Uint8Array` is included as a
+sanity check (since `BrowserBuffer` uses `Uint8Array` under the hood, `Uint8Array` will
+always be at least a bit faster). Finally, `NodeBuffer` is the node.js buffer module,
+which is included to compare against.
+
+NOTE: Performance has improved since these benchmarks were taken. PR welcome to update the README.
+
+### Chrome 38
+
+| Method | Operations | Accuracy | Sampled | Fastest |
+|:-------|:-----------|:---------|:--------|:-------:|
+| BrowserBuffer#bracket-notation | 11,457,464 ops/sec | ±0.86% | 66 | ✓ |
+| Uint8Array#bracket-notation | 10,824,332 ops/sec | ±0.74% | 65 | |
+| | | | |
+| BrowserBuffer#concat | 450,532 ops/sec | ±0.76% | 68 | |
+| Uint8Array#concat | 1,368,911 ops/sec | ±1.50% | 62 | ✓ |
+| | | | |
+| BrowserBuffer#copy(16000) | 903,001 ops/sec | ±0.96% | 67 | |
+| Uint8Array#copy(16000) | 1,422,441 ops/sec | ±1.04% | 66 | ✓ |
+| | | | |
+| BrowserBuffer#copy(16) | 11,431,358 ops/sec | ±0.46% | 69 | |
+| Uint8Array#copy(16) | 13,944,163 ops/sec | ±1.12% | 68 | ✓ |
+| | | | |
+| BrowserBuffer#new(16000) | 106,329 ops/sec | ±6.70% | 44 | |
+| Uint8Array#new(16000) | 131,001 ops/sec | ±2.85% | 31 | ✓ |
+| | | | |
+| BrowserBuffer#new(16) | 1,554,491 ops/sec | ±1.60% | 65 | |
+| Uint8Array#new(16) | 6,623,930 ops/sec | ±1.66% | 65 | ✓ |
+| | | | |
+| BrowserBuffer#readDoubleBE | 112,830 ops/sec | ±0.51% | 69 | ✓ |
+| DataView#getFloat64 | 93,500 ops/sec | ±0.57% | 68 | |
+| | | | |
+| BrowserBuffer#readFloatBE | 146,678 ops/sec | ±0.95% | 68 | ✓ |
+| DataView#getFloat32 | 99,311 ops/sec | ±0.41% | 67 | |
+| | | | |
+| BrowserBuffer#readUInt32LE | 843,214 ops/sec | ±0.70% | 69 | ✓ |
+| DataView#getUint32 | 103,024 ops/sec | ±0.64% | 67 | |
+| | | | |
+| BrowserBuffer#slice | 1,013,941 ops/sec | ±0.75% | 67 | |
+| Uint8Array#subarray | 1,903,928 ops/sec | ±0.53% | 67 | ✓ |
+| | | | |
+| BrowserBuffer#writeFloatBE | 61,387 ops/sec | ±0.90% | 67 | |
+| DataView#setFloat32 | 141,249 ops/sec | ±0.40% | 66 | ✓ |
+
+
+### Firefox 33
+
+| Method | Operations | Accuracy | Sampled | Fastest |
+|:-------|:-----------|:---------|:--------|:-------:|
+| BrowserBuffer#bracket-notation | 20,800,421 ops/sec | ±1.84% | 60 | |
+| Uint8Array#bracket-notation | 20,826,235 ops/sec | ±2.02% | 61 | ✓ |
+| | | | |
+| BrowserBuffer#concat | 153,076 ops/sec | ±2.32% | 61 | |
+| Uint8Array#concat | 1,255,674 ops/sec | ±8.65% | 52 | ✓ |
+| | | | |
+| BrowserBuffer#copy(16000) | 1,105,312 ops/sec | ±1.16% | 63 | |
+| Uint8Array#copy(16000) | 1,615,911 ops/sec | ±0.55% | 66 | ✓ |
+| | | | |
+| BrowserBuffer#copy(16) | 16,357,599 ops/sec | ±0.73% | 68 | |
+| Uint8Array#copy(16) | 31,436,281 ops/sec | ±1.05% | 68 | ✓ |
+| | | | |
+| BrowserBuffer#new(16000) | 52,995 ops/sec | ±6.01% | 35 | |
+| Uint8Array#new(16000) | 87,686 ops/sec | ±5.68% | 45 | ✓ |
+| | | | |
+| BrowserBuffer#new(16) | 252,031 ops/sec | ±1.61% | 66 | |
+| Uint8Array#new(16) | 8,477,026 ops/sec | ±0.49% | 68 | ✓ |
+| | | | |
+| BrowserBuffer#readDoubleBE | 99,871 ops/sec | ±0.41% | 69 | |
+| DataView#getFloat64 | 285,663 ops/sec | ±0.70% | 68 | ✓ |
+| | | | |
+| BrowserBuffer#readFloatBE | 115,540 ops/sec | ±0.42% | 69 | |
+| DataView#getFloat32 | 288,722 ops/sec | ±0.82% | 68 | ✓ |
+| | | | |
+| BrowserBuffer#readUInt32LE | 633,926 ops/sec | ±1.08% | 67 | ✓ |
+| DataView#getUint32 | 294,808 ops/sec | ±0.79% | 64 | |
+| | | | |
+| BrowserBuffer#slice | 349,425 ops/sec | ±0.46% | 69 | |
+| Uint8Array#subarray | 5,965,819 ops/sec | ±0.60% | 65 | ✓ |
+| | | | |
+| BrowserBuffer#writeFloatBE | 59,980 ops/sec | ±0.41% | 67 | |
+| DataView#setFloat32 | 317,634 ops/sec | ±0.63% | 68 | ✓ |
+
+### Safari 8
+
+| Method | Operations | Accuracy | Sampled | Fastest |
+|:-------|:-----------|:---------|:--------|:-------:|
+| BrowserBuffer#bracket-notation | 10,279,729 ops/sec | ±2.25% | 56 | ✓ |
+| Uint8Array#bracket-notation | 10,030,767 ops/sec | ±2.23% | 59 | |
+| | | | |
+| BrowserBuffer#concat | 144,138 ops/sec | ±1.38% | 65 | |
+| Uint8Array#concat | 4,950,764 ops/sec | ±1.70% | 63 | ✓ |
+| | | | |
+| BrowserBuffer#copy(16000) | 1,058,548 ops/sec | ±1.51% | 64 | |
+| Uint8Array#copy(16000) | 1,409,666 ops/sec | ±1.17% | 65 | ✓ |
+| | | | |
+| BrowserBuffer#copy(16) | 6,282,529 ops/sec | ±1.88% | 58 | |
+| Uint8Array#copy(16) | 11,907,128 ops/sec | ±2.87% | 58 | ✓ |
+| | | | |
+| BrowserBuffer#new(16000) | 101,663 ops/sec | ±3.89% | 57 | |
+| Uint8Array#new(16000) | 22,050,818 ops/sec | ±6.51% | 46 | ✓ |
+| | | | |
+| BrowserBuffer#new(16) | 176,072 ops/sec | ±2.13% | 64 | |
+| Uint8Array#new(16) | 24,385,731 ops/sec | ±5.01% | 51 | ✓ |
+| | | | |
+| BrowserBuffer#readDoubleBE | 41,341 ops/sec | ±1.06% | 67 | |
+| DataView#getFloat64 | 322,280 ops/sec | ±0.84% | 68 | ✓ |
+| | | | |
+| BrowserBuffer#readFloatBE | 46,141 ops/sec | ±1.06% | 65 | |
+| DataView#getFloat32 | 337,025 ops/sec | ±0.43% | 69 | ✓ |
+| | | | |
+| BrowserBuffer#readUInt32LE | 151,551 ops/sec | ±1.02% | 66 | |
+| DataView#getUint32 | 308,278 ops/sec | ±0.94% | 67 | ✓ |
+| | | | |
+| BrowserBuffer#slice | 197,365 ops/sec | ±0.95% | 66 | |
+| Uint8Array#subarray | 9,558,024 ops/sec | ±3.08% | 58 | ✓ |
+| | | | |
+| BrowserBuffer#writeFloatBE | 17,518 ops/sec | ±1.03% | 63 | |
+| DataView#setFloat32 | 319,751 ops/sec | ±0.48% | 68 | ✓ |
+
+
+### Node 0.11.14
+
+| Method | Operations | Accuracy | Sampled | Fastest |
+|:-------|:-----------|:---------|:--------|:-------:|
+| BrowserBuffer#bracket-notation | 10,489,828 ops/sec | ±3.25% | 90 | |
+| Uint8Array#bracket-notation | 10,534,884 ops/sec | ±0.81% | 92 | ✓ |
+| NodeBuffer#bracket-notation | 10,389,910 ops/sec | ±0.97% | 87 | |
+| | | | |
+| BrowserBuffer#concat | 487,830 ops/sec | ±2.58% | 88 | |
+| Uint8Array#concat | 1,814,327 ops/sec | ±1.28% | 88 | ✓ |
+| NodeBuffer#concat | 1,636,523 ops/sec | ±1.88% | 73 | |
+| | | | |
+| BrowserBuffer#copy(16000) | 1,073,665 ops/sec | ±0.77% | 90 | |
+| Uint8Array#copy(16000) | 1,348,517 ops/sec | ±0.84% | 89 | ✓ |
+| NodeBuffer#copy(16000) | 1,289,533 ops/sec | ±0.82% | 93 | |
+| | | | |
+| BrowserBuffer#copy(16) | 12,782,706 ops/sec | ±0.74% | 85 | |
+| Uint8Array#copy(16) | 14,180,427 ops/sec | ±0.93% | 92 | ✓ |
+| NodeBuffer#copy(16) | 11,083,134 ops/sec | ±1.06% | 89 | |
+| | | | |
+| BrowserBuffer#new(16000) | 141,678 ops/sec | ±3.30% | 67 | |
+| Uint8Array#new(16000) | 161,491 ops/sec | ±2.96% | 60 | |
+| NodeBuffer#new(16000) | 292,699 ops/sec | ±3.20% | 55 | ✓ |
+| | | | |
+| BrowserBuffer#new(16) | 1,655,466 ops/sec | ±2.41% | 82 | |
+| Uint8Array#new(16) | 14,399,926 ops/sec | ±0.91% | 94 | ✓ |
+| NodeBuffer#new(16) | 3,894,696 ops/sec | ±0.88% | 92 | |
+| | | | |
+| BrowserBuffer#readDoubleBE | 109,582 ops/sec | ±0.75% | 93 | ✓ |
+| DataView#getFloat64 | 91,235 ops/sec | ±0.81% | 90 | |
+| NodeBuffer#readDoubleBE | 88,593 ops/sec | ±0.96% | 81 | |
+| | | | |
+| BrowserBuffer#readFloatBE | 139,854 ops/sec | ±1.03% | 85 | ✓ |
+| DataView#getFloat32 | 98,744 ops/sec | ±0.80% | 89 | |
+| NodeBuffer#readFloatBE | 92,769 ops/sec | ±0.94% | 93 | |
+| | | | |
+| BrowserBuffer#readUInt32LE | 710,861 ops/sec | ±0.82% | 92 | |
+| DataView#getUint32 | 117,893 ops/sec | ±0.84% | 91 | |
+| NodeBuffer#readUInt32LE | 851,412 ops/sec | ±0.72% | 93 | ✓ |
+| | | | |
+| BrowserBuffer#slice | 1,673,877 ops/sec | ±0.73% | 94 | |
+| Uint8Array#subarray | 6,919,243 ops/sec | ±0.67% | 90 | ✓ |
+| NodeBuffer#slice | 4,617,604 ops/sec | ±0.79% | 93 | |
+| | | | |
+| BrowserBuffer#writeFloatBE | 66,011 ops/sec | ±0.75% | 93 | |
+| DataView#setFloat32 | 127,760 ops/sec | ±0.72% | 93 | ✓ |
+| NodeBuffer#writeFloatBE | 103,352 ops/sec | ±0.83% | 93 | |
+
+### iojs 1.8.1
+
+| Method | Operations | Accuracy | Sampled | Fastest |
+|:-------|:-----------|:---------|:--------|:-------:|
+| BrowserBuffer#bracket-notation | 10,990,488 ops/sec | ±1.11% | 91 | |
+| Uint8Array#bracket-notation | 11,268,757 ops/sec | ±0.65% | 97 | |
+| NodeBuffer#bracket-notation | 11,353,260 ops/sec | ±0.83% | 94 | ✓ |
+| | | | |
+| BrowserBuffer#concat | 378,954 ops/sec | ±0.74% | 94 | |
+| Uint8Array#concat | 1,358,288 ops/sec | ±0.97% | 87 | |
+| NodeBuffer#concat | 1,934,050 ops/sec | ±1.11% | 78 | ✓ |
+| | | | |
+| BrowserBuffer#copy(16000) | 894,538 ops/sec | ±0.56% | 84 | |
+| Uint8Array#copy(16000) | 1,442,656 ops/sec | ±0.71% | 96 | |
+| NodeBuffer#copy(16000) | 1,457,898 ops/sec | ±0.53% | 92 | ✓ |
+| | | | |
+| BrowserBuffer#copy(16) | 12,870,457 ops/sec | ±0.67% | 95 | |
+| Uint8Array#copy(16) | 16,643,989 ops/sec | ±0.61% | 93 | ✓ |
+| NodeBuffer#copy(16) | 14,885,848 ops/sec | ±0.74% | 94 | |
+| | | | |
+| BrowserBuffer#new(16000) | 109,264 ops/sec | ±4.21% | 63 | |
+| Uint8Array#new(16000) | 138,916 ops/sec | ±1.87% | 61 | |
+| NodeBuffer#new(16000) | 281,449 ops/sec | ±3.58% | 51 | ✓ |
+| | | | |
+| BrowserBuffer#new(16) | 1,362,935 ops/sec | ±0.56% | 99 | |
+| Uint8Array#new(16) | 6,193,090 ops/sec | ±0.64% | 95 | ✓ |
+| NodeBuffer#new(16) | 4,745,425 ops/sec | ±1.56% | 90 | |
+| | | | |
+| BrowserBuffer#readDoubleBE | 118,127 ops/sec | ±0.59% | 93 | ✓ |
+| DataView#getFloat64 | 107,332 ops/sec | ±0.65% | 91 | |
+| NodeBuffer#readDoubleBE | 116,274 ops/sec | ±0.94% | 95 | |
+| | | | |
+| BrowserBuffer#readFloatBE | 150,326 ops/sec | ±0.58% | 95 | ✓ |
+| DataView#getFloat32 | 110,541 ops/sec | ±0.57% | 98 | |
+| NodeBuffer#readFloatBE | 121,599 ops/sec | ±0.60% | 87 | |
+| | | | |
+| BrowserBuffer#readUInt32LE | 814,147 ops/sec | ±0.62% | 93 | |
+| DataView#getUint32 | 137,592 ops/sec | ±0.64% | 90 | |
+| NodeBuffer#readUInt32LE | 931,650 ops/sec | ±0.71% | 96 | ✓ |
+| | | | |
+| BrowserBuffer#slice | 878,590 ops/sec | ±0.68% | 93 | |
+| Uint8Array#subarray | 2,843,308 ops/sec | ±1.02% | 90 | |
+| NodeBuffer#slice | 4,998,316 ops/sec | ±0.68% | 90 | ✓ |
+| | | | |
+| BrowserBuffer#writeFloatBE | 65,927 ops/sec | ±0.74% | 93 | |
+| DataView#setFloat32 | 139,823 ops/sec | ±0.97% | 89 | ✓ |
+| NodeBuffer#writeFloatBE | 135,763 ops/sec | ±0.65% | 96 | |
+| | | | |
+
+## Testing the project
+
+First, install the project:
+
+ npm install
+
+Then, to run tests in Node.js, run:
+
+ npm run test-node
+
+To test locally in a browser, you can run:
+
+ npm run test-browser-es5-local # For ES5 browsers that don't support ES6
+ npm run test-browser-es6-local # For ES6 compliant browsers
+
+This will print out a URL that you can then open in a browser to run the tests, using [airtap](https://www.npmjs.com/package/airtap).
+
+To run automated browser tests using Saucelabs, ensure that your `SAUCE_USERNAME` and `SAUCE_ACCESS_KEY` environment variables are set, then run:
+
+ npm test
+
+This is what's run in Travis, to check against various browsers. The list of browsers is kept in the `bin/airtap-es5.yml` and `bin/airtap-es6.yml` files.
+
+## JavaScript Standard Style
+
+This module uses [JavaScript Standard Style](https://github.com/feross/standard).
+
+[](https://github.com/feross/standard)
+
+To test that the code conforms to the style, `npm install` and run:
+
+ ./node_modules/.bin/standard
+
+## credit
+
+This was originally forked from [buffer-browserify](https://github.com/toots/buffer-browserify).
+
+## Security Policies and Procedures
+
+The `buffer` team and community take all security bugs in `buffer` seriously. Please see our [security policies and procedures](https://github.com/feross/security) document to learn how to report issues.
+
+## license
+
+MIT. Copyright (C) [Feross Aboukhadijeh](http://feross.org), and other contributors. Originally forked from an MIT-licensed module by Romain Beauxis.
diff --git a/node_modules/buffer/index.d.ts b/node_modules/buffer/index.d.ts
new file mode 100644
index 0000000..5d1a804
--- /dev/null
+++ b/node_modules/buffer/index.d.ts
@@ -0,0 +1,186 @@
+export class Buffer extends Uint8Array {
+ length: number
+ write(string: string, offset?: number, length?: number, encoding?: string): number;
+ toString(encoding?: string, start?: number, end?: number): string;
+ toJSON(): { type: 'Buffer', data: any[] };
+ equals(otherBuffer: Buffer): boolean;
+ compare(otherBuffer: Buffer, targetStart?: number, targetEnd?: number, sourceStart?: number, sourceEnd?: number): number;
+ copy(targetBuffer: Buffer, targetStart?: number, sourceStart?: number, sourceEnd?: number): number;
+ slice(start?: number, end?: number): Buffer;
+ writeUIntLE(value: number, offset: number, byteLength: number, noAssert?: boolean): number;
+ writeUIntBE(value: number, offset: number, byteLength: number, noAssert?: boolean): number;
+ writeIntLE(value: number, offset: number, byteLength: number, noAssert?: boolean): number;
+ writeIntBE(value: number, offset: number, byteLength: number, noAssert?: boolean): number;
+ readUIntLE(offset: number, byteLength: number, noAssert?: boolean): number;
+ readUIntBE(offset: number, byteLength: number, noAssert?: boolean): number;
+ readIntLE(offset: number, byteLength: number, noAssert?: boolean): number;
+ readIntBE(offset: number, byteLength: number, noAssert?: boolean): number;
+ readUInt8(offset: number, noAssert?: boolean): number;
+ readUInt16LE(offset: number, noAssert?: boolean): number;
+ readUInt16BE(offset: number, noAssert?: boolean): number;
+ readUInt32LE(offset: number, noAssert?: boolean): number;
+ readUInt32BE(offset: number, noAssert?: boolean): number;
+ readInt8(offset: number, noAssert?: boolean): number;
+ readInt16LE(offset: number, noAssert?: boolean): number;
+ readInt16BE(offset: number, noAssert?: boolean): number;
+ readInt32LE(offset: number, noAssert?: boolean): number;
+ readInt32BE(offset: number, noAssert?: boolean): number;
+ readFloatLE(offset: number, noAssert?: boolean): number;
+ readFloatBE(offset: number, noAssert?: boolean): number;
+ readDoubleLE(offset: number, noAssert?: boolean): number;
+ readDoubleBE(offset: number, noAssert?: boolean): number;
+ reverse(): this;
+ swap16(): Buffer;
+ swap32(): Buffer;
+ swap64(): Buffer;
+ writeUInt8(value: number, offset: number, noAssert?: boolean): number;
+ writeUInt16LE(value: number, offset: number, noAssert?: boolean): number;
+ writeUInt16BE(value: number, offset: number, noAssert?: boolean): number;
+ writeUInt32LE(value: number, offset: number, noAssert?: boolean): number;
+ writeUInt32BE(value: number, offset: number, noAssert?: boolean): number;
+ writeInt8(value: number, offset: number, noAssert?: boolean): number;
+ writeInt16LE(value: number, offset: number, noAssert?: boolean): number;
+ writeInt16BE(value: number, offset: number, noAssert?: boolean): number;
+ writeInt32LE(value: number, offset: number, noAssert?: boolean): number;
+ writeInt32BE(value: number, offset: number, noAssert?: boolean): number;
+ writeFloatLE(value: number, offset: number, noAssert?: boolean): number;
+ writeFloatBE(value: number, offset: number, noAssert?: boolean): number;
+ writeDoubleLE(value: number, offset: number, noAssert?: boolean): number;
+ writeDoubleBE(value: number, offset: number, noAssert?: boolean): number;
+ fill(value: any, offset?: number, end?: number): this;
+ indexOf(value: string | number | Buffer, byteOffset?: number, encoding?: string): number;
+ lastIndexOf(value: string | number | Buffer, byteOffset?: number, encoding?: string): number;
+ includes(value: string | number | Buffer, byteOffset?: number, encoding?: string): boolean;
+
+ /**
+ * Allocates a new buffer containing the given {str}.
+ *
+ * @param str String to store in buffer.
+ * @param encoding encoding to use, optional. Default is 'utf8'
+ */
+ constructor (str: string, encoding?: string);
+ /**
+ * Allocates a new buffer of {size} octets.
+ *
+ * @param size count of octets to allocate.
+ */
+ constructor (size: number);
+ /**
+ * Allocates a new buffer containing the given {array} of octets.
+ *
+ * @param array The octets to store.
+ */
+ constructor (array: Uint8Array);
+ /**
+ * Produces a Buffer backed by the same allocated memory as
+ * the given {ArrayBuffer}.
+ *
+ *
+ * @param arrayBuffer The ArrayBuffer with which to share memory.
+ */
+ constructor (arrayBuffer: ArrayBuffer);
+ /**
+ * Allocates a new buffer containing the given {array} of octets.
+ *
+ * @param array The octets to store.
+ */
+ constructor (array: any[]);
+ /**
+ * Copies the passed {buffer} data onto a new {Buffer} instance.
+ *
+ * @param buffer The buffer to copy.
+ */
+ constructor (buffer: Buffer);
+ prototype: Buffer;
+ /**
+ * Allocates a new Buffer using an {array} of octets.
+ *
+ * @param array
+ */
+ static from(array: any[]): Buffer;
+ /**
+ * When passed a reference to the .buffer property of a TypedArray instance,
+ * the newly created Buffer will share the same allocated memory as the TypedArray.
+ * The optional {byteOffset} and {length} arguments specify a memory range
+ * within the {arrayBuffer} that will be shared by the Buffer.
+ *
+ * @param arrayBuffer The .buffer property of a TypedArray or a new ArrayBuffer()
+ * @param byteOffset
+ * @param length
+ */
+ static from(arrayBuffer: ArrayBuffer, byteOffset?: number, length?: number): Buffer;
+ /**
+ * Copies the passed {buffer} data onto a new Buffer instance.
+ *
+ * @param buffer
+ */
+ static from(buffer: Buffer | Uint8Array): Buffer;
+ /**
+ * Creates a new Buffer containing the given JavaScript string {str}.
+ * If provided, the {encoding} parameter identifies the character encoding.
+ * If not provided, {encoding} defaults to 'utf8'.
+ *
+ * @param str
+ */
+ static from(str: string, encoding?: string): Buffer;
+ /**
+ * Returns true if {obj} is a Buffer
+ *
+ * @param obj object to test.
+ */
+ static isBuffer(obj: any): obj is Buffer;
+ /**
+ * Returns true if {encoding} is a valid encoding argument.
+ * Valid string encodings in Node 0.12: 'ascii'|'utf8'|'utf16le'|'ucs2'(alias of 'utf16le')|'base64'|'binary'(deprecated)|'hex'
+ *
+ * @param encoding string to test.
+ */
+ static isEncoding(encoding: string): boolean;
+ /**
+ * Gives the actual byte length of a string. encoding defaults to 'utf8'.
+ * This is not the same as String.prototype.length since that returns the number of characters in a string.
+ *
+ * @param string string to test.
+ * @param encoding encoding used to evaluate (defaults to 'utf8')
+ */
+ static byteLength(string: string, encoding?: string): number;
+ /**
+ * Returns a buffer which is the result of concatenating all the buffers in the list together.
+ *
+ * If the list has no items, or if the totalLength is 0, then it returns a zero-length buffer.
+ * If the list has exactly one item, then the first item of the list is returned.
+ * If the list has more than one item, then a new Buffer is created.
+ *
+ * @param list An array of Buffer objects to concatenate
+ * @param totalLength Total length of the buffers when concatenated.
+ * If totalLength is not provided, it is read from the buffers in the list. However, this adds an additional loop to the function, so it is faster to provide the length explicitly.
+ */
+ static concat(list: Buffer[], totalLength?: number): Buffer;
+ /**
+ * The same as buf1.compare(buf2).
+ */
+ static compare(buf1: Buffer, buf2: Buffer): number;
+ /**
+ * Allocates a new buffer of {size} octets.
+ *
+ * @param size count of octets to allocate.
+ * @param fill if specified, buffer will be initialized by calling buf.fill(fill).
+ * If parameter is omitted, buffer will be filled with zeros.
+ * @param encoding encoding used for call to buf.fill while initializing
+ */
+ static alloc(size: number, fill?: string | Buffer | number, encoding?: string): Buffer;
+ /**
+ * Allocates a new buffer of {size} octets, leaving memory not initialized, so the contents
+ * of the newly created Buffer are unknown and may contain sensitive data.
+ *
+ * @param size count of octets to allocate
+ */
+ static allocUnsafe(size: number): Buffer;
+ /**
+ * Allocates a new non-pooled buffer of {size} octets, leaving memory not initialized, so the contents
+ * of the newly created Buffer are unknown and may contain sensitive data.
+ *
+ * @param size count of octets to allocate
+ */
+ static allocUnsafeSlow(size: number): Buffer;
+}
diff --git a/node_modules/buffer/index.js b/node_modules/buffer/index.js
new file mode 100644
index 0000000..609cf31
--- /dev/null
+++ b/node_modules/buffer/index.js
@@ -0,0 +1,1817 @@
+/*!
+ * The buffer module from node.js, for the browser.
+ *
+ * @author Feross Aboukhadijeh
+ * @license MIT
+ */
+/* eslint-disable no-proto */
+
+'use strict'
+
+var base64 = require('base64-js')
+var ieee754 = require('ieee754')
+var customInspectSymbol =
+ (typeof Symbol === 'function' && typeof Symbol['for'] === 'function') // eslint-disable-line dot-notation
+ ? Symbol['for']('nodejs.util.inspect.custom') // eslint-disable-line dot-notation
+ : null
+
+exports.Buffer = Buffer
+exports.SlowBuffer = SlowBuffer
+exports.INSPECT_MAX_BYTES = 50
+
+var K_MAX_LENGTH = 0x7fffffff
+exports.kMaxLength = K_MAX_LENGTH
+
+/**
+ * If `Buffer.TYPED_ARRAY_SUPPORT`:
+ * === true Use Uint8Array implementation (fastest)
+ * === false Print warning and recommend using `buffer` v4.x which has an Object
+ * implementation (most compatible, even IE6)
+ *
+ * Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,
+ * Opera 11.6+, iOS 4.2+.
+ *
+ * We report that the browser does not support typed arrays if the are not subclassable
+ * using __proto__. Firefox 4-29 lacks support for adding new properties to `Uint8Array`
+ * (See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438). IE 10 lacks support
+ * for __proto__ and has a buggy typed array implementation.
+ */
+Buffer.TYPED_ARRAY_SUPPORT = typedArraySupport()
+
+if (!Buffer.TYPED_ARRAY_SUPPORT && typeof console !== 'undefined' &&
+ typeof console.error === 'function') {
+ console.error(
+ 'This browser lacks typed array (Uint8Array) support which is required by ' +
+ '`buffer` v5.x. Use `buffer` v4.x if you require old browser support.'
+ )
+}
+
+function typedArraySupport () {
+ // Can typed array instances can be augmented?
+ try {
+ var arr = new Uint8Array(1)
+ var proto = { foo: function () { return 42 } }
+ Object.setPrototypeOf(proto, Uint8Array.prototype)
+ Object.setPrototypeOf(arr, proto)
+ return arr.foo() === 42
+ } catch (e) {
+ return false
+ }
+}
+
+Object.defineProperty(Buffer.prototype, 'parent', {
+ enumerable: true,
+ get: function () {
+ if (!Buffer.isBuffer(this)) return undefined
+ return this.buffer
+ }
+})
+
+Object.defineProperty(Buffer.prototype, 'offset', {
+ enumerable: true,
+ get: function () {
+ if (!Buffer.isBuffer(this)) return undefined
+ return this.byteOffset
+ }
+})
+
+function createBuffer (length) {
+ if (length > K_MAX_LENGTH) {
+ throw new RangeError('The value "' + length + '" is invalid for option "size"')
+ }
+ // Return an augmented `Uint8Array` instance
+ var buf = new Uint8Array(length)
+ Object.setPrototypeOf(buf, Buffer.prototype)
+ return buf
+}
+
+/**
+ * The Buffer constructor returns instances of `Uint8Array` that have their
+ * prototype changed to `Buffer.prototype`. Furthermore, `Buffer` is a subclass of
+ * `Uint8Array`, so the returned instances will have all the node `Buffer` methods
+ * and the `Uint8Array` methods. Square bracket notation works as expected -- it
+ * returns a single octet.
+ *
+ * The `Uint8Array` prototype remains unmodified.
+ */
+
+function Buffer (arg, encodingOrOffset, length) {
+ // Common case.
+ if (typeof arg === 'number') {
+ if (typeof encodingOrOffset === 'string') {
+ throw new TypeError(
+ 'The "string" argument must be of type string. Received type number'
+ )
+ }
+ return allocUnsafe(arg)
+ }
+ return from(arg, encodingOrOffset, length)
+}
+
+Buffer.poolSize = 8192 // not used by this implementation
+
+function from (value, encodingOrOffset, length) {
+ if (typeof value === 'string') {
+ return fromString(value, encodingOrOffset)
+ }
+
+ if (ArrayBuffer.isView(value)) {
+ return fromArrayView(value)
+ }
+
+ if (value == null) {
+ throw new TypeError(
+ 'The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' +
+ 'or Array-like Object. Received type ' + (typeof value)
+ )
+ }
+
+ if (isInstance(value, ArrayBuffer) ||
+ (value && isInstance(value.buffer, ArrayBuffer))) {
+ return fromArrayBuffer(value, encodingOrOffset, length)
+ }
+
+ if (typeof SharedArrayBuffer !== 'undefined' &&
+ (isInstance(value, SharedArrayBuffer) ||
+ (value && isInstance(value.buffer, SharedArrayBuffer)))) {
+ return fromArrayBuffer(value, encodingOrOffset, length)
+ }
+
+ if (typeof value === 'number') {
+ throw new TypeError(
+ 'The "value" argument must not be of type number. Received type number'
+ )
+ }
+
+ var valueOf = value.valueOf && value.valueOf()
+ if (valueOf != null && valueOf !== value) {
+ return Buffer.from(valueOf, encodingOrOffset, length)
+ }
+
+ var b = fromObject(value)
+ if (b) return b
+
+ if (typeof Symbol !== 'undefined' && Symbol.toPrimitive != null &&
+ typeof value[Symbol.toPrimitive] === 'function') {
+ return Buffer.from(
+ value[Symbol.toPrimitive]('string'), encodingOrOffset, length
+ )
+ }
+
+ throw new TypeError(
+ 'The first argument must be one of type string, Buffer, ArrayBuffer, Array, ' +
+ 'or Array-like Object. Received type ' + (typeof value)
+ )
+}
+
+/**
+ * Functionally equivalent to Buffer(arg, encoding) but throws a TypeError
+ * if value is a number.
+ * Buffer.from(str[, encoding])
+ * Buffer.from(array)
+ * Buffer.from(buffer)
+ * Buffer.from(arrayBuffer[, byteOffset[, length]])
+ **/
+Buffer.from = function (value, encodingOrOffset, length) {
+ return from(value, encodingOrOffset, length)
+}
+
+// Note: Change prototype *after* Buffer.from is defined to workaround Chrome bug:
+// https://github.com/feross/buffer/pull/148
+Object.setPrototypeOf(Buffer.prototype, Uint8Array.prototype)
+Object.setPrototypeOf(Buffer, Uint8Array)
+
+function assertSize (size) {
+ if (typeof size !== 'number') {
+ throw new TypeError('"size" argument must be of type number')
+ } else if (size < 0) {
+ throw new RangeError('The value "' + size + '" is invalid for option "size"')
+ }
+}
+
+function alloc (size, fill, encoding) {
+ assertSize(size)
+ if (size <= 0) {
+ return createBuffer(size)
+ }
+ if (fill !== undefined) {
+ // Only pay attention to encoding if it's a string. This
+ // prevents accidentally sending in a number that would
+ // be interpreted as a start offset.
+ return typeof encoding === 'string'
+ ? createBuffer(size).fill(fill, encoding)
+ : createBuffer(size).fill(fill)
+ }
+ return createBuffer(size)
+}
+
+/**
+ * Creates a new filled Buffer instance.
+ * alloc(size[, fill[, encoding]])
+ **/
+Buffer.alloc = function (size, fill, encoding) {
+ return alloc(size, fill, encoding)
+}
+
+function allocUnsafe (size) {
+ assertSize(size)
+ return createBuffer(size < 0 ? 0 : checked(size) | 0)
+}
+
+/**
+ * Equivalent to Buffer(num), by default creates a non-zero-filled Buffer instance.
+ * */
+Buffer.allocUnsafe = function (size) {
+ return allocUnsafe(size)
+}
+/**
+ * Equivalent to SlowBuffer(num), by default creates a non-zero-filled Buffer instance.
+ */
+Buffer.allocUnsafeSlow = function (size) {
+ return allocUnsafe(size)
+}
+
+function fromString (string, encoding) {
+ if (typeof encoding !== 'string' || encoding === '') {
+ encoding = 'utf8'
+ }
+
+ if (!Buffer.isEncoding(encoding)) {
+ throw new TypeError('Unknown encoding: ' + encoding)
+ }
+
+ var length = byteLength(string, encoding) | 0
+ var buf = createBuffer(length)
+
+ var actual = buf.write(string, encoding)
+
+ if (actual !== length) {
+ // Writing a hex string, for example, that contains invalid characters will
+ // cause everything after the first invalid character to be ignored. (e.g.
+ // 'abxxcd' will be treated as 'ab')
+ buf = buf.slice(0, actual)
+ }
+
+ return buf
+}
+
+function fromArrayLike (array) {
+ var length = array.length < 0 ? 0 : checked(array.length) | 0
+ var buf = createBuffer(length)
+ for (var i = 0; i < length; i += 1) {
+ buf[i] = array[i] & 255
+ }
+ return buf
+}
+
+function fromArrayView (arrayView) {
+ if (isInstance(arrayView, Uint8Array)) {
+ var copy = new Uint8Array(arrayView)
+ return fromArrayBuffer(copy.buffer, copy.byteOffset, copy.byteLength)
+ }
+ return fromArrayLike(arrayView)
+}
+
+function fromArrayBuffer (array, byteOffset, length) {
+ if (byteOffset < 0 || array.byteLength < byteOffset) {
+ throw new RangeError('"offset" is outside of buffer bounds')
+ }
+
+ if (array.byteLength < byteOffset + (length || 0)) {
+ throw new RangeError('"length" is outside of buffer bounds')
+ }
+
+ var buf
+ if (byteOffset === undefined && length === undefined) {
+ buf = new Uint8Array(array)
+ } else if (length === undefined) {
+ buf = new Uint8Array(array, byteOffset)
+ } else {
+ buf = new Uint8Array(array, byteOffset, length)
+ }
+
+ // Return an augmented `Uint8Array` instance
+ Object.setPrototypeOf(buf, Buffer.prototype)
+
+ return buf
+}
+
+function fromObject (obj) {
+ if (Buffer.isBuffer(obj)) {
+ var len = checked(obj.length) | 0
+ var buf = createBuffer(len)
+
+ if (buf.length === 0) {
+ return buf
+ }
+
+ obj.copy(buf, 0, 0, len)
+ return buf
+ }
+
+ if (obj.length !== undefined) {
+ if (typeof obj.length !== 'number' || numberIsNaN(obj.length)) {
+ return createBuffer(0)
+ }
+ return fromArrayLike(obj)
+ }
+
+ if (obj.type === 'Buffer' && Array.isArray(obj.data)) {
+ return fromArrayLike(obj.data)
+ }
+}
+
+function checked (length) {
+ // Note: cannot use `length < K_MAX_LENGTH` here because that fails when
+ // length is NaN (which is otherwise coerced to zero.)
+ if (length >= K_MAX_LENGTH) {
+ throw new RangeError('Attempt to allocate Buffer larger than maximum ' +
+ 'size: 0x' + K_MAX_LENGTH.toString(16) + ' bytes')
+ }
+ return length | 0
+}
+
+function SlowBuffer (length) {
+ if (+length != length) { // eslint-disable-line eqeqeq
+ length = 0
+ }
+ return Buffer.alloc(+length)
+}
+
+Buffer.isBuffer = function isBuffer (b) {
+ return b != null && b._isBuffer === true &&
+ b !== Buffer.prototype // so Buffer.isBuffer(Buffer.prototype) will be false
+}
+
+Buffer.compare = function compare (a, b) {
+ if (isInstance(a, Uint8Array)) a = Buffer.from(a, a.offset, a.byteLength)
+ if (isInstance(b, Uint8Array)) b = Buffer.from(b, b.offset, b.byteLength)
+ if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {
+ throw new TypeError(
+ 'The "buf1", "buf2" arguments must be one of type Buffer or Uint8Array'
+ )
+ }
+
+ if (a === b) return 0
+
+ var x = a.length
+ var y = b.length
+
+ for (var i = 0, len = Math.min(x, y); i < len; ++i) {
+ if (a[i] !== b[i]) {
+ x = a[i]
+ y = b[i]
+ break
+ }
+ }
+
+ if (x < y) return -1
+ if (y < x) return 1
+ return 0
+}
+
+Buffer.isEncoding = function isEncoding (encoding) {
+ switch (String(encoding).toLowerCase()) {
+ case 'hex':
+ case 'utf8':
+ case 'utf-8':
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ case 'base64':
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return true
+ default:
+ return false
+ }
+}
+
+Buffer.concat = function concat (list, length) {
+ if (!Array.isArray(list)) {
+ throw new TypeError('"list" argument must be an Array of Buffers')
+ }
+
+ if (list.length === 0) {
+ return Buffer.alloc(0)
+ }
+
+ var i
+ if (length === undefined) {
+ length = 0
+ for (i = 0; i < list.length; ++i) {
+ length += list[i].length
+ }
+ }
+
+ var buffer = Buffer.allocUnsafe(length)
+ var pos = 0
+ for (i = 0; i < list.length; ++i) {
+ var buf = list[i]
+ if (isInstance(buf, Uint8Array)) {
+ if (pos + buf.length > buffer.length) {
+ Buffer.from(buf).copy(buffer, pos)
+ } else {
+ Uint8Array.prototype.set.call(
+ buffer,
+ buf,
+ pos
+ )
+ }
+ } else if (!Buffer.isBuffer(buf)) {
+ throw new TypeError('"list" argument must be an Array of Buffers')
+ } else {
+ buf.copy(buffer, pos)
+ }
+ pos += buf.length
+ }
+ return buffer
+}
+
+function byteLength (string, encoding) {
+ if (Buffer.isBuffer(string)) {
+ return string.length
+ }
+ if (ArrayBuffer.isView(string) || isInstance(string, ArrayBuffer)) {
+ return string.byteLength
+ }
+ if (typeof string !== 'string') {
+ throw new TypeError(
+ 'The "string" argument must be one of type string, Buffer, or ArrayBuffer. ' +
+ 'Received type ' + typeof string
+ )
+ }
+
+ var len = string.length
+ var mustMatch = (arguments.length > 2 && arguments[2] === true)
+ if (!mustMatch && len === 0) return 0
+
+ // Use a for loop to avoid recursion
+ var loweredCase = false
+ for (;;) {
+ switch (encoding) {
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ return len
+ case 'utf8':
+ case 'utf-8':
+ return utf8ToBytes(string).length
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return len * 2
+ case 'hex':
+ return len >>> 1
+ case 'base64':
+ return base64ToBytes(string).length
+ default:
+ if (loweredCase) {
+ return mustMatch ? -1 : utf8ToBytes(string).length // assume utf8
+ }
+ encoding = ('' + encoding).toLowerCase()
+ loweredCase = true
+ }
+ }
+}
+Buffer.byteLength = byteLength
+
+function slowToString (encoding, start, end) {
+ var loweredCase = false
+
+ // No need to verify that "this.length <= MAX_UINT32" since it's a read-only
+ // property of a typed array.
+
+ // This behaves neither like String nor Uint8Array in that we set start/end
+ // to their upper/lower bounds if the value passed is out of range.
+ // undefined is handled specially as per ECMA-262 6th Edition,
+ // Section 13.3.3.7 Runtime Semantics: KeyedBindingInitialization.
+ if (start === undefined || start < 0) {
+ start = 0
+ }
+ // Return early if start > this.length. Done here to prevent potential uint32
+ // coercion fail below.
+ if (start > this.length) {
+ return ''
+ }
+
+ if (end === undefined || end > this.length) {
+ end = this.length
+ }
+
+ if (end <= 0) {
+ return ''
+ }
+
+ // Force coercion to uint32. This will also coerce falsey/NaN values to 0.
+ end >>>= 0
+ start >>>= 0
+
+ if (end <= start) {
+ return ''
+ }
+
+ if (!encoding) encoding = 'utf8'
+
+ while (true) {
+ switch (encoding) {
+ case 'hex':
+ return hexSlice(this, start, end)
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Slice(this, start, end)
+
+ case 'ascii':
+ return asciiSlice(this, start, end)
+
+ case 'latin1':
+ case 'binary':
+ return latin1Slice(this, start, end)
+
+ case 'base64':
+ return base64Slice(this, start, end)
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return utf16leSlice(this, start, end)
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)
+ encoding = (encoding + '').toLowerCase()
+ loweredCase = true
+ }
+ }
+}
+
+// This property is used by `Buffer.isBuffer` (and the `is-buffer` npm package)
+// to detect a Buffer instance. It's not possible to use `instanceof Buffer`
+// reliably in a browserify context because there could be multiple different
+// copies of the 'buffer' package in use. This method works even for Buffer
+// instances that were created from another copy of the `buffer` package.
+// See: https://github.com/feross/buffer/issues/154
+Buffer.prototype._isBuffer = true
+
+function swap (b, n, m) {
+ var i = b[n]
+ b[n] = b[m]
+ b[m] = i
+}
+
+Buffer.prototype.swap16 = function swap16 () {
+ var len = this.length
+ if (len % 2 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 16-bits')
+ }
+ for (var i = 0; i < len; i += 2) {
+ swap(this, i, i + 1)
+ }
+ return this
+}
+
+Buffer.prototype.swap32 = function swap32 () {
+ var len = this.length
+ if (len % 4 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 32-bits')
+ }
+ for (var i = 0; i < len; i += 4) {
+ swap(this, i, i + 3)
+ swap(this, i + 1, i + 2)
+ }
+ return this
+}
+
+Buffer.prototype.swap64 = function swap64 () {
+ var len = this.length
+ if (len % 8 !== 0) {
+ throw new RangeError('Buffer size must be a multiple of 64-bits')
+ }
+ for (var i = 0; i < len; i += 8) {
+ swap(this, i, i + 7)
+ swap(this, i + 1, i + 6)
+ swap(this, i + 2, i + 5)
+ swap(this, i + 3, i + 4)
+ }
+ return this
+}
+
+Buffer.prototype.toString = function toString () {
+ var length = this.length
+ if (length === 0) return ''
+ if (arguments.length === 0) return utf8Slice(this, 0, length)
+ return slowToString.apply(this, arguments)
+}
+
+Buffer.prototype.toLocaleString = Buffer.prototype.toString
+
+Buffer.prototype.equals = function equals (b) {
+ if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer')
+ if (this === b) return true
+ return Buffer.compare(this, b) === 0
+}
+
+Buffer.prototype.inspect = function inspect () {
+ var str = ''
+ var max = exports.INSPECT_MAX_BYTES
+ str = this.toString('hex', 0, max).replace(/(.{2})/g, '$1 ').trim()
+ if (this.length > max) str += ' ... '
+ return ''
+}
+if (customInspectSymbol) {
+ Buffer.prototype[customInspectSymbol] = Buffer.prototype.inspect
+}
+
+Buffer.prototype.compare = function compare (target, start, end, thisStart, thisEnd) {
+ if (isInstance(target, Uint8Array)) {
+ target = Buffer.from(target, target.offset, target.byteLength)
+ }
+ if (!Buffer.isBuffer(target)) {
+ throw new TypeError(
+ 'The "target" argument must be one of type Buffer or Uint8Array. ' +
+ 'Received type ' + (typeof target)
+ )
+ }
+
+ if (start === undefined) {
+ start = 0
+ }
+ if (end === undefined) {
+ end = target ? target.length : 0
+ }
+ if (thisStart === undefined) {
+ thisStart = 0
+ }
+ if (thisEnd === undefined) {
+ thisEnd = this.length
+ }
+
+ if (start < 0 || end > target.length || thisStart < 0 || thisEnd > this.length) {
+ throw new RangeError('out of range index')
+ }
+
+ if (thisStart >= thisEnd && start >= end) {
+ return 0
+ }
+ if (thisStart >= thisEnd) {
+ return -1
+ }
+ if (start >= end) {
+ return 1
+ }
+
+ start >>>= 0
+ end >>>= 0
+ thisStart >>>= 0
+ thisEnd >>>= 0
+
+ if (this === target) return 0
+
+ var x = thisEnd - thisStart
+ var y = end - start
+ var len = Math.min(x, y)
+
+ var thisCopy = this.slice(thisStart, thisEnd)
+ var targetCopy = target.slice(start, end)
+
+ for (var i = 0; i < len; ++i) {
+ if (thisCopy[i] !== targetCopy[i]) {
+ x = thisCopy[i]
+ y = targetCopy[i]
+ break
+ }
+ }
+
+ if (x < y) return -1
+ if (y < x) return 1
+ return 0
+}
+
+// Finds either the first index of `val` in `buffer` at offset >= `byteOffset`,
+// OR the last index of `val` in `buffer` at offset <= `byteOffset`.
+//
+// Arguments:
+// - buffer - a Buffer to search
+// - val - a string, Buffer, or number
+// - byteOffset - an index into `buffer`; will be clamped to an int32
+// - encoding - an optional encoding, relevant is val is a string
+// - dir - true for indexOf, false for lastIndexOf
+function bidirectionalIndexOf (buffer, val, byteOffset, encoding, dir) {
+ // Empty buffer means no match
+ if (buffer.length === 0) return -1
+
+ // Normalize byteOffset
+ if (typeof byteOffset === 'string') {
+ encoding = byteOffset
+ byteOffset = 0
+ } else if (byteOffset > 0x7fffffff) {
+ byteOffset = 0x7fffffff
+ } else if (byteOffset < -0x80000000) {
+ byteOffset = -0x80000000
+ }
+ byteOffset = +byteOffset // Coerce to Number.
+ if (numberIsNaN(byteOffset)) {
+ // byteOffset: it it's undefined, null, NaN, "foo", etc, search whole buffer
+ byteOffset = dir ? 0 : (buffer.length - 1)
+ }
+
+ // Normalize byteOffset: negative offsets start from the end of the buffer
+ if (byteOffset < 0) byteOffset = buffer.length + byteOffset
+ if (byteOffset >= buffer.length) {
+ if (dir) return -1
+ else byteOffset = buffer.length - 1
+ } else if (byteOffset < 0) {
+ if (dir) byteOffset = 0
+ else return -1
+ }
+
+ // Normalize val
+ if (typeof val === 'string') {
+ val = Buffer.from(val, encoding)
+ }
+
+ // Finally, search either indexOf (if dir is true) or lastIndexOf
+ if (Buffer.isBuffer(val)) {
+ // Special case: looking for empty string/buffer always fails
+ if (val.length === 0) {
+ return -1
+ }
+ return arrayIndexOf(buffer, val, byteOffset, encoding, dir)
+ } else if (typeof val === 'number') {
+ val = val & 0xFF // Search for a byte value [0-255]
+ if (typeof Uint8Array.prototype.indexOf === 'function') {
+ if (dir) {
+ return Uint8Array.prototype.indexOf.call(buffer, val, byteOffset)
+ } else {
+ return Uint8Array.prototype.lastIndexOf.call(buffer, val, byteOffset)
+ }
+ }
+ return arrayIndexOf(buffer, [val], byteOffset, encoding, dir)
+ }
+
+ throw new TypeError('val must be string, number or Buffer')
+}
+
+function arrayIndexOf (arr, val, byteOffset, encoding, dir) {
+ var indexSize = 1
+ var arrLength = arr.length
+ var valLength = val.length
+
+ if (encoding !== undefined) {
+ encoding = String(encoding).toLowerCase()
+ if (encoding === 'ucs2' || encoding === 'ucs-2' ||
+ encoding === 'utf16le' || encoding === 'utf-16le') {
+ if (arr.length < 2 || val.length < 2) {
+ return -1
+ }
+ indexSize = 2
+ arrLength /= 2
+ valLength /= 2
+ byteOffset /= 2
+ }
+ }
+
+ function read (buf, i) {
+ if (indexSize === 1) {
+ return buf[i]
+ } else {
+ return buf.readUInt16BE(i * indexSize)
+ }
+ }
+
+ var i
+ if (dir) {
+ var foundIndex = -1
+ for (i = byteOffset; i < arrLength; i++) {
+ if (read(arr, i) === read(val, foundIndex === -1 ? 0 : i - foundIndex)) {
+ if (foundIndex === -1) foundIndex = i
+ if (i - foundIndex + 1 === valLength) return foundIndex * indexSize
+ } else {
+ if (foundIndex !== -1) i -= i - foundIndex
+ foundIndex = -1
+ }
+ }
+ } else {
+ if (byteOffset + valLength > arrLength) byteOffset = arrLength - valLength
+ for (i = byteOffset; i >= 0; i--) {
+ var found = true
+ for (var j = 0; j < valLength; j++) {
+ if (read(arr, i + j) !== read(val, j)) {
+ found = false
+ break
+ }
+ }
+ if (found) return i
+ }
+ }
+
+ return -1
+}
+
+Buffer.prototype.includes = function includes (val, byteOffset, encoding) {
+ return this.indexOf(val, byteOffset, encoding) !== -1
+}
+
+Buffer.prototype.indexOf = function indexOf (val, byteOffset, encoding) {
+ return bidirectionalIndexOf(this, val, byteOffset, encoding, true)
+}
+
+Buffer.prototype.lastIndexOf = function lastIndexOf (val, byteOffset, encoding) {
+ return bidirectionalIndexOf(this, val, byteOffset, encoding, false)
+}
+
+function hexWrite (buf, string, offset, length) {
+ offset = Number(offset) || 0
+ var remaining = buf.length - offset
+ if (!length) {
+ length = remaining
+ } else {
+ length = Number(length)
+ if (length > remaining) {
+ length = remaining
+ }
+ }
+
+ var strLen = string.length
+
+ if (length > strLen / 2) {
+ length = strLen / 2
+ }
+ for (var i = 0; i < length; ++i) {
+ var parsed = parseInt(string.substr(i * 2, 2), 16)
+ if (numberIsNaN(parsed)) return i
+ buf[offset + i] = parsed
+ }
+ return i
+}
+
+function utf8Write (buf, string, offset, length) {
+ return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length)
+}
+
+function asciiWrite (buf, string, offset, length) {
+ return blitBuffer(asciiToBytes(string), buf, offset, length)
+}
+
+function base64Write (buf, string, offset, length) {
+ return blitBuffer(base64ToBytes(string), buf, offset, length)
+}
+
+function ucs2Write (buf, string, offset, length) {
+ return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length)
+}
+
+Buffer.prototype.write = function write (string, offset, length, encoding) {
+ // Buffer#write(string)
+ if (offset === undefined) {
+ encoding = 'utf8'
+ length = this.length
+ offset = 0
+ // Buffer#write(string, encoding)
+ } else if (length === undefined && typeof offset === 'string') {
+ encoding = offset
+ length = this.length
+ offset = 0
+ // Buffer#write(string, offset[, length][, encoding])
+ } else if (isFinite(offset)) {
+ offset = offset >>> 0
+ if (isFinite(length)) {
+ length = length >>> 0
+ if (encoding === undefined) encoding = 'utf8'
+ } else {
+ encoding = length
+ length = undefined
+ }
+ } else {
+ throw new Error(
+ 'Buffer.write(string, encoding, offset[, length]) is no longer supported'
+ )
+ }
+
+ var remaining = this.length - offset
+ if (length === undefined || length > remaining) length = remaining
+
+ if ((string.length > 0 && (length < 0 || offset < 0)) || offset > this.length) {
+ throw new RangeError('Attempt to write outside buffer bounds')
+ }
+
+ if (!encoding) encoding = 'utf8'
+
+ var loweredCase = false
+ for (;;) {
+ switch (encoding) {
+ case 'hex':
+ return hexWrite(this, string, offset, length)
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Write(this, string, offset, length)
+
+ case 'ascii':
+ case 'latin1':
+ case 'binary':
+ return asciiWrite(this, string, offset, length)
+
+ case 'base64':
+ // Warning: maxLength not taken into account in base64Write
+ return base64Write(this, string, offset, length)
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return ucs2Write(this, string, offset, length)
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)
+ encoding = ('' + encoding).toLowerCase()
+ loweredCase = true
+ }
+ }
+}
+
+Buffer.prototype.toJSON = function toJSON () {
+ return {
+ type: 'Buffer',
+ data: Array.prototype.slice.call(this._arr || this, 0)
+ }
+}
+
+function base64Slice (buf, start, end) {
+ if (start === 0 && end === buf.length) {
+ return base64.fromByteArray(buf)
+ } else {
+ return base64.fromByteArray(buf.slice(start, end))
+ }
+}
+
+function utf8Slice (buf, start, end) {
+ end = Math.min(buf.length, end)
+ var res = []
+
+ var i = start
+ while (i < end) {
+ var firstByte = buf[i]
+ var codePoint = null
+ var bytesPerSequence = (firstByte > 0xEF)
+ ? 4
+ : (firstByte > 0xDF)
+ ? 3
+ : (firstByte > 0xBF)
+ ? 2
+ : 1
+
+ if (i + bytesPerSequence <= end) {
+ var secondByte, thirdByte, fourthByte, tempCodePoint
+
+ switch (bytesPerSequence) {
+ case 1:
+ if (firstByte < 0x80) {
+ codePoint = firstByte
+ }
+ break
+ case 2:
+ secondByte = buf[i + 1]
+ if ((secondByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0x1F) << 0x6 | (secondByte & 0x3F)
+ if (tempCodePoint > 0x7F) {
+ codePoint = tempCodePoint
+ }
+ }
+ break
+ case 3:
+ secondByte = buf[i + 1]
+ thirdByte = buf[i + 2]
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | (thirdByte & 0x3F)
+ if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {
+ codePoint = tempCodePoint
+ }
+ }
+ break
+ case 4:
+ secondByte = buf[i + 1]
+ thirdByte = buf[i + 2]
+ fourthByte = buf[i + 3]
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | (fourthByte & 0x3F)
+ if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {
+ codePoint = tempCodePoint
+ }
+ }
+ }
+ }
+
+ if (codePoint === null) {
+ // we did not generate a valid codePoint so insert a
+ // replacement char (U+FFFD) and advance only 1 byte
+ codePoint = 0xFFFD
+ bytesPerSequence = 1
+ } else if (codePoint > 0xFFFF) {
+ // encode to utf16 (surrogate pair dance)
+ codePoint -= 0x10000
+ res.push(codePoint >>> 10 & 0x3FF | 0xD800)
+ codePoint = 0xDC00 | codePoint & 0x3FF
+ }
+
+ res.push(codePoint)
+ i += bytesPerSequence
+ }
+
+ return decodeCodePointsArray(res)
+}
+
+// Based on http://stackoverflow.com/a/22747272/680742, the browser with
+// the lowest limit is Chrome, with 0x10000 args.
+// We go 1 magnitude less, for safety
+var MAX_ARGUMENTS_LENGTH = 0x1000
+
+function decodeCodePointsArray (codePoints) {
+ var len = codePoints.length
+ if (len <= MAX_ARGUMENTS_LENGTH) {
+ return String.fromCharCode.apply(String, codePoints) // avoid extra slice()
+ }
+
+ // Decode in chunks to avoid "call stack size exceeded".
+ var res = ''
+ var i = 0
+ while (i < len) {
+ res += String.fromCharCode.apply(
+ String,
+ codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH)
+ )
+ }
+ return res
+}
+
+function asciiSlice (buf, start, end) {
+ var ret = ''
+ end = Math.min(buf.length, end)
+
+ for (var i = start; i < end; ++i) {
+ ret += String.fromCharCode(buf[i] & 0x7F)
+ }
+ return ret
+}
+
+function latin1Slice (buf, start, end) {
+ var ret = ''
+ end = Math.min(buf.length, end)
+
+ for (var i = start; i < end; ++i) {
+ ret += String.fromCharCode(buf[i])
+ }
+ return ret
+}
+
+function hexSlice (buf, start, end) {
+ var len = buf.length
+
+ if (!start || start < 0) start = 0
+ if (!end || end < 0 || end > len) end = len
+
+ var out = ''
+ for (var i = start; i < end; ++i) {
+ out += hexSliceLookupTable[buf[i]]
+ }
+ return out
+}
+
+function utf16leSlice (buf, start, end) {
+ var bytes = buf.slice(start, end)
+ var res = ''
+ // If bytes.length is odd, the last 8 bits must be ignored (same as node.js)
+ for (var i = 0; i < bytes.length - 1; i += 2) {
+ res += String.fromCharCode(bytes[i] + (bytes[i + 1] * 256))
+ }
+ return res
+}
+
+Buffer.prototype.slice = function slice (start, end) {
+ var len = this.length
+ start = ~~start
+ end = end === undefined ? len : ~~end
+
+ if (start < 0) {
+ start += len
+ if (start < 0) start = 0
+ } else if (start > len) {
+ start = len
+ }
+
+ if (end < 0) {
+ end += len
+ if (end < 0) end = 0
+ } else if (end > len) {
+ end = len
+ }
+
+ if (end < start) end = start
+
+ var newBuf = this.subarray(start, end)
+ // Return an augmented `Uint8Array` instance
+ Object.setPrototypeOf(newBuf, Buffer.prototype)
+
+ return newBuf
+}
+
+/*
+ * Need to make sure that buffer isn't trying to write out of bounds.
+ */
+function checkOffset (offset, ext, length) {
+ if ((offset % 1) !== 0 || offset < 0) throw new RangeError('offset is not uint')
+ if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length')
+}
+
+Buffer.prototype.readUintLE =
+Buffer.prototype.readUIntLE = function readUIntLE (offset, byteLength, noAssert) {
+ offset = offset >>> 0
+ byteLength = byteLength >>> 0
+ if (!noAssert) checkOffset(offset, byteLength, this.length)
+
+ var val = this[offset]
+ var mul = 1
+ var i = 0
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul
+ }
+
+ return val
+}
+
+Buffer.prototype.readUintBE =
+Buffer.prototype.readUIntBE = function readUIntBE (offset, byteLength, noAssert) {
+ offset = offset >>> 0
+ byteLength = byteLength >>> 0
+ if (!noAssert) {
+ checkOffset(offset, byteLength, this.length)
+ }
+
+ var val = this[offset + --byteLength]
+ var mul = 1
+ while (byteLength > 0 && (mul *= 0x100)) {
+ val += this[offset + --byteLength] * mul
+ }
+
+ return val
+}
+
+Buffer.prototype.readUint8 =
+Buffer.prototype.readUInt8 = function readUInt8 (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 1, this.length)
+ return this[offset]
+}
+
+Buffer.prototype.readUint16LE =
+Buffer.prototype.readUInt16LE = function readUInt16LE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ return this[offset] | (this[offset + 1] << 8)
+}
+
+Buffer.prototype.readUint16BE =
+Buffer.prototype.readUInt16BE = function readUInt16BE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ return (this[offset] << 8) | this[offset + 1]
+}
+
+Buffer.prototype.readUint32LE =
+Buffer.prototype.readUInt32LE = function readUInt32LE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return ((this[offset]) |
+ (this[offset + 1] << 8) |
+ (this[offset + 2] << 16)) +
+ (this[offset + 3] * 0x1000000)
+}
+
+Buffer.prototype.readUint32BE =
+Buffer.prototype.readUInt32BE = function readUInt32BE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return (this[offset] * 0x1000000) +
+ ((this[offset + 1] << 16) |
+ (this[offset + 2] << 8) |
+ this[offset + 3])
+}
+
+Buffer.prototype.readIntLE = function readIntLE (offset, byteLength, noAssert) {
+ offset = offset >>> 0
+ byteLength = byteLength >>> 0
+ if (!noAssert) checkOffset(offset, byteLength, this.length)
+
+ var val = this[offset]
+ var mul = 1
+ var i = 0
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul
+ }
+ mul *= 0x80
+
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength)
+
+ return val
+}
+
+Buffer.prototype.readIntBE = function readIntBE (offset, byteLength, noAssert) {
+ offset = offset >>> 0
+ byteLength = byteLength >>> 0
+ if (!noAssert) checkOffset(offset, byteLength, this.length)
+
+ var i = byteLength
+ var mul = 1
+ var val = this[offset + --i]
+ while (i > 0 && (mul *= 0x100)) {
+ val += this[offset + --i] * mul
+ }
+ mul *= 0x80
+
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength)
+
+ return val
+}
+
+Buffer.prototype.readInt8 = function readInt8 (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 1, this.length)
+ if (!(this[offset] & 0x80)) return (this[offset])
+ return ((0xff - this[offset] + 1) * -1)
+}
+
+Buffer.prototype.readInt16LE = function readInt16LE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ var val = this[offset] | (this[offset + 1] << 8)
+ return (val & 0x8000) ? val | 0xFFFF0000 : val
+}
+
+Buffer.prototype.readInt16BE = function readInt16BE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ var val = this[offset + 1] | (this[offset] << 8)
+ return (val & 0x8000) ? val | 0xFFFF0000 : val
+}
+
+Buffer.prototype.readInt32LE = function readInt32LE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return (this[offset]) |
+ (this[offset + 1] << 8) |
+ (this[offset + 2] << 16) |
+ (this[offset + 3] << 24)
+}
+
+Buffer.prototype.readInt32BE = function readInt32BE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return (this[offset] << 24) |
+ (this[offset + 1] << 16) |
+ (this[offset + 2] << 8) |
+ (this[offset + 3])
+}
+
+Buffer.prototype.readFloatLE = function readFloatLE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 4, this.length)
+ return ieee754.read(this, offset, true, 23, 4)
+}
+
+Buffer.prototype.readFloatBE = function readFloatBE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 4, this.length)
+ return ieee754.read(this, offset, false, 23, 4)
+}
+
+Buffer.prototype.readDoubleLE = function readDoubleLE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 8, this.length)
+ return ieee754.read(this, offset, true, 52, 8)
+}
+
+Buffer.prototype.readDoubleBE = function readDoubleBE (offset, noAssert) {
+ offset = offset >>> 0
+ if (!noAssert) checkOffset(offset, 8, this.length)
+ return ieee754.read(this, offset, false, 52, 8)
+}
+
+function checkInt (buf, value, offset, ext, max, min) {
+ if (!Buffer.isBuffer(buf)) throw new TypeError('"buffer" argument must be a Buffer instance')
+ if (value > max || value < min) throw new RangeError('"value" argument is out of bounds')
+ if (offset + ext > buf.length) throw new RangeError('Index out of range')
+}
+
+Buffer.prototype.writeUintLE =
+Buffer.prototype.writeUIntLE = function writeUIntLE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ byteLength = byteLength >>> 0
+ if (!noAssert) {
+ var maxBytes = Math.pow(2, 8 * byteLength) - 1
+ checkInt(this, value, offset, byteLength, maxBytes, 0)
+ }
+
+ var mul = 1
+ var i = 0
+ this[offset] = value & 0xFF
+ while (++i < byteLength && (mul *= 0x100)) {
+ this[offset + i] = (value / mul) & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeUintBE =
+Buffer.prototype.writeUIntBE = function writeUIntBE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ byteLength = byteLength >>> 0
+ if (!noAssert) {
+ var maxBytes = Math.pow(2, 8 * byteLength) - 1
+ checkInt(this, value, offset, byteLength, maxBytes, 0)
+ }
+
+ var i = byteLength - 1
+ var mul = 1
+ this[offset + i] = value & 0xFF
+ while (--i >= 0 && (mul *= 0x100)) {
+ this[offset + i] = (value / mul) & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeUint8 =
+Buffer.prototype.writeUInt8 = function writeUInt8 (value, offset, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0)
+ this[offset] = (value & 0xff)
+ return offset + 1
+}
+
+Buffer.prototype.writeUint16LE =
+Buffer.prototype.writeUInt16LE = function writeUInt16LE (value, offset, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)
+ this[offset] = (value & 0xff)
+ this[offset + 1] = (value >>> 8)
+ return offset + 2
+}
+
+Buffer.prototype.writeUint16BE =
+Buffer.prototype.writeUInt16BE = function writeUInt16BE (value, offset, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)
+ this[offset] = (value >>> 8)
+ this[offset + 1] = (value & 0xff)
+ return offset + 2
+}
+
+Buffer.prototype.writeUint32LE =
+Buffer.prototype.writeUInt32LE = function writeUInt32LE (value, offset, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)
+ this[offset + 3] = (value >>> 24)
+ this[offset + 2] = (value >>> 16)
+ this[offset + 1] = (value >>> 8)
+ this[offset] = (value & 0xff)
+ return offset + 4
+}
+
+Buffer.prototype.writeUint32BE =
+Buffer.prototype.writeUInt32BE = function writeUInt32BE (value, offset, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)
+ this[offset] = (value >>> 24)
+ this[offset + 1] = (value >>> 16)
+ this[offset + 2] = (value >>> 8)
+ this[offset + 3] = (value & 0xff)
+ return offset + 4
+}
+
+Buffer.prototype.writeIntLE = function writeIntLE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) {
+ var limit = Math.pow(2, (8 * byteLength) - 1)
+
+ checkInt(this, value, offset, byteLength, limit - 1, -limit)
+ }
+
+ var i = 0
+ var mul = 1
+ var sub = 0
+ this[offset] = value & 0xFF
+ while (++i < byteLength && (mul *= 0x100)) {
+ if (value < 0 && sub === 0 && this[offset + i - 1] !== 0) {
+ sub = 1
+ }
+ this[offset + i] = ((value / mul) >> 0) - sub & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeIntBE = function writeIntBE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) {
+ var limit = Math.pow(2, (8 * byteLength) - 1)
+
+ checkInt(this, value, offset, byteLength, limit - 1, -limit)
+ }
+
+ var i = byteLength - 1
+ var mul = 1
+ var sub = 0
+ this[offset + i] = value & 0xFF
+ while (--i >= 0 && (mul *= 0x100)) {
+ if (value < 0 && sub === 0 && this[offset + i + 1] !== 0) {
+ sub = 1
+ }
+ this[offset + i] = ((value / mul) >> 0) - sub & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeInt8 = function writeInt8 (value, offset, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80)
+ if (value < 0) value = 0xff + value + 1
+ this[offset] = (value & 0xff)
+ return offset + 1
+}
+
+Buffer.prototype.writeInt16LE = function writeInt16LE (value, offset, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)
+ this[offset] = (value & 0xff)
+ this[offset + 1] = (value >>> 8)
+ return offset + 2
+}
+
+Buffer.prototype.writeInt16BE = function writeInt16BE (value, offset, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)
+ this[offset] = (value >>> 8)
+ this[offset + 1] = (value & 0xff)
+ return offset + 2
+}
+
+Buffer.prototype.writeInt32LE = function writeInt32LE (value, offset, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)
+ this[offset] = (value & 0xff)
+ this[offset + 1] = (value >>> 8)
+ this[offset + 2] = (value >>> 16)
+ this[offset + 3] = (value >>> 24)
+ return offset + 4
+}
+
+Buffer.prototype.writeInt32BE = function writeInt32BE (value, offset, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)
+ if (value < 0) value = 0xffffffff + value + 1
+ this[offset] = (value >>> 24)
+ this[offset + 1] = (value >>> 16)
+ this[offset + 2] = (value >>> 8)
+ this[offset + 3] = (value & 0xff)
+ return offset + 4
+}
+
+function checkIEEE754 (buf, value, offset, ext, max, min) {
+ if (offset + ext > buf.length) throw new RangeError('Index out of range')
+ if (offset < 0) throw new RangeError('Index out of range')
+}
+
+function writeFloat (buf, value, offset, littleEndian, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 4, 3.4028234663852886e+38, -3.4028234663852886e+38)
+ }
+ ieee754.write(buf, value, offset, littleEndian, 23, 4)
+ return offset + 4
+}
+
+Buffer.prototype.writeFloatLE = function writeFloatLE (value, offset, noAssert) {
+ return writeFloat(this, value, offset, true, noAssert)
+}
+
+Buffer.prototype.writeFloatBE = function writeFloatBE (value, offset, noAssert) {
+ return writeFloat(this, value, offset, false, noAssert)
+}
+
+function writeDouble (buf, value, offset, littleEndian, noAssert) {
+ value = +value
+ offset = offset >>> 0
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 8, 1.7976931348623157E+308, -1.7976931348623157E+308)
+ }
+ ieee754.write(buf, value, offset, littleEndian, 52, 8)
+ return offset + 8
+}
+
+Buffer.prototype.writeDoubleLE = function writeDoubleLE (value, offset, noAssert) {
+ return writeDouble(this, value, offset, true, noAssert)
+}
+
+Buffer.prototype.writeDoubleBE = function writeDoubleBE (value, offset, noAssert) {
+ return writeDouble(this, value, offset, false, noAssert)
+}
+
+// copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)
+Buffer.prototype.copy = function copy (target, targetStart, start, end) {
+ if (!Buffer.isBuffer(target)) throw new TypeError('argument should be a Buffer')
+ if (!start) start = 0
+ if (!end && end !== 0) end = this.length
+ if (targetStart >= target.length) targetStart = target.length
+ if (!targetStart) targetStart = 0
+ if (end > 0 && end < start) end = start
+
+ // Copy 0 bytes; we're done
+ if (end === start) return 0
+ if (target.length === 0 || this.length === 0) return 0
+
+ // Fatal error conditions
+ if (targetStart < 0) {
+ throw new RangeError('targetStart out of bounds')
+ }
+ if (start < 0 || start >= this.length) throw new RangeError('Index out of range')
+ if (end < 0) throw new RangeError('sourceEnd out of bounds')
+
+ // Are we oob?
+ if (end > this.length) end = this.length
+ if (target.length - targetStart < end - start) {
+ end = target.length - targetStart + start
+ }
+
+ var len = end - start
+
+ if (this === target && typeof Uint8Array.prototype.copyWithin === 'function') {
+ // Use built-in when available, missing from IE11
+ this.copyWithin(targetStart, start, end)
+ } else {
+ Uint8Array.prototype.set.call(
+ target,
+ this.subarray(start, end),
+ targetStart
+ )
+ }
+
+ return len
+}
+
+// Usage:
+// buffer.fill(number[, offset[, end]])
+// buffer.fill(buffer[, offset[, end]])
+// buffer.fill(string[, offset[, end]][, encoding])
+Buffer.prototype.fill = function fill (val, start, end, encoding) {
+ // Handle string cases:
+ if (typeof val === 'string') {
+ if (typeof start === 'string') {
+ encoding = start
+ start = 0
+ end = this.length
+ } else if (typeof end === 'string') {
+ encoding = end
+ end = this.length
+ }
+ if (encoding !== undefined && typeof encoding !== 'string') {
+ throw new TypeError('encoding must be a string')
+ }
+ if (typeof encoding === 'string' && !Buffer.isEncoding(encoding)) {
+ throw new TypeError('Unknown encoding: ' + encoding)
+ }
+ if (val.length === 1) {
+ var code = val.charCodeAt(0)
+ if ((encoding === 'utf8' && code < 128) ||
+ encoding === 'latin1') {
+ // Fast path: If `val` fits into a single byte, use that numeric value.
+ val = code
+ }
+ }
+ } else if (typeof val === 'number') {
+ val = val & 255
+ } else if (typeof val === 'boolean') {
+ val = Number(val)
+ }
+
+ // Invalid ranges are not set to a default, so can range check early.
+ if (start < 0 || this.length < start || this.length < end) {
+ throw new RangeError('Out of range index')
+ }
+
+ if (end <= start) {
+ return this
+ }
+
+ start = start >>> 0
+ end = end === undefined ? this.length : end >>> 0
+
+ if (!val) val = 0
+
+ var i
+ if (typeof val === 'number') {
+ for (i = start; i < end; ++i) {
+ this[i] = val
+ }
+ } else {
+ var bytes = Buffer.isBuffer(val)
+ ? val
+ : Buffer.from(val, encoding)
+ var len = bytes.length
+ if (len === 0) {
+ throw new TypeError('The value "' + val +
+ '" is invalid for argument "value"')
+ }
+ for (i = 0; i < end - start; ++i) {
+ this[i + start] = bytes[i % len]
+ }
+ }
+
+ return this
+}
+
+// HELPER FUNCTIONS
+// ================
+
+var INVALID_BASE64_RE = /[^+/0-9A-Za-z-_]/g
+
+function base64clean (str) {
+ // Node takes equal signs as end of the Base64 encoding
+ str = str.split('=')[0]
+ // Node strips out invalid characters like \n and \t from the string, base64-js does not
+ str = str.trim().replace(INVALID_BASE64_RE, '')
+ // Node converts strings with length < 2 to ''
+ if (str.length < 2) return ''
+ // Node allows for non-padded base64 strings (missing trailing ===), base64-js does not
+ while (str.length % 4 !== 0) {
+ str = str + '='
+ }
+ return str
+}
+
+function utf8ToBytes (string, units) {
+ units = units || Infinity
+ var codePoint
+ var length = string.length
+ var leadSurrogate = null
+ var bytes = []
+
+ for (var i = 0; i < length; ++i) {
+ codePoint = string.charCodeAt(i)
+
+ // is surrogate component
+ if (codePoint > 0xD7FF && codePoint < 0xE000) {
+ // last char was a lead
+ if (!leadSurrogate) {
+ // no lead yet
+ if (codePoint > 0xDBFF) {
+ // unexpected trail
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ continue
+ } else if (i + 1 === length) {
+ // unpaired lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ continue
+ }
+
+ // valid lead
+ leadSurrogate = codePoint
+
+ continue
+ }
+
+ // 2 leads in a row
+ if (codePoint < 0xDC00) {
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ leadSurrogate = codePoint
+ continue
+ }
+
+ // valid surrogate pair
+ codePoint = (leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00) + 0x10000
+ } else if (leadSurrogate) {
+ // valid bmp char, but last char was a lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ }
+
+ leadSurrogate = null
+
+ // encode utf8
+ if (codePoint < 0x80) {
+ if ((units -= 1) < 0) break
+ bytes.push(codePoint)
+ } else if (codePoint < 0x800) {
+ if ((units -= 2) < 0) break
+ bytes.push(
+ codePoint >> 0x6 | 0xC0,
+ codePoint & 0x3F | 0x80
+ )
+ } else if (codePoint < 0x10000) {
+ if ((units -= 3) < 0) break
+ bytes.push(
+ codePoint >> 0xC | 0xE0,
+ codePoint >> 0x6 & 0x3F | 0x80,
+ codePoint & 0x3F | 0x80
+ )
+ } else if (codePoint < 0x110000) {
+ if ((units -= 4) < 0) break
+ bytes.push(
+ codePoint >> 0x12 | 0xF0,
+ codePoint >> 0xC & 0x3F | 0x80,
+ codePoint >> 0x6 & 0x3F | 0x80,
+ codePoint & 0x3F | 0x80
+ )
+ } else {
+ throw new Error('Invalid code point')
+ }
+ }
+
+ return bytes
+}
+
+function asciiToBytes (str) {
+ var byteArray = []
+ for (var i = 0; i < str.length; ++i) {
+ // Node's code seems to be doing this and not & 0x7F..
+ byteArray.push(str.charCodeAt(i) & 0xFF)
+ }
+ return byteArray
+}
+
+function utf16leToBytes (str, units) {
+ var c, hi, lo
+ var byteArray = []
+ for (var i = 0; i < str.length; ++i) {
+ if ((units -= 2) < 0) break
+
+ c = str.charCodeAt(i)
+ hi = c >> 8
+ lo = c % 256
+ byteArray.push(lo)
+ byteArray.push(hi)
+ }
+
+ return byteArray
+}
+
+function base64ToBytes (str) {
+ return base64.toByteArray(base64clean(str))
+}
+
+function blitBuffer (src, dst, offset, length) {
+ for (var i = 0; i < length; ++i) {
+ if ((i + offset >= dst.length) || (i >= src.length)) break
+ dst[i + offset] = src[i]
+ }
+ return i
+}
+
+// ArrayBuffer or Uint8Array objects from other contexts (i.e. iframes) do not pass
+// the `instanceof` check but they should be treated as of that type.
+// See: https://github.com/feross/buffer/issues/166
+function isInstance (obj, type) {
+ return obj instanceof type ||
+ (obj != null && obj.constructor != null && obj.constructor.name != null &&
+ obj.constructor.name === type.name)
+}
+function numberIsNaN (obj) {
+ // For IE11 support
+ return obj !== obj // eslint-disable-line no-self-compare
+}
+
+// Create lookup table for `toString('hex')`
+// See: https://github.com/feross/buffer/issues/219
+var hexSliceLookupTable = (function () {
+ var alphabet = '0123456789abcdef'
+ var table = new Array(256)
+ for (var i = 0; i < 16; ++i) {
+ var i16 = i * 16
+ for (var j = 0; j < 16; ++j) {
+ table[i16 + j] = alphabet[i] + alphabet[j]
+ }
+ }
+ return table
+})()
diff --git a/node_modules/buffer/package.json b/node_modules/buffer/package.json
new file mode 100644
index 0000000..b7bb660
--- /dev/null
+++ b/node_modules/buffer/package.json
@@ -0,0 +1,127 @@
+{
+ "_from": "buffer@^5.6.0",
+ "_id": "buffer@5.7.1",
+ "_inBundle": false,
+ "_integrity": "sha512-EHcyIPBQ4BSGlvjB16k5KgAJ27CIsHY/2JBmCRReo48y9rQ3MaUzWX3KVlBa4U7MyX02HdVj0K7C3WaB3ju7FQ==",
+ "_location": "/buffer",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "buffer@^5.6.0",
+ "name": "buffer",
+ "escapedName": "buffer",
+ "rawSpec": "^5.6.0",
+ "saveSpec": null,
+ "fetchSpec": "^5.6.0"
+ },
+ "_requiredBy": [
+ "/bson"
+ ],
+ "_resolved": "https://registry.npmjs.org/buffer/-/buffer-5.7.1.tgz",
+ "_shasum": "ba62e7c13133053582197160851a8f648e99eed0",
+ "_spec": "buffer@^5.6.0",
+ "_where": "/Users/jasonagus/Desktop/Guide Project/node_modules/bson",
+ "author": {
+ "name": "Feross Aboukhadijeh",
+ "email": "feross@feross.org",
+ "url": "https://feross.org"
+ },
+ "bugs": {
+ "url": "https://github.com/feross/buffer/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "Romain Beauxis",
+ "email": "toots@rastageeks.org"
+ },
+ {
+ "name": "James Halliday",
+ "email": "mail@substack.net"
+ }
+ ],
+ "dependencies": {
+ "base64-js": "^1.3.1",
+ "ieee754": "^1.1.13"
+ },
+ "deprecated": false,
+ "description": "Node.js Buffer API, for the browser",
+ "devDependencies": {
+ "airtap": "^3.0.0",
+ "benchmark": "^2.1.4",
+ "browserify": "^17.0.0",
+ "concat-stream": "^2.0.0",
+ "hyperquest": "^2.1.3",
+ "is-buffer": "^2.0.4",
+ "is-nan": "^1.3.0",
+ "split": "^1.0.1",
+ "standard": "*",
+ "tape": "^5.0.1",
+ "through2": "^4.0.2",
+ "uglify-js": "^3.11.3"
+ },
+ "funding": [
+ {
+ "type": "github",
+ "url": "https://github.com/sponsors/feross"
+ },
+ {
+ "type": "patreon",
+ "url": "https://www.patreon.com/feross"
+ },
+ {
+ "type": "consulting",
+ "url": "https://feross.org/support"
+ }
+ ],
+ "homepage": "https://github.com/feross/buffer",
+ "jspm": {
+ "map": {
+ "./index.js": {
+ "node": "@node/buffer"
+ }
+ }
+ },
+ "keywords": [
+ "arraybuffer",
+ "browser",
+ "browserify",
+ "buffer",
+ "compatible",
+ "dataview",
+ "uint8array"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "buffer",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/feross/buffer.git"
+ },
+ "scripts": {
+ "perf": "browserify --debug perf/bracket-notation.js > perf/bundle.js && open perf/index.html",
+ "perf-node": "node perf/bracket-notation.js && node perf/concat.js && node perf/copy-big.js && node perf/copy.js && node perf/new-big.js && node perf/new.js && node perf/readDoubleBE.js && node perf/readFloatBE.js && node perf/readUInt32LE.js && node perf/slice.js && node perf/writeFloatBE.js",
+ "size": "browserify -r ./ | uglifyjs -c -m | gzip | wc -c",
+ "test": "standard && node ./bin/test.js",
+ "test-browser-es5": "airtap -- test/*.js",
+ "test-browser-es5-local": "airtap --local -- test/*.js",
+ "test-browser-es6": "airtap -- test/*.js test/node/*.js",
+ "test-browser-es6-local": "airtap --local -- test/*.js test/node/*.js",
+ "test-node": "tape test/*.js test/node/*.js",
+ "update-authors": "./bin/update-authors.sh"
+ },
+ "standard": {
+ "ignore": [
+ "test/node/**/*.js",
+ "test/common.js",
+ "test/_polyfill.js",
+ "perf/**/*.js"
+ ],
+ "globals": [
+ "SharedArrayBuffer"
+ ]
+ },
+ "types": "index.d.ts",
+ "version": "5.7.1"
+}
diff --git a/node_modules/core-util-is/LICENSE b/node_modules/core-util-is/LICENSE
deleted file mode 100644
index d8d7f94..0000000
--- a/node_modules/core-util-is/LICENSE
+++ /dev/null
@@ -1,19 +0,0 @@
-Copyright Node.js contributors. All rights reserved.
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to
-deal in the Software without restriction, including without limitation the
-rights to use, copy, modify, merge, publish, distribute, sublicense, and/or
-sell copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in
-all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
-FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
-IN THE SOFTWARE.
diff --git a/node_modules/core-util-is/README.md b/node_modules/core-util-is/README.md
deleted file mode 100644
index 5a76b41..0000000
--- a/node_modules/core-util-is/README.md
+++ /dev/null
@@ -1,3 +0,0 @@
-# core-util-is
-
-The `util.is*` functions introduced in Node v0.12.
diff --git a/node_modules/core-util-is/float.patch b/node_modules/core-util-is/float.patch
deleted file mode 100644
index a06d5c0..0000000
--- a/node_modules/core-util-is/float.patch
+++ /dev/null
@@ -1,604 +0,0 @@
-diff --git a/lib/util.js b/lib/util.js
-index a03e874..9074e8e 100644
---- a/lib/util.js
-+++ b/lib/util.js
-@@ -19,430 +19,6 @@
- // OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
- // USE OR OTHER DEALINGS IN THE SOFTWARE.
-
--var formatRegExp = /%[sdj%]/g;
--exports.format = function(f) {
-- if (!isString(f)) {
-- var objects = [];
-- for (var i = 0; i < arguments.length; i++) {
-- objects.push(inspect(arguments[i]));
-- }
-- return objects.join(' ');
-- }
--
-- var i = 1;
-- var args = arguments;
-- var len = args.length;
-- var str = String(f).replace(formatRegExp, function(x) {
-- if (x === '%%') return '%';
-- if (i >= len) return x;
-- switch (x) {
-- case '%s': return String(args[i++]);
-- case '%d': return Number(args[i++]);
-- case '%j':
-- try {
-- return JSON.stringify(args[i++]);
-- } catch (_) {
-- return '[Circular]';
-- }
-- default:
-- return x;
-- }
-- });
-- for (var x = args[i]; i < len; x = args[++i]) {
-- if (isNull(x) || !isObject(x)) {
-- str += ' ' + x;
-- } else {
-- str += ' ' + inspect(x);
-- }
-- }
-- return str;
--};
--
--
--// Mark that a method should not be used.
--// Returns a modified function which warns once by default.
--// If --no-deprecation is set, then it is a no-op.
--exports.deprecate = function(fn, msg) {
-- // Allow for deprecating things in the process of starting up.
-- if (isUndefined(global.process)) {
-- return function() {
-- return exports.deprecate(fn, msg).apply(this, arguments);
-- };
-- }
--
-- if (process.noDeprecation === true) {
-- return fn;
-- }
--
-- var warned = false;
-- function deprecated() {
-- if (!warned) {
-- if (process.throwDeprecation) {
-- throw new Error(msg);
-- } else if (process.traceDeprecation) {
-- console.trace(msg);
-- } else {
-- console.error(msg);
-- }
-- warned = true;
-- }
-- return fn.apply(this, arguments);
-- }
--
-- return deprecated;
--};
--
--
--var debugs = {};
--var debugEnviron;
--exports.debuglog = function(set) {
-- if (isUndefined(debugEnviron))
-- debugEnviron = process.env.NODE_DEBUG || '';
-- set = set.toUpperCase();
-- if (!debugs[set]) {
-- if (new RegExp('\\b' + set + '\\b', 'i').test(debugEnviron)) {
-- var pid = process.pid;
-- debugs[set] = function() {
-- var msg = exports.format.apply(exports, arguments);
-- console.error('%s %d: %s', set, pid, msg);
-- };
-- } else {
-- debugs[set] = function() {};
-- }
-- }
-- return debugs[set];
--};
--
--
--/**
-- * Echos the value of a value. Trys to print the value out
-- * in the best way possible given the different types.
-- *
-- * @param {Object} obj The object to print out.
-- * @param {Object} opts Optional options object that alters the output.
-- */
--/* legacy: obj, showHidden, depth, colors*/
--function inspect(obj, opts) {
-- // default options
-- var ctx = {
-- seen: [],
-- stylize: stylizeNoColor
-- };
-- // legacy...
-- if (arguments.length >= 3) ctx.depth = arguments[2];
-- if (arguments.length >= 4) ctx.colors = arguments[3];
-- if (isBoolean(opts)) {
-- // legacy...
-- ctx.showHidden = opts;
-- } else if (opts) {
-- // got an "options" object
-- exports._extend(ctx, opts);
-- }
-- // set default options
-- if (isUndefined(ctx.showHidden)) ctx.showHidden = false;
-- if (isUndefined(ctx.depth)) ctx.depth = 2;
-- if (isUndefined(ctx.colors)) ctx.colors = false;
-- if (isUndefined(ctx.customInspect)) ctx.customInspect = true;
-- if (ctx.colors) ctx.stylize = stylizeWithColor;
-- return formatValue(ctx, obj, ctx.depth);
--}
--exports.inspect = inspect;
--
--
--// http://en.wikipedia.org/wiki/ANSI_escape_code#graphics
--inspect.colors = {
-- 'bold' : [1, 22],
-- 'italic' : [3, 23],
-- 'underline' : [4, 24],
-- 'inverse' : [7, 27],
-- 'white' : [37, 39],
-- 'grey' : [90, 39],
-- 'black' : [30, 39],
-- 'blue' : [34, 39],
-- 'cyan' : [36, 39],
-- 'green' : [32, 39],
-- 'magenta' : [35, 39],
-- 'red' : [31, 39],
-- 'yellow' : [33, 39]
--};
--
--// Don't use 'blue' not visible on cmd.exe
--inspect.styles = {
-- 'special': 'cyan',
-- 'number': 'yellow',
-- 'boolean': 'yellow',
-- 'undefined': 'grey',
-- 'null': 'bold',
-- 'string': 'green',
-- 'date': 'magenta',
-- // "name": intentionally not styling
-- 'regexp': 'red'
--};
--
--
--function stylizeWithColor(str, styleType) {
-- var style = inspect.styles[styleType];
--
-- if (style) {
-- return '\u001b[' + inspect.colors[style][0] + 'm' + str +
-- '\u001b[' + inspect.colors[style][1] + 'm';
-- } else {
-- return str;
-- }
--}
--
--
--function stylizeNoColor(str, styleType) {
-- return str;
--}
--
--
--function arrayToHash(array) {
-- var hash = {};
--
-- array.forEach(function(val, idx) {
-- hash[val] = true;
-- });
--
-- return hash;
--}
--
--
--function formatValue(ctx, value, recurseTimes) {
-- // Provide a hook for user-specified inspect functions.
-- // Check that value is an object with an inspect function on it
-- if (ctx.customInspect &&
-- value &&
-- isFunction(value.inspect) &&
-- // Filter out the util module, it's inspect function is special
-- value.inspect !== exports.inspect &&
-- // Also filter out any prototype objects using the circular check.
-- !(value.constructor && value.constructor.prototype === value)) {
-- var ret = value.inspect(recurseTimes, ctx);
-- if (!isString(ret)) {
-- ret = formatValue(ctx, ret, recurseTimes);
-- }
-- return ret;
-- }
--
-- // Primitive types cannot have properties
-- var primitive = formatPrimitive(ctx, value);
-- if (primitive) {
-- return primitive;
-- }
--
-- // Look up the keys of the object.
-- var keys = Object.keys(value);
-- var visibleKeys = arrayToHash(keys);
--
-- if (ctx.showHidden) {
-- keys = Object.getOwnPropertyNames(value);
-- }
--
-- // Some type of object without properties can be shortcutted.
-- if (keys.length === 0) {
-- if (isFunction(value)) {
-- var name = value.name ? ': ' + value.name : '';
-- return ctx.stylize('[Function' + name + ']', 'special');
-- }
-- if (isRegExp(value)) {
-- return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
-- }
-- if (isDate(value)) {
-- return ctx.stylize(Date.prototype.toString.call(value), 'date');
-- }
-- if (isError(value)) {
-- return formatError(value);
-- }
-- }
--
-- var base = '', array = false, braces = ['{', '}'];
--
-- // Make Array say that they are Array
-- if (isArray(value)) {
-- array = true;
-- braces = ['[', ']'];
-- }
--
-- // Make functions say that they are functions
-- if (isFunction(value)) {
-- var n = value.name ? ': ' + value.name : '';
-- base = ' [Function' + n + ']';
-- }
--
-- // Make RegExps say that they are RegExps
-- if (isRegExp(value)) {
-- base = ' ' + RegExp.prototype.toString.call(value);
-- }
--
-- // Make dates with properties first say the date
-- if (isDate(value)) {
-- base = ' ' + Date.prototype.toUTCString.call(value);
-- }
--
-- // Make error with message first say the error
-- if (isError(value)) {
-- base = ' ' + formatError(value);
-- }
--
-- if (keys.length === 0 && (!array || value.length == 0)) {
-- return braces[0] + base + braces[1];
-- }
--
-- if (recurseTimes < 0) {
-- if (isRegExp(value)) {
-- return ctx.stylize(RegExp.prototype.toString.call(value), 'regexp');
-- } else {
-- return ctx.stylize('[Object]', 'special');
-- }
-- }
--
-- ctx.seen.push(value);
--
-- var output;
-- if (array) {
-- output = formatArray(ctx, value, recurseTimes, visibleKeys, keys);
-- } else {
-- output = keys.map(function(key) {
-- return formatProperty(ctx, value, recurseTimes, visibleKeys, key, array);
-- });
-- }
--
-- ctx.seen.pop();
--
-- return reduceToSingleString(output, base, braces);
--}
--
--
--function formatPrimitive(ctx, value) {
-- if (isUndefined(value))
-- return ctx.stylize('undefined', 'undefined');
-- if (isString(value)) {
-- var simple = '\'' + JSON.stringify(value).replace(/^"|"$/g, '')
-- .replace(/'/g, "\\'")
-- .replace(/\\"/g, '"') + '\'';
-- return ctx.stylize(simple, 'string');
-- }
-- if (isNumber(value)) {
-- // Format -0 as '-0'. Strict equality won't distinguish 0 from -0,
-- // so instead we use the fact that 1 / -0 < 0 whereas 1 / 0 > 0 .
-- if (value === 0 && 1 / value < 0)
-- return ctx.stylize('-0', 'number');
-- return ctx.stylize('' + value, 'number');
-- }
-- if (isBoolean(value))
-- return ctx.stylize('' + value, 'boolean');
-- // For some reason typeof null is "object", so special case here.
-- if (isNull(value))
-- return ctx.stylize('null', 'null');
--}
--
--
--function formatError(value) {
-- return '[' + Error.prototype.toString.call(value) + ']';
--}
--
--
--function formatArray(ctx, value, recurseTimes, visibleKeys, keys) {
-- var output = [];
-- for (var i = 0, l = value.length; i < l; ++i) {
-- if (hasOwnProperty(value, String(i))) {
-- output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,
-- String(i), true));
-- } else {
-- output.push('');
-- }
-- }
-- keys.forEach(function(key) {
-- if (!key.match(/^\d+$/)) {
-- output.push(formatProperty(ctx, value, recurseTimes, visibleKeys,
-- key, true));
-- }
-- });
-- return output;
--}
--
--
--function formatProperty(ctx, value, recurseTimes, visibleKeys, key, array) {
-- var name, str, desc;
-- desc = Object.getOwnPropertyDescriptor(value, key) || { value: value[key] };
-- if (desc.get) {
-- if (desc.set) {
-- str = ctx.stylize('[Getter/Setter]', 'special');
-- } else {
-- str = ctx.stylize('[Getter]', 'special');
-- }
-- } else {
-- if (desc.set) {
-- str = ctx.stylize('[Setter]', 'special');
-- }
-- }
-- if (!hasOwnProperty(visibleKeys, key)) {
-- name = '[' + key + ']';
-- }
-- if (!str) {
-- if (ctx.seen.indexOf(desc.value) < 0) {
-- if (isNull(recurseTimes)) {
-- str = formatValue(ctx, desc.value, null);
-- } else {
-- str = formatValue(ctx, desc.value, recurseTimes - 1);
-- }
-- if (str.indexOf('\n') > -1) {
-- if (array) {
-- str = str.split('\n').map(function(line) {
-- return ' ' + line;
-- }).join('\n').substr(2);
-- } else {
-- str = '\n' + str.split('\n').map(function(line) {
-- return ' ' + line;
-- }).join('\n');
-- }
-- }
-- } else {
-- str = ctx.stylize('[Circular]', 'special');
-- }
-- }
-- if (isUndefined(name)) {
-- if (array && key.match(/^\d+$/)) {
-- return str;
-- }
-- name = JSON.stringify('' + key);
-- if (name.match(/^"([a-zA-Z_][a-zA-Z_0-9]*)"$/)) {
-- name = name.substr(1, name.length - 2);
-- name = ctx.stylize(name, 'name');
-- } else {
-- name = name.replace(/'/g, "\\'")
-- .replace(/\\"/g, '"')
-- .replace(/(^"|"$)/g, "'");
-- name = ctx.stylize(name, 'string');
-- }
-- }
--
-- return name + ': ' + str;
--}
--
--
--function reduceToSingleString(output, base, braces) {
-- var numLinesEst = 0;
-- var length = output.reduce(function(prev, cur) {
-- numLinesEst++;
-- if (cur.indexOf('\n') >= 0) numLinesEst++;
-- return prev + cur.replace(/\u001b\[\d\d?m/g, '').length + 1;
-- }, 0);
--
-- if (length > 60) {
-- return braces[0] +
-- (base === '' ? '' : base + '\n ') +
-- ' ' +
-- output.join(',\n ') +
-- ' ' +
-- braces[1];
-- }
--
-- return braces[0] + base + ' ' + output.join(', ') + ' ' + braces[1];
--}
--
--
- // NOTE: These type checking functions intentionally don't use `instanceof`
- // because it is fragile and can be easily faked with `Object.create()`.
- function isArray(ar) {
-@@ -522,166 +98,10 @@ function isPrimitive(arg) {
- exports.isPrimitive = isPrimitive;
-
- function isBuffer(arg) {
-- return arg instanceof Buffer;
-+ return Buffer.isBuffer(arg);
- }
- exports.isBuffer = isBuffer;
-
- function objectToString(o) {
- return Object.prototype.toString.call(o);
--}
--
--
--function pad(n) {
-- return n < 10 ? '0' + n.toString(10) : n.toString(10);
--}
--
--
--var months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep',
-- 'Oct', 'Nov', 'Dec'];
--
--// 26 Feb 16:19:34
--function timestamp() {
-- var d = new Date();
-- var time = [pad(d.getHours()),
-- pad(d.getMinutes()),
-- pad(d.getSeconds())].join(':');
-- return [d.getDate(), months[d.getMonth()], time].join(' ');
--}
--
--
--// log is just a thin wrapper to console.log that prepends a timestamp
--exports.log = function() {
-- console.log('%s - %s', timestamp(), exports.format.apply(exports, arguments));
--};
--
--
--/**
-- * Inherit the prototype methods from one constructor into another.
-- *
-- * The Function.prototype.inherits from lang.js rewritten as a standalone
-- * function (not on Function.prototype). NOTE: If this file is to be loaded
-- * during bootstrapping this function needs to be rewritten using some native
-- * functions as prototype setup using normal JavaScript does not work as
-- * expected during bootstrapping (see mirror.js in r114903).
-- *
-- * @param {function} ctor Constructor function which needs to inherit the
-- * prototype.
-- * @param {function} superCtor Constructor function to inherit prototype from.
-- */
--exports.inherits = function(ctor, superCtor) {
-- ctor.super_ = superCtor;
-- ctor.prototype = Object.create(superCtor.prototype, {
-- constructor: {
-- value: ctor,
-- enumerable: false,
-- writable: true,
-- configurable: true
-- }
-- });
--};
--
--exports._extend = function(origin, add) {
-- // Don't do anything if add isn't an object
-- if (!add || !isObject(add)) return origin;
--
-- var keys = Object.keys(add);
-- var i = keys.length;
-- while (i--) {
-- origin[keys[i]] = add[keys[i]];
-- }
-- return origin;
--};
--
--function hasOwnProperty(obj, prop) {
-- return Object.prototype.hasOwnProperty.call(obj, prop);
--}
--
--
--// Deprecated old stuff.
--
--exports.p = exports.deprecate(function() {
-- for (var i = 0, len = arguments.length; i < len; ++i) {
-- console.error(exports.inspect(arguments[i]));
-- }
--}, 'util.p: Use console.error() instead');
--
--
--exports.exec = exports.deprecate(function() {
-- return require('child_process').exec.apply(this, arguments);
--}, 'util.exec is now called `child_process.exec`.');
--
--
--exports.print = exports.deprecate(function() {
-- for (var i = 0, len = arguments.length; i < len; ++i) {
-- process.stdout.write(String(arguments[i]));
-- }
--}, 'util.print: Use console.log instead');
--
--
--exports.puts = exports.deprecate(function() {
-- for (var i = 0, len = arguments.length; i < len; ++i) {
-- process.stdout.write(arguments[i] + '\n');
-- }
--}, 'util.puts: Use console.log instead');
--
--
--exports.debug = exports.deprecate(function(x) {
-- process.stderr.write('DEBUG: ' + x + '\n');
--}, 'util.debug: Use console.error instead');
--
--
--exports.error = exports.deprecate(function(x) {
-- for (var i = 0, len = arguments.length; i < len; ++i) {
-- process.stderr.write(arguments[i] + '\n');
-- }
--}, 'util.error: Use console.error instead');
--
--
--exports.pump = exports.deprecate(function(readStream, writeStream, callback) {
-- var callbackCalled = false;
--
-- function call(a, b, c) {
-- if (callback && !callbackCalled) {
-- callback(a, b, c);
-- callbackCalled = true;
-- }
-- }
--
-- readStream.addListener('data', function(chunk) {
-- if (writeStream.write(chunk) === false) readStream.pause();
-- });
--
-- writeStream.addListener('drain', function() {
-- readStream.resume();
-- });
--
-- readStream.addListener('end', function() {
-- writeStream.end();
-- });
--
-- readStream.addListener('close', function() {
-- call();
-- });
--
-- readStream.addListener('error', function(err) {
-- writeStream.end();
-- call(err);
-- });
--
-- writeStream.addListener('error', function(err) {
-- readStream.destroy();
-- call(err);
-- });
--}, 'util.pump(): Use readableStream.pipe() instead');
--
--
--var uv;
--exports._errnoException = function(err, syscall) {
-- if (isUndefined(uv)) uv = process.binding('uv');
-- var errname = uv.errname(err);
-- var e = new Error(syscall + ' ' + errname);
-- e.code = errname;
-- e.errno = errname;
-- e.syscall = syscall;
-- return e;
--};
-+}
\ No newline at end of file
diff --git a/node_modules/core-util-is/lib/util.js b/node_modules/core-util-is/lib/util.js
deleted file mode 100644
index ff4c851..0000000
--- a/node_modules/core-util-is/lib/util.js
+++ /dev/null
@@ -1,107 +0,0 @@
-// Copyright Joyent, Inc. and other Node contributors.
-//
-// Permission is hereby granted, free of charge, to any person obtaining a
-// copy of this software and associated documentation files (the
-// "Software"), to deal in the Software without restriction, including
-// without limitation the rights to use, copy, modify, merge, publish,
-// distribute, sublicense, and/or sell copies of the Software, and to permit
-// persons to whom the Software is furnished to do so, subject to the
-// following conditions:
-//
-// The above copyright notice and this permission notice shall be included
-// in all copies or substantial portions of the Software.
-//
-// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
-// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
-// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN
-// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
-// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
-// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
-// USE OR OTHER DEALINGS IN THE SOFTWARE.
-
-// NOTE: These type checking functions intentionally don't use `instanceof`
-// because it is fragile and can be easily faked with `Object.create()`.
-
-function isArray(arg) {
- if (Array.isArray) {
- return Array.isArray(arg);
- }
- return objectToString(arg) === '[object Array]';
-}
-exports.isArray = isArray;
-
-function isBoolean(arg) {
- return typeof arg === 'boolean';
-}
-exports.isBoolean = isBoolean;
-
-function isNull(arg) {
- return arg === null;
-}
-exports.isNull = isNull;
-
-function isNullOrUndefined(arg) {
- return arg == null;
-}
-exports.isNullOrUndefined = isNullOrUndefined;
-
-function isNumber(arg) {
- return typeof arg === 'number';
-}
-exports.isNumber = isNumber;
-
-function isString(arg) {
- return typeof arg === 'string';
-}
-exports.isString = isString;
-
-function isSymbol(arg) {
- return typeof arg === 'symbol';
-}
-exports.isSymbol = isSymbol;
-
-function isUndefined(arg) {
- return arg === void 0;
-}
-exports.isUndefined = isUndefined;
-
-function isRegExp(re) {
- return objectToString(re) === '[object RegExp]';
-}
-exports.isRegExp = isRegExp;
-
-function isObject(arg) {
- return typeof arg === 'object' && arg !== null;
-}
-exports.isObject = isObject;
-
-function isDate(d) {
- return objectToString(d) === '[object Date]';
-}
-exports.isDate = isDate;
-
-function isError(e) {
- return (objectToString(e) === '[object Error]' || e instanceof Error);
-}
-exports.isError = isError;
-
-function isFunction(arg) {
- return typeof arg === 'function';
-}
-exports.isFunction = isFunction;
-
-function isPrimitive(arg) {
- return arg === null ||
- typeof arg === 'boolean' ||
- typeof arg === 'number' ||
- typeof arg === 'string' ||
- typeof arg === 'symbol' || // ES6 symbol
- typeof arg === 'undefined';
-}
-exports.isPrimitive = isPrimitive;
-
-exports.isBuffer = Buffer.isBuffer;
-
-function objectToString(o) {
- return Object.prototype.toString.call(o);
-}
diff --git a/node_modules/core-util-is/package.json b/node_modules/core-util-is/package.json
deleted file mode 100644
index 30d9e40..0000000
--- a/node_modules/core-util-is/package.json
+++ /dev/null
@@ -1,62 +0,0 @@
-{
- "_from": "core-util-is@~1.0.0",
- "_id": "core-util-is@1.0.2",
- "_inBundle": false,
- "_integrity": "sha1-tf1UIgqivFq1eqtxQMlAdUUDwac=",
- "_location": "/core-util-is",
- "_phantomChildren": {},
- "_requested": {
- "type": "range",
- "registry": true,
- "raw": "core-util-is@~1.0.0",
- "name": "core-util-is",
- "escapedName": "core-util-is",
- "rawSpec": "~1.0.0",
- "saveSpec": null,
- "fetchSpec": "~1.0.0"
- },
- "_requiredBy": [
- "/readable-stream"
- ],
- "_resolved": "https://registry.npmjs.org/core-util-is/-/core-util-is-1.0.2.tgz",
- "_shasum": "b5fd54220aa2bc5ab57aab7140c940754503c1a7",
- "_spec": "core-util-is@~1.0.0",
- "_where": "/Users/jasonagus/Desktop/Skyblock Guide/node_modules/readable-stream",
- "author": {
- "name": "Isaac Z. Schlueter",
- "email": "i@izs.me",
- "url": "http://blog.izs.me/"
- },
- "bugs": {
- "url": "https://github.com/isaacs/core-util-is/issues"
- },
- "bundleDependencies": false,
- "deprecated": false,
- "description": "The `util.is*` functions introduced in Node v0.12.",
- "devDependencies": {
- "tap": "^2.3.0"
- },
- "homepage": "https://github.com/isaacs/core-util-is#readme",
- "keywords": [
- "util",
- "isBuffer",
- "isArray",
- "isNumber",
- "isString",
- "isRegExp",
- "isThis",
- "isThat",
- "polyfill"
- ],
- "license": "MIT",
- "main": "lib/util.js",
- "name": "core-util-is",
- "repository": {
- "type": "git",
- "url": "git://github.com/isaacs/core-util-is.git"
- },
- "scripts": {
- "test": "tap test.js"
- },
- "version": "1.0.2"
-}
diff --git a/node_modules/core-util-is/test.js b/node_modules/core-util-is/test.js
deleted file mode 100644
index 1a490c6..0000000
--- a/node_modules/core-util-is/test.js
+++ /dev/null
@@ -1,68 +0,0 @@
-var assert = require('tap');
-
-var t = require('./lib/util');
-
-assert.equal(t.isArray([]), true);
-assert.equal(t.isArray({}), false);
-
-assert.equal(t.isBoolean(null), false);
-assert.equal(t.isBoolean(true), true);
-assert.equal(t.isBoolean(false), true);
-
-assert.equal(t.isNull(null), true);
-assert.equal(t.isNull(undefined), false);
-assert.equal(t.isNull(false), false);
-assert.equal(t.isNull(), false);
-
-assert.equal(t.isNullOrUndefined(null), true);
-assert.equal(t.isNullOrUndefined(undefined), true);
-assert.equal(t.isNullOrUndefined(false), false);
-assert.equal(t.isNullOrUndefined(), true);
-
-assert.equal(t.isNumber(null), false);
-assert.equal(t.isNumber('1'), false);
-assert.equal(t.isNumber(1), true);
-
-assert.equal(t.isString(null), false);
-assert.equal(t.isString('1'), true);
-assert.equal(t.isString(1), false);
-
-assert.equal(t.isSymbol(null), false);
-assert.equal(t.isSymbol('1'), false);
-assert.equal(t.isSymbol(1), false);
-assert.equal(t.isSymbol(Symbol()), true);
-
-assert.equal(t.isUndefined(null), false);
-assert.equal(t.isUndefined(undefined), true);
-assert.equal(t.isUndefined(false), false);
-assert.equal(t.isUndefined(), true);
-
-assert.equal(t.isRegExp(null), false);
-assert.equal(t.isRegExp('1'), false);
-assert.equal(t.isRegExp(new RegExp()), true);
-
-assert.equal(t.isObject({}), true);
-assert.equal(t.isObject([]), true);
-assert.equal(t.isObject(new RegExp()), true);
-assert.equal(t.isObject(new Date()), true);
-
-assert.equal(t.isDate(null), false);
-assert.equal(t.isDate('1'), false);
-assert.equal(t.isDate(new Date()), true);
-
-assert.equal(t.isError(null), false);
-assert.equal(t.isError({ err: true }), false);
-assert.equal(t.isError(new Error()), true);
-
-assert.equal(t.isFunction(null), false);
-assert.equal(t.isFunction({ }), false);
-assert.equal(t.isFunction(function() {}), true);
-
-assert.equal(t.isPrimitive(null), true);
-assert.equal(t.isPrimitive(''), true);
-assert.equal(t.isPrimitive(0), true);
-assert.equal(t.isPrimitive(new Date()), false);
-
-assert.equal(t.isBuffer(null), false);
-assert.equal(t.isBuffer({}), false);
-assert.equal(t.isBuffer(new Buffer(0)), true);
diff --git a/node_modules/denque/CHANGELOG.md b/node_modules/denque/CHANGELOG.md
new file mode 100644
index 0000000..bb5ffb6
--- /dev/null
+++ b/node_modules/denque/CHANGELOG.md
@@ -0,0 +1,4 @@
+## 1.5.0
+
+ - feat: adds capacity option for circular buffers (#27)
+
diff --git a/node_modules/denque/index.d.ts b/node_modules/denque/index.d.ts
index 803450f..05d7123 100644
--- a/node_modules/denque/index.d.ts
+++ b/node_modules/denque/index.d.ts
@@ -1,6 +1,7 @@
declare class Denque {
constructor();
constructor(array: T[]);
+ constructor(array: T[], options: IDenqueOptions);
push(item: T): number;
unshift(item: T): number;
@@ -23,4 +24,8 @@ declare class Denque {
length: number;
}
+interface IDenqueOptions {
+ capacity?: number
+}
+
export = Denque;
diff --git a/node_modules/denque/index.js b/node_modules/denque/index.js
index 2f03136..f664cd7 100644
--- a/node_modules/denque/index.js
+++ b/node_modules/denque/index.js
@@ -3,9 +3,12 @@
/**
* Custom implementation of a double ended queue.
*/
-function Denque(array) {
+function Denque(array, options) {
+ var options = options || {};
+
this._head = 0;
this._tail = 0;
+ this._capacity = options.capacity;
this._capacityMask = 0x3;
this._list = new Array(4);
if (Array.isArray(array)) {
@@ -41,7 +44,7 @@ Denque.prototype.peekAt = function peekAt(index) {
};
/**
- * Alias for peakAt()
+ * Alias for peekAt()
* @param i
* @returns {*}
*/
@@ -104,6 +107,7 @@ Denque.prototype.unshift = function unshift(item) {
this._head = (this._head - 1 + len) & this._capacityMask;
this._list[this._head] = item;
if (this._tail === this._head) this._growArray();
+ if (this._capacity && this.size() > this._capacity) this.pop();
if (this._head < this._tail) return this._tail - this._head;
else return this._capacityMask + 1 - (this._head - this._tail);
};
@@ -135,7 +139,9 @@ Denque.prototype.push = function push(item) {
if (this._tail === this._head) {
this._growArray();
}
-
+ if (this._capacity && this.size() > this._capacity) {
+ this.shift();
+ }
if (this._head < this._tail) return this._tail - this._head;
else return this._capacityMask + 1 - (this._head - this._tail);
};
diff --git a/node_modules/denque/package.json b/node_modules/denque/package.json
index 74106e5..8719718 100644
--- a/node_modules/denque/package.json
+++ b/node_modules/denque/package.json
@@ -1,27 +1,27 @@
{
- "_from": "denque@^1.4.1",
- "_id": "denque@1.4.1",
+ "_from": "denque@^1.5.0",
+ "_id": "denque@1.5.0",
"_inBundle": false,
- "_integrity": "sha512-OfzPuSZKGcgr96rf1oODnfjqBFmr1DVoc/TrItj3Ohe0Ah1C5WX5Baquw/9U9KovnQ88EqmJbD66rKYUQYN1tQ==",
+ "_integrity": "sha512-CYiCSgIF1p6EUByQPlGkKnP1M9g0ZV3qMIrqMqZqdwazygIA/YP2vrbcyl1h/WppKJTdl1F85cXIle+394iDAQ==",
"_location": "/denque",
"_phantomChildren": {},
"_requested": {
"type": "range",
"registry": true,
- "raw": "denque@^1.4.1",
+ "raw": "denque@^1.5.0",
"name": "denque",
"escapedName": "denque",
- "rawSpec": "^1.4.1",
+ "rawSpec": "^1.5.0",
"saveSpec": null,
- "fetchSpec": "^1.4.1"
+ "fetchSpec": "^1.5.0"
},
"_requiredBy": [
"/mongodb"
],
- "_resolved": "https://registry.npmjs.org/denque/-/denque-1.4.1.tgz",
- "_shasum": "6744ff7641c148c3f8a69c307e51235c1f4a37cf",
- "_spec": "denque@^1.4.1",
- "_where": "/Users/jasonagus/Desktop/Skyblock Guide/node_modules/mongodb",
+ "_resolved": "https://registry.npmjs.org/denque/-/denque-1.5.0.tgz",
+ "_shasum": "773de0686ff2d8ec2ff92914316a47b73b1c73de",
+ "_spec": "denque@^1.5.0",
+ "_where": "/Users/jasonagus/Desktop/Guide Project/node_modules/mongodb",
"author": {
"name": "Invertase",
"email": "oss@invertase.io",
@@ -80,5 +80,5 @@
"test": "istanbul cover --report lcov _mocha && npm run typescript",
"typescript": "tsc --project ./test/type/tsconfig.json"
},
- "version": "1.4.1"
+ "version": "1.5.0"
}
diff --git a/node_modules/ieee754/LICENSE b/node_modules/ieee754/LICENSE
new file mode 100644
index 0000000..5aac82c
--- /dev/null
+++ b/node_modules/ieee754/LICENSE
@@ -0,0 +1,11 @@
+Copyright 2008 Fair Oaks Labs, Inc.
+
+Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met:
+
+1. Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
+
+2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
+
+3. Neither the name of the copyright holder nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
+
+THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
diff --git a/node_modules/ieee754/README.md b/node_modules/ieee754/README.md
new file mode 100644
index 0000000..cb7527b
--- /dev/null
+++ b/node_modules/ieee754/README.md
@@ -0,0 +1,51 @@
+# ieee754 [![travis][travis-image]][travis-url] [![npm][npm-image]][npm-url] [![downloads][downloads-image]][downloads-url] [![javascript style guide][standard-image]][standard-url]
+
+[travis-image]: https://img.shields.io/travis/feross/ieee754/master.svg
+[travis-url]: https://travis-ci.org/feross/ieee754
+[npm-image]: https://img.shields.io/npm/v/ieee754.svg
+[npm-url]: https://npmjs.org/package/ieee754
+[downloads-image]: https://img.shields.io/npm/dm/ieee754.svg
+[downloads-url]: https://npmjs.org/package/ieee754
+[standard-image]: https://img.shields.io/badge/code_style-standard-brightgreen.svg
+[standard-url]: https://standardjs.com
+
+[![saucelabs][saucelabs-image]][saucelabs-url]
+
+[saucelabs-image]: https://saucelabs.com/browser-matrix/ieee754.svg
+[saucelabs-url]: https://saucelabs.com/u/ieee754
+
+### Read/write IEEE754 floating point numbers from/to a Buffer or array-like object.
+
+## install
+
+```
+npm install ieee754
+```
+
+## methods
+
+`var ieee754 = require('ieee754')`
+
+The `ieee754` object has the following functions:
+
+```
+ieee754.read = function (buffer, offset, isLE, mLen, nBytes)
+ieee754.write = function (buffer, value, offset, isLE, mLen, nBytes)
+```
+
+The arguments mean the following:
+
+- buffer = the buffer
+- offset = offset into the buffer
+- value = value to set (only for `write`)
+- isLe = is little endian?
+- mLen = mantissa length
+- nBytes = number of bytes
+
+## what is ieee754?
+
+The IEEE Standard for Floating-Point Arithmetic (IEEE 754) is a technical standard for floating-point computation. [Read more](http://en.wikipedia.org/wiki/IEEE_floating_point).
+
+## license
+
+BSD 3 Clause. Copyright (c) 2008, Fair Oaks Labs, Inc.
diff --git a/node_modules/ieee754/index.d.ts b/node_modules/ieee754/index.d.ts
new file mode 100644
index 0000000..f1e4354
--- /dev/null
+++ b/node_modules/ieee754/index.d.ts
@@ -0,0 +1,10 @@
+declare namespace ieee754 {
+ export function read(
+ buffer: Uint8Array, offset: number, isLE: boolean, mLen: number,
+ nBytes: number): number;
+ export function write(
+ buffer: Uint8Array, value: number, offset: number, isLE: boolean,
+ mLen: number, nBytes: number): void;
+ }
+
+ export = ieee754;
\ No newline at end of file
diff --git a/node_modules/ieee754/index.js b/node_modules/ieee754/index.js
new file mode 100644
index 0000000..81d26c3
--- /dev/null
+++ b/node_modules/ieee754/index.js
@@ -0,0 +1,85 @@
+/*! ieee754. BSD-3-Clause License. Feross Aboukhadijeh */
+exports.read = function (buffer, offset, isLE, mLen, nBytes) {
+ var e, m
+ var eLen = (nBytes * 8) - mLen - 1
+ var eMax = (1 << eLen) - 1
+ var eBias = eMax >> 1
+ var nBits = -7
+ var i = isLE ? (nBytes - 1) : 0
+ var d = isLE ? -1 : 1
+ var s = buffer[offset + i]
+
+ i += d
+
+ e = s & ((1 << (-nBits)) - 1)
+ s >>= (-nBits)
+ nBits += eLen
+ for (; nBits > 0; e = (e * 256) + buffer[offset + i], i += d, nBits -= 8) {}
+
+ m = e & ((1 << (-nBits)) - 1)
+ e >>= (-nBits)
+ nBits += mLen
+ for (; nBits > 0; m = (m * 256) + buffer[offset + i], i += d, nBits -= 8) {}
+
+ if (e === 0) {
+ e = 1 - eBias
+ } else if (e === eMax) {
+ return m ? NaN : ((s ? -1 : 1) * Infinity)
+ } else {
+ m = m + Math.pow(2, mLen)
+ e = e - eBias
+ }
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen)
+}
+
+exports.write = function (buffer, value, offset, isLE, mLen, nBytes) {
+ var e, m, c
+ var eLen = (nBytes * 8) - mLen - 1
+ var eMax = (1 << eLen) - 1
+ var eBias = eMax >> 1
+ var rt = (mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0)
+ var i = isLE ? 0 : (nBytes - 1)
+ var d = isLE ? 1 : -1
+ var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0
+
+ value = Math.abs(value)
+
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0
+ e = eMax
+ } else {
+ e = Math.floor(Math.log(value) / Math.LN2)
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--
+ c *= 2
+ }
+ if (e + eBias >= 1) {
+ value += rt / c
+ } else {
+ value += rt * Math.pow(2, 1 - eBias)
+ }
+ if (value * c >= 2) {
+ e++
+ c /= 2
+ }
+
+ if (e + eBias >= eMax) {
+ m = 0
+ e = eMax
+ } else if (e + eBias >= 1) {
+ m = ((value * c) - 1) * Math.pow(2, mLen)
+ e = e + eBias
+ } else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen)
+ e = 0
+ }
+ }
+
+ for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}
+
+ e = (e << mLen) | m
+ eLen += mLen
+ for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}
+
+ buffer[offset + i - d] |= s * 128
+}
diff --git a/node_modules/ieee754/package.json b/node_modules/ieee754/package.json
new file mode 100644
index 0000000..d35b10a
--- /dev/null
+++ b/node_modules/ieee754/package.json
@@ -0,0 +1,84 @@
+{
+ "_from": "ieee754@^1.1.13",
+ "_id": "ieee754@1.2.1",
+ "_inBundle": false,
+ "_integrity": "sha512-dcyqhDvX1C46lXZcVqCpK+FtMRQVdIMN6/Df5js2zouUsqG7I6sFxitIC+7KYK29KdXOLHdu9zL4sFnoVQnqaA==",
+ "_location": "/ieee754",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "ieee754@^1.1.13",
+ "name": "ieee754",
+ "escapedName": "ieee754",
+ "rawSpec": "^1.1.13",
+ "saveSpec": null,
+ "fetchSpec": "^1.1.13"
+ },
+ "_requiredBy": [
+ "/buffer"
+ ],
+ "_resolved": "https://registry.npmjs.org/ieee754/-/ieee754-1.2.1.tgz",
+ "_shasum": "8eb7a10a63fff25d15a57b001586d177d1b0d352",
+ "_spec": "ieee754@^1.1.13",
+ "_where": "/Users/jasonagus/Desktop/Guide Project/node_modules/buffer",
+ "author": {
+ "name": "Feross Aboukhadijeh",
+ "email": "feross@feross.org",
+ "url": "https://feross.org"
+ },
+ "bugs": {
+ "url": "https://github.com/feross/ieee754/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "Romain Beauxis",
+ "email": "toots@rastageeks.org"
+ }
+ ],
+ "deprecated": false,
+ "description": "Read/write IEEE754 floating point numbers from/to a Buffer or array-like object",
+ "devDependencies": {
+ "airtap": "^3.0.0",
+ "standard": "*",
+ "tape": "^5.0.1"
+ },
+ "funding": [
+ {
+ "type": "github",
+ "url": "https://github.com/sponsors/feross"
+ },
+ {
+ "type": "patreon",
+ "url": "https://www.patreon.com/feross"
+ },
+ {
+ "type": "consulting",
+ "url": "https://feross.org/support"
+ }
+ ],
+ "homepage": "https://github.com/feross/ieee754#readme",
+ "keywords": [
+ "IEEE 754",
+ "buffer",
+ "convert",
+ "floating point",
+ "ieee754"
+ ],
+ "license": "BSD-3-Clause",
+ "main": "index.js",
+ "name": "ieee754",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/feross/ieee754.git"
+ },
+ "scripts": {
+ "test": "standard && npm run test-node && npm run test-browser",
+ "test-browser": "airtap -- test/*.js",
+ "test-browser-local": "airtap --local -- test/*.js",
+ "test-node": "tape test/*.js"
+ },
+ "types": "index.d.ts",
+ "version": "1.2.1"
+}
diff --git a/node_modules/inherits/LICENSE b/node_modules/inherits/LICENSE
deleted file mode 100644
index dea3013..0000000
--- a/node_modules/inherits/LICENSE
+++ /dev/null
@@ -1,16 +0,0 @@
-The ISC License
-
-Copyright (c) Isaac Z. Schlueter
-
-Permission to use, copy, modify, and/or distribute this software for any
-purpose with or without fee is hereby granted, provided that the above
-copyright notice and this permission notice appear in all copies.
-
-THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH
-REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND
-FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,
-INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM
-LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR
-OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR
-PERFORMANCE OF THIS SOFTWARE.
-
diff --git a/node_modules/inherits/README.md b/node_modules/inherits/README.md
deleted file mode 100644
index b1c5665..0000000
--- a/node_modules/inherits/README.md
+++ /dev/null
@@ -1,42 +0,0 @@
-Browser-friendly inheritance fully compatible with standard node.js
-[inherits](http://nodejs.org/api/util.html#util_util_inherits_constructor_superconstructor).
-
-This package exports standard `inherits` from node.js `util` module in
-node environment, but also provides alternative browser-friendly
-implementation through [browser
-field](https://gist.github.com/shtylman/4339901). Alternative
-implementation is a literal copy of standard one located in standalone
-module to avoid requiring of `util`. It also has a shim for old
-browsers with no `Object.create` support.
-
-While keeping you sure you are using standard `inherits`
-implementation in node.js environment, it allows bundlers such as
-[browserify](https://github.com/substack/node-browserify) to not
-include full `util` package to your client code if all you need is
-just `inherits` function. It worth, because browser shim for `util`
-package is large and `inherits` is often the single function you need
-from it.
-
-It's recommended to use this package instead of
-`require('util').inherits` for any code that has chances to be used
-not only in node.js but in browser too.
-
-## usage
-
-```js
-var inherits = require('inherits');
-// then use exactly as the standard one
-```
-
-## note on version ~1.0
-
-Version ~1.0 had completely different motivation and is not compatible
-neither with 2.0 nor with standard node.js `inherits`.
-
-If you are using version ~1.0 and planning to switch to ~2.0, be
-careful:
-
-* new version uses `super_` instead of `super` for referencing
- superclass
-* new version overwrites current prototype while old one preserves any
- existing fields on it
diff --git a/node_modules/inherits/inherits.js b/node_modules/inherits/inherits.js
deleted file mode 100644
index f71f2d9..0000000
--- a/node_modules/inherits/inherits.js
+++ /dev/null
@@ -1,9 +0,0 @@
-try {
- var util = require('util');
- /* istanbul ignore next */
- if (typeof util.inherits !== 'function') throw '';
- module.exports = util.inherits;
-} catch (e) {
- /* istanbul ignore next */
- module.exports = require('./inherits_browser.js');
-}
diff --git a/node_modules/inherits/inherits_browser.js b/node_modules/inherits/inherits_browser.js
deleted file mode 100644
index 86bbb3d..0000000
--- a/node_modules/inherits/inherits_browser.js
+++ /dev/null
@@ -1,27 +0,0 @@
-if (typeof Object.create === 'function') {
- // implementation from standard node.js 'util' module
- module.exports = function inherits(ctor, superCtor) {
- if (superCtor) {
- ctor.super_ = superCtor
- ctor.prototype = Object.create(superCtor.prototype, {
- constructor: {
- value: ctor,
- enumerable: false,
- writable: true,
- configurable: true
- }
- })
- }
- };
-} else {
- // old school shim for old browsers
- module.exports = function inherits(ctor, superCtor) {
- if (superCtor) {
- ctor.super_ = superCtor
- var TempCtor = function () {}
- TempCtor.prototype = superCtor.prototype
- ctor.prototype = new TempCtor()
- ctor.prototype.constructor = ctor
- }
- }
-}
diff --git a/node_modules/inherits/package.json b/node_modules/inherits/package.json
deleted file mode 100644
index 67e1222..0000000
--- a/node_modules/inherits/package.json
+++ /dev/null
@@ -1,61 +0,0 @@
-{
- "_from": "inherits@~2.0.3",
- "_id": "inherits@2.0.4",
- "_inBundle": false,
- "_integrity": "sha512-k/vGaX4/Yla3WzyMCvTQOXYeIHvqOKtnqBduzTHpzpQZzAskKMhZ2K+EnBiSM9zGSoIFeMpXKxa4dYeZIQqewQ==",
- "_location": "/inherits",
- "_phantomChildren": {},
- "_requested": {
- "type": "range",
- "registry": true,
- "raw": "inherits@~2.0.3",
- "name": "inherits",
- "escapedName": "inherits",
- "rawSpec": "~2.0.3",
- "saveSpec": null,
- "fetchSpec": "~2.0.3"
- },
- "_requiredBy": [
- "/readable-stream"
- ],
- "_resolved": "https://registry.npmjs.org/inherits/-/inherits-2.0.4.tgz",
- "_shasum": "0fa2c64f932917c3433a0ded55363aae37416b7c",
- "_spec": "inherits@~2.0.3",
- "_where": "/Users/jasonagus/Desktop/Skyblock Guide/node_modules/readable-stream",
- "browser": "./inherits_browser.js",
- "bugs": {
- "url": "https://github.com/isaacs/inherits/issues"
- },
- "bundleDependencies": false,
- "deprecated": false,
- "description": "Browser-friendly inheritance fully compatible with standard node.js inherits()",
- "devDependencies": {
- "tap": "^14.2.4"
- },
- "files": [
- "inherits.js",
- "inherits_browser.js"
- ],
- "homepage": "https://github.com/isaacs/inherits#readme",
- "keywords": [
- "inheritance",
- "class",
- "klass",
- "oop",
- "object-oriented",
- "inherits",
- "browser",
- "browserify"
- ],
- "license": "ISC",
- "main": "./inherits.js",
- "name": "inherits",
- "repository": {
- "type": "git",
- "url": "git://github.com/isaacs/inherits.git"
- },
- "scripts": {
- "test": "tap"
- },
- "version": "2.0.4"
-}
diff --git a/node_modules/isarray/.npmignore b/node_modules/isarray/.npmignore
deleted file mode 100644
index 3c3629e..0000000
--- a/node_modules/isarray/.npmignore
+++ /dev/null
@@ -1 +0,0 @@
-node_modules
diff --git a/node_modules/isarray/.travis.yml b/node_modules/isarray/.travis.yml
deleted file mode 100644
index cc4dba2..0000000
--- a/node_modules/isarray/.travis.yml
+++ /dev/null
@@ -1,4 +0,0 @@
-language: node_js
-node_js:
- - "0.8"
- - "0.10"
diff --git a/node_modules/isarray/Makefile b/node_modules/isarray/Makefile
deleted file mode 100644
index 787d56e..0000000
--- a/node_modules/isarray/Makefile
+++ /dev/null
@@ -1,6 +0,0 @@
-
-test:
- @node_modules/.bin/tape test.js
-
-.PHONY: test
-
diff --git a/node_modules/isarray/README.md b/node_modules/isarray/README.md
deleted file mode 100644
index 16d2c59..0000000
--- a/node_modules/isarray/README.md
+++ /dev/null
@@ -1,60 +0,0 @@
-
-# isarray
-
-`Array#isArray` for older browsers.
-
-[](http://travis-ci.org/juliangruber/isarray)
-[](https://www.npmjs.org/package/isarray)
-
-[
-](https://ci.testling.com/juliangruber/isarray)
-
-## Usage
-
-```js
-var isArray = require('isarray');
-
-console.log(isArray([])); // => true
-console.log(isArray({})); // => false
-```
-
-## Installation
-
-With [npm](http://npmjs.org) do
-
-```bash
-$ npm install isarray
-```
-
-Then bundle for the browser with
-[browserify](https://github.com/substack/browserify).
-
-With [component](http://component.io) do
-
-```bash
-$ component install juliangruber/isarray
-```
-
-## License
-
-(MIT)
-
-Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
-
-Permission is hereby granted, free of charge, to any person obtaining a copy of
-this software and associated documentation files (the "Software"), to deal in
-the Software without restriction, including without limitation the rights to
-use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
-of the Software, and to permit persons to whom the Software is furnished to do
-so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/node_modules/isarray/component.json b/node_modules/isarray/component.json
deleted file mode 100644
index 9e31b68..0000000
--- a/node_modules/isarray/component.json
+++ /dev/null
@@ -1,19 +0,0 @@
-{
- "name" : "isarray",
- "description" : "Array#isArray for older browsers",
- "version" : "0.0.1",
- "repository" : "juliangruber/isarray",
- "homepage": "https://github.com/juliangruber/isarray",
- "main" : "index.js",
- "scripts" : [
- "index.js"
- ],
- "dependencies" : {},
- "keywords": ["browser","isarray","array"],
- "author": {
- "name": "Julian Gruber",
- "email": "mail@juliangruber.com",
- "url": "http://juliangruber.com"
- },
- "license": "MIT"
-}
diff --git a/node_modules/isarray/index.js b/node_modules/isarray/index.js
deleted file mode 100644
index a57f634..0000000
--- a/node_modules/isarray/index.js
+++ /dev/null
@@ -1,5 +0,0 @@
-var toString = {}.toString;
-
-module.exports = Array.isArray || function (arr) {
- return toString.call(arr) == '[object Array]';
-};
diff --git a/node_modules/isarray/package.json b/node_modules/isarray/package.json
deleted file mode 100644
index a744d63..0000000
--- a/node_modules/isarray/package.json
+++ /dev/null
@@ -1,73 +0,0 @@
-{
- "_from": "isarray@~1.0.0",
- "_id": "isarray@1.0.0",
- "_inBundle": false,
- "_integrity": "sha1-u5NdSFgsuhaMBoNJV6VKPgcSTxE=",
- "_location": "/isarray",
- "_phantomChildren": {},
- "_requested": {
- "type": "range",
- "registry": true,
- "raw": "isarray@~1.0.0",
- "name": "isarray",
- "escapedName": "isarray",
- "rawSpec": "~1.0.0",
- "saveSpec": null,
- "fetchSpec": "~1.0.0"
- },
- "_requiredBy": [
- "/readable-stream"
- ],
- "_resolved": "https://registry.npmjs.org/isarray/-/isarray-1.0.0.tgz",
- "_shasum": "bb935d48582cba168c06834957a54a3e07124f11",
- "_spec": "isarray@~1.0.0",
- "_where": "/Users/jasonagus/Desktop/Skyblock Guide/node_modules/readable-stream",
- "author": {
- "name": "Julian Gruber",
- "email": "mail@juliangruber.com",
- "url": "http://juliangruber.com"
- },
- "bugs": {
- "url": "https://github.com/juliangruber/isarray/issues"
- },
- "bundleDependencies": false,
- "dependencies": {},
- "deprecated": false,
- "description": "Array#isArray for older browsers",
- "devDependencies": {
- "tape": "~2.13.4"
- },
- "homepage": "https://github.com/juliangruber/isarray",
- "keywords": [
- "browser",
- "isarray",
- "array"
- ],
- "license": "MIT",
- "main": "index.js",
- "name": "isarray",
- "repository": {
- "type": "git",
- "url": "git://github.com/juliangruber/isarray.git"
- },
- "scripts": {
- "test": "tape test.js"
- },
- "testling": {
- "files": "test.js",
- "browsers": [
- "ie/8..latest",
- "firefox/17..latest",
- "firefox/nightly",
- "chrome/22..latest",
- "chrome/canary",
- "opera/12..latest",
- "opera/next",
- "safari/5.1..latest",
- "ipad/6.0..latest",
- "iphone/6.0..latest",
- "android-browser/4.2..latest"
- ]
- },
- "version": "1.0.0"
-}
diff --git a/node_modules/isarray/test.js b/node_modules/isarray/test.js
deleted file mode 100644
index e0c3444..0000000
--- a/node_modules/isarray/test.js
+++ /dev/null
@@ -1,20 +0,0 @@
-var isArray = require('./');
-var test = require('tape');
-
-test('is array', function(t){
- t.ok(isArray([]));
- t.notOk(isArray({}));
- t.notOk(isArray(null));
- t.notOk(isArray(false));
-
- var obj = {};
- obj[0] = true;
- t.notOk(isArray(obj));
-
- var arr = [];
- arr.foo = 'bar';
- t.ok(isArray(arr));
-
- t.end();
-});
-
diff --git a/node_modules/lodash/LICENSE b/node_modules/lodash/LICENSE
new file mode 100644
index 0000000..77c42f1
--- /dev/null
+++ b/node_modules/lodash/LICENSE
@@ -0,0 +1,47 @@
+Copyright OpenJS Foundation and other contributors
+
+Based on Underscore.js, copyright Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+This software consists of voluntary contributions made by many
+individuals. For exact contribution history, see the revision history
+available at https://github.com/lodash/lodash
+
+The following license applies to all parts of this software except as
+documented below:
+
+====
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+
+====
+
+Copyright and related rights for sample code are waived via CC0. Sample
+code is defined as all source code displayed within the prose of the
+documentation.
+
+CC0: http://creativecommons.org/publicdomain/zero/1.0/
+
+====
+
+Files located in the node_modules and vendor directories are externally
+maintained libraries used by this software which have their own
+licenses; we recommend you read them, as their terms may differ from the
+terms above.
diff --git a/node_modules/lodash/README.md b/node_modules/lodash/README.md
new file mode 100644
index 0000000..3ab1a05
--- /dev/null
+++ b/node_modules/lodash/README.md
@@ -0,0 +1,39 @@
+# lodash v4.17.21
+
+The [Lodash](https://lodash.com/) library exported as [Node.js](https://nodejs.org/) modules.
+
+## Installation
+
+Using npm:
+```shell
+$ npm i -g npm
+$ npm i --save lodash
+```
+
+In Node.js:
+```js
+// Load the full build.
+var _ = require('lodash');
+// Load the core build.
+var _ = require('lodash/core');
+// Load the FP build for immutable auto-curried iteratee-first data-last methods.
+var fp = require('lodash/fp');
+
+// Load method categories.
+var array = require('lodash/array');
+var object = require('lodash/fp/object');
+
+// Cherry-pick methods for smaller browserify/rollup/webpack bundles.
+var at = require('lodash/at');
+var curryN = require('lodash/fp/curryN');
+```
+
+See the [package source](https://github.com/lodash/lodash/tree/4.17.21-npm) for more details.
+
+**Note:**
+Install [n_](https://www.npmjs.com/package/n_) for Lodash use in the Node.js < 6 REPL.
+
+## Support
+
+Tested in Chrome 74-75, Firefox 66-67, IE 11, Edge 18, Safari 11-12, & Node.js 8-12.
+Automated [browser](https://saucelabs.com/u/lodash) & [CI](https://travis-ci.org/lodash/lodash/) test runs are available.
diff --git a/node_modules/lodash/_DataView.js b/node_modules/lodash/_DataView.js
new file mode 100644
index 0000000..ac2d57c
--- /dev/null
+++ b/node_modules/lodash/_DataView.js
@@ -0,0 +1,7 @@
+var getNative = require('./_getNative'),
+ root = require('./_root');
+
+/* Built-in method references that are verified to be native. */
+var DataView = getNative(root, 'DataView');
+
+module.exports = DataView;
diff --git a/node_modules/lodash/_Hash.js b/node_modules/lodash/_Hash.js
new file mode 100644
index 0000000..b504fe3
--- /dev/null
+++ b/node_modules/lodash/_Hash.js
@@ -0,0 +1,32 @@
+var hashClear = require('./_hashClear'),
+ hashDelete = require('./_hashDelete'),
+ hashGet = require('./_hashGet'),
+ hashHas = require('./_hashHas'),
+ hashSet = require('./_hashSet');
+
+/**
+ * Creates a hash object.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function Hash(entries) {
+ var index = -1,
+ length = entries == null ? 0 : entries.length;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+}
+
+// Add methods to `Hash`.
+Hash.prototype.clear = hashClear;
+Hash.prototype['delete'] = hashDelete;
+Hash.prototype.get = hashGet;
+Hash.prototype.has = hashHas;
+Hash.prototype.set = hashSet;
+
+module.exports = Hash;
diff --git a/node_modules/lodash/_LazyWrapper.js b/node_modules/lodash/_LazyWrapper.js
new file mode 100644
index 0000000..81786c7
--- /dev/null
+++ b/node_modules/lodash/_LazyWrapper.js
@@ -0,0 +1,28 @@
+var baseCreate = require('./_baseCreate'),
+ baseLodash = require('./_baseLodash');
+
+/** Used as references for the maximum length and index of an array. */
+var MAX_ARRAY_LENGTH = 4294967295;
+
+/**
+ * Creates a lazy wrapper object which wraps `value` to enable lazy evaluation.
+ *
+ * @private
+ * @constructor
+ * @param {*} value The value to wrap.
+ */
+function LazyWrapper(value) {
+ this.__wrapped__ = value;
+ this.__actions__ = [];
+ this.__dir__ = 1;
+ this.__filtered__ = false;
+ this.__iteratees__ = [];
+ this.__takeCount__ = MAX_ARRAY_LENGTH;
+ this.__views__ = [];
+}
+
+// Ensure `LazyWrapper` is an instance of `baseLodash`.
+LazyWrapper.prototype = baseCreate(baseLodash.prototype);
+LazyWrapper.prototype.constructor = LazyWrapper;
+
+module.exports = LazyWrapper;
diff --git a/node_modules/lodash/_ListCache.js b/node_modules/lodash/_ListCache.js
new file mode 100644
index 0000000..26895c3
--- /dev/null
+++ b/node_modules/lodash/_ListCache.js
@@ -0,0 +1,32 @@
+var listCacheClear = require('./_listCacheClear'),
+ listCacheDelete = require('./_listCacheDelete'),
+ listCacheGet = require('./_listCacheGet'),
+ listCacheHas = require('./_listCacheHas'),
+ listCacheSet = require('./_listCacheSet');
+
+/**
+ * Creates an list cache object.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function ListCache(entries) {
+ var index = -1,
+ length = entries == null ? 0 : entries.length;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+}
+
+// Add methods to `ListCache`.
+ListCache.prototype.clear = listCacheClear;
+ListCache.prototype['delete'] = listCacheDelete;
+ListCache.prototype.get = listCacheGet;
+ListCache.prototype.has = listCacheHas;
+ListCache.prototype.set = listCacheSet;
+
+module.exports = ListCache;
diff --git a/node_modules/lodash/_LodashWrapper.js b/node_modules/lodash/_LodashWrapper.js
new file mode 100644
index 0000000..c1e4d9d
--- /dev/null
+++ b/node_modules/lodash/_LodashWrapper.js
@@ -0,0 +1,22 @@
+var baseCreate = require('./_baseCreate'),
+ baseLodash = require('./_baseLodash');
+
+/**
+ * The base constructor for creating `lodash` wrapper objects.
+ *
+ * @private
+ * @param {*} value The value to wrap.
+ * @param {boolean} [chainAll] Enable explicit method chain sequences.
+ */
+function LodashWrapper(value, chainAll) {
+ this.__wrapped__ = value;
+ this.__actions__ = [];
+ this.__chain__ = !!chainAll;
+ this.__index__ = 0;
+ this.__values__ = undefined;
+}
+
+LodashWrapper.prototype = baseCreate(baseLodash.prototype);
+LodashWrapper.prototype.constructor = LodashWrapper;
+
+module.exports = LodashWrapper;
diff --git a/node_modules/lodash/_Map.js b/node_modules/lodash/_Map.js
new file mode 100644
index 0000000..b73f29a
--- /dev/null
+++ b/node_modules/lodash/_Map.js
@@ -0,0 +1,7 @@
+var getNative = require('./_getNative'),
+ root = require('./_root');
+
+/* Built-in method references that are verified to be native. */
+var Map = getNative(root, 'Map');
+
+module.exports = Map;
diff --git a/node_modules/lodash/_MapCache.js b/node_modules/lodash/_MapCache.js
new file mode 100644
index 0000000..4a4eea7
--- /dev/null
+++ b/node_modules/lodash/_MapCache.js
@@ -0,0 +1,32 @@
+var mapCacheClear = require('./_mapCacheClear'),
+ mapCacheDelete = require('./_mapCacheDelete'),
+ mapCacheGet = require('./_mapCacheGet'),
+ mapCacheHas = require('./_mapCacheHas'),
+ mapCacheSet = require('./_mapCacheSet');
+
+/**
+ * Creates a map cache object to store key-value pairs.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function MapCache(entries) {
+ var index = -1,
+ length = entries == null ? 0 : entries.length;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+}
+
+// Add methods to `MapCache`.
+MapCache.prototype.clear = mapCacheClear;
+MapCache.prototype['delete'] = mapCacheDelete;
+MapCache.prototype.get = mapCacheGet;
+MapCache.prototype.has = mapCacheHas;
+MapCache.prototype.set = mapCacheSet;
+
+module.exports = MapCache;
diff --git a/node_modules/lodash/_Promise.js b/node_modules/lodash/_Promise.js
new file mode 100644
index 0000000..247b9e1
--- /dev/null
+++ b/node_modules/lodash/_Promise.js
@@ -0,0 +1,7 @@
+var getNative = require('./_getNative'),
+ root = require('./_root');
+
+/* Built-in method references that are verified to be native. */
+var Promise = getNative(root, 'Promise');
+
+module.exports = Promise;
diff --git a/node_modules/lodash/_Set.js b/node_modules/lodash/_Set.js
new file mode 100644
index 0000000..b3c8dcb
--- /dev/null
+++ b/node_modules/lodash/_Set.js
@@ -0,0 +1,7 @@
+var getNative = require('./_getNative'),
+ root = require('./_root');
+
+/* Built-in method references that are verified to be native. */
+var Set = getNative(root, 'Set');
+
+module.exports = Set;
diff --git a/node_modules/lodash/_SetCache.js b/node_modules/lodash/_SetCache.js
new file mode 100644
index 0000000..6468b06
--- /dev/null
+++ b/node_modules/lodash/_SetCache.js
@@ -0,0 +1,27 @@
+var MapCache = require('./_MapCache'),
+ setCacheAdd = require('./_setCacheAdd'),
+ setCacheHas = require('./_setCacheHas');
+
+/**
+ *
+ * Creates an array cache object to store unique values.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [values] The values to cache.
+ */
+function SetCache(values) {
+ var index = -1,
+ length = values == null ? 0 : values.length;
+
+ this.__data__ = new MapCache;
+ while (++index < length) {
+ this.add(values[index]);
+ }
+}
+
+// Add methods to `SetCache`.
+SetCache.prototype.add = SetCache.prototype.push = setCacheAdd;
+SetCache.prototype.has = setCacheHas;
+
+module.exports = SetCache;
diff --git a/node_modules/lodash/_Stack.js b/node_modules/lodash/_Stack.js
new file mode 100644
index 0000000..80b2cf1
--- /dev/null
+++ b/node_modules/lodash/_Stack.js
@@ -0,0 +1,27 @@
+var ListCache = require('./_ListCache'),
+ stackClear = require('./_stackClear'),
+ stackDelete = require('./_stackDelete'),
+ stackGet = require('./_stackGet'),
+ stackHas = require('./_stackHas'),
+ stackSet = require('./_stackSet');
+
+/**
+ * Creates a stack cache object to store key-value pairs.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function Stack(entries) {
+ var data = this.__data__ = new ListCache(entries);
+ this.size = data.size;
+}
+
+// Add methods to `Stack`.
+Stack.prototype.clear = stackClear;
+Stack.prototype['delete'] = stackDelete;
+Stack.prototype.get = stackGet;
+Stack.prototype.has = stackHas;
+Stack.prototype.set = stackSet;
+
+module.exports = Stack;
diff --git a/node_modules/lodash/_Symbol.js b/node_modules/lodash/_Symbol.js
new file mode 100644
index 0000000..a013f7c
--- /dev/null
+++ b/node_modules/lodash/_Symbol.js
@@ -0,0 +1,6 @@
+var root = require('./_root');
+
+/** Built-in value references. */
+var Symbol = root.Symbol;
+
+module.exports = Symbol;
diff --git a/node_modules/lodash/_Uint8Array.js b/node_modules/lodash/_Uint8Array.js
new file mode 100644
index 0000000..2fb30e1
--- /dev/null
+++ b/node_modules/lodash/_Uint8Array.js
@@ -0,0 +1,6 @@
+var root = require('./_root');
+
+/** Built-in value references. */
+var Uint8Array = root.Uint8Array;
+
+module.exports = Uint8Array;
diff --git a/node_modules/lodash/_WeakMap.js b/node_modules/lodash/_WeakMap.js
new file mode 100644
index 0000000..567f86c
--- /dev/null
+++ b/node_modules/lodash/_WeakMap.js
@@ -0,0 +1,7 @@
+var getNative = require('./_getNative'),
+ root = require('./_root');
+
+/* Built-in method references that are verified to be native. */
+var WeakMap = getNative(root, 'WeakMap');
+
+module.exports = WeakMap;
diff --git a/node_modules/lodash/_apply.js b/node_modules/lodash/_apply.js
new file mode 100644
index 0000000..36436dd
--- /dev/null
+++ b/node_modules/lodash/_apply.js
@@ -0,0 +1,21 @@
+/**
+ * A faster alternative to `Function#apply`, this function invokes `func`
+ * with the `this` binding of `thisArg` and the arguments of `args`.
+ *
+ * @private
+ * @param {Function} func The function to invoke.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {Array} args The arguments to invoke `func` with.
+ * @returns {*} Returns the result of `func`.
+ */
+function apply(func, thisArg, args) {
+ switch (args.length) {
+ case 0: return func.call(thisArg);
+ case 1: return func.call(thisArg, args[0]);
+ case 2: return func.call(thisArg, args[0], args[1]);
+ case 3: return func.call(thisArg, args[0], args[1], args[2]);
+ }
+ return func.apply(thisArg, args);
+}
+
+module.exports = apply;
diff --git a/node_modules/lodash/_arrayAggregator.js b/node_modules/lodash/_arrayAggregator.js
new file mode 100644
index 0000000..d96c3ca
--- /dev/null
+++ b/node_modules/lodash/_arrayAggregator.js
@@ -0,0 +1,22 @@
+/**
+ * A specialized version of `baseAggregator` for arrays.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} setter The function to set `accumulator` values.
+ * @param {Function} iteratee The iteratee to transform keys.
+ * @param {Object} accumulator The initial aggregated object.
+ * @returns {Function} Returns `accumulator`.
+ */
+function arrayAggregator(array, setter, iteratee, accumulator) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ while (++index < length) {
+ var value = array[index];
+ setter(accumulator, value, iteratee(value), array);
+ }
+ return accumulator;
+}
+
+module.exports = arrayAggregator;
diff --git a/node_modules/lodash/_arrayEach.js b/node_modules/lodash/_arrayEach.js
new file mode 100644
index 0000000..2c5f579
--- /dev/null
+++ b/node_modules/lodash/_arrayEach.js
@@ -0,0 +1,22 @@
+/**
+ * A specialized version of `_.forEach` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns `array`.
+ */
+function arrayEach(array, iteratee) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ while (++index < length) {
+ if (iteratee(array[index], index, array) === false) {
+ break;
+ }
+ }
+ return array;
+}
+
+module.exports = arrayEach;
diff --git a/node_modules/lodash/_arrayEachRight.js b/node_modules/lodash/_arrayEachRight.js
new file mode 100644
index 0000000..976ca5c
--- /dev/null
+++ b/node_modules/lodash/_arrayEachRight.js
@@ -0,0 +1,21 @@
+/**
+ * A specialized version of `_.forEachRight` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns `array`.
+ */
+function arrayEachRight(array, iteratee) {
+ var length = array == null ? 0 : array.length;
+
+ while (length--) {
+ if (iteratee(array[length], length, array) === false) {
+ break;
+ }
+ }
+ return array;
+}
+
+module.exports = arrayEachRight;
diff --git a/node_modules/lodash/_arrayEvery.js b/node_modules/lodash/_arrayEvery.js
new file mode 100644
index 0000000..e26a918
--- /dev/null
+++ b/node_modules/lodash/_arrayEvery.js
@@ -0,0 +1,23 @@
+/**
+ * A specialized version of `_.every` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ */
+function arrayEvery(array, predicate) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ while (++index < length) {
+ if (!predicate(array[index], index, array)) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = arrayEvery;
diff --git a/node_modules/lodash/_arrayFilter.js b/node_modules/lodash/_arrayFilter.js
new file mode 100644
index 0000000..75ea254
--- /dev/null
+++ b/node_modules/lodash/_arrayFilter.js
@@ -0,0 +1,25 @@
+/**
+ * A specialized version of `_.filter` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ */
+function arrayFilter(array, predicate) {
+ var index = -1,
+ length = array == null ? 0 : array.length,
+ resIndex = 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (predicate(value, index, array)) {
+ result[resIndex++] = value;
+ }
+ }
+ return result;
+}
+
+module.exports = arrayFilter;
diff --git a/node_modules/lodash/_arrayIncludes.js b/node_modules/lodash/_arrayIncludes.js
new file mode 100644
index 0000000..3737a6d
--- /dev/null
+++ b/node_modules/lodash/_arrayIncludes.js
@@ -0,0 +1,17 @@
+var baseIndexOf = require('./_baseIndexOf');
+
+/**
+ * A specialized version of `_.includes` for arrays without support for
+ * specifying an index to search from.
+ *
+ * @private
+ * @param {Array} [array] The array to inspect.
+ * @param {*} target The value to search for.
+ * @returns {boolean} Returns `true` if `target` is found, else `false`.
+ */
+function arrayIncludes(array, value) {
+ var length = array == null ? 0 : array.length;
+ return !!length && baseIndexOf(array, value, 0) > -1;
+}
+
+module.exports = arrayIncludes;
diff --git a/node_modules/lodash/_arrayIncludesWith.js b/node_modules/lodash/_arrayIncludesWith.js
new file mode 100644
index 0000000..235fd97
--- /dev/null
+++ b/node_modules/lodash/_arrayIncludesWith.js
@@ -0,0 +1,22 @@
+/**
+ * This function is like `arrayIncludes` except that it accepts a comparator.
+ *
+ * @private
+ * @param {Array} [array] The array to inspect.
+ * @param {*} target The value to search for.
+ * @param {Function} comparator The comparator invoked per element.
+ * @returns {boolean} Returns `true` if `target` is found, else `false`.
+ */
+function arrayIncludesWith(array, value, comparator) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ while (++index < length) {
+ if (comparator(value, array[index])) {
+ return true;
+ }
+ }
+ return false;
+}
+
+module.exports = arrayIncludesWith;
diff --git a/node_modules/lodash/_arrayLikeKeys.js b/node_modules/lodash/_arrayLikeKeys.js
new file mode 100644
index 0000000..b2ec9ce
--- /dev/null
+++ b/node_modules/lodash/_arrayLikeKeys.js
@@ -0,0 +1,49 @@
+var baseTimes = require('./_baseTimes'),
+ isArguments = require('./isArguments'),
+ isArray = require('./isArray'),
+ isBuffer = require('./isBuffer'),
+ isIndex = require('./_isIndex'),
+ isTypedArray = require('./isTypedArray');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an array of the enumerable property names of the array-like `value`.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @param {boolean} inherited Specify returning inherited property names.
+ * @returns {Array} Returns the array of property names.
+ */
+function arrayLikeKeys(value, inherited) {
+ var isArr = isArray(value),
+ isArg = !isArr && isArguments(value),
+ isBuff = !isArr && !isArg && isBuffer(value),
+ isType = !isArr && !isArg && !isBuff && isTypedArray(value),
+ skipIndexes = isArr || isArg || isBuff || isType,
+ result = skipIndexes ? baseTimes(value.length, String) : [],
+ length = result.length;
+
+ for (var key in value) {
+ if ((inherited || hasOwnProperty.call(value, key)) &&
+ !(skipIndexes && (
+ // Safari 9 has enumerable `arguments.length` in strict mode.
+ key == 'length' ||
+ // Node.js 0.10 has enumerable non-index properties on buffers.
+ (isBuff && (key == 'offset' || key == 'parent')) ||
+ // PhantomJS 2 has enumerable non-index properties on typed arrays.
+ (isType && (key == 'buffer' || key == 'byteLength' || key == 'byteOffset')) ||
+ // Skip index properties.
+ isIndex(key, length)
+ ))) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+module.exports = arrayLikeKeys;
diff --git a/node_modules/lodash/_arrayMap.js b/node_modules/lodash/_arrayMap.js
new file mode 100644
index 0000000..22b2246
--- /dev/null
+++ b/node_modules/lodash/_arrayMap.js
@@ -0,0 +1,21 @@
+/**
+ * A specialized version of `_.map` for arrays without support for iteratee
+ * shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ */
+function arrayMap(array, iteratee) {
+ var index = -1,
+ length = array == null ? 0 : array.length,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = iteratee(array[index], index, array);
+ }
+ return result;
+}
+
+module.exports = arrayMap;
diff --git a/node_modules/lodash/_arrayPush.js b/node_modules/lodash/_arrayPush.js
new file mode 100644
index 0000000..7d742b3
--- /dev/null
+++ b/node_modules/lodash/_arrayPush.js
@@ -0,0 +1,20 @@
+/**
+ * Appends the elements of `values` to `array`.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to append.
+ * @returns {Array} Returns `array`.
+ */
+function arrayPush(array, values) {
+ var index = -1,
+ length = values.length,
+ offset = array.length;
+
+ while (++index < length) {
+ array[offset + index] = values[index];
+ }
+ return array;
+}
+
+module.exports = arrayPush;
diff --git a/node_modules/lodash/_arrayReduce.js b/node_modules/lodash/_arrayReduce.js
new file mode 100644
index 0000000..de8b79b
--- /dev/null
+++ b/node_modules/lodash/_arrayReduce.js
@@ -0,0 +1,26 @@
+/**
+ * A specialized version of `_.reduce` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {boolean} [initAccum] Specify using the first element of `array` as
+ * the initial value.
+ * @returns {*} Returns the accumulated value.
+ */
+function arrayReduce(array, iteratee, accumulator, initAccum) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ if (initAccum && length) {
+ accumulator = array[++index];
+ }
+ while (++index < length) {
+ accumulator = iteratee(accumulator, array[index], index, array);
+ }
+ return accumulator;
+}
+
+module.exports = arrayReduce;
diff --git a/node_modules/lodash/_arrayReduceRight.js b/node_modules/lodash/_arrayReduceRight.js
new file mode 100644
index 0000000..22d8976
--- /dev/null
+++ b/node_modules/lodash/_arrayReduceRight.js
@@ -0,0 +1,24 @@
+/**
+ * A specialized version of `_.reduceRight` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {boolean} [initAccum] Specify using the last element of `array` as
+ * the initial value.
+ * @returns {*} Returns the accumulated value.
+ */
+function arrayReduceRight(array, iteratee, accumulator, initAccum) {
+ var length = array == null ? 0 : array.length;
+ if (initAccum && length) {
+ accumulator = array[--length];
+ }
+ while (length--) {
+ accumulator = iteratee(accumulator, array[length], length, array);
+ }
+ return accumulator;
+}
+
+module.exports = arrayReduceRight;
diff --git a/node_modules/lodash/_arraySample.js b/node_modules/lodash/_arraySample.js
new file mode 100644
index 0000000..fcab010
--- /dev/null
+++ b/node_modules/lodash/_arraySample.js
@@ -0,0 +1,15 @@
+var baseRandom = require('./_baseRandom');
+
+/**
+ * A specialized version of `_.sample` for arrays.
+ *
+ * @private
+ * @param {Array} array The array to sample.
+ * @returns {*} Returns the random element.
+ */
+function arraySample(array) {
+ var length = array.length;
+ return length ? array[baseRandom(0, length - 1)] : undefined;
+}
+
+module.exports = arraySample;
diff --git a/node_modules/lodash/_arraySampleSize.js b/node_modules/lodash/_arraySampleSize.js
new file mode 100644
index 0000000..8c7e364
--- /dev/null
+++ b/node_modules/lodash/_arraySampleSize.js
@@ -0,0 +1,17 @@
+var baseClamp = require('./_baseClamp'),
+ copyArray = require('./_copyArray'),
+ shuffleSelf = require('./_shuffleSelf');
+
+/**
+ * A specialized version of `_.sampleSize` for arrays.
+ *
+ * @private
+ * @param {Array} array The array to sample.
+ * @param {number} n The number of elements to sample.
+ * @returns {Array} Returns the random elements.
+ */
+function arraySampleSize(array, n) {
+ return shuffleSelf(copyArray(array), baseClamp(n, 0, array.length));
+}
+
+module.exports = arraySampleSize;
diff --git a/node_modules/lodash/_arrayShuffle.js b/node_modules/lodash/_arrayShuffle.js
new file mode 100644
index 0000000..46313a3
--- /dev/null
+++ b/node_modules/lodash/_arrayShuffle.js
@@ -0,0 +1,15 @@
+var copyArray = require('./_copyArray'),
+ shuffleSelf = require('./_shuffleSelf');
+
+/**
+ * A specialized version of `_.shuffle` for arrays.
+ *
+ * @private
+ * @param {Array} array The array to shuffle.
+ * @returns {Array} Returns the new shuffled array.
+ */
+function arrayShuffle(array) {
+ return shuffleSelf(copyArray(array));
+}
+
+module.exports = arrayShuffle;
diff --git a/node_modules/lodash/_arraySome.js b/node_modules/lodash/_arraySome.js
new file mode 100644
index 0000000..6fd02fd
--- /dev/null
+++ b/node_modules/lodash/_arraySome.js
@@ -0,0 +1,23 @@
+/**
+ * A specialized version of `_.some` for arrays without support for iteratee
+ * shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ */
+function arraySome(array, predicate) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ while (++index < length) {
+ if (predicate(array[index], index, array)) {
+ return true;
+ }
+ }
+ return false;
+}
+
+module.exports = arraySome;
diff --git a/node_modules/lodash/_asciiSize.js b/node_modules/lodash/_asciiSize.js
new file mode 100644
index 0000000..11d29c3
--- /dev/null
+++ b/node_modules/lodash/_asciiSize.js
@@ -0,0 +1,12 @@
+var baseProperty = require('./_baseProperty');
+
+/**
+ * Gets the size of an ASCII `string`.
+ *
+ * @private
+ * @param {string} string The string inspect.
+ * @returns {number} Returns the string size.
+ */
+var asciiSize = baseProperty('length');
+
+module.exports = asciiSize;
diff --git a/node_modules/lodash/_asciiToArray.js b/node_modules/lodash/_asciiToArray.js
new file mode 100644
index 0000000..8e3dd5b
--- /dev/null
+++ b/node_modules/lodash/_asciiToArray.js
@@ -0,0 +1,12 @@
+/**
+ * Converts an ASCII `string` to an array.
+ *
+ * @private
+ * @param {string} string The string to convert.
+ * @returns {Array} Returns the converted array.
+ */
+function asciiToArray(string) {
+ return string.split('');
+}
+
+module.exports = asciiToArray;
diff --git a/node_modules/lodash/_asciiWords.js b/node_modules/lodash/_asciiWords.js
new file mode 100644
index 0000000..d765f0f
--- /dev/null
+++ b/node_modules/lodash/_asciiWords.js
@@ -0,0 +1,15 @@
+/** Used to match words composed of alphanumeric characters. */
+var reAsciiWord = /[^\x00-\x2f\x3a-\x40\x5b-\x60\x7b-\x7f]+/g;
+
+/**
+ * Splits an ASCII `string` into an array of its words.
+ *
+ * @private
+ * @param {string} The string to inspect.
+ * @returns {Array} Returns the words of `string`.
+ */
+function asciiWords(string) {
+ return string.match(reAsciiWord) || [];
+}
+
+module.exports = asciiWords;
diff --git a/node_modules/lodash/_assignMergeValue.js b/node_modules/lodash/_assignMergeValue.js
new file mode 100644
index 0000000..cb1185e
--- /dev/null
+++ b/node_modules/lodash/_assignMergeValue.js
@@ -0,0 +1,20 @@
+var baseAssignValue = require('./_baseAssignValue'),
+ eq = require('./eq');
+
+/**
+ * This function is like `assignValue` except that it doesn't assign
+ * `undefined` values.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+function assignMergeValue(object, key, value) {
+ if ((value !== undefined && !eq(object[key], value)) ||
+ (value === undefined && !(key in object))) {
+ baseAssignValue(object, key, value);
+ }
+}
+
+module.exports = assignMergeValue;
diff --git a/node_modules/lodash/_assignValue.js b/node_modules/lodash/_assignValue.js
new file mode 100644
index 0000000..4083957
--- /dev/null
+++ b/node_modules/lodash/_assignValue.js
@@ -0,0 +1,28 @@
+var baseAssignValue = require('./_baseAssignValue'),
+ eq = require('./eq');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Assigns `value` to `key` of `object` if the existing value is not equivalent
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+function assignValue(object, key, value) {
+ var objValue = object[key];
+ if (!(hasOwnProperty.call(object, key) && eq(objValue, value)) ||
+ (value === undefined && !(key in object))) {
+ baseAssignValue(object, key, value);
+ }
+}
+
+module.exports = assignValue;
diff --git a/node_modules/lodash/_assocIndexOf.js b/node_modules/lodash/_assocIndexOf.js
new file mode 100644
index 0000000..5b77a2b
--- /dev/null
+++ b/node_modules/lodash/_assocIndexOf.js
@@ -0,0 +1,21 @@
+var eq = require('./eq');
+
+/**
+ * Gets the index at which the `key` is found in `array` of key-value pairs.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} key The key to search for.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+function assocIndexOf(array, key) {
+ var length = array.length;
+ while (length--) {
+ if (eq(array[length][0], key)) {
+ return length;
+ }
+ }
+ return -1;
+}
+
+module.exports = assocIndexOf;
diff --git a/node_modules/lodash/_baseAggregator.js b/node_modules/lodash/_baseAggregator.js
new file mode 100644
index 0000000..4bc9e91
--- /dev/null
+++ b/node_modules/lodash/_baseAggregator.js
@@ -0,0 +1,21 @@
+var baseEach = require('./_baseEach');
+
+/**
+ * Aggregates elements of `collection` on `accumulator` with keys transformed
+ * by `iteratee` and values set by `setter`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} setter The function to set `accumulator` values.
+ * @param {Function} iteratee The iteratee to transform keys.
+ * @param {Object} accumulator The initial aggregated object.
+ * @returns {Function} Returns `accumulator`.
+ */
+function baseAggregator(collection, setter, iteratee, accumulator) {
+ baseEach(collection, function(value, key, collection) {
+ setter(accumulator, value, iteratee(value), collection);
+ });
+ return accumulator;
+}
+
+module.exports = baseAggregator;
diff --git a/node_modules/lodash/_baseAssign.js b/node_modules/lodash/_baseAssign.js
new file mode 100644
index 0000000..e5c4a1a
--- /dev/null
+++ b/node_modules/lodash/_baseAssign.js
@@ -0,0 +1,17 @@
+var copyObject = require('./_copyObject'),
+ keys = require('./keys');
+
+/**
+ * The base implementation of `_.assign` without support for multiple sources
+ * or `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @returns {Object} Returns `object`.
+ */
+function baseAssign(object, source) {
+ return object && copyObject(source, keys(source), object);
+}
+
+module.exports = baseAssign;
diff --git a/node_modules/lodash/_baseAssignIn.js b/node_modules/lodash/_baseAssignIn.js
new file mode 100644
index 0000000..6624f90
--- /dev/null
+++ b/node_modules/lodash/_baseAssignIn.js
@@ -0,0 +1,17 @@
+var copyObject = require('./_copyObject'),
+ keysIn = require('./keysIn');
+
+/**
+ * The base implementation of `_.assignIn` without support for multiple sources
+ * or `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @returns {Object} Returns `object`.
+ */
+function baseAssignIn(object, source) {
+ return object && copyObject(source, keysIn(source), object);
+}
+
+module.exports = baseAssignIn;
diff --git a/node_modules/lodash/_baseAssignValue.js b/node_modules/lodash/_baseAssignValue.js
new file mode 100644
index 0000000..d6f66ef
--- /dev/null
+++ b/node_modules/lodash/_baseAssignValue.js
@@ -0,0 +1,25 @@
+var defineProperty = require('./_defineProperty');
+
+/**
+ * The base implementation of `assignValue` and `assignMergeValue` without
+ * value checks.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+function baseAssignValue(object, key, value) {
+ if (key == '__proto__' && defineProperty) {
+ defineProperty(object, key, {
+ 'configurable': true,
+ 'enumerable': true,
+ 'value': value,
+ 'writable': true
+ });
+ } else {
+ object[key] = value;
+ }
+}
+
+module.exports = baseAssignValue;
diff --git a/node_modules/lodash/_baseAt.js b/node_modules/lodash/_baseAt.js
new file mode 100644
index 0000000..90e4237
--- /dev/null
+++ b/node_modules/lodash/_baseAt.js
@@ -0,0 +1,23 @@
+var get = require('./get');
+
+/**
+ * The base implementation of `_.at` without support for individual paths.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {string[]} paths The property paths to pick.
+ * @returns {Array} Returns the picked elements.
+ */
+function baseAt(object, paths) {
+ var index = -1,
+ length = paths.length,
+ result = Array(length),
+ skip = object == null;
+
+ while (++index < length) {
+ result[index] = skip ? undefined : get(object, paths[index]);
+ }
+ return result;
+}
+
+module.exports = baseAt;
diff --git a/node_modules/lodash/_baseClamp.js b/node_modules/lodash/_baseClamp.js
new file mode 100644
index 0000000..a1c5692
--- /dev/null
+++ b/node_modules/lodash/_baseClamp.js
@@ -0,0 +1,22 @@
+/**
+ * The base implementation of `_.clamp` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {number} number The number to clamp.
+ * @param {number} [lower] The lower bound.
+ * @param {number} upper The upper bound.
+ * @returns {number} Returns the clamped number.
+ */
+function baseClamp(number, lower, upper) {
+ if (number === number) {
+ if (upper !== undefined) {
+ number = number <= upper ? number : upper;
+ }
+ if (lower !== undefined) {
+ number = number >= lower ? number : lower;
+ }
+ }
+ return number;
+}
+
+module.exports = baseClamp;
diff --git a/node_modules/lodash/_baseClone.js b/node_modules/lodash/_baseClone.js
new file mode 100644
index 0000000..69f8705
--- /dev/null
+++ b/node_modules/lodash/_baseClone.js
@@ -0,0 +1,166 @@
+var Stack = require('./_Stack'),
+ arrayEach = require('./_arrayEach'),
+ assignValue = require('./_assignValue'),
+ baseAssign = require('./_baseAssign'),
+ baseAssignIn = require('./_baseAssignIn'),
+ cloneBuffer = require('./_cloneBuffer'),
+ copyArray = require('./_copyArray'),
+ copySymbols = require('./_copySymbols'),
+ copySymbolsIn = require('./_copySymbolsIn'),
+ getAllKeys = require('./_getAllKeys'),
+ getAllKeysIn = require('./_getAllKeysIn'),
+ getTag = require('./_getTag'),
+ initCloneArray = require('./_initCloneArray'),
+ initCloneByTag = require('./_initCloneByTag'),
+ initCloneObject = require('./_initCloneObject'),
+ isArray = require('./isArray'),
+ isBuffer = require('./isBuffer'),
+ isMap = require('./isMap'),
+ isObject = require('./isObject'),
+ isSet = require('./isSet'),
+ keys = require('./keys'),
+ keysIn = require('./keysIn');
+
+/** Used to compose bitmasks for cloning. */
+var CLONE_DEEP_FLAG = 1,
+ CLONE_FLAT_FLAG = 2,
+ CLONE_SYMBOLS_FLAG = 4;
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ funcTag = '[object Function]',
+ genTag = '[object GeneratorFunction]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ objectTag = '[object Object]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ symbolTag = '[object Symbol]',
+ weakMapTag = '[object WeakMap]';
+
+var arrayBufferTag = '[object ArrayBuffer]',
+ dataViewTag = '[object DataView]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+/** Used to identify `toStringTag` values supported by `_.clone`. */
+var cloneableTags = {};
+cloneableTags[argsTag] = cloneableTags[arrayTag] =
+cloneableTags[arrayBufferTag] = cloneableTags[dataViewTag] =
+cloneableTags[boolTag] = cloneableTags[dateTag] =
+cloneableTags[float32Tag] = cloneableTags[float64Tag] =
+cloneableTags[int8Tag] = cloneableTags[int16Tag] =
+cloneableTags[int32Tag] = cloneableTags[mapTag] =
+cloneableTags[numberTag] = cloneableTags[objectTag] =
+cloneableTags[regexpTag] = cloneableTags[setTag] =
+cloneableTags[stringTag] = cloneableTags[symbolTag] =
+cloneableTags[uint8Tag] = cloneableTags[uint8ClampedTag] =
+cloneableTags[uint16Tag] = cloneableTags[uint32Tag] = true;
+cloneableTags[errorTag] = cloneableTags[funcTag] =
+cloneableTags[weakMapTag] = false;
+
+/**
+ * The base implementation of `_.clone` and `_.cloneDeep` which tracks
+ * traversed objects.
+ *
+ * @private
+ * @param {*} value The value to clone.
+ * @param {boolean} bitmask The bitmask flags.
+ * 1 - Deep clone
+ * 2 - Flatten inherited properties
+ * 4 - Clone symbols
+ * @param {Function} [customizer] The function to customize cloning.
+ * @param {string} [key] The key of `value`.
+ * @param {Object} [object] The parent object of `value`.
+ * @param {Object} [stack] Tracks traversed objects and their clone counterparts.
+ * @returns {*} Returns the cloned value.
+ */
+function baseClone(value, bitmask, customizer, key, object, stack) {
+ var result,
+ isDeep = bitmask & CLONE_DEEP_FLAG,
+ isFlat = bitmask & CLONE_FLAT_FLAG,
+ isFull = bitmask & CLONE_SYMBOLS_FLAG;
+
+ if (customizer) {
+ result = object ? customizer(value, key, object, stack) : customizer(value);
+ }
+ if (result !== undefined) {
+ return result;
+ }
+ if (!isObject(value)) {
+ return value;
+ }
+ var isArr = isArray(value);
+ if (isArr) {
+ result = initCloneArray(value);
+ if (!isDeep) {
+ return copyArray(value, result);
+ }
+ } else {
+ var tag = getTag(value),
+ isFunc = tag == funcTag || tag == genTag;
+
+ if (isBuffer(value)) {
+ return cloneBuffer(value, isDeep);
+ }
+ if (tag == objectTag || tag == argsTag || (isFunc && !object)) {
+ result = (isFlat || isFunc) ? {} : initCloneObject(value);
+ if (!isDeep) {
+ return isFlat
+ ? copySymbolsIn(value, baseAssignIn(result, value))
+ : copySymbols(value, baseAssign(result, value));
+ }
+ } else {
+ if (!cloneableTags[tag]) {
+ return object ? value : {};
+ }
+ result = initCloneByTag(value, tag, isDeep);
+ }
+ }
+ // Check for circular references and return its corresponding clone.
+ stack || (stack = new Stack);
+ var stacked = stack.get(value);
+ if (stacked) {
+ return stacked;
+ }
+ stack.set(value, result);
+
+ if (isSet(value)) {
+ value.forEach(function(subValue) {
+ result.add(baseClone(subValue, bitmask, customizer, subValue, value, stack));
+ });
+ } else if (isMap(value)) {
+ value.forEach(function(subValue, key) {
+ result.set(key, baseClone(subValue, bitmask, customizer, key, value, stack));
+ });
+ }
+
+ var keysFunc = isFull
+ ? (isFlat ? getAllKeysIn : getAllKeys)
+ : (isFlat ? keysIn : keys);
+
+ var props = isArr ? undefined : keysFunc(value);
+ arrayEach(props || value, function(subValue, key) {
+ if (props) {
+ key = subValue;
+ subValue = value[key];
+ }
+ // Recursively populate clone (susceptible to call stack limits).
+ assignValue(result, key, baseClone(subValue, bitmask, customizer, key, value, stack));
+ });
+ return result;
+}
+
+module.exports = baseClone;
diff --git a/node_modules/lodash/_baseConforms.js b/node_modules/lodash/_baseConforms.js
new file mode 100644
index 0000000..947e20d
--- /dev/null
+++ b/node_modules/lodash/_baseConforms.js
@@ -0,0 +1,18 @@
+var baseConformsTo = require('./_baseConformsTo'),
+ keys = require('./keys');
+
+/**
+ * The base implementation of `_.conforms` which doesn't clone `source`.
+ *
+ * @private
+ * @param {Object} source The object of property predicates to conform to.
+ * @returns {Function} Returns the new spec function.
+ */
+function baseConforms(source) {
+ var props = keys(source);
+ return function(object) {
+ return baseConformsTo(object, source, props);
+ };
+}
+
+module.exports = baseConforms;
diff --git a/node_modules/lodash/_baseConformsTo.js b/node_modules/lodash/_baseConformsTo.js
new file mode 100644
index 0000000..e449cb8
--- /dev/null
+++ b/node_modules/lodash/_baseConformsTo.js
@@ -0,0 +1,27 @@
+/**
+ * The base implementation of `_.conformsTo` which accepts `props` to check.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property predicates to conform to.
+ * @returns {boolean} Returns `true` if `object` conforms, else `false`.
+ */
+function baseConformsTo(object, source, props) {
+ var length = props.length;
+ if (object == null) {
+ return !length;
+ }
+ object = Object(object);
+ while (length--) {
+ var key = props[length],
+ predicate = source[key],
+ value = object[key];
+
+ if ((value === undefined && !(key in object)) || !predicate(value)) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = baseConformsTo;
diff --git a/node_modules/lodash/_baseCreate.js b/node_modules/lodash/_baseCreate.js
new file mode 100644
index 0000000..ffa6a52
--- /dev/null
+++ b/node_modules/lodash/_baseCreate.js
@@ -0,0 +1,30 @@
+var isObject = require('./isObject');
+
+/** Built-in value references. */
+var objectCreate = Object.create;
+
+/**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} proto The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+var baseCreate = (function() {
+ function object() {}
+ return function(proto) {
+ if (!isObject(proto)) {
+ return {};
+ }
+ if (objectCreate) {
+ return objectCreate(proto);
+ }
+ object.prototype = proto;
+ var result = new object;
+ object.prototype = undefined;
+ return result;
+ };
+}());
+
+module.exports = baseCreate;
diff --git a/node_modules/lodash/_baseDelay.js b/node_modules/lodash/_baseDelay.js
new file mode 100644
index 0000000..1486d69
--- /dev/null
+++ b/node_modules/lodash/_baseDelay.js
@@ -0,0 +1,21 @@
+/** Error message constants. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * The base implementation of `_.delay` and `_.defer` which accepts `args`
+ * to provide to `func`.
+ *
+ * @private
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay invocation.
+ * @param {Array} args The arguments to provide to `func`.
+ * @returns {number|Object} Returns the timer id or timeout object.
+ */
+function baseDelay(func, wait, args) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ return setTimeout(function() { func.apply(undefined, args); }, wait);
+}
+
+module.exports = baseDelay;
diff --git a/node_modules/lodash/_baseDifference.js b/node_modules/lodash/_baseDifference.js
new file mode 100644
index 0000000..343ac19
--- /dev/null
+++ b/node_modules/lodash/_baseDifference.js
@@ -0,0 +1,67 @@
+var SetCache = require('./_SetCache'),
+ arrayIncludes = require('./_arrayIncludes'),
+ arrayIncludesWith = require('./_arrayIncludesWith'),
+ arrayMap = require('./_arrayMap'),
+ baseUnary = require('./_baseUnary'),
+ cacheHas = require('./_cacheHas');
+
+/** Used as the size to enable large array optimizations. */
+var LARGE_ARRAY_SIZE = 200;
+
+/**
+ * The base implementation of methods like `_.difference` without support
+ * for excluding multiple arrays or iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Array} values The values to exclude.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of filtered values.
+ */
+function baseDifference(array, values, iteratee, comparator) {
+ var index = -1,
+ includes = arrayIncludes,
+ isCommon = true,
+ length = array.length,
+ result = [],
+ valuesLength = values.length;
+
+ if (!length) {
+ return result;
+ }
+ if (iteratee) {
+ values = arrayMap(values, baseUnary(iteratee));
+ }
+ if (comparator) {
+ includes = arrayIncludesWith;
+ isCommon = false;
+ }
+ else if (values.length >= LARGE_ARRAY_SIZE) {
+ includes = cacheHas;
+ isCommon = false;
+ values = new SetCache(values);
+ }
+ outer:
+ while (++index < length) {
+ var value = array[index],
+ computed = iteratee == null ? value : iteratee(value);
+
+ value = (comparator || value !== 0) ? value : 0;
+ if (isCommon && computed === computed) {
+ var valuesIndex = valuesLength;
+ while (valuesIndex--) {
+ if (values[valuesIndex] === computed) {
+ continue outer;
+ }
+ }
+ result.push(value);
+ }
+ else if (!includes(values, computed, comparator)) {
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = baseDifference;
diff --git a/node_modules/lodash/_baseEach.js b/node_modules/lodash/_baseEach.js
new file mode 100644
index 0000000..512c067
--- /dev/null
+++ b/node_modules/lodash/_baseEach.js
@@ -0,0 +1,14 @@
+var baseForOwn = require('./_baseForOwn'),
+ createBaseEach = require('./_createBaseEach');
+
+/**
+ * The base implementation of `_.forEach` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object} Returns `collection`.
+ */
+var baseEach = createBaseEach(baseForOwn);
+
+module.exports = baseEach;
diff --git a/node_modules/lodash/_baseEachRight.js b/node_modules/lodash/_baseEachRight.js
new file mode 100644
index 0000000..0a8feec
--- /dev/null
+++ b/node_modules/lodash/_baseEachRight.js
@@ -0,0 +1,14 @@
+var baseForOwnRight = require('./_baseForOwnRight'),
+ createBaseEach = require('./_createBaseEach');
+
+/**
+ * The base implementation of `_.forEachRight` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object} Returns `collection`.
+ */
+var baseEachRight = createBaseEach(baseForOwnRight, true);
+
+module.exports = baseEachRight;
diff --git a/node_modules/lodash/_baseEvery.js b/node_modules/lodash/_baseEvery.js
new file mode 100644
index 0000000..fa52f7b
--- /dev/null
+++ b/node_modules/lodash/_baseEvery.js
@@ -0,0 +1,21 @@
+var baseEach = require('./_baseEach');
+
+/**
+ * The base implementation of `_.every` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`
+ */
+function baseEvery(collection, predicate) {
+ var result = true;
+ baseEach(collection, function(value, index, collection) {
+ result = !!predicate(value, index, collection);
+ return result;
+ });
+ return result;
+}
+
+module.exports = baseEvery;
diff --git a/node_modules/lodash/_baseExtremum.js b/node_modules/lodash/_baseExtremum.js
new file mode 100644
index 0000000..9d6aa77
--- /dev/null
+++ b/node_modules/lodash/_baseExtremum.js
@@ -0,0 +1,32 @@
+var isSymbol = require('./isSymbol');
+
+/**
+ * The base implementation of methods like `_.max` and `_.min` which accepts a
+ * `comparator` to determine the extremum value.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The iteratee invoked per iteration.
+ * @param {Function} comparator The comparator used to compare values.
+ * @returns {*} Returns the extremum value.
+ */
+function baseExtremum(array, iteratee, comparator) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ var value = array[index],
+ current = iteratee(value);
+
+ if (current != null && (computed === undefined
+ ? (current === current && !isSymbol(current))
+ : comparator(current, computed)
+ )) {
+ var computed = current,
+ result = value;
+ }
+ }
+ return result;
+}
+
+module.exports = baseExtremum;
diff --git a/node_modules/lodash/_baseFill.js b/node_modules/lodash/_baseFill.js
new file mode 100644
index 0000000..46ef9c7
--- /dev/null
+++ b/node_modules/lodash/_baseFill.js
@@ -0,0 +1,32 @@
+var toInteger = require('./toInteger'),
+ toLength = require('./toLength');
+
+/**
+ * The base implementation of `_.fill` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to fill.
+ * @param {*} value The value to fill `array` with.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns `array`.
+ */
+function baseFill(array, value, start, end) {
+ var length = array.length;
+
+ start = toInteger(start);
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = (end === undefined || end > length) ? length : toInteger(end);
+ if (end < 0) {
+ end += length;
+ }
+ end = start > end ? 0 : toLength(end);
+ while (start < end) {
+ array[start++] = value;
+ }
+ return array;
+}
+
+module.exports = baseFill;
diff --git a/node_modules/lodash/_baseFilter.js b/node_modules/lodash/_baseFilter.js
new file mode 100644
index 0000000..4678477
--- /dev/null
+++ b/node_modules/lodash/_baseFilter.js
@@ -0,0 +1,21 @@
+var baseEach = require('./_baseEach');
+
+/**
+ * The base implementation of `_.filter` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ */
+function baseFilter(collection, predicate) {
+ var result = [];
+ baseEach(collection, function(value, index, collection) {
+ if (predicate(value, index, collection)) {
+ result.push(value);
+ }
+ });
+ return result;
+}
+
+module.exports = baseFilter;
diff --git a/node_modules/lodash/_baseFindIndex.js b/node_modules/lodash/_baseFindIndex.js
new file mode 100644
index 0000000..e3f5d8a
--- /dev/null
+++ b/node_modules/lodash/_baseFindIndex.js
@@ -0,0 +1,24 @@
+/**
+ * The base implementation of `_.findIndex` and `_.findLastIndex` without
+ * support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {number} fromIndex The index to search from.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+function baseFindIndex(array, predicate, fromIndex, fromRight) {
+ var length = array.length,
+ index = fromIndex + (fromRight ? 1 : -1);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ if (predicate(array[index], index, array)) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = baseFindIndex;
diff --git a/node_modules/lodash/_baseFindKey.js b/node_modules/lodash/_baseFindKey.js
new file mode 100644
index 0000000..2e430f3
--- /dev/null
+++ b/node_modules/lodash/_baseFindKey.js
@@ -0,0 +1,23 @@
+/**
+ * The base implementation of methods like `_.findKey` and `_.findLastKey`,
+ * without support for iteratee shorthands, which iterates over `collection`
+ * using `eachFunc`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to inspect.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {Function} eachFunc The function to iterate over `collection`.
+ * @returns {*} Returns the found element or its key, else `undefined`.
+ */
+function baseFindKey(collection, predicate, eachFunc) {
+ var result;
+ eachFunc(collection, function(value, key, collection) {
+ if (predicate(value, key, collection)) {
+ result = key;
+ return false;
+ }
+ });
+ return result;
+}
+
+module.exports = baseFindKey;
diff --git a/node_modules/lodash/_baseFlatten.js b/node_modules/lodash/_baseFlatten.js
new file mode 100644
index 0000000..4b1e009
--- /dev/null
+++ b/node_modules/lodash/_baseFlatten.js
@@ -0,0 +1,38 @@
+var arrayPush = require('./_arrayPush'),
+ isFlattenable = require('./_isFlattenable');
+
+/**
+ * The base implementation of `_.flatten` with support for restricting flattening.
+ *
+ * @private
+ * @param {Array} array The array to flatten.
+ * @param {number} depth The maximum recursion depth.
+ * @param {boolean} [predicate=isFlattenable] The function invoked per iteration.
+ * @param {boolean} [isStrict] Restrict to values that pass `predicate` checks.
+ * @param {Array} [result=[]] The initial result value.
+ * @returns {Array} Returns the new flattened array.
+ */
+function baseFlatten(array, depth, predicate, isStrict, result) {
+ var index = -1,
+ length = array.length;
+
+ predicate || (predicate = isFlattenable);
+ result || (result = []);
+
+ while (++index < length) {
+ var value = array[index];
+ if (depth > 0 && predicate(value)) {
+ if (depth > 1) {
+ // Recursively flatten arrays (susceptible to call stack limits).
+ baseFlatten(value, depth - 1, predicate, isStrict, result);
+ } else {
+ arrayPush(result, value);
+ }
+ } else if (!isStrict) {
+ result[result.length] = value;
+ }
+ }
+ return result;
+}
+
+module.exports = baseFlatten;
diff --git a/node_modules/lodash/_baseFor.js b/node_modules/lodash/_baseFor.js
new file mode 100644
index 0000000..d946590
--- /dev/null
+++ b/node_modules/lodash/_baseFor.js
@@ -0,0 +1,16 @@
+var createBaseFor = require('./_createBaseFor');
+
+/**
+ * The base implementation of `baseForOwn` which iterates over `object`
+ * properties returned by `keysFunc` and invokes `iteratee` for each property.
+ * Iteratee functions may exit iteration early by explicitly returning `false`.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+var baseFor = createBaseFor();
+
+module.exports = baseFor;
diff --git a/node_modules/lodash/_baseForOwn.js b/node_modules/lodash/_baseForOwn.js
new file mode 100644
index 0000000..503d523
--- /dev/null
+++ b/node_modules/lodash/_baseForOwn.js
@@ -0,0 +1,16 @@
+var baseFor = require('./_baseFor'),
+ keys = require('./keys');
+
+/**
+ * The base implementation of `_.forOwn` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+function baseForOwn(object, iteratee) {
+ return object && baseFor(object, iteratee, keys);
+}
+
+module.exports = baseForOwn;
diff --git a/node_modules/lodash/_baseForOwnRight.js b/node_modules/lodash/_baseForOwnRight.js
new file mode 100644
index 0000000..a4b10e6
--- /dev/null
+++ b/node_modules/lodash/_baseForOwnRight.js
@@ -0,0 +1,16 @@
+var baseForRight = require('./_baseForRight'),
+ keys = require('./keys');
+
+/**
+ * The base implementation of `_.forOwnRight` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+function baseForOwnRight(object, iteratee) {
+ return object && baseForRight(object, iteratee, keys);
+}
+
+module.exports = baseForOwnRight;
diff --git a/node_modules/lodash/_baseForRight.js b/node_modules/lodash/_baseForRight.js
new file mode 100644
index 0000000..32842cd
--- /dev/null
+++ b/node_modules/lodash/_baseForRight.js
@@ -0,0 +1,15 @@
+var createBaseFor = require('./_createBaseFor');
+
+/**
+ * This function is like `baseFor` except that it iterates over properties
+ * in the opposite order.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+var baseForRight = createBaseFor(true);
+
+module.exports = baseForRight;
diff --git a/node_modules/lodash/_baseFunctions.js b/node_modules/lodash/_baseFunctions.js
new file mode 100644
index 0000000..d23bc9b
--- /dev/null
+++ b/node_modules/lodash/_baseFunctions.js
@@ -0,0 +1,19 @@
+var arrayFilter = require('./_arrayFilter'),
+ isFunction = require('./isFunction');
+
+/**
+ * The base implementation of `_.functions` which creates an array of
+ * `object` function property names filtered from `props`.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Array} props The property names to filter.
+ * @returns {Array} Returns the function names.
+ */
+function baseFunctions(object, props) {
+ return arrayFilter(props, function(key) {
+ return isFunction(object[key]);
+ });
+}
+
+module.exports = baseFunctions;
diff --git a/node_modules/lodash/_baseGet.js b/node_modules/lodash/_baseGet.js
new file mode 100644
index 0000000..a194913
--- /dev/null
+++ b/node_modules/lodash/_baseGet.js
@@ -0,0 +1,24 @@
+var castPath = require('./_castPath'),
+ toKey = require('./_toKey');
+
+/**
+ * The base implementation of `_.get` without support for default values.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the property to get.
+ * @returns {*} Returns the resolved value.
+ */
+function baseGet(object, path) {
+ path = castPath(path, object);
+
+ var index = 0,
+ length = path.length;
+
+ while (object != null && index < length) {
+ object = object[toKey(path[index++])];
+ }
+ return (index && index == length) ? object : undefined;
+}
+
+module.exports = baseGet;
diff --git a/node_modules/lodash/_baseGetAllKeys.js b/node_modules/lodash/_baseGetAllKeys.js
new file mode 100644
index 0000000..8ad204e
--- /dev/null
+++ b/node_modules/lodash/_baseGetAllKeys.js
@@ -0,0 +1,20 @@
+var arrayPush = require('./_arrayPush'),
+ isArray = require('./isArray');
+
+/**
+ * The base implementation of `getAllKeys` and `getAllKeysIn` which uses
+ * `keysFunc` and `symbolsFunc` to get the enumerable property names and
+ * symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @param {Function} symbolsFunc The function to get the symbols of `object`.
+ * @returns {Array} Returns the array of property names and symbols.
+ */
+function baseGetAllKeys(object, keysFunc, symbolsFunc) {
+ var result = keysFunc(object);
+ return isArray(object) ? result : arrayPush(result, symbolsFunc(object));
+}
+
+module.exports = baseGetAllKeys;
diff --git a/node_modules/lodash/_baseGetTag.js b/node_modules/lodash/_baseGetTag.js
new file mode 100644
index 0000000..b927ccc
--- /dev/null
+++ b/node_modules/lodash/_baseGetTag.js
@@ -0,0 +1,28 @@
+var Symbol = require('./_Symbol'),
+ getRawTag = require('./_getRawTag'),
+ objectToString = require('./_objectToString');
+
+/** `Object#toString` result references. */
+var nullTag = '[object Null]',
+ undefinedTag = '[object Undefined]';
+
+/** Built-in value references. */
+var symToStringTag = Symbol ? Symbol.toStringTag : undefined;
+
+/**
+ * The base implementation of `getTag` without fallbacks for buggy environments.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the `toStringTag`.
+ */
+function baseGetTag(value) {
+ if (value == null) {
+ return value === undefined ? undefinedTag : nullTag;
+ }
+ return (symToStringTag && symToStringTag in Object(value))
+ ? getRawTag(value)
+ : objectToString(value);
+}
+
+module.exports = baseGetTag;
diff --git a/node_modules/lodash/_baseGt.js b/node_modules/lodash/_baseGt.js
new file mode 100644
index 0000000..502d273
--- /dev/null
+++ b/node_modules/lodash/_baseGt.js
@@ -0,0 +1,14 @@
+/**
+ * The base implementation of `_.gt` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is greater than `other`,
+ * else `false`.
+ */
+function baseGt(value, other) {
+ return value > other;
+}
+
+module.exports = baseGt;
diff --git a/node_modules/lodash/_baseHas.js b/node_modules/lodash/_baseHas.js
new file mode 100644
index 0000000..1b73032
--- /dev/null
+++ b/node_modules/lodash/_baseHas.js
@@ -0,0 +1,19 @@
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * The base implementation of `_.has` without support for deep paths.
+ *
+ * @private
+ * @param {Object} [object] The object to query.
+ * @param {Array|string} key The key to check.
+ * @returns {boolean} Returns `true` if `key` exists, else `false`.
+ */
+function baseHas(object, key) {
+ return object != null && hasOwnProperty.call(object, key);
+}
+
+module.exports = baseHas;
diff --git a/node_modules/lodash/_baseHasIn.js b/node_modules/lodash/_baseHasIn.js
new file mode 100644
index 0000000..2e0d042
--- /dev/null
+++ b/node_modules/lodash/_baseHasIn.js
@@ -0,0 +1,13 @@
+/**
+ * The base implementation of `_.hasIn` without support for deep paths.
+ *
+ * @private
+ * @param {Object} [object] The object to query.
+ * @param {Array|string} key The key to check.
+ * @returns {boolean} Returns `true` if `key` exists, else `false`.
+ */
+function baseHasIn(object, key) {
+ return object != null && key in Object(object);
+}
+
+module.exports = baseHasIn;
diff --git a/node_modules/lodash/_baseInRange.js b/node_modules/lodash/_baseInRange.js
new file mode 100644
index 0000000..ec95666
--- /dev/null
+++ b/node_modules/lodash/_baseInRange.js
@@ -0,0 +1,18 @@
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * The base implementation of `_.inRange` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {number} number The number to check.
+ * @param {number} start The start of the range.
+ * @param {number} end The end of the range.
+ * @returns {boolean} Returns `true` if `number` is in the range, else `false`.
+ */
+function baseInRange(number, start, end) {
+ return number >= nativeMin(start, end) && number < nativeMax(start, end);
+}
+
+module.exports = baseInRange;
diff --git a/node_modules/lodash/_baseIndexOf.js b/node_modules/lodash/_baseIndexOf.js
new file mode 100644
index 0000000..167e706
--- /dev/null
+++ b/node_modules/lodash/_baseIndexOf.js
@@ -0,0 +1,20 @@
+var baseFindIndex = require('./_baseFindIndex'),
+ baseIsNaN = require('./_baseIsNaN'),
+ strictIndexOf = require('./_strictIndexOf');
+
+/**
+ * The base implementation of `_.indexOf` without `fromIndex` bounds checks.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} fromIndex The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+function baseIndexOf(array, value, fromIndex) {
+ return value === value
+ ? strictIndexOf(array, value, fromIndex)
+ : baseFindIndex(array, baseIsNaN, fromIndex);
+}
+
+module.exports = baseIndexOf;
diff --git a/node_modules/lodash/_baseIndexOfWith.js b/node_modules/lodash/_baseIndexOfWith.js
new file mode 100644
index 0000000..f815fe0
--- /dev/null
+++ b/node_modules/lodash/_baseIndexOfWith.js
@@ -0,0 +1,23 @@
+/**
+ * This function is like `baseIndexOf` except that it accepts a comparator.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} fromIndex The index to search from.
+ * @param {Function} comparator The comparator invoked per element.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+function baseIndexOfWith(array, value, fromIndex, comparator) {
+ var index = fromIndex - 1,
+ length = array.length;
+
+ while (++index < length) {
+ if (comparator(array[index], value)) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = baseIndexOfWith;
diff --git a/node_modules/lodash/_baseIntersection.js b/node_modules/lodash/_baseIntersection.js
new file mode 100644
index 0000000..c1d250c
--- /dev/null
+++ b/node_modules/lodash/_baseIntersection.js
@@ -0,0 +1,74 @@
+var SetCache = require('./_SetCache'),
+ arrayIncludes = require('./_arrayIncludes'),
+ arrayIncludesWith = require('./_arrayIncludesWith'),
+ arrayMap = require('./_arrayMap'),
+ baseUnary = require('./_baseUnary'),
+ cacheHas = require('./_cacheHas');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * The base implementation of methods like `_.intersection`, without support
+ * for iteratee shorthands, that accepts an array of arrays to inspect.
+ *
+ * @private
+ * @param {Array} arrays The arrays to inspect.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of shared values.
+ */
+function baseIntersection(arrays, iteratee, comparator) {
+ var includes = comparator ? arrayIncludesWith : arrayIncludes,
+ length = arrays[0].length,
+ othLength = arrays.length,
+ othIndex = othLength,
+ caches = Array(othLength),
+ maxLength = Infinity,
+ result = [];
+
+ while (othIndex--) {
+ var array = arrays[othIndex];
+ if (othIndex && iteratee) {
+ array = arrayMap(array, baseUnary(iteratee));
+ }
+ maxLength = nativeMin(array.length, maxLength);
+ caches[othIndex] = !comparator && (iteratee || (length >= 120 && array.length >= 120))
+ ? new SetCache(othIndex && array)
+ : undefined;
+ }
+ array = arrays[0];
+
+ var index = -1,
+ seen = caches[0];
+
+ outer:
+ while (++index < length && result.length < maxLength) {
+ var value = array[index],
+ computed = iteratee ? iteratee(value) : value;
+
+ value = (comparator || value !== 0) ? value : 0;
+ if (!(seen
+ ? cacheHas(seen, computed)
+ : includes(result, computed, comparator)
+ )) {
+ othIndex = othLength;
+ while (--othIndex) {
+ var cache = caches[othIndex];
+ if (!(cache
+ ? cacheHas(cache, computed)
+ : includes(arrays[othIndex], computed, comparator))
+ ) {
+ continue outer;
+ }
+ }
+ if (seen) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = baseIntersection;
diff --git a/node_modules/lodash/_baseInverter.js b/node_modules/lodash/_baseInverter.js
new file mode 100644
index 0000000..fbc337f
--- /dev/null
+++ b/node_modules/lodash/_baseInverter.js
@@ -0,0 +1,21 @@
+var baseForOwn = require('./_baseForOwn');
+
+/**
+ * The base implementation of `_.invert` and `_.invertBy` which inverts
+ * `object` with values transformed by `iteratee` and set by `setter`.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} setter The function to set `accumulator` values.
+ * @param {Function} iteratee The iteratee to transform values.
+ * @param {Object} accumulator The initial inverted object.
+ * @returns {Function} Returns `accumulator`.
+ */
+function baseInverter(object, setter, iteratee, accumulator) {
+ baseForOwn(object, function(value, key, object) {
+ setter(accumulator, iteratee(value), key, object);
+ });
+ return accumulator;
+}
+
+module.exports = baseInverter;
diff --git a/node_modules/lodash/_baseInvoke.js b/node_modules/lodash/_baseInvoke.js
new file mode 100644
index 0000000..49bcf3c
--- /dev/null
+++ b/node_modules/lodash/_baseInvoke.js
@@ -0,0 +1,24 @@
+var apply = require('./_apply'),
+ castPath = require('./_castPath'),
+ last = require('./last'),
+ parent = require('./_parent'),
+ toKey = require('./_toKey');
+
+/**
+ * The base implementation of `_.invoke` without support for individual
+ * method arguments.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the method to invoke.
+ * @param {Array} args The arguments to invoke the method with.
+ * @returns {*} Returns the result of the invoked method.
+ */
+function baseInvoke(object, path, args) {
+ path = castPath(path, object);
+ object = parent(object, path);
+ var func = object == null ? object : object[toKey(last(path))];
+ return func == null ? undefined : apply(func, object, args);
+}
+
+module.exports = baseInvoke;
diff --git a/node_modules/lodash/_baseIsArguments.js b/node_modules/lodash/_baseIsArguments.js
new file mode 100644
index 0000000..b3562cc
--- /dev/null
+++ b/node_modules/lodash/_baseIsArguments.js
@@ -0,0 +1,18 @@
+var baseGetTag = require('./_baseGetTag'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]';
+
+/**
+ * The base implementation of `_.isArguments`.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ */
+function baseIsArguments(value) {
+ return isObjectLike(value) && baseGetTag(value) == argsTag;
+}
+
+module.exports = baseIsArguments;
diff --git a/node_modules/lodash/_baseIsArrayBuffer.js b/node_modules/lodash/_baseIsArrayBuffer.js
new file mode 100644
index 0000000..a2c4f30
--- /dev/null
+++ b/node_modules/lodash/_baseIsArrayBuffer.js
@@ -0,0 +1,17 @@
+var baseGetTag = require('./_baseGetTag'),
+ isObjectLike = require('./isObjectLike');
+
+var arrayBufferTag = '[object ArrayBuffer]';
+
+/**
+ * The base implementation of `_.isArrayBuffer` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array buffer, else `false`.
+ */
+function baseIsArrayBuffer(value) {
+ return isObjectLike(value) && baseGetTag(value) == arrayBufferTag;
+}
+
+module.exports = baseIsArrayBuffer;
diff --git a/node_modules/lodash/_baseIsDate.js b/node_modules/lodash/_baseIsDate.js
new file mode 100644
index 0000000..ba67c78
--- /dev/null
+++ b/node_modules/lodash/_baseIsDate.js
@@ -0,0 +1,18 @@
+var baseGetTag = require('./_baseGetTag'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var dateTag = '[object Date]';
+
+/**
+ * The base implementation of `_.isDate` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a date object, else `false`.
+ */
+function baseIsDate(value) {
+ return isObjectLike(value) && baseGetTag(value) == dateTag;
+}
+
+module.exports = baseIsDate;
diff --git a/node_modules/lodash/_baseIsEqual.js b/node_modules/lodash/_baseIsEqual.js
new file mode 100644
index 0000000..00a68a4
--- /dev/null
+++ b/node_modules/lodash/_baseIsEqual.js
@@ -0,0 +1,28 @@
+var baseIsEqualDeep = require('./_baseIsEqualDeep'),
+ isObjectLike = require('./isObjectLike');
+
+/**
+ * The base implementation of `_.isEqual` which supports partial comparisons
+ * and tracks traversed objects.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @param {boolean} bitmask The bitmask flags.
+ * 1 - Unordered comparison
+ * 2 - Partial comparison
+ * @param {Function} [customizer] The function to customize comparisons.
+ * @param {Object} [stack] Tracks traversed `value` and `other` objects.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ */
+function baseIsEqual(value, other, bitmask, customizer, stack) {
+ if (value === other) {
+ return true;
+ }
+ if (value == null || other == null || (!isObjectLike(value) && !isObjectLike(other))) {
+ return value !== value && other !== other;
+ }
+ return baseIsEqualDeep(value, other, bitmask, customizer, baseIsEqual, stack);
+}
+
+module.exports = baseIsEqual;
diff --git a/node_modules/lodash/_baseIsEqualDeep.js b/node_modules/lodash/_baseIsEqualDeep.js
new file mode 100644
index 0000000..e3cfd6a
--- /dev/null
+++ b/node_modules/lodash/_baseIsEqualDeep.js
@@ -0,0 +1,83 @@
+var Stack = require('./_Stack'),
+ equalArrays = require('./_equalArrays'),
+ equalByTag = require('./_equalByTag'),
+ equalObjects = require('./_equalObjects'),
+ getTag = require('./_getTag'),
+ isArray = require('./isArray'),
+ isBuffer = require('./isBuffer'),
+ isTypedArray = require('./isTypedArray');
+
+/** Used to compose bitmasks for value comparisons. */
+var COMPARE_PARTIAL_FLAG = 1;
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ objectTag = '[object Object]';
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * A specialized version of `baseIsEqual` for arrays and objects which performs
+ * deep comparisons and tracks traversed objects enabling objects with circular
+ * references to be compared.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} [stack] Tracks traversed `object` and `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function baseIsEqualDeep(object, other, bitmask, customizer, equalFunc, stack) {
+ var objIsArr = isArray(object),
+ othIsArr = isArray(other),
+ objTag = objIsArr ? arrayTag : getTag(object),
+ othTag = othIsArr ? arrayTag : getTag(other);
+
+ objTag = objTag == argsTag ? objectTag : objTag;
+ othTag = othTag == argsTag ? objectTag : othTag;
+
+ var objIsObj = objTag == objectTag,
+ othIsObj = othTag == objectTag,
+ isSameTag = objTag == othTag;
+
+ if (isSameTag && isBuffer(object)) {
+ if (!isBuffer(other)) {
+ return false;
+ }
+ objIsArr = true;
+ objIsObj = false;
+ }
+ if (isSameTag && !objIsObj) {
+ stack || (stack = new Stack);
+ return (objIsArr || isTypedArray(object))
+ ? equalArrays(object, other, bitmask, customizer, equalFunc, stack)
+ : equalByTag(object, other, objTag, bitmask, customizer, equalFunc, stack);
+ }
+ if (!(bitmask & COMPARE_PARTIAL_FLAG)) {
+ var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
+ othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
+
+ if (objIsWrapped || othIsWrapped) {
+ var objUnwrapped = objIsWrapped ? object.value() : object,
+ othUnwrapped = othIsWrapped ? other.value() : other;
+
+ stack || (stack = new Stack);
+ return equalFunc(objUnwrapped, othUnwrapped, bitmask, customizer, stack);
+ }
+ }
+ if (!isSameTag) {
+ return false;
+ }
+ stack || (stack = new Stack);
+ return equalObjects(object, other, bitmask, customizer, equalFunc, stack);
+}
+
+module.exports = baseIsEqualDeep;
diff --git a/node_modules/lodash/_baseIsMap.js b/node_modules/lodash/_baseIsMap.js
new file mode 100644
index 0000000..02a4021
--- /dev/null
+++ b/node_modules/lodash/_baseIsMap.js
@@ -0,0 +1,18 @@
+var getTag = require('./_getTag'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var mapTag = '[object Map]';
+
+/**
+ * The base implementation of `_.isMap` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a map, else `false`.
+ */
+function baseIsMap(value) {
+ return isObjectLike(value) && getTag(value) == mapTag;
+}
+
+module.exports = baseIsMap;
diff --git a/node_modules/lodash/_baseIsMatch.js b/node_modules/lodash/_baseIsMatch.js
new file mode 100644
index 0000000..72494be
--- /dev/null
+++ b/node_modules/lodash/_baseIsMatch.js
@@ -0,0 +1,62 @@
+var Stack = require('./_Stack'),
+ baseIsEqual = require('./_baseIsEqual');
+
+/** Used to compose bitmasks for value comparisons. */
+var COMPARE_PARTIAL_FLAG = 1,
+ COMPARE_UNORDERED_FLAG = 2;
+
+/**
+ * The base implementation of `_.isMatch` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property values to match.
+ * @param {Array} matchData The property names, values, and compare flags to match.
+ * @param {Function} [customizer] The function to customize comparisons.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ */
+function baseIsMatch(object, source, matchData, customizer) {
+ var index = matchData.length,
+ length = index,
+ noCustomizer = !customizer;
+
+ if (object == null) {
+ return !length;
+ }
+ object = Object(object);
+ while (index--) {
+ var data = matchData[index];
+ if ((noCustomizer && data[2])
+ ? data[1] !== object[data[0]]
+ : !(data[0] in object)
+ ) {
+ return false;
+ }
+ }
+ while (++index < length) {
+ data = matchData[index];
+ var key = data[0],
+ objValue = object[key],
+ srcValue = data[1];
+
+ if (noCustomizer && data[2]) {
+ if (objValue === undefined && !(key in object)) {
+ return false;
+ }
+ } else {
+ var stack = new Stack;
+ if (customizer) {
+ var result = customizer(objValue, srcValue, key, object, source, stack);
+ }
+ if (!(result === undefined
+ ? baseIsEqual(srcValue, objValue, COMPARE_PARTIAL_FLAG | COMPARE_UNORDERED_FLAG, customizer, stack)
+ : result
+ )) {
+ return false;
+ }
+ }
+ }
+ return true;
+}
+
+module.exports = baseIsMatch;
diff --git a/node_modules/lodash/_baseIsNaN.js b/node_modules/lodash/_baseIsNaN.js
new file mode 100644
index 0000000..316f1eb
--- /dev/null
+++ b/node_modules/lodash/_baseIsNaN.js
@@ -0,0 +1,12 @@
+/**
+ * The base implementation of `_.isNaN` without support for number objects.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `NaN`, else `false`.
+ */
+function baseIsNaN(value) {
+ return value !== value;
+}
+
+module.exports = baseIsNaN;
diff --git a/node_modules/lodash/_baseIsNative.js b/node_modules/lodash/_baseIsNative.js
new file mode 100644
index 0000000..8702330
--- /dev/null
+++ b/node_modules/lodash/_baseIsNative.js
@@ -0,0 +1,47 @@
+var isFunction = require('./isFunction'),
+ isMasked = require('./_isMasked'),
+ isObject = require('./isObject'),
+ toSource = require('./_toSource');
+
+/**
+ * Used to match `RegExp`
+ * [syntax characters](http://ecma-international.org/ecma-262/7.0/#sec-patterns).
+ */
+var reRegExpChar = /[\\^$.*+?()[\]{}|]/g;
+
+/** Used to detect host constructors (Safari). */
+var reIsHostCtor = /^\[object .+?Constructor\]$/;
+
+/** Used for built-in method references. */
+var funcProto = Function.prototype,
+ objectProto = Object.prototype;
+
+/** Used to resolve the decompiled source of functions. */
+var funcToString = funcProto.toString;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Used to detect if a method is native. */
+var reIsNative = RegExp('^' +
+ funcToString.call(hasOwnProperty).replace(reRegExpChar, '\\$&')
+ .replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
+);
+
+/**
+ * The base implementation of `_.isNative` without bad shim checks.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a native function,
+ * else `false`.
+ */
+function baseIsNative(value) {
+ if (!isObject(value) || isMasked(value)) {
+ return false;
+ }
+ var pattern = isFunction(value) ? reIsNative : reIsHostCtor;
+ return pattern.test(toSource(value));
+}
+
+module.exports = baseIsNative;
diff --git a/node_modules/lodash/_baseIsRegExp.js b/node_modules/lodash/_baseIsRegExp.js
new file mode 100644
index 0000000..6cd7c1a
--- /dev/null
+++ b/node_modules/lodash/_baseIsRegExp.js
@@ -0,0 +1,18 @@
+var baseGetTag = require('./_baseGetTag'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var regexpTag = '[object RegExp]';
+
+/**
+ * The base implementation of `_.isRegExp` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a regexp, else `false`.
+ */
+function baseIsRegExp(value) {
+ return isObjectLike(value) && baseGetTag(value) == regexpTag;
+}
+
+module.exports = baseIsRegExp;
diff --git a/node_modules/lodash/_baseIsSet.js b/node_modules/lodash/_baseIsSet.js
new file mode 100644
index 0000000..6dee367
--- /dev/null
+++ b/node_modules/lodash/_baseIsSet.js
@@ -0,0 +1,18 @@
+var getTag = require('./_getTag'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var setTag = '[object Set]';
+
+/**
+ * The base implementation of `_.isSet` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a set, else `false`.
+ */
+function baseIsSet(value) {
+ return isObjectLike(value) && getTag(value) == setTag;
+}
+
+module.exports = baseIsSet;
diff --git a/node_modules/lodash/_baseIsTypedArray.js b/node_modules/lodash/_baseIsTypedArray.js
new file mode 100644
index 0000000..1edb32f
--- /dev/null
+++ b/node_modules/lodash/_baseIsTypedArray.js
@@ -0,0 +1,60 @@
+var baseGetTag = require('./_baseGetTag'),
+ isLength = require('./isLength'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ funcTag = '[object Function]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ objectTag = '[object Object]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ weakMapTag = '[object WeakMap]';
+
+var arrayBufferTag = '[object ArrayBuffer]',
+ dataViewTag = '[object DataView]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+/** Used to identify `toStringTag` values of typed arrays. */
+var typedArrayTags = {};
+typedArrayTags[float32Tag] = typedArrayTags[float64Tag] =
+typedArrayTags[int8Tag] = typedArrayTags[int16Tag] =
+typedArrayTags[int32Tag] = typedArrayTags[uint8Tag] =
+typedArrayTags[uint8ClampedTag] = typedArrayTags[uint16Tag] =
+typedArrayTags[uint32Tag] = true;
+typedArrayTags[argsTag] = typedArrayTags[arrayTag] =
+typedArrayTags[arrayBufferTag] = typedArrayTags[boolTag] =
+typedArrayTags[dataViewTag] = typedArrayTags[dateTag] =
+typedArrayTags[errorTag] = typedArrayTags[funcTag] =
+typedArrayTags[mapTag] = typedArrayTags[numberTag] =
+typedArrayTags[objectTag] = typedArrayTags[regexpTag] =
+typedArrayTags[setTag] = typedArrayTags[stringTag] =
+typedArrayTags[weakMapTag] = false;
+
+/**
+ * The base implementation of `_.isTypedArray` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a typed array, else `false`.
+ */
+function baseIsTypedArray(value) {
+ return isObjectLike(value) &&
+ isLength(value.length) && !!typedArrayTags[baseGetTag(value)];
+}
+
+module.exports = baseIsTypedArray;
diff --git a/node_modules/lodash/_baseIteratee.js b/node_modules/lodash/_baseIteratee.js
new file mode 100644
index 0000000..995c257
--- /dev/null
+++ b/node_modules/lodash/_baseIteratee.js
@@ -0,0 +1,31 @@
+var baseMatches = require('./_baseMatches'),
+ baseMatchesProperty = require('./_baseMatchesProperty'),
+ identity = require('./identity'),
+ isArray = require('./isArray'),
+ property = require('./property');
+
+/**
+ * The base implementation of `_.iteratee`.
+ *
+ * @private
+ * @param {*} [value=_.identity] The value to convert to an iteratee.
+ * @returns {Function} Returns the iteratee.
+ */
+function baseIteratee(value) {
+ // Don't store the `typeof` result in a variable to avoid a JIT bug in Safari 9.
+ // See https://bugs.webkit.org/show_bug.cgi?id=156034 for more details.
+ if (typeof value == 'function') {
+ return value;
+ }
+ if (value == null) {
+ return identity;
+ }
+ if (typeof value == 'object') {
+ return isArray(value)
+ ? baseMatchesProperty(value[0], value[1])
+ : baseMatches(value);
+ }
+ return property(value);
+}
+
+module.exports = baseIteratee;
diff --git a/node_modules/lodash/_baseKeys.js b/node_modules/lodash/_baseKeys.js
new file mode 100644
index 0000000..45e9e6f
--- /dev/null
+++ b/node_modules/lodash/_baseKeys.js
@@ -0,0 +1,30 @@
+var isPrototype = require('./_isPrototype'),
+ nativeKeys = require('./_nativeKeys');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * The base implementation of `_.keys` which doesn't treat sparse arrays as dense.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+function baseKeys(object) {
+ if (!isPrototype(object)) {
+ return nativeKeys(object);
+ }
+ var result = [];
+ for (var key in Object(object)) {
+ if (hasOwnProperty.call(object, key) && key != 'constructor') {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+module.exports = baseKeys;
diff --git a/node_modules/lodash/_baseKeysIn.js b/node_modules/lodash/_baseKeysIn.js
new file mode 100644
index 0000000..ea8a0a1
--- /dev/null
+++ b/node_modules/lodash/_baseKeysIn.js
@@ -0,0 +1,33 @@
+var isObject = require('./isObject'),
+ isPrototype = require('./_isPrototype'),
+ nativeKeysIn = require('./_nativeKeysIn');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * The base implementation of `_.keysIn` which doesn't treat sparse arrays as dense.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+function baseKeysIn(object) {
+ if (!isObject(object)) {
+ return nativeKeysIn(object);
+ }
+ var isProto = isPrototype(object),
+ result = [];
+
+ for (var key in object) {
+ if (!(key == 'constructor' && (isProto || !hasOwnProperty.call(object, key)))) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+module.exports = baseKeysIn;
diff --git a/node_modules/lodash/_baseLodash.js b/node_modules/lodash/_baseLodash.js
new file mode 100644
index 0000000..f76c790
--- /dev/null
+++ b/node_modules/lodash/_baseLodash.js
@@ -0,0 +1,10 @@
+/**
+ * The function whose prototype chain sequence wrappers inherit from.
+ *
+ * @private
+ */
+function baseLodash() {
+ // No operation performed.
+}
+
+module.exports = baseLodash;
diff --git a/node_modules/lodash/_baseLt.js b/node_modules/lodash/_baseLt.js
new file mode 100644
index 0000000..8674d29
--- /dev/null
+++ b/node_modules/lodash/_baseLt.js
@@ -0,0 +1,14 @@
+/**
+ * The base implementation of `_.lt` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is less than `other`,
+ * else `false`.
+ */
+function baseLt(value, other) {
+ return value < other;
+}
+
+module.exports = baseLt;
diff --git a/node_modules/lodash/_baseMap.js b/node_modules/lodash/_baseMap.js
new file mode 100644
index 0000000..0bf5cea
--- /dev/null
+++ b/node_modules/lodash/_baseMap.js
@@ -0,0 +1,22 @@
+var baseEach = require('./_baseEach'),
+ isArrayLike = require('./isArrayLike');
+
+/**
+ * The base implementation of `_.map` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ */
+function baseMap(collection, iteratee) {
+ var index = -1,
+ result = isArrayLike(collection) ? Array(collection.length) : [];
+
+ baseEach(collection, function(value, key, collection) {
+ result[++index] = iteratee(value, key, collection);
+ });
+ return result;
+}
+
+module.exports = baseMap;
diff --git a/node_modules/lodash/_baseMatches.js b/node_modules/lodash/_baseMatches.js
new file mode 100644
index 0000000..e56582a
--- /dev/null
+++ b/node_modules/lodash/_baseMatches.js
@@ -0,0 +1,22 @@
+var baseIsMatch = require('./_baseIsMatch'),
+ getMatchData = require('./_getMatchData'),
+ matchesStrictComparable = require('./_matchesStrictComparable');
+
+/**
+ * The base implementation of `_.matches` which doesn't clone `source`.
+ *
+ * @private
+ * @param {Object} source The object of property values to match.
+ * @returns {Function} Returns the new spec function.
+ */
+function baseMatches(source) {
+ var matchData = getMatchData(source);
+ if (matchData.length == 1 && matchData[0][2]) {
+ return matchesStrictComparable(matchData[0][0], matchData[0][1]);
+ }
+ return function(object) {
+ return object === source || baseIsMatch(object, source, matchData);
+ };
+}
+
+module.exports = baseMatches;
diff --git a/node_modules/lodash/_baseMatchesProperty.js b/node_modules/lodash/_baseMatchesProperty.js
new file mode 100644
index 0000000..24afd89
--- /dev/null
+++ b/node_modules/lodash/_baseMatchesProperty.js
@@ -0,0 +1,33 @@
+var baseIsEqual = require('./_baseIsEqual'),
+ get = require('./get'),
+ hasIn = require('./hasIn'),
+ isKey = require('./_isKey'),
+ isStrictComparable = require('./_isStrictComparable'),
+ matchesStrictComparable = require('./_matchesStrictComparable'),
+ toKey = require('./_toKey');
+
+/** Used to compose bitmasks for value comparisons. */
+var COMPARE_PARTIAL_FLAG = 1,
+ COMPARE_UNORDERED_FLAG = 2;
+
+/**
+ * The base implementation of `_.matchesProperty` which doesn't clone `srcValue`.
+ *
+ * @private
+ * @param {string} path The path of the property to get.
+ * @param {*} srcValue The value to match.
+ * @returns {Function} Returns the new spec function.
+ */
+function baseMatchesProperty(path, srcValue) {
+ if (isKey(path) && isStrictComparable(srcValue)) {
+ return matchesStrictComparable(toKey(path), srcValue);
+ }
+ return function(object) {
+ var objValue = get(object, path);
+ return (objValue === undefined && objValue === srcValue)
+ ? hasIn(object, path)
+ : baseIsEqual(srcValue, objValue, COMPARE_PARTIAL_FLAG | COMPARE_UNORDERED_FLAG);
+ };
+}
+
+module.exports = baseMatchesProperty;
diff --git a/node_modules/lodash/_baseMean.js b/node_modules/lodash/_baseMean.js
new file mode 100644
index 0000000..fa9e00a
--- /dev/null
+++ b/node_modules/lodash/_baseMean.js
@@ -0,0 +1,20 @@
+var baseSum = require('./_baseSum');
+
+/** Used as references for various `Number` constants. */
+var NAN = 0 / 0;
+
+/**
+ * The base implementation of `_.mean` and `_.meanBy` without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {number} Returns the mean.
+ */
+function baseMean(array, iteratee) {
+ var length = array == null ? 0 : array.length;
+ return length ? (baseSum(array, iteratee) / length) : NAN;
+}
+
+module.exports = baseMean;
diff --git a/node_modules/lodash/_baseMerge.js b/node_modules/lodash/_baseMerge.js
new file mode 100644
index 0000000..c98b5eb
--- /dev/null
+++ b/node_modules/lodash/_baseMerge.js
@@ -0,0 +1,42 @@
+var Stack = require('./_Stack'),
+ assignMergeValue = require('./_assignMergeValue'),
+ baseFor = require('./_baseFor'),
+ baseMergeDeep = require('./_baseMergeDeep'),
+ isObject = require('./isObject'),
+ keysIn = require('./keysIn'),
+ safeGet = require('./_safeGet');
+
+/**
+ * The base implementation of `_.merge` without support for multiple sources.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {number} srcIndex The index of `source`.
+ * @param {Function} [customizer] The function to customize merged values.
+ * @param {Object} [stack] Tracks traversed source values and their merged
+ * counterparts.
+ */
+function baseMerge(object, source, srcIndex, customizer, stack) {
+ if (object === source) {
+ return;
+ }
+ baseFor(source, function(srcValue, key) {
+ stack || (stack = new Stack);
+ if (isObject(srcValue)) {
+ baseMergeDeep(object, source, key, srcIndex, baseMerge, customizer, stack);
+ }
+ else {
+ var newValue = customizer
+ ? customizer(safeGet(object, key), srcValue, (key + ''), object, source, stack)
+ : undefined;
+
+ if (newValue === undefined) {
+ newValue = srcValue;
+ }
+ assignMergeValue(object, key, newValue);
+ }
+ }, keysIn);
+}
+
+module.exports = baseMerge;
diff --git a/node_modules/lodash/_baseMergeDeep.js b/node_modules/lodash/_baseMergeDeep.js
new file mode 100644
index 0000000..4679e8d
--- /dev/null
+++ b/node_modules/lodash/_baseMergeDeep.js
@@ -0,0 +1,94 @@
+var assignMergeValue = require('./_assignMergeValue'),
+ cloneBuffer = require('./_cloneBuffer'),
+ cloneTypedArray = require('./_cloneTypedArray'),
+ copyArray = require('./_copyArray'),
+ initCloneObject = require('./_initCloneObject'),
+ isArguments = require('./isArguments'),
+ isArray = require('./isArray'),
+ isArrayLikeObject = require('./isArrayLikeObject'),
+ isBuffer = require('./isBuffer'),
+ isFunction = require('./isFunction'),
+ isObject = require('./isObject'),
+ isPlainObject = require('./isPlainObject'),
+ isTypedArray = require('./isTypedArray'),
+ safeGet = require('./_safeGet'),
+ toPlainObject = require('./toPlainObject');
+
+/**
+ * A specialized version of `baseMerge` for arrays and objects which performs
+ * deep merges and tracks traversed objects enabling objects with circular
+ * references to be merged.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {string} key The key of the value to merge.
+ * @param {number} srcIndex The index of `source`.
+ * @param {Function} mergeFunc The function to merge values.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @param {Object} [stack] Tracks traversed source values and their merged
+ * counterparts.
+ */
+function baseMergeDeep(object, source, key, srcIndex, mergeFunc, customizer, stack) {
+ var objValue = safeGet(object, key),
+ srcValue = safeGet(source, key),
+ stacked = stack.get(srcValue);
+
+ if (stacked) {
+ assignMergeValue(object, key, stacked);
+ return;
+ }
+ var newValue = customizer
+ ? customizer(objValue, srcValue, (key + ''), object, source, stack)
+ : undefined;
+
+ var isCommon = newValue === undefined;
+
+ if (isCommon) {
+ var isArr = isArray(srcValue),
+ isBuff = !isArr && isBuffer(srcValue),
+ isTyped = !isArr && !isBuff && isTypedArray(srcValue);
+
+ newValue = srcValue;
+ if (isArr || isBuff || isTyped) {
+ if (isArray(objValue)) {
+ newValue = objValue;
+ }
+ else if (isArrayLikeObject(objValue)) {
+ newValue = copyArray(objValue);
+ }
+ else if (isBuff) {
+ isCommon = false;
+ newValue = cloneBuffer(srcValue, true);
+ }
+ else if (isTyped) {
+ isCommon = false;
+ newValue = cloneTypedArray(srcValue, true);
+ }
+ else {
+ newValue = [];
+ }
+ }
+ else if (isPlainObject(srcValue) || isArguments(srcValue)) {
+ newValue = objValue;
+ if (isArguments(objValue)) {
+ newValue = toPlainObject(objValue);
+ }
+ else if (!isObject(objValue) || isFunction(objValue)) {
+ newValue = initCloneObject(srcValue);
+ }
+ }
+ else {
+ isCommon = false;
+ }
+ }
+ if (isCommon) {
+ // Recursively merge objects and arrays (susceptible to call stack limits).
+ stack.set(srcValue, newValue);
+ mergeFunc(newValue, srcValue, srcIndex, customizer, stack);
+ stack['delete'](srcValue);
+ }
+ assignMergeValue(object, key, newValue);
+}
+
+module.exports = baseMergeDeep;
diff --git a/node_modules/lodash/_baseNth.js b/node_modules/lodash/_baseNth.js
new file mode 100644
index 0000000..0403c2a
--- /dev/null
+++ b/node_modules/lodash/_baseNth.js
@@ -0,0 +1,20 @@
+var isIndex = require('./_isIndex');
+
+/**
+ * The base implementation of `_.nth` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {Array} array The array to query.
+ * @param {number} n The index of the element to return.
+ * @returns {*} Returns the nth element of `array`.
+ */
+function baseNth(array, n) {
+ var length = array.length;
+ if (!length) {
+ return;
+ }
+ n += n < 0 ? length : 0;
+ return isIndex(n, length) ? array[n] : undefined;
+}
+
+module.exports = baseNth;
diff --git a/node_modules/lodash/_baseOrderBy.js b/node_modules/lodash/_baseOrderBy.js
new file mode 100644
index 0000000..775a017
--- /dev/null
+++ b/node_modules/lodash/_baseOrderBy.js
@@ -0,0 +1,49 @@
+var arrayMap = require('./_arrayMap'),
+ baseGet = require('./_baseGet'),
+ baseIteratee = require('./_baseIteratee'),
+ baseMap = require('./_baseMap'),
+ baseSortBy = require('./_baseSortBy'),
+ baseUnary = require('./_baseUnary'),
+ compareMultiple = require('./_compareMultiple'),
+ identity = require('./identity'),
+ isArray = require('./isArray');
+
+/**
+ * The base implementation of `_.orderBy` without param guards.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function[]|Object[]|string[]} iteratees The iteratees to sort by.
+ * @param {string[]} orders The sort orders of `iteratees`.
+ * @returns {Array} Returns the new sorted array.
+ */
+function baseOrderBy(collection, iteratees, orders) {
+ if (iteratees.length) {
+ iteratees = arrayMap(iteratees, function(iteratee) {
+ if (isArray(iteratee)) {
+ return function(value) {
+ return baseGet(value, iteratee.length === 1 ? iteratee[0] : iteratee);
+ }
+ }
+ return iteratee;
+ });
+ } else {
+ iteratees = [identity];
+ }
+
+ var index = -1;
+ iteratees = arrayMap(iteratees, baseUnary(baseIteratee));
+
+ var result = baseMap(collection, function(value, key, collection) {
+ var criteria = arrayMap(iteratees, function(iteratee) {
+ return iteratee(value);
+ });
+ return { 'criteria': criteria, 'index': ++index, 'value': value };
+ });
+
+ return baseSortBy(result, function(object, other) {
+ return compareMultiple(object, other, orders);
+ });
+}
+
+module.exports = baseOrderBy;
diff --git a/node_modules/lodash/_basePick.js b/node_modules/lodash/_basePick.js
new file mode 100644
index 0000000..09b458a
--- /dev/null
+++ b/node_modules/lodash/_basePick.js
@@ -0,0 +1,19 @@
+var basePickBy = require('./_basePickBy'),
+ hasIn = require('./hasIn');
+
+/**
+ * The base implementation of `_.pick` without support for individual
+ * property identifiers.
+ *
+ * @private
+ * @param {Object} object The source object.
+ * @param {string[]} paths The property paths to pick.
+ * @returns {Object} Returns the new object.
+ */
+function basePick(object, paths) {
+ return basePickBy(object, paths, function(value, path) {
+ return hasIn(object, path);
+ });
+}
+
+module.exports = basePick;
diff --git a/node_modules/lodash/_basePickBy.js b/node_modules/lodash/_basePickBy.js
new file mode 100644
index 0000000..85be68c
--- /dev/null
+++ b/node_modules/lodash/_basePickBy.js
@@ -0,0 +1,30 @@
+var baseGet = require('./_baseGet'),
+ baseSet = require('./_baseSet'),
+ castPath = require('./_castPath');
+
+/**
+ * The base implementation of `_.pickBy` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Object} object The source object.
+ * @param {string[]} paths The property paths to pick.
+ * @param {Function} predicate The function invoked per property.
+ * @returns {Object} Returns the new object.
+ */
+function basePickBy(object, paths, predicate) {
+ var index = -1,
+ length = paths.length,
+ result = {};
+
+ while (++index < length) {
+ var path = paths[index],
+ value = baseGet(object, path);
+
+ if (predicate(value, path)) {
+ baseSet(result, castPath(path, object), value);
+ }
+ }
+ return result;
+}
+
+module.exports = basePickBy;
diff --git a/node_modules/lodash/_baseProperty.js b/node_modules/lodash/_baseProperty.js
new file mode 100644
index 0000000..496281e
--- /dev/null
+++ b/node_modules/lodash/_baseProperty.js
@@ -0,0 +1,14 @@
+/**
+ * The base implementation of `_.property` without support for deep paths.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @returns {Function} Returns the new accessor function.
+ */
+function baseProperty(key) {
+ return function(object) {
+ return object == null ? undefined : object[key];
+ };
+}
+
+module.exports = baseProperty;
diff --git a/node_modules/lodash/_basePropertyDeep.js b/node_modules/lodash/_basePropertyDeep.js
new file mode 100644
index 0000000..1e5aae5
--- /dev/null
+++ b/node_modules/lodash/_basePropertyDeep.js
@@ -0,0 +1,16 @@
+var baseGet = require('./_baseGet');
+
+/**
+ * A specialized version of `baseProperty` which supports deep paths.
+ *
+ * @private
+ * @param {Array|string} path The path of the property to get.
+ * @returns {Function} Returns the new accessor function.
+ */
+function basePropertyDeep(path) {
+ return function(object) {
+ return baseGet(object, path);
+ };
+}
+
+module.exports = basePropertyDeep;
diff --git a/node_modules/lodash/_basePropertyOf.js b/node_modules/lodash/_basePropertyOf.js
new file mode 100644
index 0000000..4617399
--- /dev/null
+++ b/node_modules/lodash/_basePropertyOf.js
@@ -0,0 +1,14 @@
+/**
+ * The base implementation of `_.propertyOf` without support for deep paths.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Function} Returns the new accessor function.
+ */
+function basePropertyOf(object) {
+ return function(key) {
+ return object == null ? undefined : object[key];
+ };
+}
+
+module.exports = basePropertyOf;
diff --git a/node_modules/lodash/_basePullAll.js b/node_modules/lodash/_basePullAll.js
new file mode 100644
index 0000000..305720e
--- /dev/null
+++ b/node_modules/lodash/_basePullAll.js
@@ -0,0 +1,51 @@
+var arrayMap = require('./_arrayMap'),
+ baseIndexOf = require('./_baseIndexOf'),
+ baseIndexOfWith = require('./_baseIndexOfWith'),
+ baseUnary = require('./_baseUnary'),
+ copyArray = require('./_copyArray');
+
+/** Used for built-in method references. */
+var arrayProto = Array.prototype;
+
+/** Built-in value references. */
+var splice = arrayProto.splice;
+
+/**
+ * The base implementation of `_.pullAllBy` without support for iteratee
+ * shorthands.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to remove.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns `array`.
+ */
+function basePullAll(array, values, iteratee, comparator) {
+ var indexOf = comparator ? baseIndexOfWith : baseIndexOf,
+ index = -1,
+ length = values.length,
+ seen = array;
+
+ if (array === values) {
+ values = copyArray(values);
+ }
+ if (iteratee) {
+ seen = arrayMap(array, baseUnary(iteratee));
+ }
+ while (++index < length) {
+ var fromIndex = 0,
+ value = values[index],
+ computed = iteratee ? iteratee(value) : value;
+
+ while ((fromIndex = indexOf(seen, computed, fromIndex, comparator)) > -1) {
+ if (seen !== array) {
+ splice.call(seen, fromIndex, 1);
+ }
+ splice.call(array, fromIndex, 1);
+ }
+ }
+ return array;
+}
+
+module.exports = basePullAll;
diff --git a/node_modules/lodash/_basePullAt.js b/node_modules/lodash/_basePullAt.js
new file mode 100644
index 0000000..c3e9e71
--- /dev/null
+++ b/node_modules/lodash/_basePullAt.js
@@ -0,0 +1,37 @@
+var baseUnset = require('./_baseUnset'),
+ isIndex = require('./_isIndex');
+
+/** Used for built-in method references. */
+var arrayProto = Array.prototype;
+
+/** Built-in value references. */
+var splice = arrayProto.splice;
+
+/**
+ * The base implementation of `_.pullAt` without support for individual
+ * indexes or capturing the removed elements.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {number[]} indexes The indexes of elements to remove.
+ * @returns {Array} Returns `array`.
+ */
+function basePullAt(array, indexes) {
+ var length = array ? indexes.length : 0,
+ lastIndex = length - 1;
+
+ while (length--) {
+ var index = indexes[length];
+ if (length == lastIndex || index !== previous) {
+ var previous = index;
+ if (isIndex(index)) {
+ splice.call(array, index, 1);
+ } else {
+ baseUnset(array, index);
+ }
+ }
+ }
+ return array;
+}
+
+module.exports = basePullAt;
diff --git a/node_modules/lodash/_baseRandom.js b/node_modules/lodash/_baseRandom.js
new file mode 100644
index 0000000..94f76a7
--- /dev/null
+++ b/node_modules/lodash/_baseRandom.js
@@ -0,0 +1,18 @@
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeFloor = Math.floor,
+ nativeRandom = Math.random;
+
+/**
+ * The base implementation of `_.random` without support for returning
+ * floating-point numbers.
+ *
+ * @private
+ * @param {number} lower The lower bound.
+ * @param {number} upper The upper bound.
+ * @returns {number} Returns the random number.
+ */
+function baseRandom(lower, upper) {
+ return lower + nativeFloor(nativeRandom() * (upper - lower + 1));
+}
+
+module.exports = baseRandom;
diff --git a/node_modules/lodash/_baseRange.js b/node_modules/lodash/_baseRange.js
new file mode 100644
index 0000000..0fb8e41
--- /dev/null
+++ b/node_modules/lodash/_baseRange.js
@@ -0,0 +1,28 @@
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeCeil = Math.ceil,
+ nativeMax = Math.max;
+
+/**
+ * The base implementation of `_.range` and `_.rangeRight` which doesn't
+ * coerce arguments.
+ *
+ * @private
+ * @param {number} start The start of the range.
+ * @param {number} end The end of the range.
+ * @param {number} step The value to increment or decrement by.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Array} Returns the range of numbers.
+ */
+function baseRange(start, end, step, fromRight) {
+ var index = -1,
+ length = nativeMax(nativeCeil((end - start) / (step || 1)), 0),
+ result = Array(length);
+
+ while (length--) {
+ result[fromRight ? length : ++index] = start;
+ start += step;
+ }
+ return result;
+}
+
+module.exports = baseRange;
diff --git a/node_modules/lodash/_baseReduce.js b/node_modules/lodash/_baseReduce.js
new file mode 100644
index 0000000..5a1f8b5
--- /dev/null
+++ b/node_modules/lodash/_baseReduce.js
@@ -0,0 +1,23 @@
+/**
+ * The base implementation of `_.reduce` and `_.reduceRight`, without support
+ * for iteratee shorthands, which iterates over `collection` using `eachFunc`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} accumulator The initial value.
+ * @param {boolean} initAccum Specify using the first or last element of
+ * `collection` as the initial value.
+ * @param {Function} eachFunc The function to iterate over `collection`.
+ * @returns {*} Returns the accumulated value.
+ */
+function baseReduce(collection, iteratee, accumulator, initAccum, eachFunc) {
+ eachFunc(collection, function(value, index, collection) {
+ accumulator = initAccum
+ ? (initAccum = false, value)
+ : iteratee(accumulator, value, index, collection);
+ });
+ return accumulator;
+}
+
+module.exports = baseReduce;
diff --git a/node_modules/lodash/_baseRepeat.js b/node_modules/lodash/_baseRepeat.js
new file mode 100644
index 0000000..ee44c31
--- /dev/null
+++ b/node_modules/lodash/_baseRepeat.js
@@ -0,0 +1,35 @@
+/** Used as references for various `Number` constants. */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeFloor = Math.floor;
+
+/**
+ * The base implementation of `_.repeat` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {string} string The string to repeat.
+ * @param {number} n The number of times to repeat the string.
+ * @returns {string} Returns the repeated string.
+ */
+function baseRepeat(string, n) {
+ var result = '';
+ if (!string || n < 1 || n > MAX_SAFE_INTEGER) {
+ return result;
+ }
+ // Leverage the exponentiation by squaring algorithm for a faster repeat.
+ // See https://en.wikipedia.org/wiki/Exponentiation_by_squaring for more details.
+ do {
+ if (n % 2) {
+ result += string;
+ }
+ n = nativeFloor(n / 2);
+ if (n) {
+ string += string;
+ }
+ } while (n);
+
+ return result;
+}
+
+module.exports = baseRepeat;
diff --git a/node_modules/lodash/_baseRest.js b/node_modules/lodash/_baseRest.js
new file mode 100644
index 0000000..d0dc4bd
--- /dev/null
+++ b/node_modules/lodash/_baseRest.js
@@ -0,0 +1,17 @@
+var identity = require('./identity'),
+ overRest = require('./_overRest'),
+ setToString = require('./_setToString');
+
+/**
+ * The base implementation of `_.rest` which doesn't validate or coerce arguments.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @returns {Function} Returns the new function.
+ */
+function baseRest(func, start) {
+ return setToString(overRest(func, start, identity), func + '');
+}
+
+module.exports = baseRest;
diff --git a/node_modules/lodash/_baseSample.js b/node_modules/lodash/_baseSample.js
new file mode 100644
index 0000000..58582b9
--- /dev/null
+++ b/node_modules/lodash/_baseSample.js
@@ -0,0 +1,15 @@
+var arraySample = require('./_arraySample'),
+ values = require('./values');
+
+/**
+ * The base implementation of `_.sample`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to sample.
+ * @returns {*} Returns the random element.
+ */
+function baseSample(collection) {
+ return arraySample(values(collection));
+}
+
+module.exports = baseSample;
diff --git a/node_modules/lodash/_baseSampleSize.js b/node_modules/lodash/_baseSampleSize.js
new file mode 100644
index 0000000..5c90ec5
--- /dev/null
+++ b/node_modules/lodash/_baseSampleSize.js
@@ -0,0 +1,18 @@
+var baseClamp = require('./_baseClamp'),
+ shuffleSelf = require('./_shuffleSelf'),
+ values = require('./values');
+
+/**
+ * The base implementation of `_.sampleSize` without param guards.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to sample.
+ * @param {number} n The number of elements to sample.
+ * @returns {Array} Returns the random elements.
+ */
+function baseSampleSize(collection, n) {
+ var array = values(collection);
+ return shuffleSelf(array, baseClamp(n, 0, array.length));
+}
+
+module.exports = baseSampleSize;
diff --git a/node_modules/lodash/_baseSet.js b/node_modules/lodash/_baseSet.js
new file mode 100644
index 0000000..99f4fbf
--- /dev/null
+++ b/node_modules/lodash/_baseSet.js
@@ -0,0 +1,51 @@
+var assignValue = require('./_assignValue'),
+ castPath = require('./_castPath'),
+ isIndex = require('./_isIndex'),
+ isObject = require('./isObject'),
+ toKey = require('./_toKey');
+
+/**
+ * The base implementation of `_.set`.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The path of the property to set.
+ * @param {*} value The value to set.
+ * @param {Function} [customizer] The function to customize path creation.
+ * @returns {Object} Returns `object`.
+ */
+function baseSet(object, path, value, customizer) {
+ if (!isObject(object)) {
+ return object;
+ }
+ path = castPath(path, object);
+
+ var index = -1,
+ length = path.length,
+ lastIndex = length - 1,
+ nested = object;
+
+ while (nested != null && ++index < length) {
+ var key = toKey(path[index]),
+ newValue = value;
+
+ if (key === '__proto__' || key === 'constructor' || key === 'prototype') {
+ return object;
+ }
+
+ if (index != lastIndex) {
+ var objValue = nested[key];
+ newValue = customizer ? customizer(objValue, key, nested) : undefined;
+ if (newValue === undefined) {
+ newValue = isObject(objValue)
+ ? objValue
+ : (isIndex(path[index + 1]) ? [] : {});
+ }
+ }
+ assignValue(nested, key, newValue);
+ nested = nested[key];
+ }
+ return object;
+}
+
+module.exports = baseSet;
diff --git a/node_modules/lodash/_baseSetData.js b/node_modules/lodash/_baseSetData.js
new file mode 100644
index 0000000..c409947
--- /dev/null
+++ b/node_modules/lodash/_baseSetData.js
@@ -0,0 +1,17 @@
+var identity = require('./identity'),
+ metaMap = require('./_metaMap');
+
+/**
+ * The base implementation of `setData` without support for hot loop shorting.
+ *
+ * @private
+ * @param {Function} func The function to associate metadata with.
+ * @param {*} data The metadata.
+ * @returns {Function} Returns `func`.
+ */
+var baseSetData = !metaMap ? identity : function(func, data) {
+ metaMap.set(func, data);
+ return func;
+};
+
+module.exports = baseSetData;
diff --git a/node_modules/lodash/_baseSetToString.js b/node_modules/lodash/_baseSetToString.js
new file mode 100644
index 0000000..89eaca3
--- /dev/null
+++ b/node_modules/lodash/_baseSetToString.js
@@ -0,0 +1,22 @@
+var constant = require('./constant'),
+ defineProperty = require('./_defineProperty'),
+ identity = require('./identity');
+
+/**
+ * The base implementation of `setToString` without support for hot loop shorting.
+ *
+ * @private
+ * @param {Function} func The function to modify.
+ * @param {Function} string The `toString` result.
+ * @returns {Function} Returns `func`.
+ */
+var baseSetToString = !defineProperty ? identity : function(func, string) {
+ return defineProperty(func, 'toString', {
+ 'configurable': true,
+ 'enumerable': false,
+ 'value': constant(string),
+ 'writable': true
+ });
+};
+
+module.exports = baseSetToString;
diff --git a/node_modules/lodash/_baseShuffle.js b/node_modules/lodash/_baseShuffle.js
new file mode 100644
index 0000000..023077a
--- /dev/null
+++ b/node_modules/lodash/_baseShuffle.js
@@ -0,0 +1,15 @@
+var shuffleSelf = require('./_shuffleSelf'),
+ values = require('./values');
+
+/**
+ * The base implementation of `_.shuffle`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to shuffle.
+ * @returns {Array} Returns the new shuffled array.
+ */
+function baseShuffle(collection) {
+ return shuffleSelf(values(collection));
+}
+
+module.exports = baseShuffle;
diff --git a/node_modules/lodash/_baseSlice.js b/node_modules/lodash/_baseSlice.js
new file mode 100644
index 0000000..786f6c9
--- /dev/null
+++ b/node_modules/lodash/_baseSlice.js
@@ -0,0 +1,31 @@
+/**
+ * The base implementation of `_.slice` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to slice.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the slice of `array`.
+ */
+function baseSlice(array, start, end) {
+ var index = -1,
+ length = array.length;
+
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = end > length ? length : end;
+ if (end < 0) {
+ end += length;
+ }
+ length = start > end ? 0 : ((end - start) >>> 0);
+ start >>>= 0;
+
+ var result = Array(length);
+ while (++index < length) {
+ result[index] = array[index + start];
+ }
+ return result;
+}
+
+module.exports = baseSlice;
diff --git a/node_modules/lodash/_baseSome.js b/node_modules/lodash/_baseSome.js
new file mode 100644
index 0000000..58f3f44
--- /dev/null
+++ b/node_modules/lodash/_baseSome.js
@@ -0,0 +1,22 @@
+var baseEach = require('./_baseEach');
+
+/**
+ * The base implementation of `_.some` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ */
+function baseSome(collection, predicate) {
+ var result;
+
+ baseEach(collection, function(value, index, collection) {
+ result = predicate(value, index, collection);
+ return !result;
+ });
+ return !!result;
+}
+
+module.exports = baseSome;
diff --git a/node_modules/lodash/_baseSortBy.js b/node_modules/lodash/_baseSortBy.js
new file mode 100644
index 0000000..a25c92e
--- /dev/null
+++ b/node_modules/lodash/_baseSortBy.js
@@ -0,0 +1,21 @@
+/**
+ * The base implementation of `_.sortBy` which uses `comparer` to define the
+ * sort order of `array` and replaces criteria objects with their corresponding
+ * values.
+ *
+ * @private
+ * @param {Array} array The array to sort.
+ * @param {Function} comparer The function to define sort order.
+ * @returns {Array} Returns `array`.
+ */
+function baseSortBy(array, comparer) {
+ var length = array.length;
+
+ array.sort(comparer);
+ while (length--) {
+ array[length] = array[length].value;
+ }
+ return array;
+}
+
+module.exports = baseSortBy;
diff --git a/node_modules/lodash/_baseSortedIndex.js b/node_modules/lodash/_baseSortedIndex.js
new file mode 100644
index 0000000..638c366
--- /dev/null
+++ b/node_modules/lodash/_baseSortedIndex.js
@@ -0,0 +1,42 @@
+var baseSortedIndexBy = require('./_baseSortedIndexBy'),
+ identity = require('./identity'),
+ isSymbol = require('./isSymbol');
+
+/** Used as references for the maximum length and index of an array. */
+var MAX_ARRAY_LENGTH = 4294967295,
+ HALF_MAX_ARRAY_LENGTH = MAX_ARRAY_LENGTH >>> 1;
+
+/**
+ * The base implementation of `_.sortedIndex` and `_.sortedLastIndex` which
+ * performs a binary search of `array` to determine the index at which `value`
+ * should be inserted into `array` in order to maintain its sort order.
+ *
+ * @private
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {boolean} [retHighest] Specify returning the highest qualified index.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ */
+function baseSortedIndex(array, value, retHighest) {
+ var low = 0,
+ high = array == null ? low : array.length;
+
+ if (typeof value == 'number' && value === value && high <= HALF_MAX_ARRAY_LENGTH) {
+ while (low < high) {
+ var mid = (low + high) >>> 1,
+ computed = array[mid];
+
+ if (computed !== null && !isSymbol(computed) &&
+ (retHighest ? (computed <= value) : (computed < value))) {
+ low = mid + 1;
+ } else {
+ high = mid;
+ }
+ }
+ return high;
+ }
+ return baseSortedIndexBy(array, value, identity, retHighest);
+}
+
+module.exports = baseSortedIndex;
diff --git a/node_modules/lodash/_baseSortedIndexBy.js b/node_modules/lodash/_baseSortedIndexBy.js
new file mode 100644
index 0000000..c247b37
--- /dev/null
+++ b/node_modules/lodash/_baseSortedIndexBy.js
@@ -0,0 +1,67 @@
+var isSymbol = require('./isSymbol');
+
+/** Used as references for the maximum length and index of an array. */
+var MAX_ARRAY_LENGTH = 4294967295,
+ MAX_ARRAY_INDEX = MAX_ARRAY_LENGTH - 1;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeFloor = Math.floor,
+ nativeMin = Math.min;
+
+/**
+ * The base implementation of `_.sortedIndexBy` and `_.sortedLastIndexBy`
+ * which invokes `iteratee` for `value` and each element of `array` to compute
+ * their sort ranking. The iteratee is invoked with one argument; (value).
+ *
+ * @private
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {Function} iteratee The iteratee invoked per element.
+ * @param {boolean} [retHighest] Specify returning the highest qualified index.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ */
+function baseSortedIndexBy(array, value, iteratee, retHighest) {
+ var low = 0,
+ high = array == null ? 0 : array.length;
+ if (high === 0) {
+ return 0;
+ }
+
+ value = iteratee(value);
+ var valIsNaN = value !== value,
+ valIsNull = value === null,
+ valIsSymbol = isSymbol(value),
+ valIsUndefined = value === undefined;
+
+ while (low < high) {
+ var mid = nativeFloor((low + high) / 2),
+ computed = iteratee(array[mid]),
+ othIsDefined = computed !== undefined,
+ othIsNull = computed === null,
+ othIsReflexive = computed === computed,
+ othIsSymbol = isSymbol(computed);
+
+ if (valIsNaN) {
+ var setLow = retHighest || othIsReflexive;
+ } else if (valIsUndefined) {
+ setLow = othIsReflexive && (retHighest || othIsDefined);
+ } else if (valIsNull) {
+ setLow = othIsReflexive && othIsDefined && (retHighest || !othIsNull);
+ } else if (valIsSymbol) {
+ setLow = othIsReflexive && othIsDefined && !othIsNull && (retHighest || !othIsSymbol);
+ } else if (othIsNull || othIsSymbol) {
+ setLow = false;
+ } else {
+ setLow = retHighest ? (computed <= value) : (computed < value);
+ }
+ if (setLow) {
+ low = mid + 1;
+ } else {
+ high = mid;
+ }
+ }
+ return nativeMin(high, MAX_ARRAY_INDEX);
+}
+
+module.exports = baseSortedIndexBy;
diff --git a/node_modules/lodash/_baseSortedUniq.js b/node_modules/lodash/_baseSortedUniq.js
new file mode 100644
index 0000000..802159a
--- /dev/null
+++ b/node_modules/lodash/_baseSortedUniq.js
@@ -0,0 +1,30 @@
+var eq = require('./eq');
+
+/**
+ * The base implementation of `_.sortedUniq` and `_.sortedUniqBy` without
+ * support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @returns {Array} Returns the new duplicate free array.
+ */
+function baseSortedUniq(array, iteratee) {
+ var index = -1,
+ length = array.length,
+ resIndex = 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index],
+ computed = iteratee ? iteratee(value) : value;
+
+ if (!index || !eq(computed, seen)) {
+ var seen = computed;
+ result[resIndex++] = value === 0 ? 0 : value;
+ }
+ }
+ return result;
+}
+
+module.exports = baseSortedUniq;
diff --git a/node_modules/lodash/_baseSum.js b/node_modules/lodash/_baseSum.js
new file mode 100644
index 0000000..a9e84c1
--- /dev/null
+++ b/node_modules/lodash/_baseSum.js
@@ -0,0 +1,24 @@
+/**
+ * The base implementation of `_.sum` and `_.sumBy` without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {number} Returns the sum.
+ */
+function baseSum(array, iteratee) {
+ var result,
+ index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ var current = iteratee(array[index]);
+ if (current !== undefined) {
+ result = result === undefined ? current : (result + current);
+ }
+ }
+ return result;
+}
+
+module.exports = baseSum;
diff --git a/node_modules/lodash/_baseTimes.js b/node_modules/lodash/_baseTimes.js
new file mode 100644
index 0000000..0603fc3
--- /dev/null
+++ b/node_modules/lodash/_baseTimes.js
@@ -0,0 +1,20 @@
+/**
+ * The base implementation of `_.times` without support for iteratee shorthands
+ * or max array length checks.
+ *
+ * @private
+ * @param {number} n The number of times to invoke `iteratee`.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the array of results.
+ */
+function baseTimes(n, iteratee) {
+ var index = -1,
+ result = Array(n);
+
+ while (++index < n) {
+ result[index] = iteratee(index);
+ }
+ return result;
+}
+
+module.exports = baseTimes;
diff --git a/node_modules/lodash/_baseToNumber.js b/node_modules/lodash/_baseToNumber.js
new file mode 100644
index 0000000..04859f3
--- /dev/null
+++ b/node_modules/lodash/_baseToNumber.js
@@ -0,0 +1,24 @@
+var isSymbol = require('./isSymbol');
+
+/** Used as references for various `Number` constants. */
+var NAN = 0 / 0;
+
+/**
+ * The base implementation of `_.toNumber` which doesn't ensure correct
+ * conversions of binary, hexadecimal, or octal string values.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {number} Returns the number.
+ */
+function baseToNumber(value) {
+ if (typeof value == 'number') {
+ return value;
+ }
+ if (isSymbol(value)) {
+ return NAN;
+ }
+ return +value;
+}
+
+module.exports = baseToNumber;
diff --git a/node_modules/lodash/_baseToPairs.js b/node_modules/lodash/_baseToPairs.js
new file mode 100644
index 0000000..bff1991
--- /dev/null
+++ b/node_modules/lodash/_baseToPairs.js
@@ -0,0 +1,18 @@
+var arrayMap = require('./_arrayMap');
+
+/**
+ * The base implementation of `_.toPairs` and `_.toPairsIn` which creates an array
+ * of key-value pairs for `object` corresponding to the property names of `props`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} props The property names to get values for.
+ * @returns {Object} Returns the key-value pairs.
+ */
+function baseToPairs(object, props) {
+ return arrayMap(props, function(key) {
+ return [key, object[key]];
+ });
+}
+
+module.exports = baseToPairs;
diff --git a/node_modules/lodash/_baseToString.js b/node_modules/lodash/_baseToString.js
new file mode 100644
index 0000000..ada6ad2
--- /dev/null
+++ b/node_modules/lodash/_baseToString.js
@@ -0,0 +1,37 @@
+var Symbol = require('./_Symbol'),
+ arrayMap = require('./_arrayMap'),
+ isArray = require('./isArray'),
+ isSymbol = require('./isSymbol');
+
+/** Used as references for various `Number` constants. */
+var INFINITY = 1 / 0;
+
+/** Used to convert symbols to primitives and strings. */
+var symbolProto = Symbol ? Symbol.prototype : undefined,
+ symbolToString = symbolProto ? symbolProto.toString : undefined;
+
+/**
+ * The base implementation of `_.toString` which doesn't convert nullish
+ * values to empty strings.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {string} Returns the string.
+ */
+function baseToString(value) {
+ // Exit early for strings to avoid a performance hit in some environments.
+ if (typeof value == 'string') {
+ return value;
+ }
+ if (isArray(value)) {
+ // Recursively convert values (susceptible to call stack limits).
+ return arrayMap(value, baseToString) + '';
+ }
+ if (isSymbol(value)) {
+ return symbolToString ? symbolToString.call(value) : '';
+ }
+ var result = (value + '');
+ return (result == '0' && (1 / value) == -INFINITY) ? '-0' : result;
+}
+
+module.exports = baseToString;
diff --git a/node_modules/lodash/_baseTrim.js b/node_modules/lodash/_baseTrim.js
new file mode 100644
index 0000000..3e2797d
--- /dev/null
+++ b/node_modules/lodash/_baseTrim.js
@@ -0,0 +1,19 @@
+var trimmedEndIndex = require('./_trimmedEndIndex');
+
+/** Used to match leading whitespace. */
+var reTrimStart = /^\s+/;
+
+/**
+ * The base implementation of `_.trim`.
+ *
+ * @private
+ * @param {string} string The string to trim.
+ * @returns {string} Returns the trimmed string.
+ */
+function baseTrim(string) {
+ return string
+ ? string.slice(0, trimmedEndIndex(string) + 1).replace(reTrimStart, '')
+ : string;
+}
+
+module.exports = baseTrim;
diff --git a/node_modules/lodash/_baseUnary.js b/node_modules/lodash/_baseUnary.js
new file mode 100644
index 0000000..98639e9
--- /dev/null
+++ b/node_modules/lodash/_baseUnary.js
@@ -0,0 +1,14 @@
+/**
+ * The base implementation of `_.unary` without support for storing metadata.
+ *
+ * @private
+ * @param {Function} func The function to cap arguments for.
+ * @returns {Function} Returns the new capped function.
+ */
+function baseUnary(func) {
+ return function(value) {
+ return func(value);
+ };
+}
+
+module.exports = baseUnary;
diff --git a/node_modules/lodash/_baseUniq.js b/node_modules/lodash/_baseUniq.js
new file mode 100644
index 0000000..aea459d
--- /dev/null
+++ b/node_modules/lodash/_baseUniq.js
@@ -0,0 +1,72 @@
+var SetCache = require('./_SetCache'),
+ arrayIncludes = require('./_arrayIncludes'),
+ arrayIncludesWith = require('./_arrayIncludesWith'),
+ cacheHas = require('./_cacheHas'),
+ createSet = require('./_createSet'),
+ setToArray = require('./_setToArray');
+
+/** Used as the size to enable large array optimizations. */
+var LARGE_ARRAY_SIZE = 200;
+
+/**
+ * The base implementation of `_.uniqBy` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new duplicate free array.
+ */
+function baseUniq(array, iteratee, comparator) {
+ var index = -1,
+ includes = arrayIncludes,
+ length = array.length,
+ isCommon = true,
+ result = [],
+ seen = result;
+
+ if (comparator) {
+ isCommon = false;
+ includes = arrayIncludesWith;
+ }
+ else if (length >= LARGE_ARRAY_SIZE) {
+ var set = iteratee ? null : createSet(array);
+ if (set) {
+ return setToArray(set);
+ }
+ isCommon = false;
+ includes = cacheHas;
+ seen = new SetCache;
+ }
+ else {
+ seen = iteratee ? [] : result;
+ }
+ outer:
+ while (++index < length) {
+ var value = array[index],
+ computed = iteratee ? iteratee(value) : value;
+
+ value = (comparator || value !== 0) ? value : 0;
+ if (isCommon && computed === computed) {
+ var seenIndex = seen.length;
+ while (seenIndex--) {
+ if (seen[seenIndex] === computed) {
+ continue outer;
+ }
+ }
+ if (iteratee) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ else if (!includes(seen, computed, comparator)) {
+ if (seen !== result) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = baseUniq;
diff --git a/node_modules/lodash/_baseUnset.js b/node_modules/lodash/_baseUnset.js
new file mode 100644
index 0000000..eefc6e3
--- /dev/null
+++ b/node_modules/lodash/_baseUnset.js
@@ -0,0 +1,20 @@
+var castPath = require('./_castPath'),
+ last = require('./last'),
+ parent = require('./_parent'),
+ toKey = require('./_toKey');
+
+/**
+ * The base implementation of `_.unset`.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The property path to unset.
+ * @returns {boolean} Returns `true` if the property is deleted, else `false`.
+ */
+function baseUnset(object, path) {
+ path = castPath(path, object);
+ object = parent(object, path);
+ return object == null || delete object[toKey(last(path))];
+}
+
+module.exports = baseUnset;
diff --git a/node_modules/lodash/_baseUpdate.js b/node_modules/lodash/_baseUpdate.js
new file mode 100644
index 0000000..92a6237
--- /dev/null
+++ b/node_modules/lodash/_baseUpdate.js
@@ -0,0 +1,18 @@
+var baseGet = require('./_baseGet'),
+ baseSet = require('./_baseSet');
+
+/**
+ * The base implementation of `_.update`.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The path of the property to update.
+ * @param {Function} updater The function to produce the updated value.
+ * @param {Function} [customizer] The function to customize path creation.
+ * @returns {Object} Returns `object`.
+ */
+function baseUpdate(object, path, updater, customizer) {
+ return baseSet(object, path, updater(baseGet(object, path)), customizer);
+}
+
+module.exports = baseUpdate;
diff --git a/node_modules/lodash/_baseValues.js b/node_modules/lodash/_baseValues.js
new file mode 100644
index 0000000..b95faad
--- /dev/null
+++ b/node_modules/lodash/_baseValues.js
@@ -0,0 +1,19 @@
+var arrayMap = require('./_arrayMap');
+
+/**
+ * The base implementation of `_.values` and `_.valuesIn` which creates an
+ * array of `object` property values corresponding to the property names
+ * of `props`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} props The property names to get values for.
+ * @returns {Object} Returns the array of property values.
+ */
+function baseValues(object, props) {
+ return arrayMap(props, function(key) {
+ return object[key];
+ });
+}
+
+module.exports = baseValues;
diff --git a/node_modules/lodash/_baseWhile.js b/node_modules/lodash/_baseWhile.js
new file mode 100644
index 0000000..07eac61
--- /dev/null
+++ b/node_modules/lodash/_baseWhile.js
@@ -0,0 +1,26 @@
+var baseSlice = require('./_baseSlice');
+
+/**
+ * The base implementation of methods like `_.dropWhile` and `_.takeWhile`
+ * without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to query.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {boolean} [isDrop] Specify dropping elements instead of taking them.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Array} Returns the slice of `array`.
+ */
+function baseWhile(array, predicate, isDrop, fromRight) {
+ var length = array.length,
+ index = fromRight ? length : -1;
+
+ while ((fromRight ? index-- : ++index < length) &&
+ predicate(array[index], index, array)) {}
+
+ return isDrop
+ ? baseSlice(array, (fromRight ? 0 : index), (fromRight ? index + 1 : length))
+ : baseSlice(array, (fromRight ? index + 1 : 0), (fromRight ? length : index));
+}
+
+module.exports = baseWhile;
diff --git a/node_modules/lodash/_baseWrapperValue.js b/node_modules/lodash/_baseWrapperValue.js
new file mode 100644
index 0000000..443e0df
--- /dev/null
+++ b/node_modules/lodash/_baseWrapperValue.js
@@ -0,0 +1,25 @@
+var LazyWrapper = require('./_LazyWrapper'),
+ arrayPush = require('./_arrayPush'),
+ arrayReduce = require('./_arrayReduce');
+
+/**
+ * The base implementation of `wrapperValue` which returns the result of
+ * performing a sequence of actions on the unwrapped `value`, where each
+ * successive action is supplied the return value of the previous.
+ *
+ * @private
+ * @param {*} value The unwrapped value.
+ * @param {Array} actions Actions to perform to resolve the unwrapped value.
+ * @returns {*} Returns the resolved value.
+ */
+function baseWrapperValue(value, actions) {
+ var result = value;
+ if (result instanceof LazyWrapper) {
+ result = result.value();
+ }
+ return arrayReduce(actions, function(result, action) {
+ return action.func.apply(action.thisArg, arrayPush([result], action.args));
+ }, result);
+}
+
+module.exports = baseWrapperValue;
diff --git a/node_modules/lodash/_baseXor.js b/node_modules/lodash/_baseXor.js
new file mode 100644
index 0000000..8e69338
--- /dev/null
+++ b/node_modules/lodash/_baseXor.js
@@ -0,0 +1,36 @@
+var baseDifference = require('./_baseDifference'),
+ baseFlatten = require('./_baseFlatten'),
+ baseUniq = require('./_baseUniq');
+
+/**
+ * The base implementation of methods like `_.xor`, without support for
+ * iteratee shorthands, that accepts an array of arrays to inspect.
+ *
+ * @private
+ * @param {Array} arrays The arrays to inspect.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of values.
+ */
+function baseXor(arrays, iteratee, comparator) {
+ var length = arrays.length;
+ if (length < 2) {
+ return length ? baseUniq(arrays[0]) : [];
+ }
+ var index = -1,
+ result = Array(length);
+
+ while (++index < length) {
+ var array = arrays[index],
+ othIndex = -1;
+
+ while (++othIndex < length) {
+ if (othIndex != index) {
+ result[index] = baseDifference(result[index] || array, arrays[othIndex], iteratee, comparator);
+ }
+ }
+ }
+ return baseUniq(baseFlatten(result, 1), iteratee, comparator);
+}
+
+module.exports = baseXor;
diff --git a/node_modules/lodash/_baseZipObject.js b/node_modules/lodash/_baseZipObject.js
new file mode 100644
index 0000000..401f85b
--- /dev/null
+++ b/node_modules/lodash/_baseZipObject.js
@@ -0,0 +1,23 @@
+/**
+ * This base implementation of `_.zipObject` which assigns values using `assignFunc`.
+ *
+ * @private
+ * @param {Array} props The property identifiers.
+ * @param {Array} values The property values.
+ * @param {Function} assignFunc The function to assign values.
+ * @returns {Object} Returns the new object.
+ */
+function baseZipObject(props, values, assignFunc) {
+ var index = -1,
+ length = props.length,
+ valsLength = values.length,
+ result = {};
+
+ while (++index < length) {
+ var value = index < valsLength ? values[index] : undefined;
+ assignFunc(result, props[index], value);
+ }
+ return result;
+}
+
+module.exports = baseZipObject;
diff --git a/node_modules/lodash/_cacheHas.js b/node_modules/lodash/_cacheHas.js
new file mode 100644
index 0000000..2dec892
--- /dev/null
+++ b/node_modules/lodash/_cacheHas.js
@@ -0,0 +1,13 @@
+/**
+ * Checks if a `cache` value for `key` exists.
+ *
+ * @private
+ * @param {Object} cache The cache to query.
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function cacheHas(cache, key) {
+ return cache.has(key);
+}
+
+module.exports = cacheHas;
diff --git a/node_modules/lodash/_castArrayLikeObject.js b/node_modules/lodash/_castArrayLikeObject.js
new file mode 100644
index 0000000..92c75fa
--- /dev/null
+++ b/node_modules/lodash/_castArrayLikeObject.js
@@ -0,0 +1,14 @@
+var isArrayLikeObject = require('./isArrayLikeObject');
+
+/**
+ * Casts `value` to an empty array if it's not an array like object.
+ *
+ * @private
+ * @param {*} value The value to inspect.
+ * @returns {Array|Object} Returns the cast array-like object.
+ */
+function castArrayLikeObject(value) {
+ return isArrayLikeObject(value) ? value : [];
+}
+
+module.exports = castArrayLikeObject;
diff --git a/node_modules/lodash/_castFunction.js b/node_modules/lodash/_castFunction.js
new file mode 100644
index 0000000..98c91ae
--- /dev/null
+++ b/node_modules/lodash/_castFunction.js
@@ -0,0 +1,14 @@
+var identity = require('./identity');
+
+/**
+ * Casts `value` to `identity` if it's not a function.
+ *
+ * @private
+ * @param {*} value The value to inspect.
+ * @returns {Function} Returns cast function.
+ */
+function castFunction(value) {
+ return typeof value == 'function' ? value : identity;
+}
+
+module.exports = castFunction;
diff --git a/node_modules/lodash/_castPath.js b/node_modules/lodash/_castPath.js
new file mode 100644
index 0000000..017e4c1
--- /dev/null
+++ b/node_modules/lodash/_castPath.js
@@ -0,0 +1,21 @@
+var isArray = require('./isArray'),
+ isKey = require('./_isKey'),
+ stringToPath = require('./_stringToPath'),
+ toString = require('./toString');
+
+/**
+ * Casts `value` to a path array if it's not one.
+ *
+ * @private
+ * @param {*} value The value to inspect.
+ * @param {Object} [object] The object to query keys on.
+ * @returns {Array} Returns the cast property path array.
+ */
+function castPath(value, object) {
+ if (isArray(value)) {
+ return value;
+ }
+ return isKey(value, object) ? [value] : stringToPath(toString(value));
+}
+
+module.exports = castPath;
diff --git a/node_modules/lodash/_castRest.js b/node_modules/lodash/_castRest.js
new file mode 100644
index 0000000..213c66f
--- /dev/null
+++ b/node_modules/lodash/_castRest.js
@@ -0,0 +1,14 @@
+var baseRest = require('./_baseRest');
+
+/**
+ * A `baseRest` alias which can be replaced with `identity` by module
+ * replacement plugins.
+ *
+ * @private
+ * @type {Function}
+ * @param {Function} func The function to apply a rest parameter to.
+ * @returns {Function} Returns the new function.
+ */
+var castRest = baseRest;
+
+module.exports = castRest;
diff --git a/node_modules/lodash/_castSlice.js b/node_modules/lodash/_castSlice.js
new file mode 100644
index 0000000..071faeb
--- /dev/null
+++ b/node_modules/lodash/_castSlice.js
@@ -0,0 +1,18 @@
+var baseSlice = require('./_baseSlice');
+
+/**
+ * Casts `array` to a slice if it's needed.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {number} start The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the cast slice.
+ */
+function castSlice(array, start, end) {
+ var length = array.length;
+ end = end === undefined ? length : end;
+ return (!start && end >= length) ? array : baseSlice(array, start, end);
+}
+
+module.exports = castSlice;
diff --git a/node_modules/lodash/_charsEndIndex.js b/node_modules/lodash/_charsEndIndex.js
new file mode 100644
index 0000000..07908ff
--- /dev/null
+++ b/node_modules/lodash/_charsEndIndex.js
@@ -0,0 +1,19 @@
+var baseIndexOf = require('./_baseIndexOf');
+
+/**
+ * Used by `_.trim` and `_.trimEnd` to get the index of the last string symbol
+ * that is not found in the character symbols.
+ *
+ * @private
+ * @param {Array} strSymbols The string symbols to inspect.
+ * @param {Array} chrSymbols The character symbols to find.
+ * @returns {number} Returns the index of the last unmatched string symbol.
+ */
+function charsEndIndex(strSymbols, chrSymbols) {
+ var index = strSymbols.length;
+
+ while (index-- && baseIndexOf(chrSymbols, strSymbols[index], 0) > -1) {}
+ return index;
+}
+
+module.exports = charsEndIndex;
diff --git a/node_modules/lodash/_charsStartIndex.js b/node_modules/lodash/_charsStartIndex.js
new file mode 100644
index 0000000..b17afd2
--- /dev/null
+++ b/node_modules/lodash/_charsStartIndex.js
@@ -0,0 +1,20 @@
+var baseIndexOf = require('./_baseIndexOf');
+
+/**
+ * Used by `_.trim` and `_.trimStart` to get the index of the first string symbol
+ * that is not found in the character symbols.
+ *
+ * @private
+ * @param {Array} strSymbols The string symbols to inspect.
+ * @param {Array} chrSymbols The character symbols to find.
+ * @returns {number} Returns the index of the first unmatched string symbol.
+ */
+function charsStartIndex(strSymbols, chrSymbols) {
+ var index = -1,
+ length = strSymbols.length;
+
+ while (++index < length && baseIndexOf(chrSymbols, strSymbols[index], 0) > -1) {}
+ return index;
+}
+
+module.exports = charsStartIndex;
diff --git a/node_modules/lodash/_cloneArrayBuffer.js b/node_modules/lodash/_cloneArrayBuffer.js
new file mode 100644
index 0000000..c3d8f6e
--- /dev/null
+++ b/node_modules/lodash/_cloneArrayBuffer.js
@@ -0,0 +1,16 @@
+var Uint8Array = require('./_Uint8Array');
+
+/**
+ * Creates a clone of `arrayBuffer`.
+ *
+ * @private
+ * @param {ArrayBuffer} arrayBuffer The array buffer to clone.
+ * @returns {ArrayBuffer} Returns the cloned array buffer.
+ */
+function cloneArrayBuffer(arrayBuffer) {
+ var result = new arrayBuffer.constructor(arrayBuffer.byteLength);
+ new Uint8Array(result).set(new Uint8Array(arrayBuffer));
+ return result;
+}
+
+module.exports = cloneArrayBuffer;
diff --git a/node_modules/lodash/_cloneBuffer.js b/node_modules/lodash/_cloneBuffer.js
new file mode 100644
index 0000000..27c4810
--- /dev/null
+++ b/node_modules/lodash/_cloneBuffer.js
@@ -0,0 +1,35 @@
+var root = require('./_root');
+
+/** Detect free variable `exports`. */
+var freeExports = typeof exports == 'object' && exports && !exports.nodeType && exports;
+
+/** Detect free variable `module`. */
+var freeModule = freeExports && typeof module == 'object' && module && !module.nodeType && module;
+
+/** Detect the popular CommonJS extension `module.exports`. */
+var moduleExports = freeModule && freeModule.exports === freeExports;
+
+/** Built-in value references. */
+var Buffer = moduleExports ? root.Buffer : undefined,
+ allocUnsafe = Buffer ? Buffer.allocUnsafe : undefined;
+
+/**
+ * Creates a clone of `buffer`.
+ *
+ * @private
+ * @param {Buffer} buffer The buffer to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Buffer} Returns the cloned buffer.
+ */
+function cloneBuffer(buffer, isDeep) {
+ if (isDeep) {
+ return buffer.slice();
+ }
+ var length = buffer.length,
+ result = allocUnsafe ? allocUnsafe(length) : new buffer.constructor(length);
+
+ buffer.copy(result);
+ return result;
+}
+
+module.exports = cloneBuffer;
diff --git a/node_modules/lodash/_cloneDataView.js b/node_modules/lodash/_cloneDataView.js
new file mode 100644
index 0000000..9c9b7b0
--- /dev/null
+++ b/node_modules/lodash/_cloneDataView.js
@@ -0,0 +1,16 @@
+var cloneArrayBuffer = require('./_cloneArrayBuffer');
+
+/**
+ * Creates a clone of `dataView`.
+ *
+ * @private
+ * @param {Object} dataView The data view to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the cloned data view.
+ */
+function cloneDataView(dataView, isDeep) {
+ var buffer = isDeep ? cloneArrayBuffer(dataView.buffer) : dataView.buffer;
+ return new dataView.constructor(buffer, dataView.byteOffset, dataView.byteLength);
+}
+
+module.exports = cloneDataView;
diff --git a/node_modules/lodash/_cloneRegExp.js b/node_modules/lodash/_cloneRegExp.js
new file mode 100644
index 0000000..64a30df
--- /dev/null
+++ b/node_modules/lodash/_cloneRegExp.js
@@ -0,0 +1,17 @@
+/** Used to match `RegExp` flags from their coerced string values. */
+var reFlags = /\w*$/;
+
+/**
+ * Creates a clone of `regexp`.
+ *
+ * @private
+ * @param {Object} regexp The regexp to clone.
+ * @returns {Object} Returns the cloned regexp.
+ */
+function cloneRegExp(regexp) {
+ var result = new regexp.constructor(regexp.source, reFlags.exec(regexp));
+ result.lastIndex = regexp.lastIndex;
+ return result;
+}
+
+module.exports = cloneRegExp;
diff --git a/node_modules/lodash/_cloneSymbol.js b/node_modules/lodash/_cloneSymbol.js
new file mode 100644
index 0000000..bede39f
--- /dev/null
+++ b/node_modules/lodash/_cloneSymbol.js
@@ -0,0 +1,18 @@
+var Symbol = require('./_Symbol');
+
+/** Used to convert symbols to primitives and strings. */
+var symbolProto = Symbol ? Symbol.prototype : undefined,
+ symbolValueOf = symbolProto ? symbolProto.valueOf : undefined;
+
+/**
+ * Creates a clone of the `symbol` object.
+ *
+ * @private
+ * @param {Object} symbol The symbol object to clone.
+ * @returns {Object} Returns the cloned symbol object.
+ */
+function cloneSymbol(symbol) {
+ return symbolValueOf ? Object(symbolValueOf.call(symbol)) : {};
+}
+
+module.exports = cloneSymbol;
diff --git a/node_modules/lodash/_cloneTypedArray.js b/node_modules/lodash/_cloneTypedArray.js
new file mode 100644
index 0000000..7aad84d
--- /dev/null
+++ b/node_modules/lodash/_cloneTypedArray.js
@@ -0,0 +1,16 @@
+var cloneArrayBuffer = require('./_cloneArrayBuffer');
+
+/**
+ * Creates a clone of `typedArray`.
+ *
+ * @private
+ * @param {Object} typedArray The typed array to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the cloned typed array.
+ */
+function cloneTypedArray(typedArray, isDeep) {
+ var buffer = isDeep ? cloneArrayBuffer(typedArray.buffer) : typedArray.buffer;
+ return new typedArray.constructor(buffer, typedArray.byteOffset, typedArray.length);
+}
+
+module.exports = cloneTypedArray;
diff --git a/node_modules/lodash/_compareAscending.js b/node_modules/lodash/_compareAscending.js
new file mode 100644
index 0000000..8dc2791
--- /dev/null
+++ b/node_modules/lodash/_compareAscending.js
@@ -0,0 +1,41 @@
+var isSymbol = require('./isSymbol');
+
+/**
+ * Compares values to sort them in ascending order.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {number} Returns the sort order indicator for `value`.
+ */
+function compareAscending(value, other) {
+ if (value !== other) {
+ var valIsDefined = value !== undefined,
+ valIsNull = value === null,
+ valIsReflexive = value === value,
+ valIsSymbol = isSymbol(value);
+
+ var othIsDefined = other !== undefined,
+ othIsNull = other === null,
+ othIsReflexive = other === other,
+ othIsSymbol = isSymbol(other);
+
+ if ((!othIsNull && !othIsSymbol && !valIsSymbol && value > other) ||
+ (valIsSymbol && othIsDefined && othIsReflexive && !othIsNull && !othIsSymbol) ||
+ (valIsNull && othIsDefined && othIsReflexive) ||
+ (!valIsDefined && othIsReflexive) ||
+ !valIsReflexive) {
+ return 1;
+ }
+ if ((!valIsNull && !valIsSymbol && !othIsSymbol && value < other) ||
+ (othIsSymbol && valIsDefined && valIsReflexive && !valIsNull && !valIsSymbol) ||
+ (othIsNull && valIsDefined && valIsReflexive) ||
+ (!othIsDefined && valIsReflexive) ||
+ !othIsReflexive) {
+ return -1;
+ }
+ }
+ return 0;
+}
+
+module.exports = compareAscending;
diff --git a/node_modules/lodash/_compareMultiple.js b/node_modules/lodash/_compareMultiple.js
new file mode 100644
index 0000000..ad61f0f
--- /dev/null
+++ b/node_modules/lodash/_compareMultiple.js
@@ -0,0 +1,44 @@
+var compareAscending = require('./_compareAscending');
+
+/**
+ * Used by `_.orderBy` to compare multiple properties of a value to another
+ * and stable sort them.
+ *
+ * If `orders` is unspecified, all values are sorted in ascending order. Otherwise,
+ * specify an order of "desc" for descending or "asc" for ascending sort order
+ * of corresponding values.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {boolean[]|string[]} orders The order to sort by for each property.
+ * @returns {number} Returns the sort order indicator for `object`.
+ */
+function compareMultiple(object, other, orders) {
+ var index = -1,
+ objCriteria = object.criteria,
+ othCriteria = other.criteria,
+ length = objCriteria.length,
+ ordersLength = orders.length;
+
+ while (++index < length) {
+ var result = compareAscending(objCriteria[index], othCriteria[index]);
+ if (result) {
+ if (index >= ordersLength) {
+ return result;
+ }
+ var order = orders[index];
+ return result * (order == 'desc' ? -1 : 1);
+ }
+ }
+ // Fixes an `Array#sort` bug in the JS engine embedded in Adobe applications
+ // that causes it, under certain circumstances, to provide the same value for
+ // `object` and `other`. See https://github.com/jashkenas/underscore/pull/1247
+ // for more details.
+ //
+ // This also ensures a stable sort in V8 and other engines.
+ // See https://bugs.chromium.org/p/v8/issues/detail?id=90 for more details.
+ return object.index - other.index;
+}
+
+module.exports = compareMultiple;
diff --git a/node_modules/lodash/_composeArgs.js b/node_modules/lodash/_composeArgs.js
new file mode 100644
index 0000000..1ce40f4
--- /dev/null
+++ b/node_modules/lodash/_composeArgs.js
@@ -0,0 +1,39 @@
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates an array that is the composition of partially applied arguments,
+ * placeholders, and provided arguments into a single array of arguments.
+ *
+ * @private
+ * @param {Array} args The provided arguments.
+ * @param {Array} partials The arguments to prepend to those provided.
+ * @param {Array} holders The `partials` placeholder indexes.
+ * @params {boolean} [isCurried] Specify composing for a curried function.
+ * @returns {Array} Returns the new array of composed arguments.
+ */
+function composeArgs(args, partials, holders, isCurried) {
+ var argsIndex = -1,
+ argsLength = args.length,
+ holdersLength = holders.length,
+ leftIndex = -1,
+ leftLength = partials.length,
+ rangeLength = nativeMax(argsLength - holdersLength, 0),
+ result = Array(leftLength + rangeLength),
+ isUncurried = !isCurried;
+
+ while (++leftIndex < leftLength) {
+ result[leftIndex] = partials[leftIndex];
+ }
+ while (++argsIndex < holdersLength) {
+ if (isUncurried || argsIndex < argsLength) {
+ result[holders[argsIndex]] = args[argsIndex];
+ }
+ }
+ while (rangeLength--) {
+ result[leftIndex++] = args[argsIndex++];
+ }
+ return result;
+}
+
+module.exports = composeArgs;
diff --git a/node_modules/lodash/_composeArgsRight.js b/node_modules/lodash/_composeArgsRight.js
new file mode 100644
index 0000000..8dc588d
--- /dev/null
+++ b/node_modules/lodash/_composeArgsRight.js
@@ -0,0 +1,41 @@
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * This function is like `composeArgs` except that the arguments composition
+ * is tailored for `_.partialRight`.
+ *
+ * @private
+ * @param {Array} args The provided arguments.
+ * @param {Array} partials The arguments to append to those provided.
+ * @param {Array} holders The `partials` placeholder indexes.
+ * @params {boolean} [isCurried] Specify composing for a curried function.
+ * @returns {Array} Returns the new array of composed arguments.
+ */
+function composeArgsRight(args, partials, holders, isCurried) {
+ var argsIndex = -1,
+ argsLength = args.length,
+ holdersIndex = -1,
+ holdersLength = holders.length,
+ rightIndex = -1,
+ rightLength = partials.length,
+ rangeLength = nativeMax(argsLength - holdersLength, 0),
+ result = Array(rangeLength + rightLength),
+ isUncurried = !isCurried;
+
+ while (++argsIndex < rangeLength) {
+ result[argsIndex] = args[argsIndex];
+ }
+ var offset = argsIndex;
+ while (++rightIndex < rightLength) {
+ result[offset + rightIndex] = partials[rightIndex];
+ }
+ while (++holdersIndex < holdersLength) {
+ if (isUncurried || argsIndex < argsLength) {
+ result[offset + holders[holdersIndex]] = args[argsIndex++];
+ }
+ }
+ return result;
+}
+
+module.exports = composeArgsRight;
diff --git a/node_modules/lodash/_copyArray.js b/node_modules/lodash/_copyArray.js
new file mode 100644
index 0000000..cd94d5d
--- /dev/null
+++ b/node_modules/lodash/_copyArray.js
@@ -0,0 +1,20 @@
+/**
+ * Copies the values of `source` to `array`.
+ *
+ * @private
+ * @param {Array} source The array to copy values from.
+ * @param {Array} [array=[]] The array to copy values to.
+ * @returns {Array} Returns `array`.
+ */
+function copyArray(source, array) {
+ var index = -1,
+ length = source.length;
+
+ array || (array = Array(length));
+ while (++index < length) {
+ array[index] = source[index];
+ }
+ return array;
+}
+
+module.exports = copyArray;
diff --git a/node_modules/lodash/_copyObject.js b/node_modules/lodash/_copyObject.js
new file mode 100644
index 0000000..2f2a5c2
--- /dev/null
+++ b/node_modules/lodash/_copyObject.js
@@ -0,0 +1,40 @@
+var assignValue = require('./_assignValue'),
+ baseAssignValue = require('./_baseAssignValue');
+
+/**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property identifiers to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @param {Function} [customizer] The function to customize copied values.
+ * @returns {Object} Returns `object`.
+ */
+function copyObject(source, props, object, customizer) {
+ var isNew = !object;
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+
+ var newValue = customizer
+ ? customizer(object[key], source[key], key, object, source)
+ : undefined;
+
+ if (newValue === undefined) {
+ newValue = source[key];
+ }
+ if (isNew) {
+ baseAssignValue(object, key, newValue);
+ } else {
+ assignValue(object, key, newValue);
+ }
+ }
+ return object;
+}
+
+module.exports = copyObject;
diff --git a/node_modules/lodash/_copySymbols.js b/node_modules/lodash/_copySymbols.js
new file mode 100644
index 0000000..c35944a
--- /dev/null
+++ b/node_modules/lodash/_copySymbols.js
@@ -0,0 +1,16 @@
+var copyObject = require('./_copyObject'),
+ getSymbols = require('./_getSymbols');
+
+/**
+ * Copies own symbols of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy symbols from.
+ * @param {Object} [object={}] The object to copy symbols to.
+ * @returns {Object} Returns `object`.
+ */
+function copySymbols(source, object) {
+ return copyObject(source, getSymbols(source), object);
+}
+
+module.exports = copySymbols;
diff --git a/node_modules/lodash/_copySymbolsIn.js b/node_modules/lodash/_copySymbolsIn.js
new file mode 100644
index 0000000..fdf20a7
--- /dev/null
+++ b/node_modules/lodash/_copySymbolsIn.js
@@ -0,0 +1,16 @@
+var copyObject = require('./_copyObject'),
+ getSymbolsIn = require('./_getSymbolsIn');
+
+/**
+ * Copies own and inherited symbols of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy symbols from.
+ * @param {Object} [object={}] The object to copy symbols to.
+ * @returns {Object} Returns `object`.
+ */
+function copySymbolsIn(source, object) {
+ return copyObject(source, getSymbolsIn(source), object);
+}
+
+module.exports = copySymbolsIn;
diff --git a/node_modules/lodash/_coreJsData.js b/node_modules/lodash/_coreJsData.js
new file mode 100644
index 0000000..f8e5b4e
--- /dev/null
+++ b/node_modules/lodash/_coreJsData.js
@@ -0,0 +1,6 @@
+var root = require('./_root');
+
+/** Used to detect overreaching core-js shims. */
+var coreJsData = root['__core-js_shared__'];
+
+module.exports = coreJsData;
diff --git a/node_modules/lodash/_countHolders.js b/node_modules/lodash/_countHolders.js
new file mode 100644
index 0000000..718fcda
--- /dev/null
+++ b/node_modules/lodash/_countHolders.js
@@ -0,0 +1,21 @@
+/**
+ * Gets the number of `placeholder` occurrences in `array`.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} placeholder The placeholder to search for.
+ * @returns {number} Returns the placeholder count.
+ */
+function countHolders(array, placeholder) {
+ var length = array.length,
+ result = 0;
+
+ while (length--) {
+ if (array[length] === placeholder) {
+ ++result;
+ }
+ }
+ return result;
+}
+
+module.exports = countHolders;
diff --git a/node_modules/lodash/_createAggregator.js b/node_modules/lodash/_createAggregator.js
new file mode 100644
index 0000000..0be42c4
--- /dev/null
+++ b/node_modules/lodash/_createAggregator.js
@@ -0,0 +1,23 @@
+var arrayAggregator = require('./_arrayAggregator'),
+ baseAggregator = require('./_baseAggregator'),
+ baseIteratee = require('./_baseIteratee'),
+ isArray = require('./isArray');
+
+/**
+ * Creates a function like `_.groupBy`.
+ *
+ * @private
+ * @param {Function} setter The function to set accumulator values.
+ * @param {Function} [initializer] The accumulator object initializer.
+ * @returns {Function} Returns the new aggregator function.
+ */
+function createAggregator(setter, initializer) {
+ return function(collection, iteratee) {
+ var func = isArray(collection) ? arrayAggregator : baseAggregator,
+ accumulator = initializer ? initializer() : {};
+
+ return func(collection, setter, baseIteratee(iteratee, 2), accumulator);
+ };
+}
+
+module.exports = createAggregator;
diff --git a/node_modules/lodash/_createAssigner.js b/node_modules/lodash/_createAssigner.js
new file mode 100644
index 0000000..1f904c5
--- /dev/null
+++ b/node_modules/lodash/_createAssigner.js
@@ -0,0 +1,37 @@
+var baseRest = require('./_baseRest'),
+ isIterateeCall = require('./_isIterateeCall');
+
+/**
+ * Creates a function like `_.assign`.
+ *
+ * @private
+ * @param {Function} assigner The function to assign values.
+ * @returns {Function} Returns the new assigner function.
+ */
+function createAssigner(assigner) {
+ return baseRest(function(object, sources) {
+ var index = -1,
+ length = sources.length,
+ customizer = length > 1 ? sources[length - 1] : undefined,
+ guard = length > 2 ? sources[2] : undefined;
+
+ customizer = (assigner.length > 3 && typeof customizer == 'function')
+ ? (length--, customizer)
+ : undefined;
+
+ if (guard && isIterateeCall(sources[0], sources[1], guard)) {
+ customizer = length < 3 ? undefined : customizer;
+ length = 1;
+ }
+ object = Object(object);
+ while (++index < length) {
+ var source = sources[index];
+ if (source) {
+ assigner(object, source, index, customizer);
+ }
+ }
+ return object;
+ });
+}
+
+module.exports = createAssigner;
diff --git a/node_modules/lodash/_createBaseEach.js b/node_modules/lodash/_createBaseEach.js
new file mode 100644
index 0000000..d24fdd1
--- /dev/null
+++ b/node_modules/lodash/_createBaseEach.js
@@ -0,0 +1,32 @@
+var isArrayLike = require('./isArrayLike');
+
+/**
+ * Creates a `baseEach` or `baseEachRight` function.
+ *
+ * @private
+ * @param {Function} eachFunc The function to iterate over a collection.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+function createBaseEach(eachFunc, fromRight) {
+ return function(collection, iteratee) {
+ if (collection == null) {
+ return collection;
+ }
+ if (!isArrayLike(collection)) {
+ return eachFunc(collection, iteratee);
+ }
+ var length = collection.length,
+ index = fromRight ? length : -1,
+ iterable = Object(collection);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ if (iteratee(iterable[index], index, iterable) === false) {
+ break;
+ }
+ }
+ return collection;
+ };
+}
+
+module.exports = createBaseEach;
diff --git a/node_modules/lodash/_createBaseFor.js b/node_modules/lodash/_createBaseFor.js
new file mode 100644
index 0000000..94cbf29
--- /dev/null
+++ b/node_modules/lodash/_createBaseFor.js
@@ -0,0 +1,25 @@
+/**
+ * Creates a base function for methods like `_.forIn` and `_.forOwn`.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+function createBaseFor(fromRight) {
+ return function(object, iteratee, keysFunc) {
+ var index = -1,
+ iterable = Object(object),
+ props = keysFunc(object),
+ length = props.length;
+
+ while (length--) {
+ var key = props[fromRight ? length : ++index];
+ if (iteratee(iterable[key], key, iterable) === false) {
+ break;
+ }
+ }
+ return object;
+ };
+}
+
+module.exports = createBaseFor;
diff --git a/node_modules/lodash/_createBind.js b/node_modules/lodash/_createBind.js
new file mode 100644
index 0000000..07cb99f
--- /dev/null
+++ b/node_modules/lodash/_createBind.js
@@ -0,0 +1,28 @@
+var createCtor = require('./_createCtor'),
+ root = require('./_root');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_BIND_FLAG = 1;
+
+/**
+ * Creates a function that wraps `func` to invoke it with the optional `this`
+ * binding of `thisArg`.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+function createBind(func, bitmask, thisArg) {
+ var isBind = bitmask & WRAP_BIND_FLAG,
+ Ctor = createCtor(func);
+
+ function wrapper() {
+ var fn = (this && this !== root && this instanceof wrapper) ? Ctor : func;
+ return fn.apply(isBind ? thisArg : this, arguments);
+ }
+ return wrapper;
+}
+
+module.exports = createBind;
diff --git a/node_modules/lodash/_createCaseFirst.js b/node_modules/lodash/_createCaseFirst.js
new file mode 100644
index 0000000..fe8ea48
--- /dev/null
+++ b/node_modules/lodash/_createCaseFirst.js
@@ -0,0 +1,33 @@
+var castSlice = require('./_castSlice'),
+ hasUnicode = require('./_hasUnicode'),
+ stringToArray = require('./_stringToArray'),
+ toString = require('./toString');
+
+/**
+ * Creates a function like `_.lowerFirst`.
+ *
+ * @private
+ * @param {string} methodName The name of the `String` case method to use.
+ * @returns {Function} Returns the new case function.
+ */
+function createCaseFirst(methodName) {
+ return function(string) {
+ string = toString(string);
+
+ var strSymbols = hasUnicode(string)
+ ? stringToArray(string)
+ : undefined;
+
+ var chr = strSymbols
+ ? strSymbols[0]
+ : string.charAt(0);
+
+ var trailing = strSymbols
+ ? castSlice(strSymbols, 1).join('')
+ : string.slice(1);
+
+ return chr[methodName]() + trailing;
+ };
+}
+
+module.exports = createCaseFirst;
diff --git a/node_modules/lodash/_createCompounder.js b/node_modules/lodash/_createCompounder.js
new file mode 100644
index 0000000..8d4cee2
--- /dev/null
+++ b/node_modules/lodash/_createCompounder.js
@@ -0,0 +1,24 @@
+var arrayReduce = require('./_arrayReduce'),
+ deburr = require('./deburr'),
+ words = require('./words');
+
+/** Used to compose unicode capture groups. */
+var rsApos = "['\u2019]";
+
+/** Used to match apostrophes. */
+var reApos = RegExp(rsApos, 'g');
+
+/**
+ * Creates a function like `_.camelCase`.
+ *
+ * @private
+ * @param {Function} callback The function to combine each word.
+ * @returns {Function} Returns the new compounder function.
+ */
+function createCompounder(callback) {
+ return function(string) {
+ return arrayReduce(words(deburr(string).replace(reApos, '')), callback, '');
+ };
+}
+
+module.exports = createCompounder;
diff --git a/node_modules/lodash/_createCtor.js b/node_modules/lodash/_createCtor.js
new file mode 100644
index 0000000..9047aa5
--- /dev/null
+++ b/node_modules/lodash/_createCtor.js
@@ -0,0 +1,37 @@
+var baseCreate = require('./_baseCreate'),
+ isObject = require('./isObject');
+
+/**
+ * Creates a function that produces an instance of `Ctor` regardless of
+ * whether it was invoked as part of a `new` expression or by `call` or `apply`.
+ *
+ * @private
+ * @param {Function} Ctor The constructor to wrap.
+ * @returns {Function} Returns the new wrapped function.
+ */
+function createCtor(Ctor) {
+ return function() {
+ // Use a `switch` statement to work with class constructors. See
+ // http://ecma-international.org/ecma-262/7.0/#sec-ecmascript-function-objects-call-thisargument-argumentslist
+ // for more details.
+ var args = arguments;
+ switch (args.length) {
+ case 0: return new Ctor;
+ case 1: return new Ctor(args[0]);
+ case 2: return new Ctor(args[0], args[1]);
+ case 3: return new Ctor(args[0], args[1], args[2]);
+ case 4: return new Ctor(args[0], args[1], args[2], args[3]);
+ case 5: return new Ctor(args[0], args[1], args[2], args[3], args[4]);
+ case 6: return new Ctor(args[0], args[1], args[2], args[3], args[4], args[5]);
+ case 7: return new Ctor(args[0], args[1], args[2], args[3], args[4], args[5], args[6]);
+ }
+ var thisBinding = baseCreate(Ctor.prototype),
+ result = Ctor.apply(thisBinding, args);
+
+ // Mimic the constructor's `return` behavior.
+ // See https://es5.github.io/#x13.2.2 for more details.
+ return isObject(result) ? result : thisBinding;
+ };
+}
+
+module.exports = createCtor;
diff --git a/node_modules/lodash/_createCurry.js b/node_modules/lodash/_createCurry.js
new file mode 100644
index 0000000..f06c2cd
--- /dev/null
+++ b/node_modules/lodash/_createCurry.js
@@ -0,0 +1,46 @@
+var apply = require('./_apply'),
+ createCtor = require('./_createCtor'),
+ createHybrid = require('./_createHybrid'),
+ createRecurry = require('./_createRecurry'),
+ getHolder = require('./_getHolder'),
+ replaceHolders = require('./_replaceHolders'),
+ root = require('./_root');
+
+/**
+ * Creates a function that wraps `func` to enable currying.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {number} arity The arity of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+function createCurry(func, bitmask, arity) {
+ var Ctor = createCtor(func);
+
+ function wrapper() {
+ var length = arguments.length,
+ args = Array(length),
+ index = length,
+ placeholder = getHolder(wrapper);
+
+ while (index--) {
+ args[index] = arguments[index];
+ }
+ var holders = (length < 3 && args[0] !== placeholder && args[length - 1] !== placeholder)
+ ? []
+ : replaceHolders(args, placeholder);
+
+ length -= holders.length;
+ if (length < arity) {
+ return createRecurry(
+ func, bitmask, createHybrid, wrapper.placeholder, undefined,
+ args, holders, undefined, undefined, arity - length);
+ }
+ var fn = (this && this !== root && this instanceof wrapper) ? Ctor : func;
+ return apply(fn, this, args);
+ }
+ return wrapper;
+}
+
+module.exports = createCurry;
diff --git a/node_modules/lodash/_createFind.js b/node_modules/lodash/_createFind.js
new file mode 100644
index 0000000..8859ff8
--- /dev/null
+++ b/node_modules/lodash/_createFind.js
@@ -0,0 +1,25 @@
+var baseIteratee = require('./_baseIteratee'),
+ isArrayLike = require('./isArrayLike'),
+ keys = require('./keys');
+
+/**
+ * Creates a `_.find` or `_.findLast` function.
+ *
+ * @private
+ * @param {Function} findIndexFunc The function to find the collection index.
+ * @returns {Function} Returns the new find function.
+ */
+function createFind(findIndexFunc) {
+ return function(collection, predicate, fromIndex) {
+ var iterable = Object(collection);
+ if (!isArrayLike(collection)) {
+ var iteratee = baseIteratee(predicate, 3);
+ collection = keys(collection);
+ predicate = function(key) { return iteratee(iterable[key], key, iterable); };
+ }
+ var index = findIndexFunc(collection, predicate, fromIndex);
+ return index > -1 ? iterable[iteratee ? collection[index] : index] : undefined;
+ };
+}
+
+module.exports = createFind;
diff --git a/node_modules/lodash/_createFlow.js b/node_modules/lodash/_createFlow.js
new file mode 100644
index 0000000..baaddbf
--- /dev/null
+++ b/node_modules/lodash/_createFlow.js
@@ -0,0 +1,78 @@
+var LodashWrapper = require('./_LodashWrapper'),
+ flatRest = require('./_flatRest'),
+ getData = require('./_getData'),
+ getFuncName = require('./_getFuncName'),
+ isArray = require('./isArray'),
+ isLaziable = require('./_isLaziable');
+
+/** Error message constants. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_CURRY_FLAG = 8,
+ WRAP_PARTIAL_FLAG = 32,
+ WRAP_ARY_FLAG = 128,
+ WRAP_REARG_FLAG = 256;
+
+/**
+ * Creates a `_.flow` or `_.flowRight` function.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new flow function.
+ */
+function createFlow(fromRight) {
+ return flatRest(function(funcs) {
+ var length = funcs.length,
+ index = length,
+ prereq = LodashWrapper.prototype.thru;
+
+ if (fromRight) {
+ funcs.reverse();
+ }
+ while (index--) {
+ var func = funcs[index];
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ if (prereq && !wrapper && getFuncName(func) == 'wrapper') {
+ var wrapper = new LodashWrapper([], true);
+ }
+ }
+ index = wrapper ? index : length;
+ while (++index < length) {
+ func = funcs[index];
+
+ var funcName = getFuncName(func),
+ data = funcName == 'wrapper' ? getData(func) : undefined;
+
+ if (data && isLaziable(data[0]) &&
+ data[1] == (WRAP_ARY_FLAG | WRAP_CURRY_FLAG | WRAP_PARTIAL_FLAG | WRAP_REARG_FLAG) &&
+ !data[4].length && data[9] == 1
+ ) {
+ wrapper = wrapper[getFuncName(data[0])].apply(wrapper, data[3]);
+ } else {
+ wrapper = (func.length == 1 && isLaziable(func))
+ ? wrapper[funcName]()
+ : wrapper.thru(func);
+ }
+ }
+ return function() {
+ var args = arguments,
+ value = args[0];
+
+ if (wrapper && args.length == 1 && isArray(value)) {
+ return wrapper.plant(value).value();
+ }
+ var index = 0,
+ result = length ? funcs[index].apply(this, args) : value;
+
+ while (++index < length) {
+ result = funcs[index].call(this, result);
+ }
+ return result;
+ };
+ });
+}
+
+module.exports = createFlow;
diff --git a/node_modules/lodash/_createHybrid.js b/node_modules/lodash/_createHybrid.js
new file mode 100644
index 0000000..b671bd1
--- /dev/null
+++ b/node_modules/lodash/_createHybrid.js
@@ -0,0 +1,92 @@
+var composeArgs = require('./_composeArgs'),
+ composeArgsRight = require('./_composeArgsRight'),
+ countHolders = require('./_countHolders'),
+ createCtor = require('./_createCtor'),
+ createRecurry = require('./_createRecurry'),
+ getHolder = require('./_getHolder'),
+ reorder = require('./_reorder'),
+ replaceHolders = require('./_replaceHolders'),
+ root = require('./_root');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_BIND_FLAG = 1,
+ WRAP_BIND_KEY_FLAG = 2,
+ WRAP_CURRY_FLAG = 8,
+ WRAP_CURRY_RIGHT_FLAG = 16,
+ WRAP_ARY_FLAG = 128,
+ WRAP_FLIP_FLAG = 512;
+
+/**
+ * Creates a function that wraps `func` to invoke it with optional `this`
+ * binding of `thisArg`, partial application, and currying.
+ *
+ * @private
+ * @param {Function|string} func The function or method name to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {Array} [partials] The arguments to prepend to those provided to
+ * the new function.
+ * @param {Array} [holders] The `partials` placeholder indexes.
+ * @param {Array} [partialsRight] The arguments to append to those provided
+ * to the new function.
+ * @param {Array} [holdersRight] The `partialsRight` placeholder indexes.
+ * @param {Array} [argPos] The argument positions of the new function.
+ * @param {number} [ary] The arity cap of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+function createHybrid(func, bitmask, thisArg, partials, holders, partialsRight, holdersRight, argPos, ary, arity) {
+ var isAry = bitmask & WRAP_ARY_FLAG,
+ isBind = bitmask & WRAP_BIND_FLAG,
+ isBindKey = bitmask & WRAP_BIND_KEY_FLAG,
+ isCurried = bitmask & (WRAP_CURRY_FLAG | WRAP_CURRY_RIGHT_FLAG),
+ isFlip = bitmask & WRAP_FLIP_FLAG,
+ Ctor = isBindKey ? undefined : createCtor(func);
+
+ function wrapper() {
+ var length = arguments.length,
+ args = Array(length),
+ index = length;
+
+ while (index--) {
+ args[index] = arguments[index];
+ }
+ if (isCurried) {
+ var placeholder = getHolder(wrapper),
+ holdersCount = countHolders(args, placeholder);
+ }
+ if (partials) {
+ args = composeArgs(args, partials, holders, isCurried);
+ }
+ if (partialsRight) {
+ args = composeArgsRight(args, partialsRight, holdersRight, isCurried);
+ }
+ length -= holdersCount;
+ if (isCurried && length < arity) {
+ var newHolders = replaceHolders(args, placeholder);
+ return createRecurry(
+ func, bitmask, createHybrid, wrapper.placeholder, thisArg,
+ args, newHolders, argPos, ary, arity - length
+ );
+ }
+ var thisBinding = isBind ? thisArg : this,
+ fn = isBindKey ? thisBinding[func] : func;
+
+ length = args.length;
+ if (argPos) {
+ args = reorder(args, argPos);
+ } else if (isFlip && length > 1) {
+ args.reverse();
+ }
+ if (isAry && ary < length) {
+ args.length = ary;
+ }
+ if (this && this !== root && this instanceof wrapper) {
+ fn = Ctor || createCtor(fn);
+ }
+ return fn.apply(thisBinding, args);
+ }
+ return wrapper;
+}
+
+module.exports = createHybrid;
diff --git a/node_modules/lodash/_createInverter.js b/node_modules/lodash/_createInverter.js
new file mode 100644
index 0000000..6c0c562
--- /dev/null
+++ b/node_modules/lodash/_createInverter.js
@@ -0,0 +1,17 @@
+var baseInverter = require('./_baseInverter');
+
+/**
+ * Creates a function like `_.invertBy`.
+ *
+ * @private
+ * @param {Function} setter The function to set accumulator values.
+ * @param {Function} toIteratee The function to resolve iteratees.
+ * @returns {Function} Returns the new inverter function.
+ */
+function createInverter(setter, toIteratee) {
+ return function(object, iteratee) {
+ return baseInverter(object, setter, toIteratee(iteratee), {});
+ };
+}
+
+module.exports = createInverter;
diff --git a/node_modules/lodash/_createMathOperation.js b/node_modules/lodash/_createMathOperation.js
new file mode 100644
index 0000000..f1e238a
--- /dev/null
+++ b/node_modules/lodash/_createMathOperation.js
@@ -0,0 +1,38 @@
+var baseToNumber = require('./_baseToNumber'),
+ baseToString = require('./_baseToString');
+
+/**
+ * Creates a function that performs a mathematical operation on two values.
+ *
+ * @private
+ * @param {Function} operator The function to perform the operation.
+ * @param {number} [defaultValue] The value used for `undefined` arguments.
+ * @returns {Function} Returns the new mathematical operation function.
+ */
+function createMathOperation(operator, defaultValue) {
+ return function(value, other) {
+ var result;
+ if (value === undefined && other === undefined) {
+ return defaultValue;
+ }
+ if (value !== undefined) {
+ result = value;
+ }
+ if (other !== undefined) {
+ if (result === undefined) {
+ return other;
+ }
+ if (typeof value == 'string' || typeof other == 'string') {
+ value = baseToString(value);
+ other = baseToString(other);
+ } else {
+ value = baseToNumber(value);
+ other = baseToNumber(other);
+ }
+ result = operator(value, other);
+ }
+ return result;
+ };
+}
+
+module.exports = createMathOperation;
diff --git a/node_modules/lodash/_createOver.js b/node_modules/lodash/_createOver.js
new file mode 100644
index 0000000..3b94551
--- /dev/null
+++ b/node_modules/lodash/_createOver.js
@@ -0,0 +1,27 @@
+var apply = require('./_apply'),
+ arrayMap = require('./_arrayMap'),
+ baseIteratee = require('./_baseIteratee'),
+ baseRest = require('./_baseRest'),
+ baseUnary = require('./_baseUnary'),
+ flatRest = require('./_flatRest');
+
+/**
+ * Creates a function like `_.over`.
+ *
+ * @private
+ * @param {Function} arrayFunc The function to iterate over iteratees.
+ * @returns {Function} Returns the new over function.
+ */
+function createOver(arrayFunc) {
+ return flatRest(function(iteratees) {
+ iteratees = arrayMap(iteratees, baseUnary(baseIteratee));
+ return baseRest(function(args) {
+ var thisArg = this;
+ return arrayFunc(iteratees, function(iteratee) {
+ return apply(iteratee, thisArg, args);
+ });
+ });
+ });
+}
+
+module.exports = createOver;
diff --git a/node_modules/lodash/_createPadding.js b/node_modules/lodash/_createPadding.js
new file mode 100644
index 0000000..2124612
--- /dev/null
+++ b/node_modules/lodash/_createPadding.js
@@ -0,0 +1,33 @@
+var baseRepeat = require('./_baseRepeat'),
+ baseToString = require('./_baseToString'),
+ castSlice = require('./_castSlice'),
+ hasUnicode = require('./_hasUnicode'),
+ stringSize = require('./_stringSize'),
+ stringToArray = require('./_stringToArray');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeCeil = Math.ceil;
+
+/**
+ * Creates the padding for `string` based on `length`. The `chars` string
+ * is truncated if the number of characters exceeds `length`.
+ *
+ * @private
+ * @param {number} length The padding length.
+ * @param {string} [chars=' '] The string used as padding.
+ * @returns {string} Returns the padding for `string`.
+ */
+function createPadding(length, chars) {
+ chars = chars === undefined ? ' ' : baseToString(chars);
+
+ var charsLength = chars.length;
+ if (charsLength < 2) {
+ return charsLength ? baseRepeat(chars, length) : chars;
+ }
+ var result = baseRepeat(chars, nativeCeil(length / stringSize(chars)));
+ return hasUnicode(chars)
+ ? castSlice(stringToArray(result), 0, length).join('')
+ : result.slice(0, length);
+}
+
+module.exports = createPadding;
diff --git a/node_modules/lodash/_createPartial.js b/node_modules/lodash/_createPartial.js
new file mode 100644
index 0000000..e16c248
--- /dev/null
+++ b/node_modules/lodash/_createPartial.js
@@ -0,0 +1,43 @@
+var apply = require('./_apply'),
+ createCtor = require('./_createCtor'),
+ root = require('./_root');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_BIND_FLAG = 1;
+
+/**
+ * Creates a function that wraps `func` to invoke it with the `this` binding
+ * of `thisArg` and `partials` prepended to the arguments it receives.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {Array} partials The arguments to prepend to those provided to
+ * the new function.
+ * @returns {Function} Returns the new wrapped function.
+ */
+function createPartial(func, bitmask, thisArg, partials) {
+ var isBind = bitmask & WRAP_BIND_FLAG,
+ Ctor = createCtor(func);
+
+ function wrapper() {
+ var argsIndex = -1,
+ argsLength = arguments.length,
+ leftIndex = -1,
+ leftLength = partials.length,
+ args = Array(leftLength + argsLength),
+ fn = (this && this !== root && this instanceof wrapper) ? Ctor : func;
+
+ while (++leftIndex < leftLength) {
+ args[leftIndex] = partials[leftIndex];
+ }
+ while (argsLength--) {
+ args[leftIndex++] = arguments[++argsIndex];
+ }
+ return apply(fn, isBind ? thisArg : this, args);
+ }
+ return wrapper;
+}
+
+module.exports = createPartial;
diff --git a/node_modules/lodash/_createRange.js b/node_modules/lodash/_createRange.js
new file mode 100644
index 0000000..9f52c77
--- /dev/null
+++ b/node_modules/lodash/_createRange.js
@@ -0,0 +1,30 @@
+var baseRange = require('./_baseRange'),
+ isIterateeCall = require('./_isIterateeCall'),
+ toFinite = require('./toFinite');
+
+/**
+ * Creates a `_.range` or `_.rangeRight` function.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new range function.
+ */
+function createRange(fromRight) {
+ return function(start, end, step) {
+ if (step && typeof step != 'number' && isIterateeCall(start, end, step)) {
+ end = step = undefined;
+ }
+ // Ensure the sign of `-0` is preserved.
+ start = toFinite(start);
+ if (end === undefined) {
+ end = start;
+ start = 0;
+ } else {
+ end = toFinite(end);
+ }
+ step = step === undefined ? (start < end ? 1 : -1) : toFinite(step);
+ return baseRange(start, end, step, fromRight);
+ };
+}
+
+module.exports = createRange;
diff --git a/node_modules/lodash/_createRecurry.js b/node_modules/lodash/_createRecurry.js
new file mode 100644
index 0000000..eb29fb2
--- /dev/null
+++ b/node_modules/lodash/_createRecurry.js
@@ -0,0 +1,56 @@
+var isLaziable = require('./_isLaziable'),
+ setData = require('./_setData'),
+ setWrapToString = require('./_setWrapToString');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_BIND_FLAG = 1,
+ WRAP_BIND_KEY_FLAG = 2,
+ WRAP_CURRY_BOUND_FLAG = 4,
+ WRAP_CURRY_FLAG = 8,
+ WRAP_PARTIAL_FLAG = 32,
+ WRAP_PARTIAL_RIGHT_FLAG = 64;
+
+/**
+ * Creates a function that wraps `func` to continue currying.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {Function} wrapFunc The function to create the `func` wrapper.
+ * @param {*} placeholder The placeholder value.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {Array} [partials] The arguments to prepend to those provided to
+ * the new function.
+ * @param {Array} [holders] The `partials` placeholder indexes.
+ * @param {Array} [argPos] The argument positions of the new function.
+ * @param {number} [ary] The arity cap of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+function createRecurry(func, bitmask, wrapFunc, placeholder, thisArg, partials, holders, argPos, ary, arity) {
+ var isCurry = bitmask & WRAP_CURRY_FLAG,
+ newHolders = isCurry ? holders : undefined,
+ newHoldersRight = isCurry ? undefined : holders,
+ newPartials = isCurry ? partials : undefined,
+ newPartialsRight = isCurry ? undefined : partials;
+
+ bitmask |= (isCurry ? WRAP_PARTIAL_FLAG : WRAP_PARTIAL_RIGHT_FLAG);
+ bitmask &= ~(isCurry ? WRAP_PARTIAL_RIGHT_FLAG : WRAP_PARTIAL_FLAG);
+
+ if (!(bitmask & WRAP_CURRY_BOUND_FLAG)) {
+ bitmask &= ~(WRAP_BIND_FLAG | WRAP_BIND_KEY_FLAG);
+ }
+ var newData = [
+ func, bitmask, thisArg, newPartials, newHolders, newPartialsRight,
+ newHoldersRight, argPos, ary, arity
+ ];
+
+ var result = wrapFunc.apply(undefined, newData);
+ if (isLaziable(func)) {
+ setData(result, newData);
+ }
+ result.placeholder = placeholder;
+ return setWrapToString(result, func, bitmask);
+}
+
+module.exports = createRecurry;
diff --git a/node_modules/lodash/_createRelationalOperation.js b/node_modules/lodash/_createRelationalOperation.js
new file mode 100644
index 0000000..a17c6b5
--- /dev/null
+++ b/node_modules/lodash/_createRelationalOperation.js
@@ -0,0 +1,20 @@
+var toNumber = require('./toNumber');
+
+/**
+ * Creates a function that performs a relational operation on two values.
+ *
+ * @private
+ * @param {Function} operator The function to perform the operation.
+ * @returns {Function} Returns the new relational operation function.
+ */
+function createRelationalOperation(operator) {
+ return function(value, other) {
+ if (!(typeof value == 'string' && typeof other == 'string')) {
+ value = toNumber(value);
+ other = toNumber(other);
+ }
+ return operator(value, other);
+ };
+}
+
+module.exports = createRelationalOperation;
diff --git a/node_modules/lodash/_createRound.js b/node_modules/lodash/_createRound.js
new file mode 100644
index 0000000..88be5df
--- /dev/null
+++ b/node_modules/lodash/_createRound.js
@@ -0,0 +1,35 @@
+var root = require('./_root'),
+ toInteger = require('./toInteger'),
+ toNumber = require('./toNumber'),
+ toString = require('./toString');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeIsFinite = root.isFinite,
+ nativeMin = Math.min;
+
+/**
+ * Creates a function like `_.round`.
+ *
+ * @private
+ * @param {string} methodName The name of the `Math` method to use when rounding.
+ * @returns {Function} Returns the new round function.
+ */
+function createRound(methodName) {
+ var func = Math[methodName];
+ return function(number, precision) {
+ number = toNumber(number);
+ precision = precision == null ? 0 : nativeMin(toInteger(precision), 292);
+ if (precision && nativeIsFinite(number)) {
+ // Shift with exponential notation to avoid floating-point issues.
+ // See [MDN](https://mdn.io/round#Examples) for more details.
+ var pair = (toString(number) + 'e').split('e'),
+ value = func(pair[0] + 'e' + (+pair[1] + precision));
+
+ pair = (toString(value) + 'e').split('e');
+ return +(pair[0] + 'e' + (+pair[1] - precision));
+ }
+ return func(number);
+ };
+}
+
+module.exports = createRound;
diff --git a/node_modules/lodash/_createSet.js b/node_modules/lodash/_createSet.js
new file mode 100644
index 0000000..0f644ee
--- /dev/null
+++ b/node_modules/lodash/_createSet.js
@@ -0,0 +1,19 @@
+var Set = require('./_Set'),
+ noop = require('./noop'),
+ setToArray = require('./_setToArray');
+
+/** Used as references for various `Number` constants. */
+var INFINITY = 1 / 0;
+
+/**
+ * Creates a set object of `values`.
+ *
+ * @private
+ * @param {Array} values The values to add to the set.
+ * @returns {Object} Returns the new set.
+ */
+var createSet = !(Set && (1 / setToArray(new Set([,-0]))[1]) == INFINITY) ? noop : function(values) {
+ return new Set(values);
+};
+
+module.exports = createSet;
diff --git a/node_modules/lodash/_createToPairs.js b/node_modules/lodash/_createToPairs.js
new file mode 100644
index 0000000..568417a
--- /dev/null
+++ b/node_modules/lodash/_createToPairs.js
@@ -0,0 +1,30 @@
+var baseToPairs = require('./_baseToPairs'),
+ getTag = require('./_getTag'),
+ mapToArray = require('./_mapToArray'),
+ setToPairs = require('./_setToPairs');
+
+/** `Object#toString` result references. */
+var mapTag = '[object Map]',
+ setTag = '[object Set]';
+
+/**
+ * Creates a `_.toPairs` or `_.toPairsIn` function.
+ *
+ * @private
+ * @param {Function} keysFunc The function to get the keys of a given object.
+ * @returns {Function} Returns the new pairs function.
+ */
+function createToPairs(keysFunc) {
+ return function(object) {
+ var tag = getTag(object);
+ if (tag == mapTag) {
+ return mapToArray(object);
+ }
+ if (tag == setTag) {
+ return setToPairs(object);
+ }
+ return baseToPairs(object, keysFunc(object));
+ };
+}
+
+module.exports = createToPairs;
diff --git a/node_modules/lodash/_createWrap.js b/node_modules/lodash/_createWrap.js
new file mode 100644
index 0000000..33f0633
--- /dev/null
+++ b/node_modules/lodash/_createWrap.js
@@ -0,0 +1,106 @@
+var baseSetData = require('./_baseSetData'),
+ createBind = require('./_createBind'),
+ createCurry = require('./_createCurry'),
+ createHybrid = require('./_createHybrid'),
+ createPartial = require('./_createPartial'),
+ getData = require('./_getData'),
+ mergeData = require('./_mergeData'),
+ setData = require('./_setData'),
+ setWrapToString = require('./_setWrapToString'),
+ toInteger = require('./toInteger');
+
+/** Error message constants. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_BIND_FLAG = 1,
+ WRAP_BIND_KEY_FLAG = 2,
+ WRAP_CURRY_FLAG = 8,
+ WRAP_CURRY_RIGHT_FLAG = 16,
+ WRAP_PARTIAL_FLAG = 32,
+ WRAP_PARTIAL_RIGHT_FLAG = 64;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that either curries or invokes `func` with optional
+ * `this` binding and partially applied arguments.
+ *
+ * @private
+ * @param {Function|string} func The function or method name to wrap.
+ * @param {number} bitmask The bitmask flags.
+ * 1 - `_.bind`
+ * 2 - `_.bindKey`
+ * 4 - `_.curry` or `_.curryRight` of a bound function
+ * 8 - `_.curry`
+ * 16 - `_.curryRight`
+ * 32 - `_.partial`
+ * 64 - `_.partialRight`
+ * 128 - `_.rearg`
+ * 256 - `_.ary`
+ * 512 - `_.flip`
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {Array} [partials] The arguments to be partially applied.
+ * @param {Array} [holders] The `partials` placeholder indexes.
+ * @param {Array} [argPos] The argument positions of the new function.
+ * @param {number} [ary] The arity cap of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+function createWrap(func, bitmask, thisArg, partials, holders, argPos, ary, arity) {
+ var isBindKey = bitmask & WRAP_BIND_KEY_FLAG;
+ if (!isBindKey && typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ var length = partials ? partials.length : 0;
+ if (!length) {
+ bitmask &= ~(WRAP_PARTIAL_FLAG | WRAP_PARTIAL_RIGHT_FLAG);
+ partials = holders = undefined;
+ }
+ ary = ary === undefined ? ary : nativeMax(toInteger(ary), 0);
+ arity = arity === undefined ? arity : toInteger(arity);
+ length -= holders ? holders.length : 0;
+
+ if (bitmask & WRAP_PARTIAL_RIGHT_FLAG) {
+ var partialsRight = partials,
+ holdersRight = holders;
+
+ partials = holders = undefined;
+ }
+ var data = isBindKey ? undefined : getData(func);
+
+ var newData = [
+ func, bitmask, thisArg, partials, holders, partialsRight, holdersRight,
+ argPos, ary, arity
+ ];
+
+ if (data) {
+ mergeData(newData, data);
+ }
+ func = newData[0];
+ bitmask = newData[1];
+ thisArg = newData[2];
+ partials = newData[3];
+ holders = newData[4];
+ arity = newData[9] = newData[9] === undefined
+ ? (isBindKey ? 0 : func.length)
+ : nativeMax(newData[9] - length, 0);
+
+ if (!arity && bitmask & (WRAP_CURRY_FLAG | WRAP_CURRY_RIGHT_FLAG)) {
+ bitmask &= ~(WRAP_CURRY_FLAG | WRAP_CURRY_RIGHT_FLAG);
+ }
+ if (!bitmask || bitmask == WRAP_BIND_FLAG) {
+ var result = createBind(func, bitmask, thisArg);
+ } else if (bitmask == WRAP_CURRY_FLAG || bitmask == WRAP_CURRY_RIGHT_FLAG) {
+ result = createCurry(func, bitmask, arity);
+ } else if ((bitmask == WRAP_PARTIAL_FLAG || bitmask == (WRAP_BIND_FLAG | WRAP_PARTIAL_FLAG)) && !holders.length) {
+ result = createPartial(func, bitmask, thisArg, partials);
+ } else {
+ result = createHybrid.apply(undefined, newData);
+ }
+ var setter = data ? baseSetData : setData;
+ return setWrapToString(setter(result, newData), func, bitmask);
+}
+
+module.exports = createWrap;
diff --git a/node_modules/lodash/_customDefaultsAssignIn.js b/node_modules/lodash/_customDefaultsAssignIn.js
new file mode 100644
index 0000000..1f49e6f
--- /dev/null
+++ b/node_modules/lodash/_customDefaultsAssignIn.js
@@ -0,0 +1,29 @@
+var eq = require('./eq');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used by `_.defaults` to customize its `_.assignIn` use to assign properties
+ * of source objects to the destination object for all destination properties
+ * that resolve to `undefined`.
+ *
+ * @private
+ * @param {*} objValue The destination value.
+ * @param {*} srcValue The source value.
+ * @param {string} key The key of the property to assign.
+ * @param {Object} object The parent object of `objValue`.
+ * @returns {*} Returns the value to assign.
+ */
+function customDefaultsAssignIn(objValue, srcValue, key, object) {
+ if (objValue === undefined ||
+ (eq(objValue, objectProto[key]) && !hasOwnProperty.call(object, key))) {
+ return srcValue;
+ }
+ return objValue;
+}
+
+module.exports = customDefaultsAssignIn;
diff --git a/node_modules/lodash/_customDefaultsMerge.js b/node_modules/lodash/_customDefaultsMerge.js
new file mode 100644
index 0000000..4cab317
--- /dev/null
+++ b/node_modules/lodash/_customDefaultsMerge.js
@@ -0,0 +1,28 @@
+var baseMerge = require('./_baseMerge'),
+ isObject = require('./isObject');
+
+/**
+ * Used by `_.defaultsDeep` to customize its `_.merge` use to merge source
+ * objects into destination objects that are passed thru.
+ *
+ * @private
+ * @param {*} objValue The destination value.
+ * @param {*} srcValue The source value.
+ * @param {string} key The key of the property to merge.
+ * @param {Object} object The parent object of `objValue`.
+ * @param {Object} source The parent object of `srcValue`.
+ * @param {Object} [stack] Tracks traversed source values and their merged
+ * counterparts.
+ * @returns {*} Returns the value to assign.
+ */
+function customDefaultsMerge(objValue, srcValue, key, object, source, stack) {
+ if (isObject(objValue) && isObject(srcValue)) {
+ // Recursively merge objects and arrays (susceptible to call stack limits).
+ stack.set(srcValue, objValue);
+ baseMerge(objValue, srcValue, undefined, customDefaultsMerge, stack);
+ stack['delete'](srcValue);
+ }
+ return objValue;
+}
+
+module.exports = customDefaultsMerge;
diff --git a/node_modules/lodash/_customOmitClone.js b/node_modules/lodash/_customOmitClone.js
new file mode 100644
index 0000000..968db2e
--- /dev/null
+++ b/node_modules/lodash/_customOmitClone.js
@@ -0,0 +1,16 @@
+var isPlainObject = require('./isPlainObject');
+
+/**
+ * Used by `_.omit` to customize its `_.cloneDeep` use to only clone plain
+ * objects.
+ *
+ * @private
+ * @param {*} value The value to inspect.
+ * @param {string} key The key of the property to inspect.
+ * @returns {*} Returns the uncloned value or `undefined` to defer cloning to `_.cloneDeep`.
+ */
+function customOmitClone(value) {
+ return isPlainObject(value) ? undefined : value;
+}
+
+module.exports = customOmitClone;
diff --git a/node_modules/lodash/_deburrLetter.js b/node_modules/lodash/_deburrLetter.js
new file mode 100644
index 0000000..3e531ed
--- /dev/null
+++ b/node_modules/lodash/_deburrLetter.js
@@ -0,0 +1,71 @@
+var basePropertyOf = require('./_basePropertyOf');
+
+/** Used to map Latin Unicode letters to basic Latin letters. */
+var deburredLetters = {
+ // Latin-1 Supplement block.
+ '\xc0': 'A', '\xc1': 'A', '\xc2': 'A', '\xc3': 'A', '\xc4': 'A', '\xc5': 'A',
+ '\xe0': 'a', '\xe1': 'a', '\xe2': 'a', '\xe3': 'a', '\xe4': 'a', '\xe5': 'a',
+ '\xc7': 'C', '\xe7': 'c',
+ '\xd0': 'D', '\xf0': 'd',
+ '\xc8': 'E', '\xc9': 'E', '\xca': 'E', '\xcb': 'E',
+ '\xe8': 'e', '\xe9': 'e', '\xea': 'e', '\xeb': 'e',
+ '\xcc': 'I', '\xcd': 'I', '\xce': 'I', '\xcf': 'I',
+ '\xec': 'i', '\xed': 'i', '\xee': 'i', '\xef': 'i',
+ '\xd1': 'N', '\xf1': 'n',
+ '\xd2': 'O', '\xd3': 'O', '\xd4': 'O', '\xd5': 'O', '\xd6': 'O', '\xd8': 'O',
+ '\xf2': 'o', '\xf3': 'o', '\xf4': 'o', '\xf5': 'o', '\xf6': 'o', '\xf8': 'o',
+ '\xd9': 'U', '\xda': 'U', '\xdb': 'U', '\xdc': 'U',
+ '\xf9': 'u', '\xfa': 'u', '\xfb': 'u', '\xfc': 'u',
+ '\xdd': 'Y', '\xfd': 'y', '\xff': 'y',
+ '\xc6': 'Ae', '\xe6': 'ae',
+ '\xde': 'Th', '\xfe': 'th',
+ '\xdf': 'ss',
+ // Latin Extended-A block.
+ '\u0100': 'A', '\u0102': 'A', '\u0104': 'A',
+ '\u0101': 'a', '\u0103': 'a', '\u0105': 'a',
+ '\u0106': 'C', '\u0108': 'C', '\u010a': 'C', '\u010c': 'C',
+ '\u0107': 'c', '\u0109': 'c', '\u010b': 'c', '\u010d': 'c',
+ '\u010e': 'D', '\u0110': 'D', '\u010f': 'd', '\u0111': 'd',
+ '\u0112': 'E', '\u0114': 'E', '\u0116': 'E', '\u0118': 'E', '\u011a': 'E',
+ '\u0113': 'e', '\u0115': 'e', '\u0117': 'e', '\u0119': 'e', '\u011b': 'e',
+ '\u011c': 'G', '\u011e': 'G', '\u0120': 'G', '\u0122': 'G',
+ '\u011d': 'g', '\u011f': 'g', '\u0121': 'g', '\u0123': 'g',
+ '\u0124': 'H', '\u0126': 'H', '\u0125': 'h', '\u0127': 'h',
+ '\u0128': 'I', '\u012a': 'I', '\u012c': 'I', '\u012e': 'I', '\u0130': 'I',
+ '\u0129': 'i', '\u012b': 'i', '\u012d': 'i', '\u012f': 'i', '\u0131': 'i',
+ '\u0134': 'J', '\u0135': 'j',
+ '\u0136': 'K', '\u0137': 'k', '\u0138': 'k',
+ '\u0139': 'L', '\u013b': 'L', '\u013d': 'L', '\u013f': 'L', '\u0141': 'L',
+ '\u013a': 'l', '\u013c': 'l', '\u013e': 'l', '\u0140': 'l', '\u0142': 'l',
+ '\u0143': 'N', '\u0145': 'N', '\u0147': 'N', '\u014a': 'N',
+ '\u0144': 'n', '\u0146': 'n', '\u0148': 'n', '\u014b': 'n',
+ '\u014c': 'O', '\u014e': 'O', '\u0150': 'O',
+ '\u014d': 'o', '\u014f': 'o', '\u0151': 'o',
+ '\u0154': 'R', '\u0156': 'R', '\u0158': 'R',
+ '\u0155': 'r', '\u0157': 'r', '\u0159': 'r',
+ '\u015a': 'S', '\u015c': 'S', '\u015e': 'S', '\u0160': 'S',
+ '\u015b': 's', '\u015d': 's', '\u015f': 's', '\u0161': 's',
+ '\u0162': 'T', '\u0164': 'T', '\u0166': 'T',
+ '\u0163': 't', '\u0165': 't', '\u0167': 't',
+ '\u0168': 'U', '\u016a': 'U', '\u016c': 'U', '\u016e': 'U', '\u0170': 'U', '\u0172': 'U',
+ '\u0169': 'u', '\u016b': 'u', '\u016d': 'u', '\u016f': 'u', '\u0171': 'u', '\u0173': 'u',
+ '\u0174': 'W', '\u0175': 'w',
+ '\u0176': 'Y', '\u0177': 'y', '\u0178': 'Y',
+ '\u0179': 'Z', '\u017b': 'Z', '\u017d': 'Z',
+ '\u017a': 'z', '\u017c': 'z', '\u017e': 'z',
+ '\u0132': 'IJ', '\u0133': 'ij',
+ '\u0152': 'Oe', '\u0153': 'oe',
+ '\u0149': "'n", '\u017f': 's'
+};
+
+/**
+ * Used by `_.deburr` to convert Latin-1 Supplement and Latin Extended-A
+ * letters to basic Latin letters.
+ *
+ * @private
+ * @param {string} letter The matched letter to deburr.
+ * @returns {string} Returns the deburred letter.
+ */
+var deburrLetter = basePropertyOf(deburredLetters);
+
+module.exports = deburrLetter;
diff --git a/node_modules/lodash/_defineProperty.js b/node_modules/lodash/_defineProperty.js
new file mode 100644
index 0000000..b6116d9
--- /dev/null
+++ b/node_modules/lodash/_defineProperty.js
@@ -0,0 +1,11 @@
+var getNative = require('./_getNative');
+
+var defineProperty = (function() {
+ try {
+ var func = getNative(Object, 'defineProperty');
+ func({}, '', {});
+ return func;
+ } catch (e) {}
+}());
+
+module.exports = defineProperty;
diff --git a/node_modules/lodash/_equalArrays.js b/node_modules/lodash/_equalArrays.js
new file mode 100644
index 0000000..824228c
--- /dev/null
+++ b/node_modules/lodash/_equalArrays.js
@@ -0,0 +1,84 @@
+var SetCache = require('./_SetCache'),
+ arraySome = require('./_arraySome'),
+ cacheHas = require('./_cacheHas');
+
+/** Used to compose bitmasks for value comparisons. */
+var COMPARE_PARTIAL_FLAG = 1,
+ COMPARE_UNORDERED_FLAG = 2;
+
+/**
+ * A specialized version of `baseIsEqualDeep` for arrays with support for
+ * partial deep comparisons.
+ *
+ * @private
+ * @param {Array} array The array to compare.
+ * @param {Array} other The other array to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} stack Tracks traversed `array` and `other` objects.
+ * @returns {boolean} Returns `true` if the arrays are equivalent, else `false`.
+ */
+function equalArrays(array, other, bitmask, customizer, equalFunc, stack) {
+ var isPartial = bitmask & COMPARE_PARTIAL_FLAG,
+ arrLength = array.length,
+ othLength = other.length;
+
+ if (arrLength != othLength && !(isPartial && othLength > arrLength)) {
+ return false;
+ }
+ // Check that cyclic values are equal.
+ var arrStacked = stack.get(array);
+ var othStacked = stack.get(other);
+ if (arrStacked && othStacked) {
+ return arrStacked == other && othStacked == array;
+ }
+ var index = -1,
+ result = true,
+ seen = (bitmask & COMPARE_UNORDERED_FLAG) ? new SetCache : undefined;
+
+ stack.set(array, other);
+ stack.set(other, array);
+
+ // Ignore non-index properties.
+ while (++index < arrLength) {
+ var arrValue = array[index],
+ othValue = other[index];
+
+ if (customizer) {
+ var compared = isPartial
+ ? customizer(othValue, arrValue, index, other, array, stack)
+ : customizer(arrValue, othValue, index, array, other, stack);
+ }
+ if (compared !== undefined) {
+ if (compared) {
+ continue;
+ }
+ result = false;
+ break;
+ }
+ // Recursively compare arrays (susceptible to call stack limits).
+ if (seen) {
+ if (!arraySome(other, function(othValue, othIndex) {
+ if (!cacheHas(seen, othIndex) &&
+ (arrValue === othValue || equalFunc(arrValue, othValue, bitmask, customizer, stack))) {
+ return seen.push(othIndex);
+ }
+ })) {
+ result = false;
+ break;
+ }
+ } else if (!(
+ arrValue === othValue ||
+ equalFunc(arrValue, othValue, bitmask, customizer, stack)
+ )) {
+ result = false;
+ break;
+ }
+ }
+ stack['delete'](array);
+ stack['delete'](other);
+ return result;
+}
+
+module.exports = equalArrays;
diff --git a/node_modules/lodash/_equalByTag.js b/node_modules/lodash/_equalByTag.js
new file mode 100644
index 0000000..71919e8
--- /dev/null
+++ b/node_modules/lodash/_equalByTag.js
@@ -0,0 +1,112 @@
+var Symbol = require('./_Symbol'),
+ Uint8Array = require('./_Uint8Array'),
+ eq = require('./eq'),
+ equalArrays = require('./_equalArrays'),
+ mapToArray = require('./_mapToArray'),
+ setToArray = require('./_setToArray');
+
+/** Used to compose bitmasks for value comparisons. */
+var COMPARE_PARTIAL_FLAG = 1,
+ COMPARE_UNORDERED_FLAG = 2;
+
+/** `Object#toString` result references. */
+var boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ symbolTag = '[object Symbol]';
+
+var arrayBufferTag = '[object ArrayBuffer]',
+ dataViewTag = '[object DataView]';
+
+/** Used to convert symbols to primitives and strings. */
+var symbolProto = Symbol ? Symbol.prototype : undefined,
+ symbolValueOf = symbolProto ? symbolProto.valueOf : undefined;
+
+/**
+ * A specialized version of `baseIsEqualDeep` for comparing objects of
+ * the same `toStringTag`.
+ *
+ * **Note:** This function only supports comparing values with tags of
+ * `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {string} tag The `toStringTag` of the objects to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} stack Tracks traversed `object` and `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function equalByTag(object, other, tag, bitmask, customizer, equalFunc, stack) {
+ switch (tag) {
+ case dataViewTag:
+ if ((object.byteLength != other.byteLength) ||
+ (object.byteOffset != other.byteOffset)) {
+ return false;
+ }
+ object = object.buffer;
+ other = other.buffer;
+
+ case arrayBufferTag:
+ if ((object.byteLength != other.byteLength) ||
+ !equalFunc(new Uint8Array(object), new Uint8Array(other))) {
+ return false;
+ }
+ return true;
+
+ case boolTag:
+ case dateTag:
+ case numberTag:
+ // Coerce booleans to `1` or `0` and dates to milliseconds.
+ // Invalid dates are coerced to `NaN`.
+ return eq(+object, +other);
+
+ case errorTag:
+ return object.name == other.name && object.message == other.message;
+
+ case regexpTag:
+ case stringTag:
+ // Coerce regexes to strings and treat strings, primitives and objects,
+ // as equal. See http://www.ecma-international.org/ecma-262/7.0/#sec-regexp.prototype.tostring
+ // for more details.
+ return object == (other + '');
+
+ case mapTag:
+ var convert = mapToArray;
+
+ case setTag:
+ var isPartial = bitmask & COMPARE_PARTIAL_FLAG;
+ convert || (convert = setToArray);
+
+ if (object.size != other.size && !isPartial) {
+ return false;
+ }
+ // Assume cyclic values are equal.
+ var stacked = stack.get(object);
+ if (stacked) {
+ return stacked == other;
+ }
+ bitmask |= COMPARE_UNORDERED_FLAG;
+
+ // Recursively compare objects (susceptible to call stack limits).
+ stack.set(object, other);
+ var result = equalArrays(convert(object), convert(other), bitmask, customizer, equalFunc, stack);
+ stack['delete'](object);
+ return result;
+
+ case symbolTag:
+ if (symbolValueOf) {
+ return symbolValueOf.call(object) == symbolValueOf.call(other);
+ }
+ }
+ return false;
+}
+
+module.exports = equalByTag;
diff --git a/node_modules/lodash/_equalObjects.js b/node_modules/lodash/_equalObjects.js
new file mode 100644
index 0000000..cdaacd2
--- /dev/null
+++ b/node_modules/lodash/_equalObjects.js
@@ -0,0 +1,90 @@
+var getAllKeys = require('./_getAllKeys');
+
+/** Used to compose bitmasks for value comparisons. */
+var COMPARE_PARTIAL_FLAG = 1;
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * A specialized version of `baseIsEqualDeep` for objects with support for
+ * partial deep comparisons.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} stack Tracks traversed `object` and `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function equalObjects(object, other, bitmask, customizer, equalFunc, stack) {
+ var isPartial = bitmask & COMPARE_PARTIAL_FLAG,
+ objProps = getAllKeys(object),
+ objLength = objProps.length,
+ othProps = getAllKeys(other),
+ othLength = othProps.length;
+
+ if (objLength != othLength && !isPartial) {
+ return false;
+ }
+ var index = objLength;
+ while (index--) {
+ var key = objProps[index];
+ if (!(isPartial ? key in other : hasOwnProperty.call(other, key))) {
+ return false;
+ }
+ }
+ // Check that cyclic values are equal.
+ var objStacked = stack.get(object);
+ var othStacked = stack.get(other);
+ if (objStacked && othStacked) {
+ return objStacked == other && othStacked == object;
+ }
+ var result = true;
+ stack.set(object, other);
+ stack.set(other, object);
+
+ var skipCtor = isPartial;
+ while (++index < objLength) {
+ key = objProps[index];
+ var objValue = object[key],
+ othValue = other[key];
+
+ if (customizer) {
+ var compared = isPartial
+ ? customizer(othValue, objValue, key, other, object, stack)
+ : customizer(objValue, othValue, key, object, other, stack);
+ }
+ // Recursively compare objects (susceptible to call stack limits).
+ if (!(compared === undefined
+ ? (objValue === othValue || equalFunc(objValue, othValue, bitmask, customizer, stack))
+ : compared
+ )) {
+ result = false;
+ break;
+ }
+ skipCtor || (skipCtor = key == 'constructor');
+ }
+ if (result && !skipCtor) {
+ var objCtor = object.constructor,
+ othCtor = other.constructor;
+
+ // Non `Object` object instances with different constructors are not equal.
+ if (objCtor != othCtor &&
+ ('constructor' in object && 'constructor' in other) &&
+ !(typeof objCtor == 'function' && objCtor instanceof objCtor &&
+ typeof othCtor == 'function' && othCtor instanceof othCtor)) {
+ result = false;
+ }
+ }
+ stack['delete'](object);
+ stack['delete'](other);
+ return result;
+}
+
+module.exports = equalObjects;
diff --git a/node_modules/lodash/_escapeHtmlChar.js b/node_modules/lodash/_escapeHtmlChar.js
new file mode 100644
index 0000000..7ca68ee
--- /dev/null
+++ b/node_modules/lodash/_escapeHtmlChar.js
@@ -0,0 +1,21 @@
+var basePropertyOf = require('./_basePropertyOf');
+
+/** Used to map characters to HTML entities. */
+var htmlEscapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+};
+
+/**
+ * Used by `_.escape` to convert characters to HTML entities.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+var escapeHtmlChar = basePropertyOf(htmlEscapes);
+
+module.exports = escapeHtmlChar;
diff --git a/node_modules/lodash/_escapeStringChar.js b/node_modules/lodash/_escapeStringChar.js
new file mode 100644
index 0000000..44eca96
--- /dev/null
+++ b/node_modules/lodash/_escapeStringChar.js
@@ -0,0 +1,22 @@
+/** Used to escape characters for inclusion in compiled string literals. */
+var stringEscapes = {
+ '\\': '\\',
+ "'": "'",
+ '\n': 'n',
+ '\r': 'r',
+ '\u2028': 'u2028',
+ '\u2029': 'u2029'
+};
+
+/**
+ * Used by `_.template` to escape characters for inclusion in compiled string literals.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeStringChar(chr) {
+ return '\\' + stringEscapes[chr];
+}
+
+module.exports = escapeStringChar;
diff --git a/node_modules/lodash/_flatRest.js b/node_modules/lodash/_flatRest.js
new file mode 100644
index 0000000..94ab6cc
--- /dev/null
+++ b/node_modules/lodash/_flatRest.js
@@ -0,0 +1,16 @@
+var flatten = require('./flatten'),
+ overRest = require('./_overRest'),
+ setToString = require('./_setToString');
+
+/**
+ * A specialized version of `baseRest` which flattens the rest array.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @returns {Function} Returns the new function.
+ */
+function flatRest(func) {
+ return setToString(overRest(func, undefined, flatten), func + '');
+}
+
+module.exports = flatRest;
diff --git a/node_modules/lodash/_freeGlobal.js b/node_modules/lodash/_freeGlobal.js
new file mode 100644
index 0000000..bbec998
--- /dev/null
+++ b/node_modules/lodash/_freeGlobal.js
@@ -0,0 +1,4 @@
+/** Detect free variable `global` from Node.js. */
+var freeGlobal = typeof global == 'object' && global && global.Object === Object && global;
+
+module.exports = freeGlobal;
diff --git a/node_modules/lodash/_getAllKeys.js b/node_modules/lodash/_getAllKeys.js
new file mode 100644
index 0000000..a9ce699
--- /dev/null
+++ b/node_modules/lodash/_getAllKeys.js
@@ -0,0 +1,16 @@
+var baseGetAllKeys = require('./_baseGetAllKeys'),
+ getSymbols = require('./_getSymbols'),
+ keys = require('./keys');
+
+/**
+ * Creates an array of own enumerable property names and symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names and symbols.
+ */
+function getAllKeys(object) {
+ return baseGetAllKeys(object, keys, getSymbols);
+}
+
+module.exports = getAllKeys;
diff --git a/node_modules/lodash/_getAllKeysIn.js b/node_modules/lodash/_getAllKeysIn.js
new file mode 100644
index 0000000..1b46678
--- /dev/null
+++ b/node_modules/lodash/_getAllKeysIn.js
@@ -0,0 +1,17 @@
+var baseGetAllKeys = require('./_baseGetAllKeys'),
+ getSymbolsIn = require('./_getSymbolsIn'),
+ keysIn = require('./keysIn');
+
+/**
+ * Creates an array of own and inherited enumerable property names and
+ * symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names and symbols.
+ */
+function getAllKeysIn(object) {
+ return baseGetAllKeys(object, keysIn, getSymbolsIn);
+}
+
+module.exports = getAllKeysIn;
diff --git a/node_modules/lodash/_getData.js b/node_modules/lodash/_getData.js
new file mode 100644
index 0000000..a1fe7b7
--- /dev/null
+++ b/node_modules/lodash/_getData.js
@@ -0,0 +1,15 @@
+var metaMap = require('./_metaMap'),
+ noop = require('./noop');
+
+/**
+ * Gets metadata for `func`.
+ *
+ * @private
+ * @param {Function} func The function to query.
+ * @returns {*} Returns the metadata for `func`.
+ */
+var getData = !metaMap ? noop : function(func) {
+ return metaMap.get(func);
+};
+
+module.exports = getData;
diff --git a/node_modules/lodash/_getFuncName.js b/node_modules/lodash/_getFuncName.js
new file mode 100644
index 0000000..21e15b3
--- /dev/null
+++ b/node_modules/lodash/_getFuncName.js
@@ -0,0 +1,31 @@
+var realNames = require('./_realNames');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Gets the name of `func`.
+ *
+ * @private
+ * @param {Function} func The function to query.
+ * @returns {string} Returns the function name.
+ */
+function getFuncName(func) {
+ var result = (func.name + ''),
+ array = realNames[result],
+ length = hasOwnProperty.call(realNames, result) ? array.length : 0;
+
+ while (length--) {
+ var data = array[length],
+ otherFunc = data.func;
+ if (otherFunc == null || otherFunc == func) {
+ return data.name;
+ }
+ }
+ return result;
+}
+
+module.exports = getFuncName;
diff --git a/node_modules/lodash/_getHolder.js b/node_modules/lodash/_getHolder.js
new file mode 100644
index 0000000..65e94b5
--- /dev/null
+++ b/node_modules/lodash/_getHolder.js
@@ -0,0 +1,13 @@
+/**
+ * Gets the argument placeholder value for `func`.
+ *
+ * @private
+ * @param {Function} func The function to inspect.
+ * @returns {*} Returns the placeholder value.
+ */
+function getHolder(func) {
+ var object = func;
+ return object.placeholder;
+}
+
+module.exports = getHolder;
diff --git a/node_modules/lodash/_getMapData.js b/node_modules/lodash/_getMapData.js
new file mode 100644
index 0000000..17f6303
--- /dev/null
+++ b/node_modules/lodash/_getMapData.js
@@ -0,0 +1,18 @@
+var isKeyable = require('./_isKeyable');
+
+/**
+ * Gets the data for `map`.
+ *
+ * @private
+ * @param {Object} map The map to query.
+ * @param {string} key The reference key.
+ * @returns {*} Returns the map data.
+ */
+function getMapData(map, key) {
+ var data = map.__data__;
+ return isKeyable(key)
+ ? data[typeof key == 'string' ? 'string' : 'hash']
+ : data.map;
+}
+
+module.exports = getMapData;
diff --git a/node_modules/lodash/_getMatchData.js b/node_modules/lodash/_getMatchData.js
new file mode 100644
index 0000000..2cc70f9
--- /dev/null
+++ b/node_modules/lodash/_getMatchData.js
@@ -0,0 +1,24 @@
+var isStrictComparable = require('./_isStrictComparable'),
+ keys = require('./keys');
+
+/**
+ * Gets the property names, values, and compare flags of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the match data of `object`.
+ */
+function getMatchData(object) {
+ var result = keys(object),
+ length = result.length;
+
+ while (length--) {
+ var key = result[length],
+ value = object[key];
+
+ result[length] = [key, value, isStrictComparable(value)];
+ }
+ return result;
+}
+
+module.exports = getMatchData;
diff --git a/node_modules/lodash/_getNative.js b/node_modules/lodash/_getNative.js
new file mode 100644
index 0000000..97a622b
--- /dev/null
+++ b/node_modules/lodash/_getNative.js
@@ -0,0 +1,17 @@
+var baseIsNative = require('./_baseIsNative'),
+ getValue = require('./_getValue');
+
+/**
+ * Gets the native function at `key` of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the method to get.
+ * @returns {*} Returns the function if it's native, else `undefined`.
+ */
+function getNative(object, key) {
+ var value = getValue(object, key);
+ return baseIsNative(value) ? value : undefined;
+}
+
+module.exports = getNative;
diff --git a/node_modules/lodash/_getPrototype.js b/node_modules/lodash/_getPrototype.js
new file mode 100644
index 0000000..e808612
--- /dev/null
+++ b/node_modules/lodash/_getPrototype.js
@@ -0,0 +1,6 @@
+var overArg = require('./_overArg');
+
+/** Built-in value references. */
+var getPrototype = overArg(Object.getPrototypeOf, Object);
+
+module.exports = getPrototype;
diff --git a/node_modules/lodash/_getRawTag.js b/node_modules/lodash/_getRawTag.js
new file mode 100644
index 0000000..49a95c9
--- /dev/null
+++ b/node_modules/lodash/_getRawTag.js
@@ -0,0 +1,46 @@
+var Symbol = require('./_Symbol');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the
+ * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var nativeObjectToString = objectProto.toString;
+
+/** Built-in value references. */
+var symToStringTag = Symbol ? Symbol.toStringTag : undefined;
+
+/**
+ * A specialized version of `baseGetTag` which ignores `Symbol.toStringTag` values.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the raw `toStringTag`.
+ */
+function getRawTag(value) {
+ var isOwn = hasOwnProperty.call(value, symToStringTag),
+ tag = value[symToStringTag];
+
+ try {
+ value[symToStringTag] = undefined;
+ var unmasked = true;
+ } catch (e) {}
+
+ var result = nativeObjectToString.call(value);
+ if (unmasked) {
+ if (isOwn) {
+ value[symToStringTag] = tag;
+ } else {
+ delete value[symToStringTag];
+ }
+ }
+ return result;
+}
+
+module.exports = getRawTag;
diff --git a/node_modules/lodash/_getSymbols.js b/node_modules/lodash/_getSymbols.js
new file mode 100644
index 0000000..7d6eafe
--- /dev/null
+++ b/node_modules/lodash/_getSymbols.js
@@ -0,0 +1,30 @@
+var arrayFilter = require('./_arrayFilter'),
+ stubArray = require('./stubArray');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Built-in value references. */
+var propertyIsEnumerable = objectProto.propertyIsEnumerable;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeGetSymbols = Object.getOwnPropertySymbols;
+
+/**
+ * Creates an array of the own enumerable symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of symbols.
+ */
+var getSymbols = !nativeGetSymbols ? stubArray : function(object) {
+ if (object == null) {
+ return [];
+ }
+ object = Object(object);
+ return arrayFilter(nativeGetSymbols(object), function(symbol) {
+ return propertyIsEnumerable.call(object, symbol);
+ });
+};
+
+module.exports = getSymbols;
diff --git a/node_modules/lodash/_getSymbolsIn.js b/node_modules/lodash/_getSymbolsIn.js
new file mode 100644
index 0000000..cec0855
--- /dev/null
+++ b/node_modules/lodash/_getSymbolsIn.js
@@ -0,0 +1,25 @@
+var arrayPush = require('./_arrayPush'),
+ getPrototype = require('./_getPrototype'),
+ getSymbols = require('./_getSymbols'),
+ stubArray = require('./stubArray');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeGetSymbols = Object.getOwnPropertySymbols;
+
+/**
+ * Creates an array of the own and inherited enumerable symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of symbols.
+ */
+var getSymbolsIn = !nativeGetSymbols ? stubArray : function(object) {
+ var result = [];
+ while (object) {
+ arrayPush(result, getSymbols(object));
+ object = getPrototype(object);
+ }
+ return result;
+};
+
+module.exports = getSymbolsIn;
diff --git a/node_modules/lodash/_getTag.js b/node_modules/lodash/_getTag.js
new file mode 100644
index 0000000..deaf89d
--- /dev/null
+++ b/node_modules/lodash/_getTag.js
@@ -0,0 +1,58 @@
+var DataView = require('./_DataView'),
+ Map = require('./_Map'),
+ Promise = require('./_Promise'),
+ Set = require('./_Set'),
+ WeakMap = require('./_WeakMap'),
+ baseGetTag = require('./_baseGetTag'),
+ toSource = require('./_toSource');
+
+/** `Object#toString` result references. */
+var mapTag = '[object Map]',
+ objectTag = '[object Object]',
+ promiseTag = '[object Promise]',
+ setTag = '[object Set]',
+ weakMapTag = '[object WeakMap]';
+
+var dataViewTag = '[object DataView]';
+
+/** Used to detect maps, sets, and weakmaps. */
+var dataViewCtorString = toSource(DataView),
+ mapCtorString = toSource(Map),
+ promiseCtorString = toSource(Promise),
+ setCtorString = toSource(Set),
+ weakMapCtorString = toSource(WeakMap);
+
+/**
+ * Gets the `toStringTag` of `value`.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the `toStringTag`.
+ */
+var getTag = baseGetTag;
+
+// Fallback for data views, maps, sets, and weak maps in IE 11 and promises in Node.js < 6.
+if ((DataView && getTag(new DataView(new ArrayBuffer(1))) != dataViewTag) ||
+ (Map && getTag(new Map) != mapTag) ||
+ (Promise && getTag(Promise.resolve()) != promiseTag) ||
+ (Set && getTag(new Set) != setTag) ||
+ (WeakMap && getTag(new WeakMap) != weakMapTag)) {
+ getTag = function(value) {
+ var result = baseGetTag(value),
+ Ctor = result == objectTag ? value.constructor : undefined,
+ ctorString = Ctor ? toSource(Ctor) : '';
+
+ if (ctorString) {
+ switch (ctorString) {
+ case dataViewCtorString: return dataViewTag;
+ case mapCtorString: return mapTag;
+ case promiseCtorString: return promiseTag;
+ case setCtorString: return setTag;
+ case weakMapCtorString: return weakMapTag;
+ }
+ }
+ return result;
+ };
+}
+
+module.exports = getTag;
diff --git a/node_modules/lodash/_getValue.js b/node_modules/lodash/_getValue.js
new file mode 100644
index 0000000..5f7d773
--- /dev/null
+++ b/node_modules/lodash/_getValue.js
@@ -0,0 +1,13 @@
+/**
+ * Gets the value at `key` of `object`.
+ *
+ * @private
+ * @param {Object} [object] The object to query.
+ * @param {string} key The key of the property to get.
+ * @returns {*} Returns the property value.
+ */
+function getValue(object, key) {
+ return object == null ? undefined : object[key];
+}
+
+module.exports = getValue;
diff --git a/node_modules/lodash/_getView.js b/node_modules/lodash/_getView.js
new file mode 100644
index 0000000..df1e5d4
--- /dev/null
+++ b/node_modules/lodash/_getView.js
@@ -0,0 +1,33 @@
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets the view, applying any `transforms` to the `start` and `end` positions.
+ *
+ * @private
+ * @param {number} start The start of the view.
+ * @param {number} end The end of the view.
+ * @param {Array} transforms The transformations to apply to the view.
+ * @returns {Object} Returns an object containing the `start` and `end`
+ * positions of the view.
+ */
+function getView(start, end, transforms) {
+ var index = -1,
+ length = transforms.length;
+
+ while (++index < length) {
+ var data = transforms[index],
+ size = data.size;
+
+ switch (data.type) {
+ case 'drop': start += size; break;
+ case 'dropRight': end -= size; break;
+ case 'take': end = nativeMin(end, start + size); break;
+ case 'takeRight': start = nativeMax(start, end - size); break;
+ }
+ }
+ return { 'start': start, 'end': end };
+}
+
+module.exports = getView;
diff --git a/node_modules/lodash/_getWrapDetails.js b/node_modules/lodash/_getWrapDetails.js
new file mode 100644
index 0000000..3bcc6e4
--- /dev/null
+++ b/node_modules/lodash/_getWrapDetails.js
@@ -0,0 +1,17 @@
+/** Used to match wrap detail comments. */
+var reWrapDetails = /\{\n\/\* \[wrapped with (.+)\] \*/,
+ reSplitDetails = /,? & /;
+
+/**
+ * Extracts wrapper details from the `source` body comment.
+ *
+ * @private
+ * @param {string} source The source to inspect.
+ * @returns {Array} Returns the wrapper details.
+ */
+function getWrapDetails(source) {
+ var match = source.match(reWrapDetails);
+ return match ? match[1].split(reSplitDetails) : [];
+}
+
+module.exports = getWrapDetails;
diff --git a/node_modules/lodash/_hasPath.js b/node_modules/lodash/_hasPath.js
new file mode 100644
index 0000000..93dbde1
--- /dev/null
+++ b/node_modules/lodash/_hasPath.js
@@ -0,0 +1,39 @@
+var castPath = require('./_castPath'),
+ isArguments = require('./isArguments'),
+ isArray = require('./isArray'),
+ isIndex = require('./_isIndex'),
+ isLength = require('./isLength'),
+ toKey = require('./_toKey');
+
+/**
+ * Checks if `path` exists on `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path to check.
+ * @param {Function} hasFunc The function to check properties.
+ * @returns {boolean} Returns `true` if `path` exists, else `false`.
+ */
+function hasPath(object, path, hasFunc) {
+ path = castPath(path, object);
+
+ var index = -1,
+ length = path.length,
+ result = false;
+
+ while (++index < length) {
+ var key = toKey(path[index]);
+ if (!(result = object != null && hasFunc(object, key))) {
+ break;
+ }
+ object = object[key];
+ }
+ if (result || ++index != length) {
+ return result;
+ }
+ length = object == null ? 0 : object.length;
+ return !!length && isLength(length) && isIndex(key, length) &&
+ (isArray(object) || isArguments(object));
+}
+
+module.exports = hasPath;
diff --git a/node_modules/lodash/_hasUnicode.js b/node_modules/lodash/_hasUnicode.js
new file mode 100644
index 0000000..cb6ca15
--- /dev/null
+++ b/node_modules/lodash/_hasUnicode.js
@@ -0,0 +1,26 @@
+/** Used to compose unicode character classes. */
+var rsAstralRange = '\\ud800-\\udfff',
+ rsComboMarksRange = '\\u0300-\\u036f',
+ reComboHalfMarksRange = '\\ufe20-\\ufe2f',
+ rsComboSymbolsRange = '\\u20d0-\\u20ff',
+ rsComboRange = rsComboMarksRange + reComboHalfMarksRange + rsComboSymbolsRange,
+ rsVarRange = '\\ufe0e\\ufe0f';
+
+/** Used to compose unicode capture groups. */
+var rsZWJ = '\\u200d';
+
+/** Used to detect strings with [zero-width joiners or code points from the astral planes](http://eev.ee/blog/2015/09/12/dark-corners-of-unicode/). */
+var reHasUnicode = RegExp('[' + rsZWJ + rsAstralRange + rsComboRange + rsVarRange + ']');
+
+/**
+ * Checks if `string` contains Unicode symbols.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {boolean} Returns `true` if a symbol is found, else `false`.
+ */
+function hasUnicode(string) {
+ return reHasUnicode.test(string);
+}
+
+module.exports = hasUnicode;
diff --git a/node_modules/lodash/_hasUnicodeWord.js b/node_modules/lodash/_hasUnicodeWord.js
new file mode 100644
index 0000000..95d52c4
--- /dev/null
+++ b/node_modules/lodash/_hasUnicodeWord.js
@@ -0,0 +1,15 @@
+/** Used to detect strings that need a more robust regexp to match words. */
+var reHasUnicodeWord = /[a-z][A-Z]|[A-Z]{2}[a-z]|[0-9][a-zA-Z]|[a-zA-Z][0-9]|[^a-zA-Z0-9 ]/;
+
+/**
+ * Checks if `string` contains a word composed of Unicode symbols.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {boolean} Returns `true` if a word is found, else `false`.
+ */
+function hasUnicodeWord(string) {
+ return reHasUnicodeWord.test(string);
+}
+
+module.exports = hasUnicodeWord;
diff --git a/node_modules/lodash/_hashClear.js b/node_modules/lodash/_hashClear.js
new file mode 100644
index 0000000..5d4b70c
--- /dev/null
+++ b/node_modules/lodash/_hashClear.js
@@ -0,0 +1,15 @@
+var nativeCreate = require('./_nativeCreate');
+
+/**
+ * Removes all key-value entries from the hash.
+ *
+ * @private
+ * @name clear
+ * @memberOf Hash
+ */
+function hashClear() {
+ this.__data__ = nativeCreate ? nativeCreate(null) : {};
+ this.size = 0;
+}
+
+module.exports = hashClear;
diff --git a/node_modules/lodash/_hashDelete.js b/node_modules/lodash/_hashDelete.js
new file mode 100644
index 0000000..ea9dabf
--- /dev/null
+++ b/node_modules/lodash/_hashDelete.js
@@ -0,0 +1,17 @@
+/**
+ * Removes `key` and its value from the hash.
+ *
+ * @private
+ * @name delete
+ * @memberOf Hash
+ * @param {Object} hash The hash to modify.
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function hashDelete(key) {
+ var result = this.has(key) && delete this.__data__[key];
+ this.size -= result ? 1 : 0;
+ return result;
+}
+
+module.exports = hashDelete;
diff --git a/node_modules/lodash/_hashGet.js b/node_modules/lodash/_hashGet.js
new file mode 100644
index 0000000..1fc2f34
--- /dev/null
+++ b/node_modules/lodash/_hashGet.js
@@ -0,0 +1,30 @@
+var nativeCreate = require('./_nativeCreate');
+
+/** Used to stand-in for `undefined` hash values. */
+var HASH_UNDEFINED = '__lodash_hash_undefined__';
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Gets the hash value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf Hash
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function hashGet(key) {
+ var data = this.__data__;
+ if (nativeCreate) {
+ var result = data[key];
+ return result === HASH_UNDEFINED ? undefined : result;
+ }
+ return hasOwnProperty.call(data, key) ? data[key] : undefined;
+}
+
+module.exports = hashGet;
diff --git a/node_modules/lodash/_hashHas.js b/node_modules/lodash/_hashHas.js
new file mode 100644
index 0000000..281a551
--- /dev/null
+++ b/node_modules/lodash/_hashHas.js
@@ -0,0 +1,23 @@
+var nativeCreate = require('./_nativeCreate');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Checks if a hash value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf Hash
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function hashHas(key) {
+ var data = this.__data__;
+ return nativeCreate ? (data[key] !== undefined) : hasOwnProperty.call(data, key);
+}
+
+module.exports = hashHas;
diff --git a/node_modules/lodash/_hashSet.js b/node_modules/lodash/_hashSet.js
new file mode 100644
index 0000000..e105528
--- /dev/null
+++ b/node_modules/lodash/_hashSet.js
@@ -0,0 +1,23 @@
+var nativeCreate = require('./_nativeCreate');
+
+/** Used to stand-in for `undefined` hash values. */
+var HASH_UNDEFINED = '__lodash_hash_undefined__';
+
+/**
+ * Sets the hash `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf Hash
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the hash instance.
+ */
+function hashSet(key, value) {
+ var data = this.__data__;
+ this.size += this.has(key) ? 0 : 1;
+ data[key] = (nativeCreate && value === undefined) ? HASH_UNDEFINED : value;
+ return this;
+}
+
+module.exports = hashSet;
diff --git a/node_modules/lodash/_initCloneArray.js b/node_modules/lodash/_initCloneArray.js
new file mode 100644
index 0000000..078c15a
--- /dev/null
+++ b/node_modules/lodash/_initCloneArray.js
@@ -0,0 +1,26 @@
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Initializes an array clone.
+ *
+ * @private
+ * @param {Array} array The array to clone.
+ * @returns {Array} Returns the initialized clone.
+ */
+function initCloneArray(array) {
+ var length = array.length,
+ result = new array.constructor(length);
+
+ // Add properties assigned by `RegExp#exec`.
+ if (length && typeof array[0] == 'string' && hasOwnProperty.call(array, 'index')) {
+ result.index = array.index;
+ result.input = array.input;
+ }
+ return result;
+}
+
+module.exports = initCloneArray;
diff --git a/node_modules/lodash/_initCloneByTag.js b/node_modules/lodash/_initCloneByTag.js
new file mode 100644
index 0000000..f69a008
--- /dev/null
+++ b/node_modules/lodash/_initCloneByTag.js
@@ -0,0 +1,77 @@
+var cloneArrayBuffer = require('./_cloneArrayBuffer'),
+ cloneDataView = require('./_cloneDataView'),
+ cloneRegExp = require('./_cloneRegExp'),
+ cloneSymbol = require('./_cloneSymbol'),
+ cloneTypedArray = require('./_cloneTypedArray');
+
+/** `Object#toString` result references. */
+var boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ symbolTag = '[object Symbol]';
+
+var arrayBufferTag = '[object ArrayBuffer]',
+ dataViewTag = '[object DataView]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+/**
+ * Initializes an object clone based on its `toStringTag`.
+ *
+ * **Note:** This function only supports cloning values with tags of
+ * `Boolean`, `Date`, `Error`, `Map`, `Number`, `RegExp`, `Set`, or `String`.
+ *
+ * @private
+ * @param {Object} object The object to clone.
+ * @param {string} tag The `toStringTag` of the object to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the initialized clone.
+ */
+function initCloneByTag(object, tag, isDeep) {
+ var Ctor = object.constructor;
+ switch (tag) {
+ case arrayBufferTag:
+ return cloneArrayBuffer(object);
+
+ case boolTag:
+ case dateTag:
+ return new Ctor(+object);
+
+ case dataViewTag:
+ return cloneDataView(object, isDeep);
+
+ case float32Tag: case float64Tag:
+ case int8Tag: case int16Tag: case int32Tag:
+ case uint8Tag: case uint8ClampedTag: case uint16Tag: case uint32Tag:
+ return cloneTypedArray(object, isDeep);
+
+ case mapTag:
+ return new Ctor;
+
+ case numberTag:
+ case stringTag:
+ return new Ctor(object);
+
+ case regexpTag:
+ return cloneRegExp(object);
+
+ case setTag:
+ return new Ctor;
+
+ case symbolTag:
+ return cloneSymbol(object);
+ }
+}
+
+module.exports = initCloneByTag;
diff --git a/node_modules/lodash/_initCloneObject.js b/node_modules/lodash/_initCloneObject.js
new file mode 100644
index 0000000..5a13e64
--- /dev/null
+++ b/node_modules/lodash/_initCloneObject.js
@@ -0,0 +1,18 @@
+var baseCreate = require('./_baseCreate'),
+ getPrototype = require('./_getPrototype'),
+ isPrototype = require('./_isPrototype');
+
+/**
+ * Initializes an object clone.
+ *
+ * @private
+ * @param {Object} object The object to clone.
+ * @returns {Object} Returns the initialized clone.
+ */
+function initCloneObject(object) {
+ return (typeof object.constructor == 'function' && !isPrototype(object))
+ ? baseCreate(getPrototype(object))
+ : {};
+}
+
+module.exports = initCloneObject;
diff --git a/node_modules/lodash/_insertWrapDetails.js b/node_modules/lodash/_insertWrapDetails.js
new file mode 100644
index 0000000..e790808
--- /dev/null
+++ b/node_modules/lodash/_insertWrapDetails.js
@@ -0,0 +1,23 @@
+/** Used to match wrap detail comments. */
+var reWrapComment = /\{(?:\n\/\* \[wrapped with .+\] \*\/)?\n?/;
+
+/**
+ * Inserts wrapper `details` in a comment at the top of the `source` body.
+ *
+ * @private
+ * @param {string} source The source to modify.
+ * @returns {Array} details The details to insert.
+ * @returns {string} Returns the modified source.
+ */
+function insertWrapDetails(source, details) {
+ var length = details.length;
+ if (!length) {
+ return source;
+ }
+ var lastIndex = length - 1;
+ details[lastIndex] = (length > 1 ? '& ' : '') + details[lastIndex];
+ details = details.join(length > 2 ? ', ' : ' ');
+ return source.replace(reWrapComment, '{\n/* [wrapped with ' + details + '] */\n');
+}
+
+module.exports = insertWrapDetails;
diff --git a/node_modules/lodash/_isFlattenable.js b/node_modules/lodash/_isFlattenable.js
new file mode 100644
index 0000000..4cc2c24
--- /dev/null
+++ b/node_modules/lodash/_isFlattenable.js
@@ -0,0 +1,20 @@
+var Symbol = require('./_Symbol'),
+ isArguments = require('./isArguments'),
+ isArray = require('./isArray');
+
+/** Built-in value references. */
+var spreadableSymbol = Symbol ? Symbol.isConcatSpreadable : undefined;
+
+/**
+ * Checks if `value` is a flattenable `arguments` object or array.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is flattenable, else `false`.
+ */
+function isFlattenable(value) {
+ return isArray(value) || isArguments(value) ||
+ !!(spreadableSymbol && value && value[spreadableSymbol]);
+}
+
+module.exports = isFlattenable;
diff --git a/node_modules/lodash/_isIndex.js b/node_modules/lodash/_isIndex.js
new file mode 100644
index 0000000..061cd39
--- /dev/null
+++ b/node_modules/lodash/_isIndex.js
@@ -0,0 +1,25 @@
+/** Used as references for various `Number` constants. */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/** Used to detect unsigned integer values. */
+var reIsUint = /^(?:0|[1-9]\d*)$/;
+
+/**
+ * Checks if `value` is a valid array-like index.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
+ * @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
+ */
+function isIndex(value, length) {
+ var type = typeof value;
+ length = length == null ? MAX_SAFE_INTEGER : length;
+
+ return !!length &&
+ (type == 'number' ||
+ (type != 'symbol' && reIsUint.test(value))) &&
+ (value > -1 && value % 1 == 0 && value < length);
+}
+
+module.exports = isIndex;
diff --git a/node_modules/lodash/_isIterateeCall.js b/node_modules/lodash/_isIterateeCall.js
new file mode 100644
index 0000000..a0bb5a9
--- /dev/null
+++ b/node_modules/lodash/_isIterateeCall.js
@@ -0,0 +1,30 @@
+var eq = require('./eq'),
+ isArrayLike = require('./isArrayLike'),
+ isIndex = require('./_isIndex'),
+ isObject = require('./isObject');
+
+/**
+ * Checks if the given arguments are from an iteratee call.
+ *
+ * @private
+ * @param {*} value The potential iteratee value argument.
+ * @param {*} index The potential iteratee index or key argument.
+ * @param {*} object The potential iteratee object argument.
+ * @returns {boolean} Returns `true` if the arguments are from an iteratee call,
+ * else `false`.
+ */
+function isIterateeCall(value, index, object) {
+ if (!isObject(object)) {
+ return false;
+ }
+ var type = typeof index;
+ if (type == 'number'
+ ? (isArrayLike(object) && isIndex(index, object.length))
+ : (type == 'string' && index in object)
+ ) {
+ return eq(object[index], value);
+ }
+ return false;
+}
+
+module.exports = isIterateeCall;
diff --git a/node_modules/lodash/_isKey.js b/node_modules/lodash/_isKey.js
new file mode 100644
index 0000000..ff08b06
--- /dev/null
+++ b/node_modules/lodash/_isKey.js
@@ -0,0 +1,29 @@
+var isArray = require('./isArray'),
+ isSymbol = require('./isSymbol');
+
+/** Used to match property names within property paths. */
+var reIsDeepProp = /\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\\]|\\.)*?\1)\]/,
+ reIsPlainProp = /^\w*$/;
+
+/**
+ * Checks if `value` is a property name and not a property path.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {Object} [object] The object to query keys on.
+ * @returns {boolean} Returns `true` if `value` is a property name, else `false`.
+ */
+function isKey(value, object) {
+ if (isArray(value)) {
+ return false;
+ }
+ var type = typeof value;
+ if (type == 'number' || type == 'symbol' || type == 'boolean' ||
+ value == null || isSymbol(value)) {
+ return true;
+ }
+ return reIsPlainProp.test(value) || !reIsDeepProp.test(value) ||
+ (object != null && value in Object(object));
+}
+
+module.exports = isKey;
diff --git a/node_modules/lodash/_isKeyable.js b/node_modules/lodash/_isKeyable.js
new file mode 100644
index 0000000..39f1828
--- /dev/null
+++ b/node_modules/lodash/_isKeyable.js
@@ -0,0 +1,15 @@
+/**
+ * Checks if `value` is suitable for use as unique object key.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is suitable, else `false`.
+ */
+function isKeyable(value) {
+ var type = typeof value;
+ return (type == 'string' || type == 'number' || type == 'symbol' || type == 'boolean')
+ ? (value !== '__proto__')
+ : (value === null);
+}
+
+module.exports = isKeyable;
diff --git a/node_modules/lodash/_isLaziable.js b/node_modules/lodash/_isLaziable.js
new file mode 100644
index 0000000..a57c4f2
--- /dev/null
+++ b/node_modules/lodash/_isLaziable.js
@@ -0,0 +1,28 @@
+var LazyWrapper = require('./_LazyWrapper'),
+ getData = require('./_getData'),
+ getFuncName = require('./_getFuncName'),
+ lodash = require('./wrapperLodash');
+
+/**
+ * Checks if `func` has a lazy counterpart.
+ *
+ * @private
+ * @param {Function} func The function to check.
+ * @returns {boolean} Returns `true` if `func` has a lazy counterpart,
+ * else `false`.
+ */
+function isLaziable(func) {
+ var funcName = getFuncName(func),
+ other = lodash[funcName];
+
+ if (typeof other != 'function' || !(funcName in LazyWrapper.prototype)) {
+ return false;
+ }
+ if (func === other) {
+ return true;
+ }
+ var data = getData(other);
+ return !!data && func === data[0];
+}
+
+module.exports = isLaziable;
diff --git a/node_modules/lodash/_isMaskable.js b/node_modules/lodash/_isMaskable.js
new file mode 100644
index 0000000..eb98d09
--- /dev/null
+++ b/node_modules/lodash/_isMaskable.js
@@ -0,0 +1,14 @@
+var coreJsData = require('./_coreJsData'),
+ isFunction = require('./isFunction'),
+ stubFalse = require('./stubFalse');
+
+/**
+ * Checks if `func` is capable of being masked.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `func` is maskable, else `false`.
+ */
+var isMaskable = coreJsData ? isFunction : stubFalse;
+
+module.exports = isMaskable;
diff --git a/node_modules/lodash/_isMasked.js b/node_modules/lodash/_isMasked.js
new file mode 100644
index 0000000..4b0f21b
--- /dev/null
+++ b/node_modules/lodash/_isMasked.js
@@ -0,0 +1,20 @@
+var coreJsData = require('./_coreJsData');
+
+/** Used to detect methods masquerading as native. */
+var maskSrcKey = (function() {
+ var uid = /[^.]+$/.exec(coreJsData && coreJsData.keys && coreJsData.keys.IE_PROTO || '');
+ return uid ? ('Symbol(src)_1.' + uid) : '';
+}());
+
+/**
+ * Checks if `func` has its source masked.
+ *
+ * @private
+ * @param {Function} func The function to check.
+ * @returns {boolean} Returns `true` if `func` is masked, else `false`.
+ */
+function isMasked(func) {
+ return !!maskSrcKey && (maskSrcKey in func);
+}
+
+module.exports = isMasked;
diff --git a/node_modules/lodash/_isPrototype.js b/node_modules/lodash/_isPrototype.js
new file mode 100644
index 0000000..0f29498
--- /dev/null
+++ b/node_modules/lodash/_isPrototype.js
@@ -0,0 +1,18 @@
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Checks if `value` is likely a prototype object.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a prototype, else `false`.
+ */
+function isPrototype(value) {
+ var Ctor = value && value.constructor,
+ proto = (typeof Ctor == 'function' && Ctor.prototype) || objectProto;
+
+ return value === proto;
+}
+
+module.exports = isPrototype;
diff --git a/node_modules/lodash/_isStrictComparable.js b/node_modules/lodash/_isStrictComparable.js
new file mode 100644
index 0000000..b59f40b
--- /dev/null
+++ b/node_modules/lodash/_isStrictComparable.js
@@ -0,0 +1,15 @@
+var isObject = require('./isObject');
+
+/**
+ * Checks if `value` is suitable for strict equality comparisons, i.e. `===`.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` if suitable for strict
+ * equality comparisons, else `false`.
+ */
+function isStrictComparable(value) {
+ return value === value && !isObject(value);
+}
+
+module.exports = isStrictComparable;
diff --git a/node_modules/lodash/_iteratorToArray.js b/node_modules/lodash/_iteratorToArray.js
new file mode 100644
index 0000000..4768566
--- /dev/null
+++ b/node_modules/lodash/_iteratorToArray.js
@@ -0,0 +1,18 @@
+/**
+ * Converts `iterator` to an array.
+ *
+ * @private
+ * @param {Object} iterator The iterator to convert.
+ * @returns {Array} Returns the converted array.
+ */
+function iteratorToArray(iterator) {
+ var data,
+ result = [];
+
+ while (!(data = iterator.next()).done) {
+ result.push(data.value);
+ }
+ return result;
+}
+
+module.exports = iteratorToArray;
diff --git a/node_modules/lodash/_lazyClone.js b/node_modules/lodash/_lazyClone.js
new file mode 100644
index 0000000..d8a51f8
--- /dev/null
+++ b/node_modules/lodash/_lazyClone.js
@@ -0,0 +1,23 @@
+var LazyWrapper = require('./_LazyWrapper'),
+ copyArray = require('./_copyArray');
+
+/**
+ * Creates a clone of the lazy wrapper object.
+ *
+ * @private
+ * @name clone
+ * @memberOf LazyWrapper
+ * @returns {Object} Returns the cloned `LazyWrapper` object.
+ */
+function lazyClone() {
+ var result = new LazyWrapper(this.__wrapped__);
+ result.__actions__ = copyArray(this.__actions__);
+ result.__dir__ = this.__dir__;
+ result.__filtered__ = this.__filtered__;
+ result.__iteratees__ = copyArray(this.__iteratees__);
+ result.__takeCount__ = this.__takeCount__;
+ result.__views__ = copyArray(this.__views__);
+ return result;
+}
+
+module.exports = lazyClone;
diff --git a/node_modules/lodash/_lazyReverse.js b/node_modules/lodash/_lazyReverse.js
new file mode 100644
index 0000000..c5b5219
--- /dev/null
+++ b/node_modules/lodash/_lazyReverse.js
@@ -0,0 +1,23 @@
+var LazyWrapper = require('./_LazyWrapper');
+
+/**
+ * Reverses the direction of lazy iteration.
+ *
+ * @private
+ * @name reverse
+ * @memberOf LazyWrapper
+ * @returns {Object} Returns the new reversed `LazyWrapper` object.
+ */
+function lazyReverse() {
+ if (this.__filtered__) {
+ var result = new LazyWrapper(this);
+ result.__dir__ = -1;
+ result.__filtered__ = true;
+ } else {
+ result = this.clone();
+ result.__dir__ *= -1;
+ }
+ return result;
+}
+
+module.exports = lazyReverse;
diff --git a/node_modules/lodash/_lazyValue.js b/node_modules/lodash/_lazyValue.js
new file mode 100644
index 0000000..371ca8d
--- /dev/null
+++ b/node_modules/lodash/_lazyValue.js
@@ -0,0 +1,69 @@
+var baseWrapperValue = require('./_baseWrapperValue'),
+ getView = require('./_getView'),
+ isArray = require('./isArray');
+
+/** Used to indicate the type of lazy iteratees. */
+var LAZY_FILTER_FLAG = 1,
+ LAZY_MAP_FLAG = 2;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * Extracts the unwrapped value from its lazy wrapper.
+ *
+ * @private
+ * @name value
+ * @memberOf LazyWrapper
+ * @returns {*} Returns the unwrapped value.
+ */
+function lazyValue() {
+ var array = this.__wrapped__.value(),
+ dir = this.__dir__,
+ isArr = isArray(array),
+ isRight = dir < 0,
+ arrLength = isArr ? array.length : 0,
+ view = getView(0, arrLength, this.__views__),
+ start = view.start,
+ end = view.end,
+ length = end - start,
+ index = isRight ? end : (start - 1),
+ iteratees = this.__iteratees__,
+ iterLength = iteratees.length,
+ resIndex = 0,
+ takeCount = nativeMin(length, this.__takeCount__);
+
+ if (!isArr || (!isRight && arrLength == length && takeCount == length)) {
+ return baseWrapperValue(array, this.__actions__);
+ }
+ var result = [];
+
+ outer:
+ while (length-- && resIndex < takeCount) {
+ index += dir;
+
+ var iterIndex = -1,
+ value = array[index];
+
+ while (++iterIndex < iterLength) {
+ var data = iteratees[iterIndex],
+ iteratee = data.iteratee,
+ type = data.type,
+ computed = iteratee(value);
+
+ if (type == LAZY_MAP_FLAG) {
+ value = computed;
+ } else if (!computed) {
+ if (type == LAZY_FILTER_FLAG) {
+ continue outer;
+ } else {
+ break outer;
+ }
+ }
+ }
+ result[resIndex++] = value;
+ }
+ return result;
+}
+
+module.exports = lazyValue;
diff --git a/node_modules/lodash/_listCacheClear.js b/node_modules/lodash/_listCacheClear.js
new file mode 100644
index 0000000..acbe39a
--- /dev/null
+++ b/node_modules/lodash/_listCacheClear.js
@@ -0,0 +1,13 @@
+/**
+ * Removes all key-value entries from the list cache.
+ *
+ * @private
+ * @name clear
+ * @memberOf ListCache
+ */
+function listCacheClear() {
+ this.__data__ = [];
+ this.size = 0;
+}
+
+module.exports = listCacheClear;
diff --git a/node_modules/lodash/_listCacheDelete.js b/node_modules/lodash/_listCacheDelete.js
new file mode 100644
index 0000000..b1384ad
--- /dev/null
+++ b/node_modules/lodash/_listCacheDelete.js
@@ -0,0 +1,35 @@
+var assocIndexOf = require('./_assocIndexOf');
+
+/** Used for built-in method references. */
+var arrayProto = Array.prototype;
+
+/** Built-in value references. */
+var splice = arrayProto.splice;
+
+/**
+ * Removes `key` and its value from the list cache.
+ *
+ * @private
+ * @name delete
+ * @memberOf ListCache
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function listCacheDelete(key) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ if (index < 0) {
+ return false;
+ }
+ var lastIndex = data.length - 1;
+ if (index == lastIndex) {
+ data.pop();
+ } else {
+ splice.call(data, index, 1);
+ }
+ --this.size;
+ return true;
+}
+
+module.exports = listCacheDelete;
diff --git a/node_modules/lodash/_listCacheGet.js b/node_modules/lodash/_listCacheGet.js
new file mode 100644
index 0000000..f8192fc
--- /dev/null
+++ b/node_modules/lodash/_listCacheGet.js
@@ -0,0 +1,19 @@
+var assocIndexOf = require('./_assocIndexOf');
+
+/**
+ * Gets the list cache value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf ListCache
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function listCacheGet(key) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ return index < 0 ? undefined : data[index][1];
+}
+
+module.exports = listCacheGet;
diff --git a/node_modules/lodash/_listCacheHas.js b/node_modules/lodash/_listCacheHas.js
new file mode 100644
index 0000000..2adf671
--- /dev/null
+++ b/node_modules/lodash/_listCacheHas.js
@@ -0,0 +1,16 @@
+var assocIndexOf = require('./_assocIndexOf');
+
+/**
+ * Checks if a list cache value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf ListCache
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function listCacheHas(key) {
+ return assocIndexOf(this.__data__, key) > -1;
+}
+
+module.exports = listCacheHas;
diff --git a/node_modules/lodash/_listCacheSet.js b/node_modules/lodash/_listCacheSet.js
new file mode 100644
index 0000000..5855c95
--- /dev/null
+++ b/node_modules/lodash/_listCacheSet.js
@@ -0,0 +1,26 @@
+var assocIndexOf = require('./_assocIndexOf');
+
+/**
+ * Sets the list cache `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf ListCache
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the list cache instance.
+ */
+function listCacheSet(key, value) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ if (index < 0) {
+ ++this.size;
+ data.push([key, value]);
+ } else {
+ data[index][1] = value;
+ }
+ return this;
+}
+
+module.exports = listCacheSet;
diff --git a/node_modules/lodash/_mapCacheClear.js b/node_modules/lodash/_mapCacheClear.js
new file mode 100644
index 0000000..bc9ca20
--- /dev/null
+++ b/node_modules/lodash/_mapCacheClear.js
@@ -0,0 +1,21 @@
+var Hash = require('./_Hash'),
+ ListCache = require('./_ListCache'),
+ Map = require('./_Map');
+
+/**
+ * Removes all key-value entries from the map.
+ *
+ * @private
+ * @name clear
+ * @memberOf MapCache
+ */
+function mapCacheClear() {
+ this.size = 0;
+ this.__data__ = {
+ 'hash': new Hash,
+ 'map': new (Map || ListCache),
+ 'string': new Hash
+ };
+}
+
+module.exports = mapCacheClear;
diff --git a/node_modules/lodash/_mapCacheDelete.js b/node_modules/lodash/_mapCacheDelete.js
new file mode 100644
index 0000000..946ca3c
--- /dev/null
+++ b/node_modules/lodash/_mapCacheDelete.js
@@ -0,0 +1,18 @@
+var getMapData = require('./_getMapData');
+
+/**
+ * Removes `key` and its value from the map.
+ *
+ * @private
+ * @name delete
+ * @memberOf MapCache
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function mapCacheDelete(key) {
+ var result = getMapData(this, key)['delete'](key);
+ this.size -= result ? 1 : 0;
+ return result;
+}
+
+module.exports = mapCacheDelete;
diff --git a/node_modules/lodash/_mapCacheGet.js b/node_modules/lodash/_mapCacheGet.js
new file mode 100644
index 0000000..f29f55c
--- /dev/null
+++ b/node_modules/lodash/_mapCacheGet.js
@@ -0,0 +1,16 @@
+var getMapData = require('./_getMapData');
+
+/**
+ * Gets the map value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf MapCache
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function mapCacheGet(key) {
+ return getMapData(this, key).get(key);
+}
+
+module.exports = mapCacheGet;
diff --git a/node_modules/lodash/_mapCacheHas.js b/node_modules/lodash/_mapCacheHas.js
new file mode 100644
index 0000000..a1214c0
--- /dev/null
+++ b/node_modules/lodash/_mapCacheHas.js
@@ -0,0 +1,16 @@
+var getMapData = require('./_getMapData');
+
+/**
+ * Checks if a map value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf MapCache
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function mapCacheHas(key) {
+ return getMapData(this, key).has(key);
+}
+
+module.exports = mapCacheHas;
diff --git a/node_modules/lodash/_mapCacheSet.js b/node_modules/lodash/_mapCacheSet.js
new file mode 100644
index 0000000..7346849
--- /dev/null
+++ b/node_modules/lodash/_mapCacheSet.js
@@ -0,0 +1,22 @@
+var getMapData = require('./_getMapData');
+
+/**
+ * Sets the map `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf MapCache
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the map cache instance.
+ */
+function mapCacheSet(key, value) {
+ var data = getMapData(this, key),
+ size = data.size;
+
+ data.set(key, value);
+ this.size += data.size == size ? 0 : 1;
+ return this;
+}
+
+module.exports = mapCacheSet;
diff --git a/node_modules/lodash/_mapToArray.js b/node_modules/lodash/_mapToArray.js
new file mode 100644
index 0000000..fe3dd53
--- /dev/null
+++ b/node_modules/lodash/_mapToArray.js
@@ -0,0 +1,18 @@
+/**
+ * Converts `map` to its key-value pairs.
+ *
+ * @private
+ * @param {Object} map The map to convert.
+ * @returns {Array} Returns the key-value pairs.
+ */
+function mapToArray(map) {
+ var index = -1,
+ result = Array(map.size);
+
+ map.forEach(function(value, key) {
+ result[++index] = [key, value];
+ });
+ return result;
+}
+
+module.exports = mapToArray;
diff --git a/node_modules/lodash/_matchesStrictComparable.js b/node_modules/lodash/_matchesStrictComparable.js
new file mode 100644
index 0000000..f608af9
--- /dev/null
+++ b/node_modules/lodash/_matchesStrictComparable.js
@@ -0,0 +1,20 @@
+/**
+ * A specialized version of `matchesProperty` for source values suitable
+ * for strict equality comparisons, i.e. `===`.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @param {*} srcValue The value to match.
+ * @returns {Function} Returns the new spec function.
+ */
+function matchesStrictComparable(key, srcValue) {
+ return function(object) {
+ if (object == null) {
+ return false;
+ }
+ return object[key] === srcValue &&
+ (srcValue !== undefined || (key in Object(object)));
+ };
+}
+
+module.exports = matchesStrictComparable;
diff --git a/node_modules/lodash/_memoizeCapped.js b/node_modules/lodash/_memoizeCapped.js
new file mode 100644
index 0000000..7f71c8f
--- /dev/null
+++ b/node_modules/lodash/_memoizeCapped.js
@@ -0,0 +1,26 @@
+var memoize = require('./memoize');
+
+/** Used as the maximum memoize cache size. */
+var MAX_MEMOIZE_SIZE = 500;
+
+/**
+ * A specialized version of `_.memoize` which clears the memoized function's
+ * cache when it exceeds `MAX_MEMOIZE_SIZE`.
+ *
+ * @private
+ * @param {Function} func The function to have its output memoized.
+ * @returns {Function} Returns the new memoized function.
+ */
+function memoizeCapped(func) {
+ var result = memoize(func, function(key) {
+ if (cache.size === MAX_MEMOIZE_SIZE) {
+ cache.clear();
+ }
+ return key;
+ });
+
+ var cache = result.cache;
+ return result;
+}
+
+module.exports = memoizeCapped;
diff --git a/node_modules/lodash/_mergeData.js b/node_modules/lodash/_mergeData.js
new file mode 100644
index 0000000..cb570f9
--- /dev/null
+++ b/node_modules/lodash/_mergeData.js
@@ -0,0 +1,90 @@
+var composeArgs = require('./_composeArgs'),
+ composeArgsRight = require('./_composeArgsRight'),
+ replaceHolders = require('./_replaceHolders');
+
+/** Used as the internal argument placeholder. */
+var PLACEHOLDER = '__lodash_placeholder__';
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_BIND_FLAG = 1,
+ WRAP_BIND_KEY_FLAG = 2,
+ WRAP_CURRY_BOUND_FLAG = 4,
+ WRAP_CURRY_FLAG = 8,
+ WRAP_ARY_FLAG = 128,
+ WRAP_REARG_FLAG = 256;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * Merges the function metadata of `source` into `data`.
+ *
+ * Merging metadata reduces the number of wrappers used to invoke a function.
+ * This is possible because methods like `_.bind`, `_.curry`, and `_.partial`
+ * may be applied regardless of execution order. Methods like `_.ary` and
+ * `_.rearg` modify function arguments, making the order in which they are
+ * executed important, preventing the merging of metadata. However, we make
+ * an exception for a safe combined case where curried functions have `_.ary`
+ * and or `_.rearg` applied.
+ *
+ * @private
+ * @param {Array} data The destination metadata.
+ * @param {Array} source The source metadata.
+ * @returns {Array} Returns `data`.
+ */
+function mergeData(data, source) {
+ var bitmask = data[1],
+ srcBitmask = source[1],
+ newBitmask = bitmask | srcBitmask,
+ isCommon = newBitmask < (WRAP_BIND_FLAG | WRAP_BIND_KEY_FLAG | WRAP_ARY_FLAG);
+
+ var isCombo =
+ ((srcBitmask == WRAP_ARY_FLAG) && (bitmask == WRAP_CURRY_FLAG)) ||
+ ((srcBitmask == WRAP_ARY_FLAG) && (bitmask == WRAP_REARG_FLAG) && (data[7].length <= source[8])) ||
+ ((srcBitmask == (WRAP_ARY_FLAG | WRAP_REARG_FLAG)) && (source[7].length <= source[8]) && (bitmask == WRAP_CURRY_FLAG));
+
+ // Exit early if metadata can't be merged.
+ if (!(isCommon || isCombo)) {
+ return data;
+ }
+ // Use source `thisArg` if available.
+ if (srcBitmask & WRAP_BIND_FLAG) {
+ data[2] = source[2];
+ // Set when currying a bound function.
+ newBitmask |= bitmask & WRAP_BIND_FLAG ? 0 : WRAP_CURRY_BOUND_FLAG;
+ }
+ // Compose partial arguments.
+ var value = source[3];
+ if (value) {
+ var partials = data[3];
+ data[3] = partials ? composeArgs(partials, value, source[4]) : value;
+ data[4] = partials ? replaceHolders(data[3], PLACEHOLDER) : source[4];
+ }
+ // Compose partial right arguments.
+ value = source[5];
+ if (value) {
+ partials = data[5];
+ data[5] = partials ? composeArgsRight(partials, value, source[6]) : value;
+ data[6] = partials ? replaceHolders(data[5], PLACEHOLDER) : source[6];
+ }
+ // Use source `argPos` if available.
+ value = source[7];
+ if (value) {
+ data[7] = value;
+ }
+ // Use source `ary` if it's smaller.
+ if (srcBitmask & WRAP_ARY_FLAG) {
+ data[8] = data[8] == null ? source[8] : nativeMin(data[8], source[8]);
+ }
+ // Use source `arity` if one is not provided.
+ if (data[9] == null) {
+ data[9] = source[9];
+ }
+ // Use source `func` and merge bitmasks.
+ data[0] = source[0];
+ data[1] = newBitmask;
+
+ return data;
+}
+
+module.exports = mergeData;
diff --git a/node_modules/lodash/_metaMap.js b/node_modules/lodash/_metaMap.js
new file mode 100644
index 0000000..0157a0b
--- /dev/null
+++ b/node_modules/lodash/_metaMap.js
@@ -0,0 +1,6 @@
+var WeakMap = require('./_WeakMap');
+
+/** Used to store function metadata. */
+var metaMap = WeakMap && new WeakMap;
+
+module.exports = metaMap;
diff --git a/node_modules/lodash/_nativeCreate.js b/node_modules/lodash/_nativeCreate.js
new file mode 100644
index 0000000..c7aede8
--- /dev/null
+++ b/node_modules/lodash/_nativeCreate.js
@@ -0,0 +1,6 @@
+var getNative = require('./_getNative');
+
+/* Built-in method references that are verified to be native. */
+var nativeCreate = getNative(Object, 'create');
+
+module.exports = nativeCreate;
diff --git a/node_modules/lodash/_nativeKeys.js b/node_modules/lodash/_nativeKeys.js
new file mode 100644
index 0000000..479a104
--- /dev/null
+++ b/node_modules/lodash/_nativeKeys.js
@@ -0,0 +1,6 @@
+var overArg = require('./_overArg');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeKeys = overArg(Object.keys, Object);
+
+module.exports = nativeKeys;
diff --git a/node_modules/lodash/_nativeKeysIn.js b/node_modules/lodash/_nativeKeysIn.js
new file mode 100644
index 0000000..00ee505
--- /dev/null
+++ b/node_modules/lodash/_nativeKeysIn.js
@@ -0,0 +1,20 @@
+/**
+ * This function is like
+ * [`Object.keys`](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
+ * except that it includes inherited enumerable properties.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+function nativeKeysIn(object) {
+ var result = [];
+ if (object != null) {
+ for (var key in Object(object)) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+module.exports = nativeKeysIn;
diff --git a/node_modules/lodash/_nodeUtil.js b/node_modules/lodash/_nodeUtil.js
new file mode 100644
index 0000000..983d78f
--- /dev/null
+++ b/node_modules/lodash/_nodeUtil.js
@@ -0,0 +1,30 @@
+var freeGlobal = require('./_freeGlobal');
+
+/** Detect free variable `exports`. */
+var freeExports = typeof exports == 'object' && exports && !exports.nodeType && exports;
+
+/** Detect free variable `module`. */
+var freeModule = freeExports && typeof module == 'object' && module && !module.nodeType && module;
+
+/** Detect the popular CommonJS extension `module.exports`. */
+var moduleExports = freeModule && freeModule.exports === freeExports;
+
+/** Detect free variable `process` from Node.js. */
+var freeProcess = moduleExports && freeGlobal.process;
+
+/** Used to access faster Node.js helpers. */
+var nodeUtil = (function() {
+ try {
+ // Use `util.types` for Node.js 10+.
+ var types = freeModule && freeModule.require && freeModule.require('util').types;
+
+ if (types) {
+ return types;
+ }
+
+ // Legacy `process.binding('util')` for Node.js < 10.
+ return freeProcess && freeProcess.binding && freeProcess.binding('util');
+ } catch (e) {}
+}());
+
+module.exports = nodeUtil;
diff --git a/node_modules/lodash/_objectToString.js b/node_modules/lodash/_objectToString.js
new file mode 100644
index 0000000..c614ec0
--- /dev/null
+++ b/node_modules/lodash/_objectToString.js
@@ -0,0 +1,22 @@
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the
+ * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var nativeObjectToString = objectProto.toString;
+
+/**
+ * Converts `value` to a string using `Object.prototype.toString`.
+ *
+ * @private
+ * @param {*} value The value to convert.
+ * @returns {string} Returns the converted string.
+ */
+function objectToString(value) {
+ return nativeObjectToString.call(value);
+}
+
+module.exports = objectToString;
diff --git a/node_modules/lodash/_overArg.js b/node_modules/lodash/_overArg.js
new file mode 100644
index 0000000..651c5c5
--- /dev/null
+++ b/node_modules/lodash/_overArg.js
@@ -0,0 +1,15 @@
+/**
+ * Creates a unary function that invokes `func` with its argument transformed.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {Function} transform The argument transform.
+ * @returns {Function} Returns the new function.
+ */
+function overArg(func, transform) {
+ return function(arg) {
+ return func(transform(arg));
+ };
+}
+
+module.exports = overArg;
diff --git a/node_modules/lodash/_overRest.js b/node_modules/lodash/_overRest.js
new file mode 100644
index 0000000..c7cdef3
--- /dev/null
+++ b/node_modules/lodash/_overRest.js
@@ -0,0 +1,36 @@
+var apply = require('./_apply');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * A specialized version of `baseRest` which transforms the rest array.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @param {Function} transform The rest array transform.
+ * @returns {Function} Returns the new function.
+ */
+function overRest(func, start, transform) {
+ start = nativeMax(start === undefined ? (func.length - 1) : start, 0);
+ return function() {
+ var args = arguments,
+ index = -1,
+ length = nativeMax(args.length - start, 0),
+ array = Array(length);
+
+ while (++index < length) {
+ array[index] = args[start + index];
+ }
+ index = -1;
+ var otherArgs = Array(start + 1);
+ while (++index < start) {
+ otherArgs[index] = args[index];
+ }
+ otherArgs[start] = transform(array);
+ return apply(func, this, otherArgs);
+ };
+}
+
+module.exports = overRest;
diff --git a/node_modules/lodash/_parent.js b/node_modules/lodash/_parent.js
new file mode 100644
index 0000000..f174328
--- /dev/null
+++ b/node_modules/lodash/_parent.js
@@ -0,0 +1,16 @@
+var baseGet = require('./_baseGet'),
+ baseSlice = require('./_baseSlice');
+
+/**
+ * Gets the parent value at `path` of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} path The path to get the parent value of.
+ * @returns {*} Returns the parent value.
+ */
+function parent(object, path) {
+ return path.length < 2 ? object : baseGet(object, baseSlice(path, 0, -1));
+}
+
+module.exports = parent;
diff --git a/node_modules/lodash/_reEscape.js b/node_modules/lodash/_reEscape.js
new file mode 100644
index 0000000..7f47eda
--- /dev/null
+++ b/node_modules/lodash/_reEscape.js
@@ -0,0 +1,4 @@
+/** Used to match template delimiters. */
+var reEscape = /<%-([\s\S]+?)%>/g;
+
+module.exports = reEscape;
diff --git a/node_modules/lodash/_reEvaluate.js b/node_modules/lodash/_reEvaluate.js
new file mode 100644
index 0000000..6adfc31
--- /dev/null
+++ b/node_modules/lodash/_reEvaluate.js
@@ -0,0 +1,4 @@
+/** Used to match template delimiters. */
+var reEvaluate = /<%([\s\S]+?)%>/g;
+
+module.exports = reEvaluate;
diff --git a/node_modules/lodash/_reInterpolate.js b/node_modules/lodash/_reInterpolate.js
new file mode 100644
index 0000000..d02ff0b
--- /dev/null
+++ b/node_modules/lodash/_reInterpolate.js
@@ -0,0 +1,4 @@
+/** Used to match template delimiters. */
+var reInterpolate = /<%=([\s\S]+?)%>/g;
+
+module.exports = reInterpolate;
diff --git a/node_modules/lodash/_realNames.js b/node_modules/lodash/_realNames.js
new file mode 100644
index 0000000..aa0d529
--- /dev/null
+++ b/node_modules/lodash/_realNames.js
@@ -0,0 +1,4 @@
+/** Used to lookup unminified function names. */
+var realNames = {};
+
+module.exports = realNames;
diff --git a/node_modules/lodash/_reorder.js b/node_modules/lodash/_reorder.js
new file mode 100644
index 0000000..a3502b0
--- /dev/null
+++ b/node_modules/lodash/_reorder.js
@@ -0,0 +1,29 @@
+var copyArray = require('./_copyArray'),
+ isIndex = require('./_isIndex');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * Reorder `array` according to the specified indexes where the element at
+ * the first index is assigned as the first element, the element at
+ * the second index is assigned as the second element, and so on.
+ *
+ * @private
+ * @param {Array} array The array to reorder.
+ * @param {Array} indexes The arranged array indexes.
+ * @returns {Array} Returns `array`.
+ */
+function reorder(array, indexes) {
+ var arrLength = array.length,
+ length = nativeMin(indexes.length, arrLength),
+ oldArray = copyArray(array);
+
+ while (length--) {
+ var index = indexes[length];
+ array[length] = isIndex(index, arrLength) ? oldArray[index] : undefined;
+ }
+ return array;
+}
+
+module.exports = reorder;
diff --git a/node_modules/lodash/_replaceHolders.js b/node_modules/lodash/_replaceHolders.js
new file mode 100644
index 0000000..74360ec
--- /dev/null
+++ b/node_modules/lodash/_replaceHolders.js
@@ -0,0 +1,29 @@
+/** Used as the internal argument placeholder. */
+var PLACEHOLDER = '__lodash_placeholder__';
+
+/**
+ * Replaces all `placeholder` elements in `array` with an internal placeholder
+ * and returns an array of their indexes.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {*} placeholder The placeholder to replace.
+ * @returns {Array} Returns the new array of placeholder indexes.
+ */
+function replaceHolders(array, placeholder) {
+ var index = -1,
+ length = array.length,
+ resIndex = 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (value === placeholder || value === PLACEHOLDER) {
+ array[index] = PLACEHOLDER;
+ result[resIndex++] = index;
+ }
+ }
+ return result;
+}
+
+module.exports = replaceHolders;
diff --git a/node_modules/lodash/_root.js b/node_modules/lodash/_root.js
new file mode 100644
index 0000000..d2852be
--- /dev/null
+++ b/node_modules/lodash/_root.js
@@ -0,0 +1,9 @@
+var freeGlobal = require('./_freeGlobal');
+
+/** Detect free variable `self`. */
+var freeSelf = typeof self == 'object' && self && self.Object === Object && self;
+
+/** Used as a reference to the global object. */
+var root = freeGlobal || freeSelf || Function('return this')();
+
+module.exports = root;
diff --git a/node_modules/lodash/_safeGet.js b/node_modules/lodash/_safeGet.js
new file mode 100644
index 0000000..b070897
--- /dev/null
+++ b/node_modules/lodash/_safeGet.js
@@ -0,0 +1,21 @@
+/**
+ * Gets the value at `key`, unless `key` is "__proto__" or "constructor".
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the property to get.
+ * @returns {*} Returns the property value.
+ */
+function safeGet(object, key) {
+ if (key === 'constructor' && typeof object[key] === 'function') {
+ return;
+ }
+
+ if (key == '__proto__') {
+ return;
+ }
+
+ return object[key];
+}
+
+module.exports = safeGet;
diff --git a/node_modules/lodash/_setCacheAdd.js b/node_modules/lodash/_setCacheAdd.js
new file mode 100644
index 0000000..1081a74
--- /dev/null
+++ b/node_modules/lodash/_setCacheAdd.js
@@ -0,0 +1,19 @@
+/** Used to stand-in for `undefined` hash values. */
+var HASH_UNDEFINED = '__lodash_hash_undefined__';
+
+/**
+ * Adds `value` to the array cache.
+ *
+ * @private
+ * @name add
+ * @memberOf SetCache
+ * @alias push
+ * @param {*} value The value to cache.
+ * @returns {Object} Returns the cache instance.
+ */
+function setCacheAdd(value) {
+ this.__data__.set(value, HASH_UNDEFINED);
+ return this;
+}
+
+module.exports = setCacheAdd;
diff --git a/node_modules/lodash/_setCacheHas.js b/node_modules/lodash/_setCacheHas.js
new file mode 100644
index 0000000..9a49255
--- /dev/null
+++ b/node_modules/lodash/_setCacheHas.js
@@ -0,0 +1,14 @@
+/**
+ * Checks if `value` is in the array cache.
+ *
+ * @private
+ * @name has
+ * @memberOf SetCache
+ * @param {*} value The value to search for.
+ * @returns {number} Returns `true` if `value` is found, else `false`.
+ */
+function setCacheHas(value) {
+ return this.__data__.has(value);
+}
+
+module.exports = setCacheHas;
diff --git a/node_modules/lodash/_setData.js b/node_modules/lodash/_setData.js
new file mode 100644
index 0000000..e5cf3eb
--- /dev/null
+++ b/node_modules/lodash/_setData.js
@@ -0,0 +1,20 @@
+var baseSetData = require('./_baseSetData'),
+ shortOut = require('./_shortOut');
+
+/**
+ * Sets metadata for `func`.
+ *
+ * **Note:** If this function becomes hot, i.e. is invoked a lot in a short
+ * period of time, it will trip its breaker and transition to an identity
+ * function to avoid garbage collection pauses in V8. See
+ * [V8 issue 2070](https://bugs.chromium.org/p/v8/issues/detail?id=2070)
+ * for more details.
+ *
+ * @private
+ * @param {Function} func The function to associate metadata with.
+ * @param {*} data The metadata.
+ * @returns {Function} Returns `func`.
+ */
+var setData = shortOut(baseSetData);
+
+module.exports = setData;
diff --git a/node_modules/lodash/_setToArray.js b/node_modules/lodash/_setToArray.js
new file mode 100644
index 0000000..b87f074
--- /dev/null
+++ b/node_modules/lodash/_setToArray.js
@@ -0,0 +1,18 @@
+/**
+ * Converts `set` to an array of its values.
+ *
+ * @private
+ * @param {Object} set The set to convert.
+ * @returns {Array} Returns the values.
+ */
+function setToArray(set) {
+ var index = -1,
+ result = Array(set.size);
+
+ set.forEach(function(value) {
+ result[++index] = value;
+ });
+ return result;
+}
+
+module.exports = setToArray;
diff --git a/node_modules/lodash/_setToPairs.js b/node_modules/lodash/_setToPairs.js
new file mode 100644
index 0000000..36ad37a
--- /dev/null
+++ b/node_modules/lodash/_setToPairs.js
@@ -0,0 +1,18 @@
+/**
+ * Converts `set` to its value-value pairs.
+ *
+ * @private
+ * @param {Object} set The set to convert.
+ * @returns {Array} Returns the value-value pairs.
+ */
+function setToPairs(set) {
+ var index = -1,
+ result = Array(set.size);
+
+ set.forEach(function(value) {
+ result[++index] = [value, value];
+ });
+ return result;
+}
+
+module.exports = setToPairs;
diff --git a/node_modules/lodash/_setToString.js b/node_modules/lodash/_setToString.js
new file mode 100644
index 0000000..6ca8419
--- /dev/null
+++ b/node_modules/lodash/_setToString.js
@@ -0,0 +1,14 @@
+var baseSetToString = require('./_baseSetToString'),
+ shortOut = require('./_shortOut');
+
+/**
+ * Sets the `toString` method of `func` to return `string`.
+ *
+ * @private
+ * @param {Function} func The function to modify.
+ * @param {Function} string The `toString` result.
+ * @returns {Function} Returns `func`.
+ */
+var setToString = shortOut(baseSetToString);
+
+module.exports = setToString;
diff --git a/node_modules/lodash/_setWrapToString.js b/node_modules/lodash/_setWrapToString.js
new file mode 100644
index 0000000..decdc44
--- /dev/null
+++ b/node_modules/lodash/_setWrapToString.js
@@ -0,0 +1,21 @@
+var getWrapDetails = require('./_getWrapDetails'),
+ insertWrapDetails = require('./_insertWrapDetails'),
+ setToString = require('./_setToString'),
+ updateWrapDetails = require('./_updateWrapDetails');
+
+/**
+ * Sets the `toString` method of `wrapper` to mimic the source of `reference`
+ * with wrapper details in a comment at the top of the source body.
+ *
+ * @private
+ * @param {Function} wrapper The function to modify.
+ * @param {Function} reference The reference function.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @returns {Function} Returns `wrapper`.
+ */
+function setWrapToString(wrapper, reference, bitmask) {
+ var source = (reference + '');
+ return setToString(wrapper, insertWrapDetails(source, updateWrapDetails(getWrapDetails(source), bitmask)));
+}
+
+module.exports = setWrapToString;
diff --git a/node_modules/lodash/_shortOut.js b/node_modules/lodash/_shortOut.js
new file mode 100644
index 0000000..3300a07
--- /dev/null
+++ b/node_modules/lodash/_shortOut.js
@@ -0,0 +1,37 @@
+/** Used to detect hot functions by number of calls within a span of milliseconds. */
+var HOT_COUNT = 800,
+ HOT_SPAN = 16;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeNow = Date.now;
+
+/**
+ * Creates a function that'll short out and invoke `identity` instead
+ * of `func` when it's called `HOT_COUNT` or more times in `HOT_SPAN`
+ * milliseconds.
+ *
+ * @private
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new shortable function.
+ */
+function shortOut(func) {
+ var count = 0,
+ lastCalled = 0;
+
+ return function() {
+ var stamp = nativeNow(),
+ remaining = HOT_SPAN - (stamp - lastCalled);
+
+ lastCalled = stamp;
+ if (remaining > 0) {
+ if (++count >= HOT_COUNT) {
+ return arguments[0];
+ }
+ } else {
+ count = 0;
+ }
+ return func.apply(undefined, arguments);
+ };
+}
+
+module.exports = shortOut;
diff --git a/node_modules/lodash/_shuffleSelf.js b/node_modules/lodash/_shuffleSelf.js
new file mode 100644
index 0000000..8bcc4f5
--- /dev/null
+++ b/node_modules/lodash/_shuffleSelf.js
@@ -0,0 +1,28 @@
+var baseRandom = require('./_baseRandom');
+
+/**
+ * A specialized version of `_.shuffle` which mutates and sets the size of `array`.
+ *
+ * @private
+ * @param {Array} array The array to shuffle.
+ * @param {number} [size=array.length] The size of `array`.
+ * @returns {Array} Returns `array`.
+ */
+function shuffleSelf(array, size) {
+ var index = -1,
+ length = array.length,
+ lastIndex = length - 1;
+
+ size = size === undefined ? length : size;
+ while (++index < size) {
+ var rand = baseRandom(index, lastIndex),
+ value = array[rand];
+
+ array[rand] = array[index];
+ array[index] = value;
+ }
+ array.length = size;
+ return array;
+}
+
+module.exports = shuffleSelf;
diff --git a/node_modules/lodash/_stackClear.js b/node_modules/lodash/_stackClear.js
new file mode 100644
index 0000000..ce8e5a9
--- /dev/null
+++ b/node_modules/lodash/_stackClear.js
@@ -0,0 +1,15 @@
+var ListCache = require('./_ListCache');
+
+/**
+ * Removes all key-value entries from the stack.
+ *
+ * @private
+ * @name clear
+ * @memberOf Stack
+ */
+function stackClear() {
+ this.__data__ = new ListCache;
+ this.size = 0;
+}
+
+module.exports = stackClear;
diff --git a/node_modules/lodash/_stackDelete.js b/node_modules/lodash/_stackDelete.js
new file mode 100644
index 0000000..ff9887a
--- /dev/null
+++ b/node_modules/lodash/_stackDelete.js
@@ -0,0 +1,18 @@
+/**
+ * Removes `key` and its value from the stack.
+ *
+ * @private
+ * @name delete
+ * @memberOf Stack
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function stackDelete(key) {
+ var data = this.__data__,
+ result = data['delete'](key);
+
+ this.size = data.size;
+ return result;
+}
+
+module.exports = stackDelete;
diff --git a/node_modules/lodash/_stackGet.js b/node_modules/lodash/_stackGet.js
new file mode 100644
index 0000000..1cdf004
--- /dev/null
+++ b/node_modules/lodash/_stackGet.js
@@ -0,0 +1,14 @@
+/**
+ * Gets the stack value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf Stack
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function stackGet(key) {
+ return this.__data__.get(key);
+}
+
+module.exports = stackGet;
diff --git a/node_modules/lodash/_stackHas.js b/node_modules/lodash/_stackHas.js
new file mode 100644
index 0000000..16a3ad1
--- /dev/null
+++ b/node_modules/lodash/_stackHas.js
@@ -0,0 +1,14 @@
+/**
+ * Checks if a stack value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf Stack
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function stackHas(key) {
+ return this.__data__.has(key);
+}
+
+module.exports = stackHas;
diff --git a/node_modules/lodash/_stackSet.js b/node_modules/lodash/_stackSet.js
new file mode 100644
index 0000000..b790ac5
--- /dev/null
+++ b/node_modules/lodash/_stackSet.js
@@ -0,0 +1,34 @@
+var ListCache = require('./_ListCache'),
+ Map = require('./_Map'),
+ MapCache = require('./_MapCache');
+
+/** Used as the size to enable large array optimizations. */
+var LARGE_ARRAY_SIZE = 200;
+
+/**
+ * Sets the stack `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf Stack
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the stack cache instance.
+ */
+function stackSet(key, value) {
+ var data = this.__data__;
+ if (data instanceof ListCache) {
+ var pairs = data.__data__;
+ if (!Map || (pairs.length < LARGE_ARRAY_SIZE - 1)) {
+ pairs.push([key, value]);
+ this.size = ++data.size;
+ return this;
+ }
+ data = this.__data__ = new MapCache(pairs);
+ }
+ data.set(key, value);
+ this.size = data.size;
+ return this;
+}
+
+module.exports = stackSet;
diff --git a/node_modules/lodash/_strictIndexOf.js b/node_modules/lodash/_strictIndexOf.js
new file mode 100644
index 0000000..0486a49
--- /dev/null
+++ b/node_modules/lodash/_strictIndexOf.js
@@ -0,0 +1,23 @@
+/**
+ * A specialized version of `_.indexOf` which performs strict equality
+ * comparisons of values, i.e. `===`.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} fromIndex The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+function strictIndexOf(array, value, fromIndex) {
+ var index = fromIndex - 1,
+ length = array.length;
+
+ while (++index < length) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = strictIndexOf;
diff --git a/node_modules/lodash/_strictLastIndexOf.js b/node_modules/lodash/_strictLastIndexOf.js
new file mode 100644
index 0000000..d7310dc
--- /dev/null
+++ b/node_modules/lodash/_strictLastIndexOf.js
@@ -0,0 +1,21 @@
+/**
+ * A specialized version of `_.lastIndexOf` which performs strict equality
+ * comparisons of values, i.e. `===`.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} fromIndex The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+function strictLastIndexOf(array, value, fromIndex) {
+ var index = fromIndex + 1;
+ while (index--) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return index;
+}
+
+module.exports = strictLastIndexOf;
diff --git a/node_modules/lodash/_stringSize.js b/node_modules/lodash/_stringSize.js
new file mode 100644
index 0000000..17ef462
--- /dev/null
+++ b/node_modules/lodash/_stringSize.js
@@ -0,0 +1,18 @@
+var asciiSize = require('./_asciiSize'),
+ hasUnicode = require('./_hasUnicode'),
+ unicodeSize = require('./_unicodeSize');
+
+/**
+ * Gets the number of symbols in `string`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {number} Returns the string size.
+ */
+function stringSize(string) {
+ return hasUnicode(string)
+ ? unicodeSize(string)
+ : asciiSize(string);
+}
+
+module.exports = stringSize;
diff --git a/node_modules/lodash/_stringToArray.js b/node_modules/lodash/_stringToArray.js
new file mode 100644
index 0000000..d161158
--- /dev/null
+++ b/node_modules/lodash/_stringToArray.js
@@ -0,0 +1,18 @@
+var asciiToArray = require('./_asciiToArray'),
+ hasUnicode = require('./_hasUnicode'),
+ unicodeToArray = require('./_unicodeToArray');
+
+/**
+ * Converts `string` to an array.
+ *
+ * @private
+ * @param {string} string The string to convert.
+ * @returns {Array} Returns the converted array.
+ */
+function stringToArray(string) {
+ return hasUnicode(string)
+ ? unicodeToArray(string)
+ : asciiToArray(string);
+}
+
+module.exports = stringToArray;
diff --git a/node_modules/lodash/_stringToPath.js b/node_modules/lodash/_stringToPath.js
new file mode 100644
index 0000000..8f39f8a
--- /dev/null
+++ b/node_modules/lodash/_stringToPath.js
@@ -0,0 +1,27 @@
+var memoizeCapped = require('./_memoizeCapped');
+
+/** Used to match property names within property paths. */
+var rePropName = /[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\\]|\\.)*?)\2)\]|(?=(?:\.|\[\])(?:\.|\[\]|$))/g;
+
+/** Used to match backslashes in property paths. */
+var reEscapeChar = /\\(\\)?/g;
+
+/**
+ * Converts `string` to a property path array.
+ *
+ * @private
+ * @param {string} string The string to convert.
+ * @returns {Array} Returns the property path array.
+ */
+var stringToPath = memoizeCapped(function(string) {
+ var result = [];
+ if (string.charCodeAt(0) === 46 /* . */) {
+ result.push('');
+ }
+ string.replace(rePropName, function(match, number, quote, subString) {
+ result.push(quote ? subString.replace(reEscapeChar, '$1') : (number || match));
+ });
+ return result;
+});
+
+module.exports = stringToPath;
diff --git a/node_modules/lodash/_toKey.js b/node_modules/lodash/_toKey.js
new file mode 100644
index 0000000..c6d645c
--- /dev/null
+++ b/node_modules/lodash/_toKey.js
@@ -0,0 +1,21 @@
+var isSymbol = require('./isSymbol');
+
+/** Used as references for various `Number` constants. */
+var INFINITY = 1 / 0;
+
+/**
+ * Converts `value` to a string key if it's not a string or symbol.
+ *
+ * @private
+ * @param {*} value The value to inspect.
+ * @returns {string|symbol} Returns the key.
+ */
+function toKey(value) {
+ if (typeof value == 'string' || isSymbol(value)) {
+ return value;
+ }
+ var result = (value + '');
+ return (result == '0' && (1 / value) == -INFINITY) ? '-0' : result;
+}
+
+module.exports = toKey;
diff --git a/node_modules/lodash/_toSource.js b/node_modules/lodash/_toSource.js
new file mode 100644
index 0000000..a020b38
--- /dev/null
+++ b/node_modules/lodash/_toSource.js
@@ -0,0 +1,26 @@
+/** Used for built-in method references. */
+var funcProto = Function.prototype;
+
+/** Used to resolve the decompiled source of functions. */
+var funcToString = funcProto.toString;
+
+/**
+ * Converts `func` to its source code.
+ *
+ * @private
+ * @param {Function} func The function to convert.
+ * @returns {string} Returns the source code.
+ */
+function toSource(func) {
+ if (func != null) {
+ try {
+ return funcToString.call(func);
+ } catch (e) {}
+ try {
+ return (func + '');
+ } catch (e) {}
+ }
+ return '';
+}
+
+module.exports = toSource;
diff --git a/node_modules/lodash/_trimmedEndIndex.js b/node_modules/lodash/_trimmedEndIndex.js
new file mode 100644
index 0000000..139439a
--- /dev/null
+++ b/node_modules/lodash/_trimmedEndIndex.js
@@ -0,0 +1,19 @@
+/** Used to match a single whitespace character. */
+var reWhitespace = /\s/;
+
+/**
+ * Used by `_.trim` and `_.trimEnd` to get the index of the last non-whitespace
+ * character of `string`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {number} Returns the index of the last non-whitespace character.
+ */
+function trimmedEndIndex(string) {
+ var index = string.length;
+
+ while (index-- && reWhitespace.test(string.charAt(index))) {}
+ return index;
+}
+
+module.exports = trimmedEndIndex;
diff --git a/node_modules/lodash/_unescapeHtmlChar.js b/node_modules/lodash/_unescapeHtmlChar.js
new file mode 100644
index 0000000..a71fecb
--- /dev/null
+++ b/node_modules/lodash/_unescapeHtmlChar.js
@@ -0,0 +1,21 @@
+var basePropertyOf = require('./_basePropertyOf');
+
+/** Used to map HTML entities to characters. */
+var htmlUnescapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ ''': "'"
+};
+
+/**
+ * Used by `_.unescape` to convert HTML entities to characters.
+ *
+ * @private
+ * @param {string} chr The matched character to unescape.
+ * @returns {string} Returns the unescaped character.
+ */
+var unescapeHtmlChar = basePropertyOf(htmlUnescapes);
+
+module.exports = unescapeHtmlChar;
diff --git a/node_modules/lodash/_unicodeSize.js b/node_modules/lodash/_unicodeSize.js
new file mode 100644
index 0000000..68137ec
--- /dev/null
+++ b/node_modules/lodash/_unicodeSize.js
@@ -0,0 +1,44 @@
+/** Used to compose unicode character classes. */
+var rsAstralRange = '\\ud800-\\udfff',
+ rsComboMarksRange = '\\u0300-\\u036f',
+ reComboHalfMarksRange = '\\ufe20-\\ufe2f',
+ rsComboSymbolsRange = '\\u20d0-\\u20ff',
+ rsComboRange = rsComboMarksRange + reComboHalfMarksRange + rsComboSymbolsRange,
+ rsVarRange = '\\ufe0e\\ufe0f';
+
+/** Used to compose unicode capture groups. */
+var rsAstral = '[' + rsAstralRange + ']',
+ rsCombo = '[' + rsComboRange + ']',
+ rsFitz = '\\ud83c[\\udffb-\\udfff]',
+ rsModifier = '(?:' + rsCombo + '|' + rsFitz + ')',
+ rsNonAstral = '[^' + rsAstralRange + ']',
+ rsRegional = '(?:\\ud83c[\\udde6-\\uddff]){2}',
+ rsSurrPair = '[\\ud800-\\udbff][\\udc00-\\udfff]',
+ rsZWJ = '\\u200d';
+
+/** Used to compose unicode regexes. */
+var reOptMod = rsModifier + '?',
+ rsOptVar = '[' + rsVarRange + ']?',
+ rsOptJoin = '(?:' + rsZWJ + '(?:' + [rsNonAstral, rsRegional, rsSurrPair].join('|') + ')' + rsOptVar + reOptMod + ')*',
+ rsSeq = rsOptVar + reOptMod + rsOptJoin,
+ rsSymbol = '(?:' + [rsNonAstral + rsCombo + '?', rsCombo, rsRegional, rsSurrPair, rsAstral].join('|') + ')';
+
+/** Used to match [string symbols](https://mathiasbynens.be/notes/javascript-unicode). */
+var reUnicode = RegExp(rsFitz + '(?=' + rsFitz + ')|' + rsSymbol + rsSeq, 'g');
+
+/**
+ * Gets the size of a Unicode `string`.
+ *
+ * @private
+ * @param {string} string The string inspect.
+ * @returns {number} Returns the string size.
+ */
+function unicodeSize(string) {
+ var result = reUnicode.lastIndex = 0;
+ while (reUnicode.test(string)) {
+ ++result;
+ }
+ return result;
+}
+
+module.exports = unicodeSize;
diff --git a/node_modules/lodash/_unicodeToArray.js b/node_modules/lodash/_unicodeToArray.js
new file mode 100644
index 0000000..2a725c0
--- /dev/null
+++ b/node_modules/lodash/_unicodeToArray.js
@@ -0,0 +1,40 @@
+/** Used to compose unicode character classes. */
+var rsAstralRange = '\\ud800-\\udfff',
+ rsComboMarksRange = '\\u0300-\\u036f',
+ reComboHalfMarksRange = '\\ufe20-\\ufe2f',
+ rsComboSymbolsRange = '\\u20d0-\\u20ff',
+ rsComboRange = rsComboMarksRange + reComboHalfMarksRange + rsComboSymbolsRange,
+ rsVarRange = '\\ufe0e\\ufe0f';
+
+/** Used to compose unicode capture groups. */
+var rsAstral = '[' + rsAstralRange + ']',
+ rsCombo = '[' + rsComboRange + ']',
+ rsFitz = '\\ud83c[\\udffb-\\udfff]',
+ rsModifier = '(?:' + rsCombo + '|' + rsFitz + ')',
+ rsNonAstral = '[^' + rsAstralRange + ']',
+ rsRegional = '(?:\\ud83c[\\udde6-\\uddff]){2}',
+ rsSurrPair = '[\\ud800-\\udbff][\\udc00-\\udfff]',
+ rsZWJ = '\\u200d';
+
+/** Used to compose unicode regexes. */
+var reOptMod = rsModifier + '?',
+ rsOptVar = '[' + rsVarRange + ']?',
+ rsOptJoin = '(?:' + rsZWJ + '(?:' + [rsNonAstral, rsRegional, rsSurrPair].join('|') + ')' + rsOptVar + reOptMod + ')*',
+ rsSeq = rsOptVar + reOptMod + rsOptJoin,
+ rsSymbol = '(?:' + [rsNonAstral + rsCombo + '?', rsCombo, rsRegional, rsSurrPair, rsAstral].join('|') + ')';
+
+/** Used to match [string symbols](https://mathiasbynens.be/notes/javascript-unicode). */
+var reUnicode = RegExp(rsFitz + '(?=' + rsFitz + ')|' + rsSymbol + rsSeq, 'g');
+
+/**
+ * Converts a Unicode `string` to an array.
+ *
+ * @private
+ * @param {string} string The string to convert.
+ * @returns {Array} Returns the converted array.
+ */
+function unicodeToArray(string) {
+ return string.match(reUnicode) || [];
+}
+
+module.exports = unicodeToArray;
diff --git a/node_modules/lodash/_unicodeWords.js b/node_modules/lodash/_unicodeWords.js
new file mode 100644
index 0000000..e72e6e0
--- /dev/null
+++ b/node_modules/lodash/_unicodeWords.js
@@ -0,0 +1,69 @@
+/** Used to compose unicode character classes. */
+var rsAstralRange = '\\ud800-\\udfff',
+ rsComboMarksRange = '\\u0300-\\u036f',
+ reComboHalfMarksRange = '\\ufe20-\\ufe2f',
+ rsComboSymbolsRange = '\\u20d0-\\u20ff',
+ rsComboRange = rsComboMarksRange + reComboHalfMarksRange + rsComboSymbolsRange,
+ rsDingbatRange = '\\u2700-\\u27bf',
+ rsLowerRange = 'a-z\\xdf-\\xf6\\xf8-\\xff',
+ rsMathOpRange = '\\xac\\xb1\\xd7\\xf7',
+ rsNonCharRange = '\\x00-\\x2f\\x3a-\\x40\\x5b-\\x60\\x7b-\\xbf',
+ rsPunctuationRange = '\\u2000-\\u206f',
+ rsSpaceRange = ' \\t\\x0b\\f\\xa0\\ufeff\\n\\r\\u2028\\u2029\\u1680\\u180e\\u2000\\u2001\\u2002\\u2003\\u2004\\u2005\\u2006\\u2007\\u2008\\u2009\\u200a\\u202f\\u205f\\u3000',
+ rsUpperRange = 'A-Z\\xc0-\\xd6\\xd8-\\xde',
+ rsVarRange = '\\ufe0e\\ufe0f',
+ rsBreakRange = rsMathOpRange + rsNonCharRange + rsPunctuationRange + rsSpaceRange;
+
+/** Used to compose unicode capture groups. */
+var rsApos = "['\u2019]",
+ rsBreak = '[' + rsBreakRange + ']',
+ rsCombo = '[' + rsComboRange + ']',
+ rsDigits = '\\d+',
+ rsDingbat = '[' + rsDingbatRange + ']',
+ rsLower = '[' + rsLowerRange + ']',
+ rsMisc = '[^' + rsAstralRange + rsBreakRange + rsDigits + rsDingbatRange + rsLowerRange + rsUpperRange + ']',
+ rsFitz = '\\ud83c[\\udffb-\\udfff]',
+ rsModifier = '(?:' + rsCombo + '|' + rsFitz + ')',
+ rsNonAstral = '[^' + rsAstralRange + ']',
+ rsRegional = '(?:\\ud83c[\\udde6-\\uddff]){2}',
+ rsSurrPair = '[\\ud800-\\udbff][\\udc00-\\udfff]',
+ rsUpper = '[' + rsUpperRange + ']',
+ rsZWJ = '\\u200d';
+
+/** Used to compose unicode regexes. */
+var rsMiscLower = '(?:' + rsLower + '|' + rsMisc + ')',
+ rsMiscUpper = '(?:' + rsUpper + '|' + rsMisc + ')',
+ rsOptContrLower = '(?:' + rsApos + '(?:d|ll|m|re|s|t|ve))?',
+ rsOptContrUpper = '(?:' + rsApos + '(?:D|LL|M|RE|S|T|VE))?',
+ reOptMod = rsModifier + '?',
+ rsOptVar = '[' + rsVarRange + ']?',
+ rsOptJoin = '(?:' + rsZWJ + '(?:' + [rsNonAstral, rsRegional, rsSurrPair].join('|') + ')' + rsOptVar + reOptMod + ')*',
+ rsOrdLower = '\\d*(?:1st|2nd|3rd|(?![123])\\dth)(?=\\b|[A-Z_])',
+ rsOrdUpper = '\\d*(?:1ST|2ND|3RD|(?![123])\\dTH)(?=\\b|[a-z_])',
+ rsSeq = rsOptVar + reOptMod + rsOptJoin,
+ rsEmoji = '(?:' + [rsDingbat, rsRegional, rsSurrPair].join('|') + ')' + rsSeq;
+
+/** Used to match complex or compound words. */
+var reUnicodeWord = RegExp([
+ rsUpper + '?' + rsLower + '+' + rsOptContrLower + '(?=' + [rsBreak, rsUpper, '$'].join('|') + ')',
+ rsMiscUpper + '+' + rsOptContrUpper + '(?=' + [rsBreak, rsUpper + rsMiscLower, '$'].join('|') + ')',
+ rsUpper + '?' + rsMiscLower + '+' + rsOptContrLower,
+ rsUpper + '+' + rsOptContrUpper,
+ rsOrdUpper,
+ rsOrdLower,
+ rsDigits,
+ rsEmoji
+].join('|'), 'g');
+
+/**
+ * Splits a Unicode `string` into an array of its words.
+ *
+ * @private
+ * @param {string} The string to inspect.
+ * @returns {Array} Returns the words of `string`.
+ */
+function unicodeWords(string) {
+ return string.match(reUnicodeWord) || [];
+}
+
+module.exports = unicodeWords;
diff --git a/node_modules/lodash/_updateWrapDetails.js b/node_modules/lodash/_updateWrapDetails.js
new file mode 100644
index 0000000..8759fbd
--- /dev/null
+++ b/node_modules/lodash/_updateWrapDetails.js
@@ -0,0 +1,46 @@
+var arrayEach = require('./_arrayEach'),
+ arrayIncludes = require('./_arrayIncludes');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_BIND_FLAG = 1,
+ WRAP_BIND_KEY_FLAG = 2,
+ WRAP_CURRY_FLAG = 8,
+ WRAP_CURRY_RIGHT_FLAG = 16,
+ WRAP_PARTIAL_FLAG = 32,
+ WRAP_PARTIAL_RIGHT_FLAG = 64,
+ WRAP_ARY_FLAG = 128,
+ WRAP_REARG_FLAG = 256,
+ WRAP_FLIP_FLAG = 512;
+
+/** Used to associate wrap methods with their bit flags. */
+var wrapFlags = [
+ ['ary', WRAP_ARY_FLAG],
+ ['bind', WRAP_BIND_FLAG],
+ ['bindKey', WRAP_BIND_KEY_FLAG],
+ ['curry', WRAP_CURRY_FLAG],
+ ['curryRight', WRAP_CURRY_RIGHT_FLAG],
+ ['flip', WRAP_FLIP_FLAG],
+ ['partial', WRAP_PARTIAL_FLAG],
+ ['partialRight', WRAP_PARTIAL_RIGHT_FLAG],
+ ['rearg', WRAP_REARG_FLAG]
+];
+
+/**
+ * Updates wrapper `details` based on `bitmask` flags.
+ *
+ * @private
+ * @returns {Array} details The details to modify.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @returns {Array} Returns `details`.
+ */
+function updateWrapDetails(details, bitmask) {
+ arrayEach(wrapFlags, function(pair) {
+ var value = '_.' + pair[0];
+ if ((bitmask & pair[1]) && !arrayIncludes(details, value)) {
+ details.push(value);
+ }
+ });
+ return details.sort();
+}
+
+module.exports = updateWrapDetails;
diff --git a/node_modules/lodash/_wrapperClone.js b/node_modules/lodash/_wrapperClone.js
new file mode 100644
index 0000000..7bb58a2
--- /dev/null
+++ b/node_modules/lodash/_wrapperClone.js
@@ -0,0 +1,23 @@
+var LazyWrapper = require('./_LazyWrapper'),
+ LodashWrapper = require('./_LodashWrapper'),
+ copyArray = require('./_copyArray');
+
+/**
+ * Creates a clone of `wrapper`.
+ *
+ * @private
+ * @param {Object} wrapper The wrapper to clone.
+ * @returns {Object} Returns the cloned wrapper.
+ */
+function wrapperClone(wrapper) {
+ if (wrapper instanceof LazyWrapper) {
+ return wrapper.clone();
+ }
+ var result = new LodashWrapper(wrapper.__wrapped__, wrapper.__chain__);
+ result.__actions__ = copyArray(wrapper.__actions__);
+ result.__index__ = wrapper.__index__;
+ result.__values__ = wrapper.__values__;
+ return result;
+}
+
+module.exports = wrapperClone;
diff --git a/node_modules/lodash/add.js b/node_modules/lodash/add.js
new file mode 100644
index 0000000..f069515
--- /dev/null
+++ b/node_modules/lodash/add.js
@@ -0,0 +1,22 @@
+var createMathOperation = require('./_createMathOperation');
+
+/**
+ * Adds two numbers.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.4.0
+ * @category Math
+ * @param {number} augend The first number in an addition.
+ * @param {number} addend The second number in an addition.
+ * @returns {number} Returns the total.
+ * @example
+ *
+ * _.add(6, 4);
+ * // => 10
+ */
+var add = createMathOperation(function(augend, addend) {
+ return augend + addend;
+}, 0);
+
+module.exports = add;
diff --git a/node_modules/lodash/after.js b/node_modules/lodash/after.js
new file mode 100644
index 0000000..3900c97
--- /dev/null
+++ b/node_modules/lodash/after.js
@@ -0,0 +1,42 @@
+var toInteger = require('./toInteger');
+
+/** Error message constants. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * The opposite of `_.before`; this method creates a function that invokes
+ * `func` once it's called `n` or more times.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {number} n The number of calls before `func` is invoked.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var saves = ['profile', 'settings'];
+ *
+ * var done = _.after(saves.length, function() {
+ * console.log('done saving!');
+ * });
+ *
+ * _.forEach(saves, function(type) {
+ * asyncSave({ 'type': type, 'complete': done });
+ * });
+ * // => Logs 'done saving!' after the two async saves have completed.
+ */
+function after(n, func) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ n = toInteger(n);
+ return function() {
+ if (--n < 1) {
+ return func.apply(this, arguments);
+ }
+ };
+}
+
+module.exports = after;
diff --git a/node_modules/lodash/array.js b/node_modules/lodash/array.js
new file mode 100644
index 0000000..af688d3
--- /dev/null
+++ b/node_modules/lodash/array.js
@@ -0,0 +1,67 @@
+module.exports = {
+ 'chunk': require('./chunk'),
+ 'compact': require('./compact'),
+ 'concat': require('./concat'),
+ 'difference': require('./difference'),
+ 'differenceBy': require('./differenceBy'),
+ 'differenceWith': require('./differenceWith'),
+ 'drop': require('./drop'),
+ 'dropRight': require('./dropRight'),
+ 'dropRightWhile': require('./dropRightWhile'),
+ 'dropWhile': require('./dropWhile'),
+ 'fill': require('./fill'),
+ 'findIndex': require('./findIndex'),
+ 'findLastIndex': require('./findLastIndex'),
+ 'first': require('./first'),
+ 'flatten': require('./flatten'),
+ 'flattenDeep': require('./flattenDeep'),
+ 'flattenDepth': require('./flattenDepth'),
+ 'fromPairs': require('./fromPairs'),
+ 'head': require('./head'),
+ 'indexOf': require('./indexOf'),
+ 'initial': require('./initial'),
+ 'intersection': require('./intersection'),
+ 'intersectionBy': require('./intersectionBy'),
+ 'intersectionWith': require('./intersectionWith'),
+ 'join': require('./join'),
+ 'last': require('./last'),
+ 'lastIndexOf': require('./lastIndexOf'),
+ 'nth': require('./nth'),
+ 'pull': require('./pull'),
+ 'pullAll': require('./pullAll'),
+ 'pullAllBy': require('./pullAllBy'),
+ 'pullAllWith': require('./pullAllWith'),
+ 'pullAt': require('./pullAt'),
+ 'remove': require('./remove'),
+ 'reverse': require('./reverse'),
+ 'slice': require('./slice'),
+ 'sortedIndex': require('./sortedIndex'),
+ 'sortedIndexBy': require('./sortedIndexBy'),
+ 'sortedIndexOf': require('./sortedIndexOf'),
+ 'sortedLastIndex': require('./sortedLastIndex'),
+ 'sortedLastIndexBy': require('./sortedLastIndexBy'),
+ 'sortedLastIndexOf': require('./sortedLastIndexOf'),
+ 'sortedUniq': require('./sortedUniq'),
+ 'sortedUniqBy': require('./sortedUniqBy'),
+ 'tail': require('./tail'),
+ 'take': require('./take'),
+ 'takeRight': require('./takeRight'),
+ 'takeRightWhile': require('./takeRightWhile'),
+ 'takeWhile': require('./takeWhile'),
+ 'union': require('./union'),
+ 'unionBy': require('./unionBy'),
+ 'unionWith': require('./unionWith'),
+ 'uniq': require('./uniq'),
+ 'uniqBy': require('./uniqBy'),
+ 'uniqWith': require('./uniqWith'),
+ 'unzip': require('./unzip'),
+ 'unzipWith': require('./unzipWith'),
+ 'without': require('./without'),
+ 'xor': require('./xor'),
+ 'xorBy': require('./xorBy'),
+ 'xorWith': require('./xorWith'),
+ 'zip': require('./zip'),
+ 'zipObject': require('./zipObject'),
+ 'zipObjectDeep': require('./zipObjectDeep'),
+ 'zipWith': require('./zipWith')
+};
diff --git a/node_modules/lodash/ary.js b/node_modules/lodash/ary.js
new file mode 100644
index 0000000..70c87d0
--- /dev/null
+++ b/node_modules/lodash/ary.js
@@ -0,0 +1,29 @@
+var createWrap = require('./_createWrap');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_ARY_FLAG = 128;
+
+/**
+ * Creates a function that invokes `func`, with up to `n` arguments,
+ * ignoring any additional arguments.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Function
+ * @param {Function} func The function to cap arguments for.
+ * @param {number} [n=func.length] The arity cap.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Function} Returns the new capped function.
+ * @example
+ *
+ * _.map(['6', '8', '10'], _.ary(parseInt, 1));
+ * // => [6, 8, 10]
+ */
+function ary(func, n, guard) {
+ n = guard ? undefined : n;
+ n = (func && n == null) ? func.length : n;
+ return createWrap(func, WRAP_ARY_FLAG, undefined, undefined, undefined, undefined, n);
+}
+
+module.exports = ary;
diff --git a/node_modules/lodash/assign.js b/node_modules/lodash/assign.js
new file mode 100644
index 0000000..909db26
--- /dev/null
+++ b/node_modules/lodash/assign.js
@@ -0,0 +1,58 @@
+var assignValue = require('./_assignValue'),
+ copyObject = require('./_copyObject'),
+ createAssigner = require('./_createAssigner'),
+ isArrayLike = require('./isArrayLike'),
+ isPrototype = require('./_isPrototype'),
+ keys = require('./keys');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Assigns own enumerable string keyed properties of source objects to the
+ * destination object. Source objects are applied from left to right.
+ * Subsequent sources overwrite property assignments of previous sources.
+ *
+ * **Note:** This method mutates `object` and is loosely based on
+ * [`Object.assign`](https://mdn.io/Object/assign).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.10.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.assignIn
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * function Bar() {
+ * this.c = 3;
+ * }
+ *
+ * Foo.prototype.b = 2;
+ * Bar.prototype.d = 4;
+ *
+ * _.assign({ 'a': 0 }, new Foo, new Bar);
+ * // => { 'a': 1, 'c': 3 }
+ */
+var assign = createAssigner(function(object, source) {
+ if (isPrototype(source) || isArrayLike(source)) {
+ copyObject(source, keys(source), object);
+ return;
+ }
+ for (var key in source) {
+ if (hasOwnProperty.call(source, key)) {
+ assignValue(object, key, source[key]);
+ }
+ }
+});
+
+module.exports = assign;
diff --git a/node_modules/lodash/assignIn.js b/node_modules/lodash/assignIn.js
new file mode 100644
index 0000000..e663473
--- /dev/null
+++ b/node_modules/lodash/assignIn.js
@@ -0,0 +1,40 @@
+var copyObject = require('./_copyObject'),
+ createAssigner = require('./_createAssigner'),
+ keysIn = require('./keysIn');
+
+/**
+ * This method is like `_.assign` except that it iterates over own and
+ * inherited source properties.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @alias extend
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.assign
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * function Bar() {
+ * this.c = 3;
+ * }
+ *
+ * Foo.prototype.b = 2;
+ * Bar.prototype.d = 4;
+ *
+ * _.assignIn({ 'a': 0 }, new Foo, new Bar);
+ * // => { 'a': 1, 'b': 2, 'c': 3, 'd': 4 }
+ */
+var assignIn = createAssigner(function(object, source) {
+ copyObject(source, keysIn(source), object);
+});
+
+module.exports = assignIn;
diff --git a/node_modules/lodash/assignInWith.js b/node_modules/lodash/assignInWith.js
new file mode 100644
index 0000000..68fcc0b
--- /dev/null
+++ b/node_modules/lodash/assignInWith.js
@@ -0,0 +1,38 @@
+var copyObject = require('./_copyObject'),
+ createAssigner = require('./_createAssigner'),
+ keysIn = require('./keysIn');
+
+/**
+ * This method is like `_.assignIn` except that it accepts `customizer`
+ * which is invoked to produce the assigned values. If `customizer` returns
+ * `undefined`, assignment is handled by the method instead. The `customizer`
+ * is invoked with five arguments: (objValue, srcValue, key, object, source).
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @alias extendWith
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} sources The source objects.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ * @see _.assignWith
+ * @example
+ *
+ * function customizer(objValue, srcValue) {
+ * return _.isUndefined(objValue) ? srcValue : objValue;
+ * }
+ *
+ * var defaults = _.partialRight(_.assignInWith, customizer);
+ *
+ * defaults({ 'a': 1 }, { 'b': 2 }, { 'a': 3 });
+ * // => { 'a': 1, 'b': 2 }
+ */
+var assignInWith = createAssigner(function(object, source, srcIndex, customizer) {
+ copyObject(source, keysIn(source), object, customizer);
+});
+
+module.exports = assignInWith;
diff --git a/node_modules/lodash/assignWith.js b/node_modules/lodash/assignWith.js
new file mode 100644
index 0000000..7dc6c76
--- /dev/null
+++ b/node_modules/lodash/assignWith.js
@@ -0,0 +1,37 @@
+var copyObject = require('./_copyObject'),
+ createAssigner = require('./_createAssigner'),
+ keys = require('./keys');
+
+/**
+ * This method is like `_.assign` except that it accepts `customizer`
+ * which is invoked to produce the assigned values. If `customizer` returns
+ * `undefined`, assignment is handled by the method instead. The `customizer`
+ * is invoked with five arguments: (objValue, srcValue, key, object, source).
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} sources The source objects.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ * @see _.assignInWith
+ * @example
+ *
+ * function customizer(objValue, srcValue) {
+ * return _.isUndefined(objValue) ? srcValue : objValue;
+ * }
+ *
+ * var defaults = _.partialRight(_.assignWith, customizer);
+ *
+ * defaults({ 'a': 1 }, { 'b': 2 }, { 'a': 3 });
+ * // => { 'a': 1, 'b': 2 }
+ */
+var assignWith = createAssigner(function(object, source, srcIndex, customizer) {
+ copyObject(source, keys(source), object, customizer);
+});
+
+module.exports = assignWith;
diff --git a/node_modules/lodash/at.js b/node_modules/lodash/at.js
new file mode 100644
index 0000000..781ee9e
--- /dev/null
+++ b/node_modules/lodash/at.js
@@ -0,0 +1,23 @@
+var baseAt = require('./_baseAt'),
+ flatRest = require('./_flatRest');
+
+/**
+ * Creates an array of values corresponding to `paths` of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.0.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {...(string|string[])} [paths] The property paths to pick.
+ * @returns {Array} Returns the picked values.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': 3 } }, 4] };
+ *
+ * _.at(object, ['a[0].b.c', 'a[1]']);
+ * // => [3, 4]
+ */
+var at = flatRest(baseAt);
+
+module.exports = at;
diff --git a/node_modules/lodash/attempt.js b/node_modules/lodash/attempt.js
new file mode 100644
index 0000000..624d015
--- /dev/null
+++ b/node_modules/lodash/attempt.js
@@ -0,0 +1,35 @@
+var apply = require('./_apply'),
+ baseRest = require('./_baseRest'),
+ isError = require('./isError');
+
+/**
+ * Attempts to invoke `func`, returning either the result or the caught error
+ * object. Any additional arguments are provided to `func` when it's invoked.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Util
+ * @param {Function} func The function to attempt.
+ * @param {...*} [args] The arguments to invoke `func` with.
+ * @returns {*} Returns the `func` result or error object.
+ * @example
+ *
+ * // Avoid throwing errors for invalid selectors.
+ * var elements = _.attempt(function(selector) {
+ * return document.querySelectorAll(selector);
+ * }, '>_>');
+ *
+ * if (_.isError(elements)) {
+ * elements = [];
+ * }
+ */
+var attempt = baseRest(function(func, args) {
+ try {
+ return apply(func, undefined, args);
+ } catch (e) {
+ return isError(e) ? e : new Error(e);
+ }
+});
+
+module.exports = attempt;
diff --git a/node_modules/lodash/before.js b/node_modules/lodash/before.js
new file mode 100644
index 0000000..a3e0a16
--- /dev/null
+++ b/node_modules/lodash/before.js
@@ -0,0 +1,40 @@
+var toInteger = require('./toInteger');
+
+/** Error message constants. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * Creates a function that invokes `func`, with the `this` binding and arguments
+ * of the created function, while it's called less than `n` times. Subsequent
+ * calls to the created function return the result of the last `func` invocation.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Function
+ * @param {number} n The number of calls at which `func` is no longer invoked.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * jQuery(element).on('click', _.before(5, addContactToList));
+ * // => Allows adding up to 4 contacts to the list.
+ */
+function before(n, func) {
+ var result;
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ n = toInteger(n);
+ return function() {
+ if (--n > 0) {
+ result = func.apply(this, arguments);
+ }
+ if (n <= 1) {
+ func = undefined;
+ }
+ return result;
+ };
+}
+
+module.exports = before;
diff --git a/node_modules/lodash/bind.js b/node_modules/lodash/bind.js
new file mode 100644
index 0000000..b1076e9
--- /dev/null
+++ b/node_modules/lodash/bind.js
@@ -0,0 +1,57 @@
+var baseRest = require('./_baseRest'),
+ createWrap = require('./_createWrap'),
+ getHolder = require('./_getHolder'),
+ replaceHolders = require('./_replaceHolders');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_BIND_FLAG = 1,
+ WRAP_PARTIAL_FLAG = 32;
+
+/**
+ * Creates a function that invokes `func` with the `this` binding of `thisArg`
+ * and `partials` prepended to the arguments it receives.
+ *
+ * The `_.bind.placeholder` value, which defaults to `_` in monolithic builds,
+ * may be used as a placeholder for partially applied arguments.
+ *
+ * **Note:** Unlike native `Function#bind`, this method doesn't set the "length"
+ * property of bound functions.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to bind.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * function greet(greeting, punctuation) {
+ * return greeting + ' ' + this.user + punctuation;
+ * }
+ *
+ * var object = { 'user': 'fred' };
+ *
+ * var bound = _.bind(greet, object, 'hi');
+ * bound('!');
+ * // => 'hi fred!'
+ *
+ * // Bound with placeholders.
+ * var bound = _.bind(greet, object, _, '!');
+ * bound('hi');
+ * // => 'hi fred!'
+ */
+var bind = baseRest(function(func, thisArg, partials) {
+ var bitmask = WRAP_BIND_FLAG;
+ if (partials.length) {
+ var holders = replaceHolders(partials, getHolder(bind));
+ bitmask |= WRAP_PARTIAL_FLAG;
+ }
+ return createWrap(func, bitmask, thisArg, partials, holders);
+});
+
+// Assign default placeholders.
+bind.placeholder = {};
+
+module.exports = bind;
diff --git a/node_modules/lodash/bindAll.js b/node_modules/lodash/bindAll.js
new file mode 100644
index 0000000..a35706d
--- /dev/null
+++ b/node_modules/lodash/bindAll.js
@@ -0,0 +1,41 @@
+var arrayEach = require('./_arrayEach'),
+ baseAssignValue = require('./_baseAssignValue'),
+ bind = require('./bind'),
+ flatRest = require('./_flatRest'),
+ toKey = require('./_toKey');
+
+/**
+ * Binds methods of an object to the object itself, overwriting the existing
+ * method.
+ *
+ * **Note:** This method doesn't set the "length" property of bound functions.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Util
+ * @param {Object} object The object to bind and assign the bound methods to.
+ * @param {...(string|string[])} methodNames The object method names to bind.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var view = {
+ * 'label': 'docs',
+ * 'click': function() {
+ * console.log('clicked ' + this.label);
+ * }
+ * };
+ *
+ * _.bindAll(view, ['click']);
+ * jQuery(element).on('click', view.click);
+ * // => Logs 'clicked docs' when clicked.
+ */
+var bindAll = flatRest(function(object, methodNames) {
+ arrayEach(methodNames, function(key) {
+ key = toKey(key);
+ baseAssignValue(object, key, bind(object[key], object));
+ });
+ return object;
+});
+
+module.exports = bindAll;
diff --git a/node_modules/lodash/bindKey.js b/node_modules/lodash/bindKey.js
new file mode 100644
index 0000000..f7fd64c
--- /dev/null
+++ b/node_modules/lodash/bindKey.js
@@ -0,0 +1,68 @@
+var baseRest = require('./_baseRest'),
+ createWrap = require('./_createWrap'),
+ getHolder = require('./_getHolder'),
+ replaceHolders = require('./_replaceHolders');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_BIND_FLAG = 1,
+ WRAP_BIND_KEY_FLAG = 2,
+ WRAP_PARTIAL_FLAG = 32;
+
+/**
+ * Creates a function that invokes the method at `object[key]` with `partials`
+ * prepended to the arguments it receives.
+ *
+ * This method differs from `_.bind` by allowing bound functions to reference
+ * methods that may be redefined or don't yet exist. See
+ * [Peter Michaux's article](http://peter.michaux.ca/articles/lazy-function-definition-pattern)
+ * for more details.
+ *
+ * The `_.bindKey.placeholder` value, which defaults to `_` in monolithic
+ * builds, may be used as a placeholder for partially applied arguments.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.10.0
+ * @category Function
+ * @param {Object} object The object to invoke the method on.
+ * @param {string} key The key of the method.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var object = {
+ * 'user': 'fred',
+ * 'greet': function(greeting, punctuation) {
+ * return greeting + ' ' + this.user + punctuation;
+ * }
+ * };
+ *
+ * var bound = _.bindKey(object, 'greet', 'hi');
+ * bound('!');
+ * // => 'hi fred!'
+ *
+ * object.greet = function(greeting, punctuation) {
+ * return greeting + 'ya ' + this.user + punctuation;
+ * };
+ *
+ * bound('!');
+ * // => 'hiya fred!'
+ *
+ * // Bound with placeholders.
+ * var bound = _.bindKey(object, 'greet', _, '!');
+ * bound('hi');
+ * // => 'hiya fred!'
+ */
+var bindKey = baseRest(function(object, key, partials) {
+ var bitmask = WRAP_BIND_FLAG | WRAP_BIND_KEY_FLAG;
+ if (partials.length) {
+ var holders = replaceHolders(partials, getHolder(bindKey));
+ bitmask |= WRAP_PARTIAL_FLAG;
+ }
+ return createWrap(key, bitmask, object, partials, holders);
+});
+
+// Assign default placeholders.
+bindKey.placeholder = {};
+
+module.exports = bindKey;
diff --git a/node_modules/lodash/camelCase.js b/node_modules/lodash/camelCase.js
new file mode 100644
index 0000000..d7390de
--- /dev/null
+++ b/node_modules/lodash/camelCase.js
@@ -0,0 +1,29 @@
+var capitalize = require('./capitalize'),
+ createCompounder = require('./_createCompounder');
+
+/**
+ * Converts `string` to [camel case](https://en.wikipedia.org/wiki/CamelCase).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the camel cased string.
+ * @example
+ *
+ * _.camelCase('Foo Bar');
+ * // => 'fooBar'
+ *
+ * _.camelCase('--foo-bar--');
+ * // => 'fooBar'
+ *
+ * _.camelCase('__FOO_BAR__');
+ * // => 'fooBar'
+ */
+var camelCase = createCompounder(function(result, word, index) {
+ word = word.toLowerCase();
+ return result + (index ? capitalize(word) : word);
+});
+
+module.exports = camelCase;
diff --git a/node_modules/lodash/capitalize.js b/node_modules/lodash/capitalize.js
new file mode 100644
index 0000000..3e1600e
--- /dev/null
+++ b/node_modules/lodash/capitalize.js
@@ -0,0 +1,23 @@
+var toString = require('./toString'),
+ upperFirst = require('./upperFirst');
+
+/**
+ * Converts the first character of `string` to upper case and the remaining
+ * to lower case.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to capitalize.
+ * @returns {string} Returns the capitalized string.
+ * @example
+ *
+ * _.capitalize('FRED');
+ * // => 'Fred'
+ */
+function capitalize(string) {
+ return upperFirst(toString(string).toLowerCase());
+}
+
+module.exports = capitalize;
diff --git a/node_modules/lodash/castArray.js b/node_modules/lodash/castArray.js
new file mode 100644
index 0000000..e470bdb
--- /dev/null
+++ b/node_modules/lodash/castArray.js
@@ -0,0 +1,44 @@
+var isArray = require('./isArray');
+
+/**
+ * Casts `value` as an array if it's not one.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.4.0
+ * @category Lang
+ * @param {*} value The value to inspect.
+ * @returns {Array} Returns the cast array.
+ * @example
+ *
+ * _.castArray(1);
+ * // => [1]
+ *
+ * _.castArray({ 'a': 1 });
+ * // => [{ 'a': 1 }]
+ *
+ * _.castArray('abc');
+ * // => ['abc']
+ *
+ * _.castArray(null);
+ * // => [null]
+ *
+ * _.castArray(undefined);
+ * // => [undefined]
+ *
+ * _.castArray();
+ * // => []
+ *
+ * var array = [1, 2, 3];
+ * console.log(_.castArray(array) === array);
+ * // => true
+ */
+function castArray() {
+ if (!arguments.length) {
+ return [];
+ }
+ var value = arguments[0];
+ return isArray(value) ? value : [value];
+}
+
+module.exports = castArray;
diff --git a/node_modules/lodash/ceil.js b/node_modules/lodash/ceil.js
new file mode 100644
index 0000000..56c8722
--- /dev/null
+++ b/node_modules/lodash/ceil.js
@@ -0,0 +1,26 @@
+var createRound = require('./_createRound');
+
+/**
+ * Computes `number` rounded up to `precision`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.10.0
+ * @category Math
+ * @param {number} number The number to round up.
+ * @param {number} [precision=0] The precision to round up to.
+ * @returns {number} Returns the rounded up number.
+ * @example
+ *
+ * _.ceil(4.006);
+ * // => 5
+ *
+ * _.ceil(6.004, 2);
+ * // => 6.01
+ *
+ * _.ceil(6040, -2);
+ * // => 6100
+ */
+var ceil = createRound('ceil');
+
+module.exports = ceil;
diff --git a/node_modules/lodash/chain.js b/node_modules/lodash/chain.js
new file mode 100644
index 0000000..f6cd647
--- /dev/null
+++ b/node_modules/lodash/chain.js
@@ -0,0 +1,38 @@
+var lodash = require('./wrapperLodash');
+
+/**
+ * Creates a `lodash` wrapper instance that wraps `value` with explicit method
+ * chain sequences enabled. The result of such sequences must be unwrapped
+ * with `_#value`.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.3.0
+ * @category Seq
+ * @param {*} value The value to wrap.
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 },
+ * { 'user': 'pebbles', 'age': 1 }
+ * ];
+ *
+ * var youngest = _
+ * .chain(users)
+ * .sortBy('age')
+ * .map(function(o) {
+ * return o.user + ' is ' + o.age;
+ * })
+ * .head()
+ * .value();
+ * // => 'pebbles is 1'
+ */
+function chain(value) {
+ var result = lodash(value);
+ result.__chain__ = true;
+ return result;
+}
+
+module.exports = chain;
diff --git a/node_modules/lodash/chunk.js b/node_modules/lodash/chunk.js
new file mode 100644
index 0000000..5b562fe
--- /dev/null
+++ b/node_modules/lodash/chunk.js
@@ -0,0 +1,50 @@
+var baseSlice = require('./_baseSlice'),
+ isIterateeCall = require('./_isIterateeCall'),
+ toInteger = require('./toInteger');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeCeil = Math.ceil,
+ nativeMax = Math.max;
+
+/**
+ * Creates an array of elements split into groups the length of `size`.
+ * If `array` can't be split evenly, the final chunk will be the remaining
+ * elements.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to process.
+ * @param {number} [size=1] The length of each chunk
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Array} Returns the new array of chunks.
+ * @example
+ *
+ * _.chunk(['a', 'b', 'c', 'd'], 2);
+ * // => [['a', 'b'], ['c', 'd']]
+ *
+ * _.chunk(['a', 'b', 'c', 'd'], 3);
+ * // => [['a', 'b', 'c'], ['d']]
+ */
+function chunk(array, size, guard) {
+ if ((guard ? isIterateeCall(array, size, guard) : size === undefined)) {
+ size = 1;
+ } else {
+ size = nativeMax(toInteger(size), 0);
+ }
+ var length = array == null ? 0 : array.length;
+ if (!length || size < 1) {
+ return [];
+ }
+ var index = 0,
+ resIndex = 0,
+ result = Array(nativeCeil(length / size));
+
+ while (index < length) {
+ result[resIndex++] = baseSlice(array, index, (index += size));
+ }
+ return result;
+}
+
+module.exports = chunk;
diff --git a/node_modules/lodash/clamp.js b/node_modules/lodash/clamp.js
new file mode 100644
index 0000000..91a72c9
--- /dev/null
+++ b/node_modules/lodash/clamp.js
@@ -0,0 +1,39 @@
+var baseClamp = require('./_baseClamp'),
+ toNumber = require('./toNumber');
+
+/**
+ * Clamps `number` within the inclusive `lower` and `upper` bounds.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Number
+ * @param {number} number The number to clamp.
+ * @param {number} [lower] The lower bound.
+ * @param {number} upper The upper bound.
+ * @returns {number} Returns the clamped number.
+ * @example
+ *
+ * _.clamp(-10, -5, 5);
+ * // => -5
+ *
+ * _.clamp(10, -5, 5);
+ * // => 5
+ */
+function clamp(number, lower, upper) {
+ if (upper === undefined) {
+ upper = lower;
+ lower = undefined;
+ }
+ if (upper !== undefined) {
+ upper = toNumber(upper);
+ upper = upper === upper ? upper : 0;
+ }
+ if (lower !== undefined) {
+ lower = toNumber(lower);
+ lower = lower === lower ? lower : 0;
+ }
+ return baseClamp(toNumber(number), lower, upper);
+}
+
+module.exports = clamp;
diff --git a/node_modules/lodash/clone.js b/node_modules/lodash/clone.js
new file mode 100644
index 0000000..dd439d6
--- /dev/null
+++ b/node_modules/lodash/clone.js
@@ -0,0 +1,36 @@
+var baseClone = require('./_baseClone');
+
+/** Used to compose bitmasks for cloning. */
+var CLONE_SYMBOLS_FLAG = 4;
+
+/**
+ * Creates a shallow clone of `value`.
+ *
+ * **Note:** This method is loosely based on the
+ * [structured clone algorithm](https://mdn.io/Structured_clone_algorithm)
+ * and supports cloning arrays, array buffers, booleans, date objects, maps,
+ * numbers, `Object` objects, regexes, sets, strings, symbols, and typed
+ * arrays. The own enumerable properties of `arguments` objects are cloned
+ * as plain objects. An empty object is returned for uncloneable values such
+ * as error objects, functions, DOM nodes, and WeakMaps.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to clone.
+ * @returns {*} Returns the cloned value.
+ * @see _.cloneDeep
+ * @example
+ *
+ * var objects = [{ 'a': 1 }, { 'b': 2 }];
+ *
+ * var shallow = _.clone(objects);
+ * console.log(shallow[0] === objects[0]);
+ * // => true
+ */
+function clone(value) {
+ return baseClone(value, CLONE_SYMBOLS_FLAG);
+}
+
+module.exports = clone;
diff --git a/node_modules/lodash/cloneDeep.js b/node_modules/lodash/cloneDeep.js
new file mode 100644
index 0000000..4425fbe
--- /dev/null
+++ b/node_modules/lodash/cloneDeep.js
@@ -0,0 +1,29 @@
+var baseClone = require('./_baseClone');
+
+/** Used to compose bitmasks for cloning. */
+var CLONE_DEEP_FLAG = 1,
+ CLONE_SYMBOLS_FLAG = 4;
+
+/**
+ * This method is like `_.clone` except that it recursively clones `value`.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.0.0
+ * @category Lang
+ * @param {*} value The value to recursively clone.
+ * @returns {*} Returns the deep cloned value.
+ * @see _.clone
+ * @example
+ *
+ * var objects = [{ 'a': 1 }, { 'b': 2 }];
+ *
+ * var deep = _.cloneDeep(objects);
+ * console.log(deep[0] === objects[0]);
+ * // => false
+ */
+function cloneDeep(value) {
+ return baseClone(value, CLONE_DEEP_FLAG | CLONE_SYMBOLS_FLAG);
+}
+
+module.exports = cloneDeep;
diff --git a/node_modules/lodash/cloneDeepWith.js b/node_modules/lodash/cloneDeepWith.js
new file mode 100644
index 0000000..fd9c6c0
--- /dev/null
+++ b/node_modules/lodash/cloneDeepWith.js
@@ -0,0 +1,40 @@
+var baseClone = require('./_baseClone');
+
+/** Used to compose bitmasks for cloning. */
+var CLONE_DEEP_FLAG = 1,
+ CLONE_SYMBOLS_FLAG = 4;
+
+/**
+ * This method is like `_.cloneWith` except that it recursively clones `value`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to recursively clone.
+ * @param {Function} [customizer] The function to customize cloning.
+ * @returns {*} Returns the deep cloned value.
+ * @see _.cloneWith
+ * @example
+ *
+ * function customizer(value) {
+ * if (_.isElement(value)) {
+ * return value.cloneNode(true);
+ * }
+ * }
+ *
+ * var el = _.cloneDeepWith(document.body, customizer);
+ *
+ * console.log(el === document.body);
+ * // => false
+ * console.log(el.nodeName);
+ * // => 'BODY'
+ * console.log(el.childNodes.length);
+ * // => 20
+ */
+function cloneDeepWith(value, customizer) {
+ customizer = typeof customizer == 'function' ? customizer : undefined;
+ return baseClone(value, CLONE_DEEP_FLAG | CLONE_SYMBOLS_FLAG, customizer);
+}
+
+module.exports = cloneDeepWith;
diff --git a/node_modules/lodash/cloneWith.js b/node_modules/lodash/cloneWith.js
new file mode 100644
index 0000000..d2f4e75
--- /dev/null
+++ b/node_modules/lodash/cloneWith.js
@@ -0,0 +1,42 @@
+var baseClone = require('./_baseClone');
+
+/** Used to compose bitmasks for cloning. */
+var CLONE_SYMBOLS_FLAG = 4;
+
+/**
+ * This method is like `_.clone` except that it accepts `customizer` which
+ * is invoked to produce the cloned value. If `customizer` returns `undefined`,
+ * cloning is handled by the method instead. The `customizer` is invoked with
+ * up to four arguments; (value [, index|key, object, stack]).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to clone.
+ * @param {Function} [customizer] The function to customize cloning.
+ * @returns {*} Returns the cloned value.
+ * @see _.cloneDeepWith
+ * @example
+ *
+ * function customizer(value) {
+ * if (_.isElement(value)) {
+ * return value.cloneNode(false);
+ * }
+ * }
+ *
+ * var el = _.cloneWith(document.body, customizer);
+ *
+ * console.log(el === document.body);
+ * // => false
+ * console.log(el.nodeName);
+ * // => 'BODY'
+ * console.log(el.childNodes.length);
+ * // => 0
+ */
+function cloneWith(value, customizer) {
+ customizer = typeof customizer == 'function' ? customizer : undefined;
+ return baseClone(value, CLONE_SYMBOLS_FLAG, customizer);
+}
+
+module.exports = cloneWith;
diff --git a/node_modules/lodash/collection.js b/node_modules/lodash/collection.js
new file mode 100644
index 0000000..77fe837
--- /dev/null
+++ b/node_modules/lodash/collection.js
@@ -0,0 +1,30 @@
+module.exports = {
+ 'countBy': require('./countBy'),
+ 'each': require('./each'),
+ 'eachRight': require('./eachRight'),
+ 'every': require('./every'),
+ 'filter': require('./filter'),
+ 'find': require('./find'),
+ 'findLast': require('./findLast'),
+ 'flatMap': require('./flatMap'),
+ 'flatMapDeep': require('./flatMapDeep'),
+ 'flatMapDepth': require('./flatMapDepth'),
+ 'forEach': require('./forEach'),
+ 'forEachRight': require('./forEachRight'),
+ 'groupBy': require('./groupBy'),
+ 'includes': require('./includes'),
+ 'invokeMap': require('./invokeMap'),
+ 'keyBy': require('./keyBy'),
+ 'map': require('./map'),
+ 'orderBy': require('./orderBy'),
+ 'partition': require('./partition'),
+ 'reduce': require('./reduce'),
+ 'reduceRight': require('./reduceRight'),
+ 'reject': require('./reject'),
+ 'sample': require('./sample'),
+ 'sampleSize': require('./sampleSize'),
+ 'shuffle': require('./shuffle'),
+ 'size': require('./size'),
+ 'some': require('./some'),
+ 'sortBy': require('./sortBy')
+};
diff --git a/node_modules/lodash/commit.js b/node_modules/lodash/commit.js
new file mode 100644
index 0000000..fe4db71
--- /dev/null
+++ b/node_modules/lodash/commit.js
@@ -0,0 +1,33 @@
+var LodashWrapper = require('./_LodashWrapper');
+
+/**
+ * Executes the chain sequence and returns the wrapped result.
+ *
+ * @name commit
+ * @memberOf _
+ * @since 3.2.0
+ * @category Seq
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var array = [1, 2];
+ * var wrapped = _(array).push(3);
+ *
+ * console.log(array);
+ * // => [1, 2]
+ *
+ * wrapped = wrapped.commit();
+ * console.log(array);
+ * // => [1, 2, 3]
+ *
+ * wrapped.last();
+ * // => 3
+ *
+ * console.log(array);
+ * // => [1, 2, 3]
+ */
+function wrapperCommit() {
+ return new LodashWrapper(this.value(), this.__chain__);
+}
+
+module.exports = wrapperCommit;
diff --git a/node_modules/lodash/compact.js b/node_modules/lodash/compact.js
new file mode 100644
index 0000000..031fab4
--- /dev/null
+++ b/node_modules/lodash/compact.js
@@ -0,0 +1,31 @@
+/**
+ * Creates an array with all falsey values removed. The values `false`, `null`,
+ * `0`, `""`, `undefined`, and `NaN` are falsey.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to compact.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * _.compact([0, 1, false, 2, '', 3]);
+ * // => [1, 2, 3]
+ */
+function compact(array) {
+ var index = -1,
+ length = array == null ? 0 : array.length,
+ resIndex = 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (value) {
+ result[resIndex++] = value;
+ }
+ }
+ return result;
+}
+
+module.exports = compact;
diff --git a/node_modules/lodash/concat.js b/node_modules/lodash/concat.js
new file mode 100644
index 0000000..1da48a4
--- /dev/null
+++ b/node_modules/lodash/concat.js
@@ -0,0 +1,43 @@
+var arrayPush = require('./_arrayPush'),
+ baseFlatten = require('./_baseFlatten'),
+ copyArray = require('./_copyArray'),
+ isArray = require('./isArray');
+
+/**
+ * Creates a new array concatenating `array` with any additional arrays
+ * and/or values.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to concatenate.
+ * @param {...*} [values] The values to concatenate.
+ * @returns {Array} Returns the new concatenated array.
+ * @example
+ *
+ * var array = [1];
+ * var other = _.concat(array, 2, [3], [[4]]);
+ *
+ * console.log(other);
+ * // => [1, 2, 3, [4]]
+ *
+ * console.log(array);
+ * // => [1]
+ */
+function concat() {
+ var length = arguments.length;
+ if (!length) {
+ return [];
+ }
+ var args = Array(length - 1),
+ array = arguments[0],
+ index = length;
+
+ while (index--) {
+ args[index - 1] = arguments[index];
+ }
+ return arrayPush(isArray(array) ? copyArray(array) : [array], baseFlatten(args, 1));
+}
+
+module.exports = concat;
diff --git a/node_modules/lodash/cond.js b/node_modules/lodash/cond.js
new file mode 100644
index 0000000..6455598
--- /dev/null
+++ b/node_modules/lodash/cond.js
@@ -0,0 +1,60 @@
+var apply = require('./_apply'),
+ arrayMap = require('./_arrayMap'),
+ baseIteratee = require('./_baseIteratee'),
+ baseRest = require('./_baseRest');
+
+/** Error message constants. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * Creates a function that iterates over `pairs` and invokes the corresponding
+ * function of the first predicate to return truthy. The predicate-function
+ * pairs are invoked with the `this` binding and arguments of the created
+ * function.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Util
+ * @param {Array} pairs The predicate-function pairs.
+ * @returns {Function} Returns the new composite function.
+ * @example
+ *
+ * var func = _.cond([
+ * [_.matches({ 'a': 1 }), _.constant('matches A')],
+ * [_.conforms({ 'b': _.isNumber }), _.constant('matches B')],
+ * [_.stubTrue, _.constant('no match')]
+ * ]);
+ *
+ * func({ 'a': 1, 'b': 2 });
+ * // => 'matches A'
+ *
+ * func({ 'a': 0, 'b': 1 });
+ * // => 'matches B'
+ *
+ * func({ 'a': '1', 'b': '2' });
+ * // => 'no match'
+ */
+function cond(pairs) {
+ var length = pairs == null ? 0 : pairs.length,
+ toIteratee = baseIteratee;
+
+ pairs = !length ? [] : arrayMap(pairs, function(pair) {
+ if (typeof pair[1] != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ return [toIteratee(pair[0]), pair[1]];
+ });
+
+ return baseRest(function(args) {
+ var index = -1;
+ while (++index < length) {
+ var pair = pairs[index];
+ if (apply(pair[0], this, args)) {
+ return apply(pair[1], this, args);
+ }
+ }
+ });
+}
+
+module.exports = cond;
diff --git a/node_modules/lodash/conforms.js b/node_modules/lodash/conforms.js
new file mode 100644
index 0000000..5501a94
--- /dev/null
+++ b/node_modules/lodash/conforms.js
@@ -0,0 +1,35 @@
+var baseClone = require('./_baseClone'),
+ baseConforms = require('./_baseConforms');
+
+/** Used to compose bitmasks for cloning. */
+var CLONE_DEEP_FLAG = 1;
+
+/**
+ * Creates a function that invokes the predicate properties of `source` with
+ * the corresponding property values of a given object, returning `true` if
+ * all predicates return truthy, else `false`.
+ *
+ * **Note:** The created function is equivalent to `_.conformsTo` with
+ * `source` partially applied.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Util
+ * @param {Object} source The object of property predicates to conform to.
+ * @returns {Function} Returns the new spec function.
+ * @example
+ *
+ * var objects = [
+ * { 'a': 2, 'b': 1 },
+ * { 'a': 1, 'b': 2 }
+ * ];
+ *
+ * _.filter(objects, _.conforms({ 'b': function(n) { return n > 1; } }));
+ * // => [{ 'a': 1, 'b': 2 }]
+ */
+function conforms(source) {
+ return baseConforms(baseClone(source, CLONE_DEEP_FLAG));
+}
+
+module.exports = conforms;
diff --git a/node_modules/lodash/conformsTo.js b/node_modules/lodash/conformsTo.js
new file mode 100644
index 0000000..b8a93eb
--- /dev/null
+++ b/node_modules/lodash/conformsTo.js
@@ -0,0 +1,32 @@
+var baseConformsTo = require('./_baseConformsTo'),
+ keys = require('./keys');
+
+/**
+ * Checks if `object` conforms to `source` by invoking the predicate
+ * properties of `source` with the corresponding property values of `object`.
+ *
+ * **Note:** This method is equivalent to `_.conforms` when `source` is
+ * partially applied.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.14.0
+ * @category Lang
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property predicates to conform to.
+ * @returns {boolean} Returns `true` if `object` conforms, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': 2 };
+ *
+ * _.conformsTo(object, { 'b': function(n) { return n > 1; } });
+ * // => true
+ *
+ * _.conformsTo(object, { 'b': function(n) { return n > 2; } });
+ * // => false
+ */
+function conformsTo(object, source) {
+ return source == null || baseConformsTo(object, source, keys(source));
+}
+
+module.exports = conformsTo;
diff --git a/node_modules/lodash/constant.js b/node_modules/lodash/constant.js
new file mode 100644
index 0000000..655ece3
--- /dev/null
+++ b/node_modules/lodash/constant.js
@@ -0,0 +1,26 @@
+/**
+ * Creates a function that returns `value`.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.4.0
+ * @category Util
+ * @param {*} value The value to return from the new function.
+ * @returns {Function} Returns the new constant function.
+ * @example
+ *
+ * var objects = _.times(2, _.constant({ 'a': 1 }));
+ *
+ * console.log(objects);
+ * // => [{ 'a': 1 }, { 'a': 1 }]
+ *
+ * console.log(objects[0] === objects[1]);
+ * // => true
+ */
+function constant(value) {
+ return function() {
+ return value;
+ };
+}
+
+module.exports = constant;
diff --git a/node_modules/lodash/core.js b/node_modules/lodash/core.js
new file mode 100644
index 0000000..be1d567
--- /dev/null
+++ b/node_modules/lodash/core.js
@@ -0,0 +1,3877 @@
+/**
+ * @license
+ * Lodash (Custom Build)
+ * Build: `lodash core -o ./dist/lodash.core.js`
+ * Copyright OpenJS Foundation and other contributors
+ * Released under MIT license
+ * Based on Underscore.js 1.8.3
+ * Copyright Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ */
+;(function() {
+
+ /** Used as a safe reference for `undefined` in pre-ES5 environments. */
+ var undefined;
+
+ /** Used as the semantic version number. */
+ var VERSION = '4.17.21';
+
+ /** Error message constants. */
+ var FUNC_ERROR_TEXT = 'Expected a function';
+
+ /** Used to compose bitmasks for value comparisons. */
+ var COMPARE_PARTIAL_FLAG = 1,
+ COMPARE_UNORDERED_FLAG = 2;
+
+ /** Used to compose bitmasks for function metadata. */
+ var WRAP_BIND_FLAG = 1,
+ WRAP_PARTIAL_FLAG = 32;
+
+ /** Used as references for various `Number` constants. */
+ var INFINITY = 1 / 0,
+ MAX_SAFE_INTEGER = 9007199254740991;
+
+ /** `Object#toString` result references. */
+ var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ asyncTag = '[object AsyncFunction]',
+ boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ funcTag = '[object Function]',
+ genTag = '[object GeneratorFunction]',
+ numberTag = '[object Number]',
+ objectTag = '[object Object]',
+ proxyTag = '[object Proxy]',
+ regexpTag = '[object RegExp]',
+ stringTag = '[object String]';
+
+ /** Used to match HTML entities and HTML characters. */
+ var reUnescapedHtml = /[&<>"']/g,
+ reHasUnescapedHtml = RegExp(reUnescapedHtml.source);
+
+ /** Used to detect unsigned integer values. */
+ var reIsUint = /^(?:0|[1-9]\d*)$/;
+
+ /** Used to map characters to HTML entities. */
+ var htmlEscapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+ };
+
+ /** Detect free variable `global` from Node.js. */
+ var freeGlobal = typeof global == 'object' && global && global.Object === Object && global;
+
+ /** Detect free variable `self`. */
+ var freeSelf = typeof self == 'object' && self && self.Object === Object && self;
+
+ /** Used as a reference to the global object. */
+ var root = freeGlobal || freeSelf || Function('return this')();
+
+ /** Detect free variable `exports`. */
+ var freeExports = typeof exports == 'object' && exports && !exports.nodeType && exports;
+
+ /** Detect free variable `module`. */
+ var freeModule = freeExports && typeof module == 'object' && module && !module.nodeType && module;
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Appends the elements of `values` to `array`.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to append.
+ * @returns {Array} Returns `array`.
+ */
+ function arrayPush(array, values) {
+ array.push.apply(array, values);
+ return array;
+ }
+
+ /**
+ * The base implementation of `_.findIndex` and `_.findLastIndex` without
+ * support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {number} fromIndex The index to search from.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+ function baseFindIndex(array, predicate, fromIndex, fromRight) {
+ var length = array.length,
+ index = fromIndex + (fromRight ? 1 : -1);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ if (predicate(array[index], index, array)) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * The base implementation of `_.property` without support for deep paths.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @returns {Function} Returns the new accessor function.
+ */
+ function baseProperty(key) {
+ return function(object) {
+ return object == null ? undefined : object[key];
+ };
+ }
+
+ /**
+ * The base implementation of `_.propertyOf` without support for deep paths.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Function} Returns the new accessor function.
+ */
+ function basePropertyOf(object) {
+ return function(key) {
+ return object == null ? undefined : object[key];
+ };
+ }
+
+ /**
+ * The base implementation of `_.reduce` and `_.reduceRight`, without support
+ * for iteratee shorthands, which iterates over `collection` using `eachFunc`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} accumulator The initial value.
+ * @param {boolean} initAccum Specify using the first or last element of
+ * `collection` as the initial value.
+ * @param {Function} eachFunc The function to iterate over `collection`.
+ * @returns {*} Returns the accumulated value.
+ */
+ function baseReduce(collection, iteratee, accumulator, initAccum, eachFunc) {
+ eachFunc(collection, function(value, index, collection) {
+ accumulator = initAccum
+ ? (initAccum = false, value)
+ : iteratee(accumulator, value, index, collection);
+ });
+ return accumulator;
+ }
+
+ /**
+ * The base implementation of `_.values` and `_.valuesIn` which creates an
+ * array of `object` property values corresponding to the property names
+ * of `props`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} props The property names to get values for.
+ * @returns {Object} Returns the array of property values.
+ */
+ function baseValues(object, props) {
+ return baseMap(props, function(key) {
+ return object[key];
+ });
+ }
+
+ /**
+ * Used by `_.escape` to convert characters to HTML entities.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+ var escapeHtmlChar = basePropertyOf(htmlEscapes);
+
+ /**
+ * Creates a unary function that invokes `func` with its argument transformed.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {Function} transform The argument transform.
+ * @returns {Function} Returns the new function.
+ */
+ function overArg(func, transform) {
+ return function(arg) {
+ return func(transform(arg));
+ };
+ }
+
+ /*--------------------------------------------------------------------------*/
+
+ /** Used for built-in method references. */
+ var arrayProto = Array.prototype,
+ objectProto = Object.prototype;
+
+ /** Used to check objects for own properties. */
+ var hasOwnProperty = objectProto.hasOwnProperty;
+
+ /** Used to generate unique IDs. */
+ var idCounter = 0;
+
+ /**
+ * Used to resolve the
+ * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+ var nativeObjectToString = objectProto.toString;
+
+ /** Used to restore the original `_` reference in `_.noConflict`. */
+ var oldDash = root._;
+
+ /** Built-in value references. */
+ var objectCreate = Object.create,
+ propertyIsEnumerable = objectProto.propertyIsEnumerable;
+
+ /* Built-in method references for those with the same name as other `lodash` methods. */
+ var nativeIsFinite = root.isFinite,
+ nativeKeys = overArg(Object.keys, Object),
+ nativeMax = Math.max;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a `lodash` object which wraps `value` to enable implicit method
+ * chain sequences. Methods that operate on and return arrays, collections,
+ * and functions can be chained together. Methods that retrieve a single value
+ * or may return a primitive value will automatically end the chain sequence
+ * and return the unwrapped value. Otherwise, the value must be unwrapped
+ * with `_#value`.
+ *
+ * Explicit chain sequences, which must be unwrapped with `_#value`, may be
+ * enabled using `_.chain`.
+ *
+ * The execution of chained methods is lazy, that is, it's deferred until
+ * `_#value` is implicitly or explicitly called.
+ *
+ * Lazy evaluation allows several methods to support shortcut fusion.
+ * Shortcut fusion is an optimization to merge iteratee calls; this avoids
+ * the creation of intermediate arrays and can greatly reduce the number of
+ * iteratee executions. Sections of a chain sequence qualify for shortcut
+ * fusion if the section is applied to an array and iteratees accept only
+ * one argument. The heuristic for whether a section qualifies for shortcut
+ * fusion is subject to change.
+ *
+ * Chaining is supported in custom builds as long as the `_#value` method is
+ * directly or indirectly included in the build.
+ *
+ * In addition to lodash methods, wrappers have `Array` and `String` methods.
+ *
+ * The wrapper `Array` methods are:
+ * `concat`, `join`, `pop`, `push`, `shift`, `sort`, `splice`, and `unshift`
+ *
+ * The wrapper `String` methods are:
+ * `replace` and `split`
+ *
+ * The wrapper methods that support shortcut fusion are:
+ * `at`, `compact`, `drop`, `dropRight`, `dropWhile`, `filter`, `find`,
+ * `findLast`, `head`, `initial`, `last`, `map`, `reject`, `reverse`, `slice`,
+ * `tail`, `take`, `takeRight`, `takeRightWhile`, `takeWhile`, and `toArray`
+ *
+ * The chainable wrapper methods are:
+ * `after`, `ary`, `assign`, `assignIn`, `assignInWith`, `assignWith`, `at`,
+ * `before`, `bind`, `bindAll`, `bindKey`, `castArray`, `chain`, `chunk`,
+ * `commit`, `compact`, `concat`, `conforms`, `constant`, `countBy`, `create`,
+ * `curry`, `debounce`, `defaults`, `defaultsDeep`, `defer`, `delay`,
+ * `difference`, `differenceBy`, `differenceWith`, `drop`, `dropRight`,
+ * `dropRightWhile`, `dropWhile`, `extend`, `extendWith`, `fill`, `filter`,
+ * `flatMap`, `flatMapDeep`, `flatMapDepth`, `flatten`, `flattenDeep`,
+ * `flattenDepth`, `flip`, `flow`, `flowRight`, `fromPairs`, `functions`,
+ * `functionsIn`, `groupBy`, `initial`, `intersection`, `intersectionBy`,
+ * `intersectionWith`, `invert`, `invertBy`, `invokeMap`, `iteratee`, `keyBy`,
+ * `keys`, `keysIn`, `map`, `mapKeys`, `mapValues`, `matches`, `matchesProperty`,
+ * `memoize`, `merge`, `mergeWith`, `method`, `methodOf`, `mixin`, `negate`,
+ * `nthArg`, `omit`, `omitBy`, `once`, `orderBy`, `over`, `overArgs`,
+ * `overEvery`, `overSome`, `partial`, `partialRight`, `partition`, `pick`,
+ * `pickBy`, `plant`, `property`, `propertyOf`, `pull`, `pullAll`, `pullAllBy`,
+ * `pullAllWith`, `pullAt`, `push`, `range`, `rangeRight`, `rearg`, `reject`,
+ * `remove`, `rest`, `reverse`, `sampleSize`, `set`, `setWith`, `shuffle`,
+ * `slice`, `sort`, `sortBy`, `splice`, `spread`, `tail`, `take`, `takeRight`,
+ * `takeRightWhile`, `takeWhile`, `tap`, `throttle`, `thru`, `toArray`,
+ * `toPairs`, `toPairsIn`, `toPath`, `toPlainObject`, `transform`, `unary`,
+ * `union`, `unionBy`, `unionWith`, `uniq`, `uniqBy`, `uniqWith`, `unset`,
+ * `unshift`, `unzip`, `unzipWith`, `update`, `updateWith`, `values`,
+ * `valuesIn`, `without`, `wrap`, `xor`, `xorBy`, `xorWith`, `zip`,
+ * `zipObject`, `zipObjectDeep`, and `zipWith`
+ *
+ * The wrapper methods that are **not** chainable by default are:
+ * `add`, `attempt`, `camelCase`, `capitalize`, `ceil`, `clamp`, `clone`,
+ * `cloneDeep`, `cloneDeepWith`, `cloneWith`, `conformsTo`, `deburr`,
+ * `defaultTo`, `divide`, `each`, `eachRight`, `endsWith`, `eq`, `escape`,
+ * `escapeRegExp`, `every`, `find`, `findIndex`, `findKey`, `findLast`,
+ * `findLastIndex`, `findLastKey`, `first`, `floor`, `forEach`, `forEachRight`,
+ * `forIn`, `forInRight`, `forOwn`, `forOwnRight`, `get`, `gt`, `gte`, `has`,
+ * `hasIn`, `head`, `identity`, `includes`, `indexOf`, `inRange`, `invoke`,
+ * `isArguments`, `isArray`, `isArrayBuffer`, `isArrayLike`, `isArrayLikeObject`,
+ * `isBoolean`, `isBuffer`, `isDate`, `isElement`, `isEmpty`, `isEqual`,
+ * `isEqualWith`, `isError`, `isFinite`, `isFunction`, `isInteger`, `isLength`,
+ * `isMap`, `isMatch`, `isMatchWith`, `isNaN`, `isNative`, `isNil`, `isNull`,
+ * `isNumber`, `isObject`, `isObjectLike`, `isPlainObject`, `isRegExp`,
+ * `isSafeInteger`, `isSet`, `isString`, `isUndefined`, `isTypedArray`,
+ * `isWeakMap`, `isWeakSet`, `join`, `kebabCase`, `last`, `lastIndexOf`,
+ * `lowerCase`, `lowerFirst`, `lt`, `lte`, `max`, `maxBy`, `mean`, `meanBy`,
+ * `min`, `minBy`, `multiply`, `noConflict`, `noop`, `now`, `nth`, `pad`,
+ * `padEnd`, `padStart`, `parseInt`, `pop`, `random`, `reduce`, `reduceRight`,
+ * `repeat`, `result`, `round`, `runInContext`, `sample`, `shift`, `size`,
+ * `snakeCase`, `some`, `sortedIndex`, `sortedIndexBy`, `sortedLastIndex`,
+ * `sortedLastIndexBy`, `startCase`, `startsWith`, `stubArray`, `stubFalse`,
+ * `stubObject`, `stubString`, `stubTrue`, `subtract`, `sum`, `sumBy`,
+ * `template`, `times`, `toFinite`, `toInteger`, `toJSON`, `toLength`,
+ * `toLower`, `toNumber`, `toSafeInteger`, `toString`, `toUpper`, `trim`,
+ * `trimEnd`, `trimStart`, `truncate`, `unescape`, `uniqueId`, `upperCase`,
+ * `upperFirst`, `value`, and `words`
+ *
+ * @name _
+ * @constructor
+ * @category Seq
+ * @param {*} value The value to wrap in a `lodash` instance.
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * var wrapped = _([1, 2, 3]);
+ *
+ * // Returns an unwrapped value.
+ * wrapped.reduce(_.add);
+ * // => 6
+ *
+ * // Returns a wrapped value.
+ * var squares = wrapped.map(square);
+ *
+ * _.isArray(squares);
+ * // => false
+ *
+ * _.isArray(squares.value());
+ * // => true
+ */
+ function lodash(value) {
+ return value instanceof LodashWrapper
+ ? value
+ : new LodashWrapper(value);
+ }
+
+ /**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} proto The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+ var baseCreate = (function() {
+ function object() {}
+ return function(proto) {
+ if (!isObject(proto)) {
+ return {};
+ }
+ if (objectCreate) {
+ return objectCreate(proto);
+ }
+ object.prototype = proto;
+ var result = new object;
+ object.prototype = undefined;
+ return result;
+ };
+ }());
+
+ /**
+ * The base constructor for creating `lodash` wrapper objects.
+ *
+ * @private
+ * @param {*} value The value to wrap.
+ * @param {boolean} [chainAll] Enable explicit method chain sequences.
+ */
+ function LodashWrapper(value, chainAll) {
+ this.__wrapped__ = value;
+ this.__actions__ = [];
+ this.__chain__ = !!chainAll;
+ }
+
+ LodashWrapper.prototype = baseCreate(lodash.prototype);
+ LodashWrapper.prototype.constructor = LodashWrapper;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Assigns `value` to `key` of `object` if the existing value is not equivalent
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+ function assignValue(object, key, value) {
+ var objValue = object[key];
+ if (!(hasOwnProperty.call(object, key) && eq(objValue, value)) ||
+ (value === undefined && !(key in object))) {
+ baseAssignValue(object, key, value);
+ }
+ }
+
+ /**
+ * The base implementation of `assignValue` and `assignMergeValue` without
+ * value checks.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+ function baseAssignValue(object, key, value) {
+ object[key] = value;
+ }
+
+ /**
+ * The base implementation of `_.delay` and `_.defer` which accepts `args`
+ * to provide to `func`.
+ *
+ * @private
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay invocation.
+ * @param {Array} args The arguments to provide to `func`.
+ * @returns {number|Object} Returns the timer id or timeout object.
+ */
+ function baseDelay(func, wait, args) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ return setTimeout(function() { func.apply(undefined, args); }, wait);
+ }
+
+ /**
+ * The base implementation of `_.forEach` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object} Returns `collection`.
+ */
+ var baseEach = createBaseEach(baseForOwn);
+
+ /**
+ * The base implementation of `_.every` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`
+ */
+ function baseEvery(collection, predicate) {
+ var result = true;
+ baseEach(collection, function(value, index, collection) {
+ result = !!predicate(value, index, collection);
+ return result;
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of methods like `_.max` and `_.min` which accepts a
+ * `comparator` to determine the extremum value.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The iteratee invoked per iteration.
+ * @param {Function} comparator The comparator used to compare values.
+ * @returns {*} Returns the extremum value.
+ */
+ function baseExtremum(array, iteratee, comparator) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ var value = array[index],
+ current = iteratee(value);
+
+ if (current != null && (computed === undefined
+ ? (current === current && !false)
+ : comparator(current, computed)
+ )) {
+ var computed = current,
+ result = value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.filter` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ */
+ function baseFilter(collection, predicate) {
+ var result = [];
+ baseEach(collection, function(value, index, collection) {
+ if (predicate(value, index, collection)) {
+ result.push(value);
+ }
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.flatten` with support for restricting flattening.
+ *
+ * @private
+ * @param {Array} array The array to flatten.
+ * @param {number} depth The maximum recursion depth.
+ * @param {boolean} [predicate=isFlattenable] The function invoked per iteration.
+ * @param {boolean} [isStrict] Restrict to values that pass `predicate` checks.
+ * @param {Array} [result=[]] The initial result value.
+ * @returns {Array} Returns the new flattened array.
+ */
+ function baseFlatten(array, depth, predicate, isStrict, result) {
+ var index = -1,
+ length = array.length;
+
+ predicate || (predicate = isFlattenable);
+ result || (result = []);
+
+ while (++index < length) {
+ var value = array[index];
+ if (depth > 0 && predicate(value)) {
+ if (depth > 1) {
+ // Recursively flatten arrays (susceptible to call stack limits).
+ baseFlatten(value, depth - 1, predicate, isStrict, result);
+ } else {
+ arrayPush(result, value);
+ }
+ } else if (!isStrict) {
+ result[result.length] = value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `baseForOwn` which iterates over `object`
+ * properties returned by `keysFunc` and invokes `iteratee` for each property.
+ * Iteratee functions may exit iteration early by explicitly returning `false`.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+ var baseFor = createBaseFor();
+
+ /**
+ * The base implementation of `_.forOwn` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+ function baseForOwn(object, iteratee) {
+ return object && baseFor(object, iteratee, keys);
+ }
+
+ /**
+ * The base implementation of `_.functions` which creates an array of
+ * `object` function property names filtered from `props`.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Array} props The property names to filter.
+ * @returns {Array} Returns the function names.
+ */
+ function baseFunctions(object, props) {
+ return baseFilter(props, function(key) {
+ return isFunction(object[key]);
+ });
+ }
+
+ /**
+ * The base implementation of `getTag` without fallbacks for buggy environments.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the `toStringTag`.
+ */
+ function baseGetTag(value) {
+ return objectToString(value);
+ }
+
+ /**
+ * The base implementation of `_.gt` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is greater than `other`,
+ * else `false`.
+ */
+ function baseGt(value, other) {
+ return value > other;
+ }
+
+ /**
+ * The base implementation of `_.isArguments`.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ */
+ var baseIsArguments = noop;
+
+ /**
+ * The base implementation of `_.isDate` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a date object, else `false`.
+ */
+ function baseIsDate(value) {
+ return isObjectLike(value) && baseGetTag(value) == dateTag;
+ }
+
+ /**
+ * The base implementation of `_.isEqual` which supports partial comparisons
+ * and tracks traversed objects.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @param {boolean} bitmask The bitmask flags.
+ * 1 - Unordered comparison
+ * 2 - Partial comparison
+ * @param {Function} [customizer] The function to customize comparisons.
+ * @param {Object} [stack] Tracks traversed `value` and `other` objects.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ */
+ function baseIsEqual(value, other, bitmask, customizer, stack) {
+ if (value === other) {
+ return true;
+ }
+ if (value == null || other == null || (!isObjectLike(value) && !isObjectLike(other))) {
+ return value !== value && other !== other;
+ }
+ return baseIsEqualDeep(value, other, bitmask, customizer, baseIsEqual, stack);
+ }
+
+ /**
+ * A specialized version of `baseIsEqual` for arrays and objects which performs
+ * deep comparisons and tracks traversed objects enabling objects with circular
+ * references to be compared.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} [stack] Tracks traversed `object` and `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+ function baseIsEqualDeep(object, other, bitmask, customizer, equalFunc, stack) {
+ var objIsArr = isArray(object),
+ othIsArr = isArray(other),
+ objTag = objIsArr ? arrayTag : baseGetTag(object),
+ othTag = othIsArr ? arrayTag : baseGetTag(other);
+
+ objTag = objTag == argsTag ? objectTag : objTag;
+ othTag = othTag == argsTag ? objectTag : othTag;
+
+ var objIsObj = objTag == objectTag,
+ othIsObj = othTag == objectTag,
+ isSameTag = objTag == othTag;
+
+ stack || (stack = []);
+ var objStack = find(stack, function(entry) {
+ return entry[0] == object;
+ });
+ var othStack = find(stack, function(entry) {
+ return entry[0] == other;
+ });
+ if (objStack && othStack) {
+ return objStack[1] == other;
+ }
+ stack.push([object, other]);
+ stack.push([other, object]);
+ if (isSameTag && !objIsObj) {
+ var result = (objIsArr)
+ ? equalArrays(object, other, bitmask, customizer, equalFunc, stack)
+ : equalByTag(object, other, objTag, bitmask, customizer, equalFunc, stack);
+ stack.pop();
+ return result;
+ }
+ if (!(bitmask & COMPARE_PARTIAL_FLAG)) {
+ var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
+ othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
+
+ if (objIsWrapped || othIsWrapped) {
+ var objUnwrapped = objIsWrapped ? object.value() : object,
+ othUnwrapped = othIsWrapped ? other.value() : other;
+
+ var result = equalFunc(objUnwrapped, othUnwrapped, bitmask, customizer, stack);
+ stack.pop();
+ return result;
+ }
+ }
+ if (!isSameTag) {
+ return false;
+ }
+ var result = equalObjects(object, other, bitmask, customizer, equalFunc, stack);
+ stack.pop();
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.isRegExp` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a regexp, else `false`.
+ */
+ function baseIsRegExp(value) {
+ return isObjectLike(value) && baseGetTag(value) == regexpTag;
+ }
+
+ /**
+ * The base implementation of `_.iteratee`.
+ *
+ * @private
+ * @param {*} [value=_.identity] The value to convert to an iteratee.
+ * @returns {Function} Returns the iteratee.
+ */
+ function baseIteratee(func) {
+ if (typeof func == 'function') {
+ return func;
+ }
+ if (func == null) {
+ return identity;
+ }
+ return (typeof func == 'object' ? baseMatches : baseProperty)(func);
+ }
+
+ /**
+ * The base implementation of `_.lt` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is less than `other`,
+ * else `false`.
+ */
+ function baseLt(value, other) {
+ return value < other;
+ }
+
+ /**
+ * The base implementation of `_.map` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ */
+ function baseMap(collection, iteratee) {
+ var index = -1,
+ result = isArrayLike(collection) ? Array(collection.length) : [];
+
+ baseEach(collection, function(value, key, collection) {
+ result[++index] = iteratee(value, key, collection);
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.matches` which doesn't clone `source`.
+ *
+ * @private
+ * @param {Object} source The object of property values to match.
+ * @returns {Function} Returns the new spec function.
+ */
+ function baseMatches(source) {
+ var props = nativeKeys(source);
+ return function(object) {
+ var length = props.length;
+ if (object == null) {
+ return !length;
+ }
+ object = Object(object);
+ while (length--) {
+ var key = props[length];
+ if (!(key in object &&
+ baseIsEqual(source[key], object[key], COMPARE_PARTIAL_FLAG | COMPARE_UNORDERED_FLAG)
+ )) {
+ return false;
+ }
+ }
+ return true;
+ };
+ }
+
+ /**
+ * The base implementation of `_.pick` without support for individual
+ * property identifiers.
+ *
+ * @private
+ * @param {Object} object The source object.
+ * @param {string[]} paths The property paths to pick.
+ * @returns {Object} Returns the new object.
+ */
+ function basePick(object, props) {
+ object = Object(object);
+ return reduce(props, function(result, key) {
+ if (key in object) {
+ result[key] = object[key];
+ }
+ return result;
+ }, {});
+ }
+
+ /**
+ * The base implementation of `_.rest` which doesn't validate or coerce arguments.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @returns {Function} Returns the new function.
+ */
+ function baseRest(func, start) {
+ return setToString(overRest(func, start, identity), func + '');
+ }
+
+ /**
+ * The base implementation of `_.slice` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to slice.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the slice of `array`.
+ */
+ function baseSlice(array, start, end) {
+ var index = -1,
+ length = array.length;
+
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = end > length ? length : end;
+ if (end < 0) {
+ end += length;
+ }
+ length = start > end ? 0 : ((end - start) >>> 0);
+ start >>>= 0;
+
+ var result = Array(length);
+ while (++index < length) {
+ result[index] = array[index + start];
+ }
+ return result;
+ }
+
+ /**
+ * Copies the values of `source` to `array`.
+ *
+ * @private
+ * @param {Array} source The array to copy values from.
+ * @param {Array} [array=[]] The array to copy values to.
+ * @returns {Array} Returns `array`.
+ */
+ function copyArray(source) {
+ return baseSlice(source, 0, source.length);
+ }
+
+ /**
+ * The base implementation of `_.some` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ */
+ function baseSome(collection, predicate) {
+ var result;
+
+ baseEach(collection, function(value, index, collection) {
+ result = predicate(value, index, collection);
+ return !result;
+ });
+ return !!result;
+ }
+
+ /**
+ * The base implementation of `wrapperValue` which returns the result of
+ * performing a sequence of actions on the unwrapped `value`, where each
+ * successive action is supplied the return value of the previous.
+ *
+ * @private
+ * @param {*} value The unwrapped value.
+ * @param {Array} actions Actions to perform to resolve the unwrapped value.
+ * @returns {*} Returns the resolved value.
+ */
+ function baseWrapperValue(value, actions) {
+ var result = value;
+ return reduce(actions, function(result, action) {
+ return action.func.apply(action.thisArg, arrayPush([result], action.args));
+ }, result);
+ }
+
+ /**
+ * Compares values to sort them in ascending order.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {number} Returns the sort order indicator for `value`.
+ */
+ function compareAscending(value, other) {
+ if (value !== other) {
+ var valIsDefined = value !== undefined,
+ valIsNull = value === null,
+ valIsReflexive = value === value,
+ valIsSymbol = false;
+
+ var othIsDefined = other !== undefined,
+ othIsNull = other === null,
+ othIsReflexive = other === other,
+ othIsSymbol = false;
+
+ if ((!othIsNull && !othIsSymbol && !valIsSymbol && value > other) ||
+ (valIsSymbol && othIsDefined && othIsReflexive && !othIsNull && !othIsSymbol) ||
+ (valIsNull && othIsDefined && othIsReflexive) ||
+ (!valIsDefined && othIsReflexive) ||
+ !valIsReflexive) {
+ return 1;
+ }
+ if ((!valIsNull && !valIsSymbol && !othIsSymbol && value < other) ||
+ (othIsSymbol && valIsDefined && valIsReflexive && !valIsNull && !valIsSymbol) ||
+ (othIsNull && valIsDefined && valIsReflexive) ||
+ (!othIsDefined && valIsReflexive) ||
+ !othIsReflexive) {
+ return -1;
+ }
+ }
+ return 0;
+ }
+
+ /**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property identifiers to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @param {Function} [customizer] The function to customize copied values.
+ * @returns {Object} Returns `object`.
+ */
+ function copyObject(source, props, object, customizer) {
+ var isNew = !object;
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+
+ var newValue = customizer
+ ? customizer(object[key], source[key], key, object, source)
+ : undefined;
+
+ if (newValue === undefined) {
+ newValue = source[key];
+ }
+ if (isNew) {
+ baseAssignValue(object, key, newValue);
+ } else {
+ assignValue(object, key, newValue);
+ }
+ }
+ return object;
+ }
+
+ /**
+ * Creates a function like `_.assign`.
+ *
+ * @private
+ * @param {Function} assigner The function to assign values.
+ * @returns {Function} Returns the new assigner function.
+ */
+ function createAssigner(assigner) {
+ return baseRest(function(object, sources) {
+ var index = -1,
+ length = sources.length,
+ customizer = length > 1 ? sources[length - 1] : undefined;
+
+ customizer = (assigner.length > 3 && typeof customizer == 'function')
+ ? (length--, customizer)
+ : undefined;
+
+ object = Object(object);
+ while (++index < length) {
+ var source = sources[index];
+ if (source) {
+ assigner(object, source, index, customizer);
+ }
+ }
+ return object;
+ });
+ }
+
+ /**
+ * Creates a `baseEach` or `baseEachRight` function.
+ *
+ * @private
+ * @param {Function} eachFunc The function to iterate over a collection.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+ function createBaseEach(eachFunc, fromRight) {
+ return function(collection, iteratee) {
+ if (collection == null) {
+ return collection;
+ }
+ if (!isArrayLike(collection)) {
+ return eachFunc(collection, iteratee);
+ }
+ var length = collection.length,
+ index = fromRight ? length : -1,
+ iterable = Object(collection);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ if (iteratee(iterable[index], index, iterable) === false) {
+ break;
+ }
+ }
+ return collection;
+ };
+ }
+
+ /**
+ * Creates a base function for methods like `_.forIn` and `_.forOwn`.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+ function createBaseFor(fromRight) {
+ return function(object, iteratee, keysFunc) {
+ var index = -1,
+ iterable = Object(object),
+ props = keysFunc(object),
+ length = props.length;
+
+ while (length--) {
+ var key = props[fromRight ? length : ++index];
+ if (iteratee(iterable[key], key, iterable) === false) {
+ break;
+ }
+ }
+ return object;
+ };
+ }
+
+ /**
+ * Creates a function that produces an instance of `Ctor` regardless of
+ * whether it was invoked as part of a `new` expression or by `call` or `apply`.
+ *
+ * @private
+ * @param {Function} Ctor The constructor to wrap.
+ * @returns {Function} Returns the new wrapped function.
+ */
+ function createCtor(Ctor) {
+ return function() {
+ // Use a `switch` statement to work with class constructors. See
+ // http://ecma-international.org/ecma-262/7.0/#sec-ecmascript-function-objects-call-thisargument-argumentslist
+ // for more details.
+ var args = arguments;
+ var thisBinding = baseCreate(Ctor.prototype),
+ result = Ctor.apply(thisBinding, args);
+
+ // Mimic the constructor's `return` behavior.
+ // See https://es5.github.io/#x13.2.2 for more details.
+ return isObject(result) ? result : thisBinding;
+ };
+ }
+
+ /**
+ * Creates a `_.find` or `_.findLast` function.
+ *
+ * @private
+ * @param {Function} findIndexFunc The function to find the collection index.
+ * @returns {Function} Returns the new find function.
+ */
+ function createFind(findIndexFunc) {
+ return function(collection, predicate, fromIndex) {
+ var iterable = Object(collection);
+ if (!isArrayLike(collection)) {
+ var iteratee = baseIteratee(predicate, 3);
+ collection = keys(collection);
+ predicate = function(key) { return iteratee(iterable[key], key, iterable); };
+ }
+ var index = findIndexFunc(collection, predicate, fromIndex);
+ return index > -1 ? iterable[iteratee ? collection[index] : index] : undefined;
+ };
+ }
+
+ /**
+ * Creates a function that wraps `func` to invoke it with the `this` binding
+ * of `thisArg` and `partials` prepended to the arguments it receives.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {Array} partials The arguments to prepend to those provided to
+ * the new function.
+ * @returns {Function} Returns the new wrapped function.
+ */
+ function createPartial(func, bitmask, thisArg, partials) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ var isBind = bitmask & WRAP_BIND_FLAG,
+ Ctor = createCtor(func);
+
+ function wrapper() {
+ var argsIndex = -1,
+ argsLength = arguments.length,
+ leftIndex = -1,
+ leftLength = partials.length,
+ args = Array(leftLength + argsLength),
+ fn = (this && this !== root && this instanceof wrapper) ? Ctor : func;
+
+ while (++leftIndex < leftLength) {
+ args[leftIndex] = partials[leftIndex];
+ }
+ while (argsLength--) {
+ args[leftIndex++] = arguments[++argsIndex];
+ }
+ return fn.apply(isBind ? thisArg : this, args);
+ }
+ return wrapper;
+ }
+
+ /**
+ * A specialized version of `baseIsEqualDeep` for arrays with support for
+ * partial deep comparisons.
+ *
+ * @private
+ * @param {Array} array The array to compare.
+ * @param {Array} other The other array to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} stack Tracks traversed `array` and `other` objects.
+ * @returns {boolean} Returns `true` if the arrays are equivalent, else `false`.
+ */
+ function equalArrays(array, other, bitmask, customizer, equalFunc, stack) {
+ var isPartial = bitmask & COMPARE_PARTIAL_FLAG,
+ arrLength = array.length,
+ othLength = other.length;
+
+ if (arrLength != othLength && !(isPartial && othLength > arrLength)) {
+ return false;
+ }
+ // Check that cyclic values are equal.
+ var arrStacked = stack.get(array);
+ var othStacked = stack.get(other);
+ if (arrStacked && othStacked) {
+ return arrStacked == other && othStacked == array;
+ }
+ var index = -1,
+ result = true,
+ seen = (bitmask & COMPARE_UNORDERED_FLAG) ? [] : undefined;
+
+ // Ignore non-index properties.
+ while (++index < arrLength) {
+ var arrValue = array[index],
+ othValue = other[index];
+
+ var compared;
+ if (compared !== undefined) {
+ if (compared) {
+ continue;
+ }
+ result = false;
+ break;
+ }
+ // Recursively compare arrays (susceptible to call stack limits).
+ if (seen) {
+ if (!baseSome(other, function(othValue, othIndex) {
+ if (!indexOf(seen, othIndex) &&
+ (arrValue === othValue || equalFunc(arrValue, othValue, bitmask, customizer, stack))) {
+ return seen.push(othIndex);
+ }
+ })) {
+ result = false;
+ break;
+ }
+ } else if (!(
+ arrValue === othValue ||
+ equalFunc(arrValue, othValue, bitmask, customizer, stack)
+ )) {
+ result = false;
+ break;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * A specialized version of `baseIsEqualDeep` for comparing objects of
+ * the same `toStringTag`.
+ *
+ * **Note:** This function only supports comparing values with tags of
+ * `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {string} tag The `toStringTag` of the objects to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} stack Tracks traversed `object` and `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+ function equalByTag(object, other, tag, bitmask, customizer, equalFunc, stack) {
+ switch (tag) {
+
+ case boolTag:
+ case dateTag:
+ case numberTag:
+ // Coerce booleans to `1` or `0` and dates to milliseconds.
+ // Invalid dates are coerced to `NaN`.
+ return eq(+object, +other);
+
+ case errorTag:
+ return object.name == other.name && object.message == other.message;
+
+ case regexpTag:
+ case stringTag:
+ // Coerce regexes to strings and treat strings, primitives and objects,
+ // as equal. See http://www.ecma-international.org/ecma-262/7.0/#sec-regexp.prototype.tostring
+ // for more details.
+ return object == (other + '');
+
+ }
+ return false;
+ }
+
+ /**
+ * A specialized version of `baseIsEqualDeep` for objects with support for
+ * partial deep comparisons.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} stack Tracks traversed `object` and `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+ function equalObjects(object, other, bitmask, customizer, equalFunc, stack) {
+ var isPartial = bitmask & COMPARE_PARTIAL_FLAG,
+ objProps = keys(object),
+ objLength = objProps.length,
+ othProps = keys(other),
+ othLength = othProps.length;
+
+ if (objLength != othLength && !isPartial) {
+ return false;
+ }
+ var index = objLength;
+ while (index--) {
+ var key = objProps[index];
+ if (!(isPartial ? key in other : hasOwnProperty.call(other, key))) {
+ return false;
+ }
+ }
+ // Check that cyclic values are equal.
+ var objStacked = stack.get(object);
+ var othStacked = stack.get(other);
+ if (objStacked && othStacked) {
+ return objStacked == other && othStacked == object;
+ }
+ var result = true;
+
+ var skipCtor = isPartial;
+ while (++index < objLength) {
+ key = objProps[index];
+ var objValue = object[key],
+ othValue = other[key];
+
+ var compared;
+ // Recursively compare objects (susceptible to call stack limits).
+ if (!(compared === undefined
+ ? (objValue === othValue || equalFunc(objValue, othValue, bitmask, customizer, stack))
+ : compared
+ )) {
+ result = false;
+ break;
+ }
+ skipCtor || (skipCtor = key == 'constructor');
+ }
+ if (result && !skipCtor) {
+ var objCtor = object.constructor,
+ othCtor = other.constructor;
+
+ // Non `Object` object instances with different constructors are not equal.
+ if (objCtor != othCtor &&
+ ('constructor' in object && 'constructor' in other) &&
+ !(typeof objCtor == 'function' && objCtor instanceof objCtor &&
+ typeof othCtor == 'function' && othCtor instanceof othCtor)) {
+ result = false;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * A specialized version of `baseRest` which flattens the rest array.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @returns {Function} Returns the new function.
+ */
+ function flatRest(func) {
+ return setToString(overRest(func, undefined, flatten), func + '');
+ }
+
+ /**
+ * Checks if `value` is a flattenable `arguments` object or array.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is flattenable, else `false`.
+ */
+ function isFlattenable(value) {
+ return isArray(value) || isArguments(value);
+ }
+
+ /**
+ * Checks if `value` is a valid array-like index.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
+ * @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
+ */
+ function isIndex(value, length) {
+ var type = typeof value;
+ length = length == null ? MAX_SAFE_INTEGER : length;
+
+ return !!length &&
+ (type == 'number' ||
+ (type != 'symbol' && reIsUint.test(value))) &&
+ (value > -1 && value % 1 == 0 && value < length);
+ }
+
+ /**
+ * Checks if the given arguments are from an iteratee call.
+ *
+ * @private
+ * @param {*} value The potential iteratee value argument.
+ * @param {*} index The potential iteratee index or key argument.
+ * @param {*} object The potential iteratee object argument.
+ * @returns {boolean} Returns `true` if the arguments are from an iteratee call,
+ * else `false`.
+ */
+ function isIterateeCall(value, index, object) {
+ if (!isObject(object)) {
+ return false;
+ }
+ var type = typeof index;
+ if (type == 'number'
+ ? (isArrayLike(object) && isIndex(index, object.length))
+ : (type == 'string' && index in object)
+ ) {
+ return eq(object[index], value);
+ }
+ return false;
+ }
+
+ /**
+ * This function is like
+ * [`Object.keys`](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
+ * except that it includes inherited enumerable properties.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+ function nativeKeysIn(object) {
+ var result = [];
+ if (object != null) {
+ for (var key in Object(object)) {
+ result.push(key);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Converts `value` to a string using `Object.prototype.toString`.
+ *
+ * @private
+ * @param {*} value The value to convert.
+ * @returns {string} Returns the converted string.
+ */
+ function objectToString(value) {
+ return nativeObjectToString.call(value);
+ }
+
+ /**
+ * A specialized version of `baseRest` which transforms the rest array.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @param {Function} transform The rest array transform.
+ * @returns {Function} Returns the new function.
+ */
+ function overRest(func, start, transform) {
+ start = nativeMax(start === undefined ? (func.length - 1) : start, 0);
+ return function() {
+ var args = arguments,
+ index = -1,
+ length = nativeMax(args.length - start, 0),
+ array = Array(length);
+
+ while (++index < length) {
+ array[index] = args[start + index];
+ }
+ index = -1;
+ var otherArgs = Array(start + 1);
+ while (++index < start) {
+ otherArgs[index] = args[index];
+ }
+ otherArgs[start] = transform(array);
+ return func.apply(this, otherArgs);
+ };
+ }
+
+ /**
+ * Sets the `toString` method of `func` to return `string`.
+ *
+ * @private
+ * @param {Function} func The function to modify.
+ * @param {Function} string The `toString` result.
+ * @returns {Function} Returns `func`.
+ */
+ var setToString = identity;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates an array with all falsey values removed. The values `false`, `null`,
+ * `0`, `""`, `undefined`, and `NaN` are falsey.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to compact.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * _.compact([0, 1, false, 2, '', 3]);
+ * // => [1, 2, 3]
+ */
+ function compact(array) {
+ return baseFilter(array, Boolean);
+ }
+
+ /**
+ * Creates a new array concatenating `array` with any additional arrays
+ * and/or values.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to concatenate.
+ * @param {...*} [values] The values to concatenate.
+ * @returns {Array} Returns the new concatenated array.
+ * @example
+ *
+ * var array = [1];
+ * var other = _.concat(array, 2, [3], [[4]]);
+ *
+ * console.log(other);
+ * // => [1, 2, 3, [4]]
+ *
+ * console.log(array);
+ * // => [1]
+ */
+ function concat() {
+ var length = arguments.length;
+ if (!length) {
+ return [];
+ }
+ var args = Array(length - 1),
+ array = arguments[0],
+ index = length;
+
+ while (index--) {
+ args[index - 1] = arguments[index];
+ }
+ return arrayPush(isArray(array) ? copyArray(array) : [array], baseFlatten(args, 1));
+ }
+
+ /**
+ * This method is like `_.find` except that it returns the index of the first
+ * element `predicate` returns truthy for instead of the element itself.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': true }
+ * ];
+ *
+ * _.findIndex(users, function(o) { return o.user == 'barney'; });
+ * // => 0
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.findIndex(users, { 'user': 'fred', 'active': false });
+ * // => 1
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.findIndex(users, ['active', false]);
+ * // => 0
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.findIndex(users, 'active');
+ * // => 2
+ */
+ function findIndex(array, predicate, fromIndex) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return -1;
+ }
+ var index = fromIndex == null ? 0 : toInteger(fromIndex);
+ if (index < 0) {
+ index = nativeMax(length + index, 0);
+ }
+ return baseFindIndex(array, baseIteratee(predicate, 3), index);
+ }
+
+ /**
+ * Flattens `array` a single level deep.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to flatten.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * _.flatten([1, [2, [3, [4]], 5]]);
+ * // => [1, 2, [3, [4]], 5]
+ */
+ function flatten(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? baseFlatten(array, 1) : [];
+ }
+
+ /**
+ * Recursively flattens `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to flatten.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * _.flattenDeep([1, [2, [3, [4]], 5]]);
+ * // => [1, 2, 3, 4, 5]
+ */
+ function flattenDeep(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? baseFlatten(array, INFINITY) : [];
+ }
+
+ /**
+ * Gets the first element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @alias first
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {*} Returns the first element of `array`.
+ * @example
+ *
+ * _.head([1, 2, 3]);
+ * // => 1
+ *
+ * _.head([]);
+ * // => undefined
+ */
+ function head(array) {
+ return (array && array.length) ? array[0] : undefined;
+ }
+
+ /**
+ * Gets the index at which the first occurrence of `value` is found in `array`
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons. If `fromIndex` is negative, it's used as the
+ * offset from the end of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ * @example
+ *
+ * _.indexOf([1, 2, 1, 2], 2);
+ * // => 1
+ *
+ * // Search from the `fromIndex`.
+ * _.indexOf([1, 2, 1, 2], 2, 2);
+ * // => 3
+ */
+ function indexOf(array, value, fromIndex) {
+ var length = array == null ? 0 : array.length;
+ if (typeof fromIndex == 'number') {
+ fromIndex = fromIndex < 0 ? nativeMax(length + fromIndex, 0) : fromIndex;
+ } else {
+ fromIndex = 0;
+ }
+ var index = (fromIndex || 0) - 1,
+ isReflexive = value === value;
+
+ while (++index < length) {
+ var other = array[index];
+ if ((isReflexive ? other === value : other !== other)) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * Gets the last element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {*} Returns the last element of `array`.
+ * @example
+ *
+ * _.last([1, 2, 3]);
+ * // => 3
+ */
+ function last(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? array[length - 1] : undefined;
+ }
+
+ /**
+ * Creates a slice of `array` from `start` up to, but not including, `end`.
+ *
+ * **Note:** This method is used instead of
+ * [`Array#slice`](https://mdn.io/Array/slice) to ensure dense arrays are
+ * returned.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to slice.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the slice of `array`.
+ */
+ function slice(array, start, end) {
+ var length = array == null ? 0 : array.length;
+ start = start == null ? 0 : +start;
+ end = end === undefined ? length : +end;
+ return length ? baseSlice(array, start, end) : [];
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a `lodash` wrapper instance that wraps `value` with explicit method
+ * chain sequences enabled. The result of such sequences must be unwrapped
+ * with `_#value`.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.3.0
+ * @category Seq
+ * @param {*} value The value to wrap.
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 },
+ * { 'user': 'pebbles', 'age': 1 }
+ * ];
+ *
+ * var youngest = _
+ * .chain(users)
+ * .sortBy('age')
+ * .map(function(o) {
+ * return o.user + ' is ' + o.age;
+ * })
+ * .head()
+ * .value();
+ * // => 'pebbles is 1'
+ */
+ function chain(value) {
+ var result = lodash(value);
+ result.__chain__ = true;
+ return result;
+ }
+
+ /**
+ * This method invokes `interceptor` and returns `value`. The interceptor
+ * is invoked with one argument; (value). The purpose of this method is to
+ * "tap into" a method chain sequence in order to modify intermediate results.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Seq
+ * @param {*} value The value to provide to `interceptor`.
+ * @param {Function} interceptor The function to invoke.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * _([1, 2, 3])
+ * .tap(function(array) {
+ * // Mutate input array.
+ * array.pop();
+ * })
+ * .reverse()
+ * .value();
+ * // => [2, 1]
+ */
+ function tap(value, interceptor) {
+ interceptor(value);
+ return value;
+ }
+
+ /**
+ * This method is like `_.tap` except that it returns the result of `interceptor`.
+ * The purpose of this method is to "pass thru" values replacing intermediate
+ * results in a method chain sequence.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Seq
+ * @param {*} value The value to provide to `interceptor`.
+ * @param {Function} interceptor The function to invoke.
+ * @returns {*} Returns the result of `interceptor`.
+ * @example
+ *
+ * _(' abc ')
+ * .chain()
+ * .trim()
+ * .thru(function(value) {
+ * return [value];
+ * })
+ * .value();
+ * // => ['abc']
+ */
+ function thru(value, interceptor) {
+ return interceptor(value);
+ }
+
+ /**
+ * Creates a `lodash` wrapper instance with explicit method chain sequences enabled.
+ *
+ * @name chain
+ * @memberOf _
+ * @since 0.1.0
+ * @category Seq
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 }
+ * ];
+ *
+ * // A sequence without explicit chaining.
+ * _(users).head();
+ * // => { 'user': 'barney', 'age': 36 }
+ *
+ * // A sequence with explicit chaining.
+ * _(users)
+ * .chain()
+ * .head()
+ * .pick('user')
+ * .value();
+ * // => { 'user': 'barney' }
+ */
+ function wrapperChain() {
+ return chain(this);
+ }
+
+ /**
+ * Executes the chain sequence to resolve the unwrapped value.
+ *
+ * @name value
+ * @memberOf _
+ * @since 0.1.0
+ * @alias toJSON, valueOf
+ * @category Seq
+ * @returns {*} Returns the resolved unwrapped value.
+ * @example
+ *
+ * _([1, 2, 3]).value();
+ * // => [1, 2, 3]
+ */
+ function wrapperValue() {
+ return baseWrapperValue(this.__wrapped__, this.__actions__);
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Checks if `predicate` returns truthy for **all** elements of `collection`.
+ * Iteration is stopped once `predicate` returns falsey. The predicate is
+ * invoked with three arguments: (value, index|key, collection).
+ *
+ * **Note:** This method returns `true` for
+ * [empty collections](https://en.wikipedia.org/wiki/Empty_set) because
+ * [everything is true](https://en.wikipedia.org/wiki/Vacuous_truth) of
+ * elements of empty collections.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ * @example
+ *
+ * _.every([true, 1, null, 'yes'], Boolean);
+ * // => false
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': false },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.every(users, { 'user': 'barney', 'active': false });
+ * // => false
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.every(users, ['active', false]);
+ * // => true
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.every(users, 'active');
+ * // => false
+ */
+ function every(collection, predicate, guard) {
+ predicate = guard ? undefined : predicate;
+ return baseEvery(collection, baseIteratee(predicate));
+ }
+
+ /**
+ * Iterates over elements of `collection`, returning an array of all elements
+ * `predicate` returns truthy for. The predicate is invoked with three
+ * arguments: (value, index|key, collection).
+ *
+ * **Note:** Unlike `_.remove`, this method returns a new array.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ * @see _.reject
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * _.filter(users, function(o) { return !o.active; });
+ * // => objects for ['fred']
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.filter(users, { 'age': 36, 'active': true });
+ * // => objects for ['barney']
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.filter(users, ['active', false]);
+ * // => objects for ['fred']
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.filter(users, 'active');
+ * // => objects for ['barney']
+ *
+ * // Combining several predicates using `_.overEvery` or `_.overSome`.
+ * _.filter(users, _.overSome([{ 'age': 36 }, ['age', 40]]));
+ * // => objects for ['fred', 'barney']
+ */
+ function filter(collection, predicate) {
+ return baseFilter(collection, baseIteratee(predicate));
+ }
+
+ /**
+ * Iterates over elements of `collection`, returning the first element
+ * `predicate` returns truthy for. The predicate is invoked with three
+ * arguments: (value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {*} Returns the matched element, else `undefined`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false },
+ * { 'user': 'pebbles', 'age': 1, 'active': true }
+ * ];
+ *
+ * _.find(users, function(o) { return o.age < 40; });
+ * // => object for 'barney'
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.find(users, { 'age': 1, 'active': true });
+ * // => object for 'pebbles'
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.find(users, ['active', false]);
+ * // => object for 'fred'
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.find(users, 'active');
+ * // => object for 'barney'
+ */
+ var find = createFind(findIndex);
+
+ /**
+ * Iterates over elements of `collection` and invokes `iteratee` for each element.
+ * The iteratee is invoked with three arguments: (value, index|key, collection).
+ * Iteratee functions may exit iteration early by explicitly returning `false`.
+ *
+ * **Note:** As with other "Collections" methods, objects with a "length"
+ * property are iterated like arrays. To avoid this behavior use `_.forIn`
+ * or `_.forOwn` for object iteration.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @alias each
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array|Object} Returns `collection`.
+ * @see _.forEachRight
+ * @example
+ *
+ * _.forEach([1, 2], function(value) {
+ * console.log(value);
+ * });
+ * // => Logs `1` then `2`.
+ *
+ * _.forEach({ 'a': 1, 'b': 2 }, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'a' then 'b' (iteration order is not guaranteed).
+ */
+ function forEach(collection, iteratee) {
+ return baseEach(collection, baseIteratee(iteratee));
+ }
+
+ /**
+ * Creates an array of values by running each element in `collection` thru
+ * `iteratee`. The iteratee is invoked with three arguments:
+ * (value, index|key, collection).
+ *
+ * Many lodash methods are guarded to work as iteratees for methods like
+ * `_.every`, `_.filter`, `_.map`, `_.mapValues`, `_.reject`, and `_.some`.
+ *
+ * The guarded methods are:
+ * `ary`, `chunk`, `curry`, `curryRight`, `drop`, `dropRight`, `every`,
+ * `fill`, `invert`, `parseInt`, `random`, `range`, `rangeRight`, `repeat`,
+ * `sampleSize`, `slice`, `some`, `sortBy`, `split`, `take`, `takeRight`,
+ * `template`, `trim`, `trimEnd`, `trimStart`, and `words`
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ * @example
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * _.map([4, 8], square);
+ * // => [16, 64]
+ *
+ * _.map({ 'a': 4, 'b': 8 }, square);
+ * // => [16, 64] (iteration order is not guaranteed)
+ *
+ * var users = [
+ * { 'user': 'barney' },
+ * { 'user': 'fred' }
+ * ];
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.map(users, 'user');
+ * // => ['barney', 'fred']
+ */
+ function map(collection, iteratee) {
+ return baseMap(collection, baseIteratee(iteratee));
+ }
+
+ /**
+ * Reduces `collection` to a value which is the accumulated result of running
+ * each element in `collection` thru `iteratee`, where each successive
+ * invocation is supplied the return value of the previous. If `accumulator`
+ * is not given, the first element of `collection` is used as the initial
+ * value. The iteratee is invoked with four arguments:
+ * (accumulator, value, index|key, collection).
+ *
+ * Many lodash methods are guarded to work as iteratees for methods like
+ * `_.reduce`, `_.reduceRight`, and `_.transform`.
+ *
+ * The guarded methods are:
+ * `assign`, `defaults`, `defaultsDeep`, `includes`, `merge`, `orderBy`,
+ * and `sortBy`
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @returns {*} Returns the accumulated value.
+ * @see _.reduceRight
+ * @example
+ *
+ * _.reduce([1, 2], function(sum, n) {
+ * return sum + n;
+ * }, 0);
+ * // => 3
+ *
+ * _.reduce({ 'a': 1, 'b': 2, 'c': 1 }, function(result, value, key) {
+ * (result[value] || (result[value] = [])).push(key);
+ * return result;
+ * }, {});
+ * // => { '1': ['a', 'c'], '2': ['b'] } (iteration order is not guaranteed)
+ */
+ function reduce(collection, iteratee, accumulator) {
+ return baseReduce(collection, baseIteratee(iteratee), accumulator, arguments.length < 3, baseEach);
+ }
+
+ /**
+ * Gets the size of `collection` by returning its length for array-like
+ * values or the number of own enumerable string keyed properties for objects.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to inspect.
+ * @returns {number} Returns the collection size.
+ * @example
+ *
+ * _.size([1, 2, 3]);
+ * // => 3
+ *
+ * _.size({ 'a': 1, 'b': 2 });
+ * // => 2
+ *
+ * _.size('pebbles');
+ * // => 7
+ */
+ function size(collection) {
+ if (collection == null) {
+ return 0;
+ }
+ collection = isArrayLike(collection) ? collection : nativeKeys(collection);
+ return collection.length;
+ }
+
+ /**
+ * Checks if `predicate` returns truthy for **any** element of `collection`.
+ * Iteration is stopped once `predicate` returns truthy. The predicate is
+ * invoked with three arguments: (value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ * @example
+ *
+ * _.some([null, 0, 'yes', false], Boolean);
+ * // => true
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false }
+ * ];
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.some(users, { 'user': 'barney', 'active': false });
+ * // => false
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.some(users, ['active', false]);
+ * // => true
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.some(users, 'active');
+ * // => true
+ */
+ function some(collection, predicate, guard) {
+ predicate = guard ? undefined : predicate;
+ return baseSome(collection, baseIteratee(predicate));
+ }
+
+ /**
+ * Creates an array of elements, sorted in ascending order by the results of
+ * running each element in a collection thru each iteratee. This method
+ * performs a stable sort, that is, it preserves the original sort order of
+ * equal elements. The iteratees are invoked with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {...(Function|Function[])} [iteratees=[_.identity]]
+ * The iteratees to sort by.
+ * @returns {Array} Returns the new sorted array.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'fred', 'age': 48 },
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 30 },
+ * { 'user': 'barney', 'age': 34 }
+ * ];
+ *
+ * _.sortBy(users, [function(o) { return o.user; }]);
+ * // => objects for [['barney', 36], ['barney', 34], ['fred', 48], ['fred', 30]]
+ *
+ * _.sortBy(users, ['user', 'age']);
+ * // => objects for [['barney', 34], ['barney', 36], ['fred', 30], ['fred', 48]]
+ */
+ function sortBy(collection, iteratee) {
+ var index = 0;
+ iteratee = baseIteratee(iteratee);
+
+ return baseMap(baseMap(collection, function(value, key, collection) {
+ return { 'value': value, 'index': index++, 'criteria': iteratee(value, key, collection) };
+ }).sort(function(object, other) {
+ return compareAscending(object.criteria, other.criteria) || (object.index - other.index);
+ }), baseProperty('value'));
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a function that invokes `func`, with the `this` binding and arguments
+ * of the created function, while it's called less than `n` times. Subsequent
+ * calls to the created function return the result of the last `func` invocation.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Function
+ * @param {number} n The number of calls at which `func` is no longer invoked.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * jQuery(element).on('click', _.before(5, addContactToList));
+ * // => Allows adding up to 4 contacts to the list.
+ */
+ function before(n, func) {
+ var result;
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ n = toInteger(n);
+ return function() {
+ if (--n > 0) {
+ result = func.apply(this, arguments);
+ }
+ if (n <= 1) {
+ func = undefined;
+ }
+ return result;
+ };
+ }
+
+ /**
+ * Creates a function that invokes `func` with the `this` binding of `thisArg`
+ * and `partials` prepended to the arguments it receives.
+ *
+ * The `_.bind.placeholder` value, which defaults to `_` in monolithic builds,
+ * may be used as a placeholder for partially applied arguments.
+ *
+ * **Note:** Unlike native `Function#bind`, this method doesn't set the "length"
+ * property of bound functions.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to bind.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * function greet(greeting, punctuation) {
+ * return greeting + ' ' + this.user + punctuation;
+ * }
+ *
+ * var object = { 'user': 'fred' };
+ *
+ * var bound = _.bind(greet, object, 'hi');
+ * bound('!');
+ * // => 'hi fred!'
+ *
+ * // Bound with placeholders.
+ * var bound = _.bind(greet, object, _, '!');
+ * bound('hi');
+ * // => 'hi fred!'
+ */
+ var bind = baseRest(function(func, thisArg, partials) {
+ return createPartial(func, WRAP_BIND_FLAG | WRAP_PARTIAL_FLAG, thisArg, partials);
+ });
+
+ /**
+ * Defers invoking the `func` until the current call stack has cleared. Any
+ * additional arguments are provided to `func` when it's invoked.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to defer.
+ * @param {...*} [args] The arguments to invoke `func` with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.defer(function(text) {
+ * console.log(text);
+ * }, 'deferred');
+ * // => Logs 'deferred' after one millisecond.
+ */
+ var defer = baseRest(function(func, args) {
+ return baseDelay(func, 1, args);
+ });
+
+ /**
+ * Invokes `func` after `wait` milliseconds. Any additional arguments are
+ * provided to `func` when it's invoked.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay invocation.
+ * @param {...*} [args] The arguments to invoke `func` with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.delay(function(text) {
+ * console.log(text);
+ * }, 1000, 'later');
+ * // => Logs 'later' after one second.
+ */
+ var delay = baseRest(function(func, wait, args) {
+ return baseDelay(func, toNumber(wait) || 0, args);
+ });
+
+ /**
+ * Creates a function that negates the result of the predicate `func`. The
+ * `func` predicate is invoked with the `this` binding and arguments of the
+ * created function.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Function
+ * @param {Function} predicate The predicate to negate.
+ * @returns {Function} Returns the new negated function.
+ * @example
+ *
+ * function isEven(n) {
+ * return n % 2 == 0;
+ * }
+ *
+ * _.filter([1, 2, 3, 4, 5, 6], _.negate(isEven));
+ * // => [1, 3, 5]
+ */
+ function negate(predicate) {
+ if (typeof predicate != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ return function() {
+ var args = arguments;
+ return !predicate.apply(this, args);
+ };
+ }
+
+ /**
+ * Creates a function that is restricted to invoking `func` once. Repeat calls
+ * to the function return the value of the first invocation. The `func` is
+ * invoked with the `this` binding and arguments of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var initialize = _.once(createApplication);
+ * initialize();
+ * initialize();
+ * // => `createApplication` is invoked once
+ */
+ function once(func) {
+ return before(2, func);
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a shallow clone of `value`.
+ *
+ * **Note:** This method is loosely based on the
+ * [structured clone algorithm](https://mdn.io/Structured_clone_algorithm)
+ * and supports cloning arrays, array buffers, booleans, date objects, maps,
+ * numbers, `Object` objects, regexes, sets, strings, symbols, and typed
+ * arrays. The own enumerable properties of `arguments` objects are cloned
+ * as plain objects. An empty object is returned for uncloneable values such
+ * as error objects, functions, DOM nodes, and WeakMaps.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to clone.
+ * @returns {*} Returns the cloned value.
+ * @see _.cloneDeep
+ * @example
+ *
+ * var objects = [{ 'a': 1 }, { 'b': 2 }];
+ *
+ * var shallow = _.clone(objects);
+ * console.log(shallow[0] === objects[0]);
+ * // => true
+ */
+ function clone(value) {
+ if (!isObject(value)) {
+ return value;
+ }
+ return isArray(value) ? copyArray(value) : copyObject(value, nativeKeys(value));
+ }
+
+ /**
+ * Performs a
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * comparison between two values to determine if they are equivalent.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ * var other = { 'a': 1 };
+ *
+ * _.eq(object, object);
+ * // => true
+ *
+ * _.eq(object, other);
+ * // => false
+ *
+ * _.eq('a', 'a');
+ * // => true
+ *
+ * _.eq('a', Object('a'));
+ * // => false
+ *
+ * _.eq(NaN, NaN);
+ * // => true
+ */
+ function eq(value, other) {
+ return value === other || (value !== value && other !== other);
+ }
+
+ /**
+ * Checks if `value` is likely an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ * else `false`.
+ * @example
+ *
+ * _.isArguments(function() { return arguments; }());
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+ var isArguments = baseIsArguments(function() { return arguments; }()) ? baseIsArguments : function(value) {
+ return isObjectLike(value) && hasOwnProperty.call(value, 'callee') &&
+ !propertyIsEnumerable.call(value, 'callee');
+ };
+
+ /**
+ * Checks if `value` is classified as an `Array` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array, else `false`.
+ * @example
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ *
+ * _.isArray(document.body.children);
+ * // => false
+ *
+ * _.isArray('abc');
+ * // => false
+ *
+ * _.isArray(_.noop);
+ * // => false
+ */
+ var isArray = Array.isArray;
+
+ /**
+ * Checks if `value` is array-like. A value is considered array-like if it's
+ * not a function and has a `value.length` that's an integer greater than or
+ * equal to `0` and less than or equal to `Number.MAX_SAFE_INTEGER`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ * @example
+ *
+ * _.isArrayLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLike(document.body.children);
+ * // => true
+ *
+ * _.isArrayLike('abc');
+ * // => true
+ *
+ * _.isArrayLike(_.noop);
+ * // => false
+ */
+ function isArrayLike(value) {
+ return value != null && isLength(value.length) && !isFunction(value);
+ }
+
+ /**
+ * Checks if `value` is classified as a boolean primitive or object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a boolean, else `false`.
+ * @example
+ *
+ * _.isBoolean(false);
+ * // => true
+ *
+ * _.isBoolean(null);
+ * // => false
+ */
+ function isBoolean(value) {
+ return value === true || value === false ||
+ (isObjectLike(value) && baseGetTag(value) == boolTag);
+ }
+
+ /**
+ * Checks if `value` is classified as a `Date` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a date object, else `false`.
+ * @example
+ *
+ * _.isDate(new Date);
+ * // => true
+ *
+ * _.isDate('Mon April 23 2012');
+ * // => false
+ */
+ var isDate = baseIsDate;
+
+ /**
+ * Checks if `value` is an empty object, collection, map, or set.
+ *
+ * Objects are considered empty if they have no own enumerable string keyed
+ * properties.
+ *
+ * Array-like values such as `arguments` objects, arrays, buffers, strings, or
+ * jQuery-like collections are considered empty if they have a `length` of `0`.
+ * Similarly, maps and sets are considered empty if they have a `size` of `0`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is empty, else `false`.
+ * @example
+ *
+ * _.isEmpty(null);
+ * // => true
+ *
+ * _.isEmpty(true);
+ * // => true
+ *
+ * _.isEmpty(1);
+ * // => true
+ *
+ * _.isEmpty([1, 2, 3]);
+ * // => false
+ *
+ * _.isEmpty({ 'a': 1 });
+ * // => false
+ */
+ function isEmpty(value) {
+ if (isArrayLike(value) &&
+ (isArray(value) || isString(value) ||
+ isFunction(value.splice) || isArguments(value))) {
+ return !value.length;
+ }
+ return !nativeKeys(value).length;
+ }
+
+ /**
+ * Performs a deep comparison between two values to determine if they are
+ * equivalent.
+ *
+ * **Note:** This method supports comparing arrays, array buffers, booleans,
+ * date objects, error objects, maps, numbers, `Object` objects, regexes,
+ * sets, strings, symbols, and typed arrays. `Object` objects are compared
+ * by their own, not inherited, enumerable properties. Functions and DOM
+ * nodes are compared by strict equality, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ * var other = { 'a': 1 };
+ *
+ * _.isEqual(object, other);
+ * // => true
+ *
+ * object === other;
+ * // => false
+ */
+ function isEqual(value, other) {
+ return baseIsEqual(value, other);
+ }
+
+ /**
+ * Checks if `value` is a finite primitive number.
+ *
+ * **Note:** This method is based on
+ * [`Number.isFinite`](https://mdn.io/Number/isFinite).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a finite number, else `false`.
+ * @example
+ *
+ * _.isFinite(3);
+ * // => true
+ *
+ * _.isFinite(Number.MIN_VALUE);
+ * // => true
+ *
+ * _.isFinite(Infinity);
+ * // => false
+ *
+ * _.isFinite('3');
+ * // => false
+ */
+ function isFinite(value) {
+ return typeof value == 'number' && nativeIsFinite(value);
+ }
+
+ /**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+ function isFunction(value) {
+ if (!isObject(value)) {
+ return false;
+ }
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in Safari 9 which returns 'object' for typed arrays and other constructors.
+ var tag = baseGetTag(value);
+ return tag == funcTag || tag == genTag || tag == asyncTag || tag == proxyTag;
+ }
+
+ /**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This method is loosely based on
+ * [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ * @example
+ *
+ * _.isLength(3);
+ * // => true
+ *
+ * _.isLength(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isLength(Infinity);
+ * // => false
+ *
+ * _.isLength('3');
+ * // => false
+ */
+ function isLength(value) {
+ return typeof value == 'number' &&
+ value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+ }
+
+ /**
+ * Checks if `value` is the
+ * [language type](http://www.ecma-international.org/ecma-262/7.0/#sec-ecmascript-language-types)
+ * of `Object`. (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(_.noop);
+ * // => true
+ *
+ * _.isObject(null);
+ * // => false
+ */
+ function isObject(value) {
+ var type = typeof value;
+ return value != null && (type == 'object' || type == 'function');
+ }
+
+ /**
+ * Checks if `value` is object-like. A value is object-like if it's not `null`
+ * and has a `typeof` result of "object".
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ * @example
+ *
+ * _.isObjectLike({});
+ * // => true
+ *
+ * _.isObjectLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isObjectLike(_.noop);
+ * // => false
+ *
+ * _.isObjectLike(null);
+ * // => false
+ */
+ function isObjectLike(value) {
+ return value != null && typeof value == 'object';
+ }
+
+ /**
+ * Checks if `value` is `NaN`.
+ *
+ * **Note:** This method is based on
+ * [`Number.isNaN`](https://mdn.io/Number/isNaN) and is not the same as
+ * global [`isNaN`](https://mdn.io/isNaN) which returns `true` for
+ * `undefined` and other non-number values.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `NaN`, else `false`.
+ * @example
+ *
+ * _.isNaN(NaN);
+ * // => true
+ *
+ * _.isNaN(new Number(NaN));
+ * // => true
+ *
+ * isNaN(undefined);
+ * // => true
+ *
+ * _.isNaN(undefined);
+ * // => false
+ */
+ function isNaN(value) {
+ // An `NaN` primitive is the only value that is not equal to itself.
+ // Perform the `toStringTag` check first to avoid errors with some
+ // ActiveX objects in IE.
+ return isNumber(value) && value != +value;
+ }
+
+ /**
+ * Checks if `value` is `null`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `null`, else `false`.
+ * @example
+ *
+ * _.isNull(null);
+ * // => true
+ *
+ * _.isNull(void 0);
+ * // => false
+ */
+ function isNull(value) {
+ return value === null;
+ }
+
+ /**
+ * Checks if `value` is classified as a `Number` primitive or object.
+ *
+ * **Note:** To exclude `Infinity`, `-Infinity`, and `NaN`, which are
+ * classified as numbers, use the `_.isFinite` method.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a number, else `false`.
+ * @example
+ *
+ * _.isNumber(3);
+ * // => true
+ *
+ * _.isNumber(Number.MIN_VALUE);
+ * // => true
+ *
+ * _.isNumber(Infinity);
+ * // => true
+ *
+ * _.isNumber('3');
+ * // => false
+ */
+ function isNumber(value) {
+ return typeof value == 'number' ||
+ (isObjectLike(value) && baseGetTag(value) == numberTag);
+ }
+
+ /**
+ * Checks if `value` is classified as a `RegExp` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a regexp, else `false`.
+ * @example
+ *
+ * _.isRegExp(/abc/);
+ * // => true
+ *
+ * _.isRegExp('/abc/');
+ * // => false
+ */
+ var isRegExp = baseIsRegExp;
+
+ /**
+ * Checks if `value` is classified as a `String` primitive or object.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a string, else `false`.
+ * @example
+ *
+ * _.isString('abc');
+ * // => true
+ *
+ * _.isString(1);
+ * // => false
+ */
+ function isString(value) {
+ return typeof value == 'string' ||
+ (!isArray(value) && isObjectLike(value) && baseGetTag(value) == stringTag);
+ }
+
+ /**
+ * Checks if `value` is `undefined`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `undefined`, else `false`.
+ * @example
+ *
+ * _.isUndefined(void 0);
+ * // => true
+ *
+ * _.isUndefined(null);
+ * // => false
+ */
+ function isUndefined(value) {
+ return value === undefined;
+ }
+
+ /**
+ * Converts `value` to an array.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {Array} Returns the converted array.
+ * @example
+ *
+ * _.toArray({ 'a': 1, 'b': 2 });
+ * // => [1, 2]
+ *
+ * _.toArray('abc');
+ * // => ['a', 'b', 'c']
+ *
+ * _.toArray(1);
+ * // => []
+ *
+ * _.toArray(null);
+ * // => []
+ */
+ function toArray(value) {
+ if (!isArrayLike(value)) {
+ return values(value);
+ }
+ return value.length ? copyArray(value) : [];
+ }
+
+ /**
+ * Converts `value` to an integer.
+ *
+ * **Note:** This method is loosely based on
+ * [`ToInteger`](http://www.ecma-international.org/ecma-262/7.0/#sec-tointeger).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {number} Returns the converted integer.
+ * @example
+ *
+ * _.toInteger(3.2);
+ * // => 3
+ *
+ * _.toInteger(Number.MIN_VALUE);
+ * // => 0
+ *
+ * _.toInteger(Infinity);
+ * // => 1.7976931348623157e+308
+ *
+ * _.toInteger('3.2');
+ * // => 3
+ */
+ var toInteger = Number;
+
+ /**
+ * Converts `value` to a number.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to process.
+ * @returns {number} Returns the number.
+ * @example
+ *
+ * _.toNumber(3.2);
+ * // => 3.2
+ *
+ * _.toNumber(Number.MIN_VALUE);
+ * // => 5e-324
+ *
+ * _.toNumber(Infinity);
+ * // => Infinity
+ *
+ * _.toNumber('3.2');
+ * // => 3.2
+ */
+ var toNumber = Number;
+
+ /**
+ * Converts `value` to a string. An empty string is returned for `null`
+ * and `undefined` values. The sign of `-0` is preserved.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {string} Returns the converted string.
+ * @example
+ *
+ * _.toString(null);
+ * // => ''
+ *
+ * _.toString(-0);
+ * // => '-0'
+ *
+ * _.toString([1, 2, 3]);
+ * // => '1,2,3'
+ */
+ function toString(value) {
+ if (typeof value == 'string') {
+ return value;
+ }
+ return value == null ? '' : (value + '');
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Assigns own enumerable string keyed properties of source objects to the
+ * destination object. Source objects are applied from left to right.
+ * Subsequent sources overwrite property assignments of previous sources.
+ *
+ * **Note:** This method mutates `object` and is loosely based on
+ * [`Object.assign`](https://mdn.io/Object/assign).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.10.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.assignIn
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * function Bar() {
+ * this.c = 3;
+ * }
+ *
+ * Foo.prototype.b = 2;
+ * Bar.prototype.d = 4;
+ *
+ * _.assign({ 'a': 0 }, new Foo, new Bar);
+ * // => { 'a': 1, 'c': 3 }
+ */
+ var assign = createAssigner(function(object, source) {
+ copyObject(source, nativeKeys(source), object);
+ });
+
+ /**
+ * This method is like `_.assign` except that it iterates over own and
+ * inherited source properties.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @alias extend
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.assign
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * function Bar() {
+ * this.c = 3;
+ * }
+ *
+ * Foo.prototype.b = 2;
+ * Bar.prototype.d = 4;
+ *
+ * _.assignIn({ 'a': 0 }, new Foo, new Bar);
+ * // => { 'a': 1, 'b': 2, 'c': 3, 'd': 4 }
+ */
+ var assignIn = createAssigner(function(object, source) {
+ copyObject(source, nativeKeysIn(source), object);
+ });
+
+ /**
+ * Creates an object that inherits from the `prototype` object. If a
+ * `properties` object is given, its own enumerable string keyed properties
+ * are assigned to the created object.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.3.0
+ * @category Object
+ * @param {Object} prototype The object to inherit from.
+ * @param {Object} [properties] The properties to assign to the object.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * function Circle() {
+ * Shape.call(this);
+ * }
+ *
+ * Circle.prototype = _.create(Shape.prototype, {
+ * 'constructor': Circle
+ * });
+ *
+ * var circle = new Circle;
+ * circle instanceof Circle;
+ * // => true
+ *
+ * circle instanceof Shape;
+ * // => true
+ */
+ function create(prototype, properties) {
+ var result = baseCreate(prototype);
+ return properties == null ? result : assign(result, properties);
+ }
+
+ /**
+ * Assigns own and inherited enumerable string keyed properties of source
+ * objects to the destination object for all destination properties that
+ * resolve to `undefined`. Source objects are applied from left to right.
+ * Once a property is set, additional values of the same property are ignored.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.defaultsDeep
+ * @example
+ *
+ * _.defaults({ 'a': 1 }, { 'b': 2 }, { 'a': 3 });
+ * // => { 'a': 1, 'b': 2 }
+ */
+ var defaults = baseRest(function(object, sources) {
+ object = Object(object);
+
+ var index = -1;
+ var length = sources.length;
+ var guard = length > 2 ? sources[2] : undefined;
+
+ if (guard && isIterateeCall(sources[0], sources[1], guard)) {
+ length = 1;
+ }
+
+ while (++index < length) {
+ var source = sources[index];
+ var props = keysIn(source);
+ var propsIndex = -1;
+ var propsLength = props.length;
+
+ while (++propsIndex < propsLength) {
+ var key = props[propsIndex];
+ var value = object[key];
+
+ if (value === undefined ||
+ (eq(value, objectProto[key]) && !hasOwnProperty.call(object, key))) {
+ object[key] = source[key];
+ }
+ }
+ }
+
+ return object;
+ });
+
+ /**
+ * Checks if `path` is a direct property of `object`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path to check.
+ * @returns {boolean} Returns `true` if `path` exists, else `false`.
+ * @example
+ *
+ * var object = { 'a': { 'b': 2 } };
+ * var other = _.create({ 'a': _.create({ 'b': 2 }) });
+ *
+ * _.has(object, 'a');
+ * // => true
+ *
+ * _.has(object, 'a.b');
+ * // => true
+ *
+ * _.has(object, ['a', 'b']);
+ * // => true
+ *
+ * _.has(other, 'a');
+ * // => false
+ */
+ function has(object, path) {
+ return object != null && hasOwnProperty.call(object, path);
+ }
+
+ /**
+ * Creates an array of the own enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects. See the
+ * [ES spec](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
+ * for more details.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keys(new Foo);
+ * // => ['a', 'b'] (iteration order is not guaranteed)
+ *
+ * _.keys('hi');
+ * // => ['0', '1']
+ */
+ var keys = nativeKeys;
+
+ /**
+ * Creates an array of the own and inherited enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keysIn(new Foo);
+ * // => ['a', 'b', 'c'] (iteration order is not guaranteed)
+ */
+ var keysIn = nativeKeysIn;
+
+ /**
+ * Creates an object composed of the picked `object` properties.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The source object.
+ * @param {...(string|string[])} [paths] The property paths to pick.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': '2', 'c': 3 };
+ *
+ * _.pick(object, ['a', 'c']);
+ * // => { 'a': 1, 'c': 3 }
+ */
+ var pick = flatRest(function(object, paths) {
+ return object == null ? {} : basePick(object, paths);
+ });
+
+ /**
+ * This method is like `_.get` except that if the resolved value is a
+ * function it's invoked with the `this` binding of its parent object and
+ * its result is returned.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the property to resolve.
+ * @param {*} [defaultValue] The value returned for `undefined` resolved values.
+ * @returns {*} Returns the resolved value.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c1': 3, 'c2': _.constant(4) } }] };
+ *
+ * _.result(object, 'a[0].b.c1');
+ * // => 3
+ *
+ * _.result(object, 'a[0].b.c2');
+ * // => 4
+ *
+ * _.result(object, 'a[0].b.c3', 'default');
+ * // => 'default'
+ *
+ * _.result(object, 'a[0].b.c3', _.constant('default'));
+ * // => 'default'
+ */
+ function result(object, path, defaultValue) {
+ var value = object == null ? undefined : object[path];
+ if (value === undefined) {
+ value = defaultValue;
+ }
+ return isFunction(value) ? value.call(object) : value;
+ }
+
+ /**
+ * Creates an array of the own enumerable string keyed property values of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property values.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.values(new Foo);
+ * // => [1, 2] (iteration order is not guaranteed)
+ *
+ * _.values('hi');
+ * // => ['h', 'i']
+ */
+ function values(object) {
+ return object == null ? [] : baseValues(object, keys(object));
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Converts the characters "&", "<", ">", '"', and "'" in `string` to their
+ * corresponding HTML entities.
+ *
+ * **Note:** No other characters are escaped. To escape additional
+ * characters use a third-party library like [_he_](https://mths.be/he).
+ *
+ * Though the ">" character is escaped for symmetry, characters like
+ * ">" and "/" don't need escaping in HTML and have no special meaning
+ * unless they're part of a tag or unquoted attribute value. See
+ * [Mathias Bynens's article](https://mathiasbynens.be/notes/ambiguous-ampersands)
+ * (under "semi-related fun fact") for more details.
+ *
+ * When working with HTML you should always
+ * [quote attribute values](http://wonko.com/post/html-escaping) to reduce
+ * XSS vectors.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escape('fred, barney, & pebbles');
+ * // => 'fred, barney, & pebbles'
+ */
+ function escape(string) {
+ string = toString(string);
+ return (string && reHasUnescapedHtml.test(string))
+ ? string.replace(reUnescapedHtml, escapeHtmlChar)
+ : string;
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * This method returns the first argument it receives.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Util
+ * @param {*} value Any value.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ *
+ * console.log(_.identity(object) === object);
+ * // => true
+ */
+ function identity(value) {
+ return value;
+ }
+
+ /**
+ * Creates a function that invokes `func` with the arguments of the created
+ * function. If `func` is a property name, the created function returns the
+ * property value for a given element. If `func` is an array or object, the
+ * created function returns `true` for elements that contain the equivalent
+ * source properties, otherwise it returns `false`.
+ *
+ * @static
+ * @since 4.0.0
+ * @memberOf _
+ * @category Util
+ * @param {*} [func=_.identity] The value to convert to a callback.
+ * @returns {Function} Returns the callback.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.filter(users, _.iteratee({ 'user': 'barney', 'active': true }));
+ * // => [{ 'user': 'barney', 'age': 36, 'active': true }]
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.filter(users, _.iteratee(['user', 'fred']));
+ * // => [{ 'user': 'fred', 'age': 40 }]
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.map(users, _.iteratee('user'));
+ * // => ['barney', 'fred']
+ *
+ * // Create custom iteratee shorthands.
+ * _.iteratee = _.wrap(_.iteratee, function(iteratee, func) {
+ * return !_.isRegExp(func) ? iteratee(func) : function(string) {
+ * return func.test(string);
+ * };
+ * });
+ *
+ * _.filter(['abc', 'def'], /ef/);
+ * // => ['def']
+ */
+ var iteratee = baseIteratee;
+
+ /**
+ * Creates a function that performs a partial deep comparison between a given
+ * object and `source`, returning `true` if the given object has equivalent
+ * property values, else `false`.
+ *
+ * **Note:** The created function is equivalent to `_.isMatch` with `source`
+ * partially applied.
+ *
+ * Partial comparisons will match empty array and empty object `source`
+ * values against any array or object value, respectively. See `_.isEqual`
+ * for a list of supported value comparisons.
+ *
+ * **Note:** Multiple values can be checked by combining several matchers
+ * using `_.overSome`
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Util
+ * @param {Object} source The object of property values to match.
+ * @returns {Function} Returns the new spec function.
+ * @example
+ *
+ * var objects = [
+ * { 'a': 1, 'b': 2, 'c': 3 },
+ * { 'a': 4, 'b': 5, 'c': 6 }
+ * ];
+ *
+ * _.filter(objects, _.matches({ 'a': 4, 'c': 6 }));
+ * // => [{ 'a': 4, 'b': 5, 'c': 6 }]
+ *
+ * // Checking for several possible values
+ * _.filter(objects, _.overSome([_.matches({ 'a': 1 }), _.matches({ 'a': 4 })]));
+ * // => [{ 'a': 1, 'b': 2, 'c': 3 }, { 'a': 4, 'b': 5, 'c': 6 }]
+ */
+ function matches(source) {
+ return baseMatches(assign({}, source));
+ }
+
+ /**
+ * Adds all own enumerable string keyed function properties of a source
+ * object to the destination object. If `object` is a function, then methods
+ * are added to its prototype as well.
+ *
+ * **Note:** Use `_.runInContext` to create a pristine `lodash` function to
+ * avoid conflicts caused by modifying the original.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Util
+ * @param {Function|Object} [object=lodash] The destination object.
+ * @param {Object} source The object of functions to add.
+ * @param {Object} [options={}] The options object.
+ * @param {boolean} [options.chain=true] Specify whether mixins are chainable.
+ * @returns {Function|Object} Returns `object`.
+ * @example
+ *
+ * function vowels(string) {
+ * return _.filter(string, function(v) {
+ * return /[aeiou]/i.test(v);
+ * });
+ * }
+ *
+ * _.mixin({ 'vowels': vowels });
+ * _.vowels('fred');
+ * // => ['e']
+ *
+ * _('fred').vowels().value();
+ * // => ['e']
+ *
+ * _.mixin({ 'vowels': vowels }, { 'chain': false });
+ * _('fred').vowels();
+ * // => ['e']
+ */
+ function mixin(object, source, options) {
+ var props = keys(source),
+ methodNames = baseFunctions(source, props);
+
+ if (options == null &&
+ !(isObject(source) && (methodNames.length || !props.length))) {
+ options = source;
+ source = object;
+ object = this;
+ methodNames = baseFunctions(source, keys(source));
+ }
+ var chain = !(isObject(options) && 'chain' in options) || !!options.chain,
+ isFunc = isFunction(object);
+
+ baseEach(methodNames, function(methodName) {
+ var func = source[methodName];
+ object[methodName] = func;
+ if (isFunc) {
+ object.prototype[methodName] = function() {
+ var chainAll = this.__chain__;
+ if (chain || chainAll) {
+ var result = object(this.__wrapped__),
+ actions = result.__actions__ = copyArray(this.__actions__);
+
+ actions.push({ 'func': func, 'args': arguments, 'thisArg': object });
+ result.__chain__ = chainAll;
+ return result;
+ }
+ return func.apply(object, arrayPush([this.value()], arguments));
+ };
+ }
+ });
+
+ return object;
+ }
+
+ /**
+ * Reverts the `_` variable to its previous value and returns a reference to
+ * the `lodash` function.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Util
+ * @returns {Function} Returns the `lodash` function.
+ * @example
+ *
+ * var lodash = _.noConflict();
+ */
+ function noConflict() {
+ if (root._ === this) {
+ root._ = oldDash;
+ }
+ return this;
+ }
+
+ /**
+ * This method returns `undefined`.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.3.0
+ * @category Util
+ * @example
+ *
+ * _.times(2, _.noop);
+ * // => [undefined, undefined]
+ */
+ function noop() {
+ // No operation performed.
+ }
+
+ /**
+ * Generates a unique ID. If `prefix` is given, the ID is appended to it.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Util
+ * @param {string} [prefix=''] The value to prefix the ID with.
+ * @returns {string} Returns the unique ID.
+ * @example
+ *
+ * _.uniqueId('contact_');
+ * // => 'contact_104'
+ *
+ * _.uniqueId();
+ * // => '105'
+ */
+ function uniqueId(prefix) {
+ var id = ++idCounter;
+ return toString(prefix) + id;
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Computes the maximum value of `array`. If `array` is empty or falsey,
+ * `undefined` is returned.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Math
+ * @param {Array} array The array to iterate over.
+ * @returns {*} Returns the maximum value.
+ * @example
+ *
+ * _.max([4, 2, 8, 6]);
+ * // => 8
+ *
+ * _.max([]);
+ * // => undefined
+ */
+ function max(array) {
+ return (array && array.length)
+ ? baseExtremum(array, identity, baseGt)
+ : undefined;
+ }
+
+ /**
+ * Computes the minimum value of `array`. If `array` is empty or falsey,
+ * `undefined` is returned.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Math
+ * @param {Array} array The array to iterate over.
+ * @returns {*} Returns the minimum value.
+ * @example
+ *
+ * _.min([4, 2, 8, 6]);
+ * // => 2
+ *
+ * _.min([]);
+ * // => undefined
+ */
+ function min(array) {
+ return (array && array.length)
+ ? baseExtremum(array, identity, baseLt)
+ : undefined;
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ // Add methods that return wrapped values in chain sequences.
+ lodash.assignIn = assignIn;
+ lodash.before = before;
+ lodash.bind = bind;
+ lodash.chain = chain;
+ lodash.compact = compact;
+ lodash.concat = concat;
+ lodash.create = create;
+ lodash.defaults = defaults;
+ lodash.defer = defer;
+ lodash.delay = delay;
+ lodash.filter = filter;
+ lodash.flatten = flatten;
+ lodash.flattenDeep = flattenDeep;
+ lodash.iteratee = iteratee;
+ lodash.keys = keys;
+ lodash.map = map;
+ lodash.matches = matches;
+ lodash.mixin = mixin;
+ lodash.negate = negate;
+ lodash.once = once;
+ lodash.pick = pick;
+ lodash.slice = slice;
+ lodash.sortBy = sortBy;
+ lodash.tap = tap;
+ lodash.thru = thru;
+ lodash.toArray = toArray;
+ lodash.values = values;
+
+ // Add aliases.
+ lodash.extend = assignIn;
+
+ // Add methods to `lodash.prototype`.
+ mixin(lodash, lodash);
+
+ /*------------------------------------------------------------------------*/
+
+ // Add methods that return unwrapped values in chain sequences.
+ lodash.clone = clone;
+ lodash.escape = escape;
+ lodash.every = every;
+ lodash.find = find;
+ lodash.forEach = forEach;
+ lodash.has = has;
+ lodash.head = head;
+ lodash.identity = identity;
+ lodash.indexOf = indexOf;
+ lodash.isArguments = isArguments;
+ lodash.isArray = isArray;
+ lodash.isBoolean = isBoolean;
+ lodash.isDate = isDate;
+ lodash.isEmpty = isEmpty;
+ lodash.isEqual = isEqual;
+ lodash.isFinite = isFinite;
+ lodash.isFunction = isFunction;
+ lodash.isNaN = isNaN;
+ lodash.isNull = isNull;
+ lodash.isNumber = isNumber;
+ lodash.isObject = isObject;
+ lodash.isRegExp = isRegExp;
+ lodash.isString = isString;
+ lodash.isUndefined = isUndefined;
+ lodash.last = last;
+ lodash.max = max;
+ lodash.min = min;
+ lodash.noConflict = noConflict;
+ lodash.noop = noop;
+ lodash.reduce = reduce;
+ lodash.result = result;
+ lodash.size = size;
+ lodash.some = some;
+ lodash.uniqueId = uniqueId;
+
+ // Add aliases.
+ lodash.each = forEach;
+ lodash.first = head;
+
+ mixin(lodash, (function() {
+ var source = {};
+ baseForOwn(lodash, function(func, methodName) {
+ if (!hasOwnProperty.call(lodash.prototype, methodName)) {
+ source[methodName] = func;
+ }
+ });
+ return source;
+ }()), { 'chain': false });
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * The semantic version number.
+ *
+ * @static
+ * @memberOf _
+ * @type {string}
+ */
+ lodash.VERSION = VERSION;
+
+ // Add `Array` methods to `lodash.prototype`.
+ baseEach(['pop', 'join', 'replace', 'reverse', 'split', 'push', 'shift', 'sort', 'splice', 'unshift'], function(methodName) {
+ var func = (/^(?:replace|split)$/.test(methodName) ? String.prototype : arrayProto)[methodName],
+ chainName = /^(?:push|sort|unshift)$/.test(methodName) ? 'tap' : 'thru',
+ retUnwrapped = /^(?:pop|join|replace|shift)$/.test(methodName);
+
+ lodash.prototype[methodName] = function() {
+ var args = arguments;
+ if (retUnwrapped && !this.__chain__) {
+ var value = this.value();
+ return func.apply(isArray(value) ? value : [], args);
+ }
+ return this[chainName](function(value) {
+ return func.apply(isArray(value) ? value : [], args);
+ });
+ };
+ });
+
+ // Add chain sequence methods to the `lodash` wrapper.
+ lodash.prototype.toJSON = lodash.prototype.valueOf = lodash.prototype.value = wrapperValue;
+
+ /*--------------------------------------------------------------------------*/
+
+ // Some AMD build optimizers, like r.js, check for condition patterns like:
+ if (typeof define == 'function' && typeof define.amd == 'object' && define.amd) {
+ // Expose Lodash on the global object to prevent errors when Lodash is
+ // loaded by a script tag in the presence of an AMD loader.
+ // See http://requirejs.org/docs/errors.html#mismatch for more details.
+ // Use `_.noConflict` to remove Lodash from the global object.
+ root._ = lodash;
+
+ // Define as an anonymous module so, through path mapping, it can be
+ // referenced as the "underscore" module.
+ define(function() {
+ return lodash;
+ });
+ }
+ // Check for `exports` after `define` in case a build optimizer adds it.
+ else if (freeModule) {
+ // Export for Node.js.
+ (freeModule.exports = lodash)._ = lodash;
+ // Export for CommonJS support.
+ freeExports._ = lodash;
+ }
+ else {
+ // Export to the global object.
+ root._ = lodash;
+ }
+}.call(this));
diff --git a/node_modules/lodash/core.min.js b/node_modules/lodash/core.min.js
new file mode 100644
index 0000000..e425e4d
--- /dev/null
+++ b/node_modules/lodash/core.min.js
@@ -0,0 +1,29 @@
+/**
+ * @license
+ * Lodash (Custom Build) lodash.com/license | Underscore.js 1.8.3 underscorejs.org/LICENSE
+ * Build: `lodash core -o ./dist/lodash.core.js`
+ */
+;(function(){function n(n){return H(n)&&pn.call(n,"callee")&&!yn.call(n,"callee")}function t(n,t){return n.push.apply(n,t),n}function r(n){return function(t){return null==t?Z:t[n]}}function e(n,t,r,e,u){return u(n,function(n,u,o){r=e?(e=false,n):t(r,n,u,o)}),r}function u(n,t){return j(t,function(t){return n[t]})}function o(n){return n instanceof i?n:new i(n)}function i(n,t){this.__wrapped__=n,this.__actions__=[],this.__chain__=!!t}function c(n,t,r){if(typeof n!="function")throw new TypeError("Expected a function");
+return setTimeout(function(){n.apply(Z,r)},t)}function f(n,t){var r=true;return mn(n,function(n,e,u){return r=!!t(n,e,u)}),r}function a(n,t,r){for(var e=-1,u=n.length;++et}function b(n,t,r,e,u){return n===t||(null==n||null==t||!H(n)&&!H(t)?n!==n&&t!==t:y(n,t,r,e,b,u))}function y(n,t,r,e,u,o){var i=Nn(n),c=Nn(t),f=i?"[object Array]":hn.call(n),a=c?"[object Array]":hn.call(t),f="[object Arguments]"==f?"[object Object]":f,a="[object Arguments]"==a?"[object Object]":a,l="[object Object]"==f,c="[object Object]"==a,a=f==a;o||(o=[]);var p=An(o,function(t){return t[0]==n}),s=An(o,function(n){
+return n[0]==t});if(p&&s)return p[1]==t;if(o.push([n,t]),o.push([t,n]),a&&!l){if(i)r=T(n,t,r,e,u,o);else n:{switch(f){case"[object Boolean]":case"[object Date]":case"[object Number]":r=J(+n,+t);break n;case"[object Error]":r=n.name==t.name&&n.message==t.message;break n;case"[object RegExp]":case"[object String]":r=n==t+"";break n}r=false}return o.pop(),r}return 1&r||(i=l&&pn.call(n,"__wrapped__"),f=c&&pn.call(t,"__wrapped__"),!i&&!f)?!!a&&(r=B(n,t,r,e,u,o),o.pop(),r):(i=i?n.value():n,f=f?t.value():t,
+r=u(i,f,r,e,o),o.pop(),r)}function g(n){return typeof n=="function"?n:null==n?X:(typeof n=="object"?d:r)(n)}function _(n,t){return nt&&(t=-t>u?0:u+t),r=r>u?u:r,0>r&&(r+=u),u=t>r?0:r-t>>>0,t>>>=0,r=Array(u);++ei))return false;var c=o.get(n),f=o.get(t);if(c&&f)return c==t&&f==n;for(var c=-1,f=true,a=2&r?[]:Z;++cr?jn(e+r,0):r:0,r=(r||0)-1;for(var u=t===t;++rarguments.length,mn);
+}function G(n,t){var r;if(typeof t!="function")throw new TypeError("Expected a function");return n=Fn(n),function(){return 0<--n&&(r=t.apply(this,arguments)),1>=n&&(t=Z),r}}function J(n,t){return n===t||n!==n&&t!==t}function M(n){var t;return(t=null!=n)&&(t=n.length,t=typeof t=="number"&&-1=t),t&&!U(n)}function U(n){return!!V(n)&&(n=hn.call(n),"[object Function]"==n||"[object GeneratorFunction]"==n||"[object AsyncFunction]"==n||"[object Proxy]"==n)}function V(n){var t=typeof n;
+return null!=n&&("object"==t||"function"==t)}function H(n){return null!=n&&typeof n=="object"}function K(n){return typeof n=="number"||H(n)&&"[object Number]"==hn.call(n)}function L(n){return typeof n=="string"||!Nn(n)&&H(n)&&"[object String]"==hn.call(n)}function Q(n){return typeof n=="string"?n:null==n?"":n+""}function W(n){return null==n?[]:u(n,Dn(n))}function X(n){return n}function Y(n,r,e){var u=Dn(r),o=h(r,u);null!=e||V(r)&&(o.length||!u.length)||(e=r,r=n,n=this,o=h(r,Dn(r)));var i=!(V(e)&&"chain"in e&&!e.chain),c=U(n);
+return mn(o,function(e){var u=r[e];n[e]=u,c&&(n.prototype[e]=function(){var r=this.__chain__;if(i||r){var e=n(this.__wrapped__);return(e.__actions__=A(this.__actions__)).push({func:u,args:arguments,thisArg:n}),e.__chain__=r,e}return u.apply(n,t([this.value()],arguments))})}),n}var Z,nn=1/0,tn=/[&<>"']/g,rn=RegExp(tn.source),en=/^(?:0|[1-9]\d*)$/,un=typeof self=="object"&&self&&self.Object===Object&&self,on=typeof global=="object"&&global&&global.Object===Object&&global||un||Function("return this")(),cn=(un=typeof exports=="object"&&exports&&!exports.nodeType&&exports)&&typeof module=="object"&&module&&!module.nodeType&&module,fn=function(n){
+return function(t){return null==n?Z:n[t]}}({"&":"&","<":"<",">":">",'"':""","'":"'"}),an=Array.prototype,ln=Object.prototype,pn=ln.hasOwnProperty,sn=0,hn=ln.toString,vn=on._,bn=Object.create,yn=ln.propertyIsEnumerable,gn=on.isFinite,_n=function(n,t){return function(r){return n(t(r))}}(Object.keys,Object),jn=Math.max,dn=function(){function n(){}return function(t){return V(t)?bn?bn(t):(n.prototype=t,t=new n,n.prototype=Z,t):{}}}();i.prototype=dn(o.prototype),i.prototype.constructor=i;
+var mn=function(n,t){return function(r,e){if(null==r)return r;if(!M(r))return n(r,e);for(var u=r.length,o=t?u:-1,i=Object(r);(t?o--:++or&&(r=jn(e+r,0));n:{for(t=g(t),e=n.length,r+=-1;++re||o&&c&&a||!u&&a||!i){r=1;break n}if(!o&&r { '4': 1, '6': 2 }
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.countBy(['one', 'two', 'three'], 'length');
+ * // => { '3': 2, '5': 1 }
+ */
+var countBy = createAggregator(function(result, value, key) {
+ if (hasOwnProperty.call(result, key)) {
+ ++result[key];
+ } else {
+ baseAssignValue(result, key, 1);
+ }
+});
+
+module.exports = countBy;
diff --git a/node_modules/lodash/create.js b/node_modules/lodash/create.js
new file mode 100644
index 0000000..919edb8
--- /dev/null
+++ b/node_modules/lodash/create.js
@@ -0,0 +1,43 @@
+var baseAssign = require('./_baseAssign'),
+ baseCreate = require('./_baseCreate');
+
+/**
+ * Creates an object that inherits from the `prototype` object. If a
+ * `properties` object is given, its own enumerable string keyed properties
+ * are assigned to the created object.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.3.0
+ * @category Object
+ * @param {Object} prototype The object to inherit from.
+ * @param {Object} [properties] The properties to assign to the object.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * function Circle() {
+ * Shape.call(this);
+ * }
+ *
+ * Circle.prototype = _.create(Shape.prototype, {
+ * 'constructor': Circle
+ * });
+ *
+ * var circle = new Circle;
+ * circle instanceof Circle;
+ * // => true
+ *
+ * circle instanceof Shape;
+ * // => true
+ */
+function create(prototype, properties) {
+ var result = baseCreate(prototype);
+ return properties == null ? result : baseAssign(result, properties);
+}
+
+module.exports = create;
diff --git a/node_modules/lodash/curry.js b/node_modules/lodash/curry.js
new file mode 100644
index 0000000..918db1a
--- /dev/null
+++ b/node_modules/lodash/curry.js
@@ -0,0 +1,57 @@
+var createWrap = require('./_createWrap');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_CURRY_FLAG = 8;
+
+/**
+ * Creates a function that accepts arguments of `func` and either invokes
+ * `func` returning its result, if at least `arity` number of arguments have
+ * been provided, or returns a function that accepts the remaining `func`
+ * arguments, and so on. The arity of `func` may be specified if `func.length`
+ * is not sufficient.
+ *
+ * The `_.curry.placeholder` value, which defaults to `_` in monolithic builds,
+ * may be used as a placeholder for provided arguments.
+ *
+ * **Note:** This method doesn't set the "length" property of curried functions.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Function
+ * @param {Function} func The function to curry.
+ * @param {number} [arity=func.length] The arity of `func`.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Function} Returns the new curried function.
+ * @example
+ *
+ * var abc = function(a, b, c) {
+ * return [a, b, c];
+ * };
+ *
+ * var curried = _.curry(abc);
+ *
+ * curried(1)(2)(3);
+ * // => [1, 2, 3]
+ *
+ * curried(1, 2)(3);
+ * // => [1, 2, 3]
+ *
+ * curried(1, 2, 3);
+ * // => [1, 2, 3]
+ *
+ * // Curried with placeholders.
+ * curried(1)(_, 3)(2);
+ * // => [1, 2, 3]
+ */
+function curry(func, arity, guard) {
+ arity = guard ? undefined : arity;
+ var result = createWrap(func, WRAP_CURRY_FLAG, undefined, undefined, undefined, undefined, undefined, arity);
+ result.placeholder = curry.placeholder;
+ return result;
+}
+
+// Assign default placeholders.
+curry.placeholder = {};
+
+module.exports = curry;
diff --git a/node_modules/lodash/curryRight.js b/node_modules/lodash/curryRight.js
new file mode 100644
index 0000000..c85b6f3
--- /dev/null
+++ b/node_modules/lodash/curryRight.js
@@ -0,0 +1,54 @@
+var createWrap = require('./_createWrap');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_CURRY_RIGHT_FLAG = 16;
+
+/**
+ * This method is like `_.curry` except that arguments are applied to `func`
+ * in the manner of `_.partialRight` instead of `_.partial`.
+ *
+ * The `_.curryRight.placeholder` value, which defaults to `_` in monolithic
+ * builds, may be used as a placeholder for provided arguments.
+ *
+ * **Note:** This method doesn't set the "length" property of curried functions.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Function
+ * @param {Function} func The function to curry.
+ * @param {number} [arity=func.length] The arity of `func`.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Function} Returns the new curried function.
+ * @example
+ *
+ * var abc = function(a, b, c) {
+ * return [a, b, c];
+ * };
+ *
+ * var curried = _.curryRight(abc);
+ *
+ * curried(3)(2)(1);
+ * // => [1, 2, 3]
+ *
+ * curried(2, 3)(1);
+ * // => [1, 2, 3]
+ *
+ * curried(1, 2, 3);
+ * // => [1, 2, 3]
+ *
+ * // Curried with placeholders.
+ * curried(3)(1, _)(2);
+ * // => [1, 2, 3]
+ */
+function curryRight(func, arity, guard) {
+ arity = guard ? undefined : arity;
+ var result = createWrap(func, WRAP_CURRY_RIGHT_FLAG, undefined, undefined, undefined, undefined, undefined, arity);
+ result.placeholder = curryRight.placeholder;
+ return result;
+}
+
+// Assign default placeholders.
+curryRight.placeholder = {};
+
+module.exports = curryRight;
diff --git a/node_modules/lodash/date.js b/node_modules/lodash/date.js
new file mode 100644
index 0000000..cbf5b41
--- /dev/null
+++ b/node_modules/lodash/date.js
@@ -0,0 +1,3 @@
+module.exports = {
+ 'now': require('./now')
+};
diff --git a/node_modules/lodash/debounce.js b/node_modules/lodash/debounce.js
new file mode 100644
index 0000000..8f751d5
--- /dev/null
+++ b/node_modules/lodash/debounce.js
@@ -0,0 +1,191 @@
+var isObject = require('./isObject'),
+ now = require('./now'),
+ toNumber = require('./toNumber');
+
+/** Error message constants. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Creates a debounced function that delays invoking `func` until after `wait`
+ * milliseconds have elapsed since the last time the debounced function was
+ * invoked. The debounced function comes with a `cancel` method to cancel
+ * delayed `func` invocations and a `flush` method to immediately invoke them.
+ * Provide `options` to indicate whether `func` should be invoked on the
+ * leading and/or trailing edge of the `wait` timeout. The `func` is invoked
+ * with the last arguments provided to the debounced function. Subsequent
+ * calls to the debounced function return the result of the last `func`
+ * invocation.
+ *
+ * **Note:** If `leading` and `trailing` options are `true`, `func` is
+ * invoked on the trailing edge of the timeout only if the debounced function
+ * is invoked more than once during the `wait` timeout.
+ *
+ * If `wait` is `0` and `leading` is `false`, `func` invocation is deferred
+ * until to the next tick, similar to `setTimeout` with a timeout of `0`.
+ *
+ * See [David Corbacho's article](https://css-tricks.com/debouncing-throttling-explained-examples/)
+ * for details over the differences between `_.debounce` and `_.throttle`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to debounce.
+ * @param {number} [wait=0] The number of milliseconds to delay.
+ * @param {Object} [options={}] The options object.
+ * @param {boolean} [options.leading=false]
+ * Specify invoking on the leading edge of the timeout.
+ * @param {number} [options.maxWait]
+ * The maximum time `func` is allowed to be delayed before it's invoked.
+ * @param {boolean} [options.trailing=true]
+ * Specify invoking on the trailing edge of the timeout.
+ * @returns {Function} Returns the new debounced function.
+ * @example
+ *
+ * // Avoid costly calculations while the window size is in flux.
+ * jQuery(window).on('resize', _.debounce(calculateLayout, 150));
+ *
+ * // Invoke `sendMail` when clicked, debouncing subsequent calls.
+ * jQuery(element).on('click', _.debounce(sendMail, 300, {
+ * 'leading': true,
+ * 'trailing': false
+ * }));
+ *
+ * // Ensure `batchLog` is invoked once after 1 second of debounced calls.
+ * var debounced = _.debounce(batchLog, 250, { 'maxWait': 1000 });
+ * var source = new EventSource('/stream');
+ * jQuery(source).on('message', debounced);
+ *
+ * // Cancel the trailing debounced invocation.
+ * jQuery(window).on('popstate', debounced.cancel);
+ */
+function debounce(func, wait, options) {
+ var lastArgs,
+ lastThis,
+ maxWait,
+ result,
+ timerId,
+ lastCallTime,
+ lastInvokeTime = 0,
+ leading = false,
+ maxing = false,
+ trailing = true;
+
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ wait = toNumber(wait) || 0;
+ if (isObject(options)) {
+ leading = !!options.leading;
+ maxing = 'maxWait' in options;
+ maxWait = maxing ? nativeMax(toNumber(options.maxWait) || 0, wait) : maxWait;
+ trailing = 'trailing' in options ? !!options.trailing : trailing;
+ }
+
+ function invokeFunc(time) {
+ var args = lastArgs,
+ thisArg = lastThis;
+
+ lastArgs = lastThis = undefined;
+ lastInvokeTime = time;
+ result = func.apply(thisArg, args);
+ return result;
+ }
+
+ function leadingEdge(time) {
+ // Reset any `maxWait` timer.
+ lastInvokeTime = time;
+ // Start the timer for the trailing edge.
+ timerId = setTimeout(timerExpired, wait);
+ // Invoke the leading edge.
+ return leading ? invokeFunc(time) : result;
+ }
+
+ function remainingWait(time) {
+ var timeSinceLastCall = time - lastCallTime,
+ timeSinceLastInvoke = time - lastInvokeTime,
+ timeWaiting = wait - timeSinceLastCall;
+
+ return maxing
+ ? nativeMin(timeWaiting, maxWait - timeSinceLastInvoke)
+ : timeWaiting;
+ }
+
+ function shouldInvoke(time) {
+ var timeSinceLastCall = time - lastCallTime,
+ timeSinceLastInvoke = time - lastInvokeTime;
+
+ // Either this is the first call, activity has stopped and we're at the
+ // trailing edge, the system time has gone backwards and we're treating
+ // it as the trailing edge, or we've hit the `maxWait` limit.
+ return (lastCallTime === undefined || (timeSinceLastCall >= wait) ||
+ (timeSinceLastCall < 0) || (maxing && timeSinceLastInvoke >= maxWait));
+ }
+
+ function timerExpired() {
+ var time = now();
+ if (shouldInvoke(time)) {
+ return trailingEdge(time);
+ }
+ // Restart the timer.
+ timerId = setTimeout(timerExpired, remainingWait(time));
+ }
+
+ function trailingEdge(time) {
+ timerId = undefined;
+
+ // Only invoke if we have `lastArgs` which means `func` has been
+ // debounced at least once.
+ if (trailing && lastArgs) {
+ return invokeFunc(time);
+ }
+ lastArgs = lastThis = undefined;
+ return result;
+ }
+
+ function cancel() {
+ if (timerId !== undefined) {
+ clearTimeout(timerId);
+ }
+ lastInvokeTime = 0;
+ lastArgs = lastCallTime = lastThis = timerId = undefined;
+ }
+
+ function flush() {
+ return timerId === undefined ? result : trailingEdge(now());
+ }
+
+ function debounced() {
+ var time = now(),
+ isInvoking = shouldInvoke(time);
+
+ lastArgs = arguments;
+ lastThis = this;
+ lastCallTime = time;
+
+ if (isInvoking) {
+ if (timerId === undefined) {
+ return leadingEdge(lastCallTime);
+ }
+ if (maxing) {
+ // Handle invocations in a tight loop.
+ clearTimeout(timerId);
+ timerId = setTimeout(timerExpired, wait);
+ return invokeFunc(lastCallTime);
+ }
+ }
+ if (timerId === undefined) {
+ timerId = setTimeout(timerExpired, wait);
+ }
+ return result;
+ }
+ debounced.cancel = cancel;
+ debounced.flush = flush;
+ return debounced;
+}
+
+module.exports = debounce;
diff --git a/node_modules/lodash/deburr.js b/node_modules/lodash/deburr.js
new file mode 100644
index 0000000..f85e314
--- /dev/null
+++ b/node_modules/lodash/deburr.js
@@ -0,0 +1,45 @@
+var deburrLetter = require('./_deburrLetter'),
+ toString = require('./toString');
+
+/** Used to match Latin Unicode letters (excluding mathematical operators). */
+var reLatin = /[\xc0-\xd6\xd8-\xf6\xf8-\xff\u0100-\u017f]/g;
+
+/** Used to compose unicode character classes. */
+var rsComboMarksRange = '\\u0300-\\u036f',
+ reComboHalfMarksRange = '\\ufe20-\\ufe2f',
+ rsComboSymbolsRange = '\\u20d0-\\u20ff',
+ rsComboRange = rsComboMarksRange + reComboHalfMarksRange + rsComboSymbolsRange;
+
+/** Used to compose unicode capture groups. */
+var rsCombo = '[' + rsComboRange + ']';
+
+/**
+ * Used to match [combining diacritical marks](https://en.wikipedia.org/wiki/Combining_Diacritical_Marks) and
+ * [combining diacritical marks for symbols](https://en.wikipedia.org/wiki/Combining_Diacritical_Marks_for_Symbols).
+ */
+var reComboMark = RegExp(rsCombo, 'g');
+
+/**
+ * Deburrs `string` by converting
+ * [Latin-1 Supplement](https://en.wikipedia.org/wiki/Latin-1_Supplement_(Unicode_block)#Character_table)
+ * and [Latin Extended-A](https://en.wikipedia.org/wiki/Latin_Extended-A)
+ * letters to basic Latin letters and removing
+ * [combining diacritical marks](https://en.wikipedia.org/wiki/Combining_Diacritical_Marks).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to deburr.
+ * @returns {string} Returns the deburred string.
+ * @example
+ *
+ * _.deburr('déjà vu');
+ * // => 'deja vu'
+ */
+function deburr(string) {
+ string = toString(string);
+ return string && string.replace(reLatin, deburrLetter).replace(reComboMark, '');
+}
+
+module.exports = deburr;
diff --git a/node_modules/lodash/defaultTo.js b/node_modules/lodash/defaultTo.js
new file mode 100644
index 0000000..5b33359
--- /dev/null
+++ b/node_modules/lodash/defaultTo.js
@@ -0,0 +1,25 @@
+/**
+ * Checks `value` to determine whether a default value should be returned in
+ * its place. The `defaultValue` is returned if `value` is `NaN`, `null`,
+ * or `undefined`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.14.0
+ * @category Util
+ * @param {*} value The value to check.
+ * @param {*} defaultValue The default value.
+ * @returns {*} Returns the resolved value.
+ * @example
+ *
+ * _.defaultTo(1, 10);
+ * // => 1
+ *
+ * _.defaultTo(undefined, 10);
+ * // => 10
+ */
+function defaultTo(value, defaultValue) {
+ return (value == null || value !== value) ? defaultValue : value;
+}
+
+module.exports = defaultTo;
diff --git a/node_modules/lodash/defaults.js b/node_modules/lodash/defaults.js
new file mode 100644
index 0000000..c74df04
--- /dev/null
+++ b/node_modules/lodash/defaults.js
@@ -0,0 +1,64 @@
+var baseRest = require('./_baseRest'),
+ eq = require('./eq'),
+ isIterateeCall = require('./_isIterateeCall'),
+ keysIn = require('./keysIn');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Assigns own and inherited enumerable string keyed properties of source
+ * objects to the destination object for all destination properties that
+ * resolve to `undefined`. Source objects are applied from left to right.
+ * Once a property is set, additional values of the same property are ignored.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.defaultsDeep
+ * @example
+ *
+ * _.defaults({ 'a': 1 }, { 'b': 2 }, { 'a': 3 });
+ * // => { 'a': 1, 'b': 2 }
+ */
+var defaults = baseRest(function(object, sources) {
+ object = Object(object);
+
+ var index = -1;
+ var length = sources.length;
+ var guard = length > 2 ? sources[2] : undefined;
+
+ if (guard && isIterateeCall(sources[0], sources[1], guard)) {
+ length = 1;
+ }
+
+ while (++index < length) {
+ var source = sources[index];
+ var props = keysIn(source);
+ var propsIndex = -1;
+ var propsLength = props.length;
+
+ while (++propsIndex < propsLength) {
+ var key = props[propsIndex];
+ var value = object[key];
+
+ if (value === undefined ||
+ (eq(value, objectProto[key]) && !hasOwnProperty.call(object, key))) {
+ object[key] = source[key];
+ }
+ }
+ }
+
+ return object;
+});
+
+module.exports = defaults;
diff --git a/node_modules/lodash/defaultsDeep.js b/node_modules/lodash/defaultsDeep.js
new file mode 100644
index 0000000..9b5fa3e
--- /dev/null
+++ b/node_modules/lodash/defaultsDeep.js
@@ -0,0 +1,30 @@
+var apply = require('./_apply'),
+ baseRest = require('./_baseRest'),
+ customDefaultsMerge = require('./_customDefaultsMerge'),
+ mergeWith = require('./mergeWith');
+
+/**
+ * This method is like `_.defaults` except that it recursively assigns
+ * default properties.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.10.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.defaults
+ * @example
+ *
+ * _.defaultsDeep({ 'a': { 'b': 2 } }, { 'a': { 'b': 1, 'c': 3 } });
+ * // => { 'a': { 'b': 2, 'c': 3 } }
+ */
+var defaultsDeep = baseRest(function(args) {
+ args.push(undefined, customDefaultsMerge);
+ return apply(mergeWith, undefined, args);
+});
+
+module.exports = defaultsDeep;
diff --git a/node_modules/lodash/defer.js b/node_modules/lodash/defer.js
new file mode 100644
index 0000000..f6d6c6f
--- /dev/null
+++ b/node_modules/lodash/defer.js
@@ -0,0 +1,26 @@
+var baseDelay = require('./_baseDelay'),
+ baseRest = require('./_baseRest');
+
+/**
+ * Defers invoking the `func` until the current call stack has cleared. Any
+ * additional arguments are provided to `func` when it's invoked.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to defer.
+ * @param {...*} [args] The arguments to invoke `func` with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.defer(function(text) {
+ * console.log(text);
+ * }, 'deferred');
+ * // => Logs 'deferred' after one millisecond.
+ */
+var defer = baseRest(function(func, args) {
+ return baseDelay(func, 1, args);
+});
+
+module.exports = defer;
diff --git a/node_modules/lodash/delay.js b/node_modules/lodash/delay.js
new file mode 100644
index 0000000..bd55479
--- /dev/null
+++ b/node_modules/lodash/delay.js
@@ -0,0 +1,28 @@
+var baseDelay = require('./_baseDelay'),
+ baseRest = require('./_baseRest'),
+ toNumber = require('./toNumber');
+
+/**
+ * Invokes `func` after `wait` milliseconds. Any additional arguments are
+ * provided to `func` when it's invoked.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay invocation.
+ * @param {...*} [args] The arguments to invoke `func` with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.delay(function(text) {
+ * console.log(text);
+ * }, 1000, 'later');
+ * // => Logs 'later' after one second.
+ */
+var delay = baseRest(function(func, wait, args) {
+ return baseDelay(func, toNumber(wait) || 0, args);
+});
+
+module.exports = delay;
diff --git a/node_modules/lodash/difference.js b/node_modules/lodash/difference.js
new file mode 100644
index 0000000..fa28bb3
--- /dev/null
+++ b/node_modules/lodash/difference.js
@@ -0,0 +1,33 @@
+var baseDifference = require('./_baseDifference'),
+ baseFlatten = require('./_baseFlatten'),
+ baseRest = require('./_baseRest'),
+ isArrayLikeObject = require('./isArrayLikeObject');
+
+/**
+ * Creates an array of `array` values not included in the other given arrays
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons. The order and references of result values are
+ * determined by the first array.
+ *
+ * **Note:** Unlike `_.pullAll`, this method returns a new array.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {...Array} [values] The values to exclude.
+ * @returns {Array} Returns the new array of filtered values.
+ * @see _.without, _.xor
+ * @example
+ *
+ * _.difference([2, 1], [2, 3]);
+ * // => [1]
+ */
+var difference = baseRest(function(array, values) {
+ return isArrayLikeObject(array)
+ ? baseDifference(array, baseFlatten(values, 1, isArrayLikeObject, true))
+ : [];
+});
+
+module.exports = difference;
diff --git a/node_modules/lodash/differenceBy.js b/node_modules/lodash/differenceBy.js
new file mode 100644
index 0000000..2cd63e7
--- /dev/null
+++ b/node_modules/lodash/differenceBy.js
@@ -0,0 +1,44 @@
+var baseDifference = require('./_baseDifference'),
+ baseFlatten = require('./_baseFlatten'),
+ baseIteratee = require('./_baseIteratee'),
+ baseRest = require('./_baseRest'),
+ isArrayLikeObject = require('./isArrayLikeObject'),
+ last = require('./last');
+
+/**
+ * This method is like `_.difference` except that it accepts `iteratee` which
+ * is invoked for each element of `array` and `values` to generate the criterion
+ * by which they're compared. The order and references of result values are
+ * determined by the first array. The iteratee is invoked with one argument:
+ * (value).
+ *
+ * **Note:** Unlike `_.pullAllBy`, this method returns a new array.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {...Array} [values] The values to exclude.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * _.differenceBy([2.1, 1.2], [2.3, 3.4], Math.floor);
+ * // => [1.2]
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.differenceBy([{ 'x': 2 }, { 'x': 1 }], [{ 'x': 1 }], 'x');
+ * // => [{ 'x': 2 }]
+ */
+var differenceBy = baseRest(function(array, values) {
+ var iteratee = last(values);
+ if (isArrayLikeObject(iteratee)) {
+ iteratee = undefined;
+ }
+ return isArrayLikeObject(array)
+ ? baseDifference(array, baseFlatten(values, 1, isArrayLikeObject, true), baseIteratee(iteratee, 2))
+ : [];
+});
+
+module.exports = differenceBy;
diff --git a/node_modules/lodash/differenceWith.js b/node_modules/lodash/differenceWith.js
new file mode 100644
index 0000000..c0233f4
--- /dev/null
+++ b/node_modules/lodash/differenceWith.js
@@ -0,0 +1,40 @@
+var baseDifference = require('./_baseDifference'),
+ baseFlatten = require('./_baseFlatten'),
+ baseRest = require('./_baseRest'),
+ isArrayLikeObject = require('./isArrayLikeObject'),
+ last = require('./last');
+
+/**
+ * This method is like `_.difference` except that it accepts `comparator`
+ * which is invoked to compare elements of `array` to `values`. The order and
+ * references of result values are determined by the first array. The comparator
+ * is invoked with two arguments: (arrVal, othVal).
+ *
+ * **Note:** Unlike `_.pullAllWith`, this method returns a new array.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {...Array} [values] The values to exclude.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * var objects = [{ 'x': 1, 'y': 2 }, { 'x': 2, 'y': 1 }];
+ *
+ * _.differenceWith(objects, [{ 'x': 1, 'y': 2 }], _.isEqual);
+ * // => [{ 'x': 2, 'y': 1 }]
+ */
+var differenceWith = baseRest(function(array, values) {
+ var comparator = last(values);
+ if (isArrayLikeObject(comparator)) {
+ comparator = undefined;
+ }
+ return isArrayLikeObject(array)
+ ? baseDifference(array, baseFlatten(values, 1, isArrayLikeObject, true), undefined, comparator)
+ : [];
+});
+
+module.exports = differenceWith;
diff --git a/node_modules/lodash/divide.js b/node_modules/lodash/divide.js
new file mode 100644
index 0000000..8cae0cd
--- /dev/null
+++ b/node_modules/lodash/divide.js
@@ -0,0 +1,22 @@
+var createMathOperation = require('./_createMathOperation');
+
+/**
+ * Divide two numbers.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.7.0
+ * @category Math
+ * @param {number} dividend The first number in a division.
+ * @param {number} divisor The second number in a division.
+ * @returns {number} Returns the quotient.
+ * @example
+ *
+ * _.divide(6, 4);
+ * // => 1.5
+ */
+var divide = createMathOperation(function(dividend, divisor) {
+ return dividend / divisor;
+}, 1);
+
+module.exports = divide;
diff --git a/node_modules/lodash/drop.js b/node_modules/lodash/drop.js
new file mode 100644
index 0000000..d5c3cba
--- /dev/null
+++ b/node_modules/lodash/drop.js
@@ -0,0 +1,38 @@
+var baseSlice = require('./_baseSlice'),
+ toInteger = require('./toInteger');
+
+/**
+ * Creates a slice of `array` with `n` elements dropped from the beginning.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.5.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=1] The number of elements to drop.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.drop([1, 2, 3]);
+ * // => [2, 3]
+ *
+ * _.drop([1, 2, 3], 2);
+ * // => [3]
+ *
+ * _.drop([1, 2, 3], 5);
+ * // => []
+ *
+ * _.drop([1, 2, 3], 0);
+ * // => [1, 2, 3]
+ */
+function drop(array, n, guard) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return [];
+ }
+ n = (guard || n === undefined) ? 1 : toInteger(n);
+ return baseSlice(array, n < 0 ? 0 : n, length);
+}
+
+module.exports = drop;
diff --git a/node_modules/lodash/dropRight.js b/node_modules/lodash/dropRight.js
new file mode 100644
index 0000000..441fe99
--- /dev/null
+++ b/node_modules/lodash/dropRight.js
@@ -0,0 +1,39 @@
+var baseSlice = require('./_baseSlice'),
+ toInteger = require('./toInteger');
+
+/**
+ * Creates a slice of `array` with `n` elements dropped from the end.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=1] The number of elements to drop.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.dropRight([1, 2, 3]);
+ * // => [1, 2]
+ *
+ * _.dropRight([1, 2, 3], 2);
+ * // => [1]
+ *
+ * _.dropRight([1, 2, 3], 5);
+ * // => []
+ *
+ * _.dropRight([1, 2, 3], 0);
+ * // => [1, 2, 3]
+ */
+function dropRight(array, n, guard) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return [];
+ }
+ n = (guard || n === undefined) ? 1 : toInteger(n);
+ n = length - n;
+ return baseSlice(array, 0, n < 0 ? 0 : n);
+}
+
+module.exports = dropRight;
diff --git a/node_modules/lodash/dropRightWhile.js b/node_modules/lodash/dropRightWhile.js
new file mode 100644
index 0000000..9ad36a0
--- /dev/null
+++ b/node_modules/lodash/dropRightWhile.js
@@ -0,0 +1,45 @@
+var baseIteratee = require('./_baseIteratee'),
+ baseWhile = require('./_baseWhile');
+
+/**
+ * Creates a slice of `array` excluding elements dropped from the end.
+ * Elements are dropped until `predicate` returns falsey. The predicate is
+ * invoked with three arguments: (value, index, array).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': false }
+ * ];
+ *
+ * _.dropRightWhile(users, function(o) { return !o.active; });
+ * // => objects for ['barney']
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.dropRightWhile(users, { 'user': 'pebbles', 'active': false });
+ * // => objects for ['barney', 'fred']
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.dropRightWhile(users, ['active', false]);
+ * // => objects for ['barney']
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.dropRightWhile(users, 'active');
+ * // => objects for ['barney', 'fred', 'pebbles']
+ */
+function dropRightWhile(array, predicate) {
+ return (array && array.length)
+ ? baseWhile(array, baseIteratee(predicate, 3), true, true)
+ : [];
+}
+
+module.exports = dropRightWhile;
diff --git a/node_modules/lodash/dropWhile.js b/node_modules/lodash/dropWhile.js
new file mode 100644
index 0000000..903ef56
--- /dev/null
+++ b/node_modules/lodash/dropWhile.js
@@ -0,0 +1,45 @@
+var baseIteratee = require('./_baseIteratee'),
+ baseWhile = require('./_baseWhile');
+
+/**
+ * Creates a slice of `array` excluding elements dropped from the beginning.
+ * Elements are dropped until `predicate` returns falsey. The predicate is
+ * invoked with three arguments: (value, index, array).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': true }
+ * ];
+ *
+ * _.dropWhile(users, function(o) { return !o.active; });
+ * // => objects for ['pebbles']
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.dropWhile(users, { 'user': 'barney', 'active': false });
+ * // => objects for ['fred', 'pebbles']
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.dropWhile(users, ['active', false]);
+ * // => objects for ['pebbles']
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.dropWhile(users, 'active');
+ * // => objects for ['barney', 'fred', 'pebbles']
+ */
+function dropWhile(array, predicate) {
+ return (array && array.length)
+ ? baseWhile(array, baseIteratee(predicate, 3), true)
+ : [];
+}
+
+module.exports = dropWhile;
diff --git a/node_modules/lodash/each.js b/node_modules/lodash/each.js
new file mode 100644
index 0000000..8800f42
--- /dev/null
+++ b/node_modules/lodash/each.js
@@ -0,0 +1 @@
+module.exports = require('./forEach');
diff --git a/node_modules/lodash/eachRight.js b/node_modules/lodash/eachRight.js
new file mode 100644
index 0000000..3252b2a
--- /dev/null
+++ b/node_modules/lodash/eachRight.js
@@ -0,0 +1 @@
+module.exports = require('./forEachRight');
diff --git a/node_modules/lodash/endsWith.js b/node_modules/lodash/endsWith.js
new file mode 100644
index 0000000..76fc866
--- /dev/null
+++ b/node_modules/lodash/endsWith.js
@@ -0,0 +1,43 @@
+var baseClamp = require('./_baseClamp'),
+ baseToString = require('./_baseToString'),
+ toInteger = require('./toInteger'),
+ toString = require('./toString');
+
+/**
+ * Checks if `string` ends with the given target string.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to inspect.
+ * @param {string} [target] The string to search for.
+ * @param {number} [position=string.length] The position to search up to.
+ * @returns {boolean} Returns `true` if `string` ends with `target`,
+ * else `false`.
+ * @example
+ *
+ * _.endsWith('abc', 'c');
+ * // => true
+ *
+ * _.endsWith('abc', 'b');
+ * // => false
+ *
+ * _.endsWith('abc', 'b', 2);
+ * // => true
+ */
+function endsWith(string, target, position) {
+ string = toString(string);
+ target = baseToString(target);
+
+ var length = string.length;
+ position = position === undefined
+ ? length
+ : baseClamp(toInteger(position), 0, length);
+
+ var end = position;
+ position -= target.length;
+ return position >= 0 && string.slice(position, end) == target;
+}
+
+module.exports = endsWith;
diff --git a/node_modules/lodash/entries.js b/node_modules/lodash/entries.js
new file mode 100644
index 0000000..7a88df2
--- /dev/null
+++ b/node_modules/lodash/entries.js
@@ -0,0 +1 @@
+module.exports = require('./toPairs');
diff --git a/node_modules/lodash/entriesIn.js b/node_modules/lodash/entriesIn.js
new file mode 100644
index 0000000..f6c6331
--- /dev/null
+++ b/node_modules/lodash/entriesIn.js
@@ -0,0 +1 @@
+module.exports = require('./toPairsIn');
diff --git a/node_modules/lodash/eq.js b/node_modules/lodash/eq.js
new file mode 100644
index 0000000..a940688
--- /dev/null
+++ b/node_modules/lodash/eq.js
@@ -0,0 +1,37 @@
+/**
+ * Performs a
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * comparison between two values to determine if they are equivalent.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ * var other = { 'a': 1 };
+ *
+ * _.eq(object, object);
+ * // => true
+ *
+ * _.eq(object, other);
+ * // => false
+ *
+ * _.eq('a', 'a');
+ * // => true
+ *
+ * _.eq('a', Object('a'));
+ * // => false
+ *
+ * _.eq(NaN, NaN);
+ * // => true
+ */
+function eq(value, other) {
+ return value === other || (value !== value && other !== other);
+}
+
+module.exports = eq;
diff --git a/node_modules/lodash/escape.js b/node_modules/lodash/escape.js
new file mode 100644
index 0000000..9247e00
--- /dev/null
+++ b/node_modules/lodash/escape.js
@@ -0,0 +1,43 @@
+var escapeHtmlChar = require('./_escapeHtmlChar'),
+ toString = require('./toString');
+
+/** Used to match HTML entities and HTML characters. */
+var reUnescapedHtml = /[&<>"']/g,
+ reHasUnescapedHtml = RegExp(reUnescapedHtml.source);
+
+/**
+ * Converts the characters "&", "<", ">", '"', and "'" in `string` to their
+ * corresponding HTML entities.
+ *
+ * **Note:** No other characters are escaped. To escape additional
+ * characters use a third-party library like [_he_](https://mths.be/he).
+ *
+ * Though the ">" character is escaped for symmetry, characters like
+ * ">" and "/" don't need escaping in HTML and have no special meaning
+ * unless they're part of a tag or unquoted attribute value. See
+ * [Mathias Bynens's article](https://mathiasbynens.be/notes/ambiguous-ampersands)
+ * (under "semi-related fun fact") for more details.
+ *
+ * When working with HTML you should always
+ * [quote attribute values](http://wonko.com/post/html-escaping) to reduce
+ * XSS vectors.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escape('fred, barney, & pebbles');
+ * // => 'fred, barney, & pebbles'
+ */
+function escape(string) {
+ string = toString(string);
+ return (string && reHasUnescapedHtml.test(string))
+ ? string.replace(reUnescapedHtml, escapeHtmlChar)
+ : string;
+}
+
+module.exports = escape;
diff --git a/node_modules/lodash/escapeRegExp.js b/node_modules/lodash/escapeRegExp.js
new file mode 100644
index 0000000..0a58c69
--- /dev/null
+++ b/node_modules/lodash/escapeRegExp.js
@@ -0,0 +1,32 @@
+var toString = require('./toString');
+
+/**
+ * Used to match `RegExp`
+ * [syntax characters](http://ecma-international.org/ecma-262/7.0/#sec-patterns).
+ */
+var reRegExpChar = /[\\^$.*+?()[\]{}|]/g,
+ reHasRegExpChar = RegExp(reRegExpChar.source);
+
+/**
+ * Escapes the `RegExp` special characters "^", "$", "\", ".", "*", "+",
+ * "?", "(", ")", "[", "]", "{", "}", and "|" in `string`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escapeRegExp('[lodash](https://lodash.com/)');
+ * // => '\[lodash\]\(https://lodash\.com/\)'
+ */
+function escapeRegExp(string) {
+ string = toString(string);
+ return (string && reHasRegExpChar.test(string))
+ ? string.replace(reRegExpChar, '\\$&')
+ : string;
+}
+
+module.exports = escapeRegExp;
diff --git a/node_modules/lodash/every.js b/node_modules/lodash/every.js
new file mode 100644
index 0000000..25080da
--- /dev/null
+++ b/node_modules/lodash/every.js
@@ -0,0 +1,56 @@
+var arrayEvery = require('./_arrayEvery'),
+ baseEvery = require('./_baseEvery'),
+ baseIteratee = require('./_baseIteratee'),
+ isArray = require('./isArray'),
+ isIterateeCall = require('./_isIterateeCall');
+
+/**
+ * Checks if `predicate` returns truthy for **all** elements of `collection`.
+ * Iteration is stopped once `predicate` returns falsey. The predicate is
+ * invoked with three arguments: (value, index|key, collection).
+ *
+ * **Note:** This method returns `true` for
+ * [empty collections](https://en.wikipedia.org/wiki/Empty_set) because
+ * [everything is true](https://en.wikipedia.org/wiki/Vacuous_truth) of
+ * elements of empty collections.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ * @example
+ *
+ * _.every([true, 1, null, 'yes'], Boolean);
+ * // => false
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': false },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.every(users, { 'user': 'barney', 'active': false });
+ * // => false
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.every(users, ['active', false]);
+ * // => true
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.every(users, 'active');
+ * // => false
+ */
+function every(collection, predicate, guard) {
+ var func = isArray(collection) ? arrayEvery : baseEvery;
+ if (guard && isIterateeCall(collection, predicate, guard)) {
+ predicate = undefined;
+ }
+ return func(collection, baseIteratee(predicate, 3));
+}
+
+module.exports = every;
diff --git a/node_modules/lodash/extend.js b/node_modules/lodash/extend.js
new file mode 100644
index 0000000..e00166c
--- /dev/null
+++ b/node_modules/lodash/extend.js
@@ -0,0 +1 @@
+module.exports = require('./assignIn');
diff --git a/node_modules/lodash/extendWith.js b/node_modules/lodash/extendWith.js
new file mode 100644
index 0000000..dbdcb3b
--- /dev/null
+++ b/node_modules/lodash/extendWith.js
@@ -0,0 +1 @@
+module.exports = require('./assignInWith');
diff --git a/node_modules/lodash/fill.js b/node_modules/lodash/fill.js
new file mode 100644
index 0000000..ae13aa1
--- /dev/null
+++ b/node_modules/lodash/fill.js
@@ -0,0 +1,45 @@
+var baseFill = require('./_baseFill'),
+ isIterateeCall = require('./_isIterateeCall');
+
+/**
+ * Fills elements of `array` with `value` from `start` up to, but not
+ * including, `end`.
+ *
+ * **Note:** This method mutates `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.2.0
+ * @category Array
+ * @param {Array} array The array to fill.
+ * @param {*} value The value to fill `array` with.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = [1, 2, 3];
+ *
+ * _.fill(array, 'a');
+ * console.log(array);
+ * // => ['a', 'a', 'a']
+ *
+ * _.fill(Array(3), 2);
+ * // => [2, 2, 2]
+ *
+ * _.fill([4, 6, 8, 10], '*', 1, 3);
+ * // => [4, '*', '*', 10]
+ */
+function fill(array, value, start, end) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return [];
+ }
+ if (start && typeof start != 'number' && isIterateeCall(array, value, start)) {
+ start = 0;
+ end = length;
+ }
+ return baseFill(array, value, start, end);
+}
+
+module.exports = fill;
diff --git a/node_modules/lodash/filter.js b/node_modules/lodash/filter.js
new file mode 100644
index 0000000..89e0c8c
--- /dev/null
+++ b/node_modules/lodash/filter.js
@@ -0,0 +1,52 @@
+var arrayFilter = require('./_arrayFilter'),
+ baseFilter = require('./_baseFilter'),
+ baseIteratee = require('./_baseIteratee'),
+ isArray = require('./isArray');
+
+/**
+ * Iterates over elements of `collection`, returning an array of all elements
+ * `predicate` returns truthy for. The predicate is invoked with three
+ * arguments: (value, index|key, collection).
+ *
+ * **Note:** Unlike `_.remove`, this method returns a new array.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ * @see _.reject
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * _.filter(users, function(o) { return !o.active; });
+ * // => objects for ['fred']
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.filter(users, { 'age': 36, 'active': true });
+ * // => objects for ['barney']
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.filter(users, ['active', false]);
+ * // => objects for ['fred']
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.filter(users, 'active');
+ * // => objects for ['barney']
+ *
+ * // Combining several predicates using `_.overEvery` or `_.overSome`.
+ * _.filter(users, _.overSome([{ 'age': 36 }, ['age', 40]]));
+ * // => objects for ['fred', 'barney']
+ */
+function filter(collection, predicate) {
+ var func = isArray(collection) ? arrayFilter : baseFilter;
+ return func(collection, baseIteratee(predicate, 3));
+}
+
+module.exports = filter;
diff --git a/node_modules/lodash/find.js b/node_modules/lodash/find.js
new file mode 100644
index 0000000..de732cc
--- /dev/null
+++ b/node_modules/lodash/find.js
@@ -0,0 +1,42 @@
+var createFind = require('./_createFind'),
+ findIndex = require('./findIndex');
+
+/**
+ * Iterates over elements of `collection`, returning the first element
+ * `predicate` returns truthy for. The predicate is invoked with three
+ * arguments: (value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {*} Returns the matched element, else `undefined`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false },
+ * { 'user': 'pebbles', 'age': 1, 'active': true }
+ * ];
+ *
+ * _.find(users, function(o) { return o.age < 40; });
+ * // => object for 'barney'
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.find(users, { 'age': 1, 'active': true });
+ * // => object for 'pebbles'
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.find(users, ['active', false]);
+ * // => object for 'fred'
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.find(users, 'active');
+ * // => object for 'barney'
+ */
+var find = createFind(findIndex);
+
+module.exports = find;
diff --git a/node_modules/lodash/findIndex.js b/node_modules/lodash/findIndex.js
new file mode 100644
index 0000000..4689069
--- /dev/null
+++ b/node_modules/lodash/findIndex.js
@@ -0,0 +1,55 @@
+var baseFindIndex = require('./_baseFindIndex'),
+ baseIteratee = require('./_baseIteratee'),
+ toInteger = require('./toInteger');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * This method is like `_.find` except that it returns the index of the first
+ * element `predicate` returns truthy for instead of the element itself.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': true }
+ * ];
+ *
+ * _.findIndex(users, function(o) { return o.user == 'barney'; });
+ * // => 0
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.findIndex(users, { 'user': 'fred', 'active': false });
+ * // => 1
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.findIndex(users, ['active', false]);
+ * // => 0
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.findIndex(users, 'active');
+ * // => 2
+ */
+function findIndex(array, predicate, fromIndex) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return -1;
+ }
+ var index = fromIndex == null ? 0 : toInteger(fromIndex);
+ if (index < 0) {
+ index = nativeMax(length + index, 0);
+ }
+ return baseFindIndex(array, baseIteratee(predicate, 3), index);
+}
+
+module.exports = findIndex;
diff --git a/node_modules/lodash/findKey.js b/node_modules/lodash/findKey.js
new file mode 100644
index 0000000..cac0248
--- /dev/null
+++ b/node_modules/lodash/findKey.js
@@ -0,0 +1,44 @@
+var baseFindKey = require('./_baseFindKey'),
+ baseForOwn = require('./_baseForOwn'),
+ baseIteratee = require('./_baseIteratee');
+
+/**
+ * This method is like `_.find` except that it returns the key of the first
+ * element `predicate` returns truthy for instead of the element itself.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.1.0
+ * @category Object
+ * @param {Object} object The object to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {string|undefined} Returns the key of the matched element,
+ * else `undefined`.
+ * @example
+ *
+ * var users = {
+ * 'barney': { 'age': 36, 'active': true },
+ * 'fred': { 'age': 40, 'active': false },
+ * 'pebbles': { 'age': 1, 'active': true }
+ * };
+ *
+ * _.findKey(users, function(o) { return o.age < 40; });
+ * // => 'barney' (iteration order is not guaranteed)
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.findKey(users, { 'age': 1, 'active': true });
+ * // => 'pebbles'
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.findKey(users, ['active', false]);
+ * // => 'fred'
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.findKey(users, 'active');
+ * // => 'barney'
+ */
+function findKey(object, predicate) {
+ return baseFindKey(object, baseIteratee(predicate, 3), baseForOwn);
+}
+
+module.exports = findKey;
diff --git a/node_modules/lodash/findLast.js b/node_modules/lodash/findLast.js
new file mode 100644
index 0000000..70b4271
--- /dev/null
+++ b/node_modules/lodash/findLast.js
@@ -0,0 +1,25 @@
+var createFind = require('./_createFind'),
+ findLastIndex = require('./findLastIndex');
+
+/**
+ * This method is like `_.find` except that it iterates over elements of
+ * `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param {number} [fromIndex=collection.length-1] The index to search from.
+ * @returns {*} Returns the matched element, else `undefined`.
+ * @example
+ *
+ * _.findLast([1, 2, 3, 4], function(n) {
+ * return n % 2 == 1;
+ * });
+ * // => 3
+ */
+var findLast = createFind(findLastIndex);
+
+module.exports = findLast;
diff --git a/node_modules/lodash/findLastIndex.js b/node_modules/lodash/findLastIndex.js
new file mode 100644
index 0000000..7da3431
--- /dev/null
+++ b/node_modules/lodash/findLastIndex.js
@@ -0,0 +1,59 @@
+var baseFindIndex = require('./_baseFindIndex'),
+ baseIteratee = require('./_baseIteratee'),
+ toInteger = require('./toInteger');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * This method is like `_.findIndex` except that it iterates over elements
+ * of `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param {number} [fromIndex=array.length-1] The index to search from.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': false }
+ * ];
+ *
+ * _.findLastIndex(users, function(o) { return o.user == 'pebbles'; });
+ * // => 2
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.findLastIndex(users, { 'user': 'barney', 'active': true });
+ * // => 0
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.findLastIndex(users, ['active', false]);
+ * // => 2
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.findLastIndex(users, 'active');
+ * // => 0
+ */
+function findLastIndex(array, predicate, fromIndex) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return -1;
+ }
+ var index = length - 1;
+ if (fromIndex !== undefined) {
+ index = toInteger(fromIndex);
+ index = fromIndex < 0
+ ? nativeMax(length + index, 0)
+ : nativeMin(index, length - 1);
+ }
+ return baseFindIndex(array, baseIteratee(predicate, 3), index, true);
+}
+
+module.exports = findLastIndex;
diff --git a/node_modules/lodash/findLastKey.js b/node_modules/lodash/findLastKey.js
new file mode 100644
index 0000000..66fb9fb
--- /dev/null
+++ b/node_modules/lodash/findLastKey.js
@@ -0,0 +1,44 @@
+var baseFindKey = require('./_baseFindKey'),
+ baseForOwnRight = require('./_baseForOwnRight'),
+ baseIteratee = require('./_baseIteratee');
+
+/**
+ * This method is like `_.findKey` except that it iterates over elements of
+ * a collection in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Object
+ * @param {Object} object The object to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {string|undefined} Returns the key of the matched element,
+ * else `undefined`.
+ * @example
+ *
+ * var users = {
+ * 'barney': { 'age': 36, 'active': true },
+ * 'fred': { 'age': 40, 'active': false },
+ * 'pebbles': { 'age': 1, 'active': true }
+ * };
+ *
+ * _.findLastKey(users, function(o) { return o.age < 40; });
+ * // => returns 'pebbles' assuming `_.findKey` returns 'barney'
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.findLastKey(users, { 'age': 36, 'active': true });
+ * // => 'barney'
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.findLastKey(users, ['active', false]);
+ * // => 'fred'
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.findLastKey(users, 'active');
+ * // => 'pebbles'
+ */
+function findLastKey(object, predicate) {
+ return baseFindKey(object, baseIteratee(predicate, 3), baseForOwnRight);
+}
+
+module.exports = findLastKey;
diff --git a/node_modules/lodash/first.js b/node_modules/lodash/first.js
new file mode 100644
index 0000000..53f4ad1
--- /dev/null
+++ b/node_modules/lodash/first.js
@@ -0,0 +1 @@
+module.exports = require('./head');
diff --git a/node_modules/lodash/flake.lock b/node_modules/lodash/flake.lock
new file mode 100644
index 0000000..dd03252
--- /dev/null
+++ b/node_modules/lodash/flake.lock
@@ -0,0 +1,40 @@
+{
+ "nodes": {
+ "nixpkgs": {
+ "locked": {
+ "lastModified": 1613582597,
+ "narHash": "sha256-6LvipIvFuhyorHpUqK3HjySC5Y6gshXHFBhU9EJ4DoM=",
+ "path": "/nix/store/srvplqq673sqd9vyfhyc5w1p88y1gfm4-source",
+ "rev": "6b1057b452c55bb3b463f0d7055bc4ec3fd1f381",
+ "type": "path"
+ },
+ "original": {
+ "id": "nixpkgs",
+ "type": "indirect"
+ }
+ },
+ "root": {
+ "inputs": {
+ "nixpkgs": "nixpkgs",
+ "utils": "utils"
+ }
+ },
+ "utils": {
+ "locked": {
+ "lastModified": 1610051610,
+ "narHash": "sha256-U9rPz/usA1/Aohhk7Cmc2gBrEEKRzcW4nwPWMPwja4Y=",
+ "owner": "numtide",
+ "repo": "flake-utils",
+ "rev": "3982c9903e93927c2164caa727cd3f6a0e6d14cc",
+ "type": "github"
+ },
+ "original": {
+ "owner": "numtide",
+ "repo": "flake-utils",
+ "type": "github"
+ }
+ }
+ },
+ "root": "root",
+ "version": 7
+}
diff --git a/node_modules/lodash/flake.nix b/node_modules/lodash/flake.nix
new file mode 100644
index 0000000..15a451c
--- /dev/null
+++ b/node_modules/lodash/flake.nix
@@ -0,0 +1,20 @@
+{
+ inputs = {
+ utils.url = "github:numtide/flake-utils";
+ };
+
+ outputs = { self, nixpkgs, utils }:
+ utils.lib.eachDefaultSystem (system:
+ let
+ pkgs = nixpkgs.legacyPackages."${system}";
+ in rec {
+ devShell = pkgs.mkShell {
+ nativeBuildInputs = with pkgs; [
+ yarn
+ nodejs-14_x
+ nodePackages.typescript-language-server
+ nodePackages.eslint
+ ];
+ };
+ });
+}
diff --git a/node_modules/lodash/flatMap.js b/node_modules/lodash/flatMap.js
new file mode 100644
index 0000000..e668506
--- /dev/null
+++ b/node_modules/lodash/flatMap.js
@@ -0,0 +1,29 @@
+var baseFlatten = require('./_baseFlatten'),
+ map = require('./map');
+
+/**
+ * Creates a flattened array of values by running each element in `collection`
+ * thru `iteratee` and flattening the mapped results. The iteratee is invoked
+ * with three arguments: (value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * function duplicate(n) {
+ * return [n, n];
+ * }
+ *
+ * _.flatMap([1, 2], duplicate);
+ * // => [1, 1, 2, 2]
+ */
+function flatMap(collection, iteratee) {
+ return baseFlatten(map(collection, iteratee), 1);
+}
+
+module.exports = flatMap;
diff --git a/node_modules/lodash/flatMapDeep.js b/node_modules/lodash/flatMapDeep.js
new file mode 100644
index 0000000..4653d60
--- /dev/null
+++ b/node_modules/lodash/flatMapDeep.js
@@ -0,0 +1,31 @@
+var baseFlatten = require('./_baseFlatten'),
+ map = require('./map');
+
+/** Used as references for various `Number` constants. */
+var INFINITY = 1 / 0;
+
+/**
+ * This method is like `_.flatMap` except that it recursively flattens the
+ * mapped results.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.7.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * function duplicate(n) {
+ * return [[[n, n]]];
+ * }
+ *
+ * _.flatMapDeep([1, 2], duplicate);
+ * // => [1, 1, 2, 2]
+ */
+function flatMapDeep(collection, iteratee) {
+ return baseFlatten(map(collection, iteratee), INFINITY);
+}
+
+module.exports = flatMapDeep;
diff --git a/node_modules/lodash/flatMapDepth.js b/node_modules/lodash/flatMapDepth.js
new file mode 100644
index 0000000..6d72005
--- /dev/null
+++ b/node_modules/lodash/flatMapDepth.js
@@ -0,0 +1,31 @@
+var baseFlatten = require('./_baseFlatten'),
+ map = require('./map'),
+ toInteger = require('./toInteger');
+
+/**
+ * This method is like `_.flatMap` except that it recursively flattens the
+ * mapped results up to `depth` times.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.7.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {number} [depth=1] The maximum recursion depth.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * function duplicate(n) {
+ * return [[[n, n]]];
+ * }
+ *
+ * _.flatMapDepth([1, 2], duplicate, 2);
+ * // => [[1, 1], [2, 2]]
+ */
+function flatMapDepth(collection, iteratee, depth) {
+ depth = depth === undefined ? 1 : toInteger(depth);
+ return baseFlatten(map(collection, iteratee), depth);
+}
+
+module.exports = flatMapDepth;
diff --git a/node_modules/lodash/flatten.js b/node_modules/lodash/flatten.js
new file mode 100644
index 0000000..3f09f7f
--- /dev/null
+++ b/node_modules/lodash/flatten.js
@@ -0,0 +1,22 @@
+var baseFlatten = require('./_baseFlatten');
+
+/**
+ * Flattens `array` a single level deep.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to flatten.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * _.flatten([1, [2, [3, [4]], 5]]);
+ * // => [1, 2, [3, [4]], 5]
+ */
+function flatten(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? baseFlatten(array, 1) : [];
+}
+
+module.exports = flatten;
diff --git a/node_modules/lodash/flattenDeep.js b/node_modules/lodash/flattenDeep.js
new file mode 100644
index 0000000..8ad585c
--- /dev/null
+++ b/node_modules/lodash/flattenDeep.js
@@ -0,0 +1,25 @@
+var baseFlatten = require('./_baseFlatten');
+
+/** Used as references for various `Number` constants. */
+var INFINITY = 1 / 0;
+
+/**
+ * Recursively flattens `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to flatten.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * _.flattenDeep([1, [2, [3, [4]], 5]]);
+ * // => [1, 2, 3, 4, 5]
+ */
+function flattenDeep(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? baseFlatten(array, INFINITY) : [];
+}
+
+module.exports = flattenDeep;
diff --git a/node_modules/lodash/flattenDepth.js b/node_modules/lodash/flattenDepth.js
new file mode 100644
index 0000000..441fdcc
--- /dev/null
+++ b/node_modules/lodash/flattenDepth.js
@@ -0,0 +1,33 @@
+var baseFlatten = require('./_baseFlatten'),
+ toInteger = require('./toInteger');
+
+/**
+ * Recursively flatten `array` up to `depth` times.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.4.0
+ * @category Array
+ * @param {Array} array The array to flatten.
+ * @param {number} [depth=1] The maximum recursion depth.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * var array = [1, [2, [3, [4]], 5]];
+ *
+ * _.flattenDepth(array, 1);
+ * // => [1, 2, [3, [4]], 5]
+ *
+ * _.flattenDepth(array, 2);
+ * // => [1, 2, 3, [4], 5]
+ */
+function flattenDepth(array, depth) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return [];
+ }
+ depth = depth === undefined ? 1 : toInteger(depth);
+ return baseFlatten(array, depth);
+}
+
+module.exports = flattenDepth;
diff --git a/node_modules/lodash/flip.js b/node_modules/lodash/flip.js
new file mode 100644
index 0000000..c28dd78
--- /dev/null
+++ b/node_modules/lodash/flip.js
@@ -0,0 +1,28 @@
+var createWrap = require('./_createWrap');
+
+/** Used to compose bitmasks for function metadata. */
+var WRAP_FLIP_FLAG = 512;
+
+/**
+ * Creates a function that invokes `func` with arguments reversed.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Function
+ * @param {Function} func The function to flip arguments for.
+ * @returns {Function} Returns the new flipped function.
+ * @example
+ *
+ * var flipped = _.flip(function() {
+ * return _.toArray(arguments);
+ * });
+ *
+ * flipped('a', 'b', 'c', 'd');
+ * // => ['d', 'c', 'b', 'a']
+ */
+function flip(func) {
+ return createWrap(func, WRAP_FLIP_FLAG);
+}
+
+module.exports = flip;
diff --git a/node_modules/lodash/floor.js b/node_modules/lodash/floor.js
new file mode 100644
index 0000000..ab6dfa2
--- /dev/null
+++ b/node_modules/lodash/floor.js
@@ -0,0 +1,26 @@
+var createRound = require('./_createRound');
+
+/**
+ * Computes `number` rounded down to `precision`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.10.0
+ * @category Math
+ * @param {number} number The number to round down.
+ * @param {number} [precision=0] The precision to round down to.
+ * @returns {number} Returns the rounded down number.
+ * @example
+ *
+ * _.floor(4.006);
+ * // => 4
+ *
+ * _.floor(0.046, 2);
+ * // => 0.04
+ *
+ * _.floor(4060, -2);
+ * // => 4000
+ */
+var floor = createRound('floor');
+
+module.exports = floor;
diff --git a/node_modules/lodash/flow.js b/node_modules/lodash/flow.js
new file mode 100644
index 0000000..74b6b62
--- /dev/null
+++ b/node_modules/lodash/flow.js
@@ -0,0 +1,27 @@
+var createFlow = require('./_createFlow');
+
+/**
+ * Creates a function that returns the result of invoking the given functions
+ * with the `this` binding of the created function, where each successive
+ * invocation is supplied the return value of the previous.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Util
+ * @param {...(Function|Function[])} [funcs] The functions to invoke.
+ * @returns {Function} Returns the new composite function.
+ * @see _.flowRight
+ * @example
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * var addSquare = _.flow([_.add, square]);
+ * addSquare(1, 2);
+ * // => 9
+ */
+var flow = createFlow();
+
+module.exports = flow;
diff --git a/node_modules/lodash/flowRight.js b/node_modules/lodash/flowRight.js
new file mode 100644
index 0000000..1146141
--- /dev/null
+++ b/node_modules/lodash/flowRight.js
@@ -0,0 +1,26 @@
+var createFlow = require('./_createFlow');
+
+/**
+ * This method is like `_.flow` except that it creates a function that
+ * invokes the given functions from right to left.
+ *
+ * @static
+ * @since 3.0.0
+ * @memberOf _
+ * @category Util
+ * @param {...(Function|Function[])} [funcs] The functions to invoke.
+ * @returns {Function} Returns the new composite function.
+ * @see _.flow
+ * @example
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * var addSquare = _.flowRight([square, _.add]);
+ * addSquare(1, 2);
+ * // => 9
+ */
+var flowRight = createFlow(true);
+
+module.exports = flowRight;
diff --git a/node_modules/lodash/forEach.js b/node_modules/lodash/forEach.js
new file mode 100644
index 0000000..c64eaa7
--- /dev/null
+++ b/node_modules/lodash/forEach.js
@@ -0,0 +1,41 @@
+var arrayEach = require('./_arrayEach'),
+ baseEach = require('./_baseEach'),
+ castFunction = require('./_castFunction'),
+ isArray = require('./isArray');
+
+/**
+ * Iterates over elements of `collection` and invokes `iteratee` for each element.
+ * The iteratee is invoked with three arguments: (value, index|key, collection).
+ * Iteratee functions may exit iteration early by explicitly returning `false`.
+ *
+ * **Note:** As with other "Collections" methods, objects with a "length"
+ * property are iterated like arrays. To avoid this behavior use `_.forIn`
+ * or `_.forOwn` for object iteration.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @alias each
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array|Object} Returns `collection`.
+ * @see _.forEachRight
+ * @example
+ *
+ * _.forEach([1, 2], function(value) {
+ * console.log(value);
+ * });
+ * // => Logs `1` then `2`.
+ *
+ * _.forEach({ 'a': 1, 'b': 2 }, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'a' then 'b' (iteration order is not guaranteed).
+ */
+function forEach(collection, iteratee) {
+ var func = isArray(collection) ? arrayEach : baseEach;
+ return func(collection, castFunction(iteratee));
+}
+
+module.exports = forEach;
diff --git a/node_modules/lodash/forEachRight.js b/node_modules/lodash/forEachRight.js
new file mode 100644
index 0000000..7390eba
--- /dev/null
+++ b/node_modules/lodash/forEachRight.js
@@ -0,0 +1,31 @@
+var arrayEachRight = require('./_arrayEachRight'),
+ baseEachRight = require('./_baseEachRight'),
+ castFunction = require('./_castFunction'),
+ isArray = require('./isArray');
+
+/**
+ * This method is like `_.forEach` except that it iterates over elements of
+ * `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @alias eachRight
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array|Object} Returns `collection`.
+ * @see _.forEach
+ * @example
+ *
+ * _.forEachRight([1, 2], function(value) {
+ * console.log(value);
+ * });
+ * // => Logs `2` then `1`.
+ */
+function forEachRight(collection, iteratee) {
+ var func = isArray(collection) ? arrayEachRight : baseEachRight;
+ return func(collection, castFunction(iteratee));
+}
+
+module.exports = forEachRight;
diff --git a/node_modules/lodash/forIn.js b/node_modules/lodash/forIn.js
new file mode 100644
index 0000000..583a596
--- /dev/null
+++ b/node_modules/lodash/forIn.js
@@ -0,0 +1,39 @@
+var baseFor = require('./_baseFor'),
+ castFunction = require('./_castFunction'),
+ keysIn = require('./keysIn');
+
+/**
+ * Iterates over own and inherited enumerable string keyed properties of an
+ * object and invokes `iteratee` for each property. The iteratee is invoked
+ * with three arguments: (value, key, object). Iteratee functions may exit
+ * iteration early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.3.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ * @see _.forInRight
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forIn(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'a', 'b', then 'c' (iteration order is not guaranteed).
+ */
+function forIn(object, iteratee) {
+ return object == null
+ ? object
+ : baseFor(object, castFunction(iteratee), keysIn);
+}
+
+module.exports = forIn;
diff --git a/node_modules/lodash/forInRight.js b/node_modules/lodash/forInRight.js
new file mode 100644
index 0000000..4aedf58
--- /dev/null
+++ b/node_modules/lodash/forInRight.js
@@ -0,0 +1,37 @@
+var baseForRight = require('./_baseForRight'),
+ castFunction = require('./_castFunction'),
+ keysIn = require('./keysIn');
+
+/**
+ * This method is like `_.forIn` except that it iterates over properties of
+ * `object` in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ * @see _.forIn
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forInRight(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'c', 'b', then 'a' assuming `_.forIn` logs 'a', 'b', then 'c'.
+ */
+function forInRight(object, iteratee) {
+ return object == null
+ ? object
+ : baseForRight(object, castFunction(iteratee), keysIn);
+}
+
+module.exports = forInRight;
diff --git a/node_modules/lodash/forOwn.js b/node_modules/lodash/forOwn.js
new file mode 100644
index 0000000..94eed84
--- /dev/null
+++ b/node_modules/lodash/forOwn.js
@@ -0,0 +1,36 @@
+var baseForOwn = require('./_baseForOwn'),
+ castFunction = require('./_castFunction');
+
+/**
+ * Iterates over own enumerable string keyed properties of an object and
+ * invokes `iteratee` for each property. The iteratee is invoked with three
+ * arguments: (value, key, object). Iteratee functions may exit iteration
+ * early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.3.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ * @see _.forOwnRight
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forOwn(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'a' then 'b' (iteration order is not guaranteed).
+ */
+function forOwn(object, iteratee) {
+ return object && baseForOwn(object, castFunction(iteratee));
+}
+
+module.exports = forOwn;
diff --git a/node_modules/lodash/forOwnRight.js b/node_modules/lodash/forOwnRight.js
new file mode 100644
index 0000000..86f338f
--- /dev/null
+++ b/node_modules/lodash/forOwnRight.js
@@ -0,0 +1,34 @@
+var baseForOwnRight = require('./_baseForOwnRight'),
+ castFunction = require('./_castFunction');
+
+/**
+ * This method is like `_.forOwn` except that it iterates over properties of
+ * `object` in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ * @see _.forOwn
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forOwnRight(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'b' then 'a' assuming `_.forOwn` logs 'a' then 'b'.
+ */
+function forOwnRight(object, iteratee) {
+ return object && baseForOwnRight(object, castFunction(iteratee));
+}
+
+module.exports = forOwnRight;
diff --git a/node_modules/lodash/fp.js b/node_modules/lodash/fp.js
new file mode 100644
index 0000000..e372dbb
--- /dev/null
+++ b/node_modules/lodash/fp.js
@@ -0,0 +1,2 @@
+var _ = require('./lodash.min').runInContext();
+module.exports = require('./fp/_baseConvert')(_, _);
diff --git a/node_modules/lodash/fp/F.js b/node_modules/lodash/fp/F.js
new file mode 100644
index 0000000..a05a63a
--- /dev/null
+++ b/node_modules/lodash/fp/F.js
@@ -0,0 +1 @@
+module.exports = require('./stubFalse');
diff --git a/node_modules/lodash/fp/T.js b/node_modules/lodash/fp/T.js
new file mode 100644
index 0000000..e2ba8ea
--- /dev/null
+++ b/node_modules/lodash/fp/T.js
@@ -0,0 +1 @@
+module.exports = require('./stubTrue');
diff --git a/node_modules/lodash/fp/__.js b/node_modules/lodash/fp/__.js
new file mode 100644
index 0000000..4af98de
--- /dev/null
+++ b/node_modules/lodash/fp/__.js
@@ -0,0 +1 @@
+module.exports = require('./placeholder');
diff --git a/node_modules/lodash/fp/_baseConvert.js b/node_modules/lodash/fp/_baseConvert.js
new file mode 100644
index 0000000..9baf8e1
--- /dev/null
+++ b/node_modules/lodash/fp/_baseConvert.js
@@ -0,0 +1,569 @@
+var mapping = require('./_mapping'),
+ fallbackHolder = require('./placeholder');
+
+/** Built-in value reference. */
+var push = Array.prototype.push;
+
+/**
+ * Creates a function, with an arity of `n`, that invokes `func` with the
+ * arguments it receives.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {number} n The arity of the new function.
+ * @returns {Function} Returns the new function.
+ */
+function baseArity(func, n) {
+ return n == 2
+ ? function(a, b) { return func.apply(undefined, arguments); }
+ : function(a) { return func.apply(undefined, arguments); };
+}
+
+/**
+ * Creates a function that invokes `func`, with up to `n` arguments, ignoring
+ * any additional arguments.
+ *
+ * @private
+ * @param {Function} func The function to cap arguments for.
+ * @param {number} n The arity cap.
+ * @returns {Function} Returns the new function.
+ */
+function baseAry(func, n) {
+ return n == 2
+ ? function(a, b) { return func(a, b); }
+ : function(a) { return func(a); };
+}
+
+/**
+ * Creates a clone of `array`.
+ *
+ * @private
+ * @param {Array} array The array to clone.
+ * @returns {Array} Returns the cloned array.
+ */
+function cloneArray(array) {
+ var length = array ? array.length : 0,
+ result = Array(length);
+
+ while (length--) {
+ result[length] = array[length];
+ }
+ return result;
+}
+
+/**
+ * Creates a function that clones a given object using the assignment `func`.
+ *
+ * @private
+ * @param {Function} func The assignment function.
+ * @returns {Function} Returns the new cloner function.
+ */
+function createCloner(func) {
+ return function(object) {
+ return func({}, object);
+ };
+}
+
+/**
+ * A specialized version of `_.spread` which flattens the spread array into
+ * the arguments of the invoked `func`.
+ *
+ * @private
+ * @param {Function} func The function to spread arguments over.
+ * @param {number} start The start position of the spread.
+ * @returns {Function} Returns the new function.
+ */
+function flatSpread(func, start) {
+ return function() {
+ var length = arguments.length,
+ lastIndex = length - 1,
+ args = Array(length);
+
+ while (length--) {
+ args[length] = arguments[length];
+ }
+ var array = args[start],
+ otherArgs = args.slice(0, start);
+
+ if (array) {
+ push.apply(otherArgs, array);
+ }
+ if (start != lastIndex) {
+ push.apply(otherArgs, args.slice(start + 1));
+ }
+ return func.apply(this, otherArgs);
+ };
+}
+
+/**
+ * Creates a function that wraps `func` and uses `cloner` to clone the first
+ * argument it receives.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {Function} cloner The function to clone arguments.
+ * @returns {Function} Returns the new immutable function.
+ */
+function wrapImmutable(func, cloner) {
+ return function() {
+ var length = arguments.length;
+ if (!length) {
+ return;
+ }
+ var args = Array(length);
+ while (length--) {
+ args[length] = arguments[length];
+ }
+ var result = args[0] = cloner.apply(undefined, args);
+ func.apply(undefined, args);
+ return result;
+ };
+}
+
+/**
+ * The base implementation of `convert` which accepts a `util` object of methods
+ * required to perform conversions.
+ *
+ * @param {Object} util The util object.
+ * @param {string} name The name of the function to convert.
+ * @param {Function} func The function to convert.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.cap=true] Specify capping iteratee arguments.
+ * @param {boolean} [options.curry=true] Specify currying.
+ * @param {boolean} [options.fixed=true] Specify fixed arity.
+ * @param {boolean} [options.immutable=true] Specify immutable operations.
+ * @param {boolean} [options.rearg=true] Specify rearranging arguments.
+ * @returns {Function|Object} Returns the converted function or object.
+ */
+function baseConvert(util, name, func, options) {
+ var isLib = typeof name == 'function',
+ isObj = name === Object(name);
+
+ if (isObj) {
+ options = func;
+ func = name;
+ name = undefined;
+ }
+ if (func == null) {
+ throw new TypeError;
+ }
+ options || (options = {});
+
+ var config = {
+ 'cap': 'cap' in options ? options.cap : true,
+ 'curry': 'curry' in options ? options.curry : true,
+ 'fixed': 'fixed' in options ? options.fixed : true,
+ 'immutable': 'immutable' in options ? options.immutable : true,
+ 'rearg': 'rearg' in options ? options.rearg : true
+ };
+
+ var defaultHolder = isLib ? func : fallbackHolder,
+ forceCurry = ('curry' in options) && options.curry,
+ forceFixed = ('fixed' in options) && options.fixed,
+ forceRearg = ('rearg' in options) && options.rearg,
+ pristine = isLib ? func.runInContext() : undefined;
+
+ var helpers = isLib ? func : {
+ 'ary': util.ary,
+ 'assign': util.assign,
+ 'clone': util.clone,
+ 'curry': util.curry,
+ 'forEach': util.forEach,
+ 'isArray': util.isArray,
+ 'isError': util.isError,
+ 'isFunction': util.isFunction,
+ 'isWeakMap': util.isWeakMap,
+ 'iteratee': util.iteratee,
+ 'keys': util.keys,
+ 'rearg': util.rearg,
+ 'toInteger': util.toInteger,
+ 'toPath': util.toPath
+ };
+
+ var ary = helpers.ary,
+ assign = helpers.assign,
+ clone = helpers.clone,
+ curry = helpers.curry,
+ each = helpers.forEach,
+ isArray = helpers.isArray,
+ isError = helpers.isError,
+ isFunction = helpers.isFunction,
+ isWeakMap = helpers.isWeakMap,
+ keys = helpers.keys,
+ rearg = helpers.rearg,
+ toInteger = helpers.toInteger,
+ toPath = helpers.toPath;
+
+ var aryMethodKeys = keys(mapping.aryMethod);
+
+ var wrappers = {
+ 'castArray': function(castArray) {
+ return function() {
+ var value = arguments[0];
+ return isArray(value)
+ ? castArray(cloneArray(value))
+ : castArray.apply(undefined, arguments);
+ };
+ },
+ 'iteratee': function(iteratee) {
+ return function() {
+ var func = arguments[0],
+ arity = arguments[1],
+ result = iteratee(func, arity),
+ length = result.length;
+
+ if (config.cap && typeof arity == 'number') {
+ arity = arity > 2 ? (arity - 2) : 1;
+ return (length && length <= arity) ? result : baseAry(result, arity);
+ }
+ return result;
+ };
+ },
+ 'mixin': function(mixin) {
+ return function(source) {
+ var func = this;
+ if (!isFunction(func)) {
+ return mixin(func, Object(source));
+ }
+ var pairs = [];
+ each(keys(source), function(key) {
+ if (isFunction(source[key])) {
+ pairs.push([key, func.prototype[key]]);
+ }
+ });
+
+ mixin(func, Object(source));
+
+ each(pairs, function(pair) {
+ var value = pair[1];
+ if (isFunction(value)) {
+ func.prototype[pair[0]] = value;
+ } else {
+ delete func.prototype[pair[0]];
+ }
+ });
+ return func;
+ };
+ },
+ 'nthArg': function(nthArg) {
+ return function(n) {
+ var arity = n < 0 ? 1 : (toInteger(n) + 1);
+ return curry(nthArg(n), arity);
+ };
+ },
+ 'rearg': function(rearg) {
+ return function(func, indexes) {
+ var arity = indexes ? indexes.length : 0;
+ return curry(rearg(func, indexes), arity);
+ };
+ },
+ 'runInContext': function(runInContext) {
+ return function(context) {
+ return baseConvert(util, runInContext(context), options);
+ };
+ }
+ };
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Casts `func` to a function with an arity capped iteratee if needed.
+ *
+ * @private
+ * @param {string} name The name of the function to inspect.
+ * @param {Function} func The function to inspect.
+ * @returns {Function} Returns the cast function.
+ */
+ function castCap(name, func) {
+ if (config.cap) {
+ var indexes = mapping.iterateeRearg[name];
+ if (indexes) {
+ return iterateeRearg(func, indexes);
+ }
+ var n = !isLib && mapping.iterateeAry[name];
+ if (n) {
+ return iterateeAry(func, n);
+ }
+ }
+ return func;
+ }
+
+ /**
+ * Casts `func` to a curried function if needed.
+ *
+ * @private
+ * @param {string} name The name of the function to inspect.
+ * @param {Function} func The function to inspect.
+ * @param {number} n The arity of `func`.
+ * @returns {Function} Returns the cast function.
+ */
+ function castCurry(name, func, n) {
+ return (forceCurry || (config.curry && n > 1))
+ ? curry(func, n)
+ : func;
+ }
+
+ /**
+ * Casts `func` to a fixed arity function if needed.
+ *
+ * @private
+ * @param {string} name The name of the function to inspect.
+ * @param {Function} func The function to inspect.
+ * @param {number} n The arity cap.
+ * @returns {Function} Returns the cast function.
+ */
+ function castFixed(name, func, n) {
+ if (config.fixed && (forceFixed || !mapping.skipFixed[name])) {
+ var data = mapping.methodSpread[name],
+ start = data && data.start;
+
+ return start === undefined ? ary(func, n) : flatSpread(func, start);
+ }
+ return func;
+ }
+
+ /**
+ * Casts `func` to an rearged function if needed.
+ *
+ * @private
+ * @param {string} name The name of the function to inspect.
+ * @param {Function} func The function to inspect.
+ * @param {number} n The arity of `func`.
+ * @returns {Function} Returns the cast function.
+ */
+ function castRearg(name, func, n) {
+ return (config.rearg && n > 1 && (forceRearg || !mapping.skipRearg[name]))
+ ? rearg(func, mapping.methodRearg[name] || mapping.aryRearg[n])
+ : func;
+ }
+
+ /**
+ * Creates a clone of `object` by `path`.
+ *
+ * @private
+ * @param {Object} object The object to clone.
+ * @param {Array|string} path The path to clone by.
+ * @returns {Object} Returns the cloned object.
+ */
+ function cloneByPath(object, path) {
+ path = toPath(path);
+
+ var index = -1,
+ length = path.length,
+ lastIndex = length - 1,
+ result = clone(Object(object)),
+ nested = result;
+
+ while (nested != null && ++index < length) {
+ var key = path[index],
+ value = nested[key];
+
+ if (value != null &&
+ !(isFunction(value) || isError(value) || isWeakMap(value))) {
+ nested[key] = clone(index == lastIndex ? value : Object(value));
+ }
+ nested = nested[key];
+ }
+ return result;
+ }
+
+ /**
+ * Converts `lodash` to an immutable auto-curried iteratee-first data-last
+ * version with conversion `options` applied.
+ *
+ * @param {Object} [options] The options object. See `baseConvert` for more details.
+ * @returns {Function} Returns the converted `lodash`.
+ */
+ function convertLib(options) {
+ return _.runInContext.convert(options)(undefined);
+ }
+
+ /**
+ * Create a converter function for `func` of `name`.
+ *
+ * @param {string} name The name of the function to convert.
+ * @param {Function} func The function to convert.
+ * @returns {Function} Returns the new converter function.
+ */
+ function createConverter(name, func) {
+ var realName = mapping.aliasToReal[name] || name,
+ methodName = mapping.remap[realName] || realName,
+ oldOptions = options;
+
+ return function(options) {
+ var newUtil = isLib ? pristine : helpers,
+ newFunc = isLib ? pristine[methodName] : func,
+ newOptions = assign(assign({}, oldOptions), options);
+
+ return baseConvert(newUtil, realName, newFunc, newOptions);
+ };
+ }
+
+ /**
+ * Creates a function that wraps `func` to invoke its iteratee, with up to `n`
+ * arguments, ignoring any additional arguments.
+ *
+ * @private
+ * @param {Function} func The function to cap iteratee arguments for.
+ * @param {number} n The arity cap.
+ * @returns {Function} Returns the new function.
+ */
+ function iterateeAry(func, n) {
+ return overArg(func, function(func) {
+ return typeof func == 'function' ? baseAry(func, n) : func;
+ });
+ }
+
+ /**
+ * Creates a function that wraps `func` to invoke its iteratee with arguments
+ * arranged according to the specified `indexes` where the argument value at
+ * the first index is provided as the first argument, the argument value at
+ * the second index is provided as the second argument, and so on.
+ *
+ * @private
+ * @param {Function} func The function to rearrange iteratee arguments for.
+ * @param {number[]} indexes The arranged argument indexes.
+ * @returns {Function} Returns the new function.
+ */
+ function iterateeRearg(func, indexes) {
+ return overArg(func, function(func) {
+ var n = indexes.length;
+ return baseArity(rearg(baseAry(func, n), indexes), n);
+ });
+ }
+
+ /**
+ * Creates a function that invokes `func` with its first argument transformed.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {Function} transform The argument transform.
+ * @returns {Function} Returns the new function.
+ */
+ function overArg(func, transform) {
+ return function() {
+ var length = arguments.length;
+ if (!length) {
+ return func();
+ }
+ var args = Array(length);
+ while (length--) {
+ args[length] = arguments[length];
+ }
+ var index = config.rearg ? 0 : (length - 1);
+ args[index] = transform(args[index]);
+ return func.apply(undefined, args);
+ };
+ }
+
+ /**
+ * Creates a function that wraps `func` and applys the conversions
+ * rules by `name`.
+ *
+ * @private
+ * @param {string} name The name of the function to wrap.
+ * @param {Function} func The function to wrap.
+ * @returns {Function} Returns the converted function.
+ */
+ function wrap(name, func, placeholder) {
+ var result,
+ realName = mapping.aliasToReal[name] || name,
+ wrapped = func,
+ wrapper = wrappers[realName];
+
+ if (wrapper) {
+ wrapped = wrapper(func);
+ }
+ else if (config.immutable) {
+ if (mapping.mutate.array[realName]) {
+ wrapped = wrapImmutable(func, cloneArray);
+ }
+ else if (mapping.mutate.object[realName]) {
+ wrapped = wrapImmutable(func, createCloner(func));
+ }
+ else if (mapping.mutate.set[realName]) {
+ wrapped = wrapImmutable(func, cloneByPath);
+ }
+ }
+ each(aryMethodKeys, function(aryKey) {
+ each(mapping.aryMethod[aryKey], function(otherName) {
+ if (realName == otherName) {
+ var data = mapping.methodSpread[realName],
+ afterRearg = data && data.afterRearg;
+
+ result = afterRearg
+ ? castFixed(realName, castRearg(realName, wrapped, aryKey), aryKey)
+ : castRearg(realName, castFixed(realName, wrapped, aryKey), aryKey);
+
+ result = castCap(realName, result);
+ result = castCurry(realName, result, aryKey);
+ return false;
+ }
+ });
+ return !result;
+ });
+
+ result || (result = wrapped);
+ if (result == func) {
+ result = forceCurry ? curry(result, 1) : function() {
+ return func.apply(this, arguments);
+ };
+ }
+ result.convert = createConverter(realName, func);
+ result.placeholder = func.placeholder = placeholder;
+
+ return result;
+ }
+
+ /*--------------------------------------------------------------------------*/
+
+ if (!isObj) {
+ return wrap(name, func, defaultHolder);
+ }
+ var _ = func;
+
+ // Convert methods by ary cap.
+ var pairs = [];
+ each(aryMethodKeys, function(aryKey) {
+ each(mapping.aryMethod[aryKey], function(key) {
+ var func = _[mapping.remap[key] || key];
+ if (func) {
+ pairs.push([key, wrap(key, func, _)]);
+ }
+ });
+ });
+
+ // Convert remaining methods.
+ each(keys(_), function(key) {
+ var func = _[key];
+ if (typeof func == 'function') {
+ var length = pairs.length;
+ while (length--) {
+ if (pairs[length][0] == key) {
+ return;
+ }
+ }
+ func.convert = createConverter(key, func);
+ pairs.push([key, func]);
+ }
+ });
+
+ // Assign to `_` leaving `_.prototype` unchanged to allow chaining.
+ each(pairs, function(pair) {
+ _[pair[0]] = pair[1];
+ });
+
+ _.convert = convertLib;
+ _.placeholder = _;
+
+ // Assign aliases.
+ each(keys(_), function(key) {
+ each(mapping.realToAlias[key] || [], function(alias) {
+ _[alias] = _[key];
+ });
+ });
+
+ return _;
+}
+
+module.exports = baseConvert;
diff --git a/node_modules/lodash/fp/_convertBrowser.js b/node_modules/lodash/fp/_convertBrowser.js
new file mode 100644
index 0000000..bde030d
--- /dev/null
+++ b/node_modules/lodash/fp/_convertBrowser.js
@@ -0,0 +1,18 @@
+var baseConvert = require('./_baseConvert');
+
+/**
+ * Converts `lodash` to an immutable auto-curried iteratee-first data-last
+ * version with conversion `options` applied.
+ *
+ * @param {Function} lodash The lodash function to convert.
+ * @param {Object} [options] The options object. See `baseConvert` for more details.
+ * @returns {Function} Returns the converted `lodash`.
+ */
+function browserConvert(lodash, options) {
+ return baseConvert(lodash, lodash, options);
+}
+
+if (typeof _ == 'function' && typeof _.runInContext == 'function') {
+ _ = browserConvert(_.runInContext());
+}
+module.exports = browserConvert;
diff --git a/node_modules/lodash/fp/_falseOptions.js b/node_modules/lodash/fp/_falseOptions.js
new file mode 100644
index 0000000..773235e
--- /dev/null
+++ b/node_modules/lodash/fp/_falseOptions.js
@@ -0,0 +1,7 @@
+module.exports = {
+ 'cap': false,
+ 'curry': false,
+ 'fixed': false,
+ 'immutable': false,
+ 'rearg': false
+};
diff --git a/node_modules/lodash/fp/_mapping.js b/node_modules/lodash/fp/_mapping.js
new file mode 100644
index 0000000..a642ec0
--- /dev/null
+++ b/node_modules/lodash/fp/_mapping.js
@@ -0,0 +1,358 @@
+/** Used to map aliases to their real names. */
+exports.aliasToReal = {
+
+ // Lodash aliases.
+ 'each': 'forEach',
+ 'eachRight': 'forEachRight',
+ 'entries': 'toPairs',
+ 'entriesIn': 'toPairsIn',
+ 'extend': 'assignIn',
+ 'extendAll': 'assignInAll',
+ 'extendAllWith': 'assignInAllWith',
+ 'extendWith': 'assignInWith',
+ 'first': 'head',
+
+ // Methods that are curried variants of others.
+ 'conforms': 'conformsTo',
+ 'matches': 'isMatch',
+ 'property': 'get',
+
+ // Ramda aliases.
+ '__': 'placeholder',
+ 'F': 'stubFalse',
+ 'T': 'stubTrue',
+ 'all': 'every',
+ 'allPass': 'overEvery',
+ 'always': 'constant',
+ 'any': 'some',
+ 'anyPass': 'overSome',
+ 'apply': 'spread',
+ 'assoc': 'set',
+ 'assocPath': 'set',
+ 'complement': 'negate',
+ 'compose': 'flowRight',
+ 'contains': 'includes',
+ 'dissoc': 'unset',
+ 'dissocPath': 'unset',
+ 'dropLast': 'dropRight',
+ 'dropLastWhile': 'dropRightWhile',
+ 'equals': 'isEqual',
+ 'identical': 'eq',
+ 'indexBy': 'keyBy',
+ 'init': 'initial',
+ 'invertObj': 'invert',
+ 'juxt': 'over',
+ 'omitAll': 'omit',
+ 'nAry': 'ary',
+ 'path': 'get',
+ 'pathEq': 'matchesProperty',
+ 'pathOr': 'getOr',
+ 'paths': 'at',
+ 'pickAll': 'pick',
+ 'pipe': 'flow',
+ 'pluck': 'map',
+ 'prop': 'get',
+ 'propEq': 'matchesProperty',
+ 'propOr': 'getOr',
+ 'props': 'at',
+ 'symmetricDifference': 'xor',
+ 'symmetricDifferenceBy': 'xorBy',
+ 'symmetricDifferenceWith': 'xorWith',
+ 'takeLast': 'takeRight',
+ 'takeLastWhile': 'takeRightWhile',
+ 'unapply': 'rest',
+ 'unnest': 'flatten',
+ 'useWith': 'overArgs',
+ 'where': 'conformsTo',
+ 'whereEq': 'isMatch',
+ 'zipObj': 'zipObject'
+};
+
+/** Used to map ary to method names. */
+exports.aryMethod = {
+ '1': [
+ 'assignAll', 'assignInAll', 'attempt', 'castArray', 'ceil', 'create',
+ 'curry', 'curryRight', 'defaultsAll', 'defaultsDeepAll', 'floor', 'flow',
+ 'flowRight', 'fromPairs', 'invert', 'iteratee', 'memoize', 'method', 'mergeAll',
+ 'methodOf', 'mixin', 'nthArg', 'over', 'overEvery', 'overSome','rest', 'reverse',
+ 'round', 'runInContext', 'spread', 'template', 'trim', 'trimEnd', 'trimStart',
+ 'uniqueId', 'words', 'zipAll'
+ ],
+ '2': [
+ 'add', 'after', 'ary', 'assign', 'assignAllWith', 'assignIn', 'assignInAllWith',
+ 'at', 'before', 'bind', 'bindAll', 'bindKey', 'chunk', 'cloneDeepWith',
+ 'cloneWith', 'concat', 'conformsTo', 'countBy', 'curryN', 'curryRightN',
+ 'debounce', 'defaults', 'defaultsDeep', 'defaultTo', 'delay', 'difference',
+ 'divide', 'drop', 'dropRight', 'dropRightWhile', 'dropWhile', 'endsWith', 'eq',
+ 'every', 'filter', 'find', 'findIndex', 'findKey', 'findLast', 'findLastIndex',
+ 'findLastKey', 'flatMap', 'flatMapDeep', 'flattenDepth', 'forEach',
+ 'forEachRight', 'forIn', 'forInRight', 'forOwn', 'forOwnRight', 'get',
+ 'groupBy', 'gt', 'gte', 'has', 'hasIn', 'includes', 'indexOf', 'intersection',
+ 'invertBy', 'invoke', 'invokeMap', 'isEqual', 'isMatch', 'join', 'keyBy',
+ 'lastIndexOf', 'lt', 'lte', 'map', 'mapKeys', 'mapValues', 'matchesProperty',
+ 'maxBy', 'meanBy', 'merge', 'mergeAllWith', 'minBy', 'multiply', 'nth', 'omit',
+ 'omitBy', 'overArgs', 'pad', 'padEnd', 'padStart', 'parseInt', 'partial',
+ 'partialRight', 'partition', 'pick', 'pickBy', 'propertyOf', 'pull', 'pullAll',
+ 'pullAt', 'random', 'range', 'rangeRight', 'rearg', 'reject', 'remove',
+ 'repeat', 'restFrom', 'result', 'sampleSize', 'some', 'sortBy', 'sortedIndex',
+ 'sortedIndexOf', 'sortedLastIndex', 'sortedLastIndexOf', 'sortedUniqBy',
+ 'split', 'spreadFrom', 'startsWith', 'subtract', 'sumBy', 'take', 'takeRight',
+ 'takeRightWhile', 'takeWhile', 'tap', 'throttle', 'thru', 'times', 'trimChars',
+ 'trimCharsEnd', 'trimCharsStart', 'truncate', 'union', 'uniqBy', 'uniqWith',
+ 'unset', 'unzipWith', 'without', 'wrap', 'xor', 'zip', 'zipObject',
+ 'zipObjectDeep'
+ ],
+ '3': [
+ 'assignInWith', 'assignWith', 'clamp', 'differenceBy', 'differenceWith',
+ 'findFrom', 'findIndexFrom', 'findLastFrom', 'findLastIndexFrom', 'getOr',
+ 'includesFrom', 'indexOfFrom', 'inRange', 'intersectionBy', 'intersectionWith',
+ 'invokeArgs', 'invokeArgsMap', 'isEqualWith', 'isMatchWith', 'flatMapDepth',
+ 'lastIndexOfFrom', 'mergeWith', 'orderBy', 'padChars', 'padCharsEnd',
+ 'padCharsStart', 'pullAllBy', 'pullAllWith', 'rangeStep', 'rangeStepRight',
+ 'reduce', 'reduceRight', 'replace', 'set', 'slice', 'sortedIndexBy',
+ 'sortedLastIndexBy', 'transform', 'unionBy', 'unionWith', 'update', 'xorBy',
+ 'xorWith', 'zipWith'
+ ],
+ '4': [
+ 'fill', 'setWith', 'updateWith'
+ ]
+};
+
+/** Used to map ary to rearg configs. */
+exports.aryRearg = {
+ '2': [1, 0],
+ '3': [2, 0, 1],
+ '4': [3, 2, 0, 1]
+};
+
+/** Used to map method names to their iteratee ary. */
+exports.iterateeAry = {
+ 'dropRightWhile': 1,
+ 'dropWhile': 1,
+ 'every': 1,
+ 'filter': 1,
+ 'find': 1,
+ 'findFrom': 1,
+ 'findIndex': 1,
+ 'findIndexFrom': 1,
+ 'findKey': 1,
+ 'findLast': 1,
+ 'findLastFrom': 1,
+ 'findLastIndex': 1,
+ 'findLastIndexFrom': 1,
+ 'findLastKey': 1,
+ 'flatMap': 1,
+ 'flatMapDeep': 1,
+ 'flatMapDepth': 1,
+ 'forEach': 1,
+ 'forEachRight': 1,
+ 'forIn': 1,
+ 'forInRight': 1,
+ 'forOwn': 1,
+ 'forOwnRight': 1,
+ 'map': 1,
+ 'mapKeys': 1,
+ 'mapValues': 1,
+ 'partition': 1,
+ 'reduce': 2,
+ 'reduceRight': 2,
+ 'reject': 1,
+ 'remove': 1,
+ 'some': 1,
+ 'takeRightWhile': 1,
+ 'takeWhile': 1,
+ 'times': 1,
+ 'transform': 2
+};
+
+/** Used to map method names to iteratee rearg configs. */
+exports.iterateeRearg = {
+ 'mapKeys': [1],
+ 'reduceRight': [1, 0]
+};
+
+/** Used to map method names to rearg configs. */
+exports.methodRearg = {
+ 'assignInAllWith': [1, 0],
+ 'assignInWith': [1, 2, 0],
+ 'assignAllWith': [1, 0],
+ 'assignWith': [1, 2, 0],
+ 'differenceBy': [1, 2, 0],
+ 'differenceWith': [1, 2, 0],
+ 'getOr': [2, 1, 0],
+ 'intersectionBy': [1, 2, 0],
+ 'intersectionWith': [1, 2, 0],
+ 'isEqualWith': [1, 2, 0],
+ 'isMatchWith': [2, 1, 0],
+ 'mergeAllWith': [1, 0],
+ 'mergeWith': [1, 2, 0],
+ 'padChars': [2, 1, 0],
+ 'padCharsEnd': [2, 1, 0],
+ 'padCharsStart': [2, 1, 0],
+ 'pullAllBy': [2, 1, 0],
+ 'pullAllWith': [2, 1, 0],
+ 'rangeStep': [1, 2, 0],
+ 'rangeStepRight': [1, 2, 0],
+ 'setWith': [3, 1, 2, 0],
+ 'sortedIndexBy': [2, 1, 0],
+ 'sortedLastIndexBy': [2, 1, 0],
+ 'unionBy': [1, 2, 0],
+ 'unionWith': [1, 2, 0],
+ 'updateWith': [3, 1, 2, 0],
+ 'xorBy': [1, 2, 0],
+ 'xorWith': [1, 2, 0],
+ 'zipWith': [1, 2, 0]
+};
+
+/** Used to map method names to spread configs. */
+exports.methodSpread = {
+ 'assignAll': { 'start': 0 },
+ 'assignAllWith': { 'start': 0 },
+ 'assignInAll': { 'start': 0 },
+ 'assignInAllWith': { 'start': 0 },
+ 'defaultsAll': { 'start': 0 },
+ 'defaultsDeepAll': { 'start': 0 },
+ 'invokeArgs': { 'start': 2 },
+ 'invokeArgsMap': { 'start': 2 },
+ 'mergeAll': { 'start': 0 },
+ 'mergeAllWith': { 'start': 0 },
+ 'partial': { 'start': 1 },
+ 'partialRight': { 'start': 1 },
+ 'without': { 'start': 1 },
+ 'zipAll': { 'start': 0 }
+};
+
+/** Used to identify methods which mutate arrays or objects. */
+exports.mutate = {
+ 'array': {
+ 'fill': true,
+ 'pull': true,
+ 'pullAll': true,
+ 'pullAllBy': true,
+ 'pullAllWith': true,
+ 'pullAt': true,
+ 'remove': true,
+ 'reverse': true
+ },
+ 'object': {
+ 'assign': true,
+ 'assignAll': true,
+ 'assignAllWith': true,
+ 'assignIn': true,
+ 'assignInAll': true,
+ 'assignInAllWith': true,
+ 'assignInWith': true,
+ 'assignWith': true,
+ 'defaults': true,
+ 'defaultsAll': true,
+ 'defaultsDeep': true,
+ 'defaultsDeepAll': true,
+ 'merge': true,
+ 'mergeAll': true,
+ 'mergeAllWith': true,
+ 'mergeWith': true,
+ },
+ 'set': {
+ 'set': true,
+ 'setWith': true,
+ 'unset': true,
+ 'update': true,
+ 'updateWith': true
+ }
+};
+
+/** Used to map real names to their aliases. */
+exports.realToAlias = (function() {
+ var hasOwnProperty = Object.prototype.hasOwnProperty,
+ object = exports.aliasToReal,
+ result = {};
+
+ for (var key in object) {
+ var value = object[key];
+ if (hasOwnProperty.call(result, value)) {
+ result[value].push(key);
+ } else {
+ result[value] = [key];
+ }
+ }
+ return result;
+}());
+
+/** Used to map method names to other names. */
+exports.remap = {
+ 'assignAll': 'assign',
+ 'assignAllWith': 'assignWith',
+ 'assignInAll': 'assignIn',
+ 'assignInAllWith': 'assignInWith',
+ 'curryN': 'curry',
+ 'curryRightN': 'curryRight',
+ 'defaultsAll': 'defaults',
+ 'defaultsDeepAll': 'defaultsDeep',
+ 'findFrom': 'find',
+ 'findIndexFrom': 'findIndex',
+ 'findLastFrom': 'findLast',
+ 'findLastIndexFrom': 'findLastIndex',
+ 'getOr': 'get',
+ 'includesFrom': 'includes',
+ 'indexOfFrom': 'indexOf',
+ 'invokeArgs': 'invoke',
+ 'invokeArgsMap': 'invokeMap',
+ 'lastIndexOfFrom': 'lastIndexOf',
+ 'mergeAll': 'merge',
+ 'mergeAllWith': 'mergeWith',
+ 'padChars': 'pad',
+ 'padCharsEnd': 'padEnd',
+ 'padCharsStart': 'padStart',
+ 'propertyOf': 'get',
+ 'rangeStep': 'range',
+ 'rangeStepRight': 'rangeRight',
+ 'restFrom': 'rest',
+ 'spreadFrom': 'spread',
+ 'trimChars': 'trim',
+ 'trimCharsEnd': 'trimEnd',
+ 'trimCharsStart': 'trimStart',
+ 'zipAll': 'zip'
+};
+
+/** Used to track methods that skip fixing their arity. */
+exports.skipFixed = {
+ 'castArray': true,
+ 'flow': true,
+ 'flowRight': true,
+ 'iteratee': true,
+ 'mixin': true,
+ 'rearg': true,
+ 'runInContext': true
+};
+
+/** Used to track methods that skip rearranging arguments. */
+exports.skipRearg = {
+ 'add': true,
+ 'assign': true,
+ 'assignIn': true,
+ 'bind': true,
+ 'bindKey': true,
+ 'concat': true,
+ 'difference': true,
+ 'divide': true,
+ 'eq': true,
+ 'gt': true,
+ 'gte': true,
+ 'isEqual': true,
+ 'lt': true,
+ 'lte': true,
+ 'matchesProperty': true,
+ 'merge': true,
+ 'multiply': true,
+ 'overArgs': true,
+ 'partial': true,
+ 'partialRight': true,
+ 'propertyOf': true,
+ 'random': true,
+ 'range': true,
+ 'rangeRight': true,
+ 'subtract': true,
+ 'zip': true,
+ 'zipObject': true,
+ 'zipObjectDeep': true
+};
diff --git a/node_modules/lodash/fp/_util.js b/node_modules/lodash/fp/_util.js
new file mode 100644
index 0000000..1dbf36f
--- /dev/null
+++ b/node_modules/lodash/fp/_util.js
@@ -0,0 +1,16 @@
+module.exports = {
+ 'ary': require('../ary'),
+ 'assign': require('../_baseAssign'),
+ 'clone': require('../clone'),
+ 'curry': require('../curry'),
+ 'forEach': require('../_arrayEach'),
+ 'isArray': require('../isArray'),
+ 'isError': require('../isError'),
+ 'isFunction': require('../isFunction'),
+ 'isWeakMap': require('../isWeakMap'),
+ 'iteratee': require('../iteratee'),
+ 'keys': require('../_baseKeys'),
+ 'rearg': require('../rearg'),
+ 'toInteger': require('../toInteger'),
+ 'toPath': require('../toPath')
+};
diff --git a/node_modules/lodash/fp/add.js b/node_modules/lodash/fp/add.js
new file mode 100644
index 0000000..816eeec
--- /dev/null
+++ b/node_modules/lodash/fp/add.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('add', require('../add'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/after.js b/node_modules/lodash/fp/after.js
new file mode 100644
index 0000000..21a0167
--- /dev/null
+++ b/node_modules/lodash/fp/after.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('after', require('../after'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/all.js b/node_modules/lodash/fp/all.js
new file mode 100644
index 0000000..d0839f7
--- /dev/null
+++ b/node_modules/lodash/fp/all.js
@@ -0,0 +1 @@
+module.exports = require('./every');
diff --git a/node_modules/lodash/fp/allPass.js b/node_modules/lodash/fp/allPass.js
new file mode 100644
index 0000000..79b73ef
--- /dev/null
+++ b/node_modules/lodash/fp/allPass.js
@@ -0,0 +1 @@
+module.exports = require('./overEvery');
diff --git a/node_modules/lodash/fp/always.js b/node_modules/lodash/fp/always.js
new file mode 100644
index 0000000..9887703
--- /dev/null
+++ b/node_modules/lodash/fp/always.js
@@ -0,0 +1 @@
+module.exports = require('./constant');
diff --git a/node_modules/lodash/fp/any.js b/node_modules/lodash/fp/any.js
new file mode 100644
index 0000000..900ac25
--- /dev/null
+++ b/node_modules/lodash/fp/any.js
@@ -0,0 +1 @@
+module.exports = require('./some');
diff --git a/node_modules/lodash/fp/anyPass.js b/node_modules/lodash/fp/anyPass.js
new file mode 100644
index 0000000..2774ab3
--- /dev/null
+++ b/node_modules/lodash/fp/anyPass.js
@@ -0,0 +1 @@
+module.exports = require('./overSome');
diff --git a/node_modules/lodash/fp/apply.js b/node_modules/lodash/fp/apply.js
new file mode 100644
index 0000000..2b75712
--- /dev/null
+++ b/node_modules/lodash/fp/apply.js
@@ -0,0 +1 @@
+module.exports = require('./spread');
diff --git a/node_modules/lodash/fp/array.js b/node_modules/lodash/fp/array.js
new file mode 100644
index 0000000..fe939c2
--- /dev/null
+++ b/node_modules/lodash/fp/array.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../array'));
diff --git a/node_modules/lodash/fp/ary.js b/node_modules/lodash/fp/ary.js
new file mode 100644
index 0000000..8edf187
--- /dev/null
+++ b/node_modules/lodash/fp/ary.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('ary', require('../ary'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/assign.js b/node_modules/lodash/fp/assign.js
new file mode 100644
index 0000000..23f47af
--- /dev/null
+++ b/node_modules/lodash/fp/assign.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('assign', require('../assign'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/assignAll.js b/node_modules/lodash/fp/assignAll.js
new file mode 100644
index 0000000..b1d36c7
--- /dev/null
+++ b/node_modules/lodash/fp/assignAll.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('assignAll', require('../assign'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/assignAllWith.js b/node_modules/lodash/fp/assignAllWith.js
new file mode 100644
index 0000000..21e836e
--- /dev/null
+++ b/node_modules/lodash/fp/assignAllWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('assignAllWith', require('../assignWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/assignIn.js b/node_modules/lodash/fp/assignIn.js
new file mode 100644
index 0000000..6e7c65f
--- /dev/null
+++ b/node_modules/lodash/fp/assignIn.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('assignIn', require('../assignIn'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/assignInAll.js b/node_modules/lodash/fp/assignInAll.js
new file mode 100644
index 0000000..7ba75db
--- /dev/null
+++ b/node_modules/lodash/fp/assignInAll.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('assignInAll', require('../assignIn'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/assignInAllWith.js b/node_modules/lodash/fp/assignInAllWith.js
new file mode 100644
index 0000000..e766903
--- /dev/null
+++ b/node_modules/lodash/fp/assignInAllWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('assignInAllWith', require('../assignInWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/assignInWith.js b/node_modules/lodash/fp/assignInWith.js
new file mode 100644
index 0000000..acb5923
--- /dev/null
+++ b/node_modules/lodash/fp/assignInWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('assignInWith', require('../assignInWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/assignWith.js b/node_modules/lodash/fp/assignWith.js
new file mode 100644
index 0000000..eb92521
--- /dev/null
+++ b/node_modules/lodash/fp/assignWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('assignWith', require('../assignWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/assoc.js b/node_modules/lodash/fp/assoc.js
new file mode 100644
index 0000000..7648820
--- /dev/null
+++ b/node_modules/lodash/fp/assoc.js
@@ -0,0 +1 @@
+module.exports = require('./set');
diff --git a/node_modules/lodash/fp/assocPath.js b/node_modules/lodash/fp/assocPath.js
new file mode 100644
index 0000000..7648820
--- /dev/null
+++ b/node_modules/lodash/fp/assocPath.js
@@ -0,0 +1 @@
+module.exports = require('./set');
diff --git a/node_modules/lodash/fp/at.js b/node_modules/lodash/fp/at.js
new file mode 100644
index 0000000..cc39d25
--- /dev/null
+++ b/node_modules/lodash/fp/at.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('at', require('../at'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/attempt.js b/node_modules/lodash/fp/attempt.js
new file mode 100644
index 0000000..26ca42e
--- /dev/null
+++ b/node_modules/lodash/fp/attempt.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('attempt', require('../attempt'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/before.js b/node_modules/lodash/fp/before.js
new file mode 100644
index 0000000..7a2de65
--- /dev/null
+++ b/node_modules/lodash/fp/before.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('before', require('../before'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/bind.js b/node_modules/lodash/fp/bind.js
new file mode 100644
index 0000000..5cbe4f3
--- /dev/null
+++ b/node_modules/lodash/fp/bind.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('bind', require('../bind'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/bindAll.js b/node_modules/lodash/fp/bindAll.js
new file mode 100644
index 0000000..6b4a4a0
--- /dev/null
+++ b/node_modules/lodash/fp/bindAll.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('bindAll', require('../bindAll'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/bindKey.js b/node_modules/lodash/fp/bindKey.js
new file mode 100644
index 0000000..6a46c6b
--- /dev/null
+++ b/node_modules/lodash/fp/bindKey.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('bindKey', require('../bindKey'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/camelCase.js b/node_modules/lodash/fp/camelCase.js
new file mode 100644
index 0000000..87b77b4
--- /dev/null
+++ b/node_modules/lodash/fp/camelCase.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('camelCase', require('../camelCase'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/capitalize.js b/node_modules/lodash/fp/capitalize.js
new file mode 100644
index 0000000..cac74e1
--- /dev/null
+++ b/node_modules/lodash/fp/capitalize.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('capitalize', require('../capitalize'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/castArray.js b/node_modules/lodash/fp/castArray.js
new file mode 100644
index 0000000..8681c09
--- /dev/null
+++ b/node_modules/lodash/fp/castArray.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('castArray', require('../castArray'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/ceil.js b/node_modules/lodash/fp/ceil.js
new file mode 100644
index 0000000..f416b72
--- /dev/null
+++ b/node_modules/lodash/fp/ceil.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('ceil', require('../ceil'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/chain.js b/node_modules/lodash/fp/chain.js
new file mode 100644
index 0000000..604fe39
--- /dev/null
+++ b/node_modules/lodash/fp/chain.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('chain', require('../chain'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/chunk.js b/node_modules/lodash/fp/chunk.js
new file mode 100644
index 0000000..871ab08
--- /dev/null
+++ b/node_modules/lodash/fp/chunk.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('chunk', require('../chunk'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/clamp.js b/node_modules/lodash/fp/clamp.js
new file mode 100644
index 0000000..3b06c01
--- /dev/null
+++ b/node_modules/lodash/fp/clamp.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('clamp', require('../clamp'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/clone.js b/node_modules/lodash/fp/clone.js
new file mode 100644
index 0000000..cadb59c
--- /dev/null
+++ b/node_modules/lodash/fp/clone.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('clone', require('../clone'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/cloneDeep.js b/node_modules/lodash/fp/cloneDeep.js
new file mode 100644
index 0000000..a6107aa
--- /dev/null
+++ b/node_modules/lodash/fp/cloneDeep.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('cloneDeep', require('../cloneDeep'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/cloneDeepWith.js b/node_modules/lodash/fp/cloneDeepWith.js
new file mode 100644
index 0000000..6f01e44
--- /dev/null
+++ b/node_modules/lodash/fp/cloneDeepWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('cloneDeepWith', require('../cloneDeepWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/cloneWith.js b/node_modules/lodash/fp/cloneWith.js
new file mode 100644
index 0000000..aa88578
--- /dev/null
+++ b/node_modules/lodash/fp/cloneWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('cloneWith', require('../cloneWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/collection.js b/node_modules/lodash/fp/collection.js
new file mode 100644
index 0000000..fc8b328
--- /dev/null
+++ b/node_modules/lodash/fp/collection.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../collection'));
diff --git a/node_modules/lodash/fp/commit.js b/node_modules/lodash/fp/commit.js
new file mode 100644
index 0000000..130a894
--- /dev/null
+++ b/node_modules/lodash/fp/commit.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('commit', require('../commit'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/compact.js b/node_modules/lodash/fp/compact.js
new file mode 100644
index 0000000..ce8f7a1
--- /dev/null
+++ b/node_modules/lodash/fp/compact.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('compact', require('../compact'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/complement.js b/node_modules/lodash/fp/complement.js
new file mode 100644
index 0000000..93eb462
--- /dev/null
+++ b/node_modules/lodash/fp/complement.js
@@ -0,0 +1 @@
+module.exports = require('./negate');
diff --git a/node_modules/lodash/fp/compose.js b/node_modules/lodash/fp/compose.js
new file mode 100644
index 0000000..1954e94
--- /dev/null
+++ b/node_modules/lodash/fp/compose.js
@@ -0,0 +1 @@
+module.exports = require('./flowRight');
diff --git a/node_modules/lodash/fp/concat.js b/node_modules/lodash/fp/concat.js
new file mode 100644
index 0000000..e59346a
--- /dev/null
+++ b/node_modules/lodash/fp/concat.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('concat', require('../concat'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/cond.js b/node_modules/lodash/fp/cond.js
new file mode 100644
index 0000000..6a0120e
--- /dev/null
+++ b/node_modules/lodash/fp/cond.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('cond', require('../cond'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/conforms.js b/node_modules/lodash/fp/conforms.js
new file mode 100644
index 0000000..3247f64
--- /dev/null
+++ b/node_modules/lodash/fp/conforms.js
@@ -0,0 +1 @@
+module.exports = require('./conformsTo');
diff --git a/node_modules/lodash/fp/conformsTo.js b/node_modules/lodash/fp/conformsTo.js
new file mode 100644
index 0000000..aa7f41e
--- /dev/null
+++ b/node_modules/lodash/fp/conformsTo.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('conformsTo', require('../conformsTo'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/constant.js b/node_modules/lodash/fp/constant.js
new file mode 100644
index 0000000..9e406fc
--- /dev/null
+++ b/node_modules/lodash/fp/constant.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('constant', require('../constant'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/contains.js b/node_modules/lodash/fp/contains.js
new file mode 100644
index 0000000..594722a
--- /dev/null
+++ b/node_modules/lodash/fp/contains.js
@@ -0,0 +1 @@
+module.exports = require('./includes');
diff --git a/node_modules/lodash/fp/convert.js b/node_modules/lodash/fp/convert.js
new file mode 100644
index 0000000..4795dc4
--- /dev/null
+++ b/node_modules/lodash/fp/convert.js
@@ -0,0 +1,18 @@
+var baseConvert = require('./_baseConvert'),
+ util = require('./_util');
+
+/**
+ * Converts `func` of `name` to an immutable auto-curried iteratee-first data-last
+ * version with conversion `options` applied. If `name` is an object its methods
+ * will be converted.
+ *
+ * @param {string} name The name of the function to wrap.
+ * @param {Function} [func] The function to wrap.
+ * @param {Object} [options] The options object. See `baseConvert` for more details.
+ * @returns {Function|Object} Returns the converted function or object.
+ */
+function convert(name, func, options) {
+ return baseConvert(util, name, func, options);
+}
+
+module.exports = convert;
diff --git a/node_modules/lodash/fp/countBy.js b/node_modules/lodash/fp/countBy.js
new file mode 100644
index 0000000..dfa4643
--- /dev/null
+++ b/node_modules/lodash/fp/countBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('countBy', require('../countBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/create.js b/node_modules/lodash/fp/create.js
new file mode 100644
index 0000000..752025f
--- /dev/null
+++ b/node_modules/lodash/fp/create.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('create', require('../create'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/curry.js b/node_modules/lodash/fp/curry.js
new file mode 100644
index 0000000..b0b4168
--- /dev/null
+++ b/node_modules/lodash/fp/curry.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('curry', require('../curry'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/curryN.js b/node_modules/lodash/fp/curryN.js
new file mode 100644
index 0000000..2ae7d00
--- /dev/null
+++ b/node_modules/lodash/fp/curryN.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('curryN', require('../curry'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/curryRight.js b/node_modules/lodash/fp/curryRight.js
new file mode 100644
index 0000000..cb619eb
--- /dev/null
+++ b/node_modules/lodash/fp/curryRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('curryRight', require('../curryRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/curryRightN.js b/node_modules/lodash/fp/curryRightN.js
new file mode 100644
index 0000000..2495afc
--- /dev/null
+++ b/node_modules/lodash/fp/curryRightN.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('curryRightN', require('../curryRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/date.js b/node_modules/lodash/fp/date.js
new file mode 100644
index 0000000..82cb952
--- /dev/null
+++ b/node_modules/lodash/fp/date.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../date'));
diff --git a/node_modules/lodash/fp/debounce.js b/node_modules/lodash/fp/debounce.js
new file mode 100644
index 0000000..2612229
--- /dev/null
+++ b/node_modules/lodash/fp/debounce.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('debounce', require('../debounce'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/deburr.js b/node_modules/lodash/fp/deburr.js
new file mode 100644
index 0000000..96463ab
--- /dev/null
+++ b/node_modules/lodash/fp/deburr.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('deburr', require('../deburr'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/defaultTo.js b/node_modules/lodash/fp/defaultTo.js
new file mode 100644
index 0000000..d6b52a4
--- /dev/null
+++ b/node_modules/lodash/fp/defaultTo.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('defaultTo', require('../defaultTo'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/defaults.js b/node_modules/lodash/fp/defaults.js
new file mode 100644
index 0000000..e1a8e6e
--- /dev/null
+++ b/node_modules/lodash/fp/defaults.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('defaults', require('../defaults'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/defaultsAll.js b/node_modules/lodash/fp/defaultsAll.js
new file mode 100644
index 0000000..238fcc3
--- /dev/null
+++ b/node_modules/lodash/fp/defaultsAll.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('defaultsAll', require('../defaults'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/defaultsDeep.js b/node_modules/lodash/fp/defaultsDeep.js
new file mode 100644
index 0000000..1f172ff
--- /dev/null
+++ b/node_modules/lodash/fp/defaultsDeep.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('defaultsDeep', require('../defaultsDeep'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/defaultsDeepAll.js b/node_modules/lodash/fp/defaultsDeepAll.js
new file mode 100644
index 0000000..6835f2f
--- /dev/null
+++ b/node_modules/lodash/fp/defaultsDeepAll.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('defaultsDeepAll', require('../defaultsDeep'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/defer.js b/node_modules/lodash/fp/defer.js
new file mode 100644
index 0000000..ec7990f
--- /dev/null
+++ b/node_modules/lodash/fp/defer.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('defer', require('../defer'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/delay.js b/node_modules/lodash/fp/delay.js
new file mode 100644
index 0000000..556dbd5
--- /dev/null
+++ b/node_modules/lodash/fp/delay.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('delay', require('../delay'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/difference.js b/node_modules/lodash/fp/difference.js
new file mode 100644
index 0000000..2d03765
--- /dev/null
+++ b/node_modules/lodash/fp/difference.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('difference', require('../difference'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/differenceBy.js b/node_modules/lodash/fp/differenceBy.js
new file mode 100644
index 0000000..2f91491
--- /dev/null
+++ b/node_modules/lodash/fp/differenceBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('differenceBy', require('../differenceBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/differenceWith.js b/node_modules/lodash/fp/differenceWith.js
new file mode 100644
index 0000000..bcf5ad2
--- /dev/null
+++ b/node_modules/lodash/fp/differenceWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('differenceWith', require('../differenceWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/dissoc.js b/node_modules/lodash/fp/dissoc.js
new file mode 100644
index 0000000..7ec7be1
--- /dev/null
+++ b/node_modules/lodash/fp/dissoc.js
@@ -0,0 +1 @@
+module.exports = require('./unset');
diff --git a/node_modules/lodash/fp/dissocPath.js b/node_modules/lodash/fp/dissocPath.js
new file mode 100644
index 0000000..7ec7be1
--- /dev/null
+++ b/node_modules/lodash/fp/dissocPath.js
@@ -0,0 +1 @@
+module.exports = require('./unset');
diff --git a/node_modules/lodash/fp/divide.js b/node_modules/lodash/fp/divide.js
new file mode 100644
index 0000000..82048c5
--- /dev/null
+++ b/node_modules/lodash/fp/divide.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('divide', require('../divide'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/drop.js b/node_modules/lodash/fp/drop.js
new file mode 100644
index 0000000..2fa9b4f
--- /dev/null
+++ b/node_modules/lodash/fp/drop.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('drop', require('../drop'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/dropLast.js b/node_modules/lodash/fp/dropLast.js
new file mode 100644
index 0000000..174e525
--- /dev/null
+++ b/node_modules/lodash/fp/dropLast.js
@@ -0,0 +1 @@
+module.exports = require('./dropRight');
diff --git a/node_modules/lodash/fp/dropLastWhile.js b/node_modules/lodash/fp/dropLastWhile.js
new file mode 100644
index 0000000..be2a9d2
--- /dev/null
+++ b/node_modules/lodash/fp/dropLastWhile.js
@@ -0,0 +1 @@
+module.exports = require('./dropRightWhile');
diff --git a/node_modules/lodash/fp/dropRight.js b/node_modules/lodash/fp/dropRight.js
new file mode 100644
index 0000000..e98881f
--- /dev/null
+++ b/node_modules/lodash/fp/dropRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('dropRight', require('../dropRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/dropRightWhile.js b/node_modules/lodash/fp/dropRightWhile.js
new file mode 100644
index 0000000..cacaa70
--- /dev/null
+++ b/node_modules/lodash/fp/dropRightWhile.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('dropRightWhile', require('../dropRightWhile'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/dropWhile.js b/node_modules/lodash/fp/dropWhile.js
new file mode 100644
index 0000000..285f864
--- /dev/null
+++ b/node_modules/lodash/fp/dropWhile.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('dropWhile', require('../dropWhile'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/each.js b/node_modules/lodash/fp/each.js
new file mode 100644
index 0000000..8800f42
--- /dev/null
+++ b/node_modules/lodash/fp/each.js
@@ -0,0 +1 @@
+module.exports = require('./forEach');
diff --git a/node_modules/lodash/fp/eachRight.js b/node_modules/lodash/fp/eachRight.js
new file mode 100644
index 0000000..3252b2a
--- /dev/null
+++ b/node_modules/lodash/fp/eachRight.js
@@ -0,0 +1 @@
+module.exports = require('./forEachRight');
diff --git a/node_modules/lodash/fp/endsWith.js b/node_modules/lodash/fp/endsWith.js
new file mode 100644
index 0000000..17dc2a4
--- /dev/null
+++ b/node_modules/lodash/fp/endsWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('endsWith', require('../endsWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/entries.js b/node_modules/lodash/fp/entries.js
new file mode 100644
index 0000000..7a88df2
--- /dev/null
+++ b/node_modules/lodash/fp/entries.js
@@ -0,0 +1 @@
+module.exports = require('./toPairs');
diff --git a/node_modules/lodash/fp/entriesIn.js b/node_modules/lodash/fp/entriesIn.js
new file mode 100644
index 0000000..f6c6331
--- /dev/null
+++ b/node_modules/lodash/fp/entriesIn.js
@@ -0,0 +1 @@
+module.exports = require('./toPairsIn');
diff --git a/node_modules/lodash/fp/eq.js b/node_modules/lodash/fp/eq.js
new file mode 100644
index 0000000..9a3d21b
--- /dev/null
+++ b/node_modules/lodash/fp/eq.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('eq', require('../eq'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/equals.js b/node_modules/lodash/fp/equals.js
new file mode 100644
index 0000000..e6a5ce0
--- /dev/null
+++ b/node_modules/lodash/fp/equals.js
@@ -0,0 +1 @@
+module.exports = require('./isEqual');
diff --git a/node_modules/lodash/fp/escape.js b/node_modules/lodash/fp/escape.js
new file mode 100644
index 0000000..52c1fbb
--- /dev/null
+++ b/node_modules/lodash/fp/escape.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('escape', require('../escape'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/escapeRegExp.js b/node_modules/lodash/fp/escapeRegExp.js
new file mode 100644
index 0000000..369b2ef
--- /dev/null
+++ b/node_modules/lodash/fp/escapeRegExp.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('escapeRegExp', require('../escapeRegExp'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/every.js b/node_modules/lodash/fp/every.js
new file mode 100644
index 0000000..95c2776
--- /dev/null
+++ b/node_modules/lodash/fp/every.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('every', require('../every'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/extend.js b/node_modules/lodash/fp/extend.js
new file mode 100644
index 0000000..e00166c
--- /dev/null
+++ b/node_modules/lodash/fp/extend.js
@@ -0,0 +1 @@
+module.exports = require('./assignIn');
diff --git a/node_modules/lodash/fp/extendAll.js b/node_modules/lodash/fp/extendAll.js
new file mode 100644
index 0000000..cc55b64
--- /dev/null
+++ b/node_modules/lodash/fp/extendAll.js
@@ -0,0 +1 @@
+module.exports = require('./assignInAll');
diff --git a/node_modules/lodash/fp/extendAllWith.js b/node_modules/lodash/fp/extendAllWith.js
new file mode 100644
index 0000000..6679d20
--- /dev/null
+++ b/node_modules/lodash/fp/extendAllWith.js
@@ -0,0 +1 @@
+module.exports = require('./assignInAllWith');
diff --git a/node_modules/lodash/fp/extendWith.js b/node_modules/lodash/fp/extendWith.js
new file mode 100644
index 0000000..dbdcb3b
--- /dev/null
+++ b/node_modules/lodash/fp/extendWith.js
@@ -0,0 +1 @@
+module.exports = require('./assignInWith');
diff --git a/node_modules/lodash/fp/fill.js b/node_modules/lodash/fp/fill.js
new file mode 100644
index 0000000..b2d47e8
--- /dev/null
+++ b/node_modules/lodash/fp/fill.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('fill', require('../fill'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/filter.js b/node_modules/lodash/fp/filter.js
new file mode 100644
index 0000000..796d501
--- /dev/null
+++ b/node_modules/lodash/fp/filter.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('filter', require('../filter'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/find.js b/node_modules/lodash/fp/find.js
new file mode 100644
index 0000000..f805d33
--- /dev/null
+++ b/node_modules/lodash/fp/find.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('find', require('../find'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/findFrom.js b/node_modules/lodash/fp/findFrom.js
new file mode 100644
index 0000000..da8275e
--- /dev/null
+++ b/node_modules/lodash/fp/findFrom.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('findFrom', require('../find'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/findIndex.js b/node_modules/lodash/fp/findIndex.js
new file mode 100644
index 0000000..8c15fd1
--- /dev/null
+++ b/node_modules/lodash/fp/findIndex.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('findIndex', require('../findIndex'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/findIndexFrom.js b/node_modules/lodash/fp/findIndexFrom.js
new file mode 100644
index 0000000..32e98cb
--- /dev/null
+++ b/node_modules/lodash/fp/findIndexFrom.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('findIndexFrom', require('../findIndex'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/findKey.js b/node_modules/lodash/fp/findKey.js
new file mode 100644
index 0000000..475bcfa
--- /dev/null
+++ b/node_modules/lodash/fp/findKey.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('findKey', require('../findKey'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/findLast.js b/node_modules/lodash/fp/findLast.js
new file mode 100644
index 0000000..093fe94
--- /dev/null
+++ b/node_modules/lodash/fp/findLast.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('findLast', require('../findLast'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/findLastFrom.js b/node_modules/lodash/fp/findLastFrom.js
new file mode 100644
index 0000000..76c38fb
--- /dev/null
+++ b/node_modules/lodash/fp/findLastFrom.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('findLastFrom', require('../findLast'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/findLastIndex.js b/node_modules/lodash/fp/findLastIndex.js
new file mode 100644
index 0000000..36986df
--- /dev/null
+++ b/node_modules/lodash/fp/findLastIndex.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('findLastIndex', require('../findLastIndex'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/findLastIndexFrom.js b/node_modules/lodash/fp/findLastIndexFrom.js
new file mode 100644
index 0000000..34c8176
--- /dev/null
+++ b/node_modules/lodash/fp/findLastIndexFrom.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('findLastIndexFrom', require('../findLastIndex'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/findLastKey.js b/node_modules/lodash/fp/findLastKey.js
new file mode 100644
index 0000000..5f81b60
--- /dev/null
+++ b/node_modules/lodash/fp/findLastKey.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('findLastKey', require('../findLastKey'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/first.js b/node_modules/lodash/fp/first.js
new file mode 100644
index 0000000..53f4ad1
--- /dev/null
+++ b/node_modules/lodash/fp/first.js
@@ -0,0 +1 @@
+module.exports = require('./head');
diff --git a/node_modules/lodash/fp/flatMap.js b/node_modules/lodash/fp/flatMap.js
new file mode 100644
index 0000000..d01dc4d
--- /dev/null
+++ b/node_modules/lodash/fp/flatMap.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('flatMap', require('../flatMap'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/flatMapDeep.js b/node_modules/lodash/fp/flatMapDeep.js
new file mode 100644
index 0000000..569c42e
--- /dev/null
+++ b/node_modules/lodash/fp/flatMapDeep.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('flatMapDeep', require('../flatMapDeep'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/flatMapDepth.js b/node_modules/lodash/fp/flatMapDepth.js
new file mode 100644
index 0000000..6eb68fd
--- /dev/null
+++ b/node_modules/lodash/fp/flatMapDepth.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('flatMapDepth', require('../flatMapDepth'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/flatten.js b/node_modules/lodash/fp/flatten.js
new file mode 100644
index 0000000..30425d8
--- /dev/null
+++ b/node_modules/lodash/fp/flatten.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('flatten', require('../flatten'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/flattenDeep.js b/node_modules/lodash/fp/flattenDeep.js
new file mode 100644
index 0000000..aed5db2
--- /dev/null
+++ b/node_modules/lodash/fp/flattenDeep.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('flattenDeep', require('../flattenDeep'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/flattenDepth.js b/node_modules/lodash/fp/flattenDepth.js
new file mode 100644
index 0000000..ad65e37
--- /dev/null
+++ b/node_modules/lodash/fp/flattenDepth.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('flattenDepth', require('../flattenDepth'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/flip.js b/node_modules/lodash/fp/flip.js
new file mode 100644
index 0000000..0547e7b
--- /dev/null
+++ b/node_modules/lodash/fp/flip.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('flip', require('../flip'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/floor.js b/node_modules/lodash/fp/floor.js
new file mode 100644
index 0000000..a6cf335
--- /dev/null
+++ b/node_modules/lodash/fp/floor.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('floor', require('../floor'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/flow.js b/node_modules/lodash/fp/flow.js
new file mode 100644
index 0000000..cd83677
--- /dev/null
+++ b/node_modules/lodash/fp/flow.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('flow', require('../flow'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/flowRight.js b/node_modules/lodash/fp/flowRight.js
new file mode 100644
index 0000000..972a5b9
--- /dev/null
+++ b/node_modules/lodash/fp/flowRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('flowRight', require('../flowRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/forEach.js b/node_modules/lodash/fp/forEach.js
new file mode 100644
index 0000000..2f49452
--- /dev/null
+++ b/node_modules/lodash/fp/forEach.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('forEach', require('../forEach'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/forEachRight.js b/node_modules/lodash/fp/forEachRight.js
new file mode 100644
index 0000000..3ff9733
--- /dev/null
+++ b/node_modules/lodash/fp/forEachRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('forEachRight', require('../forEachRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/forIn.js b/node_modules/lodash/fp/forIn.js
new file mode 100644
index 0000000..9341749
--- /dev/null
+++ b/node_modules/lodash/fp/forIn.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('forIn', require('../forIn'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/forInRight.js b/node_modules/lodash/fp/forInRight.js
new file mode 100644
index 0000000..cecf8bb
--- /dev/null
+++ b/node_modules/lodash/fp/forInRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('forInRight', require('../forInRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/forOwn.js b/node_modules/lodash/fp/forOwn.js
new file mode 100644
index 0000000..246449e
--- /dev/null
+++ b/node_modules/lodash/fp/forOwn.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('forOwn', require('../forOwn'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/forOwnRight.js b/node_modules/lodash/fp/forOwnRight.js
new file mode 100644
index 0000000..c5e826e
--- /dev/null
+++ b/node_modules/lodash/fp/forOwnRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('forOwnRight', require('../forOwnRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/fromPairs.js b/node_modules/lodash/fp/fromPairs.js
new file mode 100644
index 0000000..f8cc596
--- /dev/null
+++ b/node_modules/lodash/fp/fromPairs.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('fromPairs', require('../fromPairs'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/function.js b/node_modules/lodash/fp/function.js
new file mode 100644
index 0000000..dfe69b1
--- /dev/null
+++ b/node_modules/lodash/fp/function.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../function'));
diff --git a/node_modules/lodash/fp/functions.js b/node_modules/lodash/fp/functions.js
new file mode 100644
index 0000000..09d1bb1
--- /dev/null
+++ b/node_modules/lodash/fp/functions.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('functions', require('../functions'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/functionsIn.js b/node_modules/lodash/fp/functionsIn.js
new file mode 100644
index 0000000..2cfeb83
--- /dev/null
+++ b/node_modules/lodash/fp/functionsIn.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('functionsIn', require('../functionsIn'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/get.js b/node_modules/lodash/fp/get.js
new file mode 100644
index 0000000..6d3a328
--- /dev/null
+++ b/node_modules/lodash/fp/get.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('get', require('../get'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/getOr.js b/node_modules/lodash/fp/getOr.js
new file mode 100644
index 0000000..7dbf771
--- /dev/null
+++ b/node_modules/lodash/fp/getOr.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('getOr', require('../get'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/groupBy.js b/node_modules/lodash/fp/groupBy.js
new file mode 100644
index 0000000..fc0bc78
--- /dev/null
+++ b/node_modules/lodash/fp/groupBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('groupBy', require('../groupBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/gt.js b/node_modules/lodash/fp/gt.js
new file mode 100644
index 0000000..9e57c80
--- /dev/null
+++ b/node_modules/lodash/fp/gt.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('gt', require('../gt'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/gte.js b/node_modules/lodash/fp/gte.js
new file mode 100644
index 0000000..4584786
--- /dev/null
+++ b/node_modules/lodash/fp/gte.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('gte', require('../gte'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/has.js b/node_modules/lodash/fp/has.js
new file mode 100644
index 0000000..b901298
--- /dev/null
+++ b/node_modules/lodash/fp/has.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('has', require('../has'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/hasIn.js b/node_modules/lodash/fp/hasIn.js
new file mode 100644
index 0000000..b3c3d1a
--- /dev/null
+++ b/node_modules/lodash/fp/hasIn.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('hasIn', require('../hasIn'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/head.js b/node_modules/lodash/fp/head.js
new file mode 100644
index 0000000..2694f0a
--- /dev/null
+++ b/node_modules/lodash/fp/head.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('head', require('../head'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/identical.js b/node_modules/lodash/fp/identical.js
new file mode 100644
index 0000000..85563f4
--- /dev/null
+++ b/node_modules/lodash/fp/identical.js
@@ -0,0 +1 @@
+module.exports = require('./eq');
diff --git a/node_modules/lodash/fp/identity.js b/node_modules/lodash/fp/identity.js
new file mode 100644
index 0000000..096415a
--- /dev/null
+++ b/node_modules/lodash/fp/identity.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('identity', require('../identity'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/inRange.js b/node_modules/lodash/fp/inRange.js
new file mode 100644
index 0000000..202d940
--- /dev/null
+++ b/node_modules/lodash/fp/inRange.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('inRange', require('../inRange'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/includes.js b/node_modules/lodash/fp/includes.js
new file mode 100644
index 0000000..1146780
--- /dev/null
+++ b/node_modules/lodash/fp/includes.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('includes', require('../includes'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/includesFrom.js b/node_modules/lodash/fp/includesFrom.js
new file mode 100644
index 0000000..683afdb
--- /dev/null
+++ b/node_modules/lodash/fp/includesFrom.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('includesFrom', require('../includes'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/indexBy.js b/node_modules/lodash/fp/indexBy.js
new file mode 100644
index 0000000..7e64bc0
--- /dev/null
+++ b/node_modules/lodash/fp/indexBy.js
@@ -0,0 +1 @@
+module.exports = require('./keyBy');
diff --git a/node_modules/lodash/fp/indexOf.js b/node_modules/lodash/fp/indexOf.js
new file mode 100644
index 0000000..524658e
--- /dev/null
+++ b/node_modules/lodash/fp/indexOf.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('indexOf', require('../indexOf'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/indexOfFrom.js b/node_modules/lodash/fp/indexOfFrom.js
new file mode 100644
index 0000000..d99c822
--- /dev/null
+++ b/node_modules/lodash/fp/indexOfFrom.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('indexOfFrom', require('../indexOf'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/init.js b/node_modules/lodash/fp/init.js
new file mode 100644
index 0000000..2f88d8b
--- /dev/null
+++ b/node_modules/lodash/fp/init.js
@@ -0,0 +1 @@
+module.exports = require('./initial');
diff --git a/node_modules/lodash/fp/initial.js b/node_modules/lodash/fp/initial.js
new file mode 100644
index 0000000..b732ba0
--- /dev/null
+++ b/node_modules/lodash/fp/initial.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('initial', require('../initial'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/intersection.js b/node_modules/lodash/fp/intersection.js
new file mode 100644
index 0000000..52936d5
--- /dev/null
+++ b/node_modules/lodash/fp/intersection.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('intersection', require('../intersection'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/intersectionBy.js b/node_modules/lodash/fp/intersectionBy.js
new file mode 100644
index 0000000..72629f2
--- /dev/null
+++ b/node_modules/lodash/fp/intersectionBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('intersectionBy', require('../intersectionBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/intersectionWith.js b/node_modules/lodash/fp/intersectionWith.js
new file mode 100644
index 0000000..e064f40
--- /dev/null
+++ b/node_modules/lodash/fp/intersectionWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('intersectionWith', require('../intersectionWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/invert.js b/node_modules/lodash/fp/invert.js
new file mode 100644
index 0000000..2d5d1f0
--- /dev/null
+++ b/node_modules/lodash/fp/invert.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('invert', require('../invert'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/invertBy.js b/node_modules/lodash/fp/invertBy.js
new file mode 100644
index 0000000..63ca97e
--- /dev/null
+++ b/node_modules/lodash/fp/invertBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('invertBy', require('../invertBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/invertObj.js b/node_modules/lodash/fp/invertObj.js
new file mode 100644
index 0000000..f1d842e
--- /dev/null
+++ b/node_modules/lodash/fp/invertObj.js
@@ -0,0 +1 @@
+module.exports = require('./invert');
diff --git a/node_modules/lodash/fp/invoke.js b/node_modules/lodash/fp/invoke.js
new file mode 100644
index 0000000..fcf17f0
--- /dev/null
+++ b/node_modules/lodash/fp/invoke.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('invoke', require('../invoke'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/invokeArgs.js b/node_modules/lodash/fp/invokeArgs.js
new file mode 100644
index 0000000..d3f2953
--- /dev/null
+++ b/node_modules/lodash/fp/invokeArgs.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('invokeArgs', require('../invoke'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/invokeArgsMap.js b/node_modules/lodash/fp/invokeArgsMap.js
new file mode 100644
index 0000000..eaa9f84
--- /dev/null
+++ b/node_modules/lodash/fp/invokeArgsMap.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('invokeArgsMap', require('../invokeMap'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/invokeMap.js b/node_modules/lodash/fp/invokeMap.js
new file mode 100644
index 0000000..6515fd7
--- /dev/null
+++ b/node_modules/lodash/fp/invokeMap.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('invokeMap', require('../invokeMap'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isArguments.js b/node_modules/lodash/fp/isArguments.js
new file mode 100644
index 0000000..1d93c9e
--- /dev/null
+++ b/node_modules/lodash/fp/isArguments.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isArguments', require('../isArguments'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isArray.js b/node_modules/lodash/fp/isArray.js
new file mode 100644
index 0000000..ba7ade8
--- /dev/null
+++ b/node_modules/lodash/fp/isArray.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isArray', require('../isArray'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isArrayBuffer.js b/node_modules/lodash/fp/isArrayBuffer.js
new file mode 100644
index 0000000..5088513
--- /dev/null
+++ b/node_modules/lodash/fp/isArrayBuffer.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isArrayBuffer', require('../isArrayBuffer'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isArrayLike.js b/node_modules/lodash/fp/isArrayLike.js
new file mode 100644
index 0000000..8f1856b
--- /dev/null
+++ b/node_modules/lodash/fp/isArrayLike.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isArrayLike', require('../isArrayLike'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isArrayLikeObject.js b/node_modules/lodash/fp/isArrayLikeObject.js
new file mode 100644
index 0000000..2108498
--- /dev/null
+++ b/node_modules/lodash/fp/isArrayLikeObject.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isArrayLikeObject', require('../isArrayLikeObject'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isBoolean.js b/node_modules/lodash/fp/isBoolean.js
new file mode 100644
index 0000000..9339f75
--- /dev/null
+++ b/node_modules/lodash/fp/isBoolean.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isBoolean', require('../isBoolean'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isBuffer.js b/node_modules/lodash/fp/isBuffer.js
new file mode 100644
index 0000000..e60b123
--- /dev/null
+++ b/node_modules/lodash/fp/isBuffer.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isBuffer', require('../isBuffer'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isDate.js b/node_modules/lodash/fp/isDate.js
new file mode 100644
index 0000000..dc41d08
--- /dev/null
+++ b/node_modules/lodash/fp/isDate.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isDate', require('../isDate'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isElement.js b/node_modules/lodash/fp/isElement.js
new file mode 100644
index 0000000..18ee039
--- /dev/null
+++ b/node_modules/lodash/fp/isElement.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isElement', require('../isElement'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isEmpty.js b/node_modules/lodash/fp/isEmpty.js
new file mode 100644
index 0000000..0f4ae84
--- /dev/null
+++ b/node_modules/lodash/fp/isEmpty.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isEmpty', require('../isEmpty'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isEqual.js b/node_modules/lodash/fp/isEqual.js
new file mode 100644
index 0000000..4138386
--- /dev/null
+++ b/node_modules/lodash/fp/isEqual.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isEqual', require('../isEqual'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isEqualWith.js b/node_modules/lodash/fp/isEqualWith.js
new file mode 100644
index 0000000..029ff5c
--- /dev/null
+++ b/node_modules/lodash/fp/isEqualWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isEqualWith', require('../isEqualWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isError.js b/node_modules/lodash/fp/isError.js
new file mode 100644
index 0000000..3dfd81c
--- /dev/null
+++ b/node_modules/lodash/fp/isError.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isError', require('../isError'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isFinite.js b/node_modules/lodash/fp/isFinite.js
new file mode 100644
index 0000000..0b647b8
--- /dev/null
+++ b/node_modules/lodash/fp/isFinite.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isFinite', require('../isFinite'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isFunction.js b/node_modules/lodash/fp/isFunction.js
new file mode 100644
index 0000000..ff8e5c4
--- /dev/null
+++ b/node_modules/lodash/fp/isFunction.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isFunction', require('../isFunction'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isInteger.js b/node_modules/lodash/fp/isInteger.js
new file mode 100644
index 0000000..67af4ff
--- /dev/null
+++ b/node_modules/lodash/fp/isInteger.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isInteger', require('../isInteger'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isLength.js b/node_modules/lodash/fp/isLength.js
new file mode 100644
index 0000000..fc101c5
--- /dev/null
+++ b/node_modules/lodash/fp/isLength.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isLength', require('../isLength'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isMap.js b/node_modules/lodash/fp/isMap.js
new file mode 100644
index 0000000..a209aa6
--- /dev/null
+++ b/node_modules/lodash/fp/isMap.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isMap', require('../isMap'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isMatch.js b/node_modules/lodash/fp/isMatch.js
new file mode 100644
index 0000000..6264ca1
--- /dev/null
+++ b/node_modules/lodash/fp/isMatch.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isMatch', require('../isMatch'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isMatchWith.js b/node_modules/lodash/fp/isMatchWith.js
new file mode 100644
index 0000000..d95f319
--- /dev/null
+++ b/node_modules/lodash/fp/isMatchWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isMatchWith', require('../isMatchWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isNaN.js b/node_modules/lodash/fp/isNaN.js
new file mode 100644
index 0000000..66a978f
--- /dev/null
+++ b/node_modules/lodash/fp/isNaN.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isNaN', require('../isNaN'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isNative.js b/node_modules/lodash/fp/isNative.js
new file mode 100644
index 0000000..3d775ba
--- /dev/null
+++ b/node_modules/lodash/fp/isNative.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isNative', require('../isNative'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isNil.js b/node_modules/lodash/fp/isNil.js
new file mode 100644
index 0000000..5952c02
--- /dev/null
+++ b/node_modules/lodash/fp/isNil.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isNil', require('../isNil'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isNull.js b/node_modules/lodash/fp/isNull.js
new file mode 100644
index 0000000..f201a35
--- /dev/null
+++ b/node_modules/lodash/fp/isNull.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isNull', require('../isNull'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isNumber.js b/node_modules/lodash/fp/isNumber.js
new file mode 100644
index 0000000..a2b5fa0
--- /dev/null
+++ b/node_modules/lodash/fp/isNumber.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isNumber', require('../isNumber'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isObject.js b/node_modules/lodash/fp/isObject.js
new file mode 100644
index 0000000..231ace0
--- /dev/null
+++ b/node_modules/lodash/fp/isObject.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isObject', require('../isObject'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isObjectLike.js b/node_modules/lodash/fp/isObjectLike.js
new file mode 100644
index 0000000..f16082e
--- /dev/null
+++ b/node_modules/lodash/fp/isObjectLike.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isObjectLike', require('../isObjectLike'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isPlainObject.js b/node_modules/lodash/fp/isPlainObject.js
new file mode 100644
index 0000000..b5bea90
--- /dev/null
+++ b/node_modules/lodash/fp/isPlainObject.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isPlainObject', require('../isPlainObject'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isRegExp.js b/node_modules/lodash/fp/isRegExp.js
new file mode 100644
index 0000000..12a1a3d
--- /dev/null
+++ b/node_modules/lodash/fp/isRegExp.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isRegExp', require('../isRegExp'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isSafeInteger.js b/node_modules/lodash/fp/isSafeInteger.js
new file mode 100644
index 0000000..7230f55
--- /dev/null
+++ b/node_modules/lodash/fp/isSafeInteger.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isSafeInteger', require('../isSafeInteger'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isSet.js b/node_modules/lodash/fp/isSet.js
new file mode 100644
index 0000000..35c01f6
--- /dev/null
+++ b/node_modules/lodash/fp/isSet.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isSet', require('../isSet'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isString.js b/node_modules/lodash/fp/isString.js
new file mode 100644
index 0000000..1fd0679
--- /dev/null
+++ b/node_modules/lodash/fp/isString.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isString', require('../isString'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isSymbol.js b/node_modules/lodash/fp/isSymbol.js
new file mode 100644
index 0000000..3867695
--- /dev/null
+++ b/node_modules/lodash/fp/isSymbol.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isSymbol', require('../isSymbol'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isTypedArray.js b/node_modules/lodash/fp/isTypedArray.js
new file mode 100644
index 0000000..8567953
--- /dev/null
+++ b/node_modules/lodash/fp/isTypedArray.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isTypedArray', require('../isTypedArray'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isUndefined.js b/node_modules/lodash/fp/isUndefined.js
new file mode 100644
index 0000000..ddbca31
--- /dev/null
+++ b/node_modules/lodash/fp/isUndefined.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isUndefined', require('../isUndefined'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isWeakMap.js b/node_modules/lodash/fp/isWeakMap.js
new file mode 100644
index 0000000..ef60c61
--- /dev/null
+++ b/node_modules/lodash/fp/isWeakMap.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isWeakMap', require('../isWeakMap'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/isWeakSet.js b/node_modules/lodash/fp/isWeakSet.js
new file mode 100644
index 0000000..c99bfaa
--- /dev/null
+++ b/node_modules/lodash/fp/isWeakSet.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('isWeakSet', require('../isWeakSet'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/iteratee.js b/node_modules/lodash/fp/iteratee.js
new file mode 100644
index 0000000..9f0f717
--- /dev/null
+++ b/node_modules/lodash/fp/iteratee.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('iteratee', require('../iteratee'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/join.js b/node_modules/lodash/fp/join.js
new file mode 100644
index 0000000..a220e00
--- /dev/null
+++ b/node_modules/lodash/fp/join.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('join', require('../join'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/juxt.js b/node_modules/lodash/fp/juxt.js
new file mode 100644
index 0000000..f71e04e
--- /dev/null
+++ b/node_modules/lodash/fp/juxt.js
@@ -0,0 +1 @@
+module.exports = require('./over');
diff --git a/node_modules/lodash/fp/kebabCase.js b/node_modules/lodash/fp/kebabCase.js
new file mode 100644
index 0000000..60737f1
--- /dev/null
+++ b/node_modules/lodash/fp/kebabCase.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('kebabCase', require('../kebabCase'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/keyBy.js b/node_modules/lodash/fp/keyBy.js
new file mode 100644
index 0000000..9a6a85d
--- /dev/null
+++ b/node_modules/lodash/fp/keyBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('keyBy', require('../keyBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/keys.js b/node_modules/lodash/fp/keys.js
new file mode 100644
index 0000000..e12bb07
--- /dev/null
+++ b/node_modules/lodash/fp/keys.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('keys', require('../keys'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/keysIn.js b/node_modules/lodash/fp/keysIn.js
new file mode 100644
index 0000000..f3eb36a
--- /dev/null
+++ b/node_modules/lodash/fp/keysIn.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('keysIn', require('../keysIn'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/lang.js b/node_modules/lodash/fp/lang.js
new file mode 100644
index 0000000..08cc9c1
--- /dev/null
+++ b/node_modules/lodash/fp/lang.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../lang'));
diff --git a/node_modules/lodash/fp/last.js b/node_modules/lodash/fp/last.js
new file mode 100644
index 0000000..0f71699
--- /dev/null
+++ b/node_modules/lodash/fp/last.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('last', require('../last'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/lastIndexOf.js b/node_modules/lodash/fp/lastIndexOf.js
new file mode 100644
index 0000000..ddf39c3
--- /dev/null
+++ b/node_modules/lodash/fp/lastIndexOf.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('lastIndexOf', require('../lastIndexOf'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/lastIndexOfFrom.js b/node_modules/lodash/fp/lastIndexOfFrom.js
new file mode 100644
index 0000000..1ff6a0b
--- /dev/null
+++ b/node_modules/lodash/fp/lastIndexOfFrom.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('lastIndexOfFrom', require('../lastIndexOf'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/lowerCase.js b/node_modules/lodash/fp/lowerCase.js
new file mode 100644
index 0000000..ea64bc1
--- /dev/null
+++ b/node_modules/lodash/fp/lowerCase.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('lowerCase', require('../lowerCase'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/lowerFirst.js b/node_modules/lodash/fp/lowerFirst.js
new file mode 100644
index 0000000..539720a
--- /dev/null
+++ b/node_modules/lodash/fp/lowerFirst.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('lowerFirst', require('../lowerFirst'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/lt.js b/node_modules/lodash/fp/lt.js
new file mode 100644
index 0000000..a31d21e
--- /dev/null
+++ b/node_modules/lodash/fp/lt.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('lt', require('../lt'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/lte.js b/node_modules/lodash/fp/lte.js
new file mode 100644
index 0000000..d795d10
--- /dev/null
+++ b/node_modules/lodash/fp/lte.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('lte', require('../lte'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/map.js b/node_modules/lodash/fp/map.js
new file mode 100644
index 0000000..cf98794
--- /dev/null
+++ b/node_modules/lodash/fp/map.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('map', require('../map'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/mapKeys.js b/node_modules/lodash/fp/mapKeys.js
new file mode 100644
index 0000000..1684587
--- /dev/null
+++ b/node_modules/lodash/fp/mapKeys.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('mapKeys', require('../mapKeys'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/mapValues.js b/node_modules/lodash/fp/mapValues.js
new file mode 100644
index 0000000..4004972
--- /dev/null
+++ b/node_modules/lodash/fp/mapValues.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('mapValues', require('../mapValues'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/matches.js b/node_modules/lodash/fp/matches.js
new file mode 100644
index 0000000..29d1e1e
--- /dev/null
+++ b/node_modules/lodash/fp/matches.js
@@ -0,0 +1 @@
+module.exports = require('./isMatch');
diff --git a/node_modules/lodash/fp/matchesProperty.js b/node_modules/lodash/fp/matchesProperty.js
new file mode 100644
index 0000000..4575bd2
--- /dev/null
+++ b/node_modules/lodash/fp/matchesProperty.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('matchesProperty', require('../matchesProperty'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/math.js b/node_modules/lodash/fp/math.js
new file mode 100644
index 0000000..e8f50f7
--- /dev/null
+++ b/node_modules/lodash/fp/math.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../math'));
diff --git a/node_modules/lodash/fp/max.js b/node_modules/lodash/fp/max.js
new file mode 100644
index 0000000..a66acac
--- /dev/null
+++ b/node_modules/lodash/fp/max.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('max', require('../max'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/maxBy.js b/node_modules/lodash/fp/maxBy.js
new file mode 100644
index 0000000..d083fd6
--- /dev/null
+++ b/node_modules/lodash/fp/maxBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('maxBy', require('../maxBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/mean.js b/node_modules/lodash/fp/mean.js
new file mode 100644
index 0000000..3117246
--- /dev/null
+++ b/node_modules/lodash/fp/mean.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('mean', require('../mean'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/meanBy.js b/node_modules/lodash/fp/meanBy.js
new file mode 100644
index 0000000..556f25e
--- /dev/null
+++ b/node_modules/lodash/fp/meanBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('meanBy', require('../meanBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/memoize.js b/node_modules/lodash/fp/memoize.js
new file mode 100644
index 0000000..638eec6
--- /dev/null
+++ b/node_modules/lodash/fp/memoize.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('memoize', require('../memoize'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/merge.js b/node_modules/lodash/fp/merge.js
new file mode 100644
index 0000000..ac66add
--- /dev/null
+++ b/node_modules/lodash/fp/merge.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('merge', require('../merge'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/mergeAll.js b/node_modules/lodash/fp/mergeAll.js
new file mode 100644
index 0000000..a3674d6
--- /dev/null
+++ b/node_modules/lodash/fp/mergeAll.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('mergeAll', require('../merge'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/mergeAllWith.js b/node_modules/lodash/fp/mergeAllWith.js
new file mode 100644
index 0000000..4bd4206
--- /dev/null
+++ b/node_modules/lodash/fp/mergeAllWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('mergeAllWith', require('../mergeWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/mergeWith.js b/node_modules/lodash/fp/mergeWith.js
new file mode 100644
index 0000000..00d44d5
--- /dev/null
+++ b/node_modules/lodash/fp/mergeWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('mergeWith', require('../mergeWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/method.js b/node_modules/lodash/fp/method.js
new file mode 100644
index 0000000..f4060c6
--- /dev/null
+++ b/node_modules/lodash/fp/method.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('method', require('../method'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/methodOf.js b/node_modules/lodash/fp/methodOf.js
new file mode 100644
index 0000000..6139905
--- /dev/null
+++ b/node_modules/lodash/fp/methodOf.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('methodOf', require('../methodOf'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/min.js b/node_modules/lodash/fp/min.js
new file mode 100644
index 0000000..d12c6b4
--- /dev/null
+++ b/node_modules/lodash/fp/min.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('min', require('../min'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/minBy.js b/node_modules/lodash/fp/minBy.js
new file mode 100644
index 0000000..fdb9e24
--- /dev/null
+++ b/node_modules/lodash/fp/minBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('minBy', require('../minBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/mixin.js b/node_modules/lodash/fp/mixin.js
new file mode 100644
index 0000000..332e6fb
--- /dev/null
+++ b/node_modules/lodash/fp/mixin.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('mixin', require('../mixin'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/multiply.js b/node_modules/lodash/fp/multiply.js
new file mode 100644
index 0000000..4dcf0b0
--- /dev/null
+++ b/node_modules/lodash/fp/multiply.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('multiply', require('../multiply'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/nAry.js b/node_modules/lodash/fp/nAry.js
new file mode 100644
index 0000000..f262a76
--- /dev/null
+++ b/node_modules/lodash/fp/nAry.js
@@ -0,0 +1 @@
+module.exports = require('./ary');
diff --git a/node_modules/lodash/fp/negate.js b/node_modules/lodash/fp/negate.js
new file mode 100644
index 0000000..8b6dc7c
--- /dev/null
+++ b/node_modules/lodash/fp/negate.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('negate', require('../negate'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/next.js b/node_modules/lodash/fp/next.js
new file mode 100644
index 0000000..140155e
--- /dev/null
+++ b/node_modules/lodash/fp/next.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('next', require('../next'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/noop.js b/node_modules/lodash/fp/noop.js
new file mode 100644
index 0000000..b9e32cc
--- /dev/null
+++ b/node_modules/lodash/fp/noop.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('noop', require('../noop'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/now.js b/node_modules/lodash/fp/now.js
new file mode 100644
index 0000000..6de2068
--- /dev/null
+++ b/node_modules/lodash/fp/now.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('now', require('../now'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/nth.js b/node_modules/lodash/fp/nth.js
new file mode 100644
index 0000000..da4fda7
--- /dev/null
+++ b/node_modules/lodash/fp/nth.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('nth', require('../nth'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/nthArg.js b/node_modules/lodash/fp/nthArg.js
new file mode 100644
index 0000000..fce3165
--- /dev/null
+++ b/node_modules/lodash/fp/nthArg.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('nthArg', require('../nthArg'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/number.js b/node_modules/lodash/fp/number.js
new file mode 100644
index 0000000..5c10b88
--- /dev/null
+++ b/node_modules/lodash/fp/number.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../number'));
diff --git a/node_modules/lodash/fp/object.js b/node_modules/lodash/fp/object.js
new file mode 100644
index 0000000..ae39a13
--- /dev/null
+++ b/node_modules/lodash/fp/object.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../object'));
diff --git a/node_modules/lodash/fp/omit.js b/node_modules/lodash/fp/omit.js
new file mode 100644
index 0000000..fd68529
--- /dev/null
+++ b/node_modules/lodash/fp/omit.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('omit', require('../omit'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/omitAll.js b/node_modules/lodash/fp/omitAll.js
new file mode 100644
index 0000000..144cf4b
--- /dev/null
+++ b/node_modules/lodash/fp/omitAll.js
@@ -0,0 +1 @@
+module.exports = require('./omit');
diff --git a/node_modules/lodash/fp/omitBy.js b/node_modules/lodash/fp/omitBy.js
new file mode 100644
index 0000000..90df738
--- /dev/null
+++ b/node_modules/lodash/fp/omitBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('omitBy', require('../omitBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/once.js b/node_modules/lodash/fp/once.js
new file mode 100644
index 0000000..f8f0a5c
--- /dev/null
+++ b/node_modules/lodash/fp/once.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('once', require('../once'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/orderBy.js b/node_modules/lodash/fp/orderBy.js
new file mode 100644
index 0000000..848e210
--- /dev/null
+++ b/node_modules/lodash/fp/orderBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('orderBy', require('../orderBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/over.js b/node_modules/lodash/fp/over.js
new file mode 100644
index 0000000..01eba7b
--- /dev/null
+++ b/node_modules/lodash/fp/over.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('over', require('../over'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/overArgs.js b/node_modules/lodash/fp/overArgs.js
new file mode 100644
index 0000000..738556f
--- /dev/null
+++ b/node_modules/lodash/fp/overArgs.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('overArgs', require('../overArgs'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/overEvery.js b/node_modules/lodash/fp/overEvery.js
new file mode 100644
index 0000000..9f5a032
--- /dev/null
+++ b/node_modules/lodash/fp/overEvery.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('overEvery', require('../overEvery'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/overSome.js b/node_modules/lodash/fp/overSome.js
new file mode 100644
index 0000000..15939d5
--- /dev/null
+++ b/node_modules/lodash/fp/overSome.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('overSome', require('../overSome'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/pad.js b/node_modules/lodash/fp/pad.js
new file mode 100644
index 0000000..f1dea4a
--- /dev/null
+++ b/node_modules/lodash/fp/pad.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('pad', require('../pad'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/padChars.js b/node_modules/lodash/fp/padChars.js
new file mode 100644
index 0000000..d6e0804
--- /dev/null
+++ b/node_modules/lodash/fp/padChars.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('padChars', require('../pad'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/padCharsEnd.js b/node_modules/lodash/fp/padCharsEnd.js
new file mode 100644
index 0000000..d4ab79a
--- /dev/null
+++ b/node_modules/lodash/fp/padCharsEnd.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('padCharsEnd', require('../padEnd'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/padCharsStart.js b/node_modules/lodash/fp/padCharsStart.js
new file mode 100644
index 0000000..a08a300
--- /dev/null
+++ b/node_modules/lodash/fp/padCharsStart.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('padCharsStart', require('../padStart'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/padEnd.js b/node_modules/lodash/fp/padEnd.js
new file mode 100644
index 0000000..a8522ec
--- /dev/null
+++ b/node_modules/lodash/fp/padEnd.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('padEnd', require('../padEnd'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/padStart.js b/node_modules/lodash/fp/padStart.js
new file mode 100644
index 0000000..f4ca79d
--- /dev/null
+++ b/node_modules/lodash/fp/padStart.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('padStart', require('../padStart'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/parseInt.js b/node_modules/lodash/fp/parseInt.js
new file mode 100644
index 0000000..27314cc
--- /dev/null
+++ b/node_modules/lodash/fp/parseInt.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('parseInt', require('../parseInt'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/partial.js b/node_modules/lodash/fp/partial.js
new file mode 100644
index 0000000..5d46015
--- /dev/null
+++ b/node_modules/lodash/fp/partial.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('partial', require('../partial'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/partialRight.js b/node_modules/lodash/fp/partialRight.js
new file mode 100644
index 0000000..7f05fed
--- /dev/null
+++ b/node_modules/lodash/fp/partialRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('partialRight', require('../partialRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/partition.js b/node_modules/lodash/fp/partition.js
new file mode 100644
index 0000000..2ebcacc
--- /dev/null
+++ b/node_modules/lodash/fp/partition.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('partition', require('../partition'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/path.js b/node_modules/lodash/fp/path.js
new file mode 100644
index 0000000..b29cfb2
--- /dev/null
+++ b/node_modules/lodash/fp/path.js
@@ -0,0 +1 @@
+module.exports = require('./get');
diff --git a/node_modules/lodash/fp/pathEq.js b/node_modules/lodash/fp/pathEq.js
new file mode 100644
index 0000000..36c027a
--- /dev/null
+++ b/node_modules/lodash/fp/pathEq.js
@@ -0,0 +1 @@
+module.exports = require('./matchesProperty');
diff --git a/node_modules/lodash/fp/pathOr.js b/node_modules/lodash/fp/pathOr.js
new file mode 100644
index 0000000..4ab5820
--- /dev/null
+++ b/node_modules/lodash/fp/pathOr.js
@@ -0,0 +1 @@
+module.exports = require('./getOr');
diff --git a/node_modules/lodash/fp/paths.js b/node_modules/lodash/fp/paths.js
new file mode 100644
index 0000000..1eb7950
--- /dev/null
+++ b/node_modules/lodash/fp/paths.js
@@ -0,0 +1 @@
+module.exports = require('./at');
diff --git a/node_modules/lodash/fp/pick.js b/node_modules/lodash/fp/pick.js
new file mode 100644
index 0000000..197393d
--- /dev/null
+++ b/node_modules/lodash/fp/pick.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('pick', require('../pick'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/pickAll.js b/node_modules/lodash/fp/pickAll.js
new file mode 100644
index 0000000..a8ecd46
--- /dev/null
+++ b/node_modules/lodash/fp/pickAll.js
@@ -0,0 +1 @@
+module.exports = require('./pick');
diff --git a/node_modules/lodash/fp/pickBy.js b/node_modules/lodash/fp/pickBy.js
new file mode 100644
index 0000000..d832d16
--- /dev/null
+++ b/node_modules/lodash/fp/pickBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('pickBy', require('../pickBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/pipe.js b/node_modules/lodash/fp/pipe.js
new file mode 100644
index 0000000..b2e1e2c
--- /dev/null
+++ b/node_modules/lodash/fp/pipe.js
@@ -0,0 +1 @@
+module.exports = require('./flow');
diff --git a/node_modules/lodash/fp/placeholder.js b/node_modules/lodash/fp/placeholder.js
new file mode 100644
index 0000000..1ce1739
--- /dev/null
+++ b/node_modules/lodash/fp/placeholder.js
@@ -0,0 +1,6 @@
+/**
+ * The default argument placeholder value for methods.
+ *
+ * @type {Object}
+ */
+module.exports = {};
diff --git a/node_modules/lodash/fp/plant.js b/node_modules/lodash/fp/plant.js
new file mode 100644
index 0000000..eca8f32
--- /dev/null
+++ b/node_modules/lodash/fp/plant.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('plant', require('../plant'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/pluck.js b/node_modules/lodash/fp/pluck.js
new file mode 100644
index 0000000..0d1e1ab
--- /dev/null
+++ b/node_modules/lodash/fp/pluck.js
@@ -0,0 +1 @@
+module.exports = require('./map');
diff --git a/node_modules/lodash/fp/prop.js b/node_modules/lodash/fp/prop.js
new file mode 100644
index 0000000..b29cfb2
--- /dev/null
+++ b/node_modules/lodash/fp/prop.js
@@ -0,0 +1 @@
+module.exports = require('./get');
diff --git a/node_modules/lodash/fp/propEq.js b/node_modules/lodash/fp/propEq.js
new file mode 100644
index 0000000..36c027a
--- /dev/null
+++ b/node_modules/lodash/fp/propEq.js
@@ -0,0 +1 @@
+module.exports = require('./matchesProperty');
diff --git a/node_modules/lodash/fp/propOr.js b/node_modules/lodash/fp/propOr.js
new file mode 100644
index 0000000..4ab5820
--- /dev/null
+++ b/node_modules/lodash/fp/propOr.js
@@ -0,0 +1 @@
+module.exports = require('./getOr');
diff --git a/node_modules/lodash/fp/property.js b/node_modules/lodash/fp/property.js
new file mode 100644
index 0000000..b29cfb2
--- /dev/null
+++ b/node_modules/lodash/fp/property.js
@@ -0,0 +1 @@
+module.exports = require('./get');
diff --git a/node_modules/lodash/fp/propertyOf.js b/node_modules/lodash/fp/propertyOf.js
new file mode 100644
index 0000000..f6273ee
--- /dev/null
+++ b/node_modules/lodash/fp/propertyOf.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('propertyOf', require('../get'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/props.js b/node_modules/lodash/fp/props.js
new file mode 100644
index 0000000..1eb7950
--- /dev/null
+++ b/node_modules/lodash/fp/props.js
@@ -0,0 +1 @@
+module.exports = require('./at');
diff --git a/node_modules/lodash/fp/pull.js b/node_modules/lodash/fp/pull.js
new file mode 100644
index 0000000..8d7084f
--- /dev/null
+++ b/node_modules/lodash/fp/pull.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('pull', require('../pull'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/pullAll.js b/node_modules/lodash/fp/pullAll.js
new file mode 100644
index 0000000..98d5c9a
--- /dev/null
+++ b/node_modules/lodash/fp/pullAll.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('pullAll', require('../pullAll'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/pullAllBy.js b/node_modules/lodash/fp/pullAllBy.js
new file mode 100644
index 0000000..876bc3b
--- /dev/null
+++ b/node_modules/lodash/fp/pullAllBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('pullAllBy', require('../pullAllBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/pullAllWith.js b/node_modules/lodash/fp/pullAllWith.js
new file mode 100644
index 0000000..f71ba4d
--- /dev/null
+++ b/node_modules/lodash/fp/pullAllWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('pullAllWith', require('../pullAllWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/pullAt.js b/node_modules/lodash/fp/pullAt.js
new file mode 100644
index 0000000..e8b3bb6
--- /dev/null
+++ b/node_modules/lodash/fp/pullAt.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('pullAt', require('../pullAt'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/random.js b/node_modules/lodash/fp/random.js
new file mode 100644
index 0000000..99d852e
--- /dev/null
+++ b/node_modules/lodash/fp/random.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('random', require('../random'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/range.js b/node_modules/lodash/fp/range.js
new file mode 100644
index 0000000..a6bb591
--- /dev/null
+++ b/node_modules/lodash/fp/range.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('range', require('../range'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/rangeRight.js b/node_modules/lodash/fp/rangeRight.js
new file mode 100644
index 0000000..fdb712f
--- /dev/null
+++ b/node_modules/lodash/fp/rangeRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('rangeRight', require('../rangeRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/rangeStep.js b/node_modules/lodash/fp/rangeStep.js
new file mode 100644
index 0000000..d72dfc2
--- /dev/null
+++ b/node_modules/lodash/fp/rangeStep.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('rangeStep', require('../range'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/rangeStepRight.js b/node_modules/lodash/fp/rangeStepRight.js
new file mode 100644
index 0000000..8b2a67b
--- /dev/null
+++ b/node_modules/lodash/fp/rangeStepRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('rangeStepRight', require('../rangeRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/rearg.js b/node_modules/lodash/fp/rearg.js
new file mode 100644
index 0000000..678e02a
--- /dev/null
+++ b/node_modules/lodash/fp/rearg.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('rearg', require('../rearg'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/reduce.js b/node_modules/lodash/fp/reduce.js
new file mode 100644
index 0000000..4cef0a0
--- /dev/null
+++ b/node_modules/lodash/fp/reduce.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('reduce', require('../reduce'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/reduceRight.js b/node_modules/lodash/fp/reduceRight.js
new file mode 100644
index 0000000..caf5bb5
--- /dev/null
+++ b/node_modules/lodash/fp/reduceRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('reduceRight', require('../reduceRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/reject.js b/node_modules/lodash/fp/reject.js
new file mode 100644
index 0000000..c163273
--- /dev/null
+++ b/node_modules/lodash/fp/reject.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('reject', require('../reject'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/remove.js b/node_modules/lodash/fp/remove.js
new file mode 100644
index 0000000..e9d1327
--- /dev/null
+++ b/node_modules/lodash/fp/remove.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('remove', require('../remove'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/repeat.js b/node_modules/lodash/fp/repeat.js
new file mode 100644
index 0000000..08470f2
--- /dev/null
+++ b/node_modules/lodash/fp/repeat.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('repeat', require('../repeat'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/replace.js b/node_modules/lodash/fp/replace.js
new file mode 100644
index 0000000..2227db6
--- /dev/null
+++ b/node_modules/lodash/fp/replace.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('replace', require('../replace'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/rest.js b/node_modules/lodash/fp/rest.js
new file mode 100644
index 0000000..c1f3d64
--- /dev/null
+++ b/node_modules/lodash/fp/rest.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('rest', require('../rest'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/restFrom.js b/node_modules/lodash/fp/restFrom.js
new file mode 100644
index 0000000..714e42b
--- /dev/null
+++ b/node_modules/lodash/fp/restFrom.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('restFrom', require('../rest'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/result.js b/node_modules/lodash/fp/result.js
new file mode 100644
index 0000000..f86ce07
--- /dev/null
+++ b/node_modules/lodash/fp/result.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('result', require('../result'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/reverse.js b/node_modules/lodash/fp/reverse.js
new file mode 100644
index 0000000..07c9f5e
--- /dev/null
+++ b/node_modules/lodash/fp/reverse.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('reverse', require('../reverse'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/round.js b/node_modules/lodash/fp/round.js
new file mode 100644
index 0000000..4c0e5c8
--- /dev/null
+++ b/node_modules/lodash/fp/round.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('round', require('../round'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sample.js b/node_modules/lodash/fp/sample.js
new file mode 100644
index 0000000..6bea125
--- /dev/null
+++ b/node_modules/lodash/fp/sample.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sample', require('../sample'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sampleSize.js b/node_modules/lodash/fp/sampleSize.js
new file mode 100644
index 0000000..359ed6f
--- /dev/null
+++ b/node_modules/lodash/fp/sampleSize.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sampleSize', require('../sampleSize'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/seq.js b/node_modules/lodash/fp/seq.js
new file mode 100644
index 0000000..d8f42b0
--- /dev/null
+++ b/node_modules/lodash/fp/seq.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../seq'));
diff --git a/node_modules/lodash/fp/set.js b/node_modules/lodash/fp/set.js
new file mode 100644
index 0000000..0b56a56
--- /dev/null
+++ b/node_modules/lodash/fp/set.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('set', require('../set'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/setWith.js b/node_modules/lodash/fp/setWith.js
new file mode 100644
index 0000000..0b58495
--- /dev/null
+++ b/node_modules/lodash/fp/setWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('setWith', require('../setWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/shuffle.js b/node_modules/lodash/fp/shuffle.js
new file mode 100644
index 0000000..aa3a1ca
--- /dev/null
+++ b/node_modules/lodash/fp/shuffle.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('shuffle', require('../shuffle'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/size.js b/node_modules/lodash/fp/size.js
new file mode 100644
index 0000000..7490136
--- /dev/null
+++ b/node_modules/lodash/fp/size.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('size', require('../size'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/slice.js b/node_modules/lodash/fp/slice.js
new file mode 100644
index 0000000..15945d3
--- /dev/null
+++ b/node_modules/lodash/fp/slice.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('slice', require('../slice'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/snakeCase.js b/node_modules/lodash/fp/snakeCase.js
new file mode 100644
index 0000000..a0ff780
--- /dev/null
+++ b/node_modules/lodash/fp/snakeCase.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('snakeCase', require('../snakeCase'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/some.js b/node_modules/lodash/fp/some.js
new file mode 100644
index 0000000..a4fa2d0
--- /dev/null
+++ b/node_modules/lodash/fp/some.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('some', require('../some'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sortBy.js b/node_modules/lodash/fp/sortBy.js
new file mode 100644
index 0000000..e0790ad
--- /dev/null
+++ b/node_modules/lodash/fp/sortBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sortBy', require('../sortBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sortedIndex.js b/node_modules/lodash/fp/sortedIndex.js
new file mode 100644
index 0000000..364a054
--- /dev/null
+++ b/node_modules/lodash/fp/sortedIndex.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sortedIndex', require('../sortedIndex'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sortedIndexBy.js b/node_modules/lodash/fp/sortedIndexBy.js
new file mode 100644
index 0000000..9593dbd
--- /dev/null
+++ b/node_modules/lodash/fp/sortedIndexBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sortedIndexBy', require('../sortedIndexBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sortedIndexOf.js b/node_modules/lodash/fp/sortedIndexOf.js
new file mode 100644
index 0000000..c9084ca
--- /dev/null
+++ b/node_modules/lodash/fp/sortedIndexOf.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sortedIndexOf', require('../sortedIndexOf'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sortedLastIndex.js b/node_modules/lodash/fp/sortedLastIndex.js
new file mode 100644
index 0000000..47fe241
--- /dev/null
+++ b/node_modules/lodash/fp/sortedLastIndex.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sortedLastIndex', require('../sortedLastIndex'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sortedLastIndexBy.js b/node_modules/lodash/fp/sortedLastIndexBy.js
new file mode 100644
index 0000000..0f9a347
--- /dev/null
+++ b/node_modules/lodash/fp/sortedLastIndexBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sortedLastIndexBy', require('../sortedLastIndexBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sortedLastIndexOf.js b/node_modules/lodash/fp/sortedLastIndexOf.js
new file mode 100644
index 0000000..0d4d932
--- /dev/null
+++ b/node_modules/lodash/fp/sortedLastIndexOf.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sortedLastIndexOf', require('../sortedLastIndexOf'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sortedUniq.js b/node_modules/lodash/fp/sortedUniq.js
new file mode 100644
index 0000000..882d283
--- /dev/null
+++ b/node_modules/lodash/fp/sortedUniq.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sortedUniq', require('../sortedUniq'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sortedUniqBy.js b/node_modules/lodash/fp/sortedUniqBy.js
new file mode 100644
index 0000000..033db91
--- /dev/null
+++ b/node_modules/lodash/fp/sortedUniqBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sortedUniqBy', require('../sortedUniqBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/split.js b/node_modules/lodash/fp/split.js
new file mode 100644
index 0000000..14de1a7
--- /dev/null
+++ b/node_modules/lodash/fp/split.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('split', require('../split'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/spread.js b/node_modules/lodash/fp/spread.js
new file mode 100644
index 0000000..2d11b70
--- /dev/null
+++ b/node_modules/lodash/fp/spread.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('spread', require('../spread'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/spreadFrom.js b/node_modules/lodash/fp/spreadFrom.js
new file mode 100644
index 0000000..0b630df
--- /dev/null
+++ b/node_modules/lodash/fp/spreadFrom.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('spreadFrom', require('../spread'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/startCase.js b/node_modules/lodash/fp/startCase.js
new file mode 100644
index 0000000..ada98c9
--- /dev/null
+++ b/node_modules/lodash/fp/startCase.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('startCase', require('../startCase'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/startsWith.js b/node_modules/lodash/fp/startsWith.js
new file mode 100644
index 0000000..985e2f2
--- /dev/null
+++ b/node_modules/lodash/fp/startsWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('startsWith', require('../startsWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/string.js b/node_modules/lodash/fp/string.js
new file mode 100644
index 0000000..773b037
--- /dev/null
+++ b/node_modules/lodash/fp/string.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../string'));
diff --git a/node_modules/lodash/fp/stubArray.js b/node_modules/lodash/fp/stubArray.js
new file mode 100644
index 0000000..cd604cb
--- /dev/null
+++ b/node_modules/lodash/fp/stubArray.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('stubArray', require('../stubArray'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/stubFalse.js b/node_modules/lodash/fp/stubFalse.js
new file mode 100644
index 0000000..3296664
--- /dev/null
+++ b/node_modules/lodash/fp/stubFalse.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('stubFalse', require('../stubFalse'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/stubObject.js b/node_modules/lodash/fp/stubObject.js
new file mode 100644
index 0000000..c6c8ec4
--- /dev/null
+++ b/node_modules/lodash/fp/stubObject.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('stubObject', require('../stubObject'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/stubString.js b/node_modules/lodash/fp/stubString.js
new file mode 100644
index 0000000..701051e
--- /dev/null
+++ b/node_modules/lodash/fp/stubString.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('stubString', require('../stubString'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/stubTrue.js b/node_modules/lodash/fp/stubTrue.js
new file mode 100644
index 0000000..9249082
--- /dev/null
+++ b/node_modules/lodash/fp/stubTrue.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('stubTrue', require('../stubTrue'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/subtract.js b/node_modules/lodash/fp/subtract.js
new file mode 100644
index 0000000..d32b16d
--- /dev/null
+++ b/node_modules/lodash/fp/subtract.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('subtract', require('../subtract'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sum.js b/node_modules/lodash/fp/sum.js
new file mode 100644
index 0000000..5cce12b
--- /dev/null
+++ b/node_modules/lodash/fp/sum.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sum', require('../sum'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/sumBy.js b/node_modules/lodash/fp/sumBy.js
new file mode 100644
index 0000000..c882656
--- /dev/null
+++ b/node_modules/lodash/fp/sumBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('sumBy', require('../sumBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/symmetricDifference.js b/node_modules/lodash/fp/symmetricDifference.js
new file mode 100644
index 0000000..78c16ad
--- /dev/null
+++ b/node_modules/lodash/fp/symmetricDifference.js
@@ -0,0 +1 @@
+module.exports = require('./xor');
diff --git a/node_modules/lodash/fp/symmetricDifferenceBy.js b/node_modules/lodash/fp/symmetricDifferenceBy.js
new file mode 100644
index 0000000..298fc7f
--- /dev/null
+++ b/node_modules/lodash/fp/symmetricDifferenceBy.js
@@ -0,0 +1 @@
+module.exports = require('./xorBy');
diff --git a/node_modules/lodash/fp/symmetricDifferenceWith.js b/node_modules/lodash/fp/symmetricDifferenceWith.js
new file mode 100644
index 0000000..70bc6fa
--- /dev/null
+++ b/node_modules/lodash/fp/symmetricDifferenceWith.js
@@ -0,0 +1 @@
+module.exports = require('./xorWith');
diff --git a/node_modules/lodash/fp/tail.js b/node_modules/lodash/fp/tail.js
new file mode 100644
index 0000000..f122f0a
--- /dev/null
+++ b/node_modules/lodash/fp/tail.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('tail', require('../tail'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/take.js b/node_modules/lodash/fp/take.js
new file mode 100644
index 0000000..9af98a7
--- /dev/null
+++ b/node_modules/lodash/fp/take.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('take', require('../take'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/takeLast.js b/node_modules/lodash/fp/takeLast.js
new file mode 100644
index 0000000..e98c84a
--- /dev/null
+++ b/node_modules/lodash/fp/takeLast.js
@@ -0,0 +1 @@
+module.exports = require('./takeRight');
diff --git a/node_modules/lodash/fp/takeLastWhile.js b/node_modules/lodash/fp/takeLastWhile.js
new file mode 100644
index 0000000..5367968
--- /dev/null
+++ b/node_modules/lodash/fp/takeLastWhile.js
@@ -0,0 +1 @@
+module.exports = require('./takeRightWhile');
diff --git a/node_modules/lodash/fp/takeRight.js b/node_modules/lodash/fp/takeRight.js
new file mode 100644
index 0000000..b82950a
--- /dev/null
+++ b/node_modules/lodash/fp/takeRight.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('takeRight', require('../takeRight'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/takeRightWhile.js b/node_modules/lodash/fp/takeRightWhile.js
new file mode 100644
index 0000000..8ffb0a2
--- /dev/null
+++ b/node_modules/lodash/fp/takeRightWhile.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('takeRightWhile', require('../takeRightWhile'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/takeWhile.js b/node_modules/lodash/fp/takeWhile.js
new file mode 100644
index 0000000..2813664
--- /dev/null
+++ b/node_modules/lodash/fp/takeWhile.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('takeWhile', require('../takeWhile'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/tap.js b/node_modules/lodash/fp/tap.js
new file mode 100644
index 0000000..d33ad6e
--- /dev/null
+++ b/node_modules/lodash/fp/tap.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('tap', require('../tap'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/template.js b/node_modules/lodash/fp/template.js
new file mode 100644
index 0000000..74857e1
--- /dev/null
+++ b/node_modules/lodash/fp/template.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('template', require('../template'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/templateSettings.js b/node_modules/lodash/fp/templateSettings.js
new file mode 100644
index 0000000..7bcc0a8
--- /dev/null
+++ b/node_modules/lodash/fp/templateSettings.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('templateSettings', require('../templateSettings'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/throttle.js b/node_modules/lodash/fp/throttle.js
new file mode 100644
index 0000000..77fff14
--- /dev/null
+++ b/node_modules/lodash/fp/throttle.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('throttle', require('../throttle'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/thru.js b/node_modules/lodash/fp/thru.js
new file mode 100644
index 0000000..d42b3b1
--- /dev/null
+++ b/node_modules/lodash/fp/thru.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('thru', require('../thru'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/times.js b/node_modules/lodash/fp/times.js
new file mode 100644
index 0000000..0dab06d
--- /dev/null
+++ b/node_modules/lodash/fp/times.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('times', require('../times'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toArray.js b/node_modules/lodash/fp/toArray.js
new file mode 100644
index 0000000..f0c360a
--- /dev/null
+++ b/node_modules/lodash/fp/toArray.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toArray', require('../toArray'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toFinite.js b/node_modules/lodash/fp/toFinite.js
new file mode 100644
index 0000000..3a47687
--- /dev/null
+++ b/node_modules/lodash/fp/toFinite.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toFinite', require('../toFinite'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toInteger.js b/node_modules/lodash/fp/toInteger.js
new file mode 100644
index 0000000..e0af6a7
--- /dev/null
+++ b/node_modules/lodash/fp/toInteger.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toInteger', require('../toInteger'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toIterator.js b/node_modules/lodash/fp/toIterator.js
new file mode 100644
index 0000000..65e6baa
--- /dev/null
+++ b/node_modules/lodash/fp/toIterator.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toIterator', require('../toIterator'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toJSON.js b/node_modules/lodash/fp/toJSON.js
new file mode 100644
index 0000000..2d718d0
--- /dev/null
+++ b/node_modules/lodash/fp/toJSON.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toJSON', require('../toJSON'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toLength.js b/node_modules/lodash/fp/toLength.js
new file mode 100644
index 0000000..b97cdd9
--- /dev/null
+++ b/node_modules/lodash/fp/toLength.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toLength', require('../toLength'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toLower.js b/node_modules/lodash/fp/toLower.js
new file mode 100644
index 0000000..616ef36
--- /dev/null
+++ b/node_modules/lodash/fp/toLower.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toLower', require('../toLower'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toNumber.js b/node_modules/lodash/fp/toNumber.js
new file mode 100644
index 0000000..d0c6f4d
--- /dev/null
+++ b/node_modules/lodash/fp/toNumber.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toNumber', require('../toNumber'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toPairs.js b/node_modules/lodash/fp/toPairs.js
new file mode 100644
index 0000000..af78378
--- /dev/null
+++ b/node_modules/lodash/fp/toPairs.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toPairs', require('../toPairs'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toPairsIn.js b/node_modules/lodash/fp/toPairsIn.js
new file mode 100644
index 0000000..66504ab
--- /dev/null
+++ b/node_modules/lodash/fp/toPairsIn.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toPairsIn', require('../toPairsIn'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toPath.js b/node_modules/lodash/fp/toPath.js
new file mode 100644
index 0000000..b4d5e50
--- /dev/null
+++ b/node_modules/lodash/fp/toPath.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toPath', require('../toPath'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toPlainObject.js b/node_modules/lodash/fp/toPlainObject.js
new file mode 100644
index 0000000..278bb86
--- /dev/null
+++ b/node_modules/lodash/fp/toPlainObject.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toPlainObject', require('../toPlainObject'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toSafeInteger.js b/node_modules/lodash/fp/toSafeInteger.js
new file mode 100644
index 0000000..367a26f
--- /dev/null
+++ b/node_modules/lodash/fp/toSafeInteger.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toSafeInteger', require('../toSafeInteger'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toString.js b/node_modules/lodash/fp/toString.js
new file mode 100644
index 0000000..cec4f8e
--- /dev/null
+++ b/node_modules/lodash/fp/toString.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toString', require('../toString'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/toUpper.js b/node_modules/lodash/fp/toUpper.js
new file mode 100644
index 0000000..54f9a56
--- /dev/null
+++ b/node_modules/lodash/fp/toUpper.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('toUpper', require('../toUpper'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/transform.js b/node_modules/lodash/fp/transform.js
new file mode 100644
index 0000000..759d088
--- /dev/null
+++ b/node_modules/lodash/fp/transform.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('transform', require('../transform'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/trim.js b/node_modules/lodash/fp/trim.js
new file mode 100644
index 0000000..e6319a7
--- /dev/null
+++ b/node_modules/lodash/fp/trim.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('trim', require('../trim'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/trimChars.js b/node_modules/lodash/fp/trimChars.js
new file mode 100644
index 0000000..c9294de
--- /dev/null
+++ b/node_modules/lodash/fp/trimChars.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('trimChars', require('../trim'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/trimCharsEnd.js b/node_modules/lodash/fp/trimCharsEnd.js
new file mode 100644
index 0000000..284bc2f
--- /dev/null
+++ b/node_modules/lodash/fp/trimCharsEnd.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('trimCharsEnd', require('../trimEnd'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/trimCharsStart.js b/node_modules/lodash/fp/trimCharsStart.js
new file mode 100644
index 0000000..ff0ee65
--- /dev/null
+++ b/node_modules/lodash/fp/trimCharsStart.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('trimCharsStart', require('../trimStart'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/trimEnd.js b/node_modules/lodash/fp/trimEnd.js
new file mode 100644
index 0000000..7190880
--- /dev/null
+++ b/node_modules/lodash/fp/trimEnd.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('trimEnd', require('../trimEnd'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/trimStart.js b/node_modules/lodash/fp/trimStart.js
new file mode 100644
index 0000000..fda902c
--- /dev/null
+++ b/node_modules/lodash/fp/trimStart.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('trimStart', require('../trimStart'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/truncate.js b/node_modules/lodash/fp/truncate.js
new file mode 100644
index 0000000..d265c1d
--- /dev/null
+++ b/node_modules/lodash/fp/truncate.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('truncate', require('../truncate'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/unapply.js b/node_modules/lodash/fp/unapply.js
new file mode 100644
index 0000000..c5dfe77
--- /dev/null
+++ b/node_modules/lodash/fp/unapply.js
@@ -0,0 +1 @@
+module.exports = require('./rest');
diff --git a/node_modules/lodash/fp/unary.js b/node_modules/lodash/fp/unary.js
new file mode 100644
index 0000000..286c945
--- /dev/null
+++ b/node_modules/lodash/fp/unary.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('unary', require('../unary'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/unescape.js b/node_modules/lodash/fp/unescape.js
new file mode 100644
index 0000000..fddcb46
--- /dev/null
+++ b/node_modules/lodash/fp/unescape.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('unescape', require('../unescape'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/union.js b/node_modules/lodash/fp/union.js
new file mode 100644
index 0000000..ef8228d
--- /dev/null
+++ b/node_modules/lodash/fp/union.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('union', require('../union'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/unionBy.js b/node_modules/lodash/fp/unionBy.js
new file mode 100644
index 0000000..603687a
--- /dev/null
+++ b/node_modules/lodash/fp/unionBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('unionBy', require('../unionBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/unionWith.js b/node_modules/lodash/fp/unionWith.js
new file mode 100644
index 0000000..65bb3a7
--- /dev/null
+++ b/node_modules/lodash/fp/unionWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('unionWith', require('../unionWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/uniq.js b/node_modules/lodash/fp/uniq.js
new file mode 100644
index 0000000..bc18524
--- /dev/null
+++ b/node_modules/lodash/fp/uniq.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('uniq', require('../uniq'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/uniqBy.js b/node_modules/lodash/fp/uniqBy.js
new file mode 100644
index 0000000..634c6a8
--- /dev/null
+++ b/node_modules/lodash/fp/uniqBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('uniqBy', require('../uniqBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/uniqWith.js b/node_modules/lodash/fp/uniqWith.js
new file mode 100644
index 0000000..0ec601a
--- /dev/null
+++ b/node_modules/lodash/fp/uniqWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('uniqWith', require('../uniqWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/uniqueId.js b/node_modules/lodash/fp/uniqueId.js
new file mode 100644
index 0000000..aa8fc2f
--- /dev/null
+++ b/node_modules/lodash/fp/uniqueId.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('uniqueId', require('../uniqueId'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/unnest.js b/node_modules/lodash/fp/unnest.js
new file mode 100644
index 0000000..5d34060
--- /dev/null
+++ b/node_modules/lodash/fp/unnest.js
@@ -0,0 +1 @@
+module.exports = require('./flatten');
diff --git a/node_modules/lodash/fp/unset.js b/node_modules/lodash/fp/unset.js
new file mode 100644
index 0000000..ea203a0
--- /dev/null
+++ b/node_modules/lodash/fp/unset.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('unset', require('../unset'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/unzip.js b/node_modules/lodash/fp/unzip.js
new file mode 100644
index 0000000..cc364b3
--- /dev/null
+++ b/node_modules/lodash/fp/unzip.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('unzip', require('../unzip'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/unzipWith.js b/node_modules/lodash/fp/unzipWith.js
new file mode 100644
index 0000000..182eaa1
--- /dev/null
+++ b/node_modules/lodash/fp/unzipWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('unzipWith', require('../unzipWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/update.js b/node_modules/lodash/fp/update.js
new file mode 100644
index 0000000..b8ce2cc
--- /dev/null
+++ b/node_modules/lodash/fp/update.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('update', require('../update'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/updateWith.js b/node_modules/lodash/fp/updateWith.js
new file mode 100644
index 0000000..d5e8282
--- /dev/null
+++ b/node_modules/lodash/fp/updateWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('updateWith', require('../updateWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/upperCase.js b/node_modules/lodash/fp/upperCase.js
new file mode 100644
index 0000000..c886f20
--- /dev/null
+++ b/node_modules/lodash/fp/upperCase.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('upperCase', require('../upperCase'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/upperFirst.js b/node_modules/lodash/fp/upperFirst.js
new file mode 100644
index 0000000..d8c04df
--- /dev/null
+++ b/node_modules/lodash/fp/upperFirst.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('upperFirst', require('../upperFirst'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/useWith.js b/node_modules/lodash/fp/useWith.js
new file mode 100644
index 0000000..d8b3df5
--- /dev/null
+++ b/node_modules/lodash/fp/useWith.js
@@ -0,0 +1 @@
+module.exports = require('./overArgs');
diff --git a/node_modules/lodash/fp/util.js b/node_modules/lodash/fp/util.js
new file mode 100644
index 0000000..18c00ba
--- /dev/null
+++ b/node_modules/lodash/fp/util.js
@@ -0,0 +1,2 @@
+var convert = require('./convert');
+module.exports = convert(require('../util'));
diff --git a/node_modules/lodash/fp/value.js b/node_modules/lodash/fp/value.js
new file mode 100644
index 0000000..555eec7
--- /dev/null
+++ b/node_modules/lodash/fp/value.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('value', require('../value'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/valueOf.js b/node_modules/lodash/fp/valueOf.js
new file mode 100644
index 0000000..f968807
--- /dev/null
+++ b/node_modules/lodash/fp/valueOf.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('valueOf', require('../valueOf'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/values.js b/node_modules/lodash/fp/values.js
new file mode 100644
index 0000000..2dfc561
--- /dev/null
+++ b/node_modules/lodash/fp/values.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('values', require('../values'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/valuesIn.js b/node_modules/lodash/fp/valuesIn.js
new file mode 100644
index 0000000..a1b2bb8
--- /dev/null
+++ b/node_modules/lodash/fp/valuesIn.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('valuesIn', require('../valuesIn'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/where.js b/node_modules/lodash/fp/where.js
new file mode 100644
index 0000000..3247f64
--- /dev/null
+++ b/node_modules/lodash/fp/where.js
@@ -0,0 +1 @@
+module.exports = require('./conformsTo');
diff --git a/node_modules/lodash/fp/whereEq.js b/node_modules/lodash/fp/whereEq.js
new file mode 100644
index 0000000..29d1e1e
--- /dev/null
+++ b/node_modules/lodash/fp/whereEq.js
@@ -0,0 +1 @@
+module.exports = require('./isMatch');
diff --git a/node_modules/lodash/fp/without.js b/node_modules/lodash/fp/without.js
new file mode 100644
index 0000000..bad9e12
--- /dev/null
+++ b/node_modules/lodash/fp/without.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('without', require('../without'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/words.js b/node_modules/lodash/fp/words.js
new file mode 100644
index 0000000..4a90141
--- /dev/null
+++ b/node_modules/lodash/fp/words.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('words', require('../words'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/wrap.js b/node_modules/lodash/fp/wrap.js
new file mode 100644
index 0000000..e93bd8a
--- /dev/null
+++ b/node_modules/lodash/fp/wrap.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('wrap', require('../wrap'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/wrapperAt.js b/node_modules/lodash/fp/wrapperAt.js
new file mode 100644
index 0000000..8f0a310
--- /dev/null
+++ b/node_modules/lodash/fp/wrapperAt.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('wrapperAt', require('../wrapperAt'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/wrapperChain.js b/node_modules/lodash/fp/wrapperChain.js
new file mode 100644
index 0000000..2a48ea2
--- /dev/null
+++ b/node_modules/lodash/fp/wrapperChain.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('wrapperChain', require('../wrapperChain'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/wrapperLodash.js b/node_modules/lodash/fp/wrapperLodash.js
new file mode 100644
index 0000000..a7162d0
--- /dev/null
+++ b/node_modules/lodash/fp/wrapperLodash.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('wrapperLodash', require('../wrapperLodash'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/wrapperReverse.js b/node_modules/lodash/fp/wrapperReverse.js
new file mode 100644
index 0000000..e1481aa
--- /dev/null
+++ b/node_modules/lodash/fp/wrapperReverse.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('wrapperReverse', require('../wrapperReverse'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/wrapperValue.js b/node_modules/lodash/fp/wrapperValue.js
new file mode 100644
index 0000000..8eb9112
--- /dev/null
+++ b/node_modules/lodash/fp/wrapperValue.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('wrapperValue', require('../wrapperValue'), require('./_falseOptions'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/xor.js b/node_modules/lodash/fp/xor.js
new file mode 100644
index 0000000..29e2819
--- /dev/null
+++ b/node_modules/lodash/fp/xor.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('xor', require('../xor'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/xorBy.js b/node_modules/lodash/fp/xorBy.js
new file mode 100644
index 0000000..b355686
--- /dev/null
+++ b/node_modules/lodash/fp/xorBy.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('xorBy', require('../xorBy'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/xorWith.js b/node_modules/lodash/fp/xorWith.js
new file mode 100644
index 0000000..8e05739
--- /dev/null
+++ b/node_modules/lodash/fp/xorWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('xorWith', require('../xorWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/zip.js b/node_modules/lodash/fp/zip.js
new file mode 100644
index 0000000..69e147a
--- /dev/null
+++ b/node_modules/lodash/fp/zip.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('zip', require('../zip'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/zipAll.js b/node_modules/lodash/fp/zipAll.js
new file mode 100644
index 0000000..efa8ccb
--- /dev/null
+++ b/node_modules/lodash/fp/zipAll.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('zipAll', require('../zip'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/zipObj.js b/node_modules/lodash/fp/zipObj.js
new file mode 100644
index 0000000..f4a3453
--- /dev/null
+++ b/node_modules/lodash/fp/zipObj.js
@@ -0,0 +1 @@
+module.exports = require('./zipObject');
diff --git a/node_modules/lodash/fp/zipObject.js b/node_modules/lodash/fp/zipObject.js
new file mode 100644
index 0000000..462dbb6
--- /dev/null
+++ b/node_modules/lodash/fp/zipObject.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('zipObject', require('../zipObject'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/zipObjectDeep.js b/node_modules/lodash/fp/zipObjectDeep.js
new file mode 100644
index 0000000..53a5d33
--- /dev/null
+++ b/node_modules/lodash/fp/zipObjectDeep.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('zipObjectDeep', require('../zipObjectDeep'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fp/zipWith.js b/node_modules/lodash/fp/zipWith.js
new file mode 100644
index 0000000..c5cf9e2
--- /dev/null
+++ b/node_modules/lodash/fp/zipWith.js
@@ -0,0 +1,5 @@
+var convert = require('./convert'),
+ func = convert('zipWith', require('../zipWith'));
+
+func.placeholder = require('./placeholder');
+module.exports = func;
diff --git a/node_modules/lodash/fromPairs.js b/node_modules/lodash/fromPairs.js
new file mode 100644
index 0000000..ee7940d
--- /dev/null
+++ b/node_modules/lodash/fromPairs.js
@@ -0,0 +1,28 @@
+/**
+ * The inverse of `_.toPairs`; this method returns an object composed
+ * from key-value `pairs`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} pairs The key-value pairs.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * _.fromPairs([['a', 1], ['b', 2]]);
+ * // => { 'a': 1, 'b': 2 }
+ */
+function fromPairs(pairs) {
+ var index = -1,
+ length = pairs == null ? 0 : pairs.length,
+ result = {};
+
+ while (++index < length) {
+ var pair = pairs[index];
+ result[pair[0]] = pair[1];
+ }
+ return result;
+}
+
+module.exports = fromPairs;
diff --git a/node_modules/lodash/function.js b/node_modules/lodash/function.js
new file mode 100644
index 0000000..b0fc6d9
--- /dev/null
+++ b/node_modules/lodash/function.js
@@ -0,0 +1,25 @@
+module.exports = {
+ 'after': require('./after'),
+ 'ary': require('./ary'),
+ 'before': require('./before'),
+ 'bind': require('./bind'),
+ 'bindKey': require('./bindKey'),
+ 'curry': require('./curry'),
+ 'curryRight': require('./curryRight'),
+ 'debounce': require('./debounce'),
+ 'defer': require('./defer'),
+ 'delay': require('./delay'),
+ 'flip': require('./flip'),
+ 'memoize': require('./memoize'),
+ 'negate': require('./negate'),
+ 'once': require('./once'),
+ 'overArgs': require('./overArgs'),
+ 'partial': require('./partial'),
+ 'partialRight': require('./partialRight'),
+ 'rearg': require('./rearg'),
+ 'rest': require('./rest'),
+ 'spread': require('./spread'),
+ 'throttle': require('./throttle'),
+ 'unary': require('./unary'),
+ 'wrap': require('./wrap')
+};
diff --git a/node_modules/lodash/functions.js b/node_modules/lodash/functions.js
new file mode 100644
index 0000000..9722928
--- /dev/null
+++ b/node_modules/lodash/functions.js
@@ -0,0 +1,31 @@
+var baseFunctions = require('./_baseFunctions'),
+ keys = require('./keys');
+
+/**
+ * Creates an array of function property names from own enumerable properties
+ * of `object`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns the function names.
+ * @see _.functionsIn
+ * @example
+ *
+ * function Foo() {
+ * this.a = _.constant('a');
+ * this.b = _.constant('b');
+ * }
+ *
+ * Foo.prototype.c = _.constant('c');
+ *
+ * _.functions(new Foo);
+ * // => ['a', 'b']
+ */
+function functions(object) {
+ return object == null ? [] : baseFunctions(object, keys(object));
+}
+
+module.exports = functions;
diff --git a/node_modules/lodash/functionsIn.js b/node_modules/lodash/functionsIn.js
new file mode 100644
index 0000000..f00345d
--- /dev/null
+++ b/node_modules/lodash/functionsIn.js
@@ -0,0 +1,31 @@
+var baseFunctions = require('./_baseFunctions'),
+ keysIn = require('./keysIn');
+
+/**
+ * Creates an array of function property names from own and inherited
+ * enumerable properties of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns the function names.
+ * @see _.functions
+ * @example
+ *
+ * function Foo() {
+ * this.a = _.constant('a');
+ * this.b = _.constant('b');
+ * }
+ *
+ * Foo.prototype.c = _.constant('c');
+ *
+ * _.functionsIn(new Foo);
+ * // => ['a', 'b', 'c']
+ */
+function functionsIn(object) {
+ return object == null ? [] : baseFunctions(object, keysIn(object));
+}
+
+module.exports = functionsIn;
diff --git a/node_modules/lodash/get.js b/node_modules/lodash/get.js
new file mode 100644
index 0000000..8805ff9
--- /dev/null
+++ b/node_modules/lodash/get.js
@@ -0,0 +1,33 @@
+var baseGet = require('./_baseGet');
+
+/**
+ * Gets the value at `path` of `object`. If the resolved value is
+ * `undefined`, the `defaultValue` is returned in its place.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.7.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the property to get.
+ * @param {*} [defaultValue] The value returned for `undefined` resolved values.
+ * @returns {*} Returns the resolved value.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': 3 } }] };
+ *
+ * _.get(object, 'a[0].b.c');
+ * // => 3
+ *
+ * _.get(object, ['a', '0', 'b', 'c']);
+ * // => 3
+ *
+ * _.get(object, 'a.b.c', 'default');
+ * // => 'default'
+ */
+function get(object, path, defaultValue) {
+ var result = object == null ? undefined : baseGet(object, path);
+ return result === undefined ? defaultValue : result;
+}
+
+module.exports = get;
diff --git a/node_modules/lodash/groupBy.js b/node_modules/lodash/groupBy.js
new file mode 100644
index 0000000..babf4f6
--- /dev/null
+++ b/node_modules/lodash/groupBy.js
@@ -0,0 +1,41 @@
+var baseAssignValue = require('./_baseAssignValue'),
+ createAggregator = require('./_createAggregator');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` thru `iteratee`. The order of grouped values
+ * is determined by the order they occur in `collection`. The corresponding
+ * value of each key is an array of elements responsible for generating the
+ * key. The iteratee is invoked with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The iteratee to transform keys.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.groupBy([6.1, 4.2, 6.3], Math.floor);
+ * // => { '4': [4.2], '6': [6.1, 6.3] }
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.groupBy(['one', 'two', 'three'], 'length');
+ * // => { '3': ['one', 'two'], '5': ['three'] }
+ */
+var groupBy = createAggregator(function(result, value, key) {
+ if (hasOwnProperty.call(result, key)) {
+ result[key].push(value);
+ } else {
+ baseAssignValue(result, key, [value]);
+ }
+});
+
+module.exports = groupBy;
diff --git a/node_modules/lodash/gt.js b/node_modules/lodash/gt.js
new file mode 100644
index 0000000..3a66282
--- /dev/null
+++ b/node_modules/lodash/gt.js
@@ -0,0 +1,29 @@
+var baseGt = require('./_baseGt'),
+ createRelationalOperation = require('./_createRelationalOperation');
+
+/**
+ * Checks if `value` is greater than `other`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.9.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is greater than `other`,
+ * else `false`.
+ * @see _.lt
+ * @example
+ *
+ * _.gt(3, 1);
+ * // => true
+ *
+ * _.gt(3, 3);
+ * // => false
+ *
+ * _.gt(1, 3);
+ * // => false
+ */
+var gt = createRelationalOperation(baseGt);
+
+module.exports = gt;
diff --git a/node_modules/lodash/gte.js b/node_modules/lodash/gte.js
new file mode 100644
index 0000000..4180a68
--- /dev/null
+++ b/node_modules/lodash/gte.js
@@ -0,0 +1,30 @@
+var createRelationalOperation = require('./_createRelationalOperation');
+
+/**
+ * Checks if `value` is greater than or equal to `other`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.9.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is greater than or equal to
+ * `other`, else `false`.
+ * @see _.lte
+ * @example
+ *
+ * _.gte(3, 1);
+ * // => true
+ *
+ * _.gte(3, 3);
+ * // => true
+ *
+ * _.gte(1, 3);
+ * // => false
+ */
+var gte = createRelationalOperation(function(value, other) {
+ return value >= other;
+});
+
+module.exports = gte;
diff --git a/node_modules/lodash/has.js b/node_modules/lodash/has.js
new file mode 100644
index 0000000..34df55e
--- /dev/null
+++ b/node_modules/lodash/has.js
@@ -0,0 +1,35 @@
+var baseHas = require('./_baseHas'),
+ hasPath = require('./_hasPath');
+
+/**
+ * Checks if `path` is a direct property of `object`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path to check.
+ * @returns {boolean} Returns `true` if `path` exists, else `false`.
+ * @example
+ *
+ * var object = { 'a': { 'b': 2 } };
+ * var other = _.create({ 'a': _.create({ 'b': 2 }) });
+ *
+ * _.has(object, 'a');
+ * // => true
+ *
+ * _.has(object, 'a.b');
+ * // => true
+ *
+ * _.has(object, ['a', 'b']);
+ * // => true
+ *
+ * _.has(other, 'a');
+ * // => false
+ */
+function has(object, path) {
+ return object != null && hasPath(object, path, baseHas);
+}
+
+module.exports = has;
diff --git a/node_modules/lodash/hasIn.js b/node_modules/lodash/hasIn.js
new file mode 100644
index 0000000..06a3686
--- /dev/null
+++ b/node_modules/lodash/hasIn.js
@@ -0,0 +1,34 @@
+var baseHasIn = require('./_baseHasIn'),
+ hasPath = require('./_hasPath');
+
+/**
+ * Checks if `path` is a direct or inherited property of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path to check.
+ * @returns {boolean} Returns `true` if `path` exists, else `false`.
+ * @example
+ *
+ * var object = _.create({ 'a': _.create({ 'b': 2 }) });
+ *
+ * _.hasIn(object, 'a');
+ * // => true
+ *
+ * _.hasIn(object, 'a.b');
+ * // => true
+ *
+ * _.hasIn(object, ['a', 'b']);
+ * // => true
+ *
+ * _.hasIn(object, 'b');
+ * // => false
+ */
+function hasIn(object, path) {
+ return object != null && hasPath(object, path, baseHasIn);
+}
+
+module.exports = hasIn;
diff --git a/node_modules/lodash/head.js b/node_modules/lodash/head.js
new file mode 100644
index 0000000..dee9d1f
--- /dev/null
+++ b/node_modules/lodash/head.js
@@ -0,0 +1,23 @@
+/**
+ * Gets the first element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @alias first
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {*} Returns the first element of `array`.
+ * @example
+ *
+ * _.head([1, 2, 3]);
+ * // => 1
+ *
+ * _.head([]);
+ * // => undefined
+ */
+function head(array) {
+ return (array && array.length) ? array[0] : undefined;
+}
+
+module.exports = head;
diff --git a/node_modules/lodash/identity.js b/node_modules/lodash/identity.js
new file mode 100644
index 0000000..2d5d963
--- /dev/null
+++ b/node_modules/lodash/identity.js
@@ -0,0 +1,21 @@
+/**
+ * This method returns the first argument it receives.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Util
+ * @param {*} value Any value.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ *
+ * console.log(_.identity(object) === object);
+ * // => true
+ */
+function identity(value) {
+ return value;
+}
+
+module.exports = identity;
diff --git a/node_modules/lodash/inRange.js b/node_modules/lodash/inRange.js
new file mode 100644
index 0000000..f20728d
--- /dev/null
+++ b/node_modules/lodash/inRange.js
@@ -0,0 +1,55 @@
+var baseInRange = require('./_baseInRange'),
+ toFinite = require('./toFinite'),
+ toNumber = require('./toNumber');
+
+/**
+ * Checks if `n` is between `start` and up to, but not including, `end`. If
+ * `end` is not specified, it's set to `start` with `start` then set to `0`.
+ * If `start` is greater than `end` the params are swapped to support
+ * negative ranges.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.3.0
+ * @category Number
+ * @param {number} number The number to check.
+ * @param {number} [start=0] The start of the range.
+ * @param {number} end The end of the range.
+ * @returns {boolean} Returns `true` if `number` is in the range, else `false`.
+ * @see _.range, _.rangeRight
+ * @example
+ *
+ * _.inRange(3, 2, 4);
+ * // => true
+ *
+ * _.inRange(4, 8);
+ * // => true
+ *
+ * _.inRange(4, 2);
+ * // => false
+ *
+ * _.inRange(2, 2);
+ * // => false
+ *
+ * _.inRange(1.2, 2);
+ * // => true
+ *
+ * _.inRange(5.2, 4);
+ * // => false
+ *
+ * _.inRange(-3, -2, -6);
+ * // => true
+ */
+function inRange(number, start, end) {
+ start = toFinite(start);
+ if (end === undefined) {
+ end = start;
+ start = 0;
+ } else {
+ end = toFinite(end);
+ }
+ number = toNumber(number);
+ return baseInRange(number, start, end);
+}
+
+module.exports = inRange;
diff --git a/node_modules/lodash/includes.js b/node_modules/lodash/includes.js
new file mode 100644
index 0000000..ae0deed
--- /dev/null
+++ b/node_modules/lodash/includes.js
@@ -0,0 +1,53 @@
+var baseIndexOf = require('./_baseIndexOf'),
+ isArrayLike = require('./isArrayLike'),
+ isString = require('./isString'),
+ toInteger = require('./toInteger'),
+ values = require('./values');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Checks if `value` is in `collection`. If `collection` is a string, it's
+ * checked for a substring of `value`, otherwise
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * is used for equality comparisons. If `fromIndex` is negative, it's used as
+ * the offset from the end of `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.reduce`.
+ * @returns {boolean} Returns `true` if `value` is found, else `false`.
+ * @example
+ *
+ * _.includes([1, 2, 3], 1);
+ * // => true
+ *
+ * _.includes([1, 2, 3], 1, 2);
+ * // => false
+ *
+ * _.includes({ 'a': 1, 'b': 2 }, 1);
+ * // => true
+ *
+ * _.includes('abcd', 'bc');
+ * // => true
+ */
+function includes(collection, value, fromIndex, guard) {
+ collection = isArrayLike(collection) ? collection : values(collection);
+ fromIndex = (fromIndex && !guard) ? toInteger(fromIndex) : 0;
+
+ var length = collection.length;
+ if (fromIndex < 0) {
+ fromIndex = nativeMax(length + fromIndex, 0);
+ }
+ return isString(collection)
+ ? (fromIndex <= length && collection.indexOf(value, fromIndex) > -1)
+ : (!!length && baseIndexOf(collection, value, fromIndex) > -1);
+}
+
+module.exports = includes;
diff --git a/node_modules/lodash/index.js b/node_modules/lodash/index.js
new file mode 100644
index 0000000..5d063e2
--- /dev/null
+++ b/node_modules/lodash/index.js
@@ -0,0 +1 @@
+module.exports = require('./lodash');
\ No newline at end of file
diff --git a/node_modules/lodash/indexOf.js b/node_modules/lodash/indexOf.js
new file mode 100644
index 0000000..3c644af
--- /dev/null
+++ b/node_modules/lodash/indexOf.js
@@ -0,0 +1,42 @@
+var baseIndexOf = require('./_baseIndexOf'),
+ toInteger = require('./toInteger');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Gets the index at which the first occurrence of `value` is found in `array`
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons. If `fromIndex` is negative, it's used as the
+ * offset from the end of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ * @example
+ *
+ * _.indexOf([1, 2, 1, 2], 2);
+ * // => 1
+ *
+ * // Search from the `fromIndex`.
+ * _.indexOf([1, 2, 1, 2], 2, 2);
+ * // => 3
+ */
+function indexOf(array, value, fromIndex) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return -1;
+ }
+ var index = fromIndex == null ? 0 : toInteger(fromIndex);
+ if (index < 0) {
+ index = nativeMax(length + index, 0);
+ }
+ return baseIndexOf(array, value, index);
+}
+
+module.exports = indexOf;
diff --git a/node_modules/lodash/initial.js b/node_modules/lodash/initial.js
new file mode 100644
index 0000000..f47fc50
--- /dev/null
+++ b/node_modules/lodash/initial.js
@@ -0,0 +1,22 @@
+var baseSlice = require('./_baseSlice');
+
+/**
+ * Gets all but the last element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.initial([1, 2, 3]);
+ * // => [1, 2]
+ */
+function initial(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? baseSlice(array, 0, -1) : [];
+}
+
+module.exports = initial;
diff --git a/node_modules/lodash/intersection.js b/node_modules/lodash/intersection.js
new file mode 100644
index 0000000..a94c135
--- /dev/null
+++ b/node_modules/lodash/intersection.js
@@ -0,0 +1,30 @@
+var arrayMap = require('./_arrayMap'),
+ baseIntersection = require('./_baseIntersection'),
+ baseRest = require('./_baseRest'),
+ castArrayLikeObject = require('./_castArrayLikeObject');
+
+/**
+ * Creates an array of unique values that are included in all given arrays
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons. The order and references of result values are
+ * determined by the first array.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @returns {Array} Returns the new array of intersecting values.
+ * @example
+ *
+ * _.intersection([2, 1], [2, 3]);
+ * // => [2]
+ */
+var intersection = baseRest(function(arrays) {
+ var mapped = arrayMap(arrays, castArrayLikeObject);
+ return (mapped.length && mapped[0] === arrays[0])
+ ? baseIntersection(mapped)
+ : [];
+});
+
+module.exports = intersection;
diff --git a/node_modules/lodash/intersectionBy.js b/node_modules/lodash/intersectionBy.js
new file mode 100644
index 0000000..31461aa
--- /dev/null
+++ b/node_modules/lodash/intersectionBy.js
@@ -0,0 +1,45 @@
+var arrayMap = require('./_arrayMap'),
+ baseIntersection = require('./_baseIntersection'),
+ baseIteratee = require('./_baseIteratee'),
+ baseRest = require('./_baseRest'),
+ castArrayLikeObject = require('./_castArrayLikeObject'),
+ last = require('./last');
+
+/**
+ * This method is like `_.intersection` except that it accepts `iteratee`
+ * which is invoked for each element of each `arrays` to generate the criterion
+ * by which they're compared. The order and references of result values are
+ * determined by the first array. The iteratee is invoked with one argument:
+ * (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {Array} Returns the new array of intersecting values.
+ * @example
+ *
+ * _.intersectionBy([2.1, 1.2], [2.3, 3.4], Math.floor);
+ * // => [2.1]
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.intersectionBy([{ 'x': 1 }], [{ 'x': 2 }, { 'x': 1 }], 'x');
+ * // => [{ 'x': 1 }]
+ */
+var intersectionBy = baseRest(function(arrays) {
+ var iteratee = last(arrays),
+ mapped = arrayMap(arrays, castArrayLikeObject);
+
+ if (iteratee === last(mapped)) {
+ iteratee = undefined;
+ } else {
+ mapped.pop();
+ }
+ return (mapped.length && mapped[0] === arrays[0])
+ ? baseIntersection(mapped, baseIteratee(iteratee, 2))
+ : [];
+});
+
+module.exports = intersectionBy;
diff --git a/node_modules/lodash/intersectionWith.js b/node_modules/lodash/intersectionWith.js
new file mode 100644
index 0000000..63cabfa
--- /dev/null
+++ b/node_modules/lodash/intersectionWith.js
@@ -0,0 +1,41 @@
+var arrayMap = require('./_arrayMap'),
+ baseIntersection = require('./_baseIntersection'),
+ baseRest = require('./_baseRest'),
+ castArrayLikeObject = require('./_castArrayLikeObject'),
+ last = require('./last');
+
+/**
+ * This method is like `_.intersection` except that it accepts `comparator`
+ * which is invoked to compare elements of `arrays`. The order and references
+ * of result values are determined by the first array. The comparator is
+ * invoked with two arguments: (arrVal, othVal).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of intersecting values.
+ * @example
+ *
+ * var objects = [{ 'x': 1, 'y': 2 }, { 'x': 2, 'y': 1 }];
+ * var others = [{ 'x': 1, 'y': 1 }, { 'x': 1, 'y': 2 }];
+ *
+ * _.intersectionWith(objects, others, _.isEqual);
+ * // => [{ 'x': 1, 'y': 2 }]
+ */
+var intersectionWith = baseRest(function(arrays) {
+ var comparator = last(arrays),
+ mapped = arrayMap(arrays, castArrayLikeObject);
+
+ comparator = typeof comparator == 'function' ? comparator : undefined;
+ if (comparator) {
+ mapped.pop();
+ }
+ return (mapped.length && mapped[0] === arrays[0])
+ ? baseIntersection(mapped, undefined, comparator)
+ : [];
+});
+
+module.exports = intersectionWith;
diff --git a/node_modules/lodash/invert.js b/node_modules/lodash/invert.js
new file mode 100644
index 0000000..8c47950
--- /dev/null
+++ b/node_modules/lodash/invert.js
@@ -0,0 +1,42 @@
+var constant = require('./constant'),
+ createInverter = require('./_createInverter'),
+ identity = require('./identity');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the
+ * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var nativeObjectToString = objectProto.toString;
+
+/**
+ * Creates an object composed of the inverted keys and values of `object`.
+ * If `object` contains duplicate values, subsequent values overwrite
+ * property assignments of previous values.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.7.0
+ * @category Object
+ * @param {Object} object The object to invert.
+ * @returns {Object} Returns the new inverted object.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': 2, 'c': 1 };
+ *
+ * _.invert(object);
+ * // => { '1': 'c', '2': 'b' }
+ */
+var invert = createInverter(function(result, value, key) {
+ if (value != null &&
+ typeof value.toString != 'function') {
+ value = nativeObjectToString.call(value);
+ }
+
+ result[value] = key;
+}, constant(identity));
+
+module.exports = invert;
diff --git a/node_modules/lodash/invertBy.js b/node_modules/lodash/invertBy.js
new file mode 100644
index 0000000..3f4f7e5
--- /dev/null
+++ b/node_modules/lodash/invertBy.js
@@ -0,0 +1,56 @@
+var baseIteratee = require('./_baseIteratee'),
+ createInverter = require('./_createInverter');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the
+ * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var nativeObjectToString = objectProto.toString;
+
+/**
+ * This method is like `_.invert` except that the inverted object is generated
+ * from the results of running each element of `object` thru `iteratee`. The
+ * corresponding inverted value of each inverted key is an array of keys
+ * responsible for generating the inverted value. The iteratee is invoked
+ * with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.1.0
+ * @category Object
+ * @param {Object} object The object to invert.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {Object} Returns the new inverted object.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': 2, 'c': 1 };
+ *
+ * _.invertBy(object);
+ * // => { '1': ['a', 'c'], '2': ['b'] }
+ *
+ * _.invertBy(object, function(value) {
+ * return 'group' + value;
+ * });
+ * // => { 'group1': ['a', 'c'], 'group2': ['b'] }
+ */
+var invertBy = createInverter(function(result, value, key) {
+ if (value != null &&
+ typeof value.toString != 'function') {
+ value = nativeObjectToString.call(value);
+ }
+
+ if (hasOwnProperty.call(result, value)) {
+ result[value].push(key);
+ } else {
+ result[value] = [key];
+ }
+}, baseIteratee);
+
+module.exports = invertBy;
diff --git a/node_modules/lodash/invoke.js b/node_modules/lodash/invoke.js
new file mode 100644
index 0000000..97d51eb
--- /dev/null
+++ b/node_modules/lodash/invoke.js
@@ -0,0 +1,24 @@
+var baseInvoke = require('./_baseInvoke'),
+ baseRest = require('./_baseRest');
+
+/**
+ * Invokes the method at `path` of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the method to invoke.
+ * @param {...*} [args] The arguments to invoke the method with.
+ * @returns {*} Returns the result of the invoked method.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': [1, 2, 3, 4] } }] };
+ *
+ * _.invoke(object, 'a[0].b.c.slice', 1, 3);
+ * // => [2, 3]
+ */
+var invoke = baseRest(baseInvoke);
+
+module.exports = invoke;
diff --git a/node_modules/lodash/invokeMap.js b/node_modules/lodash/invokeMap.js
new file mode 100644
index 0000000..8da5126
--- /dev/null
+++ b/node_modules/lodash/invokeMap.js
@@ -0,0 +1,41 @@
+var apply = require('./_apply'),
+ baseEach = require('./_baseEach'),
+ baseInvoke = require('./_baseInvoke'),
+ baseRest = require('./_baseRest'),
+ isArrayLike = require('./isArrayLike');
+
+/**
+ * Invokes the method at `path` of each element in `collection`, returning
+ * an array of the results of each invoked method. Any additional arguments
+ * are provided to each invoked method. If `path` is a function, it's invoked
+ * for, and `this` bound to, each element in `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Array|Function|string} path The path of the method to invoke or
+ * the function invoked per iteration.
+ * @param {...*} [args] The arguments to invoke each method with.
+ * @returns {Array} Returns the array of results.
+ * @example
+ *
+ * _.invokeMap([[5, 1, 7], [3, 2, 1]], 'sort');
+ * // => [[1, 5, 7], [1, 2, 3]]
+ *
+ * _.invokeMap([123, 456], String.prototype.split, '');
+ * // => [['1', '2', '3'], ['4', '5', '6']]
+ */
+var invokeMap = baseRest(function(collection, path, args) {
+ var index = -1,
+ isFunc = typeof path == 'function',
+ result = isArrayLike(collection) ? Array(collection.length) : [];
+
+ baseEach(collection, function(value) {
+ result[++index] = isFunc ? apply(path, value, args) : baseInvoke(value, path, args);
+ });
+ return result;
+});
+
+module.exports = invokeMap;
diff --git a/node_modules/lodash/isArguments.js b/node_modules/lodash/isArguments.js
new file mode 100644
index 0000000..8b9ed66
--- /dev/null
+++ b/node_modules/lodash/isArguments.js
@@ -0,0 +1,36 @@
+var baseIsArguments = require('./_baseIsArguments'),
+ isObjectLike = require('./isObjectLike');
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Built-in value references. */
+var propertyIsEnumerable = objectProto.propertyIsEnumerable;
+
+/**
+ * Checks if `value` is likely an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ * else `false`.
+ * @example
+ *
+ * _.isArguments(function() { return arguments; }());
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+var isArguments = baseIsArguments(function() { return arguments; }()) ? baseIsArguments : function(value) {
+ return isObjectLike(value) && hasOwnProperty.call(value, 'callee') &&
+ !propertyIsEnumerable.call(value, 'callee');
+};
+
+module.exports = isArguments;
diff --git a/node_modules/lodash/isArray.js b/node_modules/lodash/isArray.js
new file mode 100644
index 0000000..88ab55f
--- /dev/null
+++ b/node_modules/lodash/isArray.js
@@ -0,0 +1,26 @@
+/**
+ * Checks if `value` is classified as an `Array` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array, else `false`.
+ * @example
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ *
+ * _.isArray(document.body.children);
+ * // => false
+ *
+ * _.isArray('abc');
+ * // => false
+ *
+ * _.isArray(_.noop);
+ * // => false
+ */
+var isArray = Array.isArray;
+
+module.exports = isArray;
diff --git a/node_modules/lodash/isArrayBuffer.js b/node_modules/lodash/isArrayBuffer.js
new file mode 100644
index 0000000..12904a6
--- /dev/null
+++ b/node_modules/lodash/isArrayBuffer.js
@@ -0,0 +1,27 @@
+var baseIsArrayBuffer = require('./_baseIsArrayBuffer'),
+ baseUnary = require('./_baseUnary'),
+ nodeUtil = require('./_nodeUtil');
+
+/* Node.js helper references. */
+var nodeIsArrayBuffer = nodeUtil && nodeUtil.isArrayBuffer;
+
+/**
+ * Checks if `value` is classified as an `ArrayBuffer` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array buffer, else `false`.
+ * @example
+ *
+ * _.isArrayBuffer(new ArrayBuffer(2));
+ * // => true
+ *
+ * _.isArrayBuffer(new Array(2));
+ * // => false
+ */
+var isArrayBuffer = nodeIsArrayBuffer ? baseUnary(nodeIsArrayBuffer) : baseIsArrayBuffer;
+
+module.exports = isArrayBuffer;
diff --git a/node_modules/lodash/isArrayLike.js b/node_modules/lodash/isArrayLike.js
new file mode 100644
index 0000000..0f96680
--- /dev/null
+++ b/node_modules/lodash/isArrayLike.js
@@ -0,0 +1,33 @@
+var isFunction = require('./isFunction'),
+ isLength = require('./isLength');
+
+/**
+ * Checks if `value` is array-like. A value is considered array-like if it's
+ * not a function and has a `value.length` that's an integer greater than or
+ * equal to `0` and less than or equal to `Number.MAX_SAFE_INTEGER`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ * @example
+ *
+ * _.isArrayLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLike(document.body.children);
+ * // => true
+ *
+ * _.isArrayLike('abc');
+ * // => true
+ *
+ * _.isArrayLike(_.noop);
+ * // => false
+ */
+function isArrayLike(value) {
+ return value != null && isLength(value.length) && !isFunction(value);
+}
+
+module.exports = isArrayLike;
diff --git a/node_modules/lodash/isArrayLikeObject.js b/node_modules/lodash/isArrayLikeObject.js
new file mode 100644
index 0000000..6c4812a
--- /dev/null
+++ b/node_modules/lodash/isArrayLikeObject.js
@@ -0,0 +1,33 @@
+var isArrayLike = require('./isArrayLike'),
+ isObjectLike = require('./isObjectLike');
+
+/**
+ * This method is like `_.isArrayLike` except that it also checks if `value`
+ * is an object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array-like object,
+ * else `false`.
+ * @example
+ *
+ * _.isArrayLikeObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLikeObject(document.body.children);
+ * // => true
+ *
+ * _.isArrayLikeObject('abc');
+ * // => false
+ *
+ * _.isArrayLikeObject(_.noop);
+ * // => false
+ */
+function isArrayLikeObject(value) {
+ return isObjectLike(value) && isArrayLike(value);
+}
+
+module.exports = isArrayLikeObject;
diff --git a/node_modules/lodash/isBoolean.js b/node_modules/lodash/isBoolean.js
new file mode 100644
index 0000000..a43ed4b
--- /dev/null
+++ b/node_modules/lodash/isBoolean.js
@@ -0,0 +1,29 @@
+var baseGetTag = require('./_baseGetTag'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var boolTag = '[object Boolean]';
+
+/**
+ * Checks if `value` is classified as a boolean primitive or object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a boolean, else `false`.
+ * @example
+ *
+ * _.isBoolean(false);
+ * // => true
+ *
+ * _.isBoolean(null);
+ * // => false
+ */
+function isBoolean(value) {
+ return value === true || value === false ||
+ (isObjectLike(value) && baseGetTag(value) == boolTag);
+}
+
+module.exports = isBoolean;
diff --git a/node_modules/lodash/isBuffer.js b/node_modules/lodash/isBuffer.js
new file mode 100644
index 0000000..c103cc7
--- /dev/null
+++ b/node_modules/lodash/isBuffer.js
@@ -0,0 +1,38 @@
+var root = require('./_root'),
+ stubFalse = require('./stubFalse');
+
+/** Detect free variable `exports`. */
+var freeExports = typeof exports == 'object' && exports && !exports.nodeType && exports;
+
+/** Detect free variable `module`. */
+var freeModule = freeExports && typeof module == 'object' && module && !module.nodeType && module;
+
+/** Detect the popular CommonJS extension `module.exports`. */
+var moduleExports = freeModule && freeModule.exports === freeExports;
+
+/** Built-in value references. */
+var Buffer = moduleExports ? root.Buffer : undefined;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeIsBuffer = Buffer ? Buffer.isBuffer : undefined;
+
+/**
+ * Checks if `value` is a buffer.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a buffer, else `false`.
+ * @example
+ *
+ * _.isBuffer(new Buffer(2));
+ * // => true
+ *
+ * _.isBuffer(new Uint8Array(2));
+ * // => false
+ */
+var isBuffer = nativeIsBuffer || stubFalse;
+
+module.exports = isBuffer;
diff --git a/node_modules/lodash/isDate.js b/node_modules/lodash/isDate.js
new file mode 100644
index 0000000..7f0209f
--- /dev/null
+++ b/node_modules/lodash/isDate.js
@@ -0,0 +1,27 @@
+var baseIsDate = require('./_baseIsDate'),
+ baseUnary = require('./_baseUnary'),
+ nodeUtil = require('./_nodeUtil');
+
+/* Node.js helper references. */
+var nodeIsDate = nodeUtil && nodeUtil.isDate;
+
+/**
+ * Checks if `value` is classified as a `Date` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a date object, else `false`.
+ * @example
+ *
+ * _.isDate(new Date);
+ * // => true
+ *
+ * _.isDate('Mon April 23 2012');
+ * // => false
+ */
+var isDate = nodeIsDate ? baseUnary(nodeIsDate) : baseIsDate;
+
+module.exports = isDate;
diff --git a/node_modules/lodash/isElement.js b/node_modules/lodash/isElement.js
new file mode 100644
index 0000000..76ae29c
--- /dev/null
+++ b/node_modules/lodash/isElement.js
@@ -0,0 +1,25 @@
+var isObjectLike = require('./isObjectLike'),
+ isPlainObject = require('./isPlainObject');
+
+/**
+ * Checks if `value` is likely a DOM element.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a DOM element, else `false`.
+ * @example
+ *
+ * _.isElement(document.body);
+ * // => true
+ *
+ * _.isElement('');
+ * // => false
+ */
+function isElement(value) {
+ return isObjectLike(value) && value.nodeType === 1 && !isPlainObject(value);
+}
+
+module.exports = isElement;
diff --git a/node_modules/lodash/isEmpty.js b/node_modules/lodash/isEmpty.js
new file mode 100644
index 0000000..3597294
--- /dev/null
+++ b/node_modules/lodash/isEmpty.js
@@ -0,0 +1,77 @@
+var baseKeys = require('./_baseKeys'),
+ getTag = require('./_getTag'),
+ isArguments = require('./isArguments'),
+ isArray = require('./isArray'),
+ isArrayLike = require('./isArrayLike'),
+ isBuffer = require('./isBuffer'),
+ isPrototype = require('./_isPrototype'),
+ isTypedArray = require('./isTypedArray');
+
+/** `Object#toString` result references. */
+var mapTag = '[object Map]',
+ setTag = '[object Set]';
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Checks if `value` is an empty object, collection, map, or set.
+ *
+ * Objects are considered empty if they have no own enumerable string keyed
+ * properties.
+ *
+ * Array-like values such as `arguments` objects, arrays, buffers, strings, or
+ * jQuery-like collections are considered empty if they have a `length` of `0`.
+ * Similarly, maps and sets are considered empty if they have a `size` of `0`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is empty, else `false`.
+ * @example
+ *
+ * _.isEmpty(null);
+ * // => true
+ *
+ * _.isEmpty(true);
+ * // => true
+ *
+ * _.isEmpty(1);
+ * // => true
+ *
+ * _.isEmpty([1, 2, 3]);
+ * // => false
+ *
+ * _.isEmpty({ 'a': 1 });
+ * // => false
+ */
+function isEmpty(value) {
+ if (value == null) {
+ return true;
+ }
+ if (isArrayLike(value) &&
+ (isArray(value) || typeof value == 'string' || typeof value.splice == 'function' ||
+ isBuffer(value) || isTypedArray(value) || isArguments(value))) {
+ return !value.length;
+ }
+ var tag = getTag(value);
+ if (tag == mapTag || tag == setTag) {
+ return !value.size;
+ }
+ if (isPrototype(value)) {
+ return !baseKeys(value).length;
+ }
+ for (var key in value) {
+ if (hasOwnProperty.call(value, key)) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = isEmpty;
diff --git a/node_modules/lodash/isEqual.js b/node_modules/lodash/isEqual.js
new file mode 100644
index 0000000..5e23e76
--- /dev/null
+++ b/node_modules/lodash/isEqual.js
@@ -0,0 +1,35 @@
+var baseIsEqual = require('./_baseIsEqual');
+
+/**
+ * Performs a deep comparison between two values to determine if they are
+ * equivalent.
+ *
+ * **Note:** This method supports comparing arrays, array buffers, booleans,
+ * date objects, error objects, maps, numbers, `Object` objects, regexes,
+ * sets, strings, symbols, and typed arrays. `Object` objects are compared
+ * by their own, not inherited, enumerable properties. Functions and DOM
+ * nodes are compared by strict equality, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ * var other = { 'a': 1 };
+ *
+ * _.isEqual(object, other);
+ * // => true
+ *
+ * object === other;
+ * // => false
+ */
+function isEqual(value, other) {
+ return baseIsEqual(value, other);
+}
+
+module.exports = isEqual;
diff --git a/node_modules/lodash/isEqualWith.js b/node_modules/lodash/isEqualWith.js
new file mode 100644
index 0000000..21bdc7f
--- /dev/null
+++ b/node_modules/lodash/isEqualWith.js
@@ -0,0 +1,41 @@
+var baseIsEqual = require('./_baseIsEqual');
+
+/**
+ * This method is like `_.isEqual` except that it accepts `customizer` which
+ * is invoked to compare values. If `customizer` returns `undefined`, comparisons
+ * are handled by the method instead. The `customizer` is invoked with up to
+ * six arguments: (objValue, othValue [, index|key, object, other, stack]).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @param {Function} [customizer] The function to customize comparisons.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * function isGreeting(value) {
+ * return /^h(?:i|ello)$/.test(value);
+ * }
+ *
+ * function customizer(objValue, othValue) {
+ * if (isGreeting(objValue) && isGreeting(othValue)) {
+ * return true;
+ * }
+ * }
+ *
+ * var array = ['hello', 'goodbye'];
+ * var other = ['hi', 'goodbye'];
+ *
+ * _.isEqualWith(array, other, customizer);
+ * // => true
+ */
+function isEqualWith(value, other, customizer) {
+ customizer = typeof customizer == 'function' ? customizer : undefined;
+ var result = customizer ? customizer(value, other) : undefined;
+ return result === undefined ? baseIsEqual(value, other, undefined, customizer) : !!result;
+}
+
+module.exports = isEqualWith;
diff --git a/node_modules/lodash/isError.js b/node_modules/lodash/isError.js
new file mode 100644
index 0000000..b4f41e0
--- /dev/null
+++ b/node_modules/lodash/isError.js
@@ -0,0 +1,36 @@
+var baseGetTag = require('./_baseGetTag'),
+ isObjectLike = require('./isObjectLike'),
+ isPlainObject = require('./isPlainObject');
+
+/** `Object#toString` result references. */
+var domExcTag = '[object DOMException]',
+ errorTag = '[object Error]';
+
+/**
+ * Checks if `value` is an `Error`, `EvalError`, `RangeError`, `ReferenceError`,
+ * `SyntaxError`, `TypeError`, or `URIError` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an error object, else `false`.
+ * @example
+ *
+ * _.isError(new Error);
+ * // => true
+ *
+ * _.isError(Error);
+ * // => false
+ */
+function isError(value) {
+ if (!isObjectLike(value)) {
+ return false;
+ }
+ var tag = baseGetTag(value);
+ return tag == errorTag || tag == domExcTag ||
+ (typeof value.message == 'string' && typeof value.name == 'string' && !isPlainObject(value));
+}
+
+module.exports = isError;
diff --git a/node_modules/lodash/isFinite.js b/node_modules/lodash/isFinite.js
new file mode 100644
index 0000000..601842b
--- /dev/null
+++ b/node_modules/lodash/isFinite.js
@@ -0,0 +1,36 @@
+var root = require('./_root');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeIsFinite = root.isFinite;
+
+/**
+ * Checks if `value` is a finite primitive number.
+ *
+ * **Note:** This method is based on
+ * [`Number.isFinite`](https://mdn.io/Number/isFinite).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a finite number, else `false`.
+ * @example
+ *
+ * _.isFinite(3);
+ * // => true
+ *
+ * _.isFinite(Number.MIN_VALUE);
+ * // => true
+ *
+ * _.isFinite(Infinity);
+ * // => false
+ *
+ * _.isFinite('3');
+ * // => false
+ */
+function isFinite(value) {
+ return typeof value == 'number' && nativeIsFinite(value);
+}
+
+module.exports = isFinite;
diff --git a/node_modules/lodash/isFunction.js b/node_modules/lodash/isFunction.js
new file mode 100644
index 0000000..907a8cd
--- /dev/null
+++ b/node_modules/lodash/isFunction.js
@@ -0,0 +1,37 @@
+var baseGetTag = require('./_baseGetTag'),
+ isObject = require('./isObject');
+
+/** `Object#toString` result references. */
+var asyncTag = '[object AsyncFunction]',
+ funcTag = '[object Function]',
+ genTag = '[object GeneratorFunction]',
+ proxyTag = '[object Proxy]';
+
+/**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+function isFunction(value) {
+ if (!isObject(value)) {
+ return false;
+ }
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in Safari 9 which returns 'object' for typed arrays and other constructors.
+ var tag = baseGetTag(value);
+ return tag == funcTag || tag == genTag || tag == asyncTag || tag == proxyTag;
+}
+
+module.exports = isFunction;
diff --git a/node_modules/lodash/isInteger.js b/node_modules/lodash/isInteger.js
new file mode 100644
index 0000000..66aa87d
--- /dev/null
+++ b/node_modules/lodash/isInteger.js
@@ -0,0 +1,33 @@
+var toInteger = require('./toInteger');
+
+/**
+ * Checks if `value` is an integer.
+ *
+ * **Note:** This method is based on
+ * [`Number.isInteger`](https://mdn.io/Number/isInteger).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an integer, else `false`.
+ * @example
+ *
+ * _.isInteger(3);
+ * // => true
+ *
+ * _.isInteger(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isInteger(Infinity);
+ * // => false
+ *
+ * _.isInteger('3');
+ * // => false
+ */
+function isInteger(value) {
+ return typeof value == 'number' && value == toInteger(value);
+}
+
+module.exports = isInteger;
diff --git a/node_modules/lodash/isLength.js b/node_modules/lodash/isLength.js
new file mode 100644
index 0000000..3a95caa
--- /dev/null
+++ b/node_modules/lodash/isLength.js
@@ -0,0 +1,35 @@
+/** Used as references for various `Number` constants. */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This method is loosely based on
+ * [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ * @example
+ *
+ * _.isLength(3);
+ * // => true
+ *
+ * _.isLength(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isLength(Infinity);
+ * // => false
+ *
+ * _.isLength('3');
+ * // => false
+ */
+function isLength(value) {
+ return typeof value == 'number' &&
+ value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+}
+
+module.exports = isLength;
diff --git a/node_modules/lodash/isMap.js b/node_modules/lodash/isMap.js
new file mode 100644
index 0000000..44f8517
--- /dev/null
+++ b/node_modules/lodash/isMap.js
@@ -0,0 +1,27 @@
+var baseIsMap = require('./_baseIsMap'),
+ baseUnary = require('./_baseUnary'),
+ nodeUtil = require('./_nodeUtil');
+
+/* Node.js helper references. */
+var nodeIsMap = nodeUtil && nodeUtil.isMap;
+
+/**
+ * Checks if `value` is classified as a `Map` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a map, else `false`.
+ * @example
+ *
+ * _.isMap(new Map);
+ * // => true
+ *
+ * _.isMap(new WeakMap);
+ * // => false
+ */
+var isMap = nodeIsMap ? baseUnary(nodeIsMap) : baseIsMap;
+
+module.exports = isMap;
diff --git a/node_modules/lodash/isMatch.js b/node_modules/lodash/isMatch.js
new file mode 100644
index 0000000..9773a18
--- /dev/null
+++ b/node_modules/lodash/isMatch.js
@@ -0,0 +1,36 @@
+var baseIsMatch = require('./_baseIsMatch'),
+ getMatchData = require('./_getMatchData');
+
+/**
+ * Performs a partial deep comparison between `object` and `source` to
+ * determine if `object` contains equivalent property values.
+ *
+ * **Note:** This method is equivalent to `_.matches` when `source` is
+ * partially applied.
+ *
+ * Partial comparisons will match empty array and empty object `source`
+ * values against any array or object value, respectively. See `_.isEqual`
+ * for a list of supported value comparisons.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property values to match.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': 2 };
+ *
+ * _.isMatch(object, { 'b': 2 });
+ * // => true
+ *
+ * _.isMatch(object, { 'b': 1 });
+ * // => false
+ */
+function isMatch(object, source) {
+ return object === source || baseIsMatch(object, source, getMatchData(source));
+}
+
+module.exports = isMatch;
diff --git a/node_modules/lodash/isMatchWith.js b/node_modules/lodash/isMatchWith.js
new file mode 100644
index 0000000..187b6a6
--- /dev/null
+++ b/node_modules/lodash/isMatchWith.js
@@ -0,0 +1,41 @@
+var baseIsMatch = require('./_baseIsMatch'),
+ getMatchData = require('./_getMatchData');
+
+/**
+ * This method is like `_.isMatch` except that it accepts `customizer` which
+ * is invoked to compare values. If `customizer` returns `undefined`, comparisons
+ * are handled by the method instead. The `customizer` is invoked with five
+ * arguments: (objValue, srcValue, index|key, object, source).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property values to match.
+ * @param {Function} [customizer] The function to customize comparisons.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ * @example
+ *
+ * function isGreeting(value) {
+ * return /^h(?:i|ello)$/.test(value);
+ * }
+ *
+ * function customizer(objValue, srcValue) {
+ * if (isGreeting(objValue) && isGreeting(srcValue)) {
+ * return true;
+ * }
+ * }
+ *
+ * var object = { 'greeting': 'hello' };
+ * var source = { 'greeting': 'hi' };
+ *
+ * _.isMatchWith(object, source, customizer);
+ * // => true
+ */
+function isMatchWith(object, source, customizer) {
+ customizer = typeof customizer == 'function' ? customizer : undefined;
+ return baseIsMatch(object, source, getMatchData(source), customizer);
+}
+
+module.exports = isMatchWith;
diff --git a/node_modules/lodash/isNaN.js b/node_modules/lodash/isNaN.js
new file mode 100644
index 0000000..7d0d783
--- /dev/null
+++ b/node_modules/lodash/isNaN.js
@@ -0,0 +1,38 @@
+var isNumber = require('./isNumber');
+
+/**
+ * Checks if `value` is `NaN`.
+ *
+ * **Note:** This method is based on
+ * [`Number.isNaN`](https://mdn.io/Number/isNaN) and is not the same as
+ * global [`isNaN`](https://mdn.io/isNaN) which returns `true` for
+ * `undefined` and other non-number values.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `NaN`, else `false`.
+ * @example
+ *
+ * _.isNaN(NaN);
+ * // => true
+ *
+ * _.isNaN(new Number(NaN));
+ * // => true
+ *
+ * isNaN(undefined);
+ * // => true
+ *
+ * _.isNaN(undefined);
+ * // => false
+ */
+function isNaN(value) {
+ // An `NaN` primitive is the only value that is not equal to itself.
+ // Perform the `toStringTag` check first to avoid errors with some
+ // ActiveX objects in IE.
+ return isNumber(value) && value != +value;
+}
+
+module.exports = isNaN;
diff --git a/node_modules/lodash/isNative.js b/node_modules/lodash/isNative.js
new file mode 100644
index 0000000..f0cb8d5
--- /dev/null
+++ b/node_modules/lodash/isNative.js
@@ -0,0 +1,40 @@
+var baseIsNative = require('./_baseIsNative'),
+ isMaskable = require('./_isMaskable');
+
+/** Error message constants. */
+var CORE_ERROR_TEXT = 'Unsupported core-js use. Try https://npms.io/search?q=ponyfill.';
+
+/**
+ * Checks if `value` is a pristine native function.
+ *
+ * **Note:** This method can't reliably detect native functions in the presence
+ * of the core-js package because core-js circumvents this kind of detection.
+ * Despite multiple requests, the core-js maintainer has made it clear: any
+ * attempt to fix the detection will be obstructed. As a result, we're left
+ * with little choice but to throw an error. Unfortunately, this also affects
+ * packages, like [babel-polyfill](https://www.npmjs.com/package/babel-polyfill),
+ * which rely on core-js.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a native function,
+ * else `false`.
+ * @example
+ *
+ * _.isNative(Array.prototype.push);
+ * // => true
+ *
+ * _.isNative(_);
+ * // => false
+ */
+function isNative(value) {
+ if (isMaskable(value)) {
+ throw new Error(CORE_ERROR_TEXT);
+ }
+ return baseIsNative(value);
+}
+
+module.exports = isNative;
diff --git a/node_modules/lodash/isNil.js b/node_modules/lodash/isNil.js
new file mode 100644
index 0000000..79f0505
--- /dev/null
+++ b/node_modules/lodash/isNil.js
@@ -0,0 +1,25 @@
+/**
+ * Checks if `value` is `null` or `undefined`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is nullish, else `false`.
+ * @example
+ *
+ * _.isNil(null);
+ * // => true
+ *
+ * _.isNil(void 0);
+ * // => true
+ *
+ * _.isNil(NaN);
+ * // => false
+ */
+function isNil(value) {
+ return value == null;
+}
+
+module.exports = isNil;
diff --git a/node_modules/lodash/isNull.js b/node_modules/lodash/isNull.js
new file mode 100644
index 0000000..c0a374d
--- /dev/null
+++ b/node_modules/lodash/isNull.js
@@ -0,0 +1,22 @@
+/**
+ * Checks if `value` is `null`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `null`, else `false`.
+ * @example
+ *
+ * _.isNull(null);
+ * // => true
+ *
+ * _.isNull(void 0);
+ * // => false
+ */
+function isNull(value) {
+ return value === null;
+}
+
+module.exports = isNull;
diff --git a/node_modules/lodash/isNumber.js b/node_modules/lodash/isNumber.js
new file mode 100644
index 0000000..cd34ee4
--- /dev/null
+++ b/node_modules/lodash/isNumber.js
@@ -0,0 +1,38 @@
+var baseGetTag = require('./_baseGetTag'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var numberTag = '[object Number]';
+
+/**
+ * Checks if `value` is classified as a `Number` primitive or object.
+ *
+ * **Note:** To exclude `Infinity`, `-Infinity`, and `NaN`, which are
+ * classified as numbers, use the `_.isFinite` method.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a number, else `false`.
+ * @example
+ *
+ * _.isNumber(3);
+ * // => true
+ *
+ * _.isNumber(Number.MIN_VALUE);
+ * // => true
+ *
+ * _.isNumber(Infinity);
+ * // => true
+ *
+ * _.isNumber('3');
+ * // => false
+ */
+function isNumber(value) {
+ return typeof value == 'number' ||
+ (isObjectLike(value) && baseGetTag(value) == numberTag);
+}
+
+module.exports = isNumber;
diff --git a/node_modules/lodash/isObject.js b/node_modules/lodash/isObject.js
new file mode 100644
index 0000000..1dc8939
--- /dev/null
+++ b/node_modules/lodash/isObject.js
@@ -0,0 +1,31 @@
+/**
+ * Checks if `value` is the
+ * [language type](http://www.ecma-international.org/ecma-262/7.0/#sec-ecmascript-language-types)
+ * of `Object`. (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(_.noop);
+ * // => true
+ *
+ * _.isObject(null);
+ * // => false
+ */
+function isObject(value) {
+ var type = typeof value;
+ return value != null && (type == 'object' || type == 'function');
+}
+
+module.exports = isObject;
diff --git a/node_modules/lodash/isObjectLike.js b/node_modules/lodash/isObjectLike.js
new file mode 100644
index 0000000..301716b
--- /dev/null
+++ b/node_modules/lodash/isObjectLike.js
@@ -0,0 +1,29 @@
+/**
+ * Checks if `value` is object-like. A value is object-like if it's not `null`
+ * and has a `typeof` result of "object".
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ * @example
+ *
+ * _.isObjectLike({});
+ * // => true
+ *
+ * _.isObjectLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isObjectLike(_.noop);
+ * // => false
+ *
+ * _.isObjectLike(null);
+ * // => false
+ */
+function isObjectLike(value) {
+ return value != null && typeof value == 'object';
+}
+
+module.exports = isObjectLike;
diff --git a/node_modules/lodash/isPlainObject.js b/node_modules/lodash/isPlainObject.js
new file mode 100644
index 0000000..2387373
--- /dev/null
+++ b/node_modules/lodash/isPlainObject.js
@@ -0,0 +1,62 @@
+var baseGetTag = require('./_baseGetTag'),
+ getPrototype = require('./_getPrototype'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var objectTag = '[object Object]';
+
+/** Used for built-in method references. */
+var funcProto = Function.prototype,
+ objectProto = Object.prototype;
+
+/** Used to resolve the decompiled source of functions. */
+var funcToString = funcProto.toString;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Used to infer the `Object` constructor. */
+var objectCtorString = funcToString.call(Object);
+
+/**
+ * Checks if `value` is a plain object, that is, an object created by the
+ * `Object` constructor or one with a `[[Prototype]]` of `null`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.8.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a plain object, else `false`.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * _.isPlainObject(new Foo);
+ * // => false
+ *
+ * _.isPlainObject([1, 2, 3]);
+ * // => false
+ *
+ * _.isPlainObject({ 'x': 0, 'y': 0 });
+ * // => true
+ *
+ * _.isPlainObject(Object.create(null));
+ * // => true
+ */
+function isPlainObject(value) {
+ if (!isObjectLike(value) || baseGetTag(value) != objectTag) {
+ return false;
+ }
+ var proto = getPrototype(value);
+ if (proto === null) {
+ return true;
+ }
+ var Ctor = hasOwnProperty.call(proto, 'constructor') && proto.constructor;
+ return typeof Ctor == 'function' && Ctor instanceof Ctor &&
+ funcToString.call(Ctor) == objectCtorString;
+}
+
+module.exports = isPlainObject;
diff --git a/node_modules/lodash/isRegExp.js b/node_modules/lodash/isRegExp.js
new file mode 100644
index 0000000..76c9b6e
--- /dev/null
+++ b/node_modules/lodash/isRegExp.js
@@ -0,0 +1,27 @@
+var baseIsRegExp = require('./_baseIsRegExp'),
+ baseUnary = require('./_baseUnary'),
+ nodeUtil = require('./_nodeUtil');
+
+/* Node.js helper references. */
+var nodeIsRegExp = nodeUtil && nodeUtil.isRegExp;
+
+/**
+ * Checks if `value` is classified as a `RegExp` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a regexp, else `false`.
+ * @example
+ *
+ * _.isRegExp(/abc/);
+ * // => true
+ *
+ * _.isRegExp('/abc/');
+ * // => false
+ */
+var isRegExp = nodeIsRegExp ? baseUnary(nodeIsRegExp) : baseIsRegExp;
+
+module.exports = isRegExp;
diff --git a/node_modules/lodash/isSafeInteger.js b/node_modules/lodash/isSafeInteger.js
new file mode 100644
index 0000000..2a48526
--- /dev/null
+++ b/node_modules/lodash/isSafeInteger.js
@@ -0,0 +1,37 @@
+var isInteger = require('./isInteger');
+
+/** Used as references for various `Number` constants. */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/**
+ * Checks if `value` is a safe integer. An integer is safe if it's an IEEE-754
+ * double precision number which isn't the result of a rounded unsafe integer.
+ *
+ * **Note:** This method is based on
+ * [`Number.isSafeInteger`](https://mdn.io/Number/isSafeInteger).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a safe integer, else `false`.
+ * @example
+ *
+ * _.isSafeInteger(3);
+ * // => true
+ *
+ * _.isSafeInteger(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isSafeInteger(Infinity);
+ * // => false
+ *
+ * _.isSafeInteger('3');
+ * // => false
+ */
+function isSafeInteger(value) {
+ return isInteger(value) && value >= -MAX_SAFE_INTEGER && value <= MAX_SAFE_INTEGER;
+}
+
+module.exports = isSafeInteger;
diff --git a/node_modules/lodash/isSet.js b/node_modules/lodash/isSet.js
new file mode 100644
index 0000000..ab88bdf
--- /dev/null
+++ b/node_modules/lodash/isSet.js
@@ -0,0 +1,27 @@
+var baseIsSet = require('./_baseIsSet'),
+ baseUnary = require('./_baseUnary'),
+ nodeUtil = require('./_nodeUtil');
+
+/* Node.js helper references. */
+var nodeIsSet = nodeUtil && nodeUtil.isSet;
+
+/**
+ * Checks if `value` is classified as a `Set` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a set, else `false`.
+ * @example
+ *
+ * _.isSet(new Set);
+ * // => true
+ *
+ * _.isSet(new WeakSet);
+ * // => false
+ */
+var isSet = nodeIsSet ? baseUnary(nodeIsSet) : baseIsSet;
+
+module.exports = isSet;
diff --git a/node_modules/lodash/isString.js b/node_modules/lodash/isString.js
new file mode 100644
index 0000000..627eb9c
--- /dev/null
+++ b/node_modules/lodash/isString.js
@@ -0,0 +1,30 @@
+var baseGetTag = require('./_baseGetTag'),
+ isArray = require('./isArray'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var stringTag = '[object String]';
+
+/**
+ * Checks if `value` is classified as a `String` primitive or object.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a string, else `false`.
+ * @example
+ *
+ * _.isString('abc');
+ * // => true
+ *
+ * _.isString(1);
+ * // => false
+ */
+function isString(value) {
+ return typeof value == 'string' ||
+ (!isArray(value) && isObjectLike(value) && baseGetTag(value) == stringTag);
+}
+
+module.exports = isString;
diff --git a/node_modules/lodash/isSymbol.js b/node_modules/lodash/isSymbol.js
new file mode 100644
index 0000000..dfb60b9
--- /dev/null
+++ b/node_modules/lodash/isSymbol.js
@@ -0,0 +1,29 @@
+var baseGetTag = require('./_baseGetTag'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var symbolTag = '[object Symbol]';
+
+/**
+ * Checks if `value` is classified as a `Symbol` primitive or object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a symbol, else `false`.
+ * @example
+ *
+ * _.isSymbol(Symbol.iterator);
+ * // => true
+ *
+ * _.isSymbol('abc');
+ * // => false
+ */
+function isSymbol(value) {
+ return typeof value == 'symbol' ||
+ (isObjectLike(value) && baseGetTag(value) == symbolTag);
+}
+
+module.exports = isSymbol;
diff --git a/node_modules/lodash/isTypedArray.js b/node_modules/lodash/isTypedArray.js
new file mode 100644
index 0000000..da3f8dd
--- /dev/null
+++ b/node_modules/lodash/isTypedArray.js
@@ -0,0 +1,27 @@
+var baseIsTypedArray = require('./_baseIsTypedArray'),
+ baseUnary = require('./_baseUnary'),
+ nodeUtil = require('./_nodeUtil');
+
+/* Node.js helper references. */
+var nodeIsTypedArray = nodeUtil && nodeUtil.isTypedArray;
+
+/**
+ * Checks if `value` is classified as a typed array.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a typed array, else `false`.
+ * @example
+ *
+ * _.isTypedArray(new Uint8Array);
+ * // => true
+ *
+ * _.isTypedArray([]);
+ * // => false
+ */
+var isTypedArray = nodeIsTypedArray ? baseUnary(nodeIsTypedArray) : baseIsTypedArray;
+
+module.exports = isTypedArray;
diff --git a/node_modules/lodash/isUndefined.js b/node_modules/lodash/isUndefined.js
new file mode 100644
index 0000000..377d121
--- /dev/null
+++ b/node_modules/lodash/isUndefined.js
@@ -0,0 +1,22 @@
+/**
+ * Checks if `value` is `undefined`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `undefined`, else `false`.
+ * @example
+ *
+ * _.isUndefined(void 0);
+ * // => true
+ *
+ * _.isUndefined(null);
+ * // => false
+ */
+function isUndefined(value) {
+ return value === undefined;
+}
+
+module.exports = isUndefined;
diff --git a/node_modules/lodash/isWeakMap.js b/node_modules/lodash/isWeakMap.js
new file mode 100644
index 0000000..8d36f66
--- /dev/null
+++ b/node_modules/lodash/isWeakMap.js
@@ -0,0 +1,28 @@
+var getTag = require('./_getTag'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var weakMapTag = '[object WeakMap]';
+
+/**
+ * Checks if `value` is classified as a `WeakMap` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a weak map, else `false`.
+ * @example
+ *
+ * _.isWeakMap(new WeakMap);
+ * // => true
+ *
+ * _.isWeakMap(new Map);
+ * // => false
+ */
+function isWeakMap(value) {
+ return isObjectLike(value) && getTag(value) == weakMapTag;
+}
+
+module.exports = isWeakMap;
diff --git a/node_modules/lodash/isWeakSet.js b/node_modules/lodash/isWeakSet.js
new file mode 100644
index 0000000..e628b26
--- /dev/null
+++ b/node_modules/lodash/isWeakSet.js
@@ -0,0 +1,28 @@
+var baseGetTag = require('./_baseGetTag'),
+ isObjectLike = require('./isObjectLike');
+
+/** `Object#toString` result references. */
+var weakSetTag = '[object WeakSet]';
+
+/**
+ * Checks if `value` is classified as a `WeakSet` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a weak set, else `false`.
+ * @example
+ *
+ * _.isWeakSet(new WeakSet);
+ * // => true
+ *
+ * _.isWeakSet(new Set);
+ * // => false
+ */
+function isWeakSet(value) {
+ return isObjectLike(value) && baseGetTag(value) == weakSetTag;
+}
+
+module.exports = isWeakSet;
diff --git a/node_modules/lodash/iteratee.js b/node_modules/lodash/iteratee.js
new file mode 100644
index 0000000..61b73a8
--- /dev/null
+++ b/node_modules/lodash/iteratee.js
@@ -0,0 +1,53 @@
+var baseClone = require('./_baseClone'),
+ baseIteratee = require('./_baseIteratee');
+
+/** Used to compose bitmasks for cloning. */
+var CLONE_DEEP_FLAG = 1;
+
+/**
+ * Creates a function that invokes `func` with the arguments of the created
+ * function. If `func` is a property name, the created function returns the
+ * property value for a given element. If `func` is an array or object, the
+ * created function returns `true` for elements that contain the equivalent
+ * source properties, otherwise it returns `false`.
+ *
+ * @static
+ * @since 4.0.0
+ * @memberOf _
+ * @category Util
+ * @param {*} [func=_.identity] The value to convert to a callback.
+ * @returns {Function} Returns the callback.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.filter(users, _.iteratee({ 'user': 'barney', 'active': true }));
+ * // => [{ 'user': 'barney', 'age': 36, 'active': true }]
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.filter(users, _.iteratee(['user', 'fred']));
+ * // => [{ 'user': 'fred', 'age': 40 }]
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.map(users, _.iteratee('user'));
+ * // => ['barney', 'fred']
+ *
+ * // Create custom iteratee shorthands.
+ * _.iteratee = _.wrap(_.iteratee, function(iteratee, func) {
+ * return !_.isRegExp(func) ? iteratee(func) : function(string) {
+ * return func.test(string);
+ * };
+ * });
+ *
+ * _.filter(['abc', 'def'], /ef/);
+ * // => ['def']
+ */
+function iteratee(func) {
+ return baseIteratee(typeof func == 'function' ? func : baseClone(func, CLONE_DEEP_FLAG));
+}
+
+module.exports = iteratee;
diff --git a/node_modules/lodash/join.js b/node_modules/lodash/join.js
new file mode 100644
index 0000000..45de079
--- /dev/null
+++ b/node_modules/lodash/join.js
@@ -0,0 +1,26 @@
+/** Used for built-in method references. */
+var arrayProto = Array.prototype;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeJoin = arrayProto.join;
+
+/**
+ * Converts all elements in `array` into a string separated by `separator`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to convert.
+ * @param {string} [separator=','] The element separator.
+ * @returns {string} Returns the joined string.
+ * @example
+ *
+ * _.join(['a', 'b', 'c'], '~');
+ * // => 'a~b~c'
+ */
+function join(array, separator) {
+ return array == null ? '' : nativeJoin.call(array, separator);
+}
+
+module.exports = join;
diff --git a/node_modules/lodash/kebabCase.js b/node_modules/lodash/kebabCase.js
new file mode 100644
index 0000000..8a52be6
--- /dev/null
+++ b/node_modules/lodash/kebabCase.js
@@ -0,0 +1,28 @@
+var createCompounder = require('./_createCompounder');
+
+/**
+ * Converts `string` to
+ * [kebab case](https://en.wikipedia.org/wiki/Letter_case#Special_case_styles).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the kebab cased string.
+ * @example
+ *
+ * _.kebabCase('Foo Bar');
+ * // => 'foo-bar'
+ *
+ * _.kebabCase('fooBar');
+ * // => 'foo-bar'
+ *
+ * _.kebabCase('__FOO_BAR__');
+ * // => 'foo-bar'
+ */
+var kebabCase = createCompounder(function(result, word, index) {
+ return result + (index ? '-' : '') + word.toLowerCase();
+});
+
+module.exports = kebabCase;
diff --git a/node_modules/lodash/keyBy.js b/node_modules/lodash/keyBy.js
new file mode 100644
index 0000000..acc007a
--- /dev/null
+++ b/node_modules/lodash/keyBy.js
@@ -0,0 +1,36 @@
+var baseAssignValue = require('./_baseAssignValue'),
+ createAggregator = require('./_createAggregator');
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` thru `iteratee`. The corresponding value of
+ * each key is the last element responsible for generating the key. The
+ * iteratee is invoked with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The iteratee to transform keys.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * var array = [
+ * { 'dir': 'left', 'code': 97 },
+ * { 'dir': 'right', 'code': 100 }
+ * ];
+ *
+ * _.keyBy(array, function(o) {
+ * return String.fromCharCode(o.code);
+ * });
+ * // => { 'a': { 'dir': 'left', 'code': 97 }, 'd': { 'dir': 'right', 'code': 100 } }
+ *
+ * _.keyBy(array, 'dir');
+ * // => { 'left': { 'dir': 'left', 'code': 97 }, 'right': { 'dir': 'right', 'code': 100 } }
+ */
+var keyBy = createAggregator(function(result, value, key) {
+ baseAssignValue(result, key, value);
+});
+
+module.exports = keyBy;
diff --git a/node_modules/lodash/keys.js b/node_modules/lodash/keys.js
new file mode 100644
index 0000000..d143c71
--- /dev/null
+++ b/node_modules/lodash/keys.js
@@ -0,0 +1,37 @@
+var arrayLikeKeys = require('./_arrayLikeKeys'),
+ baseKeys = require('./_baseKeys'),
+ isArrayLike = require('./isArrayLike');
+
+/**
+ * Creates an array of the own enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects. See the
+ * [ES spec](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
+ * for more details.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keys(new Foo);
+ * // => ['a', 'b'] (iteration order is not guaranteed)
+ *
+ * _.keys('hi');
+ * // => ['0', '1']
+ */
+function keys(object) {
+ return isArrayLike(object) ? arrayLikeKeys(object) : baseKeys(object);
+}
+
+module.exports = keys;
diff --git a/node_modules/lodash/keysIn.js b/node_modules/lodash/keysIn.js
new file mode 100644
index 0000000..a62308f
--- /dev/null
+++ b/node_modules/lodash/keysIn.js
@@ -0,0 +1,32 @@
+var arrayLikeKeys = require('./_arrayLikeKeys'),
+ baseKeysIn = require('./_baseKeysIn'),
+ isArrayLike = require('./isArrayLike');
+
+/**
+ * Creates an array of the own and inherited enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keysIn(new Foo);
+ * // => ['a', 'b', 'c'] (iteration order is not guaranteed)
+ */
+function keysIn(object) {
+ return isArrayLike(object) ? arrayLikeKeys(object, true) : baseKeysIn(object);
+}
+
+module.exports = keysIn;
diff --git a/node_modules/lodash/lang.js b/node_modules/lodash/lang.js
new file mode 100644
index 0000000..a396216
--- /dev/null
+++ b/node_modules/lodash/lang.js
@@ -0,0 +1,58 @@
+module.exports = {
+ 'castArray': require('./castArray'),
+ 'clone': require('./clone'),
+ 'cloneDeep': require('./cloneDeep'),
+ 'cloneDeepWith': require('./cloneDeepWith'),
+ 'cloneWith': require('./cloneWith'),
+ 'conformsTo': require('./conformsTo'),
+ 'eq': require('./eq'),
+ 'gt': require('./gt'),
+ 'gte': require('./gte'),
+ 'isArguments': require('./isArguments'),
+ 'isArray': require('./isArray'),
+ 'isArrayBuffer': require('./isArrayBuffer'),
+ 'isArrayLike': require('./isArrayLike'),
+ 'isArrayLikeObject': require('./isArrayLikeObject'),
+ 'isBoolean': require('./isBoolean'),
+ 'isBuffer': require('./isBuffer'),
+ 'isDate': require('./isDate'),
+ 'isElement': require('./isElement'),
+ 'isEmpty': require('./isEmpty'),
+ 'isEqual': require('./isEqual'),
+ 'isEqualWith': require('./isEqualWith'),
+ 'isError': require('./isError'),
+ 'isFinite': require('./isFinite'),
+ 'isFunction': require('./isFunction'),
+ 'isInteger': require('./isInteger'),
+ 'isLength': require('./isLength'),
+ 'isMap': require('./isMap'),
+ 'isMatch': require('./isMatch'),
+ 'isMatchWith': require('./isMatchWith'),
+ 'isNaN': require('./isNaN'),
+ 'isNative': require('./isNative'),
+ 'isNil': require('./isNil'),
+ 'isNull': require('./isNull'),
+ 'isNumber': require('./isNumber'),
+ 'isObject': require('./isObject'),
+ 'isObjectLike': require('./isObjectLike'),
+ 'isPlainObject': require('./isPlainObject'),
+ 'isRegExp': require('./isRegExp'),
+ 'isSafeInteger': require('./isSafeInteger'),
+ 'isSet': require('./isSet'),
+ 'isString': require('./isString'),
+ 'isSymbol': require('./isSymbol'),
+ 'isTypedArray': require('./isTypedArray'),
+ 'isUndefined': require('./isUndefined'),
+ 'isWeakMap': require('./isWeakMap'),
+ 'isWeakSet': require('./isWeakSet'),
+ 'lt': require('./lt'),
+ 'lte': require('./lte'),
+ 'toArray': require('./toArray'),
+ 'toFinite': require('./toFinite'),
+ 'toInteger': require('./toInteger'),
+ 'toLength': require('./toLength'),
+ 'toNumber': require('./toNumber'),
+ 'toPlainObject': require('./toPlainObject'),
+ 'toSafeInteger': require('./toSafeInteger'),
+ 'toString': require('./toString')
+};
diff --git a/node_modules/lodash/last.js b/node_modules/lodash/last.js
new file mode 100644
index 0000000..cad1eaf
--- /dev/null
+++ b/node_modules/lodash/last.js
@@ -0,0 +1,20 @@
+/**
+ * Gets the last element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {*} Returns the last element of `array`.
+ * @example
+ *
+ * _.last([1, 2, 3]);
+ * // => 3
+ */
+function last(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? array[length - 1] : undefined;
+}
+
+module.exports = last;
diff --git a/node_modules/lodash/lastIndexOf.js b/node_modules/lodash/lastIndexOf.js
new file mode 100644
index 0000000..dabfb61
--- /dev/null
+++ b/node_modules/lodash/lastIndexOf.js
@@ -0,0 +1,46 @@
+var baseFindIndex = require('./_baseFindIndex'),
+ baseIsNaN = require('./_baseIsNaN'),
+ strictLastIndexOf = require('./_strictLastIndexOf'),
+ toInteger = require('./toInteger');
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * This method is like `_.indexOf` except that it iterates over elements of
+ * `array` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=array.length-1] The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ * @example
+ *
+ * _.lastIndexOf([1, 2, 1, 2], 2);
+ * // => 3
+ *
+ * // Search from the `fromIndex`.
+ * _.lastIndexOf([1, 2, 1, 2], 2, 2);
+ * // => 1
+ */
+function lastIndexOf(array, value, fromIndex) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return -1;
+ }
+ var index = length;
+ if (fromIndex !== undefined) {
+ index = toInteger(fromIndex);
+ index = index < 0 ? nativeMax(length + index, 0) : nativeMin(index, length - 1);
+ }
+ return value === value
+ ? strictLastIndexOf(array, value, index)
+ : baseFindIndex(array, baseIsNaN, index, true);
+}
+
+module.exports = lastIndexOf;
diff --git a/node_modules/lodash/lodash.js b/node_modules/lodash/lodash.js
new file mode 100644
index 0000000..4131e93
--- /dev/null
+++ b/node_modules/lodash/lodash.js
@@ -0,0 +1,17209 @@
+/**
+ * @license
+ * Lodash
+ * Copyright OpenJS Foundation and other contributors
+ * Released under MIT license
+ * Based on Underscore.js 1.8.3
+ * Copyright Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ */
+;(function() {
+
+ /** Used as a safe reference for `undefined` in pre-ES5 environments. */
+ var undefined;
+
+ /** Used as the semantic version number. */
+ var VERSION = '4.17.21';
+
+ /** Used as the size to enable large array optimizations. */
+ var LARGE_ARRAY_SIZE = 200;
+
+ /** Error message constants. */
+ var CORE_ERROR_TEXT = 'Unsupported core-js use. Try https://npms.io/search?q=ponyfill.',
+ FUNC_ERROR_TEXT = 'Expected a function',
+ INVALID_TEMPL_VAR_ERROR_TEXT = 'Invalid `variable` option passed into `_.template`';
+
+ /** Used to stand-in for `undefined` hash values. */
+ var HASH_UNDEFINED = '__lodash_hash_undefined__';
+
+ /** Used as the maximum memoize cache size. */
+ var MAX_MEMOIZE_SIZE = 500;
+
+ /** Used as the internal argument placeholder. */
+ var PLACEHOLDER = '__lodash_placeholder__';
+
+ /** Used to compose bitmasks for cloning. */
+ var CLONE_DEEP_FLAG = 1,
+ CLONE_FLAT_FLAG = 2,
+ CLONE_SYMBOLS_FLAG = 4;
+
+ /** Used to compose bitmasks for value comparisons. */
+ var COMPARE_PARTIAL_FLAG = 1,
+ COMPARE_UNORDERED_FLAG = 2;
+
+ /** Used to compose bitmasks for function metadata. */
+ var WRAP_BIND_FLAG = 1,
+ WRAP_BIND_KEY_FLAG = 2,
+ WRAP_CURRY_BOUND_FLAG = 4,
+ WRAP_CURRY_FLAG = 8,
+ WRAP_CURRY_RIGHT_FLAG = 16,
+ WRAP_PARTIAL_FLAG = 32,
+ WRAP_PARTIAL_RIGHT_FLAG = 64,
+ WRAP_ARY_FLAG = 128,
+ WRAP_REARG_FLAG = 256,
+ WRAP_FLIP_FLAG = 512;
+
+ /** Used as default options for `_.truncate`. */
+ var DEFAULT_TRUNC_LENGTH = 30,
+ DEFAULT_TRUNC_OMISSION = '...';
+
+ /** Used to detect hot functions by number of calls within a span of milliseconds. */
+ var HOT_COUNT = 800,
+ HOT_SPAN = 16;
+
+ /** Used to indicate the type of lazy iteratees. */
+ var LAZY_FILTER_FLAG = 1,
+ LAZY_MAP_FLAG = 2,
+ LAZY_WHILE_FLAG = 3;
+
+ /** Used as references for various `Number` constants. */
+ var INFINITY = 1 / 0,
+ MAX_SAFE_INTEGER = 9007199254740991,
+ MAX_INTEGER = 1.7976931348623157e+308,
+ NAN = 0 / 0;
+
+ /** Used as references for the maximum length and index of an array. */
+ var MAX_ARRAY_LENGTH = 4294967295,
+ MAX_ARRAY_INDEX = MAX_ARRAY_LENGTH - 1,
+ HALF_MAX_ARRAY_LENGTH = MAX_ARRAY_LENGTH >>> 1;
+
+ /** Used to associate wrap methods with their bit flags. */
+ var wrapFlags = [
+ ['ary', WRAP_ARY_FLAG],
+ ['bind', WRAP_BIND_FLAG],
+ ['bindKey', WRAP_BIND_KEY_FLAG],
+ ['curry', WRAP_CURRY_FLAG],
+ ['curryRight', WRAP_CURRY_RIGHT_FLAG],
+ ['flip', WRAP_FLIP_FLAG],
+ ['partial', WRAP_PARTIAL_FLAG],
+ ['partialRight', WRAP_PARTIAL_RIGHT_FLAG],
+ ['rearg', WRAP_REARG_FLAG]
+ ];
+
+ /** `Object#toString` result references. */
+ var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ asyncTag = '[object AsyncFunction]',
+ boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ domExcTag = '[object DOMException]',
+ errorTag = '[object Error]',
+ funcTag = '[object Function]',
+ genTag = '[object GeneratorFunction]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ nullTag = '[object Null]',
+ objectTag = '[object Object]',
+ promiseTag = '[object Promise]',
+ proxyTag = '[object Proxy]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ symbolTag = '[object Symbol]',
+ undefinedTag = '[object Undefined]',
+ weakMapTag = '[object WeakMap]',
+ weakSetTag = '[object WeakSet]';
+
+ var arrayBufferTag = '[object ArrayBuffer]',
+ dataViewTag = '[object DataView]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+ /** Used to match empty string literals in compiled template source. */
+ var reEmptyStringLeading = /\b__p \+= '';/g,
+ reEmptyStringMiddle = /\b(__p \+=) '' \+/g,
+ reEmptyStringTrailing = /(__e\(.*?\)|\b__t\)) \+\n'';/g;
+
+ /** Used to match HTML entities and HTML characters. */
+ var reEscapedHtml = /&(?:amp|lt|gt|quot|#39);/g,
+ reUnescapedHtml = /[&<>"']/g,
+ reHasEscapedHtml = RegExp(reEscapedHtml.source),
+ reHasUnescapedHtml = RegExp(reUnescapedHtml.source);
+
+ /** Used to match template delimiters. */
+ var reEscape = /<%-([\s\S]+?)%>/g,
+ reEvaluate = /<%([\s\S]+?)%>/g,
+ reInterpolate = /<%=([\s\S]+?)%>/g;
+
+ /** Used to match property names within property paths. */
+ var reIsDeepProp = /\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\\]|\\.)*?\1)\]/,
+ reIsPlainProp = /^\w*$/,
+ rePropName = /[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\\]|\\.)*?)\2)\]|(?=(?:\.|\[\])(?:\.|\[\]|$))/g;
+
+ /**
+ * Used to match `RegExp`
+ * [syntax characters](http://ecma-international.org/ecma-262/7.0/#sec-patterns).
+ */
+ var reRegExpChar = /[\\^$.*+?()[\]{}|]/g,
+ reHasRegExpChar = RegExp(reRegExpChar.source);
+
+ /** Used to match leading whitespace. */
+ var reTrimStart = /^\s+/;
+
+ /** Used to match a single whitespace character. */
+ var reWhitespace = /\s/;
+
+ /** Used to match wrap detail comments. */
+ var reWrapComment = /\{(?:\n\/\* \[wrapped with .+\] \*\/)?\n?/,
+ reWrapDetails = /\{\n\/\* \[wrapped with (.+)\] \*/,
+ reSplitDetails = /,? & /;
+
+ /** Used to match words composed of alphanumeric characters. */
+ var reAsciiWord = /[^\x00-\x2f\x3a-\x40\x5b-\x60\x7b-\x7f]+/g;
+
+ /**
+ * Used to validate the `validate` option in `_.template` variable.
+ *
+ * Forbids characters which could potentially change the meaning of the function argument definition:
+ * - "()," (modification of function parameters)
+ * - "=" (default value)
+ * - "[]{}" (destructuring of function parameters)
+ * - "/" (beginning of a comment)
+ * - whitespace
+ */
+ var reForbiddenIdentifierChars = /[()=,{}\[\]\/\s]/;
+
+ /** Used to match backslashes in property paths. */
+ var reEscapeChar = /\\(\\)?/g;
+
+ /**
+ * Used to match
+ * [ES template delimiters](http://ecma-international.org/ecma-262/7.0/#sec-template-literal-lexical-components).
+ */
+ var reEsTemplate = /\$\{([^\\}]*(?:\\.[^\\}]*)*)\}/g;
+
+ /** Used to match `RegExp` flags from their coerced string values. */
+ var reFlags = /\w*$/;
+
+ /** Used to detect bad signed hexadecimal string values. */
+ var reIsBadHex = /^[-+]0x[0-9a-f]+$/i;
+
+ /** Used to detect binary string values. */
+ var reIsBinary = /^0b[01]+$/i;
+
+ /** Used to detect host constructors (Safari). */
+ var reIsHostCtor = /^\[object .+?Constructor\]$/;
+
+ /** Used to detect octal string values. */
+ var reIsOctal = /^0o[0-7]+$/i;
+
+ /** Used to detect unsigned integer values. */
+ var reIsUint = /^(?:0|[1-9]\d*)$/;
+
+ /** Used to match Latin Unicode letters (excluding mathematical operators). */
+ var reLatin = /[\xc0-\xd6\xd8-\xf6\xf8-\xff\u0100-\u017f]/g;
+
+ /** Used to ensure capturing order of template delimiters. */
+ var reNoMatch = /($^)/;
+
+ /** Used to match unescaped characters in compiled string literals. */
+ var reUnescapedString = /['\n\r\u2028\u2029\\]/g;
+
+ /** Used to compose unicode character classes. */
+ var rsAstralRange = '\\ud800-\\udfff',
+ rsComboMarksRange = '\\u0300-\\u036f',
+ reComboHalfMarksRange = '\\ufe20-\\ufe2f',
+ rsComboSymbolsRange = '\\u20d0-\\u20ff',
+ rsComboRange = rsComboMarksRange + reComboHalfMarksRange + rsComboSymbolsRange,
+ rsDingbatRange = '\\u2700-\\u27bf',
+ rsLowerRange = 'a-z\\xdf-\\xf6\\xf8-\\xff',
+ rsMathOpRange = '\\xac\\xb1\\xd7\\xf7',
+ rsNonCharRange = '\\x00-\\x2f\\x3a-\\x40\\x5b-\\x60\\x7b-\\xbf',
+ rsPunctuationRange = '\\u2000-\\u206f',
+ rsSpaceRange = ' \\t\\x0b\\f\\xa0\\ufeff\\n\\r\\u2028\\u2029\\u1680\\u180e\\u2000\\u2001\\u2002\\u2003\\u2004\\u2005\\u2006\\u2007\\u2008\\u2009\\u200a\\u202f\\u205f\\u3000',
+ rsUpperRange = 'A-Z\\xc0-\\xd6\\xd8-\\xde',
+ rsVarRange = '\\ufe0e\\ufe0f',
+ rsBreakRange = rsMathOpRange + rsNonCharRange + rsPunctuationRange + rsSpaceRange;
+
+ /** Used to compose unicode capture groups. */
+ var rsApos = "['\u2019]",
+ rsAstral = '[' + rsAstralRange + ']',
+ rsBreak = '[' + rsBreakRange + ']',
+ rsCombo = '[' + rsComboRange + ']',
+ rsDigits = '\\d+',
+ rsDingbat = '[' + rsDingbatRange + ']',
+ rsLower = '[' + rsLowerRange + ']',
+ rsMisc = '[^' + rsAstralRange + rsBreakRange + rsDigits + rsDingbatRange + rsLowerRange + rsUpperRange + ']',
+ rsFitz = '\\ud83c[\\udffb-\\udfff]',
+ rsModifier = '(?:' + rsCombo + '|' + rsFitz + ')',
+ rsNonAstral = '[^' + rsAstralRange + ']',
+ rsRegional = '(?:\\ud83c[\\udde6-\\uddff]){2}',
+ rsSurrPair = '[\\ud800-\\udbff][\\udc00-\\udfff]',
+ rsUpper = '[' + rsUpperRange + ']',
+ rsZWJ = '\\u200d';
+
+ /** Used to compose unicode regexes. */
+ var rsMiscLower = '(?:' + rsLower + '|' + rsMisc + ')',
+ rsMiscUpper = '(?:' + rsUpper + '|' + rsMisc + ')',
+ rsOptContrLower = '(?:' + rsApos + '(?:d|ll|m|re|s|t|ve))?',
+ rsOptContrUpper = '(?:' + rsApos + '(?:D|LL|M|RE|S|T|VE))?',
+ reOptMod = rsModifier + '?',
+ rsOptVar = '[' + rsVarRange + ']?',
+ rsOptJoin = '(?:' + rsZWJ + '(?:' + [rsNonAstral, rsRegional, rsSurrPair].join('|') + ')' + rsOptVar + reOptMod + ')*',
+ rsOrdLower = '\\d*(?:1st|2nd|3rd|(?![123])\\dth)(?=\\b|[A-Z_])',
+ rsOrdUpper = '\\d*(?:1ST|2ND|3RD|(?![123])\\dTH)(?=\\b|[a-z_])',
+ rsSeq = rsOptVar + reOptMod + rsOptJoin,
+ rsEmoji = '(?:' + [rsDingbat, rsRegional, rsSurrPair].join('|') + ')' + rsSeq,
+ rsSymbol = '(?:' + [rsNonAstral + rsCombo + '?', rsCombo, rsRegional, rsSurrPair, rsAstral].join('|') + ')';
+
+ /** Used to match apostrophes. */
+ var reApos = RegExp(rsApos, 'g');
+
+ /**
+ * Used to match [combining diacritical marks](https://en.wikipedia.org/wiki/Combining_Diacritical_Marks) and
+ * [combining diacritical marks for symbols](https://en.wikipedia.org/wiki/Combining_Diacritical_Marks_for_Symbols).
+ */
+ var reComboMark = RegExp(rsCombo, 'g');
+
+ /** Used to match [string symbols](https://mathiasbynens.be/notes/javascript-unicode). */
+ var reUnicode = RegExp(rsFitz + '(?=' + rsFitz + ')|' + rsSymbol + rsSeq, 'g');
+
+ /** Used to match complex or compound words. */
+ var reUnicodeWord = RegExp([
+ rsUpper + '?' + rsLower + '+' + rsOptContrLower + '(?=' + [rsBreak, rsUpper, '$'].join('|') + ')',
+ rsMiscUpper + '+' + rsOptContrUpper + '(?=' + [rsBreak, rsUpper + rsMiscLower, '$'].join('|') + ')',
+ rsUpper + '?' + rsMiscLower + '+' + rsOptContrLower,
+ rsUpper + '+' + rsOptContrUpper,
+ rsOrdUpper,
+ rsOrdLower,
+ rsDigits,
+ rsEmoji
+ ].join('|'), 'g');
+
+ /** Used to detect strings with [zero-width joiners or code points from the astral planes](http://eev.ee/blog/2015/09/12/dark-corners-of-unicode/). */
+ var reHasUnicode = RegExp('[' + rsZWJ + rsAstralRange + rsComboRange + rsVarRange + ']');
+
+ /** Used to detect strings that need a more robust regexp to match words. */
+ var reHasUnicodeWord = /[a-z][A-Z]|[A-Z]{2}[a-z]|[0-9][a-zA-Z]|[a-zA-Z][0-9]|[^a-zA-Z0-9 ]/;
+
+ /** Used to assign default `context` object properties. */
+ var contextProps = [
+ 'Array', 'Buffer', 'DataView', 'Date', 'Error', 'Float32Array', 'Float64Array',
+ 'Function', 'Int8Array', 'Int16Array', 'Int32Array', 'Map', 'Math', 'Object',
+ 'Promise', 'RegExp', 'Set', 'String', 'Symbol', 'TypeError', 'Uint8Array',
+ 'Uint8ClampedArray', 'Uint16Array', 'Uint32Array', 'WeakMap',
+ '_', 'clearTimeout', 'isFinite', 'parseInt', 'setTimeout'
+ ];
+
+ /** Used to make template sourceURLs easier to identify. */
+ var templateCounter = -1;
+
+ /** Used to identify `toStringTag` values of typed arrays. */
+ var typedArrayTags = {};
+ typedArrayTags[float32Tag] = typedArrayTags[float64Tag] =
+ typedArrayTags[int8Tag] = typedArrayTags[int16Tag] =
+ typedArrayTags[int32Tag] = typedArrayTags[uint8Tag] =
+ typedArrayTags[uint8ClampedTag] = typedArrayTags[uint16Tag] =
+ typedArrayTags[uint32Tag] = true;
+ typedArrayTags[argsTag] = typedArrayTags[arrayTag] =
+ typedArrayTags[arrayBufferTag] = typedArrayTags[boolTag] =
+ typedArrayTags[dataViewTag] = typedArrayTags[dateTag] =
+ typedArrayTags[errorTag] = typedArrayTags[funcTag] =
+ typedArrayTags[mapTag] = typedArrayTags[numberTag] =
+ typedArrayTags[objectTag] = typedArrayTags[regexpTag] =
+ typedArrayTags[setTag] = typedArrayTags[stringTag] =
+ typedArrayTags[weakMapTag] = false;
+
+ /** Used to identify `toStringTag` values supported by `_.clone`. */
+ var cloneableTags = {};
+ cloneableTags[argsTag] = cloneableTags[arrayTag] =
+ cloneableTags[arrayBufferTag] = cloneableTags[dataViewTag] =
+ cloneableTags[boolTag] = cloneableTags[dateTag] =
+ cloneableTags[float32Tag] = cloneableTags[float64Tag] =
+ cloneableTags[int8Tag] = cloneableTags[int16Tag] =
+ cloneableTags[int32Tag] = cloneableTags[mapTag] =
+ cloneableTags[numberTag] = cloneableTags[objectTag] =
+ cloneableTags[regexpTag] = cloneableTags[setTag] =
+ cloneableTags[stringTag] = cloneableTags[symbolTag] =
+ cloneableTags[uint8Tag] = cloneableTags[uint8ClampedTag] =
+ cloneableTags[uint16Tag] = cloneableTags[uint32Tag] = true;
+ cloneableTags[errorTag] = cloneableTags[funcTag] =
+ cloneableTags[weakMapTag] = false;
+
+ /** Used to map Latin Unicode letters to basic Latin letters. */
+ var deburredLetters = {
+ // Latin-1 Supplement block.
+ '\xc0': 'A', '\xc1': 'A', '\xc2': 'A', '\xc3': 'A', '\xc4': 'A', '\xc5': 'A',
+ '\xe0': 'a', '\xe1': 'a', '\xe2': 'a', '\xe3': 'a', '\xe4': 'a', '\xe5': 'a',
+ '\xc7': 'C', '\xe7': 'c',
+ '\xd0': 'D', '\xf0': 'd',
+ '\xc8': 'E', '\xc9': 'E', '\xca': 'E', '\xcb': 'E',
+ '\xe8': 'e', '\xe9': 'e', '\xea': 'e', '\xeb': 'e',
+ '\xcc': 'I', '\xcd': 'I', '\xce': 'I', '\xcf': 'I',
+ '\xec': 'i', '\xed': 'i', '\xee': 'i', '\xef': 'i',
+ '\xd1': 'N', '\xf1': 'n',
+ '\xd2': 'O', '\xd3': 'O', '\xd4': 'O', '\xd5': 'O', '\xd6': 'O', '\xd8': 'O',
+ '\xf2': 'o', '\xf3': 'o', '\xf4': 'o', '\xf5': 'o', '\xf6': 'o', '\xf8': 'o',
+ '\xd9': 'U', '\xda': 'U', '\xdb': 'U', '\xdc': 'U',
+ '\xf9': 'u', '\xfa': 'u', '\xfb': 'u', '\xfc': 'u',
+ '\xdd': 'Y', '\xfd': 'y', '\xff': 'y',
+ '\xc6': 'Ae', '\xe6': 'ae',
+ '\xde': 'Th', '\xfe': 'th',
+ '\xdf': 'ss',
+ // Latin Extended-A block.
+ '\u0100': 'A', '\u0102': 'A', '\u0104': 'A',
+ '\u0101': 'a', '\u0103': 'a', '\u0105': 'a',
+ '\u0106': 'C', '\u0108': 'C', '\u010a': 'C', '\u010c': 'C',
+ '\u0107': 'c', '\u0109': 'c', '\u010b': 'c', '\u010d': 'c',
+ '\u010e': 'D', '\u0110': 'D', '\u010f': 'd', '\u0111': 'd',
+ '\u0112': 'E', '\u0114': 'E', '\u0116': 'E', '\u0118': 'E', '\u011a': 'E',
+ '\u0113': 'e', '\u0115': 'e', '\u0117': 'e', '\u0119': 'e', '\u011b': 'e',
+ '\u011c': 'G', '\u011e': 'G', '\u0120': 'G', '\u0122': 'G',
+ '\u011d': 'g', '\u011f': 'g', '\u0121': 'g', '\u0123': 'g',
+ '\u0124': 'H', '\u0126': 'H', '\u0125': 'h', '\u0127': 'h',
+ '\u0128': 'I', '\u012a': 'I', '\u012c': 'I', '\u012e': 'I', '\u0130': 'I',
+ '\u0129': 'i', '\u012b': 'i', '\u012d': 'i', '\u012f': 'i', '\u0131': 'i',
+ '\u0134': 'J', '\u0135': 'j',
+ '\u0136': 'K', '\u0137': 'k', '\u0138': 'k',
+ '\u0139': 'L', '\u013b': 'L', '\u013d': 'L', '\u013f': 'L', '\u0141': 'L',
+ '\u013a': 'l', '\u013c': 'l', '\u013e': 'l', '\u0140': 'l', '\u0142': 'l',
+ '\u0143': 'N', '\u0145': 'N', '\u0147': 'N', '\u014a': 'N',
+ '\u0144': 'n', '\u0146': 'n', '\u0148': 'n', '\u014b': 'n',
+ '\u014c': 'O', '\u014e': 'O', '\u0150': 'O',
+ '\u014d': 'o', '\u014f': 'o', '\u0151': 'o',
+ '\u0154': 'R', '\u0156': 'R', '\u0158': 'R',
+ '\u0155': 'r', '\u0157': 'r', '\u0159': 'r',
+ '\u015a': 'S', '\u015c': 'S', '\u015e': 'S', '\u0160': 'S',
+ '\u015b': 's', '\u015d': 's', '\u015f': 's', '\u0161': 's',
+ '\u0162': 'T', '\u0164': 'T', '\u0166': 'T',
+ '\u0163': 't', '\u0165': 't', '\u0167': 't',
+ '\u0168': 'U', '\u016a': 'U', '\u016c': 'U', '\u016e': 'U', '\u0170': 'U', '\u0172': 'U',
+ '\u0169': 'u', '\u016b': 'u', '\u016d': 'u', '\u016f': 'u', '\u0171': 'u', '\u0173': 'u',
+ '\u0174': 'W', '\u0175': 'w',
+ '\u0176': 'Y', '\u0177': 'y', '\u0178': 'Y',
+ '\u0179': 'Z', '\u017b': 'Z', '\u017d': 'Z',
+ '\u017a': 'z', '\u017c': 'z', '\u017e': 'z',
+ '\u0132': 'IJ', '\u0133': 'ij',
+ '\u0152': 'Oe', '\u0153': 'oe',
+ '\u0149': "'n", '\u017f': 's'
+ };
+
+ /** Used to map characters to HTML entities. */
+ var htmlEscapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+ };
+
+ /** Used to map HTML entities to characters. */
+ var htmlUnescapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ ''': "'"
+ };
+
+ /** Used to escape characters for inclusion in compiled string literals. */
+ var stringEscapes = {
+ '\\': '\\',
+ "'": "'",
+ '\n': 'n',
+ '\r': 'r',
+ '\u2028': 'u2028',
+ '\u2029': 'u2029'
+ };
+
+ /** Built-in method references without a dependency on `root`. */
+ var freeParseFloat = parseFloat,
+ freeParseInt = parseInt;
+
+ /** Detect free variable `global` from Node.js. */
+ var freeGlobal = typeof global == 'object' && global && global.Object === Object && global;
+
+ /** Detect free variable `self`. */
+ var freeSelf = typeof self == 'object' && self && self.Object === Object && self;
+
+ /** Used as a reference to the global object. */
+ var root = freeGlobal || freeSelf || Function('return this')();
+
+ /** Detect free variable `exports`. */
+ var freeExports = typeof exports == 'object' && exports && !exports.nodeType && exports;
+
+ /** Detect free variable `module`. */
+ var freeModule = freeExports && typeof module == 'object' && module && !module.nodeType && module;
+
+ /** Detect the popular CommonJS extension `module.exports`. */
+ var moduleExports = freeModule && freeModule.exports === freeExports;
+
+ /** Detect free variable `process` from Node.js. */
+ var freeProcess = moduleExports && freeGlobal.process;
+
+ /** Used to access faster Node.js helpers. */
+ var nodeUtil = (function() {
+ try {
+ // Use `util.types` for Node.js 10+.
+ var types = freeModule && freeModule.require && freeModule.require('util').types;
+
+ if (types) {
+ return types;
+ }
+
+ // Legacy `process.binding('util')` for Node.js < 10.
+ return freeProcess && freeProcess.binding && freeProcess.binding('util');
+ } catch (e) {}
+ }());
+
+ /* Node.js helper references. */
+ var nodeIsArrayBuffer = nodeUtil && nodeUtil.isArrayBuffer,
+ nodeIsDate = nodeUtil && nodeUtil.isDate,
+ nodeIsMap = nodeUtil && nodeUtil.isMap,
+ nodeIsRegExp = nodeUtil && nodeUtil.isRegExp,
+ nodeIsSet = nodeUtil && nodeUtil.isSet,
+ nodeIsTypedArray = nodeUtil && nodeUtil.isTypedArray;
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * A faster alternative to `Function#apply`, this function invokes `func`
+ * with the `this` binding of `thisArg` and the arguments of `args`.
+ *
+ * @private
+ * @param {Function} func The function to invoke.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {Array} args The arguments to invoke `func` with.
+ * @returns {*} Returns the result of `func`.
+ */
+ function apply(func, thisArg, args) {
+ switch (args.length) {
+ case 0: return func.call(thisArg);
+ case 1: return func.call(thisArg, args[0]);
+ case 2: return func.call(thisArg, args[0], args[1]);
+ case 3: return func.call(thisArg, args[0], args[1], args[2]);
+ }
+ return func.apply(thisArg, args);
+ }
+
+ /**
+ * A specialized version of `baseAggregator` for arrays.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} setter The function to set `accumulator` values.
+ * @param {Function} iteratee The iteratee to transform keys.
+ * @param {Object} accumulator The initial aggregated object.
+ * @returns {Function} Returns `accumulator`.
+ */
+ function arrayAggregator(array, setter, iteratee, accumulator) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ while (++index < length) {
+ var value = array[index];
+ setter(accumulator, value, iteratee(value), array);
+ }
+ return accumulator;
+ }
+
+ /**
+ * A specialized version of `_.forEach` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns `array`.
+ */
+ function arrayEach(array, iteratee) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ while (++index < length) {
+ if (iteratee(array[index], index, array) === false) {
+ break;
+ }
+ }
+ return array;
+ }
+
+ /**
+ * A specialized version of `_.forEachRight` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns `array`.
+ */
+ function arrayEachRight(array, iteratee) {
+ var length = array == null ? 0 : array.length;
+
+ while (length--) {
+ if (iteratee(array[length], length, array) === false) {
+ break;
+ }
+ }
+ return array;
+ }
+
+ /**
+ * A specialized version of `_.every` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ */
+ function arrayEvery(array, predicate) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ while (++index < length) {
+ if (!predicate(array[index], index, array)) {
+ return false;
+ }
+ }
+ return true;
+ }
+
+ /**
+ * A specialized version of `_.filter` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ */
+ function arrayFilter(array, predicate) {
+ var index = -1,
+ length = array == null ? 0 : array.length,
+ resIndex = 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (predicate(value, index, array)) {
+ result[resIndex++] = value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * A specialized version of `_.includes` for arrays without support for
+ * specifying an index to search from.
+ *
+ * @private
+ * @param {Array} [array] The array to inspect.
+ * @param {*} target The value to search for.
+ * @returns {boolean} Returns `true` if `target` is found, else `false`.
+ */
+ function arrayIncludes(array, value) {
+ var length = array == null ? 0 : array.length;
+ return !!length && baseIndexOf(array, value, 0) > -1;
+ }
+
+ /**
+ * This function is like `arrayIncludes` except that it accepts a comparator.
+ *
+ * @private
+ * @param {Array} [array] The array to inspect.
+ * @param {*} target The value to search for.
+ * @param {Function} comparator The comparator invoked per element.
+ * @returns {boolean} Returns `true` if `target` is found, else `false`.
+ */
+ function arrayIncludesWith(array, value, comparator) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ while (++index < length) {
+ if (comparator(value, array[index])) {
+ return true;
+ }
+ }
+ return false;
+ }
+
+ /**
+ * A specialized version of `_.map` for arrays without support for iteratee
+ * shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ */
+ function arrayMap(array, iteratee) {
+ var index = -1,
+ length = array == null ? 0 : array.length,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = iteratee(array[index], index, array);
+ }
+ return result;
+ }
+
+ /**
+ * Appends the elements of `values` to `array`.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to append.
+ * @returns {Array} Returns `array`.
+ */
+ function arrayPush(array, values) {
+ var index = -1,
+ length = values.length,
+ offset = array.length;
+
+ while (++index < length) {
+ array[offset + index] = values[index];
+ }
+ return array;
+ }
+
+ /**
+ * A specialized version of `_.reduce` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {boolean} [initAccum] Specify using the first element of `array` as
+ * the initial value.
+ * @returns {*} Returns the accumulated value.
+ */
+ function arrayReduce(array, iteratee, accumulator, initAccum) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ if (initAccum && length) {
+ accumulator = array[++index];
+ }
+ while (++index < length) {
+ accumulator = iteratee(accumulator, array[index], index, array);
+ }
+ return accumulator;
+ }
+
+ /**
+ * A specialized version of `_.reduceRight` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {boolean} [initAccum] Specify using the last element of `array` as
+ * the initial value.
+ * @returns {*} Returns the accumulated value.
+ */
+ function arrayReduceRight(array, iteratee, accumulator, initAccum) {
+ var length = array == null ? 0 : array.length;
+ if (initAccum && length) {
+ accumulator = array[--length];
+ }
+ while (length--) {
+ accumulator = iteratee(accumulator, array[length], length, array);
+ }
+ return accumulator;
+ }
+
+ /**
+ * A specialized version of `_.some` for arrays without support for iteratee
+ * shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ */
+ function arraySome(array, predicate) {
+ var index = -1,
+ length = array == null ? 0 : array.length;
+
+ while (++index < length) {
+ if (predicate(array[index], index, array)) {
+ return true;
+ }
+ }
+ return false;
+ }
+
+ /**
+ * Gets the size of an ASCII `string`.
+ *
+ * @private
+ * @param {string} string The string inspect.
+ * @returns {number} Returns the string size.
+ */
+ var asciiSize = baseProperty('length');
+
+ /**
+ * Converts an ASCII `string` to an array.
+ *
+ * @private
+ * @param {string} string The string to convert.
+ * @returns {Array} Returns the converted array.
+ */
+ function asciiToArray(string) {
+ return string.split('');
+ }
+
+ /**
+ * Splits an ASCII `string` into an array of its words.
+ *
+ * @private
+ * @param {string} The string to inspect.
+ * @returns {Array} Returns the words of `string`.
+ */
+ function asciiWords(string) {
+ return string.match(reAsciiWord) || [];
+ }
+
+ /**
+ * The base implementation of methods like `_.findKey` and `_.findLastKey`,
+ * without support for iteratee shorthands, which iterates over `collection`
+ * using `eachFunc`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to inspect.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {Function} eachFunc The function to iterate over `collection`.
+ * @returns {*} Returns the found element or its key, else `undefined`.
+ */
+ function baseFindKey(collection, predicate, eachFunc) {
+ var result;
+ eachFunc(collection, function(value, key, collection) {
+ if (predicate(value, key, collection)) {
+ result = key;
+ return false;
+ }
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.findIndex` and `_.findLastIndex` without
+ * support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {number} fromIndex The index to search from.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+ function baseFindIndex(array, predicate, fromIndex, fromRight) {
+ var length = array.length,
+ index = fromIndex + (fromRight ? 1 : -1);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ if (predicate(array[index], index, array)) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * The base implementation of `_.indexOf` without `fromIndex` bounds checks.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} fromIndex The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+ function baseIndexOf(array, value, fromIndex) {
+ return value === value
+ ? strictIndexOf(array, value, fromIndex)
+ : baseFindIndex(array, baseIsNaN, fromIndex);
+ }
+
+ /**
+ * This function is like `baseIndexOf` except that it accepts a comparator.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} fromIndex The index to search from.
+ * @param {Function} comparator The comparator invoked per element.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+ function baseIndexOfWith(array, value, fromIndex, comparator) {
+ var index = fromIndex - 1,
+ length = array.length;
+
+ while (++index < length) {
+ if (comparator(array[index], value)) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * The base implementation of `_.isNaN` without support for number objects.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `NaN`, else `false`.
+ */
+ function baseIsNaN(value) {
+ return value !== value;
+ }
+
+ /**
+ * The base implementation of `_.mean` and `_.meanBy` without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {number} Returns the mean.
+ */
+ function baseMean(array, iteratee) {
+ var length = array == null ? 0 : array.length;
+ return length ? (baseSum(array, iteratee) / length) : NAN;
+ }
+
+ /**
+ * The base implementation of `_.property` without support for deep paths.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @returns {Function} Returns the new accessor function.
+ */
+ function baseProperty(key) {
+ return function(object) {
+ return object == null ? undefined : object[key];
+ };
+ }
+
+ /**
+ * The base implementation of `_.propertyOf` without support for deep paths.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Function} Returns the new accessor function.
+ */
+ function basePropertyOf(object) {
+ return function(key) {
+ return object == null ? undefined : object[key];
+ };
+ }
+
+ /**
+ * The base implementation of `_.reduce` and `_.reduceRight`, without support
+ * for iteratee shorthands, which iterates over `collection` using `eachFunc`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} accumulator The initial value.
+ * @param {boolean} initAccum Specify using the first or last element of
+ * `collection` as the initial value.
+ * @param {Function} eachFunc The function to iterate over `collection`.
+ * @returns {*} Returns the accumulated value.
+ */
+ function baseReduce(collection, iteratee, accumulator, initAccum, eachFunc) {
+ eachFunc(collection, function(value, index, collection) {
+ accumulator = initAccum
+ ? (initAccum = false, value)
+ : iteratee(accumulator, value, index, collection);
+ });
+ return accumulator;
+ }
+
+ /**
+ * The base implementation of `_.sortBy` which uses `comparer` to define the
+ * sort order of `array` and replaces criteria objects with their corresponding
+ * values.
+ *
+ * @private
+ * @param {Array} array The array to sort.
+ * @param {Function} comparer The function to define sort order.
+ * @returns {Array} Returns `array`.
+ */
+ function baseSortBy(array, comparer) {
+ var length = array.length;
+
+ array.sort(comparer);
+ while (length--) {
+ array[length] = array[length].value;
+ }
+ return array;
+ }
+
+ /**
+ * The base implementation of `_.sum` and `_.sumBy` without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {number} Returns the sum.
+ */
+ function baseSum(array, iteratee) {
+ var result,
+ index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ var current = iteratee(array[index]);
+ if (current !== undefined) {
+ result = result === undefined ? current : (result + current);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.times` without support for iteratee shorthands
+ * or max array length checks.
+ *
+ * @private
+ * @param {number} n The number of times to invoke `iteratee`.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the array of results.
+ */
+ function baseTimes(n, iteratee) {
+ var index = -1,
+ result = Array(n);
+
+ while (++index < n) {
+ result[index] = iteratee(index);
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.toPairs` and `_.toPairsIn` which creates an array
+ * of key-value pairs for `object` corresponding to the property names of `props`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} props The property names to get values for.
+ * @returns {Object} Returns the key-value pairs.
+ */
+ function baseToPairs(object, props) {
+ return arrayMap(props, function(key) {
+ return [key, object[key]];
+ });
+ }
+
+ /**
+ * The base implementation of `_.trim`.
+ *
+ * @private
+ * @param {string} string The string to trim.
+ * @returns {string} Returns the trimmed string.
+ */
+ function baseTrim(string) {
+ return string
+ ? string.slice(0, trimmedEndIndex(string) + 1).replace(reTrimStart, '')
+ : string;
+ }
+
+ /**
+ * The base implementation of `_.unary` without support for storing metadata.
+ *
+ * @private
+ * @param {Function} func The function to cap arguments for.
+ * @returns {Function} Returns the new capped function.
+ */
+ function baseUnary(func) {
+ return function(value) {
+ return func(value);
+ };
+ }
+
+ /**
+ * The base implementation of `_.values` and `_.valuesIn` which creates an
+ * array of `object` property values corresponding to the property names
+ * of `props`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} props The property names to get values for.
+ * @returns {Object} Returns the array of property values.
+ */
+ function baseValues(object, props) {
+ return arrayMap(props, function(key) {
+ return object[key];
+ });
+ }
+
+ /**
+ * Checks if a `cache` value for `key` exists.
+ *
+ * @private
+ * @param {Object} cache The cache to query.
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+ function cacheHas(cache, key) {
+ return cache.has(key);
+ }
+
+ /**
+ * Used by `_.trim` and `_.trimStart` to get the index of the first string symbol
+ * that is not found in the character symbols.
+ *
+ * @private
+ * @param {Array} strSymbols The string symbols to inspect.
+ * @param {Array} chrSymbols The character symbols to find.
+ * @returns {number} Returns the index of the first unmatched string symbol.
+ */
+ function charsStartIndex(strSymbols, chrSymbols) {
+ var index = -1,
+ length = strSymbols.length;
+
+ while (++index < length && baseIndexOf(chrSymbols, strSymbols[index], 0) > -1) {}
+ return index;
+ }
+
+ /**
+ * Used by `_.trim` and `_.trimEnd` to get the index of the last string symbol
+ * that is not found in the character symbols.
+ *
+ * @private
+ * @param {Array} strSymbols The string symbols to inspect.
+ * @param {Array} chrSymbols The character symbols to find.
+ * @returns {number} Returns the index of the last unmatched string symbol.
+ */
+ function charsEndIndex(strSymbols, chrSymbols) {
+ var index = strSymbols.length;
+
+ while (index-- && baseIndexOf(chrSymbols, strSymbols[index], 0) > -1) {}
+ return index;
+ }
+
+ /**
+ * Gets the number of `placeholder` occurrences in `array`.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} placeholder The placeholder to search for.
+ * @returns {number} Returns the placeholder count.
+ */
+ function countHolders(array, placeholder) {
+ var length = array.length,
+ result = 0;
+
+ while (length--) {
+ if (array[length] === placeholder) {
+ ++result;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Used by `_.deburr` to convert Latin-1 Supplement and Latin Extended-A
+ * letters to basic Latin letters.
+ *
+ * @private
+ * @param {string} letter The matched letter to deburr.
+ * @returns {string} Returns the deburred letter.
+ */
+ var deburrLetter = basePropertyOf(deburredLetters);
+
+ /**
+ * Used by `_.escape` to convert characters to HTML entities.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+ var escapeHtmlChar = basePropertyOf(htmlEscapes);
+
+ /**
+ * Used by `_.template` to escape characters for inclusion in compiled string literals.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+ function escapeStringChar(chr) {
+ return '\\' + stringEscapes[chr];
+ }
+
+ /**
+ * Gets the value at `key` of `object`.
+ *
+ * @private
+ * @param {Object} [object] The object to query.
+ * @param {string} key The key of the property to get.
+ * @returns {*} Returns the property value.
+ */
+ function getValue(object, key) {
+ return object == null ? undefined : object[key];
+ }
+
+ /**
+ * Checks if `string` contains Unicode symbols.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {boolean} Returns `true` if a symbol is found, else `false`.
+ */
+ function hasUnicode(string) {
+ return reHasUnicode.test(string);
+ }
+
+ /**
+ * Checks if `string` contains a word composed of Unicode symbols.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {boolean} Returns `true` if a word is found, else `false`.
+ */
+ function hasUnicodeWord(string) {
+ return reHasUnicodeWord.test(string);
+ }
+
+ /**
+ * Converts `iterator` to an array.
+ *
+ * @private
+ * @param {Object} iterator The iterator to convert.
+ * @returns {Array} Returns the converted array.
+ */
+ function iteratorToArray(iterator) {
+ var data,
+ result = [];
+
+ while (!(data = iterator.next()).done) {
+ result.push(data.value);
+ }
+ return result;
+ }
+
+ /**
+ * Converts `map` to its key-value pairs.
+ *
+ * @private
+ * @param {Object} map The map to convert.
+ * @returns {Array} Returns the key-value pairs.
+ */
+ function mapToArray(map) {
+ var index = -1,
+ result = Array(map.size);
+
+ map.forEach(function(value, key) {
+ result[++index] = [key, value];
+ });
+ return result;
+ }
+
+ /**
+ * Creates a unary function that invokes `func` with its argument transformed.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {Function} transform The argument transform.
+ * @returns {Function} Returns the new function.
+ */
+ function overArg(func, transform) {
+ return function(arg) {
+ return func(transform(arg));
+ };
+ }
+
+ /**
+ * Replaces all `placeholder` elements in `array` with an internal placeholder
+ * and returns an array of their indexes.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {*} placeholder The placeholder to replace.
+ * @returns {Array} Returns the new array of placeholder indexes.
+ */
+ function replaceHolders(array, placeholder) {
+ var index = -1,
+ length = array.length,
+ resIndex = 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (value === placeholder || value === PLACEHOLDER) {
+ array[index] = PLACEHOLDER;
+ result[resIndex++] = index;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Converts `set` to an array of its values.
+ *
+ * @private
+ * @param {Object} set The set to convert.
+ * @returns {Array} Returns the values.
+ */
+ function setToArray(set) {
+ var index = -1,
+ result = Array(set.size);
+
+ set.forEach(function(value) {
+ result[++index] = value;
+ });
+ return result;
+ }
+
+ /**
+ * Converts `set` to its value-value pairs.
+ *
+ * @private
+ * @param {Object} set The set to convert.
+ * @returns {Array} Returns the value-value pairs.
+ */
+ function setToPairs(set) {
+ var index = -1,
+ result = Array(set.size);
+
+ set.forEach(function(value) {
+ result[++index] = [value, value];
+ });
+ return result;
+ }
+
+ /**
+ * A specialized version of `_.indexOf` which performs strict equality
+ * comparisons of values, i.e. `===`.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} fromIndex The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+ function strictIndexOf(array, value, fromIndex) {
+ var index = fromIndex - 1,
+ length = array.length;
+
+ while (++index < length) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * A specialized version of `_.lastIndexOf` which performs strict equality
+ * comparisons of values, i.e. `===`.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} fromIndex The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+ function strictLastIndexOf(array, value, fromIndex) {
+ var index = fromIndex + 1;
+ while (index--) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return index;
+ }
+
+ /**
+ * Gets the number of symbols in `string`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {number} Returns the string size.
+ */
+ function stringSize(string) {
+ return hasUnicode(string)
+ ? unicodeSize(string)
+ : asciiSize(string);
+ }
+
+ /**
+ * Converts `string` to an array.
+ *
+ * @private
+ * @param {string} string The string to convert.
+ * @returns {Array} Returns the converted array.
+ */
+ function stringToArray(string) {
+ return hasUnicode(string)
+ ? unicodeToArray(string)
+ : asciiToArray(string);
+ }
+
+ /**
+ * Used by `_.trim` and `_.trimEnd` to get the index of the last non-whitespace
+ * character of `string`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {number} Returns the index of the last non-whitespace character.
+ */
+ function trimmedEndIndex(string) {
+ var index = string.length;
+
+ while (index-- && reWhitespace.test(string.charAt(index))) {}
+ return index;
+ }
+
+ /**
+ * Used by `_.unescape` to convert HTML entities to characters.
+ *
+ * @private
+ * @param {string} chr The matched character to unescape.
+ * @returns {string} Returns the unescaped character.
+ */
+ var unescapeHtmlChar = basePropertyOf(htmlUnescapes);
+
+ /**
+ * Gets the size of a Unicode `string`.
+ *
+ * @private
+ * @param {string} string The string inspect.
+ * @returns {number} Returns the string size.
+ */
+ function unicodeSize(string) {
+ var result = reUnicode.lastIndex = 0;
+ while (reUnicode.test(string)) {
+ ++result;
+ }
+ return result;
+ }
+
+ /**
+ * Converts a Unicode `string` to an array.
+ *
+ * @private
+ * @param {string} string The string to convert.
+ * @returns {Array} Returns the converted array.
+ */
+ function unicodeToArray(string) {
+ return string.match(reUnicode) || [];
+ }
+
+ /**
+ * Splits a Unicode `string` into an array of its words.
+ *
+ * @private
+ * @param {string} The string to inspect.
+ * @returns {Array} Returns the words of `string`.
+ */
+ function unicodeWords(string) {
+ return string.match(reUnicodeWord) || [];
+ }
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Create a new pristine `lodash` function using the `context` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.1.0
+ * @category Util
+ * @param {Object} [context=root] The context object.
+ * @returns {Function} Returns a new `lodash` function.
+ * @example
+ *
+ * _.mixin({ 'foo': _.constant('foo') });
+ *
+ * var lodash = _.runInContext();
+ * lodash.mixin({ 'bar': lodash.constant('bar') });
+ *
+ * _.isFunction(_.foo);
+ * // => true
+ * _.isFunction(_.bar);
+ * // => false
+ *
+ * lodash.isFunction(lodash.foo);
+ * // => false
+ * lodash.isFunction(lodash.bar);
+ * // => true
+ *
+ * // Create a suped-up `defer` in Node.js.
+ * var defer = _.runInContext({ 'setTimeout': setImmediate }).defer;
+ */
+ var runInContext = (function runInContext(context) {
+ context = context == null ? root : _.defaults(root.Object(), context, _.pick(root, contextProps));
+
+ /** Built-in constructor references. */
+ var Array = context.Array,
+ Date = context.Date,
+ Error = context.Error,
+ Function = context.Function,
+ Math = context.Math,
+ Object = context.Object,
+ RegExp = context.RegExp,
+ String = context.String,
+ TypeError = context.TypeError;
+
+ /** Used for built-in method references. */
+ var arrayProto = Array.prototype,
+ funcProto = Function.prototype,
+ objectProto = Object.prototype;
+
+ /** Used to detect overreaching core-js shims. */
+ var coreJsData = context['__core-js_shared__'];
+
+ /** Used to resolve the decompiled source of functions. */
+ var funcToString = funcProto.toString;
+
+ /** Used to check objects for own properties. */
+ var hasOwnProperty = objectProto.hasOwnProperty;
+
+ /** Used to generate unique IDs. */
+ var idCounter = 0;
+
+ /** Used to detect methods masquerading as native. */
+ var maskSrcKey = (function() {
+ var uid = /[^.]+$/.exec(coreJsData && coreJsData.keys && coreJsData.keys.IE_PROTO || '');
+ return uid ? ('Symbol(src)_1.' + uid) : '';
+ }());
+
+ /**
+ * Used to resolve the
+ * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+ var nativeObjectToString = objectProto.toString;
+
+ /** Used to infer the `Object` constructor. */
+ var objectCtorString = funcToString.call(Object);
+
+ /** Used to restore the original `_` reference in `_.noConflict`. */
+ var oldDash = root._;
+
+ /** Used to detect if a method is native. */
+ var reIsNative = RegExp('^' +
+ funcToString.call(hasOwnProperty).replace(reRegExpChar, '\\$&')
+ .replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
+ );
+
+ /** Built-in value references. */
+ var Buffer = moduleExports ? context.Buffer : undefined,
+ Symbol = context.Symbol,
+ Uint8Array = context.Uint8Array,
+ allocUnsafe = Buffer ? Buffer.allocUnsafe : undefined,
+ getPrototype = overArg(Object.getPrototypeOf, Object),
+ objectCreate = Object.create,
+ propertyIsEnumerable = objectProto.propertyIsEnumerable,
+ splice = arrayProto.splice,
+ spreadableSymbol = Symbol ? Symbol.isConcatSpreadable : undefined,
+ symIterator = Symbol ? Symbol.iterator : undefined,
+ symToStringTag = Symbol ? Symbol.toStringTag : undefined;
+
+ var defineProperty = (function() {
+ try {
+ var func = getNative(Object, 'defineProperty');
+ func({}, '', {});
+ return func;
+ } catch (e) {}
+ }());
+
+ /** Mocked built-ins. */
+ var ctxClearTimeout = context.clearTimeout !== root.clearTimeout && context.clearTimeout,
+ ctxNow = Date && Date.now !== root.Date.now && Date.now,
+ ctxSetTimeout = context.setTimeout !== root.setTimeout && context.setTimeout;
+
+ /* Built-in method references for those with the same name as other `lodash` methods. */
+ var nativeCeil = Math.ceil,
+ nativeFloor = Math.floor,
+ nativeGetSymbols = Object.getOwnPropertySymbols,
+ nativeIsBuffer = Buffer ? Buffer.isBuffer : undefined,
+ nativeIsFinite = context.isFinite,
+ nativeJoin = arrayProto.join,
+ nativeKeys = overArg(Object.keys, Object),
+ nativeMax = Math.max,
+ nativeMin = Math.min,
+ nativeNow = Date.now,
+ nativeParseInt = context.parseInt,
+ nativeRandom = Math.random,
+ nativeReverse = arrayProto.reverse;
+
+ /* Built-in method references that are verified to be native. */
+ var DataView = getNative(context, 'DataView'),
+ Map = getNative(context, 'Map'),
+ Promise = getNative(context, 'Promise'),
+ Set = getNative(context, 'Set'),
+ WeakMap = getNative(context, 'WeakMap'),
+ nativeCreate = getNative(Object, 'create');
+
+ /** Used to store function metadata. */
+ var metaMap = WeakMap && new WeakMap;
+
+ /** Used to lookup unminified function names. */
+ var realNames = {};
+
+ /** Used to detect maps, sets, and weakmaps. */
+ var dataViewCtorString = toSource(DataView),
+ mapCtorString = toSource(Map),
+ promiseCtorString = toSource(Promise),
+ setCtorString = toSource(Set),
+ weakMapCtorString = toSource(WeakMap);
+
+ /** Used to convert symbols to primitives and strings. */
+ var symbolProto = Symbol ? Symbol.prototype : undefined,
+ symbolValueOf = symbolProto ? symbolProto.valueOf : undefined,
+ symbolToString = symbolProto ? symbolProto.toString : undefined;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a `lodash` object which wraps `value` to enable implicit method
+ * chain sequences. Methods that operate on and return arrays, collections,
+ * and functions can be chained together. Methods that retrieve a single value
+ * or may return a primitive value will automatically end the chain sequence
+ * and return the unwrapped value. Otherwise, the value must be unwrapped
+ * with `_#value`.
+ *
+ * Explicit chain sequences, which must be unwrapped with `_#value`, may be
+ * enabled using `_.chain`.
+ *
+ * The execution of chained methods is lazy, that is, it's deferred until
+ * `_#value` is implicitly or explicitly called.
+ *
+ * Lazy evaluation allows several methods to support shortcut fusion.
+ * Shortcut fusion is an optimization to merge iteratee calls; this avoids
+ * the creation of intermediate arrays and can greatly reduce the number of
+ * iteratee executions. Sections of a chain sequence qualify for shortcut
+ * fusion if the section is applied to an array and iteratees accept only
+ * one argument. The heuristic for whether a section qualifies for shortcut
+ * fusion is subject to change.
+ *
+ * Chaining is supported in custom builds as long as the `_#value` method is
+ * directly or indirectly included in the build.
+ *
+ * In addition to lodash methods, wrappers have `Array` and `String` methods.
+ *
+ * The wrapper `Array` methods are:
+ * `concat`, `join`, `pop`, `push`, `shift`, `sort`, `splice`, and `unshift`
+ *
+ * The wrapper `String` methods are:
+ * `replace` and `split`
+ *
+ * The wrapper methods that support shortcut fusion are:
+ * `at`, `compact`, `drop`, `dropRight`, `dropWhile`, `filter`, `find`,
+ * `findLast`, `head`, `initial`, `last`, `map`, `reject`, `reverse`, `slice`,
+ * `tail`, `take`, `takeRight`, `takeRightWhile`, `takeWhile`, and `toArray`
+ *
+ * The chainable wrapper methods are:
+ * `after`, `ary`, `assign`, `assignIn`, `assignInWith`, `assignWith`, `at`,
+ * `before`, `bind`, `bindAll`, `bindKey`, `castArray`, `chain`, `chunk`,
+ * `commit`, `compact`, `concat`, `conforms`, `constant`, `countBy`, `create`,
+ * `curry`, `debounce`, `defaults`, `defaultsDeep`, `defer`, `delay`,
+ * `difference`, `differenceBy`, `differenceWith`, `drop`, `dropRight`,
+ * `dropRightWhile`, `dropWhile`, `extend`, `extendWith`, `fill`, `filter`,
+ * `flatMap`, `flatMapDeep`, `flatMapDepth`, `flatten`, `flattenDeep`,
+ * `flattenDepth`, `flip`, `flow`, `flowRight`, `fromPairs`, `functions`,
+ * `functionsIn`, `groupBy`, `initial`, `intersection`, `intersectionBy`,
+ * `intersectionWith`, `invert`, `invertBy`, `invokeMap`, `iteratee`, `keyBy`,
+ * `keys`, `keysIn`, `map`, `mapKeys`, `mapValues`, `matches`, `matchesProperty`,
+ * `memoize`, `merge`, `mergeWith`, `method`, `methodOf`, `mixin`, `negate`,
+ * `nthArg`, `omit`, `omitBy`, `once`, `orderBy`, `over`, `overArgs`,
+ * `overEvery`, `overSome`, `partial`, `partialRight`, `partition`, `pick`,
+ * `pickBy`, `plant`, `property`, `propertyOf`, `pull`, `pullAll`, `pullAllBy`,
+ * `pullAllWith`, `pullAt`, `push`, `range`, `rangeRight`, `rearg`, `reject`,
+ * `remove`, `rest`, `reverse`, `sampleSize`, `set`, `setWith`, `shuffle`,
+ * `slice`, `sort`, `sortBy`, `splice`, `spread`, `tail`, `take`, `takeRight`,
+ * `takeRightWhile`, `takeWhile`, `tap`, `throttle`, `thru`, `toArray`,
+ * `toPairs`, `toPairsIn`, `toPath`, `toPlainObject`, `transform`, `unary`,
+ * `union`, `unionBy`, `unionWith`, `uniq`, `uniqBy`, `uniqWith`, `unset`,
+ * `unshift`, `unzip`, `unzipWith`, `update`, `updateWith`, `values`,
+ * `valuesIn`, `without`, `wrap`, `xor`, `xorBy`, `xorWith`, `zip`,
+ * `zipObject`, `zipObjectDeep`, and `zipWith`
+ *
+ * The wrapper methods that are **not** chainable by default are:
+ * `add`, `attempt`, `camelCase`, `capitalize`, `ceil`, `clamp`, `clone`,
+ * `cloneDeep`, `cloneDeepWith`, `cloneWith`, `conformsTo`, `deburr`,
+ * `defaultTo`, `divide`, `each`, `eachRight`, `endsWith`, `eq`, `escape`,
+ * `escapeRegExp`, `every`, `find`, `findIndex`, `findKey`, `findLast`,
+ * `findLastIndex`, `findLastKey`, `first`, `floor`, `forEach`, `forEachRight`,
+ * `forIn`, `forInRight`, `forOwn`, `forOwnRight`, `get`, `gt`, `gte`, `has`,
+ * `hasIn`, `head`, `identity`, `includes`, `indexOf`, `inRange`, `invoke`,
+ * `isArguments`, `isArray`, `isArrayBuffer`, `isArrayLike`, `isArrayLikeObject`,
+ * `isBoolean`, `isBuffer`, `isDate`, `isElement`, `isEmpty`, `isEqual`,
+ * `isEqualWith`, `isError`, `isFinite`, `isFunction`, `isInteger`, `isLength`,
+ * `isMap`, `isMatch`, `isMatchWith`, `isNaN`, `isNative`, `isNil`, `isNull`,
+ * `isNumber`, `isObject`, `isObjectLike`, `isPlainObject`, `isRegExp`,
+ * `isSafeInteger`, `isSet`, `isString`, `isUndefined`, `isTypedArray`,
+ * `isWeakMap`, `isWeakSet`, `join`, `kebabCase`, `last`, `lastIndexOf`,
+ * `lowerCase`, `lowerFirst`, `lt`, `lte`, `max`, `maxBy`, `mean`, `meanBy`,
+ * `min`, `minBy`, `multiply`, `noConflict`, `noop`, `now`, `nth`, `pad`,
+ * `padEnd`, `padStart`, `parseInt`, `pop`, `random`, `reduce`, `reduceRight`,
+ * `repeat`, `result`, `round`, `runInContext`, `sample`, `shift`, `size`,
+ * `snakeCase`, `some`, `sortedIndex`, `sortedIndexBy`, `sortedLastIndex`,
+ * `sortedLastIndexBy`, `startCase`, `startsWith`, `stubArray`, `stubFalse`,
+ * `stubObject`, `stubString`, `stubTrue`, `subtract`, `sum`, `sumBy`,
+ * `template`, `times`, `toFinite`, `toInteger`, `toJSON`, `toLength`,
+ * `toLower`, `toNumber`, `toSafeInteger`, `toString`, `toUpper`, `trim`,
+ * `trimEnd`, `trimStart`, `truncate`, `unescape`, `uniqueId`, `upperCase`,
+ * `upperFirst`, `value`, and `words`
+ *
+ * @name _
+ * @constructor
+ * @category Seq
+ * @param {*} value The value to wrap in a `lodash` instance.
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * var wrapped = _([1, 2, 3]);
+ *
+ * // Returns an unwrapped value.
+ * wrapped.reduce(_.add);
+ * // => 6
+ *
+ * // Returns a wrapped value.
+ * var squares = wrapped.map(square);
+ *
+ * _.isArray(squares);
+ * // => false
+ *
+ * _.isArray(squares.value());
+ * // => true
+ */
+ function lodash(value) {
+ if (isObjectLike(value) && !isArray(value) && !(value instanceof LazyWrapper)) {
+ if (value instanceof LodashWrapper) {
+ return value;
+ }
+ if (hasOwnProperty.call(value, '__wrapped__')) {
+ return wrapperClone(value);
+ }
+ }
+ return new LodashWrapper(value);
+ }
+
+ /**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} proto The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+ var baseCreate = (function() {
+ function object() {}
+ return function(proto) {
+ if (!isObject(proto)) {
+ return {};
+ }
+ if (objectCreate) {
+ return objectCreate(proto);
+ }
+ object.prototype = proto;
+ var result = new object;
+ object.prototype = undefined;
+ return result;
+ };
+ }());
+
+ /**
+ * The function whose prototype chain sequence wrappers inherit from.
+ *
+ * @private
+ */
+ function baseLodash() {
+ // No operation performed.
+ }
+
+ /**
+ * The base constructor for creating `lodash` wrapper objects.
+ *
+ * @private
+ * @param {*} value The value to wrap.
+ * @param {boolean} [chainAll] Enable explicit method chain sequences.
+ */
+ function LodashWrapper(value, chainAll) {
+ this.__wrapped__ = value;
+ this.__actions__ = [];
+ this.__chain__ = !!chainAll;
+ this.__index__ = 0;
+ this.__values__ = undefined;
+ }
+
+ /**
+ * By default, the template delimiters used by lodash are like those in
+ * embedded Ruby (ERB) as well as ES2015 template strings. Change the
+ * following template settings to use alternative delimiters.
+ *
+ * @static
+ * @memberOf _
+ * @type {Object}
+ */
+ lodash.templateSettings = {
+
+ /**
+ * Used to detect `data` property values to be HTML-escaped.
+ *
+ * @memberOf _.templateSettings
+ * @type {RegExp}
+ */
+ 'escape': reEscape,
+
+ /**
+ * Used to detect code to be evaluated.
+ *
+ * @memberOf _.templateSettings
+ * @type {RegExp}
+ */
+ 'evaluate': reEvaluate,
+
+ /**
+ * Used to detect `data` property values to inject.
+ *
+ * @memberOf _.templateSettings
+ * @type {RegExp}
+ */
+ 'interpolate': reInterpolate,
+
+ /**
+ * Used to reference the data object in the template text.
+ *
+ * @memberOf _.templateSettings
+ * @type {string}
+ */
+ 'variable': '',
+
+ /**
+ * Used to import variables into the compiled template.
+ *
+ * @memberOf _.templateSettings
+ * @type {Object}
+ */
+ 'imports': {
+
+ /**
+ * A reference to the `lodash` function.
+ *
+ * @memberOf _.templateSettings.imports
+ * @type {Function}
+ */
+ '_': lodash
+ }
+ };
+
+ // Ensure wrappers are instances of `baseLodash`.
+ lodash.prototype = baseLodash.prototype;
+ lodash.prototype.constructor = lodash;
+
+ LodashWrapper.prototype = baseCreate(baseLodash.prototype);
+ LodashWrapper.prototype.constructor = LodashWrapper;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a lazy wrapper object which wraps `value` to enable lazy evaluation.
+ *
+ * @private
+ * @constructor
+ * @param {*} value The value to wrap.
+ */
+ function LazyWrapper(value) {
+ this.__wrapped__ = value;
+ this.__actions__ = [];
+ this.__dir__ = 1;
+ this.__filtered__ = false;
+ this.__iteratees__ = [];
+ this.__takeCount__ = MAX_ARRAY_LENGTH;
+ this.__views__ = [];
+ }
+
+ /**
+ * Creates a clone of the lazy wrapper object.
+ *
+ * @private
+ * @name clone
+ * @memberOf LazyWrapper
+ * @returns {Object} Returns the cloned `LazyWrapper` object.
+ */
+ function lazyClone() {
+ var result = new LazyWrapper(this.__wrapped__);
+ result.__actions__ = copyArray(this.__actions__);
+ result.__dir__ = this.__dir__;
+ result.__filtered__ = this.__filtered__;
+ result.__iteratees__ = copyArray(this.__iteratees__);
+ result.__takeCount__ = this.__takeCount__;
+ result.__views__ = copyArray(this.__views__);
+ return result;
+ }
+
+ /**
+ * Reverses the direction of lazy iteration.
+ *
+ * @private
+ * @name reverse
+ * @memberOf LazyWrapper
+ * @returns {Object} Returns the new reversed `LazyWrapper` object.
+ */
+ function lazyReverse() {
+ if (this.__filtered__) {
+ var result = new LazyWrapper(this);
+ result.__dir__ = -1;
+ result.__filtered__ = true;
+ } else {
+ result = this.clone();
+ result.__dir__ *= -1;
+ }
+ return result;
+ }
+
+ /**
+ * Extracts the unwrapped value from its lazy wrapper.
+ *
+ * @private
+ * @name value
+ * @memberOf LazyWrapper
+ * @returns {*} Returns the unwrapped value.
+ */
+ function lazyValue() {
+ var array = this.__wrapped__.value(),
+ dir = this.__dir__,
+ isArr = isArray(array),
+ isRight = dir < 0,
+ arrLength = isArr ? array.length : 0,
+ view = getView(0, arrLength, this.__views__),
+ start = view.start,
+ end = view.end,
+ length = end - start,
+ index = isRight ? end : (start - 1),
+ iteratees = this.__iteratees__,
+ iterLength = iteratees.length,
+ resIndex = 0,
+ takeCount = nativeMin(length, this.__takeCount__);
+
+ if (!isArr || (!isRight && arrLength == length && takeCount == length)) {
+ return baseWrapperValue(array, this.__actions__);
+ }
+ var result = [];
+
+ outer:
+ while (length-- && resIndex < takeCount) {
+ index += dir;
+
+ var iterIndex = -1,
+ value = array[index];
+
+ while (++iterIndex < iterLength) {
+ var data = iteratees[iterIndex],
+ iteratee = data.iteratee,
+ type = data.type,
+ computed = iteratee(value);
+
+ if (type == LAZY_MAP_FLAG) {
+ value = computed;
+ } else if (!computed) {
+ if (type == LAZY_FILTER_FLAG) {
+ continue outer;
+ } else {
+ break outer;
+ }
+ }
+ }
+ result[resIndex++] = value;
+ }
+ return result;
+ }
+
+ // Ensure `LazyWrapper` is an instance of `baseLodash`.
+ LazyWrapper.prototype = baseCreate(baseLodash.prototype);
+ LazyWrapper.prototype.constructor = LazyWrapper;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a hash object.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+ function Hash(entries) {
+ var index = -1,
+ length = entries == null ? 0 : entries.length;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+ }
+
+ /**
+ * Removes all key-value entries from the hash.
+ *
+ * @private
+ * @name clear
+ * @memberOf Hash
+ */
+ function hashClear() {
+ this.__data__ = nativeCreate ? nativeCreate(null) : {};
+ this.size = 0;
+ }
+
+ /**
+ * Removes `key` and its value from the hash.
+ *
+ * @private
+ * @name delete
+ * @memberOf Hash
+ * @param {Object} hash The hash to modify.
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+ function hashDelete(key) {
+ var result = this.has(key) && delete this.__data__[key];
+ this.size -= result ? 1 : 0;
+ return result;
+ }
+
+ /**
+ * Gets the hash value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf Hash
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+ function hashGet(key) {
+ var data = this.__data__;
+ if (nativeCreate) {
+ var result = data[key];
+ return result === HASH_UNDEFINED ? undefined : result;
+ }
+ return hasOwnProperty.call(data, key) ? data[key] : undefined;
+ }
+
+ /**
+ * Checks if a hash value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf Hash
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+ function hashHas(key) {
+ var data = this.__data__;
+ return nativeCreate ? (data[key] !== undefined) : hasOwnProperty.call(data, key);
+ }
+
+ /**
+ * Sets the hash `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf Hash
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the hash instance.
+ */
+ function hashSet(key, value) {
+ var data = this.__data__;
+ this.size += this.has(key) ? 0 : 1;
+ data[key] = (nativeCreate && value === undefined) ? HASH_UNDEFINED : value;
+ return this;
+ }
+
+ // Add methods to `Hash`.
+ Hash.prototype.clear = hashClear;
+ Hash.prototype['delete'] = hashDelete;
+ Hash.prototype.get = hashGet;
+ Hash.prototype.has = hashHas;
+ Hash.prototype.set = hashSet;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates an list cache object.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+ function ListCache(entries) {
+ var index = -1,
+ length = entries == null ? 0 : entries.length;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+ }
+
+ /**
+ * Removes all key-value entries from the list cache.
+ *
+ * @private
+ * @name clear
+ * @memberOf ListCache
+ */
+ function listCacheClear() {
+ this.__data__ = [];
+ this.size = 0;
+ }
+
+ /**
+ * Removes `key` and its value from the list cache.
+ *
+ * @private
+ * @name delete
+ * @memberOf ListCache
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+ function listCacheDelete(key) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ if (index < 0) {
+ return false;
+ }
+ var lastIndex = data.length - 1;
+ if (index == lastIndex) {
+ data.pop();
+ } else {
+ splice.call(data, index, 1);
+ }
+ --this.size;
+ return true;
+ }
+
+ /**
+ * Gets the list cache value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf ListCache
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+ function listCacheGet(key) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ return index < 0 ? undefined : data[index][1];
+ }
+
+ /**
+ * Checks if a list cache value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf ListCache
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+ function listCacheHas(key) {
+ return assocIndexOf(this.__data__, key) > -1;
+ }
+
+ /**
+ * Sets the list cache `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf ListCache
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the list cache instance.
+ */
+ function listCacheSet(key, value) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ if (index < 0) {
+ ++this.size;
+ data.push([key, value]);
+ } else {
+ data[index][1] = value;
+ }
+ return this;
+ }
+
+ // Add methods to `ListCache`.
+ ListCache.prototype.clear = listCacheClear;
+ ListCache.prototype['delete'] = listCacheDelete;
+ ListCache.prototype.get = listCacheGet;
+ ListCache.prototype.has = listCacheHas;
+ ListCache.prototype.set = listCacheSet;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a map cache object to store key-value pairs.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+ function MapCache(entries) {
+ var index = -1,
+ length = entries == null ? 0 : entries.length;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+ }
+
+ /**
+ * Removes all key-value entries from the map.
+ *
+ * @private
+ * @name clear
+ * @memberOf MapCache
+ */
+ function mapCacheClear() {
+ this.size = 0;
+ this.__data__ = {
+ 'hash': new Hash,
+ 'map': new (Map || ListCache),
+ 'string': new Hash
+ };
+ }
+
+ /**
+ * Removes `key` and its value from the map.
+ *
+ * @private
+ * @name delete
+ * @memberOf MapCache
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+ function mapCacheDelete(key) {
+ var result = getMapData(this, key)['delete'](key);
+ this.size -= result ? 1 : 0;
+ return result;
+ }
+
+ /**
+ * Gets the map value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf MapCache
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+ function mapCacheGet(key) {
+ return getMapData(this, key).get(key);
+ }
+
+ /**
+ * Checks if a map value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf MapCache
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+ function mapCacheHas(key) {
+ return getMapData(this, key).has(key);
+ }
+
+ /**
+ * Sets the map `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf MapCache
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the map cache instance.
+ */
+ function mapCacheSet(key, value) {
+ var data = getMapData(this, key),
+ size = data.size;
+
+ data.set(key, value);
+ this.size += data.size == size ? 0 : 1;
+ return this;
+ }
+
+ // Add methods to `MapCache`.
+ MapCache.prototype.clear = mapCacheClear;
+ MapCache.prototype['delete'] = mapCacheDelete;
+ MapCache.prototype.get = mapCacheGet;
+ MapCache.prototype.has = mapCacheHas;
+ MapCache.prototype.set = mapCacheSet;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ *
+ * Creates an array cache object to store unique values.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [values] The values to cache.
+ */
+ function SetCache(values) {
+ var index = -1,
+ length = values == null ? 0 : values.length;
+
+ this.__data__ = new MapCache;
+ while (++index < length) {
+ this.add(values[index]);
+ }
+ }
+
+ /**
+ * Adds `value` to the array cache.
+ *
+ * @private
+ * @name add
+ * @memberOf SetCache
+ * @alias push
+ * @param {*} value The value to cache.
+ * @returns {Object} Returns the cache instance.
+ */
+ function setCacheAdd(value) {
+ this.__data__.set(value, HASH_UNDEFINED);
+ return this;
+ }
+
+ /**
+ * Checks if `value` is in the array cache.
+ *
+ * @private
+ * @name has
+ * @memberOf SetCache
+ * @param {*} value The value to search for.
+ * @returns {number} Returns `true` if `value` is found, else `false`.
+ */
+ function setCacheHas(value) {
+ return this.__data__.has(value);
+ }
+
+ // Add methods to `SetCache`.
+ SetCache.prototype.add = SetCache.prototype.push = setCacheAdd;
+ SetCache.prototype.has = setCacheHas;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a stack cache object to store key-value pairs.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+ function Stack(entries) {
+ var data = this.__data__ = new ListCache(entries);
+ this.size = data.size;
+ }
+
+ /**
+ * Removes all key-value entries from the stack.
+ *
+ * @private
+ * @name clear
+ * @memberOf Stack
+ */
+ function stackClear() {
+ this.__data__ = new ListCache;
+ this.size = 0;
+ }
+
+ /**
+ * Removes `key` and its value from the stack.
+ *
+ * @private
+ * @name delete
+ * @memberOf Stack
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+ function stackDelete(key) {
+ var data = this.__data__,
+ result = data['delete'](key);
+
+ this.size = data.size;
+ return result;
+ }
+
+ /**
+ * Gets the stack value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf Stack
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+ function stackGet(key) {
+ return this.__data__.get(key);
+ }
+
+ /**
+ * Checks if a stack value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf Stack
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+ function stackHas(key) {
+ return this.__data__.has(key);
+ }
+
+ /**
+ * Sets the stack `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf Stack
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the stack cache instance.
+ */
+ function stackSet(key, value) {
+ var data = this.__data__;
+ if (data instanceof ListCache) {
+ var pairs = data.__data__;
+ if (!Map || (pairs.length < LARGE_ARRAY_SIZE - 1)) {
+ pairs.push([key, value]);
+ this.size = ++data.size;
+ return this;
+ }
+ data = this.__data__ = new MapCache(pairs);
+ }
+ data.set(key, value);
+ this.size = data.size;
+ return this;
+ }
+
+ // Add methods to `Stack`.
+ Stack.prototype.clear = stackClear;
+ Stack.prototype['delete'] = stackDelete;
+ Stack.prototype.get = stackGet;
+ Stack.prototype.has = stackHas;
+ Stack.prototype.set = stackSet;
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates an array of the enumerable property names of the array-like `value`.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @param {boolean} inherited Specify returning inherited property names.
+ * @returns {Array} Returns the array of property names.
+ */
+ function arrayLikeKeys(value, inherited) {
+ var isArr = isArray(value),
+ isArg = !isArr && isArguments(value),
+ isBuff = !isArr && !isArg && isBuffer(value),
+ isType = !isArr && !isArg && !isBuff && isTypedArray(value),
+ skipIndexes = isArr || isArg || isBuff || isType,
+ result = skipIndexes ? baseTimes(value.length, String) : [],
+ length = result.length;
+
+ for (var key in value) {
+ if ((inherited || hasOwnProperty.call(value, key)) &&
+ !(skipIndexes && (
+ // Safari 9 has enumerable `arguments.length` in strict mode.
+ key == 'length' ||
+ // Node.js 0.10 has enumerable non-index properties on buffers.
+ (isBuff && (key == 'offset' || key == 'parent')) ||
+ // PhantomJS 2 has enumerable non-index properties on typed arrays.
+ (isType && (key == 'buffer' || key == 'byteLength' || key == 'byteOffset')) ||
+ // Skip index properties.
+ isIndex(key, length)
+ ))) {
+ result.push(key);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * A specialized version of `_.sample` for arrays.
+ *
+ * @private
+ * @param {Array} array The array to sample.
+ * @returns {*} Returns the random element.
+ */
+ function arraySample(array) {
+ var length = array.length;
+ return length ? array[baseRandom(0, length - 1)] : undefined;
+ }
+
+ /**
+ * A specialized version of `_.sampleSize` for arrays.
+ *
+ * @private
+ * @param {Array} array The array to sample.
+ * @param {number} n The number of elements to sample.
+ * @returns {Array} Returns the random elements.
+ */
+ function arraySampleSize(array, n) {
+ return shuffleSelf(copyArray(array), baseClamp(n, 0, array.length));
+ }
+
+ /**
+ * A specialized version of `_.shuffle` for arrays.
+ *
+ * @private
+ * @param {Array} array The array to shuffle.
+ * @returns {Array} Returns the new shuffled array.
+ */
+ function arrayShuffle(array) {
+ return shuffleSelf(copyArray(array));
+ }
+
+ /**
+ * This function is like `assignValue` except that it doesn't assign
+ * `undefined` values.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+ function assignMergeValue(object, key, value) {
+ if ((value !== undefined && !eq(object[key], value)) ||
+ (value === undefined && !(key in object))) {
+ baseAssignValue(object, key, value);
+ }
+ }
+
+ /**
+ * Assigns `value` to `key` of `object` if the existing value is not equivalent
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+ function assignValue(object, key, value) {
+ var objValue = object[key];
+ if (!(hasOwnProperty.call(object, key) && eq(objValue, value)) ||
+ (value === undefined && !(key in object))) {
+ baseAssignValue(object, key, value);
+ }
+ }
+
+ /**
+ * Gets the index at which the `key` is found in `array` of key-value pairs.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} key The key to search for.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+ function assocIndexOf(array, key) {
+ var length = array.length;
+ while (length--) {
+ if (eq(array[length][0], key)) {
+ return length;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * Aggregates elements of `collection` on `accumulator` with keys transformed
+ * by `iteratee` and values set by `setter`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} setter The function to set `accumulator` values.
+ * @param {Function} iteratee The iteratee to transform keys.
+ * @param {Object} accumulator The initial aggregated object.
+ * @returns {Function} Returns `accumulator`.
+ */
+ function baseAggregator(collection, setter, iteratee, accumulator) {
+ baseEach(collection, function(value, key, collection) {
+ setter(accumulator, value, iteratee(value), collection);
+ });
+ return accumulator;
+ }
+
+ /**
+ * The base implementation of `_.assign` without support for multiple sources
+ * or `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @returns {Object} Returns `object`.
+ */
+ function baseAssign(object, source) {
+ return object && copyObject(source, keys(source), object);
+ }
+
+ /**
+ * The base implementation of `_.assignIn` without support for multiple sources
+ * or `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @returns {Object} Returns `object`.
+ */
+ function baseAssignIn(object, source) {
+ return object && copyObject(source, keysIn(source), object);
+ }
+
+ /**
+ * The base implementation of `assignValue` and `assignMergeValue` without
+ * value checks.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+ function baseAssignValue(object, key, value) {
+ if (key == '__proto__' && defineProperty) {
+ defineProperty(object, key, {
+ 'configurable': true,
+ 'enumerable': true,
+ 'value': value,
+ 'writable': true
+ });
+ } else {
+ object[key] = value;
+ }
+ }
+
+ /**
+ * The base implementation of `_.at` without support for individual paths.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {string[]} paths The property paths to pick.
+ * @returns {Array} Returns the picked elements.
+ */
+ function baseAt(object, paths) {
+ var index = -1,
+ length = paths.length,
+ result = Array(length),
+ skip = object == null;
+
+ while (++index < length) {
+ result[index] = skip ? undefined : get(object, paths[index]);
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.clamp` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {number} number The number to clamp.
+ * @param {number} [lower] The lower bound.
+ * @param {number} upper The upper bound.
+ * @returns {number} Returns the clamped number.
+ */
+ function baseClamp(number, lower, upper) {
+ if (number === number) {
+ if (upper !== undefined) {
+ number = number <= upper ? number : upper;
+ }
+ if (lower !== undefined) {
+ number = number >= lower ? number : lower;
+ }
+ }
+ return number;
+ }
+
+ /**
+ * The base implementation of `_.clone` and `_.cloneDeep` which tracks
+ * traversed objects.
+ *
+ * @private
+ * @param {*} value The value to clone.
+ * @param {boolean} bitmask The bitmask flags.
+ * 1 - Deep clone
+ * 2 - Flatten inherited properties
+ * 4 - Clone symbols
+ * @param {Function} [customizer] The function to customize cloning.
+ * @param {string} [key] The key of `value`.
+ * @param {Object} [object] The parent object of `value`.
+ * @param {Object} [stack] Tracks traversed objects and their clone counterparts.
+ * @returns {*} Returns the cloned value.
+ */
+ function baseClone(value, bitmask, customizer, key, object, stack) {
+ var result,
+ isDeep = bitmask & CLONE_DEEP_FLAG,
+ isFlat = bitmask & CLONE_FLAT_FLAG,
+ isFull = bitmask & CLONE_SYMBOLS_FLAG;
+
+ if (customizer) {
+ result = object ? customizer(value, key, object, stack) : customizer(value);
+ }
+ if (result !== undefined) {
+ return result;
+ }
+ if (!isObject(value)) {
+ return value;
+ }
+ var isArr = isArray(value);
+ if (isArr) {
+ result = initCloneArray(value);
+ if (!isDeep) {
+ return copyArray(value, result);
+ }
+ } else {
+ var tag = getTag(value),
+ isFunc = tag == funcTag || tag == genTag;
+
+ if (isBuffer(value)) {
+ return cloneBuffer(value, isDeep);
+ }
+ if (tag == objectTag || tag == argsTag || (isFunc && !object)) {
+ result = (isFlat || isFunc) ? {} : initCloneObject(value);
+ if (!isDeep) {
+ return isFlat
+ ? copySymbolsIn(value, baseAssignIn(result, value))
+ : copySymbols(value, baseAssign(result, value));
+ }
+ } else {
+ if (!cloneableTags[tag]) {
+ return object ? value : {};
+ }
+ result = initCloneByTag(value, tag, isDeep);
+ }
+ }
+ // Check for circular references and return its corresponding clone.
+ stack || (stack = new Stack);
+ var stacked = stack.get(value);
+ if (stacked) {
+ return stacked;
+ }
+ stack.set(value, result);
+
+ if (isSet(value)) {
+ value.forEach(function(subValue) {
+ result.add(baseClone(subValue, bitmask, customizer, subValue, value, stack));
+ });
+ } else if (isMap(value)) {
+ value.forEach(function(subValue, key) {
+ result.set(key, baseClone(subValue, bitmask, customizer, key, value, stack));
+ });
+ }
+
+ var keysFunc = isFull
+ ? (isFlat ? getAllKeysIn : getAllKeys)
+ : (isFlat ? keysIn : keys);
+
+ var props = isArr ? undefined : keysFunc(value);
+ arrayEach(props || value, function(subValue, key) {
+ if (props) {
+ key = subValue;
+ subValue = value[key];
+ }
+ // Recursively populate clone (susceptible to call stack limits).
+ assignValue(result, key, baseClone(subValue, bitmask, customizer, key, value, stack));
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.conforms` which doesn't clone `source`.
+ *
+ * @private
+ * @param {Object} source The object of property predicates to conform to.
+ * @returns {Function} Returns the new spec function.
+ */
+ function baseConforms(source) {
+ var props = keys(source);
+ return function(object) {
+ return baseConformsTo(object, source, props);
+ };
+ }
+
+ /**
+ * The base implementation of `_.conformsTo` which accepts `props` to check.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property predicates to conform to.
+ * @returns {boolean} Returns `true` if `object` conforms, else `false`.
+ */
+ function baseConformsTo(object, source, props) {
+ var length = props.length;
+ if (object == null) {
+ return !length;
+ }
+ object = Object(object);
+ while (length--) {
+ var key = props[length],
+ predicate = source[key],
+ value = object[key];
+
+ if ((value === undefined && !(key in object)) || !predicate(value)) {
+ return false;
+ }
+ }
+ return true;
+ }
+
+ /**
+ * The base implementation of `_.delay` and `_.defer` which accepts `args`
+ * to provide to `func`.
+ *
+ * @private
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay invocation.
+ * @param {Array} args The arguments to provide to `func`.
+ * @returns {number|Object} Returns the timer id or timeout object.
+ */
+ function baseDelay(func, wait, args) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ return setTimeout(function() { func.apply(undefined, args); }, wait);
+ }
+
+ /**
+ * The base implementation of methods like `_.difference` without support
+ * for excluding multiple arrays or iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Array} values The values to exclude.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of filtered values.
+ */
+ function baseDifference(array, values, iteratee, comparator) {
+ var index = -1,
+ includes = arrayIncludes,
+ isCommon = true,
+ length = array.length,
+ result = [],
+ valuesLength = values.length;
+
+ if (!length) {
+ return result;
+ }
+ if (iteratee) {
+ values = arrayMap(values, baseUnary(iteratee));
+ }
+ if (comparator) {
+ includes = arrayIncludesWith;
+ isCommon = false;
+ }
+ else if (values.length >= LARGE_ARRAY_SIZE) {
+ includes = cacheHas;
+ isCommon = false;
+ values = new SetCache(values);
+ }
+ outer:
+ while (++index < length) {
+ var value = array[index],
+ computed = iteratee == null ? value : iteratee(value);
+
+ value = (comparator || value !== 0) ? value : 0;
+ if (isCommon && computed === computed) {
+ var valuesIndex = valuesLength;
+ while (valuesIndex--) {
+ if (values[valuesIndex] === computed) {
+ continue outer;
+ }
+ }
+ result.push(value);
+ }
+ else if (!includes(values, computed, comparator)) {
+ result.push(value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.forEach` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object} Returns `collection`.
+ */
+ var baseEach = createBaseEach(baseForOwn);
+
+ /**
+ * The base implementation of `_.forEachRight` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object} Returns `collection`.
+ */
+ var baseEachRight = createBaseEach(baseForOwnRight, true);
+
+ /**
+ * The base implementation of `_.every` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`
+ */
+ function baseEvery(collection, predicate) {
+ var result = true;
+ baseEach(collection, function(value, index, collection) {
+ result = !!predicate(value, index, collection);
+ return result;
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of methods like `_.max` and `_.min` which accepts a
+ * `comparator` to determine the extremum value.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The iteratee invoked per iteration.
+ * @param {Function} comparator The comparator used to compare values.
+ * @returns {*} Returns the extremum value.
+ */
+ function baseExtremum(array, iteratee, comparator) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ var value = array[index],
+ current = iteratee(value);
+
+ if (current != null && (computed === undefined
+ ? (current === current && !isSymbol(current))
+ : comparator(current, computed)
+ )) {
+ var computed = current,
+ result = value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.fill` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to fill.
+ * @param {*} value The value to fill `array` with.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns `array`.
+ */
+ function baseFill(array, value, start, end) {
+ var length = array.length;
+
+ start = toInteger(start);
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = (end === undefined || end > length) ? length : toInteger(end);
+ if (end < 0) {
+ end += length;
+ }
+ end = start > end ? 0 : toLength(end);
+ while (start < end) {
+ array[start++] = value;
+ }
+ return array;
+ }
+
+ /**
+ * The base implementation of `_.filter` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ */
+ function baseFilter(collection, predicate) {
+ var result = [];
+ baseEach(collection, function(value, index, collection) {
+ if (predicate(value, index, collection)) {
+ result.push(value);
+ }
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.flatten` with support for restricting flattening.
+ *
+ * @private
+ * @param {Array} array The array to flatten.
+ * @param {number} depth The maximum recursion depth.
+ * @param {boolean} [predicate=isFlattenable] The function invoked per iteration.
+ * @param {boolean} [isStrict] Restrict to values that pass `predicate` checks.
+ * @param {Array} [result=[]] The initial result value.
+ * @returns {Array} Returns the new flattened array.
+ */
+ function baseFlatten(array, depth, predicate, isStrict, result) {
+ var index = -1,
+ length = array.length;
+
+ predicate || (predicate = isFlattenable);
+ result || (result = []);
+
+ while (++index < length) {
+ var value = array[index];
+ if (depth > 0 && predicate(value)) {
+ if (depth > 1) {
+ // Recursively flatten arrays (susceptible to call stack limits).
+ baseFlatten(value, depth - 1, predicate, isStrict, result);
+ } else {
+ arrayPush(result, value);
+ }
+ } else if (!isStrict) {
+ result[result.length] = value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `baseForOwn` which iterates over `object`
+ * properties returned by `keysFunc` and invokes `iteratee` for each property.
+ * Iteratee functions may exit iteration early by explicitly returning `false`.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+ var baseFor = createBaseFor();
+
+ /**
+ * This function is like `baseFor` except that it iterates over properties
+ * in the opposite order.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+ var baseForRight = createBaseFor(true);
+
+ /**
+ * The base implementation of `_.forOwn` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+ function baseForOwn(object, iteratee) {
+ return object && baseFor(object, iteratee, keys);
+ }
+
+ /**
+ * The base implementation of `_.forOwnRight` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+ function baseForOwnRight(object, iteratee) {
+ return object && baseForRight(object, iteratee, keys);
+ }
+
+ /**
+ * The base implementation of `_.functions` which creates an array of
+ * `object` function property names filtered from `props`.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Array} props The property names to filter.
+ * @returns {Array} Returns the function names.
+ */
+ function baseFunctions(object, props) {
+ return arrayFilter(props, function(key) {
+ return isFunction(object[key]);
+ });
+ }
+
+ /**
+ * The base implementation of `_.get` without support for default values.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the property to get.
+ * @returns {*} Returns the resolved value.
+ */
+ function baseGet(object, path) {
+ path = castPath(path, object);
+
+ var index = 0,
+ length = path.length;
+
+ while (object != null && index < length) {
+ object = object[toKey(path[index++])];
+ }
+ return (index && index == length) ? object : undefined;
+ }
+
+ /**
+ * The base implementation of `getAllKeys` and `getAllKeysIn` which uses
+ * `keysFunc` and `symbolsFunc` to get the enumerable property names and
+ * symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @param {Function} symbolsFunc The function to get the symbols of `object`.
+ * @returns {Array} Returns the array of property names and symbols.
+ */
+ function baseGetAllKeys(object, keysFunc, symbolsFunc) {
+ var result = keysFunc(object);
+ return isArray(object) ? result : arrayPush(result, symbolsFunc(object));
+ }
+
+ /**
+ * The base implementation of `getTag` without fallbacks for buggy environments.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the `toStringTag`.
+ */
+ function baseGetTag(value) {
+ if (value == null) {
+ return value === undefined ? undefinedTag : nullTag;
+ }
+ return (symToStringTag && symToStringTag in Object(value))
+ ? getRawTag(value)
+ : objectToString(value);
+ }
+
+ /**
+ * The base implementation of `_.gt` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is greater than `other`,
+ * else `false`.
+ */
+ function baseGt(value, other) {
+ return value > other;
+ }
+
+ /**
+ * The base implementation of `_.has` without support for deep paths.
+ *
+ * @private
+ * @param {Object} [object] The object to query.
+ * @param {Array|string} key The key to check.
+ * @returns {boolean} Returns `true` if `key` exists, else `false`.
+ */
+ function baseHas(object, key) {
+ return object != null && hasOwnProperty.call(object, key);
+ }
+
+ /**
+ * The base implementation of `_.hasIn` without support for deep paths.
+ *
+ * @private
+ * @param {Object} [object] The object to query.
+ * @param {Array|string} key The key to check.
+ * @returns {boolean} Returns `true` if `key` exists, else `false`.
+ */
+ function baseHasIn(object, key) {
+ return object != null && key in Object(object);
+ }
+
+ /**
+ * The base implementation of `_.inRange` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {number} number The number to check.
+ * @param {number} start The start of the range.
+ * @param {number} end The end of the range.
+ * @returns {boolean} Returns `true` if `number` is in the range, else `false`.
+ */
+ function baseInRange(number, start, end) {
+ return number >= nativeMin(start, end) && number < nativeMax(start, end);
+ }
+
+ /**
+ * The base implementation of methods like `_.intersection`, without support
+ * for iteratee shorthands, that accepts an array of arrays to inspect.
+ *
+ * @private
+ * @param {Array} arrays The arrays to inspect.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of shared values.
+ */
+ function baseIntersection(arrays, iteratee, comparator) {
+ var includes = comparator ? arrayIncludesWith : arrayIncludes,
+ length = arrays[0].length,
+ othLength = arrays.length,
+ othIndex = othLength,
+ caches = Array(othLength),
+ maxLength = Infinity,
+ result = [];
+
+ while (othIndex--) {
+ var array = arrays[othIndex];
+ if (othIndex && iteratee) {
+ array = arrayMap(array, baseUnary(iteratee));
+ }
+ maxLength = nativeMin(array.length, maxLength);
+ caches[othIndex] = !comparator && (iteratee || (length >= 120 && array.length >= 120))
+ ? new SetCache(othIndex && array)
+ : undefined;
+ }
+ array = arrays[0];
+
+ var index = -1,
+ seen = caches[0];
+
+ outer:
+ while (++index < length && result.length < maxLength) {
+ var value = array[index],
+ computed = iteratee ? iteratee(value) : value;
+
+ value = (comparator || value !== 0) ? value : 0;
+ if (!(seen
+ ? cacheHas(seen, computed)
+ : includes(result, computed, comparator)
+ )) {
+ othIndex = othLength;
+ while (--othIndex) {
+ var cache = caches[othIndex];
+ if (!(cache
+ ? cacheHas(cache, computed)
+ : includes(arrays[othIndex], computed, comparator))
+ ) {
+ continue outer;
+ }
+ }
+ if (seen) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.invert` and `_.invertBy` which inverts
+ * `object` with values transformed by `iteratee` and set by `setter`.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} setter The function to set `accumulator` values.
+ * @param {Function} iteratee The iteratee to transform values.
+ * @param {Object} accumulator The initial inverted object.
+ * @returns {Function} Returns `accumulator`.
+ */
+ function baseInverter(object, setter, iteratee, accumulator) {
+ baseForOwn(object, function(value, key, object) {
+ setter(accumulator, iteratee(value), key, object);
+ });
+ return accumulator;
+ }
+
+ /**
+ * The base implementation of `_.invoke` without support for individual
+ * method arguments.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the method to invoke.
+ * @param {Array} args The arguments to invoke the method with.
+ * @returns {*} Returns the result of the invoked method.
+ */
+ function baseInvoke(object, path, args) {
+ path = castPath(path, object);
+ object = parent(object, path);
+ var func = object == null ? object : object[toKey(last(path))];
+ return func == null ? undefined : apply(func, object, args);
+ }
+
+ /**
+ * The base implementation of `_.isArguments`.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ */
+ function baseIsArguments(value) {
+ return isObjectLike(value) && baseGetTag(value) == argsTag;
+ }
+
+ /**
+ * The base implementation of `_.isArrayBuffer` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array buffer, else `false`.
+ */
+ function baseIsArrayBuffer(value) {
+ return isObjectLike(value) && baseGetTag(value) == arrayBufferTag;
+ }
+
+ /**
+ * The base implementation of `_.isDate` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a date object, else `false`.
+ */
+ function baseIsDate(value) {
+ return isObjectLike(value) && baseGetTag(value) == dateTag;
+ }
+
+ /**
+ * The base implementation of `_.isEqual` which supports partial comparisons
+ * and tracks traversed objects.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @param {boolean} bitmask The bitmask flags.
+ * 1 - Unordered comparison
+ * 2 - Partial comparison
+ * @param {Function} [customizer] The function to customize comparisons.
+ * @param {Object} [stack] Tracks traversed `value` and `other` objects.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ */
+ function baseIsEqual(value, other, bitmask, customizer, stack) {
+ if (value === other) {
+ return true;
+ }
+ if (value == null || other == null || (!isObjectLike(value) && !isObjectLike(other))) {
+ return value !== value && other !== other;
+ }
+ return baseIsEqualDeep(value, other, bitmask, customizer, baseIsEqual, stack);
+ }
+
+ /**
+ * A specialized version of `baseIsEqual` for arrays and objects which performs
+ * deep comparisons and tracks traversed objects enabling objects with circular
+ * references to be compared.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} [stack] Tracks traversed `object` and `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+ function baseIsEqualDeep(object, other, bitmask, customizer, equalFunc, stack) {
+ var objIsArr = isArray(object),
+ othIsArr = isArray(other),
+ objTag = objIsArr ? arrayTag : getTag(object),
+ othTag = othIsArr ? arrayTag : getTag(other);
+
+ objTag = objTag == argsTag ? objectTag : objTag;
+ othTag = othTag == argsTag ? objectTag : othTag;
+
+ var objIsObj = objTag == objectTag,
+ othIsObj = othTag == objectTag,
+ isSameTag = objTag == othTag;
+
+ if (isSameTag && isBuffer(object)) {
+ if (!isBuffer(other)) {
+ return false;
+ }
+ objIsArr = true;
+ objIsObj = false;
+ }
+ if (isSameTag && !objIsObj) {
+ stack || (stack = new Stack);
+ return (objIsArr || isTypedArray(object))
+ ? equalArrays(object, other, bitmask, customizer, equalFunc, stack)
+ : equalByTag(object, other, objTag, bitmask, customizer, equalFunc, stack);
+ }
+ if (!(bitmask & COMPARE_PARTIAL_FLAG)) {
+ var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
+ othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
+
+ if (objIsWrapped || othIsWrapped) {
+ var objUnwrapped = objIsWrapped ? object.value() : object,
+ othUnwrapped = othIsWrapped ? other.value() : other;
+
+ stack || (stack = new Stack);
+ return equalFunc(objUnwrapped, othUnwrapped, bitmask, customizer, stack);
+ }
+ }
+ if (!isSameTag) {
+ return false;
+ }
+ stack || (stack = new Stack);
+ return equalObjects(object, other, bitmask, customizer, equalFunc, stack);
+ }
+
+ /**
+ * The base implementation of `_.isMap` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a map, else `false`.
+ */
+ function baseIsMap(value) {
+ return isObjectLike(value) && getTag(value) == mapTag;
+ }
+
+ /**
+ * The base implementation of `_.isMatch` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property values to match.
+ * @param {Array} matchData The property names, values, and compare flags to match.
+ * @param {Function} [customizer] The function to customize comparisons.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ */
+ function baseIsMatch(object, source, matchData, customizer) {
+ var index = matchData.length,
+ length = index,
+ noCustomizer = !customizer;
+
+ if (object == null) {
+ return !length;
+ }
+ object = Object(object);
+ while (index--) {
+ var data = matchData[index];
+ if ((noCustomizer && data[2])
+ ? data[1] !== object[data[0]]
+ : !(data[0] in object)
+ ) {
+ return false;
+ }
+ }
+ while (++index < length) {
+ data = matchData[index];
+ var key = data[0],
+ objValue = object[key],
+ srcValue = data[1];
+
+ if (noCustomizer && data[2]) {
+ if (objValue === undefined && !(key in object)) {
+ return false;
+ }
+ } else {
+ var stack = new Stack;
+ if (customizer) {
+ var result = customizer(objValue, srcValue, key, object, source, stack);
+ }
+ if (!(result === undefined
+ ? baseIsEqual(srcValue, objValue, COMPARE_PARTIAL_FLAG | COMPARE_UNORDERED_FLAG, customizer, stack)
+ : result
+ )) {
+ return false;
+ }
+ }
+ }
+ return true;
+ }
+
+ /**
+ * The base implementation of `_.isNative` without bad shim checks.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a native function,
+ * else `false`.
+ */
+ function baseIsNative(value) {
+ if (!isObject(value) || isMasked(value)) {
+ return false;
+ }
+ var pattern = isFunction(value) ? reIsNative : reIsHostCtor;
+ return pattern.test(toSource(value));
+ }
+
+ /**
+ * The base implementation of `_.isRegExp` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a regexp, else `false`.
+ */
+ function baseIsRegExp(value) {
+ return isObjectLike(value) && baseGetTag(value) == regexpTag;
+ }
+
+ /**
+ * The base implementation of `_.isSet` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a set, else `false`.
+ */
+ function baseIsSet(value) {
+ return isObjectLike(value) && getTag(value) == setTag;
+ }
+
+ /**
+ * The base implementation of `_.isTypedArray` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a typed array, else `false`.
+ */
+ function baseIsTypedArray(value) {
+ return isObjectLike(value) &&
+ isLength(value.length) && !!typedArrayTags[baseGetTag(value)];
+ }
+
+ /**
+ * The base implementation of `_.iteratee`.
+ *
+ * @private
+ * @param {*} [value=_.identity] The value to convert to an iteratee.
+ * @returns {Function} Returns the iteratee.
+ */
+ function baseIteratee(value) {
+ // Don't store the `typeof` result in a variable to avoid a JIT bug in Safari 9.
+ // See https://bugs.webkit.org/show_bug.cgi?id=156034 for more details.
+ if (typeof value == 'function') {
+ return value;
+ }
+ if (value == null) {
+ return identity;
+ }
+ if (typeof value == 'object') {
+ return isArray(value)
+ ? baseMatchesProperty(value[0], value[1])
+ : baseMatches(value);
+ }
+ return property(value);
+ }
+
+ /**
+ * The base implementation of `_.keys` which doesn't treat sparse arrays as dense.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+ function baseKeys(object) {
+ if (!isPrototype(object)) {
+ return nativeKeys(object);
+ }
+ var result = [];
+ for (var key in Object(object)) {
+ if (hasOwnProperty.call(object, key) && key != 'constructor') {
+ result.push(key);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.keysIn` which doesn't treat sparse arrays as dense.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+ function baseKeysIn(object) {
+ if (!isObject(object)) {
+ return nativeKeysIn(object);
+ }
+ var isProto = isPrototype(object),
+ result = [];
+
+ for (var key in object) {
+ if (!(key == 'constructor' && (isProto || !hasOwnProperty.call(object, key)))) {
+ result.push(key);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.lt` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is less than `other`,
+ * else `false`.
+ */
+ function baseLt(value, other) {
+ return value < other;
+ }
+
+ /**
+ * The base implementation of `_.map` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ */
+ function baseMap(collection, iteratee) {
+ var index = -1,
+ result = isArrayLike(collection) ? Array(collection.length) : [];
+
+ baseEach(collection, function(value, key, collection) {
+ result[++index] = iteratee(value, key, collection);
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.matches` which doesn't clone `source`.
+ *
+ * @private
+ * @param {Object} source The object of property values to match.
+ * @returns {Function} Returns the new spec function.
+ */
+ function baseMatches(source) {
+ var matchData = getMatchData(source);
+ if (matchData.length == 1 && matchData[0][2]) {
+ return matchesStrictComparable(matchData[0][0], matchData[0][1]);
+ }
+ return function(object) {
+ return object === source || baseIsMatch(object, source, matchData);
+ };
+ }
+
+ /**
+ * The base implementation of `_.matchesProperty` which doesn't clone `srcValue`.
+ *
+ * @private
+ * @param {string} path The path of the property to get.
+ * @param {*} srcValue The value to match.
+ * @returns {Function} Returns the new spec function.
+ */
+ function baseMatchesProperty(path, srcValue) {
+ if (isKey(path) && isStrictComparable(srcValue)) {
+ return matchesStrictComparable(toKey(path), srcValue);
+ }
+ return function(object) {
+ var objValue = get(object, path);
+ return (objValue === undefined && objValue === srcValue)
+ ? hasIn(object, path)
+ : baseIsEqual(srcValue, objValue, COMPARE_PARTIAL_FLAG | COMPARE_UNORDERED_FLAG);
+ };
+ }
+
+ /**
+ * The base implementation of `_.merge` without support for multiple sources.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {number} srcIndex The index of `source`.
+ * @param {Function} [customizer] The function to customize merged values.
+ * @param {Object} [stack] Tracks traversed source values and their merged
+ * counterparts.
+ */
+ function baseMerge(object, source, srcIndex, customizer, stack) {
+ if (object === source) {
+ return;
+ }
+ baseFor(source, function(srcValue, key) {
+ stack || (stack = new Stack);
+ if (isObject(srcValue)) {
+ baseMergeDeep(object, source, key, srcIndex, baseMerge, customizer, stack);
+ }
+ else {
+ var newValue = customizer
+ ? customizer(safeGet(object, key), srcValue, (key + ''), object, source, stack)
+ : undefined;
+
+ if (newValue === undefined) {
+ newValue = srcValue;
+ }
+ assignMergeValue(object, key, newValue);
+ }
+ }, keysIn);
+ }
+
+ /**
+ * A specialized version of `baseMerge` for arrays and objects which performs
+ * deep merges and tracks traversed objects enabling objects with circular
+ * references to be merged.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {string} key The key of the value to merge.
+ * @param {number} srcIndex The index of `source`.
+ * @param {Function} mergeFunc The function to merge values.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @param {Object} [stack] Tracks traversed source values and their merged
+ * counterparts.
+ */
+ function baseMergeDeep(object, source, key, srcIndex, mergeFunc, customizer, stack) {
+ var objValue = safeGet(object, key),
+ srcValue = safeGet(source, key),
+ stacked = stack.get(srcValue);
+
+ if (stacked) {
+ assignMergeValue(object, key, stacked);
+ return;
+ }
+ var newValue = customizer
+ ? customizer(objValue, srcValue, (key + ''), object, source, stack)
+ : undefined;
+
+ var isCommon = newValue === undefined;
+
+ if (isCommon) {
+ var isArr = isArray(srcValue),
+ isBuff = !isArr && isBuffer(srcValue),
+ isTyped = !isArr && !isBuff && isTypedArray(srcValue);
+
+ newValue = srcValue;
+ if (isArr || isBuff || isTyped) {
+ if (isArray(objValue)) {
+ newValue = objValue;
+ }
+ else if (isArrayLikeObject(objValue)) {
+ newValue = copyArray(objValue);
+ }
+ else if (isBuff) {
+ isCommon = false;
+ newValue = cloneBuffer(srcValue, true);
+ }
+ else if (isTyped) {
+ isCommon = false;
+ newValue = cloneTypedArray(srcValue, true);
+ }
+ else {
+ newValue = [];
+ }
+ }
+ else if (isPlainObject(srcValue) || isArguments(srcValue)) {
+ newValue = objValue;
+ if (isArguments(objValue)) {
+ newValue = toPlainObject(objValue);
+ }
+ else if (!isObject(objValue) || isFunction(objValue)) {
+ newValue = initCloneObject(srcValue);
+ }
+ }
+ else {
+ isCommon = false;
+ }
+ }
+ if (isCommon) {
+ // Recursively merge objects and arrays (susceptible to call stack limits).
+ stack.set(srcValue, newValue);
+ mergeFunc(newValue, srcValue, srcIndex, customizer, stack);
+ stack['delete'](srcValue);
+ }
+ assignMergeValue(object, key, newValue);
+ }
+
+ /**
+ * The base implementation of `_.nth` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {Array} array The array to query.
+ * @param {number} n The index of the element to return.
+ * @returns {*} Returns the nth element of `array`.
+ */
+ function baseNth(array, n) {
+ var length = array.length;
+ if (!length) {
+ return;
+ }
+ n += n < 0 ? length : 0;
+ return isIndex(n, length) ? array[n] : undefined;
+ }
+
+ /**
+ * The base implementation of `_.orderBy` without param guards.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function[]|Object[]|string[]} iteratees The iteratees to sort by.
+ * @param {string[]} orders The sort orders of `iteratees`.
+ * @returns {Array} Returns the new sorted array.
+ */
+ function baseOrderBy(collection, iteratees, orders) {
+ if (iteratees.length) {
+ iteratees = arrayMap(iteratees, function(iteratee) {
+ if (isArray(iteratee)) {
+ return function(value) {
+ return baseGet(value, iteratee.length === 1 ? iteratee[0] : iteratee);
+ }
+ }
+ return iteratee;
+ });
+ } else {
+ iteratees = [identity];
+ }
+
+ var index = -1;
+ iteratees = arrayMap(iteratees, baseUnary(getIteratee()));
+
+ var result = baseMap(collection, function(value, key, collection) {
+ var criteria = arrayMap(iteratees, function(iteratee) {
+ return iteratee(value);
+ });
+ return { 'criteria': criteria, 'index': ++index, 'value': value };
+ });
+
+ return baseSortBy(result, function(object, other) {
+ return compareMultiple(object, other, orders);
+ });
+ }
+
+ /**
+ * The base implementation of `_.pick` without support for individual
+ * property identifiers.
+ *
+ * @private
+ * @param {Object} object The source object.
+ * @param {string[]} paths The property paths to pick.
+ * @returns {Object} Returns the new object.
+ */
+ function basePick(object, paths) {
+ return basePickBy(object, paths, function(value, path) {
+ return hasIn(object, path);
+ });
+ }
+
+ /**
+ * The base implementation of `_.pickBy` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Object} object The source object.
+ * @param {string[]} paths The property paths to pick.
+ * @param {Function} predicate The function invoked per property.
+ * @returns {Object} Returns the new object.
+ */
+ function basePickBy(object, paths, predicate) {
+ var index = -1,
+ length = paths.length,
+ result = {};
+
+ while (++index < length) {
+ var path = paths[index],
+ value = baseGet(object, path);
+
+ if (predicate(value, path)) {
+ baseSet(result, castPath(path, object), value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * A specialized version of `baseProperty` which supports deep paths.
+ *
+ * @private
+ * @param {Array|string} path The path of the property to get.
+ * @returns {Function} Returns the new accessor function.
+ */
+ function basePropertyDeep(path) {
+ return function(object) {
+ return baseGet(object, path);
+ };
+ }
+
+ /**
+ * The base implementation of `_.pullAllBy` without support for iteratee
+ * shorthands.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to remove.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns `array`.
+ */
+ function basePullAll(array, values, iteratee, comparator) {
+ var indexOf = comparator ? baseIndexOfWith : baseIndexOf,
+ index = -1,
+ length = values.length,
+ seen = array;
+
+ if (array === values) {
+ values = copyArray(values);
+ }
+ if (iteratee) {
+ seen = arrayMap(array, baseUnary(iteratee));
+ }
+ while (++index < length) {
+ var fromIndex = 0,
+ value = values[index],
+ computed = iteratee ? iteratee(value) : value;
+
+ while ((fromIndex = indexOf(seen, computed, fromIndex, comparator)) > -1) {
+ if (seen !== array) {
+ splice.call(seen, fromIndex, 1);
+ }
+ splice.call(array, fromIndex, 1);
+ }
+ }
+ return array;
+ }
+
+ /**
+ * The base implementation of `_.pullAt` without support for individual
+ * indexes or capturing the removed elements.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {number[]} indexes The indexes of elements to remove.
+ * @returns {Array} Returns `array`.
+ */
+ function basePullAt(array, indexes) {
+ var length = array ? indexes.length : 0,
+ lastIndex = length - 1;
+
+ while (length--) {
+ var index = indexes[length];
+ if (length == lastIndex || index !== previous) {
+ var previous = index;
+ if (isIndex(index)) {
+ splice.call(array, index, 1);
+ } else {
+ baseUnset(array, index);
+ }
+ }
+ }
+ return array;
+ }
+
+ /**
+ * The base implementation of `_.random` without support for returning
+ * floating-point numbers.
+ *
+ * @private
+ * @param {number} lower The lower bound.
+ * @param {number} upper The upper bound.
+ * @returns {number} Returns the random number.
+ */
+ function baseRandom(lower, upper) {
+ return lower + nativeFloor(nativeRandom() * (upper - lower + 1));
+ }
+
+ /**
+ * The base implementation of `_.range` and `_.rangeRight` which doesn't
+ * coerce arguments.
+ *
+ * @private
+ * @param {number} start The start of the range.
+ * @param {number} end The end of the range.
+ * @param {number} step The value to increment or decrement by.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Array} Returns the range of numbers.
+ */
+ function baseRange(start, end, step, fromRight) {
+ var index = -1,
+ length = nativeMax(nativeCeil((end - start) / (step || 1)), 0),
+ result = Array(length);
+
+ while (length--) {
+ result[fromRight ? length : ++index] = start;
+ start += step;
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.repeat` which doesn't coerce arguments.
+ *
+ * @private
+ * @param {string} string The string to repeat.
+ * @param {number} n The number of times to repeat the string.
+ * @returns {string} Returns the repeated string.
+ */
+ function baseRepeat(string, n) {
+ var result = '';
+ if (!string || n < 1 || n > MAX_SAFE_INTEGER) {
+ return result;
+ }
+ // Leverage the exponentiation by squaring algorithm for a faster repeat.
+ // See https://en.wikipedia.org/wiki/Exponentiation_by_squaring for more details.
+ do {
+ if (n % 2) {
+ result += string;
+ }
+ n = nativeFloor(n / 2);
+ if (n) {
+ string += string;
+ }
+ } while (n);
+
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.rest` which doesn't validate or coerce arguments.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @returns {Function} Returns the new function.
+ */
+ function baseRest(func, start) {
+ return setToString(overRest(func, start, identity), func + '');
+ }
+
+ /**
+ * The base implementation of `_.sample`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to sample.
+ * @returns {*} Returns the random element.
+ */
+ function baseSample(collection) {
+ return arraySample(values(collection));
+ }
+
+ /**
+ * The base implementation of `_.sampleSize` without param guards.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to sample.
+ * @param {number} n The number of elements to sample.
+ * @returns {Array} Returns the random elements.
+ */
+ function baseSampleSize(collection, n) {
+ var array = values(collection);
+ return shuffleSelf(array, baseClamp(n, 0, array.length));
+ }
+
+ /**
+ * The base implementation of `_.set`.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The path of the property to set.
+ * @param {*} value The value to set.
+ * @param {Function} [customizer] The function to customize path creation.
+ * @returns {Object} Returns `object`.
+ */
+ function baseSet(object, path, value, customizer) {
+ if (!isObject(object)) {
+ return object;
+ }
+ path = castPath(path, object);
+
+ var index = -1,
+ length = path.length,
+ lastIndex = length - 1,
+ nested = object;
+
+ while (nested != null && ++index < length) {
+ var key = toKey(path[index]),
+ newValue = value;
+
+ if (key === '__proto__' || key === 'constructor' || key === 'prototype') {
+ return object;
+ }
+
+ if (index != lastIndex) {
+ var objValue = nested[key];
+ newValue = customizer ? customizer(objValue, key, nested) : undefined;
+ if (newValue === undefined) {
+ newValue = isObject(objValue)
+ ? objValue
+ : (isIndex(path[index + 1]) ? [] : {});
+ }
+ }
+ assignValue(nested, key, newValue);
+ nested = nested[key];
+ }
+ return object;
+ }
+
+ /**
+ * The base implementation of `setData` without support for hot loop shorting.
+ *
+ * @private
+ * @param {Function} func The function to associate metadata with.
+ * @param {*} data The metadata.
+ * @returns {Function} Returns `func`.
+ */
+ var baseSetData = !metaMap ? identity : function(func, data) {
+ metaMap.set(func, data);
+ return func;
+ };
+
+ /**
+ * The base implementation of `setToString` without support for hot loop shorting.
+ *
+ * @private
+ * @param {Function} func The function to modify.
+ * @param {Function} string The `toString` result.
+ * @returns {Function} Returns `func`.
+ */
+ var baseSetToString = !defineProperty ? identity : function(func, string) {
+ return defineProperty(func, 'toString', {
+ 'configurable': true,
+ 'enumerable': false,
+ 'value': constant(string),
+ 'writable': true
+ });
+ };
+
+ /**
+ * The base implementation of `_.shuffle`.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to shuffle.
+ * @returns {Array} Returns the new shuffled array.
+ */
+ function baseShuffle(collection) {
+ return shuffleSelf(values(collection));
+ }
+
+ /**
+ * The base implementation of `_.slice` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to slice.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the slice of `array`.
+ */
+ function baseSlice(array, start, end) {
+ var index = -1,
+ length = array.length;
+
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = end > length ? length : end;
+ if (end < 0) {
+ end += length;
+ }
+ length = start > end ? 0 : ((end - start) >>> 0);
+ start >>>= 0;
+
+ var result = Array(length);
+ while (++index < length) {
+ result[index] = array[index + start];
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.some` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ */
+ function baseSome(collection, predicate) {
+ var result;
+
+ baseEach(collection, function(value, index, collection) {
+ result = predicate(value, index, collection);
+ return !result;
+ });
+ return !!result;
+ }
+
+ /**
+ * The base implementation of `_.sortedIndex` and `_.sortedLastIndex` which
+ * performs a binary search of `array` to determine the index at which `value`
+ * should be inserted into `array` in order to maintain its sort order.
+ *
+ * @private
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {boolean} [retHighest] Specify returning the highest qualified index.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ */
+ function baseSortedIndex(array, value, retHighest) {
+ var low = 0,
+ high = array == null ? low : array.length;
+
+ if (typeof value == 'number' && value === value && high <= HALF_MAX_ARRAY_LENGTH) {
+ while (low < high) {
+ var mid = (low + high) >>> 1,
+ computed = array[mid];
+
+ if (computed !== null && !isSymbol(computed) &&
+ (retHighest ? (computed <= value) : (computed < value))) {
+ low = mid + 1;
+ } else {
+ high = mid;
+ }
+ }
+ return high;
+ }
+ return baseSortedIndexBy(array, value, identity, retHighest);
+ }
+
+ /**
+ * The base implementation of `_.sortedIndexBy` and `_.sortedLastIndexBy`
+ * which invokes `iteratee` for `value` and each element of `array` to compute
+ * their sort ranking. The iteratee is invoked with one argument; (value).
+ *
+ * @private
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {Function} iteratee The iteratee invoked per element.
+ * @param {boolean} [retHighest] Specify returning the highest qualified index.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ */
+ function baseSortedIndexBy(array, value, iteratee, retHighest) {
+ var low = 0,
+ high = array == null ? 0 : array.length;
+ if (high === 0) {
+ return 0;
+ }
+
+ value = iteratee(value);
+ var valIsNaN = value !== value,
+ valIsNull = value === null,
+ valIsSymbol = isSymbol(value),
+ valIsUndefined = value === undefined;
+
+ while (low < high) {
+ var mid = nativeFloor((low + high) / 2),
+ computed = iteratee(array[mid]),
+ othIsDefined = computed !== undefined,
+ othIsNull = computed === null,
+ othIsReflexive = computed === computed,
+ othIsSymbol = isSymbol(computed);
+
+ if (valIsNaN) {
+ var setLow = retHighest || othIsReflexive;
+ } else if (valIsUndefined) {
+ setLow = othIsReflexive && (retHighest || othIsDefined);
+ } else if (valIsNull) {
+ setLow = othIsReflexive && othIsDefined && (retHighest || !othIsNull);
+ } else if (valIsSymbol) {
+ setLow = othIsReflexive && othIsDefined && !othIsNull && (retHighest || !othIsSymbol);
+ } else if (othIsNull || othIsSymbol) {
+ setLow = false;
+ } else {
+ setLow = retHighest ? (computed <= value) : (computed < value);
+ }
+ if (setLow) {
+ low = mid + 1;
+ } else {
+ high = mid;
+ }
+ }
+ return nativeMin(high, MAX_ARRAY_INDEX);
+ }
+
+ /**
+ * The base implementation of `_.sortedUniq` and `_.sortedUniqBy` without
+ * support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @returns {Array} Returns the new duplicate free array.
+ */
+ function baseSortedUniq(array, iteratee) {
+ var index = -1,
+ length = array.length,
+ resIndex = 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index],
+ computed = iteratee ? iteratee(value) : value;
+
+ if (!index || !eq(computed, seen)) {
+ var seen = computed;
+ result[resIndex++] = value === 0 ? 0 : value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.toNumber` which doesn't ensure correct
+ * conversions of binary, hexadecimal, or octal string values.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {number} Returns the number.
+ */
+ function baseToNumber(value) {
+ if (typeof value == 'number') {
+ return value;
+ }
+ if (isSymbol(value)) {
+ return NAN;
+ }
+ return +value;
+ }
+
+ /**
+ * The base implementation of `_.toString` which doesn't convert nullish
+ * values to empty strings.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {string} Returns the string.
+ */
+ function baseToString(value) {
+ // Exit early for strings to avoid a performance hit in some environments.
+ if (typeof value == 'string') {
+ return value;
+ }
+ if (isArray(value)) {
+ // Recursively convert values (susceptible to call stack limits).
+ return arrayMap(value, baseToString) + '';
+ }
+ if (isSymbol(value)) {
+ return symbolToString ? symbolToString.call(value) : '';
+ }
+ var result = (value + '');
+ return (result == '0' && (1 / value) == -INFINITY) ? '-0' : result;
+ }
+
+ /**
+ * The base implementation of `_.uniqBy` without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new duplicate free array.
+ */
+ function baseUniq(array, iteratee, comparator) {
+ var index = -1,
+ includes = arrayIncludes,
+ length = array.length,
+ isCommon = true,
+ result = [],
+ seen = result;
+
+ if (comparator) {
+ isCommon = false;
+ includes = arrayIncludesWith;
+ }
+ else if (length >= LARGE_ARRAY_SIZE) {
+ var set = iteratee ? null : createSet(array);
+ if (set) {
+ return setToArray(set);
+ }
+ isCommon = false;
+ includes = cacheHas;
+ seen = new SetCache;
+ }
+ else {
+ seen = iteratee ? [] : result;
+ }
+ outer:
+ while (++index < length) {
+ var value = array[index],
+ computed = iteratee ? iteratee(value) : value;
+
+ value = (comparator || value !== 0) ? value : 0;
+ if (isCommon && computed === computed) {
+ var seenIndex = seen.length;
+ while (seenIndex--) {
+ if (seen[seenIndex] === computed) {
+ continue outer;
+ }
+ }
+ if (iteratee) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ else if (!includes(seen, computed, comparator)) {
+ if (seen !== result) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.unset`.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The property path to unset.
+ * @returns {boolean} Returns `true` if the property is deleted, else `false`.
+ */
+ function baseUnset(object, path) {
+ path = castPath(path, object);
+ object = parent(object, path);
+ return object == null || delete object[toKey(last(path))];
+ }
+
+ /**
+ * The base implementation of `_.update`.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The path of the property to update.
+ * @param {Function} updater The function to produce the updated value.
+ * @param {Function} [customizer] The function to customize path creation.
+ * @returns {Object} Returns `object`.
+ */
+ function baseUpdate(object, path, updater, customizer) {
+ return baseSet(object, path, updater(baseGet(object, path)), customizer);
+ }
+
+ /**
+ * The base implementation of methods like `_.dropWhile` and `_.takeWhile`
+ * without support for iteratee shorthands.
+ *
+ * @private
+ * @param {Array} array The array to query.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {boolean} [isDrop] Specify dropping elements instead of taking them.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Array} Returns the slice of `array`.
+ */
+ function baseWhile(array, predicate, isDrop, fromRight) {
+ var length = array.length,
+ index = fromRight ? length : -1;
+
+ while ((fromRight ? index-- : ++index < length) &&
+ predicate(array[index], index, array)) {}
+
+ return isDrop
+ ? baseSlice(array, (fromRight ? 0 : index), (fromRight ? index + 1 : length))
+ : baseSlice(array, (fromRight ? index + 1 : 0), (fromRight ? length : index));
+ }
+
+ /**
+ * The base implementation of `wrapperValue` which returns the result of
+ * performing a sequence of actions on the unwrapped `value`, where each
+ * successive action is supplied the return value of the previous.
+ *
+ * @private
+ * @param {*} value The unwrapped value.
+ * @param {Array} actions Actions to perform to resolve the unwrapped value.
+ * @returns {*} Returns the resolved value.
+ */
+ function baseWrapperValue(value, actions) {
+ var result = value;
+ if (result instanceof LazyWrapper) {
+ result = result.value();
+ }
+ return arrayReduce(actions, function(result, action) {
+ return action.func.apply(action.thisArg, arrayPush([result], action.args));
+ }, result);
+ }
+
+ /**
+ * The base implementation of methods like `_.xor`, without support for
+ * iteratee shorthands, that accepts an array of arrays to inspect.
+ *
+ * @private
+ * @param {Array} arrays The arrays to inspect.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of values.
+ */
+ function baseXor(arrays, iteratee, comparator) {
+ var length = arrays.length;
+ if (length < 2) {
+ return length ? baseUniq(arrays[0]) : [];
+ }
+ var index = -1,
+ result = Array(length);
+
+ while (++index < length) {
+ var array = arrays[index],
+ othIndex = -1;
+
+ while (++othIndex < length) {
+ if (othIndex != index) {
+ result[index] = baseDifference(result[index] || array, arrays[othIndex], iteratee, comparator);
+ }
+ }
+ }
+ return baseUniq(baseFlatten(result, 1), iteratee, comparator);
+ }
+
+ /**
+ * This base implementation of `_.zipObject` which assigns values using `assignFunc`.
+ *
+ * @private
+ * @param {Array} props The property identifiers.
+ * @param {Array} values The property values.
+ * @param {Function} assignFunc The function to assign values.
+ * @returns {Object} Returns the new object.
+ */
+ function baseZipObject(props, values, assignFunc) {
+ var index = -1,
+ length = props.length,
+ valsLength = values.length,
+ result = {};
+
+ while (++index < length) {
+ var value = index < valsLength ? values[index] : undefined;
+ assignFunc(result, props[index], value);
+ }
+ return result;
+ }
+
+ /**
+ * Casts `value` to an empty array if it's not an array like object.
+ *
+ * @private
+ * @param {*} value The value to inspect.
+ * @returns {Array|Object} Returns the cast array-like object.
+ */
+ function castArrayLikeObject(value) {
+ return isArrayLikeObject(value) ? value : [];
+ }
+
+ /**
+ * Casts `value` to `identity` if it's not a function.
+ *
+ * @private
+ * @param {*} value The value to inspect.
+ * @returns {Function} Returns cast function.
+ */
+ function castFunction(value) {
+ return typeof value == 'function' ? value : identity;
+ }
+
+ /**
+ * Casts `value` to a path array if it's not one.
+ *
+ * @private
+ * @param {*} value The value to inspect.
+ * @param {Object} [object] The object to query keys on.
+ * @returns {Array} Returns the cast property path array.
+ */
+ function castPath(value, object) {
+ if (isArray(value)) {
+ return value;
+ }
+ return isKey(value, object) ? [value] : stringToPath(toString(value));
+ }
+
+ /**
+ * A `baseRest` alias which can be replaced with `identity` by module
+ * replacement plugins.
+ *
+ * @private
+ * @type {Function}
+ * @param {Function} func The function to apply a rest parameter to.
+ * @returns {Function} Returns the new function.
+ */
+ var castRest = baseRest;
+
+ /**
+ * Casts `array` to a slice if it's needed.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {number} start The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the cast slice.
+ */
+ function castSlice(array, start, end) {
+ var length = array.length;
+ end = end === undefined ? length : end;
+ return (!start && end >= length) ? array : baseSlice(array, start, end);
+ }
+
+ /**
+ * A simple wrapper around the global [`clearTimeout`](https://mdn.io/clearTimeout).
+ *
+ * @private
+ * @param {number|Object} id The timer id or timeout object of the timer to clear.
+ */
+ var clearTimeout = ctxClearTimeout || function(id) {
+ return root.clearTimeout(id);
+ };
+
+ /**
+ * Creates a clone of `buffer`.
+ *
+ * @private
+ * @param {Buffer} buffer The buffer to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Buffer} Returns the cloned buffer.
+ */
+ function cloneBuffer(buffer, isDeep) {
+ if (isDeep) {
+ return buffer.slice();
+ }
+ var length = buffer.length,
+ result = allocUnsafe ? allocUnsafe(length) : new buffer.constructor(length);
+
+ buffer.copy(result);
+ return result;
+ }
+
+ /**
+ * Creates a clone of `arrayBuffer`.
+ *
+ * @private
+ * @param {ArrayBuffer} arrayBuffer The array buffer to clone.
+ * @returns {ArrayBuffer} Returns the cloned array buffer.
+ */
+ function cloneArrayBuffer(arrayBuffer) {
+ var result = new arrayBuffer.constructor(arrayBuffer.byteLength);
+ new Uint8Array(result).set(new Uint8Array(arrayBuffer));
+ return result;
+ }
+
+ /**
+ * Creates a clone of `dataView`.
+ *
+ * @private
+ * @param {Object} dataView The data view to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the cloned data view.
+ */
+ function cloneDataView(dataView, isDeep) {
+ var buffer = isDeep ? cloneArrayBuffer(dataView.buffer) : dataView.buffer;
+ return new dataView.constructor(buffer, dataView.byteOffset, dataView.byteLength);
+ }
+
+ /**
+ * Creates a clone of `regexp`.
+ *
+ * @private
+ * @param {Object} regexp The regexp to clone.
+ * @returns {Object} Returns the cloned regexp.
+ */
+ function cloneRegExp(regexp) {
+ var result = new regexp.constructor(regexp.source, reFlags.exec(regexp));
+ result.lastIndex = regexp.lastIndex;
+ return result;
+ }
+
+ /**
+ * Creates a clone of the `symbol` object.
+ *
+ * @private
+ * @param {Object} symbol The symbol object to clone.
+ * @returns {Object} Returns the cloned symbol object.
+ */
+ function cloneSymbol(symbol) {
+ return symbolValueOf ? Object(symbolValueOf.call(symbol)) : {};
+ }
+
+ /**
+ * Creates a clone of `typedArray`.
+ *
+ * @private
+ * @param {Object} typedArray The typed array to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the cloned typed array.
+ */
+ function cloneTypedArray(typedArray, isDeep) {
+ var buffer = isDeep ? cloneArrayBuffer(typedArray.buffer) : typedArray.buffer;
+ return new typedArray.constructor(buffer, typedArray.byteOffset, typedArray.length);
+ }
+
+ /**
+ * Compares values to sort them in ascending order.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {number} Returns the sort order indicator for `value`.
+ */
+ function compareAscending(value, other) {
+ if (value !== other) {
+ var valIsDefined = value !== undefined,
+ valIsNull = value === null,
+ valIsReflexive = value === value,
+ valIsSymbol = isSymbol(value);
+
+ var othIsDefined = other !== undefined,
+ othIsNull = other === null,
+ othIsReflexive = other === other,
+ othIsSymbol = isSymbol(other);
+
+ if ((!othIsNull && !othIsSymbol && !valIsSymbol && value > other) ||
+ (valIsSymbol && othIsDefined && othIsReflexive && !othIsNull && !othIsSymbol) ||
+ (valIsNull && othIsDefined && othIsReflexive) ||
+ (!valIsDefined && othIsReflexive) ||
+ !valIsReflexive) {
+ return 1;
+ }
+ if ((!valIsNull && !valIsSymbol && !othIsSymbol && value < other) ||
+ (othIsSymbol && valIsDefined && valIsReflexive && !valIsNull && !valIsSymbol) ||
+ (othIsNull && valIsDefined && valIsReflexive) ||
+ (!othIsDefined && valIsReflexive) ||
+ !othIsReflexive) {
+ return -1;
+ }
+ }
+ return 0;
+ }
+
+ /**
+ * Used by `_.orderBy` to compare multiple properties of a value to another
+ * and stable sort them.
+ *
+ * If `orders` is unspecified, all values are sorted in ascending order. Otherwise,
+ * specify an order of "desc" for descending or "asc" for ascending sort order
+ * of corresponding values.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {boolean[]|string[]} orders The order to sort by for each property.
+ * @returns {number} Returns the sort order indicator for `object`.
+ */
+ function compareMultiple(object, other, orders) {
+ var index = -1,
+ objCriteria = object.criteria,
+ othCriteria = other.criteria,
+ length = objCriteria.length,
+ ordersLength = orders.length;
+
+ while (++index < length) {
+ var result = compareAscending(objCriteria[index], othCriteria[index]);
+ if (result) {
+ if (index >= ordersLength) {
+ return result;
+ }
+ var order = orders[index];
+ return result * (order == 'desc' ? -1 : 1);
+ }
+ }
+ // Fixes an `Array#sort` bug in the JS engine embedded in Adobe applications
+ // that causes it, under certain circumstances, to provide the same value for
+ // `object` and `other`. See https://github.com/jashkenas/underscore/pull/1247
+ // for more details.
+ //
+ // This also ensures a stable sort in V8 and other engines.
+ // See https://bugs.chromium.org/p/v8/issues/detail?id=90 for more details.
+ return object.index - other.index;
+ }
+
+ /**
+ * Creates an array that is the composition of partially applied arguments,
+ * placeholders, and provided arguments into a single array of arguments.
+ *
+ * @private
+ * @param {Array} args The provided arguments.
+ * @param {Array} partials The arguments to prepend to those provided.
+ * @param {Array} holders The `partials` placeholder indexes.
+ * @params {boolean} [isCurried] Specify composing for a curried function.
+ * @returns {Array} Returns the new array of composed arguments.
+ */
+ function composeArgs(args, partials, holders, isCurried) {
+ var argsIndex = -1,
+ argsLength = args.length,
+ holdersLength = holders.length,
+ leftIndex = -1,
+ leftLength = partials.length,
+ rangeLength = nativeMax(argsLength - holdersLength, 0),
+ result = Array(leftLength + rangeLength),
+ isUncurried = !isCurried;
+
+ while (++leftIndex < leftLength) {
+ result[leftIndex] = partials[leftIndex];
+ }
+ while (++argsIndex < holdersLength) {
+ if (isUncurried || argsIndex < argsLength) {
+ result[holders[argsIndex]] = args[argsIndex];
+ }
+ }
+ while (rangeLength--) {
+ result[leftIndex++] = args[argsIndex++];
+ }
+ return result;
+ }
+
+ /**
+ * This function is like `composeArgs` except that the arguments composition
+ * is tailored for `_.partialRight`.
+ *
+ * @private
+ * @param {Array} args The provided arguments.
+ * @param {Array} partials The arguments to append to those provided.
+ * @param {Array} holders The `partials` placeholder indexes.
+ * @params {boolean} [isCurried] Specify composing for a curried function.
+ * @returns {Array} Returns the new array of composed arguments.
+ */
+ function composeArgsRight(args, partials, holders, isCurried) {
+ var argsIndex = -1,
+ argsLength = args.length,
+ holdersIndex = -1,
+ holdersLength = holders.length,
+ rightIndex = -1,
+ rightLength = partials.length,
+ rangeLength = nativeMax(argsLength - holdersLength, 0),
+ result = Array(rangeLength + rightLength),
+ isUncurried = !isCurried;
+
+ while (++argsIndex < rangeLength) {
+ result[argsIndex] = args[argsIndex];
+ }
+ var offset = argsIndex;
+ while (++rightIndex < rightLength) {
+ result[offset + rightIndex] = partials[rightIndex];
+ }
+ while (++holdersIndex < holdersLength) {
+ if (isUncurried || argsIndex < argsLength) {
+ result[offset + holders[holdersIndex]] = args[argsIndex++];
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Copies the values of `source` to `array`.
+ *
+ * @private
+ * @param {Array} source The array to copy values from.
+ * @param {Array} [array=[]] The array to copy values to.
+ * @returns {Array} Returns `array`.
+ */
+ function copyArray(source, array) {
+ var index = -1,
+ length = source.length;
+
+ array || (array = Array(length));
+ while (++index < length) {
+ array[index] = source[index];
+ }
+ return array;
+ }
+
+ /**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property identifiers to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @param {Function} [customizer] The function to customize copied values.
+ * @returns {Object} Returns `object`.
+ */
+ function copyObject(source, props, object, customizer) {
+ var isNew = !object;
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+
+ var newValue = customizer
+ ? customizer(object[key], source[key], key, object, source)
+ : undefined;
+
+ if (newValue === undefined) {
+ newValue = source[key];
+ }
+ if (isNew) {
+ baseAssignValue(object, key, newValue);
+ } else {
+ assignValue(object, key, newValue);
+ }
+ }
+ return object;
+ }
+
+ /**
+ * Copies own symbols of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy symbols from.
+ * @param {Object} [object={}] The object to copy symbols to.
+ * @returns {Object} Returns `object`.
+ */
+ function copySymbols(source, object) {
+ return copyObject(source, getSymbols(source), object);
+ }
+
+ /**
+ * Copies own and inherited symbols of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy symbols from.
+ * @param {Object} [object={}] The object to copy symbols to.
+ * @returns {Object} Returns `object`.
+ */
+ function copySymbolsIn(source, object) {
+ return copyObject(source, getSymbolsIn(source), object);
+ }
+
+ /**
+ * Creates a function like `_.groupBy`.
+ *
+ * @private
+ * @param {Function} setter The function to set accumulator values.
+ * @param {Function} [initializer] The accumulator object initializer.
+ * @returns {Function} Returns the new aggregator function.
+ */
+ function createAggregator(setter, initializer) {
+ return function(collection, iteratee) {
+ var func = isArray(collection) ? arrayAggregator : baseAggregator,
+ accumulator = initializer ? initializer() : {};
+
+ return func(collection, setter, getIteratee(iteratee, 2), accumulator);
+ };
+ }
+
+ /**
+ * Creates a function like `_.assign`.
+ *
+ * @private
+ * @param {Function} assigner The function to assign values.
+ * @returns {Function} Returns the new assigner function.
+ */
+ function createAssigner(assigner) {
+ return baseRest(function(object, sources) {
+ var index = -1,
+ length = sources.length,
+ customizer = length > 1 ? sources[length - 1] : undefined,
+ guard = length > 2 ? sources[2] : undefined;
+
+ customizer = (assigner.length > 3 && typeof customizer == 'function')
+ ? (length--, customizer)
+ : undefined;
+
+ if (guard && isIterateeCall(sources[0], sources[1], guard)) {
+ customizer = length < 3 ? undefined : customizer;
+ length = 1;
+ }
+ object = Object(object);
+ while (++index < length) {
+ var source = sources[index];
+ if (source) {
+ assigner(object, source, index, customizer);
+ }
+ }
+ return object;
+ });
+ }
+
+ /**
+ * Creates a `baseEach` or `baseEachRight` function.
+ *
+ * @private
+ * @param {Function} eachFunc The function to iterate over a collection.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+ function createBaseEach(eachFunc, fromRight) {
+ return function(collection, iteratee) {
+ if (collection == null) {
+ return collection;
+ }
+ if (!isArrayLike(collection)) {
+ return eachFunc(collection, iteratee);
+ }
+ var length = collection.length,
+ index = fromRight ? length : -1,
+ iterable = Object(collection);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ if (iteratee(iterable[index], index, iterable) === false) {
+ break;
+ }
+ }
+ return collection;
+ };
+ }
+
+ /**
+ * Creates a base function for methods like `_.forIn` and `_.forOwn`.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+ function createBaseFor(fromRight) {
+ return function(object, iteratee, keysFunc) {
+ var index = -1,
+ iterable = Object(object),
+ props = keysFunc(object),
+ length = props.length;
+
+ while (length--) {
+ var key = props[fromRight ? length : ++index];
+ if (iteratee(iterable[key], key, iterable) === false) {
+ break;
+ }
+ }
+ return object;
+ };
+ }
+
+ /**
+ * Creates a function that wraps `func` to invoke it with the optional `this`
+ * binding of `thisArg`.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+ function createBind(func, bitmask, thisArg) {
+ var isBind = bitmask & WRAP_BIND_FLAG,
+ Ctor = createCtor(func);
+
+ function wrapper() {
+ var fn = (this && this !== root && this instanceof wrapper) ? Ctor : func;
+ return fn.apply(isBind ? thisArg : this, arguments);
+ }
+ return wrapper;
+ }
+
+ /**
+ * Creates a function like `_.lowerFirst`.
+ *
+ * @private
+ * @param {string} methodName The name of the `String` case method to use.
+ * @returns {Function} Returns the new case function.
+ */
+ function createCaseFirst(methodName) {
+ return function(string) {
+ string = toString(string);
+
+ var strSymbols = hasUnicode(string)
+ ? stringToArray(string)
+ : undefined;
+
+ var chr = strSymbols
+ ? strSymbols[0]
+ : string.charAt(0);
+
+ var trailing = strSymbols
+ ? castSlice(strSymbols, 1).join('')
+ : string.slice(1);
+
+ return chr[methodName]() + trailing;
+ };
+ }
+
+ /**
+ * Creates a function like `_.camelCase`.
+ *
+ * @private
+ * @param {Function} callback The function to combine each word.
+ * @returns {Function} Returns the new compounder function.
+ */
+ function createCompounder(callback) {
+ return function(string) {
+ return arrayReduce(words(deburr(string).replace(reApos, '')), callback, '');
+ };
+ }
+
+ /**
+ * Creates a function that produces an instance of `Ctor` regardless of
+ * whether it was invoked as part of a `new` expression or by `call` or `apply`.
+ *
+ * @private
+ * @param {Function} Ctor The constructor to wrap.
+ * @returns {Function} Returns the new wrapped function.
+ */
+ function createCtor(Ctor) {
+ return function() {
+ // Use a `switch` statement to work with class constructors. See
+ // http://ecma-international.org/ecma-262/7.0/#sec-ecmascript-function-objects-call-thisargument-argumentslist
+ // for more details.
+ var args = arguments;
+ switch (args.length) {
+ case 0: return new Ctor;
+ case 1: return new Ctor(args[0]);
+ case 2: return new Ctor(args[0], args[1]);
+ case 3: return new Ctor(args[0], args[1], args[2]);
+ case 4: return new Ctor(args[0], args[1], args[2], args[3]);
+ case 5: return new Ctor(args[0], args[1], args[2], args[3], args[4]);
+ case 6: return new Ctor(args[0], args[1], args[2], args[3], args[4], args[5]);
+ case 7: return new Ctor(args[0], args[1], args[2], args[3], args[4], args[5], args[6]);
+ }
+ var thisBinding = baseCreate(Ctor.prototype),
+ result = Ctor.apply(thisBinding, args);
+
+ // Mimic the constructor's `return` behavior.
+ // See https://es5.github.io/#x13.2.2 for more details.
+ return isObject(result) ? result : thisBinding;
+ };
+ }
+
+ /**
+ * Creates a function that wraps `func` to enable currying.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {number} arity The arity of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+ function createCurry(func, bitmask, arity) {
+ var Ctor = createCtor(func);
+
+ function wrapper() {
+ var length = arguments.length,
+ args = Array(length),
+ index = length,
+ placeholder = getHolder(wrapper);
+
+ while (index--) {
+ args[index] = arguments[index];
+ }
+ var holders = (length < 3 && args[0] !== placeholder && args[length - 1] !== placeholder)
+ ? []
+ : replaceHolders(args, placeholder);
+
+ length -= holders.length;
+ if (length < arity) {
+ return createRecurry(
+ func, bitmask, createHybrid, wrapper.placeholder, undefined,
+ args, holders, undefined, undefined, arity - length);
+ }
+ var fn = (this && this !== root && this instanceof wrapper) ? Ctor : func;
+ return apply(fn, this, args);
+ }
+ return wrapper;
+ }
+
+ /**
+ * Creates a `_.find` or `_.findLast` function.
+ *
+ * @private
+ * @param {Function} findIndexFunc The function to find the collection index.
+ * @returns {Function} Returns the new find function.
+ */
+ function createFind(findIndexFunc) {
+ return function(collection, predicate, fromIndex) {
+ var iterable = Object(collection);
+ if (!isArrayLike(collection)) {
+ var iteratee = getIteratee(predicate, 3);
+ collection = keys(collection);
+ predicate = function(key) { return iteratee(iterable[key], key, iterable); };
+ }
+ var index = findIndexFunc(collection, predicate, fromIndex);
+ return index > -1 ? iterable[iteratee ? collection[index] : index] : undefined;
+ };
+ }
+
+ /**
+ * Creates a `_.flow` or `_.flowRight` function.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new flow function.
+ */
+ function createFlow(fromRight) {
+ return flatRest(function(funcs) {
+ var length = funcs.length,
+ index = length,
+ prereq = LodashWrapper.prototype.thru;
+
+ if (fromRight) {
+ funcs.reverse();
+ }
+ while (index--) {
+ var func = funcs[index];
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ if (prereq && !wrapper && getFuncName(func) == 'wrapper') {
+ var wrapper = new LodashWrapper([], true);
+ }
+ }
+ index = wrapper ? index : length;
+ while (++index < length) {
+ func = funcs[index];
+
+ var funcName = getFuncName(func),
+ data = funcName == 'wrapper' ? getData(func) : undefined;
+
+ if (data && isLaziable(data[0]) &&
+ data[1] == (WRAP_ARY_FLAG | WRAP_CURRY_FLAG | WRAP_PARTIAL_FLAG | WRAP_REARG_FLAG) &&
+ !data[4].length && data[9] == 1
+ ) {
+ wrapper = wrapper[getFuncName(data[0])].apply(wrapper, data[3]);
+ } else {
+ wrapper = (func.length == 1 && isLaziable(func))
+ ? wrapper[funcName]()
+ : wrapper.thru(func);
+ }
+ }
+ return function() {
+ var args = arguments,
+ value = args[0];
+
+ if (wrapper && args.length == 1 && isArray(value)) {
+ return wrapper.plant(value).value();
+ }
+ var index = 0,
+ result = length ? funcs[index].apply(this, args) : value;
+
+ while (++index < length) {
+ result = funcs[index].call(this, result);
+ }
+ return result;
+ };
+ });
+ }
+
+ /**
+ * Creates a function that wraps `func` to invoke it with optional `this`
+ * binding of `thisArg`, partial application, and currying.
+ *
+ * @private
+ * @param {Function|string} func The function or method name to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {Array} [partials] The arguments to prepend to those provided to
+ * the new function.
+ * @param {Array} [holders] The `partials` placeholder indexes.
+ * @param {Array} [partialsRight] The arguments to append to those provided
+ * to the new function.
+ * @param {Array} [holdersRight] The `partialsRight` placeholder indexes.
+ * @param {Array} [argPos] The argument positions of the new function.
+ * @param {number} [ary] The arity cap of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+ function createHybrid(func, bitmask, thisArg, partials, holders, partialsRight, holdersRight, argPos, ary, arity) {
+ var isAry = bitmask & WRAP_ARY_FLAG,
+ isBind = bitmask & WRAP_BIND_FLAG,
+ isBindKey = bitmask & WRAP_BIND_KEY_FLAG,
+ isCurried = bitmask & (WRAP_CURRY_FLAG | WRAP_CURRY_RIGHT_FLAG),
+ isFlip = bitmask & WRAP_FLIP_FLAG,
+ Ctor = isBindKey ? undefined : createCtor(func);
+
+ function wrapper() {
+ var length = arguments.length,
+ args = Array(length),
+ index = length;
+
+ while (index--) {
+ args[index] = arguments[index];
+ }
+ if (isCurried) {
+ var placeholder = getHolder(wrapper),
+ holdersCount = countHolders(args, placeholder);
+ }
+ if (partials) {
+ args = composeArgs(args, partials, holders, isCurried);
+ }
+ if (partialsRight) {
+ args = composeArgsRight(args, partialsRight, holdersRight, isCurried);
+ }
+ length -= holdersCount;
+ if (isCurried && length < arity) {
+ var newHolders = replaceHolders(args, placeholder);
+ return createRecurry(
+ func, bitmask, createHybrid, wrapper.placeholder, thisArg,
+ args, newHolders, argPos, ary, arity - length
+ );
+ }
+ var thisBinding = isBind ? thisArg : this,
+ fn = isBindKey ? thisBinding[func] : func;
+
+ length = args.length;
+ if (argPos) {
+ args = reorder(args, argPos);
+ } else if (isFlip && length > 1) {
+ args.reverse();
+ }
+ if (isAry && ary < length) {
+ args.length = ary;
+ }
+ if (this && this !== root && this instanceof wrapper) {
+ fn = Ctor || createCtor(fn);
+ }
+ return fn.apply(thisBinding, args);
+ }
+ return wrapper;
+ }
+
+ /**
+ * Creates a function like `_.invertBy`.
+ *
+ * @private
+ * @param {Function} setter The function to set accumulator values.
+ * @param {Function} toIteratee The function to resolve iteratees.
+ * @returns {Function} Returns the new inverter function.
+ */
+ function createInverter(setter, toIteratee) {
+ return function(object, iteratee) {
+ return baseInverter(object, setter, toIteratee(iteratee), {});
+ };
+ }
+
+ /**
+ * Creates a function that performs a mathematical operation on two values.
+ *
+ * @private
+ * @param {Function} operator The function to perform the operation.
+ * @param {number} [defaultValue] The value used for `undefined` arguments.
+ * @returns {Function} Returns the new mathematical operation function.
+ */
+ function createMathOperation(operator, defaultValue) {
+ return function(value, other) {
+ var result;
+ if (value === undefined && other === undefined) {
+ return defaultValue;
+ }
+ if (value !== undefined) {
+ result = value;
+ }
+ if (other !== undefined) {
+ if (result === undefined) {
+ return other;
+ }
+ if (typeof value == 'string' || typeof other == 'string') {
+ value = baseToString(value);
+ other = baseToString(other);
+ } else {
+ value = baseToNumber(value);
+ other = baseToNumber(other);
+ }
+ result = operator(value, other);
+ }
+ return result;
+ };
+ }
+
+ /**
+ * Creates a function like `_.over`.
+ *
+ * @private
+ * @param {Function} arrayFunc The function to iterate over iteratees.
+ * @returns {Function} Returns the new over function.
+ */
+ function createOver(arrayFunc) {
+ return flatRest(function(iteratees) {
+ iteratees = arrayMap(iteratees, baseUnary(getIteratee()));
+ return baseRest(function(args) {
+ var thisArg = this;
+ return arrayFunc(iteratees, function(iteratee) {
+ return apply(iteratee, thisArg, args);
+ });
+ });
+ });
+ }
+
+ /**
+ * Creates the padding for `string` based on `length`. The `chars` string
+ * is truncated if the number of characters exceeds `length`.
+ *
+ * @private
+ * @param {number} length The padding length.
+ * @param {string} [chars=' '] The string used as padding.
+ * @returns {string} Returns the padding for `string`.
+ */
+ function createPadding(length, chars) {
+ chars = chars === undefined ? ' ' : baseToString(chars);
+
+ var charsLength = chars.length;
+ if (charsLength < 2) {
+ return charsLength ? baseRepeat(chars, length) : chars;
+ }
+ var result = baseRepeat(chars, nativeCeil(length / stringSize(chars)));
+ return hasUnicode(chars)
+ ? castSlice(stringToArray(result), 0, length).join('')
+ : result.slice(0, length);
+ }
+
+ /**
+ * Creates a function that wraps `func` to invoke it with the `this` binding
+ * of `thisArg` and `partials` prepended to the arguments it receives.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {Array} partials The arguments to prepend to those provided to
+ * the new function.
+ * @returns {Function} Returns the new wrapped function.
+ */
+ function createPartial(func, bitmask, thisArg, partials) {
+ var isBind = bitmask & WRAP_BIND_FLAG,
+ Ctor = createCtor(func);
+
+ function wrapper() {
+ var argsIndex = -1,
+ argsLength = arguments.length,
+ leftIndex = -1,
+ leftLength = partials.length,
+ args = Array(leftLength + argsLength),
+ fn = (this && this !== root && this instanceof wrapper) ? Ctor : func;
+
+ while (++leftIndex < leftLength) {
+ args[leftIndex] = partials[leftIndex];
+ }
+ while (argsLength--) {
+ args[leftIndex++] = arguments[++argsIndex];
+ }
+ return apply(fn, isBind ? thisArg : this, args);
+ }
+ return wrapper;
+ }
+
+ /**
+ * Creates a `_.range` or `_.rangeRight` function.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new range function.
+ */
+ function createRange(fromRight) {
+ return function(start, end, step) {
+ if (step && typeof step != 'number' && isIterateeCall(start, end, step)) {
+ end = step = undefined;
+ }
+ // Ensure the sign of `-0` is preserved.
+ start = toFinite(start);
+ if (end === undefined) {
+ end = start;
+ start = 0;
+ } else {
+ end = toFinite(end);
+ }
+ step = step === undefined ? (start < end ? 1 : -1) : toFinite(step);
+ return baseRange(start, end, step, fromRight);
+ };
+ }
+
+ /**
+ * Creates a function that performs a relational operation on two values.
+ *
+ * @private
+ * @param {Function} operator The function to perform the operation.
+ * @returns {Function} Returns the new relational operation function.
+ */
+ function createRelationalOperation(operator) {
+ return function(value, other) {
+ if (!(typeof value == 'string' && typeof other == 'string')) {
+ value = toNumber(value);
+ other = toNumber(other);
+ }
+ return operator(value, other);
+ };
+ }
+
+ /**
+ * Creates a function that wraps `func` to continue currying.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @param {Function} wrapFunc The function to create the `func` wrapper.
+ * @param {*} placeholder The placeholder value.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {Array} [partials] The arguments to prepend to those provided to
+ * the new function.
+ * @param {Array} [holders] The `partials` placeholder indexes.
+ * @param {Array} [argPos] The argument positions of the new function.
+ * @param {number} [ary] The arity cap of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+ function createRecurry(func, bitmask, wrapFunc, placeholder, thisArg, partials, holders, argPos, ary, arity) {
+ var isCurry = bitmask & WRAP_CURRY_FLAG,
+ newHolders = isCurry ? holders : undefined,
+ newHoldersRight = isCurry ? undefined : holders,
+ newPartials = isCurry ? partials : undefined,
+ newPartialsRight = isCurry ? undefined : partials;
+
+ bitmask |= (isCurry ? WRAP_PARTIAL_FLAG : WRAP_PARTIAL_RIGHT_FLAG);
+ bitmask &= ~(isCurry ? WRAP_PARTIAL_RIGHT_FLAG : WRAP_PARTIAL_FLAG);
+
+ if (!(bitmask & WRAP_CURRY_BOUND_FLAG)) {
+ bitmask &= ~(WRAP_BIND_FLAG | WRAP_BIND_KEY_FLAG);
+ }
+ var newData = [
+ func, bitmask, thisArg, newPartials, newHolders, newPartialsRight,
+ newHoldersRight, argPos, ary, arity
+ ];
+
+ var result = wrapFunc.apply(undefined, newData);
+ if (isLaziable(func)) {
+ setData(result, newData);
+ }
+ result.placeholder = placeholder;
+ return setWrapToString(result, func, bitmask);
+ }
+
+ /**
+ * Creates a function like `_.round`.
+ *
+ * @private
+ * @param {string} methodName The name of the `Math` method to use when rounding.
+ * @returns {Function} Returns the new round function.
+ */
+ function createRound(methodName) {
+ var func = Math[methodName];
+ return function(number, precision) {
+ number = toNumber(number);
+ precision = precision == null ? 0 : nativeMin(toInteger(precision), 292);
+ if (precision && nativeIsFinite(number)) {
+ // Shift with exponential notation to avoid floating-point issues.
+ // See [MDN](https://mdn.io/round#Examples) for more details.
+ var pair = (toString(number) + 'e').split('e'),
+ value = func(pair[0] + 'e' + (+pair[1] + precision));
+
+ pair = (toString(value) + 'e').split('e');
+ return +(pair[0] + 'e' + (+pair[1] - precision));
+ }
+ return func(number);
+ };
+ }
+
+ /**
+ * Creates a set object of `values`.
+ *
+ * @private
+ * @param {Array} values The values to add to the set.
+ * @returns {Object} Returns the new set.
+ */
+ var createSet = !(Set && (1 / setToArray(new Set([,-0]))[1]) == INFINITY) ? noop : function(values) {
+ return new Set(values);
+ };
+
+ /**
+ * Creates a `_.toPairs` or `_.toPairsIn` function.
+ *
+ * @private
+ * @param {Function} keysFunc The function to get the keys of a given object.
+ * @returns {Function} Returns the new pairs function.
+ */
+ function createToPairs(keysFunc) {
+ return function(object) {
+ var tag = getTag(object);
+ if (tag == mapTag) {
+ return mapToArray(object);
+ }
+ if (tag == setTag) {
+ return setToPairs(object);
+ }
+ return baseToPairs(object, keysFunc(object));
+ };
+ }
+
+ /**
+ * Creates a function that either curries or invokes `func` with optional
+ * `this` binding and partially applied arguments.
+ *
+ * @private
+ * @param {Function|string} func The function or method name to wrap.
+ * @param {number} bitmask The bitmask flags.
+ * 1 - `_.bind`
+ * 2 - `_.bindKey`
+ * 4 - `_.curry` or `_.curryRight` of a bound function
+ * 8 - `_.curry`
+ * 16 - `_.curryRight`
+ * 32 - `_.partial`
+ * 64 - `_.partialRight`
+ * 128 - `_.rearg`
+ * 256 - `_.ary`
+ * 512 - `_.flip`
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {Array} [partials] The arguments to be partially applied.
+ * @param {Array} [holders] The `partials` placeholder indexes.
+ * @param {Array} [argPos] The argument positions of the new function.
+ * @param {number} [ary] The arity cap of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+ function createWrap(func, bitmask, thisArg, partials, holders, argPos, ary, arity) {
+ var isBindKey = bitmask & WRAP_BIND_KEY_FLAG;
+ if (!isBindKey && typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ var length = partials ? partials.length : 0;
+ if (!length) {
+ bitmask &= ~(WRAP_PARTIAL_FLAG | WRAP_PARTIAL_RIGHT_FLAG);
+ partials = holders = undefined;
+ }
+ ary = ary === undefined ? ary : nativeMax(toInteger(ary), 0);
+ arity = arity === undefined ? arity : toInteger(arity);
+ length -= holders ? holders.length : 0;
+
+ if (bitmask & WRAP_PARTIAL_RIGHT_FLAG) {
+ var partialsRight = partials,
+ holdersRight = holders;
+
+ partials = holders = undefined;
+ }
+ var data = isBindKey ? undefined : getData(func);
+
+ var newData = [
+ func, bitmask, thisArg, partials, holders, partialsRight, holdersRight,
+ argPos, ary, arity
+ ];
+
+ if (data) {
+ mergeData(newData, data);
+ }
+ func = newData[0];
+ bitmask = newData[1];
+ thisArg = newData[2];
+ partials = newData[3];
+ holders = newData[4];
+ arity = newData[9] = newData[9] === undefined
+ ? (isBindKey ? 0 : func.length)
+ : nativeMax(newData[9] - length, 0);
+
+ if (!arity && bitmask & (WRAP_CURRY_FLAG | WRAP_CURRY_RIGHT_FLAG)) {
+ bitmask &= ~(WRAP_CURRY_FLAG | WRAP_CURRY_RIGHT_FLAG);
+ }
+ if (!bitmask || bitmask == WRAP_BIND_FLAG) {
+ var result = createBind(func, bitmask, thisArg);
+ } else if (bitmask == WRAP_CURRY_FLAG || bitmask == WRAP_CURRY_RIGHT_FLAG) {
+ result = createCurry(func, bitmask, arity);
+ } else if ((bitmask == WRAP_PARTIAL_FLAG || bitmask == (WRAP_BIND_FLAG | WRAP_PARTIAL_FLAG)) && !holders.length) {
+ result = createPartial(func, bitmask, thisArg, partials);
+ } else {
+ result = createHybrid.apply(undefined, newData);
+ }
+ var setter = data ? baseSetData : setData;
+ return setWrapToString(setter(result, newData), func, bitmask);
+ }
+
+ /**
+ * Used by `_.defaults` to customize its `_.assignIn` use to assign properties
+ * of source objects to the destination object for all destination properties
+ * that resolve to `undefined`.
+ *
+ * @private
+ * @param {*} objValue The destination value.
+ * @param {*} srcValue The source value.
+ * @param {string} key The key of the property to assign.
+ * @param {Object} object The parent object of `objValue`.
+ * @returns {*} Returns the value to assign.
+ */
+ function customDefaultsAssignIn(objValue, srcValue, key, object) {
+ if (objValue === undefined ||
+ (eq(objValue, objectProto[key]) && !hasOwnProperty.call(object, key))) {
+ return srcValue;
+ }
+ return objValue;
+ }
+
+ /**
+ * Used by `_.defaultsDeep` to customize its `_.merge` use to merge source
+ * objects into destination objects that are passed thru.
+ *
+ * @private
+ * @param {*} objValue The destination value.
+ * @param {*} srcValue The source value.
+ * @param {string} key The key of the property to merge.
+ * @param {Object} object The parent object of `objValue`.
+ * @param {Object} source The parent object of `srcValue`.
+ * @param {Object} [stack] Tracks traversed source values and their merged
+ * counterparts.
+ * @returns {*} Returns the value to assign.
+ */
+ function customDefaultsMerge(objValue, srcValue, key, object, source, stack) {
+ if (isObject(objValue) && isObject(srcValue)) {
+ // Recursively merge objects and arrays (susceptible to call stack limits).
+ stack.set(srcValue, objValue);
+ baseMerge(objValue, srcValue, undefined, customDefaultsMerge, stack);
+ stack['delete'](srcValue);
+ }
+ return objValue;
+ }
+
+ /**
+ * Used by `_.omit` to customize its `_.cloneDeep` use to only clone plain
+ * objects.
+ *
+ * @private
+ * @param {*} value The value to inspect.
+ * @param {string} key The key of the property to inspect.
+ * @returns {*} Returns the uncloned value or `undefined` to defer cloning to `_.cloneDeep`.
+ */
+ function customOmitClone(value) {
+ return isPlainObject(value) ? undefined : value;
+ }
+
+ /**
+ * A specialized version of `baseIsEqualDeep` for arrays with support for
+ * partial deep comparisons.
+ *
+ * @private
+ * @param {Array} array The array to compare.
+ * @param {Array} other The other array to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} stack Tracks traversed `array` and `other` objects.
+ * @returns {boolean} Returns `true` if the arrays are equivalent, else `false`.
+ */
+ function equalArrays(array, other, bitmask, customizer, equalFunc, stack) {
+ var isPartial = bitmask & COMPARE_PARTIAL_FLAG,
+ arrLength = array.length,
+ othLength = other.length;
+
+ if (arrLength != othLength && !(isPartial && othLength > arrLength)) {
+ return false;
+ }
+ // Check that cyclic values are equal.
+ var arrStacked = stack.get(array);
+ var othStacked = stack.get(other);
+ if (arrStacked && othStacked) {
+ return arrStacked == other && othStacked == array;
+ }
+ var index = -1,
+ result = true,
+ seen = (bitmask & COMPARE_UNORDERED_FLAG) ? new SetCache : undefined;
+
+ stack.set(array, other);
+ stack.set(other, array);
+
+ // Ignore non-index properties.
+ while (++index < arrLength) {
+ var arrValue = array[index],
+ othValue = other[index];
+
+ if (customizer) {
+ var compared = isPartial
+ ? customizer(othValue, arrValue, index, other, array, stack)
+ : customizer(arrValue, othValue, index, array, other, stack);
+ }
+ if (compared !== undefined) {
+ if (compared) {
+ continue;
+ }
+ result = false;
+ break;
+ }
+ // Recursively compare arrays (susceptible to call stack limits).
+ if (seen) {
+ if (!arraySome(other, function(othValue, othIndex) {
+ if (!cacheHas(seen, othIndex) &&
+ (arrValue === othValue || equalFunc(arrValue, othValue, bitmask, customizer, stack))) {
+ return seen.push(othIndex);
+ }
+ })) {
+ result = false;
+ break;
+ }
+ } else if (!(
+ arrValue === othValue ||
+ equalFunc(arrValue, othValue, bitmask, customizer, stack)
+ )) {
+ result = false;
+ break;
+ }
+ }
+ stack['delete'](array);
+ stack['delete'](other);
+ return result;
+ }
+
+ /**
+ * A specialized version of `baseIsEqualDeep` for comparing objects of
+ * the same `toStringTag`.
+ *
+ * **Note:** This function only supports comparing values with tags of
+ * `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {string} tag The `toStringTag` of the objects to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} stack Tracks traversed `object` and `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+ function equalByTag(object, other, tag, bitmask, customizer, equalFunc, stack) {
+ switch (tag) {
+ case dataViewTag:
+ if ((object.byteLength != other.byteLength) ||
+ (object.byteOffset != other.byteOffset)) {
+ return false;
+ }
+ object = object.buffer;
+ other = other.buffer;
+
+ case arrayBufferTag:
+ if ((object.byteLength != other.byteLength) ||
+ !equalFunc(new Uint8Array(object), new Uint8Array(other))) {
+ return false;
+ }
+ return true;
+
+ case boolTag:
+ case dateTag:
+ case numberTag:
+ // Coerce booleans to `1` or `0` and dates to milliseconds.
+ // Invalid dates are coerced to `NaN`.
+ return eq(+object, +other);
+
+ case errorTag:
+ return object.name == other.name && object.message == other.message;
+
+ case regexpTag:
+ case stringTag:
+ // Coerce regexes to strings and treat strings, primitives and objects,
+ // as equal. See http://www.ecma-international.org/ecma-262/7.0/#sec-regexp.prototype.tostring
+ // for more details.
+ return object == (other + '');
+
+ case mapTag:
+ var convert = mapToArray;
+
+ case setTag:
+ var isPartial = bitmask & COMPARE_PARTIAL_FLAG;
+ convert || (convert = setToArray);
+
+ if (object.size != other.size && !isPartial) {
+ return false;
+ }
+ // Assume cyclic values are equal.
+ var stacked = stack.get(object);
+ if (stacked) {
+ return stacked == other;
+ }
+ bitmask |= COMPARE_UNORDERED_FLAG;
+
+ // Recursively compare objects (susceptible to call stack limits).
+ stack.set(object, other);
+ var result = equalArrays(convert(object), convert(other), bitmask, customizer, equalFunc, stack);
+ stack['delete'](object);
+ return result;
+
+ case symbolTag:
+ if (symbolValueOf) {
+ return symbolValueOf.call(object) == symbolValueOf.call(other);
+ }
+ }
+ return false;
+ }
+
+ /**
+ * A specialized version of `baseIsEqualDeep` for objects with support for
+ * partial deep comparisons.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
+ * @param {Function} customizer The function to customize comparisons.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Object} stack Tracks traversed `object` and `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+ function equalObjects(object, other, bitmask, customizer, equalFunc, stack) {
+ var isPartial = bitmask & COMPARE_PARTIAL_FLAG,
+ objProps = getAllKeys(object),
+ objLength = objProps.length,
+ othProps = getAllKeys(other),
+ othLength = othProps.length;
+
+ if (objLength != othLength && !isPartial) {
+ return false;
+ }
+ var index = objLength;
+ while (index--) {
+ var key = objProps[index];
+ if (!(isPartial ? key in other : hasOwnProperty.call(other, key))) {
+ return false;
+ }
+ }
+ // Check that cyclic values are equal.
+ var objStacked = stack.get(object);
+ var othStacked = stack.get(other);
+ if (objStacked && othStacked) {
+ return objStacked == other && othStacked == object;
+ }
+ var result = true;
+ stack.set(object, other);
+ stack.set(other, object);
+
+ var skipCtor = isPartial;
+ while (++index < objLength) {
+ key = objProps[index];
+ var objValue = object[key],
+ othValue = other[key];
+
+ if (customizer) {
+ var compared = isPartial
+ ? customizer(othValue, objValue, key, other, object, stack)
+ : customizer(objValue, othValue, key, object, other, stack);
+ }
+ // Recursively compare objects (susceptible to call stack limits).
+ if (!(compared === undefined
+ ? (objValue === othValue || equalFunc(objValue, othValue, bitmask, customizer, stack))
+ : compared
+ )) {
+ result = false;
+ break;
+ }
+ skipCtor || (skipCtor = key == 'constructor');
+ }
+ if (result && !skipCtor) {
+ var objCtor = object.constructor,
+ othCtor = other.constructor;
+
+ // Non `Object` object instances with different constructors are not equal.
+ if (objCtor != othCtor &&
+ ('constructor' in object && 'constructor' in other) &&
+ !(typeof objCtor == 'function' && objCtor instanceof objCtor &&
+ typeof othCtor == 'function' && othCtor instanceof othCtor)) {
+ result = false;
+ }
+ }
+ stack['delete'](object);
+ stack['delete'](other);
+ return result;
+ }
+
+ /**
+ * A specialized version of `baseRest` which flattens the rest array.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @returns {Function} Returns the new function.
+ */
+ function flatRest(func) {
+ return setToString(overRest(func, undefined, flatten), func + '');
+ }
+
+ /**
+ * Creates an array of own enumerable property names and symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names and symbols.
+ */
+ function getAllKeys(object) {
+ return baseGetAllKeys(object, keys, getSymbols);
+ }
+
+ /**
+ * Creates an array of own and inherited enumerable property names and
+ * symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names and symbols.
+ */
+ function getAllKeysIn(object) {
+ return baseGetAllKeys(object, keysIn, getSymbolsIn);
+ }
+
+ /**
+ * Gets metadata for `func`.
+ *
+ * @private
+ * @param {Function} func The function to query.
+ * @returns {*} Returns the metadata for `func`.
+ */
+ var getData = !metaMap ? noop : function(func) {
+ return metaMap.get(func);
+ };
+
+ /**
+ * Gets the name of `func`.
+ *
+ * @private
+ * @param {Function} func The function to query.
+ * @returns {string} Returns the function name.
+ */
+ function getFuncName(func) {
+ var result = (func.name + ''),
+ array = realNames[result],
+ length = hasOwnProperty.call(realNames, result) ? array.length : 0;
+
+ while (length--) {
+ var data = array[length],
+ otherFunc = data.func;
+ if (otherFunc == null || otherFunc == func) {
+ return data.name;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Gets the argument placeholder value for `func`.
+ *
+ * @private
+ * @param {Function} func The function to inspect.
+ * @returns {*} Returns the placeholder value.
+ */
+ function getHolder(func) {
+ var object = hasOwnProperty.call(lodash, 'placeholder') ? lodash : func;
+ return object.placeholder;
+ }
+
+ /**
+ * Gets the appropriate "iteratee" function. If `_.iteratee` is customized,
+ * this function returns the custom method, otherwise it returns `baseIteratee`.
+ * If arguments are provided, the chosen function is invoked with them and
+ * its result is returned.
+ *
+ * @private
+ * @param {*} [value] The value to convert to an iteratee.
+ * @param {number} [arity] The arity of the created iteratee.
+ * @returns {Function} Returns the chosen function or its result.
+ */
+ function getIteratee() {
+ var result = lodash.iteratee || iteratee;
+ result = result === iteratee ? baseIteratee : result;
+ return arguments.length ? result(arguments[0], arguments[1]) : result;
+ }
+
+ /**
+ * Gets the data for `map`.
+ *
+ * @private
+ * @param {Object} map The map to query.
+ * @param {string} key The reference key.
+ * @returns {*} Returns the map data.
+ */
+ function getMapData(map, key) {
+ var data = map.__data__;
+ return isKeyable(key)
+ ? data[typeof key == 'string' ? 'string' : 'hash']
+ : data.map;
+ }
+
+ /**
+ * Gets the property names, values, and compare flags of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the match data of `object`.
+ */
+ function getMatchData(object) {
+ var result = keys(object),
+ length = result.length;
+
+ while (length--) {
+ var key = result[length],
+ value = object[key];
+
+ result[length] = [key, value, isStrictComparable(value)];
+ }
+ return result;
+ }
+
+ /**
+ * Gets the native function at `key` of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the method to get.
+ * @returns {*} Returns the function if it's native, else `undefined`.
+ */
+ function getNative(object, key) {
+ var value = getValue(object, key);
+ return baseIsNative(value) ? value : undefined;
+ }
+
+ /**
+ * A specialized version of `baseGetTag` which ignores `Symbol.toStringTag` values.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the raw `toStringTag`.
+ */
+ function getRawTag(value) {
+ var isOwn = hasOwnProperty.call(value, symToStringTag),
+ tag = value[symToStringTag];
+
+ try {
+ value[symToStringTag] = undefined;
+ var unmasked = true;
+ } catch (e) {}
+
+ var result = nativeObjectToString.call(value);
+ if (unmasked) {
+ if (isOwn) {
+ value[symToStringTag] = tag;
+ } else {
+ delete value[symToStringTag];
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Creates an array of the own enumerable symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of symbols.
+ */
+ var getSymbols = !nativeGetSymbols ? stubArray : function(object) {
+ if (object == null) {
+ return [];
+ }
+ object = Object(object);
+ return arrayFilter(nativeGetSymbols(object), function(symbol) {
+ return propertyIsEnumerable.call(object, symbol);
+ });
+ };
+
+ /**
+ * Creates an array of the own and inherited enumerable symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of symbols.
+ */
+ var getSymbolsIn = !nativeGetSymbols ? stubArray : function(object) {
+ var result = [];
+ while (object) {
+ arrayPush(result, getSymbols(object));
+ object = getPrototype(object);
+ }
+ return result;
+ };
+
+ /**
+ * Gets the `toStringTag` of `value`.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the `toStringTag`.
+ */
+ var getTag = baseGetTag;
+
+ // Fallback for data views, maps, sets, and weak maps in IE 11 and promises in Node.js < 6.
+ if ((DataView && getTag(new DataView(new ArrayBuffer(1))) != dataViewTag) ||
+ (Map && getTag(new Map) != mapTag) ||
+ (Promise && getTag(Promise.resolve()) != promiseTag) ||
+ (Set && getTag(new Set) != setTag) ||
+ (WeakMap && getTag(new WeakMap) != weakMapTag)) {
+ getTag = function(value) {
+ var result = baseGetTag(value),
+ Ctor = result == objectTag ? value.constructor : undefined,
+ ctorString = Ctor ? toSource(Ctor) : '';
+
+ if (ctorString) {
+ switch (ctorString) {
+ case dataViewCtorString: return dataViewTag;
+ case mapCtorString: return mapTag;
+ case promiseCtorString: return promiseTag;
+ case setCtorString: return setTag;
+ case weakMapCtorString: return weakMapTag;
+ }
+ }
+ return result;
+ };
+ }
+
+ /**
+ * Gets the view, applying any `transforms` to the `start` and `end` positions.
+ *
+ * @private
+ * @param {number} start The start of the view.
+ * @param {number} end The end of the view.
+ * @param {Array} transforms The transformations to apply to the view.
+ * @returns {Object} Returns an object containing the `start` and `end`
+ * positions of the view.
+ */
+ function getView(start, end, transforms) {
+ var index = -1,
+ length = transforms.length;
+
+ while (++index < length) {
+ var data = transforms[index],
+ size = data.size;
+
+ switch (data.type) {
+ case 'drop': start += size; break;
+ case 'dropRight': end -= size; break;
+ case 'take': end = nativeMin(end, start + size); break;
+ case 'takeRight': start = nativeMax(start, end - size); break;
+ }
+ }
+ return { 'start': start, 'end': end };
+ }
+
+ /**
+ * Extracts wrapper details from the `source` body comment.
+ *
+ * @private
+ * @param {string} source The source to inspect.
+ * @returns {Array} Returns the wrapper details.
+ */
+ function getWrapDetails(source) {
+ var match = source.match(reWrapDetails);
+ return match ? match[1].split(reSplitDetails) : [];
+ }
+
+ /**
+ * Checks if `path` exists on `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path to check.
+ * @param {Function} hasFunc The function to check properties.
+ * @returns {boolean} Returns `true` if `path` exists, else `false`.
+ */
+ function hasPath(object, path, hasFunc) {
+ path = castPath(path, object);
+
+ var index = -1,
+ length = path.length,
+ result = false;
+
+ while (++index < length) {
+ var key = toKey(path[index]);
+ if (!(result = object != null && hasFunc(object, key))) {
+ break;
+ }
+ object = object[key];
+ }
+ if (result || ++index != length) {
+ return result;
+ }
+ length = object == null ? 0 : object.length;
+ return !!length && isLength(length) && isIndex(key, length) &&
+ (isArray(object) || isArguments(object));
+ }
+
+ /**
+ * Initializes an array clone.
+ *
+ * @private
+ * @param {Array} array The array to clone.
+ * @returns {Array} Returns the initialized clone.
+ */
+ function initCloneArray(array) {
+ var length = array.length,
+ result = new array.constructor(length);
+
+ // Add properties assigned by `RegExp#exec`.
+ if (length && typeof array[0] == 'string' && hasOwnProperty.call(array, 'index')) {
+ result.index = array.index;
+ result.input = array.input;
+ }
+ return result;
+ }
+
+ /**
+ * Initializes an object clone.
+ *
+ * @private
+ * @param {Object} object The object to clone.
+ * @returns {Object} Returns the initialized clone.
+ */
+ function initCloneObject(object) {
+ return (typeof object.constructor == 'function' && !isPrototype(object))
+ ? baseCreate(getPrototype(object))
+ : {};
+ }
+
+ /**
+ * Initializes an object clone based on its `toStringTag`.
+ *
+ * **Note:** This function only supports cloning values with tags of
+ * `Boolean`, `Date`, `Error`, `Map`, `Number`, `RegExp`, `Set`, or `String`.
+ *
+ * @private
+ * @param {Object} object The object to clone.
+ * @param {string} tag The `toStringTag` of the object to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the initialized clone.
+ */
+ function initCloneByTag(object, tag, isDeep) {
+ var Ctor = object.constructor;
+ switch (tag) {
+ case arrayBufferTag:
+ return cloneArrayBuffer(object);
+
+ case boolTag:
+ case dateTag:
+ return new Ctor(+object);
+
+ case dataViewTag:
+ return cloneDataView(object, isDeep);
+
+ case float32Tag: case float64Tag:
+ case int8Tag: case int16Tag: case int32Tag:
+ case uint8Tag: case uint8ClampedTag: case uint16Tag: case uint32Tag:
+ return cloneTypedArray(object, isDeep);
+
+ case mapTag:
+ return new Ctor;
+
+ case numberTag:
+ case stringTag:
+ return new Ctor(object);
+
+ case regexpTag:
+ return cloneRegExp(object);
+
+ case setTag:
+ return new Ctor;
+
+ case symbolTag:
+ return cloneSymbol(object);
+ }
+ }
+
+ /**
+ * Inserts wrapper `details` in a comment at the top of the `source` body.
+ *
+ * @private
+ * @param {string} source The source to modify.
+ * @returns {Array} details The details to insert.
+ * @returns {string} Returns the modified source.
+ */
+ function insertWrapDetails(source, details) {
+ var length = details.length;
+ if (!length) {
+ return source;
+ }
+ var lastIndex = length - 1;
+ details[lastIndex] = (length > 1 ? '& ' : '') + details[lastIndex];
+ details = details.join(length > 2 ? ', ' : ' ');
+ return source.replace(reWrapComment, '{\n/* [wrapped with ' + details + '] */\n');
+ }
+
+ /**
+ * Checks if `value` is a flattenable `arguments` object or array.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is flattenable, else `false`.
+ */
+ function isFlattenable(value) {
+ return isArray(value) || isArguments(value) ||
+ !!(spreadableSymbol && value && value[spreadableSymbol]);
+ }
+
+ /**
+ * Checks if `value` is a valid array-like index.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
+ * @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
+ */
+ function isIndex(value, length) {
+ var type = typeof value;
+ length = length == null ? MAX_SAFE_INTEGER : length;
+
+ return !!length &&
+ (type == 'number' ||
+ (type != 'symbol' && reIsUint.test(value))) &&
+ (value > -1 && value % 1 == 0 && value < length);
+ }
+
+ /**
+ * Checks if the given arguments are from an iteratee call.
+ *
+ * @private
+ * @param {*} value The potential iteratee value argument.
+ * @param {*} index The potential iteratee index or key argument.
+ * @param {*} object The potential iteratee object argument.
+ * @returns {boolean} Returns `true` if the arguments are from an iteratee call,
+ * else `false`.
+ */
+ function isIterateeCall(value, index, object) {
+ if (!isObject(object)) {
+ return false;
+ }
+ var type = typeof index;
+ if (type == 'number'
+ ? (isArrayLike(object) && isIndex(index, object.length))
+ : (type == 'string' && index in object)
+ ) {
+ return eq(object[index], value);
+ }
+ return false;
+ }
+
+ /**
+ * Checks if `value` is a property name and not a property path.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {Object} [object] The object to query keys on.
+ * @returns {boolean} Returns `true` if `value` is a property name, else `false`.
+ */
+ function isKey(value, object) {
+ if (isArray(value)) {
+ return false;
+ }
+ var type = typeof value;
+ if (type == 'number' || type == 'symbol' || type == 'boolean' ||
+ value == null || isSymbol(value)) {
+ return true;
+ }
+ return reIsPlainProp.test(value) || !reIsDeepProp.test(value) ||
+ (object != null && value in Object(object));
+ }
+
+ /**
+ * Checks if `value` is suitable for use as unique object key.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is suitable, else `false`.
+ */
+ function isKeyable(value) {
+ var type = typeof value;
+ return (type == 'string' || type == 'number' || type == 'symbol' || type == 'boolean')
+ ? (value !== '__proto__')
+ : (value === null);
+ }
+
+ /**
+ * Checks if `func` has a lazy counterpart.
+ *
+ * @private
+ * @param {Function} func The function to check.
+ * @returns {boolean} Returns `true` if `func` has a lazy counterpart,
+ * else `false`.
+ */
+ function isLaziable(func) {
+ var funcName = getFuncName(func),
+ other = lodash[funcName];
+
+ if (typeof other != 'function' || !(funcName in LazyWrapper.prototype)) {
+ return false;
+ }
+ if (func === other) {
+ return true;
+ }
+ var data = getData(other);
+ return !!data && func === data[0];
+ }
+
+ /**
+ * Checks if `func` has its source masked.
+ *
+ * @private
+ * @param {Function} func The function to check.
+ * @returns {boolean} Returns `true` if `func` is masked, else `false`.
+ */
+ function isMasked(func) {
+ return !!maskSrcKey && (maskSrcKey in func);
+ }
+
+ /**
+ * Checks if `func` is capable of being masked.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `func` is maskable, else `false`.
+ */
+ var isMaskable = coreJsData ? isFunction : stubFalse;
+
+ /**
+ * Checks if `value` is likely a prototype object.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a prototype, else `false`.
+ */
+ function isPrototype(value) {
+ var Ctor = value && value.constructor,
+ proto = (typeof Ctor == 'function' && Ctor.prototype) || objectProto;
+
+ return value === proto;
+ }
+
+ /**
+ * Checks if `value` is suitable for strict equality comparisons, i.e. `===`.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` if suitable for strict
+ * equality comparisons, else `false`.
+ */
+ function isStrictComparable(value) {
+ return value === value && !isObject(value);
+ }
+
+ /**
+ * A specialized version of `matchesProperty` for source values suitable
+ * for strict equality comparisons, i.e. `===`.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @param {*} srcValue The value to match.
+ * @returns {Function} Returns the new spec function.
+ */
+ function matchesStrictComparable(key, srcValue) {
+ return function(object) {
+ if (object == null) {
+ return false;
+ }
+ return object[key] === srcValue &&
+ (srcValue !== undefined || (key in Object(object)));
+ };
+ }
+
+ /**
+ * A specialized version of `_.memoize` which clears the memoized function's
+ * cache when it exceeds `MAX_MEMOIZE_SIZE`.
+ *
+ * @private
+ * @param {Function} func The function to have its output memoized.
+ * @returns {Function} Returns the new memoized function.
+ */
+ function memoizeCapped(func) {
+ var result = memoize(func, function(key) {
+ if (cache.size === MAX_MEMOIZE_SIZE) {
+ cache.clear();
+ }
+ return key;
+ });
+
+ var cache = result.cache;
+ return result;
+ }
+
+ /**
+ * Merges the function metadata of `source` into `data`.
+ *
+ * Merging metadata reduces the number of wrappers used to invoke a function.
+ * This is possible because methods like `_.bind`, `_.curry`, and `_.partial`
+ * may be applied regardless of execution order. Methods like `_.ary` and
+ * `_.rearg` modify function arguments, making the order in which they are
+ * executed important, preventing the merging of metadata. However, we make
+ * an exception for a safe combined case where curried functions have `_.ary`
+ * and or `_.rearg` applied.
+ *
+ * @private
+ * @param {Array} data The destination metadata.
+ * @param {Array} source The source metadata.
+ * @returns {Array} Returns `data`.
+ */
+ function mergeData(data, source) {
+ var bitmask = data[1],
+ srcBitmask = source[1],
+ newBitmask = bitmask | srcBitmask,
+ isCommon = newBitmask < (WRAP_BIND_FLAG | WRAP_BIND_KEY_FLAG | WRAP_ARY_FLAG);
+
+ var isCombo =
+ ((srcBitmask == WRAP_ARY_FLAG) && (bitmask == WRAP_CURRY_FLAG)) ||
+ ((srcBitmask == WRAP_ARY_FLAG) && (bitmask == WRAP_REARG_FLAG) && (data[7].length <= source[8])) ||
+ ((srcBitmask == (WRAP_ARY_FLAG | WRAP_REARG_FLAG)) && (source[7].length <= source[8]) && (bitmask == WRAP_CURRY_FLAG));
+
+ // Exit early if metadata can't be merged.
+ if (!(isCommon || isCombo)) {
+ return data;
+ }
+ // Use source `thisArg` if available.
+ if (srcBitmask & WRAP_BIND_FLAG) {
+ data[2] = source[2];
+ // Set when currying a bound function.
+ newBitmask |= bitmask & WRAP_BIND_FLAG ? 0 : WRAP_CURRY_BOUND_FLAG;
+ }
+ // Compose partial arguments.
+ var value = source[3];
+ if (value) {
+ var partials = data[3];
+ data[3] = partials ? composeArgs(partials, value, source[4]) : value;
+ data[4] = partials ? replaceHolders(data[3], PLACEHOLDER) : source[4];
+ }
+ // Compose partial right arguments.
+ value = source[5];
+ if (value) {
+ partials = data[5];
+ data[5] = partials ? composeArgsRight(partials, value, source[6]) : value;
+ data[6] = partials ? replaceHolders(data[5], PLACEHOLDER) : source[6];
+ }
+ // Use source `argPos` if available.
+ value = source[7];
+ if (value) {
+ data[7] = value;
+ }
+ // Use source `ary` if it's smaller.
+ if (srcBitmask & WRAP_ARY_FLAG) {
+ data[8] = data[8] == null ? source[8] : nativeMin(data[8], source[8]);
+ }
+ // Use source `arity` if one is not provided.
+ if (data[9] == null) {
+ data[9] = source[9];
+ }
+ // Use source `func` and merge bitmasks.
+ data[0] = source[0];
+ data[1] = newBitmask;
+
+ return data;
+ }
+
+ /**
+ * This function is like
+ * [`Object.keys`](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
+ * except that it includes inherited enumerable properties.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+ function nativeKeysIn(object) {
+ var result = [];
+ if (object != null) {
+ for (var key in Object(object)) {
+ result.push(key);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Converts `value` to a string using `Object.prototype.toString`.
+ *
+ * @private
+ * @param {*} value The value to convert.
+ * @returns {string} Returns the converted string.
+ */
+ function objectToString(value) {
+ return nativeObjectToString.call(value);
+ }
+
+ /**
+ * A specialized version of `baseRest` which transforms the rest array.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @param {Function} transform The rest array transform.
+ * @returns {Function} Returns the new function.
+ */
+ function overRest(func, start, transform) {
+ start = nativeMax(start === undefined ? (func.length - 1) : start, 0);
+ return function() {
+ var args = arguments,
+ index = -1,
+ length = nativeMax(args.length - start, 0),
+ array = Array(length);
+
+ while (++index < length) {
+ array[index] = args[start + index];
+ }
+ index = -1;
+ var otherArgs = Array(start + 1);
+ while (++index < start) {
+ otherArgs[index] = args[index];
+ }
+ otherArgs[start] = transform(array);
+ return apply(func, this, otherArgs);
+ };
+ }
+
+ /**
+ * Gets the parent value at `path` of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} path The path to get the parent value of.
+ * @returns {*} Returns the parent value.
+ */
+ function parent(object, path) {
+ return path.length < 2 ? object : baseGet(object, baseSlice(path, 0, -1));
+ }
+
+ /**
+ * Reorder `array` according to the specified indexes where the element at
+ * the first index is assigned as the first element, the element at
+ * the second index is assigned as the second element, and so on.
+ *
+ * @private
+ * @param {Array} array The array to reorder.
+ * @param {Array} indexes The arranged array indexes.
+ * @returns {Array} Returns `array`.
+ */
+ function reorder(array, indexes) {
+ var arrLength = array.length,
+ length = nativeMin(indexes.length, arrLength),
+ oldArray = copyArray(array);
+
+ while (length--) {
+ var index = indexes[length];
+ array[length] = isIndex(index, arrLength) ? oldArray[index] : undefined;
+ }
+ return array;
+ }
+
+ /**
+ * Gets the value at `key`, unless `key` is "__proto__" or "constructor".
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the property to get.
+ * @returns {*} Returns the property value.
+ */
+ function safeGet(object, key) {
+ if (key === 'constructor' && typeof object[key] === 'function') {
+ return;
+ }
+
+ if (key == '__proto__') {
+ return;
+ }
+
+ return object[key];
+ }
+
+ /**
+ * Sets metadata for `func`.
+ *
+ * **Note:** If this function becomes hot, i.e. is invoked a lot in a short
+ * period of time, it will trip its breaker and transition to an identity
+ * function to avoid garbage collection pauses in V8. See
+ * [V8 issue 2070](https://bugs.chromium.org/p/v8/issues/detail?id=2070)
+ * for more details.
+ *
+ * @private
+ * @param {Function} func The function to associate metadata with.
+ * @param {*} data The metadata.
+ * @returns {Function} Returns `func`.
+ */
+ var setData = shortOut(baseSetData);
+
+ /**
+ * A simple wrapper around the global [`setTimeout`](https://mdn.io/setTimeout).
+ *
+ * @private
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay invocation.
+ * @returns {number|Object} Returns the timer id or timeout object.
+ */
+ var setTimeout = ctxSetTimeout || function(func, wait) {
+ return root.setTimeout(func, wait);
+ };
+
+ /**
+ * Sets the `toString` method of `func` to return `string`.
+ *
+ * @private
+ * @param {Function} func The function to modify.
+ * @param {Function} string The `toString` result.
+ * @returns {Function} Returns `func`.
+ */
+ var setToString = shortOut(baseSetToString);
+
+ /**
+ * Sets the `toString` method of `wrapper` to mimic the source of `reference`
+ * with wrapper details in a comment at the top of the source body.
+ *
+ * @private
+ * @param {Function} wrapper The function to modify.
+ * @param {Function} reference The reference function.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @returns {Function} Returns `wrapper`.
+ */
+ function setWrapToString(wrapper, reference, bitmask) {
+ var source = (reference + '');
+ return setToString(wrapper, insertWrapDetails(source, updateWrapDetails(getWrapDetails(source), bitmask)));
+ }
+
+ /**
+ * Creates a function that'll short out and invoke `identity` instead
+ * of `func` when it's called `HOT_COUNT` or more times in `HOT_SPAN`
+ * milliseconds.
+ *
+ * @private
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new shortable function.
+ */
+ function shortOut(func) {
+ var count = 0,
+ lastCalled = 0;
+
+ return function() {
+ var stamp = nativeNow(),
+ remaining = HOT_SPAN - (stamp - lastCalled);
+
+ lastCalled = stamp;
+ if (remaining > 0) {
+ if (++count >= HOT_COUNT) {
+ return arguments[0];
+ }
+ } else {
+ count = 0;
+ }
+ return func.apply(undefined, arguments);
+ };
+ }
+
+ /**
+ * A specialized version of `_.shuffle` which mutates and sets the size of `array`.
+ *
+ * @private
+ * @param {Array} array The array to shuffle.
+ * @param {number} [size=array.length] The size of `array`.
+ * @returns {Array} Returns `array`.
+ */
+ function shuffleSelf(array, size) {
+ var index = -1,
+ length = array.length,
+ lastIndex = length - 1;
+
+ size = size === undefined ? length : size;
+ while (++index < size) {
+ var rand = baseRandom(index, lastIndex),
+ value = array[rand];
+
+ array[rand] = array[index];
+ array[index] = value;
+ }
+ array.length = size;
+ return array;
+ }
+
+ /**
+ * Converts `string` to a property path array.
+ *
+ * @private
+ * @param {string} string The string to convert.
+ * @returns {Array} Returns the property path array.
+ */
+ var stringToPath = memoizeCapped(function(string) {
+ var result = [];
+ if (string.charCodeAt(0) === 46 /* . */) {
+ result.push('');
+ }
+ string.replace(rePropName, function(match, number, quote, subString) {
+ result.push(quote ? subString.replace(reEscapeChar, '$1') : (number || match));
+ });
+ return result;
+ });
+
+ /**
+ * Converts `value` to a string key if it's not a string or symbol.
+ *
+ * @private
+ * @param {*} value The value to inspect.
+ * @returns {string|symbol} Returns the key.
+ */
+ function toKey(value) {
+ if (typeof value == 'string' || isSymbol(value)) {
+ return value;
+ }
+ var result = (value + '');
+ return (result == '0' && (1 / value) == -INFINITY) ? '-0' : result;
+ }
+
+ /**
+ * Converts `func` to its source code.
+ *
+ * @private
+ * @param {Function} func The function to convert.
+ * @returns {string} Returns the source code.
+ */
+ function toSource(func) {
+ if (func != null) {
+ try {
+ return funcToString.call(func);
+ } catch (e) {}
+ try {
+ return (func + '');
+ } catch (e) {}
+ }
+ return '';
+ }
+
+ /**
+ * Updates wrapper `details` based on `bitmask` flags.
+ *
+ * @private
+ * @returns {Array} details The details to modify.
+ * @param {number} bitmask The bitmask flags. See `createWrap` for more details.
+ * @returns {Array} Returns `details`.
+ */
+ function updateWrapDetails(details, bitmask) {
+ arrayEach(wrapFlags, function(pair) {
+ var value = '_.' + pair[0];
+ if ((bitmask & pair[1]) && !arrayIncludes(details, value)) {
+ details.push(value);
+ }
+ });
+ return details.sort();
+ }
+
+ /**
+ * Creates a clone of `wrapper`.
+ *
+ * @private
+ * @param {Object} wrapper The wrapper to clone.
+ * @returns {Object} Returns the cloned wrapper.
+ */
+ function wrapperClone(wrapper) {
+ if (wrapper instanceof LazyWrapper) {
+ return wrapper.clone();
+ }
+ var result = new LodashWrapper(wrapper.__wrapped__, wrapper.__chain__);
+ result.__actions__ = copyArray(wrapper.__actions__);
+ result.__index__ = wrapper.__index__;
+ result.__values__ = wrapper.__values__;
+ return result;
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates an array of elements split into groups the length of `size`.
+ * If `array` can't be split evenly, the final chunk will be the remaining
+ * elements.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to process.
+ * @param {number} [size=1] The length of each chunk
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Array} Returns the new array of chunks.
+ * @example
+ *
+ * _.chunk(['a', 'b', 'c', 'd'], 2);
+ * // => [['a', 'b'], ['c', 'd']]
+ *
+ * _.chunk(['a', 'b', 'c', 'd'], 3);
+ * // => [['a', 'b', 'c'], ['d']]
+ */
+ function chunk(array, size, guard) {
+ if ((guard ? isIterateeCall(array, size, guard) : size === undefined)) {
+ size = 1;
+ } else {
+ size = nativeMax(toInteger(size), 0);
+ }
+ var length = array == null ? 0 : array.length;
+ if (!length || size < 1) {
+ return [];
+ }
+ var index = 0,
+ resIndex = 0,
+ result = Array(nativeCeil(length / size));
+
+ while (index < length) {
+ result[resIndex++] = baseSlice(array, index, (index += size));
+ }
+ return result;
+ }
+
+ /**
+ * Creates an array with all falsey values removed. The values `false`, `null`,
+ * `0`, `""`, `undefined`, and `NaN` are falsey.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to compact.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * _.compact([0, 1, false, 2, '', 3]);
+ * // => [1, 2, 3]
+ */
+ function compact(array) {
+ var index = -1,
+ length = array == null ? 0 : array.length,
+ resIndex = 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (value) {
+ result[resIndex++] = value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Creates a new array concatenating `array` with any additional arrays
+ * and/or values.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to concatenate.
+ * @param {...*} [values] The values to concatenate.
+ * @returns {Array} Returns the new concatenated array.
+ * @example
+ *
+ * var array = [1];
+ * var other = _.concat(array, 2, [3], [[4]]);
+ *
+ * console.log(other);
+ * // => [1, 2, 3, [4]]
+ *
+ * console.log(array);
+ * // => [1]
+ */
+ function concat() {
+ var length = arguments.length;
+ if (!length) {
+ return [];
+ }
+ var args = Array(length - 1),
+ array = arguments[0],
+ index = length;
+
+ while (index--) {
+ args[index - 1] = arguments[index];
+ }
+ return arrayPush(isArray(array) ? copyArray(array) : [array], baseFlatten(args, 1));
+ }
+
+ /**
+ * Creates an array of `array` values not included in the other given arrays
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons. The order and references of result values are
+ * determined by the first array.
+ *
+ * **Note:** Unlike `_.pullAll`, this method returns a new array.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {...Array} [values] The values to exclude.
+ * @returns {Array} Returns the new array of filtered values.
+ * @see _.without, _.xor
+ * @example
+ *
+ * _.difference([2, 1], [2, 3]);
+ * // => [1]
+ */
+ var difference = baseRest(function(array, values) {
+ return isArrayLikeObject(array)
+ ? baseDifference(array, baseFlatten(values, 1, isArrayLikeObject, true))
+ : [];
+ });
+
+ /**
+ * This method is like `_.difference` except that it accepts `iteratee` which
+ * is invoked for each element of `array` and `values` to generate the criterion
+ * by which they're compared. The order and references of result values are
+ * determined by the first array. The iteratee is invoked with one argument:
+ * (value).
+ *
+ * **Note:** Unlike `_.pullAllBy`, this method returns a new array.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {...Array} [values] The values to exclude.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * _.differenceBy([2.1, 1.2], [2.3, 3.4], Math.floor);
+ * // => [1.2]
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.differenceBy([{ 'x': 2 }, { 'x': 1 }], [{ 'x': 1 }], 'x');
+ * // => [{ 'x': 2 }]
+ */
+ var differenceBy = baseRest(function(array, values) {
+ var iteratee = last(values);
+ if (isArrayLikeObject(iteratee)) {
+ iteratee = undefined;
+ }
+ return isArrayLikeObject(array)
+ ? baseDifference(array, baseFlatten(values, 1, isArrayLikeObject, true), getIteratee(iteratee, 2))
+ : [];
+ });
+
+ /**
+ * This method is like `_.difference` except that it accepts `comparator`
+ * which is invoked to compare elements of `array` to `values`. The order and
+ * references of result values are determined by the first array. The comparator
+ * is invoked with two arguments: (arrVal, othVal).
+ *
+ * **Note:** Unlike `_.pullAllWith`, this method returns a new array.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {...Array} [values] The values to exclude.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * var objects = [{ 'x': 1, 'y': 2 }, { 'x': 2, 'y': 1 }];
+ *
+ * _.differenceWith(objects, [{ 'x': 1, 'y': 2 }], _.isEqual);
+ * // => [{ 'x': 2, 'y': 1 }]
+ */
+ var differenceWith = baseRest(function(array, values) {
+ var comparator = last(values);
+ if (isArrayLikeObject(comparator)) {
+ comparator = undefined;
+ }
+ return isArrayLikeObject(array)
+ ? baseDifference(array, baseFlatten(values, 1, isArrayLikeObject, true), undefined, comparator)
+ : [];
+ });
+
+ /**
+ * Creates a slice of `array` with `n` elements dropped from the beginning.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.5.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=1] The number of elements to drop.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.drop([1, 2, 3]);
+ * // => [2, 3]
+ *
+ * _.drop([1, 2, 3], 2);
+ * // => [3]
+ *
+ * _.drop([1, 2, 3], 5);
+ * // => []
+ *
+ * _.drop([1, 2, 3], 0);
+ * // => [1, 2, 3]
+ */
+ function drop(array, n, guard) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return [];
+ }
+ n = (guard || n === undefined) ? 1 : toInteger(n);
+ return baseSlice(array, n < 0 ? 0 : n, length);
+ }
+
+ /**
+ * Creates a slice of `array` with `n` elements dropped from the end.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=1] The number of elements to drop.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.dropRight([1, 2, 3]);
+ * // => [1, 2]
+ *
+ * _.dropRight([1, 2, 3], 2);
+ * // => [1]
+ *
+ * _.dropRight([1, 2, 3], 5);
+ * // => []
+ *
+ * _.dropRight([1, 2, 3], 0);
+ * // => [1, 2, 3]
+ */
+ function dropRight(array, n, guard) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return [];
+ }
+ n = (guard || n === undefined) ? 1 : toInteger(n);
+ n = length - n;
+ return baseSlice(array, 0, n < 0 ? 0 : n);
+ }
+
+ /**
+ * Creates a slice of `array` excluding elements dropped from the end.
+ * Elements are dropped until `predicate` returns falsey. The predicate is
+ * invoked with three arguments: (value, index, array).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': false }
+ * ];
+ *
+ * _.dropRightWhile(users, function(o) { return !o.active; });
+ * // => objects for ['barney']
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.dropRightWhile(users, { 'user': 'pebbles', 'active': false });
+ * // => objects for ['barney', 'fred']
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.dropRightWhile(users, ['active', false]);
+ * // => objects for ['barney']
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.dropRightWhile(users, 'active');
+ * // => objects for ['barney', 'fred', 'pebbles']
+ */
+ function dropRightWhile(array, predicate) {
+ return (array && array.length)
+ ? baseWhile(array, getIteratee(predicate, 3), true, true)
+ : [];
+ }
+
+ /**
+ * Creates a slice of `array` excluding elements dropped from the beginning.
+ * Elements are dropped until `predicate` returns falsey. The predicate is
+ * invoked with three arguments: (value, index, array).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': true }
+ * ];
+ *
+ * _.dropWhile(users, function(o) { return !o.active; });
+ * // => objects for ['pebbles']
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.dropWhile(users, { 'user': 'barney', 'active': false });
+ * // => objects for ['fred', 'pebbles']
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.dropWhile(users, ['active', false]);
+ * // => objects for ['pebbles']
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.dropWhile(users, 'active');
+ * // => objects for ['barney', 'fred', 'pebbles']
+ */
+ function dropWhile(array, predicate) {
+ return (array && array.length)
+ ? baseWhile(array, getIteratee(predicate, 3), true)
+ : [];
+ }
+
+ /**
+ * Fills elements of `array` with `value` from `start` up to, but not
+ * including, `end`.
+ *
+ * **Note:** This method mutates `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.2.0
+ * @category Array
+ * @param {Array} array The array to fill.
+ * @param {*} value The value to fill `array` with.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = [1, 2, 3];
+ *
+ * _.fill(array, 'a');
+ * console.log(array);
+ * // => ['a', 'a', 'a']
+ *
+ * _.fill(Array(3), 2);
+ * // => [2, 2, 2]
+ *
+ * _.fill([4, 6, 8, 10], '*', 1, 3);
+ * // => [4, '*', '*', 10]
+ */
+ function fill(array, value, start, end) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return [];
+ }
+ if (start && typeof start != 'number' && isIterateeCall(array, value, start)) {
+ start = 0;
+ end = length;
+ }
+ return baseFill(array, value, start, end);
+ }
+
+ /**
+ * This method is like `_.find` except that it returns the index of the first
+ * element `predicate` returns truthy for instead of the element itself.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': true }
+ * ];
+ *
+ * _.findIndex(users, function(o) { return o.user == 'barney'; });
+ * // => 0
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.findIndex(users, { 'user': 'fred', 'active': false });
+ * // => 1
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.findIndex(users, ['active', false]);
+ * // => 0
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.findIndex(users, 'active');
+ * // => 2
+ */
+ function findIndex(array, predicate, fromIndex) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return -1;
+ }
+ var index = fromIndex == null ? 0 : toInteger(fromIndex);
+ if (index < 0) {
+ index = nativeMax(length + index, 0);
+ }
+ return baseFindIndex(array, getIteratee(predicate, 3), index);
+ }
+
+ /**
+ * This method is like `_.findIndex` except that it iterates over elements
+ * of `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param {number} [fromIndex=array.length-1] The index to search from.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': false }
+ * ];
+ *
+ * _.findLastIndex(users, function(o) { return o.user == 'pebbles'; });
+ * // => 2
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.findLastIndex(users, { 'user': 'barney', 'active': true });
+ * // => 0
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.findLastIndex(users, ['active', false]);
+ * // => 2
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.findLastIndex(users, 'active');
+ * // => 0
+ */
+ function findLastIndex(array, predicate, fromIndex) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return -1;
+ }
+ var index = length - 1;
+ if (fromIndex !== undefined) {
+ index = toInteger(fromIndex);
+ index = fromIndex < 0
+ ? nativeMax(length + index, 0)
+ : nativeMin(index, length - 1);
+ }
+ return baseFindIndex(array, getIteratee(predicate, 3), index, true);
+ }
+
+ /**
+ * Flattens `array` a single level deep.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to flatten.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * _.flatten([1, [2, [3, [4]], 5]]);
+ * // => [1, 2, [3, [4]], 5]
+ */
+ function flatten(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? baseFlatten(array, 1) : [];
+ }
+
+ /**
+ * Recursively flattens `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to flatten.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * _.flattenDeep([1, [2, [3, [4]], 5]]);
+ * // => [1, 2, 3, 4, 5]
+ */
+ function flattenDeep(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? baseFlatten(array, INFINITY) : [];
+ }
+
+ /**
+ * Recursively flatten `array` up to `depth` times.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.4.0
+ * @category Array
+ * @param {Array} array The array to flatten.
+ * @param {number} [depth=1] The maximum recursion depth.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * var array = [1, [2, [3, [4]], 5]];
+ *
+ * _.flattenDepth(array, 1);
+ * // => [1, 2, [3, [4]], 5]
+ *
+ * _.flattenDepth(array, 2);
+ * // => [1, 2, 3, [4], 5]
+ */
+ function flattenDepth(array, depth) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return [];
+ }
+ depth = depth === undefined ? 1 : toInteger(depth);
+ return baseFlatten(array, depth);
+ }
+
+ /**
+ * The inverse of `_.toPairs`; this method returns an object composed
+ * from key-value `pairs`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} pairs The key-value pairs.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * _.fromPairs([['a', 1], ['b', 2]]);
+ * // => { 'a': 1, 'b': 2 }
+ */
+ function fromPairs(pairs) {
+ var index = -1,
+ length = pairs == null ? 0 : pairs.length,
+ result = {};
+
+ while (++index < length) {
+ var pair = pairs[index];
+ result[pair[0]] = pair[1];
+ }
+ return result;
+ }
+
+ /**
+ * Gets the first element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @alias first
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {*} Returns the first element of `array`.
+ * @example
+ *
+ * _.head([1, 2, 3]);
+ * // => 1
+ *
+ * _.head([]);
+ * // => undefined
+ */
+ function head(array) {
+ return (array && array.length) ? array[0] : undefined;
+ }
+
+ /**
+ * Gets the index at which the first occurrence of `value` is found in `array`
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons. If `fromIndex` is negative, it's used as the
+ * offset from the end of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ * @example
+ *
+ * _.indexOf([1, 2, 1, 2], 2);
+ * // => 1
+ *
+ * // Search from the `fromIndex`.
+ * _.indexOf([1, 2, 1, 2], 2, 2);
+ * // => 3
+ */
+ function indexOf(array, value, fromIndex) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return -1;
+ }
+ var index = fromIndex == null ? 0 : toInteger(fromIndex);
+ if (index < 0) {
+ index = nativeMax(length + index, 0);
+ }
+ return baseIndexOf(array, value, index);
+ }
+
+ /**
+ * Gets all but the last element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.initial([1, 2, 3]);
+ * // => [1, 2]
+ */
+ function initial(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? baseSlice(array, 0, -1) : [];
+ }
+
+ /**
+ * Creates an array of unique values that are included in all given arrays
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons. The order and references of result values are
+ * determined by the first array.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @returns {Array} Returns the new array of intersecting values.
+ * @example
+ *
+ * _.intersection([2, 1], [2, 3]);
+ * // => [2]
+ */
+ var intersection = baseRest(function(arrays) {
+ var mapped = arrayMap(arrays, castArrayLikeObject);
+ return (mapped.length && mapped[0] === arrays[0])
+ ? baseIntersection(mapped)
+ : [];
+ });
+
+ /**
+ * This method is like `_.intersection` except that it accepts `iteratee`
+ * which is invoked for each element of each `arrays` to generate the criterion
+ * by which they're compared. The order and references of result values are
+ * determined by the first array. The iteratee is invoked with one argument:
+ * (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {Array} Returns the new array of intersecting values.
+ * @example
+ *
+ * _.intersectionBy([2.1, 1.2], [2.3, 3.4], Math.floor);
+ * // => [2.1]
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.intersectionBy([{ 'x': 1 }], [{ 'x': 2 }, { 'x': 1 }], 'x');
+ * // => [{ 'x': 1 }]
+ */
+ var intersectionBy = baseRest(function(arrays) {
+ var iteratee = last(arrays),
+ mapped = arrayMap(arrays, castArrayLikeObject);
+
+ if (iteratee === last(mapped)) {
+ iteratee = undefined;
+ } else {
+ mapped.pop();
+ }
+ return (mapped.length && mapped[0] === arrays[0])
+ ? baseIntersection(mapped, getIteratee(iteratee, 2))
+ : [];
+ });
+
+ /**
+ * This method is like `_.intersection` except that it accepts `comparator`
+ * which is invoked to compare elements of `arrays`. The order and references
+ * of result values are determined by the first array. The comparator is
+ * invoked with two arguments: (arrVal, othVal).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of intersecting values.
+ * @example
+ *
+ * var objects = [{ 'x': 1, 'y': 2 }, { 'x': 2, 'y': 1 }];
+ * var others = [{ 'x': 1, 'y': 1 }, { 'x': 1, 'y': 2 }];
+ *
+ * _.intersectionWith(objects, others, _.isEqual);
+ * // => [{ 'x': 1, 'y': 2 }]
+ */
+ var intersectionWith = baseRest(function(arrays) {
+ var comparator = last(arrays),
+ mapped = arrayMap(arrays, castArrayLikeObject);
+
+ comparator = typeof comparator == 'function' ? comparator : undefined;
+ if (comparator) {
+ mapped.pop();
+ }
+ return (mapped.length && mapped[0] === arrays[0])
+ ? baseIntersection(mapped, undefined, comparator)
+ : [];
+ });
+
+ /**
+ * Converts all elements in `array` into a string separated by `separator`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to convert.
+ * @param {string} [separator=','] The element separator.
+ * @returns {string} Returns the joined string.
+ * @example
+ *
+ * _.join(['a', 'b', 'c'], '~');
+ * // => 'a~b~c'
+ */
+ function join(array, separator) {
+ return array == null ? '' : nativeJoin.call(array, separator);
+ }
+
+ /**
+ * Gets the last element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {*} Returns the last element of `array`.
+ * @example
+ *
+ * _.last([1, 2, 3]);
+ * // => 3
+ */
+ function last(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? array[length - 1] : undefined;
+ }
+
+ /**
+ * This method is like `_.indexOf` except that it iterates over elements of
+ * `array` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=array.length-1] The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ * @example
+ *
+ * _.lastIndexOf([1, 2, 1, 2], 2);
+ * // => 3
+ *
+ * // Search from the `fromIndex`.
+ * _.lastIndexOf([1, 2, 1, 2], 2, 2);
+ * // => 1
+ */
+ function lastIndexOf(array, value, fromIndex) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return -1;
+ }
+ var index = length;
+ if (fromIndex !== undefined) {
+ index = toInteger(fromIndex);
+ index = index < 0 ? nativeMax(length + index, 0) : nativeMin(index, length - 1);
+ }
+ return value === value
+ ? strictLastIndexOf(array, value, index)
+ : baseFindIndex(array, baseIsNaN, index, true);
+ }
+
+ /**
+ * Gets the element at index `n` of `array`. If `n` is negative, the nth
+ * element from the end is returned.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.11.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=0] The index of the element to return.
+ * @returns {*} Returns the nth element of `array`.
+ * @example
+ *
+ * var array = ['a', 'b', 'c', 'd'];
+ *
+ * _.nth(array, 1);
+ * // => 'b'
+ *
+ * _.nth(array, -2);
+ * // => 'c';
+ */
+ function nth(array, n) {
+ return (array && array.length) ? baseNth(array, toInteger(n)) : undefined;
+ }
+
+ /**
+ * Removes all given values from `array` using
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * **Note:** Unlike `_.without`, this method mutates `array`. Use `_.remove`
+ * to remove elements from an array by predicate.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Array
+ * @param {Array} array The array to modify.
+ * @param {...*} [values] The values to remove.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = ['a', 'b', 'c', 'a', 'b', 'c'];
+ *
+ * _.pull(array, 'a', 'c');
+ * console.log(array);
+ * // => ['b', 'b']
+ */
+ var pull = baseRest(pullAll);
+
+ /**
+ * This method is like `_.pull` except that it accepts an array of values to remove.
+ *
+ * **Note:** Unlike `_.difference`, this method mutates `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to remove.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = ['a', 'b', 'c', 'a', 'b', 'c'];
+ *
+ * _.pullAll(array, ['a', 'c']);
+ * console.log(array);
+ * // => ['b', 'b']
+ */
+ function pullAll(array, values) {
+ return (array && array.length && values && values.length)
+ ? basePullAll(array, values)
+ : array;
+ }
+
+ /**
+ * This method is like `_.pullAll` except that it accepts `iteratee` which is
+ * invoked for each element of `array` and `values` to generate the criterion
+ * by which they're compared. The iteratee is invoked with one argument: (value).
+ *
+ * **Note:** Unlike `_.differenceBy`, this method mutates `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to remove.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = [{ 'x': 1 }, { 'x': 2 }, { 'x': 3 }, { 'x': 1 }];
+ *
+ * _.pullAllBy(array, [{ 'x': 1 }, { 'x': 3 }], 'x');
+ * console.log(array);
+ * // => [{ 'x': 2 }]
+ */
+ function pullAllBy(array, values, iteratee) {
+ return (array && array.length && values && values.length)
+ ? basePullAll(array, values, getIteratee(iteratee, 2))
+ : array;
+ }
+
+ /**
+ * This method is like `_.pullAll` except that it accepts `comparator` which
+ * is invoked to compare elements of `array` to `values`. The comparator is
+ * invoked with two arguments: (arrVal, othVal).
+ *
+ * **Note:** Unlike `_.differenceWith`, this method mutates `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.6.0
+ * @category Array
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to remove.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = [{ 'x': 1, 'y': 2 }, { 'x': 3, 'y': 4 }, { 'x': 5, 'y': 6 }];
+ *
+ * _.pullAllWith(array, [{ 'x': 3, 'y': 4 }], _.isEqual);
+ * console.log(array);
+ * // => [{ 'x': 1, 'y': 2 }, { 'x': 5, 'y': 6 }]
+ */
+ function pullAllWith(array, values, comparator) {
+ return (array && array.length && values && values.length)
+ ? basePullAll(array, values, undefined, comparator)
+ : array;
+ }
+
+ /**
+ * Removes elements from `array` corresponding to `indexes` and returns an
+ * array of removed elements.
+ *
+ * **Note:** Unlike `_.at`, this method mutates `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to modify.
+ * @param {...(number|number[])} [indexes] The indexes of elements to remove.
+ * @returns {Array} Returns the new array of removed elements.
+ * @example
+ *
+ * var array = ['a', 'b', 'c', 'd'];
+ * var pulled = _.pullAt(array, [1, 3]);
+ *
+ * console.log(array);
+ * // => ['a', 'c']
+ *
+ * console.log(pulled);
+ * // => ['b', 'd']
+ */
+ var pullAt = flatRest(function(array, indexes) {
+ var length = array == null ? 0 : array.length,
+ result = baseAt(array, indexes);
+
+ basePullAt(array, arrayMap(indexes, function(index) {
+ return isIndex(index, length) ? +index : index;
+ }).sort(compareAscending));
+
+ return result;
+ });
+
+ /**
+ * Removes all elements from `array` that `predicate` returns truthy for
+ * and returns an array of the removed elements. The predicate is invoked
+ * with three arguments: (value, index, array).
+ *
+ * **Note:** Unlike `_.filter`, this method mutates `array`. Use `_.pull`
+ * to pull elements from an array by value.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Array
+ * @param {Array} array The array to modify.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new array of removed elements.
+ * @example
+ *
+ * var array = [1, 2, 3, 4];
+ * var evens = _.remove(array, function(n) {
+ * return n % 2 == 0;
+ * });
+ *
+ * console.log(array);
+ * // => [1, 3]
+ *
+ * console.log(evens);
+ * // => [2, 4]
+ */
+ function remove(array, predicate) {
+ var result = [];
+ if (!(array && array.length)) {
+ return result;
+ }
+ var index = -1,
+ indexes = [],
+ length = array.length;
+
+ predicate = getIteratee(predicate, 3);
+ while (++index < length) {
+ var value = array[index];
+ if (predicate(value, index, array)) {
+ result.push(value);
+ indexes.push(index);
+ }
+ }
+ basePullAt(array, indexes);
+ return result;
+ }
+
+ /**
+ * Reverses `array` so that the first element becomes the last, the second
+ * element becomes the second to last, and so on.
+ *
+ * **Note:** This method mutates `array` and is based on
+ * [`Array#reverse`](https://mdn.io/Array/reverse).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to modify.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = [1, 2, 3];
+ *
+ * _.reverse(array);
+ * // => [3, 2, 1]
+ *
+ * console.log(array);
+ * // => [3, 2, 1]
+ */
+ function reverse(array) {
+ return array == null ? array : nativeReverse.call(array);
+ }
+
+ /**
+ * Creates a slice of `array` from `start` up to, but not including, `end`.
+ *
+ * **Note:** This method is used instead of
+ * [`Array#slice`](https://mdn.io/Array/slice) to ensure dense arrays are
+ * returned.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to slice.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the slice of `array`.
+ */
+ function slice(array, start, end) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return [];
+ }
+ if (end && typeof end != 'number' && isIterateeCall(array, start, end)) {
+ start = 0;
+ end = length;
+ }
+ else {
+ start = start == null ? 0 : toInteger(start);
+ end = end === undefined ? length : toInteger(end);
+ }
+ return baseSlice(array, start, end);
+ }
+
+ /**
+ * Uses a binary search to determine the lowest index at which `value`
+ * should be inserted into `array` in order to maintain its sort order.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ * @example
+ *
+ * _.sortedIndex([30, 50], 40);
+ * // => 1
+ */
+ function sortedIndex(array, value) {
+ return baseSortedIndex(array, value);
+ }
+
+ /**
+ * This method is like `_.sortedIndex` except that it accepts `iteratee`
+ * which is invoked for `value` and each element of `array` to compute their
+ * sort ranking. The iteratee is invoked with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ * @example
+ *
+ * var objects = [{ 'x': 4 }, { 'x': 5 }];
+ *
+ * _.sortedIndexBy(objects, { 'x': 4 }, function(o) { return o.x; });
+ * // => 0
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.sortedIndexBy(objects, { 'x': 4 }, 'x');
+ * // => 0
+ */
+ function sortedIndexBy(array, value, iteratee) {
+ return baseSortedIndexBy(array, value, getIteratee(iteratee, 2));
+ }
+
+ /**
+ * This method is like `_.indexOf` except that it performs a binary
+ * search on a sorted `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ * @example
+ *
+ * _.sortedIndexOf([4, 5, 5, 5, 6], 5);
+ * // => 1
+ */
+ function sortedIndexOf(array, value) {
+ var length = array == null ? 0 : array.length;
+ if (length) {
+ var index = baseSortedIndex(array, value);
+ if (index < length && eq(array[index], value)) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * This method is like `_.sortedIndex` except that it returns the highest
+ * index at which `value` should be inserted into `array` in order to
+ * maintain its sort order.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ * @example
+ *
+ * _.sortedLastIndex([4, 5, 5, 5, 6], 5);
+ * // => 4
+ */
+ function sortedLastIndex(array, value) {
+ return baseSortedIndex(array, value, true);
+ }
+
+ /**
+ * This method is like `_.sortedLastIndex` except that it accepts `iteratee`
+ * which is invoked for `value` and each element of `array` to compute their
+ * sort ranking. The iteratee is invoked with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ * @example
+ *
+ * var objects = [{ 'x': 4 }, { 'x': 5 }];
+ *
+ * _.sortedLastIndexBy(objects, { 'x': 4 }, function(o) { return o.x; });
+ * // => 1
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.sortedLastIndexBy(objects, { 'x': 4 }, 'x');
+ * // => 1
+ */
+ function sortedLastIndexBy(array, value, iteratee) {
+ return baseSortedIndexBy(array, value, getIteratee(iteratee, 2), true);
+ }
+
+ /**
+ * This method is like `_.lastIndexOf` except that it performs a binary
+ * search on a sorted `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to search for.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ * @example
+ *
+ * _.sortedLastIndexOf([4, 5, 5, 5, 6], 5);
+ * // => 3
+ */
+ function sortedLastIndexOf(array, value) {
+ var length = array == null ? 0 : array.length;
+ if (length) {
+ var index = baseSortedIndex(array, value, true) - 1;
+ if (eq(array[index], value)) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * This method is like `_.uniq` except that it's designed and optimized
+ * for sorted arrays.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @returns {Array} Returns the new duplicate free array.
+ * @example
+ *
+ * _.sortedUniq([1, 1, 2]);
+ * // => [1, 2]
+ */
+ function sortedUniq(array) {
+ return (array && array.length)
+ ? baseSortedUniq(array)
+ : [];
+ }
+
+ /**
+ * This method is like `_.uniqBy` except that it's designed and optimized
+ * for sorted arrays.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {Function} [iteratee] The iteratee invoked per element.
+ * @returns {Array} Returns the new duplicate free array.
+ * @example
+ *
+ * _.sortedUniqBy([1.1, 1.2, 2.3, 2.4], Math.floor);
+ * // => [1.1, 2.3]
+ */
+ function sortedUniqBy(array, iteratee) {
+ return (array && array.length)
+ ? baseSortedUniq(array, getIteratee(iteratee, 2))
+ : [];
+ }
+
+ /**
+ * Gets all but the first element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.tail([1, 2, 3]);
+ * // => [2, 3]
+ */
+ function tail(array) {
+ var length = array == null ? 0 : array.length;
+ return length ? baseSlice(array, 1, length) : [];
+ }
+
+ /**
+ * Creates a slice of `array` with `n` elements taken from the beginning.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=1] The number of elements to take.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.take([1, 2, 3]);
+ * // => [1]
+ *
+ * _.take([1, 2, 3], 2);
+ * // => [1, 2]
+ *
+ * _.take([1, 2, 3], 5);
+ * // => [1, 2, 3]
+ *
+ * _.take([1, 2, 3], 0);
+ * // => []
+ */
+ function take(array, n, guard) {
+ if (!(array && array.length)) {
+ return [];
+ }
+ n = (guard || n === undefined) ? 1 : toInteger(n);
+ return baseSlice(array, 0, n < 0 ? 0 : n);
+ }
+
+ /**
+ * Creates a slice of `array` with `n` elements taken from the end.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=1] The number of elements to take.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.takeRight([1, 2, 3]);
+ * // => [3]
+ *
+ * _.takeRight([1, 2, 3], 2);
+ * // => [2, 3]
+ *
+ * _.takeRight([1, 2, 3], 5);
+ * // => [1, 2, 3]
+ *
+ * _.takeRight([1, 2, 3], 0);
+ * // => []
+ */
+ function takeRight(array, n, guard) {
+ var length = array == null ? 0 : array.length;
+ if (!length) {
+ return [];
+ }
+ n = (guard || n === undefined) ? 1 : toInteger(n);
+ n = length - n;
+ return baseSlice(array, n < 0 ? 0 : n, length);
+ }
+
+ /**
+ * Creates a slice of `array` with elements taken from the end. Elements are
+ * taken until `predicate` returns falsey. The predicate is invoked with
+ * three arguments: (value, index, array).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': false }
+ * ];
+ *
+ * _.takeRightWhile(users, function(o) { return !o.active; });
+ * // => objects for ['fred', 'pebbles']
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.takeRightWhile(users, { 'user': 'pebbles', 'active': false });
+ * // => objects for ['pebbles']
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.takeRightWhile(users, ['active', false]);
+ * // => objects for ['fred', 'pebbles']
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.takeRightWhile(users, 'active');
+ * // => []
+ */
+ function takeRightWhile(array, predicate) {
+ return (array && array.length)
+ ? baseWhile(array, getIteratee(predicate, 3), false, true)
+ : [];
+ }
+
+ /**
+ * Creates a slice of `array` with elements taken from the beginning. Elements
+ * are taken until `predicate` returns falsey. The predicate is invoked with
+ * three arguments: (value, index, array).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': true }
+ * ];
+ *
+ * _.takeWhile(users, function(o) { return !o.active; });
+ * // => objects for ['barney', 'fred']
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.takeWhile(users, { 'user': 'barney', 'active': false });
+ * // => objects for ['barney']
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.takeWhile(users, ['active', false]);
+ * // => objects for ['barney', 'fred']
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.takeWhile(users, 'active');
+ * // => []
+ */
+ function takeWhile(array, predicate) {
+ return (array && array.length)
+ ? baseWhile(array, getIteratee(predicate, 3))
+ : [];
+ }
+
+ /**
+ * Creates an array of unique values, in order, from all given arrays using
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @returns {Array} Returns the new array of combined values.
+ * @example
+ *
+ * _.union([2], [1, 2]);
+ * // => [2, 1]
+ */
+ var union = baseRest(function(arrays) {
+ return baseUniq(baseFlatten(arrays, 1, isArrayLikeObject, true));
+ });
+
+ /**
+ * This method is like `_.union` except that it accepts `iteratee` which is
+ * invoked for each element of each `arrays` to generate the criterion by
+ * which uniqueness is computed. Result values are chosen from the first
+ * array in which the value occurs. The iteratee is invoked with one argument:
+ * (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {Array} Returns the new array of combined values.
+ * @example
+ *
+ * _.unionBy([2.1], [1.2, 2.3], Math.floor);
+ * // => [2.1, 1.2]
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.unionBy([{ 'x': 1 }], [{ 'x': 2 }, { 'x': 1 }], 'x');
+ * // => [{ 'x': 1 }, { 'x': 2 }]
+ */
+ var unionBy = baseRest(function(arrays) {
+ var iteratee = last(arrays);
+ if (isArrayLikeObject(iteratee)) {
+ iteratee = undefined;
+ }
+ return baseUniq(baseFlatten(arrays, 1, isArrayLikeObject, true), getIteratee(iteratee, 2));
+ });
+
+ /**
+ * This method is like `_.union` except that it accepts `comparator` which
+ * is invoked to compare elements of `arrays`. Result values are chosen from
+ * the first array in which the value occurs. The comparator is invoked
+ * with two arguments: (arrVal, othVal).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of combined values.
+ * @example
+ *
+ * var objects = [{ 'x': 1, 'y': 2 }, { 'x': 2, 'y': 1 }];
+ * var others = [{ 'x': 1, 'y': 1 }, { 'x': 1, 'y': 2 }];
+ *
+ * _.unionWith(objects, others, _.isEqual);
+ * // => [{ 'x': 1, 'y': 2 }, { 'x': 2, 'y': 1 }, { 'x': 1, 'y': 1 }]
+ */
+ var unionWith = baseRest(function(arrays) {
+ var comparator = last(arrays);
+ comparator = typeof comparator == 'function' ? comparator : undefined;
+ return baseUniq(baseFlatten(arrays, 1, isArrayLikeObject, true), undefined, comparator);
+ });
+
+ /**
+ * Creates a duplicate-free version of an array, using
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons, in which only the first occurrence of each element
+ * is kept. The order of result values is determined by the order they occur
+ * in the array.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @returns {Array} Returns the new duplicate free array.
+ * @example
+ *
+ * _.uniq([2, 1, 2]);
+ * // => [2, 1]
+ */
+ function uniq(array) {
+ return (array && array.length) ? baseUniq(array) : [];
+ }
+
+ /**
+ * This method is like `_.uniq` except that it accepts `iteratee` which is
+ * invoked for each element in `array` to generate the criterion by which
+ * uniqueness is computed. The order of result values is determined by the
+ * order they occur in the array. The iteratee is invoked with one argument:
+ * (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {Array} Returns the new duplicate free array.
+ * @example
+ *
+ * _.uniqBy([2.1, 1.2, 2.3], Math.floor);
+ * // => [2.1, 1.2]
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.uniqBy([{ 'x': 1 }, { 'x': 2 }, { 'x': 1 }], 'x');
+ * // => [{ 'x': 1 }, { 'x': 2 }]
+ */
+ function uniqBy(array, iteratee) {
+ return (array && array.length) ? baseUniq(array, getIteratee(iteratee, 2)) : [];
+ }
+
+ /**
+ * This method is like `_.uniq` except that it accepts `comparator` which
+ * is invoked to compare elements of `array`. The order of result values is
+ * determined by the order they occur in the array.The comparator is invoked
+ * with two arguments: (arrVal, othVal).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new duplicate free array.
+ * @example
+ *
+ * var objects = [{ 'x': 1, 'y': 2 }, { 'x': 2, 'y': 1 }, { 'x': 1, 'y': 2 }];
+ *
+ * _.uniqWith(objects, _.isEqual);
+ * // => [{ 'x': 1, 'y': 2 }, { 'x': 2, 'y': 1 }]
+ */
+ function uniqWith(array, comparator) {
+ comparator = typeof comparator == 'function' ? comparator : undefined;
+ return (array && array.length) ? baseUniq(array, undefined, comparator) : [];
+ }
+
+ /**
+ * This method is like `_.zip` except that it accepts an array of grouped
+ * elements and creates an array regrouping the elements to their pre-zip
+ * configuration.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.2.0
+ * @category Array
+ * @param {Array} array The array of grouped elements to process.
+ * @returns {Array} Returns the new array of regrouped elements.
+ * @example
+ *
+ * var zipped = _.zip(['a', 'b'], [1, 2], [true, false]);
+ * // => [['a', 1, true], ['b', 2, false]]
+ *
+ * _.unzip(zipped);
+ * // => [['a', 'b'], [1, 2], [true, false]]
+ */
+ function unzip(array) {
+ if (!(array && array.length)) {
+ return [];
+ }
+ var length = 0;
+ array = arrayFilter(array, function(group) {
+ if (isArrayLikeObject(group)) {
+ length = nativeMax(group.length, length);
+ return true;
+ }
+ });
+ return baseTimes(length, function(index) {
+ return arrayMap(array, baseProperty(index));
+ });
+ }
+
+ /**
+ * This method is like `_.unzip` except that it accepts `iteratee` to specify
+ * how regrouped values should be combined. The iteratee is invoked with the
+ * elements of each group: (...group).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.8.0
+ * @category Array
+ * @param {Array} array The array of grouped elements to process.
+ * @param {Function} [iteratee=_.identity] The function to combine
+ * regrouped values.
+ * @returns {Array} Returns the new array of regrouped elements.
+ * @example
+ *
+ * var zipped = _.zip([1, 2], [10, 20], [100, 200]);
+ * // => [[1, 10, 100], [2, 20, 200]]
+ *
+ * _.unzipWith(zipped, _.add);
+ * // => [3, 30, 300]
+ */
+ function unzipWith(array, iteratee) {
+ if (!(array && array.length)) {
+ return [];
+ }
+ var result = unzip(array);
+ if (iteratee == null) {
+ return result;
+ }
+ return arrayMap(result, function(group) {
+ return apply(iteratee, undefined, group);
+ });
+ }
+
+ /**
+ * Creates an array excluding all given values using
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * **Note:** Unlike `_.pull`, this method returns a new array.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {...*} [values] The values to exclude.
+ * @returns {Array} Returns the new array of filtered values.
+ * @see _.difference, _.xor
+ * @example
+ *
+ * _.without([2, 1, 2, 3], 1, 2);
+ * // => [3]
+ */
+ var without = baseRest(function(array, values) {
+ return isArrayLikeObject(array)
+ ? baseDifference(array, values)
+ : [];
+ });
+
+ /**
+ * Creates an array of unique values that is the
+ * [symmetric difference](https://en.wikipedia.org/wiki/Symmetric_difference)
+ * of the given arrays. The order of result values is determined by the order
+ * they occur in the arrays.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.4.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @returns {Array} Returns the new array of filtered values.
+ * @see _.difference, _.without
+ * @example
+ *
+ * _.xor([2, 1], [2, 3]);
+ * // => [1, 3]
+ */
+ var xor = baseRest(function(arrays) {
+ return baseXor(arrayFilter(arrays, isArrayLikeObject));
+ });
+
+ /**
+ * This method is like `_.xor` except that it accepts `iteratee` which is
+ * invoked for each element of each `arrays` to generate the criterion by
+ * which by which they're compared. The order of result values is determined
+ * by the order they occur in the arrays. The iteratee is invoked with one
+ * argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * _.xorBy([2.1, 1.2], [2.3, 3.4], Math.floor);
+ * // => [1.2, 3.4]
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.xorBy([{ 'x': 1 }], [{ 'x': 2 }, { 'x': 1 }], 'x');
+ * // => [{ 'x': 2 }]
+ */
+ var xorBy = baseRest(function(arrays) {
+ var iteratee = last(arrays);
+ if (isArrayLikeObject(iteratee)) {
+ iteratee = undefined;
+ }
+ return baseXor(arrayFilter(arrays, isArrayLikeObject), getIteratee(iteratee, 2));
+ });
+
+ /**
+ * This method is like `_.xor` except that it accepts `comparator` which is
+ * invoked to compare elements of `arrays`. The order of result values is
+ * determined by the order they occur in the arrays. The comparator is invoked
+ * with two arguments: (arrVal, othVal).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @param {Function} [comparator] The comparator invoked per element.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * var objects = [{ 'x': 1, 'y': 2 }, { 'x': 2, 'y': 1 }];
+ * var others = [{ 'x': 1, 'y': 1 }, { 'x': 1, 'y': 2 }];
+ *
+ * _.xorWith(objects, others, _.isEqual);
+ * // => [{ 'x': 2, 'y': 1 }, { 'x': 1, 'y': 1 }]
+ */
+ var xorWith = baseRest(function(arrays) {
+ var comparator = last(arrays);
+ comparator = typeof comparator == 'function' ? comparator : undefined;
+ return baseXor(arrayFilter(arrays, isArrayLikeObject), undefined, comparator);
+ });
+
+ /**
+ * Creates an array of grouped elements, the first of which contains the
+ * first elements of the given arrays, the second of which contains the
+ * second elements of the given arrays, and so on.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to process.
+ * @returns {Array} Returns the new array of grouped elements.
+ * @example
+ *
+ * _.zip(['a', 'b'], [1, 2], [true, false]);
+ * // => [['a', 1, true], ['b', 2, false]]
+ */
+ var zip = baseRest(unzip);
+
+ /**
+ * This method is like `_.fromPairs` except that it accepts two arrays,
+ * one of property identifiers and one of corresponding values.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.4.0
+ * @category Array
+ * @param {Array} [props=[]] The property identifiers.
+ * @param {Array} [values=[]] The property values.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * _.zipObject(['a', 'b'], [1, 2]);
+ * // => { 'a': 1, 'b': 2 }
+ */
+ function zipObject(props, values) {
+ return baseZipObject(props || [], values || [], assignValue);
+ }
+
+ /**
+ * This method is like `_.zipObject` except that it supports property paths.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.1.0
+ * @category Array
+ * @param {Array} [props=[]] The property identifiers.
+ * @param {Array} [values=[]] The property values.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * _.zipObjectDeep(['a.b[0].c', 'a.b[1].d'], [1, 2]);
+ * // => { 'a': { 'b': [{ 'c': 1 }, { 'd': 2 }] } }
+ */
+ function zipObjectDeep(props, values) {
+ return baseZipObject(props || [], values || [], baseSet);
+ }
+
+ /**
+ * This method is like `_.zip` except that it accepts `iteratee` to specify
+ * how grouped values should be combined. The iteratee is invoked with the
+ * elements of each group: (...group).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.8.0
+ * @category Array
+ * @param {...Array} [arrays] The arrays to process.
+ * @param {Function} [iteratee=_.identity] The function to combine
+ * grouped values.
+ * @returns {Array} Returns the new array of grouped elements.
+ * @example
+ *
+ * _.zipWith([1, 2], [10, 20], [100, 200], function(a, b, c) {
+ * return a + b + c;
+ * });
+ * // => [111, 222]
+ */
+ var zipWith = baseRest(function(arrays) {
+ var length = arrays.length,
+ iteratee = length > 1 ? arrays[length - 1] : undefined;
+
+ iteratee = typeof iteratee == 'function' ? (arrays.pop(), iteratee) : undefined;
+ return unzipWith(arrays, iteratee);
+ });
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a `lodash` wrapper instance that wraps `value` with explicit method
+ * chain sequences enabled. The result of such sequences must be unwrapped
+ * with `_#value`.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.3.0
+ * @category Seq
+ * @param {*} value The value to wrap.
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 },
+ * { 'user': 'pebbles', 'age': 1 }
+ * ];
+ *
+ * var youngest = _
+ * .chain(users)
+ * .sortBy('age')
+ * .map(function(o) {
+ * return o.user + ' is ' + o.age;
+ * })
+ * .head()
+ * .value();
+ * // => 'pebbles is 1'
+ */
+ function chain(value) {
+ var result = lodash(value);
+ result.__chain__ = true;
+ return result;
+ }
+
+ /**
+ * This method invokes `interceptor` and returns `value`. The interceptor
+ * is invoked with one argument; (value). The purpose of this method is to
+ * "tap into" a method chain sequence in order to modify intermediate results.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Seq
+ * @param {*} value The value to provide to `interceptor`.
+ * @param {Function} interceptor The function to invoke.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * _([1, 2, 3])
+ * .tap(function(array) {
+ * // Mutate input array.
+ * array.pop();
+ * })
+ * .reverse()
+ * .value();
+ * // => [2, 1]
+ */
+ function tap(value, interceptor) {
+ interceptor(value);
+ return value;
+ }
+
+ /**
+ * This method is like `_.tap` except that it returns the result of `interceptor`.
+ * The purpose of this method is to "pass thru" values replacing intermediate
+ * results in a method chain sequence.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Seq
+ * @param {*} value The value to provide to `interceptor`.
+ * @param {Function} interceptor The function to invoke.
+ * @returns {*} Returns the result of `interceptor`.
+ * @example
+ *
+ * _(' abc ')
+ * .chain()
+ * .trim()
+ * .thru(function(value) {
+ * return [value];
+ * })
+ * .value();
+ * // => ['abc']
+ */
+ function thru(value, interceptor) {
+ return interceptor(value);
+ }
+
+ /**
+ * This method is the wrapper version of `_.at`.
+ *
+ * @name at
+ * @memberOf _
+ * @since 1.0.0
+ * @category Seq
+ * @param {...(string|string[])} [paths] The property paths to pick.
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': 3 } }, 4] };
+ *
+ * _(object).at(['a[0].b.c', 'a[1]']).value();
+ * // => [3, 4]
+ */
+ var wrapperAt = flatRest(function(paths) {
+ var length = paths.length,
+ start = length ? paths[0] : 0,
+ value = this.__wrapped__,
+ interceptor = function(object) { return baseAt(object, paths); };
+
+ if (length > 1 || this.__actions__.length ||
+ !(value instanceof LazyWrapper) || !isIndex(start)) {
+ return this.thru(interceptor);
+ }
+ value = value.slice(start, +start + (length ? 1 : 0));
+ value.__actions__.push({
+ 'func': thru,
+ 'args': [interceptor],
+ 'thisArg': undefined
+ });
+ return new LodashWrapper(value, this.__chain__).thru(function(array) {
+ if (length && !array.length) {
+ array.push(undefined);
+ }
+ return array;
+ });
+ });
+
+ /**
+ * Creates a `lodash` wrapper instance with explicit method chain sequences enabled.
+ *
+ * @name chain
+ * @memberOf _
+ * @since 0.1.0
+ * @category Seq
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 }
+ * ];
+ *
+ * // A sequence without explicit chaining.
+ * _(users).head();
+ * // => { 'user': 'barney', 'age': 36 }
+ *
+ * // A sequence with explicit chaining.
+ * _(users)
+ * .chain()
+ * .head()
+ * .pick('user')
+ * .value();
+ * // => { 'user': 'barney' }
+ */
+ function wrapperChain() {
+ return chain(this);
+ }
+
+ /**
+ * Executes the chain sequence and returns the wrapped result.
+ *
+ * @name commit
+ * @memberOf _
+ * @since 3.2.0
+ * @category Seq
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var array = [1, 2];
+ * var wrapped = _(array).push(3);
+ *
+ * console.log(array);
+ * // => [1, 2]
+ *
+ * wrapped = wrapped.commit();
+ * console.log(array);
+ * // => [1, 2, 3]
+ *
+ * wrapped.last();
+ * // => 3
+ *
+ * console.log(array);
+ * // => [1, 2, 3]
+ */
+ function wrapperCommit() {
+ return new LodashWrapper(this.value(), this.__chain__);
+ }
+
+ /**
+ * Gets the next value on a wrapped object following the
+ * [iterator protocol](https://mdn.io/iteration_protocols#iterator).
+ *
+ * @name next
+ * @memberOf _
+ * @since 4.0.0
+ * @category Seq
+ * @returns {Object} Returns the next iterator value.
+ * @example
+ *
+ * var wrapped = _([1, 2]);
+ *
+ * wrapped.next();
+ * // => { 'done': false, 'value': 1 }
+ *
+ * wrapped.next();
+ * // => { 'done': false, 'value': 2 }
+ *
+ * wrapped.next();
+ * // => { 'done': true, 'value': undefined }
+ */
+ function wrapperNext() {
+ if (this.__values__ === undefined) {
+ this.__values__ = toArray(this.value());
+ }
+ var done = this.__index__ >= this.__values__.length,
+ value = done ? undefined : this.__values__[this.__index__++];
+
+ return { 'done': done, 'value': value };
+ }
+
+ /**
+ * Enables the wrapper to be iterable.
+ *
+ * @name Symbol.iterator
+ * @memberOf _
+ * @since 4.0.0
+ * @category Seq
+ * @returns {Object} Returns the wrapper object.
+ * @example
+ *
+ * var wrapped = _([1, 2]);
+ *
+ * wrapped[Symbol.iterator]() === wrapped;
+ * // => true
+ *
+ * Array.from(wrapped);
+ * // => [1, 2]
+ */
+ function wrapperToIterator() {
+ return this;
+ }
+
+ /**
+ * Creates a clone of the chain sequence planting `value` as the wrapped value.
+ *
+ * @name plant
+ * @memberOf _
+ * @since 3.2.0
+ * @category Seq
+ * @param {*} value The value to plant.
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * var wrapped = _([1, 2]).map(square);
+ * var other = wrapped.plant([3, 4]);
+ *
+ * other.value();
+ * // => [9, 16]
+ *
+ * wrapped.value();
+ * // => [1, 4]
+ */
+ function wrapperPlant(value) {
+ var result,
+ parent = this;
+
+ while (parent instanceof baseLodash) {
+ var clone = wrapperClone(parent);
+ clone.__index__ = 0;
+ clone.__values__ = undefined;
+ if (result) {
+ previous.__wrapped__ = clone;
+ } else {
+ result = clone;
+ }
+ var previous = clone;
+ parent = parent.__wrapped__;
+ }
+ previous.__wrapped__ = value;
+ return result;
+ }
+
+ /**
+ * This method is the wrapper version of `_.reverse`.
+ *
+ * **Note:** This method mutates the wrapped array.
+ *
+ * @name reverse
+ * @memberOf _
+ * @since 0.1.0
+ * @category Seq
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var array = [1, 2, 3];
+ *
+ * _(array).reverse().value()
+ * // => [3, 2, 1]
+ *
+ * console.log(array);
+ * // => [3, 2, 1]
+ */
+ function wrapperReverse() {
+ var value = this.__wrapped__;
+ if (value instanceof LazyWrapper) {
+ var wrapped = value;
+ if (this.__actions__.length) {
+ wrapped = new LazyWrapper(this);
+ }
+ wrapped = wrapped.reverse();
+ wrapped.__actions__.push({
+ 'func': thru,
+ 'args': [reverse],
+ 'thisArg': undefined
+ });
+ return new LodashWrapper(wrapped, this.__chain__);
+ }
+ return this.thru(reverse);
+ }
+
+ /**
+ * Executes the chain sequence to resolve the unwrapped value.
+ *
+ * @name value
+ * @memberOf _
+ * @since 0.1.0
+ * @alias toJSON, valueOf
+ * @category Seq
+ * @returns {*} Returns the resolved unwrapped value.
+ * @example
+ *
+ * _([1, 2, 3]).value();
+ * // => [1, 2, 3]
+ */
+ function wrapperValue() {
+ return baseWrapperValue(this.__wrapped__, this.__actions__);
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` thru `iteratee`. The corresponding value of
+ * each key is the number of times the key was returned by `iteratee`. The
+ * iteratee is invoked with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.5.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The iteratee to transform keys.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.countBy([6.1, 4.2, 6.3], Math.floor);
+ * // => { '4': 1, '6': 2 }
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.countBy(['one', 'two', 'three'], 'length');
+ * // => { '3': 2, '5': 1 }
+ */
+ var countBy = createAggregator(function(result, value, key) {
+ if (hasOwnProperty.call(result, key)) {
+ ++result[key];
+ } else {
+ baseAssignValue(result, key, 1);
+ }
+ });
+
+ /**
+ * Checks if `predicate` returns truthy for **all** elements of `collection`.
+ * Iteration is stopped once `predicate` returns falsey. The predicate is
+ * invoked with three arguments: (value, index|key, collection).
+ *
+ * **Note:** This method returns `true` for
+ * [empty collections](https://en.wikipedia.org/wiki/Empty_set) because
+ * [everything is true](https://en.wikipedia.org/wiki/Vacuous_truth) of
+ * elements of empty collections.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ * @example
+ *
+ * _.every([true, 1, null, 'yes'], Boolean);
+ * // => false
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': false },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.every(users, { 'user': 'barney', 'active': false });
+ * // => false
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.every(users, ['active', false]);
+ * // => true
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.every(users, 'active');
+ * // => false
+ */
+ function every(collection, predicate, guard) {
+ var func = isArray(collection) ? arrayEvery : baseEvery;
+ if (guard && isIterateeCall(collection, predicate, guard)) {
+ predicate = undefined;
+ }
+ return func(collection, getIteratee(predicate, 3));
+ }
+
+ /**
+ * Iterates over elements of `collection`, returning an array of all elements
+ * `predicate` returns truthy for. The predicate is invoked with three
+ * arguments: (value, index|key, collection).
+ *
+ * **Note:** Unlike `_.remove`, this method returns a new array.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ * @see _.reject
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * _.filter(users, function(o) { return !o.active; });
+ * // => objects for ['fred']
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.filter(users, { 'age': 36, 'active': true });
+ * // => objects for ['barney']
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.filter(users, ['active', false]);
+ * // => objects for ['fred']
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.filter(users, 'active');
+ * // => objects for ['barney']
+ *
+ * // Combining several predicates using `_.overEvery` or `_.overSome`.
+ * _.filter(users, _.overSome([{ 'age': 36 }, ['age', 40]]));
+ * // => objects for ['fred', 'barney']
+ */
+ function filter(collection, predicate) {
+ var func = isArray(collection) ? arrayFilter : baseFilter;
+ return func(collection, getIteratee(predicate, 3));
+ }
+
+ /**
+ * Iterates over elements of `collection`, returning the first element
+ * `predicate` returns truthy for. The predicate is invoked with three
+ * arguments: (value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {*} Returns the matched element, else `undefined`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false },
+ * { 'user': 'pebbles', 'age': 1, 'active': true }
+ * ];
+ *
+ * _.find(users, function(o) { return o.age < 40; });
+ * // => object for 'barney'
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.find(users, { 'age': 1, 'active': true });
+ * // => object for 'pebbles'
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.find(users, ['active', false]);
+ * // => object for 'fred'
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.find(users, 'active');
+ * // => object for 'barney'
+ */
+ var find = createFind(findIndex);
+
+ /**
+ * This method is like `_.find` except that it iterates over elements of
+ * `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param {number} [fromIndex=collection.length-1] The index to search from.
+ * @returns {*} Returns the matched element, else `undefined`.
+ * @example
+ *
+ * _.findLast([1, 2, 3, 4], function(n) {
+ * return n % 2 == 1;
+ * });
+ * // => 3
+ */
+ var findLast = createFind(findLastIndex);
+
+ /**
+ * Creates a flattened array of values by running each element in `collection`
+ * thru `iteratee` and flattening the mapped results. The iteratee is invoked
+ * with three arguments: (value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * function duplicate(n) {
+ * return [n, n];
+ * }
+ *
+ * _.flatMap([1, 2], duplicate);
+ * // => [1, 1, 2, 2]
+ */
+ function flatMap(collection, iteratee) {
+ return baseFlatten(map(collection, iteratee), 1);
+ }
+
+ /**
+ * This method is like `_.flatMap` except that it recursively flattens the
+ * mapped results.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.7.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * function duplicate(n) {
+ * return [[[n, n]]];
+ * }
+ *
+ * _.flatMapDeep([1, 2], duplicate);
+ * // => [1, 1, 2, 2]
+ */
+ function flatMapDeep(collection, iteratee) {
+ return baseFlatten(map(collection, iteratee), INFINITY);
+ }
+
+ /**
+ * This method is like `_.flatMap` except that it recursively flattens the
+ * mapped results up to `depth` times.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.7.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {number} [depth=1] The maximum recursion depth.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * function duplicate(n) {
+ * return [[[n, n]]];
+ * }
+ *
+ * _.flatMapDepth([1, 2], duplicate, 2);
+ * // => [[1, 1], [2, 2]]
+ */
+ function flatMapDepth(collection, iteratee, depth) {
+ depth = depth === undefined ? 1 : toInteger(depth);
+ return baseFlatten(map(collection, iteratee), depth);
+ }
+
+ /**
+ * Iterates over elements of `collection` and invokes `iteratee` for each element.
+ * The iteratee is invoked with three arguments: (value, index|key, collection).
+ * Iteratee functions may exit iteration early by explicitly returning `false`.
+ *
+ * **Note:** As with other "Collections" methods, objects with a "length"
+ * property are iterated like arrays. To avoid this behavior use `_.forIn`
+ * or `_.forOwn` for object iteration.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @alias each
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array|Object} Returns `collection`.
+ * @see _.forEachRight
+ * @example
+ *
+ * _.forEach([1, 2], function(value) {
+ * console.log(value);
+ * });
+ * // => Logs `1` then `2`.
+ *
+ * _.forEach({ 'a': 1, 'b': 2 }, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'a' then 'b' (iteration order is not guaranteed).
+ */
+ function forEach(collection, iteratee) {
+ var func = isArray(collection) ? arrayEach : baseEach;
+ return func(collection, getIteratee(iteratee, 3));
+ }
+
+ /**
+ * This method is like `_.forEach` except that it iterates over elements of
+ * `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @alias eachRight
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array|Object} Returns `collection`.
+ * @see _.forEach
+ * @example
+ *
+ * _.forEachRight([1, 2], function(value) {
+ * console.log(value);
+ * });
+ * // => Logs `2` then `1`.
+ */
+ function forEachRight(collection, iteratee) {
+ var func = isArray(collection) ? arrayEachRight : baseEachRight;
+ return func(collection, getIteratee(iteratee, 3));
+ }
+
+ /**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` thru `iteratee`. The order of grouped values
+ * is determined by the order they occur in `collection`. The corresponding
+ * value of each key is an array of elements responsible for generating the
+ * key. The iteratee is invoked with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The iteratee to transform keys.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.groupBy([6.1, 4.2, 6.3], Math.floor);
+ * // => { '4': [4.2], '6': [6.1, 6.3] }
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.groupBy(['one', 'two', 'three'], 'length');
+ * // => { '3': ['one', 'two'], '5': ['three'] }
+ */
+ var groupBy = createAggregator(function(result, value, key) {
+ if (hasOwnProperty.call(result, key)) {
+ result[key].push(value);
+ } else {
+ baseAssignValue(result, key, [value]);
+ }
+ });
+
+ /**
+ * Checks if `value` is in `collection`. If `collection` is a string, it's
+ * checked for a substring of `value`, otherwise
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * is used for equality comparisons. If `fromIndex` is negative, it's used as
+ * the offset from the end of `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to inspect.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.reduce`.
+ * @returns {boolean} Returns `true` if `value` is found, else `false`.
+ * @example
+ *
+ * _.includes([1, 2, 3], 1);
+ * // => true
+ *
+ * _.includes([1, 2, 3], 1, 2);
+ * // => false
+ *
+ * _.includes({ 'a': 1, 'b': 2 }, 1);
+ * // => true
+ *
+ * _.includes('abcd', 'bc');
+ * // => true
+ */
+ function includes(collection, value, fromIndex, guard) {
+ collection = isArrayLike(collection) ? collection : values(collection);
+ fromIndex = (fromIndex && !guard) ? toInteger(fromIndex) : 0;
+
+ var length = collection.length;
+ if (fromIndex < 0) {
+ fromIndex = nativeMax(length + fromIndex, 0);
+ }
+ return isString(collection)
+ ? (fromIndex <= length && collection.indexOf(value, fromIndex) > -1)
+ : (!!length && baseIndexOf(collection, value, fromIndex) > -1);
+ }
+
+ /**
+ * Invokes the method at `path` of each element in `collection`, returning
+ * an array of the results of each invoked method. Any additional arguments
+ * are provided to each invoked method. If `path` is a function, it's invoked
+ * for, and `this` bound to, each element in `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Array|Function|string} path The path of the method to invoke or
+ * the function invoked per iteration.
+ * @param {...*} [args] The arguments to invoke each method with.
+ * @returns {Array} Returns the array of results.
+ * @example
+ *
+ * _.invokeMap([[5, 1, 7], [3, 2, 1]], 'sort');
+ * // => [[1, 5, 7], [1, 2, 3]]
+ *
+ * _.invokeMap([123, 456], String.prototype.split, '');
+ * // => [['1', '2', '3'], ['4', '5', '6']]
+ */
+ var invokeMap = baseRest(function(collection, path, args) {
+ var index = -1,
+ isFunc = typeof path == 'function',
+ result = isArrayLike(collection) ? Array(collection.length) : [];
+
+ baseEach(collection, function(value) {
+ result[++index] = isFunc ? apply(path, value, args) : baseInvoke(value, path, args);
+ });
+ return result;
+ });
+
+ /**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` thru `iteratee`. The corresponding value of
+ * each key is the last element responsible for generating the key. The
+ * iteratee is invoked with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The iteratee to transform keys.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * var array = [
+ * { 'dir': 'left', 'code': 97 },
+ * { 'dir': 'right', 'code': 100 }
+ * ];
+ *
+ * _.keyBy(array, function(o) {
+ * return String.fromCharCode(o.code);
+ * });
+ * // => { 'a': { 'dir': 'left', 'code': 97 }, 'd': { 'dir': 'right', 'code': 100 } }
+ *
+ * _.keyBy(array, 'dir');
+ * // => { 'left': { 'dir': 'left', 'code': 97 }, 'right': { 'dir': 'right', 'code': 100 } }
+ */
+ var keyBy = createAggregator(function(result, value, key) {
+ baseAssignValue(result, key, value);
+ });
+
+ /**
+ * Creates an array of values by running each element in `collection` thru
+ * `iteratee`. The iteratee is invoked with three arguments:
+ * (value, index|key, collection).
+ *
+ * Many lodash methods are guarded to work as iteratees for methods like
+ * `_.every`, `_.filter`, `_.map`, `_.mapValues`, `_.reject`, and `_.some`.
+ *
+ * The guarded methods are:
+ * `ary`, `chunk`, `curry`, `curryRight`, `drop`, `dropRight`, `every`,
+ * `fill`, `invert`, `parseInt`, `random`, `range`, `rangeRight`, `repeat`,
+ * `sampleSize`, `slice`, `some`, `sortBy`, `split`, `take`, `takeRight`,
+ * `template`, `trim`, `trimEnd`, `trimStart`, and `words`
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ * @example
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * _.map([4, 8], square);
+ * // => [16, 64]
+ *
+ * _.map({ 'a': 4, 'b': 8 }, square);
+ * // => [16, 64] (iteration order is not guaranteed)
+ *
+ * var users = [
+ * { 'user': 'barney' },
+ * { 'user': 'fred' }
+ * ];
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.map(users, 'user');
+ * // => ['barney', 'fred']
+ */
+ function map(collection, iteratee) {
+ var func = isArray(collection) ? arrayMap : baseMap;
+ return func(collection, getIteratee(iteratee, 3));
+ }
+
+ /**
+ * This method is like `_.sortBy` except that it allows specifying the sort
+ * orders of the iteratees to sort by. If `orders` is unspecified, all values
+ * are sorted in ascending order. Otherwise, specify an order of "desc" for
+ * descending or "asc" for ascending sort order of corresponding values.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Array[]|Function[]|Object[]|string[]} [iteratees=[_.identity]]
+ * The iteratees to sort by.
+ * @param {string[]} [orders] The sort orders of `iteratees`.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.reduce`.
+ * @returns {Array} Returns the new sorted array.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'fred', 'age': 48 },
+ * { 'user': 'barney', 'age': 34 },
+ * { 'user': 'fred', 'age': 40 },
+ * { 'user': 'barney', 'age': 36 }
+ * ];
+ *
+ * // Sort by `user` in ascending order and by `age` in descending order.
+ * _.orderBy(users, ['user', 'age'], ['asc', 'desc']);
+ * // => objects for [['barney', 36], ['barney', 34], ['fred', 48], ['fred', 40]]
+ */
+ function orderBy(collection, iteratees, orders, guard) {
+ if (collection == null) {
+ return [];
+ }
+ if (!isArray(iteratees)) {
+ iteratees = iteratees == null ? [] : [iteratees];
+ }
+ orders = guard ? undefined : orders;
+ if (!isArray(orders)) {
+ orders = orders == null ? [] : [orders];
+ }
+ return baseOrderBy(collection, iteratees, orders);
+ }
+
+ /**
+ * Creates an array of elements split into two groups, the first of which
+ * contains elements `predicate` returns truthy for, the second of which
+ * contains elements `predicate` returns falsey for. The predicate is
+ * invoked with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the array of grouped elements.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': false },
+ * { 'user': 'fred', 'age': 40, 'active': true },
+ * { 'user': 'pebbles', 'age': 1, 'active': false }
+ * ];
+ *
+ * _.partition(users, function(o) { return o.active; });
+ * // => objects for [['fred'], ['barney', 'pebbles']]
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.partition(users, { 'age': 1, 'active': false });
+ * // => objects for [['pebbles'], ['barney', 'fred']]
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.partition(users, ['active', false]);
+ * // => objects for [['barney', 'pebbles'], ['fred']]
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.partition(users, 'active');
+ * // => objects for [['fred'], ['barney', 'pebbles']]
+ */
+ var partition = createAggregator(function(result, value, key) {
+ result[key ? 0 : 1].push(value);
+ }, function() { return [[], []]; });
+
+ /**
+ * Reduces `collection` to a value which is the accumulated result of running
+ * each element in `collection` thru `iteratee`, where each successive
+ * invocation is supplied the return value of the previous. If `accumulator`
+ * is not given, the first element of `collection` is used as the initial
+ * value. The iteratee is invoked with four arguments:
+ * (accumulator, value, index|key, collection).
+ *
+ * Many lodash methods are guarded to work as iteratees for methods like
+ * `_.reduce`, `_.reduceRight`, and `_.transform`.
+ *
+ * The guarded methods are:
+ * `assign`, `defaults`, `defaultsDeep`, `includes`, `merge`, `orderBy`,
+ * and `sortBy`
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @returns {*} Returns the accumulated value.
+ * @see _.reduceRight
+ * @example
+ *
+ * _.reduce([1, 2], function(sum, n) {
+ * return sum + n;
+ * }, 0);
+ * // => 3
+ *
+ * _.reduce({ 'a': 1, 'b': 2, 'c': 1 }, function(result, value, key) {
+ * (result[value] || (result[value] = [])).push(key);
+ * return result;
+ * }, {});
+ * // => { '1': ['a', 'c'], '2': ['b'] } (iteration order is not guaranteed)
+ */
+ function reduce(collection, iteratee, accumulator) {
+ var func = isArray(collection) ? arrayReduce : baseReduce,
+ initAccum = arguments.length < 3;
+
+ return func(collection, getIteratee(iteratee, 4), accumulator, initAccum, baseEach);
+ }
+
+ /**
+ * This method is like `_.reduce` except that it iterates over elements of
+ * `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @returns {*} Returns the accumulated value.
+ * @see _.reduce
+ * @example
+ *
+ * var array = [[0, 1], [2, 3], [4, 5]];
+ *
+ * _.reduceRight(array, function(flattened, other) {
+ * return flattened.concat(other);
+ * }, []);
+ * // => [4, 5, 2, 3, 0, 1]
+ */
+ function reduceRight(collection, iteratee, accumulator) {
+ var func = isArray(collection) ? arrayReduceRight : baseReduce,
+ initAccum = arguments.length < 3;
+
+ return func(collection, getIteratee(iteratee, 4), accumulator, initAccum, baseEachRight);
+ }
+
+ /**
+ * The opposite of `_.filter`; this method returns the elements of `collection`
+ * that `predicate` does **not** return truthy for.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ * @see _.filter
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': false },
+ * { 'user': 'fred', 'age': 40, 'active': true }
+ * ];
+ *
+ * _.reject(users, function(o) { return !o.active; });
+ * // => objects for ['fred']
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.reject(users, { 'age': 40, 'active': true });
+ * // => objects for ['barney']
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.reject(users, ['active', false]);
+ * // => objects for ['fred']
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.reject(users, 'active');
+ * // => objects for ['barney']
+ */
+ function reject(collection, predicate) {
+ var func = isArray(collection) ? arrayFilter : baseFilter;
+ return func(collection, negate(getIteratee(predicate, 3)));
+ }
+
+ /**
+ * Gets a random element from `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to sample.
+ * @returns {*} Returns the random element.
+ * @example
+ *
+ * _.sample([1, 2, 3, 4]);
+ * // => 2
+ */
+ function sample(collection) {
+ var func = isArray(collection) ? arraySample : baseSample;
+ return func(collection);
+ }
+
+ /**
+ * Gets `n` random elements at unique keys from `collection` up to the
+ * size of `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to sample.
+ * @param {number} [n=1] The number of elements to sample.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Array} Returns the random elements.
+ * @example
+ *
+ * _.sampleSize([1, 2, 3], 2);
+ * // => [3, 1]
+ *
+ * _.sampleSize([1, 2, 3], 4);
+ * // => [2, 3, 1]
+ */
+ function sampleSize(collection, n, guard) {
+ if ((guard ? isIterateeCall(collection, n, guard) : n === undefined)) {
+ n = 1;
+ } else {
+ n = toInteger(n);
+ }
+ var func = isArray(collection) ? arraySampleSize : baseSampleSize;
+ return func(collection, n);
+ }
+
+ /**
+ * Creates an array of shuffled values, using a version of the
+ * [Fisher-Yates shuffle](https://en.wikipedia.org/wiki/Fisher-Yates_shuffle).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to shuffle.
+ * @returns {Array} Returns the new shuffled array.
+ * @example
+ *
+ * _.shuffle([1, 2, 3, 4]);
+ * // => [4, 1, 3, 2]
+ */
+ function shuffle(collection) {
+ var func = isArray(collection) ? arrayShuffle : baseShuffle;
+ return func(collection);
+ }
+
+ /**
+ * Gets the size of `collection` by returning its length for array-like
+ * values or the number of own enumerable string keyed properties for objects.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to inspect.
+ * @returns {number} Returns the collection size.
+ * @example
+ *
+ * _.size([1, 2, 3]);
+ * // => 3
+ *
+ * _.size({ 'a': 1, 'b': 2 });
+ * // => 2
+ *
+ * _.size('pebbles');
+ * // => 7
+ */
+ function size(collection) {
+ if (collection == null) {
+ return 0;
+ }
+ if (isArrayLike(collection)) {
+ return isString(collection) ? stringSize(collection) : collection.length;
+ }
+ var tag = getTag(collection);
+ if (tag == mapTag || tag == setTag) {
+ return collection.size;
+ }
+ return baseKeys(collection).length;
+ }
+
+ /**
+ * Checks if `predicate` returns truthy for **any** element of `collection`.
+ * Iteration is stopped once `predicate` returns truthy. The predicate is
+ * invoked with three arguments: (value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ * @example
+ *
+ * _.some([null, 0, 'yes', false], Boolean);
+ * // => true
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false }
+ * ];
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.some(users, { 'user': 'barney', 'active': false });
+ * // => false
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.some(users, ['active', false]);
+ * // => true
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.some(users, 'active');
+ * // => true
+ */
+ function some(collection, predicate, guard) {
+ var func = isArray(collection) ? arraySome : baseSome;
+ if (guard && isIterateeCall(collection, predicate, guard)) {
+ predicate = undefined;
+ }
+ return func(collection, getIteratee(predicate, 3));
+ }
+
+ /**
+ * Creates an array of elements, sorted in ascending order by the results of
+ * running each element in a collection thru each iteratee. This method
+ * performs a stable sort, that is, it preserves the original sort order of
+ * equal elements. The iteratees are invoked with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Collection
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {...(Function|Function[])} [iteratees=[_.identity]]
+ * The iteratees to sort by.
+ * @returns {Array} Returns the new sorted array.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'fred', 'age': 48 },
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 30 },
+ * { 'user': 'barney', 'age': 34 }
+ * ];
+ *
+ * _.sortBy(users, [function(o) { return o.user; }]);
+ * // => objects for [['barney', 36], ['barney', 34], ['fred', 48], ['fred', 30]]
+ *
+ * _.sortBy(users, ['user', 'age']);
+ * // => objects for [['barney', 34], ['barney', 36], ['fred', 30], ['fred', 48]]
+ */
+ var sortBy = baseRest(function(collection, iteratees) {
+ if (collection == null) {
+ return [];
+ }
+ var length = iteratees.length;
+ if (length > 1 && isIterateeCall(collection, iteratees[0], iteratees[1])) {
+ iteratees = [];
+ } else if (length > 2 && isIterateeCall(iteratees[0], iteratees[1], iteratees[2])) {
+ iteratees = [iteratees[0]];
+ }
+ return baseOrderBy(collection, baseFlatten(iteratees, 1), []);
+ });
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Gets the timestamp of the number of milliseconds that have elapsed since
+ * the Unix epoch (1 January 1970 00:00:00 UTC).
+ *
+ * @static
+ * @memberOf _
+ * @since 2.4.0
+ * @category Date
+ * @returns {number} Returns the timestamp.
+ * @example
+ *
+ * _.defer(function(stamp) {
+ * console.log(_.now() - stamp);
+ * }, _.now());
+ * // => Logs the number of milliseconds it took for the deferred invocation.
+ */
+ var now = ctxNow || function() {
+ return root.Date.now();
+ };
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * The opposite of `_.before`; this method creates a function that invokes
+ * `func` once it's called `n` or more times.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {number} n The number of calls before `func` is invoked.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var saves = ['profile', 'settings'];
+ *
+ * var done = _.after(saves.length, function() {
+ * console.log('done saving!');
+ * });
+ *
+ * _.forEach(saves, function(type) {
+ * asyncSave({ 'type': type, 'complete': done });
+ * });
+ * // => Logs 'done saving!' after the two async saves have completed.
+ */
+ function after(n, func) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ n = toInteger(n);
+ return function() {
+ if (--n < 1) {
+ return func.apply(this, arguments);
+ }
+ };
+ }
+
+ /**
+ * Creates a function that invokes `func`, with up to `n` arguments,
+ * ignoring any additional arguments.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Function
+ * @param {Function} func The function to cap arguments for.
+ * @param {number} [n=func.length] The arity cap.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Function} Returns the new capped function.
+ * @example
+ *
+ * _.map(['6', '8', '10'], _.ary(parseInt, 1));
+ * // => [6, 8, 10]
+ */
+ function ary(func, n, guard) {
+ n = guard ? undefined : n;
+ n = (func && n == null) ? func.length : n;
+ return createWrap(func, WRAP_ARY_FLAG, undefined, undefined, undefined, undefined, n);
+ }
+
+ /**
+ * Creates a function that invokes `func`, with the `this` binding and arguments
+ * of the created function, while it's called less than `n` times. Subsequent
+ * calls to the created function return the result of the last `func` invocation.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Function
+ * @param {number} n The number of calls at which `func` is no longer invoked.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * jQuery(element).on('click', _.before(5, addContactToList));
+ * // => Allows adding up to 4 contacts to the list.
+ */
+ function before(n, func) {
+ var result;
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ n = toInteger(n);
+ return function() {
+ if (--n > 0) {
+ result = func.apply(this, arguments);
+ }
+ if (n <= 1) {
+ func = undefined;
+ }
+ return result;
+ };
+ }
+
+ /**
+ * Creates a function that invokes `func` with the `this` binding of `thisArg`
+ * and `partials` prepended to the arguments it receives.
+ *
+ * The `_.bind.placeholder` value, which defaults to `_` in monolithic builds,
+ * may be used as a placeholder for partially applied arguments.
+ *
+ * **Note:** Unlike native `Function#bind`, this method doesn't set the "length"
+ * property of bound functions.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to bind.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * function greet(greeting, punctuation) {
+ * return greeting + ' ' + this.user + punctuation;
+ * }
+ *
+ * var object = { 'user': 'fred' };
+ *
+ * var bound = _.bind(greet, object, 'hi');
+ * bound('!');
+ * // => 'hi fred!'
+ *
+ * // Bound with placeholders.
+ * var bound = _.bind(greet, object, _, '!');
+ * bound('hi');
+ * // => 'hi fred!'
+ */
+ var bind = baseRest(function(func, thisArg, partials) {
+ var bitmask = WRAP_BIND_FLAG;
+ if (partials.length) {
+ var holders = replaceHolders(partials, getHolder(bind));
+ bitmask |= WRAP_PARTIAL_FLAG;
+ }
+ return createWrap(func, bitmask, thisArg, partials, holders);
+ });
+
+ /**
+ * Creates a function that invokes the method at `object[key]` with `partials`
+ * prepended to the arguments it receives.
+ *
+ * This method differs from `_.bind` by allowing bound functions to reference
+ * methods that may be redefined or don't yet exist. See
+ * [Peter Michaux's article](http://peter.michaux.ca/articles/lazy-function-definition-pattern)
+ * for more details.
+ *
+ * The `_.bindKey.placeholder` value, which defaults to `_` in monolithic
+ * builds, may be used as a placeholder for partially applied arguments.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.10.0
+ * @category Function
+ * @param {Object} object The object to invoke the method on.
+ * @param {string} key The key of the method.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var object = {
+ * 'user': 'fred',
+ * 'greet': function(greeting, punctuation) {
+ * return greeting + ' ' + this.user + punctuation;
+ * }
+ * };
+ *
+ * var bound = _.bindKey(object, 'greet', 'hi');
+ * bound('!');
+ * // => 'hi fred!'
+ *
+ * object.greet = function(greeting, punctuation) {
+ * return greeting + 'ya ' + this.user + punctuation;
+ * };
+ *
+ * bound('!');
+ * // => 'hiya fred!'
+ *
+ * // Bound with placeholders.
+ * var bound = _.bindKey(object, 'greet', _, '!');
+ * bound('hi');
+ * // => 'hiya fred!'
+ */
+ var bindKey = baseRest(function(object, key, partials) {
+ var bitmask = WRAP_BIND_FLAG | WRAP_BIND_KEY_FLAG;
+ if (partials.length) {
+ var holders = replaceHolders(partials, getHolder(bindKey));
+ bitmask |= WRAP_PARTIAL_FLAG;
+ }
+ return createWrap(key, bitmask, object, partials, holders);
+ });
+
+ /**
+ * Creates a function that accepts arguments of `func` and either invokes
+ * `func` returning its result, if at least `arity` number of arguments have
+ * been provided, or returns a function that accepts the remaining `func`
+ * arguments, and so on. The arity of `func` may be specified if `func.length`
+ * is not sufficient.
+ *
+ * The `_.curry.placeholder` value, which defaults to `_` in monolithic builds,
+ * may be used as a placeholder for provided arguments.
+ *
+ * **Note:** This method doesn't set the "length" property of curried functions.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Function
+ * @param {Function} func The function to curry.
+ * @param {number} [arity=func.length] The arity of `func`.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Function} Returns the new curried function.
+ * @example
+ *
+ * var abc = function(a, b, c) {
+ * return [a, b, c];
+ * };
+ *
+ * var curried = _.curry(abc);
+ *
+ * curried(1)(2)(3);
+ * // => [1, 2, 3]
+ *
+ * curried(1, 2)(3);
+ * // => [1, 2, 3]
+ *
+ * curried(1, 2, 3);
+ * // => [1, 2, 3]
+ *
+ * // Curried with placeholders.
+ * curried(1)(_, 3)(2);
+ * // => [1, 2, 3]
+ */
+ function curry(func, arity, guard) {
+ arity = guard ? undefined : arity;
+ var result = createWrap(func, WRAP_CURRY_FLAG, undefined, undefined, undefined, undefined, undefined, arity);
+ result.placeholder = curry.placeholder;
+ return result;
+ }
+
+ /**
+ * This method is like `_.curry` except that arguments are applied to `func`
+ * in the manner of `_.partialRight` instead of `_.partial`.
+ *
+ * The `_.curryRight.placeholder` value, which defaults to `_` in monolithic
+ * builds, may be used as a placeholder for provided arguments.
+ *
+ * **Note:** This method doesn't set the "length" property of curried functions.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Function
+ * @param {Function} func The function to curry.
+ * @param {number} [arity=func.length] The arity of `func`.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Function} Returns the new curried function.
+ * @example
+ *
+ * var abc = function(a, b, c) {
+ * return [a, b, c];
+ * };
+ *
+ * var curried = _.curryRight(abc);
+ *
+ * curried(3)(2)(1);
+ * // => [1, 2, 3]
+ *
+ * curried(2, 3)(1);
+ * // => [1, 2, 3]
+ *
+ * curried(1, 2, 3);
+ * // => [1, 2, 3]
+ *
+ * // Curried with placeholders.
+ * curried(3)(1, _)(2);
+ * // => [1, 2, 3]
+ */
+ function curryRight(func, arity, guard) {
+ arity = guard ? undefined : arity;
+ var result = createWrap(func, WRAP_CURRY_RIGHT_FLAG, undefined, undefined, undefined, undefined, undefined, arity);
+ result.placeholder = curryRight.placeholder;
+ return result;
+ }
+
+ /**
+ * Creates a debounced function that delays invoking `func` until after `wait`
+ * milliseconds have elapsed since the last time the debounced function was
+ * invoked. The debounced function comes with a `cancel` method to cancel
+ * delayed `func` invocations and a `flush` method to immediately invoke them.
+ * Provide `options` to indicate whether `func` should be invoked on the
+ * leading and/or trailing edge of the `wait` timeout. The `func` is invoked
+ * with the last arguments provided to the debounced function. Subsequent
+ * calls to the debounced function return the result of the last `func`
+ * invocation.
+ *
+ * **Note:** If `leading` and `trailing` options are `true`, `func` is
+ * invoked on the trailing edge of the timeout only if the debounced function
+ * is invoked more than once during the `wait` timeout.
+ *
+ * If `wait` is `0` and `leading` is `false`, `func` invocation is deferred
+ * until to the next tick, similar to `setTimeout` with a timeout of `0`.
+ *
+ * See [David Corbacho's article](https://css-tricks.com/debouncing-throttling-explained-examples/)
+ * for details over the differences between `_.debounce` and `_.throttle`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to debounce.
+ * @param {number} [wait=0] The number of milliseconds to delay.
+ * @param {Object} [options={}] The options object.
+ * @param {boolean} [options.leading=false]
+ * Specify invoking on the leading edge of the timeout.
+ * @param {number} [options.maxWait]
+ * The maximum time `func` is allowed to be delayed before it's invoked.
+ * @param {boolean} [options.trailing=true]
+ * Specify invoking on the trailing edge of the timeout.
+ * @returns {Function} Returns the new debounced function.
+ * @example
+ *
+ * // Avoid costly calculations while the window size is in flux.
+ * jQuery(window).on('resize', _.debounce(calculateLayout, 150));
+ *
+ * // Invoke `sendMail` when clicked, debouncing subsequent calls.
+ * jQuery(element).on('click', _.debounce(sendMail, 300, {
+ * 'leading': true,
+ * 'trailing': false
+ * }));
+ *
+ * // Ensure `batchLog` is invoked once after 1 second of debounced calls.
+ * var debounced = _.debounce(batchLog, 250, { 'maxWait': 1000 });
+ * var source = new EventSource('/stream');
+ * jQuery(source).on('message', debounced);
+ *
+ * // Cancel the trailing debounced invocation.
+ * jQuery(window).on('popstate', debounced.cancel);
+ */
+ function debounce(func, wait, options) {
+ var lastArgs,
+ lastThis,
+ maxWait,
+ result,
+ timerId,
+ lastCallTime,
+ lastInvokeTime = 0,
+ leading = false,
+ maxing = false,
+ trailing = true;
+
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ wait = toNumber(wait) || 0;
+ if (isObject(options)) {
+ leading = !!options.leading;
+ maxing = 'maxWait' in options;
+ maxWait = maxing ? nativeMax(toNumber(options.maxWait) || 0, wait) : maxWait;
+ trailing = 'trailing' in options ? !!options.trailing : trailing;
+ }
+
+ function invokeFunc(time) {
+ var args = lastArgs,
+ thisArg = lastThis;
+
+ lastArgs = lastThis = undefined;
+ lastInvokeTime = time;
+ result = func.apply(thisArg, args);
+ return result;
+ }
+
+ function leadingEdge(time) {
+ // Reset any `maxWait` timer.
+ lastInvokeTime = time;
+ // Start the timer for the trailing edge.
+ timerId = setTimeout(timerExpired, wait);
+ // Invoke the leading edge.
+ return leading ? invokeFunc(time) : result;
+ }
+
+ function remainingWait(time) {
+ var timeSinceLastCall = time - lastCallTime,
+ timeSinceLastInvoke = time - lastInvokeTime,
+ timeWaiting = wait - timeSinceLastCall;
+
+ return maxing
+ ? nativeMin(timeWaiting, maxWait - timeSinceLastInvoke)
+ : timeWaiting;
+ }
+
+ function shouldInvoke(time) {
+ var timeSinceLastCall = time - lastCallTime,
+ timeSinceLastInvoke = time - lastInvokeTime;
+
+ // Either this is the first call, activity has stopped and we're at the
+ // trailing edge, the system time has gone backwards and we're treating
+ // it as the trailing edge, or we've hit the `maxWait` limit.
+ return (lastCallTime === undefined || (timeSinceLastCall >= wait) ||
+ (timeSinceLastCall < 0) || (maxing && timeSinceLastInvoke >= maxWait));
+ }
+
+ function timerExpired() {
+ var time = now();
+ if (shouldInvoke(time)) {
+ return trailingEdge(time);
+ }
+ // Restart the timer.
+ timerId = setTimeout(timerExpired, remainingWait(time));
+ }
+
+ function trailingEdge(time) {
+ timerId = undefined;
+
+ // Only invoke if we have `lastArgs` which means `func` has been
+ // debounced at least once.
+ if (trailing && lastArgs) {
+ return invokeFunc(time);
+ }
+ lastArgs = lastThis = undefined;
+ return result;
+ }
+
+ function cancel() {
+ if (timerId !== undefined) {
+ clearTimeout(timerId);
+ }
+ lastInvokeTime = 0;
+ lastArgs = lastCallTime = lastThis = timerId = undefined;
+ }
+
+ function flush() {
+ return timerId === undefined ? result : trailingEdge(now());
+ }
+
+ function debounced() {
+ var time = now(),
+ isInvoking = shouldInvoke(time);
+
+ lastArgs = arguments;
+ lastThis = this;
+ lastCallTime = time;
+
+ if (isInvoking) {
+ if (timerId === undefined) {
+ return leadingEdge(lastCallTime);
+ }
+ if (maxing) {
+ // Handle invocations in a tight loop.
+ clearTimeout(timerId);
+ timerId = setTimeout(timerExpired, wait);
+ return invokeFunc(lastCallTime);
+ }
+ }
+ if (timerId === undefined) {
+ timerId = setTimeout(timerExpired, wait);
+ }
+ return result;
+ }
+ debounced.cancel = cancel;
+ debounced.flush = flush;
+ return debounced;
+ }
+
+ /**
+ * Defers invoking the `func` until the current call stack has cleared. Any
+ * additional arguments are provided to `func` when it's invoked.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to defer.
+ * @param {...*} [args] The arguments to invoke `func` with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.defer(function(text) {
+ * console.log(text);
+ * }, 'deferred');
+ * // => Logs 'deferred' after one millisecond.
+ */
+ var defer = baseRest(function(func, args) {
+ return baseDelay(func, 1, args);
+ });
+
+ /**
+ * Invokes `func` after `wait` milliseconds. Any additional arguments are
+ * provided to `func` when it's invoked.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay invocation.
+ * @param {...*} [args] The arguments to invoke `func` with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.delay(function(text) {
+ * console.log(text);
+ * }, 1000, 'later');
+ * // => Logs 'later' after one second.
+ */
+ var delay = baseRest(function(func, wait, args) {
+ return baseDelay(func, toNumber(wait) || 0, args);
+ });
+
+ /**
+ * Creates a function that invokes `func` with arguments reversed.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Function
+ * @param {Function} func The function to flip arguments for.
+ * @returns {Function} Returns the new flipped function.
+ * @example
+ *
+ * var flipped = _.flip(function() {
+ * return _.toArray(arguments);
+ * });
+ *
+ * flipped('a', 'b', 'c', 'd');
+ * // => ['d', 'c', 'b', 'a']
+ */
+ function flip(func) {
+ return createWrap(func, WRAP_FLIP_FLAG);
+ }
+
+ /**
+ * Creates a function that memoizes the result of `func`. If `resolver` is
+ * provided, it determines the cache key for storing the result based on the
+ * arguments provided to the memoized function. By default, the first argument
+ * provided to the memoized function is used as the map cache key. The `func`
+ * is invoked with the `this` binding of the memoized function.
+ *
+ * **Note:** The cache is exposed as the `cache` property on the memoized
+ * function. Its creation may be customized by replacing the `_.memoize.Cache`
+ * constructor with one whose instances implement the
+ * [`Map`](http://ecma-international.org/ecma-262/7.0/#sec-properties-of-the-map-prototype-object)
+ * method interface of `clear`, `delete`, `get`, `has`, and `set`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to have its output memoized.
+ * @param {Function} [resolver] The function to resolve the cache key.
+ * @returns {Function} Returns the new memoized function.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': 2 };
+ * var other = { 'c': 3, 'd': 4 };
+ *
+ * var values = _.memoize(_.values);
+ * values(object);
+ * // => [1, 2]
+ *
+ * values(other);
+ * // => [3, 4]
+ *
+ * object.a = 2;
+ * values(object);
+ * // => [1, 2]
+ *
+ * // Modify the result cache.
+ * values.cache.set(object, ['a', 'b']);
+ * values(object);
+ * // => ['a', 'b']
+ *
+ * // Replace `_.memoize.Cache`.
+ * _.memoize.Cache = WeakMap;
+ */
+ function memoize(func, resolver) {
+ if (typeof func != 'function' || (resolver != null && typeof resolver != 'function')) {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ var memoized = function() {
+ var args = arguments,
+ key = resolver ? resolver.apply(this, args) : args[0],
+ cache = memoized.cache;
+
+ if (cache.has(key)) {
+ return cache.get(key);
+ }
+ var result = func.apply(this, args);
+ memoized.cache = cache.set(key, result) || cache;
+ return result;
+ };
+ memoized.cache = new (memoize.Cache || MapCache);
+ return memoized;
+ }
+
+ // Expose `MapCache`.
+ memoize.Cache = MapCache;
+
+ /**
+ * Creates a function that negates the result of the predicate `func`. The
+ * `func` predicate is invoked with the `this` binding and arguments of the
+ * created function.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Function
+ * @param {Function} predicate The predicate to negate.
+ * @returns {Function} Returns the new negated function.
+ * @example
+ *
+ * function isEven(n) {
+ * return n % 2 == 0;
+ * }
+ *
+ * _.filter([1, 2, 3, 4, 5, 6], _.negate(isEven));
+ * // => [1, 3, 5]
+ */
+ function negate(predicate) {
+ if (typeof predicate != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ return function() {
+ var args = arguments;
+ switch (args.length) {
+ case 0: return !predicate.call(this);
+ case 1: return !predicate.call(this, args[0]);
+ case 2: return !predicate.call(this, args[0], args[1]);
+ case 3: return !predicate.call(this, args[0], args[1], args[2]);
+ }
+ return !predicate.apply(this, args);
+ };
+ }
+
+ /**
+ * Creates a function that is restricted to invoking `func` once. Repeat calls
+ * to the function return the value of the first invocation. The `func` is
+ * invoked with the `this` binding and arguments of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var initialize = _.once(createApplication);
+ * initialize();
+ * initialize();
+ * // => `createApplication` is invoked once
+ */
+ function once(func) {
+ return before(2, func);
+ }
+
+ /**
+ * Creates a function that invokes `func` with its arguments transformed.
+ *
+ * @static
+ * @since 4.0.0
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to wrap.
+ * @param {...(Function|Function[])} [transforms=[_.identity]]
+ * The argument transforms.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * function doubled(n) {
+ * return n * 2;
+ * }
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * var func = _.overArgs(function(x, y) {
+ * return [x, y];
+ * }, [square, doubled]);
+ *
+ * func(9, 3);
+ * // => [81, 6]
+ *
+ * func(10, 5);
+ * // => [100, 10]
+ */
+ var overArgs = castRest(function(func, transforms) {
+ transforms = (transforms.length == 1 && isArray(transforms[0]))
+ ? arrayMap(transforms[0], baseUnary(getIteratee()))
+ : arrayMap(baseFlatten(transforms, 1), baseUnary(getIteratee()));
+
+ var funcsLength = transforms.length;
+ return baseRest(function(args) {
+ var index = -1,
+ length = nativeMin(args.length, funcsLength);
+
+ while (++index < length) {
+ args[index] = transforms[index].call(this, args[index]);
+ }
+ return apply(func, this, args);
+ });
+ });
+
+ /**
+ * Creates a function that invokes `func` with `partials` prepended to the
+ * arguments it receives. This method is like `_.bind` except it does **not**
+ * alter the `this` binding.
+ *
+ * The `_.partial.placeholder` value, which defaults to `_` in monolithic
+ * builds, may be used as a placeholder for partially applied arguments.
+ *
+ * **Note:** This method doesn't set the "length" property of partially
+ * applied functions.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.2.0
+ * @category Function
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * function greet(greeting, name) {
+ * return greeting + ' ' + name;
+ * }
+ *
+ * var sayHelloTo = _.partial(greet, 'hello');
+ * sayHelloTo('fred');
+ * // => 'hello fred'
+ *
+ * // Partially applied with placeholders.
+ * var greetFred = _.partial(greet, _, 'fred');
+ * greetFred('hi');
+ * // => 'hi fred'
+ */
+ var partial = baseRest(function(func, partials) {
+ var holders = replaceHolders(partials, getHolder(partial));
+ return createWrap(func, WRAP_PARTIAL_FLAG, undefined, partials, holders);
+ });
+
+ /**
+ * This method is like `_.partial` except that partially applied arguments
+ * are appended to the arguments it receives.
+ *
+ * The `_.partialRight.placeholder` value, which defaults to `_` in monolithic
+ * builds, may be used as a placeholder for partially applied arguments.
+ *
+ * **Note:** This method doesn't set the "length" property of partially
+ * applied functions.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.0.0
+ * @category Function
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * function greet(greeting, name) {
+ * return greeting + ' ' + name;
+ * }
+ *
+ * var greetFred = _.partialRight(greet, 'fred');
+ * greetFred('hi');
+ * // => 'hi fred'
+ *
+ * // Partially applied with placeholders.
+ * var sayHelloTo = _.partialRight(greet, 'hello', _);
+ * sayHelloTo('fred');
+ * // => 'hello fred'
+ */
+ var partialRight = baseRest(function(func, partials) {
+ var holders = replaceHolders(partials, getHolder(partialRight));
+ return createWrap(func, WRAP_PARTIAL_RIGHT_FLAG, undefined, partials, holders);
+ });
+
+ /**
+ * Creates a function that invokes `func` with arguments arranged according
+ * to the specified `indexes` where the argument value at the first index is
+ * provided as the first argument, the argument value at the second index is
+ * provided as the second argument, and so on.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Function
+ * @param {Function} func The function to rearrange arguments for.
+ * @param {...(number|number[])} indexes The arranged argument indexes.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var rearged = _.rearg(function(a, b, c) {
+ * return [a, b, c];
+ * }, [2, 0, 1]);
+ *
+ * rearged('b', 'c', 'a')
+ * // => ['a', 'b', 'c']
+ */
+ var rearg = flatRest(function(func, indexes) {
+ return createWrap(func, WRAP_REARG_FLAG, undefined, undefined, undefined, indexes);
+ });
+
+ /**
+ * Creates a function that invokes `func` with the `this` binding of the
+ * created function and arguments from `start` and beyond provided as
+ * an array.
+ *
+ * **Note:** This method is based on the
+ * [rest parameter](https://mdn.io/rest_parameters).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Function
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var say = _.rest(function(what, names) {
+ * return what + ' ' + _.initial(names).join(', ') +
+ * (_.size(names) > 1 ? ', & ' : '') + _.last(names);
+ * });
+ *
+ * say('hello', 'fred', 'barney', 'pebbles');
+ * // => 'hello fred, barney, & pebbles'
+ */
+ function rest(func, start) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ start = start === undefined ? start : toInteger(start);
+ return baseRest(func, start);
+ }
+
+ /**
+ * Creates a function that invokes `func` with the `this` binding of the
+ * create function and an array of arguments much like
+ * [`Function#apply`](http://www.ecma-international.org/ecma-262/7.0/#sec-function.prototype.apply).
+ *
+ * **Note:** This method is based on the
+ * [spread operator](https://mdn.io/spread_operator).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.2.0
+ * @category Function
+ * @param {Function} func The function to spread arguments over.
+ * @param {number} [start=0] The start position of the spread.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var say = _.spread(function(who, what) {
+ * return who + ' says ' + what;
+ * });
+ *
+ * say(['fred', 'hello']);
+ * // => 'fred says hello'
+ *
+ * var numbers = Promise.all([
+ * Promise.resolve(40),
+ * Promise.resolve(36)
+ * ]);
+ *
+ * numbers.then(_.spread(function(x, y) {
+ * return x + y;
+ * }));
+ * // => a Promise of 76
+ */
+ function spread(func, start) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ start = start == null ? 0 : nativeMax(toInteger(start), 0);
+ return baseRest(function(args) {
+ var array = args[start],
+ otherArgs = castSlice(args, 0, start);
+
+ if (array) {
+ arrayPush(otherArgs, array);
+ }
+ return apply(func, this, otherArgs);
+ });
+ }
+
+ /**
+ * Creates a throttled function that only invokes `func` at most once per
+ * every `wait` milliseconds. The throttled function comes with a `cancel`
+ * method to cancel delayed `func` invocations and a `flush` method to
+ * immediately invoke them. Provide `options` to indicate whether `func`
+ * should be invoked on the leading and/or trailing edge of the `wait`
+ * timeout. The `func` is invoked with the last arguments provided to the
+ * throttled function. Subsequent calls to the throttled function return the
+ * result of the last `func` invocation.
+ *
+ * **Note:** If `leading` and `trailing` options are `true`, `func` is
+ * invoked on the trailing edge of the timeout only if the throttled function
+ * is invoked more than once during the `wait` timeout.
+ *
+ * If `wait` is `0` and `leading` is `false`, `func` invocation is deferred
+ * until to the next tick, similar to `setTimeout` with a timeout of `0`.
+ *
+ * See [David Corbacho's article](https://css-tricks.com/debouncing-throttling-explained-examples/)
+ * for details over the differences between `_.throttle` and `_.debounce`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {Function} func The function to throttle.
+ * @param {number} [wait=0] The number of milliseconds to throttle invocations to.
+ * @param {Object} [options={}] The options object.
+ * @param {boolean} [options.leading=true]
+ * Specify invoking on the leading edge of the timeout.
+ * @param {boolean} [options.trailing=true]
+ * Specify invoking on the trailing edge of the timeout.
+ * @returns {Function} Returns the new throttled function.
+ * @example
+ *
+ * // Avoid excessively updating the position while scrolling.
+ * jQuery(window).on('scroll', _.throttle(updatePosition, 100));
+ *
+ * // Invoke `renewToken` when the click event is fired, but not more than once every 5 minutes.
+ * var throttled = _.throttle(renewToken, 300000, { 'trailing': false });
+ * jQuery(element).on('click', throttled);
+ *
+ * // Cancel the trailing throttled invocation.
+ * jQuery(window).on('popstate', throttled.cancel);
+ */
+ function throttle(func, wait, options) {
+ var leading = true,
+ trailing = true;
+
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ if (isObject(options)) {
+ leading = 'leading' in options ? !!options.leading : leading;
+ trailing = 'trailing' in options ? !!options.trailing : trailing;
+ }
+ return debounce(func, wait, {
+ 'leading': leading,
+ 'maxWait': wait,
+ 'trailing': trailing
+ });
+ }
+
+ /**
+ * Creates a function that accepts up to one argument, ignoring any
+ * additional arguments.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Function
+ * @param {Function} func The function to cap arguments for.
+ * @returns {Function} Returns the new capped function.
+ * @example
+ *
+ * _.map(['6', '8', '10'], _.unary(parseInt));
+ * // => [6, 8, 10]
+ */
+ function unary(func) {
+ return ary(func, 1);
+ }
+
+ /**
+ * Creates a function that provides `value` to `wrapper` as its first
+ * argument. Any additional arguments provided to the function are appended
+ * to those provided to the `wrapper`. The wrapper is invoked with the `this`
+ * binding of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Function
+ * @param {*} value The value to wrap.
+ * @param {Function} [wrapper=identity] The wrapper function.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var p = _.wrap(_.escape, function(func, text) {
+ * return '' + func(text) + '
';
+ * });
+ *
+ * p('fred, barney, & pebbles');
+ * // => 'fred, barney, & pebbles
'
+ */
+ function wrap(value, wrapper) {
+ return partial(castFunction(wrapper), value);
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Casts `value` as an array if it's not one.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.4.0
+ * @category Lang
+ * @param {*} value The value to inspect.
+ * @returns {Array} Returns the cast array.
+ * @example
+ *
+ * _.castArray(1);
+ * // => [1]
+ *
+ * _.castArray({ 'a': 1 });
+ * // => [{ 'a': 1 }]
+ *
+ * _.castArray('abc');
+ * // => ['abc']
+ *
+ * _.castArray(null);
+ * // => [null]
+ *
+ * _.castArray(undefined);
+ * // => [undefined]
+ *
+ * _.castArray();
+ * // => []
+ *
+ * var array = [1, 2, 3];
+ * console.log(_.castArray(array) === array);
+ * // => true
+ */
+ function castArray() {
+ if (!arguments.length) {
+ return [];
+ }
+ var value = arguments[0];
+ return isArray(value) ? value : [value];
+ }
+
+ /**
+ * Creates a shallow clone of `value`.
+ *
+ * **Note:** This method is loosely based on the
+ * [structured clone algorithm](https://mdn.io/Structured_clone_algorithm)
+ * and supports cloning arrays, array buffers, booleans, date objects, maps,
+ * numbers, `Object` objects, regexes, sets, strings, symbols, and typed
+ * arrays. The own enumerable properties of `arguments` objects are cloned
+ * as plain objects. An empty object is returned for uncloneable values such
+ * as error objects, functions, DOM nodes, and WeakMaps.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to clone.
+ * @returns {*} Returns the cloned value.
+ * @see _.cloneDeep
+ * @example
+ *
+ * var objects = [{ 'a': 1 }, { 'b': 2 }];
+ *
+ * var shallow = _.clone(objects);
+ * console.log(shallow[0] === objects[0]);
+ * // => true
+ */
+ function clone(value) {
+ return baseClone(value, CLONE_SYMBOLS_FLAG);
+ }
+
+ /**
+ * This method is like `_.clone` except that it accepts `customizer` which
+ * is invoked to produce the cloned value. If `customizer` returns `undefined`,
+ * cloning is handled by the method instead. The `customizer` is invoked with
+ * up to four arguments; (value [, index|key, object, stack]).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to clone.
+ * @param {Function} [customizer] The function to customize cloning.
+ * @returns {*} Returns the cloned value.
+ * @see _.cloneDeepWith
+ * @example
+ *
+ * function customizer(value) {
+ * if (_.isElement(value)) {
+ * return value.cloneNode(false);
+ * }
+ * }
+ *
+ * var el = _.cloneWith(document.body, customizer);
+ *
+ * console.log(el === document.body);
+ * // => false
+ * console.log(el.nodeName);
+ * // => 'BODY'
+ * console.log(el.childNodes.length);
+ * // => 0
+ */
+ function cloneWith(value, customizer) {
+ customizer = typeof customizer == 'function' ? customizer : undefined;
+ return baseClone(value, CLONE_SYMBOLS_FLAG, customizer);
+ }
+
+ /**
+ * This method is like `_.clone` except that it recursively clones `value`.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.0.0
+ * @category Lang
+ * @param {*} value The value to recursively clone.
+ * @returns {*} Returns the deep cloned value.
+ * @see _.clone
+ * @example
+ *
+ * var objects = [{ 'a': 1 }, { 'b': 2 }];
+ *
+ * var deep = _.cloneDeep(objects);
+ * console.log(deep[0] === objects[0]);
+ * // => false
+ */
+ function cloneDeep(value) {
+ return baseClone(value, CLONE_DEEP_FLAG | CLONE_SYMBOLS_FLAG);
+ }
+
+ /**
+ * This method is like `_.cloneWith` except that it recursively clones `value`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to recursively clone.
+ * @param {Function} [customizer] The function to customize cloning.
+ * @returns {*} Returns the deep cloned value.
+ * @see _.cloneWith
+ * @example
+ *
+ * function customizer(value) {
+ * if (_.isElement(value)) {
+ * return value.cloneNode(true);
+ * }
+ * }
+ *
+ * var el = _.cloneDeepWith(document.body, customizer);
+ *
+ * console.log(el === document.body);
+ * // => false
+ * console.log(el.nodeName);
+ * // => 'BODY'
+ * console.log(el.childNodes.length);
+ * // => 20
+ */
+ function cloneDeepWith(value, customizer) {
+ customizer = typeof customizer == 'function' ? customizer : undefined;
+ return baseClone(value, CLONE_DEEP_FLAG | CLONE_SYMBOLS_FLAG, customizer);
+ }
+
+ /**
+ * Checks if `object` conforms to `source` by invoking the predicate
+ * properties of `source` with the corresponding property values of `object`.
+ *
+ * **Note:** This method is equivalent to `_.conforms` when `source` is
+ * partially applied.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.14.0
+ * @category Lang
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property predicates to conform to.
+ * @returns {boolean} Returns `true` if `object` conforms, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': 2 };
+ *
+ * _.conformsTo(object, { 'b': function(n) { return n > 1; } });
+ * // => true
+ *
+ * _.conformsTo(object, { 'b': function(n) { return n > 2; } });
+ * // => false
+ */
+ function conformsTo(object, source) {
+ return source == null || baseConformsTo(object, source, keys(source));
+ }
+
+ /**
+ * Performs a
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * comparison between two values to determine if they are equivalent.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ * var other = { 'a': 1 };
+ *
+ * _.eq(object, object);
+ * // => true
+ *
+ * _.eq(object, other);
+ * // => false
+ *
+ * _.eq('a', 'a');
+ * // => true
+ *
+ * _.eq('a', Object('a'));
+ * // => false
+ *
+ * _.eq(NaN, NaN);
+ * // => true
+ */
+ function eq(value, other) {
+ return value === other || (value !== value && other !== other);
+ }
+
+ /**
+ * Checks if `value` is greater than `other`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.9.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is greater than `other`,
+ * else `false`.
+ * @see _.lt
+ * @example
+ *
+ * _.gt(3, 1);
+ * // => true
+ *
+ * _.gt(3, 3);
+ * // => false
+ *
+ * _.gt(1, 3);
+ * // => false
+ */
+ var gt = createRelationalOperation(baseGt);
+
+ /**
+ * Checks if `value` is greater than or equal to `other`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.9.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is greater than or equal to
+ * `other`, else `false`.
+ * @see _.lte
+ * @example
+ *
+ * _.gte(3, 1);
+ * // => true
+ *
+ * _.gte(3, 3);
+ * // => true
+ *
+ * _.gte(1, 3);
+ * // => false
+ */
+ var gte = createRelationalOperation(function(value, other) {
+ return value >= other;
+ });
+
+ /**
+ * Checks if `value` is likely an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ * else `false`.
+ * @example
+ *
+ * _.isArguments(function() { return arguments; }());
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+ var isArguments = baseIsArguments(function() { return arguments; }()) ? baseIsArguments : function(value) {
+ return isObjectLike(value) && hasOwnProperty.call(value, 'callee') &&
+ !propertyIsEnumerable.call(value, 'callee');
+ };
+
+ /**
+ * Checks if `value` is classified as an `Array` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array, else `false`.
+ * @example
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ *
+ * _.isArray(document.body.children);
+ * // => false
+ *
+ * _.isArray('abc');
+ * // => false
+ *
+ * _.isArray(_.noop);
+ * // => false
+ */
+ var isArray = Array.isArray;
+
+ /**
+ * Checks if `value` is classified as an `ArrayBuffer` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array buffer, else `false`.
+ * @example
+ *
+ * _.isArrayBuffer(new ArrayBuffer(2));
+ * // => true
+ *
+ * _.isArrayBuffer(new Array(2));
+ * // => false
+ */
+ var isArrayBuffer = nodeIsArrayBuffer ? baseUnary(nodeIsArrayBuffer) : baseIsArrayBuffer;
+
+ /**
+ * Checks if `value` is array-like. A value is considered array-like if it's
+ * not a function and has a `value.length` that's an integer greater than or
+ * equal to `0` and less than or equal to `Number.MAX_SAFE_INTEGER`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ * @example
+ *
+ * _.isArrayLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLike(document.body.children);
+ * // => true
+ *
+ * _.isArrayLike('abc');
+ * // => true
+ *
+ * _.isArrayLike(_.noop);
+ * // => false
+ */
+ function isArrayLike(value) {
+ return value != null && isLength(value.length) && !isFunction(value);
+ }
+
+ /**
+ * This method is like `_.isArrayLike` except that it also checks if `value`
+ * is an object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array-like object,
+ * else `false`.
+ * @example
+ *
+ * _.isArrayLikeObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLikeObject(document.body.children);
+ * // => true
+ *
+ * _.isArrayLikeObject('abc');
+ * // => false
+ *
+ * _.isArrayLikeObject(_.noop);
+ * // => false
+ */
+ function isArrayLikeObject(value) {
+ return isObjectLike(value) && isArrayLike(value);
+ }
+
+ /**
+ * Checks if `value` is classified as a boolean primitive or object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a boolean, else `false`.
+ * @example
+ *
+ * _.isBoolean(false);
+ * // => true
+ *
+ * _.isBoolean(null);
+ * // => false
+ */
+ function isBoolean(value) {
+ return value === true || value === false ||
+ (isObjectLike(value) && baseGetTag(value) == boolTag);
+ }
+
+ /**
+ * Checks if `value` is a buffer.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a buffer, else `false`.
+ * @example
+ *
+ * _.isBuffer(new Buffer(2));
+ * // => true
+ *
+ * _.isBuffer(new Uint8Array(2));
+ * // => false
+ */
+ var isBuffer = nativeIsBuffer || stubFalse;
+
+ /**
+ * Checks if `value` is classified as a `Date` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a date object, else `false`.
+ * @example
+ *
+ * _.isDate(new Date);
+ * // => true
+ *
+ * _.isDate('Mon April 23 2012');
+ * // => false
+ */
+ var isDate = nodeIsDate ? baseUnary(nodeIsDate) : baseIsDate;
+
+ /**
+ * Checks if `value` is likely a DOM element.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a DOM element, else `false`.
+ * @example
+ *
+ * _.isElement(document.body);
+ * // => true
+ *
+ * _.isElement('');
+ * // => false
+ */
+ function isElement(value) {
+ return isObjectLike(value) && value.nodeType === 1 && !isPlainObject(value);
+ }
+
+ /**
+ * Checks if `value` is an empty object, collection, map, or set.
+ *
+ * Objects are considered empty if they have no own enumerable string keyed
+ * properties.
+ *
+ * Array-like values such as `arguments` objects, arrays, buffers, strings, or
+ * jQuery-like collections are considered empty if they have a `length` of `0`.
+ * Similarly, maps and sets are considered empty if they have a `size` of `0`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is empty, else `false`.
+ * @example
+ *
+ * _.isEmpty(null);
+ * // => true
+ *
+ * _.isEmpty(true);
+ * // => true
+ *
+ * _.isEmpty(1);
+ * // => true
+ *
+ * _.isEmpty([1, 2, 3]);
+ * // => false
+ *
+ * _.isEmpty({ 'a': 1 });
+ * // => false
+ */
+ function isEmpty(value) {
+ if (value == null) {
+ return true;
+ }
+ if (isArrayLike(value) &&
+ (isArray(value) || typeof value == 'string' || typeof value.splice == 'function' ||
+ isBuffer(value) || isTypedArray(value) || isArguments(value))) {
+ return !value.length;
+ }
+ var tag = getTag(value);
+ if (tag == mapTag || tag == setTag) {
+ return !value.size;
+ }
+ if (isPrototype(value)) {
+ return !baseKeys(value).length;
+ }
+ for (var key in value) {
+ if (hasOwnProperty.call(value, key)) {
+ return false;
+ }
+ }
+ return true;
+ }
+
+ /**
+ * Performs a deep comparison between two values to determine if they are
+ * equivalent.
+ *
+ * **Note:** This method supports comparing arrays, array buffers, booleans,
+ * date objects, error objects, maps, numbers, `Object` objects, regexes,
+ * sets, strings, symbols, and typed arrays. `Object` objects are compared
+ * by their own, not inherited, enumerable properties. Functions and DOM
+ * nodes are compared by strict equality, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ * var other = { 'a': 1 };
+ *
+ * _.isEqual(object, other);
+ * // => true
+ *
+ * object === other;
+ * // => false
+ */
+ function isEqual(value, other) {
+ return baseIsEqual(value, other);
+ }
+
+ /**
+ * This method is like `_.isEqual` except that it accepts `customizer` which
+ * is invoked to compare values. If `customizer` returns `undefined`, comparisons
+ * are handled by the method instead. The `customizer` is invoked with up to
+ * six arguments: (objValue, othValue [, index|key, object, other, stack]).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @param {Function} [customizer] The function to customize comparisons.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * function isGreeting(value) {
+ * return /^h(?:i|ello)$/.test(value);
+ * }
+ *
+ * function customizer(objValue, othValue) {
+ * if (isGreeting(objValue) && isGreeting(othValue)) {
+ * return true;
+ * }
+ * }
+ *
+ * var array = ['hello', 'goodbye'];
+ * var other = ['hi', 'goodbye'];
+ *
+ * _.isEqualWith(array, other, customizer);
+ * // => true
+ */
+ function isEqualWith(value, other, customizer) {
+ customizer = typeof customizer == 'function' ? customizer : undefined;
+ var result = customizer ? customizer(value, other) : undefined;
+ return result === undefined ? baseIsEqual(value, other, undefined, customizer) : !!result;
+ }
+
+ /**
+ * Checks if `value` is an `Error`, `EvalError`, `RangeError`, `ReferenceError`,
+ * `SyntaxError`, `TypeError`, or `URIError` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an error object, else `false`.
+ * @example
+ *
+ * _.isError(new Error);
+ * // => true
+ *
+ * _.isError(Error);
+ * // => false
+ */
+ function isError(value) {
+ if (!isObjectLike(value)) {
+ return false;
+ }
+ var tag = baseGetTag(value);
+ return tag == errorTag || tag == domExcTag ||
+ (typeof value.message == 'string' && typeof value.name == 'string' && !isPlainObject(value));
+ }
+
+ /**
+ * Checks if `value` is a finite primitive number.
+ *
+ * **Note:** This method is based on
+ * [`Number.isFinite`](https://mdn.io/Number/isFinite).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a finite number, else `false`.
+ * @example
+ *
+ * _.isFinite(3);
+ * // => true
+ *
+ * _.isFinite(Number.MIN_VALUE);
+ * // => true
+ *
+ * _.isFinite(Infinity);
+ * // => false
+ *
+ * _.isFinite('3');
+ * // => false
+ */
+ function isFinite(value) {
+ return typeof value == 'number' && nativeIsFinite(value);
+ }
+
+ /**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+ function isFunction(value) {
+ if (!isObject(value)) {
+ return false;
+ }
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in Safari 9 which returns 'object' for typed arrays and other constructors.
+ var tag = baseGetTag(value);
+ return tag == funcTag || tag == genTag || tag == asyncTag || tag == proxyTag;
+ }
+
+ /**
+ * Checks if `value` is an integer.
+ *
+ * **Note:** This method is based on
+ * [`Number.isInteger`](https://mdn.io/Number/isInteger).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an integer, else `false`.
+ * @example
+ *
+ * _.isInteger(3);
+ * // => true
+ *
+ * _.isInteger(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isInteger(Infinity);
+ * // => false
+ *
+ * _.isInteger('3');
+ * // => false
+ */
+ function isInteger(value) {
+ return typeof value == 'number' && value == toInteger(value);
+ }
+
+ /**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This method is loosely based on
+ * [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ * @example
+ *
+ * _.isLength(3);
+ * // => true
+ *
+ * _.isLength(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isLength(Infinity);
+ * // => false
+ *
+ * _.isLength('3');
+ * // => false
+ */
+ function isLength(value) {
+ return typeof value == 'number' &&
+ value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+ }
+
+ /**
+ * Checks if `value` is the
+ * [language type](http://www.ecma-international.org/ecma-262/7.0/#sec-ecmascript-language-types)
+ * of `Object`. (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(_.noop);
+ * // => true
+ *
+ * _.isObject(null);
+ * // => false
+ */
+ function isObject(value) {
+ var type = typeof value;
+ return value != null && (type == 'object' || type == 'function');
+ }
+
+ /**
+ * Checks if `value` is object-like. A value is object-like if it's not `null`
+ * and has a `typeof` result of "object".
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ * @example
+ *
+ * _.isObjectLike({});
+ * // => true
+ *
+ * _.isObjectLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isObjectLike(_.noop);
+ * // => false
+ *
+ * _.isObjectLike(null);
+ * // => false
+ */
+ function isObjectLike(value) {
+ return value != null && typeof value == 'object';
+ }
+
+ /**
+ * Checks if `value` is classified as a `Map` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a map, else `false`.
+ * @example
+ *
+ * _.isMap(new Map);
+ * // => true
+ *
+ * _.isMap(new WeakMap);
+ * // => false
+ */
+ var isMap = nodeIsMap ? baseUnary(nodeIsMap) : baseIsMap;
+
+ /**
+ * Performs a partial deep comparison between `object` and `source` to
+ * determine if `object` contains equivalent property values.
+ *
+ * **Note:** This method is equivalent to `_.matches` when `source` is
+ * partially applied.
+ *
+ * Partial comparisons will match empty array and empty object `source`
+ * values against any array or object value, respectively. See `_.isEqual`
+ * for a list of supported value comparisons.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property values to match.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': 2 };
+ *
+ * _.isMatch(object, { 'b': 2 });
+ * // => true
+ *
+ * _.isMatch(object, { 'b': 1 });
+ * // => false
+ */
+ function isMatch(object, source) {
+ return object === source || baseIsMatch(object, source, getMatchData(source));
+ }
+
+ /**
+ * This method is like `_.isMatch` except that it accepts `customizer` which
+ * is invoked to compare values. If `customizer` returns `undefined`, comparisons
+ * are handled by the method instead. The `customizer` is invoked with five
+ * arguments: (objValue, srcValue, index|key, object, source).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property values to match.
+ * @param {Function} [customizer] The function to customize comparisons.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ * @example
+ *
+ * function isGreeting(value) {
+ * return /^h(?:i|ello)$/.test(value);
+ * }
+ *
+ * function customizer(objValue, srcValue) {
+ * if (isGreeting(objValue) && isGreeting(srcValue)) {
+ * return true;
+ * }
+ * }
+ *
+ * var object = { 'greeting': 'hello' };
+ * var source = { 'greeting': 'hi' };
+ *
+ * _.isMatchWith(object, source, customizer);
+ * // => true
+ */
+ function isMatchWith(object, source, customizer) {
+ customizer = typeof customizer == 'function' ? customizer : undefined;
+ return baseIsMatch(object, source, getMatchData(source), customizer);
+ }
+
+ /**
+ * Checks if `value` is `NaN`.
+ *
+ * **Note:** This method is based on
+ * [`Number.isNaN`](https://mdn.io/Number/isNaN) and is not the same as
+ * global [`isNaN`](https://mdn.io/isNaN) which returns `true` for
+ * `undefined` and other non-number values.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `NaN`, else `false`.
+ * @example
+ *
+ * _.isNaN(NaN);
+ * // => true
+ *
+ * _.isNaN(new Number(NaN));
+ * // => true
+ *
+ * isNaN(undefined);
+ * // => true
+ *
+ * _.isNaN(undefined);
+ * // => false
+ */
+ function isNaN(value) {
+ // An `NaN` primitive is the only value that is not equal to itself.
+ // Perform the `toStringTag` check first to avoid errors with some
+ // ActiveX objects in IE.
+ return isNumber(value) && value != +value;
+ }
+
+ /**
+ * Checks if `value` is a pristine native function.
+ *
+ * **Note:** This method can't reliably detect native functions in the presence
+ * of the core-js package because core-js circumvents this kind of detection.
+ * Despite multiple requests, the core-js maintainer has made it clear: any
+ * attempt to fix the detection will be obstructed. As a result, we're left
+ * with little choice but to throw an error. Unfortunately, this also affects
+ * packages, like [babel-polyfill](https://www.npmjs.com/package/babel-polyfill),
+ * which rely on core-js.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a native function,
+ * else `false`.
+ * @example
+ *
+ * _.isNative(Array.prototype.push);
+ * // => true
+ *
+ * _.isNative(_);
+ * // => false
+ */
+ function isNative(value) {
+ if (isMaskable(value)) {
+ throw new Error(CORE_ERROR_TEXT);
+ }
+ return baseIsNative(value);
+ }
+
+ /**
+ * Checks if `value` is `null`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `null`, else `false`.
+ * @example
+ *
+ * _.isNull(null);
+ * // => true
+ *
+ * _.isNull(void 0);
+ * // => false
+ */
+ function isNull(value) {
+ return value === null;
+ }
+
+ /**
+ * Checks if `value` is `null` or `undefined`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is nullish, else `false`.
+ * @example
+ *
+ * _.isNil(null);
+ * // => true
+ *
+ * _.isNil(void 0);
+ * // => true
+ *
+ * _.isNil(NaN);
+ * // => false
+ */
+ function isNil(value) {
+ return value == null;
+ }
+
+ /**
+ * Checks if `value` is classified as a `Number` primitive or object.
+ *
+ * **Note:** To exclude `Infinity`, `-Infinity`, and `NaN`, which are
+ * classified as numbers, use the `_.isFinite` method.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a number, else `false`.
+ * @example
+ *
+ * _.isNumber(3);
+ * // => true
+ *
+ * _.isNumber(Number.MIN_VALUE);
+ * // => true
+ *
+ * _.isNumber(Infinity);
+ * // => true
+ *
+ * _.isNumber('3');
+ * // => false
+ */
+ function isNumber(value) {
+ return typeof value == 'number' ||
+ (isObjectLike(value) && baseGetTag(value) == numberTag);
+ }
+
+ /**
+ * Checks if `value` is a plain object, that is, an object created by the
+ * `Object` constructor or one with a `[[Prototype]]` of `null`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.8.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a plain object, else `false`.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * _.isPlainObject(new Foo);
+ * // => false
+ *
+ * _.isPlainObject([1, 2, 3]);
+ * // => false
+ *
+ * _.isPlainObject({ 'x': 0, 'y': 0 });
+ * // => true
+ *
+ * _.isPlainObject(Object.create(null));
+ * // => true
+ */
+ function isPlainObject(value) {
+ if (!isObjectLike(value) || baseGetTag(value) != objectTag) {
+ return false;
+ }
+ var proto = getPrototype(value);
+ if (proto === null) {
+ return true;
+ }
+ var Ctor = hasOwnProperty.call(proto, 'constructor') && proto.constructor;
+ return typeof Ctor == 'function' && Ctor instanceof Ctor &&
+ funcToString.call(Ctor) == objectCtorString;
+ }
+
+ /**
+ * Checks if `value` is classified as a `RegExp` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a regexp, else `false`.
+ * @example
+ *
+ * _.isRegExp(/abc/);
+ * // => true
+ *
+ * _.isRegExp('/abc/');
+ * // => false
+ */
+ var isRegExp = nodeIsRegExp ? baseUnary(nodeIsRegExp) : baseIsRegExp;
+
+ /**
+ * Checks if `value` is a safe integer. An integer is safe if it's an IEEE-754
+ * double precision number which isn't the result of a rounded unsafe integer.
+ *
+ * **Note:** This method is based on
+ * [`Number.isSafeInteger`](https://mdn.io/Number/isSafeInteger).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a safe integer, else `false`.
+ * @example
+ *
+ * _.isSafeInteger(3);
+ * // => true
+ *
+ * _.isSafeInteger(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isSafeInteger(Infinity);
+ * // => false
+ *
+ * _.isSafeInteger('3');
+ * // => false
+ */
+ function isSafeInteger(value) {
+ return isInteger(value) && value >= -MAX_SAFE_INTEGER && value <= MAX_SAFE_INTEGER;
+ }
+
+ /**
+ * Checks if `value` is classified as a `Set` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a set, else `false`.
+ * @example
+ *
+ * _.isSet(new Set);
+ * // => true
+ *
+ * _.isSet(new WeakSet);
+ * // => false
+ */
+ var isSet = nodeIsSet ? baseUnary(nodeIsSet) : baseIsSet;
+
+ /**
+ * Checks if `value` is classified as a `String` primitive or object.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a string, else `false`.
+ * @example
+ *
+ * _.isString('abc');
+ * // => true
+ *
+ * _.isString(1);
+ * // => false
+ */
+ function isString(value) {
+ return typeof value == 'string' ||
+ (!isArray(value) && isObjectLike(value) && baseGetTag(value) == stringTag);
+ }
+
+ /**
+ * Checks if `value` is classified as a `Symbol` primitive or object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a symbol, else `false`.
+ * @example
+ *
+ * _.isSymbol(Symbol.iterator);
+ * // => true
+ *
+ * _.isSymbol('abc');
+ * // => false
+ */
+ function isSymbol(value) {
+ return typeof value == 'symbol' ||
+ (isObjectLike(value) && baseGetTag(value) == symbolTag);
+ }
+
+ /**
+ * Checks if `value` is classified as a typed array.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a typed array, else `false`.
+ * @example
+ *
+ * _.isTypedArray(new Uint8Array);
+ * // => true
+ *
+ * _.isTypedArray([]);
+ * // => false
+ */
+ var isTypedArray = nodeIsTypedArray ? baseUnary(nodeIsTypedArray) : baseIsTypedArray;
+
+ /**
+ * Checks if `value` is `undefined`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `undefined`, else `false`.
+ * @example
+ *
+ * _.isUndefined(void 0);
+ * // => true
+ *
+ * _.isUndefined(null);
+ * // => false
+ */
+ function isUndefined(value) {
+ return value === undefined;
+ }
+
+ /**
+ * Checks if `value` is classified as a `WeakMap` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a weak map, else `false`.
+ * @example
+ *
+ * _.isWeakMap(new WeakMap);
+ * // => true
+ *
+ * _.isWeakMap(new Map);
+ * // => false
+ */
+ function isWeakMap(value) {
+ return isObjectLike(value) && getTag(value) == weakMapTag;
+ }
+
+ /**
+ * Checks if `value` is classified as a `WeakSet` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a weak set, else `false`.
+ * @example
+ *
+ * _.isWeakSet(new WeakSet);
+ * // => true
+ *
+ * _.isWeakSet(new Set);
+ * // => false
+ */
+ function isWeakSet(value) {
+ return isObjectLike(value) && baseGetTag(value) == weakSetTag;
+ }
+
+ /**
+ * Checks if `value` is less than `other`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.9.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is less than `other`,
+ * else `false`.
+ * @see _.gt
+ * @example
+ *
+ * _.lt(1, 3);
+ * // => true
+ *
+ * _.lt(3, 3);
+ * // => false
+ *
+ * _.lt(3, 1);
+ * // => false
+ */
+ var lt = createRelationalOperation(baseLt);
+
+ /**
+ * Checks if `value` is less than or equal to `other`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.9.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is less than or equal to
+ * `other`, else `false`.
+ * @see _.gte
+ * @example
+ *
+ * _.lte(1, 3);
+ * // => true
+ *
+ * _.lte(3, 3);
+ * // => true
+ *
+ * _.lte(3, 1);
+ * // => false
+ */
+ var lte = createRelationalOperation(function(value, other) {
+ return value <= other;
+ });
+
+ /**
+ * Converts `value` to an array.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {Array} Returns the converted array.
+ * @example
+ *
+ * _.toArray({ 'a': 1, 'b': 2 });
+ * // => [1, 2]
+ *
+ * _.toArray('abc');
+ * // => ['a', 'b', 'c']
+ *
+ * _.toArray(1);
+ * // => []
+ *
+ * _.toArray(null);
+ * // => []
+ */
+ function toArray(value) {
+ if (!value) {
+ return [];
+ }
+ if (isArrayLike(value)) {
+ return isString(value) ? stringToArray(value) : copyArray(value);
+ }
+ if (symIterator && value[symIterator]) {
+ return iteratorToArray(value[symIterator]());
+ }
+ var tag = getTag(value),
+ func = tag == mapTag ? mapToArray : (tag == setTag ? setToArray : values);
+
+ return func(value);
+ }
+
+ /**
+ * Converts `value` to a finite number.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.12.0
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {number} Returns the converted number.
+ * @example
+ *
+ * _.toFinite(3.2);
+ * // => 3.2
+ *
+ * _.toFinite(Number.MIN_VALUE);
+ * // => 5e-324
+ *
+ * _.toFinite(Infinity);
+ * // => 1.7976931348623157e+308
+ *
+ * _.toFinite('3.2');
+ * // => 3.2
+ */
+ function toFinite(value) {
+ if (!value) {
+ return value === 0 ? value : 0;
+ }
+ value = toNumber(value);
+ if (value === INFINITY || value === -INFINITY) {
+ var sign = (value < 0 ? -1 : 1);
+ return sign * MAX_INTEGER;
+ }
+ return value === value ? value : 0;
+ }
+
+ /**
+ * Converts `value` to an integer.
+ *
+ * **Note:** This method is loosely based on
+ * [`ToInteger`](http://www.ecma-international.org/ecma-262/7.0/#sec-tointeger).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {number} Returns the converted integer.
+ * @example
+ *
+ * _.toInteger(3.2);
+ * // => 3
+ *
+ * _.toInteger(Number.MIN_VALUE);
+ * // => 0
+ *
+ * _.toInteger(Infinity);
+ * // => 1.7976931348623157e+308
+ *
+ * _.toInteger('3.2');
+ * // => 3
+ */
+ function toInteger(value) {
+ var result = toFinite(value),
+ remainder = result % 1;
+
+ return result === result ? (remainder ? result - remainder : result) : 0;
+ }
+
+ /**
+ * Converts `value` to an integer suitable for use as the length of an
+ * array-like object.
+ *
+ * **Note:** This method is based on
+ * [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {number} Returns the converted integer.
+ * @example
+ *
+ * _.toLength(3.2);
+ * // => 3
+ *
+ * _.toLength(Number.MIN_VALUE);
+ * // => 0
+ *
+ * _.toLength(Infinity);
+ * // => 4294967295
+ *
+ * _.toLength('3.2');
+ * // => 3
+ */
+ function toLength(value) {
+ return value ? baseClamp(toInteger(value), 0, MAX_ARRAY_LENGTH) : 0;
+ }
+
+ /**
+ * Converts `value` to a number.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to process.
+ * @returns {number} Returns the number.
+ * @example
+ *
+ * _.toNumber(3.2);
+ * // => 3.2
+ *
+ * _.toNumber(Number.MIN_VALUE);
+ * // => 5e-324
+ *
+ * _.toNumber(Infinity);
+ * // => Infinity
+ *
+ * _.toNumber('3.2');
+ * // => 3.2
+ */
+ function toNumber(value) {
+ if (typeof value == 'number') {
+ return value;
+ }
+ if (isSymbol(value)) {
+ return NAN;
+ }
+ if (isObject(value)) {
+ var other = typeof value.valueOf == 'function' ? value.valueOf() : value;
+ value = isObject(other) ? (other + '') : other;
+ }
+ if (typeof value != 'string') {
+ return value === 0 ? value : +value;
+ }
+ value = baseTrim(value);
+ var isBinary = reIsBinary.test(value);
+ return (isBinary || reIsOctal.test(value))
+ ? freeParseInt(value.slice(2), isBinary ? 2 : 8)
+ : (reIsBadHex.test(value) ? NAN : +value);
+ }
+
+ /**
+ * Converts `value` to a plain object flattening inherited enumerable string
+ * keyed properties of `value` to own properties of the plain object.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {Object} Returns the converted plain object.
+ * @example
+ *
+ * function Foo() {
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.assign({ 'a': 1 }, new Foo);
+ * // => { 'a': 1, 'b': 2 }
+ *
+ * _.assign({ 'a': 1 }, _.toPlainObject(new Foo));
+ * // => { 'a': 1, 'b': 2, 'c': 3 }
+ */
+ function toPlainObject(value) {
+ return copyObject(value, keysIn(value));
+ }
+
+ /**
+ * Converts `value` to a safe integer. A safe integer can be compared and
+ * represented correctly.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {number} Returns the converted integer.
+ * @example
+ *
+ * _.toSafeInteger(3.2);
+ * // => 3
+ *
+ * _.toSafeInteger(Number.MIN_VALUE);
+ * // => 0
+ *
+ * _.toSafeInteger(Infinity);
+ * // => 9007199254740991
+ *
+ * _.toSafeInteger('3.2');
+ * // => 3
+ */
+ function toSafeInteger(value) {
+ return value
+ ? baseClamp(toInteger(value), -MAX_SAFE_INTEGER, MAX_SAFE_INTEGER)
+ : (value === 0 ? value : 0);
+ }
+
+ /**
+ * Converts `value` to a string. An empty string is returned for `null`
+ * and `undefined` values. The sign of `-0` is preserved.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {string} Returns the converted string.
+ * @example
+ *
+ * _.toString(null);
+ * // => ''
+ *
+ * _.toString(-0);
+ * // => '-0'
+ *
+ * _.toString([1, 2, 3]);
+ * // => '1,2,3'
+ */
+ function toString(value) {
+ return value == null ? '' : baseToString(value);
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Assigns own enumerable string keyed properties of source objects to the
+ * destination object. Source objects are applied from left to right.
+ * Subsequent sources overwrite property assignments of previous sources.
+ *
+ * **Note:** This method mutates `object` and is loosely based on
+ * [`Object.assign`](https://mdn.io/Object/assign).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.10.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.assignIn
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * function Bar() {
+ * this.c = 3;
+ * }
+ *
+ * Foo.prototype.b = 2;
+ * Bar.prototype.d = 4;
+ *
+ * _.assign({ 'a': 0 }, new Foo, new Bar);
+ * // => { 'a': 1, 'c': 3 }
+ */
+ var assign = createAssigner(function(object, source) {
+ if (isPrototype(source) || isArrayLike(source)) {
+ copyObject(source, keys(source), object);
+ return;
+ }
+ for (var key in source) {
+ if (hasOwnProperty.call(source, key)) {
+ assignValue(object, key, source[key]);
+ }
+ }
+ });
+
+ /**
+ * This method is like `_.assign` except that it iterates over own and
+ * inherited source properties.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @alias extend
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.assign
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * function Bar() {
+ * this.c = 3;
+ * }
+ *
+ * Foo.prototype.b = 2;
+ * Bar.prototype.d = 4;
+ *
+ * _.assignIn({ 'a': 0 }, new Foo, new Bar);
+ * // => { 'a': 1, 'b': 2, 'c': 3, 'd': 4 }
+ */
+ var assignIn = createAssigner(function(object, source) {
+ copyObject(source, keysIn(source), object);
+ });
+
+ /**
+ * This method is like `_.assignIn` except that it accepts `customizer`
+ * which is invoked to produce the assigned values. If `customizer` returns
+ * `undefined`, assignment is handled by the method instead. The `customizer`
+ * is invoked with five arguments: (objValue, srcValue, key, object, source).
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @alias extendWith
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} sources The source objects.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ * @see _.assignWith
+ * @example
+ *
+ * function customizer(objValue, srcValue) {
+ * return _.isUndefined(objValue) ? srcValue : objValue;
+ * }
+ *
+ * var defaults = _.partialRight(_.assignInWith, customizer);
+ *
+ * defaults({ 'a': 1 }, { 'b': 2 }, { 'a': 3 });
+ * // => { 'a': 1, 'b': 2 }
+ */
+ var assignInWith = createAssigner(function(object, source, srcIndex, customizer) {
+ copyObject(source, keysIn(source), object, customizer);
+ });
+
+ /**
+ * This method is like `_.assign` except that it accepts `customizer`
+ * which is invoked to produce the assigned values. If `customizer` returns
+ * `undefined`, assignment is handled by the method instead. The `customizer`
+ * is invoked with five arguments: (objValue, srcValue, key, object, source).
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} sources The source objects.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ * @see _.assignInWith
+ * @example
+ *
+ * function customizer(objValue, srcValue) {
+ * return _.isUndefined(objValue) ? srcValue : objValue;
+ * }
+ *
+ * var defaults = _.partialRight(_.assignWith, customizer);
+ *
+ * defaults({ 'a': 1 }, { 'b': 2 }, { 'a': 3 });
+ * // => { 'a': 1, 'b': 2 }
+ */
+ var assignWith = createAssigner(function(object, source, srcIndex, customizer) {
+ copyObject(source, keys(source), object, customizer);
+ });
+
+ /**
+ * Creates an array of values corresponding to `paths` of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.0.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {...(string|string[])} [paths] The property paths to pick.
+ * @returns {Array} Returns the picked values.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': 3 } }, 4] };
+ *
+ * _.at(object, ['a[0].b.c', 'a[1]']);
+ * // => [3, 4]
+ */
+ var at = flatRest(baseAt);
+
+ /**
+ * Creates an object that inherits from the `prototype` object. If a
+ * `properties` object is given, its own enumerable string keyed properties
+ * are assigned to the created object.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.3.0
+ * @category Object
+ * @param {Object} prototype The object to inherit from.
+ * @param {Object} [properties] The properties to assign to the object.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * function Circle() {
+ * Shape.call(this);
+ * }
+ *
+ * Circle.prototype = _.create(Shape.prototype, {
+ * 'constructor': Circle
+ * });
+ *
+ * var circle = new Circle;
+ * circle instanceof Circle;
+ * // => true
+ *
+ * circle instanceof Shape;
+ * // => true
+ */
+ function create(prototype, properties) {
+ var result = baseCreate(prototype);
+ return properties == null ? result : baseAssign(result, properties);
+ }
+
+ /**
+ * Assigns own and inherited enumerable string keyed properties of source
+ * objects to the destination object for all destination properties that
+ * resolve to `undefined`. Source objects are applied from left to right.
+ * Once a property is set, additional values of the same property are ignored.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.defaultsDeep
+ * @example
+ *
+ * _.defaults({ 'a': 1 }, { 'b': 2 }, { 'a': 3 });
+ * // => { 'a': 1, 'b': 2 }
+ */
+ var defaults = baseRest(function(object, sources) {
+ object = Object(object);
+
+ var index = -1;
+ var length = sources.length;
+ var guard = length > 2 ? sources[2] : undefined;
+
+ if (guard && isIterateeCall(sources[0], sources[1], guard)) {
+ length = 1;
+ }
+
+ while (++index < length) {
+ var source = sources[index];
+ var props = keysIn(source);
+ var propsIndex = -1;
+ var propsLength = props.length;
+
+ while (++propsIndex < propsLength) {
+ var key = props[propsIndex];
+ var value = object[key];
+
+ if (value === undefined ||
+ (eq(value, objectProto[key]) && !hasOwnProperty.call(object, key))) {
+ object[key] = source[key];
+ }
+ }
+ }
+
+ return object;
+ });
+
+ /**
+ * This method is like `_.defaults` except that it recursively assigns
+ * default properties.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.10.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.defaults
+ * @example
+ *
+ * _.defaultsDeep({ 'a': { 'b': 2 } }, { 'a': { 'b': 1, 'c': 3 } });
+ * // => { 'a': { 'b': 2, 'c': 3 } }
+ */
+ var defaultsDeep = baseRest(function(args) {
+ args.push(undefined, customDefaultsMerge);
+ return apply(mergeWith, undefined, args);
+ });
+
+ /**
+ * This method is like `_.find` except that it returns the key of the first
+ * element `predicate` returns truthy for instead of the element itself.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.1.0
+ * @category Object
+ * @param {Object} object The object to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {string|undefined} Returns the key of the matched element,
+ * else `undefined`.
+ * @example
+ *
+ * var users = {
+ * 'barney': { 'age': 36, 'active': true },
+ * 'fred': { 'age': 40, 'active': false },
+ * 'pebbles': { 'age': 1, 'active': true }
+ * };
+ *
+ * _.findKey(users, function(o) { return o.age < 40; });
+ * // => 'barney' (iteration order is not guaranteed)
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.findKey(users, { 'age': 1, 'active': true });
+ * // => 'pebbles'
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.findKey(users, ['active', false]);
+ * // => 'fred'
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.findKey(users, 'active');
+ * // => 'barney'
+ */
+ function findKey(object, predicate) {
+ return baseFindKey(object, getIteratee(predicate, 3), baseForOwn);
+ }
+
+ /**
+ * This method is like `_.findKey` except that it iterates over elements of
+ * a collection in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Object
+ * @param {Object} object The object to inspect.
+ * @param {Function} [predicate=_.identity] The function invoked per iteration.
+ * @returns {string|undefined} Returns the key of the matched element,
+ * else `undefined`.
+ * @example
+ *
+ * var users = {
+ * 'barney': { 'age': 36, 'active': true },
+ * 'fred': { 'age': 40, 'active': false },
+ * 'pebbles': { 'age': 1, 'active': true }
+ * };
+ *
+ * _.findLastKey(users, function(o) { return o.age < 40; });
+ * // => returns 'pebbles' assuming `_.findKey` returns 'barney'
+ *
+ * // The `_.matches` iteratee shorthand.
+ * _.findLastKey(users, { 'age': 36, 'active': true });
+ * // => 'barney'
+ *
+ * // The `_.matchesProperty` iteratee shorthand.
+ * _.findLastKey(users, ['active', false]);
+ * // => 'fred'
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.findLastKey(users, 'active');
+ * // => 'pebbles'
+ */
+ function findLastKey(object, predicate) {
+ return baseFindKey(object, getIteratee(predicate, 3), baseForOwnRight);
+ }
+
+ /**
+ * Iterates over own and inherited enumerable string keyed properties of an
+ * object and invokes `iteratee` for each property. The iteratee is invoked
+ * with three arguments: (value, key, object). Iteratee functions may exit
+ * iteration early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.3.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ * @see _.forInRight
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forIn(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'a', 'b', then 'c' (iteration order is not guaranteed).
+ */
+ function forIn(object, iteratee) {
+ return object == null
+ ? object
+ : baseFor(object, getIteratee(iteratee, 3), keysIn);
+ }
+
+ /**
+ * This method is like `_.forIn` except that it iterates over properties of
+ * `object` in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ * @see _.forIn
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forInRight(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'c', 'b', then 'a' assuming `_.forIn` logs 'a', 'b', then 'c'.
+ */
+ function forInRight(object, iteratee) {
+ return object == null
+ ? object
+ : baseForRight(object, getIteratee(iteratee, 3), keysIn);
+ }
+
+ /**
+ * Iterates over own enumerable string keyed properties of an object and
+ * invokes `iteratee` for each property. The iteratee is invoked with three
+ * arguments: (value, key, object). Iteratee functions may exit iteration
+ * early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.3.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ * @see _.forOwnRight
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forOwn(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'a' then 'b' (iteration order is not guaranteed).
+ */
+ function forOwn(object, iteratee) {
+ return object && baseForOwn(object, getIteratee(iteratee, 3));
+ }
+
+ /**
+ * This method is like `_.forOwn` except that it iterates over properties of
+ * `object` in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.0.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ * @see _.forOwn
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forOwnRight(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => Logs 'b' then 'a' assuming `_.forOwn` logs 'a' then 'b'.
+ */
+ function forOwnRight(object, iteratee) {
+ return object && baseForOwnRight(object, getIteratee(iteratee, 3));
+ }
+
+ /**
+ * Creates an array of function property names from own enumerable properties
+ * of `object`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns the function names.
+ * @see _.functionsIn
+ * @example
+ *
+ * function Foo() {
+ * this.a = _.constant('a');
+ * this.b = _.constant('b');
+ * }
+ *
+ * Foo.prototype.c = _.constant('c');
+ *
+ * _.functions(new Foo);
+ * // => ['a', 'b']
+ */
+ function functions(object) {
+ return object == null ? [] : baseFunctions(object, keys(object));
+ }
+
+ /**
+ * Creates an array of function property names from own and inherited
+ * enumerable properties of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns the function names.
+ * @see _.functions
+ * @example
+ *
+ * function Foo() {
+ * this.a = _.constant('a');
+ * this.b = _.constant('b');
+ * }
+ *
+ * Foo.prototype.c = _.constant('c');
+ *
+ * _.functionsIn(new Foo);
+ * // => ['a', 'b', 'c']
+ */
+ function functionsIn(object) {
+ return object == null ? [] : baseFunctions(object, keysIn(object));
+ }
+
+ /**
+ * Gets the value at `path` of `object`. If the resolved value is
+ * `undefined`, the `defaultValue` is returned in its place.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.7.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the property to get.
+ * @param {*} [defaultValue] The value returned for `undefined` resolved values.
+ * @returns {*} Returns the resolved value.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': 3 } }] };
+ *
+ * _.get(object, 'a[0].b.c');
+ * // => 3
+ *
+ * _.get(object, ['a', '0', 'b', 'c']);
+ * // => 3
+ *
+ * _.get(object, 'a.b.c', 'default');
+ * // => 'default'
+ */
+ function get(object, path, defaultValue) {
+ var result = object == null ? undefined : baseGet(object, path);
+ return result === undefined ? defaultValue : result;
+ }
+
+ /**
+ * Checks if `path` is a direct property of `object`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path to check.
+ * @returns {boolean} Returns `true` if `path` exists, else `false`.
+ * @example
+ *
+ * var object = { 'a': { 'b': 2 } };
+ * var other = _.create({ 'a': _.create({ 'b': 2 }) });
+ *
+ * _.has(object, 'a');
+ * // => true
+ *
+ * _.has(object, 'a.b');
+ * // => true
+ *
+ * _.has(object, ['a', 'b']);
+ * // => true
+ *
+ * _.has(other, 'a');
+ * // => false
+ */
+ function has(object, path) {
+ return object != null && hasPath(object, path, baseHas);
+ }
+
+ /**
+ * Checks if `path` is a direct or inherited property of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path to check.
+ * @returns {boolean} Returns `true` if `path` exists, else `false`.
+ * @example
+ *
+ * var object = _.create({ 'a': _.create({ 'b': 2 }) });
+ *
+ * _.hasIn(object, 'a');
+ * // => true
+ *
+ * _.hasIn(object, 'a.b');
+ * // => true
+ *
+ * _.hasIn(object, ['a', 'b']);
+ * // => true
+ *
+ * _.hasIn(object, 'b');
+ * // => false
+ */
+ function hasIn(object, path) {
+ return object != null && hasPath(object, path, baseHasIn);
+ }
+
+ /**
+ * Creates an object composed of the inverted keys and values of `object`.
+ * If `object` contains duplicate values, subsequent values overwrite
+ * property assignments of previous values.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.7.0
+ * @category Object
+ * @param {Object} object The object to invert.
+ * @returns {Object} Returns the new inverted object.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': 2, 'c': 1 };
+ *
+ * _.invert(object);
+ * // => { '1': 'c', '2': 'b' }
+ */
+ var invert = createInverter(function(result, value, key) {
+ if (value != null &&
+ typeof value.toString != 'function') {
+ value = nativeObjectToString.call(value);
+ }
+
+ result[value] = key;
+ }, constant(identity));
+
+ /**
+ * This method is like `_.invert` except that the inverted object is generated
+ * from the results of running each element of `object` thru `iteratee`. The
+ * corresponding inverted value of each inverted key is an array of keys
+ * responsible for generating the inverted value. The iteratee is invoked
+ * with one argument: (value).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.1.0
+ * @category Object
+ * @param {Object} object The object to invert.
+ * @param {Function} [iteratee=_.identity] The iteratee invoked per element.
+ * @returns {Object} Returns the new inverted object.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': 2, 'c': 1 };
+ *
+ * _.invertBy(object);
+ * // => { '1': ['a', 'c'], '2': ['b'] }
+ *
+ * _.invertBy(object, function(value) {
+ * return 'group' + value;
+ * });
+ * // => { 'group1': ['a', 'c'], 'group2': ['b'] }
+ */
+ var invertBy = createInverter(function(result, value, key) {
+ if (value != null &&
+ typeof value.toString != 'function') {
+ value = nativeObjectToString.call(value);
+ }
+
+ if (hasOwnProperty.call(result, value)) {
+ result[value].push(key);
+ } else {
+ result[value] = [key];
+ }
+ }, getIteratee);
+
+ /**
+ * Invokes the method at `path` of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the method to invoke.
+ * @param {...*} [args] The arguments to invoke the method with.
+ * @returns {*} Returns the result of the invoked method.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': [1, 2, 3, 4] } }] };
+ *
+ * _.invoke(object, 'a[0].b.c.slice', 1, 3);
+ * // => [2, 3]
+ */
+ var invoke = baseRest(baseInvoke);
+
+ /**
+ * Creates an array of the own enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects. See the
+ * [ES spec](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
+ * for more details.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keys(new Foo);
+ * // => ['a', 'b'] (iteration order is not guaranteed)
+ *
+ * _.keys('hi');
+ * // => ['0', '1']
+ */
+ function keys(object) {
+ return isArrayLike(object) ? arrayLikeKeys(object) : baseKeys(object);
+ }
+
+ /**
+ * Creates an array of the own and inherited enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keysIn(new Foo);
+ * // => ['a', 'b', 'c'] (iteration order is not guaranteed)
+ */
+ function keysIn(object) {
+ return isArrayLike(object) ? arrayLikeKeys(object, true) : baseKeysIn(object);
+ }
+
+ /**
+ * The opposite of `_.mapValues`; this method creates an object with the
+ * same values as `object` and keys generated by running each own enumerable
+ * string keyed property of `object` thru `iteratee`. The iteratee is invoked
+ * with three arguments: (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.8.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Object} Returns the new mapped object.
+ * @see _.mapValues
+ * @example
+ *
+ * _.mapKeys({ 'a': 1, 'b': 2 }, function(value, key) {
+ * return key + value;
+ * });
+ * // => { 'a1': 1, 'b2': 2 }
+ */
+ function mapKeys(object, iteratee) {
+ var result = {};
+ iteratee = getIteratee(iteratee, 3);
+
+ baseForOwn(object, function(value, key, object) {
+ baseAssignValue(result, iteratee(value, key, object), value);
+ });
+ return result;
+ }
+
+ /**
+ * Creates an object with the same keys as `object` and values generated
+ * by running each own enumerable string keyed property of `object` thru
+ * `iteratee`. The iteratee is invoked with three arguments:
+ * (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @since 2.4.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @returns {Object} Returns the new mapped object.
+ * @see _.mapKeys
+ * @example
+ *
+ * var users = {
+ * 'fred': { 'user': 'fred', 'age': 40 },
+ * 'pebbles': { 'user': 'pebbles', 'age': 1 }
+ * };
+ *
+ * _.mapValues(users, function(o) { return o.age; });
+ * // => { 'fred': 40, 'pebbles': 1 } (iteration order is not guaranteed)
+ *
+ * // The `_.property` iteratee shorthand.
+ * _.mapValues(users, 'age');
+ * // => { 'fred': 40, 'pebbles': 1 } (iteration order is not guaranteed)
+ */
+ function mapValues(object, iteratee) {
+ var result = {};
+ iteratee = getIteratee(iteratee, 3);
+
+ baseForOwn(object, function(value, key, object) {
+ baseAssignValue(result, key, iteratee(value, key, object));
+ });
+ return result;
+ }
+
+ /**
+ * This method is like `_.assign` except that it recursively merges own and
+ * inherited enumerable string keyed properties of source objects into the
+ * destination object. Source properties that resolve to `undefined` are
+ * skipped if a destination value exists. Array and plain object properties
+ * are merged recursively. Other objects and value types are overridden by
+ * assignment. Source objects are applied from left to right. Subsequent
+ * sources overwrite property assignments of previous sources.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.5.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var object = {
+ * 'a': [{ 'b': 2 }, { 'd': 4 }]
+ * };
+ *
+ * var other = {
+ * 'a': [{ 'c': 3 }, { 'e': 5 }]
+ * };
+ *
+ * _.merge(object, other);
+ * // => { 'a': [{ 'b': 2, 'c': 3 }, { 'd': 4, 'e': 5 }] }
+ */
+ var merge = createAssigner(function(object, source, srcIndex) {
+ baseMerge(object, source, srcIndex);
+ });
+
+ /**
+ * This method is like `_.merge` except that it accepts `customizer` which
+ * is invoked to produce the merged values of the destination and source
+ * properties. If `customizer` returns `undefined`, merging is handled by the
+ * method instead. The `customizer` is invoked with six arguments:
+ * (objValue, srcValue, key, object, source, stack).
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} sources The source objects.
+ * @param {Function} customizer The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function customizer(objValue, srcValue) {
+ * if (_.isArray(objValue)) {
+ * return objValue.concat(srcValue);
+ * }
+ * }
+ *
+ * var object = { 'a': [1], 'b': [2] };
+ * var other = { 'a': [3], 'b': [4] };
+ *
+ * _.mergeWith(object, other, customizer);
+ * // => { 'a': [1, 3], 'b': [2, 4] }
+ */
+ var mergeWith = createAssigner(function(object, source, srcIndex, customizer) {
+ baseMerge(object, source, srcIndex, customizer);
+ });
+
+ /**
+ * The opposite of `_.pick`; this method creates an object composed of the
+ * own and inherited enumerable property paths of `object` that are not omitted.
+ *
+ * **Note:** This method is considerably slower than `_.pick`.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The source object.
+ * @param {...(string|string[])} [paths] The property paths to omit.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': '2', 'c': 3 };
+ *
+ * _.omit(object, ['a', 'c']);
+ * // => { 'b': '2' }
+ */
+ var omit = flatRest(function(object, paths) {
+ var result = {};
+ if (object == null) {
+ return result;
+ }
+ var isDeep = false;
+ paths = arrayMap(paths, function(path) {
+ path = castPath(path, object);
+ isDeep || (isDeep = path.length > 1);
+ return path;
+ });
+ copyObject(object, getAllKeysIn(object), result);
+ if (isDeep) {
+ result = baseClone(result, CLONE_DEEP_FLAG | CLONE_FLAT_FLAG | CLONE_SYMBOLS_FLAG, customOmitClone);
+ }
+ var length = paths.length;
+ while (length--) {
+ baseUnset(result, paths[length]);
+ }
+ return result;
+ });
+
+ /**
+ * The opposite of `_.pickBy`; this method creates an object composed of
+ * the own and inherited enumerable string keyed properties of `object` that
+ * `predicate` doesn't return truthy for. The predicate is invoked with two
+ * arguments: (value, key).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The source object.
+ * @param {Function} [predicate=_.identity] The function invoked per property.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': '2', 'c': 3 };
+ *
+ * _.omitBy(object, _.isNumber);
+ * // => { 'b': '2' }
+ */
+ function omitBy(object, predicate) {
+ return pickBy(object, negate(getIteratee(predicate)));
+ }
+
+ /**
+ * Creates an object composed of the picked `object` properties.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The source object.
+ * @param {...(string|string[])} [paths] The property paths to pick.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': '2', 'c': 3 };
+ *
+ * _.pick(object, ['a', 'c']);
+ * // => { 'a': 1, 'c': 3 }
+ */
+ var pick = flatRest(function(object, paths) {
+ return object == null ? {} : basePick(object, paths);
+ });
+
+ /**
+ * Creates an object composed of the `object` properties `predicate` returns
+ * truthy for. The predicate is invoked with two arguments: (value, key).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The source object.
+ * @param {Function} [predicate=_.identity] The function invoked per property.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': '2', 'c': 3 };
+ *
+ * _.pickBy(object, _.isNumber);
+ * // => { 'a': 1, 'c': 3 }
+ */
+ function pickBy(object, predicate) {
+ if (object == null) {
+ return {};
+ }
+ var props = arrayMap(getAllKeysIn(object), function(prop) {
+ return [prop];
+ });
+ predicate = getIteratee(predicate);
+ return basePickBy(object, props, function(value, path) {
+ return predicate(value, path[0]);
+ });
+ }
+
+ /**
+ * This method is like `_.get` except that if the resolved value is a
+ * function it's invoked with the `this` binding of its parent object and
+ * its result is returned.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the property to resolve.
+ * @param {*} [defaultValue] The value returned for `undefined` resolved values.
+ * @returns {*} Returns the resolved value.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c1': 3, 'c2': _.constant(4) } }] };
+ *
+ * _.result(object, 'a[0].b.c1');
+ * // => 3
+ *
+ * _.result(object, 'a[0].b.c2');
+ * // => 4
+ *
+ * _.result(object, 'a[0].b.c3', 'default');
+ * // => 'default'
+ *
+ * _.result(object, 'a[0].b.c3', _.constant('default'));
+ * // => 'default'
+ */
+ function result(object, path, defaultValue) {
+ path = castPath(path, object);
+
+ var index = -1,
+ length = path.length;
+
+ // Ensure the loop is entered when path is empty.
+ if (!length) {
+ length = 1;
+ object = undefined;
+ }
+ while (++index < length) {
+ var value = object == null ? undefined : object[toKey(path[index])];
+ if (value === undefined) {
+ index = length;
+ value = defaultValue;
+ }
+ object = isFunction(value) ? value.call(object) : value;
+ }
+ return object;
+ }
+
+ /**
+ * Sets the value at `path` of `object`. If a portion of `path` doesn't exist,
+ * it's created. Arrays are created for missing index properties while objects
+ * are created for all other missing properties. Use `_.setWith` to customize
+ * `path` creation.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.7.0
+ * @category Object
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The path of the property to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': 3 } }] };
+ *
+ * _.set(object, 'a[0].b.c', 4);
+ * console.log(object.a[0].b.c);
+ * // => 4
+ *
+ * _.set(object, ['x', '0', 'y', 'z'], 5);
+ * console.log(object.x[0].y.z);
+ * // => 5
+ */
+ function set(object, path, value) {
+ return object == null ? object : baseSet(object, path, value);
+ }
+
+ /**
+ * This method is like `_.set` except that it accepts `customizer` which is
+ * invoked to produce the objects of `path`. If `customizer` returns `undefined`
+ * path creation is handled by the method instead. The `customizer` is invoked
+ * with three arguments: (nsValue, key, nsObject).
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The path of the property to set.
+ * @param {*} value The value to set.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var object = {};
+ *
+ * _.setWith(object, '[0][1]', 'a', Object);
+ * // => { '0': { '1': 'a' } }
+ */
+ function setWith(object, path, value, customizer) {
+ customizer = typeof customizer == 'function' ? customizer : undefined;
+ return object == null ? object : baseSet(object, path, value, customizer);
+ }
+
+ /**
+ * Creates an array of own enumerable string keyed-value pairs for `object`
+ * which can be consumed by `_.fromPairs`. If `object` is a map or set, its
+ * entries are returned.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @alias entries
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the key-value pairs.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.toPairs(new Foo);
+ * // => [['a', 1], ['b', 2]] (iteration order is not guaranteed)
+ */
+ var toPairs = createToPairs(keys);
+
+ /**
+ * Creates an array of own and inherited enumerable string keyed-value pairs
+ * for `object` which can be consumed by `_.fromPairs`. If `object` is a map
+ * or set, its entries are returned.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @alias entriesIn
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the key-value pairs.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.toPairsIn(new Foo);
+ * // => [['a', 1], ['b', 2], ['c', 3]] (iteration order is not guaranteed)
+ */
+ var toPairsIn = createToPairs(keysIn);
+
+ /**
+ * An alternative to `_.reduce`; this method transforms `object` to a new
+ * `accumulator` object which is the result of running each of its own
+ * enumerable string keyed properties thru `iteratee`, with each invocation
+ * potentially mutating the `accumulator` object. If `accumulator` is not
+ * provided, a new object with the same `[[Prototype]]` will be used. The
+ * iteratee is invoked with four arguments: (accumulator, value, key, object).
+ * Iteratee functions may exit iteration early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.3.0
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [accumulator] The custom accumulator value.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * _.transform([2, 3, 4], function(result, n) {
+ * result.push(n *= n);
+ * return n % 2 == 0;
+ * }, []);
+ * // => [4, 9]
+ *
+ * _.transform({ 'a': 1, 'b': 2, 'c': 1 }, function(result, value, key) {
+ * (result[value] || (result[value] = [])).push(key);
+ * }, {});
+ * // => { '1': ['a', 'c'], '2': ['b'] }
+ */
+ function transform(object, iteratee, accumulator) {
+ var isArr = isArray(object),
+ isArrLike = isArr || isBuffer(object) || isTypedArray(object);
+
+ iteratee = getIteratee(iteratee, 4);
+ if (accumulator == null) {
+ var Ctor = object && object.constructor;
+ if (isArrLike) {
+ accumulator = isArr ? new Ctor : [];
+ }
+ else if (isObject(object)) {
+ accumulator = isFunction(Ctor) ? baseCreate(getPrototype(object)) : {};
+ }
+ else {
+ accumulator = {};
+ }
+ }
+ (isArrLike ? arrayEach : baseForOwn)(object, function(value, index, object) {
+ return iteratee(accumulator, value, index, object);
+ });
+ return accumulator;
+ }
+
+ /**
+ * Removes the property at `path` of `object`.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The path of the property to unset.
+ * @returns {boolean} Returns `true` if the property is deleted, else `false`.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': 7 } }] };
+ * _.unset(object, 'a[0].b.c');
+ * // => true
+ *
+ * console.log(object);
+ * // => { 'a': [{ 'b': {} }] };
+ *
+ * _.unset(object, ['a', '0', 'b', 'c']);
+ * // => true
+ *
+ * console.log(object);
+ * // => { 'a': [{ 'b': {} }] };
+ */
+ function unset(object, path) {
+ return object == null ? true : baseUnset(object, path);
+ }
+
+ /**
+ * This method is like `_.set` except that accepts `updater` to produce the
+ * value to set. Use `_.updateWith` to customize `path` creation. The `updater`
+ * is invoked with one argument: (value).
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.6.0
+ * @category Object
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The path of the property to set.
+ * @param {Function} updater The function to produce the updated value.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': 3 } }] };
+ *
+ * _.update(object, 'a[0].b.c', function(n) { return n * n; });
+ * console.log(object.a[0].b.c);
+ * // => 9
+ *
+ * _.update(object, 'x[0].y.z', function(n) { return n ? n + 1 : 0; });
+ * console.log(object.x[0].y.z);
+ * // => 0
+ */
+ function update(object, path, updater) {
+ return object == null ? object : baseUpdate(object, path, castFunction(updater));
+ }
+
+ /**
+ * This method is like `_.update` except that it accepts `customizer` which is
+ * invoked to produce the objects of `path`. If `customizer` returns `undefined`
+ * path creation is handled by the method instead. The `customizer` is invoked
+ * with three arguments: (nsValue, key, nsObject).
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.6.0
+ * @category Object
+ * @param {Object} object The object to modify.
+ * @param {Array|string} path The path of the property to set.
+ * @param {Function} updater The function to produce the updated value.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var object = {};
+ *
+ * _.updateWith(object, '[0][1]', _.constant('a'), Object);
+ * // => { '0': { '1': 'a' } }
+ */
+ function updateWith(object, path, updater, customizer) {
+ customizer = typeof customizer == 'function' ? customizer : undefined;
+ return object == null ? object : baseUpdate(object, path, castFunction(updater), customizer);
+ }
+
+ /**
+ * Creates an array of the own enumerable string keyed property values of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property values.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.values(new Foo);
+ * // => [1, 2] (iteration order is not guaranteed)
+ *
+ * _.values('hi');
+ * // => ['h', 'i']
+ */
+ function values(object) {
+ return object == null ? [] : baseValues(object, keys(object));
+ }
+
+ /**
+ * Creates an array of the own and inherited enumerable string keyed property
+ * values of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property values.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.valuesIn(new Foo);
+ * // => [1, 2, 3] (iteration order is not guaranteed)
+ */
+ function valuesIn(object) {
+ return object == null ? [] : baseValues(object, keysIn(object));
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Clamps `number` within the inclusive `lower` and `upper` bounds.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Number
+ * @param {number} number The number to clamp.
+ * @param {number} [lower] The lower bound.
+ * @param {number} upper The upper bound.
+ * @returns {number} Returns the clamped number.
+ * @example
+ *
+ * _.clamp(-10, -5, 5);
+ * // => -5
+ *
+ * _.clamp(10, -5, 5);
+ * // => 5
+ */
+ function clamp(number, lower, upper) {
+ if (upper === undefined) {
+ upper = lower;
+ lower = undefined;
+ }
+ if (upper !== undefined) {
+ upper = toNumber(upper);
+ upper = upper === upper ? upper : 0;
+ }
+ if (lower !== undefined) {
+ lower = toNumber(lower);
+ lower = lower === lower ? lower : 0;
+ }
+ return baseClamp(toNumber(number), lower, upper);
+ }
+
+ /**
+ * Checks if `n` is between `start` and up to, but not including, `end`. If
+ * `end` is not specified, it's set to `start` with `start` then set to `0`.
+ * If `start` is greater than `end` the params are swapped to support
+ * negative ranges.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.3.0
+ * @category Number
+ * @param {number} number The number to check.
+ * @param {number} [start=0] The start of the range.
+ * @param {number} end The end of the range.
+ * @returns {boolean} Returns `true` if `number` is in the range, else `false`.
+ * @see _.range, _.rangeRight
+ * @example
+ *
+ * _.inRange(3, 2, 4);
+ * // => true
+ *
+ * _.inRange(4, 8);
+ * // => true
+ *
+ * _.inRange(4, 2);
+ * // => false
+ *
+ * _.inRange(2, 2);
+ * // => false
+ *
+ * _.inRange(1.2, 2);
+ * // => true
+ *
+ * _.inRange(5.2, 4);
+ * // => false
+ *
+ * _.inRange(-3, -2, -6);
+ * // => true
+ */
+ function inRange(number, start, end) {
+ start = toFinite(start);
+ if (end === undefined) {
+ end = start;
+ start = 0;
+ } else {
+ end = toFinite(end);
+ }
+ number = toNumber(number);
+ return baseInRange(number, start, end);
+ }
+
+ /**
+ * Produces a random number between the inclusive `lower` and `upper` bounds.
+ * If only one argument is provided a number between `0` and the given number
+ * is returned. If `floating` is `true`, or either `lower` or `upper` are
+ * floats, a floating-point number is returned instead of an integer.
+ *
+ * **Note:** JavaScript follows the IEEE-754 standard for resolving
+ * floating-point values which can produce unexpected results.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.7.0
+ * @category Number
+ * @param {number} [lower=0] The lower bound.
+ * @param {number} [upper=1] The upper bound.
+ * @param {boolean} [floating] Specify returning a floating-point number.
+ * @returns {number} Returns the random number.
+ * @example
+ *
+ * _.random(0, 5);
+ * // => an integer between 0 and 5
+ *
+ * _.random(5);
+ * // => also an integer between 0 and 5
+ *
+ * _.random(5, true);
+ * // => a floating-point number between 0 and 5
+ *
+ * _.random(1.2, 5.2);
+ * // => a floating-point number between 1.2 and 5.2
+ */
+ function random(lower, upper, floating) {
+ if (floating && typeof floating != 'boolean' && isIterateeCall(lower, upper, floating)) {
+ upper = floating = undefined;
+ }
+ if (floating === undefined) {
+ if (typeof upper == 'boolean') {
+ floating = upper;
+ upper = undefined;
+ }
+ else if (typeof lower == 'boolean') {
+ floating = lower;
+ lower = undefined;
+ }
+ }
+ if (lower === undefined && upper === undefined) {
+ lower = 0;
+ upper = 1;
+ }
+ else {
+ lower = toFinite(lower);
+ if (upper === undefined) {
+ upper = lower;
+ lower = 0;
+ } else {
+ upper = toFinite(upper);
+ }
+ }
+ if (lower > upper) {
+ var temp = lower;
+ lower = upper;
+ upper = temp;
+ }
+ if (floating || lower % 1 || upper % 1) {
+ var rand = nativeRandom();
+ return nativeMin(lower + (rand * (upper - lower + freeParseFloat('1e-' + ((rand + '').length - 1)))), upper);
+ }
+ return baseRandom(lower, upper);
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Converts `string` to [camel case](https://en.wikipedia.org/wiki/CamelCase).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the camel cased string.
+ * @example
+ *
+ * _.camelCase('Foo Bar');
+ * // => 'fooBar'
+ *
+ * _.camelCase('--foo-bar--');
+ * // => 'fooBar'
+ *
+ * _.camelCase('__FOO_BAR__');
+ * // => 'fooBar'
+ */
+ var camelCase = createCompounder(function(result, word, index) {
+ word = word.toLowerCase();
+ return result + (index ? capitalize(word) : word);
+ });
+
+ /**
+ * Converts the first character of `string` to upper case and the remaining
+ * to lower case.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to capitalize.
+ * @returns {string} Returns the capitalized string.
+ * @example
+ *
+ * _.capitalize('FRED');
+ * // => 'Fred'
+ */
+ function capitalize(string) {
+ return upperFirst(toString(string).toLowerCase());
+ }
+
+ /**
+ * Deburrs `string` by converting
+ * [Latin-1 Supplement](https://en.wikipedia.org/wiki/Latin-1_Supplement_(Unicode_block)#Character_table)
+ * and [Latin Extended-A](https://en.wikipedia.org/wiki/Latin_Extended-A)
+ * letters to basic Latin letters and removing
+ * [combining diacritical marks](https://en.wikipedia.org/wiki/Combining_Diacritical_Marks).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to deburr.
+ * @returns {string} Returns the deburred string.
+ * @example
+ *
+ * _.deburr('déjà vu');
+ * // => 'deja vu'
+ */
+ function deburr(string) {
+ string = toString(string);
+ return string && string.replace(reLatin, deburrLetter).replace(reComboMark, '');
+ }
+
+ /**
+ * Checks if `string` ends with the given target string.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to inspect.
+ * @param {string} [target] The string to search for.
+ * @param {number} [position=string.length] The position to search up to.
+ * @returns {boolean} Returns `true` if `string` ends with `target`,
+ * else `false`.
+ * @example
+ *
+ * _.endsWith('abc', 'c');
+ * // => true
+ *
+ * _.endsWith('abc', 'b');
+ * // => false
+ *
+ * _.endsWith('abc', 'b', 2);
+ * // => true
+ */
+ function endsWith(string, target, position) {
+ string = toString(string);
+ target = baseToString(target);
+
+ var length = string.length;
+ position = position === undefined
+ ? length
+ : baseClamp(toInteger(position), 0, length);
+
+ var end = position;
+ position -= target.length;
+ return position >= 0 && string.slice(position, end) == target;
+ }
+
+ /**
+ * Converts the characters "&", "<", ">", '"', and "'" in `string` to their
+ * corresponding HTML entities.
+ *
+ * **Note:** No other characters are escaped. To escape additional
+ * characters use a third-party library like [_he_](https://mths.be/he).
+ *
+ * Though the ">" character is escaped for symmetry, characters like
+ * ">" and "/" don't need escaping in HTML and have no special meaning
+ * unless they're part of a tag or unquoted attribute value. See
+ * [Mathias Bynens's article](https://mathiasbynens.be/notes/ambiguous-ampersands)
+ * (under "semi-related fun fact") for more details.
+ *
+ * When working with HTML you should always
+ * [quote attribute values](http://wonko.com/post/html-escaping) to reduce
+ * XSS vectors.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escape('fred, barney, & pebbles');
+ * // => 'fred, barney, & pebbles'
+ */
+ function escape(string) {
+ string = toString(string);
+ return (string && reHasUnescapedHtml.test(string))
+ ? string.replace(reUnescapedHtml, escapeHtmlChar)
+ : string;
+ }
+
+ /**
+ * Escapes the `RegExp` special characters "^", "$", "\", ".", "*", "+",
+ * "?", "(", ")", "[", "]", "{", "}", and "|" in `string`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escapeRegExp('[lodash](https://lodash.com/)');
+ * // => '\[lodash\]\(https://lodash\.com/\)'
+ */
+ function escapeRegExp(string) {
+ string = toString(string);
+ return (string && reHasRegExpChar.test(string))
+ ? string.replace(reRegExpChar, '\\$&')
+ : string;
+ }
+
+ /**
+ * Converts `string` to
+ * [kebab case](https://en.wikipedia.org/wiki/Letter_case#Special_case_styles).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the kebab cased string.
+ * @example
+ *
+ * _.kebabCase('Foo Bar');
+ * // => 'foo-bar'
+ *
+ * _.kebabCase('fooBar');
+ * // => 'foo-bar'
+ *
+ * _.kebabCase('__FOO_BAR__');
+ * // => 'foo-bar'
+ */
+ var kebabCase = createCompounder(function(result, word, index) {
+ return result + (index ? '-' : '') + word.toLowerCase();
+ });
+
+ /**
+ * Converts `string`, as space separated words, to lower case.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the lower cased string.
+ * @example
+ *
+ * _.lowerCase('--Foo-Bar--');
+ * // => 'foo bar'
+ *
+ * _.lowerCase('fooBar');
+ * // => 'foo bar'
+ *
+ * _.lowerCase('__FOO_BAR__');
+ * // => 'foo bar'
+ */
+ var lowerCase = createCompounder(function(result, word, index) {
+ return result + (index ? ' ' : '') + word.toLowerCase();
+ });
+
+ /**
+ * Converts the first character of `string` to lower case.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the converted string.
+ * @example
+ *
+ * _.lowerFirst('Fred');
+ * // => 'fred'
+ *
+ * _.lowerFirst('FRED');
+ * // => 'fRED'
+ */
+ var lowerFirst = createCaseFirst('toLowerCase');
+
+ /**
+ * Pads `string` on the left and right sides if it's shorter than `length`.
+ * Padding characters are truncated if they can't be evenly divided by `length`.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to pad.
+ * @param {number} [length=0] The padding length.
+ * @param {string} [chars=' '] The string used as padding.
+ * @returns {string} Returns the padded string.
+ * @example
+ *
+ * _.pad('abc', 8);
+ * // => ' abc '
+ *
+ * _.pad('abc', 8, '_-');
+ * // => '_-abc_-_'
+ *
+ * _.pad('abc', 3);
+ * // => 'abc'
+ */
+ function pad(string, length, chars) {
+ string = toString(string);
+ length = toInteger(length);
+
+ var strLength = length ? stringSize(string) : 0;
+ if (!length || strLength >= length) {
+ return string;
+ }
+ var mid = (length - strLength) / 2;
+ return (
+ createPadding(nativeFloor(mid), chars) +
+ string +
+ createPadding(nativeCeil(mid), chars)
+ );
+ }
+
+ /**
+ * Pads `string` on the right side if it's shorter than `length`. Padding
+ * characters are truncated if they exceed `length`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category String
+ * @param {string} [string=''] The string to pad.
+ * @param {number} [length=0] The padding length.
+ * @param {string} [chars=' '] The string used as padding.
+ * @returns {string} Returns the padded string.
+ * @example
+ *
+ * _.padEnd('abc', 6);
+ * // => 'abc '
+ *
+ * _.padEnd('abc', 6, '_-');
+ * // => 'abc_-_'
+ *
+ * _.padEnd('abc', 3);
+ * // => 'abc'
+ */
+ function padEnd(string, length, chars) {
+ string = toString(string);
+ length = toInteger(length);
+
+ var strLength = length ? stringSize(string) : 0;
+ return (length && strLength < length)
+ ? (string + createPadding(length - strLength, chars))
+ : string;
+ }
+
+ /**
+ * Pads `string` on the left side if it's shorter than `length`. Padding
+ * characters are truncated if they exceed `length`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category String
+ * @param {string} [string=''] The string to pad.
+ * @param {number} [length=0] The padding length.
+ * @param {string} [chars=' '] The string used as padding.
+ * @returns {string} Returns the padded string.
+ * @example
+ *
+ * _.padStart('abc', 6);
+ * // => ' abc'
+ *
+ * _.padStart('abc', 6, '_-');
+ * // => '_-_abc'
+ *
+ * _.padStart('abc', 3);
+ * // => 'abc'
+ */
+ function padStart(string, length, chars) {
+ string = toString(string);
+ length = toInteger(length);
+
+ var strLength = length ? stringSize(string) : 0;
+ return (length && strLength < length)
+ ? (createPadding(length - strLength, chars) + string)
+ : string;
+ }
+
+ /**
+ * Converts `string` to an integer of the specified radix. If `radix` is
+ * `undefined` or `0`, a `radix` of `10` is used unless `value` is a
+ * hexadecimal, in which case a `radix` of `16` is used.
+ *
+ * **Note:** This method aligns with the
+ * [ES5 implementation](https://es5.github.io/#x15.1.2.2) of `parseInt`.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.1.0
+ * @category String
+ * @param {string} string The string to convert.
+ * @param {number} [radix=10] The radix to interpret `value` by.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {number} Returns the converted integer.
+ * @example
+ *
+ * _.parseInt('08');
+ * // => 8
+ *
+ * _.map(['6', '08', '10'], _.parseInt);
+ * // => [6, 8, 10]
+ */
+ function parseInt(string, radix, guard) {
+ if (guard || radix == null) {
+ radix = 0;
+ } else if (radix) {
+ radix = +radix;
+ }
+ return nativeParseInt(toString(string).replace(reTrimStart, ''), radix || 0);
+ }
+
+ /**
+ * Repeats the given string `n` times.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to repeat.
+ * @param {number} [n=1] The number of times to repeat the string.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {string} Returns the repeated string.
+ * @example
+ *
+ * _.repeat('*', 3);
+ * // => '***'
+ *
+ * _.repeat('abc', 2);
+ * // => 'abcabc'
+ *
+ * _.repeat('abc', 0);
+ * // => ''
+ */
+ function repeat(string, n, guard) {
+ if ((guard ? isIterateeCall(string, n, guard) : n === undefined)) {
+ n = 1;
+ } else {
+ n = toInteger(n);
+ }
+ return baseRepeat(toString(string), n);
+ }
+
+ /**
+ * Replaces matches for `pattern` in `string` with `replacement`.
+ *
+ * **Note:** This method is based on
+ * [`String#replace`](https://mdn.io/String/replace).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category String
+ * @param {string} [string=''] The string to modify.
+ * @param {RegExp|string} pattern The pattern to replace.
+ * @param {Function|string} replacement The match replacement.
+ * @returns {string} Returns the modified string.
+ * @example
+ *
+ * _.replace('Hi Fred', 'Fred', 'Barney');
+ * // => 'Hi Barney'
+ */
+ function replace() {
+ var args = arguments,
+ string = toString(args[0]);
+
+ return args.length < 3 ? string : string.replace(args[1], args[2]);
+ }
+
+ /**
+ * Converts `string` to
+ * [snake case](https://en.wikipedia.org/wiki/Snake_case).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the snake cased string.
+ * @example
+ *
+ * _.snakeCase('Foo Bar');
+ * // => 'foo_bar'
+ *
+ * _.snakeCase('fooBar');
+ * // => 'foo_bar'
+ *
+ * _.snakeCase('--FOO-BAR--');
+ * // => 'foo_bar'
+ */
+ var snakeCase = createCompounder(function(result, word, index) {
+ return result + (index ? '_' : '') + word.toLowerCase();
+ });
+
+ /**
+ * Splits `string` by `separator`.
+ *
+ * **Note:** This method is based on
+ * [`String#split`](https://mdn.io/String/split).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category String
+ * @param {string} [string=''] The string to split.
+ * @param {RegExp|string} separator The separator pattern to split by.
+ * @param {number} [limit] The length to truncate results to.
+ * @returns {Array} Returns the string segments.
+ * @example
+ *
+ * _.split('a-b-c', '-', 2);
+ * // => ['a', 'b']
+ */
+ function split(string, separator, limit) {
+ if (limit && typeof limit != 'number' && isIterateeCall(string, separator, limit)) {
+ separator = limit = undefined;
+ }
+ limit = limit === undefined ? MAX_ARRAY_LENGTH : limit >>> 0;
+ if (!limit) {
+ return [];
+ }
+ string = toString(string);
+ if (string && (
+ typeof separator == 'string' ||
+ (separator != null && !isRegExp(separator))
+ )) {
+ separator = baseToString(separator);
+ if (!separator && hasUnicode(string)) {
+ return castSlice(stringToArray(string), 0, limit);
+ }
+ }
+ return string.split(separator, limit);
+ }
+
+ /**
+ * Converts `string` to
+ * [start case](https://en.wikipedia.org/wiki/Letter_case#Stylistic_or_specialised_usage).
+ *
+ * @static
+ * @memberOf _
+ * @since 3.1.0
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the start cased string.
+ * @example
+ *
+ * _.startCase('--foo-bar--');
+ * // => 'Foo Bar'
+ *
+ * _.startCase('fooBar');
+ * // => 'Foo Bar'
+ *
+ * _.startCase('__FOO_BAR__');
+ * // => 'FOO BAR'
+ */
+ var startCase = createCompounder(function(result, word, index) {
+ return result + (index ? ' ' : '') + upperFirst(word);
+ });
+
+ /**
+ * Checks if `string` starts with the given target string.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category String
+ * @param {string} [string=''] The string to inspect.
+ * @param {string} [target] The string to search for.
+ * @param {number} [position=0] The position to search from.
+ * @returns {boolean} Returns `true` if `string` starts with `target`,
+ * else `false`.
+ * @example
+ *
+ * _.startsWith('abc', 'a');
+ * // => true
+ *
+ * _.startsWith('abc', 'b');
+ * // => false
+ *
+ * _.startsWith('abc', 'b', 1);
+ * // => true
+ */
+ function startsWith(string, target, position) {
+ string = toString(string);
+ position = position == null
+ ? 0
+ : baseClamp(toInteger(position), 0, string.length);
+
+ target = baseToString(target);
+ return string.slice(position, position + target.length) == target;
+ }
+
+ /**
+ * Creates a compiled template function that can interpolate data properties
+ * in "interpolate" delimiters, HTML-escape interpolated data properties in
+ * "escape" delimiters, and execute JavaScript in "evaluate" delimiters. Data
+ * properties may be accessed as free variables in the template. If a setting
+ * object is given, it takes precedence over `_.templateSettings` values.
+ *
+ * **Note:** In the development build `_.template` utilizes
+ * [sourceURLs](http://www.html5rocks.com/en/tutorials/developertools/sourcemaps/#toc-sourceurl)
+ * for easier debugging.
+ *
+ * For more information on precompiling templates see
+ * [lodash's custom builds documentation](https://lodash.com/custom-builds).
+ *
+ * For more information on Chrome extension sandboxes see
+ * [Chrome's extensions documentation](https://developer.chrome.com/extensions/sandboxingEval).
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The template string.
+ * @param {Object} [options={}] The options object.
+ * @param {RegExp} [options.escape=_.templateSettings.escape]
+ * The HTML "escape" delimiter.
+ * @param {RegExp} [options.evaluate=_.templateSettings.evaluate]
+ * The "evaluate" delimiter.
+ * @param {Object} [options.imports=_.templateSettings.imports]
+ * An object to import into the template as free variables.
+ * @param {RegExp} [options.interpolate=_.templateSettings.interpolate]
+ * The "interpolate" delimiter.
+ * @param {string} [options.sourceURL='lodash.templateSources[n]']
+ * The sourceURL of the compiled template.
+ * @param {string} [options.variable='obj']
+ * The data object variable name.
+ * @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
+ * @returns {Function} Returns the compiled template function.
+ * @example
+ *
+ * // Use the "interpolate" delimiter to create a compiled template.
+ * var compiled = _.template('hello <%= user %>!');
+ * compiled({ 'user': 'fred' });
+ * // => 'hello fred!'
+ *
+ * // Use the HTML "escape" delimiter to escape data property values.
+ * var compiled = _.template('<%- value %>');
+ * compiled({ 'value': '